question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
most-profitable-path-in-a-tree | C++✅✅| O(n) | Simplest approach🚀🚀 | c-on-simplest-approach-by-aayu_t-qhqt | Complexity
Time complexity: O(N)
Space complexity: O(N)
Code | aayu_t | NORMAL | 2025-03-22T11:54:48.999281+00:00 | 2025-03-22T11:54:48.999281+00:00 | 10 | false |
# Complexity
- Time complexity: O(N)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(N)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int mostProfitablePath(vector<vector<int>>& edges, int bob, vector<int>& amount) {
int n = amount.size();
vector<vector<int>> adj(n);
vector<int> vis(n, 0);
for(auto& edge : edges) {
adj[edge[0]].push_back(edge[1]);
adj[edge[1]].push_back(edge[0]);
}
function<bool(int, int)> dfsBob = [&](int node, int time) {
vis[node] = time;
if(node == 0) return true;
for(auto& it : adj[node]) {
if(vis[it] == 0 && dfsBob(it, time + 1)) return true;
}
vis[node] = 0;
return false;
};
dfsBob(bob, 1);
int ans = INT_MIN;
function<void(int, int, int)> dfsAlice = [&](int node, int amt, int time) {
if(vis[node] == 0 || vis[node] > time) amt += amount[node];
else if(vis[node] == time) amt += amount[node] / 2;
if(adj[node].size() == 1 && node != 0) ans = max(ans, amt);
vis[node] = -1;
for(auto& it : adj[node]) {
if(vis[it] != -1) dfsAlice(it, amt, time + 1);
}
};
dfsAlice(0, 0, 1);
return ans;
}
};
``` | 1 | 0 | ['Array', 'Tree', 'Depth-First Search', 'Graph', 'C++'] | 0 |
most-profitable-path-in-a-tree | python easy solution💯💯💀💀 | python-easy-solution-by-rode_atharva-9a3y | Code | rode_atharva | NORMAL | 2025-03-12T10:04:21.608354+00:00 | 2025-03-12T10:04:21.608354+00:00 | 8 | false | # Code
```python3 []
class Solution:
def mostProfitablePath(self, edges: List[List[int]], bob: int, amount: List[int]) -> int:
nodes = len(amount)
adj = [ [] for _ in range(nodes)]
parent = [-1] * nodes
for u, v in edges:
adj[u].append(v)
adj[v].append(u)
queue = deque([(0, 0)])
distance = [float('inf')] * nodes
distance[0] = 0
while queue:
dist, node = queue.popleft()
for v in adj[node]:
if distance[v] == float('inf'):
distance[v] = dist+1
queue.append((dist+1, v))
parent[v] = node
dist = 0
bob_path = {}
while bob != -1:
bob_path[bob] = dist
bob = parent[bob]
dist += 1
queue = deque([(amount[0], 0, 0)]) #amt, node, time
max_collected = float('-inf')
while queue:
amt, node, time = queue.popleft()
if node in bob_path:
if bob_path[node] == time:
amt -= (amount[node] // 2)
elif bob_path[node] < time:
amt -= amount[node]
for v in adj[node]:
if v == parent[node]:
continue
queue.append((amt+amount[v], v, time+1))
if node != 0 and len(adj[node]) == 1:
max_collected = max(max_collected, amt)
return max_collected
``` | 1 | 0 | ['Python3'] | 0 |
most-profitable-path-in-a-tree | C# | c-by-adchoudhary-kge8 | Code | adchoudhary | NORMAL | 2025-02-26T04:46:13.717653+00:00 | 2025-02-26T04:46:13.717653+00:00 | 9 | false | # Code
```csharp []
public class Solution {
private class Node
{
public int distFromBob = int.MaxValue;
public int pathForBob = int.MaxValue;
public readonly List<int> neighbors = new();
}
public int MostProfitablePath(int[][] edges, int bob, int[] amount) {
// build initial array of nodes
Node[] nodes = new Node[amount.Length];
for (int ni = 0; ni < amount.Length; ni++)
{
nodes[ni] = new Node();
}
// connect the nodes
foreach (var e in edges)
{
int ni1 = e[0], ni2 = e[1];
nodes[ni1].neighbors.Add(ni2);
nodes[ni2].neighbors.Add(ni1);
}
// BFS from Bob outward to record distance
List<int> curr = new() { bob }, next = new();
int distFromBob = 0;
bool[] visited = new bool[nodes.Length];
bool found0 = false;
while (!found0 && curr.Count > 0)
{
foreach (var ci in curr)
{
visited[ci] = true;
Node node = nodes[ci];
node.distFromBob = distFromBob;
if (ci == 0)
{
found0 = true;
break;
}
foreach (var ni in node.neighbors)
{
if (!visited[ni])
{
next.Add(ni);
}
}
}
curr.Clear();
(curr, next) = (next, curr);
distFromBob++;
}
// Retrace Bob's path to 0
for (int ni = 0; ;)
{
Node node = nodes[ni];
node.pathForBob = node.distFromBob;
if (ni == bob)
{
break;
}
int nextDist = node.distFromBob - 1;
foreach (var nni in node.neighbors)
{
if (nodes[nni].distFromBob == nextDist)
{
ni = nni;
break;
}
}
}
Array.Clear(visited);
visited[0] = true;
// recursively find best path for Alice starting from node 0
return BestPath(0, 0);
int BestPath(int ni, int distFrom0)
{
Node node = nodes[ni];
int sum = amount[ni], pathForBob = node.pathForBob;
// adjust amount if Bob's been here already
if (distFrom0 == pathForBob) sum >>= 1;
if (distFrom0 > pathForBob) sum = 0;
distFrom0++;
// Recursively find the best path going down unvisited neighbors
int best = int.MinValue, bestNode = -1;
foreach (var nni in node.neighbors)
{
if (!visited[nni])
{
visited[nni] = true;
int s = BestPath(nni, distFrom0);
if (s > best)
{
best = s;
bestNode = nni;
}
visited[nni] = false;
}
}
// adjust sum if we found a unvisited neighbor
if (bestNode >= 0)
{
sum += best;
visited[bestNode] = true;
}
return sum;
}
}
}
``` | 1 | 0 | ['C#'] | 0 |
most-profitable-path-in-a-tree | DFS Approach | dfs-approach-by-tthieu0409-r043 | IntuitionApproachOne important note is no. edges = no. nodes - 1. This means there is no cycle!
There is only one path that Bob can go (to the node 0). Save his | tthieu0409 | NORMAL | 2025-02-25T17:43:44.760856+00:00 | 2025-02-25T17:43:44.760856+00:00 | 5 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
One important note is no. edges = no. nodes - 1. This means there is no cycle!
There is only one path that Bob can go (to the node 0). Save his path and then dfs for alice move which maximize the outcome.
# Complexity
- Time complexity:
O(n)
- Space complexity:
O(n)
# Code
```java []
class Solution {
boolean bobMove(List<List<Integer>> graph, int curIdx, int[] bobPath, int step) {
bobPath[curIdx] = step;
if (curIdx == 0) {
return true;
}
for (var node : graph.get(curIdx)) {
if (bobPath[node] != 0) continue;
if (bobMove(graph, node, bobPath, step + 1))
return true;
}
bobPath[curIdx] = 0;
return false;
}
int aliceMove(List<List<Integer>> graph, int curIdx, int[] amount, int parent, int[] bobPath, int step) {
var val = amount[curIdx];
if (bobPath[curIdx] != 0 && bobPath[curIdx] < step) {
val = 0;
} else if (bobPath[curIdx] == step) {
val /= 2;
}
var maxNet = Integer.MIN_VALUE;
boolean isLeaf = true;
for (var node : graph.get(curIdx)) {
if (parent == node)
continue;
isLeaf = false;
maxNet = Math.max(maxNet, aliceMove(graph, node, amount, curIdx, bobPath, step + 1));
}
return isLeaf ? val : val + maxNet;
}
List<List<Integer>> constructGraph(int[][] edges) {
List<List<Integer>> graph = new ArrayList<>();
for (int[] edge : edges) {
while (graph.size() <= Math.max(edge[0], edge[1])) {
graph.add(new ArrayList<>());
}
graph.get(edge[0]).add(edge[1]);
graph.get(edge[1]).add(edge[0]);
}
return graph;
}
public int mostProfitablePath(int[][] edges, int bob, int[] amount) {
List<List<Integer>> graph = constructGraph(edges);
int[] bobPath = new int[edges.length + 1];
bobMove(graph, bob, bobPath, 1);
return aliceMove(graph, 0, amount, -1, bobPath, 1);
}
}
``` | 1 | 0 | ['Java'] | 0 |
most-profitable-path-in-a-tree | Python | Beats 100% | 😊 | Explanation | python-beats-100-by-ashishgupta291-ym8r | Intuitionbob's path is fixed. if alice and bob travels on same path they can meet each other at the mid of root-bob path. bob can open first half of the gates i | ashishgupta291 | NORMAL | 2025-02-25T05:14:30.776425+00:00 | 2025-02-25T05:15:21.719423+00:00 | 8 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
bob's path is fixed. if alice and bob travels on same path they can meet each other at the mid of root-bob path. bob can open first half of the gates in his path.
if alice diverts from bob's path before meeting bob. in this case alice can never visit the node which bob has already visited, note that in any case bob will be able to open the first half of the gates in its path.
so we can directly make zero amount for first half of the nodes in bob's path and calculate maximum path sum from root-leaf for alice with simple dfs.
# Approach
<!-- Describe your approach to solving the problem. -->
using backtracking to get bob's path otherwise gets MLE. making amount zero for the first half of the nodes of bob's path. then do dfs for alice.
# Complexity
- Time complexity: O(n)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(n)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
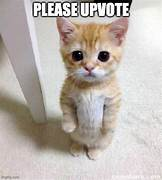
# Code
```python []
class Solution(object):
def mostProfitablePath(self, edges, bob, amount):
adja = {}
for e in edges:
if e[0] in adja:
adja[e[0]].append(e[1])
else:
adja[e[0]]=[e[1]]
if e[1] in adja:
adja[e[1]].append(e[0])
else:
adja[e[1]]=[e[0]]
def bob_p(p, root):
path.append(root)
if root == bob:
return True
for c in adja[root]:
if c!=p:
if bob_p(root, c):
return True
path.pop()
return False
path=[]
bob_p(None, 0)
l = len(path)
for i in range(l//2):
amount[path.pop()] =0
if l%2:
t = path.pop()
amount[t] = amount[t]//2
self.out = float('-inf')
def alice(p, root, tot):
tot+=amount[root]
flag = True
for c in adja[root]:
if c!=p:
flag = False
alice(root, c, tot)
if flag:
self.out = max(self.out, tot)
alice(None, 0, 0)
return self.out
``` | 1 | 0 | ['Python'] | 0 |
most-profitable-path-in-a-tree | ✅ 🎯 📌 Simple Solution || DFS+BFS || Fastest Solution ✅ 🎯 📌 | simple-solution-dfsbfs-fastest-solution-vlam1 | Code | vvnpais | NORMAL | 2025-02-24T21:17:46.478678+00:00 | 2025-02-24T21:17:46.478678+00:00 | 19 | false | # Code
```python3 []
class Solution:
def mostProfitablePath(self, edges: List[List[int]], bob: int, amount: List[int]) -> int:
d=defaultdict(list)
for u,v in edges:
d[u].append(v)
d[v].append(u)
bobs={i:float('inf') for i in range(len(edges)+1)}
def dfs(node,par,time):
if node==0:
bobs[node]=time
return True
for neighbour in d[node]:
if neighbour==par: continue
if dfs(neighbour,node,time+1):
bobs[node]=time
return True
return False
dfs(bob,-1,0)
dq=deque([(0,0,-1,amount[0])]) #node, time, parent, totalprof
ans=float('-inf')
while dq:
node,time,par,profit=dq.popleft()
for neighbour in d[node]:
if neighbour==par: continue
neighbour_time=time+1
neighbour_profit=amount[neighbour]
if neighbour_time>bobs[neighbour]:
neighbour_profit=0
if neighbour_time==bobs[neighbour]:
neighbour_profit>>=1
dq.append((neighbour,neighbour_time,node,profit+neighbour_profit))
if len(d[neighbour])==1 and profit+neighbour_profit>ans:
ans=profit+neighbour_profit
return ans
``` | 1 | 0 | ['Array', 'Tree', 'Depth-First Search', 'Breadth-First Search', 'Graph', 'Python3'] | 0 |
most-profitable-path-in-a-tree | java and erlang solution | java-and-erlang-solution-by-sidraq-amrb | the erlang one needs some tweakingCode | sidraq | NORMAL | 2025-02-24T20:00:16.726793+00:00 | 2025-02-24T20:00:16.726793+00:00 | 15 | false | # the erlang one needs some tweaking
# Code
```erlang
-export([most_profitable_path/3]).
most_profitable_path(Edges, Bob, Amount) ->
Graph = build_graph(Edges, maps:new()),
BobPath = find_bob_path(Bob, 0, Graph, []),
BobPathMap = lists:foldl(fun({Node, Index}, Acc) -> maps:put(Node, Index, Acc) end, maps:new(), lists:zip(BobPath, lists:seq(0, length(BobPath) - 1))),
get_alice_max_score(0, -1, 0, BobPathMap, Graph, 0, Amount).
build_graph([], Graph) -> Graph;
build_graph([[A, B] | Rest], Graph) ->
Graph1 = maps:update_with(A, fun(V) -> [B | V] end, [B], Graph),
Graph2 = maps:update_with(B, fun(V) -> [A | V] end, [A], Graph1),
build_graph(Rest, Graph2).
find_bob_path(0, _, _, Path) -> lists:reverse([0 | Path]);
find_bob_path(Node, Parent, Graph, Path) ->
case maps:get(Node, Graph, []) of
[] -> [];
Neighbors ->
lists:foldl(
fun(Neighbor, Acc) when Neighbor /= Parent ->
NewPath = [Node | Path],
Result = find_bob_path(Neighbor, Node, Graph, NewPath),
if Result /= [] -> Result; true -> Acc end;
(_, Acc) -> Acc
end,
[],
Neighbors
)
end.
get_alice_max_score(Node, Parent, CurrScore, BobPath, Graph, Timestamp, Amount) ->
BobTime = maps:get(Node, BobPath, -1),
NodeAmount = lists:nth(Node + 1, Amount),
NewScore = case BobTime of
-1 -> CurrScore + NodeAmount;
T when T > Timestamp -> CurrScore + NodeAmount;
T when T =:= Timestamp -> CurrScore + (NodeAmount div 2);
_ -> CurrScore
end,
case lists:filter(fun(N) -> N /= Parent end, maps:get(Node, Graph, [])) of
[] -> NewScore;
Neighbors -> lists:max([get_alice_max_score(Neighbor, Node, NewScore, BobPath, Graph, Timestamp + 1, Amount) || Neighbor <- Neighbors])
end.
```
```java []
class Solution {
public int mostProfitablePath(int[][] edges, int bob, int[] amount) {
ArrayList<Integer>[] graph = new ArrayList[amount.length];
for (int i = 0; i < graph.length; i++) graph[i] = new ArrayList<>();
for (int[] edge : edges) {
graph[edge[0]].add(edge[1]);
graph[edge[1]].add(edge[0]);
}
int[] bobPath = new int[amount.length];
Arrays.fill(bobPath, -1);
ArrayList<Integer> path = new ArrayList<>();
fillBobPath(bob, -1, path, graph);
for (int i = 0; i < path.size(); i++) {
bobPath[path.get(i)] = i;
}
return getAliceMaxScore(0, -1, 0, bobPath, graph, 0, amount);
}
private boolean fillBobPath(int node, int parentNode, ArrayList<Integer> path, ArrayList<Integer>[] graph) {
if (node == 0) return true;
for (int neighborNode : graph[node]) {
if (neighborNode != parentNode) {
path.add(node);
if (fillBobPath(neighborNode, node, path, graph)) return true;
path.remove(path.size() - 1);
}
}
return false;
}
private int getAliceMaxScore(int node, int parentNode, int currScore, int[] bobPath, ArrayList<Integer>[] graph, int timestamp, int[] amount) {
if (bobPath[node] == -1 || bobPath[node] > timestamp) {
currScore += amount[node];
} else if (bobPath[node] == timestamp) {
currScore += amount[node] / 2;
}
if (graph[node].size() == 1 && node != 0) return currScore;
int maxScore = Integer.MIN_VALUE;
for (int neighborNode : graph[node]) {
if (neighborNode != parentNode) {
maxScore = Math.max(maxScore, getAliceMaxScore(neighborNode, node, currScore, bobPath, graph, timestamp + 1, amount));
}
}
return maxScore;
}
}
``` | 1 | 0 | ['Java'] | 0 |
most-profitable-path-in-a-tree | Best easy and simple explanation in python 🚀 | best-easy-and-simple-explanation-in-pyth-s77d | IntuitionThe problem involves two players, Alice and Bob, traversing a tree represented as an undirected graph. Alice aims to maximize her profit, while Bob fol | harshit197 | NORMAL | 2025-02-24T19:38:25.211258+00:00 | 2025-02-24T19:38:25.211258+00:00 | 14 | false | # Intuition
The problem involves two players, Alice and Bob, traversing a tree represented as an undirected graph. Alice aims to maximize her profit, while Bob follows a specific path to the root. The key observation is that:
- Bob's movement can be simulated using **DFS**, where we track the time he reaches each node in path to root. BOB doesn't have any choice to make. So we need to check only nodes in path to root.
- Alice's movement can be simulated using **BFS**, while adjusting the profit at each node based on Bob's presence. Alice have choices to make to reach any leaf note. So we need to check the profit at each leaf node and return whichever is maximum.
# Approach
## 1. Graph Construction
- Use an **adjacency list** (dictionary of lists) to store edges because it is easier that way.
## 2. Simulate Bob's Path (DFS)
- Perform a **DFS** from Bob's starting node towards the root (node `0`).
- Track the **visit time** for each node in a dictionary `bob_visit`.
## 3. Simulate Alice's Path (BFS)
- Perform **BFS** from the root (`0`).
- Maintain a queue storing tuples `(node, parent, time, profit)`.
- Adjust the profit at each node:
- If Bob reaches the node **before Alice**, profit is `0`.
- If Bob and Alice reach at the **same time**, profit is **halved**.
## 4. Track Maximum Profit
- Update the result when Alice reaches a **leaf node**.
# Complexity
| Complexity | Analysis |
|------------|------------|
| **Time Complexity** | $$O(n)$$ - DFS + BFS traverse each node once |
| **Space Complexity** | $$O(n)$$ - Adjacency list, queue, and hash table |
# Code
```python
class Solution:
def mostProfitablePath(
self, edges: List[List[int]], bob: int, amount: List[int]
) -> int:
# Construct adjacency list
adj = defaultdict(list)
for v1, v2 in edges:
adj[v1].append(v2)
adj[v2].append(v1)
# Bob's Path Simulation (DFS)
bob_visit = {} # Stores nodes Bob visits with their timestamps
def dfs(src, parent, time):
if src == 0:
bob_visit[src] = time
return True
for nei in adj[src]:
if nei == parent:
continue
if dfs(nei, src, time + 1):
bob_visit[src] = time
return True
return False
dfs(bob, -1, 0)
# Alice's Path Simulation (BFS)
q = deque([(0, -1, 0, amount[0])]) # (node, parent, time, profit)
res = float("-inf")
while q:
node, parent, time, profit = q.popleft()
for nei in adj[node]:
if nei == parent:
continue
nei_time = time + 1
nei_profit = amount[nei]
# Adjust profit based on Bob's visit
if nei in bob_visit:
if nei_time > bob_visit[nei]:
nei_profit = 0 # Bob already took it
elif nei_time == bob_visit[nei]:
nei_profit //= 2 # Split profit
q.append((nei, node, nei_time, profit + nei_profit))
# Update result if Alice reaches a leaf
if len(adj[nei]) == 1:
res = max(res, profit + nei_profit)
return res | 1 | 0 | ['Array', 'Tree', 'Depth-First Search', 'Breadth-First Search', 'Graph', 'Simulation', 'Python3'] | 0 |
most-profitable-path-in-a-tree | Java Solution for Most Profitable Path In a Tree Problem | java-solution-for-most-profitable-path-i-emw9 | IntuitionThe problem involves a tree structure where Alice and Bob move simultaneously in opposite directions. Alice tries to maximize her profit by moving towa | Aman_Raj_Sinha | NORMAL | 2025-02-24T18:52:10.458298+00:00 | 2025-02-24T18:52:10.458298+00:00 | 36 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
The problem involves a tree structure where Alice and Bob move simultaneously in opposite directions. Alice tries to maximize her profit by moving towards a leaf, while Bob moves towards the root, potentially blocking Alice’s access to some rewards. The key observations are:
• If Alice reaches a node first, she gets the full amount.
• If Bob reaches a node first, Alice gets nothing.
• If they reach simultaneously, they split the amount.
• Alice should choose the most profitable leaf path.
# Approach
<!-- Describe your approach to solving the problem. -->
1. Graph Representation (Adjacency List)
• Convert the edge list into an adjacency list for easier tree traversal.
2. Find Bob’s Path to Root
• Use DFS from Bob’s starting position to record the depth of each node on his path.
• This helps determine whether Bob reaches a node before, after, or at the same time as Alice.
3. Depth-First Search (DFS) for Alice
• Start DFS from the root (Alice’s initial position).
• At each node:
• Determine Alice’s profit based on Bob’s arrival.
• If the node is a leaf, return the profit.
• Recursively explore all child nodes and maximize the profit.
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
• Building the adjacency list takes O(n).
• Finding Bob’s path requires a single DFS traversal, taking O(n).
• Alice’s DFS traversal also takes O(n) in the worst case.
• Overall complexity: O(n) + O(n) = O(n).
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
• Adjacency list: O(n) (for storing edges).
• Bob’s distance array: O(n).
• Recursive stack depth (for DFS calls): O(n) in the worst case (if the tree is skewed).
• Total space: O(n) + O(n) + O(n) = O(n).
# Code
```java []
class Solution {
public int mostProfitablePath(int[][] edges, int bob, int[] amount) {
int n = amount.length;
List<Integer>[] tree = new ArrayList[n];
for (int i = 0; i < n; i++) {
tree[i] = new ArrayList<>();
}
for (int[] edge : edges) {
tree[edge[0]].add(edge[1]);
tree[edge[1]].add(edge[0]);
}
int[] bobDist = new int[n];
Arrays.fill(bobDist, -1);
findBobPath(bob, -1, 0, tree, bobDist);
return dfsAlice(0, -1, 0, 0, tree, bobDist, amount);
}
private boolean findBobPath(int node, int parent, int depth, List<Integer>[] tree, int[] bobDist) {
bobDist[node] = depth;
if (node == 0) return true;
for (int neighbor : tree[node]) {
if (neighbor == parent) continue;
if (findBobPath(neighbor, node, depth + 1, tree, bobDist)) {
return true;
}
}
bobDist[node] = -1;
return false;
}
private int dfsAlice(int node, int parent, int depth, int currentProfit, List<Integer>[] tree, int[] bobDist, int[] amount) {
if (bobDist[node] == -1 || depth < bobDist[node]) {
currentProfit += amount[node];
} else if (depth == bobDist[node]) {
currentProfit += amount[node] / 2;
}
if (tree[node].size() == 1 && node != 0) {
return currentProfit;
}
int maxProfit = Integer.MIN_VALUE;
for (int neighbor : tree[node]) {
if (neighbor == parent) continue;
maxProfit = Math.max(maxProfit, dfsAlice(neighbor, node, depth + 1, currentProfit, tree, bobDist, amount));
}
return maxProfit;
}
}
``` | 1 | 0 | ['Java'] | 0 |
most-profitable-path-in-a-tree | Best Easy Solution - Most Profitable Path in a Tree | best-easy-solution-most-profitable-path-jbir9 | Code | deepakkoshta | NORMAL | 2025-02-24T18:39:07.889547+00:00 | 2025-02-24T18:39:07.889547+00:00 | 11 | false | # Code
```cpp []
class Solution {
public:
unordered_map<int, vector<int>> umpv;
unordered_map<int, int> bobtime;
int aliceprofit = INT_MIN;
bool DFSBob(int currnode, int time, vector<bool>& visited) {
visited[currnode] = true;
bobtime[currnode] = time;
if (currnode == 0)
return true;
for (auto& ngbr : umpv[currnode]) {
if (!visited[ngbr])
if (DFSBob(ngbr, time + 1, visited) == true)
return true;
}
bobtime.erase(currnode);
return false;
}
void DFSAlice(int currnode, int time, int income, vector<bool>& visited, vector<int>& amount) {
visited[currnode] = true;
if (bobtime.find(currnode) == bobtime.end() || time < bobtime[currnode])
income += amount[currnode];
else if (time == bobtime[currnode])
income += amount[currnode] / 2;
if (umpv[currnode].size() == 1 && currnode != 0)
aliceprofit = max(aliceprofit, income);
for (auto& ngbr : umpv[currnode]) {
if (!visited[ngbr])
DFSAlice(ngbr, time + 1, income, visited, amount);
}
}
int mostProfitablePath(vector<vector<int>>& edges, int bob, vector<int>& amount) {
int n = amount.size();
for (auto edge : edges) {
umpv[edge[0]].push_back(edge[1]);
umpv[edge[1]].push_back(edge[0]);
}
vector<bool> visited(n, false);
DFSBob(bob, 0, visited);
int income = 0;
visited.assign(n, false);
DFSAlice(0, 0, income, visited, amount);
return aliceprofit;
}
};
``` | 1 | 0 | ['C++'] | 0 |
most-profitable-path-in-a-tree | 2467 LeetCode - Most Profitable Path in a Tree | 2467-leetcode-most-profitable-path-in-a-4gplg | 2467 LeetCode - Most Profitable Path in a TreeProblem StatementYou are given an undirected tree with n nodes labeled from 0 to n-1, where the root node is 0. Th | ayushrawat220804 | NORMAL | 2025-02-24T18:07:11.984953+00:00 | 2025-02-24T18:07:11.984953+00:00 | 35 | false | ## **2467 LeetCode - Most Profitable Path in a Tree**
### Problem Statement
You are given an undirected tree with `n` nodes labeled from `0` to `n-1`, where the root node is `0`. The tree is described by an array `edges`, where `edges[i] = [ui, vi]` means that there is an undirected edge between the nodes `ui` and `vi`.
Each node has a value stored in an array `amount`, where `amount[i]` represents the profit at node `i`.
A game is played between Alice and Bob:
- Alice starts at node `0` and can move to any connected node.
- Bob starts at a given node `bob` and moves towards node `0` using the shortest path.
- If both Alice and Bob reach the same node at the same time, Alice only earns half the profit from that node.
- Alice’s goal is to maximize her total profit.
Return the **maximum profit Alice can earn** by choosing an optimal path.
---
## **Intuition**
1. **Bob's Shortest Path Calculation**:
- Bob moves towards node `0`, so we must determine the time he takes to reach each node.
- We use **DFS** to record the exact time when Bob reaches a node.
2. **Alice's DFS for Maximum Profit**:
- Alice starts from node `0` and explores all possible paths.
- If Bob has not visited a node, Alice gets the full amount.
- If Bob and Alice arrive at the same time, Alice gets **half** the amount.
- If Bob has already passed through a node, Alice gets **nothing**.
3. **Finding Maximum Profit**:
- Alice explores all paths using **DFS**, keeping track of profit.
- At leaf nodes (nodes with no children except the parent), we update the maximum profit.
---
## **Approach - Using DFS for Bob and Alice**
### **Algorithm**
1. **Build the adjacency list** from the given edges.
2. **Find Bob's path** to `0`:
- Use a DFS (`DFSBob`) to record the time Bob takes to reach each node.
- Store Bob's arrival times in `bobMap`.
3. **Simulate Alice's movement**:
- Use another DFS (`DFSAlice`) to explore all paths.
- Track Alice’s profit based on Bob’s recorded arrival times.
- Update `aliceIncome` whenever a leaf node is reached.
### **Complexity Analysis**
- **Time Complexity**: `O(n)` since each node is visited at most twice (once for Bob, once for Alice).
- **Space Complexity**: `O(n)` for recursion stack and adjacency list storage.
---
## **Code Implementation**
```cpp
/**
* Approach - Using DFS for Bob and Alice
* Time Complexity : O(n)
* Space Complexity : O(n) (for recursion stack and adjacency list)
*/
class Solution {
public:
unordered_map<int, vector<int>> adj;
unordered_map<int, int> bobMap;
int aliceIncome;
// DFS for Bob to record the time taken to reach each node
bool DFSBob(int curr, int t, vector<bool>& visited) {
visited[curr] = true;
bobMap[curr] = t; // Store time taken to reach this node
if (curr == 0) // Reached root
return true;
for (auto &ngbr : adj[curr]) {
if (!visited[ngbr]) {
if (DFSBob(ngbr, t + 1, visited))
return true;
}
}
bobMap.erase(curr); // Remove node if it's not in the shortest path
return false;
}
// DFS for Alice to maximize profit
void DFSAlice(int curr, int t, int income, vector<bool>& visited, vector<int>& amount) {
visited[curr] = true;
if (bobMap.find(curr) == bobMap.end() || t < bobMap[curr]) {
income += amount[curr]; // Alice gets full profit if Bob is not there yet
} else if (t == bobMap[curr]) {
income += (amount[curr] / 2); // Split profit if they arrive at the same time
}
// If current node is a leaf node, update maximum profit
if (adj[curr].size() == 1 && curr != 0) {
aliceIncome = max(aliceIncome, income);
}
// Explore neighbors
for (int &ngbr : adj[curr]) {
if (!visited[ngbr]) {
DFSAlice(ngbr, t + 1, income, visited, amount);
}
}
}
int mostProfitablePath(vector<vector<int>>& edges, int bob, vector<int>& amount) {
int n = amount.size(); // Number of nodes
aliceIncome = INT_MIN;
for (vector<int>& edge : edges) {
int u = edge[0];
int v = edge[1];
adj[u].push_back(v);
adj[v].push_back(u);
}
// DFS on Bob to determine his path to node 0
int time = 0;
vector<bool> visited(n, false);
DFSBob(bob, time, visited);
// DFS for Alice to maximize profit
int income = 0;
visited.assign(n, false);
DFSAlice(0, 0, income, visited, amount);
return aliceIncome;
}
};
```
---
## **Explanation with Example**
### **Example 1**
#### **Input:**
```cpp
edges = [[0,1],[1,2],[1,3],[3,4]]
bob = 3
amount = [-2,4,2,-4,6]
```
#### **Output:**
`6`
#### **Explanation:**
```
0
|
1
/ \
2 3
|
4
```
- Bob moves from `3 → 1 → 0`.
- Alice moves from `0 → 1 → 3 → 4`.
- Alice collects: `amount[0] + amount[1] + amount[3]/2 + amount[4] = -2 + 4 + (-4)/2 + 6 = 6`.
---
### **Example 2**
#### **Input:**
```cpp
edges = [[0,1],[1,2],[1,3],[3,4],[3,5]]
bob = 4
amount = [5,3,2,1,4,-1]
```
#### **Output:**
`7`
#### **Explanation:**
```
0
|
1
/ \
2 3
/ \
4 5
```
- Bob moves from `4 → 3 → 1 → 0`.
- Alice moves from `0 → 1 → 3 → 4`.
- Alice collects: `amount[0] + amount[1] + amount[3]/2 + amount[4] = 5 + 3 + (1)/2 + 4 = 7`.
---
## **Conclusion**
- We efficiently calculate **Bob's shortest path** to the root using DFS.
- We simulate **Alice's movement** using DFS to find the optimal path for maximum profit.
- The algorithm runs in **O(n) time**, making it efficient for large trees.
**Hope this helps! 🚀** | 1 | 0 | ['C++'] | 0 |
most-profitable-path-in-a-tree | Most Profitable Path in a Tree | most-profitable-path-in-a-tree-by-dinesh-4qra | IntuitionApproachComplexity
Time complexity:O(V+E)
Space complexity:O(V)
Code | Dinesh-Saladi | NORMAL | 2025-02-24T17:56:25.372013+00:00 | 2025-02-24T17:56:25.372013+00:00 | 25 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity: $$O(V+E)$$
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: $$O(V)$$
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int bob0(vector<vector<int>>& adj, int bob, int visited,
vector<int>& obob) {
if (bob == 0) {
obob.push_back(bob);
return 0;
}
for (int i = 0; i < adj[bob].size(); i++) {
if (adj[bob][i] != visited) {
int s = bob0(adj, adj[bob][i], bob, obob);
if (s == 0) {
obob.push_back(bob);
return 0;
}
}
}
return 1;
}
void alice(int i, vector<vector<int>>& adj, int cur, int visited,
vector<int>& bobvis, int sum, int& opt, vector<int>& amount) {
if (i < bobvis[cur]) {
sum += amount[cur];
} else if (i == bobvis[cur]) {
sum += amount[cur] / 2;
}
bool isLeaf = true;
for (int j = 0; j < adj[cur].size(); j++) {
if (adj[cur][j] != visited) {
isLeaf = false;
alice(i + 1, adj, adj[cur][j], cur, bobvis, sum, opt, amount);
}
}
if (isLeaf) {
opt = max(opt, sum);
}
}
int mostProfitablePath(vector<vector<int>>& edges, int bob,
vector<int>& amount) {
vector<vector<int>> adj(amount.size());
for (auto v : edges) {
adj[v[0]].push_back(v[1]);
adj[v[1]].push_back(v[0]);
}
vector<int> obob;
bob0(adj, bob, -1, obob);
reverse(obob.begin(), obob.end());
vector<int> bobvis(amount.size(), 1e7);
for (int i = 0; i < obob.size(); i++) {
bobvis[obob[i]] = i;
}
int opt = INT_MIN;
alice(0, adj, 0, -1, bobvis, 0, opt, amount);
return opt;
}
};
``` | 1 | 0 | ['Array', 'Depth-First Search', 'Breadth-First Search', 'Graph', 'C++'] | 0 |
most-profitable-path-in-a-tree | ✅ 🌟 JAVA SOLUTION ||🔥 BEATS 100% PROOF🔥|| 💡 CONCISE CODE ✅ || 🧑💻 BEGINNER FRIENDLY | java-solution-beats-100-proof-concise-co-gple | Complexity
Time complexity:O(n)
Space complexity:O(n)
Code | Shyam_jee_ | NORMAL | 2025-02-24T17:40:36.055369+00:00 | 2025-02-24T17:40:36.055369+00:00 | 35 | false | # Complexity
- Time complexity:$$O(n)$$
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:$$O(n)$$
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
HashMap<Integer, List<Integer>> adj=new HashMap<>();
HashMap<Integer, Integer> bobMap=new HashMap<>();
int aliceIncome;
public int mostProfitablePath(int[][] edges, int bob, int[] amount) {
int n=amount.length;
aliceIncome=Integer.MIN_VALUE;
for(int[] edge:edges)
{
int u=edge[0];
int v=edge[1];
adj.computeIfAbsent(u, k->new ArrayList<>()).add(v);
adj.computeIfAbsent(v, k->new ArrayList<>()).add(u);
}
boolean[] visited=new boolean[n];
dfsBob(bob, 0, visited);
int income=0;
Arrays.fill(visited, false);
dfsAlice(0, 0, income, visited, amount);
return aliceIncome;
}
private boolean dfsBob(int curr, int t, boolean[] visited)
{
visited[curr]=true;
bobMap.put(curr, t);
if(curr==0) return true;
for(int ngbr:adj.getOrDefault(curr, new ArrayList<>()))
{
if(!visited[ngbr])
{
if(dfsBob(ngbr, t+1, visited)) return true;
}
}
bobMap.remove(curr);
visited[curr]=false;
return false;
}
private void dfsAlice(int curr, int t, int income, boolean[] visited, int[] amount)
{
visited[curr]=true;
if(!bobMap.containsKey(curr) || t<bobMap.get(curr)) income+=amount[curr];
else if(t==bobMap.get(curr)) income+=amount[curr]/2;
if(adj.getOrDefault(curr, new ArrayList<>()).size()==1 && curr!=0)
aliceIncome=Math.max(income, aliceIncome);
for(int ngbr : adj.getOrDefault(curr, new ArrayList<>()))
{
if(!visited[ngbr])
{
dfsAlice(ngbr, t+1, income, visited, amount);
}
}
}
}
``` | 1 | 0 | ['Array', 'Tree', 'Depth-First Search', 'Graph', 'Java'] | 0 |
most-profitable-path-in-a-tree | Most Profitable path in the Tree easy solution in c++ | most-profitable-path-in-the-tree-easy-so-zb5h | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Tushar_1920 | NORMAL | 2025-02-24T16:55:28.752568+00:00 | 2025-02-24T16:55:28.752568+00:00 | 108 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int mostProfitablePath(vector<vector<int>>& edges, int bob,
vector<int>& amount) {
int n = amount.size(), maxIncome = INT_MIN;
tree.resize(n);
visited.assign(n, false);
queue<vector<int>> nodeQueue;
nodeQueue.push({0, 0, 0});
for (vector<int> edge : edges) {
tree[edge[0]].push_back(edge[1]);
tree[edge[1]].push_back(edge[0]);
}
findBobPath(bob, 0);
visited.assign(n, false);
while (!nodeQueue.empty()) {
int sourceNode = nodeQueue.front()[0], time = nodeQueue.front()[1],
income = nodeQueue.front()[2];
if (bobPath.find(sourceNode) == bobPath.end() ||
time < bobPath[sourceNode]) {
income += amount[sourceNode];
}
else if (time == bobPath[sourceNode]) {
income += (amount[sourceNode] / 2);
}
if (tree[sourceNode].size() == 1 && sourceNode != 0) {
maxIncome = max(maxIncome, income);
}
for (int adjacentNode : tree[sourceNode]) {
if (!visited[adjacentNode]) {
nodeQueue.push({adjacentNode, time + 1, income});
}
}
visited[sourceNode] = true;
nodeQueue.pop();
}
return maxIncome;
}
private:
unordered_map<int, int> bobPath;
vector<bool> visited;
vector<vector<int>> tree;
bool findBobPath(int sourceNode, int time) {
bobPath[sourceNode] = time;
visited[sourceNode] = true;
if (sourceNode == 0) {
return true;
}
for (auto adjacentNode : tree[sourceNode]) {
if (!visited[adjacentNode]) {
if (findBobPath(adjacentNode, time + 1)) {
return true;
}
}
}
bobPath.erase(sourceNode);
return false;
}
};
``` | 1 | 0 | ['C++'] | 1 |
most-profitable-path-in-a-tree | hehheh | hehheh-by-abhay0_20-gphc | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | abhay0_20 | NORMAL | 2025-02-24T16:51:31.753043+00:00 | 2025-02-24T16:51:31.753043+00:00 | 22 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def mostProfitablePath(self, edges: List[List[int]], bob: int, amount: List[int]) -> int:
n=len(edges)+1
adj=[[] for _ in range(n)]
parent=[-1]*n
Bob=[float('inf')]*n
for u, v in edges:
adj[u].append(v)
adj[v].append(u)
def dfs(i, p):
parent[i] = p
for j in adj[i]:
if j == p: continue
dfs(j, i)
dfs(0, -1)
x=bob
move=0
while x!=-1:
Bob[x]=move
move+=1
x=parent[x]
def dfs_sum(i, dist, prev):
alice=0
if dist < Bob[i]:
alice=amount[i] # Alice takes full amount
elif dist==Bob[i]:
alice=amount[i]//2 # Both reach at the same time
isLeaf=True
maxLeafSum=-float('inf')
for j in adj[i]:
if j == prev: continue
isLeaf=False
maxLeafSum = max(maxLeafSum, dfs_sum(j, dist+1, i))
return alice if isLeaf else alice + maxLeafSum
return dfs_sum(0, 0, -1)
``` | 1 | 0 | ['Python3'] | 0 |
most-profitable-path-in-a-tree | Python Solution: | python-solution-by-a_nishkumar-t92i | Code | A_nishkumar | NORMAL | 2025-02-24T16:31:20.971057+00:00 | 2025-02-24T16:31:20.971057+00:00 | 27 | false | # Code
```python3 []
class Solution:
def mostProfitablePath(self, edges: List[List[int]], bob: int, amount: List[int]) -> int:
n=len(edges)+1
adj=[[] for _ in range(n)]
parent=[-1]*n
Bob=[float('inf')]*n
for u, v in edges:
adj[u].append(v)
adj[v].append(u)
# Step 1: Build parent information using DFS
def dfs(i, p):
parent[i] = p
for j in adj[i]:
if j == p: continue
dfs(j, i)
dfs(0, -1) # Start with -1 as the parent of root
# Step 2: Compute Bob's arrival times
x=bob
move=0
while x!=-1:
Bob[x]=move
move+=1
x=parent[x]
# Step 3: DFS to compute Alice's best profit path
def dfs_sum(i, dist, prev):
alice=0
if dist < Bob[i]:
alice=amount[i] # Alice takes full amount
elif dist==Bob[i]:
alice=amount[i]//2 # Both reach at the same time
isLeaf=True
maxLeafSum=-float('inf')
for j in adj[i]:
if j == prev: continue
isLeaf=False
maxLeafSum = max(maxLeafSum, dfs_sum(j, dist+1, i))
return alice if isLeaf else alice + maxLeafSum
return dfs_sum(0, 0, -1)
``` | 1 | 0 | ['Python3'] | 0 |
most-profitable-path-in-a-tree | Very Easy Intuitive Simple BFS Solution, No DFS Used | very-easy-intuitive-simple-bfs-solution-e8evu | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | utk50090 | NORMAL | 2025-02-24T16:20:50.930969+00:00 | 2025-02-24T16:20:50.930969+00:00 | 44 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int mostProfitablePath(vector<vector<int>>& edges, int bob, vector<int>& amount) {
int n = amount.size();
vector<int> adj[n];
for(auto it : edges){
int u = it[0];
int v = it[1];
adj[u].push_back(v);
adj[v].push_back(u);
}
vector<int> distAlice(n,-1);
vector<int> distBob(n,-1);
distAlice[0] = 0;
queue<pair<int,int>> q;
q.push({0,0});
while(!q.empty()){
auto it = q.front();
q.pop();
int node = it.first;
int dist = it.second;
for(auto adjNode : adj[node]){
if(distAlice[adjNode] == -1){
distAlice[adjNode] = dist+1;
q.push({adjNode,dist+1});
}
}
}
vector<int> parent(n,-1);
queue<int> q2;
q2.push(0);
parent[0] = -1;
while(!q2.empty()){
int node = q2.front();
q2.pop();
for(auto adjNode : adj[node]){
if(parent[adjNode] == -1 && adjNode != 0){
parent[adjNode] = node;
q2.push(adjNode);
}
}
}
vector<int> bobPath;
int currNode = bob;
while(currNode != -1){
bobPath.push_back(currNode);
currNode = parent[currNode];
}
for(int i=0; i<bobPath.size(); i++){
distBob[bobPath[i]] = i;
}
queue<pair<int,int>> q3;
q3.push({0,amount[0]});
int ans = INT_MIN;
vector<int> vis(n,0);
vis[0] = 1;
while(!q3.empty()){
auto it = q3.front();
q3.pop();
int node = it.first;
int profit = it.second;
bool flag = 1;
//ans = max(ans,profit);
//cout<<profit<<" ";
for(auto adjNode : adj[node]){
if(!vis[adjNode]){
flag = 0;
if(distBob[adjNode] != -1 && distBob[adjNode] == distAlice[adjNode]){
q3.push({adjNode,profit+(amount[adjNode]/2)});
}else if(distBob[adjNode] != -1
&& distBob[adjNode] < distAlice[adjNode]){
q3.push({adjNode,profit});
}
else{
q3.push({adjNode,profit + amount[adjNode]});
}
vis[adjNode] = 1;
}
}
if(flag){
ans = max(ans,profit);
}
}
return ans;
}
};
``` | 1 | 0 | ['C++'] | 0 |
most-profitable-path-in-a-tree | Two-Pass O(n) Approach: Most Profitable Path | two-pass-on-approach-most-profitable-pat-g45b | IntuitionImagine the problem as a map of connected locations (nodes) with money amounts at each location. We have Alice starting at location 0 and Bob starting | rashid_sid | NORMAL | 2025-02-24T15:20:14.581443+00:00 | 2025-02-24T15:57:35.803548+00:00 | 53 | false | # Intuition
Imagine the problem as a map of connected locations (nodes) with money amounts at each location. We have Alice starting at location 0 and Bob starting at another location. Bob moves towards location 0, and Alice also moves towards one of the leaf nodes.
We want to find the path Alice can take that gives her the maximum income, considering Bob's movements and how they affect the amounts at each location.
# Approach
1. Build the Map:
We first create a map (adjacency list) representing the connections between the locations.
2. Find Bob's Path and Timestamps:
We then find the path Bob takes from his starting location to location 0.
As Bob moves, we record the time he reaches each location along his path. This "timestamp" tells us when Bob was at each location.
3. Calculate Alice's Maximum Profit:
We use a depth-first search (DFS) to explore all possible paths Alice can take from location 0.
As Alice moves, we check:
If Alice and Bob reach the same location at the same time, Alice gets half the money.
If Bob reached the location before Alice, Alice gets no money.
Otherwise, Alice gets the full money amount.
We keep track of the maximum profit Alice can get by exploring all paths.
4. Return the Result:
We return the maximum profit.
# Complexity
- Time complexity: $$O(n)$$
This is because we visit each node at most once during the Bob's path finding and the DFS.
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: $$O(n)$$
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int mostProfitablePath(vector<vector<int>>& edges, int bob, vector<int>& amount) {
int n = amount.size();
vector<vector<int>> adj(n);
for(auto i: edges){
adj[i[0]].push_back(i[1]);
adj[i[1]].push_back(i[0]);
}
vector<int> timeStamp(n, 1e6);
vector<int> parent(n, -1);
function<bool(int, int, int)> findBobPath = [&](int node, int par, int time) {
if (node == 0) {
timeStamp[node] = time;
return true;
}
for (int neighbor : adj[node]) {
if (neighbor != par) {
if (findBobPath(neighbor, node, time + 1)) {
timeStamp[node] = time;
return true;
}
}
}
return false;
};
findBobPath(bob, -1, 0);
function<int(int, int, int)> dfs = [&](int node, int par, int time) {
int profit = amount[node];
if (timeStamp[node] == time) {
profit /= 2;
} else if (timeStamp[node] < time) {
profit = 0;
}
int maxChildProfit = INT_MIN;
bool isLeaf = true;
for (int neighbor : adj[node]) {
if (neighbor != par) {
isLeaf = false;
maxChildProfit = max(maxChildProfit, dfs(neighbor, node, time + 1));
}
}
if (isLeaf) {
return profit;
} else {
return profit + maxChildProfit;
}
};
return dfs(0, -1, 0);
}
};
``` | 1 | 0 | ['C++'] | 0 |
most-profitable-path-in-a-tree | Using DFS and BFS | using-dfs-and-bfs-by-sanjoli_121-0bm1 | IntuitionThe problem requires us to maximize Alice's profit as she traverses a tree while Bob moves towards the root. Since Bob and Alice move independently, we | Sanjoli_121 | NORMAL | 2025-02-24T14:58:30.048079+00:00 | 2025-02-24T14:58:30.048079+00:00 | 58 | false | # Intuition
The problem requires us to maximize Alice's profit as she traverses a tree while Bob moves towards the root. Since Bob and Alice move independently, we need to track Bob’s path to correctly apply the reward-sharing rules.
# Approach
1. **Build the Tree**: Convert the given `edges` list into an adjacency list representation.
2. **Find Bob's Path to Root**: Use DFS from Bob's starting node to track the time Bob reaches each node.
3. **Simulate Alice's Movement**: Use BFS to explore Alice’s paths and calculate her maximum possible income:
- If Alice reaches a node first, she collects the full reward.
- If Alice and Bob reach a node simultaneously, they split the reward.
- If Bob reaches first, Alice gets nothing from that node.
4. **Identify Leaf Nodes**: Track Alice’s income only when she reaches a leaf node and update the maximum profit.
# Complexity
- **Time Complexity**: $$O(n)$$
- Constructing the tree takes $$O(n)$$.
- Bob’s DFS takes $$O(n)$$.
- Alice’s BFS takes $$O(n)$$.
- **Space Complexity**: $$O(n)$$
- The adjacency list, visited array, and queue all use $$O(n)$$ space.
# Code
```python
from collections import defaultdict, deque
class Solution(object):
def mostProfitablePath(self, edges, bob, amount):
"""
:type edges: List[List[int]]
:type bob: int
:type amount: List[int]
:rtype: int
"""
n = len(amount)
self.tree = [[] for i in range(n)]
self.bob_t = {}
self.is_open = [False] * n
profit = -float("inf")
# Build adjacency list representation of the tree
for u, v in edges:
self.tree[u].append(v)
self.tree[v].append(u)
# Bob's DFS to determine time to reach each node
self.dfs(bob, 0)
# Alice's BFS traversal
self.is_open = [False] * n
q = deque([(0, 0, 0)]) # (node, time, income)
while q:
s, t, inc = q.popleft()
# Apply reward-sharing rules
if s not in self.bob_t or t < self.bob_t[s]:
inc += amount[s]
elif t == self.bob_t[s]:
inc += amount[s] // 2
# Check if it's a leaf node (except root)
if len(self.tree[s]) == 1 and s != 0:
profit = max(inc, profit)
# Expand search to child nodes
for node in self.tree[s]:
if not self.is_open[node]:
q.append((node, t + 1, inc))
# Mark current node as visited
self.is_open[s] = True
return profit
def dfs(self, s, t):
""" DFS to find Bob’s time of arrival at each node """
self.is_open[s] = True
self.bob_t[s] = t
if s == 0:
return True
for node in self.tree[s]:
if not self.is_open[node]:
if self.dfs(node, t + 1):
return True
self.bob_t.pop(s, None)
return False
``` | 1 | 0 | ['Python'] | 0 |
most-profitable-path-in-a-tree | Beats 100% || Basic and Simple | beats-100-basic-and-simple-by-akshatchaw-2yil | IntuitionStore path of Bob from node 'bob' to root. Also store timestamps for each node in the path.Now for Alice, just find max sum path from root to leaf with | akshatchawla1307 | NORMAL | 2025-02-24T14:14:59.086177+00:00 | 2025-02-24T14:14:59.086177+00:00 | 74 | false | # Intuition
**Store path of Bob from node 'bob' to root. Also store timestamps for each node in the path.
Now for Alice, just find max sum path from root to leaf with conditions:**
1. if Alice reaches a node that is not in the path of bob, then Alice will get the full amount of profit or loss for that node.
2. else if Alice reaches the node before Bob, then also Alice will get full amount of profit or loss.
3. else if Alice reaches the node in the same time as Bob then Alice will get half the amount of profit or loss.
4. else Alice will not get any profit or loss.
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity: O(V+E)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(V)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int helper(vector<vector<int>>&adj,vector<int>&path,vector<int>&amount,vector<bool>&visited,int node,int time)
{
int sum=0;
visited[node]=1;
if(path[node]==-1 || path[node]>time)
sum+=amount[node];
else if(path[node]==time)
sum+=(amount[node]/2);
int sum1=INT_MIN;
for(int i=0;i<adj[node].size();i++)
{
if(!visited[adj[node][i]])
sum1=max(sum1,helper(adj,path,amount,visited,adj[node][i],time+1));
}
if(sum1!=INT_MIN)
sum+=sum1;
return sum;
}
int mostProfitablePath(vector<vector<int>>& edges, int bob, vector<int>& amount) {
int n=edges.size()+1;
vector<int>bpath;
vector<vector<int>>adj(n);
for(vector<int>i:edges)
{
adj[i[0]].push_back(i[1]);
adj[i[1]].push_back(i[0]);
}
queue<int>q;
vector<bool>visited(n,0);
vector<int>parent(n,-1);
q.push(0);
visited[0]=1;
while(!q.empty())
{
int node=q.front();
q.pop();
for(int i=0;i<adj[node].size();i++)
{
if(visited[adj[node][i]])
continue;
visited[adj[node][i]]=1;
parent[adj[node][i]]=node;
q.push(adj[node][i]);
if(adj[node][i]==bob)
{
int temp=adj[node][i];
while(temp!=-1)
{
bpath.push_back(temp);
temp=parent[temp];
}
break;
}
}
if(bpath.size())
break;
}
vector<int>path(n,-1);
for(int i=0;i<bpath.size();i++)
path[bpath[i]]=i;
fill(visited.begin(),visited.end(),0);
return helper(adj,path,amount,visited,0,0);
}
};
``` | 1 | 0 | ['C++'] | 0 |
most-profitable-path-in-a-tree | Beats 87.15% | C++ | DFS Approach | Linear Solution 🔥🔥🔥 | beats-8715-c-dfs-approach-linear-solutio-p35s | IntuitionIn this problem, we need to find the most profitable path for Alice, given that Bob also moves towards the root node (0). Since the input is a tree, th | kdds598 | NORMAL | 2025-02-24T13:53:53.408353+00:00 | 2025-02-24T13:53:53.408353+00:00 | 44 | false | # Intuition
In this problem, we need to find the most profitable path for Alice, given that Bob also moves towards the root node (0). Since the input is a tree, there exists only one unique path between any two nodes, which simplifies our traversal.
Key observations:
1. Since the input is a tree (i.e., a connected acyclic graph), we can use DFS to explore paths efficiently.
2. Bob will always move towards node `0`, meaning we can precompute Bob’s path.
3. Alice explores the tree using DFS while collecting profits based on the condition that Bob may have already visited or be present at certain nodes.
# Approach
1. **Graph Representation:**
Convert the `edges` list into an adjacency list representation of the tree.
2. **Finding Bob’s Path:**
- Use DFS to trace Bob’s unique path from `bob` to the root (`0`).
- Store Bob’s path to later adjust the `amount` values accordingly.
3. **Modifying Profits Based on Bob’s Path:**
- Nodes in the first half of Bob’s path are set to `0` (as Bob reaches them before Alice).
- If Bob and Alice reach a node simultaneously, we divide the `amount` by `2`.
4. **DFS for Maximum Profit Calculation:**
- Alice starts at `0` and explores the tree recursively.
- The goal is to find the path that yields the maximum total profit.
# Complexity
- **Time Complexity:**
- Constructing the adjacency list takes **O(n)**.
- Finding Bob’s path using DFS takes **O(n)**.
- Modifying the amount array takes **O(n)**.
- The final DFS traversal takes **O(n)**.
- Overall, the complexity is **O(n)**.
- **Space Complexity:**
- The adjacency list takes **O(n)**.
- Storing Bob’s path takes **O(n)** in the worst case.
- The recursion stack for DFS takes **O(n)** in the worst case.
- Hence, the overall space complexity is **O(n)**.
# Code
```cpp
class Solution {
public:
// Function to find Bob's path from his start position to node 0
bool findBobPath(vector<vector<int>> &adj, vector<int> &curr, vector<int> &bobpath, int start, int parent) {
if (start == 0) { // If we reached root node, store the path
bobpath = curr;
return true;
}
curr.push_back(start);
for (auto j : adj[start]) {
if (j != parent) {
if (findBobPath(adj, curr, bobpath, j, start))
return true;
}
}
curr.pop_back();
return false;
}
// DFS to calculate maximum profit for Alice
int dfs(vector<vector<int>> &adj, int start, int parent, vector<int>& amount) {
int maxProfit = INT_MIN;
for (auto nbr : adj[start]) {
if (nbr != parent) {
int subTreeProfit = amount[start] + dfs(adj, nbr, start, amount);
maxProfit = max(maxProfit, subTreeProfit);
}
}
return maxProfit == INT_MIN ? amount[start] : maxProfit;
}
int mostProfitablePath(vector<vector<int>>& edges, int bob, vector<int>& amount) {
int n = edges.size() + 1;
vector<vector<int>> adj(n);
// Construct adjacency list
for (auto &edge : edges) {
adj[edge[0]].push_back(edge[1]);
adj[edge[1]].push_back(edge[0]);
}
vector<int> bobpath, curr;
findBobPath(adj, curr, bobpath, bob, -1);
int pathLen = bobpath.size();
// Adjust amount array based on Bob's path
for (int i = 0; i < pathLen / 2; i++) {
amount[bobpath[i]] = 0;
}
if (pathLen % 2 == 1) {
amount[bobpath[pathLen / 2]] = 0;
} else {
amount[bobpath[pathLen / 2]] /= 2;
}
// Alice starts DFS from node 0
return dfs(adj, 0, -1, amount);
}
};
| 1 | 0 | ['Tree', 'Depth-First Search', 'Graph', 'C++'] | 0 |
most-profitable-path-in-a-tree | Traversing Alice and Bob Path at the same time | Bidirectional Graph | Backtracking DFS + DP | | traversing-alice-and-bob-path-at-the-sam-hatt | IntuitionThere are 2 ways to solve this problem.1. To simulate both Alice and Bob path simultaneously.2. To seperatly calculate Bob Path and use it with time co | Prateek_Sarna_24 | NORMAL | 2025-02-24T13:25:34.719401+00:00 | 2025-02-24T20:03:52.679499+00:00 | 46 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
#### There are 2 ways to solve this problem.
#### 1. To simulate both Alice and Bob path simultaneously.
#### 2. To seperatly calculate Bob Path and use it with time constraints while traversing Alice path.
### We will use the 1st Approach.
## Note : We are given a bidirectional Graph.
---
# Approach
<!-- Describe your approach to solving the problem. -->
#### 1. Requirments :
1. Find `N` or the total number of nodes by traversing the given edge list and finding the maxValue. Then Since indexed 0 based, so do N++.
2. Create an edge list of format `{0 : {child1, child2...}, 1: ...}` This could either be a Map OR a vector, since the keys are `[0, N)`.
3. Create GateState vector, this signifies the state of node at any point of traversal. This is different from Visited Array.
4. Make visited Array, since we are traversing a graph, we dont want to traverse a visited node again.
5. Use BFS to find parents of each Node, Make a Parent Vactor.
#### 2. Backtracking :
1. Use a backtracking DFS function to explore Alice Path, But also note that we are simtaneously traversing bob path as well.
`void func(CurrNode, BobNode, AliceIncome, visited, gateStateVector, EdgeList, AmountVector)`
2. At currNode, we check if BobNode == AliceNode, if that is the case then we share the amount, **Note : At this point the gate will always be closed, since no one reached it before**.
3. we mark the gateState as open, and also store the current information in a boolean variable which we will be using further.
4. If the they are on different nodes, then also check if gates are closed then only add to income. and mark open.
5. Make dfs calls. **Bob call will go to Parent[bobNode]**. Also note that we only call the unvisited child.
6. After we are back from recursive calls, we backtrack the state of visited array, and gateStates of both Alice and Bob.
#### Base case : Although the code acts a self base case, but here we need a base case to update the maxNetIncome, So if all children of the node are Visited, then that means we are at leaf node, and we update the maxNetIncome.
---
# Complexity
- Time complexity: $$O(V + E)$$
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: $$O(V + E)$$
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
---
# Code
```cpp []
class Solution {
private:
// final answer
int maxNetIncome = INT_MIN;
// function to find parent of each Node.
void bfsFindParent(vector<vector<int>>& edgeList, vector<bool>& visited, vector<int>& parent) {
queue<pair<int, int>> q;
q.push({0, 0});
visited[0] = 1;
while (q.size()) {
auto front = q.front();
q.pop();
int Parent = front.first;
int child = front.second;
parent[child] = Parent;
for (int& childNode : edgeList[child]) {
if (!visited[childNode]) {
visited[childNode] = 1;
q.push({child, childNode});
}
}
}
}
// function to calcuate optimal path of Alice.
void dfsBacktrack(vector<vector<int>>& edgeList, vector<int>& amount, vector<int>& parent, int aliceNode, int bobNode, int aliceIncome, vector<bool>& gateState, vector<bool>& visited) {
// mark visited
visited[aliceNode] = 1;
// check gateState and if Alice and Bob are at same Node or not.
bool AliceGateOpened = false;
bool bobGateOpened = false;
if (aliceNode == bobNode) {
aliceIncome += amount[aliceNode] / 2;
gateState[aliceNode] = true;
AliceGateOpened = true;
bobGateOpened = true;
}
else {
if (!gateState[aliceNode]) {
AliceGateOpened = true;
gateState[aliceNode] = true;
aliceIncome += amount[aliceNode];
}
if (!gateState[bobNode]) {
bobGateOpened = true;
gateState[bobNode] = true;
}
}
bool allChildUnvisited = true;
for (int& childNode : edgeList[aliceNode]) {
if (!visited[childNode]) {
allChildUnvisited = false;
// make recursive call
dfsBacktrack(edgeList, amount, parent, childNode, parent[bobNode], aliceIncome, gateState, visited);
}
}
// BASE CASE, IF CURR Node is Leaf node or not, if it is then update maxIncome
if (allChildUnvisited) {
maxNetIncome = max(maxNetIncome, aliceIncome);
}
// backtrack the states of visited array and gateState
if (AliceGateOpened) {
gateState[aliceNode] = false;
}
if (bobGateOpened) {
gateState[bobNode] = false;
}
visited[aliceNode] = 0;
}
public:
int mostProfitablePath(vector<vector<int>>& edges, int bob, vector<int>& amount) {
// Find the total number of Nodes 'N'.
int n = 0;
for (auto& edge : edges) {
n = max(n, max(edge[0], edge[1]));
}
n++;
vector<vector<int>> edgeList(n);
vector<int> parent(n, 0);
// create required ege list
for (auto& edge : edges) {
int u = edge[0];
int v = edge[1];
edgeList[u].push_back(v);
edgeList[v].push_back(u);
}
vector<bool> gateState(n, 0);
vector<bool> visited(n, 0);
bfsFindParent(edgeList, visited, parent);
for (int i = 0; i < n; i++) {
visited[i] = 0;
}
dfsBacktrack(edgeList, amount, parent, 0, bob, 0, gateState, visited);
// return the result
return maxNetIncome;
}
};
``` | 1 | 0 | ['Hash Table', 'Dynamic Programming', 'Backtracking', 'Greedy', 'Tree', 'Depth-First Search', 'Breadth-First Search', 'Graph', 'Shortest Path', 'C++'] | 0 |
most-profitable-path-in-a-tree | Easy C++ Approach | DFS + BFS 🔥🔥🔥 | easy-c-approach-dfs-bfs-by-rayleigh_123-drui | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | RayLeigh_123 | NORMAL | 2025-02-24T13:25:11.163588+00:00 | 2025-02-24T13:25:11.163588+00:00 | 10 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
bool filltimer(vector<int>& timer, int node,
unordered_map<int, list<int>>& adj,vector<bool>&vis,int count) {
if(node==0){
return true;
}
vis[node] = true;
for(auto neigh:adj[node]){
if(!vis[neigh]){
if(filltimer(timer,neigh,adj,vis,count+1)){
timer[neigh] = count+1;
return true;
}
}
}
return false;
}
int mostProfitablePath(vector<vector<int>>& edges, int bob,
vector<int>& amount) {
unordered_map<int, list<int>> adj;
for (auto edge : edges) {
int u = edge[0];
int v = edge[1];
adj[u].push_back(v);
adj[v].push_back(u);
}
int n = edges.size();
vector<int> timer(n + 1, -1);
vector<bool> vis1(timer.size(), false);
filltimer(timer, bob, adj,vis1,0);
timer[bob] =0;
int counter = 1;
queue<int> q;
q.push(0);
vector<int> nodemax(n + 1, 0);
nodemax[0] = amount[0];
vector<bool> vis(timer.size(), false);
vis[0] = true;
while (!q.empty()) {
int sz = q.size();
for (int i = 0; i < sz; i++) {
auto node = q.front();
q.pop();
for (auto neigh : adj[node]) {
if (!vis[neigh]) {
vis[neigh] = true;
if (timer[neigh] == counter) {
nodemax[neigh] =nodemax[node] + (amount[neigh]) / 2;
}else if(timer[neigh]==-1 ||timer[neigh] > counter ){
nodemax[neigh] = amount[neigh]+ nodemax[node];
}else if (timer[neigh] < counter) {
nodemax[neigh] = nodemax[node];
}
q.push(neigh);
}
}
}
counter++;
}
int maxi = -1e9;
for(int i=1;i<=n;i++){
if(adj[i].size()==1){
maxi = max(maxi,nodemax[i]);
}
}
return maxi;
}
};
``` | 1 | 0 | ['C++'] | 0 |
most-profitable-path-in-a-tree | simple thought process | simple-thought-process-by-harismanzar977-x0t1 | Code | harismanzar977 | NORMAL | 2025-02-24T13:02:58.745599+00:00 | 2025-02-24T13:02:58.745599+00:00 | 49 | false | # Code
```cpp []
class Solution {
public:
vector<int> leafnodes(int n, vector<vector<int>>& adj) {
vector<int> leaf;
for (int i = 0; i < n; i++) {
if (adj[i].size() == 1) {
leaf.push_back(i);
}
}
sort(leaf.begin(), leaf.end());
return leaf;
}
int dfs(int node, vector<vector<int>> &adj, vector<int> &amount, vector<bool> &vis) {
vis[node] = true;
if (adj[node].size() == 1 && node != 0) {
return amount[node];
}
int maxProfit = INT_MIN;
for (auto &neighbor : adj[node]) {
if (!vis[neighbor]) {
maxProfit = max(maxProfit, dfs(neighbor, adj, amount, vis));
}
}
vis[node] = false;
return amount[node] + (maxProfit == INT_MIN ? 0 : maxProfit);
}
bool boba(int node, vector<vector<int>>& adj, vector<int>& v,
vector<bool>& vis) {
vis[node] = 1;
if (node == 0) {
v.push_back(node);
return true;
}
for (auto& it : adj[node]) {
if (!vis[it]) {
if (boba(it, adj, v, vis)) {
v.push_back(node);
return true;
}
}
}
return false;
}
int mostProfitablePath(vector<vector<int>>& edges, int bob,
vector<int>& amount) {
int n = edges.size();
vector<vector<int>> adj(n + 1);
for (auto& it : edges) {
adj[it[0]].push_back(it[1]);
adj[it[1]].push_back(it[0]);
}
vector<int> store(n + 1);
for (int i = 0; i <= n; i++) {
store[i] = amount[i];
}
// for (auto &it : adj) {
// for (auto &i : it) {
// cout << i << " ";
// }
// cout << endl;
// }
vector<int> leafs = leafnodes(n + 1, adj);
vector<int> b;
vector<bool> vis(n + 1, 0);
boba(bob, adj, b, vis);
// for (auto& it : b) {
// cout << it << " ";
// }
// cout << endl;
int z = b.size();
for (int i = b.size() - 1; i > b.size() / 2; i--) {
store[b[i]] = 0;
}
// int z = b.size();
if (z % 2 != 0) {
int midIndex = z / 2;
store[b[midIndex]] /= 2;
}
else {
store[b[z/2]]=0;
}
// for (int i = 0; i <= n; i++) {
// cout<<store[i]<<" ";
// }
// cout<<endl;
vector<bool> visited(n+1,false);
int ans = dfs(0,adj,store,visited);
return ans;
}
};
``` | 1 | 0 | ['Tree', 'Depth-First Search', 'Breadth-First Search', 'Graph', 'C++'] | 0 |
most-profitable-path-in-a-tree | Easiest solution in Java !!!!!!!!!!!!!!!!!!!!!! | easiest-solution-in-java-by-bips7aa-7t95 | IntuitionThe problem presents Alice and Bob navigating through a tree structure, where Alice seeks to maximize her net income by reaching leaf nodes while Bob r | bips7aa | NORMAL | 2025-02-24T12:17:36.277082+00:00 | 2025-02-24T12:17:36.277082+00:00 | 21 | false | # Intuition
The problem presents Alice and Bob navigating through a tree structure, where Alice seeks to maximize her net income by reaching leaf nodes while Bob returns to the root. The gates at each node either cost money (which negatively impacts Alice’s income) or provide cash rewards (which boosts Alice's income).
To maximize her net income, Alice needs to strategically navigate the tree towards leaf nodes while considering Bob's path towards the root. In particular, we need to keep track of nodes where Alice and Bob might meet (their paths overlap), and how this influences Alice's total result.
# Approach
1. Graph Representation:
First, represent the tree using an adjacency list from the given edges.
2. Breadth-First Search (BFS):
To determine the depth of each node and also find out how Bob will traverse the tree back to the root, perform a BFS from the root node (0).
Maintain two arrays: one for the depth of each node and another to keep track of Bob’s travel path to each node.
3. DFS for Alice:
Perform a Depth-First Search (DFS) from the root node (0) to explore all paths, aiming to reach every leaf node.
As you explore each node, calculate Alice’s net income:
If Alice arrives at a node before Bob, she collects the full reward or pays the full cost.
If they arrive simultaneously, they split the cost/reward.
Keep track of the maximum net income at each leaf node.
4. Calculating the Net Income:
For each node Alice visits during her DFS, determine the cost/reward based on the timing of her and Bob's arrival.
Aggregate the income and update the maximum when she reaches a leaf node.
5. Optimization:
Since there can be many paths (due to the potential size of n), ensure that the algorithm remains efficient by avoiding redundant calculations.
# Complexity
- Time complexity: The overall time complexity is O(n) since both BFS and DFS will traverse each node and edge once, leading to a linear relationship with the number of nodes.
- Space complexity: The space complexity is also O(n) due to the adjacency list representation of the tree and the additional space used for the depth and net income calculations.
# Code
```java []
class Solution {
public int mostProfitablePath(int[][] edges, int bob, int[] amount) {
final int n = amount.length;
List<Integer>[] tree = new List[n];
int[] parent = new int[n];
int[] aliceDist = new int[n];
Arrays.fill(aliceDist, -1);
for (int i = 0; i < n; ++i)
tree[i] = new ArrayList<>();
for (int[] edge : edges) {
final int u = edge[0];
final int v = edge[1];
tree[u].add(v);
tree[v].add(u);
}
dfs(tree, 0, -1, 0, parent, aliceDist);
// Modify the amount along the path from node Bob to node 0.
// For each node,
// 1. If Bob reaches earlier than Alice does, change the amount to 0.
// 2. If Bob and Alice reach simultaneously, devide the amount by 2.
for (int u = bob, bobDist = 0; u != 0; u = parent[u], ++bobDist)
if (bobDist < aliceDist[u])
amount[u] = 0;
else if (bobDist == aliceDist[u])
amount[u] /= 2;
return getMoney(tree, 0, -1, amount);
}
// Fills `parent` and `dist`.
private void dfs(List<Integer>[] tree, int u, int prev, int d, int[] parent, int[] dist) {
parent[u] = prev;
dist[u] = d;
for (final int v : tree[u]) {
if (dist[v] == -1)
dfs(tree, v, u, d + 1, parent, dist);
}
}
private int getMoney(List<Integer>[] tree, int u, int prev, int[] amount) {
// a leaf node
if (tree[u].size() == 1 && tree[u].get(0) == prev)
return amount[u];
int maxPath = Integer.MIN_VALUE;
for (final int v : tree[u])
if (v != prev)
maxPath = Math.max(maxPath, getMoney(tree, v, u, amount));
return amount[u] + maxPath;
}
}
``` | 1 | 0 | ['Java'] | 0 |
most-profitable-path-in-a-tree | Beat 44% complexity | beat-44-complexity-by-usac_07-atud | Code | Usac_07 | NORMAL | 2025-02-24T12:13:09.366527+00:00 | 2025-02-24T12:13:09.366527+00:00 | 43 | false | # Code
```python3 []
class Solution:
def mostProfitablePath(self, edges: List[List[int]], bob: int, amount: List[int]) -> int:
n=len(edges)+1
adj=[[] for _ in range(n)]
parent=[-1]*n
Bob=[float('inf')]*n
for u, v in edges:
adj[u].append(v)
adj[v].append(u)
# Step 1: Build parent information using DFS
def dfs(i, p):
parent[i] = p
for j in adj[i]:
if j == p: continue
dfs(j, i)
dfs(0, -1) # Start with -1 as the parent of root
# Step 2: Compute Bob's arrival times
x=bob
move=0
while x!=-1:
Bob[x]=move
move+=1
x=parent[x]
# Step 3: DFS to compute Alice's best profit path
def dfs_sum(i, dist, prev):
alice=0
if dist < Bob[i]:
alice=amount[i] # Alice takes full amount
elif dist==Bob[i]:
alice=amount[i]//2 # Both reach at the same time
isLeaf=True
maxLeafSum=-float('inf')
for j in adj[i]:
if j == prev: continue
isLeaf=False
maxLeafSum = max(maxLeafSum, dfs_sum(j, dist+1, i))
return alice if isLeaf else alice + maxLeafSum
return dfs_sum(0, 0, -1)
``` | 1 | 0 | ['Python3'] | 0 |
most-profitable-path-in-a-tree | Python3: Map nodes to levels, build Bob's path, simulate Alice's path (BFS) | python3-map-nodes-to-levels-build-bobs-p-ccvh | Intuition & ApproachThere's only one way of going from a leaf to the root, so we calculate Bob's path first.In order to do that, we can just move Bob up the tre | bb-ctrl | NORMAL | 2025-02-24T11:18:14.124194+00:00 | 2025-02-24T11:18:14.124194+00:00 | 47 | false | # Intuition & Approach
There's only one way of going from a leaf to the root, so we calculate Bob's path first.
In order to do that, we can just move Bob up the tree.
To know what is "up", we can pre-calculate the level of each node (BFS).
Then we move Alice down the tree, level by level (BFS), while simultaneously moving Bob along his calculated path.
At each level, we first halve the amount at the node that Bob is currently visiting, then we process the level from Alice's perspective.
Whenever Alice encounters a node that doesn't have deeper adjacent nodes, we know that it's a leaf, so we update the best possible score accordingly.
Technically, we could probably build Bob's path as we go (in the simulation for Alice), but it wouldn't change the time complexity.
# Complexity
- Time complexity:
$$O(n)$$ - We traverse each node/edge a constant number of times.
- Space complexity:
$$O(n)$$ - We're keeping the level for each node, seen nodes (for the first BFS) and Bob's path, all of which are O(n).
# Code
```python3 []
from collections import defaultdict
import heapq
class Solution:
def mostProfitablePath(self, edges: List[List[int]], bob: int, amount: List[int]) -> int:
# Build adjacencies
adjs = defaultdict(list)
for a, b in edges:
adjs[a].append(b)
adjs[b].append(a)
# Map each node to its level
levels_by_node = dict()
q = deque([0])
seen = set()
level = 0
while q:
for _ in range(len(q)):
x = q.popleft()
seen.add(x)
levels_by_node[x] = level
for adj in adjs[x]:
if adj not in seen:
q.append(adj)
level += 1
# Calculate Bob's path
bob_path = []
q = deque([bob])
while q:
x = q.popleft()
bob_path.append(x)
for adj in adjs[x]:
if levels_by_node[adj] == levels_by_node[x] - 1:
q.append(adj)
# Do BFS from Alice's perspective
q = deque([(0,0)]) # (node, total_score)
level = 0
bob_idx = 0
best = -inf
while q:
if bob_idx < len(bob_path):
amount[bob_path[bob_idx]] //= 2
for _ in range(len(q)):
x, score = q.popleft()
score += amount[x]
is_leaf = True
for adj in adjs[x]:
if levels_by_node[adj] == levels_by_node[x] + 1:
q.append((adj, score))
is_leaf = False
if is_leaf:
best = max(best, score)
if bob_idx < len(bob_path):
# Not strictly necessary, since we won't be returning to the node
amount[bob_path[bob_idx]] = 0
bob_idx += 1
return best
``` | 1 | 0 | ['Python3'] | 1 |
most-profitable-path-in-a-tree | easy dfs solution | easy-dfs-solution-by-afatwapas-i06q | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | afatwapas | NORMAL | 2025-02-24T10:35:06.622301+00:00 | 2025-02-24T10:35:06.622301+00:00 | 58 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->O(v+e)
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->O(v^2)
# Code
```cpp []
class Solution {
vector<int> path;
bool dfs(int node,int pa,vector<vector<int>>& adj,int d){
if(node==0 ){
return true;
}
bool f=0;
for(auto i:adj[node]){
if(i!= pa && path[i]==-1){
path[i]= d;
if(dfs(i,node,adj,d+1)) return true;
path[i]=-1;
}
}
return 0;
}
int solve(int node,int pa,vector<vector<int>>& adj,int d,vector<int>& amount){
int maxi=INT_MIN;
int x=0;
if(path[node] ==-1 || path[node]> d) x+= amount[node];
else if(path[node]==d) x+= amount[node]/2;
for(auto i:adj[node]){
if(i!= pa){
maxi= max(maxi,solve(i,node,adj,d+1,amount)+x);
}
}
if(maxi== INT_MIN) return x;
return maxi;
}
public:
int mostProfitablePath(vector<vector<int>>& edges, int bob, vector<int>& amount) {
int n= edges.size()+1;
vector<vector<int>> adj(n);
for(auto i: edges){
adj[i[0]].push_back(i[1]);
adj[i[1]].push_back(i[0]);
}
path.resize(n,-1);
path[bob]=0;
dfs(bob,-1,adj,1);
int ans= solve(0,-1,adj,0,amount);
return ans;
}
};
``` | 1 | 0 | ['Array', 'Tree', 'Depth-First Search', 'Graph', 'C++'] | 0 |
most-profitable-path-in-a-tree | Two DFS. Simple solution | two-dfs-simple-solution-by-xxxxkav-0jz8 | null | xxxxkav | NORMAL | 2025-02-24T10:20:41.016784+00:00 | 2025-02-24T12:08:56.593182+00:00 | 72 | false | ```
class Solution:
def mostProfitablePath(self, edges: List[List[int]], bob: int, amount: List[int]) -> int:
def dfs_bob(node, parent, time):
if node == 0:
reach_time[node] = time
return True
for neighbor in graph[node]:
if neighbor != parent and dfs_bob(neighbor, node, time+1):
reach_time[node] = time
return True
return False
def dfs_alice(node, parent, time):
income = -inf
for neighbor in graph[node]:
if neighbor != parent:
income = max(dfs_alice(neighbor, node, time+1), income)
if income == -inf: # Current node is a leaf
income = 0
if time < reach_time[node]:
return income + amount[node]
if time == reach_time[node]:
return income + amount[node] // 2
return income
graph = [[] for _ in range(len(edges)+1)]
for u, v in edges:
graph[u].append(v)
graph[v].append(u)
reach_time = [inf]*(len(edges)+1)
dfs_bob(bob, -1, 0)
return dfs_alice(0, -1, 0)
``` | 1 | 0 | ['Depth-First Search', 'Python3'] | 0 |
big-countries | Union and OR and the Explanation | union-and-or-and-the-explanation-by-new2-5xav | Two obvious solutions:\n\n#OR\nSELECT name, population, area\nFROM World\nWHERE area > 3000000 OR population > 25000000\n\nAnd Faster Union\n\n#Union\nSELECT na | new2500 | NORMAL | 2017-07-16T03:27:02.692000+00:00 | 2018-10-19T00:22:35.565708+00:00 | 39,133 | false | Two obvious solutions:\n```\n#OR\nSELECT name, population, area\nFROM World\nWHERE area > 3000000 OR population > 25000000\n```\nAnd Faster Union\n```\n#Union\nSELECT name, population, area\nFROM World\nWHERE area > 3000000 \n\nUNION\n\nSELECT name, population, area\nFROM World\nWHERE population > 25000000\n```\n\nWhy `Union` is faster than `OR`? \n\nStrictly speaking, Using ` UNION` is faster when it comes to cases like **scan two different column like this**. \n\n(Of course using `UNION ALL` is much faster than `UNION` since we don't need to sort the result. But it violates the requirements)\n\n\nSuppose we are searching `population` and `area`, Given that MySQL usually uses one one index per table in a given query, so when it uses the 1st index rather than 2nd index, it would still have to do a table-scan to find rows that fit the 2nd index. \n\nWhen using `UNION`, each sub-query can use the index of its search, then combine the sub-query by `UNION`.\n\n\nI quote from a [benchmark](http://www.sql-server-performance.com/2011/union-or-sql-server-queries/) about `UNION` and `OR`, feel free to check it out:\n\n```\nScenario 3: Selecting all columns for different fields\n CPU Reads Duration Row Counts\nOR 47 1278 443 1228\nUNION 31 1334 400 1228\n\nScenario 4: Selecting Clustered index columns for different fields\n CPU Reads Duration Row Counts\nOR 0 319 366 1228\nUNION 0 50 193 1228\n``` | 300 | 6 | [] | 29 |
big-countries | One Line Of Code using UNION and OR | one-line-of-code-using-union-and-or-by-g-wfl5 | \n\n# 1. Using OR\n\nselect name,population,area from world where (area>=3000000 or population>=25000000)\n\n# 2. Using UNION\n\nselect area,population,name\nfr | GANJINAVEEN | NORMAL | 2023-03-31T13:15:07.772449+00:00 | 2023-03-31T13:15:07.772491+00:00 | 20,529 | false | \n\n# 1. Using OR\n```\nselect name,population,area from world where (area>=3000000 or population>=25000000)\n```\n# 2. Using UNION\n```\nselect area,population,name\nfrom world\nwhere area>=3000000\nunion\nselect area,population,name\nfrom world\nwhere population>=25000000\n\n```\n# please upvote me it would encourage me alot\n | 93 | 0 | ['MySQL'] | 5 |
big-countries | ✅EASY MY SQL SOLUTION | easy-my-sql-solution-by-swayam28-s1da | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | swayam28 | NORMAL | 2024-08-07T11:35:32.260080+00:00 | 2024-08-10T08:20:42.291407+00:00 | 19,774 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n# Write your MySQL query statement below\nselect name ,population,area from world where area>=3000000 or population>=25000000;\n```\n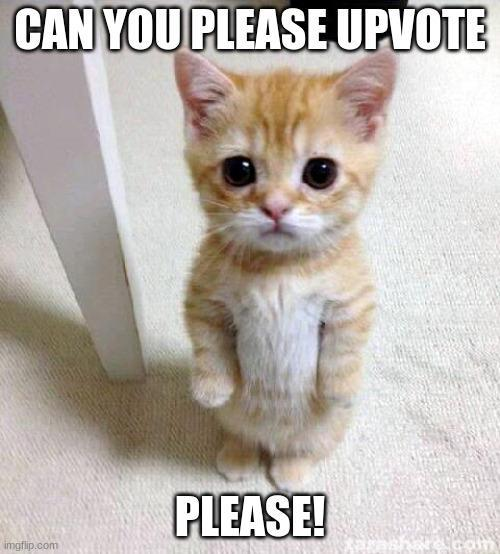 | 68 | 0 | ['MySQL'] | 2 |
big-countries | Pandas vs SQL | Elegant & Short | All 30 Days of Pandas solutions ✅ | pandas-vs-sql-elegant-short-all-30-days-4d3qr | Complexity\n- Time complexity: O(n)\n- Space complexity: O(n)\n\n# Code\nPython []\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n return world[\n | Kyrylo-Ktl | NORMAL | 2023-08-01T14:25:24.294468+00:00 | 2023-08-06T16:37:10.291172+00:00 | 12,759 | false | # Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(n)$$\n\n# Code\n```Python []\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n return world[\n (world[\'area\'] >= 3_000_000) | \\\n (world[\'population\'] >= 25_000_000)\n ][[\'name\', \'population\', \'area\']]\n```\n```SQL []\nSELECT name,\n population,\n area\n FROM World\n WHERE population >= 25000000\n OR area >= 3000000;\n```\n\n# Important!\n###### If you like the solution or find it useful, feel free to **upvote** for it, it will support me in creating high quality solutions)\n\n# 30 Days of Pandas solutions\n\n### Data Filtering \u2705\n- [Big Countries](https://leetcode.com/problems/big-countries/solutions/3848474/pandas-elegant-short-1-line/)\n- [Recyclable and Low Fat Products](https://leetcode.com/problems/recyclable-and-low-fat-products/solutions/3848500/pandas-elegant-short-1-line/)\n- [Customers Who Never Order](https://leetcode.com/problems/customers-who-never-order/solutions/3848527/pandas-elegant-short-1-line/)\n- [Article Views I](https://leetcode.com/problems/article-views-i/solutions/3867192/pandas-elegant-short-1-line/)\n\n\n### String Methods \u2705\n- [Invalid Tweets](https://leetcode.com/problems/invalid-tweets/solutions/3849121/pandas-elegant-short-1-line/)\n- [Calculate Special Bonus](https://leetcode.com/problems/calculate-special-bonus/solutions/3867209/pandas-elegant-short-1-line/)\n- [Fix Names in a Table](https://leetcode.com/problems/fix-names-in-a-table/solutions/3849167/pandas-elegant-short-1-line/)\n- [Find Users With Valid E-Mails](https://leetcode.com/problems/find-users-with-valid-e-mails/solutions/3849177/pandas-elegant-short-1-line/)\n- [Patients With a Condition](https://leetcode.com/problems/patients-with-a-condition/solutions/3849196/pandas-elegant-short-1-line-regex/)\n\n\n### Data Manipulation \u2705\n- [Nth Highest Salary](https://leetcode.com/problems/nth-highest-salary/solutions/3867257/pandas-elegant-short-1-line/)\n- [Second Highest Salary](https://leetcode.com/problems/second-highest-salary/solutions/3867278/pandas-elegant-short/)\n- [Department Highest Salary](https://leetcode.com/problems/department-highest-salary/solutions/3867312/pandas-elegant-short-1-line/)\n- [Rank Scores](https://leetcode.com/problems/rank-scores/solutions/3872817/pandas-elegant-short-1-line-all-30-days-of-pandas-solutions/)\n- [Delete Duplicate Emails](https://leetcode.com/problems/delete-duplicate-emails/solutions/3849211/pandas-elegant-short/)\n- [Rearrange Products Table](https://leetcode.com/problems/rearrange-products-table/solutions/3849226/pandas-elegant-short-1-line/)\n\n\n### Statistics \u2705\n- [The Number of Rich Customers](https://leetcode.com/problems/the-number-of-rich-customers/solutions/3849251/pandas-elegant-short-1-line/)\n- [Immediate Food Delivery I](https://leetcode.com/problems/immediate-food-delivery-i/solutions/3872719/pandas-elegant-short-1-line-all-30-days-of-pandas-solutions/)\n- [Count Salary Categories](https://leetcode.com/problems/count-salary-categories/solutions/3872801/pandas-elegant-short-1-line-all-30-days-of-pandas-solutions/)\n\n\n### Data Aggregation \u2705\n- [Find Total Time Spent by Each Employee](https://leetcode.com/problems/find-total-time-spent-by-each-employee/solutions/3872715/pandas-elegant-short-1-line-all-30-days-of-pandas-solutions/)\n- [Game Play Analysis I](https://leetcode.com/problems/game-play-analysis-i/solutions/3863223/pandas-elegant-short-1-line/)\n- [Number of Unique Subjects Taught by Each Teacher](https://leetcode.com/problems/number-of-unique-subjects-taught-by-each-teacher/solutions/3863239/pandas-elegant-short-1-line/)\n- [Classes More Than 5 Students](https://leetcode.com/problems/classes-more-than-5-students/solutions/3863249/pandas-elegant-short/)\n- [Customer Placing the Largest Number of Orders](https://leetcode.com/problems/customer-placing-the-largest-number-of-orders/solutions/3863257/pandas-elegant-short-1-line/)\n- [Group Sold Products By The Date](https://leetcode.com/problems/group-sold-products-by-the-date/solutions/3863267/pandas-elegant-short-1-line/)\n- [Daily Leads and Partners](https://leetcode.com/problems/daily-leads-and-partners/solutions/3863279/pandas-elegant-short-1-line/)\n\n\n### Data Aggregation \u2705\n- [Actors and Directors Who Cooperated At Least Three Times](https://leetcode.com/problems/actors-and-directors-who-cooperated-at-least-three-times/solutions/3863309/pandas-elegant-short/)\n- [Replace Employee ID With The Unique Identifier](https://leetcode.com/problems/replace-employee-id-with-the-unique-identifier/solutions/3872822/pandas-elegant-short-1-line-all-30-days-of-pandas-solutions/)\n- [Students and Examinations](https://leetcode.com/problems/students-and-examinations/solutions/3872699/pandas-elegant-short-1-line-all-30-days-of-pandas-solutions/)\n- [Managers with at Least 5 Direct Reports](https://leetcode.com/problems/managers-with-at-least-5-direct-reports/solutions/3872861/pandas-elegant-short/)\n- [Sales Person](https://leetcode.com/problems/sales-person/solutions/3872712/pandas-elegant-short-1-line-all-30-days-of-pandas-solutions/)\n | 41 | 0 | ['Python', 'Python3', 'MySQL', 'Pandas'] | 4 |
big-countries | FULL Explanation UNLIKE ANYOTHERS - PANDAS SOLUTION 🤔🧠🤯 | full-explanation-unlike-anyothers-pandas-y5me | Intuition\nAs a begginer with pandas , I\'ll try my best to explain it.\nSo , What is pandas ? \nIt\'s a data visualization library in python and it\'s extremle | Sarah-2002 | NORMAL | 2023-08-02T08:28:36.838387+00:00 | 2023-08-02T08:28:36.838412+00:00 | 3,396 | false | # Intuition\nAs a begginer with pandas , I\'ll try my best to explain it.\n***So , What is pandas ?*** \nIt\'s a data visualization library in python and it\'s extremley helpful for data science and data analysis .\n\n***What is our problem here ?***\n1. We have 1 table called "World"\n2. 5 rows\n*We need to return the name , population and area of big countries*\n\n\n***What makes a country Big ?***\n- if it has an area of at least three million (i.e., 3000000 km2)\n- if it has a population of at least twenty-five million (i.e., 25000000).\n# Lets get started :)\n\n# Approach\n- We first import the pandas library to be able to use it as \'pd \'\n- Define a function called \'big_countries\' in which it takes a dataframe called \'world\'and must return a dataframe .\n- Moving on to our third line of code , we create a new dataframe called \' big_countries_df \' .\n- The new dataframe filters our exisiting dataframe \'world\' to match our condition of areas and population as follows :\n\n```\nworld[(world[\'area\'] >= 3000000) | (world[\'population\'] >= 25000000)]\n```\n- We then create a results dataframe , which only contains the name , population and area returned .\n- Finally we return the results dataframe \n# A QUESTION YOU MIGHT BE ASKING : WHAT\'S A DATAFRAME ?\nIn pandas , a dataframe is a datastructure and it\'s similar to a table in Relational database . If you\'re comming from SQL Background , you can think of it as a 2-D tabular dara with rows and columns .\n\n\n**Very Important : In pandas we take dataframe as an input and return one as an output , it\'s very important to remmember this !**\n\n\n\n\nNow that\'s it , hope my explnation was clear enough : )\n\n# Code\n```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n\n big_countries_df = world[(world[\'area\'] >= 3000000) | (world[\'population\'] >= 25000000)]\n\n\n result_df = big_countries_df[[\'name\', \'population\', \'area\']]\n\n return result_df\n``` | 30 | 0 | ['Brainteaser', 'Pandas'] | 2 |
big-countries | Easy and Simple Solution | easy-and-simple-solution-by-mayankluthya-69b5 | Please Like ❤️IntuitionThe task is to filter countries from the World table based on their population or area. The condition for a country to be included is:
It | mayankluthyagi | NORMAL | 2025-01-28T01:22:38.017121+00:00 | 2025-01-28T01:22:38.017121+00:00 | 8,194 | false | ```mysql
SELECT name, population, area
FROM World
WHERE area >= 3000000 OR population >= 25000000;
```
# Please Like ❤️
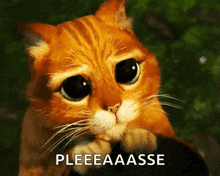
# Intuition
The task is to filter countries from the `World` table based on their population or area. The condition for a country to be included is:
1. Its area is greater than or equal to 3,000,000 square kilometers.
2. Its population is greater than or equal to 25,000,000.
# Approach
1. **Filter Conditions**: Use the `WHERE` clause with the logical operator `OR` to check for either of the conditions:
- `area >= 3000000`
- `population >= 25000000`
2. **Select Columns**: Return the `name`, `population`, and `area` columns for the filtered countries.
# Complexity
- **Time complexity**:
$$O(n)$$, where $$n$$ is the number of rows in the `World` table.
- **Space complexity**:
$$O(1)$$, as no additional data structures are used. | 25 | 0 | ['MySQL'] | 0 |
big-countries | Simple Two liner pandas code. Very easy to understand!!! | simple-two-liner-pandas-code-very-easy-t-9qnt | \n# Approach\n Describe your approach to solving the problem. \n- Use boolean indexing to filter the rows where either the "area" is greater than or equal to 3 | sriganesh777 | NORMAL | 2023-08-01T13:22:56.855820+00:00 | 2023-08-01T13:22:56.855852+00:00 | 12,844 | false | \n# Approach\n<!-- Describe your approach to solving the problem. -->\n- Use boolean indexing to filter the rows where either the "area" is greater than or equal to 3 million or the "population" is greater than or equal to 25 million.\n- Select only the columns "name," "population," and "area" from the filtered DataFrame.\n- Return the resulting DataFrame containing the name, population, and area of the big countries.\n\n# Code\n```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n big_countries_df = world[(world[\'area\'] >= 3000000) | (world[\'population\'] >= 25000000)]\n return big_countries_df[[\'name\', \'population\', \'area\']]\n\n``` | 19 | 0 | ['Database', 'Pandas'] | 6 |
big-countries | 📌 Simple MySql query easy to understand | simple-mysql-query-easy-to-understand-by-oyhv | \nselect name, population, area from World where area>=3000000 or population>=25000000\n | dark_wolf_jss | NORMAL | 2022-06-24T10:33:58.774222+00:00 | 2022-06-24T10:33:58.774258+00:00 | 5,211 | false | ```\nselect name, population, area from World where area>=3000000 or population>=25000000\n``` | 19 | 0 | ['MySQL'] | 1 |
big-countries | Easy AC | easy-ac-by-leiyu-ma9n | \nSELECT name,population,area \nFROM World \nWHERE population>25000000 OR area>3000000;\n | leiyu | NORMAL | 2017-05-22T12:16:38.899000+00:00 | 2017-05-22T12:16:38.899000+00:00 | 12,904 | false | ```\nSELECT name,population,area \nFROM World \nWHERE population>25000000 OR area>3000000;\n``` | 18 | 3 | [] | 2 |
big-countries | ✅💯Beats 98.63% of users || Best solution in MySQL || Beginner friendly👍🤝 | beats-9863-of-users-best-solution-in-mys-gg7c | PLEASE DO UPVOTE\n\n\n\n# Code\nMySQL []\n# Write your MySQL query statement below\nselect name, population, area from World where area>=3000000 \nunion \nselec | anand18402 | NORMAL | 2024-01-13T12:03:06.155874+00:00 | 2024-01-13T12:03:06.155904+00:00 | 2,268 | false | # PLEASE DO UPVOTE\n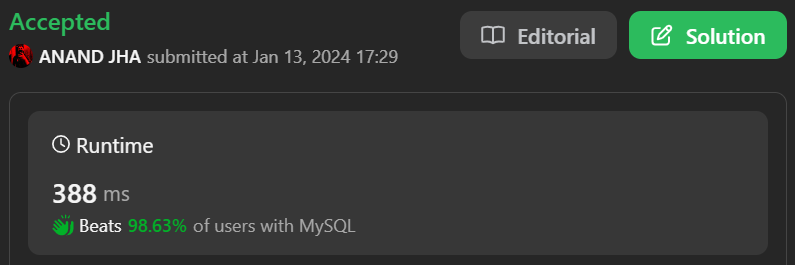\n\n\n# Code\n```MySQL []\n# Write your MySQL query statement below\nselect name, population, area from World where area>=3000000 \nunion \nselect name, population, area from World where population>=25000000\n```\n\n | 13 | 0 | ['Database', 'MySQL'] | 1 |
big-countries | SQL | Oracle Union and OR | sql-oracle-union-and-or-by-jainshubham76-c13r | \nIs Union is faster than OR?\n\n\nUsing UNION is faster in some cases when it comes to cases like scan two different column like this.\n\n(Of course using UNIO | jainshubham766 | NORMAL | 2022-04-20T19:41:58.906689+00:00 | 2022-04-20T19:41:58.906722+00:00 | 2,589 | false | ```\nIs Union is faster than OR?\n```\n\nUsing UNION is faster in some cases when it comes to cases like scan two different column like this.\n\n(Of course using UNION ALL is much faster than UNION since we don\'t need to sort the result. But it violates the requirements)\n\nUnion not always faster than or!\nMost good DBMSs use an internal query optimizer to combine the SELECT statements\nbefore they are even processed. In theory, this means that from a performance\nperspective, there should be no real difference between using multiple WHERE clause\nconditions or a UNION. I say in theory, because, in practice, most query optimizers\ndon\u2019t always do as good a job as they should. Your best bet is to test both methods to\nsee which will work best for you.\n\n```\n/* Write your PL/SQL query statement below */\n \n -- Approach 01: Using OR Operator\n SELECT name, population, area\n FROM World\n Where (area >= 3000000 ) OR (population >=25000000);\n \n \n -- Approach 02: Using UNION Operator --faster than OR\n SELECT name, population, area\n FROM World\n Where (area >= 3000000 ) \n \n UNION\n\n SELECT name, population, area\n FROM World\n Where (population >=25000000);\n```\n\n | 12 | 0 | ['MySQL', 'Oracle'] | 2 |
big-countries | pandas || 1 line, filter || T/S: 81% / 57% | pandas-1-line-filter-ts-81-57-by-spauldi-xams | \nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n \n return world.loc[(world[\'population\'] >= 25_000_000) |\n | Spaulding_ | NORMAL | 2024-05-14T07:42:38.579723+00:00 | 2024-05-29T05:30:03.352116+00:00 | 3,709 | false | ```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n \n return world.loc[(world[\'population\'] >= 25_000_000) |\n (world[\'area\'] >= 3_000_000)].iloc[:,[0,3,2]]\n \n```\n[https://leetcode.com/problems/big-countries/submissions/1265055097/](https://leetcode.com/problems/big-countries/submissions/1265055097/)\n\n\n\nI could be wrong, but I think that time complexity is *O*(*N*) and space complexity is *O*(*N*), in which *N* ~ `len(world)`. | 11 | 0 | ['Pandas'] | 0 |
big-countries | Simplest SOlution (With Approach) 😎😎 -> 😮😮 -> 🤯🤯 | simplest-solution-with-approach-by-jeele-zb98 | Intuition
The query retrieves information (name, population, and area) for countries that either have a large area (over 3 million) or a high population (over 2 | jeeleej | NORMAL | 2024-12-30T16:05:24.975773+00:00 | 2024-12-30T16:05:24.975773+00:00 | 1,338 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
- The query retrieves information (`name`, `population`, and `area`) for countries that either have a large area (over 3 million) or a high population (over 25 million). It uses the `OR` operator to include countries that meet at least one of these criteria.
# Approach
<!-- Describe your approach to solving the problem. -->
- **Table and Columns:** The query operates on the `World` table, filtering by `name`, `population`, and `area` columns.
2. **Conditions:** It filters rows where `area >= 3000000` or `population >= 25000000`.
3. **Combination of Conditions:** The `OR` operator ensures that if either condition is true, the row is included.
4. **Selection:** It returns `name`, `population`, and `area` for the matching rows.
---
# Code
```PostgreSQL []
-- Write your PostgreSQL query statement below
SELECT name , population , area
FROM World
WHERE area >= 3000000 OR population >= 25000000;
```
```MySQL []
-- Write your PostgreSQL query statement below
SELECT name , population , area
FROM World
WHERE area >= 3000000 OR population >= 25000000;
```
```MSSQL []
-- Write your PostgreSQL query statement below
SELECT name , population , area
FROM World
WHERE area >= 3000000 OR population >= 25000000;
```
```Oracle []
-- Write your PostgreSQL query statement below
SELECT name , population , area
FROM World
WHERE area >= 3000000 OR population >= 25000000;
```
---
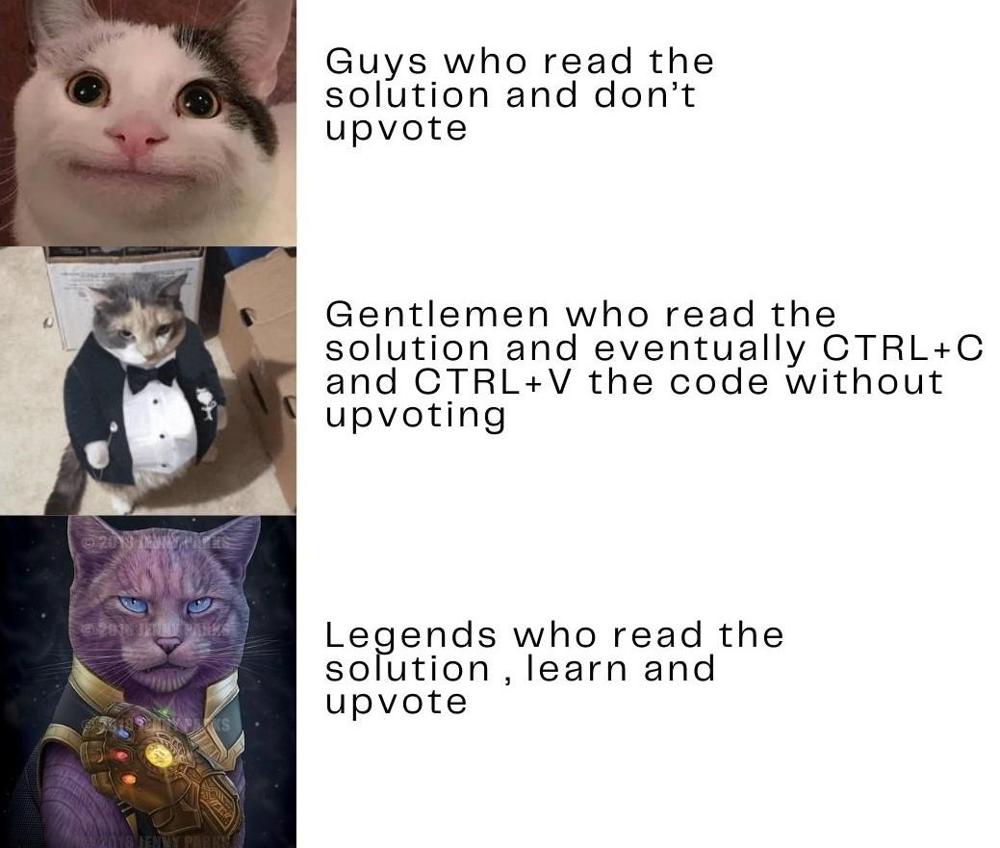
| 10 | 0 | ['Database', 'MySQL', 'Oracle', 'MS SQL Server', 'PostgreSQL'] | 1 |
big-countries | ✅100% Beast Solution || Easy Sql Query | 100-beast-solution-easy-sql-query-by-gou-ngeu | Intuition\nThis SQL query selects the name, population, and area columns from the World table where either the population is greater than or equal to 25,000,000 | gouravsharma2806 | NORMAL | 2024-02-10T06:31:18.087768+00:00 | 2024-02-10T06:31:18.087801+00:00 | 6,986 | false | # Intuition\nThis SQL query selects the name, population, and area columns from the World table where either the population is greater than or equal to 25,000,000 or the area is greater than or equal to 3,000,000.\n\n# Approach\nThe approach is to filter rows from the World table based on the conditions specified in the WHERE clause. We want to retrieve countries or entities with either a large population or a large area.\n\n# Complexity\n- Time Complexity: The time complexity depends on the indexing of the population and area columns in the World table. If properly indexed, the time complexity will be O(log n) where n is the number of rows in the World table. Otherwise, it could be O(n) if a full table scan is required.\n- Space Complexity: The space complexity is O(1) because we are only retrieving a fixed number of columns (name, population, and area) and it doesn\'t depend on the size of the data being queried.\n\n# Code\n```\nselect \nname,population,area\nfrom World\nwhere population>=25000000 or area>=3000000;\n\n``` | 9 | 0 | ['MySQL'] | 0 |
big-countries | Easiest MYSQL Solution with EXPLANATION✅ | easiest-mysql-solution-with-explanation-u8ito | 1. Understanding the Question :\n\nThe question wants us to fetch 3 columns ( name, population, area) from the world table. The condition is to return the rows | aftabalam2209 | NORMAL | 2022-10-28T10:18:43.410491+00:00 | 2022-10-28T10:18:43.410638+00:00 | 4,279 | false | **1. Understanding the Question :**\n\nThe question wants us to fetch 3 columns ( name, population, area) from the world table. The condition is to return the rows where the area is atleast 3000000 (which means - area>=3000000) and the population is atleast 25000000 (i.e population>=25000000 ).\n\n**2. Simply structure the query using SELECT and WHERE keyword.**\n\n```\nSELECT name,population,area FROM world WHERE area>=3000000 OR population>=25000000;\n```\n\n | 9 | 0 | ['MySQL', 'Oracle'] | 0 |
big-countries | ✅3/50 | All SQL50 Explained | 350-all-sql50-explained-by-piotr_maminsk-1zl5 | \nmysql [MySQL]\nSELECT \n name\n ,population\n ,area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000\n;\n\nmssql [MS SQL Server]\nSELECT | Piotr_Maminski | NORMAL | 2024-09-25T20:45:01.288591+00:00 | 2024-10-04T14:24:04.346424+00:00 | 2,431 | false | \n```mysql [MySQL]\nSELECT \n name\n ,population\n ,area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000\n;\n```\n```mssql [MS SQL Server]\nSELECT \n name\n ,population\n ,area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000\n;\n```\n```oraclesql [Oracle]\nSELECT \n name\n ,population\n ,area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000\n;\n```\n```postgresql [PostgreSQL]\nSELECT \n name\n ,population\n ,area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000\n;\n```\n```pythondata [Pandas]\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n return world[\n (world[\'area\'] >= 3_000_000) | (world[\'population\'] >= 25_000_000)\n ][[\'name\', \'population\', \'area\']]\n```\n\n# Explained\n\n- **WHERE area >= 3000000 OR population >= 25000000**\nThe **WHERE** clause is used to filter records based on specified conditions. Here, it filters the rows to include only those where either:\nThe area column has a value greater than or equal to 3,000,000 (indicating the country has a large area), or\nThe population column has a value greater than or equal to 25,000,000 (indicating the country has a large population).\nThe **OR** operator ensures that a row is included if either of the conditions is true.\n# Order of Execution \n\n**FROM** World > **WHERE** area >= 3000000 OR population >= 25000000 > **SELECT** name, population, area\n\n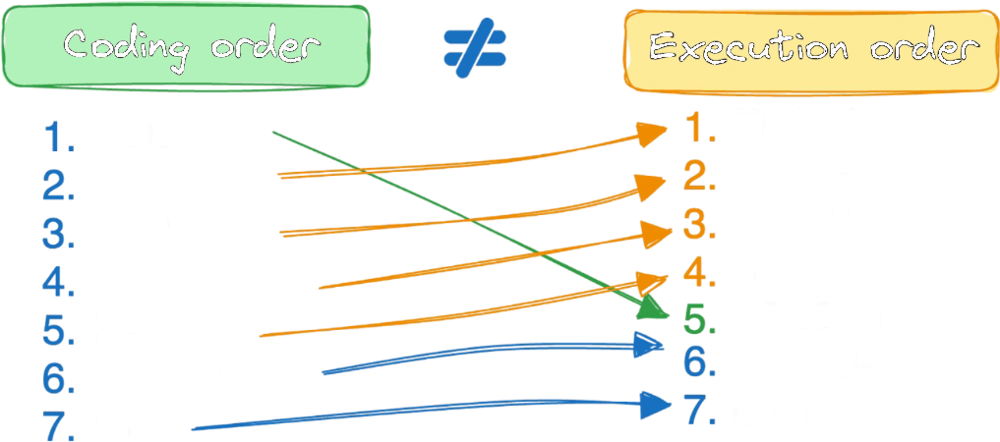\n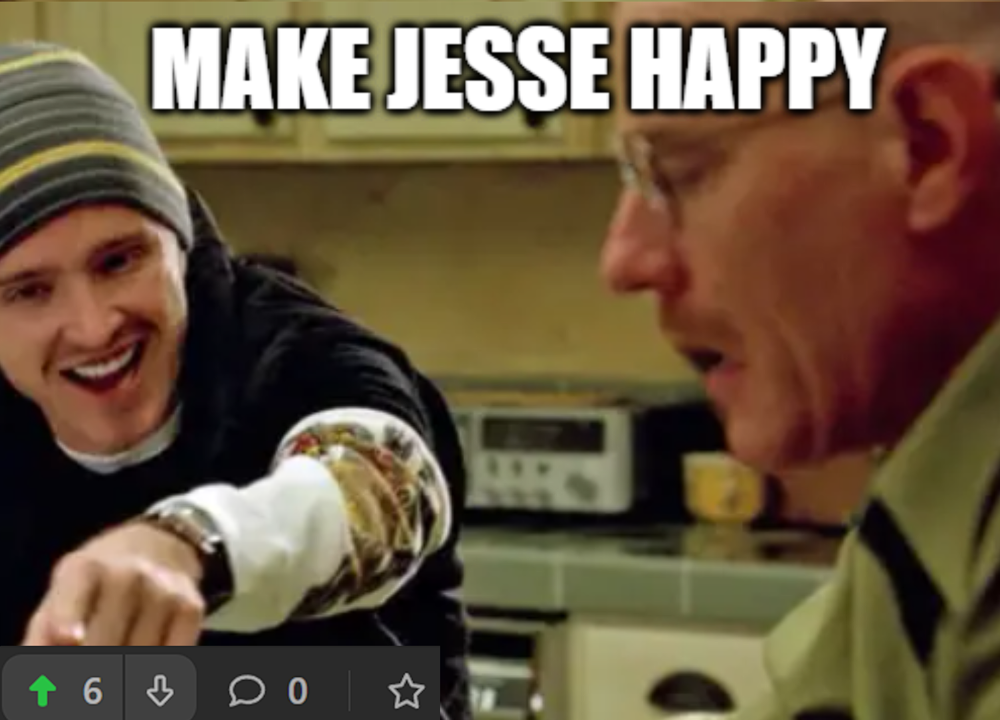\n | 8 | 0 | ['Python', 'Python3', 'MySQL', 'Oracle', 'MS SQL Server', 'PostgreSQL', 'Pandas'] | 0 |
big-countries | 📌 Simple MySql query || use of OR ✅ | simple-mysql-query-use-of-or-by-mayankgu-qc2a | upvote if you like the sol \uD83D\uDC4D\n# Code\n\n# Write your MySQL query statement below\nselect name , population , area from world where area >= 3000000 or | mayankgurjar1570 | NORMAL | 2024-06-03T11:36:49.303950+00:00 | 2024-06-03T11:36:49.303971+00:00 | 5,228 | false | # upvote if you like the sol \uD83D\uDC4D\n# Code\n```\n# Write your MySQL query statement below\nselect name , population , area from world where area >= 3000000 or population >=25000000;\n\n``` | 8 | 0 | ['MySQL'] | 1 |
big-countries | MySql | simple one | mysql-simple-one-by-ahmedna126-prmv | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | ahmedna126 | NORMAL | 2023-04-20T18:49:08.798416+00:00 | 2023-11-07T11:48:44.908188+00:00 | 1,349 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n# Write your MySQL query statement below\nSELECT name,population,area FROM World WHERE area >= 3000000 OR population >= 25000000;\n```\n\n## For additional problem-solving solutions, you can explore my repository on GitHub: [GitHub Repository](https://github.com/ahmedna126/java_leetcode_challenges)\n\n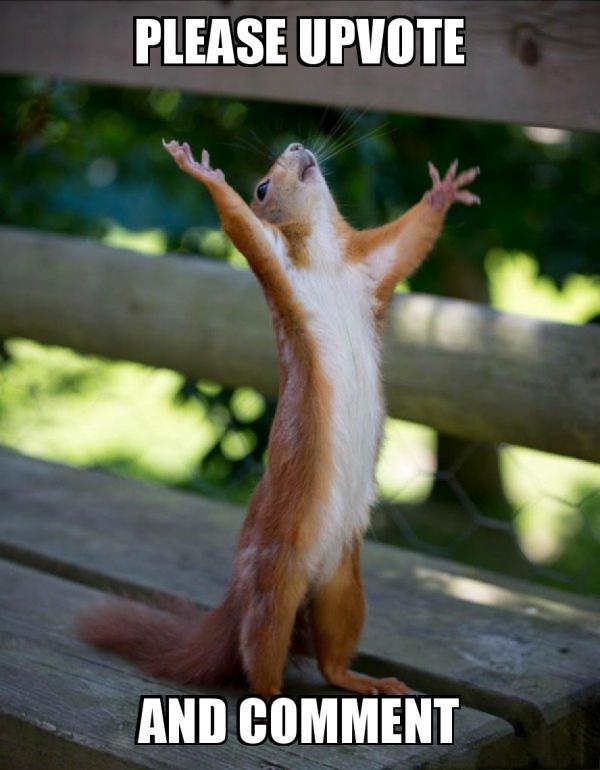\n | 8 | 0 | ['MySQL'] | 0 |
big-countries | SQL easy solution | sql-easy-solution-by-tovam-szbb | \nselect name, population, area \nfrom World\nwhere area > 3000000 or population > 25000000\n\n\nLike it ? please upvote ! | TovAm | NORMAL | 2021-10-24T16:22:42.156986+00:00 | 2021-10-24T16:22:42.157027+00:00 | 1,951 | false | ```\nselect name, population, area \nfrom World\nwhere area > 3000000 or population > 25000000\n```\n\n**Like it ? please upvote !** | 8 | 2 | ['MySQL'] | 1 |
big-countries | ✔✔✔EZ - beats 99%⚡⚡⚡MySQL || MS SQL Server || Oracle || PostgreSQL⚡⚡⚡ | ez-beats-99mysql-ms-sql-server-oracle-po-x8fu | Code\n\nSELECT name, population, area\nFROM World\nWHERE area >= \'3000000\' OR population >= \'25000000\'\n\n\n# for all LeetCode SQL50 solutions... | anish_sule | NORMAL | 2024-02-27T05:31:09.498552+00:00 | 2024-04-25T05:27:34.978028+00:00 | 1,795 | false | # Code\n```\nSELECT name, population, area\nFROM World\nWHERE area >= \'3000000\' OR population >= \'25000000\'\n```\n\n# [for all LeetCode SQL50 solutions...](https://github.com/anish-sule/LeetCode-SQL-50) | 7 | 0 | ['MySQL', 'Oracle', 'MS SQL Server', 'PostgreSQL'] | 2 |
big-countries | Easy solution using Where | easy-solution-using-where-by-swayam248-d5ln | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Swayam248 | NORMAL | 2024-10-01T17:03:33.302976+00:00 | 2024-10-01T17:03:33.303007+00:00 | 6,752 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```mysql []\n# Write your MySQL query statement below\nSELECT name, population, area from World where area >= 3000000 OR population >= 25000000;\n``` | 6 | 0 | ['MySQL'] | 3 |
big-countries | MySQL Clean Solution | mysql-clean-solution-by-shree_govind_jee-abct | Code\n\n# Write your MySQL query statement below\nSELECT name, population, area FROM World\nWHERE population >= 25000000 OR area >= 3000000;\n | Shree_Govind_Jee | NORMAL | 2024-07-03T15:50:29.564134+00:00 | 2024-07-03T15:50:29.564158+00:00 | 4,932 | false | # Code\n```\n# Write your MySQL query statement below\nSELECT name, population, area FROM World\nWHERE population >= 25000000 OR area >= 3000000;\n``` | 6 | 0 | ['Database', 'MySQL'] | 0 |
big-countries | ✅Easiest Basic SQL Solution | 3 Approaches | Beginner Level🏆 | easiest-basic-sql-solution-3-approaches-f7748 | \n# Approach 1\nSELECT \nname, population, area\nFROM World\nHAVING area >= 3000000 OR population >= 25000000;\n\n or\n \nSELECT\nname,population,area\nFROM Wor | dev_yash_ | NORMAL | 2024-02-25T11:45:48.612696+00:00 | 2024-03-17T09:26:00.139555+00:00 | 651 | false | ```\n# Approach 1\nSELECT \nname, population, area\nFROM World\nHAVING area >= 3000000 OR population >= 25000000;\n ```\n or\n ```\nSELECT\nname,population,area\nFROM World\nWHERE area>=3000000 OR population>=25000000;\n```\n\nApproach to achieve the same result is by using a UNION of two queries: one selecting countries based on area criteria and the other based on population criteria. Here\'s how you can do it:\n```\n\n#Approach 2\nSELECT name, population, area\nFROM World\nWHERE area >= 3000000\n\nUNION\n\nSELECT name, population, area\nFROM World\nWHERE population >= 25000000;\n```\n\n\nAnother approach involves using a CASE statement to check each country\'s area and population against the defined thresholds. We can use this to create a new column indicating whether a country is considered big or not based on the given criteria, and then filter out the rows where this column equals 1. Here\'s how you can implement it:\n```\n#Approach 3\nSELECT name, population, area\nFROM (\n SELECT \n name, population, area,\n CASE\n WHEN area >= 3000000 OR population >= 25000000 THEN 1\n ELSE 0\n END AS is_big\n FROM World\n) AS big_countries\nWHERE is_big = 1;\n```\n | 6 | 0 | ['Union Find', 'MySQL', 'MS SQL Server'] | 2 |
big-countries | Simple Two liner SQL code. Very easy to understand!!! | simple-two-liner-sql-code-very-easy-to-u-arb0 | - This SQL query selects the name, population, and area columns from the World table and filters the results to include countries with either a large area (grea | komronabdulloev | NORMAL | 2023-10-10T05:48:03.694359+00:00 | 2023-10-10T05:48:03.694387+00:00 | 783 | false | ##### ***- This SQL query selects the name, population, and area columns from the World table and filters the results to include countries with either a large area (greater than or equal to 3,000,000 square units) or a large population (greater than or equal to 25,000,000 people).***\n\n# Code\nSELECT name, population, area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000;\n``` | 6 | 0 | ['MySQL'] | 1 |
big-countries | Easy Code || MySQL | easy-code-mysql-by-me_avi-3der | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | me_avi | NORMAL | 2023-10-05T18:04:31.704718+00:00 | 2023-10-05T18:04:31.704747+00:00 | 2,949 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n# Write your MySQL query statement below\nselect name,population,area\nfrom World\nwhere area >= 3000000 or population >=25000000\n``` | 6 | 0 | ['MySQL'] | 1 |
big-countries | Beats 91.03% || Easy || Fast || Clean Solution with detailed explanation | beats-9103-easy-fast-clean-solution-with-tc9l | \n# Approach\n1. Data Creation: The code starts by creating a pandas DataFrame world using the provided data dictionary. The DataFrame consists of columns \'nam | rimapanja | NORMAL | 2023-08-01T02:15:14.840718+00:00 | 2023-08-01T02:15:14.840738+00:00 | 4,339 | false | \n# Approach\n1. **Data Creation**: The code starts by creating a pandas DataFrame `world` using the provided data dictionary. The DataFrame consists of columns \'name\', \'continent\', \'area\', \'population\', and \'gdp\', where each row represents information about a country.\n\n2. **Filtering**: The code then filters the DataFrame using boolean indexing. It creates a new DataFrame `big_countries_df`, which contains only the rows that satisfy the specified conditions:\n - Rows with an area greater than or equal to 3,000,000 square kilometers (3 million km\xB2).\n - Rows with a population greater than or equal to 25,000,000 people (25 million).\n\n3. **Column Selection**: After filtering, the code selects only the \'name\', \'population\', and \'area\' columns from the `big_countries_df`. This creates the final result DataFrame `result_df` containing the name, population, and area of the big countries.\n\n4. **Output**: Finally, the code prints the `result_df`, which shows the names, populations, and areas of the countries that meet the criteria for being big.\n\nOverall, the code efficiently uses pandas DataFrame operations to filter and select the relevant data, resulting in a concise and readable solution to find the big countries based on the given conditions.\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- **Time complexity**:\n1. DataFrame Creation: The time complexity of creating the pandas DataFrame `world` from the input data dictionary is O(n), where n is the number of rows in the DataFrame. This is because each row\'s data needs to be processed and inserted into the DataFrame.\n\n2. Filtering: The time complexity of the filtering step using boolean indexing is O(n), where n is the number of rows in the DataFrame. This is because it iterates through each row to check the conditions (area >= 3000000 or population >= 25000000) and keeps the rows that satisfy these conditions.\n\n3. Column Selection: The time complexity of selecting the \'name\', \'population\', and \'area\' columns from the filtered DataFrame is O(1), as it\'s a constant-time operation to extract specific columns from the DataFrame.\n\n**Overall Time Complexity: O(n)**, where n is the number of rows in the DataFrame.\n\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- **Space complexity**:\n1. DataFrame: The space complexity of the `world` DataFrame is O(n), as it stores all the rows of the data.\n\n2. Filtering: The space complexity of the `big_countries_df` DataFrame, which contains the filtered rows, is also O(n) as it keeps all the rows that meet the filtering conditions.\n\n3. Result DataFrame: The space complexity of the `result_df` DataFrame, which contains the \'name\', \'population\', and \'area\' columns of the big countries, is O(m), where m is the number of big countries (the number of rows that meet the filtering conditions).\n\n**Overall Space Complexity: O(n)**, as the space complexity is primarily dominated by the DataFrame storing all the data and the filtered rows.\n\nNote: The space complexity does not consider the space taken by temporary variables or data structures used during the intermediate steps, as they usually have constant space requirements and do not grow with the input size.\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n # Filtering the big countries\n big_countries_df = world[(world[\'area\'] >= 3000000) | (world[\'population\'] >= 25000000)]\n\n # Selecting the desired columns\n result_df = big_countries_df[[\'name\', \'population\', \'area\']]\n\n return result_df\n``` | 6 | 0 | ['Pandas'] | 3 |
big-countries | My Solution | my-solution-by-momo89-u7uf | \nSELECT name, population, area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000\n | momo89 | NORMAL | 2022-09-20T08:08:19.796639+00:00 | 2022-09-22T06:12:11.173276+00:00 | 2,142 | false | ```\nSELECT name, population, area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000\n``` | 6 | 0 | [] | 0 |
big-countries | Day 1 || Pandas || Clean Code || Python | day-1-pandas-clean-code-python-by-nadeem-s76z | \nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n # selecting the required columns\n df = world[[\'name\',\'population\', | nadeem_ansari14 | NORMAL | 2023-08-01T08:26:19.553117+00:00 | 2023-08-01T09:14:54.401746+00:00 | 974 | false | ```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n # selecting the required columns\n df = world[[\'name\',\'population\',\'area\']]\n \n\t# operation to get result\n resdf = df[(df[\'population\']>=25000000) | (df[\'area\']>=3000000)]\n \n return resdf\n``` | 5 | 0 | ['Python3'] | 0 |
big-countries | MySQL || Best Solution ✅ | mysql-best-solution-by-primordial_black-w9ix | Code\n\n# Write your MySQL query statement below\nSELECT name,population,area FROM World\nWHERE area >= 3000000 or population >= 25000000;\n\n> Please Upvote if | Primordial_Black | NORMAL | 2023-01-31T11:23:16.452485+00:00 | 2023-01-31T11:23:49.272224+00:00 | 3,745 | false | # Code\n```\n# Write your MySQL query statement below\nSELECT name,population,area FROM World\nWHERE area >= 3000000 or population >= 25000000;\n```\n> Please Upvote if it was Helpful. | 5 | 0 | ['MySQL'] | 0 |
big-countries | ✅ MySQL || Straightforward && SIMPLE SQL query | mysql-straightforward-simple-sql-query-b-dlad | \n\n\n\tSELECT name, population, area FROM world WHERE (population >= 25000000 OR area >= 3000000 ); | abhinav_0107 | NORMAL | 2023-01-16T18:42:02.740354+00:00 | 2023-01-16T18:42:02.740390+00:00 | 3,842 | false | 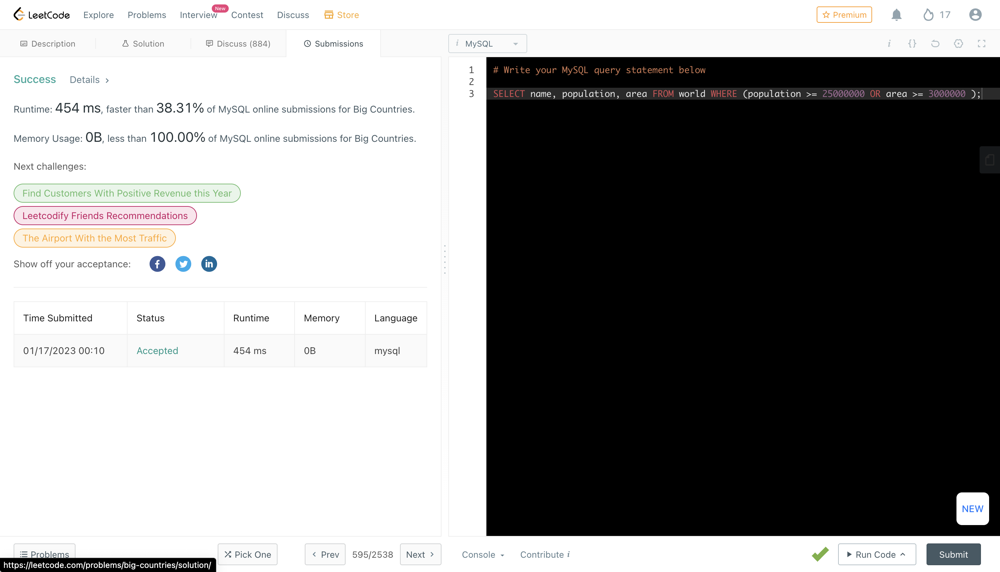\n\n\n\tSELECT name, population, area FROM world WHERE (population >= 25000000 OR area >= 3000000 ); | 5 | 0 | ['MySQL'] | 0 |
big-countries | MySql | simple one | mysql-simple-one-by-venkat089-ekwn | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Venkat089 | NORMAL | 2022-12-29T06:02:53.209936+00:00 | 2022-12-29T06:02:53.209991+00:00 | 4,005 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n# Write your MySQL query statement below\nselect name,population,area from World\nwhere area >=3000000 or population >= 25000000;\n``` | 5 | 0 | ['MySQL'] | 1 |
big-countries | ✔️ easy mySQL solution | easy-mysql-solution-by-coding_menance-l7xr | Remember, mySQL is case-insensitive. Typing the table names in lower case or all upper case makes on difference. Same goes for data contents within the table.\n | coding_menance | NORMAL | 2022-10-24T18:48:03.851552+00:00 | 2022-10-27T08:09:47.637742+00:00 | 1,755 | false | Remember, mySQL is case-insensitive. Typing the table names in lower case or all upper case makes on difference. Same goes for data contents within the table.\n\n```\nSELECT name, population, area FROM World WHERE area>=3000000 OR population>=25000000;\n``` | 5 | 0 | ['MySQL'] | 0 |
big-countries | [MY SQL] Easy and Faster than 89% | my-sql-easy-and-faster-than-89-by-overec-mjao | ```\nselect name,population,area\nfrom World\nwhere (World.area >=3000000) or (World.population >=25000000) ; | overeckon | NORMAL | 2022-08-12T09:55:50.862773+00:00 | 2022-08-12T09:55:50.862815+00:00 | 2,650 | false | ```\nselect name,population,area\nfrom World\nwhere (World.area >=3000000) or (World.population >=25000000) ; | 5 | 0 | ['MySQL'] | 1 |
big-countries | Easy to Understand | SQL | Union | OR | easy-to-understand-sql-union-or-by-sai72-w29l | \n#OR\nselect \nname, population, area \nfrom world \nwhere area>=3000000 \nor population>=25000000;\n\n\n#UNION\nselect \nname, population, area \nfrom world\n | sai721 | NORMAL | 2022-04-17T05:44:49.571818+00:00 | 2022-04-17T05:44:49.571859+00:00 | 578 | false | ```\n#OR\nselect \nname, population, area \nfrom world \nwhere area>=3000000 \nor population>=25000000;\n\n\n#UNION\nselect \nname, population, area \nfrom world\nwhere area>=3000000\n\nunion\n\nselect \nname, population, area \nfrom world \nwhere \npopulation>=25000000;\n```\n\n**UNION** is faster than **OR**\n\n\nIf you have any **doubts**, feel **free to ask**...\nIf you understand the **concept**. Don\'t Forget to **upvote**\n\n | 5 | 0 | ['Union Find', 'MySQL'] | 1 |
big-countries | Very Fast Solution using WITH clause:Runtime: 492 ms, faster than 99.03% | very-fast-solution-using-with-clauserunt-eqbf | \nwith \n a AS\n (select name, population, area\n from world\n where area > 3000000),\n b AS\n (select name, population, area\n | apewithakeyboard | NORMAL | 2020-01-31T05:41:01.590126+00:00 | 2020-01-31T05:43:24.756573+00:00 | 1,280 | false | ```\nwith \n a AS\n (select name, population, area\n from world\n where area > 3000000),\n b AS\n (select name, population, area\n from world\n where population > 25000000)\nselect * from a\nunion\nselect * from b\n;\n```\n\nSolution here will compute the aggregation first, give it a variable and allow us to reference it later in the query(could be multiple times too). The result from two querys are materialized(this boosts performence), oracle database optimizes the query by treating the query name as either an inline view or as a temporary table.\n | 5 | 0 | ['Oracle'] | 2 |
big-countries | Help! Same code getting run time error when using Microsoft SQL Server | help-same-code-getting-run-time-error-wh-fbu3 | Same code as below works in MySQL but returns run-time error using SQL Server. What have I missed? Anybody knows? Thanks!\n\n\nSELECT name, population, area\nFR | __nick__ | NORMAL | 2019-03-04T00:52:35.853395+00:00 | 2019-03-04T00:52:35.853438+00:00 | 2,473 | false | Same code as below works in MySQL but returns run-time error using SQL Server. What have I missed? Anybody knows? Thanks!\n\n```\nSELECT name, population, area\nFROM World\nWHERE area>3000000 OR population>25000000;\n```\n\nError message:\n```\nRuntime Error Message:\nsql: insert into World (name, continent, area, population, gdp) values (?, ?, ?, ?, ?), values: [(\'Afghanistan\', \'Asia\', 652230, 25500100, 20343000000), (\'Albania\', \'Europe\', 28748, 2831741, 12960000000), (\'Algeria\', \'Africa\', 2381741, 37100000, 188681000000), (\'Andorra\', \'Europe\', 468, 78115, 3712000000), (\'Angola\', \'Africa\', 1246700, 20609294, 100990000000)]\n``` | 5 | 0 | [] | 16 |
big-countries | ✅💯🔥✔️Simple My SQL Code || 🔥✔️✅💯 | simple-my-sql-code-by-patilprajakta-ul4o | We need to find the name, population, and area of the big countries. A "big" country is defined as one with either an area greater than or equal to 3,000,000 or | patilprajakta | NORMAL | 2025-01-12T14:01:12.926686+00:00 | 2025-01-12T14:01:12.926686+00:00 | 1,601 | false | We need to find the name, population, and area of the big countries. A **"big"** country is defined as one with either an area greater than or equal to 3,000,000 or a population greater than or equal to 25,000,000.
To do this, we first select the columns name, population, and area from the table.
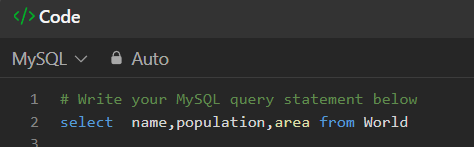
Then, we add a WHERE condition to filter the results, using OR to check if the area is greater than or equal to 3,000,000 or the population is greater than or equal to 25,000,000.exity here, e.g. $$O(n)$$ -->
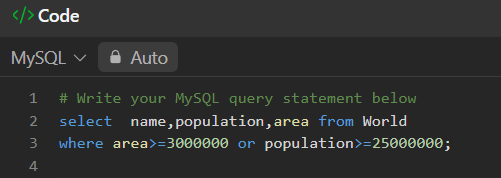
# Code
select name,population,area from World
where area>=3000000 or population>=25000000;
| 4 | 0 | ['MySQL'] | 0 |
big-countries | 1 line solution | 1-line-solution-by-victorhpxavier-aviq | \n# Code\n\n# Write your MySQL query statement below\nSELECT name, population, area FROM World WHERE area >= 3000000 or population >= 25000000;\n | VictorhpXavier | NORMAL | 2024-07-30T20:32:46.530916+00:00 | 2024-07-30T20:32:46.530938+00:00 | 446 | false | \n# Code\n```\n# Write your MySQL query statement below\nSELECT name, population, area FROM World WHERE area >= 3000000 or population >= 25000000;\n``` | 4 | 0 | ['MySQL'] | 0 |
big-countries | Beats 94% | Python pandas | beats-94-python-pandas-by-u23cs159-0amu | \n\n\n\n# Code\n\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n return world[(world.area >= 3000000) | (world.population > | u23cs159 | NORMAL | 2024-06-12T17:22:49.487516+00:00 | 2024-06-12T17:22:49.487539+00:00 | 3,042 | false | 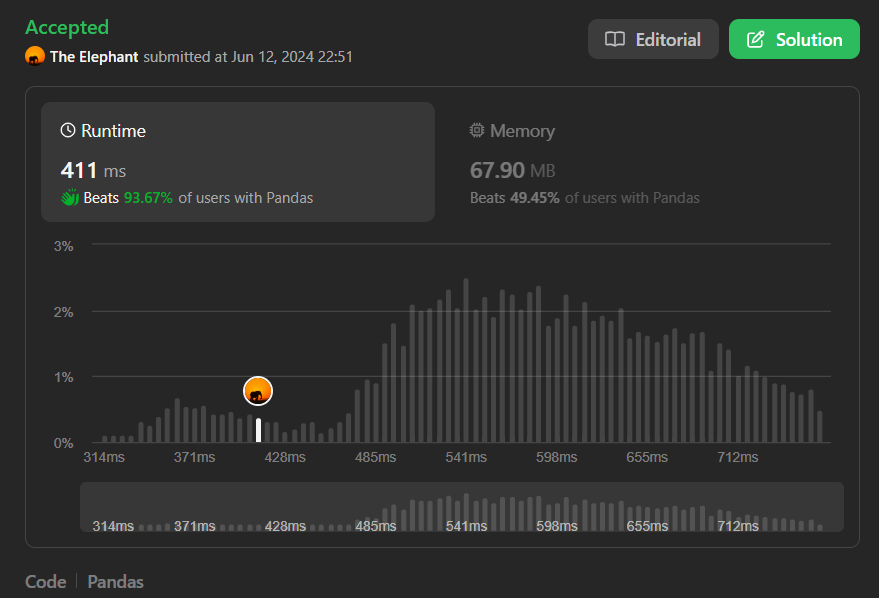\n\n\n\n# Code\n```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n return world[(world.area >= 3000000) | (world.population >= 25000000)][[\'name\',\'population\',\'area\']]\n``` | 4 | 0 | ['Pandas'] | 1 |
big-countries | 🔥 Clean & Easy || Oracle 🔥 | clean-easy-oracle-by-someonescoding-krdw | Code\n\nSELECT name, population, area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000\n\n\n | SomeonesCoding | NORMAL | 2024-04-11T07:05:05.112190+00:00 | 2024-04-11T07:05:05.112234+00:00 | 2,355 | false | # Code\n```\nSELECT name, population, area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000\n```\n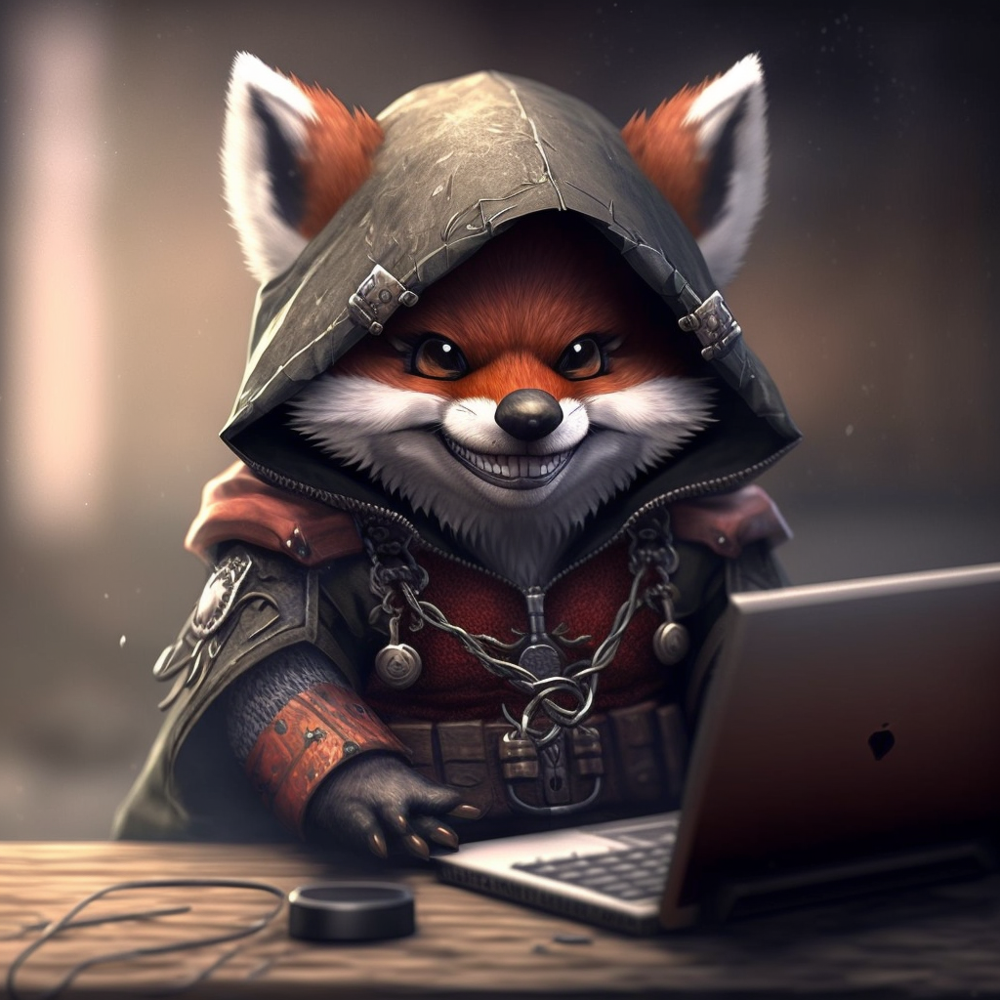\n | 4 | 0 | ['Oracle'] | 0 |
big-countries | Easy SQL Quary | easy-sql-quary-by-deepakumar-developer-oycc | \n\n# Quary\n\nselect name,population,area\nfrom World \nwhere population >= 25000000\nor area >= 3000000\n | deepakumar-developer | NORMAL | 2023-12-27T15:19:31.647417+00:00 | 2023-12-27T15:19:31.647439+00:00 | 1,809 | false | \n\n# Quary\n```\nselect name,population,area\nfrom World \nwhere population >= 25000000\nor area >= 3000000\n``` | 4 | 0 | ['MySQL'] | 2 |
big-countries | Filtering and applying conditional selection | filtering-and-applying-conditional-selec-yoap | Intuition\n Describe your first thoughts on how to solve this problem. \n We need to select certain entities which meet our condition, then we should select the | abdelazizSalah | NORMAL | 2023-09-15T18:01:00.696095+00:00 | 2023-09-15T18:01:00.696116+00:00 | 152 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n* We need to select certain entities which meet our condition, then we should select the name, population, and area columns only. \n* This will be done using conditional selection\n* Then applying filtering function\n# Approach\n<!-- Describe your approach to solving the problem. -->\n* Apply conditionl selection by selecting elements which are \n 1. (world.population >= 25000000)\n 2. (world.area >= 3000000)\n* After that, use the filter function, to select the needed columns only\n * .filter(items=[\'name\', \'population\', \'area\'])\n\n# Code\n```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n # world = world[(world.population >= 25000000) | (world.area >= 3000000)]\n\n # # Drop all columns except \'name\',\'population\',\'area\'\n # columns_to_keep = [\'name\',\'population\',\'area\',]\n\n # world = world[columns_to_keep]\n # return world\n return world[(world.population >= 25000000) | (world.area >= 3000000)].filter(items=[\'name\', \'population\', \'area\'])\n\n``` | 4 | 0 | ['Pandas'] | 0 |
big-countries | One Liner Solution - Optimum Solution | one-liner-solution-optimum-solution-by-d-8ogk | Please Upvote my solution, if you find it helpful ;)\n\n# Intuition\nThe problem asks us to find "big" countries based on certain conditions related to their ar | deepankyadav | NORMAL | 2023-05-30T16:25:15.275780+00:00 | 2023-05-30T20:30:53.475361+00:00 | 395 | false | ## ***Please Upvote my solution, if you find it helpful ;)***\n\n# Intuition\nThe problem asks us to find "big" countries based on certain conditions related to their area and population. We need to retrieve the name, population, and area of countries that have either an area greater than or equal to 3,000,000 or a population greater than or equal to 25,000,000. To do this, we will query the **\'World\'** table and apply the necessary filtering conditions.\n\n# Approach\nHere is a step-by-step explanation of your approach:\n\n1. We start by selecting the name, population, and area columns from the World table. This allows us to retrieve the necessary information about the countries.\n1. We use the WHERE clause to apply the filtering conditions. In this case, the conditions state that we want to include countries with an area greater than or equal to 3,000,000 or a population greater than or equal to 25,000,000.\n1. Finally, we retrieve the name, population, and area of countries that satisfy the filtering conditions.\n\n# Complexity\n- Time complexity:\nThe time complexity of your solution depends on the number of countries in the World table. We need to scan through each row in the table to retrieve the required information. Therefore, the time complexity can be considered $$O(N)$$, where N is the number of countries.\n\n- Space complexity:\nThe space complexity of your solution depends on the number of "big" countries that satisfy the given conditions. Since we are selecting the name, population, and area columns, the space required to store the result set will depend on the number of countries that meet the criteria. Let\'s assume this is M countries. Therefore, the space complexity can be considered $$O(M)$$.\n\n# Code\n```\n# Write your MySQL query statement below\nselect name, population, area from World where area>= 3000000 or population>=25000000\n```\n***Please Upvote my solution, if you find it helpful ;)***\n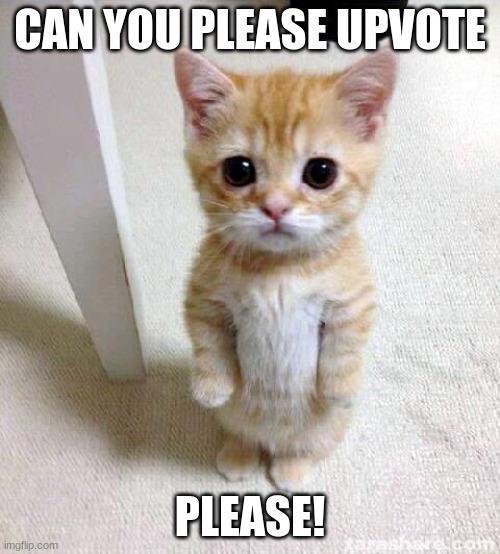\n\n | 4 | 0 | ['Database', 'MySQL'] | 0 |
big-countries | 595: Solution with step by step explanation | 595-solution-with-step-by-step-explanati-yjfs | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Marlen09 | NORMAL | 2023-03-17T04:54:22.780058+00:00 | 2023-03-17T04:54:22.780107+00:00 | 3,148 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nSELECT name, population, area \nFROM World \nWHERE area >= 3000000 OR population >= 25000000;\n\n``` | 4 | 0 | ['Database', 'MySQL'] | 0 |
big-countries | MySQL Solution | mysql-solution-by-pranto1209-nsjj | Code\n\n# Write your MySQL query statement below\nselect name, population, area from World \nwhere area >= 3000000 or population >= 25000000;\n | pranto1209 | NORMAL | 2023-03-07T17:29:03.032597+00:00 | 2023-03-13T16:01:15.206501+00:00 | 5,541 | false | # Code\n```\n# Write your MySQL query statement below\nselect name, population, area from World \nwhere area >= 3000000 or population >= 25000000;\n``` | 4 | 0 | ['MySQL'] | 0 |
big-countries | Easy-peezy SQL Query Solution | easy-peezy-sql-query-solution-by-anshika-oopa | 1) Simple Method\n\nselect name, population, area from World where area>=3000000 or population>=25000000\n\n\n2) Using Union\n```\nselect name, population, area | anshika_yadav | NORMAL | 2022-06-05T06:33:20.469425+00:00 | 2022-06-05T06:36:13.513019+00:00 | 368 | false | 1) Simple Method\n```\nselect name, population, area from World where area>=3000000 or population>=25000000\n```\n\n2) Using Union\n```\nselect name, population, area \nfrom World \nwhere area >= 3000000\n\nunion\n\nselect name, population, area \nfrom World \nwhere population >= 25000000\n | 4 | 0 | ['MySQL'] | 0 |
big-countries | SQL easy-peasy | sql-easy-peasy-by-yehudisk-77jv | \nSELECT name, population, area\nFROM World\nWHERE area > 3000000 or population > 25000000\n | yehudisk | NORMAL | 2020-08-20T14:14:29.893271+00:00 | 2020-08-20T14:14:29.893324+00:00 | 697 | false | ```\nSELECT name, population, area\nFROM World\nWHERE area > 3000000 or population > 25000000\n``` | 4 | 0 | [] | 1 |
big-countries | Easy and Simple Solution | Beginner-friendly🚀 | easy-and-simple-solution-beginner-friend-vc6h | SQL QueryIf you found this solution helpful, please give it an upvote! 🌟✨ It really motivates me to share more solutions like this. 😊👍
Happy coding! 💻🐱🏍
Intuit | Yadav_Akash_ | NORMAL | 2025-01-30T18:19:17.387984+00:00 | 2025-01-30T18:19:17.387984+00:00 | 634 | false | ## **SQL Query**
```sql
SELECT name, population, area
FROM World
WHERE area >= 3000000 OR population >= 25000000;
```
**If you found this solution helpful, please give it an upvote! 🌟✨ It really motivates me to share more solutions like this. 😊👍
Happy coding! 💻🐱🏍**
<img src="https://assets.leetcode.com/users/images/6e8c623a-1111-4d21-9a5b-54e00e7feabb_1738093236.165277.jpeg" width=250px>
## **Intuition**
We need to find countries that are considered **big**, which means they must satisfy at least one of these conditions:
1. **Area is at least 3,000,000 km²** (`area >= 3000000`).
2. **Population is at least 25,000,000** (`population >= 25000000`).
Since a country only needs to meet **one** of these conditions, we use the **OR** operator in the `WHERE` clause.
---
## **Approach**
1. **Select the required columns**: `name`, `population`, and `area`.
2. **Filter big countries** using the `WHERE` clause:
- `area >= 3000000` OR
- `population >= 25000000`.
3. **Return the result in any order** as sorting is not required.
---
## **Complexity Analysis**
- **Time Complexity**: **O(N)** → The query scans all `N` rows and applies the `WHERE` conditions.
- **Space Complexity**: **O(1)** → The query does not use extra space beyond the result set.
| 3 | 0 | ['MySQL'] | 0 |
big-countries | Easy solution on select query using MySQL | easy-solution-on-select-query-using-mysq-na74 | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Siddarth9911 | NORMAL | 2024-12-19T07:30:29.346844+00:00 | 2024-12-19T07:30:29.346844+00:00 | 1,189 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```mysql []
SELECT name,population,area FROM World where population>=25000000 or area>=3000000;
``` | 3 | 0 | ['MySQL'] | 0 |
big-countries | simple and easy solution || MySQL | simple-and-easy-solution-mysql-by-shishi-tw1t | \n# if it\'s help, please up \u2B06 vote! \u2764\uFE0F\n\n###### Let\'s Connect on LinkedIn: www.linkedin.com/in/shishirrsiam\n###### Let\'s Connect on Facebook | shishirRsiam | NORMAL | 2024-09-27T14:37:27.529667+00:00 | 2024-09-27T14:37:27.529709+00:00 | 2,873 | false | \n# if it\'s help, please up \u2B06 vote! \u2764\uFE0F\n\n###### Let\'s Connect on LinkedIn: www.linkedin.com/in/shishirrsiam\n###### Let\'s Connect on Facebook: www.fb.com/shishirrsiam\n\n# Code\n```mysql []\nselect name, population, area from World\nwhere population >= 25000000 or area >= 3000000;\n``` | 3 | 0 | ['Database', 'MySQL'] | 6 |
big-countries | Big Countries | big-countries-by-tejdekiwadiya-81ba | SQL Query\n\nsql\nSELECT name, population, area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000;\n\n\n# Explanation\n\nThis SQL query is designed t | tejdekiwadiya | NORMAL | 2024-07-03T18:41:14.582442+00:00 | 2024-07-03T18:41:14.582473+00:00 | 973 | false | # SQL Query\n\n```sql\nSELECT name, population, area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000;\n```\n\n# Explanation\n\nThis SQL query is designed to retrieve specific columns from a table named `World`. Let\'s break down each part:\n\n1. **SELECT Clause**:\n - `SELECT name, population, area`: This specifies the columns to be returned in the query result. It selects the `name`, `population`, and `area` columns from the `World` table.\n\n2. **FROM Clause**:\n - `FROM World`: This specifies the table from which to retrieve the data. The data is being selected from a table named `World`.\n\n3. **WHERE Clause**:\n - `WHERE area >= 3000000 OR population >= 25000000`: This is the condition that filters the rows to be returned.\n - `area >= 3000000`: This condition checks if the area of a country is greater than or equal to 3,000,000 square kilometers.\n - `OR population >= 25000000`: This condition checks if the population of a country is greater than or equal to 25,000,000 people.\n - The `OR` operator means that rows that meet either one of these conditions will be included in the result set.\n\n# Query Result\nThe query returns the `name`, `population`, and `area` of countries that either have an area of at least 3,000,000 square kilometers or a population of at least 25,000,000 people.\n\nThis query can be used to find large or highly populated countries based on the criteria defined in the `WHERE` clause. The use of the `OR` operator ensures that countries meeting either of the conditions will be included in the results, making it possible to identify significant countries based on either their geographical size or their population. | 3 | 0 | ['Database', 'MySQL'] | 0 |
big-countries | 100 % FAST && EASY SQL Query || Well - Explained || Beats 99.9 % | 100-fast-easy-sql-query-well-explained-b-dlru | Intuition\n Describe your first thoughts on how to solve this problem. \nSELECT --> for show the table...\nname, population, area --> column names...\nFROM --> | ganpatinath07 | NORMAL | 2024-01-27T14:39:42.739589+00:00 | 2024-01-27T14:39:42.739607+00:00 | 2,167 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nSELECT --> for show the table...\nname, population, area --> column names...\nFROM --> for association...\nWorld --> Table name...\nWHERE --> Clause...\narea >= 3000000 --> Condition 01...\nOR --> Connector...\npopulation >= 25000000 --> Condition 02...\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nUsing Fundamentals of SQL...\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nSELECT name, population, area FROM World\nWHERE area >= 3000000 OR population >= 25000000;\n``` | 3 | 0 | ['Database', 'MySQL'] | 0 |
big-countries | Simple Two liner pandas code. | simple-two-liner-pandas-code-by-ankita29-45n1 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\nUse boolean indexing to filter the rows where either the "area" is greate | Ankita2905 | NORMAL | 2023-11-21T09:20:01.388935+00:00 | 2023-11-21T09:20:01.388963+00:00 | 3,157 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nUse boolean indexing to filter the rows where either the "area" is greater than or equal to 3 million or the "population" is greater than or equal to 25 million.\nSelect only the columns "name," "population," and "area" from the filtered DataFrame.\nReturn the resulting DataFrame containing the name, population, and area of the big countries.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n big_countries_df = world[(world[\'area\'] >= 3000000) | (world[\'population\'] >= 25000000)]\n return big_countries_df[[\'name\', \'population\', \'area\']] \n``` | 3 | 0 | ['Pandas'] | 1 |
big-countries | Find Big Countries using MySQL | find-big-countries-using-mysql-by-samabd-zj24 | Intuition\n Describe your first thoughts on how to solve this problem. \nWe need to find countries that meet the specified conditions.\n\n# Approach\n Describe | samabdullaev | NORMAL | 2023-10-13T21:35:00.158868+00:00 | 2023-10-13T21:35:00.158885+00:00 | 956 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe need to find countries that meet the specified conditions.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n1. Select specific columns from the table.\n\n > `SELECT` \u2192 This command retrieves data from a database\n\n2. Check if they meet the given conditions.\n\n > `WHERE` \u2192 This command filters a result set to include only records that fulfill a specified condition\n\n > `OR` \u2192 This operator displays a record if any of the conditions separated by it are `TRUE`\n\n > `>=` \u2192 This operator compares if one value is greater than or equal to another value\n\n# Complexity\n- Time complexity: $O(n)$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n`n` is the number of rows in the table. This is because the query examines each row in the table once to check the conditions.\n\n# Code\n```\nSELECT name, population, area\nFROM World\nWHERE area >= 3000000 OR population >= 25000000\n``` | 3 | 0 | ['MySQL'] | 0 |
big-countries | Python/Pandas One Liner Solution, Common Error addressed | pythonpandas-one-liner-solution-common-e-k5jy | Intuition\n Describe your first thoughts on how to solve this problem. \nA mistake that might be hard to debug for beginners is that the \'or\' and \'and\' Pyth | sonalibasu24 | NORMAL | 2023-08-06T17:42:16.871923+00:00 | 2023-08-06T17:42:58.967961+00:00 | 1,354 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nA mistake that might be hard to debug for beginners is that the \'or\' and \'and\' Python statements require truth-values, resulting in the following error:\n```\nValueError: The truth value of a Series is ambiguous.\n Use a.empty, a.bool(), a.item(), a.any() or a.all().\n```\n\nThis happens because for pandas, these are considered ambiguous\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nUse "bitwise" | (or) or & (and) operations. \nThese are overriden for the dataframe structures and allow us to perform the SQL equivalent of and/or in a where clause.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n) - Checks through all rows to validate specified conditions.\n\n\n# Code\n```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n return world[(world.population >= 25000000) | (world.area >= 3000000)].filter(items=[\'name\', \'population\', \'area\'])\n``` | 3 | 0 | ['Database', 'Python3', 'Pandas'] | 0 |
big-countries | SIMPLE 2 LINE SOLUTION|| ❤️❤️ | simple-2-line-solution-by-khushantgola-4fnq | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \nIt is very simple just | KhushantGola | NORMAL | 2023-08-03T18:52:21.927611+00:00 | 2023-08-03T18:52:21.927642+00:00 | 190 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n*It is very simple just create a DataFrame \n*Put the condition into the DataFrame\n*Return the DataFrame\n(You can also store the required DataFrame into another DataFrame)\n\n# Code\n```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n df=world[(world[\'area\']>=3000000)| (world[\'population\']>=25000000)]\n return df[[\'name\',\'population\',\'area\']]\n\n# if want to store values into another dataframe just use thiS approach---->\n\n\ndf=world[(world[\'area\']>=3000000)| (world[\'population\']>=25000000)]\nresult=df[[\'name\',\'population\',\'area\']]\nreturn result\n\n\n\n``` | 3 | 0 | ['Pandas'] | 0 |
big-countries | Python easy solutions | python-easy-solutions-by-purvi85-0u0t | Intuition\nThe problem requires finding and displaying information about countries that are considered "big" based on specific criteria. The two criteria for a | Purvi85 | NORMAL | 2023-08-02T05:51:20.755026+00:00 | 2023-08-02T05:51:20.755057+00:00 | 644 | false | # Intuition\nThe problem requires finding and displaying information about countries that are considered "big" based on specific criteria. The two criteria for a country to be considered big are:\n\n* Area >= 3000000 (3 million square kilometers)\n* Population >= 25000000 (25 million people)\n\nThe task is to filter out countries from the given data that meet either of these criteria and then present their names, populations, and areas.\n\n# Approach\n(world[\'area\'] >= 3000000) and (world[\'area\'] >= 3000000)\nThese are the two conditons we are using an return with specific columns.\n\n# Code\n```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n big_countries_df = world[(world[\'area\'] >= 3000000) | (world[\'population\'] >= 25000000)]\n result = big_countries_df[[\'name\', \'population\', \'area\']]\n return result\n``` | 3 | 0 | ['Pandas'] | 0 |
big-countries | 1-line solution | 1-line-solution-by-ost_k-con5 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Ost_K | NORMAL | 2023-08-01T15:21:58.307671+00:00 | 2023-08-01T15:21:58.307701+00:00 | 608 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n return world[(world[\'area\'] >= 3_000_000) | ( world[\'population\'] >= 25_000_000)][[\'name\', \'population\', \'area\']]\n``` | 3 | 0 | ['Pandas'] | 0 |
big-countries | simple solution | simple-solution-by-amevide-5qzn | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | amevide | NORMAL | 2023-08-01T13:42:42.537706+00:00 | 2023-08-01T13:42:42.537735+00:00 | 1,831 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport pandas as pd\n\ndef big_countries(world: pd.DataFrame) -> pd.DataFrame:\n world = world[(world.area >= 3000000) | (world.population >= 25000000)]\n return world[[\'name\', \'population\', \'area\']]\n``` | 3 | 0 | ['Pandas'] | 1 |
big-countries | Easy Mysql Solution | easy-mysql-solution-by-2005115-aomv | \n\n# Code\n\n# Write your MySQL query statement below\nSELECT name , population ,area from World where area >=3000000 OR population >=25000000;\n | 2005115 | NORMAL | 2023-07-16T15:51:19.984039+00:00 | 2023-07-16T15:51:19.984068+00:00 | 2,409 | false | \n\n# Code\n```\n# Write your MySQL query statement below\nSELECT name , population ,area from World where area >=3000000 OR population >=25000000;\n``` | 3 | 0 | ['MySQL'] | 1 |
big-countries | sql || simple Straight forward approach | sql-simple-straight-forward-approach-by-znu9s | \n# Write your MySQL query statement below\n\nselect name, population, area from World\nwhere area >= 3000000 or population >= 25000000;\n\n\n\n\nplease upvote! | haneelkumar | NORMAL | 2023-07-01T13:15:29.198684+00:00 | 2023-07-01T13:15:29.198703+00:00 | 345 | false | ```\n# Write your MySQL query statement below\n\nselect name, population, area from World\nwhere area >= 3000000 or population >= 25000000;\n```\n\n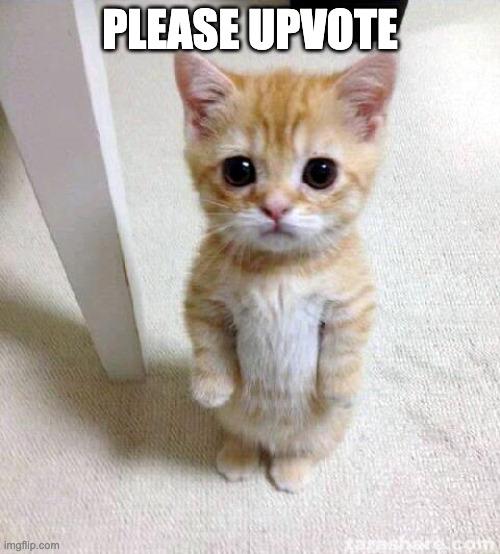\n\n**please upvote!! if you like.**\ncomment below\uD83D\uDC47 | 3 | 0 | ['MySQL'] | 1 |
big-countries | EASILY UNDERSTANDABLE SOLUTION | easily-understandable-solution-by-himans-d9nc | \nSelect name,population,area from world \nwhere area >= 3000000 or population >=25000000\n``` | himanshu__mehra__ | NORMAL | 2023-06-28T22:32:32.303025+00:00 | 2023-06-28T22:32:32.303052+00:00 | 4,046 | false | \nSelect name,population,area from world \nwhere area >= 3000000 or population >=25000000\n``` | 3 | 0 | ['MySQL'] | 3 |
big-countries | MySQL Solution for Big Countries Problem | mysql-solution-for-big-countries-problem-vsqg | Intuition\n Describe your first thoughts on how to solve this problem. \nThe given query selects the name, population, and area of countries from the "World" ta | Aman_Raj_Sinha | NORMAL | 2023-05-16T03:59:55.955947+00:00 | 2023-05-16T03:59:55.955980+00:00 | 4,101 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe given query selects the name, population, and area of countries from the "World" table where either the area is greater than or equal to 3,000,000 or the population is greater than or equal to 25,000,000.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. It selects the name, population, and area columns from the "World" table.\n2. The query applies the filter condition area >= 3000000 or population >= 25000000 to select records where either the area is greater than or equal to 3,000,000 or the population is greater than or equal to 25,000,000.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity of this query depends on the size of the "World" table and the efficiency of indexing on the area and population columns. The query involves scanning the entire table and applying the filter condition, which has a time complexity of O(N), where N is the number of rows in the table. The performance can be further improved if there are indexes on the area and population columns, as it allows for faster filtering.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity of this query is determined by the memory required to process the query and store the result set. The space complexity is proportional to the size of the result set, which depends on the number of countries where either the area is greater than or equal to 3,000,000 or the population is greater than or equal to 25,000,000. If the result set is large, it may require additional memory resources to store and return the data. However, if the result set is small compared to the total table size, the impact on space complexity is minimal.\n\n# Code\n```\n# Write your MySQL query statement below\nselect name, population, area from World \nwhere area >= 3000000 or population >= 25000000\n``` | 3 | 0 | ['MySQL'] | 0 |
big-countries | EASY SOLUTION | easy-solution-by-sarah-2002-sw3m | \n\n# Code\n\n# Write your MySQL query statement below\nSelect name,population,area from world \nwhere area >= 3000000 or population >=25000000\n | Sarah-2002 | NORMAL | 2023-05-05T10:35:30.329880+00:00 | 2023-05-05T10:35:30.329907+00:00 | 8,791 | false | \n\n# Code\n```\n# Write your MySQL query statement below\nSelect name,population,area from world \nwhere area >= 3000000 or population >=25000000\n``` | 3 | 0 | ['MySQL'] | 0 |
big-countries | ✔ Beats 99% || Easy SQL Solution | beats-99-easy-sql-solution-by-gourav_sin-54lg | \n\n# Complexity\n- Time complexity:\nBeats 99%\n\n# Code\n\n# Write your MySQL query statement below\n\nSELECT name,population,area FROM WORLD WHERE population | Gourav_Sinha | NORMAL | 2023-04-26T20:52:42.603565+00:00 | 2023-04-26T20:53:37.072592+00:00 | 2,177 | false | \n\n# Complexity\n- Time complexity:\nBeats 99%\n\n# Code\n```\n# Write your MySQL query statement below\n\nSELECT name,population,area FROM WORLD WHERE population >= 25000000 or area >= 3000000\n``` | 3 | 0 | ['MySQL'] | 0 |
big-countries | SQL Server CLEAN & EASY | sql-server-clean-easy-by-rhazem13-rz71 | \n/* Write your T-SQL query statement below */\nSELECT name, population, area\nFROM World\nWHERE area>=3000000 OR population >= 25000000\n | rhazem13 | NORMAL | 2023-03-08T15:37:25.752933+00:00 | 2023-03-08T15:37:25.752978+00:00 | 881 | false | ```\n/* Write your T-SQL query statement below */\nSELECT name, population, area\nFROM World\nWHERE area>=3000000 OR population >= 25000000\n``` | 3 | 0 | [] | 0 |
big-countries | Easy Simple || MySQL Solution ✔✔ | easy-simple-mysql-solution-by-akanksha98-yt0h | Code\n\n# Write your MySQL query statement below\nSELECT name, population, area\nFROM World\nWHERE area>= 3000000 or population>= 25000000 ; \n | akanksha984 | NORMAL | 2023-02-26T14:21:07.699983+00:00 | 2023-02-26T14:21:07.700024+00:00 | 4,081 | false | ## Code\n```\n# Write your MySQL query statement below\nSELECT name, population, area\nFROM World\nWHERE area>= 3000000 or population>= 25000000 ; \n``` | 3 | 0 | ['MySQL'] | 0 |
big-countries | || BASIC SQL COMMAND || ONE LINERS || | basic-sql-command-one-liners-by-ujjwalva-xxyi | \n# Code\n\nselect name,population,area\nfrom World\nwhere area>=3000000 or population>=25000000;\n | ujjwalvarma6948 | NORMAL | 2023-02-17T16:38:37.751862+00:00 | 2023-02-17T16:38:37.751904+00:00 | 1,717 | false | \n# Code\n```\nselect name,population,area\nfrom World\nwhere area>=3000000 or population>=25000000;\n``` | 3 | 0 | ['MySQL'] | 0 |
big-countries | Easy MySQL || beginner solution | easy-mysql-beginner-solution-by-nikhil_m-8fqa | \n\n# Code\n\n# Write your MySQL query statement below\nselect name, population, area\nfrom world\nwhere (area >= 3000000 or population >= 25000000);\n | nikhil_mane | NORMAL | 2022-11-05T17:14:47.887287+00:00 | 2022-11-05T17:14:47.887323+00:00 | 1,864 | false | \n\n# Code\n```\n# Write your MySQL query statement below\nselect name, population, area\nfrom world\nwhere (area >= 3000000 or population >= 25000000);\n``` | 3 | 0 | ['MySQL'] | 2 |
big-countries | ✅MySQL-1 Liner Solution || Beginner level||Simple-Short -Solution✅ | mysql-1-liner-solution-beginner-levelsim-49kj | Please upvote to motivate me in my quest of documenting all leetcode solutions. HAPPY CODING:)\nAny suggestions and improvements are always welcome.\n========== | Anos | NORMAL | 2022-08-15T22:39:40.720867+00:00 | 2022-08-15T22:41:22.138843+00:00 | 431 | false | **Please upvote to motivate me in my quest of documenting all leetcode solutions. HAPPY CODING:)\nAny suggestions and improvements are always welcome.***\n*====================================================================*\n\u2705 **MySQL Code :**\n**Runtime:** 253 ms, faster than 91.58% of MySQL online submissions for Big Countries.\n```\nSELECT name,area,population FROM World WHERE area>=3000000 OR population>=25000000\n```\n**Runtime:** 253 ms\n**Memory Usage:** 0B\n________________________________\n__________________________________\n\nIf you like the solution, please upvote \uD83D\uDD3C\nFor any questions, or discussions, comment below. \uD83D\uDC47\uFE0F | 3 | 0 | ['MySQL'] | 1 |
big-countries | Oracle | Memory 100% | oracle-memory-100-by-sirojiddinnematov-eq4v | ```\nSELECT name, population, area FROM world WHERE area >= 3000000\nUNION \nSELECT name, population, area FROM world WHERE population >= 25000000 | sirojiddinnematov | NORMAL | 2022-07-05T08:34:32.393652+00:00 | 2022-07-05T08:34:32.393702+00:00 | 248 | false | ```\nSELECT name, population, area FROM world WHERE area >= 3000000\nUNION \nSELECT name, population, area FROM world WHERE population >= 25000000 | 3 | 0 | [] | 0 |
Subsets and Splits