question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
shortest-impossible-sequence-of-rolls | 1 + Number of K windows | 1-number-of-k-windows-by-vats_lc-684t | Code | vats_lc | NORMAL | 2024-12-29T07:56:44.335074+00:00 | 2024-12-29T07:56:44.335074+00:00 | 2 | false | # Code
```cpp []
class Solution {
public:
int shortestSequence(vector<int>& rolls, int k) {
int n = rolls.size(), ans = 0;
set<int> st;
for (int i = 0; i < n; i++) {
st.insert(rolls[i]);
if (st.size() == k) {
ans++;
st.clear();
}
}
return ans + 1;
}
};
``` | 0 | 0 | ['C++'] | 0 |
shortest-impossible-sequence-of-rolls | Easiest O(N) Python solution | easiest-on-python-solution-by-duckysolut-2voc | The idea is to keep track of what permutations of numbers are possible as you go through the list. Keeping tracking of the real permutations though is impossibl | DuckySolutions | NORMAL | 2024-12-03T01:37:26.436240+00:00 | 2024-12-03T01:37:26.436277+00:00 | 8 | false | The idea is to keep track of what permutations of numbers are possible as you go through the list. Keeping tracking of the real permutations though is impossible as there are an exponential amount of possible permutations.\n\nOne thing to note though, is that for (1) or (2) to be possible, they have to appear in the list.\n\nAnd for (2, 1) to be possible, 1 has to appear at least once after 2. For (1, 2) to be possible, 2 has to appear at least once after 1.\n\nBecause of this ordered manner, you only have to keep track of how many times you can collect at least one of every number [1, k], as you go left to right through the array. If you only collected all the numbers once, that means that, although (1, 2) may have appeared in the array. (2, 1), might\'ve not.\n\nThis follows the property that all permutations of length K are just a convolution of all permutations of length K-1 and length 1. (As in, Permutations(K) = Permutations(K-1) * Permutations(1))\nSo for some index i, if you\'ve currently calculated minimum length to be K-1, that means you couldn\'t collect all unique combinations of length 1 after index i.\n\nYou can have a set/hashset of all the numbers you\'ve picked up so far, and once you\'ve collected all the numbers, the set will be length K. You can empty the set, and add 1 to your length.\n\nReturn the length in the end\n\n# Complexity\n- Time complexity:\n$$O(N + K*minLengthPermutationNotInList) = O(N)$$\nThis is O(N) as N is roughly equal to K. It is impossible for for the minLengthPermutationNotInList to be equal to N (for large values of N), as there are an exponential amount of permutations of length N.\n\n- Space complexity:\n$$O(K)$$\n\n# Code\n```python3 []\nclass Solution:\n def shortestSequence(self, rolls: List[int], k: int) -> int:\n length = 1\n numbers = set()\n \n for i in range(len(rolls)):\n numbers.add(rolls[i])\n if len(numbers) >= k:\n numbers = set()\n length += 1\n \n \n return length\n``` | 0 | 0 | ['Python3'] | 0 |
shortest-impossible-sequence-of-rolls | scala oneliner | scala-oneliner-by-vititov-lbkt | scala []\nobject Solution {\n import scala.util.chaining._\n def shortestSequence(rolls: Array[Int], k: Int): Int = \n rolls.iterator.foldLeft((0,Set.empty | vititov | NORMAL | 2024-11-08T19:17:10.509099+00:00 | 2024-11-08T19:17:10.509128+00:00 | 0 | false | ```scala []\nobject Solution {\n import scala.util.chaining._\n def shortestSequence(rolls: Array[Int], k: Int): Int = \n rolls.iterator.foldLeft((0,Set.empty[Int])){case ((acc,aSet),roll) =>\n (aSet + roll).pipe{bSet =>if(bSet.size < k) (acc,bSet) else (acc+1,Set())}\n }._1+1\n}\n``` | 0 | 0 | ['Hash Table', 'Greedy', 'Scala'] | 0 |
shortest-impossible-sequence-of-rolls | Greedy find full segments | greedy-find-full-segments-by-fredzqm-ei8p | Code\njava []\nclass Solution {\n public int shortestSequence(int[] rolls, int k) {\n Set<Integer> s = new HashSet<>();\n int numSeg = 0;\n | fredzqm | NORMAL | 2024-11-03T18:53:45.902260+00:00 | 2024-11-03T18:53:45.902287+00:00 | 2 | false | # Code\n```java []\nclass Solution {\n public int shortestSequence(int[] rolls, int k) {\n Set<Integer> s = new HashSet<>();\n int numSeg = 0;\n for (int i = 0; i < rolls.length; i++) {\n s.add(rolls[i]);\n if (s.size() == k) {\n numSeg++;\n s = new HashSet<>();\n }\n }\n return numSeg+1;\n }\n}\n``` | 0 | 0 | ['Java'] | 0 |
shortest-impossible-sequence-of-rolls | Python (Simple Maths) | python-simple-maths-by-rnotappl-ryem | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | rnotappl | NORMAL | 2024-10-25T06:58:40.768529+00:00 | 2024-10-25T06:58:40.768559+00:00 | 4 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python3 []\nclass Solution:\n def shortestSequence(self, rolls, k):\n n, count, res = len(rolls), 0, set()\n\n for i in range(n):\n res.add(rolls[i])\n\n if len(res) == k:\n count += 1 \n res = set()\n\n return count + 1 \n``` | 0 | 0 | ['Python3'] | 0 |
shortest-impossible-sequence-of-rolls | Simple And Fast Solution | simple-and-fast-solution-by-nishk2-rl5q | Code\nphp []\nclass Solution {\n\tfunction shortestSequence($rolls, $k){\n\t\t$len = 1;\n\t\t$met = [];\n\n\t\tforeach ($rolls as $roll) {\n\t\t\tif (!isset($me | nishk2 | NORMAL | 2024-10-08T10:08:19.839617+00:00 | 2024-10-08T10:08:19.839675+00:00 | 0 | false | # Code\n```php []\nclass Solution {\n\tfunction shortestSequence($rolls, $k){\n\t\t$len = 1;\n\t\t$met = [];\n\n\t\tforeach ($rolls as $roll) {\n\t\t\tif (!isset($met[$roll])) {\n\t\t\t\t$met[$roll] = 0;\n\t\t\t}\n\n\t\t\tif (count($met) === $k) {\n\t\t\t\t$len++;\n\t\t\t\t$met = [];\n\t\t\t}\n\t\t}\n\n\t\treturn $len;\n\t}\n}\n``` | 0 | 0 | ['PHP'] | 0 |
shortest-impossible-sequence-of-rolls | [Accepted] Swift | accepted-swift-by-vasilisiniak-msvk | \nclass Solution {\n func shortestSequence(_ rolls: [Int], _ k: Int) -> Int {\n \n var s = Set<Int>()\n var tgt = 1\n\n for r in | vasilisiniak | NORMAL | 2024-09-20T11:25:56.809777+00:00 | 2024-09-20T11:25:56.809816+00:00 | 0 | false | ```\nclass Solution {\n func shortestSequence(_ rolls: [Int], _ k: Int) -> Int {\n \n var s = Set<Int>()\n var tgt = 1\n\n for r in rolls {\n s.insert(r)\n \n if s.count == k {\n s.removeAll()\n tgt += 1\n }\n }\n\n return tgt\n }\n}\n``` | 0 | 0 | ['Swift'] | 0 |
shortest-impossible-sequence-of-rolls | Python || Simple solution || Sets | python-simple-solution-sets-by-vilaparth-osrq | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | vilaparthibhaskar | NORMAL | 2024-08-27T22:32:46.634582+00:00 | 2024-08-27T22:32:46.634613+00:00 | 14 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\no(n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\no(k)\n\n# Code\n```python3 []\nclass Solution:\n def shortestSequence(self, rolls: List[int], k: int) -> int:\n ans = 1\n i = len(rolls) - 1\n s = set()\n while i >= 0:\n while i >= 0 and len(s) < k:\n s.add(rolls[i])\n i -= 1\n if len(s) < k:return ans\n ans += 1\n s.clear()\n return ans\n``` | 0 | 0 | ['Array', 'Greedy', 'Python3'] | 0 |
shortest-impossible-sequence-of-rolls | C++ | c-by-user5976fh-pvoc | \nclass Solution {\npublic:\n int shortestSequence(vector<int>& r, int k) {\n int ans = 1;\n unordered_set<int> s;\n for (auto& n : r){\ | user5976fh | NORMAL | 2024-08-14T15:52:52.624528+00:00 | 2024-08-14T15:52:52.624561+00:00 | 0 | false | ```\nclass Solution {\npublic:\n int shortestSequence(vector<int>& r, int k) {\n int ans = 1;\n unordered_set<int> s;\n for (auto& n : r){\n s.insert(n);\n if (s.size() == k)\n ++ans, s = {};\n }\n return ans;\n }\n};\n``` | 0 | 0 | [] | 0 |
shortest-impossible-sequence-of-rolls | Solution with Javascript | solution-with-javascript-by-manhhungit37-93nj | Code\n\n/**\n * @param {number[]} rolls\n * @param {number} k\n * @return {number}\n */\nvar shortestSequence = function(rolls, k) {\n const set = new Set(); | manhhungit37 | NORMAL | 2024-08-12T07:40:18.586115+00:00 | 2024-08-12T07:40:18.586151+00:00 | 0 | false | # Code\n```\n/**\n * @param {number[]} rolls\n * @param {number} k\n * @return {number}\n */\nvar shortestSequence = function(rolls, k) {\n const set = new Set();\n let count = 1;\n for (let roll of rolls) {\n set.add(roll);\n if (set.size === k) {\n set.clear();\n count++;\n }\n }\n return count;\n};\n``` | 0 | 0 | ['JavaScript'] | 0 |
shortest-impossible-sequence-of-rolls | Python || Easy Greedy | python-easy-greedy-by-subscriber6436-v85r | Intuition\nWe\'d like to make such sequence of dice\'s rolls in a way, that it doesn\'t exist in a list of rolls. So, how to do this? If you have, as an example | subscriber6436 | NORMAL | 2024-08-06T15:25:45.611714+00:00 | 2024-08-06T15:26:57.038273+00:00 | 10 | false | # Intuition\nWe\'d like to make such sequence of dice\'s rolls in a way, that it **doesn\'t** exist in a list of `rolls`. So, how to do this? If you have, as an example, `k=3` and `rolls=[1,2,3]`, how you can extract such a sequence?\nWell, if make all the combinations, you\'ll find, that **it\'s impossible** to make `1,2,3` or `1,2` etc., but `1,1,1` will be acceptable, `1,2,2`, `2,1` and so on.\nTry to think this way. If you meet all the possible sides in **ONE** pass, **then it\'s IMPOSSIBLE** to make such a sequence with unique sides. So, simple add this **unlucky step** to the `ans`, fix this index and move forward.\n\n# Complexity\n- Time complexity: **O(N)**\n\n- Space complexity: **O(k)**\n\n# Code\n```python\nclass Solution:\n def shortestSequence(self, rolls: List[int], k: int) -> int:\n ans = 1\n cache = set()\n\n for num in rolls:\n cache.add(num)\n\n if len(cache) == k:\n ans += 1\n cache.clear()\n\n return ans\n\n\n\n``` | 0 | 0 | ['Greedy', 'Python3'] | 0 |
shortest-impossible-sequence-of-rolls | to take a track of how many set possible such that it give complete k elemant take each | to-take-a-track-of-how-many-set-possible-g9r4 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \nfrom it and then af | amit_shinde | NORMAL | 2024-07-31T18:03:42.882081+00:00 | 2024-07-31T18:03:42.882150+00:00 | 0 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nfrom it and then after the end we have and extra elemant that are not in the uncomplete set \n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int shortestSequence(vector<int>& rolls, int k) {\n set<int>st ;\n int cnt = 1 ;\n for (int i = 0 ; i<rolls.size() ; i++){\n st.insert(rolls[i]) ;\n if(st.size() == k){\n cnt++;\n st.clear() ;\n }\n }\n return cnt ;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
shortest-impossible-sequence-of-rolls | C++ || Easy To Understand | c-easy-to-understand-by-sanskarkumar028-5jgo | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | sanskarkumar028 | NORMAL | 2024-07-30T10:17:20.307124+00:00 | 2024-07-30T10:18:04.763448+00:00 | 0 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(N * K)\n- Space complexity:\nO(K)\n# Code\n```\nclass Solution {\npublic:\n bool check(unordered_map<int, int>& mp, int k)\n {\n if(mp.size() < k) return false;\n for(auto i : mp)\n {\n if(i.second < 1) return false;\n }\n return true;\n }\n int shortestSequence(vector<int>& rolls, int k) {\n int ans = 1;\n int n = rolls.size();\n unordered_map<int, int> mp;\n for(int i = 0; i < n; i++)\n {\n mp[rolls[i]]++;\n if(check(mp, k))\n {\n for(auto &i : mp) i.second = 0;\n ans++;\n }\n }\n if(check(mp, k)) ans++;\n return ans;\n }\n};\n``` | 0 | 0 | ['Array', 'Hash Table', 'Greedy', 'C++'] | 0 |
shortest-impossible-sequence-of-rolls | Easy Java solution || 1D DP | easy-java-solution-1d-dp-by-mnnit1prakha-ye7j | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | mnnit_prakharg | NORMAL | 2024-07-15T14:28:51.630412+00:00 | 2024-07-15T14:28:51.630449+00:00 | 0 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport java.util.*;\nimport java.io.*;\nclass Solution {\n public int shortestSequence(int[] arr, int k) {\n int n = arr.length;\n int[] typeArr = new int[k + 1];\n int mini = 0, updates = 0;\n for(int i = n - 1; i >= 0; i--){\n int myVal = arr[i];\n int nextEle = mini + 1;\n if(typeArr[myVal] != nextEle){\n typeArr[myVal] = nextEle;\n updates++;\n }\n if(updates == k){\n updates = 0;\n mini++;\n }\n }\n return mini + 1;\n }\n}\n``` | 0 | 0 | ['Java'] | 0 |
shortest-impossible-sequence-of-rolls | Python (Simple Maths) | python-simple-maths-by-rnotappl-2wve | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | rnotappl | NORMAL | 2024-07-13T07:26:25.645207+00:00 | 2024-07-13T07:26:25.645247+00:00 | 0 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def shortestSequence(self, rolls, k):\n count, ans = 0, set()\n\n for i in rolls:\n ans.add(i)\n if len(ans) == k:\n count += 1 \n ans.clear()\n\n return count+1\n``` | 0 | 0 | ['Python3'] | 0 |
shortest-impossible-sequence-of-rolls | Simple python code using set | simple-python-code-using-set-by-thomanan-8mn9 | \n\n# Complexity\n- Time complexity: O(n)\n\n\n- Space complexity: O(k)\n\n# Code\n\nclass Solution:\n def shortestSequence(self, rolls: List[int], k: int) - | thomanani | NORMAL | 2024-07-05T05:48:22.089300+00:00 | 2024-07-05T05:49:56.465131+00:00 | 16 | false | \n\n# Complexity\n- Time complexity: $$O(n)$$\n\n\n- Space complexity: $$O(k)$$\n\n# Code\n```\nclass Solution:\n def shortestSequence(self, rolls: List[int], k: int) -> int:\n s = set()\n curr = 1\n for i in range(len(rolls)-1,-1,-1):\n s.add(rolls[i])\n if len(s) == k:\n curr+=1\n s = set()\n return curr\n \n``` | 0 | 0 | ['Python3'] | 0 |
shortest-impossible-sequence-of-rolls | Very Simple Solution || Easy to understand || Explained | very-simple-solution-easy-to-understand-1ibhe | Complexity\n- Time complexity: O(n) \n\n- Space complexity: O(k) \n\n# Code\n\nclass Solution {\npublic:\n int shortestSequence(vector<int>& rolls, int k) {\ | coder_rastogi_21 | NORMAL | 2024-06-18T05:32:54.106052+00:00 | 2024-06-18T05:32:54.106089+00:00 | 1 | false | # Complexity\n- Time complexity: $$O(n)$$ \n\n- Space complexity: $$O(k)$$ \n\n# Code\n```\nclass Solution {\npublic:\n int shortestSequence(vector<int>& rolls, int k) {\n int ans = 0;\n unordered_set<int> seen;\n\n for(auto it : rolls) {\n seen.insert(it);\n if(seen.size() == k) { //if I have seen all numbers from 1 to k \n //till here we can form all subsequences of length (ans+1)\n seen.clear(); //make the set empty\n ans++; //increment ans (now we will look for this length)\n }\n }\n return ans+1; //we cannot make a subsequence of length (ans+1)\n }\n};\n``` | 0 | 0 | ['Greedy', 'C++'] | 0 |
shortest-impossible-sequence-of-rolls | Shortest PHP solution | shortest-php-solution-by-wischerdson-zt5c | \nclass Solution\n{\n\t/**\n\t * @param int[] $rolls\n\t * @param int $k\n\t * @return int\n\t */\n\tpublic function shortestSequence($rolls, $k)\n\t{\n\t\t$len | wischerdson | NORMAL | 2024-06-11T19:27:29.175292+00:00 | 2024-06-11T19:27:29.175326+00:00 | 1 | false | ```\nclass Solution\n{\n\t/**\n\t * @param int[] $rolls\n\t * @param int $k\n\t * @return int\n\t */\n\tpublic function shortestSequence($rolls, $k)\n\t{\n\t\t$len = 1;\n\t\t$met = [];\n\n\t\tforeach ($rolls as $roll) {\n\t\t\tif (!isset($met[$roll])) {\n\t\t\t\t$met[$roll] = 0;\n\t\t\t}\n\n\t\t\tif (count($met) === $k) {\n\t\t\t\t$len++;\n\t\t\t\t$met = [];\n\t\t\t}\n\t\t}\n\n\t\treturn $len;\n\t}\n}\n``` | 0 | 0 | ['PHP'] | 0 |
shortest-impossible-sequence-of-rolls | WebCanape [AMZ] | webcanape-amz-by-eb2hr8vpgm-exi9 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | eB2HR8vpgM | NORMAL | 2024-06-11T16:14:29.132457+00:00 | 2024-06-11T16:14:29.132499+00:00 | 8 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n k = 0\n rolls = []\n sequence_count = 0\n last_number = 0\n index_first_number = 0\n\n def find_last_number(self):\n numbers = [0 for num in range(1, self.k + 1)]\n\n for num in range(len(self.rolls) - 1, -1, -1):\n if numbers[self.rolls[num] - 1] == 0:\n numbers[self.rolls[num] - 1] = num\n\n if 0 not in numbers:\n self.last_number = self.rolls[num]\n break\n\n def find_first_number(self):\n numbers = [0 for num in range(1, self.k + 1)]\n\n for num in range(0, len(self.rolls)):\n\n if numbers[self.rolls[num] - 1] == 0:\n numbers[self.rolls[num] - 1] = self.rolls[num]\n\n if 0 not in numbers:\n self.index_first_number = num\n self.sequence_count += 1\n break\n\n def is_incorrect_sequence(self):\n numbers = {num for num in range(1, self.k + 1)}\n unique_rolls = set(self.rolls)\n\n return bool(numbers - unique_rolls)\n\n def shortestSequence(self, rolls: List[int], k: int) -> int:\n self.k = k\n self.rolls = rolls\n\n if self.is_incorrect_sequence():\n return 1\n\n if len(set(rolls)) == 1:\n return len(rolls) + 1\n\n if k == 1 or len(rolls) == k:\n return 2\n\n self.sequence_count = 0\n\n self.find_last_number()\n\n while self.last_number in self.rolls:\n self.find_first_number()\n self.rolls = self.rolls[self.index_first_number + 1:]\n\n self.sequence_count += 1\n\n return self.sequence_count\n``` | 0 | 0 | ['Python3'] | 0 |
shortest-impossible-sequence-of-rolls | WebCanape [AMZ Solution] | webcanape-amz-solution-by-eb2hr8vpgm-mdw7 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | eB2HR8vpgM | NORMAL | 2024-06-11T16:00:15.246444+00:00 | 2024-06-11T16:01:57.622820+00:00 | 1 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n k = 0\n rolls = []\n searched_sequence = []\n last_number = 0\n index_first_number = 0\n\n def find_last_number(self):\n numbers = [0 for num in range(1, self.k + 1)]\n\n for num in range(len(self.rolls) - 1, -1, -1):\n if numbers[self.rolls[num] - 1] == 0:\n numbers[self.rolls[num] - 1] = num\n\n if 0 not in numbers:\n self.last_number = self.rolls[num]\n break\n\n def find_first_number(self):\n numbers = [0 for num in range(1, self.k + 1)]\n\n for num in range(0, len(self.rolls)):\n\n if numbers[self.rolls[num] - 1] == 0:\n numbers[self.rolls[num] - 1] = self.rolls[num]\n\n if 0 not in numbers:\n self.index_first_number = num\n self.searched_sequence.append(self.rolls[num])\n break\n\n def is_incorrect_sequence(self):\n numbers = {num for num in range(1, self.k + 1)}\n unique_rolls = set(self.rolls)\n\n return bool(numbers - unique_rolls)\n\n\n def shortestSequence(self, rolls: List[int], k: int) -> int:\n if self.is_incorrect_sequence():\n return 1\n\n if len(rolls) == k:\n return 2\n\n if len(set(rolls)) == 1:\n return len(rolls) + 1\n\n if k == 1:\n return 2\n\n self.k = k\n self.rolls = rolls\n self.searched_sequence = []\n\n self.find_last_number()\n\n while self.last_number in self.rolls:\n self.find_first_number()\n self.rolls = self.rolls[self.index_first_number + 1:]\n\n self.searched_sequence.append(self.last_number)\n print(self.searched_sequence, len(self.searched_sequence))\n return len(self.searched_sequence)\n``` | 0 | 0 | ['Python3'] | 0 |
shortest-impossible-sequence-of-rolls | simple char line soln hai padhna hai to padho | simple-char-line-soln-hai-padhna-hai-to-38mit | the idea is simple check weather all k\'s from 1 to k have occurred in a segment or not and just count how many such segments and return cnt+1\n\nreason is our | Priyanshu_gupta_9620 | NORMAL | 2024-05-31T20:16:54.663535+00:00 | 2024-05-31T20:16:54.663561+00:00 | 4 | false | the idea is simple check weather all k\'s from 1 to k have occurred in a segment or not and just count how many such segments and return cnt+1\n\nreason is our greedy soln is when we pick the first first occurence of last element from 1-k \n\neg. [1,2,2,1,3,2,2,3,1,2,3] k=3\n\nnow our greedy ans which will minimize our seq will be \n3 (index-4 wala) then 1 (index-8 wala) and then 1 (which is not is array)\n\nso [3,1,1] is never possible and this is the req ans \n\n# Code\n```\nclass Solution {\npublic:\n int shortestSequence(vector<int>& v, int k) {\n map<int,int> m;\n int n=v.size();\n int ans=0;\n for(int i=0;i<n;i++){\n m[v[i]]++;\n if(m.size()==k){\n m.clear();\n ans++;\n }\n }\n return ans+1;\n }\n};\n``` | 0 | 0 | ['Greedy', 'Ordered Map', 'C++'] | 0 |
shortest-impossible-sequence-of-rolls | Simple Proof || 100% Beats O(n) || Combination logic || Mathematical Proof | simple-proof-100-beats-on-combination-lo-coq1 | Simple Understanding Read Point 2\n1. Iterate the array from left to right\n# if previosly n size sequence possible and currently we have k unique elements next | shubham6762 | NORMAL | 2024-05-23T10:46:47.102286+00:00 | 2024-05-23T10:46:47.102315+00:00 | 3 | false | # Simple Understanding Read Point 2\n1. Iterate the array from left to right\n# **if previosly n size sequence possible and currently we have `k` unique elements next to n sequences then `n + 1` combinations are possible.**\n3. So Just find out number of `k` unique sequences in array\n\n# Code\n```\nclass Solution {\npublic:\n int shortestSequence(vector<int>& rolls, int k) {\n int ans = 0;\n set<int>s;\n\n // here we want to find first impossible sequence \n //i.e. if n sequence can be possible till index i and \n //there are another k element next to i then n + 1 sequences are possible\n for (auto num : rolls)\n {\n s.insert(num);\n if (s.size() == k)\n {\n ans ++;\n s.clear();\n }\n }\n\n return ans + 1;\n }\n};\n``` | 0 | 0 | ['Math', 'Simulation', 'Combinatorics', 'Ordered Set', 'C++'] | 0 |
shortest-impossible-sequence-of-rolls | simple 7 line | simple-7-line-by-yoongyeom-ube4 | Code\n\nclass Solution:\n def shortestSequence(self, rolls: List[int], k: int) -> int:\n cnt = 1\n seen = set()\n for i in range(len(rol | YoonGyeom | NORMAL | 2024-05-02T03:15:27.079706+00:00 | 2024-05-02T03:15:27.079744+00:00 | 9 | false | # Code\n```\nclass Solution:\n def shortestSequence(self, rolls: List[int], k: int) -> int:\n cnt = 1\n seen = set()\n for i in range(len(rolls)):\n seen.add(rolls[i])\n if len(seen) == k:\n seen = set()\n cnt += 1\n return cnt\n``` | 0 | 0 | ['Python3'] | 0 |
shortest-impossible-sequence-of-rolls | complexity 0(n) +simple observation | complexity-0n-simple-observation-by-asad-sb20 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \napproach is very simple | asadali0786 | NORMAL | 2024-04-15T06:29:34.731428+00:00 | 2024-04-15T06:29:34.731456+00:00 | 0 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\napproach is very simple you have to just think what is the smallest sequence you need to create all possible combination of N elments in you sequence ,lets take an example\nN=1 k=1 1\nN=2 k=2 12 \nN=6 k=3 123123 (see in this example in this if you append k digits 2 times it will give us all possible combination which have size 2)\n.\n.\n.\nso the solution is the number of times you find (1,2,..,k )+1 and the \nfor example\n1 3 3 2 1 3\nin this example if k=3\nwe find our 1 to k in index 4(1 based indexing)\nafter that repeat again \n \n\ni hope you get little intuition you can ask in comment.\n\n\n# Complexity\n- Time complexity:\n- O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int shortestSequence(vector<int>& r, int k) {\n int a = r.size();\n\n \n\n map<int,int>s;\n int ans=0;\n int count=1;\n for (int j = 0; j < a; j++) {\n // if(r[j]==count)count++;\n s[r[j]]++;\n while(s[count]){\n s[count]--;\n count++;\n }\n\n if(count>k){\n // cout<<j<<endl;\n ans++;\n count=1;\n s.clear();\n }\n\n }\n if(ans<a){\n return ans+1;\n }\n \n return a+1;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
shortest-impossible-sequence-of-rolls | C++ || beats 100% | c-beats-100-by-adnan_alkam-uhc4 | \n# Code\n\nclass Solution {\npublic:\n int shortestSequence(vector<int>& rolls, int k) {\n ios_base::sync_with_stdio(false);\n\t\tcin.tie(NULL);\n\t\ | Adnan_Alkam | NORMAL | 2024-03-26T02:34:31.022863+00:00 | 2024-03-26T02:34:31.022942+00:00 | 3 | false | \n# Code\n```\nclass Solution {\npublic:\n int shortestSequence(vector<int>& rolls, int k) {\n ios_base::sync_with_stdio(false);\n\t\tcin.tie(NULL);\n\t\tcout.tie(NULL);\n\n int n = rolls.size(), ans = 1;\n vector<bool> visited(k, false);\n int k2 = 0;\n for(int i = 0; i < n; i++){\n int index = rolls[i]-1;\n\n if (visited[index]) continue;\n visited[index] = true;\n k2++;\n if (k2 == k) {\n ans++;\n k2 = 0;\n for (int j = 0 ; j < k; j++)\n visited[j] = false;\n }\n\n }\n return ans;\n }\n};\n``` | 0 | 0 | ['Hash Table', 'C++'] | 0 |
shortest-impossible-sequence-of-rolls | Beats 83% || Easy C++ Solution | beats-83-easy-c-solution-by-krishiiitp-4kty | Code\n\nclass Solution {\npublic:\n int shortestSequence(vector<int>& rolls, int k) {\n int n=rolls.size(),ans=0;\n unordered_map<int,int> mm;\ | krishiiitp | NORMAL | 2024-01-29T11:14:08.803999+00:00 | 2024-01-29T11:14:08.804019+00:00 | 2 | false | # Code\n```\nclass Solution {\npublic:\n int shortestSequence(vector<int>& rolls, int k) {\n int n=rolls.size(),ans=0;\n unordered_map<int,int> mm;\n for(int i=0;i<n;i++){\n mm[rolls[i]]++;\n if(mm.size()==k){\n ans++;\n mm.clear();\n }\n }\n ans++;\n return ans;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
shortest-impossible-sequence-of-rolls | Linear Time Solution in python | linear-time-solution-in-python-by-muthur-c89o | Intuition\nFind out the minimum index that contains all the elements for specific frequency.\n\n\n# Complexity\n- Time complexity:\nO(N)\n\n- Space complexity:\ | muthuramkumar | NORMAL | 2024-01-27T05:14:59.380885+00:00 | 2024-01-27T05:14:59.380916+00:00 | 11 | false | # Intuition\nFind out the minimum index that contains all the elements for specific frequency.\n\n\n# Complexity\n- Time complexity:\nO(N)\n\n- Space complexity:\nO(N)\n\n# Code\n```\nclass Solution:\n def shortestSequence(self, rolls: List[int], k: int) -> int:\n n, i = 0, 0\n visited = set()\n while i < len(rolls):\n if len(visited) == k:\n n += 1\n visited = set()\n visited.add(rolls[i])\n i += 1\n if len(visited) == k:\n n += 1\n\n return n + 1\n``` | 0 | 0 | ['Python3'] | 0 |
shortest-impossible-sequence-of-rolls | Efficient JS solution - Counting + Greedy (Beat 100% time and 91% memory) | efficient-js-solution-counting-greedy-be-i75v | \n\nPro tip: Love \u8C22\u8C22 \uD83D\uDC9C\nI saw you guys using Set.clear or filling the counting list. Why do you need that when we can do it more efficientl | CuteTN | NORMAL | 2024-01-23T17:32:20.746778+00:00 | 2024-01-23T17:32:20.746818+00:00 | 2 | false | 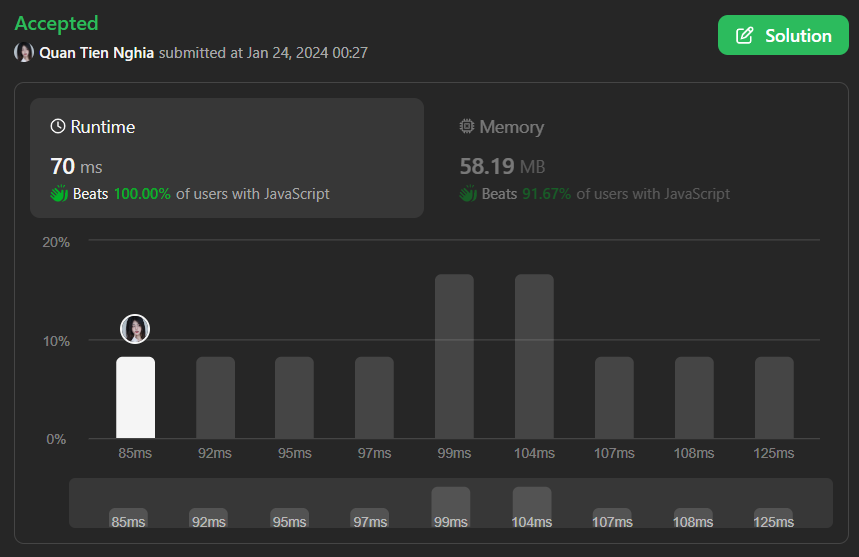\n\nPro tip: *Love \u8C22\u8C22* \uD83D\uDC9C\nI saw you guys using Set.clear or filling the counting list. Why do you need that when we can do it more efficiently with just parities! By switching a parity we can mark the whole counting array as "outdated" (Please analyze the code below yourself).\n\n# Complexity\n- Time complexity: $$O(n + k)$$\n- Space complexity: $$O(k)$$\n\n# Code\n```js\nlet seen = new Uint8Array(100001);\n\n/**\n * @param {number[]} rolls\n * @param {number} k\n * @return {number}\n */\nvar shortestSequence = function (rolls, k) {\n let n = rolls.length;\n if (k == 1) return n + 1;\n\n let res = 1;\n seen.fill(0, 0, k + 1);\n let cnt = 0;\n let parity = 1;\n\n for (let i = 0; i < n; ++i) {\n if (seen[rolls[i]] ^ parity) {\n ++cnt;\n seen[rolls[i]] ^= 1;\n if (cnt == k) {\n ++res;\n parity ^= 1;\n cnt = 0;\n }\n }\n }\n\n return res;\n};\n``` | 0 | 0 | ['Greedy', 'Counting', 'JavaScript'] | 0 |
shortest-impossible-sequence-of-rolls | ruby 1-liner | ruby-1-liner-by-_-k-388i | \ndef shortest_sequence(rolls, k, s=Set[]) =\n\n rolls.count{ s.clear if (s<<_1).size==k } + 1\n | _-k- | NORMAL | 2024-01-15T17:09:17.922120+00:00 | 2024-01-15T17:09:17.922161+00:00 | 6 | false | ```\ndef shortest_sequence(rolls, k, s=Set[]) =\n\n rolls.count{ s.clear if (s<<_1).size==k } + 1\n``` | 0 | 0 | ['Ruby'] | 0 |
shortest-impossible-sequence-of-rolls | Easy Solution in Javascript | easy-solution-in-javascript-by-chetannad-6ylv | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | chetannada | NORMAL | 2023-12-28T18:12:20.385430+00:00 | 2023-12-28T18:12:20.385454+00:00 | 0 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n/**\n * @param {number[]} rolls\n * @param {number} k\n * @return {number}\n */\nvar shortestSequence = function (rolls, k) {\n let allElementGroups = 0;\n let set = new Set();\n\n for (let ele of rolls) {\n set.add(ele);\n\n if (set.size === k) {\n allElementGroups += 1;\n set = new Set();\n }\n }\n\n return allElementGroups + 1;\n};\n``` | 0 | 0 | ['JavaScript'] | 0 |
shortest-impossible-sequence-of-rolls | Python Hard | python-hard-by-lucasschnee-vhzh | \nclass Solution:\n def shortestSequence(self, rolls: List[int], k: int) -> int:\n \'\'\'\n initial thought: sliding window\n\n Note tha | lucasschnee | NORMAL | 2023-11-20T21:23:26.537717+00:00 | 2023-11-20T21:23:26.537739+00:00 | 5 | false | ```\nclass Solution:\n def shortestSequence(self, rolls: List[int], k: int) -> int:\n \'\'\'\n initial thought: sliding window\n\n Note that the sequence taken does not have to be consecutive as long as it is in order. \n\n contiguous\n\n meaning for every \n\n\n\n 3\n\n 1 2 3\n\n 12 13 23 21 31 32\n\n 123 132 312 321 213 231\n\n \n\n longest = kPk\n\n maybe bfs\n\n\n \n \'\'\'\n N = len(rolls)\n level = 1\n \n\n r = 0\n \n while True:\n\n d = defaultdict(int)\n\n while len(d) != k:\n if r == N:\n return level\n d[rolls[r]] += 1\n r += 1\n\n level += 1\n\n \n return 0\n\n\n\n \n``` | 0 | 0 | ['Python3'] | 0 |
goat-latin | [C++/Java/Python] Straight Forward Solution | cjavapython-straight-forward-solution-by-xklq | Explanation\n1. Build a vowel set.(optional)\n2. Split \'S\' to words.\n3. For each word, check if it starts with a vowel. (O(1) complexity with set).\n4. If it | lee215 | NORMAL | 2018-04-29T06:45:49.651714+00:00 | 2020-08-19T15:49:05.023667+00:00 | 18,802 | false | # Explanation\n1. Build a vowel set.(optional)\n2. Split \'S\' to words.\n3. For each word, check if it starts with a vowel. (O(1) complexity with set).\n4. If it does, keep going. If it does not, rotate the first letter to the end.\n5. Add it to result string.\n<br>\n\n**C++:**\n```cpp\n string toGoatLatin(string S) {\n unordered_set<char> vowel({\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\'});\n istringstream iss(S);\n string res, w;\n int i = 0, j;\n while (iss >> w) {\n res += \' \' + (vowel.count(w[0]) == 1 ? w : w.substr(1) + w[0]) + "ma";\n for (j = 0, ++i; j < i; ++j) res += "a";\n }\n return res.substr(1);\n }\n```\n**Java:**\n```java\n public String toGoatLatin(String S) {\n Set<Character> vowels = new HashSet<>(Arrays.asList(\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\'));\n String res = "";\n int i = 0, j = 0;\n for (String w : S.split("\\\\s")) {\n res += \' \' + (vowels.contains(w.charAt(0)) ? w : w.substring(1) + w.charAt(0)) + "ma";\n for (j = 0, ++i; j < i; ++j) {\n res += "a";\n }\n };\n return res.substring(1);\n }\n```\n**Python:**\n```py\n def toGoatLatin(self, S):\n vowel = set(\'aeiouAEIOU\')\n def latin(w, i):\n if w[0] not in vowel:\n w = w[1:] + w[0]\n return w + \'ma\' + \'a\' * (i + 1)\n return \' \'.join(latin(w, i) for i, w in enumerate(S.split()))\n```\n\n**1-line Python:**\n```py\n def toGoatLatin(self, S):\n return \' \'.join((w if w[0].lower() in \'aeiou\' else w[1:] + w[0]) + \'ma\' + \'a\' * (i + 1) for i, w in enumerate(S.split()))\n```\n | 125 | 6 | [] | 37 |
goat-latin | Short C++ solution using i/o stringstream | short-c-solution-using-io-stringstream-b-0eh2 | \nstring toGoatLatin(string S) {\n stringstream iss(S), oss;\n string vowels("aeiouAEIOU"), word, a;\n while (iss >> word) {\n a.push_back(\'a\' | mzchen | NORMAL | 2018-04-29T03:29:57.338152+00:00 | 2018-09-26T15:51:43.337918+00:00 | 6,405 | false | ```\nstring toGoatLatin(string S) {\n stringstream iss(S), oss;\n string vowels("aeiouAEIOU"), word, a;\n while (iss >> word) {\n a.push_back(\'a\');\n if (vowels.find_first_of(word[0]) != string::npos) // begin with a vowel\n oss << \' \' << word << "ma" << a;\n else // begin with a consonant\n oss << \' \' << word.substr(1) << word[0] << "ma" << a;\n }\n return oss.str().substr(1);\n}\n``` | 68 | 1 | [] | 17 |
goat-latin | Java 2 ms solution with time and space complexity explanation | java-2-ms-solution-with-time-and-space-c-fgu5 | java\n/**\n * Time Complexity: O(3*L + N^2) = O(Split + Substring + Adding word to sb +\n * Adding suffix to sb)\n *\n * Space Complexity: O(L + N^2 + N) = O(In | wtc2021 | NORMAL | 2018-05-03T07:39:09.029005+00:00 | 2019-09-09T07:38:42.334440+00:00 | 6,230 | false | ```java\n/**\n * Time Complexity: O(3*L + N^2) = O(Split + Substring + Adding word to sb +\n * Adding suffix to sb)\n *\n * Space Complexity: O(L + N^2 + N) = O(Input string + Suffix for all words +\n * Suffix sb)\n *\n * L = Length of the input string. N = Number of words in the input string.\n */\nclass Solution {\n private static final HashSet<Character> vowels = new HashSet<>(\n Arrays.asList(\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\'));\n\n public String toGoatLatin(String S) {\n if (S == null || S.length() == 0) {\n return "";\n }\n\n StringBuilder sb = new StringBuilder();\n StringBuilder suffix = new StringBuilder("a");\n\n for (String w : S.split(" ")) {\n if (sb.length() != 0) {\n sb.append(" ");\n }\n\n char fChar = w.charAt(0);\n if (vowels.contains(fChar)) {\n sb.append(w);\n } else {\n sb.append(w.substring(1));\n sb.append(fChar);\n }\n\n sb.append("ma").append(suffix);\n\n suffix.append("a");\n }\n\n return sb.toString();\n }\n}\n``` | 35 | 2 | [] | 7 |
goat-latin | [Python] Just do what is asked, explained | python-just-do-what-is-asked-explained-b-3khs | This is easy prolem, where you need just to do what is asked, without really thinking.\n\n1. Split our sentence into words, using .split(). If it is not allowed | dbabichev | NORMAL | 2020-08-19T07:34:10.741782+00:00 | 2020-08-19T12:02:06.056621+00:00 | 2,297 | false | This is easy prolem, where you need just to do what is asked, without really thinking.\n\n1. Split our sentence into words, using `.split()`. If it is not allowed we can just traverse it and find spaces.\n2. For every word check if it starts with vowel, if it is, add `ma` and `i+1` letters `a` to the end. It is `i+1`, because we start from zero index.\n3. If first letter is not vowel, remove first letter and add it to the end, also add `ma` and letters `a`.\n\n**Complexity**: time and space complexity is `O(n) + O(k^2)`, where `n` is number of symbols in our string and `k` is number of words: do not forget about letters `a` in the end: we add `1 + 2 + ... + k = O(k^2)` letters in the end.\n\n```\nclass Solution:\n def toGoatLatin(self, S):\n words = S.split()\n vowels = set("AEIOUaeiou")\n for i in range(len(words)):\n if words[i][0] in vowels:\n words[i] = words[i] + "ma" + "a"*(i+1)\n else:\n words[i] = words[i][1:] + words[i][0] + "ma" + "a"*(i+1)\n \n return " ".join(words)\n```\n\nIf you have any questions, feel free to ask. If you like solution and explanations, please **Upvote!** | 32 | 5 | [] | 3 |
goat-latin | [Python] (Very Easy to understand solution) | python-very-easy-to-understand-solution-hsfxx | \nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n temp = []\n ans = " " # Here space must be present. (So, after joining the words, | not_a_cp_coder | NORMAL | 2020-08-19T07:45:52.603609+00:00 | 2020-08-19T07:46:16.250234+00:00 | 2,496 | false | ```\nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n temp = []\n ans = " " # Here space must be present. (So, after joining the words, they wil be separated by space)\n i = 1\n vowel = [\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\']\n for word in S.split(" "):\n if word[0] in vowel:\n word = word + "ma"\n else:\n word = word[1:] + word[0] + "ma"\n word = word + "a"*i\n i = i + 1\n temp.append(word)\n\t\t# Joins all the list elements.\n return ans.join(temp)\n \n```\nIf you have any doubts, head over the **comment** section.\nIf you like this solution, do **UPVOTE**.\nHappy Coding :) | 18 | 1 | ['Python', 'Python3'] | 4 |
goat-latin | C++, easy to understand, just as we think! | c-easy-to-understand-just-as-we-think-by-x23t | \nclass Solution {\nprivate:\n bool isVowel(char c) {\n c = tolower(c);\n return (c == \'a\' || c == \'e\' || c == \'i\' || c == \'o\' || c == | vasant17 | NORMAL | 2018-06-17T02:58:07.752706+00:00 | 2018-10-25T14:12:29.657310+00:00 | 2,101 | false | ```\nclass Solution {\nprivate:\n bool isVowel(char c) {\n c = tolower(c);\n return (c == \'a\' || c == \'e\' || c == \'i\' || c == \'o\' || c == \'u\');\n }\n \npublic:\n string toGoatLatin(string S) {\n string result;\n string append = "maa", w;\n istringstream line(S);\n while (line >> w) {\n if (!isVowel(w[0])) {\n w += w[0]; // Add the first charater to last\n w.erase(0, 1); // Erase the first charater\n }\n w += append; // Append maa, maaa, maaaa, ......\n\t\t\t\t\t\t\n result += w + " "; // Add the word to result\n append += \'a\'; // Prepare the next maaa, maaaa, maaaaa, ...\n }\n result.erase(result.size()-1, 1); // Don\'t forget to remove the whitespace at the end!\n return result;\n }\n};\n``` | 14 | 0 | [] | 3 |
goat-latin | Java 1ms 100% - Clean & Efficient O(n) Solution (Faster Solution) | java-1ms-100-clean-efficient-on-solution-7jl0 | Hit the UPVOTE! Thankyou <3\n\nclass Solution {\n public String toGoatLatin(String sentence) {\n StringBuilder sb = new StringBuilder();\n Stri | talhashaikh1998 | NORMAL | 2021-09-17T20:06:15.142701+00:00 | 2021-09-17T20:06:15.142738+00:00 | 1,517 | false | **Hit the UPVOTE! Thankyou <3**\n```\nclass Solution {\n public String toGoatLatin(String sentence) {\n StringBuilder sb = new StringBuilder();\n String[] words = sentence.split(" ");\n int r = 1;\n for(String word : words){\n char ch = word.charAt(0);\n if(ch == \'a\' || ch == \'e\' || ch == \'i\' || ch == \'o\' || ch == \'u\' ||ch == \'A\' || ch == \'E\' || ch == \'I\' || ch == \'O\' || ch == \'U\')\n sb.append(word).append("ma").append("a".repeat(r++)).append(" ");\n else\n sb.append(word.substring(1,word.length())).append(word.charAt(0)).append("ma").append("a".repeat(r++)).append(" "); \n } \n return sb.toString().trim();\n }\n}\n``` | 12 | 1 | ['Java'] | 4 |
goat-latin | C++ Clean&Clear Solution 100% faster using istringstream with explanations | c-cleanclear-solution-100-faster-using-i-yq7n | \nclass Solution {\npublic:\n string toGoatLatin(string S) \n {\n // result\n string goatLatin = "";\n \n static const string | debbiealter | NORMAL | 2020-08-19T08:48:37.814861+00:00 | 2020-08-19T08:58:05.929284+00:00 | 1,340 | false | ```\nclass Solution {\npublic:\n string toGoatLatin(string S) \n {\n // result\n string goatLatin = "";\n \n static const string VOWELS = "aAeEiIoOuU";\n \n // to keep count of how many "a"s to add\n size_t count = 1;\n \n\t\t// for spliting sentence to words\n std::istringstream ss(S); \n \n \twhile (ss)\n\t { \n \tstd::string word; \n \tss >> word;\n \n\t\t // convert word to goat latin according to case\n if(!word.empty())\n {\n goatLatin += VOWELS.find(word[0]) != string::npos ? word + "ma" + std::string(count, \'a\') + " " : word.substr(1, word.length() - 1) + word[0] + "ma" + std::string(count, \'a\') + " ";\n }\n \n // increment for next word\n count ++;\n\t }\n\t\t// return sentence without last space\n return goatLatin.substr(0, goatLatin.length() - 1);\n }\n};\n``` | 10 | 1 | ['C++'] | 1 |
goat-latin | JavaScript two lines | javascript-two-lines-by-shengdade-bu6l | javascript\n/**\n * @param {string} S\n * @return {string}\n */\nvar toGoatLatin = function(S) {\n const vowels = new Set([\'a\', \'e\', \'i\', \'o\', \'u\', \ | shengdade | NORMAL | 2020-02-15T15:11:51.151663+00:00 | 2020-02-15T15:11:51.151694+00:00 | 631 | false | ```javascript\n/**\n * @param {string} S\n * @return {string}\n */\nvar toGoatLatin = function(S) {\n const vowels = new Set([\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\']);\n return S.split(\' \')\n .map((w, i) =>\n vowels.has(w[0])\n ? w + \'ma\' + \'a\'.repeat(i + 1)\n : w.slice(1) + w[0] + \'ma\' + \'a\'.repeat(i + 1)\n )\n .join(\' \');\n};\n``` | 9 | 2 | ['JavaScript'] | 1 |
goat-latin | C++ Soolution, 0ms, 9MB(less than 100%) | c-soolution-0ms-9mbless-than-100-by-pooj-u6sr | Runtime: 0 ms, faster than 100.00% of C++ online submissions for Goat Latin.\nMemory Usage: 9 MB, less than 100.00% of C++ online submissions for Goat Latin.\n\ | pooja0406 | NORMAL | 2019-09-10T10:32:13.139131+00:00 | 2019-09-10T10:32:13.139182+00:00 | 699 | false | Runtime: 0 ms, faster than 100.00% of C++ online submissions for Goat Latin.\nMemory Usage: 9 MB, less than 100.00% of C++ online submissions for Goat Latin.\n\n```\nstring toGoatLatin(string S) {\n \n int front = 0;\n int count = 1;\n int n = S.size();\n string res;\n \n for(int i=0; i<=n; i++)\n {\n if(i == n || S[i] == \' \')\n {\n string word(S.begin() + front, S.begin() + i);\n \n if(word[0] == \'a\' || word[0] == \'A\' || word[0] == \'e\' || word[0] == \'E\' || word[0] == \'i\' || word[0] == \'I\' || word[0] == \'o\' || word[0] == \'O\' || word[0] == \'u\' || word[0] == \'U\')\n res += word + "ma";\n else\n res += word.substr(1) + word[0] + "ma";\n \n for(int k=0; k<count; k++)\n res += \'a\';\n \n if(i != n)\n res += " ";\n front = i+1;\n count++;\n }\n }\n \n return res;\n } | 9 | 2 | ['C'] | 2 |
goat-latin | [C++/Python] Challenge me for shorter solution (1Line python & 3Line C++) | cpython-challenge-me-for-shorter-solutio-w17p | Idea:\nFuzzy grammar between string and set in python make coding simple.\nC++ does not have such freedom as Python in this case.\n\nSolution 1:\n\nclass Soluti | codedayday | NORMAL | 2020-08-19T20:23:04.911626+00:00 | 2022-10-31T16:51:14.898975+00:00 | 863 | false | Idea:\nFuzzy grammar between string and set in python make coding simple.\nC++ does not have such freedom as Python in this case.\n\nSolution 1:\n```\nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n vowel=\'aeiouAEIOU\'\n sList=S.strip().split()\n result = \'\'\n for i, w in enumerate(sList):\n if w[0] in vowel: result+=\' \' + w + \'ma\' + \'a\'*(i+1)\n else:result +=\' \' + w[1:] + w[0] + \'ma\' + \'a\'*(i+1)\n return \'\' if result == \'\' else result[1:]\n```\n\nSolution 2:\n```\ndef toGoatLatin(self, S):\n\t\t#return \' \'.join((w if w[0].lower() in \'aeiou\' else w[1:] + w[0]) + \'ma\' + \'a\' * (i + 1) for i, w in enumerate(S.split()))\n\t\treturn \' \'.join((w if w[0].lower() in \'aeiou\' else w[1:] + w[0]) + \'ma\' + \'a\' * i for i, w in enumerate(S.split(), 1)) #Thanks @PonomarevIK\n```\n\nSolution 3: the shortest one (Challenge me for shorter)\n```\nclass Solution:\n def toGoatLatin(self, S: str) -> str: \n\t\treturn \' \'.join([w[1:] + w[0], w][w[0].lower() in \'aeiou\'] + \'maa\' + \'a\' * i for i, w in enumerate(S.split())) # Thanks @susanxie123\n\t\t#return \' \'.join([w[1:] + w[0], w][w[0] in \'aeiouAEIOU\'] + \'maa\' + \'a\' * i for i, w in enumerate(S.split())) # shorter but takes more in checking vowel property\n```\n\nSolution 4: C++ 3-line version\n```\nclass Solution {\npublic:\n string toGoatLatin(string S) {\n stringstream ss(S), oss; \n for(string vowels("aeiouAEIOU"), word, a; ss>>word; oss <<"maa"<<a, a+=\'a\') \n vowels.find_first_of(word[0]) !=string::npos ? oss<<\' \' <<word: oss<<\' \'<< word.substr(1)<<word[0];\n return oss.str().substr(1); \n }\n};\n``` | 7 | 0 | ['C', 'Python'] | 4 |
goat-latin | C++ 0ms , faster than 100% solutions | c-0ms-faster-than-100-solutions-by-paran-evu3 | class Solution {\npublic:\n string toGoatLatin(string s) {\n \n int n = s.size();\n string ans = "";\n int x =1; // last m string m \ | paranoid-619 | NORMAL | 2021-12-13T09:40:56.178308+00:00 | 2021-12-13T09:40:56.178337+00:00 | 860 | false | class Solution {\npublic:\n string toGoatLatin(string s) {\n \n int n = s.size();\n string ans = "";\n int x =1; // last m string m \'a\' add krne k liye ( index 1 pr 1 \'a\' , index 2 pr 2 \'a\' and sp on)//\n for(int i = 0;i<s.size();i++){\n int j =i;\n if(s[i] == \'a\' || s[i] == \'e\' || s[i] == \'i\' || s[i] == \'o\' || s[i] == \'u\' || s[i] == \'A\' || s[i] == \'E\' || s[i] == \'I\' || s[i] == \'O\' || s[i] == \'U\'){\n while(j<n and s[j]!=\' \'){\n ans+=s[j];\n j++;\n }\n }\n else{\n j++;\n while(j<n and s[j]!=\' \'){\n ans+=s[j];\n j++;\n }\n ans+=s[i]; // firsct char add krre m string m\n }\n ans+="ma";\n // int c = x;\n // while(c>0){\n // ans+=\'a\';\n // c--;\n // }\n int t = x;\n ans.append(t,\'a\');\n x++;\n if(j<n)ans+=\' \'; // taaki last character m bhi space na dal jaaye\n i = j; // taaki i jaha j h wha direct pahuch jaaye\n }\n return ans;\n \n }\n};\n\n | 6 | 0 | ['String', 'C', 'C++'] | 3 |
goat-latin | concise python solution | concise-python-solution-by-yuzhoul-6q7f | ```\nclass Solution:\n def toGoatLatin(self, S):\n """\n :type S: str\n :rtype: str\n """\n words = S.split()\n vow | yuzhoul | NORMAL | 2018-05-01T20:51:29.892909+00:00 | 2018-05-01T20:51:29.892909+00:00 | 1,064 | false | ```\nclass Solution:\n def toGoatLatin(self, S):\n """\n :type S: str\n :rtype: str\n """\n words = S.split()\n vowels = \'AEIOUaeiou\'\n def transform(stuff):\n idx, word = stuff\n if word[0] in vowels:\n word += \'ma\'\n else:\n word = word[1:] + word[0] + \'ma\'\n word += \'a\'*(idx+1) \n return word\n return " ".join(list(map(transform, enumerate(words)))) | 6 | 1 | [] | 0 |
goat-latin | Easy Java solution, 13 ms | easy-java-solution-13-ms-by-lifeisnotcod-czwi | public String toGoatLatin(String S) {\n \n List list=new ArrayList(Arrays.asList(\'a\',\'e\',\'i\',\'o\',\'u\',\'A\',\'E\',\'I\',\'O\',\'U\'));\n | LifeIsNotCoding | NORMAL | 2018-04-30T01:01:03.517689+00:00 | 2018-04-30T01:01:03.517689+00:00 | 1,151 | false | public String toGoatLatin(String S) {\n \n List<Character> list=new ArrayList<Character>(Arrays.asList(\'a\',\'e\',\'i\',\'o\',\'u\',\'A\',\'E\',\'I\',\'O\',\'U\'));\n String arr[]=S.split(" ");\n String a="a",test="";\n StringBuffer sb=new StringBuffer();\n \n for(int i=0;i<arr.length;i++)\n {\n test=arr[i];\n \n if(list.contains(test.charAt(0)))\n {\n sb.append(test+"ma");\n }\n else\n {\n sb.append(test.substring(1)+test.charAt(0)+"ma");\n }\n \n sb.append(a);\n a=a+"a";\n sb.append(" ");\n }\n \n \n return sb.toString().trim();\n } | 6 | 0 | [] | 1 |
goat-latin | Kotlin Best Solution | kotlin-best-solution-by-progp-ijdu | \n# Code\n\nclass Solution {\nfun toGoatLatin(S: String): String {\n val a = StringBuilder("a")\n return S.split(" ").joinToString(" ") {\n if ("ae | progp | NORMAL | 2023-12-10T08:02:26.047273+00:00 | 2023-12-10T08:02:26.047304+00:00 | 104 | false | \n# Code\n```\nclass Solution {\nfun toGoatLatin(S: String): String {\n val a = StringBuilder("a")\n return S.split(" ").joinToString(" ") {\n if ("aeiou".contains(it[0].toLowerCase())) (it + "ma" + a).also { a.append("a") }\n else (it.substring(1) + it[0] + "ma" + a).also { a.append("a") }\n }\n}\n}\n``` | 5 | 0 | ['Kotlin'] | 0 |
goat-latin | Python // 36 ms // 2-liner & 4-liner | python-36-ms-2-liner-4-liner-by-cenkay-tn93 | \nclass Solution:\n def toGoatLatin(self, S):\n s, vowels = S.split(), {"a", "e", "i", "o", "u"} \n return " ".join([(s[i][0].lower() in vowels | cenkay | NORMAL | 2018-04-29T03:18:21.490753+00:00 | 2018-10-25T14:12:54.965105+00:00 | 945 | false | ```\nclass Solution:\n def toGoatLatin(self, S):\n s, vowels = S.split(), {"a", "e", "i", "o", "u"} \n return " ".join([(s[i][0].lower() in vowels and s[i] or s[i][1:] + s[i][0]) + "m" + "a" * (i + 2) for i in range(len(s))])\n```\n* 4 line solution for better readability:\n```\nclass Solution:\n def toGoatLatin(self, S):\n s, vowels = S.split(), {"a", "e", "i", "o", "u"}\n for i, char in enumerate(s):\n s[i] = char[1:] + char[0] + "ma" + "a" * (i + 1) if char[0].lower() not in vowels else char + "ma" + "a" * (i + 1) \n return " ".join(s)\n``` | 5 | 0 | [] | 3 |
goat-latin | [Python 3] - Simple Solution w Explanation | python-3-simple-solution-w-explanation-b-s1yc | Still fairly new to Python so open to any suggestions or improvements! \n\nclass Solution:\n def toGoatLatin(self, sentence: str) -> str:\n new = sent | IvanTsukei | NORMAL | 2022-03-23T18:37:04.396400+00:00 | 2022-03-23T18:37:04.396482+00:00 | 1,297 | false | Still fairly new to Python so open to any suggestions or improvements! \n```\nclass Solution:\n def toGoatLatin(self, sentence: str) -> str:\n new = sentence.split() # Breaks up the input into individual sentences\n count = 1 # Starting at 1 since we only have one "a" to begin with.\n \n for x in range(len(new)):\n if new[x][0].casefold() in \'aeiou\': # Checks if the first value of x is a vowel. The casefold, can be replaced with lower, lowers the case. Can also just be removed and have "in \'aeiouAEIOU\'\n new[x] = new[x] + \'ma\' + \'a\'*count # Brings it together with the count multiplying number of "a"\'s as needed.\n count += 1\n elif new[x].casefold() not in \'aeiou\': # Same comment as above.\n new[x] = new[x][1:] + new[x][0] + \'ma\' + \'a\'*count # Just moves the first value to the end then does the a.\n count += 1\n \n return " ".join(x for x in new) # Converts the list back into a string.\n```\n | 4 | 0 | ['Python', 'Python3'] | 1 |
goat-latin | [C++] 0 ms, Easy to understand solution | c-0-ms-easy-to-understand-solution-by-an-59td | \n string toGoatLatin(string S) \n {\n vector<char> vowel{\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\'};\n string res=" | anjali_cs | NORMAL | 2021-03-11T19:17:08.245378+00:00 | 2021-03-12T19:16:03.885237+00:00 | 305 | false | ```\n string toGoatLatin(string S) \n {\n vector<char> vowel{\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\'};\n string res="";\n stringstream s(S); //for splitting sentence to words\n string word;\n int curr=1; //keeping a counter to append \'a\'\n while(s>>word)\n {\n if(count(vowel.begin(),vowel.end(),word[0])) //if 1st character is a vowel\n {\n word+="ma";\n word.append(curr,\'a\'); //appending \'a\' curr times\n res+=word;\n res.push_back(\' \'); //space after a word\n curr++; \n }\n else //if 1st character is a consonant \n {\n char c=word[0];\n word.erase(0,1);\n word.push_back(c);\n word+="ma";\n word.append(curr,\'a\');\n res+=word;\n res.push_back(\' \');\n curr++; \n \n }\n }\n res.pop_back(); //removing extra space at the end\n return res;\n }\n``` | 4 | 0 | ['C'] | 0 |
goat-latin | Python super-simple intuitive solution | python-super-simple-intuitive-solution-b-vbd4 | Like it? please upvote...\n\nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n count = 0\n res = []\n for word in S.split():\n | yehudisk | NORMAL | 2020-08-19T07:13:20.909941+00:00 | 2020-08-19T07:19:52.952726+00:00 | 276 | false | **Like it? please upvote...**\n```\nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n count = 0\n res = []\n for word in S.split():\n count+=1\n if word[0].lower() in [\'a\', \'e\', \'i\', \'o\', \'u\']:\n res.append(word+"ma"+\'a\'*count)\n else:\n res.append(word[1:]+word[0]+"ma"+\'a\'*count)\n return " ".join(res)\n``` | 4 | 0 | ['Python3'] | 0 |
goat-latin | cpp with 100% ms | cpp-with-100-ms-by-ahmed_433-lqg9 | Code | Ahmed_433 | NORMAL | 2024-12-30T21:59:20.659567+00:00 | 2024-12-30T21:59:20.659567+00:00 | 254 | false |
# Code
```cpp []
class Solution {
public:
string toGoatLatin(string sentence) {
deque<string>a;
string ans="";
string trill="";
string s;
int cnt=0;
for(int i=0;i<sentence.size()+1;i++)
{if(sentence[i]!=' ')
{
s+=sentence[i];
}else
{
cnt++;
a.push_back(s);
s="";
}
}
s=s.substr(0,s.size()-1);
a.push_back(s);
for(auto it:a)
{
trill=trill+'a';
if(it[0]=='a'||it[0]=='e'||it[0]=='i'||it[0]=='o'||it[0]=='u'||it[0]=='A'||it[0]=='E'||it[0]=='I'||it[0]=='O'||it[0]=='U')
{
it+="ma"+trill;
}else
{
it=it.substr(1,it.size())+it[0]+"ma"+trill;
}
ans+=it+' ';
}
ans=ans.substr(0,ans.size()-1);
return ans;
}
};
``` | 3 | 0 | ['C++'] | 2 |
goat-latin | Beats 100%🔥|| easy JAVA Solution✅ | beats-100-easy-java-solution-by-priyansh-tl9n | Code\n\nclass Solution {\n public String toGoatLatin(String sentence) {\n String[] str=sentence.split(" ");\n StringBuilder ans=new StringBuild | priyanshu1078 | NORMAL | 2024-02-05T10:51:11.578079+00:00 | 2024-02-05T10:51:11.578154+00:00 | 477 | false | # Code\n```\nclass Solution {\n public String toGoatLatin(String sentence) {\n String[] str=sentence.split(" ");\n StringBuilder ans=new StringBuilder();\n StringBuilder x=new StringBuilder();\n for(int i=0;i<str.length;i++){\n x.append("a");\n if(isVowel(str[i])){\n ans.append(str[i]);\n }else{\n ans.append(str[i].substring(1));\n ans.append(str[i].charAt(0));\n }\n ans.append("ma");\n ans.append(x);\n if(i<str.length-1) ans.append(" ");\n }\n return ans.toString();\n }\n public boolean isVowel(String s){\n char ch=s.charAt(0);\n if(ch==\'a\' || ch==\'e\' || ch==\'i\' || ch==\'o\' || ch==\'u\' || ch==\'A\' || ch==\'E\' || ch==\'I\' || ch==\'O\' || ch==\'U\') return true;\n return false;\n }\n}\n``` | 3 | 0 | ['Java'] | 0 |
goat-latin | NOOB CODE : EASY TO UNDERSTAND | noob-code-easy-to-understand-by-harshava-ga30 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n1. If a word begins with a vowel (a, e, i, o, u, A, E, I, O, U), append " | HARSHAVARDHAN_15 | NORMAL | 2023-09-30T09:17:18.162781+00:00 | 2023-09-30T09:17:18.162803+00:00 | 949 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. If a word begins with a vowel (a, e, i, o, u, A, E, I, O, U), append "ma" to the end of the word.\n2. If a word begins with a consonant, remove the first letter and append it to the end of the word, followed by "ma."\n3. For each word, add \'a\' (in increasing order starting with \'a\') to the end of the resulting word.\n\nLet\'s break down the code step by step:\n\n1. `l = sentence.split()`: The input sentence is split into words, and the resulting list of words is stored in the `l` variable.\n\n2. `v = set([\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\'])`: A set `v` is created to hold vowels in both lowercase and uppercase.\n\n3. `str1 = \'\'`: An empty string `str1` is initialized to store the transformed sentence.\n\n4. `r = \'\'`, `at = \'\'`, `st = \'\'`: These variables are initialized for temporary storage within the loop.\n\n5. The code then iterates through each word in the `l` list (representing words in the input sentence):\n\n a. `at = \'a\' * (intr + 1)`: This line calculates the \'a\' sequence based on the word\'s position in the sentence. The \'a\' sequence increases by one with each word.\n\n b. `st = l[intr]`: Retrieves the current word from the list.\n\n c. Checks if the first letter of the word (`st[0]`) is in the set of vowels (`v`). If it is, it means the word starts with a vowel, so:\n - `r = st + \'ma\'`: The word is appended with "ma."\n\n d. If the word doesn\'t start with a vowel (i.e., it starts with a consonant), it performs the following steps:\n - A loop is used to iterate through the characters of the word starting from the second character (`st[1:]`), and they are concatenated to the `r` variable.\n - The first character of the word (`st[0]`) is then added to the end of the `r` variable.\n - Finally, "ma" is appended to the `r` variable.\n\n e. The \'a\' sequence calculated earlier (`at`) is added to the end of the `r` variable.\n\n f. The `r` variable, representing the transformed word, is appended to the `str1` variable with a space.\n\n6. Finally, the code returns `str1[1:]`, which removes the leading space, providing the correctly formatted Goat Latin sentence.\n\n# Complexity\n- Time complexity: ***O(n * m)***\nwhere n is the number of words in the sentence, and m is the average length of a word\n- Space complexity: ***O(n)***\n\n\n# Code\n```\nclass Solution:\n def toGoatLatin(self, sentence: str) -> str:\n l = sentence.split()\n v = set([\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\'])\n str1 = \'\'\n r = \'\'\n at = \'\'\n st = \'\'\n for intr in range(len(l)):\n r = \'\'\n at = \'a\' * (intr + 1)\n st = l[intr]\n if st[0] in v:\n r = st + \'ma\'\n else:\n if len(st) > 1:\n for m in range(1, len(st)):\n r = r + st[m]\n r = r + st[0]\n r = r + \'ma\'\n r = r + at\n str1 = str1 + \' \' + r\n return str1[1:]\n\n``` | 3 | 0 | ['Python3'] | 0 |
goat-latin | Java Easy Solution Beats 100% runtime & 99.21% memory | java-easy-solution-beats-100-runtime-992-7327 | Intuition\nThe intuition behind this solution is to iterate through each word in the input sentence, apply the Goat Latin rules to each word, and then build the | Laziz_511 | NORMAL | 2023-09-07T10:03:17.504582+00:00 | 2023-09-07T10:03:17.504602+00:00 | 336 | false | # Intuition\nThe intuition behind this solution is to iterate through each word in the input sentence, apply the Goat Latin rules to each word, and then build the final Goat Latin sentence by appending the modified words together.\n\n# Approach\n1. Initialize a `StringBuilder` named `goatLatin` to store the Goat Latin sentence.\n\n2. Split the input sentence into an array of `words` using space as the delimiter.\n\n4. Create a string vowels containing all lowercase and uppercase `vowels`: "aeiouAEIOU".\n\n5. Iterate through each word in the words array using a for loop with index `i`:\n a. Get the current word from the `words` array.\n\n b. Retrieve the first character of the word using `word.charAt(0) `and store it in `firstChar`.\n\n c. Check if firstChar is a vowel by using v`owels.indexOf(firstChar) != -1`. If it is a vowel, append the original word to the goatLatin StringBuilder; otherwise, append the modified version of the word following the Goat Latin rules.\n\n d. Append "ma" to the `goatLatin` StringBuilder.\n\n e. Append "a" repeated `i + 1` times to the goatLatin StringBuilder to satisfy the index-based requirement.\n\n f. If i is not the last word (i.e., `i < words.length - 1`), append a space " " to separate words in the Goat Latin sentence.\n\n5. After processing all words, return the Goat Latin sentence as a string by calling `goatLatin.toString()`.\n\n# Complexity\n- Time Complexity: The algorithm iterates through each word in the input sentence exactly once, and the operations performed within the loop are constant time. Therefore, the time complexity is $$O(n)$$, where n is the total number of characters in the input sentence.\n\n- Space Complexity: The space complexity is $$O(n)$$, where n is the length of the input sentence. This is because we split the sentence into an array of words, and the goatLatin StringBuilder stores the Goat Latin sentence.\n\n# Code\n```\nclass Solution {\n public String toGoatLatin(String sentence) {\n StringBuilder goatLatin = new StringBuilder();\n String[] words = sentence.split(" ");\n String vowels = "aeiouAEIOU";\n\n for (int i = 0; i < words.length; i++) {\n String word = words[i];\n char firstChar = word.charAt(0);\n\n if (vowels.indexOf(firstChar) != -1) {\n goatLatin.append(word);\n } else {\n goatLatin.append(word.substring(1)).append(firstChar);\n }\n\n goatLatin.append("ma").append("a".repeat(i + 1));\n\n if (i < words.length - 1) {\n goatLatin.append(" ");\n }\n }\n\n return goatLatin.toString();\n }\n}\n\n``` | 3 | 0 | ['Java'] | 1 |
goat-latin | Use StringBuilder || JAVA || Just 1 loop | use-stringbuilder-java-just-1-loop-by-im-0qsb | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | im_obid | NORMAL | 2022-11-27T16:45:05.411565+00:00 | 2022-11-27T16:45:05.411715+00:00 | 680 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public String toGoatLatin(String sentence) {\n String[] words = sentence.split("\\\\s+");\n StringBuilder res=new StringBuilder("");\n StringBuilder a= new StringBuilder("");\n for(int i=0;i<words.length;i++){\n if(!"aeiouAEIOU".contains(words[i].charAt(0)+"")){\n res.append(words[i].substring(1)).append(words[i].charAt(0)).append("maa").append(a+" ");\n }else{\n res.append(words[i]).append("maa").append(a+" ");\n }\n a.append("a");\n }\n return res.toString().trim();\n }\n}\n``` | 3 | 0 | ['Java'] | 0 |
goat-latin | ✔️[C++]||Easy Solution | ceasy-solution-by-nikhil909-zr43 | class Solution {\nprivate:\n\n\n bool isVowel(char ch){\n if(ch==\'a\'||ch==\'e\'||ch==\'i\'||ch==\'o\'||ch==\'u\'||ch==\'A\'||ch==\'E\'||ch==\'I\'||c | nikhil909 | NORMAL | 2022-08-29T20:36:39.587516+00:00 | 2022-08-29T20:36:39.587559+00:00 | 610 | false | class Solution {\nprivate:\n\n\n bool isVowel(char ch){\n if(ch==\'a\'||ch==\'e\'||ch==\'i\'||ch==\'o\'||ch==\'u\'||ch==\'A\'||ch==\'E\'||ch==\'I\'||ch==\'O\'||ch==\'U\'){\n return true;\n }\n return false;\n }\npublic:\n\n string toGoatLatin(string sentence) {\n int len = sentence.length();\n string str = "ma";\n int count=2;\n for(int i=0; i<sentence.length(); i++){\n int j=i;\n \n while( j<sentence.length() && sentence[j]!=\' \' ){\n j++;\n }\n \n str+=\'a\';\n if(isVowel(sentence[i])){\n sentence.insert(j,str);\n }\n else{\n string ch = sentence[i]+str;\n sentence.insert(j,ch);\n sentence.erase(i,1);\n }\n count++;\n i=j+count;//becuase old string is modified so we cant just do i=j;each word is modified with one a evertime so count ++ \n }\n return sentence;\n }\n}; | 3 | 0 | ['C', 'C++'] | 0 |
goat-latin | [JAVA] BEATS 100.00% MEMORY/SPEED 0ms // APRIL 2022 | java-beats-10000-memoryspeed-0ms-april-2-56et | \n\tclass Solution {\n\n public String toGoatLatin(String sentence) {\n StringBuilder sb = new StringBuilder();\n String[] words = sentence.spl | darian-catalin-cucer | NORMAL | 2022-04-20T06:41:59.630003+00:00 | 2022-04-20T06:41:59.630059+00:00 | 285 | false | \n\tclass Solution {\n\n public String toGoatLatin(String sentence) {\n StringBuilder sb = new StringBuilder();\n String[] words = sentence.split(" ");\n int r = 1;\n for(String word : words){\n char ch = word.charAt(0);\n if(ch == \'a\' || ch == \'e\' || ch == \'i\' || ch == \'o\' || ch == \'u\' ||ch == \'A\' || ch == \'E\' || ch == \'I\' || ch == \'O\' || ch == \'U\')\n sb.append(word).append("ma").append("a".repeat(r++)).append(" ");\n else\n sb.append(word.substring(1,word.length())).append(word.charAt(0)).append("ma").append("a".repeat(r++)).append(" "); \n } \n return sb.toString().trim();\n }\n\t} | 3 | 0 | ['Java'] | 0 |
goat-latin | Simple solution in Golang | simple-solution-in-golang-by-bhargavsnv1-56yn | Code\n====\n\ngo\nfunc isVowel(s string) bool {\n\tletter := strings.ToLower(string(s[0]))\n\n\tswitch letter {\n\tcase "a", "e", "i", "o", "u":\n\t\treturn tru | bhargavsnv100 | NORMAL | 2020-11-06T18:17:34.799082+00:00 | 2020-11-06T18:17:34.799138+00:00 | 147 | false | Code\n====\n\n```go\nfunc isVowel(s string) bool {\n\tletter := strings.ToLower(string(s[0]))\n\n\tswitch letter {\n\tcase "a", "e", "i", "o", "u":\n\t\treturn true\n\t}\n\n\treturn false\n}\n\nfunc toGoatLatin(S string) string {\n\twords := strings.Split(S, " ")\n\tsolution := ""\n\n\tfor i, word := range words {\n\t\ta := strings.Repeat("a", i+1)\n\t\tgoatWord := ""\n\n\t\tif isVowel(word) {\n\t\t\tgoatWord = word + "ma"\n\t\t} else {\n\t\t\tgoatWord = word[1:] + string(word[0]) + "ma"\n\t\t}\n\n\t\tsolution = solution + goatWord + a + " "\n\t}\n\n\treturn solution[:len(solution)-1]\n}\n```\n | 3 | 0 | ['Go'] | 0 |
goat-latin | golang | golang-by-zz12345-nejy | \nfunc toGoatLatin(S string) string {\n\tif len(S) == 0 {\n\t\treturn ""\n\t}\n\n\tstrs := strings.Split(S, " ")\n\tfor i := 0; i < len(strs); i++ {\n\t\tswitch | zz12345 | NORMAL | 2020-02-21T22:37:28.892446+00:00 | 2020-02-21T22:37:28.892496+00:00 | 75 | false | ```\nfunc toGoatLatin(S string) string {\n\tif len(S) == 0 {\n\t\treturn ""\n\t}\n\n\tstrs := strings.Split(S, " ")\n\tfor i := 0; i < len(strs); i++ {\n\t\tswitch s := strs[i]; s[0] {\n\t\tcase \'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\':\n\t\t\tstrs[i] = s + "ma"\n\t\tdefault:\n\t\t\tstrs[i] = s[1:] + string(s[0]) + "ma"\n\t\t}\n\t\tfor j := 0; j < i+1; j++ {\n\t\t\tstrs[i] = strs[i] + "a"\n\t\t}\n\t}\n\treturn strings.Join(strs, " ")\n}\n``` | 3 | 0 | [] | 0 |
goat-latin | 1ms 99.95% JAVA Solution | 1ms-9995-java-solution-by-imranansari-76xg | \nclass Solution {\n public String toGoatLatin(String str) {\n String[] words = str.split(" ");\n StringBuilder sb = new StringBuilder();\n | imranansari | NORMAL | 2020-02-07T11:28:12.382941+00:00 | 2020-02-07T11:28:12.383025+00:00 | 450 | false | ```\nclass Solution {\n public String toGoatLatin(String str) {\n String[] words = str.split(" ");\n StringBuilder sb = new StringBuilder();\n int k = 1;\n for (String word : words) {\n char ch = word.charAt(0);\n if (ch == \'a\' || ch == \'e\' || ch == \'i\' || ch == \'o\' || ch == \'u\' || ch == \'A\' || ch == \'E\' || ch == \'I\' || ch == \'O\' || ch == \'U\') {\n sb.append(word);\n sb.append("ma");\n } else {\n sb.append(word.substring(1,word.length()));\n sb.append(ch);\n sb.append("ma");\n }\n int i = 0;\n while (i < k) {\n sb.append("a");\n ++i;\n }\n ++k;\n sb.append(" ");\n }\n \n int index = sb.lastIndexOf(" ");\n String s = sb.toString().substring(0, index);\n return s;\n }\n}\n``` | 3 | 0 | ['Java'] | 0 |
goat-latin | Accepted Python3 using a simple regular expression | accepted-python3-using-a-simple-regular-mk50l | \nimport re\n\nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n s = S.split()\n out = \'\'\n for i, w in enumerate(s):\n | i-i | NORMAL | 2019-12-17T16:32:05.253361+00:00 | 2019-12-17T16:32:05.253442+00:00 | 320 | false | ```\nimport re\n\nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n s = S.split()\n out = \'\'\n for i, w in enumerate(s):\n if re.match(r\'(?i)^[aeiou]\', w):\n out += w + \'ma\' + (i + 1) * \'a\' + \' \'\n else:\n out += w[1:] + w[0] + \'ma\' + (i + 1) * \'a\' + \' \'\n\n return out.strip()\n```\n\n | 3 | 0 | ['String', 'Python', 'Python3'] | 0 |
goat-latin | Solution in Python 3 (beats ~100%) (one line) | solution-in-python-3-beats-100-one-line-9zvfd | ```\nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n \treturn " ".join([(s if s[0].lower() in \'aeiou\' else s[1:]+s[0])+\'maa\'+\'a\'*i for i,s | junaidmansuri | NORMAL | 2019-08-07T20:18:24.548110+00:00 | 2019-08-07T20:20:05.283064+00:00 | 245 | false | ```\nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n \treturn " ".join([(s if s[0].lower() in \'aeiou\' else s[1:]+s[0])+\'maa\'+\'a\'*i for i,s in enumerate(S.split())])\n\n\n- Junaid Mansuri\n(LeetCode ID)@hotmail.com | 3 | 1 | ['Python', 'Python3'] | 0 |
goat-latin | C Solution | c-solution-by-sunsfz-di27 | \nchar* toGoatLatin(char* S) {\n int aCount = 1;\n int ansSize = 0;\n int ansIndex = 0;\n char* ans = malloc(0);\n char s[1] = {\' \'};\n char | sunsfz | NORMAL | 2019-02-16T17:42:31.499175+00:00 | 2019-02-16T17:42:31.499213+00:00 | 378 | false | ```\nchar* toGoatLatin(char* S) {\n int aCount = 1;\n int ansSize = 0;\n int ansIndex = 0;\n char* ans = malloc(0);\n char s[1] = {\' \'};\n char* token;\n char vowel;\n \n token = strtok(S, s); \n while(1)\n {\n ansSize += strlen(token) + 4 + aCount;\n ans = realloc(ans, sizeof(char)*ansSize);\n vowel = tolower(token[0]);\n if(vowel == \'a\' || vowel == \'e\' || vowel == \'i\' || vowel == \'o\' || vowel == \'u\' )\n {\n ansIndex += sprintf(&ans[ansIndex], "%sma", token);\n }\n else\n {\n ansIndex += sprintf(&ans[ansIndex], "%s%cma", &token[1], token[0]);\n \n }\n for(int i = 0; i < aCount; i++)\n {\n ansIndex += sprintf(&ans[ansIndex], "a");\n }\n aCount++;\n \n token = strtok(NULL, s);\n if(token)\n {\n ansIndex += sprintf(&ans[ansIndex], " ");\n }\n else\n {\n break;\n }\n }\n return ans;\n}\n``` | 3 | 0 | ['C'] | 0 |
goat-latin | Python solution | python-solution-by-zitaowang-i397 | Time complexity: O(n), space complexity: O(n), where n is the number of characters in S.\n\n\nclass Solution:\n def toGoatLatin(self, S):\n """\n | zitaowang | NORMAL | 2019-01-22T08:58:22.320826+00:00 | 2019-01-22T08:58:22.320882+00:00 | 236 | false | Time complexity: `O(n)`, space complexity: `O(n)`, where `n` is the number of characters in `S`.\n\n```\nclass Solution:\n def toGoatLatin(self, S):\n """\n :type S: str\n :rtype: str\n """\n vowels = {\'a\',\'e\',\'i\',\'o\',\'u\',\'A\',\'E\',\'I\',\'O\',\'U\'}\n word_list = S.split()\n for i, word in enumerate(word_list):\n if word[0] in vowels:\n word_list[i] = word+\'ma\'+\'a\'*(i+1)\n else:\n word_list[i] = word[1:]+word[0]+\'ma\'+\'a\'*(i+1)\n return " ".join(word_list)\n``` | 3 | 0 | [] | 0 |
goat-latin | Javascript solution beats 100% | javascript-solution-beats-100-by-thidkya-k6bd | \nfunction goatLatin(s) {\n return s\n .split(" ")\n .map(word => {\n if (word.match(/^[aeiouAEIOU]/g)) {\n return word + "ma";\n } else | thidkyar | NORMAL | 2018-12-18T20:09:38.986332+00:00 | 2018-12-18T20:09:38.986408+00:00 | 351 | false | ```\nfunction goatLatin(s) {\n return s\n .split(" ")\n .map(word => {\n if (word.match(/^[aeiouAEIOU]/g)) {\n return word + "ma";\n } else {\n return word.slice(1) + word.charAt(0) + "ma";\n }\n })\n .map((word, i) => {\n return word + "a".repeat(i + 1);\n }).join(\' \')\n}\n``` | 3 | 0 | [] | 2 |
goat-latin | Easy solution, readable, using StringBuilder and a foreach. | easy-solution-readable-using-stringbuild-hjby | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | jandujo | NORMAL | 2024-11-13T03:41:02.612570+00:00 | 2024-11-13T03:41:02.612653+00:00 | 44 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```csharp []\npublic class Solution {\n public string ToGoatLatin(string sentence) {\n StringBuilder result = new();\n int wordCount = 1;\n var words = sentence.Split(\' \');\n var vowels = "aeiouAEIOU";\n foreach(string word in words)\n {\n if(wordCount > 1)\n result.Append(" ");\n if(vowels.IndexOf(word[0]) > -1)\n result.Append(word).Append("ma");\n else\n result.Append(word.Substring(1,word.Length-1)).Append(word[0]).Append("ma");\n\n for(int i=0; i<wordCount; i++)\n result.Append("a");\n \n wordCount++;\n }\n\n return result.ToString();\n }\n}\n``` | 2 | 0 | ['C#'] | 0 |
goat-latin | Brute Force Approach || Python3 | brute-force-approach-python3-by-lavanya_-kl82 | Intuition\nWhen transforming the input sentence into "Goat Latin," the process primarily revolves around manipulating individual words in the sentence based on | lavanya_immaneni | NORMAL | 2024-10-19T04:48:53.854212+00:00 | 2024-10-19T04:48:53.854236+00:00 | 471 | false | # Intuition\nWhen transforming the input sentence into "Goat Latin," the process primarily revolves around manipulating individual words in the sentence based on whether they start with a vowel or consonant. Additionally, a suffix ("ma") and increasing "a"s are added to each word.\n\n# Approach\n1. Split the sentence: Break the sentence into a list of words.\n2. Process each word:\n * If the word starts with a consonant, move the first letter to the end and append "ma".\n * If the word starts with a vowel, append "ma" without changing the word.\n3. Add suffix: Append a number of "a"s equal to the word\'s position in the sentence (starting with one "a" for the first word, two for the second, etc.).\n4. Rebuild the sentence: Join the transformed words back into a sentence, ensuring proper spacing.\n# Complexity\n- Time complexity: $$O(n)$$ \n\n- Space complexity: $$O(n)$$ \n\n# Code\n```python3 []\nclass Solution:\n def toGoatLatin(self, sentence: str) -> str:\n sentence = sentence.split(" ")\n s = \'a\'\n l = []\n print(sentence)\n for i in sentence:\n if i[0] not in "aeiouAEIOU":\n l.append(i[1:] + i[0] + \'ma\' + s)\n else:\n l.append(i + \'ma\' + s)\n s += \'a\'\n l.append(" ")\n l.pop() \n return "".join(l)\n\n \n``` | 2 | 0 | ['Python3'] | 0 |
goat-latin | Very simple solution. | very-simple-solution-by-srh_abhay-w4kk | Code\npython3 []\nclass Solution:\n def toGoatLatin(self, sentence: str) -> str:\n words = sentence.split()\n vowels = \'aeiouAEIOU\'\n | srh_abhay | NORMAL | 2024-10-12T14:22:11.691689+00:00 | 2024-10-12T14:22:11.691721+00:00 | 450 | false | # Code\n```python3 []\nclass Solution:\n def toGoatLatin(self, sentence: str) -> str:\n words = sentence.split()\n vowels = \'aeiouAEIOU\'\n goat_latin_words = []\n\n for index, word in enumerate(words):\n if word[0] in vowels:\n goat_word = word + "ma"\n else:\n goat_word = word[1:] + word[0] + "ma"\n \n # Add \'a\' based on the word index (1-indexed)\n goat_word += \'a\' * (index + 1)\n goat_latin_words.append(goat_word)\n\n return \' \'.join(goat_latin_words)\n\n\n\n \n``` | 2 | 0 | ['Python3'] | 0 |
goat-latin | Java solution || beats 100% || with explanation | java-solution-beats-100-with-explanation-ahw3 | Intuition\nThe problem seems to require transforming a sentence into "Goat Latin" according to some rules, such as appending "ma" to each word and adding a suff | vidojevica | NORMAL | 2024-05-14T23:07:55.897232+00:00 | 2024-05-14T23:07:55.897263+00:00 | 233 | false | # Intuition\nThe problem seems to require transforming a sentence into "Goat Latin" according to some rules, such as appending "ma" to each word and adding a suffix based on the word\'s position.\n\n# Approach\n1. Split the input sentence into words.\n2. Iterate through each word.\n3. Check if the word starts with a vowel or a consonant.\n4. Append "ma" to each word.\n5. Append a suffix based on the position of the word.\n6. Join the words back into a sentence.\n\n# Complexity\n- Time complexity:\nO(n^2) where n is the average length of words in the\nsentence due to the repeat function.\n\n- Space complexity:\nO(n) for the space used by the new sentence.\n\n# Code\n```\nclass Solution {\n public String toGoatLatin(String sentence) \n {\n String vowels = "aeiouAEIOU";\n\n String[] words = sentence.split(" ");\n StringBuffer newSentence = new StringBuffer();\n int i = 1;\n\n for (String word : words)\n {\n char c = word.charAt(0);\n\n if (vowels.indexOf(c) != -1)\n {\n newSentence.append(word);\n }\n else\n {\n newSentence.append(word.substring(1));\n newSentence.append(c);\n }\n\n newSentence.append("ma");\n newSentence.append("a".repeat(i++));\n newSentence.append(" ");\n } \n\n return newSentence.toString().substring(0, newSentence.length() - 1);\n }\n}\n``` | 2 | 0 | ['String', 'Java'] | 1 |
goat-latin | C++ Simple Solution | c-simple-solution-by-ayushsachan123-dq2k | Complexity\n- Time complexity:\no(n)\n\n- Space complexity:\no(n) \nn -> size of the string\n\n# Code\n\nclass Solution {\npublic:\n\nstring solve(string &s){\n | ayushsachan123 | NORMAL | 2023-12-29T17:38:21.239967+00:00 | 2023-12-29T17:38:21.239999+00:00 | 357 | false | # Complexity\n- Time complexity:\no(n)\n\n- Space complexity:\no(n) \nn -> size of the string\n\n# Code\n```\nclass Solution {\npublic:\n\nstring solve(string &s){\n int n = s.size();\n string ans = "";\n int k=0;\n for(int i=0;i<n;i++){\n int j = i;\n k++;\n string temp = "";\n string res = "";\n while(j<n && s[j] != \' \'){\n if(j==i){\n if(s[j] == \'a\' || s[j] == \'e\' || s[j] == \'i\' || s[j] == \'o\' || s[j] == \'u\' \n || s[j] == \'A\' || s[j] == \'E\' || s[j] == \'I\' || s[j] == \'O\' || s[j] == \'U\'){\n temp += "ma";\n res +=s[j];\n }\n else{\n temp+=s[j];\n temp+="ma";\n }\n }\n else{\n res+=s[j];\n }\n j++;\n }\n res+=temp;\n res.append(k,\'a\');\n ans+=res;\n ans+=" ";\n i=j;\n } \n ans.pop_back();\n return ans;\n}\n\n string toGoatLatin(string sentence) {\n return solve(sentence);\n }\n};\n``` | 2 | 0 | ['String', 'C++'] | 0 |
goat-latin | Python3 code | python3-code-by-manasaanand1711-34fg | Intuition\n1.Split the input sentence into individual words using the .split() method, and store them in a list called w.\n2.Initialize empty lists l and v to s | manasaanand1711 | NORMAL | 2023-10-02T15:23:28.278410+00:00 | 2023-10-02T15:23:28.278436+00:00 | 265 | false | # Intuition\n1.Split the input sentence into individual words using the .split() method, and store them in a list called w.\n2.Initialize empty lists l and v to store the modified words and the final modified words, respectively.\n3.Iterate over each word i in the list w.\n4.Check if the first letter of the word i is a vowel (either lowercase or uppercase). If it is, append the string "ma" to the end of the word i and add it to the list l.\n5.If the first letter of the word i is not a vowel, move the first letter to the end of the word, append "ma" to it, and add it to the list l.\n6.Iterate over the modified words in the list l. For each word at index i, append "a" repeated (i+1) times to the end of the word, and add it to the list v.\n7.Join all the modified words in the list v into a single string, separated by spaces, and store it in the variable m.\n8.Return the final modified string m.\n\n# Approach\nThe input string is first split into words, and then each word is processed individually. \nIf a word starts with a vowel (either lower or upper case), then the string "ma" is appended to the end of the word. Otherwise, the first letter of the word is moved to the end of the word, and then "ma" is appended.\nAfter processing all the words, each modified word is further modified by appending "a" characters to the end of the word, where the number of "a" characters depends on the position of the word in the sentence (starting from 1). \nFinally, all the modified words are joined together into a single string, separated by spaces, and returned as the output of the method.\n\n# Complexity\n- Time complexity:\nO(n^2)\n\n- Space complexity:\nO(n^2)\n\n# Code\n```\nclass Solution:\n def toGoatLatin(self, sentence: str) -> str:\n w = sentence.split()\n l=[]\n v=[]\n for i in w:\n a = 1*i \n if(i[0]==\'a\'or i[0]==\'e\'or i[0]==\'i\' or i[0]==\'o\' or i[0]==\'u\' or i[0]==\'A\'or i[0]==\'E\'or i[0]==\'I\' or i[0]==\'O\' or i[0]==\'U\'):\n i = i+"ma"\n l.append(i)\n else:\n w = i[1:]+i[0]+"ma"\n l.append(w)\n for i in range(len(l)):\n q = l[i]+"a"*(i+1)\n v.append(q)\n m = " ".join(v)\n\n \n return m\n \n``` | 2 | 0 | ['Python3'] | 1 |
goat-latin | easy | easy-by-ratelime-gt8u | \n\nclass Solution:\n def toGoatLatin(self, sentence: str) -> str:\n k = 0\n p = ""\n for x in sentence.split(" "):\n if x[0] | RateLime | NORMAL | 2023-08-11T13:45:07.907000+00:00 | 2023-08-11T13:45:07.907026+00:00 | 281 | false | \n```\nclass Solution:\n def toGoatLatin(self, sentence: str) -> str:\n k = 0\n p = ""\n for x in sentence.split(" "):\n if x[0] not in ("aeiouAEIOU"): \n x = x[1:]+x[0]\n p+=x+"maa"+"a" * k\n k += 1\n if k == len(sentence.split(" ")):\n return p\n p+=" "\n``` | 2 | 0 | ['Python3'] | 1 |
goat-latin | Goat Latin Solution in C++ | goat-latin-solution-in-c-by-the_kunal_si-lnyx | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | The_Kunal_Singh | NORMAL | 2023-05-31T05:08:13.781130+00:00 | 2023-05-31T05:08:13.781169+00:00 | 100 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n*m)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(n)\n# Code\n```\nclass Solution {\npublic:\n string toGoatLatin(string sentence) {\n int i, j, k=1, flag=0;\n string ans="";\n string temp="";\n for(i=0 ; i<sentence.size() ; i++)\n {\n if(flag==0)\n {\n flag=1;\n if(tolower(sentence[i])==\'a\' || tolower(sentence[i])==\'e\' || tolower(sentence[i])==\'i\' || tolower(sentence[i])==\'o\' || tolower(sentence[i])==\'u\')\n {\n ans += sentence[i];\n }\n else\n {\n temp += sentence[i];\n }\n }\n else if(sentence[i]==\' \')\n {\n if(temp.length()>0)\n {\n ans += temp;\n temp.clear();\n }\n ans += "ma";\n for(j=0 ; j<k ; j++)\n {\n ans += \'a\';\n }\n ans += \' \';\n flag=0;\n k++;\n }\n else\n {\n ans += sentence[i];\n }\n }\n if(temp.length()>0)\n {\n ans += temp;\n temp.clear();\n }\n ans += "ma";\n for(j=0 ; j<k ; j++)\n {\n ans += \'a\';\n }\n return ans;\n }\n};\n```\n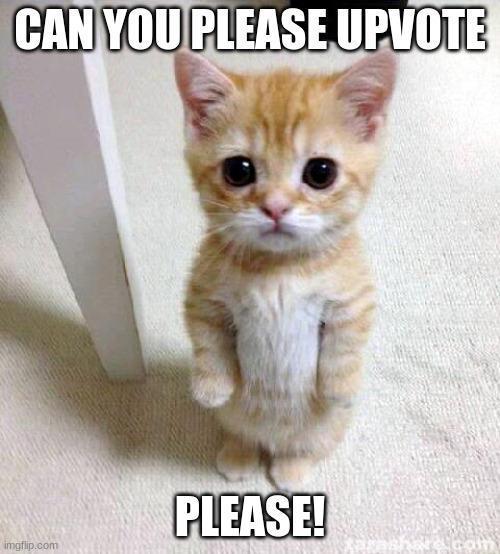\n | 2 | 0 | ['C++'] | 0 |
goat-latin | Solution | solution-by-deleted_user-oybo | C++ []\nclass Solution {\npublic:\n string toGoatLatin(string s) {\n string res;\n int count=0;\n for(int i=0; i<s.length(); i++){\n | deleted_user | NORMAL | 2023-05-03T12:34:02.823879+00:00 | 2023-05-03T13:46:58.996653+00:00 | 1,058 | false | ```C++ []\nclass Solution {\npublic:\n string toGoatLatin(string s) {\n string res;\n int count=0;\n for(int i=0; i<s.length(); i++){\n string temp;\n while(s[i]!=\' \' && i<s.length()){\n temp +=s[i];\n i++;\n }\n count++;\n if(temp[0]!=\'a\' && temp[0]!=\'e\' && temp[0]!=\'i\' && temp[0]!=\'o\' && temp[0]!=\'u\'&& temp[0]!=\'A\' && temp[0]!=\'E\' && temp[0]!=\'I\' && temp[0]!=\'O\' && temp[0]!=\'U\'){\n char t;\n t = temp[0];\n for(int k=0; k<temp.length()-1; k++){\n temp[k]=temp[k+1];\n }\n temp[temp.length()-1]=t;\n }\n temp+="ma";\n for(int j=0; j<count; j++){\n temp+="a";\n }\n res+=temp+" ";\n }\n return res.substr(0,res.length()-1);\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def toGoatLatin(self, sentence: str) -> str:\n \n vowels = [\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\']\n words = sentence.split()\n for i in range(len(words)):\n if words[i][0] in vowels:\n words[i] += \'ma\'\n else:\n words[i] = words[i][1:] + words[i][0] + \'ma\'\n words[i] += \'a\' * (i+1)\n return \' \'.join(words)\n```\n\n```Java []\nclass Solution {\n static final char[] vowels = new char[10];\n static {\n vowels[0] = \'A\';\n vowels[1] = \'E\';\n vowels[2] = \'I\';\n vowels[3] = \'O\';\n vowels[4] = \'U\';\n vowels[5] = \'a\';\n vowels[6] = \'e\';\n vowels[7] = \'i\';\n vowels[8] = \'o\';\n vowels[9] = \'u\';\n }\n private static boolean isConsonant(char ch) {\n return Arrays.binarySearch(vowels, ch) < 0;\n }\n public String toGoatLatin(String sentence) {\n final StringBuilder sb = new StringBuilder();\n int count = 0;\n final char[] chars = sentence.toCharArray();\n final int length = chars.length;\n for (int index = 0; index < length;) {\n if (index > 0) {\n sb.append(\' \');\n }\n final char firstChar = chars[index];\n char next = firstChar;\n final boolean isConsonant = isConsonant(firstChar);\n if (isConsonant) {\n if (++index >= length) {\n next = \' \';\n } else {\n next = chars[index];\n }\n }\n while (next != \' \') {\n sb.append(next);\n if (++index >= length) {\n break;\n }\n next = chars[index];\n }\n if (isConsonant) {\n sb.append(firstChar);\n }\n sb.append("ma");\n ++count;\n for (int i = 0; i < count; i++) {\n sb.append(\'a\');\n }\n ++index;\n }\n return sb.toString();\n }\n}\n```\n | 2 | 0 | ['C++', 'Java', 'Python3'] | 0 |
goat-latin | Easy to Understand Code || Code for beginners ✅✅✅ | easy-to-understand-code-code-for-beginne-4uzf | ```\nstring toGoatLatin(string sentence) {\n string res,word,a="a";\n stringstream ss(sentence);\n while(ss>>word){ \n\t\t\tif(word[0]== | kunalbatham2701 | NORMAL | 2023-02-08T13:46:03.176373+00:00 | 2023-02-08T13:46:03.176401+00:00 | 293 | false | ```\nstring toGoatLatin(string sentence) {\n string res,word,a="a";\n stringstream ss(sentence);\n while(ss>>word){ \n\t\t\tif(word[0]==\'a\'||word[0]==\'e\'||word[0]==\'i\'||word[0]==\'o\'||word[0]==\'u\'||word[0]==\'A\'||word[0]==\'E\'||word[0]==\'I\'||word[0]==\'O\'||word[0]==\'U\')\n res+=word+"ma";\n else\n res+=word.substr(1)+word[0]+"ma";\n res+=a+" ";\n a+="a";\n }\n return res.substr(0,res.size()-1);\n } | 2 | 0 | ['C'] | 0 |
goat-latin | Java solution without string split | java-solution-without-string-split-by-co-3ayy | ```\nclass Solution {\n \n static final char[] vowels = new char[10];\n static {\n vowels[0] = \'A\';\n vowels[1] = \'E\';\n vowel | coffeeminator | NORMAL | 2022-11-23T18:29:19.683489+00:00 | 2022-11-23T18:29:19.683584+00:00 | 524 | false | ```\nclass Solution {\n \n static final char[] vowels = new char[10];\n static {\n vowels[0] = \'A\';\n vowels[1] = \'E\';\n vowels[2] = \'I\';\n vowels[3] = \'O\';\n vowels[4] = \'U\';\n vowels[5] = \'a\';\n vowels[6] = \'e\';\n vowels[7] = \'i\';\n vowels[8] = \'o\';\n vowels[9] = \'u\';\n }\n\n private static boolean isConsonant(char ch) {\n return Arrays.binarySearch(vowels, ch) < 0;\n }\n \n public String toGoatLatin(String sentence) {\n final StringBuilder sb = new StringBuilder();\n \n int count = 0;\n final char[] chars = sentence.toCharArray();\n final int length = chars.length;\n for (int index = 0; index < length;) {\n if (index > 0) {\n sb.append(\' \');\n }\n final char firstChar = chars[index];\n char next = firstChar;\n final boolean isConsonant = isConsonant(firstChar);\n if (isConsonant) {\n if (++index >= length) {\n next = \' \';\n } else {\n next = chars[index];\n }\n }\n while (next != \' \') {\n sb.append(next);\n if (++index >= length) {\n break;\n }\n next = chars[index];\n }\n if (isConsonant) {\n sb.append(firstChar);\n }\n sb.append("ma");\n ++count;\n for (int i = 0; i < count; i++) {\n sb.append(\'a\');\n }\n ++index;\n }\n \n return sb.toString();\n }\n} | 2 | 0 | ['Binary Tree', 'Java'] | 0 |
goat-latin | Python Easy | python-easy-by-lucasschnee-jt3q | \tclass Solution:\n\t\tdef toGoatLatin(self, sentence: str) -> str:\n\t\t\tlst = sentence.split(" ")\n\n\t\t\tlst2 = []\n\n\t\t\tvowels = ["a", "e", "i", "o", " | lucasschnee | NORMAL | 2022-10-25T13:31:07.186124+00:00 | 2022-10-25T13:31:07.186159+00:00 | 626 | false | \tclass Solution:\n\t\tdef toGoatLatin(self, sentence: str) -> str:\n\t\t\tlst = sentence.split(" ")\n\n\t\t\tlst2 = []\n\n\t\t\tvowels = ["a", "e", "i", "o", "u"]\n\n\t\t\tfor i, word in enumerate(lst):\n\t\t\t\ttmp = word\n\n\t\t\t\tif (word[0].lower() in vowels):\n\t\t\t\t\ttmp = tmp + "ma"\n\n\t\t\t\telse: \n\t\t\t\t\ttmp2 = tmp[1:] + tmp[0] + "ma" \n\t\t\t\t\ttmp = tmp2\n\n\t\t\t\ttmp = tmp + ("a" * (i + 1))\n\n\t\t\t\tlst2.append(tmp)\n\n\t\t\tsentence_latin = " ".join(lst2)\n\n\t\t\treturn sentence_latin\n\n\n | 2 | 0 | ['Python', 'Python3'] | 0 |
goat-latin | Java Solution || Faster than 97% (Normal String Manipulation) | java-solution-faster-than-97-normal-stri-s1qe | Please upvote if you like the solution-->\n\n\nclass Solution {\n public static boolean isVowel(char c){\n return c == \'a\' || c == \'e\' || c == \'i | Ritabrata_1080 | NORMAL | 2022-10-23T15:57:52.739164+00:00 | 2022-10-23T15:57:52.739206+00:00 | 1,442 | false | # Please upvote if you like the solution-->\n\n```\nclass Solution {\n public static boolean isVowel(char c){\n return c == \'a\' || c == \'e\' || c == \'i\' || c == \'o\' || c == \'u\';\n }\n public String toGoatLatin(String sentence) {\n List<String> list = new ArrayList<>();\n String arr[] = sentence.split(" ");\n StringBuilder sb = new StringBuilder();\n for(int i = 0; i < arr.length;i++){\n char detachedOriginal = arr[i].charAt(0);\n char detach = arr[i].toLowerCase().charAt(0);\n String rem = arr[i].substring(1);\n if(isVowel(detach)){\n sb.append(arr[i]);\n sb.append("ma");\n for(int j = 0;j<=i;j++){\n sb.append("a");\n }\n }\n else{\n sb.append(rem);\n sb.append(detachedOriginal);\n sb.append("ma");\n for(int j = 0;j<=i;j++){\n sb.append("a");\n }\n }\n list.add(sb.toString());\n sb.setLength(0);\n }\n for(int i = 0;i<list.size() - 1;i++){\n sb.append(list.get(i));\n sb.append(" ");\n }\n sb.append(list.get(list.size()-1));\n return sb.toString();\n }\n}\n``` | 2 | 0 | ['String', 'Java'] | 0 |
goat-latin | 🐌 C# - linq | c-linq-by-user7166uf-v5bd | \npublic class Solution\n{\n public string ToGoatLatin(string sentence) \n => string.Join(\' \', sentence.Split(\' \').Select(ConvertWordToGoatLatin)) | user7166Uf | NORMAL | 2022-06-13T18:17:25.286780+00:00 | 2022-06-13T18:17:25.286817+00:00 | 81 | false | ```\npublic class Solution\n{\n public string ToGoatLatin(string sentence) \n => string.Join(\' \', sentence.Split(\' \').Select(ConvertWordToGoatLatin));\n\n private static string ConvertWordToGoatLatin(string word, int index)\n => char.ToLower(word[0]) is not (\'a\' or \'e\' or \'i\' or \'o\' or \'u\')\n ? word[1..] + word[0] + "ma" + new string(\'a\', index + 1)\n : word + "ma" + new string(\'a\', index + 1);\n}\n``` | 2 | 0 | ['C#'] | 0 |
goat-latin | Easy CPP Solution | easy-cpp-solution-by-siddharthphalle-0p6o | ```\nclass Solution {\npublic:\n string toGoatLatin(string sentence) {\n unordered_set vowels = {\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\ | SiddharthPhalle | NORMAL | 2022-05-30T15:22:38.819548+00:00 | 2022-05-30T15:22:38.819577+00:00 | 313 | false | ```\nclass Solution {\npublic:\n string toGoatLatin(string sentence) {\n unordered_set<char> vowels = {\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\'};\n string word = "", res= "";\n stringstream ss(sentence);\n int cnt = 1;\n while(ss>>word)\n { \n if(!vowels.count(word[0]))\n word = word.substr(1, word.length()-1) + word[0];\n res += word + "ma" + addA(cnt++);\n }\n res.erase(res.end()-1); //To remove last space \n return res;\n }\n \n string addA(int cnt) {\n string a = "";\n for(int i=0; i<cnt; i++)\n a += "a";\n return a+" ";\n }\n}; | 2 | 0 | ['C'] | 0 |
goat-latin | Easy Python code beats 99.8% | easy-python-code-beats-998-by-vistrit-yc89 | \n def toGoatLatin(self, sentence: str) -> str:\n words = sentence.split()\n vowels = "AEIOUaeiou"\n m="maa"\n l=[]\n for | vistrit | NORMAL | 2021-11-03T03:33:46.557862+00:00 | 2021-11-03T03:33:46.557904+00:00 | 125 | false | ```\n def toGoatLatin(self, sentence: str) -> str:\n words = sentence.split()\n vowels = "AEIOUaeiou"\n m="maa"\n l=[]\n for i in words:\n if i[0] in vowels:\n l.append(i + m)\n else:\n l.append(i[1:] + i[0] + m)\n m+="a" \n return " ".join(l)\n``` | 2 | 0 | ['Python'] | 0 |
goat-latin | Time and Space Complexity of Python Solution | time-and-space-complexity-of-python-solu-kfz3 | \n \n vowels = set("aeiouAEIOU")\n ma="ma"\n \n def goat_latin(word, index):\n \n end = "a"(index+1)\n | venom736 | NORMAL | 2021-07-24T09:00:31.756049+00:00 | 2021-07-24T09:06:58.601762+00:00 | 68 | false | \n \n vowels = set("aeiouAEIOU")\n ma="ma"\n \n def goat_latin(word, index):\n \n end = "a"*(index+1)\n if word[0] not in vowels:\n \n word = word[1:] + word[0]\n \n return word + ma + end\n \n return " ".join(goat_latin(w,i) for i,w in enumerate(sentence.split()))\n\n*N = Length of String, W = Number of Words in the string*\n\n**Time Complexity:**\n\n* sentence.split() --> O(N)\n\n* Looping over every word enumerate(sentence.split()) -> O(W)\nFor each loop above\n\t1. Suffix creation - > 1+2+3+ .... +W \n\t2. New Word Creation -> O(W)\n\n\t*Total for Loop -> O(W^2)*\n\n* Stiching back the result string O(N + new_added_letters)\n\n\tnew_added_letters -> Suffix + \'ma\' = (W^2 - W)/2 + 2W = (W^2 + 3W)/2\n\tStiching back the result string O(N + (W^2 + 3W)/2) = O(N+W+W^2)\n\n\n*Total Time Complexity ->\nO(N) + O(W^2) + O(N+W+W^2)*\n\n*As avg length of words is 4.7(google) .W = N/4.7\nO(N+W+W^2) equivalent to O(W^2)*\n\n\n**Space Complexity**\nsentence.split() -> O(N)\n\nCreating Suffix + New Word -> O(W^2 + W)\n\nSitching back new string -> O(N+W+W^2)\n\nTotal Space Complexity: O(N+W^2 + W)\n\nAgain considering 4.7 avg length of words. W = N/4.7\n\nTotal Space Complexity: O(N+W^2 + W) equivalent to O(W^2)\n\n\n\n\n\n\n\n | 2 | 0 | [] | 0 |
goat-latin | (C++) 824. Goat Latin | c-824-goat-latin-by-qeetcode-2jtz | \n\nclass Solution {\npublic:\n string toGoatLatin(string S) {\n string ans, word, vowels = "aeiouAEIOU", suffix; \n istringstream iss(S); \n | qeetcode | NORMAL | 2021-04-15T19:55:44.299431+00:00 | 2021-04-15T19:55:44.299475+00:00 | 190 | false | \n```\nclass Solution {\npublic:\n string toGoatLatin(string S) {\n string ans, word, vowels = "aeiouAEIOU", suffix; \n istringstream iss(S); \n ostringstream oss; \n \n while (iss >> word) {\n suffix.push_back(\'a\'); \n if (vowels.find(word[0]) == string::npos) \n word = word.substr(1) + word[0]; \n oss << " " << word << "ma" << suffix; \n }\n return oss.str().substr(1); \n }\n};\n``` | 2 | 0 | ['C'] | 1 |
goat-latin | Simple Solution In Java | simple-solution-in-java-by-s34-k8f4 | \nclass Solution {\n public String toGoatLatin(String S) {\n String[] tmp=S.split(" ");\n String res="";\n Set<Character> s=new HashSet< | s34 | NORMAL | 2021-02-22T01:36:43.900573+00:00 | 2021-02-22T01:36:43.900612+00:00 | 253 | false | ```\nclass Solution {\n public String toGoatLatin(String S) {\n String[] tmp=S.split(" ");\n String res="";\n Set<Character> s=new HashSet<>();\n s.add(\'a\');s.add(\'e\');s.add(\'i\');s.add(\'o\');s.add(\'u\');\n s.add(\'A\');s.add(\'E\');s.add(\'I\');s.add(\'O\');s.add(\'U\');\n \n for(int i=0;i<tmp.length;i++){\n //Vowel\n if(s.contains(tmp[i].charAt(0))){\n res+=tmp[i]+"ma";\n }\n else{\n res+=tmp[i].substring(1,tmp[i].length())+tmp[i].charAt(0)+"ma";\n }\n int j=0;\n while(j<i+1){\n res+="a";\n j++;\n }\n if(i!=tmp.length-1)\n res+=" ";\n }\n return res;\n }\n}\n```\nPlease **upvote**, if you like the solution:) | 2 | 0 | [] | 0 |
goat-latin | Simple Python Solution | simple-python-solution-by-lokeshsk1-92r3 | \nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n out=\'\'\n l=S.split()\n for i in range(len(l)):\n if l[i][0] in | lokeshsk1 | NORMAL | 2020-11-24T18:26:05.502944+00:00 | 2020-11-24T18:26:33.632849+00:00 | 193 | false | ```\nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n out=\'\'\n l=S.split()\n for i in range(len(l)):\n if l[i][0] in \'aeiouAEIOU\':\n out+=l[i]+\'m\'+\'a\'*(i+2)+\' \'\n else:\n out+=l[i][1:]+l[i][0]+\'m\'+\'a\'*(i+2)+\' \'\n return out[:-1]\n``` | 2 | 0 | ['Python', 'Python3'] | 0 |
goat-latin | Clean JavaScript Solution | clean-javascript-solution-by-shimphillip-pyv3 | \n// time O(n^2) time O(1)\nvar toGoatLatin = function(S) {\n const vowels = \'aeiouAEIOU\'\n const arr = S.split(\' \')\n \n for(let i=0; i<arr.len | shimphillip | NORMAL | 2020-11-09T03:03:19.505683+00:00 | 2020-11-09T03:03:19.505732+00:00 | 418 | false | ```\n// time O(n^2) time O(1)\nvar toGoatLatin = function(S) {\n const vowels = \'aeiouAEIOU\'\n const arr = S.split(\' \')\n \n for(let i=0; i<arr.length; i++) {\n let word = arr[i]\n const firstChar = word[0]\n\n if(!vowels.includes(firstChar)) {\n word = word.slice(1) + firstChar\n }\n \n word += \'ma\'\n \n for(let j=-1; j<i; j++) {\n word += \'a\'\n }\n \n arr[i] = word\n }\n \n return arr.join(\' \')\n};\n``` | 2 | 0 | ['JavaScript'] | 1 |
goat-latin | [Kotlin] 2 lines of code, the power of FP | kotlin-2-lines-of-code-the-power-of-fp-b-4asx | \nfun toGoatLatin(S: String): String {\n val a = StringBuilder("a")\n return S.split(" ").joinToString(" ") {\n if ("aeiou".contains(it[0].toLowerC | nurbekinho | NORMAL | 2020-08-20T12:35:11.888189+00:00 | 2020-08-20T12:35:11.888236+00:00 | 86 | false | ```\nfun toGoatLatin(S: String): String {\n val a = StringBuilder("a")\n return S.split(" ").joinToString(" ") {\n if ("aeiou".contains(it[0].toLowerCase())) (it + "ma" + a).also { a.append("a") }\n else (it.substring(1) + it[0] + "ma" + a).also { a.append("a") }\n }\n}\n``` | 2 | 0 | ['Kotlin'] | 0 |
goat-latin | Java solution | java-solution-by-anand_ms-83pe | \nclass Solution {\n public String toGoatLatin(String s) {\n\n String splitSentence[] = s.split(" ");\n String appendStr = "a";\n\n for | anand_ms | NORMAL | 2020-08-20T03:14:42.374852+00:00 | 2020-08-20T03:14:42.374887+00:00 | 120 | false | ```\nclass Solution {\n public String toGoatLatin(String s) {\n\n String splitSentence[] = s.split(" ");\n String appendStr = "a";\n\n for (int i = 0; i < splitSentence.length; i++) {\n\n String word = splitSentence[i];\n char firstLetter = Character.toLowerCase(word.charAt(0));\n\n if (firstLetter == \'a\' || firstLetter == \'e\' || firstLetter == \'i\' || firstLetter == \'o\' || firstLetter == \'u\') {\n\n splitSentence[i] = splitSentence[i] + "ma" + appendStr;\n } else {\n\n String char1 = String.valueOf(splitSentence[i].charAt(0));\n splitSentence[i] = splitSentence[i].substring(1) + char1 + "ma" + appendStr;\n\n }\n\n appendStr += "a";\n }\n\n return String.join(" ", splitSentence);\n }\n}\n``` | 2 | 0 | [] | 0 |
goat-latin | [C++] Best Method, Run Time 0ms, 100% | c-best-method-run-time-0ms-100-by-ravire-tuf0 | Goat Latin Best Solution, Leetcode\n\nRuntime: 8 ms\nMemory: 6.5 MB, less than Top 84.27%\n\n\n\nclass Solution\n{\npublic:\n vector<string> split(string st | ravireddy07 | NORMAL | 2020-08-19T20:24:03.499023+00:00 | 2020-08-22T18:38:05.074252+00:00 | 105 | false | ### Goat Latin Best Solution, Leetcode\n\n**Runtime**: 8 ms\n**Memory**: 6.5 MB, less than Top 84.27%\n\n\n```\nclass Solution\n{\npublic:\n vector<string> split(string str, string token)\n {\n vector<string> result;\n while (str.size())\n {\n int index = str.find(token);\n if (index != string::npos)\n {\n result.push_back(str.substr(0, index));\n str = str.substr(index + token.size());\n if (str.size() == 0)\n result.push_back(str);\n }\n else\n {\n result.push_back(str);\n str = "";\n }\n }\n return result;\n }\n\n string toGoatLatin(string S)\n {\n vector<string> a;\n int count = 0, ll, checkVowel;\n string temp, rule1 = "ma", rule3 = "a", result;\n char f;\n a = split(S, " ");\n for (int i = 0; i < a.size(); i++)\n {\n temp = a[i];\n f = temp[0];\n checkVowel = (f == \'a\' || f == \'e\' || f == \'i\' || f == \'o\' || f == \'u\' || f == \'A\' || f == \'E\' || f == \'I\' || f == \'O\' || f == \'U\');\n if (checkVowel)\n {\n temp.append(rule1);\n count++;\n }\n else\n {\n ll = temp.length();\n for (int k = 0; k < ll - 1; k++)\n {\n temp[k] = temp[k + 1];\n }\n temp[ll - 1] = f;\n temp.append(rule1);\n count++;\n }\n\n for (int d = 0; d < count; d++)\n {\n temp.append(rule3);\n }\n\n if (i == 0)\n {\n result.append(temp);\n }\n else\n {\n result.append(" ");\n result.append(temp);\n }\n }\n return result;\n }\n} ;\n``` | 2 | 0 | [] | 0 |
goat-latin | 2 different JS solution | 2-different-js-solution-by-hon9g-wd21 | vowels in set\n- Time Complexity: O(N)\n- Space Complexity: O(N)\nJavaScript\nconst vowels = new Set([\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \' | hon9g | NORMAL | 2020-08-19T16:00:05.502282+00:00 | 2020-08-19T16:00:05.502334+00:00 | 249 | false | ## vowels in set\n- Time Complexity: O(N)\n- Space Complexity: O(N)\n```JavaScript\nconst vowels = new Set([\'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\'])\n/**\n * @param {string} S\n * @return {string}\n */\nvar toGoatLatin = function(S) {\n return S.split(\' \').map(\n (word, i) => `${vowels.has(word[0]) ? word : word.slice(1) + word[0]}ma${"a".repeat(i+1)}`\n ).join(\' \')\n};\n````\n\n## vowels with regex\n```JavaScript\n/**\n * @param {string} S\n * @return {string}\n */\nvar toGoatLatin = function(S) {\n return S.split(\' \').map(\n (word, i) => `${/[aeiou]/i.test(word[0]) ? word : word.slice(1) + word[0]}ma${"a".repeat(i+1)}`\n ).join(\' \')\n};\n``` | 2 | 0 | ['Ordered Set', 'JavaScript'] | 0 |
goat-latin | faster than 100%, easy solution | faster-than-100-easy-solution-by-jinkim-r10j | \nclass Solution {\npublic:\n string toGoatLatin(string S) {\n string word = "";\n vector<string> words;\n string res = "";\n for | jinkim | NORMAL | 2020-08-19T14:40:00.370207+00:00 | 2020-08-19T14:40:00.370263+00:00 | 238 | false | ```\nclass Solution {\npublic:\n string toGoatLatin(string S) {\n string word = "";\n vector<string> words;\n string res = "";\n for (char c: S) {\n if (c == \' \') {\n words.push_back(word);\n word = "";\n } else {\n word += c;\n }\n }\n if (word != "") words.push_back(word);\n int c = 1;\n // words all separated\n string curr = "";\n for (string word: words) {\n if (isVowel(word[0])) { // vowel case\n curr = word + "ma";\n } else { // consonant case\n for (size_t i = 1; i < word.size(); i++) {\n curr += word[i];\n }\n curr += word[0];\n curr += "ma";\n }\n // add \'a\'\n for (size_t i = 0; i < c; i++) {\n curr += \'a\';\n }\n res = res + curr + \' \';\n c++;\n curr = "";\n }\n if (c != 1) res.pop_back(); // placeholder space removed\n return res;\n }\n \n bool isVowel(char c) {\n if (tolower(c) == \'a\' || tolower(c) == \'e\' || tolower(c) == \'i\' || \n tolower(c) == \'o\' || tolower(c) == \'u\') {\n return true;\n } else {\n return false;\n }\n }\n};\n``` | 2 | 2 | ['C'] | 0 |
goat-latin | Cpp code faster than 100% | Straight solution | Just do what is asked | cpp-code-faster-than-100-straight-soluti-fkxb | \nclass Solution {\npublic:\n bool checkVowel(char ch)\n {\n if(ch==\'a\' || ch==\'e\' || ch==\'i\' || ch==\'o\' || ch==\'u\' || ch==\'A\' || ch==\ | mayanktolani19 | NORMAL | 2020-08-19T11:34:04.197800+00:00 | 2020-08-19T11:34:04.197834+00:00 | 127 | false | ```\nclass Solution {\npublic:\n bool checkVowel(char ch)\n {\n if(ch==\'a\' || ch==\'e\' || ch==\'i\' || ch==\'o\' || ch==\'u\' || ch==\'A\' || ch==\'E\' || ch==\'I\' || ch==\'O\' || ch==\'U\')\n return true;\n return false;\n }\n string toGoatLatin(string S) {\n S = S+" ";\n int i, l = S.size(), a = 0;\n string app = "a", word = "", ans = "";\n for(i=0;i<l;i++)\n {\n if(S[i]==\' \')\n {\n word = S.substr(a,i-a);\n if(!checkVowel(word[0]))\n word = word.substr(1)+word[0];\n word += ("ma"+app);\n app += "a";\n ans += (word+" ");\n a = i+1;\n }\n }\n return ans.substr(0,ans.size()-1);\n }\n};\n``` | 2 | 0 | [] | 0 |
goat-latin | Python Super Simple&Clear Solution with explanations | python-super-simpleclear-solution-with-e-c6ql | \nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n\t\n\t\t# split sentence to words\n splited_sentence = S.split(" ")\n\t\t\n\t\t# list of go | debbiealter | NORMAL | 2020-08-19T07:50:21.274575+00:00 | 2020-08-19T08:11:50.868967+00:00 | 49 | false | ```\nclass Solution:\n def toGoatLatin(self, S: str) -> str:\n\t\n\t\t# split sentence to words\n splited_sentence = S.split(" ")\n\t\t\n\t\t# list of goat latin words\n goat_latin = []\n\t\t\n vowels = "aAeEiIoOuU"\n\t\t\n\t\t# to keep count of how many "a"s to add\n index = 1\n \n for word in splited_sentence:\n\t\t\n\t\t\t# convert word to goat latin according to case\n goat_latin_word = word + "ma" + "a" * index if word[0] in vowels else word[1:] + word[0] + "ma" + "a" * index\n \n\t\t\t # increment for next word\n index += 1\n\t\t\t#add goat latin word to list \n goat_latin.append(goat_latin_word)\n \n\t\t\t\n\t\t# join all goat latin words to a sentence\n return " ".join(goat_latin)\n``` | 2 | 2 | ['Python3'] | 0 |
goat-latin | C++ || Easy and simple | c-easy-and-simple-by-satifer-7ag7 | ```\nclass Solution {\npublic:\n string convert(string s, int count)\n {\n int len = s.length();\n string temp = "";\n if(s[0] == \'a | satifer | NORMAL | 2020-07-21T16:26:33.508986+00:00 | 2020-07-21T16:26:33.509040+00:00 | 301 | false | ```\nclass Solution {\npublic:\n string convert(string s, int count)\n {\n int len = s.length();\n string temp = "";\n if(s[0] == \'a\' || s[0] == \'e\' || s[0] == \'i\' || s[0] == \'o\' || s[0] == \'u\' || s[0] == \'A\' || s[0] == \'E\' || s[0] == \'I\' || s[0] == \'O\' || s[0] == \'U\')\n {\n temp = s;\n temp += "ma";\n }\n else\n {\n char first = s[0];\n temp = s.substr(1, len-1);\n temp += first;\n temp += "ma";\n }\n for(int i=0; i<count; i++)\n temp += "a";\n string res = temp + " ";\n return res;\n }\n string toGoatLatin(string S) \n {\n stringstream ss(S);\n string word, temp;\n int count = 1;\n while(ss >> word)\n {\n temp += convert(word, count);\n count++;\n }\n return temp.substr(0, temp.length()-1);\n }\n}; | 2 | 0 | ['C', 'C++'] | 0 |
goat-latin | [go] double 100% straightforward solution | go-double-100-straightforward-solution-b-k8fn | go\n// Runtime: 0 ms, faster than 100.00% of Go online submissions for Goat Latin.\n// Memory Usage: 2.2 MB, less than 100.00% of Go online submissions for Goat | wenfengg | NORMAL | 2020-03-20T00:48:22.708339+00:00 | 2020-03-20T00:48:22.708394+00:00 | 60 | false | ```go\n// Runtime: 0 ms, faster than 100.00% of Go online submissions for Goat Latin.\n// Memory Usage: 2.2 MB, less than 100.00% of Go online submissions for Goat Latin.\nfunc toGoatLatin(S string) string {\n\tstrs := strings.Split(S, " ")\n\tvar as string\n\tfor i := range strs {\n\t\tas += "a"\n\t\tswitch strs[i][0] {\n\t\tcase \'a\', \'e\', \'i\', \'o\', \'u\', \'A\', \'E\', \'I\', \'O\', \'U\':\n\t\tdefault:\n\t\t\tstrs[i] = (strs[i] + string(strs[i][0]))[1:]\n\t\t}\n\t\tstrs[i] += "ma" + as\n\t}\n\treturn strings.Join(strs, " ")\n}\n``` | 2 | 0 | [] | 1 |
goat-latin | JAVA - Very Easy Solution (Highly Efficient) | java-very-easy-solution-highly-efficient-29gz | \npublic String toGoatLatin(String S) {\n\tString[] words = S.split(" ");\n\tStringBuilder a = new StringBuilder(""), sol = new StringBuilder();\n\tSet<Characte | anubhavjindal | NORMAL | 2019-11-28T07:37:55.954310+00:00 | 2019-11-28T07:38:55.280887+00:00 | 191 | false | ```\npublic String toGoatLatin(String S) {\n\tString[] words = S.split(" ");\n\tStringBuilder a = new StringBuilder(""), sol = new StringBuilder();\n\tSet<Character> vowels = new HashSet<>();\n\tvowels.add(\'a\'); vowels.add(\'e\'); vowels.add(\'i\'); vowels.add(\'o\'); vowels.add(\'u\'); \n\tfor(int i = 0; i<words.length; i++) {\n\t\tString w = words[i];\n\t\ta.append("a");\n\t\tchar first = Character.toLowerCase(w.charAt(0));\n\t\tif(vowels.contains(first))\n\t\t\tsol.append(w).append("ma").append(a);\n\t\telse\n\t\t\tsol.append(w.substring(1,w.length())).append(w.charAt(0)).append("ma").append(a);\n\t\tif(i!=words.length-1) sol.append(" ");\n\t}\n\treturn sol.toString();\n }\n``` | 2 | 1 | [] | 1 |
goat-latin | easy peasy python solution - split into list | easy-peasy-python-solution-split-into-li-y1xg | \tdef toGoatLatin(self, S: str) -> str:\n ls = S.split()\n for i in range(len(ls)):\n if ls[i][0].lower() in [\'a\', \'e\', \'i\', \'o\ | lostworld21 | NORMAL | 2019-08-18T13:38:55.330326+00:00 | 2019-08-18T13:38:55.330382+00:00 | 149 | false | \tdef toGoatLatin(self, S: str) -> str:\n ls = S.split()\n for i in range(len(ls)):\n if ls[i][0].lower() in [\'a\', \'e\', \'i\', \'o\', \'u\']:\n ls[i] = ls[i] + "ma" + (i+1)*"a"\n else:\n ls[i] = ls[i][1:] + ls[i][0] + "ma" + + (i+1)*"a"\n \n \n return " ".join(ls) | 2 | 0 | [] | 0 |
goat-latin | Javascript (four 1-line functions) | javascript-four-1-line-functions-by-stro-f1fy | \nconst toGoatLatin = S => S.split(\' \').map((w, i) => isVow(w) ? vow(w, i) : con(w, i)).join(\' \');\nconst isVow = w => [\'a\', \'e\', \'i\', \'o\', \'u\'].i | strom415 | NORMAL | 2019-07-07T03:18:08.407723+00:00 | 2019-07-07T03:18:08.407754+00:00 | 104 | false | ```\nconst toGoatLatin = S => S.split(\' \').map((w, i) => isVow(w) ? vow(w, i) : con(w, i)).join(\' \');\nconst isVow = w => [\'a\', \'e\', \'i\', \'o\', \'u\'].includes(w[0].toLowerCase());\nconst vow = (w, i) => w + \'ma\' + \'a\'.repeat(i + 1);\nconst con = (w, i) => w.slice(1, w.length) + w[0] + \'ma\' + \'a\'.repeat(i + 1);\n``` | 2 | 0 | [] | 1 |
goat-latin | Haskell and Python Solution | haskell-and-python-solution-by-code_repo-49zk | Python:\n\ndef translate(i: int, w: str) -> str:\n return (w if w[0].lower() in \'aeiou\' else w[1:] + w[0]) + \'ma\' + i * \'a\'\n\nclass Solution:\n def | code_report | NORMAL | 2019-04-27T07:34:31.344374+00:00 | 2019-04-27T07:34:31.344511+00:00 | 147 | false | **Python:**\n```\ndef translate(i: int, w: str) -> str:\n return (w if w[0].lower() in \'aeiou\' else w[1:] + w[0]) + \'ma\' + i * \'a\'\n\nclass Solution:\n def toGoatLatin(self, s: str) -> str:\n return \' \'.join(translate(i, w) for i, w in enumerate(s.split(), 1))\n```\n**Haskell:**\n```\ntranslate :: (Int, String) -> String\ntranslate (i, w)\n | elem (toLower (head w)) "aeiou" = w ++ as\n | otherwise = (tail w) ++ [head w] ++ as\n where as = "ma" ++ replicate i \'a\'\n\nsolve :: String -> String\nsolve = unwords . map translate . zip [1..] . words\n``` | 2 | 0 | ['Python3'] | 0 |
goat-latin | JavaScript solution 52 ms beats 100% submissions | javascript-solution-52-ms-beats-100-subm-d9ns | js\n/**\n * @param {string} S\n * @return {string}\n */\nvar toGoatLatin = function (S) {\n const VOWELS = new Set([\'A\', \'a\', \'E\', \'e\', \'I\', \'i\', | naansensical | NORMAL | 2018-10-23T16:44:22.348767+00:00 | 2019-11-19T03:46:42.245699+00:00 | 139 | false | ```js\n/**\n * @param {string} S\n * @return {string}\n */\nvar toGoatLatin = function (S) {\n const VOWELS = new Set([\'A\', \'a\', \'E\', \'e\', \'I\', \'i\', \'O\', \'o\', \'U\', \'u\']);\n\n return S.split(\' \').map((word, i) => {\n const res = VOWELS.has(word[0]) ? word : word.substring(1) + word[0];\n return res + \'ma\' + \'a\'.repeat(i + 1);\n }).join(\' \');\n};\n``` | 2 | 0 | [] | 0 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.