licenses
sequencelengths 1
3
| version
stringclasses 677
values | tree_hash
stringlengths 40
40
| path
stringclasses 1
value | type
stringclasses 2
values | size
stringlengths 2
8
| text
stringlengths 25
67.1M
| package_name
stringlengths 2
41
| repo
stringlengths 33
86
|
---|---|---|---|---|---|---|---|---|
[
"MIT"
] | 0.2.2 | c81897832613bbcf7cc19639fd3a1a0a154abe1b | code | 3273 | # perform point-to-point ICP with provided correspondence and distance score functions
# TO DO: for MultiPointSets, choose the appropriate number of weights
function iterate_kabsch(P, Q, wp=ones(size(P,2)), wq=ones(size(Q,2)); iterations=1000, correspondence = hungarian_assignment)
# initial correspondences
matches = correspondence(P,Q)
prevmatches = matches
tform = identity
# iterate until convergence
it = 0
score = Inf
prevscore = Inf
while it < iterations
it += 1
# TO DO: make sure that, for hungarian assignment, the proper weights are selected
prevscore = score
tform = kabsch(P, Q, matches, wp, wq)
score = squared_deviation(tform(P),Q,matches)
if prevscore < score
matches = prevmatches
break
end
prevmatches = matches
matches = correspondence(tform(P),Q)
if matches == prevmatches
break
end
end
return matches
end
iterate_kabsch(P::AbstractPointSet, Q::AbstractSet; kwargs...) = iterate_kabsch(P.coords, Q.coords, P.weights, Q.weights; kwargs...)
iterate_kabsch(P::AbstractMultiPointSet, Q::AbstractMultiPointSet; kwargs...) = iterate_kabsch(P, Q, weights(P), weights(Q); kwargs...)
function icp(P::AbstractMatrix, Q::AbstractMatrix, wp=ones(size(P,2)), wq=ones(size(P,2)); kdtree = KDTree(Q, Euclidean()), kwargs...)
return iterate_kabsch(P, Q, wp, wq; correspondence = f(p,q) = closest_points(p, kdtree), kwargs...)
end
icp(P::AbstractSinglePointSet, Q::AbstractSinglePointSet; kwargs...) = icp(P.coords, Q.coords, P.weights, Q.weights; kwargs...)
iterative_hungarian(args...; kwargs...) = iterate_kabsch(args...; correspondence = hungarian_assignment, kwargs...)
function local_matching_alignment(x::AbstractPointSet, y::AbstractPointSet, block::SearchRegion; matching_fun = iterative_hungarian, kwargs...)
tformedx = block.R*x + block.T
matches = matching_fun(tformedx, y; kwargs...)
tform = kabsch(x, y, matches)
score = squared_deviation(tform(x), y, matches)
R = RotationVec(tform.linear)
params = (R.sx, R.sy, R.sz, tform.translation...)
return (score, params)
end
function local_matching_alignment(x::AbstractPointSet, y::AbstractPointSet, block::RotationRegion; matching_fun = iterative_hungarian, kwargs...)
tformedx = block.R*x + block.T
matches = matching_fun(tformedx, y; kwargs...)
tform = kabsch(x, y, matches)
score = squared_deviation(tform(x), y, matches)
R = RotationVec(tform.linear)
params = (R.sx, R.sy, R.sz)
return (score, params)
end
function local_matching_alignment(x::AbstractPointSet, y::AbstractPointSet, block::TranslationRegion; matching_fun = iterative_hungarian, kwargs...)
tformedx = block.R*x + block.T
matches = matching_fun(tformedx, y; kwargs...)
tform = kabsch(x, y, matches)
score = squared_deviation(tform(x), y, matches)
params = (tform.translation...,)
return (score, params)
end
local_icp(x, y, block::SearchRegion; kwargs...) = local_matching_alignment(x, y, block; matching_fun = icp, kwargs...)
local_iterative_hungarian(x, y, block::SearchRegion; kwargs...) = local_matching_alignment(x, y, block; matching_fun = iterative_hungarian, kwargs...)
| GaussianMixtureAlignment | https://github.com/tmcgrath325/GaussianMixtureAlignment.jl.git |
|
[
"MIT"
] | 0.2.2 | c81897832613bbcf7cc19639fd3a1a0a154abe1b | code | 3409 | # All matrices are DxN, where N is the number of positions and D is the dimensionality
# Here, P is the probe (to be rotated) and Q is the refereence
# https://en.wikipedia.org/wiki/Kabsch_algorithm
# This has been generalized to support weighted points:
# https://igl.ethz.ch/projects/ARAP/svd_rot.pdf
# assuming P and Q are already centered at the origin
# returns the rotation for alignment
function kabsch_centered(P,Q,w)
@assert size(P) == size(Q)
W = diagm(w/sum(w)) # here, the weights are assumed to sum to 1
H = P*W*Q'
D = Matrix{Float64}(I,size(H,1), size(H,2))
# U,Σ,V = svd(H)
U,Σ,V = GenericLinearAlgebra.svd(H)
D[end] = sign(det(V*U'))
return LinearMap(V * D * U')
end
function kabsch_centered(P,Q,matches::AbstractVector{<:Tuple{Int,Int}},wp=ones(size(P,2)),wq=ones(size(Q,2)))
matchedP, matchedQ = matched_points(P,Q,matches)
w = [wp[i]*wq[j] for (i,j) in matches]
return kabsch_centered(matchedP, matchedQ, w)
end
kabsch_centered(P::PointSet, Q::PointSet) = kabsch_centered(P.coords, Q.coords, P.weights .* Q.weights);
kabsch_centered(P::PointSet, Q::PointSet, matches::AbstractVector{<:Tuple{Int,Int}}) = kabsch_centered(P.coords, Q.coords, matches, P.weights, Q.weights);
# transform DxN matrices
function (tform::Translation)(A::AbstractMatrix)
return hcat([tform(A[:,i]) for i=1:size(A,2)]...)
end
# P and Q are not necessarily centered
# returns the transformation for alignment
function kabsch(P, Q, w::AbstractVector=ones(size(P,2)))
@assert !any(w.<0) && sum(w)>0
wn = w/sum(w) # weights should sum to 1 for computing the centroid
centerP, centerQ = center_translation(P,wn), center_translation(Q,wn)
R = kabsch_centered(centerP(P), centerQ(Q), wn)
return inv(centerQ) ∘ R ∘ centerP
end
function kabsch(P,Q,matches::AbstractVector{<:Tuple{Int,Int}},wp=ones(size(P,2)),wq=ones(size(Q,2)))
matchedP, matchedQ = matched_points(P,Q,matches)
w = [wp[i]*wq[j] for (i,j) in matches]
return kabsch(matchedP, matchedQ, w)
end
kabsch(P::PointSet, Q::PointSet) = kabsch(P.coords, Q.coords, P.weights .* Q.weights);
kabsch(P::PointSet, Q::PointSet, matches::AbstractVector{<:Tuple{Int,Int}}) = kabsch(P.coords, Q.coords, matches, P.weights, Q.weights);
function kabsch(P::AbstractMultiPointSet{N,T,K}, Q::AbstractMultiPointSet{N,T,K}, matchesdict, wp = weights(P), wq = weights(Q)) where {N,T,K}
matchedP, matchedQ = matched_points(P,Q,matchesdict)
w = Vector{T}()
for (key, matches) in matchesdict
for m in matches
push!(w, wp[key][m[1]] * wq[key][m[2]])
end
end
return kabsch(matchedP, matchedQ, w)
end
# align via translation only (no rotation)
function translation_align(P,Q,w)
@assert size(P) == size(Q)
wnormalized = w/sum(w)
dists = (Q - P) .* wnormalized'
return Translation(sum(dists; dims=2))
end
function translation_align(P,Q,matches::AbstractVector{<:Tuple{Int,Int}},wp=ones(size(P,2)),wq=ones(size(Q,2)))
matchedP, matchedQ = matched_points(P,Q,matches)
w = [wp[i]*wq[j] for (i,j) in matches]
return translation_align(matchedP, matchedQ, w)
end
translation_align(P::PointSet, Q::PointSet) = translation_align(P.coords, Q.coords, P.weights .* Q.weights)
translation_align(P::PointSet, Q::PointSet, matches::AbstractVector{<:Tuple{Int,Int}}) = translation_align(P.coords, Q.coords, matches, P.weights .* Q.weights) | GaussianMixtureAlignment | https://github.com/tmcgrath325/GaussianMixtureAlignment.jl.git |
|
[
"MIT"
] | 0.2.2 | c81897832613bbcf7cc19639fd3a1a0a154abe1b | code | 2461 | function align_local_points(P, Q; maxevals=1000, tformfun=AffineMap)
# set initial guess at the center of the block
initial_X = zeros(tformfun === AffineMap ? 6 : 3)
trlim = tformfun !== LinearMap ? translation_limit(P, Q) : 0;
lower = tformfun === AffineMap ? [-π, -π, -π, -trlim, -trlim, -trlim] :
tformfun === LinearMap ? [-π, -π, -π] :
[-trlim, -trlim, -trlim]
upper = tformfun === AffineMap ? [π, π, π, trlim, trlim, trlim] :
tformfun === LinearMap ? [π, π, π] :
[trlim, trlim, trlim]
# local optimization within the block
function f(X)
tform = tformfun((X...,))
score = squared_deviation(tform(P), Q)
return score
end
# res = optimize(f, initial_X, LBFGS(), Optim.Options(f_calls_limit=maxevals)) # ; autodiff = :forward)
res = optimize(f, lower, upper, initial_X, Fminbox(LBFGS()), Optim.Options(f_calls_limit=maxevals)) #; autodiff = :forward)
# @show res.iterations
return Optim.minimum(res), tuple(Optim.minimizer(res)...)
end
function iterate_local_alignment(P, Q; correspondence = hungarian_assignment, iterations=100, tformfun=AffineMap, kwargs...)
matches = correspondence(P, Q)
prevmatches = matches
score = Inf
tformparams = zeros(tformfun === AffineMap ? 6 : 3);
it = 0
while (it < iterations)
it += 1
matchedP, matchedQ = matched_points(P,Q,matches)
score, tformparams = align_local_points(matchedP, matchedQ; tformfun=tformfun, kwargs...)
tform = tformfun(tformparams)
prevmatches = matches
matches = correspondence(tform(P), Q)
if matches == prevmatches
break
end
end
return score, tformparams
end
function iterate_local_alignment(P, Q, block; tformfun=AffineMap, kwargs...)
block_tform = AffineMap(block.R, block.T)
tformedP = block_tform(P)
score, opt_tformparams = iterate_local_alignment(tformedP, Q; tformfun=tformfun, kwargs...)
opt_tform = tformfun(opt_tformparams)
tform = opt_tform ∘ block_tform
tform = AffineMap(RotationVec(tform.linear), tform.translation)
tformparams = tformfun === LinearMap ? (tform.linear.sx, tform.linear.sy, tform.linear.sz) :
tformfun === Translation ? (tform.translation...,) :
(tform.linear.sx, tform.linear.sy, tform.linear.sz, tform.translation...)
return score, tformparams
end | GaussianMixtureAlignment | https://github.com/tmcgrath325/GaussianMixtureAlignment.jl.git |
|
[
"MIT"
] | 0.2.2 | c81897832613bbcf7cc19639fd3a1a0a154abe1b | code | 5812 | import Base: eltype, length, size, getindex, iterate, convert, promote_rule, keys
abstract type AbstractPoint{N,T} end
abstract type AbstractPointSet{N,T} <: AbstractModel{N,T} end
abstract type AbstractSinglePointSet{N,T} <: AbstractPointSet{N,T} end
abstract type AbstractMultiPointSet{N,T,K} <: AbstractPointSet{N,T} end
# Base methods for Points
numbertype(::AbstractPoint{N,T}) where {N,T} = T
dims(::AbstractPoint{N,T}) where {N,T} = N
length(::AbstractPoint{N,T}) where {N,T} = N
size(::AbstractPoint{N,T}) where {N,T} = (N,)
size(::AbstractPoint{N,T}, idx::Int) where {N,T} = (N,)[idx]
# Base methods for point sets
numbertype(::AbstractPointSet{N,T}) where {N,T} = T
dims(::AbstractPointSet{N,T}) where {N,T} = N
length(x::AbstractSinglePointSet{N,T}) where {N,T} = size(x.coords,2)
getindex(x::AbstractSinglePointSet, idx) = getpoint(x, idx)
iterate(x::AbstractSinglePointSet) = iterate(x, 1)
iterate(x::AbstractSinglePointSet, i) = i > length(x) ? nothing : (getindex(x, i), i+1)
size(x::AbstractSinglePointSet{N,T}) where {N,T} = (N,length(x))
size(x::AbstractSinglePointSet{N,T}, idx::Int) where {N,T} = size(x)[idx]
coords(x::AbstractSinglePointSet) = x.coords
weights(x::AbstractSinglePointSet) = x.weights
widths(x::AbstractSinglePointSet{N,T}) where {N,T} = fill(zero(T), length(x))
length(x::AbstractMultiPointSet) = length(x.pointsets)
getindex(x::AbstractMultiPointSet, key) = x.pointsets[key]
keys(x::AbstractMultiPointSet) = keys(x.pointsets)
iterate(x::AbstractMultiPointSet) = iterate(x.pointsets)
iterate(x::AbstractMultiPointSet, i) = iterate(x.pointsets, i)
size(x::AbstractMultiPointSet{N,T,K}) where {N,T,K} = (length(x.pointsets), N)
size(x::AbstractMultiPointSet{N,T,K}, idx::Int) where {N,T,K} = (length(x.pointsets), N)[idx]
coords(x::AbstractMultiPointSet) = hcat([coords(ps) for (k,ps) in x.pointsets]...)
weights(x::AbstractMultiPointSet) = vcat([weights(ps) for (k,ps) in x.pointsets]...)
widths(x::AbstractMultiPointSet) = vcat([widths(ps) for (k,ps) in x.pointsets]...)
"""
A coordinate position and a weight, to be used as part of a point set.
"""
struct Point{N,T} <: AbstractPoint{N,T}
coords::SVector{N,T}
weight::T
end
convert(::Type{Point{N,T}}, p::AbstractPoint) where {N,T} = Point(SVector{N,T}(p.coords), T(p.weight))
promote_rule(::Type{Point{N,T}}, ::Type{Point{N,S}}) where {N,T<:Real,S<:Real} = Point{N,promote_type(T,S)}
function Base.:*(R::AbstractMatrix, p::Point)
return Point(R*p.coords, p.weight)
end
function Base.:-(p::Point, T::AbstractVector)
return Point(p.coords-T, p.weight)
end
"""
A point set made consisting of a matrix of coordinate positions with corresponding weights.
"""
struct PointSet{N,T} <: AbstractSinglePointSet{N,T}
coords::Matrix{T}
weights::Vector{T}
end
getpoint(x::PointSet{N,T}, idx::Int) where {N,T} = Point{N,T}(x.coords[:,idx], x.weights[idx])
eltype(::Type{PointSet{N,T}}) where {N,T} = Point{N,T}
convert(t::Type{PointSet}, p::AbstractPointSet) = t(p.coords, p.weights)
promote_rule(::Type{PointSet{N,T}}, ::Type{PointSet{N,S}}) where {T,S,N} = PointSet{N,promote_type(T,S)}
weights(ps::PointSet) = ps.weights
function Base.:*(R::AbstractMatrix, p::PointSet)
return PointSet(R*p.coords, p.weights)
end
function Base.:+(p::PointSet, T::AbstractVector)
return PointSet(p.coords.+T, p.weights)
end
function Base.:-(p::PointSet, T::AbstractVector)
return PointSet(p.coords.-T, p.weights)
end
function PointSet(coords::AbstractMatrix{S}, weights::AbstractVector{T} = ones(S, size(coords,2))) where {S,T}
dim = size(coords,1)
numtype = promote_type(S,T)
return PointSet{dim,numtype}(Matrix{numtype}(coords), Vector{numtype}(weights))
end
PointSet(coords::AbstractVector{<:AbstractVector{T}}, weights::AbstractVector{T} = ones(T, length(coords))) where T = PointSet(hcat(coords...), weights)
"""
A collection of labeled point sets, to each be considered separately during an alignment procedure. That is,
only alignment scores between point sets with the same key are considered when aligning two `MultiPointSet`s.
"""
struct MultiPointSet{N,T,K} <: AbstractMultiPointSet{N,T,K}
pointsets::Dict{K, <:AbstractSinglePointSet{N,T}}
end
MultiPointSet(x::AbstractMultiPointSet) = MultiPointSet(x.pointsets)
function MultiPointSet(pointsetpairs::AbstractVector{<:Pair{K, S}}) where {K, S<:AbstractSinglePointSet}
psdict = Dict{K,S}()
for pair in pointsetpairs
push!(psdict, pair)
end
return MultiPointSet(psdict)
end
eltype(::Type{MultiPointSet{N,T,K}}) where {N,T,K} = Pair{K, PointSet{N,T}}
convert(t::Type{MultiPointSet}, x::AbstractMultiPointSet) = t(x.pointsets)
promote_rule(::Type{MultiPointSet{N,T,K}}, ::Type{MultiPointSet{N,S,L}}) where {N,T,S,K,L} = MultiPointSet{N,promote_type(T,S), promote_type(K,L)}
function weights(mps::MultiPointSet{N,T,K}) where {N,T,K}
w = Dict{K, Vector{T}}()
for (key, ps) in mps.pointsets
push!(w, key => ps.weights)
end
return w
end
function Base.:*(R::AbstractMatrix, p::MultiPointSet)
return MultiPointSet([key => R*p[key] for key in keys(p)])
end
function Base.:+(p::MultiPointSet, T::AbstractVector)
return MultiPointSet([key => p[key]+T for key in keys(p)])
end
function Base.:-(p::MultiPointSet, T::AbstractVector)
return MultiPointSet([key => p[key]-T for key in keys(p)])
end
Base.show(io::IO, p::AbstractPoint) = println(io,
summary(p),
" with μ = $(p.coords) and ϕ = $(p.weight).\n"
)
Base.show(io::IO, ps::AbstractSinglePointSet) = println(io,
summary(ps),
" with $(length(ps)) points."
)
Base.show(io::IO, mps::AbstractMultiPointSet) = println(io,
summary(mps),
" with $(length(mps)) labeled $(eltype(mps.pointsets).parameters[2]) sets and a total of $(sum([length(ps) for (key,ps) in mps.pointsets])) points."
) | GaussianMixtureAlignment | https://github.com/tmcgrath325/GaussianMixtureAlignment.jl.git |
|
[
"MIT"
] | 0.2.2 | c81897832613bbcf7cc19639fd3a1a0a154abe1b | code | 1056 | squared_deviation(P::AbstractMatrix, Q::AbstractMatrix, w::AbstractVector=ones(size(P,2))) = sum(w .* colwise(SqEuclidean(), P, Q))
squared_deviation(P::AbstractMatrix, Q::AbstractMatrix, matches::AbstractVector{<:Tuple{Int,Int}}, wp=ones(size(P,2)), wq=ones(size(Q,2))) = squared_deviation(matched_points(P, Q, matches)..., [wp[i]*wq[j] for (i,j) in matches])
squared_deviation(P::AbstractPointSet, Q::AbstractPointSet, matches::AbstractVector{<:Tuple{Int,Int}}) = squared_deviation(P.coords, Q.coords, matches, P.weights, Q.weights)
squared_deviation(P::AbstractPointSet, Q::AbstractPointSet) = squared_deviation(P.coords, Q.coords, hungarian_assignment(P.coords,Q.coords), P.weights, Q.weights)
function squared_deviation(P::AbstractMultiPointSet{N,T,K}, Q::AbstractMultiPointSet{N,T,K}, matchesdict) where {N,T,K}
sqdev = zero(T)
for (key, matches) in matchesdict
sqdev += squared_deviation(P.pointsets[key], Q.pointsets[key], matches)
end
return sqdev
end
rmsd(P, Q, args...) = sqrt(squared_deviation(P,Q,args...)/size(P,2))
| GaussianMixtureAlignment | https://github.com/tmcgrath325/GaussianMixtureAlignment.jl.git |
|
[
"MIT"
] | 0.2.2 | c81897832613bbcf7cc19639fd3a1a0a154abe1b | code | 1480 | """
tset = tivpointset(ps::PointSet, c=Inf)
tset = tivpointset(mps::MultiPointSet, c=Inf)
Returns a new `PointSet` or `MultiPointSet` containing up to `c*length(gmm)` translation invariant vectors (TIVs) connecting point coordinates in the input model.
TIVs are chosen to maximize length multiplied by the weights of the connected distributions.
See [Li et. al. (2019)](https://arxiv.org/abs/1812.11307) for a description of TIV construction.
"""
function tivpointset(x::AbstractSinglePointSet, c=Inf)
t = numbertype(x)
npts = length(x)
n = ceil(c)*npts
if npts^2 < n
n = npts^2
end
scores = fill(zero(t), npts, npts)
for i=1:npts
for j = i+1:npts
scores[i,j] = scores[j,i] = norm(x[i].coords - x[j].coords) * √(x[i].weight * x[j].weight)
end
end
tivcoords = SVector{3,t}[]
tivweights = t[]
order = sortperm(vec(scores), rev=true)
for idx in order[1:Int(n)]
i = Int(floor((idx-1)/npts)+1)
j = mod(idx-1, npts)+1
push!(tivcoords, x[i].coords - x[j].coords)
push!(tivweights, √(x[i].weight * x[j].weight))
end
return PointSet(hcat(tivcoords...), tivweights)
end
function tivpointset(mps::AbstractMultiPointSet, c=Inf)
pointsets = Dict{Symbol, PointSet{dims(mps),numbertype(mps)}}()
for key in keys(mps.pointsets)
push!(pointsets, Pair(key, tivpointset(mps.pointsets[key], c)))
end
return MultiPointSet(pointsets)
end
| GaussianMixtureAlignment | https://github.com/tmcgrath325/GaussianMixtureAlignment.jl.git |
|
[
"MIT"
] | 0.2.2 | c81897832613bbcf7cc19639fd3a1a0a154abe1b | code | 3984 | struct ROCSAlignmentResult{D,S,T,F<:AbstractAffineMap,X<:AbstractGMM{D,S},Y<:AbstractGMM{D,T}} <: AlignmentResults
x::X
y::Y
minimum::T
tform::F
end
"""
m = second_moment(gmm, center, dim1, dim2)
Returns the second order moment of `gmm`
"""
function mass_matrix(positions::AbstractMatrix{<:Real},
weights=ones(eltype(positions),size(positions,2)),
widths=zeros(eltype(positions),size(positions,2)),
center=centroid(positions,weights))
t = eltype(positions)
npts = size(positions,2)
M = fill(zero(t), 3, 3)
dists = [positions[:,i].-center for i=1:npts]
# diagonal terms
for i=1:3
for j=1:npts
M[i,i] += weights[j] * (dists[j][i]^2 + widths[j]^2)
end
end
# off-diagonal terms
for i=1:3
for j=i+1:3
for k=1:npts
inc = weights[k] * dists[k][i] * dists[k][j]
M[i,j] += inc
M[j,i] += inc
end
end
end
return M
end
"""
tforms = inertial_transforms(gmm)
Returns 10 transformations to put `gmm` in an inertial frame. That is, the mass matrix of the GMM
is made diagonal, and the GMM center of mass is made the origin.
"""
function inertial_transforms(positions::AbstractMatrix{<:Real},
weights=ones(eltype(positions),size(positions,2)),
widths=zeros(eltype(positions),size(positions,2));
invert = false)
com = centroid(positions, weights / sum(weights))
massmat = mass_matrix(positions, weights, widths, com)
evecs = eigvecs(massmat)
T = eltype(com)
N = length(com)
# obtain all rotations that align the mass matrix to the coordinate system
tforms = AffineMap{SMatrix{N,N,T,N^2},SVector{N,T}}[]
# first, align the the eigenvectors to the coordinate system axes
# make sure that a reflection is not performed
if det(evecs) < 0
evecs[:,end] = -evecs[:,end]
end
push!(tforms, inv(LinearMap(SMatrix{length(com),length(com)}(evecs))) ∘ Translation(-com))
# then consider unique rotations made up of 180 degree rotations about the coordinate axes (out of all 2^3, there are 4 unique poses)
for i=1:3
axis = zeros(T,N)
axis[i] = one(T)
push!(tforms, LinearMap(AngleAxis(π, axis...)) ∘ tforms[1])
end
if invert
return [inv(tform) for tform in tforms]
else
return tforms
end
end
inertial_transforms(x::AbstractModel; kwargs...) = inertial_transforms(coords(x), weights(x), widths(x); kwargs...)
"""
score, tform, nevals = rocs_align_gmms(gmmfixed, gmmmoving; maxevals=1000)
Finds the optimal alignment between the two supplied GMMs using steric multipoles,
based on the [ROCS alignment algorithm.](https://docs.eyesopen.com/applications/rocs/theory/shape_shape.html)
"""
function rocs_align(gmmmoving::AbstractGMM, gmmfixed::AbstractGMM; kwargs...)
# align both GMMs to their inertial frames
tformfixed = inertial_transforms(gmmfixed)[1] # Only need one alignment for the fixed GMM
tformsmoving = inertial_transforms(gmmmoving) # Take all 4 rotations for the GMM to be aligned
# perform local alignment starting at each inertial rotation for the "moving" GMM
results = Tuple{Float64, NTuple{6,Float64}}[]
for tformm in tformsmoving
push!(results, local_align(tformm(gmmmoving), tformfixed(gmmfixed); kwargs...))
end
# combine the inertial transform with the subsequent alignment transform for the best result
minoverlap, mindex = findmin([r[1] for r in results])
tformmoving = AffineMap(results[mindex][2]) ∘ tformsmoving[mindex]
# Apply the inverse of `tformfixed` to the optimized transformation
alignment_tform = inv(tformfixed) ∘ tformmoving
# return the result
return ROCSAlignmentResult(gmmmoving, gmmfixed, minoverlap, alignment_tform)
end | GaussianMixtureAlignment | https://github.com/tmcgrath325/GaussianMixtureAlignment.jl.git |
|
[
"MIT"
] | 0.2.2 | c81897832613bbcf7cc19639fd3a1a0a154abe1b | code | 985 | # Speed up rotation search of TIV-GOGMA by using the ROCS alignment algorithm to produce a best rotation
function rocs_gogma_align(gmmx::AbstractGMM, gmmy::AbstractGMM; kwargs...)
t = promote_type(numbertype(gmmx),numbertype(gmmy))
p = t(π)
# rotation alignment
rocs_res = rocs_align(gmmx, gmmy)
rotpos = rot_to_axis(rocs_res.tform.linear)
# translation alignment
trl_res = trl_gogma_align(gmmx, gmmy; rot=rotpos, kwargs...)
# local alignment in the full transformation space
pos = NTuple{6, t}([rotpos..., trl_res.tform_params...])
trlim = translation_limit(gmmx, gmmy)
localblock = Block(((-p,p), (-p,p), (-p,p), (-trlim,trlim), (-trlim,trlim), (-trlim,trlim)), pos, trl_res.upperbound, -Inf)
min, bestpos = local_align(gmmx, gmmy, localblock)
return GMAlignmentResult(gmmx, gmmy, min, trl_res.lowerbound, AffineMap(bestpos...), bestpos, trl_res.obj_calls, trl_res.num_splits, trl_res.num_blocks, trl_res.stagnant_splits)
end | GaussianMixtureAlignment | https://github.com/tmcgrath325/GaussianMixtureAlignment.jl.git |
|
[
"MIT"
] | 0.2.2 | c81897832613bbcf7cc19639fd3a1a0a154abe1b | code | 692 | # Smoke tests for drawing functions
using Makie # load GLMakie or CairoMakie externally
using GaussianMixtureAlignment
using Test
@testset "drawing" begin
tetrahedral = [
[0.,0.,1.],
[sqrt(8/9), 0., -1/3],
[-sqrt(2/9),sqrt(2/3),-1/3],
[-sqrt(2/9),-sqrt(2/3),-1/3]
]
gmm = IsotropicGMM([IsotropicGaussian(x, 1.2, 1) for x in tetrahedral])
gmmdisplay(gmm)
ch_g = IsotropicGaussian(tetrahedral[1], 1.0, 1.0)
s_gs = [IsotropicGaussian(x, 0.5, 1.0) for (i,x) in enumerate(tetrahedral)]
mgmmx = IsotropicMultiGMM(Dict(
:positive => IsotropicGMM([ch_g]),
:steric => IsotropicGMM(s_gs)
))
gmmdisplay(mgmmx)
end
| GaussianMixtureAlignment | https://github.com/tmcgrath325/GaussianMixtureAlignment.jl.git |
|
[
"MIT"
] | 0.2.2 | c81897832613bbcf7cc19639fd3a1a0a154abe1b | code | 11723 | using GaussianMixtureAlignment
using Test
using IntervalSets
using LinearAlgebra
using StaticArrays
using Rotations
using CoordinateTransformations
using ForwardDiff
using GaussianMixtureAlignment: UncertaintyRegion, RotationRegion, TranslationRegion
using GaussianMixtureAlignment: tight_distance_bounds, loose_distance_bounds, gauss_l2_bounds, subranges, sqrt3, UncertaintyRegion, subregions, branchbound, rocs_align, overlap, gogma_align, tiv_gogma_align, tiv_goih_align, overlapobj
const GMA = GaussianMixtureAlignment
@testset "search space bounds" begin
μx = SVector(3,0,0)
μy = SVector(-4,0,0)
σ = ϕ = 1
ndims = 3
sqrt2 = √2
x, y = IsotropicGaussian(μx, σ, ϕ), IsotropicGaussian(μy, σ, ϕ)
### tight_distance_bounds
# anti-aligned (no rotation) and aligned (180 degree rotation)
lbdist, ubdist = tight_distance_bounds(x,y,π,0)
@test ubdist ≈ 7
@test lbdist ≈ 1
# region with closest alignment at 90 degree rotation
lbdist, ubdist = tight_distance_bounds(x,y,π/2/sqrt3,0)
@test lbdist ≈ 5
# translation region centered at origin
lbdist, ubdist = tight_distance_bounds(x,y,0,1/√3)
@test lbdist ≈ 6
@test ubdist ≈ 7
# centered at x = 1
lbdist, ubdist = tight_distance_bounds(x+SVector(1,0,0),y,0,1/sqrt3)
@test lbdist ≈ 7
@test ubdist ≈ 8
### loose_distance_bounds
### Gaussian L2 bounds
# rotation distances, no translation
# anti-aligned (no rotation) and aligned (180 degree rotation)
lb, ub = gauss_l2_bounds(x,y,RotationRegion(0.))
@test lb ≈ -GMA.overlap(7^2,2*σ^2,ϕ*ϕ) atol=1e-16
@test ub ≈ -GMA.overlap(7^2,2*σ^2,ϕ*ϕ)
lb, ub = gauss_l2_bounds(x,y,RotationRegion(RotationVec(0.,0.,π),SVector{3}(0.,0.,0.),0.))
@test lb ≈ ub ≈ -GMA.overlap(1,2*σ^2,ϕ*ϕ)
# region with closest alignment at 90 degree rotation
lb = gauss_l2_bounds(x,y,RotationRegion(π/2/sqrt3))[1]
@test lb ≈ -GMA.overlap(5^2,2*σ^2,ϕ*ϕ)
lb = gauss_l2_bounds(x,y,RotationRegion(RotationVec(0,0,π/4),SVector{3}(0.,0.,0.),π/4/(sqrt3)))[1]
@test lb ≈ -GMA.overlap(5^2,2*σ^2,ϕ*ϕ)
# translation distance, no rotation
# translation region centered at origin
lb, ub = gauss_l2_bounds(x,y,TranslationRegion(1/sqrt3))
@test lb ≈ -GMA.overlap(6^2,2*σ^2,ϕ*ϕ)
@test ub ≈ -GMA.overlap(7^2,2*σ^2,ϕ*ϕ)
# centered with translation of 1 in +x
lb, ub = gauss_l2_bounds(x+SVector(1,0,0),y,TranslationRegion(1/sqrt3))
@test lb ≈ -GMA.overlap(7^2,2*σ^2,ϕ*ϕ)
@test ub ≈ -GMA.overlap(8^2,2*σ^2,ϕ*ϕ)
# centered with translation of 3 in +y
lb, ub = gauss_l2_bounds(x+SVector(0,3,0),y,TranslationRegion(1/sqrt3))
@test lb ≈ -GMA.overlap((√(58)-1)^2,2*σ^2,ϕ*ϕ)
@test ub ≈ -GMA.overlap(58,2*σ^2,ϕ*ϕ)
end
@testset "divide a searchspace" begin
blk1 = NTuple{6,Tuple{Float64,Float64}}(((-π,π), (-π,π), (-π,π), (-1,1), (-1,1), (-1,1)))
subblks1 = subranges(blk1, 2)
@test length(subblks1) == 2^6
for i=1:6
intv = OpenInterval(0,0)
for sblk in subblks1
rng = sblk[i]
intv = union(intv, ClosedInterval(rng[1], rng[2]))
end
@test intv == ClosedInterval(blk1[i][1], blk1[i][2])
end
# @show subblks1[length(subblks1)]
blk2 = NTuple{6,Tuple{Float64,Float64}}(((0,π), (0,π), (0,π), (0,1), (0,1), (0,1)))
subblks2 = subranges(blk2, 4)
@test length(subblks2) == 4^6
for i=1:6
intv = OpenInterval(0,0)
for sblk in subblks2
rng = sblk[i]
intv = union(intv, ClosedInterval(rng[1], rng[2]))
end
@test intv == ClosedInterval(blk2[i][1], blk2[i][2])
end
end
@testset "GMM interface" begin
tetrahedral = [
[0.,0.,1.],
[sqrt(8/9), 0., -1/3],
[-sqrt(2/9),sqrt(2/3),-1/3],
[-sqrt(2/9),-sqrt(2/3),-1/3]
]
ch_g = IsotropicGaussian(tetrahedral[1], 1.0, 1.0)
s_gs = [IsotropicGaussian(x, 0.5, 1.0) for (i,x) in enumerate(tetrahedral)]
gmm = IsotropicGMM(s_gs)
@test length(gmm) == 4
@test gmm[2] == s_gs[2]
@test collect(gmm) == s_gs # tests iterate
@test eltype(gmm) === eltype(typeof(gmm)) === IsotropicGaussian{3,Float64}
@test convert(IsotropicGMM{3,Float32}, gmm) isa IsotropicGMM{3,Float32}
@test_throws DimensionMismatch convert(IsotropicGMM{2,Float64}, gmm)
mgmmx = IsotropicMultiGMM(Dict(
:positive => IsotropicGMM([ch_g]),
:steric => gmm
))
@test keys(mgmmx) == Set([:positive, :steric])
@test keytype(mgmmx) === keytype(typeof(mgmmx)) === Symbol
@test valtype(mgmmx) === valtype(typeof(mgmmx)) === IsotropicGMM{3,Float64}
@test eltype(mgmmx) === eltype(typeof(mgmmx)) === Pair{Symbol, IsotropicGMM{3,Float64}}
@test length(mgmmx) == 2
@test length(mgmmx[:steric]) == 4
@test mgmmx[:steric][2] == s_gs[2]
@test collect(mgmmx) == collect(mgmmx.gmms) # tests iterate
@test get!(valtype(mgmmx), mgmmx, :positive) == mgmmx[:positive]
gmm = get!(valtype(mgmmx), mgmmx, :acceptor)
@test isempty(gmm) && gmm isa IsotropicGMM{3,Float64}
push!(gmm, ch_g)
@test length(gmm) == 1
pop!(gmm)
@test isempty(gmm)
push!(gmm, ch_g)
empty!(gmm)
@test isempty(gmm)
delete!(mgmmx, :acceptor)
@test !haskey(mgmmx, :acceptor)
empty!(mgmmx)
@test isempty(mgmmx)
end
@testset "bounds for shrinking searchspace around an optimum" begin
# two sets of points, each forming a 3-4-5 triangle
xpts = [[0.,0.,0.], [3.,0.,0.,], [0.,4.,0.]]
ypts = [[1.,1.,1.], [1.,-2.,1.], [1.,1.,-3.]]
σ = ϕ = 1.
gmmx = IsotropicGMM([IsotropicGaussian(x, σ, ϕ) for x in xpts])
gmmy = IsotropicGMM([IsotropicGaussian(y, σ, ϕ) for y in ypts])
# aligning a GMM to itself
bigblock = UncertaintyRegion(gmmx, gmmx)
(lb,ub) = gauss_l2_bounds(gmmx, gmmx, bigblock)
@test lb ≈ -length(gmmx.gaussians)^2 # / √(4π)^3
blk = UncertaintyRegion(RotationVec{Float64}(π/2, π/2, π/2), SVector{3,Float64}(1.0, 1.0, 1.0), π/2, 1.0)
(lb,ub) = gauss_l2_bounds(gmmx, gmmx, blk)
for i = 1:20
blk = subregions(blk)[1]
(newlb,newub) = gauss_l2_bounds(gmmx, gmmx, blk)
@test newlb >= lb
@test newub <= ub
(lb,ub) = (newlb, newub)
end
end
@testset "GOGMA runs without errors" begin
# two sets of points, each forming a 3-4-5 triangle
xpts = [[0.,0.,0.], [3.,0.,0.,], [0.,4.,0.]]
ypts = [[1.,1.,1.], [1.,-2.,1.], [1.,1.,-3.]]
σ = ϕ = 1.
gmmx = IsotropicGMM([IsotropicGaussian(x, σ, ϕ) for x in xpts])
gmmy = IsotropicGMM([IsotropicGaussian(y, σ, ϕ) for y in ypts])
# make sure this runs without an error
res1 = gogma_align(gmmx, gmmy; maxsplits=1E3)
res2 = tiv_gogma_align(gmmx, gmmy; maxsplits=1E3)
mgmmx = IsotropicMultiGMM(Dict(:x => gmmx, :y => gmmx))
mgmmy = IsotropicMultiGMM(Dict(:x => gmmy, :y => gmmy))
res3 = gogma_align(mgmmx, mgmmy; maxsplits=1E3)
res4 = tiv_gogma_align(mgmmx, mgmmy)
# ROCS alignment should work perfectly for these GMMs
@test isapprox(rocs_align(gmmx, gmmy).minimum, -overlap(gmmx,gmmx); atol=1E-12)
end
@testset "Evaluation at a point" begin
pts = [[0.,0.,0.], [3.,0.,0.,], [0.,4.,0.]]
σ = ϕ = 1.
gmm = IsotropicGMM([IsotropicGaussian(x, σ, ϕ) for x in pts])
pt = [1, 1, 1]
gpt = [g(pt) for g in gmm.gaussians]
@test gpt ≈ [exp(-3/2), exp(-6/2), exp(-11/2)]
@test gmm(pt) == sum(gpt)
end
@testset "MultiGMMs with interactions" begin
tetrahedral = [
[0.,0.,1.],
[sqrt(8/9), 0., -1/3],
[-sqrt(2/9),sqrt(2/3),-1/3],
[-sqrt(2/9),-sqrt(2/3),-1/3]
]
ch_g = IsotropicGaussian(tetrahedral[1], 1.0, 1.0)
s_gs = [IsotropicGaussian(x, 0.5, 1.0) for (i,x) in enumerate(tetrahedral)]
mgmmx = IsotropicMultiGMM(Dict(
:positive => IsotropicGMM([ch_g]),
:steric => IsotropicGMM(s_gs)
))
mgmmy = IsotropicMultiGMM(Dict(
:negative => IsotropicGMM([ch_g]),
:steric => IsotropicGMM(s_gs)
))
# interaction validation
interactions = Dict(
(:positive, :negative) => 1.0,
(:negative, :positive) => 1.0,
(:positive, :positive) => -1.0,
(:negative, :negative) => -1.0,
(:steric, :steric) => -1.0,
)
@test_throws AssertionError GMA.pairwise_consts(mgmmx, mgmmy, interactions)
interactions = Dict(
(:positive, :negative) => 1.0,
(:positive, :positive) => -1.0,
(:negative, :negative) => -1.0,
(:steric, :steric) => -1.0,
)
randtform = AffineMap(RotationVec(π*0.1rand(3)...), SVector{3}(0.1*rand(3)...))
res = gogma_align(randtform(mgmmx), mgmmy; interactions=interactions, maxsplits=5e3, nextblockfun=GMA.randomblock)
end
@testset "Forces" begin
μx = randn(SVector{3,Float64})
μy = randn(SVector{3,Float64})
σx = 1 + rand()
σy = 1 + rand()
ϕx = 1 + rand()
ϕy = 1 + rand()
x = IsotropicGaussian(μx, σx, ϕx)
y = IsotropicGaussian(μy, σy, ϕy)
f = zeros(3)
force!(f, x, y)
ovlp(μ) = overlap(IsotropicGaussian(μ, σx, ϕx), y)
@test f ≈ ForwardDiff.gradient(ovlp, μx)
tetrahedral = [
[0.,0.,1.],
[sqrt(8/9), 0., -1/3],
[-sqrt(2/9),sqrt(2/3),-1/3],
[-sqrt(2/9),-sqrt(2/3),-1/3]
]
ch_g = IsotropicGaussian(tetrahedral[1], 1.0, 1.0)
s_gs = [IsotropicGaussian(x, 0.5, 1.0) for (i,x) in enumerate(tetrahedral)]
mgmmx = IsotropicMultiGMM(Dict(
:positive => IsotropicGMM([ch_g]),
:steric => IsotropicGMM(s_gs)
))
mgmmy = IsotropicMultiGMM(Dict(
:negative => IsotropicGMM([ch_g]),
:steric => IsotropicGMM(s_gs)
))
fliptform = AffineMap(RotationVec(π,0,0),[0,0,3]) ∘ AffineMap(RotationVec(0,0,π),[0,0,0])
mgmmy = fliptform(mgmmy)
interactions = Dict(
(:positive, :negative) => 1.0,
(:positive, :positive) => -1.0,
(:negative, :negative) => -1.0,
(:steric, :steric) => -1.0,
)
f = zeros(3)
force!(f, mgmmx, mgmmy; interactions=interactions)
movlp(μ) = overlap(IsotropicMultiGMM(Dict(:positive => IsotropicGMM([ch_g + μ]),:steric => IsotropicGMM([g + μ for g in s_gs]))), mgmmy, nothing, nothing, interactions)
@test f ≈ ForwardDiff.gradient(movlp, zeros(3))
end
@testset "GO-ICP and GO-IH run without errors" begin
xpts = [[0.,0.,0.], [3.,0.,0.,], [0.,4.,0.]]
ypts = [[1.,1.,1.], [1.,-2.,1.], [1.,1.,-3.]]
xset = PointSet(xpts);
yset = PointSet(ypts);
goicp_res = goicp_align(xset, yset)
@test goicp_res.tform(xset.coords) ≈ yset.coords
goih_res = goih_align(xset, yset)
@test goih_res.tform(xset.coords) ≈ yset.coords
end
@testset "Kabsch" begin
xpts = [[0.,0.,0.], [3.,0.,0.,], [0.,4.,0.]]
ypts = [[1.,1.,1.], [1.,-2.,1.], [1.,1.,-3.]]
xset = PointSet(xpts, ones(3))
yset = PointSet(ypts, ones(3))
tform = kabsch(xset, yset)
@test yset.coords ≈ tform(xset).coords
end
@testset "GO-ICP" begin
ycoords = rand(3,50) * 5 .- 10;
randtform = AffineMap(RotationVec(π*rand(3)...), SVector{3}(5*rand(3)...))
xcoords = randtform(ycoords)
xset = PointSet(xcoords, ones(size(xcoords,2)))
yset = PointSet(xcoords, ones(size(ycoords,2)))
res = goicp_align(yset, xset)
@test res.lowerbound == 0
@test res.upperbound < 1e-15
end
@testset "globally optimal iterative hungarian" begin
ycoords = rand(3,5) * 5 .- 10;
randtform = AffineMap(RotationVec(π*rand(3)...), SVector{3}(5*rand(3)...))
xcoords = randtform(ycoords)
xset = PointSet(xcoords, ones(size(xcoords,2)))
yset = PointSet(xcoords, ones(size(ycoords,2)))
res = goih_align(yset, xset)
@test res.lowerbound == 0
@test res.upperbound < 1e-15
end
| GaussianMixtureAlignment | https://github.com/tmcgrath325/GaussianMixtureAlignment.jl.git |
|
[
"MIT"
] | 0.2.2 | c81897832613bbcf7cc19639fd3a1a0a154abe1b | docs | 5157 | # GaussianMixtureAlignment.jl
A Julia implementation of the Globally-Optimal Gaussian Mixture Alignment (GOGMA) algorithm [(Campbell, 2016)](https://arxiv.org/abs/1603.00150), with modifications inspired by
[Li et. al. (2018)](https://arxiv.org/abs/1812.11307).
The GOGMA algorithm uses a branch-and-bound procedure to return a globally optimal alignment of point sets via rigid transformation. In order to improve speed for small point sets, the alignment problem can be split to separately optimize rotational and translational alignments, while still guaranteeing global optimality, through the using of translation invariant vectors (TIVs).
Becaues the runtime of the GOGMA algorithm is O(n^2), and that of the TIV-GOGMA algorithm is O(n^4), they may be unsuitable for use with large point sets without downsampling.
## Construct Isotropic Gaussian Mixture Models (GMMs) for alignment
```julia
julia> using GaussianMixtureAlignment; GMA = GaussianMixtureAlignment;
julia> # These are very simple point sets that can be perfectly aligned
julia> xpts = [[0.,0.,0.], [3.,0.,0.,], [0.,4.,0.]];
julia> ypts = [[1.,1.,1.], [1.,-2.,1.], [1.,1.,-3.]];
julia> σ = ϕ = 1.;
julia> gmmx = IsotropicGMM([IsotropicGaussian(x, σ, ϕ) for x in xpts])
IsotropicGMM{3, Float64} with 3 IsotropicGaussian{3, Float64} distributions.
julia> gmmy = IsotropicGMM([IsotropicGaussian(y, σ, ϕ) for y in ypts])
IsotropicGMM{3, Float64} with 3 IsotropicGaussian{3, Float64} distributions.
julia> # Compute the overlap between the two GMMs
julia> overlap(gmmx, gmmy)
1.1908057504684806
```
## Align Isotropic GMMs with GOGMA
```julia
julia> res = gogma_align(gmmx, gmmy; nextblockfun=GMA.randomblock, maxsplits=10000);
julia> # upper and lower bounds of alignmnet objective function at search termination
julia> res.upperbound, res.lowerbound
(-3.251290635173653, -5.528700740172087)
julia> # rotation component of the best transformation
julia> res.tform.linear
3×3 AngleAxis{Float64} with indices SOneTo(3)×SOneTo(3)(2.0944, -0.57735, 0.57735, -0.57735):
-1.38948e-11 -2.5226e-11 1.0
-1.0 9.12343e-11 -1.38948e-11
-9.12343e-11 -1.0 -2.52261e-11
julia> # translation component of the best transformation
julia> res.tform.translation
3-element StaticArrays.SVector{3, Float64} with indices SOneTo(3):
1.0000000000115654
1.000000000557716
0.9999999997059433
julia> # Compute the overlap between the GMMs after alignment (equal to res.upperbound)
julia> overlap(res.tform(gmmx), gmmy)
3.251290635173653
```
## Plot Isotropic GMMs
```julia
julia> using GLMakie
julia> # Draw the unaligned GMMs
julia> gmmdisplay(gmmx,gmmy)
```
<img src="./assets/image/example.png" width="400"/>
```julia
julia> # Draw the aligned GMMs
julia> gmmdisplay(res.tform(gmmx), gmmy)
```
<img src="./assets/image/example_aligned.png" width="400"/>
## Align GMMs with different types of interacting features
```julia
julia> # four points defining a tetrahedron
julia> tetrahedron = [
[0.,0.,1.],
[sqrt(8/9), 0., -1/3],
[-sqrt(2/9),sqrt(2/3),-1/3],
[-sqrt(2/9),-sqrt(2/3),-1/3]
];
julia> # each feature type is given its own IsotropicGMM
julia> chargedGMM = IsotropicGMM([IsotropicGaussian(tetrahedron[1], 1.0, 1.0)]);
julia> stericGMM = IsotropicGMM([IsotropicGaussian(p, 0.5, 1.0) for p in tetrahedron]);
julia> mgmmx = IsotropicMultiGMM(Dict(
:positive => chargedGMM,
:steric => stericGMM
))
IsotropicMultiGMM{3, Float64, Symbol} with 2 labeled IsotropicGMM{3, Float64} models made up of a total of 5 IsotropicGMM{3, Float64} distributions.
julia> mgmmy = IsotropicMultiGMM(Dict(
:negative => chargedGMM,
:steric => stericGMM
))
IsotropicMultiGMM{3, Float64, Symbol} with 2 labeled IsotropicGMM{3, Float64} models made up of a total of 5 IsotropicGMM{3, Float64} distributions.
julia> # define interactions between each type of feature
julia> interactions = Dict(
:positive => Dict(
:positive => -1.0,
:negative => 1.0,
),
:negative => Dict(
:positive => 1.0,
:negative => -1.0,
),
:steric => Dict(
:steric => -1.0,
),
);
julia> # apply a random rigid transformation to one of the models
julia> using CoordinateTransformations; randtform = AffineMap((rand(), rand(), rand(), rand(), rand(), rand()));
julia> mgmmx = randtform(mgmmx);
julia> # compute alignment between the two MultiIsotropicGMMs
julia> res = gogma_align(mgmmx, mgmmy; interactions=interactions, nextblockfun=GMA.randomblock, maxsplits=10000);
```
## Plot MultiGMMs
```
julia> # Draw the unaligned GMMs
julia> gmmdisplay(mgmmx)
```
<img src="./assets/image/multi_example_x.png" width="400"/>
```
julia> gmmdisplay(mgmmy)
```
<img src="./assets/image/multi_example_y.png" width="400"/>
```julia
julia> # Draw the aligned GMMs. Note that the "steric" features (green) repulse one another.
julia> gmmdisplay(res.tform(mgmmx), mgmmy)
```
<img src="./assets/image/multi_example_aligned.png" width="400"/> | GaussianMixtureAlignment | https://github.com/tmcgrath325/GaussianMixtureAlignment.jl.git |
|
[
"MIT"
] | 0.2.2 | c81897832613bbcf7cc19639fd3a1a0a154abe1b | docs | 259 | ```@meta
CurrentModule = GaussianMixtureAlignment
```
# GaussianMixtureAlignment
Documentation for [GaussianMixtureAlignment](https://github.com/tmcgrath325/GaussianMixtureAlignment.jl).
```@index
```
```@autodocs
Modules = [GaussianMixtureAlignment]
```
| GaussianMixtureAlignment | https://github.com/tmcgrath325/GaussianMixtureAlignment.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 103 | push!(LOAD_PATH,"../src/")
using Documenter, SymEngine
makedocs(sitename="Symengine Julia API Docs")
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 3950 | module SymEngineSymbolicUtilsExt
using SymEngine
using SymbolicUtils
import SymEngine: SymbolicType
#
function is_number(a::SymEngine.Basic)
cls = SymEngine.get_symengine_class(a)
any(==(cls), SymEngine.number_types) && return true
false
end
λ(x::SymEngine.SymbolicType) = λ(Val(SymEngine.get_symengine_class(x)))
λ(::Val{T}) where {T} = getfield(Main, Symbol(lowercase(string(T))))
λ(::Val{:Add}) = +; λ(::Val{:Sub}) = -
λ(::Val{:Mul}) = *; λ(::Val{:Div}) = /
λ(::Val{:Pow}) = ^
λ(::Val{:re}) = real; λ(::Val{:im}) = imag
λ(::Val{:Abs}) = abs
λ(::Val{:Log}) = log
λ(::Val{:Sin}) = sin; λ(::Val{:Cos}) = cos; λ(::Val{:Tan}) = tan
λ(::Val{:Csc}) = csc; λ(::Val{:Sec}) = sec; λ(::Val{:Cot}) = cot
λ(::Val{:Asin}) = asin; λ(::Val{:Acos}) = acos; λ(::Val{:Atan}) = atan
λ(::Val{:Acsc}) = acsc; λ(::Val{:Asec}) = asec; λ(::Val{:Acot}) = acot
λ(::Val{:Sinh}) = sinh; λ(::Val{:Cosh}) = cosh; λ(::Val{:Tanh}) = tanh
λ(::Val{:Csch}) = csch; λ(::Val{:Sech}) = sech; λ(::Val{:Coth}) = coth
λ(::Val{:Asinh}) = asinh; λ(::Val{:Acosh}) = acosh; λ(::Val{:Atanh}) = atanh
λ(::Val{:Acsch}) = acsch; λ(::Val{:Asech}) = asech; λ(::Val{:Acoth}) = acoth
λ(::Val{:Gamma}) = gamma; λ(::Val{:Zeta}) = zeta; λ(::Val{:LambertW}) = lambertw
#==
Check if x represents an expression tree. If returns true, it will be assumed that operation(::T) and arguments(::T) methods are defined. Definining these three should allow use of SymbolicUtils.simplify on custom types. Optionally symtype(x) can be defined to return the expected type of the symbolic expression.
==#
function SymbolicUtils.istree(x::SymEngine.SymbolicType)
cls = SymEngine.get_symengine_class(x)
cls == :Symbol && return false
cls == :Constant && return false
any(==(cls), SymEngine.number_types) && return false
return true
end
SymbolicUtils.issym(x::SymEngine.SymbolicType) = SymEngine.get_symengine_class(x) == :Symbol
Base.nameof(x::SymEngine.SymbolicType) = Symbol(x)
# no metadata(x), metadata(x, data)
#==
Returns the head (a function object) performed by an expression tree. Called only if istree(::T) is true. Part of the API required for simplify to work. Other required methods are arguments and istree
==#
function SymbolicUtils.operation(x::SymEngine.SymbolicType)
istree(x) || error("$(typeof(x)) doesn't have an operation!")
return λ(x)
end
#==
Returns the arguments (a Vector) for an expression tree. Called only if istree(x) is true. Part of the API required for simplify to work. Other required methods are operation and istree
==#
function SymbolicUtils.arguments(x::SymEngine.SymbolicType)
get_args(x)
end
#==
Construct a new term with the operation f and arguments args, the term should be similar to t in type. if t is a SymbolicUtils.Term object a new Term is created with the same symtype as t. If not, the result is computed as f(args...). Defining this method for your term type will reduce any performance loss in performing f(args...) (esp. the splatting, and redundant type computation). T is the symtype of the output term. You can use SymbolicUtils.promote_symtype to infer this type. The exprhead keyword argument is useful when creating Exprs.
==#
function SymbolicUtils.similarterm(t::SymEngine.SymbolicType, f, args, symtype=nothing;
metadata=nothing, exprhead=:call)
f(args...) # default
end
# Needed for some simplification routines
# a total order <ₑ
import SymbolicUtils: <ₑ, isterm, isadd, ismul, issym, get_degrees, monomial_lt, _arglen
function SymbolicUtils.:<ₑ(a::SymEngine.Basic, b::SymEngine.Basic)
da, db = get_degrees(a), get_degrees(b)
fw = monomial_lt(da, db)
bw = monomial_lt(db, da)
if fw === bw && !isequal(a, b)
if _arglen(a) == _arglen(b)
return (operation(a), arguments(a)...,) <ₑ (operation(b), arguments(b)...,)
else
return _arglen(a) < _arglen(b)
end
else
return fw
end
end
end
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 986 | module SymEngine
using SymEngine_jll
import Base: show, convert, real, imag, MathConstants.γ, MathConstants.e, MathConstants.φ, MathConstants.catalan, invokelatest
import Compat: String, unsafe_string, @compat, denominator, numerator, Cvoid, Nothing, finalizer, reduce, mapreduce
import Serialization
import LinearAlgebra, Libdl
export Basic, symbols, @vars, @funs, SymFunction
export free_symbols, function_symbols, get_name, get_args
export coeff
export ascii_art
export subs, lambdify, N, cse
export series
export expand
include("utils.jl")
const have_mpfr = have_component("mpfr")
const have_mpc = have_component("mpc")
const libversion = get_libversion()
include("exceptions.jl")
include("types.jl")
include("ctypes.jl")
include("display.jl")
include("mathops.jl")
include("mathfuns.jl")
include("simplify.jl")
include("subs.jl")
include("numerics.jl")
include("calculus.jl")
include("recipes.jl")
include("dense-matrix.jl")
function __init__()
init_constants()
end
end
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 1854 | import Base: diff
## diff(ex, x) f'
## what is the rest of the interface. This does:
## diff(ex, x, n) f^(n)
## diff(ex, x, y, ...) f_{xy...} # also diff(ex, (x,y))
## no support for diff(ex, x,n1, y,n2, ...), but can do diff(ex, (x,y), (n1, n2))
function diff(b1::SymbolicType, b2::BasicType{Val{:Symbol}})
a = Basic()
ret = ccall((:basic_diff, libsymengine), Int, (Ref{Basic}, Ref{Basic}, Ref{Basic}), a, b1, b2)
return a
end
diff(b1::SymbolicType, b2::BasicType) =
throw(ArgumentError("Second argument must be of Symbol type"))
function diff(b1::SymbolicType, b2::SymbolicType, n::Integer=1)
n < 0 && throw(DomainError("n must be non-negative integer"))
n==0 && return b1
n==1 && return diff(b1, BasicType(b2))
n > 1 && return diff(diff(b1, BasicType(b2)), BasicType(b2), n-1)
end
function diff(b1::SymbolicType, b2::SymbolicType, b3::SymbolicType)
isa(BasicType(b3), BasicType{Val{:Integer}}) ? diff(b1, b2, N(b3)) : diff(b1, (b2, b3))
end
diff(b1::SymbolicType, b2::SymbolicType, b3::SymbolicType, b4::SymbolicType, b5...) =
diff(b1, (b2,b3,b4,b5...))
## mixed partials
diff(ex::SymbolicType, bs::Tuple) = reduce((ex, x) -> diff(ex, x), bs, init=ex)
diff(ex::SymbolicType, bs::Tuple, ns::Tuple) =
reduce((ex, x) -> diff(ex, x[1],x[2]), zip(bs, ns), init=ex)
diff(b1::SymbolicType, x::Union{String,Symbol}) = diff(b1, Basic(x))
"""
Series expansion to order `n` about point `x0`
"""
function series(ex::SymbolicType, x::SymbolicType, x0=0, n::Union{Integer, Basic}=6)
(!isa(N(n), Integer) || n < 0) && throw(DomainError("n must be non-negative integer"))
fc = subs(ex, x, x0)
n==0 && return fc
fp = ex
nfact = Basic(1)
for k in 1:n
fp = diff(fp, x)
nfact = k * nfact
fc = fc + subs(fp, x, x0) * (x-x0)^k / nfact
end
fc
end
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 4762 | # types from SymEngine to Julia
## CSetBasic
mutable struct CSetBasic
ptr::Ptr{Cvoid}
end
function CSetBasic()
z = CSetBasic(ccall((:setbasic_new, libsymengine), Ptr{Cvoid}, ()))
finalizer(CSetBasic_free, z)
z
end
function CSetBasic_free(x::CSetBasic)
if x.ptr != C_NULL
ccall((:setbasic_free, libsymengine), Nothing, (Ptr{Cvoid},), x.ptr)
x.ptr = C_NULL
end
end
function Base.length(s::CSetBasic)
ccall((:setbasic_size, libsymengine), UInt, (Ptr{Cvoid},), s.ptr)
end
function Base.getindex(s::CSetBasic, n::UInt)
result = Basic()
ccall((:setbasic_get, libsymengine), Nothing, (Ptr{Cvoid}, UInt, Ref{Basic}), s.ptr, n, result)
result
end
function Base.convert(::Type{Vector}, x::CSetBasic)
n = Base.length(x)
[x[i-1] for i in 1:n]
end
Base.convert(::Type{Set}, x::CSetBasic) = Set(convert(Vector, x))
## VecBasic Need this for get_args...
mutable struct CVecBasic
ptr::Ptr{Cvoid}
end
function CVecBasic()
z = CVecBasic(ccall((:vecbasic_new, libsymengine), Ptr{Cvoid}, ()))
finalizer(CVecBasic_free, z)
z
end
function CVecBasic_free(x::CVecBasic)
if x.ptr != C_NULL
ccall((:vecbasic_free, libsymengine), Nothing, (Ptr{Cvoid},), x.ptr)
x.ptr = C_NULL
end
end
function Base.length(s::CVecBasic)
ccall((:vecbasic_size, libsymengine), UInt, (Ptr{Cvoid},), s.ptr)
end
function Base.getindex(s::CVecBasic, n)
result = Basic()
ccall((:vecbasic_get, libsymengine), Nothing, (Ptr{Cvoid}, UInt, Ref{Basic}), s.ptr, UInt(n), result)
result
end
function Base.convert(::Type{Vector}, x::CVecBasic)
n = Base.length(x)
[x[i-1] for i in 1:n]
end
start(s::CVecBasic) = 0
done(s::CVecBasic, i) = (i == Base.length(s))
next(s::CVecBasic, i) = s[i], i+1
function Base.iterate(s::CVecBasic, i=start(s))
done(s, i) && return nothing
next(s, i)
end
## CMapBasicBasic
mutable struct CMapBasicBasic
ptr::Ptr{Cvoid}
end
function CMapBasicBasic()
z = CMapBasicBasic(ccall((:mapbasicbasic_new, libsymengine), Ptr{Cvoid}, ()))
finalizer(CMapBasicBasic_free, z)
z
end
function CMapBasicBasic(dict::AbstractDict)
c = CMapBasicBasic()
for (key, value) in dict
c[Basic(key)] = Basic(value)
end
return c
end
function CMapBasicBasic_free(x::CMapBasicBasic)
if x.ptr != C_NULL
ccall((:mapbasicbasic_free, libsymengine), Nothing, (Ptr{Cvoid},), x.ptr)
x.ptr = C_NULL
end
end
function Base.length(s::CMapBasicBasic)
ccall((:mapbasicbasic_size, libsymengine), UInt, (Ptr{Cvoid},), s.ptr)
end
function Base.getindex(s::CMapBasicBasic, k::Basic)
result = Basic()
ret = ccall((:mapbasicbasic_get, libsymengine), Cint, (Ptr{Cvoid}, Ref{Basic}, Ref{Basic}), s.ptr, k, result)
if ret == 0
throw(KeyError("Key not found"))
end
result
end
function Base.setindex!(s::CMapBasicBasic, v::Basic, k::Basic)
ccall((:mapbasicbasic_insert, libsymengine), Nothing, (Ptr{Cvoid}, Ref{Basic}, Ref{Basic}), s.ptr, k, v)
end
Base.convert(::Type{CMapBasicBasic}, x::AbstractDict) = CMapBasicBasic(x)
## Dense matrix
mutable struct CDenseMatrix <: DenseArray{Basic, 2}
ptr::Ptr{Cvoid}
end
Base.promote_rule(::Type{CDenseMatrix}, ::Type{Matrix{T}} ) where {T <: Basic} = CDenseMatrix
function CDenseMatrix_free(x::CDenseMatrix)
if x.ptr != C_NULL
ccall((:dense_matrix_free, libsymengine), Nothing, (Ptr{Cvoid},), x.ptr)
x.ptr = C_NULL
end
end
function CDenseMatrix()
z = CDenseMatrix(ccall((:dense_matrix_new, libsymengine), Ptr{Cvoid}, ()))
finalizer(CDenseMatrix_free, z)
z
end
function CDenseMatrix(m::Int, n::Int)
z = CDenseMatrix(ccall((:dense_matrix_new_rows_cols, libsymengine), Ptr{Cvoid}, (Int, Int), m, n))
finalizer(CDenseMatrix_free, z)
z
end
function CDenseMatrix(x::Array{T, 2}) where T
r,c = size(x)
M = CDenseMatrix(r, c)
for j in 1:c
for i in 1:r ## fill column by column
M[i,j] = x[i,j]
end
end
M
end
function Base.convert(::Type{Matrix}, x::CDenseMatrix)
m,n = Base.size(x)
[x[i,j] for i in 1:m, j in 1:n]
end
Base.convert(::Type{CDenseMatrix}, x::Array{T, 2}) where {T} = CDenseMatrix(x)
Base.convert(::Type{CDenseMatrix}, x::Array{T, 1}) where {T} = convert(CDenseMatrix, reshape(x, length(x), 1))
function toString(b::CDenseMatrix)
a = ccall((:dense_matrix_str, libsymengine), Cstring, (Ptr{Cvoid}, ), b.ptr)
string = unsafe_string(a)
ccall((:basic_str_free, libsymengine), Nothing, (Cstring, ), a)
string = replace(string, "**", "^") # de pythonify
return string
end
function Base.show(io::IO, m::CDenseMatrix)
r, c = size(m)
println(io, "CDenseMatrix: $r x $c")
println(io, toString(m))
end
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 1395 | using Pkg
Pkg.activate(".")
using SymEngine
struct CustomFunc
name::String
cfunc::Base.CFunction
func1
func2
end
struct CFunctionWrapperClass
name::Ptr{Cchar}
create_ptr::Ptr{Cvoid}
diff_ptr::Ptr{Cvoid}
end
function create_func(name, f)
function _create_func(a::Basic, args_ptr::Ptr{Cvoid})::Cint
args = SymEngine.CVecBasic(args_ptr)
try
res = f(convert(Vector, args))
catch e
res = nothing
end
if res == nothing
return 1
else
res = Basic(res)
ccall((:basic_assign, SymEngine.libsymengine), Nothing, (Ref{Basic}, Ref{Basic}), a, res)
return 0
end
end
create_ptr = @cfunction($_create_func, Cint, (Ref{Basic}, Ptr{Cvoid}))
return CustomFunc(name, create_ptr, f, _create_func)
end
function my_real(x::Vector{Basic})
return real(N(x[1]))
end
function my_norm(x::Vector{Basic})
a, b = N(x[1]), N(x[2])
return sqrt(a^2+b^2)
end
@vars x
function (c::CustomFunc)(args...)
v = convert(SymEngine.CVecBasic, args...)
a = Basic()
ccall((:basic_create_function_wrapper, SymEngine.libsymengine), Nothing, (Ref{Basic}, Ptr{Cvoid}, Cstring, Ptr{Cvoid}, Ptr{Cvoid}), a, v.ptr, pointer(c.name), c.cfunc.ptr, C_NULL)
return a
end
c = create_func("Re", my_real)
c(x)
d = create_func("Norm", my_norm)
d(2, 3)
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 6840 | ## Dense matrix interface
dense_matrix_rows(s::CDenseMatrix) = ccall((:dense_matrix_rows, libsymengine), Int, (Ptr{Cvoid}, ), s.ptr)
dense_matrix_cols(s::CDenseMatrix) = ccall((:dense_matrix_cols, libsymengine), Int, (Ptr{Cvoid}, ), s.ptr)
function dense_matrix_rows_cols(mat::CDenseMatrix, r::UInt, c::UInt)
ccall((:dense_matrix_rows_cols, libsymengine), Int, (Ptr{Cvoid}, UInt, UInt), s.ptr, r, c)
end
function dense_matrix_get_basic(s::CDenseMatrix, r::Int, c::Int)
result = Basic()
ccall((:dense_matrix_get_basic, libsymengine), Nothing, (Ref{Basic}, Ptr{Cvoid}, UInt, UInt), result, s.ptr, UInt(r), UInt(c))
result
end
function dense_matrix_set_basic(s::CDenseMatrix, val, r::Int, c::Int)
value = Basic(val)
ccall((:dense_matrix_set_basic, libsymengine), Nothing, (Ptr{Cvoid}, UInt, UInt, Ref{Basic}), s.ptr, UInt(r), UInt(c), value)
value
end
## Basic operations det, inv, transpose
function dense_matrix_det(s::CDenseMatrix)
result = Basic()
ccall((:dense_matrix_det, libsymengine), Nothing, (Ref{Basic}, Ptr{Cvoid}), result, s.ptr)
result
end
function dense_matrix_inv(s::CDenseMatrix)
result = CDenseMatrix()
ccall((:dense_matrix_inv, libsymengine), Nothing, (Ptr{Cvoid}, Ptr{Cvoid}), result.ptr, s.ptr)
result
end
function dense_matrix_transpose(s::CDenseMatrix)
result = CDenseMatrix()
ccall((:dense_matrix_transpose, libsymengine), Nothing, (Ptr{Cvoid}, Ptr{Cvoid}), result.ptr, s.ptr)
result
end
function dense_matrix_submatrix(mat::CDenseMatrix, r1::Int, c1::Int, r2::Int, c2::Int, r::Int, c::Int)
s = CDenseMatrix()
ccall((:dense_matrix_inv, libsymengine), Nothing, (Ptr{Cvoid}, Ptr{Cvoid}, UInt, UInt, UInt, UInt, UInt, UInt),
s.ptr, mat.ptr, UInt(r1), UInt(c1), UInt(r2), UInt(c2), UInt(r), UInt(c))
s
end
## some matrix arithmetic methods
## These are unncecessary, as +,-,*,^ are inhertied by the AbstractArray Interface. Those return Array{Basic,2}. These
## return CDenseMatrix objects.
function dense_matrix_add_matrix(a::CDenseMatrix, b::CDenseMatrix)
result = CDenseMatrix()
ccall((:dense_matrix_add_matrix, libsymengine), Nothing, (Ptr{Cvoid}, Ptr{Cvoid}, Ptr{Cvoid}), result.ptr, a.ptr, b.ptr)
result
end
function dense_matrix_mul_matrix(a::CDenseMatrix, b::CDenseMatrix)
result = CDenseMatrix()
ccall((:dense_matrix_mul_matrix, libsymengine), Nothing, (Ptr{Cvoid}, Ptr{Cvoid}, Ptr{Cvoid}), result.ptr, a.ptr, b.ptr)
result
end
function dense_matrix_add_scalar(a::CDenseMatrix, b::Basic)
result = CDenseMatrix()
ccall((:dense_matrix_add_scalar, libsymengine), Nothing, (Ptr{Cvoid}, Ptr{Cvoid}, Ptr{Cvoid}), result.ptr, a.ptr, b.ptr)
result
end
function dense_matrix_mul_scalar(a::CDenseMatrix, b::Basic)
result = CDenseMatrix()
ccall((:dense_matrix_mul_scalar, libsymengine), Nothing, (Ptr{Cvoid}, Ptr{Cvoid}, Ptr{Cvoid}), result.ptr, a.ptr, b.ptr)
result
end
## Factorizations ##################################################
function dense_matrix_LU(mat::CDenseMatrix)
## need square?
L = CDenseMatrix()
U = CDenseMatrix()
ccall((:dense_matrix_LU, libsymengine), Nothing, (Ptr{Cvoid}, Ptr{Cvoid}, Ptr{Cvoid}), L.ptr, U.ptr, mat.ptr)
(L, U)
end
## LDL decomposition
function dense_matrix_LDL(mat::CDenseMatrix)
L = CDenseMatrix()
D = CDenseMatrix()
ccall((:dense_matrix_LDL, libsymengine), Nothing, (Ptr{Cvoid}, Ptr{Cvoid}, Ptr{Cvoid}), L.ptr, D.ptr, mat.ptr)
(L, D)
end
## Fraction free LU factorization
function dense_matrix_FFLU(mat::CDenseMatrix)
LU = CDenseMatrix()
ccall((:dense_matrix_FFLU, libsymengine), Nothing, (Ptr{Cvoid}, Ptr{Cvoid}), LU.ptr, mat.ptr)
LU
end
## Fraction free LDU factorization
function dense_matrix_FFLDU(mat::CDenseMatrix)
L = CDenseMatrix()
D = CDenseMatrix()
U = CDenseMatrix()
ccall((:dense_matrix_FFLDU, libsymengine), Nothing, (Ptr{Cvoid}, Ptr{Cvoid}, Ptr{Cvoid}, Ptr{Cvoid}), L.ptr, D.ptr, U.ptr, mat.ptr)
(L, D, U)
end
function dense_matrix_LU_solve(A::CDenseMatrix, b::CDenseMatrix)
x = CDenseMatrix()
ccall((:dense_matrix_LU_solve, libsymengine), Nothing, (Ptr{Cvoid}, Ptr{Cvoid}, Ptr{Cvoid}), x.ptr, A.ptr, b.ptr)
x
end
function dense_matrix_zeros(::Type{CDenseMatrix}, r::Int, c::Int)
result = CDenseMatrix()
ccall((:dense_matrix_zeros, libsymengine), Nothing, (Ptr{Cvoid}, UInt, UInt), result.ptr, UInt(r), UInt(c))
result
end
function dense_matrix_ones(r::Int, c::Int)
result = CDenseMatrix()
ccall((:dense_matrix_ones, libsymengine), Nothing, (Ptr{Cvoid}, UInt, UInt), result.ptr, UInt(r), UInt(c))
result
end
## dense_matrix_diag XXX don't have CVecBasic constructor
function dense_matrix_eye(N::Int, M::Int, k::Int=0)
s = CDenseMatrix()
ccall((:dense_matrix_eye, libsymengine), Nothing, (Ptr{Cvoid}, UInt, UInt, Int), s.ptr, UInt(N), UInt(M), k)
s
end
dense_matrix_eq(a::CDenseMatrix, b::CDenseMatrix) = ccall((:dense_matrix_eq, libsymengine), Int, (Ptr{Cvoid},Ptr{Cvoid}), a.ptr, b.ptr)
## Plug into Julia's interface ##################################################
import Base: ==
==(a::CDenseMatrix, b::CDenseMatrix) = dense_matrix_eq(a, b) == 1
## Abstract Array Interface
Base.size(s::CDenseMatrix) = (dense_matrix_rows(s), dense_matrix_cols(s))
Base.getindex(s::CDenseMatrix, r::Int, c::Int) = dense_matrix_get_basic(s, r-1, c-1)
Base.setindex!(s::CDenseMatrix, val, r::Int, c::Int) = dense_matrix_set_basic(s, val, r-1, c-1)
## special matrices
Base.zeros(::Type{CDenseMatrix}, r::Int, c::Int) = dense_matrix_zeros(s, r-1, c-1)
Base.ones(::Type{CDenseMatrix}, r::Int, c::Int) = dense_matrix_ones(r-1, c-1)
## basic functions
LinearAlgebra.det(s::CDenseMatrix) = dense_matrix_det(s)
LinearAlgebra.det(s::Array{Basic,2}) = dense_matrix_det(CDenseMatrix(s))
Base.inv(s::CDenseMatrix) = dense_matrix_inv(s)
Base.inv(s::Array{Basic,2}) = Array{Basic,2}(dense_matrix_inv(CDenseMatrix(s)))
Base.transpose(s::CDenseMatrix) = dense_matrix_transpose(s)
Base.transpose(s::Array{Basic,2}) = Array{Basic,2}(dense_matrix_transpose(CDenseMatrix(s)))
LinearAlgebra.factorize(M::CDenseMatrix) = factorize(convert(Matrix, M))
"""
LU decomposition for CDenseMatrix, dense matrices of symbolic values
Also: lufact(a, val{:false}) for non-pivoting lu factorization
"""
function LinearAlgebra.lu(a::CDenseMatrix)
l, u = dense_matrix_LU(a)
L, U = convert(Matrix, l), convert(Matrix, u)
n = size(L, 1)
L[1:n+1:end] .-= one(eltype(L))
return LinearAlgebra.LU(L + U, collect(1:n), 0)
end
LinearAlgebra.lu(a::Array{Basic,2}) = LinearAlgebra.lu(CDenseMatrix(a))
# solve using LU_solve
import Base: \
\(A::CDenseMatrix, b::CDenseMatrix) = dense_matrix_LU_solve(A, b)
\(A::CDenseMatrix, b::Vector) = A \ convert(CDenseMatrix, [convert(Basic,u) for u in b])
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 645 |
function toString(b::SymbolicType)
b = Basic(b)
if b.ptr == C_NULL
error("Trying to print an uninitialized SymEngine Basic variable.")
end
a = ccall((:basic_str_julia, libsymengine), Cstring, (Ref{Basic}, ), b)
string = unsafe_string(a)
ccall((:basic_str_free, libsymengine), Nothing, (Cstring, ), a)
return string
end
Base.show(io::IO, b::SymbolicType) = print(io, toString(b))
" show symengine logo "
mutable struct AsciiArt x end
function ascii_art()
out = ccall((:ascii_art_str, libsymengine), Ptr{UInt8}, ())
AsciiArt(unsafe_string(out))
end
Base.show(io::IO, x::AsciiArt) = print(io, x.x)
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 659 | @inline function throw_if_error(error_code::Cuint, str=nothing)
if error_code == 0
return
else
throw(get_error(error_code, str))
end
end
function get_error(error_code::Cuint, str=nothing)
if error_code == 1
return ErrorException("Unknown SymEngine Exception")
elseif error_code == 2
return DivideError()
elseif error_code == 3
return ErrorException("Not implemented SymEngine feature")
elseif error_code == 4
return DomainError(str)
elseif error_code == 5
return Meta.ParseError(str)
else
return ErrorException("Unexpected SymEngine error code")
end
end
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 5181 | using SpecialFunctions
function IMPLEMENT_ONE_ARG_FUNC(meth, symnm; lib=:basic_)
@eval begin
function ($meth)(b::SymbolicType)
a = Basic()
err_code = ccall(($(string(lib,symnm)), libsymengine), Cuint, (Ref{Basic}, Ref{Basic}), a, b)
throw_if_error(err_code, $(string(meth)))
return a
end
end
end
function IMPLEMENT_TWO_ARG_FUNC(meth, symnm; lib=:basic_)
@eval begin
function ($meth)(b1::SymbolicType, b2::Number)
a = Basic()
b1, b2 = promote(b1, b2)
err_code = ccall(($(string(lib,symnm)), libsymengine), Cuint, (Ref{Basic}, Ref{Basic}, Ref{Basic}), a, b1, b2)
throw_if_error(err_code, $(string(meth)))
return a
end
end
end
## import from base one argument functions
## these are from cwrapper.cpp, one arg func
for (meth, libnm, modu) in [
(:abs,:abs,:Base),
(:sin,:sin,:Base),
(:cos,:cos,:Base),
(:tan,:tan,:Base),
(:csc,:csc,:Base),
(:sec,:sec,:Base),
(:cot,:cot,:Base),
(:asin,:asin,:Base),
(:acos,:acos,:Base),
(:asec,:asec,:Base),
(:acsc,:acsc,:Base),
(:atan,:atan,:Base),
(:acot,:acot,:Base),
(:sinh,:sinh,:Base),
(:cosh,:cosh,:Base),
(:tanh,:tanh,:Base),
(:csch,:csch,:Base),
(:sech,:sech,:Base),
(:coth,:coth,:Base),
(:asinh,:asinh,:Base),
(:acosh,:acosh,:Base),
(:asech,:asech,:Base),
(:acsch,:acsch,:Base),
(:atanh,:atanh,:Base),
(:acoth,:acoth,:Base),
(:gamma,:gamma,:SpecialFunctions),
(:log,:log,:Base),
(:sqrt,:sqrt,:Base),
(:exp,:exp,:Base),
(:eta,:dirichlet_eta,:SpecialFunctions),
(:zeta,:zeta,:SpecialFunctions),
]
eval(:(import $modu.$meth))
IMPLEMENT_ONE_ARG_FUNC(:($modu.$meth), libnm)
end
Base.abs2(x::SymEngine.Basic) = abs(x)^2
if get_symbol(:basic_atan2) != C_NULL
import Base.atan
IMPLEMENT_TWO_ARG_FUNC(:(Base.atan), :atan2)
end
# export not import
for (meth, libnm) in [
(:lambertw,:lambertw), # in add-on packages, not base
]
IMPLEMENT_ONE_ARG_FUNC(meth, libnm)
eval(Expr(:export, meth))
end
## add these in until they are wrapped
Base.cbrt(a::SymbolicType) = a^(1//3)
for (meth, fn) in [(:sind, :sin), (:cosd, :cos), (:tand, :tan), (:secd, :sec), (:cscd, :csc), (:cotd, :cot)]
eval(:(import Base.$meth))
@eval begin
$(meth)(a::SymbolicType) = $(fn)(a*PI/180)
end
end
## Number theory module from cppwrapper
for (meth, libnm) in [(:gcd, :gcd),
(:lcm, :lcm),
(:div, :quotient),
(:mod, :mod_f),
]
eval(:(import Base.$meth))
IMPLEMENT_TWO_ARG_FUNC(:(Base.$meth), libnm, lib=:ntheory_)
end
Base.binomial(n::Basic, k::Number) = binomial(N(n), N(k)) #ntheory_binomial seems wrong
Base.binomial(n::Basic, k::Integer) = binomial(N(n), N(k)) #Fix dispatch ambiguity / MethodError
Base.rem(a::SymbolicType, b::SymbolicType) = a - (a ÷ b) * b
Base.factorial(n::SymbolicType, k) = factorial(N(n), N(k))
## but not (:fibonacci,:fibonacci), (:lucas, :lucas) (Basic type is not the signature)
for (meth, libnm) in [(:nextprime,:nextprime)
]
IMPLEMENT_ONE_ARG_FUNC(meth, libnm, lib=:ntheory_)
eval(Expr(:export, meth))
end
function Base.convert(::Type{CVecBasic}, x::Vector{T}) where T
vec = CVecBasic()
for i in x
b::Basic = Basic(i)
ccall((:vecbasic_push_back, libsymengine), Nothing, (Ptr{Cvoid}, Ref{Basic}), vec.ptr, b)
end
return vec
end
Base.convert(::Type{CVecBasic}, x...) = Base.convert(CVecBasic, collect(promote(x...)))
Base.convert(::Type{CVecBasic}, x::CVecBasic) = x
mutable struct SymFunction
name::String
end
SymFunction(s::Symbol) = SymFunction(string(s))
function (f::SymFunction)(x::CVecBasic)
a = Basic()
ccall((:function_symbol_set, libsymengine), Nothing, (Ref{Basic}, Ptr{Int8}, Ptr{Cvoid}), a, f.name, x.ptr)
return a
end
(f::SymFunction)(x::Vector{T}) where {T} = (f::SymFunction)(convert(CVecBasic, x))
(f::SymFunction)(x...) = (f::SymFunction)(convert(CVecBasic, x...))
macro funs(x...)
q=Expr(:block)
if length(x) == 1 && isa(x[1],Expr)
@assert x[1].head === :tuple "@funs expected a list of symbols"
x = x[1].args
end
for s in x
@assert isa(s,Symbol) "@funs expected a list of symbols"
push!(q.args, Expr(:(=), esc(s), Expr(:call, :(SymEngine.SymFunction), Expr(:quote, s))))
end
push!(q.args, Expr(:tuple, map(esc, x)...))
q
end
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 4945 | import Base: +, -, ^, /, //, \, *, ==, sum, prod
## equality
function ==(b1::SymbolicType, b2::SymbolicType)
b1,b2 = map(Basic, (b1, b2))
ccall((:basic_eq, libsymengine), Int, (Ref{Basic}, Ref{Basic}), b1, b2) == 1
end
## main ops
for (op, libnm) in ((:+, :add), (:-, :sub), (:*, :mul), (:/, :div), (://, :div), (:^, :pow))
tup = (Base.Symbol("basic_$libnm"), libsymengine)
@eval begin
function ($op)(b1::Basic, b2::Basic)
a = Basic()
err_code = ccall($tup, Cuint, (Ref{Basic}, Ref{Basic}, Ref{Basic}), a, b1, b2)
throw_if_error(err_code, $(string(libnm)))
return a
end
($op)(b1::BasicType, b2::BasicType) = ($op)(Basic(b1), Basic(b2))
end
end
^(a::T, b::S) where {T<:SymbolicType, S <: Integer} = Basic(a)^Basic(b)
^(a::T, b::S) where {T<:SymbolicType, S <: Rational} = Basic(a)^Basic(b)
+(b::SymbolicType) = b
-(b::SymbolicType) = 0 - b
\(b1::SymbolicType, b2::SymbolicType) = b2 / b1
# In contrast to other standard operations such as `+`, `*`, `-`, and `/`,
# Julia doesn't implement a general fallback of `//` for `Number`s promoting
# the input arguments. Thus, we implement this here explicitly.
Base.:(//)(b1::SymbolicType, b2::Number) = //(promote(b1, b2)...)
Base.:(//)(b1::Number, b2::SymbolicType) = //(promote(b1, b2)...)
function sum(v::CVecBasic)
a = Basic()
err_code = ccall((:basic_add_vec, libsymengine), Cuint, (Ref{Basic}, Ptr{Cvoid}), a, v.ptr)
throw_if_error(err_code, "add_vec")
return a
end
+(b1::Basic, b2::Basic, b3::Basic, bs...) = sum(convert(CVecBasic, [b1, b2, b3, bs...]))
+(b1::Basic, b2::Basic, b3, bs...) = +(Basic(b1), Basic(b2), Basic(b3), bs...)
+(b1, b2::Basic, b3::Basic, bs...) = +(Basic(b1), Basic(b2), Basic(b3), bs...)
+(b1::Basic, b2, b3::Basic, bs...) = +(Basic(b1), Basic(b2), Basic(b3), bs...)
+(b1::Basic, b2, b3, bs...) = +(Basic(b1), Basic(b2), Basic(b3), bs...)
+(b1, b2::Basic, b3, bs...) = +(Basic(b1), Basic(b2), Basic(b3), bs...)
+(b1, b2, b3::Basic, bs...) = +(Basic(b1), Basic(b2), Basic(b3), bs...)
function prod(v::CVecBasic)
a = Basic()
err_code = ccall((:basic_mul_vec, libsymengine), Cuint, (Ref{Basic}, Ptr{Cvoid}), a, v.ptr)
throw_if_error(err_code, "mul_vec")
return a
end
*(b1::Basic, b2::Basic, b3::Basic, bs::Vararg{Number, N}) where {N} = prod(convert(CVecBasic, [b1, b2, b3, bs...]))
*(b1::Basic, b2::Basic, b3::Number, bs::Vararg{Number, N}) where {N} = *(Basic(b1), Basic(b2), Basic(b3), bs...)
*(b1::Number, b2::Basic, b3::Basic, bs::Vararg{Number, N}) where {N} = *(Basic(b1), Basic(b2), Basic(b3), bs...)
*(b1::Basic, b2::Number, b3::Basic, bs::Vararg{Number, N}) where {N} = *(Basic(b1), Basic(b2), Basic(b3), bs...)
*(b1::Basic, b2::Number, b3::Number, bs::Vararg{Number, N}) where {N} = *(Basic(b1), Basic(b2), Basic(b3), bs...)
*(b1::Number, b2::Basic, b3::Number, bs::Vararg{Number, N}) where {N} = *(Basic(b1), Basic(b2), Basic(b3), bs...)
*(b1::Number, b2::Number, b3::Basic, bs::Vararg{Number, N}) where {N} = *(Basic(b1), Basic(b2), Basic(b3), bs...)
## ## constants
Base.zero(x::Basic) = Basic(0)
Base.zero(::Type{T}) where {T<:Basic} = Basic(0)
Base.one(x::Basic) = Basic(1)
Base.one(::Type{T}) where {T<:Basic} = Basic(1)
Base.zero(x::BasicType) = BasicType(Basic(0))
Base.zero(::Type{T}) where {T<:BasicType} = BasicType(Basic(0))
Base.one(x::BasicType) = BasicType(Basic(1))
Base.one(::Type{T}) where {T<:BasicType} = BasicType(Basic(1))
## Math constants
## no oo!
for op in [:IM, :PI, :E, :EulerGamma, :Catalan, :oo, :zoo, :NAN]
@eval begin
const $op = Basic(C_NULL)
end
eval(Expr(:export, op))
end
macro init_constant(op, libnm)
tup = (Base.Symbol("basic_const_$libnm"), libsymengine)
alloc_tup = (:basic_new_stack, libsymengine)
:(
begin
ccall($alloc_tup, Nothing, (Ref{Basic}, ), $op)
ccall($tup, Nothing, (Ref{Basic}, ), $op)
finalizer(basic_free, $op)
end
)
end
function init_constants()
@init_constant IM I
@init_constant PI pi
@init_constant E E
@init_constant EulerGamma EulerGamma
@init_constant Catalan Catalan
@init_constant oo infinity
@init_constant zoo complex_infinity
@init_constant NAN nan
end
## ## Conversions
Base.convert(::Type{Basic}, x::Irrational{:π}) = PI
Base.convert(::Type{Basic}, x::Irrational{:e}) = E
Base.convert(::Type{Basic}, x::Irrational{:γ}) = EulerGamma
Base.convert(::Type{Basic}, x::Irrational{:catalan}) = Catalan
Base.convert(::Type{Basic}, x::Irrational{:φ}) = (1 + Basic(5)^Basic(1//2))/2
Base.convert(::Type{BasicType}, x::Irrational) = BasicType(convert(Basic, x))
## Logical operators
Base.:<(x::SymbolicType, y::SymbolicType) = N(x) < N(y)
Base.:<(x::SymbolicType, y) = <(promote(x,y)...)
Base.:<(x, y::SymbolicType) = <(promote(x,y)...)
## Other Basic Operations
Base.copysign(x::SymEngine.Basic,y::SymEngine.BasicType) = sign(y)*abs(x)
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 7994 | import Base: convert, real, imag, float, eps, conj
import Base: isfinite, isnan, isinf, isless
import Base: trunc, ceil, floor, round
function evalf(b::Basic, bits::Integer=53, real::Bool=false)
c = Basic()
bits > 53 && real && (have_mpfr || throw(ArgumentError("libsymengine has to be compiled with MPFR for this feature")))
bits > 53 && !real && (have_mpc || throw(ArgumentError("libsymengine has to be compiled with MPC for this feature")))
status = ccall((:basic_evalf, libsymengine), Cint, (Ref{Basic}, Ref{Basic}, Culong, Cint), c, b, Culong(bits), Int(real))
if status == 0
return c
else
throw(ArgumentError("symbolic value cannot be evaluated to a numeric value"))
end
end
## Conversions from SymEngine -> Julia at the ccall level
function convert(::Type{BigInt}, b::BasicType{Val{:Integer}})
a = BigInt()
c = Basic(b)
ccall((:integer_get_mpz, libsymengine), Nothing, (Ref{BigInt}, Ref{Basic}), a, c)
return a
end
function convert(::Type{BigFloat}, b::BasicType{Val{:RealMPFR}})
c = Basic(b)
a = BigFloat()
ccall((:real_mpfr_get, libsymengine), Nothing, (Ref{BigFloat}, Ref{Basic}), a, c)
return a
end
function convert(::Type{Cdouble}, b::BasicType{Val{:RealDouble}})
c = Basic(b)
return ccall((:real_double_get_d, libsymengine), Cdouble, (Ref{Basic},), c)
end
if SymEngine.libversion >= VersionNumber("0.4.0")
function real(b::BasicComplexNumber)
c = Basic(b)
a = Basic()
ccall((:complex_base_real_part, libsymengine), Nothing, (Ref{Basic}, Ref{Basic}), a, c)
return a
end
function imag(b::BasicComplexNumber)
c = Basic(b)
a = Basic()
ccall((:complex_base_imaginary_part, libsymengine), Nothing, (Ref{Basic}, Ref{Basic}), a, c)
return a
end
else
function real(b::BasicType{Val{:ComplexDouble}})
c = Basic(b)
a = Basic()
ccall((:complex_double_real_part, libsymengine), Nothing, (Ref{Basic}, Ref{Basic}), a, c)
return a
end
function imag(b::BasicType{Val{:ComplexDouble}})
c = Basic(b)
a = Basic()
ccall((:complex_double_imaginary_part, libsymengine), Nothing, (Ref{Basic}, Ref{Basic}), a, c)
return a
end
function real(b::BasicType{Val{:Complex}})
c = Basic(b)
a = Basic()
ccall((:complex_real_part, libsymengine), Nothing, (Ref{Basic}, Ref{Basic}), a, c)
return a
end
function imag(b::BasicType{Val{:Complex}})
c = Basic(b)
a = Basic()
ccall((:complex_imaginary_part, libsymengine), Nothing, (Ref{Basic}, Ref{Basic}), a, c)
return a
end
function real(b::BasicType{Val{:ComplexMPC}})
c = Basic(b)
a = Basic()
ccall((:complex_mpc_real_part, libsymengine), Nothing, (Ref{Basic}, Ref{Basic}), a, c)
return a
end
function imag(b::BasicType{Val{:ComplexMPC}})
c = Basic(b)
a = Basic()
ccall((:complex_mpc_imaginary_part, libsymengine), Nothing, (Ref{Basic}, Ref{Basic}), a, c)
return a
end
end
##################################################
# N
"""
Convert a SymEngine numeric value into a Julian number
"""
N(a::Integer) = a
N(a::Rational) = a
N(a::Complex) = a
N(b::Basic) = N(BasicType(b))
function N(b::BasicType{Val{:Integer}})
a = convert(BigInt, b)
if (a.size > 1 || a.size < -1)
return a
elseif (a.size == 0)
return 0
else
u = unsafe_load(a.d, 1)
w = signed(u)
if w > 0
return w * a.size
elseif a.size == 1
return u
else
return a
end
end
end
N(b::BasicType{Val{:Rational}}) = Rational(N(numerator(b)), N(denominator(b))) # TODO: conditionally wrap rational_get_mpq from cwrapper.h
N(b::BasicType{Val{:RealDouble}}) = convert(Cdouble, b)
N(b::BasicType{Val{:RealMPFR}}) = convert(BigFloat, b)
N(b::BasicType{Val{:NaN}}) = NaN
N(b::BasicType{Val{:Infty}}) = (string(b) == "-inf") ? -Inf : Inf
## Mapping of SymEngine Constants into julia values
constant_map = Dict("pi" => π, "eulergamma" => γ, "exp(1)" => e, "catalan" => catalan,
"goldenratio" => φ)
N(b::BasicType{Val{:Constant}}) = constant_map[toString(b)]
N(b::BasicComplexNumber) = complex(N(real(b)), N(imag(b)))
function N(b::BasicType)
b = convert(Basic, b)
fs = free_symbols(b)
if length(fs) > 0
throw(ArgumentError("Object can have no free symbols"))
end
out = evalf(b)
imag(out) == Basic(0.0) ? real(out) : out
end
## Conversions SymEngine -> Julia
function as_numer_denom(x::Basic)
a, b = Basic(), Basic()
ccall((:basic_as_numer_denom, libsymengine), Nothing, (Ref{Basic}, Ref{Basic}, Ref{Basic}), a, b, x)
return a, b
end
as_numer_denom(x::BasicType) = as_numer_denom(Basic(x))
denominator(x::SymbolicType) = as_numer_denom(x)[2]
numerator(x::SymbolicType) = as_numer_denom(x)[1]
## Complex
real(x::Basic) = Basic(real(SymEngine.BasicType(x)))
real(x::SymEngine.BasicType) = x
imag(x::Basic) = Basic(imag(SymEngine.BasicType(x)))
imag(x::BasicType{Val{:Integer}}) = Basic(0)
imag(x::BasicType{Val{:RealDouble}}) = Basic(0)
imag(x::BasicType{Val{:RealMPFR}}) = Basic(0)
imag(x::BasicType{Val{:Rational}}) = Basic(0)
imag(x::SymEngine.BasicType) = throw(InexactError())
# Because of the definitions above, `real(x) == x` for `x::Basic`
# such as `x = symbols("x")`. Thus, it is consistent to define the
conj(x::Basic) = Basic(conj(SymEngine.BasicType(x)))
# To allow future extension, we define the fallback on `BasicType``.
conj(x::BasicType) = 2 * real(x.x) - x.x
## define convert(T, x) methods leveraging N()
convert(::Type{Float64}, x::Basic) = convert(Float64, N(evalf(x, 53, true)))
convert(::Type{BigFloat}, x::Basic) = convert(BigFloat, N(evalf(x, precision(BigFloat), true)))
convert(::Type{Complex{Float64}}, x::Basic) = convert(Complex{Float64}, N(evalf(x, 53, false)))
convert(::Type{Complex{BigFloat}}, x::Basic) = convert(Complex{BigFloat}, N(evalf(x, precision(BigFloat), false)))
convert(::Type{Number}, x::Basic) = x
convert(::Type{T}, x::Basic) where {T <: Real} = convert(T, N(x))
convert(::Type{Complex{T}}, x::Basic) where {T <: Real} = convert(Complex{T}, N(x))
# Constructors no longer fall back to `convert` methods
Base.Int64(x::Basic) = convert(Int64, x)
Base.Int32(x::Basic) = convert(Int32, x)
Base.Float32(x::Basic) = convert(Float32, x)
Base.Float64(x::Basic) = convert(Float64, x)
Base.BigInt(x::Basic) = convert(BigInt, x)
Base.Real(x::Basic) = convert(Real, x)
## For generic programming in Julia
float(x::Basic) = float(N(x))
# trunc, flooor, ceil, round, rem, mod, cld, fld,
isfinite(x::Basic) = x-x == 0
isnan(x::Basic) = ( x == NAN )
isinf(x::Basic) = !isnan(x) & !isfinite(x)
isless(x::Basic, y::Basic) = isless(N(x), N(y))
## These should have support in symengine-wrapper, but currently don't
trunc(x::Basic, args...) = Basic(trunc(N(x), args...))
trunc(::Type{T},x::Basic, args...) where {T <: Integer} = convert(T, trunc(x,args...))
ceil(x::Basic) = Basic(ceil(N(x)))
ceil(::Type{T},x::Basic) where {T <: Integer} = convert(T, ceil(x))
floor(x::Basic) = Basic(floor(N(x)))
floor(::Type{T},x::Basic) where {T <: Integer} = convert(T, floor(x))
round(x::Basic; kwargs...) = Basic(round(N(x); kwargs...))
round(::Type{T},x::Basic; kwargs...) where {T <: Integer} = convert(T, round(x; kwargs...))
prec(x::BasicType{Val{:RealMPFR}}) = ccall((:real_mpfr_get_prec, libsymengine), Clong, (Ref{Basic},), x)
# eps
eps(x::Basic) = eps(BasicType(x))
eps(x::BasicType{T}) where {T} = eps(typeof(x))
eps(::Type{T}) where {T <: BasicType} = 0
eps(::Type{T}) where {T <: Basic} = 0
eps(::Type{BasicType{Val{:RealDouble}}}) = 2^-52
eps(::Type{BasicType{Val{:ComplexDouble}}}) = 2^-52
eps(x::BasicType{Val{:RealMPFR}}) = evalf(Basic(2), prec(x), true) ^ (-prec(x)+1)
eps(x::BasicType{Val{:ComplexMPFR}}) = eps(real(x))
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 255 | using RecipesBase
## Plug into RecipesBase so plots of expressions treated like plots of functions
@recipe f(::Type{T}, v::T) where {T<:Basic} = lambdify(v)
@recipe f(::Type{S}, ss::S) where {S<:AbstractVector{Basic}} = Function[lambdify(s) for s in ss]
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 604 | IMPLEMENT_ONE_ARG_FUNC(:expand, :expand)
if get_symbol(:basic_cse) != C_NULL
function cse(exprs...)
vec = convert(CVecBasic, exprs...)
replacement_syms = CVecBasic()
replacement_exprs = CVecBasic()
reduced_exprs = CVecBasic()
ccall((:basic_cse, libsymengine), Nothing, (Ptr{Cvoid},Ptr{Cvoid},Ptr{Cvoid},Ptr{Cvoid}),
replacement_syms.ptr, replacement_exprs.ptr, reduced_exprs.ptr,vec.ptr)
return replacement_syms, replacement_exprs, reduced_exprs
end
else
function cse(exprs...)
error("libsymengine is too old")
end
end
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 4582 |
"""
subs
Substitute values into a symbolic expression.
Examples
```
@vars x y
ex = x^2 + y^2
subs(ex, x, 1) # 1 + y^2
subs(ex, (x, 1)) # 1 + y^2
subs(ex, (x, 1), (y,x)) # 1 + x^2, values are substituted left to right.
subs(ex, x=>1) # alternate to subs(x, (x,1))
subs(ex, x=>1, y=>1) # ditto
```
"""
function subs(ex::T, var::S, val) where {T<:SymbolicType, S<:SymbolicType}
s = Basic()
err_code = ccall((:basic_subs2, libsymengine), Cuint, (Ref{Basic}, Ref{Basic}, Ref{Basic}, Ref{Basic}), s, ex, var, val)
throw_if_error(err_code, ex)
return s
end
function subs(ex::T, d::CMapBasicBasic) where T<:SymbolicType
s = Basic()
err_code = ccall((:basic_subs, libsymengine), Cuint, (Ref{Basic}, Ref{Basic}, Ptr{Cvoid}), s, ex, d.ptr)
throw_if_error(err_code, ex)
return s
end
subs(ex::T, d::AbstractDict) where {T<:SymbolicType} = subs(ex, CMapBasicBasic(d))
subs(ex::T, y::Tuple{S, Any}) where {T <: SymbolicType, S<:SymbolicType} = subs(ex, y[1], y[2])
subs(ex::T, y::Tuple{S, Any}, args...) where {T <: SymbolicType, S<:SymbolicType} = subs(subs(ex, y), args...)
subs(ex::T, d::Pair...) where {T <: SymbolicType} = subs(ex, [(p.first, p.second) for p in d]...)
## Allow an expression to be called, as with ex(2). When there is more than one symbol, one can rely on order of `free_symbols` or
## be explicit by passing in pairs : `ex(x=>1, y=>2)` or a dict `ex(Dict(x=>1, y=>2))`.
function (ex::Basic)(args...)
xs = free_symbols(ex)
subs(ex, collect(zip(xs, args))...)
end
(ex::Basic)(x::AbstractDict) = subs(ex, x)
(ex::Basic)(x::Pair...) = subs(ex, x...)
## Lambdify
## Map symengine classes to function names
fn_map = Dict(
:Add => :+,
:Sub => :-,
:Mul => :*,
:Div => :/,
:Pow => :^,
:re => :real,
:im => :imag,
:Abs => :abs # not really needed as default in map_fn covers this
)
map_fn(key, fn_map) = haskey(fn_map, key) ? fn_map[key] : Symbol(lowercase(string(key)))
function _convert(::Type{Expr}, ex::Basic)
fn = get_symengine_class(ex)
if fn == :Symbol
return Symbol(toString(ex))
elseif (fn in number_types) || (fn == :Constant)
return N(ex)
end
as = get_args(ex)
Expr(:call, map_fn(fn, fn_map), [_convert(Expr,a) for a in as]...)
end
function convert(::Type{Expr}, ex::Basic)
fn = get_symengine_class(ex)
if fn == :Symbol
return Expr(:call, :*, Symbol(toString(ex)), 1)
elseif (fn in number_types) || (fn == :Constant)
return Expr(:call, :*, N(ex), 1)
end
return _convert(Expr, ex)
end
function convert(::Type{Expr}, m::AbstractArray{Basic, 2})
col_args = []
for i = 1:size(m, 1)
row_args = []
for j = 1:size(m, 2)
push!(row_args, convert(Expr, m[i, j]))
end
row = Expr(:hcat, row_args...)
push!(col_args, row)
end
Expr(:vcat, col_args...)
end
function convert(::Type{Expr}, m::AbstractArray{Basic, 1})
row_args = []
for j = 1:size(m, 1)
push!(row_args, convert(Expr, m[j]))
end
Expr(:vcat, row_args...)
end
walk_expression(b) = convert(Expr, b)
"""
lambdify
evaluates a symbolless expression or returns a function
"""
function lambdify(ex, vars=[])
if length(vars) == 0
vars = free_symbols(ex)
end
body = convert(Expr, ex)
lambdify(body, vars)
end
function lambdify(ex::Basic, vars=[]; cse=false)
if length(vars) == 0
vars = free_symbols(ex)
end
if !cse
body = convert(Expr, ex)
return lambdify(body, vars)
end
replace_syms, replace_exprs, new_exprs = SymEngine.cse(ex)
body_args = []
for (i, j) in zip(replace_syms, replace_exprs)
append!(body_args, [Expr(:(=), Symbol(toString(i)), convert(Expr, j))])
end
append!(body_args, [convert(Expr, new_exprs[0])])
body = Expr(:block, body_args...)
lambdify(body, vars)
end
lambdify(ex::BasicType, vars=[]) = lambdify(Basic(ex), vars)
function lambdify(ex::Expr, vars)
if length(vars) == 0
# return a number
eval(ex)
else
# return a function
_lambdify(ex, vars)
end
end
function _lambdify(ex::Expr, vars)
try
fn = eval(Expr(:function,
Expr(:call, gensym(), map(Symbol,vars)...),
ex))
(args...) -> invokelatest(fn, args...) # https://github.com/JuliaLang/julia/pull/19784
catch err
throw(ArgumentError("Expression does not lambdify"))
end
end
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 10456 | ## We have different types:
##
## Basic: holds a ptr to a symengine object. Faster, so is default type
##
## BasicType{Val{:XXX}}: types that can be use to control dispatch
##
## SymbolicType: is a type union of the two
##
## Basic(x::BasicType) gives a basic object; BasicType(x::Basic) gives a BasicType object. (This name needs change)
##
## To control dispatch, one might have `N(b::Basic) = N(BasicType(b))` and then define `N` for types of interest
## Hold a reference to a SymEngine object
mutable struct Basic <: Number
ptr::Ptr{Cvoid}
function Basic()
z = new(C_NULL)
ccall((:basic_new_stack, libsymengine), Nothing, (Ref{Basic}, ), z)
finalizer(basic_free, z)
return z
end
function Basic(v::Ptr{Cvoid})
z = new(v)
return z
end
end
basic_free(b::Basic) = ccall((:basic_free_stack, libsymengine), Nothing, (Ref{Basic}, ), b)
function convert(::Type{Basic}, x::Clong)
a = Basic()
ccall((:integer_set_si, libsymengine), Nothing, (Ref{Basic}, Clong), a, x)
return a
end
function convert(::Type{Basic}, x::Culong)
a = Basic()
ccall((:integer_set_ui, libsymengine), Nothing, (Ref{Basic}, Culong), a, x)
return a
end
function convert(::Type{Basic}, x::BigInt)
a = Basic()
ccall((:integer_set_mpz, libsymengine), Nothing, (Ref{Basic}, Ref{BigInt}), a, x)
return a
end
function convert(::Type{Basic}, s::String)
a = Basic()
b = ccall((:basic_parse, libsymengine), Cuint, (Ref{Basic}, Ptr{Int8}), a, s)
throw_if_error(b, s)
return a
end
function convert(::Type{Basic}, ex::Expr)
expr = copy(ex)
Basic(string(expr))
end
convert(::Type{Basic}, ex::Symbol) = Basic(string(ex))
function convert(::Type{Basic}, x::Cdouble)
a = Basic()
ccall((:real_double_set_d, libsymengine), Nothing, (Ref{Basic}, Cdouble), a, x)
return a
end
function convert(::Type{Basic}, x::BigFloat)
if (x.prec <= 53)
return convert(Basic, Cdouble(x))
elseif have_mpfr
a = Basic()
ccall((:real_mpfr_set, libsymengine), Nothing, (Ref{Basic}, Ref{BigFloat}), a, x)
return a
else
warn("SymEngine is not compiled with MPFR support. Converting will lose precision.")
return convert(Basic, Cdouble(x))
end
end
if Clong == Int32
convert(::Type{Basic}, x::Union{Int8, Int16}) = Basic(convert(Clong, x))
convert(::Type{Basic}, x::Union{UInt8, UInt16}) = Basic(convert(Culong, x))
else
convert(::Type{Basic}, x::Union{Int8, Int16, Int32}) = Basic(convert(Clong, x))
convert(::Type{Basic}, x::Union{UInt8, UInt16, UInt32}) = Basic(convert(Culong, x))
end
convert(::Type{Basic}, x::Union{Float16, Float32}) = Basic(convert(Cdouble, x))
convert(::Type{Basic}, x::Integer) = Basic(BigInt(x))
convert(::Type{Basic}, x::Rational) = Basic(numerator(x)) / Basic(denominator(x))
convert(::Type{Basic}, x::Complex) = Basic(real(x)) + Basic(imag(x)) * IM
Basic(x::T) where {T} = convert(Basic, x)
Basic(x::Basic) = x
Base.promote_rule(::Type{Basic}, ::Type{S}) where {S<:Number} = Basic
Base.promote_rule(::Type{S}, ::Type{Basic}) where {S<:Number} = Basic
Base.promote_rule(::Type{S}, ::Type{Basic}) where {S<:AbstractIrrational} = Basic
## Class ID
get_type(s::Basic) = ccall((:basic_get_type, libsymengine), UInt, (Ref{Basic},), s)
function get_class_from_id(id::UInt)
a = ccall((:basic_get_class_from_id, libsymengine), Ptr{UInt8}, (Int,), id)
str = unsafe_string(a)
ccall((:basic_str_free, libsymengine), Nothing, (Ptr{UInt8}, ), a)
str
end
"Get SymEngine class of an object (e.g. 1=>:Integer, 1//2 =:Rational, sin(x) => :Sin, ..."
get_symengine_class(s::Basic) = Symbol(get_class_from_id(get_type(s)))
## Construct symbolic objects
## renamed, as `Symbol` conflicts with Base.Symbol
function _symbol(s::String)
a = Basic()
ccall((:symbol_set, libsymengine), Nothing, (Ref{Basic}, Ptr{Int8}), a, s)
return a
end
_symbol(s::Symbol) = _symbol(string(s))
## use SymPy name here, but no assumptions
"""
`symbols(::Symbol)` construct symbolic value
Examples:
```
a = symbols(:a)
x = symbols("x")
x,y = symbols("x y")
x,y,z = symbols("x,y,z")
```
"""
symbols(s::Symbol) = _symbol(s)
function symbols(s::String)
## handle space or comma sparation
s = replace(s, ","=> " ")
by_space = split(s, r"\s+")
Base.length(by_space) == 1 && return symbols(Symbol(s))
tuple([_symbol(Symbol(o)) for o in by_space]...)
end
## Follow, somewhat, the python names: symbols to construct symbols, @vars
"""
Macro to define 1 or more variables in the main workspace.
Symbolic values are defined with `_symbol`. This is a convenience
Example
```
@vars x y z
```
"""
macro vars(x...)
q=Expr(:block)
if length(x) == 1 && isa(x[1],Expr)
@assert x[1].head === :tuple "@syms expected a list of symbols"
x = x[1].args
end
for s in x
@assert isa(s,Symbol) "@syms expected a list of symbols"
push!(q.args, Expr(:(=), esc(s), Expr(:call, :(SymEngine._symbol), Expr(:quote, s))))
end
push!(q.args, Expr(:tuple, map(esc, x)...))
q
end
## We also have a wrapper type that can be used to control dispatch
## pros: wrapping adds overhead, so if possible best to use Basic
## cons: have to write methods meth(x::Basic, ...) = meth(BasicType(x),...)
## Parameterized type allowing for dispatch on Julia side by type of objecton SymEngine side
## Use as BasicType{Val{:Integer}}(...)
## To take advantage of this, define
## meth(x::Basic) = meth(BasicType(x))
## and then
## meth(x::BasicType{Val{:Integer}}) = ... or
## meth(x::BasicNumber) = ...
mutable struct BasicType{T} <: Number
x::Basic
end
convert(::Type{Basic}, x::Basic) = x
SymbolicType = Union{Basic, BasicType}
convert(::Type{Basic}, x::BasicType) = x.x
Basic(x::BasicType) = x.x
BasicType(val::Basic) = BasicType{Val{get_symengine_class(val)}}(val)
convert(::Type{BasicType{T}}, val::Basic) where {T} = BasicType{Val{get_symengine_class(val)}}(val)
# Needed for julia v0.4.7
convert(::Type{T}, x::Basic) where {T<:BasicType} = BasicType(x)
## We have Basic and BasicType{...}. We go back and forth with:
## Basic(b::BasicType) and BasicType(b::Basic)
# for mathops
Base.promote_rule(::Type{T}, ::Type{S} ) where {T<:BasicType, S<:Number} = T
Base.promote_rule(::Type{S}, ::Type{T} ) where {T<:BasicType, S<:Number} = T
# to intersperse BasicType and Basic in math ops
Base.promote_rule(::Type{T}, ::Type{Basic} ) where {T<:BasicType} = T
Base.promote_rule( ::Type{Basic}, ::Type{T} ) where {T<:BasicType} = T
Base.promote_rule(::Type{S}, ::Type{T}) where {S<:AbstractIrrational, T<:BasicType} = T
## needed for mathops
convert(::Type{T}, val::Number) where {T<:BasicType} = T(Basic(val))
## Julia v0.6 errors with ambiguous error if this method is not defined.
convert(::Type{T}, val::T) where {T<:BasicType} = val
## some type unions possibly useful for dispatch
## Names here match those returned by get_symengine_class()
real_number_types = [:Integer, :RealDouble, :Rational, :RealMPFR]
complex_number_types = [:Complex, :ComplexDouble, :ComplexMPC]
number_types = vcat(real_number_types, complex_number_types)
BasicNumber = Union{[SymEngine.BasicType{Val{i}} for i in number_types]...}
BasicRealNumber = Union{[SymEngine.BasicType{Val{i}} for i in real_number_types]...}
BasicComplexNumber = Union{[SymEngine.BasicType{Val{i}} for i in complex_number_types]...}
op_types = [:Mul, :Add, :Pow, :Symbol, :Const]
BasicOp = Union{[SymEngine.BasicType{Val{i}} for i in op_types]...}
trig_types = [:Sin, :Cos, :Tan, :Csc, :Sec, :Cot, :ASin, :ACos, :ATan, :ACsc, :ASec, :ACot]
BasicTrigFunction = Union{[SymEngine.BasicType{Val{i}} for i in trig_types]...}
###
" Return free symbols in an expression as a `Set`"
function free_symbols(ex::Basic)
syms = CSetBasic()
ccall((:basic_free_symbols, libsymengine), Nothing, (Ref{Basic}, Ptr{Cvoid}), ex, syms.ptr)
convert(Vector, syms)
end
free_symbols(ex::BasicType) = free_symbols(Basic(ex))
_flat(A) = mapreduce(x->isa(x,Array) ? _flat(x) : x, vcat, A, init=Basic[]) # from rosetta code example
free_symbols(exs::Array{T}) where {T<:SymbolicType} = unique(_flat([free_symbols(ex) for ex in exs]))
free_symbols(exs::Tuple) = unique(_flat([free_symbols(ex) for ex in exs]))
"Return function symbols in an expression as a `Set`"
function function_symbols(ex::Basic)
syms = CSetBasic()
ccall((:basic_function_symbols, libsymengine), Nothing, (Ptr{Cvoid}, Ref{Basic}), syms.ptr, ex)
convert(Vector, syms)
end
function_symbols(ex::BasicType) = function_symbols(Basic(ex))
function_symbols(exs::Array{T}) where {T<:SymbolicType} = unique(_flat([function_symbols(ex) for ex in exs]))
function_symbols(exs::Tuple) = unique(_flat([function_symbols(ex) for ex in exs]))
"Return name of function symbol"
function get_name(ex::Basic)
a = ccall((:function_symbol_get_name, libsymengine), Cstring, (Ref{Basic}, ), ex)
string = unsafe_string(a)
ccall((:basic_str_free, libsymengine), Nothing, (Cstring, ), a)
return string
end
"Return arguments of a function call as a vector of `Basic` objects"
function get_args(ex::Basic)
args = CVecBasic()
ccall((:basic_get_args, libsymengine), Nothing, (Ref{Basic}, Ptr{Cvoid}), ex, args.ptr)
convert(Vector, args)
end
## so that Dicts will work
basic_hash(ex::Basic) = ccall((:basic_hash, libsymengine), UInt, (Ref{Basic}, ), ex)
# similar definition as in Base for general objects
Base.hash(ex::Basic, h::UInt) = Base.hash_uint(3h - basic_hash(ex))
Base.hash(ex::BasicType, h::UInt) = hash(Basic(ex), h)
function coeff(b::Basic, x::Basic, n::Basic)
c = Basic()
ccall((:basic_coeff, libsymengine), Nothing, (Ref{Basic}, Ref{Basic}, Ref{Basic}, Ref{Basic}), c, b, x, n)
return c
end
coeff(b::Basic, x::Basic) = coeff(b, x, one(Basic))
function Serialization.serialize(s::Serialization.AbstractSerializer, m::Basic)
Serialization.serialize_type(s, typeof(m))
size = Ref{UInt64}(0)
serialized = ccall((:basic_dumps, libsymengine),
Ptr{Int8}, (Ref{Basic}, Ptr{UInt64}), m, size)
write(s.io, size[])
unsafe_write(s.io, serialized, size[])
end
function Serialization.deserialize(s::Serialization.AbstractSerializer, ::Type{Basic})
size = read(s.io, UInt64)
serialized_data = read(s.io, size)
a = Basic()
res = ccall((:basic_loads, libsymengine),
Cuint, (Ref{Basic}, Ptr{Int8}, UInt64), a, serialized_data, size)
throw_if_error(res)
return a
end
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 955 | "Helper function to lookup a symbol from libsymengine"
function get_symbol(sym::Symbol)
handle = Libdl.dlopen_e(libsymengine)
if handle == C_NULL
C_NULL
else
Libdl.dlsym_e(handle, sym)
end
end
"Get libsymengine version"
function get_libversion()
func = get_symbol(:symengine_version)
if func != C_NULL
a = ccall(func, Ptr{UInt8}, ())
VersionNumber(unsafe_string(a))
else
# Above method is not in 0.2.0 which is the earliest version supported
VersionNumber("0.2.0")
end
end
"Check whether libsymengine was compiled with comp"
function have_component(comp::String)
func = get_symbol(:symengine_have_component)
if func != C_NULL
ccall(func, Cint, (Ptr{UInt8},), comp) == 1
elseif comp == "mpfr"
get_symbol(:real_mpfr_set_d) != C_NULL
elseif comp == "mpc"
get_symbol(:complex_mpc_real_part) != C_NULL
else
false
end
end
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 8108 | using SymEngine
using Compat
using Test
using Serialization
import Base: MathConstants.γ, MathConstants.e, MathConstants.φ, MathConstants.catalan
VERSION >= v"1.9" && include("test-SymbolicUtils.jl")
include("test-dense-matrix.jl")
x = symbols("x")
y = symbols(:y)
@vars z
# Check Basic conversions
@test eltype([Basic(u) for u in [1, 1/2, 1//2, pi, e]]) == Basic
# make sure @vars defines in a local scope
let
@vars w
end
@test_throws UndefVarError isdefined(w)
@test_throws Exception show(Basic())
a = x^2 + x/2 - x*y*5
b = diff(a, x)
@test b == 2*x + 1//2 - 5*y
c = x + Rational(1, 5)
c = expand(c * 5)
@test c == 5*x + 1
c = sum(convert(SymEngine.CVecBasic, [x, x, 1]))
@test c == 2*x + 1
@test x + x + 1 == 2*x + 1
@test x + 1 + 1 == x + 2
@test 1 + x + 1 == x + 2
c = prod(convert(SymEngine.CVecBasic, [x, x, 2]))
@test c == 2*x^2
@test x * x * 2 == 2*x^2
@test x * 2 * 3 == 6 * x
@test 2 * 3 * x == 6 * x
@test 2 * x * 3 == 6 * x
@test 3 * 2 * x == 6 * x
# https://github.com/symengine/SymEngine.jl/issues/259
@testset "Issue #259" begin
let (a, b, c) = symbols("a b c")
@test a * [b, c] == [a * b, a * c]
@test a * 1//3 * [b, c] == [a * b / 3, a * c / 3]
@test a * 1//3 * 3//4 * [b, c] == [a * b / 4, a * c / 4]
@test a * 1//3 * 3//4 * 4//5 * [b, c] == [a * b / 5, a * c / 5]
end
end
c = x ^ 5
@test diff(c, x) == 5 * x ^ 4
c = x ^ y
@test c != y^x
c = Basic(-5)
@test abs(c) == 5
@test abs(c) != 4
show(a)
println()
show(b)
println()
@test 1 // x == 1 / x
@test x // 2 == (1//2) * x
@test x // y == x / y
## mathfuns
@test abs(Basic(-1)) == 1
@test sin(Basic(1)) == subs(sin(x), x, 1)
@test sin(PI) == 0
@test subs(sin(x), x, pi) == 0
@test sind(Basic(30)) == 1 // 2
## calculus
x,y = symbols("x y")
n = Basic(2)
ex = sin(x*y)
@test diff(log(x),x) == 1/x
@test diff(ex, x) == y * cos(x*y)
@test diff(ex, x, 2) == diff(diff(ex,x), x)
@test diff(ex, x, n) == diff(diff(ex,x), x)
@test diff(ex, x, y) == diff(diff(ex,x), y)
@test diff(ex, x, y,x) == diff(diff(diff(ex,x), y), x)
@test series(sin(x), x, 0, 2) == x
@test series(sin(x), x, 0, 3) == x - x^3/6
## ntheory
@test mod(Basic(10), Basic(4)) == 2
for j in [-3, 3], p in [-5,5]
@test mod(Basic(j), Basic(p)) == mod(j, p)
end
@test mod(Basic(10), 4) == 2 # mod(::Basic, ::Number)
@test_throws MethodError mod(10, Basic(4)) # no mod(::Number, ::Basic)
@test gcd(Basic(10), Basic(4)) == 2
@test lcm(Basic(10), Basic(4)) == 20
@test binomial(Basic(5), 2) == 10
## type information
a = Basic(1)
b = Basic(1//2)
c = Basic(0.125)
@test isa(SymEngine.BasicType(a+a), SymEngine.BasicType{Val{:Integer}})
@test isa(SymEngine.BasicType(a+b), SymEngine.BasicType{Val{:Rational}})
@test isa(SymEngine.BasicType(a+c), SymEngine.BasicType{Val{:RealDouble}})
@test isa(SymEngine.BasicType(b+c), SymEngine.BasicType{Val{:RealDouble}})
@test isa(SymEngine.BasicType(c+c), SymEngine.BasicType{Val{:RealDouble}})
## can we do math with items of BasicType?
a1 = SymEngine.BasicType(a)
tot = a1
for i in 1:100
global tot
tot = tot + a1
end
@test tot == 101
sin(a1)
# samples of different types:
# (Int, Rational{Int}, Complex{Int}, Float64, Complex{Float64})
samples = (1, 1//2, (1 + 2im), 1.0, (1.0 + 0im))
## subs - check all different syntaxes and types
ex = x^2 + y^2
for val in samples
@test subs(ex, x, val) == val^2 + y^2
@test subs(ex, (x, val)) == val^2 + y^2
@test subs(ex, x => val) == val^2 + y^2
@test subs(ex, SymEngine.CMapBasicBasic(Dict(x=>val))) == val^2 + y^2
@test subs(ex, Dict(x=>val)) == val^2 + y^2
end
# This probably results in a number of redundant tests (operator order).
for val1 in samples, val2 in samples
@test subs(ex, (x, val1), (y, val2)) == val1^2 + val2^2
@test subs(ex, x => val1, y => val2) == val1^2 + val2^2
end
## lambidfy
@test abs(lambdify(sin(Basic(1))) - sin(1)) <= 1e-14
fn = lambdify(exp(PI/2*x))
@test abs(fn(1) - exp(pi/2)) <= 1e-14
for val in samples
ex2 = sin(x + val)
fn2 = lambdify(ex2)
@test abs(fn2(val) - sin(2*val)) <= 1e-14
end
@test lambdify(x^2)(3) == 9
A = [x 2; x 1]
@test lambdify(A, [x])(0) == [0 2; 0 1]
@test lambdify(A)(0) == [0 2; 0 1]
A = [x 2]
@test lambdify(A, [x])(1) == [1 2]
@test lambdify(A)(1) == [1 2]
@test isa(convert.(Expr, [0 x x+1]), Array{Expr})
## N
for val in samples
@test N(Basic(val)) == val
end
for val in [π, γ, e, φ, catalan]
@test N(Basic(val)) == val
end
@test !isnan(x)
@test isnan(Basic(0)/0)
## generic linear algebra
x = symbols("x")
A = [x 2; x 1]
#@test det(A) == -x
#@test det(inv(A)) == - 1/x
#(A \ [1,2])[1] == 3/x
## check that unique work (hash)
x,y,z = symbols("x y z")
@test length(SymEngine.free_symbols([x*y, y,z])) == 3
## check that callable symengine expressions can be used as functions for duck-typed functions
@vars x
function simple_newton(f, fp, x0)
x = float(x0)
while abs(f(x)) >= 1e-14
x = x - f(x)/fp(x)
end
x
end
@test abs(simple_newton(sin(x), diff(sin(x), x), 3) - pi) <= 1e-14
## Check conversions SymEngine -> Julia
z,flt, rat, ima, cplx = btypes = [Basic(1), Basic(1.23), Basic(3//5), Basic(2im), Basic(1 + 2im)]
@test Int(z) == 1
@test BigInt(z) == 1
@test Float64(flt) ≈ 1.23
@test Real(flt) ≈ 1.23
@test convert(Rational{Int}, rat) == 3//5
@test convert(Complex{Int}, ima) == 2im
@test convert(Complex{Int}, cplx) == 1 + 2im
@test_throws InexactError convert(Int, flt)
@test_throws InexactError convert(Int, rat)
x = symbols("x")
Number[1 2 3 x]
@test_throws ArgumentError Int[1 2 3 x]
t = BigFloat(1.23)
@test !SymEngine.have_component("mpfr") || t == convert(BigFloat, convert(Basic, t))
@test typeof(N(Basic(-1))) != BigInt
# Check that libversion works. VersionNumber should always be >= 0.2.0
# since 0.2.0 is the first public release
@test SymEngine.libversion >= VersionNumber("0.2.0")
# Check that constructing Basic from Expr works
@vars x y
@test Basic(:(-2*x)) == -2*x
@test Basic(:(-3*x*y)) == -3*x*y
@test Basic(:((x-y)*-3)) == (x-y)*(-3)
@test Basic(:(-y)) == -y
@test Basic(:(-2*(x-2*y))) == -2*(x-2*y)
@test Basic(0)/0 == NAN
@test subs(1/x, x, 0) == Basic(1)/0
d = Dict(x=>y, y=>x)
@test subs(x + 2*y, d) == y + 2*x
@test sin(x+PI/4) != sin(x)
@test sin(PI/2-x) == cos(x)
f = SymFunction("f")
@test string(f(x, y)) == "f(x, y)"
@test string(f([x, y])) == "f(x, y)"
@test string(f(2*x)) == "f(2*x)"
@funs g, h
@test string(g(x, y)) == "g(x, y)"
@test string(h(x, y)) == "h(x, y)"
# Function symbols
@funs f, g, h, s
@vars n, m
expr = Basic("f(n) + f(n+1) + g(m)^2 - 3*h(n)*s(n)^3")
@test function_symbols(expr) == [h(n), f(n), s(n), g(m), f(n+1)]
@test get_name(f(n+1)) == "f"
@test get_args(f(n+1)) == [n+1]
@test get_args(f(n, m^2)) == [n, m^2]
# Coefficients
@vars x y
expr = x^3 + 3*x^2*y + 3*x*y^2 + y^3 + 1
@test coeff(expr, x, Basic(3)) == 1
@test coeff(expr, x, Basic(2)) == 3*y
@test coeff(expr, x, Basic(1)) == 3*y^2
@test coeff(expr, x, Basic(0)) == y^3 + 1
# Check that infinities are handled correctly
@test_throws DomainError exp(zoo)
@test_throws DomainError sin(zoo)
@test_throws DomainError sin(oo)
@test_throws DomainError subs(sin(log(y - y/x)), x => 1)
@test_throws DomainError exp(zoo+x)/exp(x)
# Some basic checks for complex numbers
@testset "Complex numbers" begin
for T in (Int, Float64, BigFloat)
j = one(T) * IM
@test j == imag(j) * IM
@test conj(j) == -j
end
end
@test round(Basic(3.14)) == 3.0
@test round(Basic(3.14); digits=1) == 3.1
function is_serialization_correct(expr :: Basic)
iobuf = IOBuffer()
serialize(iobuf, expr)
seek(iobuf, 0)
deserialized = deserialize(iobuf)
close(iobuf)
return expr == deserialized
end
@vars x y
@testset "Serialization and deserialization" begin
@test is_serialization_correct(Basic(1.0))
@test is_serialization_correct(Basic(pi))
@test is_serialization_correct(Basic(x + y))
@test is_serialization_correct(Basic(sin(cos(pi*x + y)) + y^2))
# Complex type test
iobuf = IOBuffer()
data = [x+y, x+y]
serialize(iobuf, data)
seek(iobuf, 0)
deserialized = deserialize(iobuf)
close(iobuf)
@test deserialized == data
end
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 1869 | using Test
using SymEngine
import SymbolicUtils: simplify, @rule, @acrule, Chain, Fixpoint
@testset "SymbolicUtils" begin
# from SymbolicUtils.jl docs
# https://symbolicutils.juliasymbolics.org/rewrite/#rule-based_rewriting
@vars w x y z
@vars α β
@vars a b c d
@test simplify(cos(x)^2 + sin(x)^2) == 1
r1 = @rule sin(2(~x)) => 2sin(~x)*cos(~x)
@test r1(sin(2z)) == 2*cos(z)*sin(z)
@test r1(sin(3z)) === nothing
@test r1(sin(2*(w-z))) == 2cos(w - z)*sin(w - z)
@test r1(sin(2*(w+z)*(α+β))) === nothing
r2 = @rule sin(~x + ~y) => sin(~x)*cos(~y) + cos(~x)*sin(~y);
@test r2(sin(α+β)) == sin(α)*cos(β) + cos(α)*sin(β)
xs = @rule(+(~~xs) => ~~xs)(x + y + z) # segment variable
@test Set(xs) == Set([x,y,z])
r3 = @rule ~x * +(~~ys) => sum(map(y-> ~x * y, ~~ys));
@test r3(2 * (w+w+α+β)) == 4w + 2α + 2β
r4 = @rule ~x + ~~y::(ys->iseven(length(ys))) => "odd terms"; # Predicates for matching
@test r4(a + b + c + d) == nothing
@test r4(b + c + d) == "odd terms"
@test r4(b + c + b) == nothing
@test r4(a + b) == nothing
sqexpand = @rule (~x + ~y)^2 => (~x)^2 + (~y)^2 + 2 * ~x * ~y
@test sqexpand((cos(x) + sin(x))^2) == cos(x)^2 + sin(x)^2 + 2cos(x)*sin(x)
pyid = @rule sin(~x)^2 + cos(~x)^2 => 1
@test_broken pyid(cos(x)^2 + sin(x)^2) === nothing # order should matter, but this works
acpyid = @acrule sin(~x)^2 + cos(~x)^2 => 1 # acrule is commutative
@test acpyid(cos(x)^2 + sin(x)^2 + 2cos(x)*sin(x)) == 1 + 2cos(x)*sin(x)
csa = Chain([sqexpand, acpyid]) # chain composes rules
@test csa((cos(x) + sin(x))^2) == 1 + 2cos(x)*sin(x)
cas = Chain([acpyid, sqexpand]) # order matters
@test cas((cos(x) + sin(x))^2) == cos(x)^2 + sin(x)^2 + 2cos(x)*sin(x)
@test Fixpoint(cas)((cos(x) + sin(x))^2) == 1 + 2cos(x)*sin(x)
end
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | code | 1225 | using Test
using SymEngine
import LinearAlgebra: lu, det, zeros, dot
CDenseMatrix = SymEngine.CDenseMatrix
@vars x y
# constructors
A = [x 1 2; 3 x 4; 5 6 x]
M = convert(CDenseMatrix, A)
M = CDenseMatrix(A)
# abstract interface
@test M[1,1] == A[1,1]
for i in eachindex(M)
@test M[i] == A[i]
end
M[1,1] = x^2
@test M[1,1] == x^2
M[1,1] = x
# inherits from DenseArray
@test all(M .+ 3 .== A .+ 3)
@test all(M * 3 .== A * 3)
@test all(M + M .== A + A)
@test all(M * M .== A * A)
@test all(transpose(M) .== transpose(A))
# generic det
@test prod([subs(det(M) - det(A), x, i) == 0 for i in 2:10]) == true
@test inv(M) - inv(A) == zeros(Basic, 3,3)
# factorizations
L1, U1 = lu(M)
L2, U2, P2 = lu(A)
@test iszero(expand.(L1 - L2))
@test iszero(expand.(U1 - U2))
A = [x 1 2; 0 x 4; 0 0 x]
b = [1, 2, 3]
M = convert(CDenseMatrix, A)
out = M \ b
@test M * out - b == zeros(Basic, 3, 1)
@test SymEngine.dense_matrix_eye(2,2,0) == Basic[1 0; 0 1]
# dot product
@test dot(x, x) == x^2
@test dot([1, x, 0], [y, -2, 1]) == y - 2x
@testset "dense matrix" begin
@vars a b c d x y
A = [a b; c d]
B = [x, y]
res = A \ B
@test res == [(x - b*(y - c*x/a)/(d - b*c/a))/a,
(y - c*x/a)/(d - b*c/a)]
end | SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | docs | 3025 | # SymEngine.jl
[](https://github.com/symengine/SymEngine.jl/actions)
[](https://ci.appveyor.com/project/isuruf/symengine-jl-pj80f/branch/master)
[](http://codecov.io/github/symengine/SymEngine.jl?branch=master)
[](https://coveralls.io/github/symengine/SymEngine.jl?branch=master)
Julia Wrappers for [SymEngine](https://github.com/symengine/symengine), a fast symbolic manipulation library, written in C++.
## Installation
You can install `SymEngine.jl` by giving the following command.
```julia
julia> Pkg.add("SymEngine")
```
## Quick Start
### Working with scalar variables
#### Defining variables
One can define variables in a few ways. The following three examples are equivalent.
Defining two symbolic variables with the names `a` and `b`, and assigning them to julia variables with the same name.
``` julia
julia> a=symbols(:a); b=symbols(:b)
b
julia> a,b = symbols("a b")
(a, b)
julia> @vars a b
(a, b)
```
### Simple expressions
We are going to define an expression using the variables from earlier:
``` julia
julia> ex1 = a + 2(b+2)^2 + 2a + 3(a+1)
3*a + 3*(1 + a) + 2*(2 + b)^2
```
One can see that values are grouped, but no expansion is done.
### Working with vector and matrix variables
### Defining vectors of variables
A vector of variables can be defined using list comprehension and string interpolation.
```julia
julia> [symbols("α_$i") for i in 1:3]
3-element Array{SymEngine.Basic,1}:
α_1
α_2
α_3
```
#### Defining matrices of variables
Some times one might want to define a matrix of variables.
One can use a matrix comprehension, and string interpolation to create a matrix of variables.
```julia
julia> W = [symbols("W_$i$j") for i in 1:3, j in 1:4]
3×4 Array{Basic,2}:
W_11 W_12 W_13 W_14
W_21 W_22 W_23 W_24
W_31 W_32 W_33 W_34
```
#### Matrix-vector multiplication
Now using the matrix we can perform matrix operations:
```julia
julia> W*[1.0, 2.0, 3.0, 4.0]
3-element Array{Basic,1}:
1.0*W_11 + 2.0*W_12 + 3.0*W_13 + 4.0*W_14
1.0*W_21 + 2.0*W_22 + 3.0*W_23 + 4.0*W_24
1.0*W_31 + 2.0*W_32 + 3.0*W_33 + 4.0*W_34
```
### Operations
#### `expand`
```julia
julia> expand(a + 2(b+2)^2 + 2a + 3(a+1))
11 + 6*a + 8*b + 2*b^2
```
#### `subs`
Performs substitution.
```julia
julia> subs(a^2+(b-2)^2, b=>a)
a^2 + (-2 + a)^2
julia> subs(a^2+(b-2)^2, b=>2)
a^2
julia> subs(a^2+(b-2)^2, a=>2)
4 + (-2 + b)^2
julia> subs(a^2+(b-2)^2, a^2=>2)
2 + (-2 + b)^2
julia> subs(a^2+(b-2)^2, a=>2, b=>3)
5
```
#### `diff`
Peforms differentiation
```julia
julia> diff(a + 2(b+2)^2 + 2a + 3(a+1), b)
4*(2 + b)
```
## License
`SymEngine.jl` is licensed under MIT open source license.
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | docs | 99 | # API Documentation
## SymEngine
```@autodocs
Modules = [SymEngine]
```
## Index
```@index
```
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | docs | 2284 | # Quick Start
Derived from the project `README` [on GitHub](https://github.com/symengine/SymEngine.jl).
## Scalar Variables
### Definitions
Multiple equivalent methods of variable declaration are supported.
```jldoctest
julia> using SymEngine
julia> a=symbols(:a); b=symbols(:b)
b
julia> a,b = symbols("a b")
(a, b)
julia> @vars a b
(a, b)
```
### Simple Expressions
Note that by default, values are grouped but no expansion takes place.
```jldoctest
julia> using SymEngine
julia> @vars a b
(a, b)
julia> ex1 = a + 2(b+2)^2 + 2a + 3(a+1)
3*a + 3*(1 + a) + 2*(2 + b)^2
```
## Vectors and Matrices
### Definitions
#### Vectors
Vectors can be defined through list comprehension and string interpolation.
```jldoctest
julia> using SymEngine
julia> [symbols("α_$i") for i in 1:3]
3-element Vector{Basic}:
α_1
α_2
α_3
```
#### Matrices
In an analogous manner, matrices are declared with a combination of string interpolation and matrix comprehension.
```jldoctest
julia> using SymEngine
julia> W = [symbols("W_$i$j") for i in 1:3, j in 1:4]
3×4 Matrix{Basic}:
W_11 W_12 W_13 W_14
W_21 W_22 W_23 W_24
W_31 W_32 W_33 W_34
```
### Matrix Vector Operations
Consider the canonical example of **matrix vector multiplication**.
```jldoctest
julia> using SymEngine
julia> W = [symbols("W_$i$j") for i in 1:3, j in 1:4]
3×4 Matrix{Basic}:
W_11 W_12 W_13 W_14
W_21 W_22 W_23 W_24
W_31 W_32 W_33 W_34
julia> W*[1.0, 2.0, 3.0, 4.0]
3-element Vector{Basic}:
1.0*W_11 + 2.0*W_12 + 3.0*W_13 + 4.0*W_14
1.0*W_21 + 2.0*W_22 + 3.0*W_23 + 4.0*W_24
1.0*W_31 + 2.0*W_32 + 3.0*W_33 + 4.0*W_34
```
## Operations
We will demonstrate the most common operations as follows.
### Expansion
```jldoctest
julia> using SymEngine
julia> @vars a b
(a, b)
julia> expand(a + 2(b+2)^2 + 2a + 3(a+1))
11 + 6*a + 8*b + 2*b^2
```
### Substitution
```jldoctest
julia> using SymEngine
julia> @vars a b
(a, b)
julia> subs(a^2+(b-2)^2, b=>a)
a^2 + (-2 + a)^2
julia> subs(a^2+(b-2)^2, b=>2)
a^2
julia> subs(a^2+(b-2)^2, a=>2)
4 + (-2 + b)^2
julia> subs(a^2+(b-2)^2, a^2=>2)
2 + (-2 + b)^2
julia> subs(a^2+(b-2)^2, a=>2, b=>3)
5
```
### Differentiation
```jldoctest
julia> using SymEngine
julia> @vars a b
(a, b)
julia> diff(a + 2(b+2)^2 + 2a + 3(a+1), b)
4*(2 + b)
```
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"MIT"
] | 0.12.0 | 9a66fef88d10cfa264c61181221e405d7276f08b | docs | 320 | # SymEngine.jl Documentation
## About
This site contains the documentation and `julia` language bindings for the [Symengine project](https://symengine.org).
### Contents
```@contents
```
## Installation
The package and dependencies can be obtained with standard `Pkg` calls.
```julia
julia> Pkg.add("SymEngine")
```
| SymEngine | https://github.com/symengine/SymEngine.jl.git |
|
[
"Apache-2.0"
] | 0.1.0 | 3e076ca96e34a47e98a46657b2bec2655a366d80 | code | 2616 | module GilbertCurves
export gilbertindices
"""
gilbertindices(dims::Tuple{Int,Int}; majdim=dims[1] >= dims[2] ? 1 : 2)
Constructs a vector of `CartesianIndex` objects, orderd by a generalized Hilbert
space-filling, starting at `CartesianIndex(1,1)`. It will end at
`CartesianIndex(dims[1],1)` if `majdim==1`, or `CartesianIndex(1,dims[2])` if `majdim==2`
(or the closest feasible point).
"""
gilbertindices(dims::Tuple{Int,Int}; kwargs...) =
gilbertorder(CartesianIndices(dims); kwargs...)
"""
gilbertorder(mat::AbstractMatrix; majdim=size(mat,1) >= size(mat,2) ? 1 : 2)
Constructs a vector of the elements of `mat`, ordered by a generalized Hilbert
space-filling curve. The list will start at `mat[1,1]`, and end at `mat[end,1]` if
`majdim==1` or `mat[1,end]` if `majdim==2` (or the closest feasible point).
"""
function gilbertorder(mat::AbstractMatrix{T}; majdim=size(mat,1) >= size(mat,2) ? 1 : 2) where {T}
list = sizehint!(T[], length(mat))
if majdim == 1
append_gilbert!(list, mat)
else
append_gilbert!(list, permutedims(mat,(2,1)))
end
end
function append_gilbert!(list, mat::AbstractMatrix)
# 1 |* |
# | ) |
# a |v |
a,b = size(mat)
if a == 1 || b == 1
# single in one dimension
append!(list, mat)
elseif 2a > 3b
# long case: split into two
# +-----+
# 1 |* |
# || |
# a2|v |
# +-----+
# |* |
# || |
# a |v |
# +-----+
a2 = div(a,2)
if isodd(a2) && a > 2
a2 += 1
end
append_gilbert!(list, mat[1:a2,:])
append_gilbert!(list, mat[a2+1:a,:])
else
# standard case: split into three
# b2
# +---+---+
# 1 |*->|* |
# | || |
# a2| || |
# +---+| |
# | || |
# a |<-*|v |
# +---+----+
a2 = div(a,2)
b2 = div(b,2)
if isodd(b2) && b > 2
b2 += 1
end
append_gilbert!(list, permutedims(mat[1:a2,1:b2],(2,1)))
append_gilbert!(list, mat[:,b2+1:b])
append_gilbert!(list, permutedims(mat[a:-1:a2+1,b2:-1:1],(2,1)))
end
end
"""
linearindices(list::Vector{CartesianIndex{2}})
Construct an integer matrix `M` containing the integers `1:length(list)` such that
`M[list[i]] == i`.
"""
function linearindices(list::Vector{CartesianIndex{2}})
cmax = maximum(list)
L = zeros(Int,cmax.I)
for (i,c) in enumerate(list)
L[c] = i
end
return L
end
end
| GilbertCurves | https://github.com/CliMA/GilbertCurves.jl.git |
|
[
"Apache-2.0"
] | 0.1.0 | 3e076ca96e34a47e98a46657b2bec2655a366d80 | code | 858 | using GilbertCurves
using Test
@testset "size $m,$n" for m = 1:20, n = 1:20
list = gilbertindices((m,n))
@test length(list) == m*n
@test list[1] == CartesianIndex(1,1)
if m >= n
if !(n == 2 && isodd(m))
@test list[end] == CartesianIndex(m,1)
end
else
if !(m == 2 && isodd(n))
@test list[end] == CartesianIndex(1,n)
end
end
ndiag = 0
for i = 1:m*n-1
Δ = map(abs, (list[i+1] - list[i]).I)
@test Δ[1] <= 1
@test Δ[2] <= 1
if Δ[1] + Δ[2] > 1
ndiag += 1
end
end
if m > n && isodd(m) && iseven(n) || m < n && isodd(n) && iseven(m)
@test ndiag <= 1
else
@test ndiag == 0
end
L = GilbertCurves.linearindices(list)
@test !any(iszero, L)
@test sum(L) == sum(1:m*n)
end
| GilbertCurves | https://github.com/CliMA/GilbertCurves.jl.git |
|
[
"Apache-2.0"
] | 0.1.0 | 3e076ca96e34a47e98a46657b2bec2655a366d80 | docs | 2422 | # GilbertCurves.jl
[](https://github.com/CliMA/GilbertCurves.jl/actions/workflows/CI.yml?query=branch%3Amain)
This is a Julia implementation of the generalized Hilbert ("gilbert") space-filling curve algorithm, by Jakub Červený (https://github.com/jakubcerveny/gilbert).
It provides space-filling curves for ectangular domains of arbitrary (non-power-of-two) sizes. Currently only 2D domains are supported, but it could be extended to 3D.
# Usage
Currently it exports one function, `gilbertindices` which returns a vector of
`CartesianIndex{2}` objects corresponding to their order on the curve:
```julia
julia> using GilbertCurves
julia> list = gilbertindices((5,5))
25-element Vector{CartesianIndex{2}}:
CartesianIndex(1, 1)
CartesianIndex(2, 1)
CartesianIndex(2, 2)
CartesianIndex(1, 2)
CartesianIndex(1, 3)
CartesianIndex(1, 4)
CartesianIndex(1, 5)
⋮
CartesianIndex(5, 3)
CartesianIndex(5, 2)
CartesianIndex(4, 2)
CartesianIndex(3, 2)
CartesianIndex(3, 1)
CartesianIndex(4, 1)
CartesianIndex(5, 1)
```
Two non-exported functions are also provided. `GilbertCurves.linearindices` takes the output of
`gilbertindices`, returning an integer-valued matrix of the gilbert indices of each component.
```julia
julia> GilbertCurves.linearindices(list)
5×5 Matrix{Int64}:
1 4 5 6 7
2 3 10 9 8
23 22 11 12 13
24 21 18 17 14
25 20 19 16 15
```
`GilbertCurves.gilbertorder` constructs a vector containing the elements of a matrix in the
gilbert curve order.
```julia
julia> GilbertCurves.gilbertorder(reshape(1:9,3,3))
9-element Vector{Int64}:
1
4
7
8
9
6
5
2
3
```
# Example
```julia
julia> using Plots
julia> list = gilbertindices((67,29));
julia> plot([c[1] for c in list], [c[2] for c in list], line_z=1:length(list), legend=false)
```
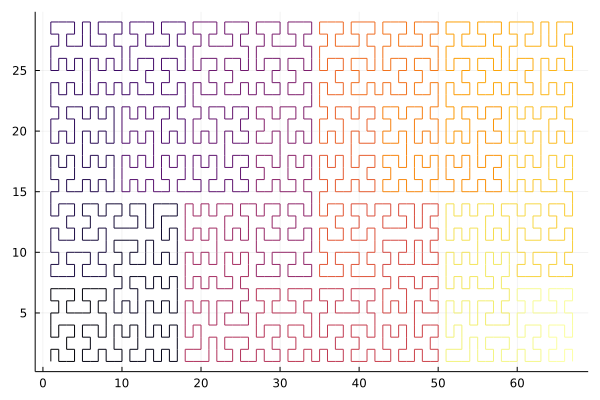
# Notes
The algorithm is not able to avoid non-diagonal moves in the case when the
larger dimension is odd and the smaller is even.
```julia
julia> list = gilbertindices((15,12));
julia> plot([c[1] for c in list], [c[2] for c in list], line_z=1:length(list), legend=false)
```
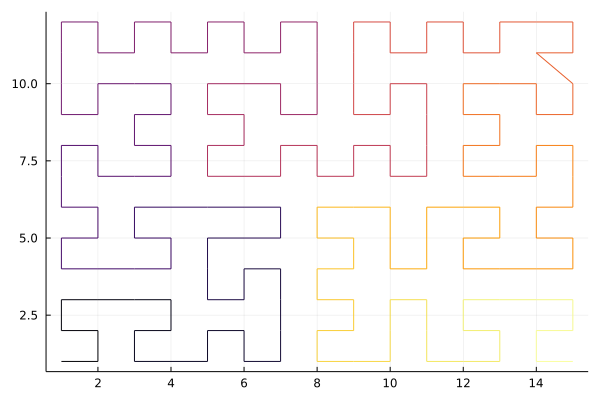
| GilbertCurves | https://github.com/CliMA/GilbertCurves.jl.git |
|
[
"MIT"
] | 0.1.0 | 130a26c6126dff88bf0ad5e265cc81bb7196e38c | code | 15433 | """
CLI for grep.
Matches patterns in input text. Supports simple patterns and regular expressions
Generated via FigCLIGen. Do not edit directly.
Edit the specification file and run the generator instead.
"""
module CLI
const OptsType = Base.Dict{Base.Symbol,Base.Any}
"""
CommandLine execution context.
`exec`: a no argument function that provides the base command to execute in a julia `do` block.
`cmdopts`: keyword arguments that should be used to further customize the `Cmd` creation
`pipelineopts`: keyword arguments that should be used to further customize the `pipeline` creation
"""
Base.@kwdef struct CommandLine
exec::Base.Function = (f) -> f("grep")
cmdopts::OptsType = OptsType()
pipelineopts::OptsType = OptsType()
runopts::OptsType = OptsType()
end
""" grep
Run the grep command.
Matches patterns in input text. Supports simple patterns and regular expressions
Options:
- help::Bool - Print a usage message briefly summarizing these command-line options and the bug-reporting address, then exit
- extended_regexp::Bool - Interpret PATTERN as an extended regular expression (-E is specified by POSIX.)
- fixed_string::Bool - Interpret PATTERN as a list of fixed strings, separated by newlines, any of which is to be matched. (-F is specified by POSIX.)
- basic_regexp::Bool - Interpret PATTERN as a basic regular expression (BRE, see below). This is the default
- regexp::AbstractString - Use PATTERN as the pattern. This can be used to specify multiple search patterns, or to protect a pattern beginning with a hyphen (-). (-e is specified by POSIX.)
- ignore_case::Bool - Ignore case distinctions in both the PATTERN and the input files. (-i is specified by POSIX.)
- invert_match::Bool - Invert the sense of matching, to select non-matching lines. (-v is specified by POSIX.)
- word_regexp::Bool - Select only those lines containing matches that form whole words. The test is that the matching substring must either be at the beginning of the line, or preceded by a non-word constituent character. Similarly, it must be either at the end of the line or followed by a non-word constituent character. Word-constituent characters are letters, digits, and the underscore
- line_regexp::Bool - Select only those matches that exactly match the whole line. (-x is specified by POSIX.)
- count::Bool - Suppress normal output; instead print a count of matching lines for each input file. With the -v, --invert-match option, count non-matching lines. (-c is specified by POSIX.)
- color::AbstractString - Surround the matched (non-empty) strings, matching lines, context lines, file names, line numbers, byte offsets, and separators (for fields and groups of context lines) with escape sequences to display them in color on the terminal. The colors are defined by the environment variable GREP_COLORS. The deprecated environment variable GREP_COLOR is still supported, but its setting does not have priority
- files_without_match::Bool - Suppress normal output; instead print the name of each input file from which no output would normally have been printed. The scanning will stop on the first match
- files_with_matches::Bool - Suppress normal output; instead print the name of each input file from which output would normally have been printed. The scanning will stop on the first match. (-l is specified by POSIX.)
- max_count::AbstractString - Stop reading a file after NUM matching lines. If the input is standard input from a regular file, and NUM matching lines are output, grep ensures that the standard input is positioned to just after the last matching line before exiting, regardless of the presence of trailing context lines. This enables a calling process to resume a search. When grep stops after NUM matching lines, it outputs any trailing context lines. When the -c or --count option is also used, grep does not output a count greater than NUM. When the -v or --invert-match option is also used, grep stops after outputting NUM non-matching lines
- only_matching::Bool - Print only the matched (non-empty) parts of a matching line, with each such part on a separate output line
- quiet::Bool - Quiet; do not write anything to standard output. Exit immediately with zero status if any match is found, even if an error was detected. Also see the -s or --no-messages option. (-q is specified by POSIX.)
- no_messages::Bool - Suppress error messages about nonexistent or unreadable files. Portability note: unlike GNU grep, 7th Edition Unix grep did not conform to POSIX, because it lacked -q and its -s option behaved like GNU grep's -q option. USG -style grep also lacked -q but its -s option behaved like GNU grep. Portable shell scripts should avoid both -q and -s and should redirect standard and error output to /dev/null instead. (-s is specified by POSIX.)
- byte_offset::Bool - Print the 0-based byte offset within the input file before each line of output. If -o (--only-matching) is specified, print the offset of the matching part itself
- with_filename::Bool - Print the file name for each match. This is the default when there is more than one file to search
- no_filename::Bool - Suppress the prefixing of file names on output. This is the default when there is only one file (or only standard input) to search
- label::AbstractString - Display input actually coming from standard input as input coming from file LABEL. This is especially useful when implementing tools like zgrep, e.g., gzip -cd foo.gz | grep --label=foo -H something
- line_number::Bool - Prefix each line of output with the 1-based line number within its input file. (-n is specified by POSIX.)
- initial_tab::Bool - Make sure that the first character of actual line content lies on a tab stop, so that the alignment of tabs looks normal. This is useful with options that prefix their output to the actual content: -H,-n, and -b. In order to improve the probability that lines from a single file will all start at the same column, this also causes the line number and byte offset (if present) to be printed in a minimum size field width
- unix_byte_offsets::Bool - Report Unix-style byte offsets. This switch causes grep to report byte offsets as if the file were a Unix-style text file, i.e., with CR characters stripped off. This will produce results identical to running grep on a Unix machine. This option has no effect unless -b option is also used; it has no effect on platforms other than MS-DOS and MS -Windows
- null::Bool - Output a zero byte (the ASCII NUL character) instead of the character that normally follows a file name. For example, grep -lZ outputs a zero byte after each file name instead of the usual newline. This option makes the output unambiguous, even in the presence of file names containing unusual characters like newlines. This option can be used with commands like find -print0, perl -0, sort -z, and xargs -0 to process arbitrary file names, even those that contain newline characters
- after_context::AbstractString - Print num lines of trailing context after each match
- before_context::AbstractString - Print num lines of leading context before each match. See also the -A and -C options
- context::AbstractString - Print NUM lines of output context. Places a line containing a group separator (--) between contiguous groups of matches. With the -o or --only-matching option, this has no effect and a warning is given
- text::Bool - Treat all files as ASCII text. Normally grep will simply print ``Binary file ... matches'' if files contain binary characters. Use of this option forces grep to output lines matching the specified pattern
- binary_files::AbstractString - Controls searching and printing of binary files
- devices::AbstractString - Specify the demanded action for devices, FIFOs and sockets
- directories::AbstractString - Specify the demanded action for directories
- exclude::AbstractString - Note that --exclude patterns take priority over --include patterns, and if no --include pattern is specified, all files are searched that are not excluded. Patterns are matched to the full path specified, not only to the filename component
- exclude_dir::AbstractString - If -R is specified, only directories matching the given filename pattern are searched. Note that --exclude-dir patterns take priority over --include-dir patterns
- I::Bool - Ignore binary files. This option is equivalent to --binary-file=without-match option
- include::AbstractString - If specified, only files matching the given filename pattern are searched. Note that --exclude patterns take priority over --include patterns. Patterns are matched to the full path specified, not only to the filename component
- include_dir::AbstractString - If -R is specified, only directories matching the given filename pattern are searched. Note that --exclude-dir patterns take priority over --include-dir patterns
- recursive::Bool - Recursively search subdirectories listed
- line_buffered::Bool - Force output to be line buffered. By default, output is line buffered when standard output is a terminal and block buffered otherwise
- binary::Bool - Search binary files, but do not attempt to print them
- J::Bool - Decompress the bzip2(1) compressed file before looking for the text
- version::Bool - Print version number of grep to the standard output stream
- perl_regexp::Bool - Interpret pattern as a Perl regular expression
- file::AbstractString - Obtain patterns from FILE, one per line. The empty file contains zero patterns, and therefore matches nothing. (-f is specified by POSIX.)
"""
function grep(ctx::CommandLine, _args...; help::Union{Nothing,Bool} = false, extended_regexp::Union{Nothing,Bool} = false, fixed_string::Union{Nothing,Bool} = false, basic_regexp::Union{Nothing,Bool} = false, regexp::Union{Nothing,AbstractString} = nothing, ignore_case::Union{Nothing,Bool} = false, invert_match::Union{Nothing,Bool} = false, word_regexp::Union{Nothing,Bool} = false, line_regexp::Union{Nothing,Bool} = false, count::Union{Nothing,Bool} = false, color::Union{Nothing,AbstractString} = "auto", files_without_match::Union{Nothing,Bool} = false, files_with_matches::Union{Nothing,Bool} = false, max_count::Union{Nothing,AbstractString} = nothing, only_matching::Union{Nothing,Bool} = false, quiet::Union{Nothing,Bool} = false, no_messages::Union{Nothing,Bool} = false, byte_offset::Union{Nothing,Bool} = false, with_filename::Union{Nothing,Bool} = false, no_filename::Union{Nothing,Bool} = false, label::Union{Nothing,AbstractString} = nothing, line_number::Union{Nothing,Bool} = false, initial_tab::Union{Nothing,Bool} = false, unix_byte_offsets::Union{Nothing,Bool} = false, null::Union{Nothing,Bool} = false, after_context::Union{Nothing,AbstractString} = nothing, before_context::Union{Nothing,AbstractString} = nothing, context::Union{Nothing,AbstractString} = nothing, text::Union{Nothing,Bool} = false, binary_files::Union{Nothing,AbstractString} = "binary", devices::Union{Nothing,AbstractString} = "read", directories::Union{Nothing,AbstractString} = "read", exclude::Union{Nothing,AbstractString} = nothing, exclude_dir::Union{Nothing,AbstractString} = nothing, I::Union{Nothing,Bool} = false, include::Union{Nothing,AbstractString} = nothing, include_dir::Union{Nothing,AbstractString} = nothing, recursive::Union{Nothing,Bool} = false, line_buffered::Union{Nothing,Bool} = false, binary::Union{Nothing,Bool} = false, J::Union{Nothing,Bool} = false, version::Union{Nothing,Bool} = false, perl_regexp::Union{Nothing,Bool} = false, file::Union{Nothing,AbstractString} = nothing, )
ctx.exec() do cmdstr
cmd = [cmdstr]
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
!Base.isnothing(extended_regexp) && extended_regexp && Base.push!(cmd, "--extended-regexp")
!Base.isnothing(fixed_string) && fixed_string && Base.push!(cmd, "--fixed-string")
!Base.isnothing(basic_regexp) && basic_regexp && Base.push!(cmd, "--basic-regexp")
Base.isnothing(regexp) || Base.push!(cmd, "--regexp=$(regexp)")
!Base.isnothing(ignore_case) && ignore_case && Base.push!(cmd, "--ignore-case")
!Base.isnothing(invert_match) && invert_match && Base.push!(cmd, "--invert-match")
!Base.isnothing(word_regexp) && word_regexp && Base.push!(cmd, "--word-regexp")
!Base.isnothing(line_regexp) && line_regexp && Base.push!(cmd, "--line-regexp")
!Base.isnothing(count) && count && Base.push!(cmd, "--count")
Base.isnothing(color) || Base.push!(cmd, "--color=$(color)")
!Base.isnothing(files_without_match) && files_without_match && Base.push!(cmd, "--files-without-match")
!Base.isnothing(files_with_matches) && files_with_matches && Base.push!(cmd, "--files-with-matches")
Base.isnothing(max_count) || Base.push!(cmd, "--max-count=$(max_count)")
!Base.isnothing(only_matching) && only_matching && Base.push!(cmd, "--only-matching")
!Base.isnothing(quiet) && quiet && Base.push!(cmd, "--quiet")
!Base.isnothing(no_messages) && no_messages && Base.push!(cmd, "--no-messages")
!Base.isnothing(byte_offset) && byte_offset && Base.push!(cmd, "--byte-offset")
!Base.isnothing(with_filename) && with_filename && Base.push!(cmd, "--with-filename")
!Base.isnothing(no_filename) && no_filename && Base.push!(cmd, "--no-filename")
Base.isnothing(label) || Base.push!(cmd, "--label=$(label)")
!Base.isnothing(line_number) && line_number && Base.push!(cmd, "--line-number")
!Base.isnothing(initial_tab) && initial_tab && Base.push!(cmd, "--initial-tab")
!Base.isnothing(unix_byte_offsets) && unix_byte_offsets && Base.push!(cmd, "--unix-byte-offsets")
!Base.isnothing(null) && null && Base.push!(cmd, "--null")
Base.isnothing(after_context) || Base.push!(cmd, "--after-context=$(after_context)")
Base.isnothing(before_context) || Base.push!(cmd, "--before-context=$(before_context)")
Base.isnothing(context) || Base.push!(cmd, "--context=$(context)")
!Base.isnothing(text) && text && Base.push!(cmd, "--text")
Base.isnothing(binary_files) || Base.push!(cmd, "--binary-files=$(binary_files)")
Base.isnothing(devices) || Base.push!(cmd, "--devices=$(devices)")
Base.isnothing(directories) || Base.push!(cmd, "--directories=$(directories)")
Base.isnothing(exclude) || Base.push!(cmd, "--exclude=$(exclude)")
Base.isnothing(exclude_dir) || Base.push!(cmd, "--exclude-dir=$(exclude_dir)")
!Base.isnothing(I) && I && Base.push!(cmd, "-I")
Base.isnothing(include) || Base.push!(cmd, "--include=$(include)")
Base.isnothing(include_dir) || Base.push!(cmd, "--include-dir=$(include_dir)")
!Base.isnothing(recursive) && recursive && Base.push!(cmd, "--recursive")
!Base.isnothing(line_buffered) && line_buffered && Base.push!(cmd, "--line-buffered")
!Base.isnothing(binary) && binary && Base.push!(cmd, "--binary")
!Base.isnothing(J) && J && Base.push!(cmd, "-J")
!Base.isnothing(version) && version && Base.push!(cmd, "--version")
!Base.isnothing(perl_regexp) && perl_regexp && Base.push!(cmd, "--perl-regexp")
Base.isnothing(file) || Base.push!(cmd, "--file=$(file)")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
end # module CLI
| FigCLIGen | https://github.com/tanmaykm/FigCLIGen.jl.git |
|
[
"MIT"
] | 0.1.0 | 130a26c6126dff88bf0ad5e265cc81bb7196e38c | code | 38466 | """
CLI for opa.
Open Policy Agent (OPA)
Generated via FigCLIGen. Do not edit directly.
Edit the specification file and run the generator instead.
"""
module CLI
const OptsType = Base.Dict{Base.Symbol,Base.Any}
"""
CommandLine execution context.
`exec`: a no argument function that provides the base command to execute in a julia `do` block.
`cmdopts`: keyword arguments that should be used to further customize the `Cmd` creation
`pipelineopts`: keyword arguments that should be used to further customize the `pipeline` creation
"""
Base.@kwdef struct CommandLine
exec::Base.Function = (f) -> f("opa")
cmdopts::OptsType = OptsType()
pipelineopts::OptsType = OptsType()
runopts::OptsType = OptsType()
end
""" opa
Run the opa command.
Open Policy Agent (OPA)
Options:
- help::Bool - Help for opa
"""
function opa(ctx::CommandLine, _args...; help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr]
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" bench
Run the bench subcommand of opa command.
Benchmark a Rego query
Options:
- benchmem::Bool - Report memory allocations with benchmark results
- bundle::AbstractString - Set bundle file(s) or directory path(s). This flag can be repeated
- count::AbstractString - Number of times to repeat each benchmark
- data::AbstractString - Set policy or data file(s). This flag can be repeated
- fail::Bool - Exits with non-zero exit code on undefined/empty result and errors
- format::AbstractString - Set output format
- ignore::AbstractString - Set file and directory names to ignore during loading (e.g., '.*' excludes hidden files)
- _import::AbstractString - Set query import(s). This flag can be repeated
- input::AbstractString - Set input file path
- metrics::Bool - Report query performance metrics
- package::AbstractString - Set query package
- partial::Bool - Perform partial evaluation
- schema::AbstractString - Set schema file path or directory path
- stdin::Bool - Read query from stdin
- stdin_input::Bool - Read input document from stdin
- target::AbstractString - Set the runtime to exercise
- unknowns::AbstractString - Set paths to treat as unknown during partial evaluation
- help::Bool - Help for bench
"""
function bench(ctx::CommandLine, _args...; benchmem::Union{Nothing,Bool} = false, bundle::Union{Nothing,AbstractString} = nothing, count::Union{Nothing,AbstractString} = "1", data::Union{Nothing,AbstractString} = nothing, fail::Union{Nothing,Bool} = false, format::Union{Nothing,AbstractString} = "pretty", ignore::Union{Nothing,AbstractString} = nothing, _import::Union{Nothing,AbstractString} = nothing, input::Union{Nothing,AbstractString} = nothing, metrics::Union{Nothing,Bool} = false, package::Union{Nothing,AbstractString} = nothing, partial::Union{Nothing,Bool} = false, schema::Union{Nothing,AbstractString} = nothing, stdin::Union{Nothing,Bool} = false, stdin_input::Union{Nothing,Bool} = false, target::Union{Nothing,AbstractString} = "rego", unknowns::Union{Nothing,AbstractString} = "[input]", help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "bench"]
!Base.isnothing(benchmem) && benchmem && Base.push!(cmd, "--benchmem")
Base.isnothing(bundle) || Base.push!(cmd, "--bundle=$(bundle)")
Base.isnothing(count) || Base.push!(cmd, "--count=$(count)")
Base.isnothing(data) || Base.push!(cmd, "--data=$(data)")
!Base.isnothing(fail) && fail && Base.push!(cmd, "--fail")
Base.isnothing(format) || Base.push!(cmd, "--format=$(format)")
Base.isnothing(ignore) || Base.push!(cmd, "--ignore=$(ignore)")
Base.isnothing(_import) || Base.push!(cmd, "--import=$(_import)")
Base.isnothing(input) || Base.push!(cmd, "--input=$(input)")
!Base.isnothing(metrics) && metrics && Base.push!(cmd, "--metrics")
Base.isnothing(package) || Base.push!(cmd, "--package=$(package)")
!Base.isnothing(partial) && partial && Base.push!(cmd, "--partial")
Base.isnothing(schema) || Base.push!(cmd, "--schema=$(schema)")
!Base.isnothing(stdin) && stdin && Base.push!(cmd, "--stdin")
!Base.isnothing(stdin_input) && stdin_input && Base.push!(cmd, "--stdin-input")
Base.isnothing(target) || Base.push!(cmd, "--target=$(target)")
Base.isnothing(unknowns) || Base.push!(cmd, "--unknowns=$(unknowns)")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" build
Run the build subcommand of opa command.
Build an OPA bundle
Options:
- bundle::Bool - Load paths as bundle files or root directories
- capabilities::AbstractString - Set capabilities.json file path
- claims_file::AbstractString - Set path of JSON file containing optional claims (see: https://openpolicyagent.org/docs/latest/management/#signature-format)
- debug::Bool - Enable debug output
- entrypoint::AbstractString - Set slash separated entrypoint path
- exclude_files_verify::AbstractString - Set file names to exclude during bundle verification
- ignore::AbstractString - Set file and directory names to ignore during loading (e.g., '.*' excludes hidden files)
- optimize::AbstractString - Set optimization level
- output::AbstractString - Set the output filename
- revision::AbstractString - Set output bundle revision
- scope::AbstractString - Scope to use for bundle signature verification
- signing_alg::AbstractString - Name of the signing algorithm
- signing_key::AbstractString - Set the secret (HMAC) or path of the PEM file containing the private key (RSA and ECDSA)
- signing_plugin::AbstractString - Name of the plugin to use for signing/verification (see https://openpolicyagent.org/docs/latest/management/#signature-plugin
- target::AbstractString - Set the output bundle target type
- verification_key::AbstractString - Set the secret (HMAC) or path of the PEM file containing the public key (RSA and ECDSA)
- verification_key_id::AbstractString - Name assigned to the verification key used for bundle verification
- help::Bool - Help for build
"""
function build(ctx::CommandLine, _args...; bundle::Union{Nothing,Bool} = false, capabilities::Union{Nothing,AbstractString} = nothing, claims_file::Union{Nothing,AbstractString} = nothing, debug::Union{Nothing,Bool} = false, entrypoint::Union{Nothing,AbstractString} = nothing, exclude_files_verify::Union{Nothing,AbstractString} = nothing, ignore::Union{Nothing,AbstractString} = nothing, optimize::Union{Nothing,AbstractString} = "0", output::Union{Nothing,AbstractString} = "bundle.tar.gz", revision::Union{Nothing,AbstractString} = nothing, scope::Union{Nothing,AbstractString} = nothing, signing_alg::Union{Nothing,AbstractString} = "RS256", signing_key::Union{Nothing,AbstractString} = nothing, signing_plugin::Union{Nothing,AbstractString} = nothing, target::Union{Nothing,AbstractString} = "rego", verification_key::Union{Nothing,AbstractString} = nothing, verification_key_id::Union{Nothing,AbstractString} = "default", help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "build"]
!Base.isnothing(bundle) && bundle && Base.push!(cmd, "--bundle")
Base.isnothing(capabilities) || Base.push!(cmd, "--capabilities=$(capabilities)")
Base.isnothing(claims_file) || Base.push!(cmd, "--claims-file=$(claims_file)")
!Base.isnothing(debug) && debug && Base.push!(cmd, "--debug")
Base.isnothing(entrypoint) || Base.push!(cmd, "--entrypoint=$(entrypoint)")
Base.isnothing(exclude_files_verify) || Base.push!(cmd, "--exclude-files-verify=$(exclude_files_verify)")
Base.isnothing(ignore) || Base.push!(cmd, "--ignore=$(ignore)")
Base.isnothing(optimize) || Base.push!(cmd, "--optimize=$(optimize)")
Base.isnothing(output) || Base.push!(cmd, "--output=$(output)")
Base.isnothing(revision) || Base.push!(cmd, "--revision=$(revision)")
Base.isnothing(scope) || Base.push!(cmd, "--scope=$(scope)")
Base.isnothing(signing_alg) || Base.push!(cmd, "--signing-alg=$(signing_alg)")
Base.isnothing(signing_key) || Base.push!(cmd, "--signing-key=$(signing_key)")
Base.isnothing(signing_plugin) || Base.push!(cmd, "--signing-plugin=$(signing_plugin)")
Base.isnothing(target) || Base.push!(cmd, "--target=$(target)")
Base.isnothing(verification_key) || Base.push!(cmd, "--verification-key=$(verification_key)")
Base.isnothing(verification_key_id) || Base.push!(cmd, "--verification-key-id=$(verification_key_id)")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" check
Run the check subcommand of opa command.
Check Rego source files
Options:
- bundle::Bool - Load paths as bundle files or root directories
- capabilities::AbstractString - Set capabilities.json file path
- format::AbstractString - Set output format
- ignore::AbstractString - Set file and directory names to ignore during loading (e.g., '.*' excludes hidden files)
- max_errors::AbstractString - Set the number of errors to allow before compilation fails early
- schema::AbstractString - Set schema file path or directory path
- strict::Bool - Enable compiler strict mode
- help::Bool - Help for check
"""
function check(ctx::CommandLine, _args...; bundle::Union{Nothing,Bool} = false, capabilities::Union{Nothing,AbstractString} = nothing, format::Union{Nothing,AbstractString} = "pretty", ignore::Union{Nothing,AbstractString} = nothing, max_errors::Union{Nothing,AbstractString} = "10", schema::Union{Nothing,AbstractString} = nothing, strict::Union{Nothing,Bool} = false, help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "check"]
!Base.isnothing(bundle) && bundle && Base.push!(cmd, "--bundle")
Base.isnothing(capabilities) || Base.push!(cmd, "--capabilities=$(capabilities)")
Base.isnothing(format) || Base.push!(cmd, "--format=$(format)")
Base.isnothing(ignore) || Base.push!(cmd, "--ignore=$(ignore)")
Base.isnothing(max_errors) || Base.push!(cmd, "--max-errors=$(max_errors)")
Base.isnothing(schema) || Base.push!(cmd, "--schema=$(schema)")
!Base.isnothing(strict) && strict && Base.push!(cmd, "--strict")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" completion
Run the completion subcommand of opa command.
Generate the autocompletion script for the specified shell
Options:
- help::Bool - Help for completion
"""
function completion(ctx::CommandLine, _args...; help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "completion"]
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" deps
Run the deps subcommand of opa command.
Analyze Rego query dependencies
Options:
- bundle::AbstractString - Set bundle file(s) or directory path(s). This flag can be repeated
- data::AbstractString - Set policy or data file(s). This flag can be repeated
- format::AbstractString - Set output format
- ignore::AbstractString - Set file and directory names to ignore during loading (e.g., '.*' excludes hidden files)
- help::Bool - Help for deps
"""
function deps(ctx::CommandLine, _args...; bundle::Union{Nothing,AbstractString} = nothing, data::Union{Nothing,AbstractString} = nothing, format::Union{Nothing,AbstractString} = "pretty", ignore::Union{Nothing,AbstractString} = nothing, help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "deps"]
Base.isnothing(bundle) || Base.push!(cmd, "--bundle=$(bundle)")
Base.isnothing(data) || Base.push!(cmd, "--data=$(data)")
Base.isnothing(format) || Base.push!(cmd, "--format=$(format)")
Base.isnothing(ignore) || Base.push!(cmd, "--ignore=$(ignore)")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" eval
Run the eval subcommand of opa command.
Evaluate a Rego query
Options:
- bundle::AbstractString - Set bundle file(s) or directory path(s). This flag can be repeated
- capabilities::AbstractString - Set capabilities.json file path
- count::AbstractString - Number of times to repeat each benchmark
- coverage::Bool - Report coverage
- data::AbstractString - Set policy or data file(s). This flag can be repeated
- disable_early_exit::Bool - Disable 'early exit' optimizations
- disable_indexing::Bool - Disable indexing optimizations
- disable_inlining::AbstractString - Set paths of documents to exclude from inlining
- explain::AbstractString - Enable query explanations
- fail::Bool - Exits with non-zero exit code on undefined/empty result and errors
- fail_defined::Bool - Exits with non-zero exit code on defined/non-empty result and errors
- format::AbstractString - Set output format
- ignore::AbstractString - Set file and directory names to ignore during loading (e.g., '.*' excludes hidden files)
- _import::AbstractString - Set query import(s). This flag can be repeated
- input::AbstractString - Set input file path
- instrument::Bool - Enable query instrumentation metrics (implies --metrics)
- metrics::Bool - Report query performance metrics
- package::AbstractString - Set query package
- partial::Bool - Perform partial evaluation
- pretty_limit::AbstractString - Set limit after which pretty output gets truncated
- profile::Bool - Perform expression profiling
- profile_limit::AbstractString - Set number of profiling results to show
- profile_sort::AbstractString - Set sort order of expression profiler results
- schema::AbstractString - Set schema file path or directory path
- shallow_inlining::Bool - Disable inlining of rules that depend on unknowns
- stdin::Bool - Read query from stdin
- stdin_input::Bool - Read input document from stdin
- strict_builtin_errors::Bool - Treat built-in function errors as fatal
- target::AbstractString - Set the runtime to exercise
- timeout::AbstractString - Set eval timeout (default unlimited)
- unknowns::AbstractString - Set paths to treat as unknown during partial evaluation
- help::Bool - Help for eval
"""
function eval(ctx::CommandLine, _args...; bundle::Union{Nothing,AbstractString} = nothing, capabilities::Union{Nothing,AbstractString} = nothing, count::Union{Nothing,AbstractString} = "1", coverage::Union{Nothing,Bool} = false, data::Union{Nothing,AbstractString} = nothing, disable_early_exit::Union{Nothing,Bool} = false, disable_indexing::Union{Nothing,Bool} = false, disable_inlining::Union{Nothing,AbstractString} = nothing, explain::Union{Nothing,AbstractString} = "off", fail::Union{Nothing,Bool} = false, fail_defined::Union{Nothing,Bool} = false, format::Union{Nothing,AbstractString} = "json", ignore::Union{Nothing,AbstractString} = nothing, _import::Union{Nothing,AbstractString} = nothing, input::Union{Nothing,AbstractString} = nothing, instrument::Union{Nothing,Bool} = false, metrics::Union{Nothing,Bool} = false, package::Union{Nothing,AbstractString} = nothing, partial::Union{Nothing,Bool} = false, pretty_limit::Union{Nothing,AbstractString} = "80", profile::Union{Nothing,Bool} = false, profile_limit::Union{Nothing,AbstractString} = "10", profile_sort::Union{Nothing,AbstractString} = nothing, schema::Union{Nothing,AbstractString} = nothing, shallow_inlining::Union{Nothing,Bool} = false, stdin::Union{Nothing,Bool} = false, stdin_input::Union{Nothing,Bool} = false, strict_builtin_errors::Union{Nothing,Bool} = false, target::Union{Nothing,AbstractString} = "rego", timeout::Union{Nothing,AbstractString} = "0s", unknowns::Union{Nothing,AbstractString} = "[input]", help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "eval"]
Base.isnothing(bundle) || Base.push!(cmd, "--bundle=$(bundle)")
Base.isnothing(capabilities) || Base.push!(cmd, "--capabilities=$(capabilities)")
Base.isnothing(count) || Base.push!(cmd, "--count=$(count)")
!Base.isnothing(coverage) && coverage && Base.push!(cmd, "--coverage")
Base.isnothing(data) || Base.push!(cmd, "--data=$(data)")
!Base.isnothing(disable_early_exit) && disable_early_exit && Base.push!(cmd, "--disable-early-exit")
!Base.isnothing(disable_indexing) && disable_indexing && Base.push!(cmd, "--disable-indexing")
Base.isnothing(disable_inlining) || Base.push!(cmd, "--disable-inlining=$(disable_inlining)")
Base.isnothing(explain) || Base.push!(cmd, "--explain=$(explain)")
!Base.isnothing(fail) && fail && Base.push!(cmd, "--fail")
!Base.isnothing(fail_defined) && fail_defined && Base.push!(cmd, "--fail-defined")
Base.isnothing(format) || Base.push!(cmd, "--format=$(format)")
Base.isnothing(ignore) || Base.push!(cmd, "--ignore=$(ignore)")
Base.isnothing(_import) || Base.push!(cmd, "--import=$(_import)")
Base.isnothing(input) || Base.push!(cmd, "--input=$(input)")
!Base.isnothing(instrument) && instrument && Base.push!(cmd, "--instrument")
!Base.isnothing(metrics) && metrics && Base.push!(cmd, "--metrics")
Base.isnothing(package) || Base.push!(cmd, "--package=$(package)")
!Base.isnothing(partial) && partial && Base.push!(cmd, "--partial")
Base.isnothing(pretty_limit) || Base.push!(cmd, "--pretty-limit=$(pretty_limit)")
!Base.isnothing(profile) && profile && Base.push!(cmd, "--profile")
Base.isnothing(profile_limit) || Base.push!(cmd, "--profile-limit=$(profile_limit)")
Base.isnothing(profile_sort) || Base.push!(cmd, "--profile-sort=$(profile_sort)")
Base.isnothing(schema) || Base.push!(cmd, "--schema=$(schema)")
!Base.isnothing(shallow_inlining) && shallow_inlining && Base.push!(cmd, "--shallow-inlining")
!Base.isnothing(stdin) && stdin && Base.push!(cmd, "--stdin")
!Base.isnothing(stdin_input) && stdin_input && Base.push!(cmd, "--stdin-input")
!Base.isnothing(strict_builtin_errors) && strict_builtin_errors && Base.push!(cmd, "--strict-builtin-errors")
Base.isnothing(target) || Base.push!(cmd, "--target=$(target)")
Base.isnothing(timeout) || Base.push!(cmd, "--timeout=$(timeout)")
Base.isnothing(unknowns) || Base.push!(cmd, "--unknowns=$(unknowns)")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" exec
Run the exec subcommand of opa command.
Execute against input files
Options:
- bundle::AbstractString - Set bundle file(s) or directory path(s). This flag can be repeated
- config_file::AbstractString - Set path of configuration file
- decision::AbstractString - Set decision to evaluate
- format::AbstractString - Set output format
- log_format::AbstractString - Set log format
- log_level::AbstractString - Set log level
- set::AbstractString - Override config values on the command line (use commas to specify multiple values)
- set_file::AbstractString - Override config values with files on the command line (use commas to specify multiple values)
- help::Bool - Help for exec
"""
function exec(ctx::CommandLine, _args...; bundle::Union{Nothing,AbstractString} = nothing, config_file::Union{Nothing,AbstractString} = nothing, decision::Union{Nothing,AbstractString} = nothing, format::Union{Nothing,AbstractString} = "pretty", log_format::Union{Nothing,AbstractString} = "json", log_level::Union{Nothing,AbstractString} = "error", set::Union{Nothing,AbstractString} = nothing, set_file::Union{Nothing,AbstractString} = nothing, help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "exec"]
Base.isnothing(bundle) || Base.push!(cmd, "--bundle=$(bundle)")
Base.isnothing(config_file) || Base.push!(cmd, "--config-file=$(config_file)")
Base.isnothing(decision) || Base.push!(cmd, "--decision=$(decision)")
Base.isnothing(format) || Base.push!(cmd, "--format=$(format)")
Base.isnothing(log_format) || Base.push!(cmd, "--log-format=$(log_format)")
Base.isnothing(log_level) || Base.push!(cmd, "--log-level=$(log_level)")
Base.isnothing(set) || Base.push!(cmd, "--set=$(set)")
Base.isnothing(set_file) || Base.push!(cmd, "--set-file=$(set_file)")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" fmt
Run the fmt subcommand of opa command.
Format Rego source files
Options:
- diff::Bool - Only display a diff of the changes
- fail::Bool - Non zero exit code on reformat
- list::Bool - List all files who would change when formatted
- write::Bool - Overwrite the original source file
- help::Bool - Help for fmt
"""
function fmt(ctx::CommandLine, _args...; diff::Union{Nothing,Bool} = false, fail::Union{Nothing,Bool} = false, list::Union{Nothing,Bool} = false, write::Union{Nothing,Bool} = false, help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "fmt"]
!Base.isnothing(diff) && diff && Base.push!(cmd, "--diff")
!Base.isnothing(fail) && fail && Base.push!(cmd, "--fail")
!Base.isnothing(list) && list && Base.push!(cmd, "--list")
!Base.isnothing(write) && write && Base.push!(cmd, "--write")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" inspect
Run the inspect subcommand of opa command.
Inspect OPA bundle(s)
Options:
- format::AbstractString - Set output format
- help::Bool - Help for inspect
"""
function inspect(ctx::CommandLine, _args...; format::Union{Nothing,AbstractString} = "pretty", help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "inspect"]
Base.isnothing(format) || Base.push!(cmd, "--format=$(format)")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" parse
Run the parse subcommand of opa command.
Parse Rego source file
Options:
- format::AbstractString - Set output format
- help::Bool - Help for parse
"""
function parse(ctx::CommandLine, _args...; format::Union{Nothing,AbstractString} = "pretty", help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "parse"]
Base.isnothing(format) || Base.push!(cmd, "--format=$(format)")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" run
Run the run subcommand of opa command.
Start OPA in interactive or server mode
Options:
- addr::AbstractString - Set listening address of the server (e.g., [ip]:<port> for TCP, unix://<path> for UNIX domain socket)
- authentication::AbstractString - Set authentication scheme
- authorization::AbstractString - Set authorization scheme
- bundle::Bool - Load paths as bundle files or root directories
- config_file::AbstractString - Set path of configuration file
- diagnostic_addr::AbstractString - Set read-only diagnostic listening address of the server for /health and /metric APIs (e.g., [ip]:<port> for TCP, unix://<path> for UNIX domain socket)
- exclude_files_verify::AbstractString - Set file names to exclude during bundle verification
- format::AbstractString - Set shell output format, i.e, pretty, json
- h2c::Bool - Enable H2C for HTTP listeners
- history::AbstractString - Set path of history file
- ignore::AbstractString - Set file and directory names to ignore during loading (e.g., '.*' excludes hidden files)
- log_format::AbstractString - Set log format
- log_level::AbstractString - Set log level
- max_errors::AbstractString - Set the number of errors to allow before compilation fails early
- min_tls_version::AbstractString - Set minimum TLS version to be used by OPA's server
- pprof::Bool - Enables pprof endpoints
- ready_timeout::AbstractString - Wait (in seconds) for configured plugins before starting server (value <= 0 disables ready check)
- scope::AbstractString - Scope to use for bundle signature verification
- server::Bool - Start the runtime in server mode
- set::AbstractString - Override config values on the command line (use commas to specify multiple values)
- set_file::AbstractString - Override config values with files on the command line (use commas to specify multiple values)
- shutdown_grace_period::AbstractString - Set the time (in seconds) that the server will wait to gracefully shut down
- shutdown_wait_period::AbstractString - Set the time (in seconds) that the server will wait before initiating shutdown
- signing_alg::AbstractString - Name of the signing algorithm
- skip_verify::Bool - Disables bundle signature verification
- skip_version_check::Bool - Disables anonymous version reporting (see: https://openpolicyagent.org/docs/latest/privacy)
- tls_ca_cert_file::AbstractString - Set path of TLS CA cert file
- tls_cert_file::AbstractString - Set path of TLS certificate file
- tls_cert_refresh_period::AbstractString - Set certificate refresh period
- tls_private_key_file::AbstractString - Set path of TLS private key file
- verification_key::AbstractString - Set the secret (HMAC) or path of the PEM file containing the public key (RSA and ECDSA)
- verification_key_id::AbstractString - Name assigned to the verification key used for bundle verification
- watch::Bool - Watch command line files for changes
- help::Bool - Help for run
"""
function run(ctx::CommandLine, _args...; addr::Union{Nothing,AbstractString} = "[:8181]", authentication::Union{Nothing,AbstractString} = "off", authorization::Union{Nothing,AbstractString} = "off", bundle::Union{Nothing,Bool} = false, config_file::Union{Nothing,AbstractString} = nothing, diagnostic_addr::Union{Nothing,AbstractString} = nothing, exclude_files_verify::Union{Nothing,AbstractString} = nothing, format::Union{Nothing,AbstractString} = "pretty", h2c::Union{Nothing,Bool} = false, history::Union{Nothing,AbstractString} = "/Users/batuhan.apaydin/.opa_history", ignore::Union{Nothing,AbstractString} = nothing, log_format::Union{Nothing,AbstractString} = "json", log_level::Union{Nothing,AbstractString} = "info", max_errors::Union{Nothing,AbstractString} = "10", min_tls_version::Union{Nothing,AbstractString} = "1.2", pprof::Union{Nothing,Bool} = false, ready_timeout::Union{Nothing,AbstractString} = "0", scope::Union{Nothing,AbstractString} = nothing, server::Union{Nothing,Bool} = false, set::Union{Nothing,AbstractString} = nothing, set_file::Union{Nothing,AbstractString} = nothing, shutdown_grace_period::Union{Nothing,AbstractString} = "10", shutdown_wait_period::Union{Nothing,AbstractString} = "0", signing_alg::Union{Nothing,AbstractString} = "RS256", skip_verify::Union{Nothing,Bool} = false, skip_version_check::Union{Nothing,Bool} = false, tls_ca_cert_file::Union{Nothing,AbstractString} = nothing, tls_cert_file::Union{Nothing,AbstractString} = nothing, tls_cert_refresh_period::Union{Nothing,AbstractString} = "0s", tls_private_key_file::Union{Nothing,AbstractString} = nothing, verification_key::Union{Nothing,AbstractString} = nothing, verification_key_id::Union{Nothing,AbstractString} = "default", watch::Union{Nothing,Bool} = false, help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "run"]
Base.isnothing(addr) || Base.push!(cmd, "--addr=$(addr)")
Base.isnothing(authentication) || Base.push!(cmd, "--authentication=$(authentication)")
Base.isnothing(authorization) || Base.push!(cmd, "--authorization=$(authorization)")
!Base.isnothing(bundle) && bundle && Base.push!(cmd, "--bundle")
Base.isnothing(config_file) || Base.push!(cmd, "--config-file=$(config_file)")
Base.isnothing(diagnostic_addr) || Base.push!(cmd, "--diagnostic-addr=$(diagnostic_addr)")
Base.isnothing(exclude_files_verify) || Base.push!(cmd, "--exclude-files-verify=$(exclude_files_verify)")
Base.isnothing(format) || Base.push!(cmd, "--format=$(format)")
!Base.isnothing(h2c) && h2c && Base.push!(cmd, "--h2c")
Base.isnothing(history) || Base.push!(cmd, "--history=$(history)")
Base.isnothing(ignore) || Base.push!(cmd, "--ignore=$(ignore)")
Base.isnothing(log_format) || Base.push!(cmd, "--log-format=$(log_format)")
Base.isnothing(log_level) || Base.push!(cmd, "--log-level=$(log_level)")
Base.isnothing(max_errors) || Base.push!(cmd, "--max-errors=$(max_errors)")
Base.isnothing(min_tls_version) || Base.push!(cmd, "--min-tls-version=$(min_tls_version)")
!Base.isnothing(pprof) && pprof && Base.push!(cmd, "--pprof")
Base.isnothing(ready_timeout) || Base.push!(cmd, "--ready-timeout=$(ready_timeout)")
Base.isnothing(scope) || Base.push!(cmd, "--scope=$(scope)")
!Base.isnothing(server) && server && Base.push!(cmd, "--server")
Base.isnothing(set) || Base.push!(cmd, "--set=$(set)")
Base.isnothing(set_file) || Base.push!(cmd, "--set-file=$(set_file)")
Base.isnothing(shutdown_grace_period) || Base.push!(cmd, "--shutdown-grace-period=$(shutdown_grace_period)")
Base.isnothing(shutdown_wait_period) || Base.push!(cmd, "--shutdown-wait-period=$(shutdown_wait_period)")
Base.isnothing(signing_alg) || Base.push!(cmd, "--signing-alg=$(signing_alg)")
!Base.isnothing(skip_verify) && skip_verify && Base.push!(cmd, "--skip-verify")
!Base.isnothing(skip_version_check) && skip_version_check && Base.push!(cmd, "--skip-version-check")
Base.isnothing(tls_ca_cert_file) || Base.push!(cmd, "--tls-ca-cert-file=$(tls_ca_cert_file)")
Base.isnothing(tls_cert_file) || Base.push!(cmd, "--tls-cert-file=$(tls_cert_file)")
Base.isnothing(tls_cert_refresh_period) || Base.push!(cmd, "--tls-cert-refresh-period=$(tls_cert_refresh_period)")
Base.isnothing(tls_private_key_file) || Base.push!(cmd, "--tls-private-key-file=$(tls_private_key_file)")
Base.isnothing(verification_key) || Base.push!(cmd, "--verification-key=$(verification_key)")
Base.isnothing(verification_key_id) || Base.push!(cmd, "--verification-key-id=$(verification_key_id)")
!Base.isnothing(watch) && watch && Base.push!(cmd, "--watch")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" sign
Run the sign subcommand of opa command.
Generate an OPA bundle signature
Options:
- bundle::Bool - Load paths as bundle files or root directories
- claims_file::AbstractString - Set path of JSON file containing optional claims (see: https://openpolicyagent.org/docs/latest/management/#signature-format)
- output_file_path::AbstractString - Set the location for the .signatures.json file
- signing_alg::AbstractString - Name of the signing algorithm
- signing_key::AbstractString - Set the secret (HMAC) or path of the PEM file containing the private key (RSA and ECDSA)
- signing_plugin::AbstractString - Name of the plugin to use for signing/verification (see https://openpolicyagent.org/docs/latest/management/#signature-plugin
- help::Bool - Help for sign
"""
function sign(ctx::CommandLine, _args...; bundle::Union{Nothing,Bool} = false, claims_file::Union{Nothing,AbstractString} = nothing, output_file_path::Union{Nothing,AbstractString} = ".", signing_alg::Union{Nothing,AbstractString} = "RS256", signing_key::Union{Nothing,AbstractString} = nothing, signing_plugin::Union{Nothing,AbstractString} = nothing, help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "sign"]
!Base.isnothing(bundle) && bundle && Base.push!(cmd, "--bundle")
Base.isnothing(claims_file) || Base.push!(cmd, "--claims-file=$(claims_file)")
Base.isnothing(output_file_path) || Base.push!(cmd, "--output-file-path=$(output_file_path)")
Base.isnothing(signing_alg) || Base.push!(cmd, "--signing-alg=$(signing_alg)")
Base.isnothing(signing_key) || Base.push!(cmd, "--signing-key=$(signing_key)")
Base.isnothing(signing_plugin) || Base.push!(cmd, "--signing-plugin=$(signing_plugin)")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" test
Run the test subcommand of opa command.
Execute Rego test cases
Options:
- bench::Bool - Benchmark the unit tests
- benchmem::Bool - Report memory allocations with benchmark results
- bundle::Bool - Load paths as bundle files or root directories
- count::AbstractString - Number of times to repeat each test
- coverage::Bool - Report coverage (overrides debug tracing)
- exit_zero_on_skipped::Bool - Skipped tests return status 0
- explain::AbstractString - Enable query explanations
- format::AbstractString - Set output format
- ignore::AbstractString - Set file and directory names to ignore during loading (e.g., '.*' excludes hidden files)
- max_errors::AbstractString - Set the number of errors to allow before compilation fails early
- run::AbstractString - Run only test cases matching the regular expression
- show_failure_line::Bool - Show test failure line
- target::AbstractString - Set the runtime to exercise
- threshold::AbstractString - Set coverage threshold and exit with non-zero status if coverage is less than threshold %
- timeout::AbstractString - Set test timeout (default 5s, 30s when benchmarking)
- verbose::Bool - Set verbose reporting mode
- help::Bool - Help for test
"""
function test(ctx::CommandLine, _args...; bench::Union{Nothing,Bool} = false, benchmem::Union{Nothing,Bool} = false, bundle::Union{Nothing,Bool} = false, count::Union{Nothing,AbstractString} = "1", coverage::Union{Nothing,Bool} = false, exit_zero_on_skipped::Union{Nothing,Bool} = false, explain::Union{Nothing,AbstractString} = "fails", format::Union{Nothing,AbstractString} = "pretty", ignore::Union{Nothing,AbstractString} = nothing, max_errors::Union{Nothing,AbstractString} = "10", run::Union{Nothing,AbstractString} = nothing, show_failure_line::Union{Nothing,Bool} = false, target::Union{Nothing,AbstractString} = "rego", threshold::Union{Nothing,AbstractString} = "0", timeout::Union{Nothing,AbstractString} = "0s", verbose::Union{Nothing,Bool} = false, help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "test"]
!Base.isnothing(bench) && bench && Base.push!(cmd, "--bench")
!Base.isnothing(benchmem) && benchmem && Base.push!(cmd, "--benchmem")
!Base.isnothing(bundle) && bundle && Base.push!(cmd, "--bundle")
Base.isnothing(count) || Base.push!(cmd, "--count=$(count)")
!Base.isnothing(coverage) && coverage && Base.push!(cmd, "--coverage")
!Base.isnothing(exit_zero_on_skipped) && exit_zero_on_skipped && Base.push!(cmd, "--exit-zero-on-skipped")
Base.isnothing(explain) || Base.push!(cmd, "--explain=$(explain)")
Base.isnothing(format) || Base.push!(cmd, "--format=$(format)")
Base.isnothing(ignore) || Base.push!(cmd, "--ignore=$(ignore)")
Base.isnothing(max_errors) || Base.push!(cmd, "--max-errors=$(max_errors)")
Base.isnothing(run) || Base.push!(cmd, "--run=$(run)")
!Base.isnothing(show_failure_line) && show_failure_line && Base.push!(cmd, "--show-failure-line")
Base.isnothing(target) || Base.push!(cmd, "--target=$(target)")
Base.isnothing(threshold) || Base.push!(cmd, "--threshold=$(threshold)")
Base.isnothing(timeout) || Base.push!(cmd, "--timeout=$(timeout)")
!Base.isnothing(verbose) && verbose && Base.push!(cmd, "--verbose")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" version
Run the version subcommand of opa command.
Print the version of OPA
Options:
- check::Bool - Check for latest OPA release
- help::Bool - Help for version
"""
function version(ctx::CommandLine, _args...; check::Union{Nothing,Bool} = false, help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "version"]
!Base.isnothing(check) && check && Base.push!(cmd, "--check")
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""" help
Run the help subcommand of opa command.
Help about any command
Options:
- help::Bool - Help for help
"""
function help(ctx::CommandLine, _args...; help::Union{Nothing,Bool} = false, )
ctx.exec() do cmdstr
cmd = [cmdstr, "help"]
!Base.isnothing(help) && help && Base.push!(cmd, "--help")
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
end # module CLI
| FigCLIGen | https://github.com/tanmaykm/FigCLIGen.jl.git |
|
[
"MIT"
] | 0.1.0 | 130a26c6126dff88bf0ad5e265cc81bb7196e38c | code | 78 | module FigCLIGen
using JSON
include("generator.jl")
end # module FigCLIGen
| FigCLIGen | https://github.com/tanmaykm/FigCLIGen.jl.git |
|
[
"MIT"
] | 0.1.0 | 130a26c6126dff88bf0ad5e265cc81bb7196e38c | code | 6321 | const sdir = @__DIR__
const SPEC = joinpath(sdir, "opa.json")
const CLIMODULE = joinpath(sdir, "cli.jl")
const RESERVED_WORDS = [
"if", "else", "elseif", "while", "for", "begin", "end", "quote",
"try", "catch", "return", "local", "function", "macro", "ccall", "finally", "break", "continue",
"global", "module", "using", "import", "export", "const", "let", "do", "baremodule",
"Type", "Enum", "Any", "DataType", "Base"
]
function default_base_command(spec::Dict{String,Any})
return """
\"\"\"
CommandLine execution context.
`exec`: a no argument function that provides the base command to execute in a julia `do` block.
`cmdopts`: keyword arguments that should be used to further customize the `Cmd` creation
`pipelineopts`: keyword arguments that should be used to further customize the `pipeline` creation
\"\"\"
Base.@kwdef struct CommandLine
exec::Base.Function = (f) -> f(\"$(spec["name"])\")
cmdopts::OptsType = OptsType()
pipelineopts::OptsType = OptsType()
runopts::OptsType = OptsType()
end
"""
end
function wrap_module(f, io::IO, spec::Dict{String,Any}; custom_include::Union{Nothing,AbstractString}=nothing, ignore_base_command::Bool=false)
println(io, """
\"\"\"
CLI for $(spec["name"]).
$(spec["description"])
Generated via $(@__MODULE__). Do not edit directly.
Edit the specification file and run the generator instead.
\"\"\"
module CLI
const OptsType = Base.Dict{Base.Symbol,Base.Any}
""")
if !isnothing(custom_include)
println(io, custom_include)
end
if !ignore_base_command
println(io, default_base_command(spec))
end
f(io, spec)
println(io, """
end # module CLI
""")
return nothing
end
function strip_leading_dashes(s::AbstractString)
if startswith(s, "--")
return strip_leading_dashes(s[3:end])
elseif startswith(s, "-")
return strip_leading_dashes(s[2:end])
else
return s
end
end
function convert_dashes_to_underscores(s::AbstractString)
return replace(s, "-" => "_")
end
function escape_reserved_word(s::AbstractString)
if s in RESERVED_WORDS
return "_$(s)"
else
return s
end
end
function get_option_name(option::Dict{String,Any})
optname = optname_spec = option["name"]
if isa(optname_spec, Vector)
for n in optname
if startswith(n, "--")
optname = n
break
end
end
if isa(optname, Vector)
optname = first(optname_spec)
end
end
julia_optname = escape_reserved_word(convert_dashes_to_underscores(strip_leading_dashes(optname)))
return julia_optname, optname
end
function gen_command(io::IO, spec::Dict{String,Any}; parent::Union{Nothing,String}=nothing)
name = spec["name"]
description = get(spec, "description", "")
options = get(spec, "options", Dict{String,Any}())
docstring = """$(name)
Run the $(name)"""
if !isnothing(parent)
docstring *= " subcommand of $(parent) command."
else
docstring *= " command."
end
if !isempty(description)
docstring *= "\n$(description)"
end
kwargs_str = ""
kwargs = []
if !isempty(options)
docstring *= "\n\nOptions:\n"
kwargs_str = "; "
for option in options
julia_optname, optname = get_option_name(option)
isbool = !(haskey(option, "args") && !isempty(option["args"]))
opttype = isbool ? "Bool" : "AbstractString"
opt_default = if isbool
"false"
else
default = get(option["args"], "default", nothing)
if isnothing(default)
"nothing"
else
"\"$(default)\""
end
end
docstring *= "- $(julia_optname)::$(opttype) - $(option["description"])\n"
kwargs_str *= "$(julia_optname)::Union{Nothing,$(opttype)} = $(opt_default), "
push!(kwargs, (optname, julia_optname, isbool))
end
end
println(io, """\"\"\" $(docstring) \"\"\"
function $(name)(ctx::CommandLine, _args...$(kwargs_str))
ctx.exec() do cmdstr""")
if isnothing(parent)
println(io, """ cmd = [cmdstr]""")
else
println(io, """ cmd = [cmdstr, \"$(name)\"]""")
end
for (optname, julia_optname, isbool) in kwargs
if isbool
println(io, """ !Base.isnothing($(julia_optname)) && $(julia_optname) && Base.push!(cmd, \"$(optname)\")""")
else
println(io, """ Base.isnothing($(julia_optname)) || Base.push!(cmd, \"$(optname)=\$($julia_optname)\")""")
end
end
println(io, """
Base.append!(cmd, Base.string.(_args))
Base.run(Base.pipeline(Base.Cmd(Cmd(cmd); ctx.cmdopts...); ctx.pipelineopts...); ctx.runopts...)
end
end
""")
end
function generate_internals(io, spec)
gen_command(io, spec)
subcommands = get(spec, "subcommands", Dict{String,Any}())
for subcommand in subcommands
gen_command(io, subcommand; parent=spec["name"])
end
end
"""
Generate a Julia module for a CLI based on a specification file.
Arguments:
- `specfile`: path to the specification file
- `outputfile`: path to the output file
Keyword arguments:
- `custom_include`: a string to include at the top of the generated module
- `ignore_base_command`: if `true`, do not generate the base command.
The base command must be made available by the caller,
either by including it in `custom_include` or by
defining it in the module before calling `generate`.
"""
function generate(specfile::AbstractString, outputfile::AbstractString; kwargs...)
spec = JSON.parsefile(specfile)
generate(spec, outputfile; kwargs...)
end
function generate(spec::Dict{String,Any}, outputfile::AbstractString; kwargs...)
open(outputfile, "w") do io
generate(spec::Dict{String,Any}, io; kwargs...)
end
end
function generate(spec::Dict{String,Any}, io::IO; kwargs...)
wrap_module(generate_internals, io, spec; kwargs...)
return nothing
end
| FigCLIGen | https://github.com/tanmaykm/FigCLIGen.jl.git |
|
[
"MIT"
] | 0.1.0 | 130a26c6126dff88bf0ad5e265cc81bb7196e38c | code | 441 | include("grep.jl")
outbuf_stdout = IOBuffer()
outbuf_stderr = IOBuffer()
ctx = CLI.CommandLine(; exec = (f)->f("grep"))
ctx.pipelineopts[:stdout] = outbuf_stdout
ctx.pipelineopts[:stderr] = outbuf_stderr
CLI.grep(ctx, "CLI", "greptest.jl"; ignore_case=true, count=true)
output = strip(string(strip(String(take!(outbuf_stderr))), strip(String(take!(outbuf_stdout)))))
if output != "2"
error("expected output to be 2, got $output")
end | FigCLIGen | https://github.com/tanmaykm/FigCLIGen.jl.git |
|
[
"MIT"
] | 0.1.0 | 130a26c6126dff88bf0ad5e265cc81bb7196e38c | code | 1196 | using Test
using FigCLIGen
function rungen()
mktempdir() do tmpdir
for file in readdir(joinpath(@__DIR__, "specs"))
if endswith(file, ".json")
testname = replace(file, ".json" => "")
specfile = joinpath(@__DIR__, "specs", file)
outputfile = joinpath(tmpdir, testname * ".jl")
@info("testing", specfile, outputfile)
@eval @testset $testname begin
FigCLIGen.generate($specfile, $outputfile)
@test isfile($outputfile)
end
end
end
end
end
function test_grep()
mktempdir() do tmpdir
specfile = joinpath(@__DIR__, "specs", "grep.json")
outputfile = joinpath(tmpdir, "grep.jl")
FigCLIGen.generate(specfile, outputfile)
@test isfile(outputfile)
cp(joinpath(@__DIR__, "greptest.jl"), joinpath(tmpdir, "greptest.jl"))
cd(tmpdir) do
proc = run(`julia greptest.jl`)
@test proc.exitcode == 0
end
end
end
@testset "FigCLIGen" begin
rungen()
# run test_grep only on Linux
if Sys.islinux()
test_grep()
end
end
| FigCLIGen | https://github.com/tanmaykm/FigCLIGen.jl.git |
|
[
"MIT"
] | 0.1.0 | 130a26c6126dff88bf0ad5e265cc81bb7196e38c | docs | 3405 | # FigCLIGen.jl
[](https://github.com/tanmaykm/FigCLIGen.jl/actions?query=workflow%3ACI+branch%3Amain)
[](http://codecov.io/github/tanmaykm/FigCLIGen.jl?branch=main)
Generate Julia code that provide functions to run CLI programs.
The specification to generate this code is similar to what [Fig](https://fig.io/) uses, but in JSON format. So for popular CLIs, a starting point can be the spec found in the [Fig autocomplete repo](https://github.com/withfig/autocomplete). Remember to convert from `js` to `json` format.
## Code generation
```julia
generate(
specfile::AbstractString,
outputfile::AbstractString;
kwargs...
)
```
Generate a Julia module for a CLI based on a specification file.
Refer to [Generated Code Structure](#generated-code-structure) for more details of the code generated.
Arguments:
- `specfile`: path to the specification file
- `outputfile`: path to the output file
Keyword arguments:
- `custom_include`: a string to include at the top of the generated module
- `ignore_base_command`: if `true`, do not generate the base command.
The base command must be made available by the caller,
either by including it in `custom_include` or by
defining it in the module before calling `generate`.
## Generated Code Structure
Code is generated into a module named `CLI`. The module name is fixed, the intent being for it to be wrapped within another module or package.
The primary command line options are generated as methods within the module, with names same as the option name. The optional parameters are keyword arguments. And the methods accept other arguments to be passed to the command.
An example that generates a CLI for `grep` is included:
- `grep` [specs](test/specs/grep.json)
- `grep` [generated code](examples/grep.jl)
- `grep` [example use of generated code](test/greptest.jl)
A more complex spec is that of Open Policy Agent:
- `opa` [specs](test/specs/opa.json)
- `opa` [generated code](examples/opa.jl)
Example:
```julia
function grep(
ctx::CommandLine,
_args...;
help::Union{Nothing,Bool} = false,
extended_regexp::Union{Nothing,Bool} = false,
...,
file::Union{Nothing,AbstractString} = nothing,
)
```
Each method accepts an instance of `CommandLine` as the first argument that holds the execution context. It has the following members:
- `exec`: a no argument function that provides the base command to execute in a julia `do` block.
- `cmdopts`: keyword arguments that should be used to further customize the `Cmd` creation
- `pipelineopts`: keyword arguments that should be used to further customize the `pipeline` creation
`CommandLine` is generated by default, and is termed as the "base command". It can be overridden during code generation by passing the optional `ignore_base_command` and `custom_include` keyword arguments. See ["Code generation"](#code-generation).
## Using the Generated Code
Create a `CommandLine` and invoke methods. Example:
```julia
julia> include("grep.jl");
julia> ctx = CLI.CommandLine();
julia> CLI.grep(ctx, "CLI", "grep.jl"; ignore_case=true, count=true);
4
julia> CLI.grep(ctx, "the", "grep.jl"; ignore_case=true, count=true);
40
```
| FigCLIGen | https://github.com/tanmaykm/FigCLIGen.jl.git |
|
[
"MIT"
] | 0.5.0 | 777bb6bb7ffa65b15c5f3ca8d9ef92d8efa7ffe7 | code | 358 | module PkgUtils
# deps
using Pkg, Pkg.TOML, Statistics, LightGraphs, MetaGraphs
using SnoopCompile
# file includes
include("dependencies.jl")
# include("treeshaker.jl")
include("snapshot.jl")
include("environments.jl")
# exports
export get_dependents, get_dependencies, get_latest_version
export snapshot!, undo!
export @project!_str, @manifest!_str
end
| PkgUtils | https://github.com/arnavs/PkgUtils.jl.git |
|
[
"MIT"
] | 0.5.0 | 777bb6bb7ffa65b15c5f3ca8d9ef92d8efa7ffe7 | code | 3259 | # Thanks to Seth Bromberger for writing almost all of this code, and giving permission to use it
REGDIR = joinpath(DEPOT_PATH[1], "registries", "General")
version_in_range(v, s) = v in Pkg.Types.VersionSpec(s)
get_pkg_dir(s) = "$REGDIR/$(uppercase(s[1]))/$s"
# "Internal" utils and build the graph.
"""
Get all the dependencies of a package by
reading Deps.toml.
"""
function get_dep_dict(s)
deps = Dict{String, Vector{String}}()
d = get_pkg_dir(s)
vrange = ""
!isfile("$d/Deps.toml") && return deps
for l in readlines("$d/Deps.toml")
if startswith(l, "[")
vrange = strip(l, ['[',']','\"'])
# println("l = $l, vrange = $vrange")
deps[vrange] = Vector{String}()
else
pkg = split(l, " ")[1]
if pkg != ""
push!(deps[vrange], pkg)
end
end
end
return deps
end
"""
Get the latest version of a package by
reading Versions.toml.
"""
function get_latest_version(s)
d = get_pkg_dir(s)
maxver = v"0.0.0"
!isfile("$d/Versions.toml") && return maxver
for l in readlines("$d/Versions.toml")
if startswith(l, "[")
v = VersionNumber(strip(l, ['[',']','\"']))
if v > maxver
maxver = v
end
end
end
return maxver
end
"""
Given a package name, return a vector
of all _direct_ depedencies.
"""
function get_deps(s)
depdict = get_dep_dict(s)
depset = Set{String}()
maxver = get_latest_version(s)
for (vrange, deps) in depdict
# println("vrange = $vrange, deps = $deps")
if version_in_range(maxver, vrange)
union!(depset, deps)
end
end
return collect(depset)
end
function make_pkg_list(r, omit_packages)
f = "$r/Registry.toml"
t = Pkg.TOML.parsefile(f)["packages"]
omitset = Set(omit_packages)
pkglist = Set(v["name"] for (_, v) in t)
for p in pkglist
# println("making $p")
depset = Set(get_deps(p))
union!(pkglist, depset)
end
setdiff!(pkglist, omitset)
return sort(collect(pkglist))
end
function build_graph(r=REGDIR; omit_packages=[])
pkglist = make_pkg_list(r, omit_packages)
pkgrev = Dict((k, v) for (v, k) in enumerate(pkglist))
g = MetaDiGraph(length(pkglist))
for p in pkglist
v = pkgrev[p]
set_prop!(g, v, :name, p)
deps = get_deps(p)
setdiff!(deps, omit_packages)
for d in deps
w = pkgrev[d]
add_edge!(g, v, w)
end
end
set_indexing_prop!(g, :name)
return (g, reverse(g), pkglist, pkgrev)
end
function __init__()
global g, rev_g, pkglist, pkgrev = build_graph()
end
#===
"Customer-facing" functions.
===#
"""
See all n-th order dependents of a package.
"""
function get_dependents(s, n = 1)
s_index = pkgrev[s]
new_g = egonet(rev_g, s_index, n)
dependents = [props(new_g, dependent)[:name] for dependent in unique(vertices(new_g)[2:end])]
end
"""
See all n-th order dependencies of a package.
"""
function get_dependencies(s, n = 1)
s_index = pkgrev[s]
new_g = egonet(g, s_index, n)
dependents = [props(new_g, dependent)[:name] for dependent in unique(vertices(new_g)[2:end])]
end
| PkgUtils | https://github.com/arnavs/PkgUtils.jl.git |
|
[
"MIT"
] | 0.5.0 | 777bb6bb7ffa65b15c5f3ca8d9ef92d8efa7ffe7 | code | 1302 | """
manifest!"..."
Create and activate a throwaway environment based on the contents of a `Manifest.toml`.
The environment is deleted at exit.
Use this macro to paste a manifest file into a Julia script,
ensuring that it will always run with the exact set of desired dependencies.
"""
macro manifest!_str(s::AbstractString)
quote
env = _env_dir()
write(joinpath(env, "Manifest.toml"), $s)
deps = Dict(name => pkgs[1]["uuid"] for (name, pkgs) in TOML.parse($s))
project = Dict("deps" => deps)
open(io -> TOML.print(io, project), joinpath(env, "Project.toml"), "w")
_activate(env)
end
end
"""
project!"..."
Create and activate a throwaway environment based on the contents of a `Project.toml`.
The environment is deleted at exit.
Use this macro to paste a project file into a Julia script,
ensuring that it will always run with the defined set of desired dependencies.
"""
macro project!_str(s::AbstractString)
quote
env = _env_dir()
write(joinpath(env, "Project.toml"), $s)
_activate(env)
end
end
function _env_dir()
dir = mktempdir()
atexit(() -> rm(dir; recursive=true, force=true))
return dir
end
function _activate(env::AbstractString)
Pkg.activate(env)
Pkg.instantiate()
end
| PkgUtils | https://github.com/arnavs/PkgUtils.jl.git |
|
[
"MIT"
] | 0.5.0 | 777bb6bb7ffa65b15c5f3ca8d9ef92d8efa7ffe7 | code | 807 | function snapshot!()
ctx = Pkg.Types.Context();
proj = ctx.env.project_file;
man = ctx.env.manifest_file;
cp(proj, joinpath(dirname(proj), "project_snapshot.toml"), force = true);
cp(man, joinpath(dirname(proj), "manifest_snapshot.toml"), force = true);
end
function undo!()
ctx = Pkg.Types.Context();
snap_proj = joinpath(dirname(ctx.env.project_file), "project_snapshot.toml")
snap_man = joinpath(dirname(ctx.env.manifest_file), "manifest_snapshot.toml")
if isfile(snap_proj) && isfile(snap_man)
cp(snap_proj, joinpath(dirname(snap_proj), "Project.toml"), force = true);
cp(snap_man, joinpath(dirname(snap_proj), "Manifest.toml"), force = true);
Pkg.activate(dirname(snap_proj))
else
@info "No valid snapshot found."
end
end | PkgUtils | https://github.com/arnavs/PkgUtils.jl.git |
|
[
"MIT"
] | 0.5.0 | 777bb6bb7ffa65b15c5f3ca8d9ef92d8efa7ffe7 | code | 649 | # See @ianshmean for name
"""
Returns a list of dependencies required by a package that aren't actually used.
"""
function trim_dependencies(s)
named_deps = get_dependencies(s)
test_path = joinpath(Pkg.dir(s), "test", "runtests.jl")
@info "Snooping $test_path"
# snoop the tests
SnoopCompile.@snoopc "/tmp/$s.log" begin
using ColorTypes, Pkg
include(joinpath(dirname(dirname(pathof(ColorTypes))), "test", "runtests.jl"))
end
# parse the test coverage
snoopfile = read("/tmp/$s.log")
used_deps = filter(dep -> occursin(dep, snoopfile), named_deps)
return setdiff(named_deps, used_deps)
end
| PkgUtils | https://github.com/arnavs/PkgUtils.jl.git |
|
[
"MIT"
] | 0.5.0 | 777bb6bb7ffa65b15c5f3ca8d9ef92d8efa7ffe7 | code | 225 | deps = get_dependencies("Expectations")
@test deps == ["Compat", "Distributions", "FastGaussQuadrature", "SpecialFunctions"]
@test PkgUtils.get_latest_version("Expectations") == v"1.4.0" # will fail if this version is bumped
| PkgUtils | https://github.com/arnavs/PkgUtils.jl.git |
|
[
"MIT"
] | 0.5.0 | 777bb6bb7ffa65b15c5f3ca8d9ef92d8efa7ffe7 | code | 934 | function do_env_test(min::VersionNumber, max::VersionNumber)
if isdefined(Pkg, :dependencies)
deps = Pkg.dependencies()
uuid = UUID("7876af07-990d-54b4-ab0e-23690620f79a")
@test haskey(deps, uuid) && min <= deps[uuid].version <= max
else
deps = Pkg.installed()
@test haskey(deps, "Example") && min <= deps["Example"] <= max
end
end
proj = Base.active_project()
try
project!"""
[deps]
Example = "7876af07-990d-54b4-ab0e-23690620f79a"
[compat]
Example = "0.4"
"""
do_env_test(v"0.4", v"0.4.99")
manifest!"""
# This file is machine-generated - editing it directly is not advised
[[Example]]
git-tree-sha1 = "46e44e869b4d90b96bd8ed1fdcf32244fddfb6cc"
uuid = "7876af07-990d-54b4-ab0e-23690620f79a"
version = "0.5.3"
"""
do_env_test(v"0.5.3", v"0.5.3")
finally
proj === nothing ? Pkg.activate() : Pkg.activate(proj)
end
| PkgUtils | https://github.com/arnavs/PkgUtils.jl.git |
|
[
"MIT"
] | 0.5.0 | 777bb6bb7ffa65b15c5f3ca8d9ef92d8efa7ffe7 | code | 200 | using PkgUtils
using Pkg, Test, UUIDs
@elapsed begin
@time @testset "Dependencies" begin include("dependencies.jl") end
@time @testset "Environments" begin include("environments.jl") end
end
| PkgUtils | https://github.com/arnavs/PkgUtils.jl.git |
|
[
"MIT"
] | 0.5.0 | 777bb6bb7ffa65b15c5f3ca8d9ef92d8efa7ffe7 | docs | 1356 | # PkgUtils
[](https://travis-ci.org/arnavs/PkgUtils.jl)
Some small utilities to help Julia package users and authors. This package is basically a smorgasbord of code that others have submitted and I could never have written myself. https://github.com/ianshmean and https://github.com/harryscholes also provided lots of emotional support.
## Environments
* `manifest!"..."` creates and activates a throwaway environment based on the contents of a `Manifest.toml`.
* `project!"..."` creates and activates a throwaway environment based on the contents of a `Project.toml`.
Thanks to https://github.com/christopher-DG and https://github.com/oxinabox for this feature.
## Dependencies and Dependents
* `get_dependents("MyPackage", n = 1)` returns n-th order dependents of `MyPackage` (in the `General` registry.)
* `get_dependencies("SomePackage", n = 1)` returns n-th order dependencies of `SomePackage`.
* `get_latest_version("SomePackage")` will get the latest version of that package.
Thanks to https://github.com/sbromberger for most of this code.
## Snapshot
* `snapshot!()` will save a copy of your current project and manifest file in the relevant `environments/` folder.
* `undo!()` will revert the current project and manifest to the most recent snapshot.
| PkgUtils | https://github.com/arnavs/PkgUtils.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | code | 1191 | using Plots, SumOfExpVPMR
function direct_sum(f::Function, q_1::Vector{T}, q_2::Vector{T}, x::Vector{T}) where{T}
sum_result = zero(T)
N = length(x)
for i in 1:N
for j in 1:N
sum_result += q_1[i] * q_2[j] * f(abs(x[i] - x[j]))
end
end
return sum_result
end
begin
f = x -> exp(-x^2)
soepara, σ = VPMR_cal(f, 6.0, 60, 200, 16)
end
begin
time_direct = Float64[]
time_FET = Float64[]
# warm up
direct_sum(f, rand(2), rand(2), rand(2))
FET1d(rand(2), rand(2), rand(2), soepara)
N_array = [100, 200, 400, 800, 1600, 3200, 6400, 12800, 25600]
for N in N_array
q_1 = rand(N)
q_2 = rand(N)
x = rand(N)
sort_x = sortperm(x)
push!(time_direct, @elapsed direct_sum(f, q_1, q_2, x))
push!(time_FET, @elapsed FET1d(q_1, q_2, x, soepara, sort_x = sort_x))
end
end
begin
plot(xlabel = "log(N)", ylabel = "log(time)", dpi = 500, title = "Compare direct sum and FET")
plot!(log10.(N_array), log10.(time_direct), label = "direct sum", marker = :circle)
plot!(log10.(N_array), log10.(time_FET), label = "FET", marker = :square)
savefig("FET.png")
end | SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | code | 861 | # Approximating Gaussian
using Plots, SumOfExpVPMR
begin
f = x -> exp(-x^2)
# 4 terms approximation
sw4, σ4 = VPMR_cal(f, 4.0, 40, 100, 4)
error4 = soe_error(f, sw4)
# 8 terms approximation
sw8, σ8 = VPMR_cal(f, 4.0, 40, 100, 8)
error8 = soe_error(f, sw8)
# 12 terms approximation
sw12, σ12 = VPMR_cal(f, 4.0, 40, 100, 12)
error12 = soe_error(f, sw12)
# 16 terms approximation
sw16, σ16 = VPMR_cal(f, 6.0, 60, 200, 16)
error16 = soe_error(f, sw16)
end
begin
x = [0.0:0.01:10.0...]
plot(xlabel = "x", ylabel = "error", dpi = 500, title = "Approximating Gaussian via soe")
plot!(x, log10.(error4), label = "p = 4")
plot!(x, log10.(error8), label = "p = 8")
plot!(x, log10.(error12), label = "p = 12")
plot!(x, log10.(error16), label = "p = 16")
savefig("Gaussian.png")
end | SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | code | 1224 | struct GaussParameter{T}
sw::Vector{NTuple{2, T}}
end
function GaussParameter(Step::Int)
legendre_sw = legendre(Step)
return GaussParameter{Float64}([tuple(legendre_sw[1][i], legendre_sw[2][i]) for i in 1:Step])
end
function GaussParameter(T::DataType, Step::Int)
legendre_sw = legendre(T, Step)
return GaussParameter{T}([tuple(legendre_sw[1][i], legendre_sw[2][i]) for i in 1:Step])
end
@inline function Gauss_int(integrand::Function, Gaussian::GaussParameter{TG}; region::NTuple{2, T} = ((-1.0), (1.0))) where {T <: Number, TG <: Number}
a, b = TG.(region)
result = zero(TG)
for i in 1:size(Gaussian.sw, 1)
result += integrand((b + a) / TG(2) + (b - a) * Gaussian.sw[i][1] / TG(2)) * (b - a) * Gaussian.sw[i][2] / TG(2)
end
return result
end
@inline function Gauss_int_vector(integrand::Function, Gaussian::GaussParameter{TG}; region::NTuple{2, T} = (-1.0, 1.0)) where {T <: Number, TG <: Number}
a, b = TG.(region)
result = zeros(TG, size(Gaussian.sw, 1))
for i in 1:size(Gaussian.sw, 1)
result[i] = integrand((b + a) / TG(2) + (b - a) * Gaussian.sw[i][1] / TG(2)) * (b - a) * Gaussian.sw[i][2] / TG(2)
end
return result
end | SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | code | 973 | function MR_cal(s::Vector{BigFloat}, w::Vector{BigFloat}, p::Int; T1::DataType = ComplexF64, T2::DataType = Float64, digit::Int = 1024)
@assert iszero(digit % 256)
s, w, σ = setprecision(digit) do
MR(s, w, p)
end
return T1.(s), T1.(w), T2(σ)
end
function MR(s::Vector{BigFloat}, w::Vector{BigFloat}, p::Int)
n = length(s)
@assert p ≤ n
A = diagm(- s)
B = sqrt.(abs.(w))
C = sign.(w) .* B
P = lyapc(A, B * B')
Q = lyapc(A, C * C')
S = cholesky(P).L
L = cholesky(Q).L
STL = S' * L
F = svd(STL)
Tt = S * F.U * diagm(inv.(sqrt.(F.S)))
At = inv(Tt) * A * Tt
Bt = inv(Tt) * B
Ct = C' * Tt
σ = 2.0 * sum([F.S[i] for i in p+1:n])
Ad = At[1:p, 1:p]
Bd = Bt[1:p]
Cd = (Ct[1:p])'
Ad_eigen = eigen(Ad)
s = - Ad_eigen.values
B_mr = inv(Ad_eigen.vectors) * Bd
C_mr = Cd * Ad_eigen.vectors
w = [C_mr[i] * B_mr[i] for i in 1:p]
return s, w, σ
end | SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | code | 1414 | module SumOfExpVPMR
using LinearAlgebra, SpecialFunctions, GaussQuadrature, SpecialFunctions, MatrixEquations, GenericLinearAlgebra, GenericSchur
export GaussParameter, Gauss_int
export VP_cal, MR_cal, VPMR_cal
export soe, soe_error, max_error, SoePara
export FET1d
include("Gaussian_integral.jl")
include("VP.jl")
include("MR.jl")
include("soe.jl")
include("fast_exp_transform.jl")
function VPMR_cal(f::Function, nc::T, n::Int, N::Int, p::Int; region::Tuple{TR1, TR2} = (0.0, π), T1::DataType = ComplexF64, T2::DataType = Float64, digit::Int = 1024, print_info::Bool=false) where{T, TR1, TR2}
@assert iszero(digit % 256)
s, w = setprecision(digit) do
VP(f, nc, n, N, region)
end
if print_info
x = [- nc * log((1.0 + cos(region[1])) / 2.0):0.001: 10.0...]
error_VP = max_error(f, s, w, x = x)
@info "VP error: $error_VP"
end
smr, wmr, σ = setprecision(digit) do
MR(s, w, p)
end
if print_info
x = [- nc * log((1.0 + cos(region[1])) / 2.0):0.001: 10.0...]
error_MR = max_error(f, smr, wmr, x = x)
@info "MR error: $error_MR"
end
Ts, Tw, Tσ = T1.(smr), T1.(wmr), T2(σ)
if print_info
x = [- nc * log((1.0 + cos(region[1])) / 2.0):0.001: 10.0...]
error_TMR = max_error(f, Ts, Tw, x = x)
@info "Truncated MR error: $error_TMR"
end
return SoePara(Ts, Tw), Tσ
end
end
| SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | code | 3240 | function VP_kernel(f::Function, nc::T, j::Int) where{T}
VP_K = r -> f(- nc * log((T(1.0) + cos(T(r))) / T(2))) * cos(T(j) * T(r))
return VP_K
end
function VP_k(f::Function, nc::TN, n::Int, j::Int, gausspara::GaussParameter{T}, region::NTuple{2, T}) where{TN, T}
nc = T(nc)
K = VP_kernel(f, nc, j)
∫K = Gauss_int(K, gausspara; region = region)
kj = max(T(2)/T(π), (T(4) * n - T(2) * j) / (n * T(π))) * ∫K
return kj
end
function cos_array(j::Int, gausspara::GaussParameter{T}, region::NTuple{2, T}) where{T}
cos_array = Vector{T}()
a, b = T.(region)
for i in 1:size(gausspara.sw, 1)
push!(cos_array, cos(T(j) * T((b + a) / T(2) + (b - a) * gausspara.sw[i][1] / T(2))))
end
return cos_array
end
function VP_k_array(f::Function, nc::TN, n::Int, gausspara::GaussParameter{T}, region::NTuple{2, T}) where{TN, T}
Kj_array = Vector{T}()
nc = T(nc)
K = VP_kernel(f, nc, 0)
∫K = Gauss_int_vector(K, gausspara; region = T.(region))
for j in 1:2 * n - 1
cos_array_j = cos_array(j, gausspara, T.(region))
kj = max(T(2)/T(π), (T(4) * T(n) - T(2) * T(j)) / (n * T(π))) * dot(cos_array_j, ∫K)
push!(Kj_array, kj)
end
return Kj_array
end
function sign_bit(l::BigInt)
return l % 2 == 0 ? big(1) : big(-1)
end
function VP_cn(n::BigInt)
cn = Vector{Vector{BigFloat}}()
for j in 0 : 2*n-1
cnj = Vector{BigFloat}()
for l in 1 : n
cnjl = sign_bit(n + l - j) * (big(1.0) - l / n) * (n + l) / (n + l + j) * binomial(n + l + j, n + l - j)
push!(cnj, cnjl)
end
push!(cn, cnj)
end
return cn
end
function get_cn(cn::Vector{Vector{BigFloat}}, j::T, l::T) where{T<:Integer}
return cn[j + 1][l]
end
function VP(f::Function, nc::T, n::Int, N::Int, region::Tuple{TR1, TR2}) where{T, TR1, TR2}
gausspara = GaussParameter(BigFloat, N)
region = BigFloat.(region)
k0 = VP_k(f, nc, n, 0, gausspara, region)
k = big.( VP_k_array(f, nc, n, gausspara, region))
s_array = [big(j) / big(nc) for j in 1:2*n - 1]
w_array = Vector{BigFloat}()
n = BigInt(n)
cn = VP_cn(n)
# j = 0
w0 = 2 * k0 + sum([sign_bit(l) * n / (big(2) * n - l) * k[l] for l in 1:n]) + sum([sign_bit(n + l) * (n - l) / (2 * n - l) * k[n + l] for l in 1:n - 1])
# push!(w_array, w0)
#1 ≤ j ≤ n
for j in 1:n
wj = sum([sign_bit(l - j) * n * l / ((l + j) * (2 * n - l)) * binomial(l + j, l - j) * k[l] for l in j:n])
wj += sum([get_cn(cn, j, l) * n / (2 * n - l) * k[n + l] for l in 1:n-1])
wj *= (big(4.0))^j
push!(w_array, wj)
end
#n + 1 ≤ j ≤ 2n - 1
for j in n + 1:2 * n - 1
wj = sum([n * get_cn(cn, j, l) * k[n + l] / (2 * n - l) for l in j-n:n-1])
wj *= (big(4.0))^j
push!(w_array, wj)
end
# return [(s_array[i], w_array[i]) for i in 1:length(s_array)]
return s_array, w_array
end
function VP_cal(f::Function, nc::T, n::Int, N::Int; digit::Int = 1024, region::Tuple{TR1, TR2} = (0.0, π)) where{T, TR1, TR2}
@assert iszero(digit % 256)
s_array, w_array = setprecision(digit) do
VP(f, nc, n, N, region)
end
return s_array, w_array
end | SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | code | 1321 |
"""
function FGT1d(q_1, q_2, x, soepara, α)
Calculate the summation sum_{i,j} q_1[i] * q_2[j] * f(|x[i] - x[j]| / α) in O(N) steps, via SOE approximation.
"""
function FET1d(q_1::Vector{TQ}, q_2::Vector{TQ}, x::Vector{T}, soepara::SoePara{TC}; α::T = one(T), sort_x::Vector{Int64} = sortperm(x)) where{TQ, T, TC}
sum_result = zero(ComplexF64)
N = length(x)
@assert length(q_1) == length(q_2) == N
for i in 1:N
sum_result += q_1[i] * q_2[i]
end
for (s, w) in soepara.sw
A = ComplexF64(q_2[sort_x[N]])
sum_result += w * q_1[sort_x[N - 1]] * exp(s * (x[sort_x[N - 1]] - x[sort_x[N]]) / α) * A
for i in N-2:-1:1
l = sort_x[i]
m = sort_x[i + 1]
A = A * exp( - s * (x[sort_x[i + 2]] - x[sort_x[i + 1]]) / α) + q_2[sort_x[i + 1]]
sum_result += w * q_1[l] * exp(s * (x[l] - x[m]) / α) * A
end
B = ComplexF64(q_2[sort_x[1]])
sum_result += w * q_1[sort_x[2]] * exp(- s * (x[sort_x[1]] - x[sort_x[2]]) / α) * B
for i in 3:N
l = sort_x[i]
m = sort_x[i - 1]
B = B * exp(s * (x[sort_x[i - 2]] - x[sort_x[i - 1]]) / α) + q_2[sort_x[i - 1]]
sum_result += w * q_1[l] * exp(s * (x[m] - x[l]) / α) * B
end
end
return sum_result
end | SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | code | 1294 | struct SoePara{T}
sw::Vector{Tuple{T, T}}
end
function SoePara(s::Vector{T}, w::Vector{T}) where{T}
return SoePara{T}([tuple(s[i], w[i]) for i in 1:length(s)])
end
function soe(x::T1, sw::SoePara{T2}; T::DataType = Float64) where{T1<:Real, T2}
x = abs(x)
sum = zero(T2)
for (s, w) in sw.sw
sum += w * exp(- s * x)
end
return T(real(sum))
end
function soe(x::T, s::Vector{T1}, w::Vector{T2}) where{T, T1, T2}
return sum([w[i] * exp(-s[i] * x) for i in 1:length(s)])
end
function soe_error(f::Function, s::Vector{T2}, w::Vector{T2}; x::Vector{T1} = big.([0.0:0.01:10.0...])) where{T1<:Real, T2}
error = [abs(soe(x[i], s, w) - f(x[i])) for i in 1:size(x, 1)]
return error
end
function max_error(f::Function, s::Vector{T2}, w::Vector{T2}; x::Vector{T1} = big.([0.0:0.01:10.0...])) where{T1<:Real, T2}
error = soe_error(f, s, w, x = x)
return maximum(error)
end
function soe_error(f::Function, sw::SoePara{T2}; x::Vector{T1} = big.([0.0:0.01:10.0...])) where{T1<:Real, T2}
error = [abs(soe(x[i], sw) - f(x[i])) for i in 1:size(x, 1)]
return error
end
function max_error(f::Function, sw::SoePara{T2}; x::Vector{T1} = big.([0.0:0.01:10.0...])) where{T1<:Real, T2}
error = soe_error(f, sw, x = x)
return maximum(error)
end | SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | code | 294 | @testset "VP kernel accuracy, exp(-x^2)" begin
f = x -> exp(-x^2)
s, w = VP_cal(f, 4.0, 30, 100)
@test max_error(f, s, w) < 1e-8
end
@testset "VP kernel accuracy, erfc(x)" begin
f = x -> erfc(x)
s, w = VP_cal(f, 4.0, 30, 100)
@test max_error(f, s, w) < 1e-8
end | SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | code | 586 | @testset "VPMR, Gaussian" begin
f = x -> exp(-x^2)
sw, σ = VPMR_cal(f, 4.0, 40, 100, 8)
@test max_error(f, sw) < 1e-7
sw, σ = VPMR_cal(f, 4.0, 40, 100, 12)
@test max_error(f, sw) < 1e-10
sw, σ = VPMR_cal(f, 6.0, 60, 200, 16)
@test max_error(f, sw) < 1e-12
end
@testset "VPMR, error function" begin
f = x -> erfc(x)
sw, σ = VPMR_cal(f, 4.0, 40, 100, 8)
@test max_error(f, sw) < 1e-7
sw, σ = VPMR_cal(f, 4.0, 40, 100, 12)
@test max_error(f, sw) < 1e-10
sw, σ = VPMR_cal(f, 6.0, 60, 200, 16)
@test max_error(f, sw) < 1e-12
end | SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | code | 577 | @testset "test fast exponentials transform 1d" begin
f = x -> exp(-x^2)
soepara = VPMR_cal(f, 6.0, 60, 200, 16)[1]
for T in [Float64, ComplexF64]
q_1 = rand(T, 100)
q_2 = rand(T, 100)
x = rand(100)
α = rand()
sum_exact = zero(ComplexF64)
for i in 1:length(x)
for j in 1:length(x)
sum_exact += q_1[i] * q_2[j] * f(abs(x[i] - x[j])/ α)
end
end
sum_soe = FET1d(q_1, q_2, x, soepara, α = α)
@test isapprox((sum_exact), (sum_soe), rtol = 1e-8)
end
end | SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | code | 172 | using SumOfExpVPMR
using Test
using SpecialFunctions
@testset "SumOfExpVPRM.jl" begin
include("VP.jl")
include("VPMR.jl")
include("fast_exp_transform.jl")
end
| SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 0.1.1 | 124d55aa9a072c5681a4c90ff733142abf378376 | docs | 3035 | # SumOfExpVPMR.jl
[](https://github.com/HPMolSim/SumOfExpVPMR.jl/actions/workflows/CI.yml?query=branch%3Amain)
[](https://travis-ci.com/HPMolSim/SumOfExpVPMR.jl)
[](https://codecov.io/gh/HPMolSim/SumOfExpVPMR.jl)
`SumOfExpVPMR.jl` is a `Julia` implentation the VPMR method by Zixuan Gao, Jiuyang Liang and Zhenli Xu in [A Kernel-Independent Sum-of-Exponentials Method](https://link.springer.com/10.1007/s10915-022-01999-1), which can be used to represent rapid decaying kernels via sum of exponentials.
## Getting Started
Add this package in julia by typing `]` in Julia REPL and then
```julia
pkg> add SumOfExpVPMR
```
to install the package.
The main function is
```julia
function VPMR_cal(
f::Function, # function to be approximated, be like f(x::T) where{T<:Real}, and make sure it can produce highly accurate result for BigFloat
nc::T, # width of soe
n::Int, # terms of soe in VP
N::Int, # order of Gaussian integral
p::Int; # terms of MR
T1::DataType = ComplexF64, # output type for s and w
T2::DataType = Float64, # output type for \sigma
digit::Int = 1024,
print_info::Bool=false
) where{T}
```
for details please refer to the article.
Here is an example of using VPMR, where we find a 12 term approximation for Gaussian function:
```julia
julia> using SumOfExpVPMR
julia> f = x -> exp(-x^2)
#58 (generic function with 1 method)
julia> sw12, σ12 = VPMR_cal(f, 4.0, 40, 100, 12, print_info = true);
[ Info: VP error: 1.2159077902284768438357759665040000333168536446025393484114289668230574315365e-12
[ Info: MR error: 1.331145872202156879583878373566408054787581766492969993446545399873793531071339e-11
[ Info: Truncated MR error: 1.3310907931440852e-11
```
More benchmark is shown in the following figure, the code can be found in the `expamle` folder.

## Usage
The SOE approach is quite useful since the $\exp(\cdot)$ function is easy to be handled.
One of the most important usage is called the fast exponential transform (FET), which can be used to calculate the summation $\sum_{i,j} q_{1}^{i} q_{2}^{j} f \left( α^{-1} |x_i - x_j| \right)$ in $O(N)$ steps.
For details, please see the implentation in `src/fast_exp_transform.jl`.
Here we compare the cost of calculating $\sum_{i,j} q_{1}^{i} q_{2}^{j} \exp \left( -(x_i - x_j)^2 \right)$ directly and via FET, as shown in fig below.

## How to Contribute
If you find any bug or have any suggestion, please open an issue.
## References
1. The VPMR article [A Kernel-Independent Sum-of-Exponentials Method](https://link.springer.com/10.1007/s10915-022-01999-1).
2. [Matlab implementation of VPMR](https://github.com/ZXGao97/VPMR)
3. [C++ implementation of VPMR](https://github.com/TLCFEM/vpmr)
| SumOfExpVPMR | https://github.com/HPMolSim/SumOfExpVPMR.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 8185 | """
Format.jl
This package provides various functions to provide formatted output,
either in a fashion similar to C printf or Python format strings.
## Python-style Types and Functions
#### Types to Represent Formats
This package has two types ``FormatSpec`` and ``FormatExpr`` to represent a format specification.
In particular, ``FormatSpec`` is used to capture the specification of a single entry. One can compile a format specification string into a ``FormatSpec`` instance as
```julia
fspec = FormatSpec("d")
fspec = FormatSpec("<8.4f")
```
Please refer to [Python's format specification language](http://docs.python.org/2/library/string.html#formatspec) for details.
``FormatExpr`` captures a formatting expression that may involve multiple items. One can compile a formatting string into a ``FormatExpr`` instance as
```julia
fe = FormatExpr("{1} + {2}")
fe = FormatExpr("{1:d} + {2:08.4e} + {3|>abs2}")
```
Please refer to [Python's format string syntax](http://docs.python.org/2/library/string.html#format-string-syntax) for details.
**Note:** If the same format is going to be applied for multiple times. It is more efficient to first compile it.
#### Formatted Printing
One can use ``printfmt`` and ``printfmtln`` for formatted printing:
- **printfmt**(io, fe, args...)
- **printfmt**(fe, args...)
Print given arguments using given format ``fe``. Here ``fe`` can be a formatting string, an instance of ``FormatSpec`` or ``FormatExpr``.
**Examples**
```julia
printfmt("{1:>4s} + {2:.2f}", "abc", 12) # --> print(" abc + 12.00")
printfmt("{} = {:#04x}", "abc", 12) # --> print("abc = 0x0c")
fs = FormatSpec("#04x")
printfmt(fs, 12) # --> print("0x0c")
fe = FormatExpr("{} = {:#04x}")
printfmt(fe, "abc", 12) # --> print("abc = 0x0c")
```
**Notes**
If the first argument is a string, it will be first compiled into a ``FormatExpr``, which implies that you can not use specification-only string in the first argument.
```julia
printfmt("{1:d}", 10) # OK, "{1:d}" can be compiled into a FormatExpr instance
printfmt("d", 10) # Error, "d" can not be compiled into a FormatExpr instance
# such a string to specify a format specification for single argument
printfmt(FormatSpec("d"), 10) # OK
printfmt(FormatExpr("{1:d}", 10)) # OK
```
- **printfmtln**(io, fe, args...)
- **printfmtln**(fe, args...)
Similar to ``printfmt`` except that this function print a newline at the end.
#### Formatted String
One can use ``pyfmt`` to format a single value into a string, or ``format`` to format one to multiple arguments into a string using an format expression.
- **pyfmt**(fspec, a)
Format a single value using a format specification given by ``fspec``, where ``fspec`` can be either a string or an instance of ``FormatSpec``.
- **format**(fe, args...)
Format arguments using a format expression given by ``fe``, where ``fe`` can be either a string or an instance of ``FormatSpec``.
#### Difference from Python's Format
At this point, this package implements a subset of Python's formatting language (with slight modification). Here is a summary of the differences:
- In terms of argument specification, it supports natural ordering (e.g. ``{} + {}``), explicit position (e.g. ``{1} + {2}``). It hasn't supported named arguments or fields extraction yet. Note that mixing these two modes is not allowed (e.g. ``{1} + {}``).
- The package provides support for filtering (for explicitly positioned arguments), such as ``{1|>lowercase}`` by allowing one to embed the ``|>`` operator, which the Python counter part does not support.
## C-style functions
The c-style part of this package aims to get around the limitation that
`@sprintf` has to take a literal string argument.
The core part is basically a c-style print formatter using the standard
`@sprintf` macro.
It also adds functionalities such as commas separator (thousands), parenthesis for negatives,
stripping trailing zeros, and mixed fractions.
### Usage and Implementation
The idea here is that the package compiles a function only once for each unique
format string within the `Format.*` name space, so repeated use is faster.
Unrelated parts of a session using the same format string would reuse the same
function, avoiding redundant compilation. To avoid the proliferation of
functions, we limit the usage to only 1 argument. Practical consideration
would suggest that only dozens of functions would be created in a session, which
seems manageable.
Usage
```julia
using Format
fmt = "%10.3f"
s = cfmt( fmt, 3.14159 ) # usage 1. Quite performant. Easiest to switch to.
fmtrfunc = generate_formatter( fmt ) # usage 2. This bypasses repeated lookup of cached function. Most performant.
s = fmtrfunc( 3.14159 )
s = format( 3.14159, precision=3 ) # usage 3. Most flexible, with some non-printf options. Least performant.
```
### Commas
This package also supplements the lack of thousand separator e.g. `"%'d"`, `"%'f"`, `"%'g"`.
Note: `"%'g"` behavior is that for small enough floating point (but not too small),
thousand separator would be used. If the number needs to be represented by `"%e"`, no
separator is used.
### Flexible `format` function
This package contains a run-time number formatter `format` function, which goes beyond
the standard `sprintf` functionality.
An example:
```julia
s = format( 1234, commas=true ) # 1,234
s = format( -1234, commas=true, parens=true ) # (1,234)
```
The keyword arguments are (Bold keywards are not printf standard)
* width. Integer. Try to fit the output into this many characters. May not be successful.
Sacrifice space first, then commas.
* precision. Integer. How many decimal places.
* leftjustified. Boolean
* zeropadding. Boolean
* commas. Boolean. Thousands-group separator.
* signed. Boolean. Always show +/- sign?
* positivespace. Boolean. Prepend an extra space for positive numbers? (so they align nicely with negative numbers)
* **parens**. Boolean. Use parenthesis instead of "-". e.g. `(1.01)` instead of `-1.01`. Useful in finance. Note that
you cannot use `signed` and `parens` option at the same time.
* **stripzeros**. Boolean. Strip trailing '0' to the right of the decimal (and to the left of 'e', if any ).
* It may strip the decimal point itself if all trailing places are zeros.
* This is true by default if precision is not given, and vice versa.
* alternative. Boolean. See `#` alternative form explanation in standard printf documentation
* conversion. length=1 string. Default is type dependent. It can be one of `aAeEfFoxX`. See standard
printf documentation.
* **mixedfraction**. Boolean. If the number is rational, format it in mixed fraction e.g. `1_1/2` instead of `3/2`
* **mixedfractionsep**. Default `_`
* **fractionsep**. Default `/`
* **fractionwidth**. Integer. Try to pad zeros to the numerator until the fractional part has this width
* **tryden**. Integer. Try to use this denominator instead of a smaller one. No-op if it'd lose precision.
* **suffix**. String. This strings will be appended to the output. Useful for units/%
* **autoscale**. Symbol, default `:none`. It could be `:metric`, `:binary`, or `:finance`.
* `:metric` implements common SI symbols for large and small numbers e.g. `M`, `k`, `μ`, `n`
* `:binary` implements common ISQ symbols for large numbers e.g. `Ti`, `Gi`, `Mi`, `Ki`
* `:finance` implements common finance/news symbols for large numbers e.g. `b` (billion), `m` (millions)
"""
module Format
import Base.show
_stdout() = stdout
_codeunits(s) = Vector{UInt8}(codeunits(s))
m_eval(expr) = Core.eval(@__MODULE__, expr)
export FormatSpec, FormatExpr, printfmt, printfmtln, format, generate_formatter
export pyfmt, cfmt, fmt
export fmt_default, fmt_default!, reset!, default_spec, default_spec!
# Deal with mess from #16058
# Later, use Strs package!
isdefined(Main, :ASCIIStr) || (const ASCIIStr = String)
isdefined(Main, :UTF8Str) || (const UTF8Str = String)
include("cformat.jl" )
include("fmtspec.jl")
include("fmtcore.jl")
include("formatexpr.jl")
include("fmt.jl")
end # module Format
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 12424 | include("printf.jl")
const _formatters = Dict{ASCIIStr,FmtSpec}()
function _get_formatter(fmt)
global _formatters
chkfmt = get(_formatters, fmt, nothing)
chkfmt === nothing || return chkfmt
_formatters[fmt] = FmtSpec(fmt)
end
_cfmt_comma(fspec::FmtSpec, x) = addcommasreal(_cfmt(fspec, x), Char(fspec.tsep))
_cfmt_comma(fspec::FmtSpec{FmtStr}, x::Rational) = addcommasrat(_cfmt(fspec, x), Char(fspec.tsep))
_cfmt_comma(fspec::FmtSpec{<:FmtInts}, x) = checkcommas(_cfmt(fspec, x), Char(fspec.tsep))
function _cfmt(fspec::FmtSpec, x)
sv = Base.StringVector(23) # Trust that lower level code will expand if necessary
pos = _fmt(sv, 1, fspec, x)
resize!(sv, pos - 1)
String(sv)
end
cfmt(fspec::FmtSpec, x) = fspec.tsep == 0 ? _cfmt(fspec, x) : _cfmt_comma(fspec, x)
cfmt(fmtstr::ASCIIStr, x) = cfmt(_get_formatter(fmtstr), x)
function generate_formatter(fmt::ASCIIStr)
fspec = _get_formatter(fmt)
fspec.tsep == 0 ? x -> _cfmt(fspec, x) : x -> _cfmt_comma(fspec, x)
end
function addcommasreal(s, sep)
len = length(s)
dpos = findfirst( isequal('.'), s )
dpos !== nothing && return addcommas(s, len, dpos-1, sep)
# find the rightmost digit
for i in len:-1:1
isdigit( s[i] ) && return addcommas(s, len, i, sep)
end
s
end
# commas are added to only the numerator
addcommasrat(s, sep) = addcommas(s, length(s), findfirst( isequal('/'), s )-1, sep)
function checkcommas(s, sep)
len = length(s)
for i in len:-1:1
isdigit( s[i] ) && return addcommas(s, len, i, sep)
end
s
end
function addcommas(s::T, len, lst, sep) where {T<:AbstractString}
lst < 4 && return s
beg = 1
while beg < len
isdigit(s[beg]) && break
beg += 1
end
dig = lst - beg + 1
dig < 4 && return s
commas = div(dig - 1, 3)
sv = Base.StringVector(len + commas)
for i = 1:beg-1; sv[i] = s[i]; end
cnt = dig - commas*3
pos = beg - 1
for i = beg:lst-3
sv[pos += 1] = s[i]
(cnt -= 1) == 0 && (cnt = 3; sv[pos += 1] = sep)
end
for i = lst-2:len; sv[i+commas] = s[i]; end
T(sv)
end
addcommas(s, sep) = (l = length(s); addcommas(s, l, l, sep))
function generate_format_string(;
width::Int=-1,
precision::Int= -1,
leftjustified::Bool=false,
zeropadding::Bool=false,
commas::Bool=false,
signed::Bool=false,
positivespace::Bool=false,
alternative::Bool=false,
conversion::ASCIIStr="f" #aAdecEfFiosxX
)
s = ['%'%UInt8]
commas &&
push!(s, '\'')
alternative && in( conversion[1], "aAeEfFoxX" ) &&
push!(s, '#')
zeropadding && !leftjustified && width != -1 &&
push!(s, '0')
if signed
push!(s, '+')
elseif positivespace
push!(s, ' ')
end
if width != -1
leftjustified && push!(s, '-')
append!(s, _codeunits(string( width )))
end
precision != -1 &&
append!(s, _codeunits(string( '.', precision )))
String(append!(s, _codeunits(conversion)))
end
"""
Format a value, using the following keyword arguments to control formatting
(Bold keywords are not printf standard):
* width. Integer. Try to fit the output into this many characters. May not be successful.
Sacrifice space first, then commas.
* precision. Integer. How many decimal places.
* leftjustified. Boolean
* zeropadding. Boolean
* commas. Boolean. Thousands-group separator.
* signed. Boolean. Always show +/- sign?
* positivespace. Boolean. Prepend an extra space for positive numbers? (so they align nicely with negative numbers)
* **parens**. Boolean. Use parenthesis instead of "-". e.g. `(1.01)` instead of `-1.01`. Useful in finance. Note that
you cannot use `signed` and `parens` option at the same time.
* **stripzeros**. Boolean. Strip trailing '0' to the right of the decimal (and to the left of 'e', if any ).
* It may strip the decimal point itself if all trailing places are zeros.
* This is true by default if precision is not given, and vice versa.
* alternative. Boolean. See `#` alternative form explanation in standard printf documentation
* conversion. length=1 string. Default is type dependent. It can be one of `aAeEfFoxX`. See standard
printf documentation.
* **mixedfraction**. Boolean. If the number is rational, format it in mixed fraction e.g. `1_1/2` instead of `3/2`
* **mixedfractionsep**. Default `_`
* **fractionsep**. Default `/`
* **fractionwidth**. Integer. Try to pad zeros to the numerator until the fractional part has this width
* **tryden**. Integer. Try to use this denominator instead of a smaller one. No-op if it'd lose precision.
* **suffix**. String. This strings will be appended to the output. Useful for units/%
* **autoscale**. Symbol, default `:none`. It could be `:metric`, `:binary`, or `:finance`.
* `:metric` implements common SI symbols for large and small numbers e.g. `M`, `k`, `μ`, `n`
* `:binary` implements common ISQ symbols for large numbers e.g. `Ti`, `Gi`, `Mi`, `Ki`
* `:finance` implements common finance/news symbols for large numbers e.g. `b` (billion), `m` (millions)
"""
function format( x::T;
width::Int=-1,
precision::Int= -1,
leftjustified::Bool=false,
zeropadding::Bool=false, # when right-justified, use 0 instead of space to fill
commas::Bool=false,
signed::Bool=false, # +/- prefix
positivespace::Bool=false,
stripzeros::Bool=(precision== -1),
parens::Bool=false, # use (1.00) instead of -1.00. Used in finance
alternative::Bool=false, # usually for hex
mixedfraction::Bool=false,
mixedfractionsep::UTF8Str="_",
fractionsep::UTF8Str="/", # num / den
fractionwidth::Int = 0,
tryden::Int = 0, # if 2 or higher,
# try to use this denominator, without losing precision
suffix::UTF8Str="", # useful for units/%
autoscale::Symbol=:none, # :metric, :binary or :finance
conversion::ASCIIStr=""
) where {T<:Real}
checkwidth = commas
if conversion == ""
if T <: AbstractFloat || T <: Rational && precision != -1
actualconv = "f"
elseif T <: Unsigned
actualconv = "x"
elseif T <: Integer
actualconv = "d"
else
conversion = "s"
actualconv = "s"
end
else
actualconv = conversion
end
signed && commas && error( "You cannot use signed (+/-) AND commas at the same time")
T <: Rational && conversion == "s" && (stripzeros = false)
if ( T <: AbstractFloat && actualconv == "f" || T <: Integer ) && autoscale != :none
actualconv = "f"
if autoscale == :metric
scales = [
(1e24, "Y" ),
(1e21, "Z" ),
(1e18, "E" ),
(1e15, "P" ),
(1e12, "T" ),
(1e9, "G"),
(1e6, "M"),
(1e3, "k") ]
if abs(x) > 1
for (mag, sym) in scales
if abs(x) >= mag
x /= mag
suffix = string(sym, suffix)
break
end
end
elseif T <: AbstractFloat
smallscales = [
( 1e-12, "p" ),
( 1e-9, "n" ),
( 1e-6, "μ" ),
( 1e-3, "m" ) ]
for (mag,sym) in smallscales
if abs(x) < mag*10
x /= mag
suffix = string(sym, suffix)
break
end
end
end
else
if autoscale == :binary
scales = [
(1024.0 ^8, "Yi" ),
(1024.0 ^7, "Zi" ),
(1024.0 ^6, "Ei" ),
(1024.0 ^5, "Pi" ),
(1024.0 ^4, "Ti" ),
(1024.0 ^3, "Gi"),
(1024.0 ^2, "Mi"),
(1024.0, "Ki")
]
else # :finance
scales = [
(1e12, "t" ),
(1e9, "b"),
(1e6, "m"),
(1e3, "k") ]
end
for (mag, sym) in scales
if abs(x) >= mag
x /= mag
suffix = string(sym, suffix)
break
end
end
end
end
nonneg = x >= 0
fractional = 0
if T <: Rational && mixedfraction
actualconv = "d"
actualx = trunc( Int, x )
fractional = abs(x) - abs(actualx)
else
actualx = (parens && !in( actualconv[1], "xX" )) ? abs(x) : x
end
s = cfmt( generate_format_string( width=width,
precision=precision,
leftjustified=leftjustified,
zeropadding=zeropadding,
commas=commas,
signed=signed,
positivespace=positivespace,
alternative=alternative,
conversion=actualconv
),
actualx)
if T <:Rational && conversion == "s"
if mixedfraction && fractional != 0
num = fractional.num
den = fractional.den
if tryden >= 2 && mod( tryden, den ) == 0
num *= div(tryden,den)
den = tryden
end
fs = string( num, fractionsep, den)
length(fs) < fractionwidth &&
(fs = string(repeat( "0", fractionwidth - length(fs) ), fs))
s = (actualx != 0
? string(rstrip(s), mixedfractionsep, fs)
: (nonneg ? fs : string('-', fs)))
checkwidth = true
elseif !mixedfraction
s = replace( s, "//" => fractionsep )
checkwidth = true
end
elseif stripzeros && in( actualconv[1], "fFeEs" )
dpos = findfirst( isequal('.'), s )
dpos === nothing && (dpos = length(s))
if actualconv[1] in "eEs"
epos = findfirst(isequal(actualconv[1] == 'E' ? 'E' : 'e'), s)
rpos = (epos === nothing) ? length( s ) : (epos-1)
else
rpos = length(s)
end
# rpos at this point is the rightmost possible char to start
# stripping
stripfrom = rpos+1
for i = rpos:-1:dpos+1
if s[i] == '0'
stripfrom = i
elseif s[i] ==' '
continue
else
break
end
end
if stripfrom <= rpos
# everything after decimal is 0, so strip the decimal too
s = string(s[1:stripfrom-1-(stripfrom == dpos+1)], s[rpos+1:end])
checkwidth = true
end
end
s = string(s, suffix)
if parens && !in( actualconv[1], "xX" )
# if zero or positive, we still need 1 white space on the right
s = nonneg ? string(' ', strip(s), ' ') : string('(', strip(s), ')')
checkwidth = true
end
if checkwidth && width != -1
if (len = length(s) - width) > 0
s = replace( s, " " => ""; count=len )
if (len = length(s) - width) > 0
endswith(s, " ") && (s = reverse(replace(reverse(s), " " => ""; count=len)))
(len = length(s) - width) > 0 && (s = replace( s, "," => ""; count=len ))
end
elseif len < 0
# Todo: should use lpad or rpad here, can be more efficient
s = leftjustified ? string(s, repeat( " ", -len )) : string(repeat( " ", -len), s)
end
end
s
end
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 8012 | # interface proposal by Tom Breloff (@tbreloff)... comments welcome
# This uses the more basic formatting based on FormatSpec and the pyfmt method
# (formerly called fmt, which I repurposed)
# TODO: swap out FormatSpec for something that is able to use the "format" method,
# which has more options for units, prefixes, etc
# TODO: support rational numbers, autoscale, etc as in "format"
# --------------------------------------------------------------------------------------------------
# the DefaultSpec object is just something to hold onto the current FormatSpec.
# we keep the typechar around specifically for the reset! function,
# to go back to the starting state
mutable struct DefaultSpec
typechar::Char
kwargs::Any
fspec::FormatSpec
end
DefaultSpec(c::AbstractChar) = DefaultSpec(Char(c), (), FormatSpec(c))
function DefaultSpec(c::AbstractChar, syms...; kwargs...)
# otherwise update the spec
if isempty(syms)
DefaultSpec(Char(c), kwargs, FormatSpec(c; kwargs...))
else
kw = _add_kwargs_from_symbols(kwargs, syms...)
DefaultSpec(Char(c), tuple(kw...), FormatSpec(c; kw...))
end
end
const DEFAULT_FORMATTERS = Dict{DataType, DefaultSpec}()
# adds a new default formatter for this type
default_spec!(::Type{T}, c::AbstractChar) where {T} =
(DEFAULT_FORMATTERS[T] = DefaultSpec(c); nothing)
# note: types T and K will now both share K's default
default_spec!(::Type{T}, ::Type{K}) where {T,K} =
(DEFAULT_FORMATTERS[T] = DEFAULT_FORMATTERS[K]; nothing)
default_spec!(::Type{T}, c::AbstractChar, syms...; kwargs...) where {T} =
(DEFAULT_FORMATTERS[T] = DefaultSpec(c, syms...; kwargs...); nothing)
function reset!(::Type{T}) where {T}
dspec = default_spec(T)
dspec.fspec = FormatSpec(dspec.typechar; dspec.kwargs...)
nothing
end
# --------------------------------------------------------------------------------------------------
function _add_kwargs_from_symbols(kwargs, syms::Symbol...)
d = Dict{Symbol, Any}(kwargs)
for s in syms
if s == :ljust || s == :left
d[:align] = '<'
elseif s == :rjust || s == :right
d[:align] = '>'
elseif s == :center
d[:align] = '^'
elseif s == :commas
d[:tsep] = true
elseif s == :zpad || s == :zeropad
d[:zpad] = true
elseif s == :ipre || s == :prefix
d[:ipre] = true
end
end
d
end
# --------------------------------------------------------------------------------------------------
# methods to get the current default objects
# note: if you want to set a default for an abstract type (i.e. AbstractFloat)
# you'll need to extend this method like here:
default_spec(::Type{<:Integer}) = DEFAULT_FORMATTERS[Integer]
default_spec(::Type{<:AbstractFloat}) = DEFAULT_FORMATTERS[AbstractFloat]
default_spec(::Type{<:AbstractString}) = DEFAULT_FORMATTERS[AbstractString]
default_spec(::Type{<:AbstractChar}) = DEFAULT_FORMATTERS[AbstractChar]
default_spec(::Type{<:AbstractIrrational}) = DEFAULT_FORMATTERS[AbstractIrrational]
default_spec(::Type{<:Number}) = DEFAULT_FORMATTERS[Number]
default_spec(::Type{T}) where {T} =
get(DEFAULT_FORMATTERS, T) do
error("Missing default spec for type $T... call default!(T, c): $DEFAULT_FORMATTERS")
end
default_spec(x) = default_spec(typeof(x))
fmt_default(::Type{T}) where {T} = default_spec(T).fspec
fmt_default(x) = default_spec(x).fspec
# first resets the fmt_default spec to the given arg,
# then continue by updating with args and kwargs
fmt_default!(::Type{T}, c::AbstractChar, args...; kwargs...) where {T} =
(default_spec!(T,c); fmt_default!(T, args...; kwargs...))
fmt_default!(::Type{T}, ::Type{K}, args...; kwargs...) where {T,K} =
(default_spec!(T,K); fmt_default!(T, args...; kwargs...))
# update the fmt_default for a specific type
function fmt_default!(::Type{T}, syms::Symbol...; kwargs...) where {T}
if isempty(syms)
# if there are no arguments, reset to initial defaults
if isempty(kwargs)
reset!(T)
return
end
# otherwise update the spec
dspec = default_spec(T)
dspec.fspec = FormatSpec(dspec.fspec; kwargs...)
else
d = _add_kwargs_from_symbols(kwargs, syms...)
fmt_default!(T; d...)
end
nothing
end
# update the fmt_default for all types
function fmt_default!(syms::Symbol...; kwargs...)
if isempty(syms)
for k in keys(DEFAULT_FORMATTERS)
fmt_default!(k; kwargs...)
end
else
d = _add_kwargs_from_symbols(kwargs, syms...)
fmt_default!(; d...)
end
nothing
end
# --------------------------------------------------------------------------------------------------
# TODO: get rid of this entire hack by moving commas into pyfmt
function _optional_commas(x::Real, s::AbstractString, fspec::FormatSpec)
prevwidth = length(s)
dpos = findfirst( isequal('.'), s)
ns = addcommas(s, prevwidth, dpos === nothing ? prevwidth : dpos - 1, fspec.sep)
# check for excess width from commas
w = length(s)
(fspec.width > 0 && w > fspec.width && w > prevwidth) ? s : ns
end
_optional_commas(x, s::AbstractString, fspec::FormatSpec) = s
# --------------------------------------------------------------------------------------------------
"""
Creates a new FormatSpec by overriding the defaults and passes it to pyfmt
Optionally width and precision can be passed positionally, after the value to be formatted.
Some keyword arguments can be passed simply as symbols:
```
Symbol | Meaning
------------------|------------------------------------------
:ljust or :left | Left justified, same as < for FormatSpec
:rjust or :right | Right justified, same as > for FormatSpec
:center | Center justified, same as ^ for FormatSpec
:zpad or :zeropad | Pad with 0s on left
:ipre or :prefix | Whether to prefix 0b, 0o, or 0x
:commas | Add commas every 3 digits
```
Also, keyword arguments can be given:
```
Keyword | Type | Meaning | Default
--------|------|---------------------------|-------
fill | Char | Fill character | ' '
align | Char | Alignment character | '\\0'
sign | Char | Sign character | '-'
width | Int | Field width | -1, i.e. ignored
prec | Int | Floating Precision | -1, i.e. ignored
ipre | Bool | Use 0b, 0o, or 0x prefix? | false
zpad | Bool | Pad with 0s on left | false
tsep | Bool | Use thousands separator? | false
```
"""
function fmt end
# TODO: do more caching to optimize repeated calls
# creates a new FormatSpec by overriding the defaults and passes it to pyfmt
# note: adding kwargs is only appropriate for one-off formatting.
# normally it will be much faster to change the fmt_default formatting as needed
function fmt(x; kwargs...)
fspec = fmt_default(x)
isempty(kwargs) || (fspec = FormatSpec(fspec; kwargs...))
pyfmt(fspec, x)
end
# some helper method calls, which just convert to kwargs
fmt(x, width::Int, args...; kwargs...) = fmt(x, args...; width=width, kwargs...)
fmt(x, width::Int, prec::Int, args...; kwargs...) =
fmt(x, args...; width=width, prec=prec, kwargs...)
# integrate some symbol shorthands into the keyword args
# note: as above, this will generate relevant kwargs, so to format in a tight loop,
# you should probably update the fmt_default
function fmt(x, syms::Symbol...; kwargs...)
d = _add_kwargs_from_symbols(kwargs, syms...)
fmt(x; d...)
end
# --------------------------------------------------------------------------------------------------
# seed it with some basic default formatters
for (t, c) in [(Integer,'d'),
(AbstractFloat,'f'),
(AbstractChar,'c'),
(AbstractString,'s')]
default_spec!(t, c)
end
default_spec!(Number, 's', :right)
default_spec!(AbstractIrrational, 's', :right)
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 8769 | # core formatting functions
### auxiliary functions
### print char n times
function _repprint(out::IO, c::AbstractChar, n::Int)
wid = textwidth(c)
n < wid && return
while n > 0
print(out, c)
n -= wid
end
end
### print string or char
function _truncstr(s::AbstractString, slen, prec)
prec == 0 && return ("", 0)
i, n = 0, 1
siz = ncodeunits(s)
while n <= siz
(prec -= textwidth(s[n])) < 0 && break
i = n
n = nextind(s, i)
end
str = SubString(s, 1, i)
return (str, textwidth(str))
end
_truncstr(s::AbstractChar, slen, prec) = ("", 0)
function _pfmt_s(out::IO, fs::FormatSpec, s::Union{AbstractString,AbstractChar})
slen = textwidth(s)
str, slen = 0 <= fs.prec < slen ? _truncstr(s, slen, fs.prec) : (s, slen)
prepad = postpad = 0
pad = fs.width - slen
if pad > 0
if fs.align == '<'
postpad = pad
elseif fs.align == '^'
prepad, postpad = pad>>1, (pad+1)>>1
else
prepad = pad
end
end
# left padding
prepad == 0 || _repprint(out, fs.fill, prepad)
# print string
print(out, str)
# right padding
postpad == 0 || _repprint(out, fs.fill, postpad)
end
_unsigned_abs(x::Signed) = unsigned(abs(x))
_unsigned_abs(x::Bool) = UInt(x)
_unsigned_abs(x::Unsigned) = x
# Special case because unsigned fails for BigInt
_unsigned_abs(x::BigInt) = abs(x)
_ndigits(x, ::_Dec) = ndigits(x)
_ndigits(x, ::_Bin) = ndigits(x, base=2)
_ndigits(x, ::_Oct) = ndigits(x, base=8)
_ndigits(x, ::Union{_Hex, _HEX}) = ndigits(x, base=16)
_sepcnt(::_Dec) = 3
_sepcnt(::Any) = 4
_mul(x::Integer, ::_Dec) = x * 10
_mul(x::Integer, ::_Bin) = x << 1
_mul(x::Integer, ::_Oct) = x << 3
_mul(x::Integer, ::Union{_Hex, _HEX}) = x << 4
_div(x::Integer, ::_Dec) = div(x, 10)
_div(x::Integer, ::_Bin) = x >> 1
_div(x::Integer, ::_Oct) = x >> 3
_div(x::Integer, ::Union{_Hex, _HEX}) = x >> 4
_ipre(op) = ""
_ipre(::_Oct) = "0o"
_ipre(::_Bin) = "0b"
_ipre(::_Hex) = "0x"
_ipre(::_HEX) = "0X"
_digitchar(x::Integer, ::_Bin) = x == 0 ? '0' : '1'
_digitchar(x::Integer, ::_Dec) = Char(Int('0') + x)
_digitchar(x::Integer, ::_Oct) = Char(Int('0') + x)
_digitchar(x::Integer, ::_Hex) = Char(x < 10 ? '0' + x : 'a' + (x - 10))
_digitchar(x::Integer, ::_HEX) = Char(x < 10 ? '0' + x : 'A' + (x - 10))
_signchar(x::Real, s::AbstractChar) = signbit(x) ? '-' : s == '+' ? '+' : s == ' ' ? ' ' : '\0'
### output integers (with or without separators)
function _outint(out::IO, ax::T, op::Op=_Dec()) where {Op, T<:Integer}
b_lb = _div(ax, op)
b = one(T)
while b <= b_lb
b = _mul(b, op)
end
r = ax
while b > 0
(q, r) = divrem(r, b)
print(out, _digitchar(q, op))
b = _div(b, op)
end
end
function _outint(out::IO, ax::T, op::Op, numini, sep) where {Op, T<:Integer}
b_lb = _div(ax, op)
b = one(T)
while b <= b_lb
b = _mul(b, op)
end
r = ax
while true
(q, r) = divrem(r, b)
print(out, _digitchar(q, op))
b = _div(b, op)
b == 0 && break
if numini == 0
numini = _sepcnt(op)
print(out, sep)
end
numini -= 1
end
end
# Print integer
function _pfmt_i(out::IO, fs::FormatSpec, x::Integer, op::Op) where {Op}
# calculate actual length
ax = _unsigned_abs(x)
xlen = _ndigits(ax, op)
numsep, numini = fs.tsep ? divrem(xlen-1, _sepcnt(op)) : (0, 0)
xlen += numsep
# sign char
sch = _signchar(x, fs.sign)
xlen += (sch != '\0')
# prefix (e.g. 0x, 0b, 0o)
ip = ""
if fs.ipre
ip = _ipre(op)
xlen += length(ip)
end
# printing
pad = fs.width - xlen
prepad = postpad = zpad = 0
if pad > 0
if fs.zpad
zpad = pad
elseif fs.align == '<'
postpad = pad
elseif fs.align == '^'
prepad, postpad = pad>>1, (pad+1)>>1
else
prepad = pad
end
end
# left padding
prepad == 0 || _repprint(out, fs.fill, prepad)
# print sign
sch != '\0' && print(out, sch)
# print prefix
!isempty(ip) && print(out, ip)
# print padding zeros
zpad > 0 && _repprint(out, '0', zpad)
# print actual digits
ax == 0 ? print(out, '0') :
numsep == 0 ? _outint(out, ax, op) : _outint(out, ax, op, numini, fs.sep)
# right padding
postpad == 0 || _repprint(out, fs.fill, postpad)
end
function _truncval(v)
try
return trunc(Integer, v)
catch e
e isa InexactError || rethrow(e)
end
try
return trunc(Int128, v)
catch e
e isa InexactError || rethrow(e)
end
trunc(BigInt, v)
end
### print floating point numbers
function _pfmt_f(out::IO, fs::FormatSpec, x::AbstractFloat)
# Handle %
percentflag = (fs.typ == '%')
percentflag && (x *= 100)
# separate sign, integer, and decimal part
prec = fs.prec
rax = round(abs(x); digits = prec)
sch = _signchar(x, fs.sign)
intv = _truncval(rax)
decv = rax - intv
# calculate length
xlen = ndigits(intv)
numsep, numini = fs.tsep ? divrem(xlen - 1, 3) : (0, 0)
xlen += ifelse(prec > 0, prec + 1, 0) + (sch != '\0') + numsep + percentflag
# calculate padding needed
pad = fs.width - xlen
prepad = postpad = zpad = 0
if pad > 0
if fs.zpad
zpad = pad
elseif fs.align == '<'
postpad = pad
elseif fs.align == '^'
prepad, postpad = pad>>1, (pad+1)>>1
else
prepad = pad
end
end
# left padding
prepad == 0 || _repprint(out, fs.fill, prepad)
# print sign
sch != '\0' && print(out, sch)
# print padding zeros
zpad > 0 && _repprint(out, '0', zpad)
# print integer part
intv == 0 ? print(out, '0') :
numsep == 0 ? _outint(out, intv) : _outint(out, intv, _Dec(), numini, fs.sep)
# print decimal part
if prec > 0
print(out, '.')
idecv = round(Integer, decv * exp10(prec))
nd = ndigits(idecv)
nd < prec && _repprint(out, '0', prec - nd)
_outint(out, idecv)
end
# print trailing percent sign
percentflag && print(out, '%')
# right padding
postpad == 0 || _repprint(out, fs.fill, postpad)
end
function _pfmt_e(out::IO, fs::FormatSpec, x::AbstractFloat)
# extract sign, significand, and exponent
prec = fs.prec
ax = abs(x)
sch = _signchar(x, fs.sign)
if ax == 0.0
e = 0
u = zero(x)
else
rax = round(ax; sigdigits = prec + 1)
e = floor(Integer, log10(rax)) # exponent
u = round(rax * exp10(-e); sigdigits = prec + 1) # significand
i = 0
v10 = 1
while isinf(u)
i += 1
i > 18 && (u = 0.0; e = 0; break)
v10 *= 10
u = round(v10 * rax * exp10(-e - i); sigdigits = prec + 1)
end
end
# calculate length
xlen = 6 + prec + (sch != '\0') + (abs(e) > 99 ? ndigits(abs(e)) - 2 : 0)
# calculate padding
pad = fs.width - xlen
prepad = postpad = zpad = 0
if pad > 0
if fs.zpad
zpad = pad
elseif fs.align == '<'
postpad = pad
elseif fs.align == '^'
prepad, postpad = pad>>1, (pad+1)>>1
else
prepad = pad
end
end
# left padding
prepad == 0 || _repprint(out, fs.fill, prepad)
# print sign
sch != '\0' && print(out, sch)
# print padding zeros
zpad > 0 && _repprint(out, '0', zpad)
# print actual digits
intv = trunc(Integer, u)
decv = u - intv
if intv == 0 && decv != 0
intv = 1
decv -= 1
end
# print integer part (should always be 0-9)
print(out, Char(Int('0') + intv))
# print decimal part
if prec > 0
print(out, '.')
idecv = round(Integer, decv * exp10(prec))
nd = ndigits(idecv)
nd < prec && _repprint(out, '0', prec - nd)
_outint(out, idecv)
end
# print exponent
print(out, isuppercase(fs.typ) ? 'E' : 'e')
if e >= 0
print(out, '+')
else
print(out, '-')
e = -e
end
e < 10 && print(out, '0')
_outint(out, e)
# right padding
postpad == 0 || _repprint(out, fs.fill, postpad)
end
function _pfmt_g(out::IO, fs::FormatSpec, x::AbstractFloat)
# number decomposition
ax = abs(x)
if 1.0e-4 <= ax < 1.0e6
_pfmt_f(out, fs, x)
else
_pfmt_e(out, fs, x)
end
end
function _pfmt_specialf(out::IO, fs::FormatSpec, x::AbstractFloat)
if isinf(x)
_pfmt_s(out, fs, x > 0 ? "Inf" : "-Inf")
else
@assert isnan(x)
_pfmt_s(out, fs, "NaN")
end
end
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 6255 | # formatting specification
# formatting specification language
#
# spec ::= [[fill]align][sign][#][0][width][,_][.prec][type]
# fill ::= <any character>
# align ::= '<' | '^' | '>'
# sign ::= '+' | '-' | ' '
# width ::= <integer>
# prec ::= <integer>
# type ::= 'b' | 'c' | 'd' | 'e' | 'E' | 'f' | 'F' | 'g' | 'G' |
# 'n' | 'o' | 'x' | 'X' | 's' | '%'
#
# Please refer to http://docs.python.org/2/library/string.html#formatspec
# for more details
#
## FormatSpec type
const _numtypchars = Set(['b', 'd', 'e', 'E', 'f', 'F', 'g', 'G', 'n', 'o', 'x', 'X', '%'])
_tycls(c::AbstractChar) =
(c == 'd' || c == 'n' || c == 'b' || c == 'o' || c == 'x') ? 'i' :
(c == 'e' || c == 'f' || c == 'g' || c == '%') ? 'f' :
(c == 'c') ? 'c' :
(c == 's') ? 's' :
error("Invalid type char $(c)")
struct FormatSpec
cls::Char # category: 'i' | 'f' | 'c' | 's'
typ::Char
fill::Char
align::Char
sign::Char
width::Int
prec::Int
ipre::Bool # whether to prefix 0b, 0o, or 0x
zpad::Bool # whether to do zero-padding
tsep::Bool # whether to use thousand-separator
sep::Char
function FormatSpec(typ::AbstractChar;
fill::AbstractChar=' ',
align::AbstractChar='\0',
sign::AbstractChar='-',
width::Int=-1,
prec::Int=-1,
ipre::Bool=false,
zpad::Bool=false,
tsep=nothing)
align == '\0' && (align = (typ in _numtypchars) ? '>' : '<')
cls = _tycls(lowercase(typ))
cls == 'f' && prec < 0 && (prec = 6)
if tsep === true
sep = ','
elseif tsep isa AbstractChar
sep = Char(tsep)
tsep = true
else
sep = '\0'
tsep = false
end
new(cls, Char(typ), Char(fill), Char(align), Char(sign), width, prec, ipre, zpad,
tsep, sep)
end
# copy constructor with overrides
function FormatSpec(spec::FormatSpec;
fill::AbstractChar=spec.fill,
align::AbstractChar=spec.align,
sign::AbstractChar=spec.sign,
width::Int=spec.width,
prec::Int=spec.prec,
ipre::Bool=spec.ipre,
zpad::Bool=spec.zpad,
tsep=nothing)
if tsep === nothing
tsep = spec.tsep
sep = spec.sep
elseif tsep === true
sep = ','
elseif tsep isa AbstractChar
sep = Char(tsep)
tsep = true
else
sep = '\0'
tsep = false
end
new(spec.cls, spec.typ, Char(fill), Char(align), Char(sign), width, prec, ipre, zpad,
tsep, sep)
end
end
function show(io::IO, fs::FormatSpec)
println(io, typeof(fs))
println(io, " cls = ", fs.cls)
println(io, " typ = ", fs.typ)
println(io, " fill = ", fs.fill)
println(io, " align = ", fs.align)
println(io, " sign = ", fs.sign)
println(io, " width = ", fs.width)
println(io, " prec = ", fs.prec)
println(io, " ipre = ", fs.ipre)
println(io, " zpad = ", fs.zpad)
println(io, " tsep = ", fs.tsep ? repr(fs.sep) : "false")
end
## parse FormatSpec from a string
const _spec_regex = r"^(.?[<^>])?([ +-])?(#)?(\d+)?([,_])?(.\d+)?([bcdeEfFgGnosxX%])?$"
function FormatSpec(s::AbstractString)
# default spec
_fill = ' '
_align = '\0'
_sign = '-'
_width = -1
_prec = -1
_ipre = false
_zpad = false
_tsep = false
_typ = 's'
if !isempty(s)
m = match(_spec_regex, s)
m == nothing && error("Invalid formatting spec: $(s)")
(a1, a2, a3, a4, a5, a6, a7) = m.captures
# a1: [[fill]align]
if a1 != nothing
if length(a1) == 1
_align = a1[1]
else
_fill = a1[1]
_align = a1[nextind(a1, 1)]
end
end
# a2: [sign]
a2 == nothing || (_sign = a2[1])
# a3: [#]
a3 == nothing || (_ipre = true)
# a4: [0][width]
if a4 != nothing
if a4[1] == '0'
_zpad = true
length(a4) > 1 && (_width = parse(Int, a4[2:end]))
else
_width = parse(Int, a4)
end
end
# a5: [,_]
a5 == nothing || (_tsep = a5[1])
# a6 [.prec]
a6 == nothing || (_prec = parse(Int, a6[2:end]))
# a7: [type]
a7 == nothing || (_typ = a7[1])
end
FormatSpec(_typ;
fill=_fill,
align=_align,
sign=_sign,
width=_width,
prec=_prec,
ipre=_ipre,
zpad=_zpad,
tsep=_tsep)
end # function FormatSpec
## formatted printing using a format spec
struct _Dec end
struct _Oct end
struct _Hex end
struct _HEX end
struct _Bin end
_srepr(x) = repr(x)
_srepr(x::AbstractString) = x
_srepr(x::Symbol) = string(x)
_srepr(x::AbstractChar) = string(x)
_srepr(x::Enum) = string(x)
function printfmt(io::IO, fs::FormatSpec, x)
cls = fs.cls
ty = fs.typ
if cls == 'i'
ix = Integer(x)
ty == 'd' || ty == 'n' ? _pfmt_i(io, fs, ix, _Dec()) :
ty == 'x' ? _pfmt_i(io, fs, ix, _Hex()) :
ty == 'X' ? _pfmt_i(io, fs, ix, _HEX()) :
ty == 'o' ? _pfmt_i(io, fs, ix, _Oct()) :
_pfmt_i(io, fs, ix, _Bin())
elseif cls == 'f'
fx = float(x)
if isfinite(fx)
ty == 'f' || ty == 'F' || ty == '%' ? _pfmt_f(io, fs, fx) :
ty == 'e' || ty == 'E' ? _pfmt_e(io, fs, fx) : _pfmt_g(io, fs, fx)
else
_pfmt_specialf(io, fs, fx)
end
elseif cls == 's'
_pfmt_s(io, fs, _srepr(x))
else # cls == 'c'
_pfmt_s(io, fs, Char(x))
end
end
printfmt(fs::FormatSpec, x) = printfmt(_stdout(), fs, x)
pyfmt(fs::FormatSpec, x) = (buf = IOBuffer(); printfmt(buf, fs, x); String(take!(buf)))
pyfmt(spec::AbstractString, x) = pyfmt(FormatSpec(spec), x)
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 4647 | # formatting expression
### Argument specification
struct ArgSpec
argidx::Int
hasfilter::Bool
filter::Function
function ArgSpec(idx::Int, hasfil::Bool, filter::Function)
idx != 0 || error("Argument index cannot be zero.")
new(idx, hasfil, filter)
end
end
getarg(args, sp::ArgSpec) =
(a = args[sp.argidx]; sp.hasfilter ? sp.filter(a) : a)
# pos > 0: must not have iarg in expression (use pos+1), return (entry, pos + 1)
# pos < 0: must have iarg in expression, return (entry, -1)
# pos = 0: no positional argument before, can be either, return (entry, 1) or (entry, -1)
function make_argspec(s::AbstractString, pos::Int)
# for argument position
iarg::Int = -1
hasfil::Bool = false
ff::Function = Base.identity
if !isempty(s)
filrange = findfirst("|>", s)
if filrange === nothing
iarg = parse(Int, s)
else
ifil = first(filrange)
iarg = ifil > 1 ? parse(Int, s[1:prevind(s, ifil)]) : -1
hasfil = true
ff = m_eval(Symbol(s[ifil+2:end]))
end
end
if pos > 0
iarg < 0 || error("entry with and without argument index must not coexist.")
iarg = (pos += 1)
elseif pos < 0
iarg > 0 || error("entry with and without argument index must not coexist.")
else # pos == 0
if iarg < 0
iarg = pos = 1
else
pos = -1
end
end
return (ArgSpec(iarg, hasfil, ff), pos)
end
### Format entry
struct FormatEntry
argspec::ArgSpec
spec::FormatSpec
end
function make_formatentry(s::AbstractString, pos::Int)
@assert s[1] == '{' && s[end] == '}'
sc = s[2:prevind(s, lastindex(s))]
icolon = findfirst(isequal(':'), sc)
if icolon === nothing # no colon
(argspec, pos) = make_argspec(sc, pos)
spec = FormatSpec('s')
else
(argspec, pos) = make_argspec(sc[1:prevind(sc, icolon)], pos)
spec = FormatSpec(sc[nextind(sc, icolon):end])
end
return (FormatEntry(argspec, spec), pos)
end
### Format expression
mutable struct FormatExpr
prefix::UTF8Str
suffix::UTF8Str
entries::Vector{FormatEntry}
inter::Vector{UTF8Str}
end
_raise_unmatched_lbrace() = error("Unmatched { in format expression.")
function find_next_entry_open(s::AbstractString, si::Int)
slen = lastindex(s)
p = findnext(isequal('{'), s, si)
(p === nothing || p < slen) || _raise_unmatched_lbrace()
while p !== nothing && s[p+1] == '{' # escape `{{`
p = findnext(isequal('{'), s, p+2)
(p === nothing || p < slen) || _raise_unmatched_lbrace()
end
pre = p !== nothing ? s[si:prevind(s, p)] : s[si:end]
if !isempty(pre)
pre = replace(pre, "{{" => '{')
pre = replace(pre, "}}" => '}')
end
return (p, convert(UTF8Str, pre))
end
function find_next_entry_close(s::AbstractString, si::Int)
p = findnext(isequal('}'), s, si)
p !== nothing || _raise_unmatched_lbrace()
return p
end
function FormatExpr(s::AbstractString)
slen = length(s)
# init
prefix = UTF8Str("")
suffix = UTF8Str("")
entries = FormatEntry[]
inter = UTF8Str[]
# scan
(p, prefix) = find_next_entry_open(s, 1)
if p !== nothing
q = find_next_entry_close(s, p+1)
(e, pos) = make_formatentry(s[p:q], 0)
push!(entries, e)
(p, pre) = find_next_entry_open(s, q+1)
while p !== nothing
push!(inter, pre)
q = find_next_entry_close(s, p+1)
(e, pos) = make_formatentry(s[p:q], pos)
push!(entries, e)
(p, pre) = find_next_entry_open(s, q+1)
end
suffix = pre
end
FormatExpr(prefix, suffix, entries, inter)
end
function printfmt(io::IO, fe::FormatExpr, args...)
isempty(fe.prefix) || print(io, fe.prefix)
ents = fe.entries
ne = length(ents)
if ne > 0
e = ents[1]
printfmt(io, e.spec, getarg(args, e.argspec))
for i = 2:ne
print(io, fe.inter[i-1])
e = ents[i]
printfmt(io, e.spec, getarg(args, e.argspec))
end
end
isempty(fe.suffix) || print(io, fe.suffix)
end
const StringOrFE = Union{AbstractString, FormatExpr}
printfmt(io::IO, fe::AbstractString, args...) = printfmt(io, FormatExpr(fe), args...)
printfmt(fe::StringOrFE, args...) = printfmt(_stdout(), fe, args...)
printfmtln(io::IO, fe::StringOrFE, args...) = (printfmt(io, fe, args...); println(io))
printfmtln(fe::StringOrFE, args...) = printfmtln(_stdout(), fe, args...)
format(fe::StringOrFE, args...) = sprint(printfmt, fe, args...)
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 19160 | # C-style formatting functions, originally based on Printf.jl
using Base.Ryu
abstract type FmtType end
struct FmtDec <: FmtType end
struct FmtOct <: FmtType end
struct FmtHex <: FmtType end
struct FmtChr <: FmtType end
struct FmtStr <: FmtType end
struct FmtPtr <: FmtType end
struct FmtFltE <: FmtType end
struct FmtFltF <: FmtType end
struct FmtFltG <: FmtType end
struct FmtFltA <: FmtType end
struct FmtN <: FmtType end
const VALID_FMTS = b"duoxefgacsipnDUOXEFGACS"
const FMT_TYPES = [FmtDec, FmtDec, FmtOct, FmtHex, FmtFltE, FmtFltF, FmtFltG, FmtFltA, FmtChr, FmtStr,
FmtDec, FmtPtr, FmtN]
# format specifier categories
const FmtInts = Union{FmtDec, FmtOct, FmtHex}
const FmtFlts = Union{FmtFltE, FmtFltF, FmtFltG, FmtFltA}
"""
Typed representation of a format specifier.
Fields are the various modifiers allowed for various format specifiers.
"""
struct FmtSpec{T<:FmtType}
char::UInt8
tsep::UInt8 # thousands separator (default ',')
leftalign::Bool
plus::Bool
space::Bool
zero::Bool
altf::Bool # alternate formatting ('#' flag)
width::Int # negative means *, 0 means no width specified
prec::Int # -2 means *, -1 means no precision specified
end
# recreate the format specifier string from a typed FmtSpec
Base.string(f::FmtSpec; modifier::String="") =
string("%",
f.leftalign ? "-" : "",
f.plus ? "+" : "",
f.space ? " " : "",
f.zero ? "0" : "",
f.altf ? "#" : "",
f.tsep == 0 ? "" : "'",
f.width > 0 ? "$(f.width)" : f.width < 0 ? "*" : "",
f.prec < -1 ? "*" : f.prec >= 0 ? ".$(f.prec)" : "",
modifier,
Char(f.char))
Base.show(io::IO, f::FmtSpec) = print(io, string(f))
floatfmt(s::FmtSpec) =
FmtSpec{FmtFltF}(s.char, s.tsep, s.leftalign, s.plus, s.space, s.zero, s.altf, s.width, 0)
ptrfmt(s::FmtSpec, x) =
FmtSpec{FmtHex}(s.char, s.tsep, s.leftalign, s.plus, s.space, s.zero, true, s.width,
ifelse(sizeof(x) == 8, 16, 8))
function FmtSpec(s::FmtSpec{F}; width=s.width, prec=s.prec) where {F}
s.width < 0 || throw(ArgumentError("Already has width $(s.width)"))
s.prec < -1 || throw(ArgumentError("Already has precision $(s.prec)"))
width < 0 && throw(ArgumentError("Width $(width) must be >= 0"))
prec < -1 && throw(ArgumentError("Precision $(prec) must be >= -1"))
FmtSpec{F}(s.char, s.tsep, s.leftalign, s.plus, s.space, s.zero, s.altf, width, prec)
end
# parse format string
function FmtSpec(f::AbstractString)
isempty(f) && throw(ArgumentError("empty format string"))
bytes = codeunits(f) # Note: codeunits are not necessarily *bytes*!
len = length(bytes)
bytes[1] === UInt8('%') || throw(ArgumentError("Format string doesn't start with %"))
b = bytes[2]
pos = 3
# positioned at start of first format str %
# parse flags
leftalign = plus = space = zero = altf = false
tsep = UInt8(0)
while true
if b == UInt8('-')
leftalign = true
elseif b == UInt8('+')
plus = true
elseif b == UInt8(' ')
space = true
elseif b == UInt8('0')
zero = true
elseif b == UInt8('#')
altf = true
elseif b == UInt8('\'')
tsep = UInt8(',')
else
break
end
pos > len && throw(ArgumentError("incomplete format string: '$f'"))
b = bytes[pos]
pos += 1
end
leftalign && (zero = false)
# parse width
if b == UInt('*')
width = -1
else
width = 0
while b - UInt8('0') < 0x0a
width = 10 * width + (b - UInt8('0'))
b = bytes[pos]
pos += 1
pos > len && break
end
end
# parse prec
prec = 0
parsedprecdigits = false
if b == UInt8('.')
pos > len && throw(ArgumentError("incomplete format string: '$f'"))
parsedprecdigits = true
b = bytes[pos]
pos += 1
if pos <= len
if b == UInt('*')
prec == -2
else
prec = 0
while b - UInt8('0') < 0x0a
prec = 10 * prec + (b - UInt8('0'))
b = bytes[pos]
pos += 1
pos > len && break
end
end
end
end
# parse length modifier (ignored)
if b == UInt8('h') || b == UInt8('l')
prev = b
pos > len &&
throw(ArgumentError("format string - length modifier is missing type specifier: '$f'"))
b = bytes[pos]
pos += 1
if b == prev
pos > len && throw(ArgumentError("invalid format string: '$f'"))
b = bytes[pos]
pos += 1
end
elseif b in b"Ljqtz"
pos > len &&
throw(ArgumentError("format string - length modifier is missing type specifier: '$f'"))
b = bytes[pos]
pos += 1
end
# parse type
fmtind = findfirst(isequal(b), VALID_FMTS)
fmtind === nothing &&
throw(ArgumentError(string("invalid format string: '$f', ",
"invalid type specifier: '$(Char(b))'")))
# Check for uppercase variants
fmtind > 13 && (fmtind -= 13)
fmttyp = FMT_TYPES[fmtind]
if fmttyp <: FmtInts && prec > 0
zero = false
elseif !parsedprecdigits
if (fmttyp === FmtStr || fmttyp === FmtChr || fmttyp === FmtFltA)
prec = -1
elseif fmttyp <: FmtFlts
prec = 6
end
end
FmtSpec{fmttyp}(b, tsep, leftalign, plus, space, zero, altf, width, prec)
end
@inline gethexbase(spec) = spec.char < UInt8('a') ? b"0123456789ABCDEF" : b"0123456789abcdef"
@inline upchar(spec, ch) = (spec.char & 0x20) | UInt8(ch)
# write out a single arg according to format options
# char
@inline function writechar(buf, pos, c::Char)
u = bswap(reinterpret(UInt32, c))
while true
@inbounds buf[pos] = u % UInt8
pos += 1
(u >>= 8) == 0 && break
end
return pos
end
@inline function padn(buf, pos, cnt)
@inbounds for _ = 1:cnt
buf[pos] = UInt8(' ')
pos += 1
end
pos
end
@inline function padzero(buf, pos, cnt)
@inbounds for _ = 1:cnt
buf[pos] = UInt8('0')
pos += 1
end
pos
end
function _fmt(buf, pos, spec::FmtSpec{FmtChr}, arg)
ch = arg isa String ? arg[1] : Char(arg)
width = spec.width - textwidth(ch)
width <= 0 && return writechar(buf, pos, ch)
if spec.leftalign
padn(buf, writechar(buf, pos, ch), width)
else
writechar(buf, padn(buf, pos, width), ch)
end
end
@inline function outch(buf, pos, ch)
@inbounds buf[pos] = UInt8(ch)
pos + 1
end
@inline function outch(buf, pos, c1, c2)
@inbounds buf[pos] = UInt8(c1)
@inbounds buf[pos + 1] = UInt8(c2)
pos + 2
end
@inline function outch(buf, pos, c1, c2, c3)
@inbounds buf[pos] = UInt8(c1)
@inbounds buf[pos + 1] = UInt8(c2)
@inbounds buf[pos + 2] = UInt8(c3)
pos + 3
end
# strings
function _fmt(buf, pos, spec::FmtSpec{FmtStr}, arg)
altf, width, prec = spec.altf, spec.width, spec.prec
s = altf && (arg isa Symbol || arg isa AbstractString) ? repr(arg) : string(arg)
slen = textwidth(s)
str, slen = 0 <= prec < slen ? _truncstr(s, slen, prec) : (s, slen)
prepad = postpad = 0
pad = width - slen
if pad > 0
if spec.leftalign
postpad = pad
else
prepad = pad
end
end
# Make sure there is enough room in buffer
nlen = pos + prepad + sizeof(str) + postpad
nlen > sizeof(buf) && resize!(buf, nlen)
prepad == 0 || (pos = padn(buf, pos, prepad))
for c in str
pos = writechar(buf, pos, c)
end
return postpad == 0 ? pos : padn(buf, pos, postpad)
end
const BaseInt = Union{Int8, Int16, Int32, Int64, Int128}
const BaseUns = Union{UInt8, UInt16, UInt32, UInt64, UInt128}
base(::Type{FmtOct}) = 8
base(::Type{FmtDec}) = 10
base(::Type{FmtHex}) = 16
# integers
_fmt(buf, pos, spec::FmtSpec{<:FmtInts}, arg::AbstractFloat) =
_fmt(buf, pos, floatfmt(spec), arg)
_fmt(buf, pos, spec::FmtSpec{T}, arg::Real) where {T<:FmtInts} =
_fmt(buf, pos, spec, arg < 0, string(abs(arg); base=base(T)))
_fmt(buf, pos, spec::FmtSpec{<:FmtInts}, arg::BaseUns) =
_fmt(buf, pos, spec, false, arg)
_fmt(buf, pos, spec::FmtSpec{<:FmtInts}, arg::BaseInt) =
_fmt(buf, pos, spec, arg < 0, unsigned(abs(arg)))
hex_len(x) = x == 0 ? 1 : (sizeof(x)<<1) - (leading_zeros(x)>>2)
oct_len(x) = x == 0 ? 1 : div((sizeof(x)<<3) - leading_zeros(x)+2, 3)
function _fmt(buf, pos, spec::FmtSpec{F}, neg, x::T) where {F<:FmtInts,T<:Union{String,BaseUns}}
n = T === String ? sizeof(x) :
F === FmtDec ? dec_len(x) : F === FmtHex ? hex_len(x) : oct_len(x)
i = n
arglen = n + (neg || (spec.plus | spec.space)) +
((spec.altf && (F !== FmtDec)) ? ifelse(F === FmtOct, 1, 2) : 0)
width, prec = spec.width, spec.prec
precpad = max(0, prec - n)
# Calculate width including padding due to width or precision
arglen2 = arglen < width && prec > 0 ? arglen + min(precpad, width - arglen) : arglen
# Make sure that remaining output buffer is large enough
# This means that it isn't necessary to preallocate for cases that usually will never happen
buflen = pos + max(width, arglen + precpad)
buflen > sizeof(buf) && resize!(buf, buflen)
!spec.leftalign && !spec.zero && arglen2 < width &&
(pos = padn(buf, pos, width - arglen2))
if neg
pos = outch(buf, pos, '-')
elseif spec.plus # plus overrides space
pos = outch(buf, pos, '+')
elseif spec.space
pos = outch(buf, pos, ' ')
end
if spec.altf
if F === FmtOct
pos = outch(buf, pos, '0')
elseif F === FmtHex
pos = outch(buf, pos, '0', upchar(spec, 'X'))
end
end
if spec.zero && arglen2 < width
pos = padzero(buf, pos, width - arglen2)
elseif n < prec
pos = padzero(buf, pos, precpad)
elseif arglen < arglen2
pos = padzero(buf, pos, arglen2 - arglen)
end
if T === String
GC.@preserve buf x begin
unsafe_copyto!(pointer(buf, pos), pointer(x), n)
end
elseif F === FmtDec
while i > 0
d, r = divrem(x, 10)
@inbounds buf[pos + i - 1] = UInt8('0') + r
x = oftype(x, d)
i -= 1
end
elseif F === FmtHex
hexbase = gethexbase(spec)
while i > 0
@inbounds buf[pos + i - 1] = hexbase[(x & 0x0f) + 1]
x >>= 4
i -= 1
end
else # F === FmtOct
while i > 0
@inbounds buf[pos + i - 1] = UInt8('0') + (x & 0x07)
x >>= 3
i -= 1
end
end
(spec.leftalign && arglen2 < width) ? padn(buf, pos + n, width - arglen2) : (pos + n)
end
# floats
"""
tofloat(x)
Convert an argument to a Base float type for printf formatting.
By default, arguments are converted to `Float64` via `Float64(x)`.
Custom numeric types that have a conversion to a Base float type
that wish to hook into printf formatting can extend this method like:
```julia
Printf.tofloat(x::MyCustomType) = convert_my_custom_type_to_float(x)
```
For arbitrary precision numerics, you might extend the method like:
```julia
Printf.tofloat(x::MyArbitraryPrecisionType) = BigFloat(x)
```
"""
tofloat(x) = Float64(x)
tofloat(x::Base.IEEEFloat) = x
function output_fmt_a(buf, pos, spec, neg, x)
if neg
pos = outch(buf, pos, '-')
elseif spec.plus
pos = outch(buf, pos, '+')
elseif spec.space
pos = outch(buf, pos, ' ')
end
isnan(x) && return outch(buf, pos, 'N', 'a', 'N')
isfinite(x) || return outch(buf, pos, 'I', 'n', 'f')
prec = spec.prec
pos = outch(buf, pos, '0', upchar(spec, 'X'))
if x == 0
pos = outch(buf, pos, '0')
prec > 0 && (pos = outch(buf, pos, '.'); pos = padzero(buf, pos, prec))
return outch(buf, pos, upchar(spec, 'P'), '+', '0')
end
s, p = frexp(x)
prec = spec.prec
if prec > -1
sigbits = 4 * min(prec, 13)
s = 0.25 * round(ldexp(s, 1 + sigbits))
# ensure last 2 exponent bits either 01 or 10
u = (reinterpret(UInt64, s) & 0x003f_ffff_ffff_ffff) >> (52 - sigbits)
i = n = (sizeof(u) << 1) - (leading_zeros(u) >> 2)
else
s *= 2.0
u = (reinterpret(UInt64, s) & 0x001f_ffff_ffff_ffff)
t = (trailing_zeros(u) >> 2)
u >>= (t << 2)
i = n = 14 - t
end
frac = u > 9 || spec.altf || prec > 0
hexbase = gethexbase(spec)
while i > 1
buf[pos + i] = hexbase[(u & 0x0f) + 1]
u >>= 4
i -= 1
prec -= 1
end
frac && (buf[pos + 1] = UInt8('.'))
buf[pos] = hexbase[(u & 0x0f) + 1]
pos += n + frac
while prec > 0
pos = outch(buf, pos, '0')
prec -= 1
end
pos = outch(buf, pos, upchar(spec, 'P'))
p -= 1
pos = outch(buf, pos, p < 0 ? UInt8('-') : UInt8('+'))
p = p < 0 ? -p : p
n = i = ndigits(p, base=10, pad=1)
while i > 0
d, r = divrem(p, 10)
buf[pos + i - 1] = UInt8('0') + r
p = oftype(p, d)
i -= 1
end
return pos + n
end
const _strspec = Dict{FmtSpec,ASCIIStr}()
"""Get the format specification, prepared for use with MPFR for BigFloats"""
function _get_strspec(spec)
global _strspec
chkspec = get(_strspec, spec, nothing)
chkspec === nothing || return chkspec
_strspec[spec] = string(spec; modifier="R")
end
@static if VERSION < v"1.5"
_snprintf(ptr, siz, spec, arg) =
ccall((:mpfr_snprintf,:libmpfr), Int32,
(Ptr{UInt8}, Culong, Ptr{UInt8}, Ref{BigFloat}...),
ptr, siz, spec, arg)
else
_snprintf(ptr, siz, spec, arg) =
@ccall "libmpfr".mpfr_snprintf(ptr::Ptr{UInt8}, siz::Csize_t, spec::Ptr{UInt8};
arg::Ref{BigFloat})::Cint
end
function _fmt(buf, pos, spec::FmtSpec{<:FmtFlts}, arg::BigFloat)
isfinite(arg) || return _fmt(buf, pos, spec, Float64(arg))
strspec = _get_strspec(spec)
siz = length(buf) - pos + 1
# Calculate size needed for most common outputs
len = max(spec.width, ceil(Int, precision(arg) * log(2)/log(10)) + 24)
if len > siz
resize!(buf, pos + len + 1)
siz = length(buf) - pos + 1
end
GC.@preserve buf begin
len = _snprintf(pointer(buf, pos), siz, strspec, arg)
if len > siz
resize!(buf, pos + len + 1)
len = _snprintf(pointer(buf, pos), len + 1, strspec, arg)
end
end
len > 0 || error("invalid printf formatting for BigFloat")
pos + len
end
function _fmt(buf, pos, spec::FmtSpec{T}, arg) where {T <: FmtFlts}
# Make sure there is enough room
width, prec, plus, space, hash = spec.width, spec.prec, spec.plus, spec.space, spec.altf
buflen = sizeof(buf) - pos
needed = max(width, 309 + 17 + 5)
buflen < needed && resize!(buf, pos + needed)
x = tofloat(arg)
if T === FmtFltE
newpos = Ryu.writeexp(buf, pos, x, prec, plus, space, hash, upchar(spec, 'E'), UInt8('.'))
elseif T === FmtFltF
newpos = Ryu.writefixed(buf, pos, x, prec, plus, space, hash, UInt8('.'))
elseif T === FmtFltG
if isinf(x) || isnan(x)
newpos = Ryu.writeshortest(buf, pos, x, plus, space)
else
# C11-compliant general format
prec = prec == 0 ? 1 : prec
# format the value in scientific notation and parse the exponent part
exp = let p = Ryu.writeexp(buf, pos, x, prec)
b1, b2, b3, b4 = buf[p-4], buf[p-3], buf[p-2], buf[p-1]
Z = UInt8('0')
if b1 == UInt8('e')
# two-digit exponent
sign = b2 == UInt8('+') ? 1 : -1
exp = 10 * (b3 - Z) + (b4 - Z)
else
# three-digit exponent
sign = b1 == UInt8('+') ? 1 : -1
exp = 100 * (b2 - Z) + 10 * (b3 - Z) + (b4 - Z)
end
flipsign(exp, sign)
end
if -4 ≤ exp < prec
newpos = Ryu.writefixed(buf, pos, x, prec - (exp + 1), plus, space, hash,
UInt8('.'), !hash)
else
newpos = Ryu.writeexp(buf, pos, x, prec - 1, plus, space, hash,
upchar(spec, 'E'), UInt8('.'), !hash)
end
end
elseif T === FmtFltA
x, neg = x < 0 || x === -Base.zero(x) ? (-x, true) : (x, false)
newpos = output_fmt_a(buf, pos, spec, neg, x)
end
if newpos - pos < width
# need to pad
if spec.leftalign
# easy case, just pad spaces after number
newpos = padn(buf, newpos, width - (newpos - pos))
else
# right aligned
n = width - (newpos - pos)
if spec.zero && isfinite(x)
ex = (arg < 0 || (plus | space)) + ifelse(T === FmtFltA, 2, 0)
so = pos + ex
len = (newpos - pos) - ex
copyto!(buf, so + n, buf, so, len)
for i = so:(so + n - 1)
buf[i] = UInt8('0')
end
newpos += n
else
copyto!(buf, pos + n, buf, pos, newpos - pos)
for i = pos:(pos + n - 1)
buf[i] = UInt8(' ')
end
newpos += n
end
end
end
return newpos
end
# pointers
_fmt(buf, pos, spec::FmtSpec{FmtPtr}, arg) =
_fmt(buf, pos, ptrfmt(spec, arg), UInt64(arg))
@inline _dec_len1(v) = ifelse(v < 100, ifelse(v < 10, 1, 2), 3)
@inline _dec_len2(v) = v < 1_000 ? _dec_len1(v) : ifelse(v < 10_000, 4, 5)
@inline _dec_len4(v) = v < 100_000 ? _dec_len2(v) :
(v < 10_000_000
? ifelse(v < 1_000_000, 6, 7)
: ifelse(v < 100_000_000, 8, ifelse(v < 1_000_000_000, 9, 10)))
@inline function _dec_len8(v)
if v < 1_000_000_000 # 1 - 9 digits
_dec_len4(v)
elseif v < 10_000_000_000_000 # 10 - 13 digits
if v < 100_000_000_000
ifelse(v < 10_000_000_000, 10, 11)
else
ifelse(v < 1_000_000_000_000, 12, 13)
end
elseif v < 100_000_000_000_000_000 # 14 - 17 digits
if v < 1_000_000_000_000_000
ifelse(v < 100_000_000_000_000, 14, 15)
else
ifelse(v < 10_000_000_000_000_000, 16, 17)
end
else
v < 10_000_000_000_000_000_000 ? ifelse(v < 1_000_000_000_000_000_000, 18, 19) : 20
end
end
@inline dec_len(v::UInt8) = _dec_len1(v)
@inline dec_len(v::UInt16) = _dec_len2(v)
@inline dec_len(v::UInt32) = _dec_len4(v)
@inline dec_len(v::UInt64) = _dec_len8(v)
@inline function dec_len(v::UInt128)
v <= typemax(UInt64) && return _dec_len8(v%UInt64)
v = div(v, 0x8ac7230489e80000) # 10^19
v <= typemax(UInt64) ? _dec_len8(v%UInt64) + 19 : 39
end
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 6315 | _erfinv(z) = sqrt(π) * Base.Math.@horner(z, 0, 1, 0, π/12, 0, 7π^2/480, 0, 127π^3/40320, 0,
4369π^4/5806080, 0, 34807π^5/182476800) / 2
set_seed!(seed) = Random.seed!(seed)
function test_equality()
println( "test cformat equality...")
set_seed!(10)
fmts = [ (x->@sprintf("%10.4f",x), "%10.4f"),
(x->@sprintf("%f", x), "%f"),
(x->@sprintf("%e", x), "%e"),
(x->@sprintf("%10f", x), "%10f"),
(x->@sprintf("%.3f", x), "%.3f"),
(x->@sprintf("%.3e", x), "%.3e")]
for (mfmtr,fmt) in fmts
for i in 1:10000
n = _erfinv( rand() * 1.99 - 1.99/2.0 )
expect = mfmtr( n )
actual = cfmt( fmt, n )
@test expect == actual
end
end
fmts = [ (x->@sprintf("%d",x), "%d"),
(x->@sprintf("%10d",x), "%10d"),
(x->@sprintf("%010d",x), "%010d"),
(x->@sprintf("%-10d",x), "%-10d")]
for (mfmtr,fmt) in fmts
for i in 1:10000
j = round(Int, _erfinv( rand() * 1.99 - 1.99/2.0 ) * 100000 )
expect = mfmtr( j )
actual = cfmt( fmt, j )
@test expect == actual
end
end
println( "...Done" )
end
@time test_equality()
include("speedtest.jl")
@testset "test commas..." begin
@test cfmt( "%'d", 1000 ) == "1,000"
@test cfmt( "%'d", -1000 ) == "-1,000"
@test cfmt( "%'d", 100 ) == "100"
@test cfmt( "%'d", -100 ) == "-100"
@test cfmt( "%'f", Inf ) == "Inf"
@test cfmt( "%'f", -Inf ) == "-Inf"
@test cfmt( "%'s", 1000.0 ) == "1,000.0"
@test cfmt( "%'s", 1234567.0 ) == "1.234567e6"
@test cfmt( "%'g", 1000.0 ) == "1,000"
end
@testset "Test bug introduced by stdlib/Printf rewrite" begin
@test cfmt( "%4.2s", "a" ) == " a"
end
@testset "Test precision with wide characters" begin
@test cfmt( "%10.9s", "\U1f355" ^ 6) == " " * "\U1f355" ^ 4
@test cfmt( "%10.10s", "\U1f355" ^ 6) == "\U1f355" ^ 5
end
@testset "test format..." begin
@test format( 10 ) == "10"
@test format( 10.0 ) == "10"
@test format( 10.0, precision=2 ) == "10.00"
@test format( 111//100, precision=2 ) == "1.11"
@test format( 111//100 ) == "111/100"
@test format( 1234, commas=true ) == "1,234"
@test format( 1234, conversion="f", precision=2 ) == "1234.00"
@test format( 1.23, precision=3 ) == "1.230"
@test format( 1.23, precision=3, stripzeros=true ) == "1.23"
@test format( 1.00, precision=3, stripzeros=true ) == "1"
@test format( 1.0, conversion="e", stripzeros=true ) == "1e+00"
@test format( 1.0, conversion="e", precision=4 ) == "1.0000e+00"
@test format( 1.0, signed=true ) == "+1"
@test format( 1.0, positivespace=true ) == " 1"
@test_throws ErrorException format( 1234.56, signed=true, commas=true )
@test format( 1.0, width=6, precision=4, stripzeros=true, leftjustified=true) == "1 "
end
@testset "hex output" begin
@test format( 1118, conversion="x" ) == "45e"
@test format( 1118, width=4, conversion="x" ) == " 45e"
@test format( 1118, width=4, zeropadding=true, conversion="x" ) == "045e"
@test format( 1118, alternative=true, conversion="x" ) == "0x45e"
@test format( 1118, width=4, alternative=true, conversion="x" ) == "0x45e"
@test format( 1118, width=6, alternative=true, conversion="x", zeropadding=true ) == "0x045e"
end
@testset "mixed fractions" begin
@test format( 3//2, mixedfraction=true ) == "1_1/2"
@test format( -3//2, mixedfraction=true ) == "-1_1/2"
@test format( 3//100, mixedfraction=true ) == "3/100"
@test format( -3//100, mixedfraction=true ) == "-3/100"
@test format( 307//100, mixedfraction=true ) == "3_7/100"
@test format( -307//100, mixedfraction=true ) == "-3_7/100"
@test format( 307//100, mixedfraction=true, fractionwidth=6 ) == "3_07/100"
@test format( -307//100, mixedfraction=true, fractionwidth=6 ) == "-3_07/100"
@test format( -302//100, mixedfraction=true ) == "-3_1/50"
# try to make the denominator 100
@test format( -302//100, mixedfraction=true,tryden = 100 ) == "-3_2/100"
@test format( -302//30, mixedfraction=true,tryden = 100 ) == "-10_1/15" # lose precision otherwise
@test format( -302//100, mixedfraction=true,tryden = 100,fractionwidth=6 ) == "-3_02/100" # lose precision otherwise
end
@testset "commas" begin
@test format( 12345678, width=10, commas=true ) == "12,345,678"
# it would try to squeeze out the commas
@test format( 12345678, width=9, commas=true ) == "12345,678"
# until it can't anymore
@test format( 12345678, width=8, commas=true ) == "12345678"
@test format( 12345678, width=7, commas=true ) == "12345678"
# only the numerator would have commas
@test format( 1111//1000, commas=true ) == "1,111/1000"
# this shows how, with enough space, parens line up with empty spaces
@test format( 12345678, width=12, commas=true, parens=true )== " 12,345,678 "
@test format( -12345678, width=12, commas=true, parens=true )== "(12,345,678)"
# same with unspecified width
@test format( 12345678, commas=true, parens=true )== " 12,345,678 "
@test format( -12345678, commas=true, parens=true )== "(12,345,678)"
end
@testset "autoscale" begin
@test format( 1.2e9, autoscale = :metric ) == "1.2G"
@test format( 1.2e6, autoscale = :metric ) == "1.2M"
@test format( 1.2e3, autoscale = :metric ) == "1.2k"
@test format( 1.2e-6, autoscale = :metric ) == "1.2μ"
@test format( 1.2e-9, autoscale = :metric ) == "1.2n"
@test format( 1.2e-12, autoscale = :metric ) == "1.2p"
@test format( 1.2e9, autoscale = :finance ) == "1.2b"
@test format( 1.2e6, autoscale = :finance ) == "1.2m"
@test format( 1.2e3, autoscale = :finance ) == "1.2k"
@test format( 0x40000000, autoscale = :binary ) == "1Gi"
@test format( 0x100000, autoscale = :binary ) == "1Mi"
@test format( 0x800, autoscale = :binary ) == "2Ki"
@test format( 0x400, autoscale = :binary ) == "1Ki"
end
@testset "suffix" begin
@test format( 100.00, precision=2, suffix="%" ) == "100.00%"
@test format( 100, precision=2, suffix="%" ) == "100%"
@test format( 100, precision=2, suffix="%", conversion="f" ) == "100.00%"
end
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 1315 | # some basic functionality testing
x = 1234.56789
@test fmt(x) == "1234.567890"
@test fmt(x;prec=2) == "1234.57"
@test fmt(x,10,3) == " 1234.568"
@test fmt(x,10,3,:left) == "1234.568 "
@test fmt(x,10,3,:ljust) == "1234.568 "
@test fmt(x,10,3,:right) == " 1234.568"
@test fmt(x,10,3,:rjust) == " 1234.568"
@test fmt(x,10,3,:center) == " 1234.568 "
@test fmt(x,10,3,:zpad) == "001234.568"
@test fmt(x,10,3,:zeropad) == "001234.568"
@test fmt(x,:commas) == "1,234.567890"
@test fmt(x,10,3,:left,:commas) == "1,234.568 "
@test fmt(x,:ipre) == "1234.567890"
@test fmt(x,12) == " 1234.567890"
i = 1234567
@test fmt(i) == "1234567"
@test fmt(i,:commas) == "1,234,567"
# These are not handled
#@test_throws ErrorException fmt_default(Real)
#@test_throws ErrorException fmt_default(Complex)
fmt_default!(Int, :commas, width = 12)
@test fmt(i) == " 1,234,567"
@test fmt(x) == "1234.567890" # default hasn't changed
fmt_default!(:commas)
@test fmt(i) == " 1,234,567"
@test fmt(x) == "1,234.567890" # width hasn't changed, but added commas
fmt_default!(Int) # resets Integer defaults
@test fmt(i) == "1234567"
@test fmt(i,:commas) == "1,234,567"
reset!(Int)
fmt_default!(UInt16, 'd', :commas)
@test fmt(0xffff) == "65,535"
fmt_default!(UInt32, UInt16, width=20)
@test fmt(0xfffff) == " 1,048,575"
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 13623 | # test format spec parsing
# default spec
@testset "Default spec" begin
fs = FormatSpec("")
@test fs.typ == 's'
@test fs.fill == ' '
@test fs.align == '<'
@test fs.sign == '-'
@test fs.width == -1
@test fs.prec == -1
@test fs.ipre == false
@test fs.zpad == false
@test fs.tsep == false
end
_contains(s, r) = occursin(r, s)
@testset "Show" begin
x = FormatSpec("#8,d")
io = IOBuffer()
show(io, x)
str = String(take!(io))
@test _contains(str, "width = 8")
end
@testset "Literal incorrect" begin
@test_throws ErrorException FormatSpec("Z")
end
# more cases
@testset "FormatSpec(\"d\")" begin
fs = FormatSpec("d")
@test fs == FormatSpec('d')
@test fs.align == '>'
@test FormatSpec("8x") == FormatSpec('x'; width=8)
@test FormatSpec("08b") == FormatSpec('b'; width=8, zpad=true)
@test FormatSpec("12f") == FormatSpec('f'; width=12, prec=6)
@test FormatSpec("12.7f") == FormatSpec('f'; width=12, prec=7)
@test FormatSpec("+08o") == FormatSpec('o'; width=8, zpad=true, sign='+')
@test FormatSpec("8") == FormatSpec('s'; width=8)
@test FormatSpec(".6f") == FormatSpec('f'; prec=6)
@test FormatSpec("<8d") == FormatSpec('d'; width=8, align='<')
@test FormatSpec("#<8d") == FormatSpec('d'; width=8, fill='#', align='<')
@test FormatSpec("⋆<8d") == FormatSpec('d'; width=8, fill='⋆', align='<')
@test FormatSpec("#^8d") == FormatSpec('d'; width=8, fill='#', align='^')
@test FormatSpec("#8,d") == FormatSpec('d'; width=8, ipre=true, tsep=true)
end
@testset "Format prefix" begin
@test pyfmt("#b", 6) == "0b110"
@test pyfmt("#o", 6) == "0o6"
@test pyfmt("#x", 6) == "0x6"
@test pyfmt("#X", 10) == "0XA"
end
@testset "Format string" begin
@test pyfmt("", "abc") == "abc"
@test pyfmt("", "αβγ") == "αβγ"
@test pyfmt("s", "abc") == "abc"
@test pyfmt("s", "αβγ") == "αβγ"
@test pyfmt("2s", "abc") == "abc"
@test pyfmt("2s", "αβγ") == "αβγ"
@test pyfmt("5s", "abc") == "abc "
@test pyfmt("5s", "αβγ") == "αβγ "
@test pyfmt(">5s", "abc") == " abc"
@test pyfmt(">5s", "αβγ") == " αβγ"
@test pyfmt("^5s", "abc") == " abc "
@test pyfmt("^5s", "αβγ") == " αβγ "
@test pyfmt("*>5s", "abc") == "**abc"
@test pyfmt("⋆>5s", "αβγ") == "⋆⋆αβγ"
@test pyfmt("*^5s", "abc") == "*abc*"
@test pyfmt("⋆^5s", "αβγ") == "⋆αβγ⋆"
@test pyfmt("*<5s", "abc") == "abc**"
@test pyfmt("⋆<5s", "αβγ") == "αβγ⋆⋆"
# Issue #83 (in Formatting.jl)
@test pyfmt(".3s", "\U1f595\U1f596") == "🖕"
@test pyfmt(".4s", "\U1f595\U1f596") == "🖕🖖"
@test pyfmt(".5s", "\U1f595\U1f596") == "🖕🖖"
@test pyfmt("6.3s", "\U1f595\U1f596") == "🖕 "
@test pyfmt("6.4s", "\U1f595\U1f596") == "🖕🖖 "
@test pyfmt("6.5s", "\U1f595\U1f596") == "🖕🖖 "
end
@testset "Format Symbol" begin
@test pyfmt("", :abc) == "abc"
@test pyfmt("s", :abc) == "abc"
end
@testset "Format Char" begin
@test pyfmt("", 'c') == "c"
@test pyfmt("", 'γ') == "γ"
@test pyfmt("c", 'c') == "c"
@test pyfmt("c", 'γ') == "γ"
@test pyfmt("3c", 'c') == "c "
@test pyfmt("3c", 'γ') == "γ "
@test pyfmt(">3c", 'c') == " c"
@test pyfmt(">3c", 'γ') == " γ"
@test pyfmt("^3c", 'c') == " c "
@test pyfmt("^3c", 'γ') == " γ "
@test pyfmt("*>3c", 'c') == "**c"
@test pyfmt("⋆>3c", 'γ') == "⋆⋆γ"
@test pyfmt("*^3c", 'c') == "*c*"
@test pyfmt("⋆^3c", 'γ') == "⋆γ⋆"
@test pyfmt("*<3c", 'c') == "c**"
@test pyfmt("⋆<3c", 'γ') == "γ⋆⋆"
end
@testset "Format integer" begin
@test pyfmt("", 1234) == "1234"
@test pyfmt("d", 1234) == "1234"
@test pyfmt("n", 1234) == "1234"
@test pyfmt("x", 0x2ab) == "2ab"
@test pyfmt("X", 0x2ab) == "2AB"
@test pyfmt("o", 0o123) == "123"
@test pyfmt("b", 0b1101) == "1101"
@test pyfmt("d", 0) == "0"
@test pyfmt("d", 9) == "9"
@test pyfmt("d", 10) == "10"
@test pyfmt("d", 99) == "99"
@test pyfmt("d", 100) == "100"
@test pyfmt("d", 1000) == "1000"
@test pyfmt("06d", 123) == "000123"
@test pyfmt("+6d", 123) == " +123"
@test pyfmt("+06d", 123) == "+00123"
@test pyfmt(" d", 123) == " 123"
@test pyfmt(" 6d", 123) == " 123"
@test pyfmt("<6d", 123) == "123 "
@test pyfmt(">6d", 123) == " 123"
@test pyfmt("^6d", 123) == " 123 "
@test pyfmt("*<6d", 123) == "123***"
@test pyfmt("⋆<6d", 123) == "123⋆⋆⋆"
@test pyfmt("*^6d", 123) == "*123**"
@test pyfmt("⋆^6d", 123) == "⋆123⋆⋆"
@test pyfmt("*>6d", 123) == "***123"
@test pyfmt("⋆>6d", 123) == "⋆⋆⋆123"
@test pyfmt("< 6d", 123) == " 123 "
@test pyfmt("<+6d", 123) == "+123 "
@test pyfmt("^ 6d", 123) == " 123 "
@test pyfmt("^+6d", 123) == " +123 "
@test pyfmt("> 6d", 123) == " 123"
@test pyfmt(">+6d", 123) == " +123"
@test pyfmt("+d", -123) == "-123"
@test pyfmt("-d", -123) == "-123"
@test pyfmt(" d", -123) == "-123"
@test pyfmt("06d", -123) == "-00123"
@test pyfmt("<6d", -123) == "-123 "
@test pyfmt("^6d", -123) == " -123 "
@test pyfmt(">6d", -123) == " -123"
# Issue #110 (in Formatting.jl)
f = FormatExpr("{:+d}")
for T in (Int8, Int16, Int32, Int64, Int128)
@test format(f, typemin(T)) == string(typemin(T))
end
end
@testset "Python separators (, and _)" begin
# May need to change so that 'd' is a default, if none specified for integer value
@test pyfmt(",d", 1) == "1"
@test pyfmt(",d", 12) == "12"
@test pyfmt(",d", 123) == "123"
@test pyfmt(",d", 1234) == "1,234"
@test pyfmt(",d", 12345) == "12,345"
@test pyfmt(",d", 123456) == "123,456"
@test pyfmt(",d", 1234567) == "1,234,567"
@test pyfmt(",d", 12345678) == "12,345,678"
@test pyfmt(",d", 123456789) == "123,456,789"
@test pyfmt(",d", 1234567890) == "1,234,567,890"
@test pyfmt("_d", 1) == "1"
@test pyfmt("_d", 12) == "12"
@test pyfmt("_d", 123) == "123"
@test pyfmt("_d", 1234) == "1_234"
@test pyfmt("_d", 12345) == "12_345"
@test pyfmt("_d", 123456) == "123_456"
@test pyfmt("_d", 1234567) == "1_234_567"
@test pyfmt("_d", 12345678) == "12_345_678"
@test pyfmt("_d", 123456789) == "123_456_789"
@test pyfmt("_d", 1234567890) == "1_234_567_890"
@test pyfmt("_x", 0x1) == "1"
@test pyfmt("_x", 0x12) == "12"
@test pyfmt("_x", 0x123) == "123"
@test pyfmt("_x", 0x1234) == "1234"
@test pyfmt("_x", 0x12345) == "1_2345"
@test pyfmt("_x", 0x123456) == "12_3456"
@test pyfmt("_x", 0x1234567) == "123_4567"
@test pyfmt("_x", 0x12345678) == "1234_5678"
@test pyfmt("_x", 0x123456789) == "1_2345_6789"
@test pyfmt("_x", 0x123456789a) == "12_3456_789a"
@test pyfmt("_b", 0b1) == "1"
@test pyfmt("_b", 0b10) == "10"
@test pyfmt("_b", 0b101) == "101"
@test pyfmt("_b", 0b1010) == "1010"
@test pyfmt("_b", 0b10101) == "1_0101"
@test pyfmt("_b", 0b101010) == "10_1010"
@test pyfmt("_o", 0o1) == "1"
@test pyfmt("_o", 0o12) == "12"
@test pyfmt("_o", 0o123) == "123"
@test pyfmt("_o", 0o1234) == "1234"
@test pyfmt("_o", 0o12345) == "1_2345"
@test pyfmt("_o", 0o123456) == "12_3456"
@test pyfmt("15,d", 123456789) == " 123,456,789"
@test pyfmt("15,d", -123456789) == " -123,456,789"
@test pyfmt("06,d", 1234) == "01,234"
@test pyfmt("07,d", 1234) == "001,234"
# May need to change how separators are handled with zero padding,
# to produce the same results as Python
# @test pyfmt("08,d", 1234) == "0,001,234"
# @test pyfmt("09,d", 1234) == "0,001,234"
# @test pyfmt("010,d", 1234) == "00,001,234"
@test pyfmt("+#012_b", 42) == "+0b0010_1010"
# @test pyfmt("+#013_b", 42) == "+0b0_0010_1010"
# @test pyfmt("+#014_b", 42) == "+0b0_0010_1010"
end
@testset "Format floating point (f)" begin
@test pyfmt("", 0.125) == "0.125"
@test pyfmt("f", 0.0) == "0.000000"
@test pyfmt("f", -0.0) == "-0.000000"
@test pyfmt("f", 0.001) == "0.001000"
@test pyfmt("f", 0.125) == "0.125000"
@test pyfmt("f", 1.0/3) == "0.333333"
@test pyfmt("f", 1.0/6) == "0.166667"
@test pyfmt("f", -0.125) == "-0.125000"
@test pyfmt("f", -1.0/3) == "-0.333333"
@test pyfmt("f", -1.0/6) == "-0.166667"
@test pyfmt("f", 1234.5678) == "1234.567800"
@test pyfmt("8f", 1234.5678) == "1234.567800"
@test pyfmt("8.2f", 8.376) == " 8.38"
@test pyfmt("<8.2f", 8.376) == "8.38 "
@test pyfmt("^8.2f", 8.376) == " 8.38 "
@test pyfmt(">8.2f", 8.376) == " 8.38"
@test pyfmt("8.2f", -8.376) == " -8.38"
@test pyfmt("<8.2f", -8.376) == "-8.38 "
@test pyfmt("^8.2f", -8.376) == " -8.38 "
@test pyfmt(">8.2f", -8.376) == " -8.38"
@test pyfmt(".0f", 8.376) == "8"
# Note: these act differently than Python, but it looks like Python might be wrong
# in at least some of these cases (zero padding should be *after* the sign, IMO)
@test pyfmt("<08.2f", 8.376) == "00008.38"
@test pyfmt("^08.2f", 8.376) == "00008.38"
@test pyfmt(">08.2f", 8.376) == "00008.38"
@test pyfmt("<08.2f", -8.376) == "-0008.38"
@test pyfmt("^08.2f", -8.376) == "-0008.38"
@test pyfmt(">08.2f", -8.376) == "-0008.38"
@test pyfmt("*<8.2f", 8.376) == "8.38****"
@test pyfmt("⋆<8.2f", 8.376) == "8.38⋆⋆⋆⋆"
@test pyfmt("*^8.2f", 8.376) == "**8.38**"
@test pyfmt("⋆^8.2f", 8.376) == "⋆⋆8.38⋆⋆"
@test pyfmt("*>8.2f", 8.376) == "****8.38"
@test pyfmt("⋆>8.2f", 8.376) == "⋆⋆⋆⋆8.38"
@test pyfmt("*<8.2f", -8.376) == "-8.38***"
@test pyfmt("⋆<8.2f", -8.376) == "-8.38⋆⋆⋆"
@test pyfmt("*^8.2f", -8.376) == "*-8.38**"
@test pyfmt("⋆^8.2f", -8.376) == "⋆-8.38⋆⋆"
@test pyfmt("*>8.2f", -8.376) == "***-8.38"
@test pyfmt("⋆>8.2f", -8.376) == "⋆⋆⋆-8.38"
@test pyfmt(".2f", 0.999) == "1.00"
@test pyfmt(".2f", 0.996) == "1.00"
@test pyfmt("6.2f", 9.999) == " 10.00"
# Floating point error can upset this one (i.e. 0.99500000 or 0.994999999)
@test (pyfmt(".2f", 0.995) == "1.00" || pyfmt(".2f", 0.995) == "0.99")
@test pyfmt(".2f", 0.994) == "0.99"
# issue #102 (from Formatting.jl)
@test pyfmt("15.6f", 1e20) == "100000000000000000000.000000"
end
@testset "Format floating point (e)" begin
@test pyfmt("E", 0.0) == "0.000000E+00"
@test pyfmt("e", 0.0) == "0.000000e+00"
@test pyfmt("e", 0.001) == "1.000000e-03"
@test pyfmt("e", 0.125) == "1.250000e-01"
@test pyfmt("e", 100/3) == "3.333333e+01"
@test pyfmt("e", -0.125) == "-1.250000e-01"
@test pyfmt("e", -100/6) == "-1.666667e+01"
@test pyfmt("e", 1234.5678) == "1.234568e+03"
@test pyfmt("8e", 1234.5678) == "1.234568e+03"
@test pyfmt("<12.2e", 13.89) == "1.39e+01 "
@test pyfmt("^12.2e", 13.89) == " 1.39e+01 "
@test pyfmt(">12.2e", 13.89) == " 1.39e+01"
@test pyfmt("*<12.2e", 13.89) == "1.39e+01****"
@test pyfmt("⋆<12.2e", 13.89) == "1.39e+01⋆⋆⋆⋆"
@test pyfmt("*^12.2e", 13.89) == "**1.39e+01**"
@test pyfmt("⋆^12.2e", 13.89) == "⋆⋆1.39e+01⋆⋆"
@test pyfmt("*>12.2e", 13.89) == "****1.39e+01"
@test pyfmt("⋆>12.2e", 13.89) == "⋆⋆⋆⋆1.39e+01"
@test pyfmt("012.2e", 13.89) == "00001.39e+01"
@test pyfmt("012.2e", -13.89) == "-0001.39e+01"
@test pyfmt("+012.2e", 13.89) == "+0001.39e+01"
@test pyfmt(".1e", 0.999) == "1.0e+00"
@test pyfmt(".1e", 0.996) == "1.0e+00"
# Floating point error can upset this one (i.e. 0.99500000 or 0.994999999)
@test (pyfmt(".1e", 0.995) == "1.0e+00" || pyfmt(".1e", 0.995) == "9.9e-01")
@test pyfmt(".1e", 0.994) == "9.9e-01"
@test pyfmt(".1e", 0.6) == "6.0e-01"
@test pyfmt(".1e", 0.9) == "9.0e-01"
# issue #61 (from Formatting.jl)
@test pyfmt("1.0e", 1e-21) == "1e-21"
@test pyfmt("1.1e", 1e-21) == "1.0e-21"
@test pyfmt("10.2e", 1.2e100) == " 1.20e+100"
@test pyfmt("11.2e", BigFloat("1.2e1000")) == " 1.20e+1000"
@test pyfmt("11.2e", BigFloat("1.2e-1000")) == " 1.20e-1000"
@test pyfmt("9.2e", 9.999e9) == " 1.00e+10"
@test pyfmt("10.2e", 9.999e99) == " 1.00e+100"
@test pyfmt("11.2e", BigFloat("9.999e999")) == " 1.00e+1000"
@test pyfmt("10.2e", -9.999e-100) == " -1.00e-99"
# issue #84 (from Formatting.jl)
@test pyfmt("+11.3e", 1.0e-309) == "+1.000e-309"
@test pyfmt("+11.3e", 1.0e-313) == "+1.000e-313"
# issue #108 (from Formatting.jl)
@test pyfmt(".1e", 0.0003) == "3.0e-04"
@test pyfmt(".1e", 0.0006) == "6.0e-04"
end
@testset "Format percentage (%)" begin
@test pyfmt("8.2%", 0.123456) == " 12.35%"
@test pyfmt("<8.2%", 0.123456) == "12.35% "
@test pyfmt("^8.2%", 0.123456) == " 12.35% "
@test pyfmt(">8.2%", 0.123456) == " 12.35%"
end
@testset "Format special floating point value" begin
@test pyfmt("f", NaN) == "NaN"
@test pyfmt("e", NaN) == "NaN"
@test pyfmt("f", NaN32) == "NaN"
@test pyfmt("e", NaN32) == "NaN"
@test pyfmt("f", Inf) == "Inf"
@test pyfmt("e", Inf) == "Inf"
@test pyfmt("f", Inf32) == "Inf"
@test pyfmt("e", Inf32) == "Inf"
@test pyfmt("f", -Inf) == "-Inf"
@test pyfmt("e", -Inf) == "-Inf"
@test pyfmt("f", -Inf32) == "-Inf"
@test pyfmt("e", -Inf32) == "-Inf"
@test pyfmt("<5f", Inf) == "Inf "
@test pyfmt("^5f", Inf) == " Inf "
@test pyfmt(">5f", Inf) == " Inf"
@test pyfmt("*<5f", Inf) == "Inf**"
@test pyfmt("⋆<5f", Inf) == "Inf⋆⋆"
@test pyfmt("*^5f", Inf) == "*Inf*"
@test pyfmt("⋆^5f", Inf) == "⋆Inf⋆"
@test pyfmt("*>5f", Inf) == "**Inf"
@test pyfmt("⋆>5f", Inf) == "⋆⋆Inf"
end
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 2623 | @testset "format with positional arguments" begin
@test format("{1}", 10) == "10"
@test format("αβγ {1}", 10) == "αβγ 10"
@test format("{1} efg", 10) == "10 efg"
@test format("{1} ϵζη", 10) == "10 ϵζη"
@test format("abc {1} efg", 10) == "abc 10 efg"
@test format("αβγ{1}ϵζη", 10) == "αβγ10ϵζη"
@test format("{1} + {2}", 10, "xyz") == "10 + xyz"
@test format("{1} + {2}", 10, "χψω") == "10 + χψω"
@test format("abc {1} + {2}", 10, "xyz") == "abc 10 + xyz"
@test format("αβγ {1} + {2}", 10, "χψω") == "αβγ 10 + χψω"
@test format("{1} + {2} efg", 10, "xyz") == "10 + xyz efg"
@test format("{1} + {2} ϵζη", 10, "χψω") == "10 + χψω ϵζη"
@test format("abc {1} + {2} efg", 10, "xyz") == "abc 10 + xyz efg"
@test format("αβγ {1} + {2} ϵζη", 10, "χψω") == "αβγ 10 + χψω ϵζη"
@test format("abc {1}{2} efg", 10, "xyz") == "abc 10xyz efg"
@test format("αβγ {1}{2} ϵζη", 10, "χψω") == "αβγ 10χψω ϵζη"
@test format("{1:d} + {2:s}", 10, "xyz") == "10 + xyz"
@test format("{1:d} + {2:s}", 10, "χψω") == "10 + χψω"
@test format("{1:04d} + {2:*>5}", 10, "xyz") == "0010 + **xyz"
@test format("{1:04d} + {2:⋆>5}", 10, "χψω") == "0010 + ⋆⋆χψω"
@test format("let {2:<5} := {1:.4f};", 12.3, "var") == "let var := 12.3000;"
@test format("let {2:<5} := {1:.4f};", 12.3, "χψω") == "let χψω := 12.3000;"
@test format("{}", 10) == "10"
@test format("{} + {}", 10, 20) == "10 + 20"
@test format("{} + {:04d}", 10, 20) == "10 + 0020"
@test format("{:03d} + {}", 10, 20) == "010 + 20"
@test format("{:03d} + {:04d}", 10, 20) == "010 + 0020"
@test_throws(ErrorException, format("{1} + {}", 10, 20) )
@test_throws(ErrorException, format("{} + {1}", 10, 20) )
@test_throws(ErrorException, format("{1", 10) )
f = FormatExpr("{1:s}")
printfmt(f,"")
printfmtln(f,"")
io = IOBuffer()
printfmt(io,f,"foobar")
@test io.size == 6
printfmtln(io,f,"ugly")
@test io.size == 11
end
@testset "format escape {{ and }}" begin
@test format("{{}}") == "{}"
@test format("{{{1}}}", 10) == "{10}"
@test format("v: {{{2}}} = {1:.4f}", 1.2, "ab") == "v: {ab} = 1.2000"
@test format("χ: {{{2}}} = {1:.4f}", 1.2, "αβ") == "χ: {αβ} = 1.2000"
end
@testset "format with filter" begin
@test format("{1|>abs2} + {2|>abs2:.2f}", 2, 3) == "4 + 9.00"
end
@testset "test typemin/typemax edge cases" begin
f = FormatExpr("{1:+d}")
for T in (Int8,Int16,Int32,Int64)
@test format(f, typemin(T)) == string(typemin(T))
@test format(f, typemax(T)) == "+"*string(typemax(T))
end
end
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 491 | # performance testing
using Format
# performance of format parsing
fp1() = FormatExpr("{1} + {2} + {3}")
fp1()
@time for i=1:10000; fp1(); end
fp2() = FormatExpr("abc {1:*<5d} efg {2:*>8.4e} hij")
fp2()
@time for i=1:10000; fp2(); end
# performance of string formatting
const fe1 = fp1()
const fe2 = fp2()
sf1(x, y, z) = format(fe1, x, y, z)
sf1(10, 20, 30)
@time for i=1:10000; sf1(10, 20, 30); end
sf2(x, y) = format(fe2, x, y)
sf2(10, 20)
@time for i=1:10000; sf2(10, 20); end
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 31616 | # Based on stdlib Printf.jl runtests.jl
using Format
using Test
@testset "cfmt" begin
@testset "%p" begin
# pointers
if Sys.WORD_SIZE == 64
@test cfmt("%20p", 0) == " 0x0000000000000000"
@test cfmt("%-20p", 0) == "0x0000000000000000 "
@test cfmt("%20p", C_NULL) == " 0x0000000000000000"
@test cfmt("%-20p", C_NULL) == "0x0000000000000000 "
elseif Sys.WORD_SIZE == 32
@test cfmt("%20p", 0) == " 0x00000000"
@test cfmt("%-20p", 0) == "0x00000000 "
@test cfmt("%20p", C_NULL) == " 0x00000000"
@test cfmt("%-20p", C_NULL) == "0x00000000 "
end
# Printf.jl #40318
@test cfmt("%p", 0xfffffffffffe0000) == "0xfffffffffffe0000"
end
@testset "%a" begin
# hex float
@test cfmt("%a", 0.0) == "0x0p+0"
@test cfmt("%a", -0.0) == "-0x0p+0"
@test cfmt("%.3a", 0.0) == "0x0.000p+0"
@test cfmt("%a", 1.5) == "0x1.8p+0"
@test cfmt("%a", 1.5f0) == "0x1.8p+0"
@test cfmt("%a", big"1.5") == "0x1.8p+0"
@test cfmt("%#.0a", 1.5) == "0x2.p+0"
@test cfmt("%+30a", 1/3) == " +0x1.5555555555555p-2"
@test cfmt("%a", 1.5) == "0x1.8p+0"
@test cfmt("%a", 3.14) == "0x1.91eb851eb851fp+1"
@test cfmt("%.0a", 3.14) == "0x2p+1"
@test cfmt("%.1a", 3.14) == "0x1.9p+1"
@test cfmt("%.2a", 3.14) == "0x1.92p+1"
@test cfmt("%#a", 3.14) == "0x1.91eb851eb851fp+1"
@test cfmt("%#.0a", 3.14) == "0x2.p+1"
@test cfmt("%#.1a", 3.14) == "0x1.9p+1"
@test cfmt("%#.2a", 3.14) == "0x1.92p+1"
@test cfmt("%.6a", 1.5) == "0x1.800000p+0"
end
@testset "%g" begin
# %g
for (val, res) in ((12345678., "1.23457e+07"),
(1234567.8, "1.23457e+06"),
(123456.78, "123457"),
(12345.678, "12345.7"),
(12340000.0, "1.234e+07"))
@test cfmt("%.6g", val) == res
end
for (val, res) in ((big"12345678.", "1.23457e+07"),
(big"1234567.8", "1.23457e+06"),
(big"123456.78", "123457"),
(big"12345.678", "12345.7"))
@test cfmt("%.6g", val) == res
end
for (fmt, val) in (("%10.5g", " 123.4"),
("%+10.5g", " +123.4"),
("% 10.5g", " 123.4"),
("%#10.5g", " 123.40"),
("%-10.5g", "123.4 "),
("%-+10.5g", "+123.4 "),
("%010.5g", "00000123.4")),
num in (123.4, big"123.4")
@test cfmt(Format.FmtSpec(fmt), num) == val
end
@test cfmt( "%10.5g", -123.4 ) == " -123.4"
@test cfmt( "%010.5g", -123.4 ) == "-0000123.4"
@test cfmt( "%.6g", 12340000.0 ) == "1.234e+07"
@test cfmt( "%#.6g", 12340000.0 ) == "1.23400e+07"
@test cfmt( "%10.5g", big"-123.4" ) == " -123.4"
@test cfmt( "%010.5g", big"-123.4" ) == "-0000123.4"
@test cfmt( "%.6g", big"12340000.0" ) == "1.234e+07"
@test cfmt( "%#.6g", big"12340000.0") == "1.23400e+07"
# %g regression gh #14331
@test cfmt( "%.5g", 42) == "42"
@test cfmt( "%#.2g", 42) == "42."
@test cfmt( "%#.5g", 42) == "42.000"
@test cfmt("%g", 0.00012) == "0.00012"
@test cfmt("%g", 0.000012) == "1.2e-05"
@test cfmt("%g", 123456.7) == "123457"
@test cfmt("%g", 1234567.8) == "1.23457e+06"
# %g regression gh #41631
for (val, res) in ((Inf, "Inf"),
(-Inf, "-Inf"),
(NaN, "NaN"),
(-NaN, "NaN"))
@test cfmt("%g", val) == res
@test cfmt("%G", val) == res
end
# zeros
@test cfmt("%.15g", 0) == "0"
@test cfmt("%#.15g", 0) == "0.00000000000000"
end
@testset "%f" begin
# Inf / NaN handling
@test cfmt("%f", Inf) == "Inf"
@test cfmt("%+f", Inf) == "+Inf"
@test cfmt("% f", Inf) == " Inf"
@test cfmt("% #f", Inf) == " Inf"
@test cfmt("%07f", Inf) == " Inf"
@test cfmt("%f", -Inf) == "-Inf"
@test cfmt("%+f", -Inf) == "-Inf"
@test cfmt("%07f", -Inf) == " -Inf"
@test cfmt("%f", NaN) == "NaN"
@test cfmt("%+f", NaN) == "+NaN"
@test cfmt("% f", NaN) == " NaN"
@test cfmt("% #f", NaN) == " NaN"
@test cfmt("%07f", NaN) == " NaN"
@test cfmt("%e", big"Inf") == "Inf"
@test cfmt("%e", big"NaN") == "NaN"
@test cfmt("%.0f", 3e142) == "29999999999999997463140672961703247153805615792184250659629251954072073858354858644285983761764971823910371920726635399393477049701891710124032"
@test cfmt("%f", 1.234) == "1.234000"
@test cfmt("%F", 1.234) == "1.234000"
@test cfmt("%+f", 1.234) == "+1.234000"
@test cfmt("% f", 1.234) == " 1.234000"
@test cfmt("%f", -1.234) == "-1.234000"
@test cfmt("%+f", -1.234) == "-1.234000"
@test cfmt("% f", -1.234) == "-1.234000"
@test cfmt("%#f", 1.234) == "1.234000"
@test cfmt("%.2f", 1.234) == "1.23"
@test cfmt("%.2f", 1.235) == "1.24"
@test cfmt("%.2f", 0.235) == "0.23"
@test cfmt("%4.1f", 1.234) == " 1.2"
@test cfmt("%8.1f", 1.234) == " 1.2"
@test cfmt("%+8.1f", 1.234) == " +1.2"
@test cfmt("% 8.1f", 1.234) == " 1.2"
@test cfmt("% 7.1f", 1.234) == " 1.2"
@test cfmt("% 08.1f", 1.234) == " 00001.2"
@test cfmt("%08.1f", 1.234) == "000001.2"
@test cfmt("%-08.1f", 1.234) == "1.2 "
@test cfmt("%-8.1f", 1.234) == "1.2 "
@test cfmt("%08.1f", -1.234) == "-00001.2"
@test cfmt("%09.1f", -1.234) == "-000001.2"
@test cfmt("%09.1f", 1.234) == "0000001.2"
@test cfmt("%+09.1f", 1.234) == "+000001.2"
@test cfmt("% 09.1f", 1.234) == " 000001.2"
@test cfmt("%+ 09.1f", 1.234) == "+000001.2"
@test cfmt("%+ 09.1f", 1.234) == "+000001.2"
@test cfmt("%+ 09.0f", 1.234) == "+00000001"
@test cfmt("%+ #09.0f", 1.234) == "+0000001."
# Printf.jl issue #40303
@test cfmt("%+7.1f", 9.96) == " +10.0"
@test cfmt("% 7.1f", 9.96) == " 10.0"
end
@testset "%e" begin
# Inf / NaN handling
@test cfmt("%e", Inf) == "Inf"
@test cfmt("%+e", Inf) == "+Inf"
@test cfmt("% e", Inf) == " Inf"
@test cfmt("% #e", Inf) == " Inf"
@test cfmt("%07e", Inf) == " Inf"
@test cfmt("%e", -Inf) == "-Inf"
@test cfmt("%+e", -Inf) == "-Inf"
@test cfmt("%07e", -Inf) == " -Inf"
@test cfmt("%e", NaN) == "NaN"
@test cfmt("%+e", NaN) == "+NaN"
@test cfmt("% e", NaN) == " NaN"
@test cfmt("% #e", NaN) == " NaN"
@test cfmt("%07e", NaN) == " NaN"
@test cfmt("%e", big"Inf") == "Inf"
@test cfmt("%e", big"NaN") == "NaN"
# scientific notation
@test cfmt("%.0e", 3e142) == "3e+142"
@test cfmt("%#.0e", 3e142) == "3.e+142"
@test cfmt("%.0e", big"3e142") == "3e+142"
@test cfmt("%#.0e", big"3e142") == "3.e+142"
@test cfmt("%.0e", big"3e1042") == "3e+1042"
@test cfmt("%e", 3e42) == "3.000000e+42"
@test cfmt("%E", 3e42) == "3.000000E+42"
@test cfmt("%e", 3e-42) == "3.000000e-42"
@test cfmt("%E", 3e-42) == "3.000000E-42"
@test cfmt("%e", 1.234) == "1.234000e+00"
@test cfmt("%E", 1.234) == "1.234000E+00"
@test cfmt("%+e", 1.234) == "+1.234000e+00"
@test cfmt("% e", 1.234) == " 1.234000e+00"
@test cfmt("%e", -1.234) == "-1.234000e+00"
@test cfmt("%+e", -1.234) == "-1.234000e+00"
@test cfmt("% e", -1.234) == "-1.234000e+00"
@test cfmt("%#e", 1.234) == "1.234000e+00"
@test cfmt("%.2e", 1.234) == "1.23e+00"
@test cfmt("%.2e", 1.235) == "1.24e+00"
@test cfmt("%.2e", 0.235) == "2.35e-01"
@test cfmt("%4.1e", 1.234) == "1.2e+00"
@test cfmt("%8.1e", 1.234) == " 1.2e+00"
@test cfmt("%+8.1e", 1.234) == "+1.2e+00"
@test cfmt("% 8.1e", 1.234) == " 1.2e+00"
@test cfmt("% 7.1e", 1.234) == " 1.2e+00"
@test cfmt("% 08.1e", 1.234) == " 1.2e+00"
@test cfmt("%08.1e", 1.234) == "01.2e+00"
@test cfmt("%-08.1e", 1.234) == "1.2e+00 "
@test cfmt("%-8.1e", 1.234) == "1.2e+00 "
@test cfmt("%-8.1e", 1.234) == "1.2e+00 "
@test cfmt("%08.1e", -1.234) == "-1.2e+00"
@test cfmt("%09.1e", -1.234) == "-01.2e+00"
@test cfmt("%09.1e", 1.234) == "001.2e+00"
@test cfmt("%+09.1e", 1.234) == "+01.2e+00"
@test cfmt("% 09.1e", 1.234) == " 01.2e+00"
@test cfmt("%+ 09.1e", 1.234) == "+01.2e+00"
@test cfmt("%+ 09.1e", 1.234) == "+01.2e+00"
@test cfmt("%+ 09.0e", 1.234) == "+0001e+00"
@test cfmt("%+ #09.0e", 1.234) == "+001.e+00"
# Printf.jl #40303
@test cfmt("%+9.1e", 9.96) == " +1.0e+01"
@test cfmt("% 9.1e", 9.96) == " 1.0e+01"
end
@testset "strings" begin
@test cfmt("%.1s", "foo") == "f"
@test cfmt("%s", "%%%%") == "%%%%"
@test cfmt("%s", "Hallo heimur") == "Hallo heimur"
@test cfmt("%+s", "Hallo heimur") == "Hallo heimur"
@test cfmt("% s", "Hallo heimur") == "Hallo heimur"
@test cfmt("%+ s", "Hallo heimur") == "Hallo heimur"
@test cfmt("%1s", "Hallo heimur") == "Hallo heimur"
@test cfmt("%20s", "Hallo") == " Hallo"
@test cfmt("%-20s", "Hallo") == "Hallo "
@test cfmt("%0-20s", "Hallo") == "Hallo "
@test cfmt("%.20s", "Hallo heimur") == "Hallo heimur"
@test cfmt("%20.5s", "Hallo heimur") == " Hallo"
@test cfmt("%.0s", "Hallo heimur") == ""
@test cfmt("%20.0s", "Hallo heimur") == " "
@test cfmt("%.s", "Hallo heimur") == ""
@test cfmt("%20.s", "Hallo heimur") == " "
@test cfmt("%s", "test") == "test"
@test cfmt("%s", "tést") == "tést"
@test cfmt("%8s", "test") == " test"
@test cfmt("%-8s", "test") == "test "
@test cfmt("%s", :test) == "test"
@test cfmt("%#s", :test) == ":test"
@test cfmt("%#8s", :test) == " :test"
@test cfmt("%#-8s", :test) == ":test "
@test cfmt("%8.3s", "test") == " tes"
@test cfmt("%#8.3s", "test") == " \"te"
@test cfmt("%-8.3s", "test") == "tes "
@test cfmt("%#-8.3s", "test") == "\"te "
@test cfmt("%.3s", "test") == "tes"
@test cfmt("%#.3s", "test") == "\"te"
@test cfmt("%-.3s", "test") == "tes"
@test cfmt("%#-.3s", "test") == "\"te"
# Printf.jl issue #41068
@test cfmt("%.2s", "föó") == "fö"
@test cfmt("%5s", "föó") == " föó"
@test cfmt("%6s", "😍🍕") == " 😍🍕"
@test cfmt("%2c", '🍕') == "🍕"
@test cfmt("%3c", '🍕') == " 🍕"
end
@testset "chars" begin
@test cfmt("%c", 'a') == "a"
@test cfmt("%c", 32) == " "
@test cfmt("%c", 36) == "\$"
@test cfmt("%3c", 'a') == " a"
@test cfmt( "%c", 'x') == "x"
@test cfmt("%+c", 'x') == "x"
@test cfmt("% c", 'x') == "x"
@test cfmt("%+ c", 'x') == "x"
@test cfmt("%1c", 'x') == "x"
@test cfmt("%20c", 'x') == " x"
@test cfmt("%-20c", 'x') == "x "
@test cfmt("%-020c", 'x') == "x "
@test cfmt("%c", 65) == "A"
@test cfmt("%c", 'A') == "A"
@test cfmt("%3c", 'A') == " A"
@test cfmt("%-3c", 'A') == "A "
@test cfmt("%c", 248) == "ø"
@test cfmt("%c", 'ø') == "ø"
@test cfmt("%c", "ø") == "ø"
@test cfmt("%c", '𐀀') == "𐀀"
end
function _test_flags(val, vflag::AbstractString, fmt::AbstractString, res::AbstractString, prefix::AbstractString)
vflag = string("%", vflag)
space_fmt = string(length(res) + length(prefix) + 3, fmt)
fsign = string((val < 0 ? "-" : "+"), prefix)
nsign = string((val < 0 ? "-" : " "), prefix)
osign = val < 0 ? string("-", prefix) : string(prefix, "0")
esign = string(val < 0 ? "-" : "", prefix)
esignend = val < 0 ? "" : " "
for (flag::AbstractString, ans::AbstractString) in (
("", string(" ", nsign, res)),
("+", string(" ", fsign, res)),
(" ", string(" ", nsign, res)),
("0", string(osign, "00", res)),
("-", string(esign, res, " ", esignend)),
("0+", string(fsign, "00", res)),
("0 ", string(nsign, "00", res)),
("-+", string(fsign, res, " ")),
("- ", string(nsign, res, " ")),
)
fmt_string = string(vflag, flag, space_fmt)
@test cfmt(fmt_string, val) == ans
end
end
@testset "basics" begin
@test cfmt("%10.5d", 4) == " 00004"
@test cfmt("%d", typemax(Int64)) == "9223372036854775807"
for (fmt, val) in (("%7.2f", " 1.23"),
("%-7.2f", "1.23 "),
("%07.2f", "0001.23"),
("%.0f", "1"),
("%#.0f", "1."),
("%.4e", "1.2345e+00"),
("%.4E", "1.2345E+00"),
("%.2a", "0x1.3cp+0"),
("%.2A", "0X1.3CP+0")),
num in (1.2345, big"1.2345")
@test cfmt(Format.FmtSpec(fmt), num) == val
end
for (fmt, val) in (("%i", "42"),
("%u", "42"),
#("Test: %i", "Test: 42"),
("%#x", "0x2a"),
("%x", "2a"),
("%X", "2A"),
("% i", " 42"),
("%+i", "+42"),
("%4i", " 42"),
("%-4i", "42 "),
("%f", "42.000000"),
("%g", "42"),
("%e", "4.200000e+01")),
num in (UInt16(42), UInt32(42), UInt64(42), UInt128(42),
Int16(42), Int32(42), Int64(42), Int128(42)) #, big"42") #Fix!
@test cfmt(Format.FmtSpec(fmt), num) == val
end
for i in (
(42, "", "i", "42", ""),
(42, "", "d", "42", ""),
(42, "", "u", "42", ""),
(42, "", "x", "2a", ""),
(42, "", "X", "2A", ""),
(42, "", "o", "52", ""),
(42, "#", "x", "2a", "0x"),
(42, "#", "X", "2A", "0X"),
(42, "#", "o", "052", ""),
(1.2345, "", ".2f", "1.23", ""),
(1.2345, "", ".2e", "1.23e+00", ""),
(1.2345, "", ".2E", "1.23E+00", ""),
(1.2345, "#", ".0f", "1.", ""),
(1.2345, "#", ".0e", "1.e+00", ""),
(1.2345, "#", ".0E", "1.E+00", ""),
(1.2345, "", ".2a", "1.3cp+0", "0x"),
(1.2345, "", ".2A", "1.3CP+0", "0X"),
)
_test_flags(i...)
_test_flags(-i[1], i[2:5]...)
end
# Check bug with trailing nul printing BigFloat
@test cfmt("%.330f", BigFloat(1))[end] != '\0'
# Check bugs with truncated output printing BigFloat
@test cfmt("%f", parse(BigFloat, "1e400")) ==
"10000000000000000000000000000000000000000000000000000000000000000000000000000025262527574416492004687051900140830217136998040684679611623086405387447100385714565637522507383770691831689647535911648520404034824470543643098638520633064715221151920028135130764414460468236314621044034960475540018328999334468948008954289495190631358190153259681118693204411689043999084305348398480210026863210192871358464.000000"
# Check that Printf does not attempt to output more than 8KiB worth of digits
#@test_throws ArgumentError cfmt("%f", parse(BigFloat, "1e99999"))
# Check bug with precision > length of string
@test cfmt("%4.2s", "a") == " a"
# Printf.jl issue #29662
@test cfmt("%12.3e", pi*1e100) == " 3.142e+100"
#@test string(Printf.Format("%a").formats[1]) == "%a"
#@test string(Printf.Format("%a").formats[1]; modifier="R") == "%Ra"
@test cfmt("%d", 3.14) == "3"
@test cfmt("%2d", 3.14) == " 3"
@test_broken cfmt("%2d", big(3.14)) == " 3"
@test cfmt("%s", 1) == "1"
@test cfmt("%f", 1) == "1.000000"
@test cfmt("%e", 1) == "1.000000e+00"
@test cfmt("%g", 1) == "1"
# Printf.jl issue #39748
@test cfmt("%.16g", 194.4778127560983) == "194.4778127560983"
@test cfmt("%.17g", 194.4778127560983) == "194.4778127560983"
@test cfmt("%.18g", 194.4778127560983) == "194.477812756098302"
@test cfmt("%.1g", 1.7976931348623157e308) == "2e+308"
@test cfmt("%.2g", 1.7976931348623157e308) == "1.8e+308"
@test cfmt("%.3g", 1.7976931348623157e308) == "1.8e+308"
# print float as %d uses round(x)
@test cfmt("%d", 25.5) == "26"
# Printf.jl issue #37539
#@test @sprintf(" %.1e\n", 0.999) == " 1.0e+00\n"
#@test @sprintf(" %.1f", 9.999) == " 10.0"
# Printf.jl issue #37552
@test cfmt("%d", 1.0e100) == "10000000000000000159028911097599180468360808563945281389781327557747838772170381060813469985856815104"
@test_broken cfmt("%d", 3//1) == "3"
@test cfmt("%d", Inf) == "Inf"
end
@testset "integers" begin
@test cfmt("% d", 42) == " 42"
@test cfmt("% d", -42) == "-42"
@test cfmt("% 5d", 42) == " 42"
@test cfmt("% 5d", -42) == " -42"
@test cfmt("% 15d", 42) == " 42"
@test cfmt("% 15d", -42) == " -42"
@test cfmt("%+d", 42) == "+42"
@test cfmt("%+d", -42) == "-42"
@test cfmt("%+5d", 42) == " +42"
@test cfmt("%+5d", -42) == " -42"
@test cfmt("%+15d", 42) == " +42"
@test cfmt("%+15d", -42) == " -42"
@test cfmt("%0d", 42) == "42"
@test cfmt("%0d", -42) == "-42"
@test cfmt("%05d", 42) == "00042"
@test cfmt("%05d", -42) == "-0042"
@test cfmt("%015d", 42) == "000000000000042"
@test cfmt("%015d", -42) == "-00000000000042"
@test cfmt("%-d", 42) == "42"
@test cfmt("%-d", -42) == "-42"
@test cfmt("%-5d", 42) == "42 "
@test cfmt("%-5d", -42) == "-42 "
@test cfmt("%-15d", 42) == "42 "
@test cfmt("%-15d", -42) == "-42 "
@test cfmt("%-0d", 42) == "42"
@test cfmt("%-0d", -42) == "-42"
@test cfmt("%-05d", 42) == "42 "
@test cfmt("%-05d", -42) == "-42 "
@test cfmt("%-015d", 42) == "42 "
@test cfmt("%-015d", -42) == "-42 "
@test cfmt("%0-d", 42) == "42"
@test cfmt("%0-d", -42) == "-42"
@test cfmt("%0-5d", 42) == "42 "
@test cfmt("%0-5d", -42) == "-42 "
@test cfmt("%0-15d", 42) == "42 "
@test cfmt("%0-15d", -42) == "-42 "
@test cfmt("%lld", 18446744065119617025) == "18446744065119617025"
@test cfmt("%+8lld", 100) == " +100"
@test cfmt("%+.8lld", 100) == "+00000100"
@test cfmt("%+10.8lld", 100) == " +00000100"
#@test_throws Printf.InvalidFormatStringError Printf.Format("%_1lld")
@test cfmt("%-1.5lld", -100) == "-00100"
@test cfmt("%5lld", 100) == " 100"
@test cfmt("%5lld", -100) == " -100"
@test cfmt("%-5lld", 100) == "100 "
@test cfmt("%-5lld", -100) == "-100 "
@test cfmt("%-.5lld", 100) == "00100"
@test cfmt("%-.5lld", -100) == "-00100"
@test cfmt("%-8.5lld", 100) == "00100 "
@test cfmt("%-8.5lld", -100) == "-00100 "
@test cfmt("%05lld", 100) == "00100"
@test cfmt("%05lld", -100) == "-0100"
@test cfmt("% lld", 100) == " 100"
@test cfmt("% lld", -100) == "-100"
@test cfmt("% 5lld", 100) == " 100"
@test cfmt("% 5lld", -100) == " -100"
@test cfmt("% .5lld", 100) == " 00100"
@test cfmt("% .5lld", -100) == "-00100"
@test cfmt("% 8.5lld", 100) == " 00100"
@test cfmt("% 8.5lld", -100) == " -00100"
@test cfmt("%.0lld", 0) == "0"
@test cfmt("%#+21.18llx", -100) == "-0x000000000000000064"
@test cfmt("%#.25llo", -100) == "-00000000000000000000000144"
@test cfmt("%#+24.20llo", -100) == " -000000000000000000144"
@test cfmt("%#+18.21llX", -100) == "-0X000000000000000000064"
@test cfmt("%#+20.24llo", -100) == "-0000000000000000000000144"
@test cfmt("%#+25.22llu", -1) == " -0000000000000000000001"
@test cfmt("%#+25.22llu", -1) == " -0000000000000000000001"
@test cfmt("%#+30.25llu", -1) == " -0000000000000000000000001"
@test cfmt("%+#25.22lld", -1) == " -0000000000000000000001"
@test cfmt("%#-8.5llo", 100) == "000144 "
@test cfmt("%#-+ 08.5lld", 100) == "+00100 "
@test cfmt("%#-+ 08.5lld", 100) == "+00100 "
@test cfmt("%.40lld", 1) == "0000000000000000000000000000000000000001"
@test cfmt("% .40lld", 1) == " 0000000000000000000000000000000000000001"
@test cfmt("% .40d", 1) == " 0000000000000000000000000000000000000001"
@test cfmt("%lld", 18446744065119617025) == "18446744065119617025"
@test cfmt("%#012x", 1) == "0x0000000001"
@test cfmt("%#04.8x", 1) == "0x00000001"
@test cfmt("%#-08.2x", 1) == "0x01 "
@test cfmt("%#08o", 1) == "00000001"
@test cfmt("%d", 1024) == "1024"
@test cfmt("%d", -1024) == "-1024"
@test cfmt("%i", 1024) == "1024"
@test cfmt("%i", -1024) == "-1024"
@test cfmt("%u", 1024) == "1024"
@test cfmt("%u", UInt(4294966272)) == "4294966272"
@test cfmt("%o", 511) == "777"
@test cfmt("%o", UInt(4294966785)) == "37777777001"
@test cfmt("%x", 305441741) == "1234abcd"
@test cfmt("%x", UInt(3989525555)) == "edcb5433"
@test cfmt("%X", 305441741) == "1234ABCD"
@test cfmt("%X", UInt(3989525555)) == "EDCB5433"
@test cfmt("%+d", 1024) == "+1024"
@test cfmt("%+d", -1024) == "-1024"
@test cfmt("%+i", 1024) == "+1024"
@test cfmt("%+i", -1024) == "-1024"
@test cfmt("%+u", 1024) == "+1024"
@test cfmt("%+u", UInt(4294966272)) == "+4294966272"
@test cfmt("%+o", 511) == "+777"
@test cfmt("%+o", UInt(4294966785)) == "+37777777001"
@test cfmt("%+x", 305441741) == "+1234abcd"
@test cfmt("%+x", UInt(3989525555)) == "+edcb5433"
@test cfmt("%+X", 305441741) == "+1234ABCD"
@test cfmt("%+X", UInt(3989525555)) == "+EDCB5433"
@test cfmt("% d", 1024) == " 1024"
@test cfmt("% d", -1024) == "-1024"
@test cfmt("% i", 1024) == " 1024"
@test cfmt("% i", -1024) == "-1024"
@test cfmt("% u", 1024) == " 1024"
@test cfmt("% u", UInt(4294966272)) == " 4294966272"
@test cfmt("% o", 511) == " 777"
@test cfmt("% o", UInt(4294966785)) == " 37777777001"
@test cfmt("% x", 305441741) == " 1234abcd"
@test cfmt("% x", UInt(3989525555)) == " edcb5433"
@test cfmt("% X", 305441741) == " 1234ABCD"
@test cfmt("% X", UInt(3989525555)) == " EDCB5433"
@test cfmt("%+ d", 1024) == "+1024"
@test cfmt("%+ d", -1024) == "-1024"
@test cfmt("%+ i", 1024) == "+1024"
@test cfmt("%+ i", -1024) == "-1024"
@test cfmt("%+ u", 1024) == "+1024"
@test cfmt("%+ u", UInt(4294966272)) == "+4294966272"
@test cfmt("%+ o", 511) == "+777"
@test cfmt("%+ o", UInt(4294966785)) == "+37777777001"
@test cfmt("%+ x", 305441741) == "+1234abcd"
@test cfmt("%+ x", UInt(3989525555)) == "+edcb5433"
@test cfmt("%+ X", 305441741) == "+1234ABCD"
@test cfmt("%+ X", UInt(3989525555)) == "+EDCB5433"
@test cfmt("%#o", 511) == "0777"
@test cfmt("%#o", UInt(4294966785)) == "037777777001"
@test cfmt("%#x", 305441741) == "0x1234abcd"
@test cfmt("%#x", UInt(3989525555)) == "0xedcb5433"
@test cfmt("%#X", 305441741) == "0X1234ABCD"
@test cfmt("%#X", UInt(3989525555)) == "0XEDCB5433"
@test cfmt("%#o", UInt(0)) == "00"
@test cfmt("%#x", UInt(0)) == "0x0"
@test cfmt("%#X", UInt(0)) == "0X0"
@test cfmt("%1d", 1024) == "1024"
@test cfmt("%1d", -1024) == "-1024"
@test cfmt("%1i", 1024) == "1024"
@test cfmt("%1i", -1024) == "-1024"
@test cfmt("%1u", 1024) == "1024"
@test cfmt("%1u", UInt(4294966272)) == "4294966272"
@test cfmt("%1o", 511) == "777"
@test cfmt("%1o", UInt(4294966785)) == "37777777001"
@test cfmt("%1x", 305441741) == "1234abcd"
@test cfmt("%1x", UInt(3989525555)) == "edcb5433"
@test cfmt("%1X", 305441741) == "1234ABCD"
@test cfmt("%1X", UInt(3989525555)) == "EDCB5433"
@test cfmt("%20d", 1024) == " 1024"
@test cfmt("%20d", -1024) == " -1024"
@test cfmt("%20i", 1024) == " 1024"
@test cfmt("%20i", -1024) == " -1024"
@test cfmt("%20u", 1024) == " 1024"
@test cfmt("%20u", UInt(4294966272)) == " 4294966272"
@test cfmt("%20o", 511) == " 777"
@test cfmt("%20o", UInt(4294966785)) == " 37777777001"
@test cfmt("%20x", 305441741) == " 1234abcd"
@test cfmt("%20x", UInt(3989525555)) == " edcb5433"
@test cfmt("%20X", 305441741) == " 1234ABCD"
@test cfmt("%20X", UInt(3989525555)) == " EDCB5433"
@test cfmt("%-20d", 1024) == "1024 "
@test cfmt("%-20d", -1024) == "-1024 "
@test cfmt("%-20i", 1024) == "1024 "
@test cfmt("%-20i", -1024) == "-1024 "
@test cfmt("%-20u", 1024) == "1024 "
@test cfmt("%-20u", UInt(4294966272)) == "4294966272 "
@test cfmt("%-20o", 511) == "777 "
@test cfmt("%-20o", UInt(4294966785)) == "37777777001 "
@test cfmt("%-20x", 305441741) == "1234abcd "
@test cfmt("%-20x", UInt(3989525555)) == "edcb5433 "
@test cfmt("%-20X", 305441741) == "1234ABCD "
@test cfmt("%-20X", UInt(3989525555)) == "EDCB5433 "
@test cfmt("%020d", 1024) == "00000000000000001024"
@test cfmt("%020d", -1024) == "-0000000000000001024"
@test cfmt("%020i", 1024) == "00000000000000001024"
@test cfmt("%020i", -1024) == "-0000000000000001024"
@test cfmt("%020u", 1024) == "00000000000000001024"
@test cfmt("%020u", UInt(4294966272)) == "00000000004294966272"
@test cfmt("%020o", 511) == "00000000000000000777"
@test cfmt("%020o", UInt(4294966785)) == "00000000037777777001"
@test cfmt("%020x", 305441741) == "0000000000001234abcd"
@test cfmt("%020x", UInt(3989525555)) == "000000000000edcb5433"
@test cfmt("%020X", 305441741) == "0000000000001234ABCD"
@test cfmt("%020X", UInt(3989525555)) == "000000000000EDCB5433"
@test cfmt("%#20o", 511) == " 0777"
@test cfmt("%#20o", UInt(4294966785)) == " 037777777001"
@test cfmt("%#20x", 305441741) == " 0x1234abcd"
@test cfmt("%#20x", UInt(3989525555)) == " 0xedcb5433"
@test cfmt("%#20X", 305441741) == " 0X1234ABCD"
@test cfmt("%#20X", UInt(3989525555)) == " 0XEDCB5433"
@test cfmt("%#020o", 511) == "00000000000000000777"
@test cfmt("%#020o", UInt(4294966785)) == "00000000037777777001"
@test cfmt("%#020x", 305441741) == "0x00000000001234abcd"
@test cfmt("%#020x", UInt(3989525555)) == "0x0000000000edcb5433"
@test cfmt("%#020X", 305441741) == "0X00000000001234ABCD"
@test cfmt("%#020X", UInt(3989525555)) == "0X0000000000EDCB5433"
@test cfmt("%0-20d", 1024) == "1024 "
@test cfmt("%0-20d", -1024) == "-1024 "
@test cfmt("%0-20i", 1024) == "1024 "
@test cfmt("%0-20i", -1024) == "-1024 "
@test cfmt("%0-20u", 1024) == "1024 "
@test cfmt("%0-20u", UInt(4294966272)) == "4294966272 "
@test cfmt("%-020o", 511) == "777 "
@test cfmt("%-020o", UInt(4294966785)) == "37777777001 "
@test cfmt("%-020x", 305441741) == "1234abcd "
@test cfmt("%-020x", UInt(3989525555)) == "edcb5433 "
@test cfmt("%-020X", 305441741) == "1234ABCD "
@test cfmt("%-020X", UInt(3989525555)) == "EDCB5433 "
@test cfmt("%.20d", 1024) == "00000000000000001024"
@test cfmt("%.20d", -1024) == "-00000000000000001024"
@test cfmt("%.20i", 1024) == "00000000000000001024"
@test cfmt("%.20i", -1024) == "-00000000000000001024"
@test cfmt("%.20u", 1024) == "00000000000000001024"
@test cfmt("%.20u", UInt(4294966272)) == "00000000004294966272"
@test cfmt("%.20o", 511) == "00000000000000000777"
@test cfmt("%.20o", UInt(4294966785)) == "00000000037777777001"
@test cfmt("%.20x", 305441741) == "0000000000001234abcd"
@test cfmt("%.20x", UInt(3989525555)) == "000000000000edcb5433"
@test cfmt("%.20X", 305441741) == "0000000000001234ABCD"
@test cfmt("%.20X", UInt(3989525555)) == "000000000000EDCB5433"
@test cfmt("%20.5d", 1024) == " 01024"
@test cfmt("%20.5d", -1024) == " -01024"
@test cfmt("%20.5i", 1024) == " 01024"
@test cfmt("%20.5i", -1024) == " -01024"
@test cfmt("%20.5u", 1024) == " 01024"
@test cfmt("%20.5u", UInt(4294966272)) == " 4294966272"
@test cfmt("%20.5o", 511) == " 00777"
@test cfmt("%20.5o", UInt(4294966785)) == " 37777777001"
@test cfmt("%20.5x", 305441741) == " 1234abcd"
@test cfmt("%20.10x", UInt(3989525555)) == " 00edcb5433"
@test cfmt("%20.5X", 305441741) == " 1234ABCD"
@test cfmt("%20.10X", UInt(3989525555)) == " 00EDCB5433"
@test cfmt("%020.5d", 1024) == " 01024"
@test cfmt("%020.5d", -1024) == " -01024"
@test cfmt("%020.5i", 1024) == " 01024"
@test cfmt("%020.5i", -1024) == " -01024"
@test cfmt("%020.5u", 1024) == " 01024"
@test cfmt("%020.5u", UInt(4294966272)) == " 4294966272"
@test cfmt("%020.5o", 511) == " 00777"
@test cfmt("%020.5o", UInt(4294966785)) == " 37777777001"
@test cfmt("%020.5x", 305441741) == " 1234abcd"
@test cfmt("%020.10x", UInt(3989525555)) == " 00edcb5433"
@test cfmt("%020.5X", 305441741) == " 1234ABCD"
@test cfmt("%020.10X", UInt(3989525555)) == " 00EDCB5433"
@test cfmt("%20.0d", 1024) == " 1024"
@test cfmt("%20.d", -1024) == " -1024"
@test cfmt("%20.d", 0) == " 0"
@test cfmt("%20.0i", 1024) == " 1024"
@test cfmt("%20.i", -1024) == " -1024"
@test cfmt("%20.i", 0) == " 0"
@test cfmt("%20.u", 1024) == " 1024"
@test cfmt("%20.0u", UInt(4294966272)) == " 4294966272"
@test cfmt("%20.u", UInt(0)) == " 0"
@test cfmt("%20.o", 511) == " 777"
@test cfmt("%20.0o", UInt(4294966785)) == " 37777777001"
@test cfmt("%20.o", UInt(0)) == " 0"
@test cfmt("%20.x", 305441741) == " 1234abcd"
@test cfmt("%20.0x", UInt(3989525555)) == " edcb5433"
@test cfmt("%20.x", UInt(0)) == " 0"
@test cfmt("%20.X", 305441741) == " 1234ABCD"
@test cfmt("%20.0X", UInt(3989525555)) == " EDCB5433"
@test cfmt("%20.X", UInt(0)) == " 0"
# Printf.jl issue #41971
@test cfmt("%4d", typemin(Int8)) == "-128"
@test cfmt("%4d", typemax(Int8)) == " 127"
@test cfmt("%6d", typemin(Int16)) == "-32768"
@test cfmt("%6d", typemax(Int16)) == " 32767"
@test cfmt("%11d", typemin(Int32)) == "-2147483648"
@test cfmt("%11d", typemax(Int32)) == " 2147483647"
@test cfmt("%20d", typemin(Int64)) == "-9223372036854775808"
@test cfmt("%20d", typemax(Int64)) == " 9223372036854775807"
@test cfmt("%40d", typemin(Int128)) == "-170141183460469231731687303715884105728"
@test cfmt("%40d", typemax(Int128)) == " 170141183460469231731687303715884105727"
end
# Printf.jl issue #52749
@test cfmt("%.160g", 1.38e-23) == "1.380000000000000060010582465734078799297660966782642624395399644741944111814291318296454846858978271484375e-23"
end # @testset "cfmt"
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 329 | using Format
using Test
using Printf
using Random
@testset "cformat" begin include( "cformat.jl" ) end
@testset "printf" begin include( "printf.jl" ) end
@testset "fmtspec" begin include( "fmtspec.jl" ) end
@testset "formatexpr" begin include( "formatexpr.jl" ) end
@testset "fmt" begin include( "fmt.jl" ) end
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | code | 1969 | println( "\nTest speed" )
const BENCH_REP = 200_000
function native_int()
for i in 1:BENCH_REP
@sprintf( "%10d", i )
end
end
@static if VERSION >= v"1.6"
function format_int()
fmt = Printf.Format( "%10d" )
for i in 1:BENCH_REP
Printf.format( fmt, i )
end
end
end
function runtime_int()
for i in 1:BENCH_REP
cfmt( "%10d", i )
end
end
function runtime_int_spec()
fs = Format.FmtSpec("%10d")
for i in 1:BENCH_REP
cfmt( fs, i )
end
end
function runtime_int_bypass()
f = generate_formatter( "%10d" )
for i in 1:BENCH_REP
f( i )
end
end
println( "integer @sprintf speed")
@time native_int()
@static if VERSION >= v"1.6"
println( "integer format speed")
@time format_int()
end
println( "integer cfmt speed")
@time runtime_int()
println( "integer cfmt spec speed")
@time runtime_int_spec()
println( "integer cfmt speed, bypass repeated lookup")
@time runtime_int_bypass()
set_seed!(10)
const testflts = [ _erfinv( rand() ) for i in 1:200_000 ]
function native_float()
for v in testflts
@sprintf( "%10.4f", v)
end
end
@static if VERSION >= v"1.6"
function format_float()
fmt = Printf.Format( "%10.4f" )
for v in testflts
Printf.format( fmt, v )
end
end
end
function runtime_float()
for v in testflts
cfmt( "%10.4f", v)
end
end
function runtime_float_spec()
fs = Format.FmtSpec("%10.4f")
for v in testflts
cfmt( fs, v )
end
end
function runtime_float_bypass()
f = generate_formatter( "%10.4f" )
for v in testflts
f( v )
end
end
println()
println( "float64 @sprintf speed")
@time native_float()
@static if VERSION >= v"1.6"
println( "float64 format speed")
@time format_float()
end
println( "float64 cfmt speed")
@time runtime_float()
println( "float64 cfmt spec speed")
@time runtime_float_spec()
println( "float64 cfmt speed, bypass repeated lookup")
@time runtime_float_bypass()
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 1.3.7 | 9c68794ef81b08086aeb32eeaf33531668d5f5fc | docs | 9679 | # Format
This package offers Python-style general formatting and c-style numerical formatting (for speed).
[pkg-url]: https://github.com/JuliaString/Format.jl.git
[julia-url]: https://github.com/JuliaLang/Julia
[julia-release]:https://img.shields.io/github/release/JuliaLang/julia.svg
[release]: https://img.shields.io/github/release/JuliaString/Format.jl.svg
[release-date]: https://img.shields.io/github/release-date/JuliaString/Format.jl.svg
[license-img]: http://img.shields.io/badge/license-MIT-brightgreen.svg?style=flat
[license-url]: LICENSE.md
[gitter-img]: https://badges.gitter.im/Join%20Chat.svg
[gitter-url]: https://gitter.im/JuliaString/Lobby?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge
[travis-url]: https://travis-ci.org/JuliaString/Format.jl
[travis-s-img]: https://travis-ci.org/JuliaString/Format.jl.svg
[travis-m-img]: https://travis-ci.org/JuliaString/Format.jl.svg?branch=master
[codecov-url]: https://codecov.io/gh/JuliaString/Format.jl
[codecov-img]: https://codecov.io/gh/JuliaString/Format.jl/branch/master/graph/badge.svg
[contrib]: https://img.shields.io/badge/contributions-welcome-brightgreen.svg?style=flat
[![][release]][pkg-url] [![][release-date]][pkg-url] [![][license-img]][license-url] [![contributions welcome][contrib]](https://github.com/JuliaString/Format.jl/issues)
| **Julia Version** | **Unit Tests** | **Coverage** |
|:------------------:|:------------------:|:---------------------:|
| [![][julia-release]][julia-url] | [![][travis-s-img]][travis-url] | [![][codecov-img]][codecov-url]
| Julia Latest | [![][travis-m-img]][travis-url] | [![][codecov-img]][codecov-url]
## Getting Started
This package is pure Julia. Setting up this package is like setting up other Julia packages:
```julia
Pkg.add("Format")
```
or
```julia
]add Format
```
It is forked off of [Formatting.jl](https://github.com/JuliaIO/Formatting.jl), and I try to keep the oldmaster branch up to date with the master branch of that, and cherry pick or port all necessary changes to `Format`).
To start using the package, you can simply write
```julia
using Format
```
This package depends on Julia of version 1.4 or above, and. The package is MIT-licensed.
## Python-style Types and Functions
#### Types to Represent Formats
This package has two types ``FormatSpec`` and ``FormatExpr`` to represent a format specification.
In particular, ``FormatSpec`` is used to capture the specification of a single entry. One can compile a format specification string into a ``FormatSpec`` instance as
```julia
fspec = FormatSpec("d")
fspec = FormatSpec("<8.4f")
```
Please refer to [Python's format specification language](http://docs.python.org/2/library/string.html#formatspec) for details.
``FormatExpr`` captures a formatting expression that may involve multiple items. One can compile a formatting string into a ``FormatExpr`` instance as
```julia
fe = FormatExpr("{1} + {2}")
fe = FormatExpr("{1:d} + {2:08.4e} + {3|>abs2}")
```
Please refer to [Python's format string syntax](http://docs.python.org/2/library/string.html#format-string-syntax) for details.
**Note:** If the same format is going to be applied for multiple times. It is more efficient to first compile it.
#### Formatted Printing
One can use ``printfmt`` and ``printfmtln`` for formatted printing:
- **printfmt**(io, fe, args...)
- **printfmt**(fe, args...)
Print given arguments using given format ``fe``. Here ``fe`` can be a formatting string, an instance of ``FormatSpec`` or ``FormatExpr``.
**Examples**
```julia
printfmt("{1:>4s} + {2:.2f}", "abc", 12) # --> print(" abc + 12.00")
printfmt("{} = {:#04x}", "abc", 12) # --> print("abc = 0x0c")
fs = FormatSpec("#04x")
printfmt(fs, 12) # --> print("0x0c")
fe = FormatExpr("{} = {:#04x}")
printfmt(fe, "abc", 12) # --> print("abc = 0x0c")
```
**Notes**
If the first argument is a string, it will be first compiled into a ``FormatExpr``, which implies that you can not use specification-only string in the first argument.
```julia
printfmt("{1:d}", 10) # OK, "{1:d}" can be compiled into a FormatExpr instance
printfmt("d", 10) # Error, "d" can not be compiled into a FormatExpr instance
# such a string to specify a format specification for single argument
printfmt(FormatSpec("d"), 10) # OK
printfmt(FormatExpr("{1:d}", 10)) # OK
```
- **printfmtln**(io, fe, args...)
- **printfmtln**(fe, args...)
Similar to ``printfmt`` except that this function print a newline at the end.
#### Formatted String
One can use ``pyfmt`` to format a single value into a string, or ``format`` to format one to multiple arguments into a string using an format expression.
- **pyfmt**(fspec, a)
Format a single value using a format specification given by ``fspec``, where ``fspec`` can be either a string or an instance of ``FormatSpec``.
- **format**(fe, args...)
Format arguments using a format expression given by ``fe``, where ``fe`` can be either a string or an instance of ``FormatSpec``.
#### Difference from Python's Format
At this point, this package implements a subset of Python's formatting language (with slight modification). Here is a summary of the differences:
- In terms of argument specification, it supports natural ordering (e.g. ``{} + {}``), explicit position (e.g. ``{1} + {2}``). It hasn't supported named arguments or fields extraction yet. Note that mixing these two modes is not allowed (e.g. ``{1} + {}``).
- The package provides support for filtering (for explicitly positioned arguments), such as ``{1|>lowercase}`` by allowing one to embed the ``|>`` operator, which the Python counter part does not support.
## C-style functions
The c-style part of this package aims to get around the limitation that
`@sprintf` has to take a literal string argument.
The core part is basically a c-style print formatter using the standard
`@sprintf` macro.
It also adds functionalities such as commas separator (thousands), parenthesis for negatives,
stripping trailing zeros, and mixed fractions.
### Usage and Implementation
The idea here is that the package compiles a function only once for each unique
format string within the `Format.*` name space, so repeated use is faster.
Unrelated parts of a session using the same format string would reuse the same
function, avoiding redundant compilation. To avoid the proliferation of
functions, we limit the usage to only 1 argument. Practical consideration
would suggest that only dozens of functions would be created in a session, which
seems manageable.
Usage
```julia
using Format
fmt = "%10.3f"
s = cfmt( fmt, 3.14159 ) # usage 1. Quite performant. Easiest to switch to.
fmtrfunc = generate_formatter( fmt ) # usage 2. This bypass repeated lookup of cached function. Most performant.
s = fmtrfunc( 3.14159 )
s = format( 3.14159, precision=3 ) # usage 3. Most flexible, with some non-printf options. Least performant.
```
### Commas
This package also supplements the lack of thousand separator e.g. `"%'d"`, `"%'f"`, `"%'g"`.
Note: `"%'g"` behavior is that for small enough floating point (but not too small),
thousand separator would be used. If the number needs to be represented by `"%e"`, no
separator is used.
### Flexible `format` function
This package contains a run-time number formatter `format` function, which goes beyond
the standard `sprintf` functionality.
An example:
```julia
s = format( 1234, commas=true ) # 1,234
s = format( -1234, commas=true, parens=true ) # (1,234)
```
The keyword arguments are (Bold keywords are not printf standard)
* width. Integer. Try to fit the output into this many characters. May not be successful.
Sacrifice space first, then commas.
* precision. Integer. How many decimal places.
* leftjustified. Boolean
* zeropadding. Boolean
* commas. Boolean. Thousands-group separator.
* signed. Boolean. Always show +/- sign?
* positivespace. Boolean. Prepend an extra space for positive numbers? (so they align nicely with negative numbers)
* **parens**. Boolean. Use parenthesis instead of "-". e.g. `(1.01)` instead of `-1.01`. Useful in finance. Note that
you cannot use `signed` and `parens` option at the same time.
* **stripzeros**. Boolean. Strip trailing '0' to the right of the decimal (and to the left of 'e', if any ).
* It may strip the decimal point itself if all trailing places are zeros.
* This is true by default if precision is not given, and vice versa.
* alternative. Boolean. See `#` alternative form explanation in standard printf documentation
* conversion. length=1 string. Default is type dependent. It can be one of `aAeEfFoxX`. See standard
printf documentation.
* **mixedfraction**. Boolean. If the number is rational, format it in mixed fraction e.g. `1_1/2` instead of `3/2`
* **mixedfractionsep**. Default `_`
* **fractionsep**. Default `/`
* **fractionwidth**. Integer. Try to pad zeros to the numerator until the fractional part has this width
* **tryden**. Integer. Try to use this denominator instead of a smaller one. No-op if it'd lose precision.
* **suffix**. String. This strings will be appended to the output. Useful for units/%
* **autoscale**. Symbol, default `:none`. It could be `:metric`, `:binary`, or `:finance`.
* `:metric` implements common SI symbols for large and small numbers e.g. `M`, `k`, `μ`, `n`
* `:binary` implements common ISQ symbols for large numbers e.g. `Ti`, `Gi`, `Mi`, `Ki`
* `:finance` implements common finance/news symbols for large numbers e.g. `b` (billion), `m` (millions)
See the test script for more examples.
| Format | https://github.com/JuliaString/Format.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | code | 455 | push!(LOAD_PATH,"../src/")
using Documenter
using PrincipalMomentAnalysis
makedocs(
sitename="PrincipalMomentAnalysis.jl",
modules = [PrincipalMomentAnalysis],
format = Documenter.HTML(prettyurls = get(ENV, "CI", nothing) == "true"),
pages = [
"Home" => "index.md",
"Tutorial" => "tutorial.md",
"Reference" => "reference.md",
]
)
deploydocs(
repo = "github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git",
)
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | code | 361 | module PrincipalMomentAnalysis
using LinearAlgebra
using SparseArrays
using Statistics
using NearestNeighbors
include("svd.jl")
include("simplices.jl")
include("pma.jl")
include("util.jl")
export
PMA,
pma,
SimplexGraph,
groupsimplices,
timeseriessimplices,
neighborsimplices,
normalizemean!,
normalizemean,
normalizemeanstd!,
normalizemeanstd
end
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | code | 2532 | """
PMA <: Factorization
The output of `pma` representing the Principal Moment Analysis factorization of a matrix `A`.
If `F::PMA` is the factorization object, `U`, `S`, `V` and `Vt` can be obtained via `F.U`, `F.S`, `F.V` and `F.Vt` such as A ≈ U * Diagonal(S) * Vt'.
See also `pma`.
"""
struct PMA{T} <: Factorization{T}
U::Matrix{T}
S::Vector{T}
Vt::Matrix{T}
VV::Matrix{T}
end
Base.size(F::PMA, dim::Integer) = dim == 1 ? size(F.U, dim) : size(F.Vt, dim)
Base.size(F::PMA) = (size(F, 1), size(F, 2))
function Base.getproperty(F::PMA,name::Symbol)
name==:V && return F.Vt'
getfield(F,name)
end
Base.propertynames(::PMA) = (:U,:S,:V,:Vt,:VV)
function simplices2kernelmatrix(sg::SimplexGraph)
A = simplices2kernelmatrixroot(sg, simplify=false)
A*A'
end
function simplices2kernelmatrixroot(sg::SimplexGraph; simplify=true)::SparseMatrixCSC
s = vec(sum(sg.G,dims=1))
L = sg.G * Diagonal(sqrt.(sg.w./(s.*(s.+1))))
D = Diagonal(sqrt.(vec(sum(x->x^2,L,dims=2))))
simplify || return [L D]
QR = qr(vcat(sparse(L'), D))
sparse(QR.R[:,invperm(QR.pcol)]')
end
function _pma(A::AbstractMatrix, S::AbstractMatrix; nsv::Integer=6)
P,N = size(A)
L = size(S,2)
Y = A*S
K = Symmetric(Y'Y)
nsv = min(nsv, P, N)
F = eigen(K, L-nsv+1:L)
Σ = sqrt.(max.(0.,reverse(F.values)))
VV = F.vectors[:,end:-1:1] # Coordinates of simplex equivalents, not interesting in practice.
U = Y*VV ./ Σ'
Vt = U'A ./ Σ # Coordinates of original sample points in low dimensional space.
PMA(U,Σ,Vt,VV)
end
"""
pma(A, G::SimplexGraph; nsv=6)
Computes the Principal Moment Analysis of the matrix `A` (variables × samples) using the sample SimplexGraph `G`.
Each column in `G` is a boolean vector representing one simplex. True means that a vertex is part of the simplex and false that it is not.
Set `nsv` to control the number of singular values and vectors returned.
Returns a `PMA` struct.
See also `PMA`, `SimplexGraph`.
"""
pma(A::AbstractMatrix, sg::SimplexGraph; kwargs...) = _pma(A, simplices2kernelmatrixroot(sg); kwargs...)
"""
pma(A, G::AbstractMatrix{Bool}; nsv=6)
Computes the Principal Moment Analysis of the matrix `A` (variables × samples).
Each column in `G` is a boolean vector representing one simplex. True means that a vertex is part of the simplex and false that it is not.
Set `nsv` to control the number of singular values and vectors returned.
Returns a `PMA` struct.
See also `PMA`, `SimplexGraph`.
"""
pma(A::AbstractMatrix, G::AbstractMatrix{Bool}; kwargs...) = pma(A, SimplexGraph(G); kwargs...)
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | code | 5958 | """
SimplexGraph{T<:AbstractMatrix{Bool}, S<:Union{Number,AbstractVector{<:Number}}}
Struct describing a set of simplices with vertices numbered from 1:N.
Optionally, each simplex can be assigned a weight.
Usage
--------------
SimplexGraph(G, w=true)
Construct a SimplexGraph. `G` is a `MxN` matrix of booleans, where `M` is the number of vertices and `N` is the number of simplices.
Each column in `G` represents one simplex. True means that a vertex is part of the simplex and false that it is not.
Optionally, a vector `w` of length N can be used to specify a weight (total mass) for each simplex. The weight defaults to 1 (true).
See also `pma`, `groupsimplices`, `timeseriessimplices`, `neighborsimplices`.
"""
struct SimplexGraph{T<:AbstractMatrix{Bool}, S<:Union{Number,AbstractVector{<:Number}}}
G::T
w::S
function SimplexGraph(G::T, w::S=true) where {T<:AbstractMatrix{Bool}, S<:Union{Number,AbstractVector{<:Number}}}
@assert ndims(w)==0 || (size(w,1)==size(G,2))
new{T,S}(G,w)
end
end
"""
groupsimplices(groupby::AbstractVector)
Create SimplexGraph connecting elements with identical values in the groupby vector.
# Examples
```
julia> sg = groupsimplices(["A","A","B","C","B"]);
julia> sg.G
5×3 BitArray{2}:
1 0 0
1 0 0
0 1 0
0 0 1
0 1 0
julia> sg.w
3-element Array{Int64,1}:
2
2
1
```
"""
function groupsimplices(groupby::AbstractVector)
u = unique(groupby)
ind = indexin(groupby,u)
I = Vector{Int}(undef,length(groupby))
J = Vector{Int}(undef,length(groupby))
w = zeros(Int,length(u))
for (i,j) in enumerate(ind)
I[i] = i
J[i] = j
w[j] += 1
end
SimplexGraph(sparse(I,J,true),w)
end
"""
timeseriessimplices(time::AbstractVector; groupby::AbstractVector)
Create SimplexGraph connecting elements adjacent in time.
In case of ties, **all** elements at a unique timepoint will be connected to the **all** elements at the previous, current and next timepoints.
If `groupby` is specified, the elements are first divided by group, and then connected by time.
# Examples
```
julia> sg = timeseriessimplices([0.5, 1.0, 4.0]);
julia> sg.G
3×3 BitArray{2}:
1 1 0
1 1 1
0 1 1
julia> sg = timeseriessimplices([0.5, 1.0, 1.0, 4.0]);
julia> sg.G
4×3 BitArray{2}:
1 1 0
1 1 1
1 1 1
0 1 1
julia> sg.w
3-element Array{Int64,1}:
1
2
1
julia> sg = timeseriessimplices([2, 4, 6, 2, 4, 8]; groupby=["A","A","A","B","B","B"]);
julia> sg.G
6×6 BitArray{2}:
1 1 0 0 0 0
1 1 1 0 0 0
0 1 1 0 0 0
0 0 0 1 1 0
0 0 0 1 1 1
0 0 0 0 1 1
```
"""
function timeseriessimplices(time::AbstractVector; groupby::AbstractVector=falses(length(time)))
N = length(groupby)
I,J = Int[],Int[]
w = Int[]
# TODO: Rewrite. Can be simplified and optimized.
col = 1
for g in unique(groupby)
ind = findall( groupby.==g )
time2 = time[ind]
uniqueTimes = sort(unique(time2))
currInd = Int[]
nextInd = ind[time2.==uniqueTimes[1]]
for t in Iterators.drop(uniqueTimes,1)
prevInd = currInd
currInd = nextInd
nextInd = ind[time2.==t]
append!(I, prevInd)
append!(I, currInd)
append!(I, nextInd)
append!(J, repeat([col],outer=length(prevInd)+length(currInd)+length(nextInd)))
push!(w, length(currInd))
col += 1
end
if length(uniqueTimes)==2 # Two timepoints means the same simplex is used for both.
w[end] += length(nextInd)
else
append!(I, currInd)
append!(I, nextInd)
append!(J, repeat([col],outer=length(currInd)+length(nextInd)))
push!(w, length(nextInd))
col += 1
end
end
SimplexGraph(sparse(I,J,true),w)
end
"""
neighborsimplices(A::AbstractMatrix; k, r, dim, symmetric, normalizedist, groupby)
Create simplex graph connecting nearest neighbor samples.
# Inputs
* `A`: Data matrix (variables × samples).
* `k`: Number of nearest neighbors to connect. Default: `0`.
* `r`: Connected all neighbors with disctance `≤r`. Default: `0.0`.
* `dim`: Reduce the dimension to `dim` before computing distances. Useful to reduce noise. Default: Disabled.
* `symmetric`: Make the simplex graph symmetric. Default: `false`.
* `normalizedist`: Normalize distances to the scale [0.0,1.0] such that the maximal distance from a point to the origin is 0.5. Affects the `r` parameter. Default: `true`.
* `groupby`: Only connected samples within the specified groups. Default: Disabled.
# Examples
```
julia> sg = neighborsimplices([0 0 2 2; 0 1 1 0]; k=1); sg.G
4×4 BitArray{2}:
1 1 0 0
1 1 0 0
0 0 1 1
0 0 1 1
julia> sg = neighborsimplices([0 0 2 2; 0 1 1 0]; r=0.45); sg.G
4×4 BitArray{2}:
1 1 0 1
1 1 1 0
0 1 1 1
1 0 1 1
```
"""
function neighborsimplices(A::AbstractMatrix; dim::Integer=typemax(Int), kwargs...)
if dim<minimum(size(A))
F = svdbyeigen(A; nsv=dim)
A = Diagonal(F.S)*F.Vt
end
_neighborsimplices(A; kwargs...)
end
function _neighborsimplices(A::AbstractMatrix; k::Integer=0, r::Real=0.0, symmetric=false, normalizedist=true, groupby=nothing)
k==0 && r==0.0 && return SimplexGraph(sparse(Diagonal(trues(size(A,2))))) # trivial case, avoid some computations.
r>0.0 && normalizedist && (r *= 2*sqrt(maximum(sum(x->x^2, A, dims=1))))
N = size(A,2)
rowInd,colInd = Int[],Int[]
if groupby===nothing
_neighborsimplices!(rowInd, colInd, A, identity, k, r)
else
for g in unique(groupby)
ind = findall( groupby.==g )
_neighborsimplices!(rowInd, colInd, A[:,ind], x->ind[x], k, r)
end
end
SimplexGraph(symmetric ? sparse([rowInd;colInd],[colInd;rowInd],true) : sparse(rowInd,colInd,true))
end
function _neighborsimplices!(rowInd, colInd, A, indfun, k, r)
tree = BallTree(A)
if k>0
indices,_ = knn(tree, A, min(k+1,size(A,2)))
for (j,I) in enumerate(indices)
append!(rowInd,indfun.(I))
append!(colInd,repeat([indfun(j)],outer=length(I)))
end
end
if r>0.0
indices = inrange(tree, A, r)
for (j,I) in enumerate(indices)
append!(rowInd,indfun.(I))
append!(colInd,repeat([indfun(j)],outer=length(I)))
end
end
end
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | code | 243 | function svdbyeigen(A; nsv::Integer=3)
P,N = size(A)
K = Symmetric(N<=P ? A'A : A*A')
M = size(K,1)
F = eigen(K, M-nsv+1:M)
S = sqrt.(max.(0.,reverse(F.values)))
V = F.vectors[:,end:-1:1]
N<=P ? SVD(A*V./S',S,V') : SVD(V,S,V'A./S)
end
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | code | 1074 | """
normalizemean!(A)
In place removal of the variable mean from the P×N matrix `A` where P is the number of variables and N is the number of samples.
"""
normalizemean!(A::AbstractMatrix) = A .-= mean(A,dims=2)
"""
normalizemean(A)
Remove the variable mean from the P×N matrix `A` where P is the number of variables and N is the number of samples.
"""
normalizemean(A::AbstractMatrix) = A .- mean(A,dims=2)
"""
normalizemeanstd!(A)
In place normalization of variables to be mean zero and standard deviation one of the P×N matrix `A` where P is the number of variables and N is the number of samples.
"""
function normalizemeanstd!(A::AbstractMatrix)
normalizemean!(A::AbstractMatrix)
A ./= max.(1e-12, std(A,dims=2)) # max to avoid div by zero
end
"""
normalizemeanstd!(A)
Normalize variables to be mean zero and standard deviation one in the P×N matrix `A` where P is the number of variables and N is the number of samples.
"""
function normalizemeanstd(A::AbstractMatrix)
A = normalizemean(A)
A ./= max.(1e-12, std(A,dims=2)) # max to avoid div by zero
end
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | code | 757 | using PrincipalMomentAnalysis
using Test
using LinearAlgebra
using Statistics
# change back to this simple oneliner if we can just ignore problems in StaticArrays that is imported by NearestNeighbors
# @test [] == detect_ambiguities(Base, Core, PrincipalMomentAnalysis)
@testset "Method ambiguities" begin
ambiguities = detect_ambiguities(Base, Core, PrincipalMomentAnalysis)
filter!(x->x[1].module==PrincipalMomentAnalysis || x[2].module==PrincipalMomentAnalysis, ambiguities)
@test ambiguities == []
end
const simplices2kernelmatrix = PrincipalMomentAnalysis.simplices2kernelmatrix
const simplices2kernelmatrixroot = PrincipalMomentAnalysis.simplices2kernelmatrixroot
include("test_simplices.jl")
include("test_pma.jl")
include("test_util.jl")
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | code | 4408 | @testset "PMA" begin
function factorizationcmp(F1,F2)
@test size(F1)==size(F2)
@test size(F1.U)==size(F2.U)
@test size(F1.S)==size(F2.S)
@test size(F1.V)==size(F2.V)
@test size(F1.Vt)==size(F2.Vt)
@test F1.S ≈ F2.S
sgn = sign.(diag(F1.U'F2.U))
@test F1.U.*sgn' ≈ F2.U
@test F1.V.*sgn' ≈ F2.V
@test F1.Vt.*sgn ≈ F2.Vt
nothing
end
extractdims(F::SVD,dims) = SVD(F.U[:,dims], F.S[dims], F.Vt[dims,:])
A = [-8 0 6 0 0 0; -1 9 6 -2 0 -1; -1 0 -2 0 0 0; 0 1 0 1 0 -2; -9 -3 0 0 0 -5; -6 3 -8 -2 -4 8; 0 -1 -1 0 -3 -1; 4 0 0 -6 0 2]
@testset "PCA" begin
FAns = svd(A)
FPMA = pma(A,Diagonal(trues(6)); nsv=6)
factorizationcmp(FAns,FPMA)
FPMA4 = pma(A,Diagonal(trues(6)); nsv=4)
factorizationcmp(extractdims(FAns,1:4),FPMA4)
end
@testset "properties" begin
F = pma(A,Diagonal(trues(6)); nsv=3)
@test all(in(propertynames(F)), fieldnames(PMA))
@test :V in propertynames(F)
for p in propertynames(F)
@test getproperty(F,p)!=nothing # test that it doesn't throw
end
end
@testset "TotalSimplexMass" begin
A2 = hcat(repeat(A[:,1],1,5), repeat(A[:,2],1,2), A[:,3:end]) # five copies of first sample and two copies of second
G = Matrix(Diagonal(trues(11)))
G[1:5,1:5] .= true # Connect (identical) samples 1-5
G[6:7,6:7] .= true # Connect (identical) samples 6-7
FAns = svd(A2)
# FPMA = pma(A2,G; nsv=11) # TODO: Add this test case if we add support for computing U,V columns corresponding to zero-valued singular values.
# factorizationcmp(FAns,FPMA)
FPMA6 = pma(A2,G; nsv=6) # non-zero singular values
factorizationcmp(extractdims(FAns,1:6),FPMA6)
FPMA4 = pma(A2,G; nsv=4)
factorizationcmp(extractdims(FAns,1:4),FPMA4)
end
@testset "TotalSimplexMass2" begin
A2 = hcat(repeat(A[:,1],1,4), A[:,2:end]) # four copies of first sample
G = Matrix(Diagonal(trues(9)))
G[1:4,1:4] .= LowerTriangular(ones(4,4)) # Simplices of dimensions 1-4 connecting (some of) the 4 identical samples
FAns = svd(A2)
# FPMA = pma(A2,G; nsv=9) # TODO: Add this test case if we add support for computing U,V columns corresponding to zero-valued singular values.
# factorizationcmp(FAns,FPMA)
FPMA6 = pma(A2,G; nsv=6) # non-zero singular values
factorizationcmp(extractdims(FAns,1:6),FPMA6)
FPMA4 = pma(A2,G; nsv=4)
factorizationcmp(extractdims(FAns,1:4),FPMA4)
end
@testset "DisjointCliques" begin
G = Bool[1 0 0 0 0 0; 0 1 1 0 0 0; 0 1 1 0 0 0; 0 0 0 1 1 1; 0 0 0 1 1 1; 0 0 0 1 1 1]
# S = [1 0 0 0 0 0; 0 2/3 1/3 0 0 0; 0 1/3 2/3 0 0 0; 0 0 0 1/2 1/4 1/4; 0 0 0 1/4 1/2 1/4; 0 0 0 1/4 1/4 1/2] # corresponding S matrix
a,b,c,d = 1/2+sqrt(1/12), 1/2-sqrt(1/12), 2/3, 1/6
Ssq = [1 0 0 0 0 0; 0 a b 0 0 0; 0 b a 0 0 0; 0 0 0 c d d; 0 0 0 d c d; 0 0 0 d d c ]
F = svd(A*Ssq)
FVt = (F.U'A)./F.S
FAns = SVD(F.U, F.S, FVt)
FPMA = pma(A,G; nsv=6)
factorizationcmp(FAns,FPMA)
FPMA4 = pma(A,G; nsv=4)
factorizationcmp(extractdims(FAns,1:4),FPMA4)
end
@testset "SymmetricSimplexGraph" begin
G = Bool[1 0 0 0 1 0; 0 1 1 0 1 1; 0 1 1 1 1 0; 0 0 1 1 1 0; 1 1 1 1 1 1; 0 1 0 0 1 1]
S = [8/21 1/42 1/42 1/42 4/21 1/42; 1/42 29/70 13/105 31/420 29/140 11/70; 1/42 13/105 29/70 11/70 29/140 31/420; 1/42 31/420 11/70 11/35 11/70 1/42; 4/21 29/140 29/140 11/70 32/35 11/70; 1/42 11/70 31/420 1/42 11/70 11/35]
Ssq = sqrt(S);
F = svd(A*Ssq)
FVt = (F.U'A)./F.S
FAns = SVD(F.U, F.S, FVt)
FPMA = pma(A,G; nsv=6)
factorizationcmp(FAns,FPMA)
FPMA4 = pma(A,G; nsv=4)
factorizationcmp(extractdims(FAns,1:4),FPMA4)
end
@testset "AsymmetricSimplexGraph" begin
G = Bool[1 0 0 0 0 0; 0 1 1 1 1 0; 0 1 1 0 1 1; 0 1 1 1 0 1; 0 0 0 0 1 1; 1 0 0 0 0 1]
S = [1/3 0 0 0 0 1/6; 0 5/6 1/4 1/3 1/12 0; 0 1/4 3/5 13/60 2/15 1/20; 0 1/3 13/60 23/30 1/20 1/20; 0 1/12 2/15 1/20 4/15 1/20; 1/6 0 1/20 1/20 1/20 13/30]
Ssq = sqrt(S);
F = svd(A*Ssq)
FVt = (F.U'A)./F.S
FAns = SVD(F.U, F.S, FVt)
FPMA = pma(A,G; nsv=6)
factorizationcmp(FAns,FPMA)
FPMA4 = pma(A,G; nsv=4)
factorizationcmp(extractdims(FAns,1:4),FPMA4)
end
@testset "AsymmetricSimplexGraph2" begin
G = Bool[1 0 0 0 0 0; 0 0 1 1 1 0; 0 1 1 0 1 1; 0 1 1 0 0 1; 0 0 0 0 1 1; 1 0 0 0 0 0]
S = [1/3 0 0 0 0 1/6; 0 4/3 1/6 1/12 1/12 0; 0 1/6 5/6 1/3 1/6 0; 0 1/12 1/3 2/3 1/12 0; 0 1/12 1/6 1/12 1/3 0; 1/6 0 0 0 0 1/3]
Ssq = sqrt(S);
F = svd(A*Ssq)
FVt = (F.U'A)./F.S
FAns = SVD(F.U, F.S, FVt)
FPMA = pma(A,G; nsv=6)
factorizationcmp(FAns,FPMA)
FPMA4 = pma(A,G; nsv=4)
factorizationcmp(extractdims(FAns,1:4),FPMA4)
end
end
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | code | 16712 | @testset "Simplices" begin
# checks that to SimplexGraphs are identical up to a permutation of the simplices.
function simplexgraphcmp(sg1,sg2)
@test size(sg1.G)==size(sg2.G)
@test size(sg1.w)==size(sg2.w)
N = size(sg1.G,2)
if sg1.w isa AbstractVector
perm1 = sortperm(1:N, by=i->(sg1.w[i], sg1.G[:,i]))
perm2 = sortperm(1:N, by=i->(sg2.w[i], sg2.G[:,i]))
@test sg1.w[perm1] == sg2.w[perm2]
else
perm1 = sortperm(1:N, by=i->sg1.G[:,i])
perm2 = sortperm(1:N, by=i->sg2.G[:,i])
end
@test sg1.G[:,perm1] == sg2.G[:,perm2]
nothing
end
@testset "ThreeGroups" begin
sgAnswer = SimplexGraph(Bool[1 0 0; 1 0 0; 0 1 0; 0 0 1], [2,1,1])
SAnswer = [2/3 1/3 0 0; 1/3 2/3 0 0; 0 0 1 0; 0 0 0 1]
annot = ["A","A","B","C"]
sg = groupsimplices(annot)
simplexgraphcmp(sg,sgAnswer)
S = simplices2kernelmatrix(sg)
@test S ≈ SAnswer
# try permuted version
perm = [2, 3, 4, 1]
sg = groupsimplices(annot[perm])
simplexgraphcmp(sg, SimplexGraph(sgAnswer.G[perm,:],sgAnswer.w))
S = simplices2kernelmatrix(sg)
@test S ≈ SAnswer[perm,perm]
end
@testset "OneSample" begin
annot = ["A"]
sg = groupsimplices(annot)
simplexgraphcmp(sg, SimplexGraph(trues(1,1),[1]))
S = simplices2kernelmatrix(sg)
@test S ≈ ones(1,1)
end
@testset "OneGroup" begin
annot = ["A", "A", "A"]
sg = groupsimplices(annot)
simplexgraphcmp(sg, SimplexGraph(trues(3,1),[3]))
S = simplices2kernelmatrix(sg)
@test S ≈ (Diagonal(ones(3)) + ones(3,3))/4
end
@testset "UniqueGroups" begin
annot = ["A", "B", "C", "D"]
sg = groupsimplices(annot)
simplexgraphcmp(sg, SimplexGraph(Diagonal(trues(4)),ones(Int,4)))
S = simplices2kernelmatrix(sg)
@test S ≈ Diagonal(ones(4))
end
@testset "OneTimeSeries" begin
N = 6
annot = repeat(["A"], N)
t = 1:N
sg = timeseriessimplices(t, groupby=annot)
simplexgraphcmp(sg, SimplexGraph(SymTridiagonal(trues(N),trues(N-1)), ones(Int,N)))
S = simplices2kernelmatrix(sg)
@test S ≈ [1/2 1/4 1/12 0 0 0; 1/4 2/3 1/6 1/12 0 0; 1/12 1/6 1/2 1/6 1/12 0; 0 1/12 1/6 1/2 1/6 1/12; 0 0 1/12 1/6 2/3 1/4; 0 0 0 1/12 1/4 1/2]
end
@testset "TwoTimeSeries" begin
N = 6
annot = repeat(["A","B"], inner=N)
t = repeat(1:N, 2)
sg = timeseriessimplices(t, groupby=annot)
T = SymTridiagonal(trues(N),trues(N-1))
Z = falses(N,N)
simplexgraphcmp(sg, SimplexGraph([T Z; Z T], ones(Int,2N)))
S = simplices2kernelmatrix(sg)
S1 = [1/2 1/4 1/12 0 0 0; 1/4 2/3 1/6 1/12 0 0; 1/12 1/6 1/2 1/6 1/12 0; 0 1/12 1/6 1/2 1/6 1/12; 0 0 1/12 1/6 2/3 1/4; 0 0 0 1/12 1/4 1/2]
@test S ≈ [S1 Z; Z S1]
end
@testset "OneTimeSeries2" begin
N = 6
annot = repeat(["A"], 2N)
t = div.(0:2N-1,2)
sg = timeseriessimplices(t, groupby=annot)
simplexgraphcmp(sg, SimplexGraph(repeat(SymTridiagonal(trues(N),trues(N-1)), inner=(2,1)), 2*ones(N)))
S = simplices2kernelmatrix(sg)
@test S ≈ [31/105 31/210 31/210 31/210 1/21 1/21 0 0 0 0 0 0; 31/210 31/105 31/210 31/210 1/21 1/21 0 0 0 0 0 0; 31/210 31/210 41/105 41/210 2/21 2/21 1/21 1/21 0 0 0 0; 31/210 31/210 41/210 41/105 2/21 2/21 1/21 1/21 0 0 0 0; 1/21 1/21 2/21 2/21 2/7 1/7 2/21 2/21 1/21 1/21 0 0; 1/21 1/21 2/21 2/21 1/7 2/7 2/21 2/21 1/21 1/21 0 0; 0 0 1/21 1/21 2/21 2/21 2/7 1/7 2/21 2/21 1/21 1/21; 0 0 1/21 1/21 2/21 2/21 1/7 2/7 2/21 2/21 1/21 1/21; 0 0 0 0 1/21 1/21 2/21 2/21 41/105 41/210 31/210 31/210; 0 0 0 0 1/21 1/21 2/21 2/21 41/210 41/105 31/210 31/210; 0 0 0 0 0 0 1/21 1/21 31/210 31/210 31/105 31/210; 0 0 0 0 0 0 1/21 1/21 31/210 31/210 31/210 31/105]
end
@testset "MixedTimeSeries" begin
GAnswer = Bool[1 1 0 0 0 0 0 0 0 0; 1 1 1 0 0 0 0 0 0 0; 1 1 1 0 0 0 0 0 0 0; 0 1 1 0 0 0 0 0 0 0; 0 1 1 0 0 0 0 0 0 0; 0 1 1 0 0 0 0 0 0 0; 0 0 0 1 0 0 0 0 0 0; 0 0 0 0 1 0 0 0 0 0; 0 0 0 0 1 0 0 0 0 0; 0 0 0 0 1 0 0 0 0 0; 0 0 0 0 0 1 1 0 0 0; 0 0 0 0 0 1 1 0 0 0; 0 0 0 0 0 1 1 1 0 0; 0 0 0 0 0 0 1 1 1 0; 0 0 0 0 0 0 0 1 1 1; 0 0 0 0 0 0 0 1 1 1; 0 0 0 0 0 0 0 1 1 1; 0 0 0 0 0 0 0 0 1 1]
wAnswer = Int[1, 2, 3, 1, 3, 2, 1, 1, 3, 1]
SAnswer = [11/42 11/84 11/84 1/21 1/21 1/21 0 0 0 0 0 0 0 0 0 0 0 0; 11/84 97/210 97/420 31/210 31/210 31/210 0 0 0 0 0 0 0 0 0 0 0 0; 11/84 97/420 97/210 31/210 31/210 31/210 0 0 0 0 0 0 0 0 0 0 0 0; 1/21 31/210 31/210 31/105 31/210 31/210 0 0 0 0 0 0 0 0 0 0 0 0; 1/21 31/210 31/210 31/210 31/105 31/210 0 0 0 0 0 0 0 0 0 0 0 0; 1/21 31/210 31/210 31/210 31/210 31/105 0 0 0 0 0 0 0 0 0 0 0 0; 0 0 0 0 0 0 1/1 0 0 0 0 0 0 0 0 0 0 0; 0 0 0 0 0 0 0 1/2 1/4 1/4 0 0 0 0 0 0 0 0; 0 0 0 0 0 0 0 1/4 1/2 1/4 0 0 0 0 0 0 0 0; 0 0 0 0 0 0 0 1/4 1/4 1/2 0 0 0 0 0 0 0 0; 0 0 0 0 0 0 0 0 0 0 13/30 13/60 13/60 1/20 0 0 0 0; 0 0 0 0 0 0 0 0 0 0 13/60 13/30 13/60 1/20 0 0 0 0; 0 0 0 0 0 0 0 0 0 0 13/60 13/60 1/2 1/12 1/30 1/30 1/30 0; 0 0 0 0 0 0 0 0 0 0 1/20 1/20 1/12 11/30 2/15 2/15 2/15 1/10; 0 0 0 0 0 0 0 0 0 0 0 0 1/30 2/15 11/30 11/60 11/60 3/20; 0 0 0 0 0 0 0 0 0 0 0 0 1/30 2/15 11/60 11/30 11/60 3/20; 0 0 0 0 0 0 0 0 0 0 0 0 1/30 2/15 11/60 11/60 11/30 3/20; 0 0 0 0 0 0 0 0 0 0 0 0 0 1/10 3/20 3/20 3/20 3/10]
annot = ["A", "A", "A", "A", "A", "A", "B", "C", "C", "C", "D", "D", "D", "D", "D", "D", "D", "D" ]
t = [ 0, 5, 5, 7, 7, 7, 8, 4, 4, 8, 1, 1, 2, 3, 4, 4, 4, 5 ]
sg = timeseriessimplices(t, groupby=annot)
simplexgraphcmp(sg, SimplexGraph(GAnswer,wAnswer))
S = simplices2kernelmatrix(sg)
@test S ≈ SAnswer
# try permuted version
perm = [3, 9, 13, 12, 2, 4, 17, 10, 7, 14, 11, 1, 6, 8, 5, 15, 18, 16]
sg = timeseriessimplices(t[perm], groupby=annot[perm])
simplexgraphcmp(sg, SimplexGraph(GAnswer[perm,:],wAnswer))
S = simplices2kernelmatrix(sg)
@test S ≈ SAnswer[perm,perm]
end
@testset "kNN" begin
A = Float64[9 1 3 9 3 3 7 3 5 5; 0 2 3.99 7 1 1 8 4 7.01 1; 0 6 3 7 0 9 0 2 0 6; 8 8 7 6 5 9 1 4 2 5];
sg = neighborsimplices(A)
@test sg.G == Diagonal(trues(10))
S = simplices2kernelmatrix(sg)
@test S ≈ Diagonal(ones(10))
sg = neighborsimplices(A; k=1)
@test sg.G == Bool[1 0 0 0 0 0 0 0 0 0; 0 1 0 0 0 1 0 0 0 0; 0 0 1 0 0 0 0 1 0 1; 0 0 0 1 0 0 0 0 0 0; 1 0 0 0 1 0 0 0 0 0; 0 1 0 0 0 1 0 0 0 0; 0 0 0 0 0 0 1 0 1 0; 0 0 1 0 1 0 0 1 0 0; 0 0 0 0 0 0 1 0 1 0; 0 0 0 1 0 0 0 0 0 1]
S = simplices2kernelmatrix(sg)
@test S ≈ [1/3 0 0 0 1/6 0 0 0 0 0; 0 2/3 0 0 0 1/3 0 0 0 0; 0 0 1 0 0 0 0 1/3 0 1/6; 0 0 0 1/3 0 0 0 0 0 1/6; 1/6 0 0 0 2/3 0 0 1/6 0 0; 0 1/3 0 0 0 2/3 0 0 0 0; 0 0 0 0 0 0 2/3 0 1/3 0; 0 0 1/3 0 1/6 0 0 1 0 0; 0 0 0 0 0 0 1/3 0 2/3 0; 0 0 1/6 1/6 0 0 0 0 0 2/3]
sg = neighborsimplices(A; k=2)
@test sg.G == Bool[1 0 0 0 0 0 0 0 0 0; 0 1 1 0 0 1 0 0 0 1; 1 1 1 1 1 0 0 1 0 1; 0 0 0 1 0 0 0 0 0 0; 1 0 0 0 1 0 0 1 0 0; 0 1 0 0 0 1 0 0 0 0; 0 0 0 0 0 0 1 0 1 0; 0 0 1 0 1 0 1 1 1 0; 0 0 0 0 0 0 1 0 1 0; 0 0 0 1 0 1 0 0 0 1]
S = simplices2kernelmatrix(sg)
@test S ≈ [1/6 0 1/12 0 1/12 0 0 0 0 0; 0 2/3 1/4 0 0 1/6 0 1/12 0 1/6; 1/12 1/4 7/6 1/12 1/4 1/12 0 1/4 0 1/6; 0 0 1/12 1/6 0 0 0 0 0 1/12; 1/12 0 1/4 0 1/2 0 0 1/6 0 0; 0 1/6 1/12 0 0 1/3 0 0 0 1/12; 0 0 0 0 0 0 1/3 1/6 1/6 0; 0 1/12 1/4 0 1/6 0 1/6 5/6 1/6 0; 0 0 0 0 0 0 1/6 1/6 1/3 0; 0 1/6 1/6 1/12 0 1/12 0 0 0 1/2]
sg = neighborsimplices(A; k=6)
@test sg.G == Bool[1 0 0 0 1 0 0 0 0 0; 0 1 1 0 1 1 0 1 0 1; 1 1 1 1 1 1 1 1 1 1; 1 1 0 1 0 1 1 0 1 1; 1 1 1 0 1 1 1 1 1 1; 0 1 1 1 0 1 0 0 0 1; 0 0 0 1 0 0 1 1 1 0; 1 1 1 1 1 1 1 1 1 1; 1 0 1 1 1 0 1 1 1 0; 1 1 1 1 1 1 1 1 1 1]
S = simplices2kernelmatrix(sg)
@test S ≈ [1/14 1/56 1/28 1/56 1/28 0 0 1/28 1/28 1/28; 1/56 3/14 3/28 3/56 3/28 1/14 1/56 3/28 3/56 3/28; 1/28 3/28 5/14 1/8 9/56 5/56 1/14 5/28 1/8 5/28; 1/56 3/56 1/8 1/4 3/28 1/14 3/56 1/8 1/14 1/8; 1/28 3/28 9/56 3/28 9/28 1/14 3/56 9/56 3/28 9/56; 0 1/14 5/56 1/14 1/14 5/28 1/56 5/56 1/28 5/56; 0 1/56 1/14 3/56 3/56 1/56 1/7 1/14 1/14 1/14; 1/28 3/28 5/28 1/8 9/56 5/56 1/14 5/14 1/8 5/28; 1/28 3/56 1/8 1/14 3/28 1/28 1/14 1/8 1/4 1/8; 1/28 3/28 5/28 1/8 9/56 5/56 1/14 5/28 1/8 5/14]
sg = neighborsimplices(A; k=9)
@test sg.G == trues(10,10)
S = simplices2kernelmatrix(sg)
@test S ≈ (ones(10,10)+Diagonal(ones(10)))/11
end
@testset "NNDist" begin
A = Float64[9 1 0 4 0 9 0 8 7 2; 3 6 4 4 0 6 4 2 5 4; 0 7 7 7 8 7 6 5 0 2; 5 6 8 9 5 5 5 1 7 9]
sg = neighborsimplices(A; r=0.05)
@test sg.G == Diagonal(trues(10))
S = simplices2kernelmatrix(sg)
@test S ≈ Diagonal(ones(10))
sg = neighborsimplices(A; r=0.225)
@test sg.G == Bool[1 0 0 0 0 0 0 0 1 0; 0 1 1 1 0 0 1 0 0 0; 0 1 1 1 1 0 1 0 0 1; 0 1 1 1 0 0 1 0 0 1; 0 0 1 0 1 0 1 0 0 0; 0 0 0 0 0 1 0 1 0 0; 0 1 1 1 1 0 1 0 0 1; 0 0 0 0 0 1 0 1 0 0; 1 0 0 0 0 0 0 0 1 1; 0 0 1 1 0 0 1 0 1 1]
S = simplices2kernelmatrix(sg)
@test S ≈ [1/2 0 0 0 0 0 0 0 1/4 1/12; 0 11/42 11/84 11/84 1/21 0 11/84 0 0 17/210; 0 11/84 52/105 23/140 11/84 0 26/105 0 1/30 4/35; 0 11/84 23/140 23/70 1/21 0 23/140 0 1/30 4/35; 0 1/21 11/84 1/21 11/42 0 11/84 0 0 1/21; 0 0 0 0 0 2/3 0 1/3 0 0; 0 11/84 26/105 23/140 11/84 0 52/105 0 1/30 4/35; 0 0 0 0 0 1/3 0 2/3 0 0; 1/4 0 1/30 1/30 0 0 1/30 0 17/30 7/60; 1/12 17/210 4/35 4/35 1/21 0 4/35 0 7/60 83/210]
sg = neighborsimplices(A; r=0.36)
@test sg.G == Bool[1 0 0 1 0 1 0 1 1 1; 0 1 1 1 1 1 1 1 1 1; 0 1 1 1 1 1 1 0 0 1; 1 1 1 1 1 1 1 1 1 1; 0 1 1 1 1 0 1 1 0 1; 1 1 1 1 0 1 1 1 1 1; 0 1 1 1 1 1 1 1 1 1; 1 1 0 1 1 1 1 1 1 0; 1 1 0 1 0 1 1 1 1 1; 1 1 1 1 1 1 1 0 1 1]
S = simplices2kernelmatrix(sg)
@test S ≈ [383/2310 13/220 31/990 383/4620 3/88 383/4620 13/220 199/2772 383/4620 1913/27720; 13/220 811/3465 1237/13860 811/6930 2551/27720 2749/27720 811/6930 2441/27720 161/1980 953/9240; 31/990 1237/13860 1237/6930 1237/13860 361/4620 1979/27720 1237/13860 557/9240 53/990 1237/13860; 383/4620 811/6930 1237/13860 976/3465 2551/27720 487/3960 811/6930 443/3960 1457/13860 391/3080; 3/88 2551/27720 361/4620 2551/27720 2551/13860 257/3465 2551/27720 437/6930 223/3960 361/4620; 383/4620 2749/27720 1979/27720 487/3960 257/3465 487/1980 2749/27720 1303/13860 1457/13860 6/55; 13/220 811/6930 1237/13860 811/6930 2551/27720 2749/27720 811/3465 2441/27720 161/1980 953/9240; 199/2772 2441/27720 557/9240 443/3960 437/6930 1303/13860 2441/27720 443/1980 1303/13860 97/990; 383/4620 161/1980 53/990 1457/13860 223/3960 1457/13860 161/1980 1303/13860 1457/6930 281/3080; 1913/27720 953/9240 1237/13860 391/3080 361/4620 6/55 953/9240 97/990 281/3080 391/1540]
sg = neighborsimplices(A; r=1.0)
@test sg.G == trues(10,10)
S = simplices2kernelmatrix(sg)
@test S ≈ (Diagonal(ones(10)) + ones(10,10))/11
end
@testset "kNNDist" begin
A = Float64[1 0 -9 0 0 -6 3 -3 -1 7; -1 0 -5 1 1 0 -6 0 0 -1; 0 -5 2 2 0.01 -1 -2 0 0 4; -4 -2 0 -7 -5 -4 0 0 -5 0.01]
sg = neighborsimplices(A; k=2, r=0.28)
@test sg.G == Bool[1 1 0 1 1 0 1 1 1 1; 1 1 0 0 0 0 1 0 0 0; 0 0 1 0 0 0 0 0 0 0; 1 0 0 1 1 0 0 0 1 0; 1 0 0 1 1 0 0 0 1 0; 0 0 1 0 0 1 0 1 1 0; 0 0 0 0 0 0 1 0 0 1; 1 0 1 0 0 1 0 1 1 0; 1 1 0 1 1 1 0 1 1 0; 0 0 0 0 0 0 0 0 0 1]
S = simplices2kernelmatrix(sg)
@test S ≈ [94/105 4/21 0 31/210 31/210 31/420 1/6 41/420 59/210 1/12; 4/21 8/21 0 1/42 1/42 0 1/12 1/42 3/28 0; 0 0 1/6 0 0 1/12 0 1/12 0 0; 31/210 1/42 0 31/105 31/210 1/42 0 1/21 31/210 0; 31/210 1/42 0 31/210 31/105 1/42 0 1/21 31/210 0; 31/420 0 1/12 1/42 1/42 101/210 0 101/420 11/70 0; 1/6 1/12 0 0 0 0 1/3 0 0 1/12; 41/420 1/42 1/12 1/21 1/21 101/420 0 37/70 19/105 0; 59/210 3/28 0 31/210 31/210 11/70 0 19/105 51/70 0; 1/12 0 0 0 0 0 1/12 0 0 1/6]
end
@testset "kNNDistReduced" begin
A = Float64[76 48 40 14 -15 7 -42 -57 -37 -34; 84 23 59 76 22 -8 -123 -44 -77 -12; -56 -28 36 -59 37 50 -21 8 23 10; -19 -59 -84 42 50 22 -5 38 -19 34; 33 56 -9 -97 56 2 -2 -44 -19 24; 36 98 -13 32 19 25 -81 53 -50 -119]
sg = neighborsimplices(A; k=2, r=0.32, dim=1)
@test sg.G == Bool[1 1 1 1 0 0 0 0 0 0; 1 1 1 1 0 0 0 0 0 0; 1 1 1 1 1 1 0 1 0 0; 1 1 1 1 1 1 0 0 0 0; 0 0 1 1 1 1 0 1 0 0; 0 0 1 1 1 1 0 1 0 0; 0 0 0 0 0 0 1 0 1 1; 0 0 1 0 1 1 0 1 1 1; 0 0 0 0 0 0 1 1 1 1; 0 0 0 0 0 0 1 1 1 1]
S = simplices2kernelmatrix(sg)
@test S ≈ [17/60 17/120 17/120 17/120 1/24 1/24 0 1/56 0 0; 17/120 17/60 17/120 17/120 1/24 1/24 0 1/56 0 0; 17/120 17/120 13/28 5/24 37/280 37/280 0 13/120 1/42 1/42; 17/120 17/120 5/24 5/12 13/120 13/120 0 71/840 0 0; 1/24 1/24 37/280 13/120 37/140 37/280 0 13/120 1/42 1/42; 1/24 1/24 37/280 13/120 37/280 37/140 0 13/120 1/42 1/42; 0 0 0 0 0 0 11/30 1/10 11/60 11/60; 1/56 1/56 13/120 71/840 13/120 13/120 1/10 5/12 13/105 13/105; 0 0 1/42 0 1/42 1/42 11/60 13/105 29/70 29/140; 0 0 1/42 0 1/42 1/42 11/60 13/105 29/140 29/70]
sg = neighborsimplices(A; k=2, r=0.42, dim=3)
@test sg.G == Bool[1 1 1 1 0 0 0 0 0 0; 1 1 1 0 1 0 0 0 0 0; 1 1 1 0 1 1 0 0 0 0; 0 0 0 1 0 0 0 0 0 0; 0 1 1 0 1 1 0 1 1 0; 0 0 1 1 1 1 0 1 1 0; 0 0 0 0 0 0 1 0 1 1; 0 0 0 0 1 1 0 1 0 0; 0 0 0 0 1 1 1 0 1 1; 0 0 0 0 0 0 1 0 1 1]
S = simplices2kernelmatrix(sg)
@test S ≈ [1/2 1/6 1/6 1/12 1/12 7/60 0 0 0 0; 1/6 8/21 4/21 0 3/28 2/35 0 1/42 1/42 0; 1/6 4/21 47/105 0 59/420 19/210 0 2/35 2/35 0; 1/12 0 0 1/6 0 1/12 0 0 0 0; 1/12 3/28 59/420 0 18/35 29/140 1/30 59/420 19/210 1/30; 7/60 2/35 19/210 1/12 29/140 61/105 1/30 59/420 19/210 1/30; 0 0 0 0 1/30 1/30 2/5 0 1/5 1/5; 0 1/42 2/35 0 59/420 59/420 0 59/210 2/35 0; 0 1/42 2/35 0 19/210 19/210 1/5 2/35 18/35 1/5; 0 0 0 0 1/30 1/30 1/5 0 1/5 2/5]
sg = neighborsimplices(A; k=2, r=0.45, dim=5)
@test sg.G == Bool[1 1 1 1 0 0 0 0 0 0; 1 1 0 0 0 0 0 0 0 0; 1 0 1 0 0 1 0 0 0 0; 0 0 0 1 0 0 0 0 0 0; 0 0 0 0 1 1 0 1 0 0; 0 1 1 0 1 1 0 1 1 0; 0 0 0 0 0 0 1 0 1 1; 0 0 0 1 1 1 0 1 1 0; 0 0 0 0 0 1 1 1 1 1; 0 0 0 0 0 0 1 0 1 1]
S = simplices2kernelmatrix(sg)
@test S ≈ [2/3 1/6 1/6 1/12 0 1/6 0 1/12 0 0; 1/6 1/3 1/12 0 0 1/12 0 0 0 0; 1/6 1/12 2/5 0 1/30 7/60 0 1/30 1/30 0; 1/12 0 0 1/6 0 0 0 1/12 0 0; 0 0 1/30 0 1/3 1/6 0 1/6 1/12 0; 1/6 1/12 7/60 0 1/6 11/15 1/30 1/5 7/60 1/30; 0 0 0 0 0 1/30 2/5 1/30 1/5 1/5; 1/12 0 1/30 1/12 1/6 1/5 1/30 17/30 7/60 1/30; 0 0 1/30 0 1/12 7/60 1/5 7/60 17/30 1/5; 0 0 0 0 0 1/30 1/5 1/30 1/5 2/5]
sg = neighborsimplices(A'; k=1, r=0.4, dim=1)
@test sg.G == Bool[1 1 0 0 1 1; 1 1 0 0 0 1; 0 0 1 1 1 0; 0 0 1 1 1 0; 1 0 1 1 1 1; 1 1 0 0 1 1]
S = simplices2kernelmatrix(sg)
@test S ≈ [13/30 11/60 1/30 1/30 2/15 13/60; 11/60 11/30 0 0 1/10 11/60; 1/30 0 2/5 1/5 1/5 1/30; 1/30 0 1/5 2/5 1/5 1/30; 2/15 1/10 1/5 1/5 3/5 2/15; 13/60 11/60 1/30 1/30 2/15 13/30]
sg = neighborsimplices(A'; k=1, r=0.45, dim=3)
@test sg.G == Bool[1 1 1 0 1 1; 1 1 0 0 0 0; 1 0 1 1 1 0; 0 0 1 1 0 0; 1 0 1 0 1 0; 1 0 0 0 0 1]
S = simplices2kernelmatrix(sg)
@test S ≈ [1/1 1/5 1/6 1/20 1/6 1/5; 1/5 2/5 1/30 0 1/30 1/30; 1/6 1/30 2/3 13/60 1/6 1/30; 1/20 0 13/60 13/30 1/20 0; 1/6 1/30 1/6 1/20 1/3 1/30; 1/5 1/30 1/30 0 1/30 2/5]
sg = neighborsimplices(A'; k=1, r=0.55, dim=5)
@test sg.G == Bool[1 1 1 0 1 1; 1 1 0 0 0 1; 1 0 1 1 1 0; 0 0 1 1 1 0; 1 0 1 1 1 0; 1 1 0 0 0 1]
S = simplices2kernelmatrix(sg)
@test S ≈ [3/5 1/5 2/15 1/10 2/15 1/5; 1/5 2/5 1/30 0 1/30 1/5; 2/15 1/30 13/30 11/60 13/60 1/30; 1/10 0 11/60 11/30 11/60 0; 2/15 1/30 13/60 11/60 13/30 1/30; 1/5 1/5 1/30 0 1/30 2/5]
end
@testset "NNGrouped" begin
A = Float64[76 48 40 14 -15 7 -42 -57 -37 -34; 84 23 59 76 22 -8 -123 -44 -77 -12; -56 -28 36 -59 37 50 -21 8 23 10; -19 -59 -84 42 50 22 -5 38 -19 34; 33 56 -9 -97 56 2 -2 -44 -19 24; 36 98 -13 32 19 25 -81 53 -50 -119]
annot = ["A","B","A","A","B","A","A","B","C","B"]
sg = neighborsimplices(A; k=1, groupby=annot)
simplexgraphcmp(sg, SimplexGraph(Bool[1 0 0 1 0 0 0 0 0 0; 0 1 0 0 0 0 0 0 0 0; 1 0 1 0 0 1 0 0 0 0; 0 0 0 1 0 0 0 0 0 0; 0 1 0 0 1 0 0 1 0 1; 0 0 1 0 0 1 1 0 0 0; 0 0 0 0 0 0 1 0 0 0; 0 0 0 0 1 0 0 1 0 0; 0 0 0 0 0 0 0 0 1 0; 0 0 0 0 0 0 0 0 0 1]))
sg = neighborsimplices(A; k=2, groupby=annot)
simplexgraphcmp(sg, SimplexGraph(Bool[1 0 1 1 0 1 0 0 0 0; 0 1 0 0 0 0 0 0 0 0; 1 0 1 0 0 1 1 0 0 0; 1 0 0 1 0 0 0 0 0 0; 0 1 0 0 1 0 0 1 0 1; 0 0 1 1 0 1 1 0 0 0; 0 0 0 0 0 0 1 0 0 0; 0 1 0 0 1 0 0 1 0 1; 0 0 0 0 0 0 0 0 1 0; 0 0 0 0 1 0 0 1 0 1]))
sg = neighborsimplices(A; k=4, groupby=annot)
simplexgraphcmp(sg, SimplexGraph(Bool[1 0 1 1 0 1 1 0 0 0; 0 1 0 0 1 0 0 1 0 1; 1 0 1 1 0 1 1 0 0 0; 1 0 1 1 0 1 1 0 0 0; 0 1 0 0 1 0 0 1 0 1; 1 0 1 1 0 1 1 0 0 0; 1 0 1 1 0 1 1 0 0 0; 0 1 0 0 1 0 0 1 0 1; 0 0 0 0 0 0 0 0 1 0; 0 1 0 0 1 0 0 1 0 1]))
sg = neighborsimplices(A; r=0.6, groupby=annot)
simplexgraphcmp(sg, SimplexGraph(Bool[1 0 1 1 0 1 0 0 0 0; 0 1 0 0 1 0 0 0 0 0; 1 0 1 0 0 1 0 0 0 0; 1 0 0 1 0 1 0 0 0 0; 0 1 0 0 1 0 0 1 0 1; 1 0 1 1 0 1 1 0 0 0; 0 0 0 0 0 1 1 0 0 0; 0 0 0 0 1 0 0 1 0 0; 0 0 0 0 0 0 0 0 1 0; 0 0 0 0 1 0 0 0 0 1]))
sg = neighborsimplices(A; r=2.0, groupby=annot)
simplexgraphcmp(sg, SimplexGraph(Bool[1 0 1 1 0 1 1 0 0 0; 0 1 0 0 1 0 0 1 0 1; 1 0 1 1 0 1 1 0 0 0; 1 0 1 1 0 1 1 0 0 0; 0 1 0 0 1 0 0 1 0 1; 1 0 1 1 0 1 1 0 0 0; 1 0 1 1 0 1 1 0 0 0; 0 1 0 0 1 0 0 1 0 1; 0 0 0 0 0 0 0 0 1 0; 0 1 0 0 1 0 0 1 0 1]))
end
end
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | code | 1635 | @testset "util" begin
A = Int[7 0 -1 0 -4 0 3; -9 4 2 -5 3 1 0; 0 0 2 0 1 -7 -2; 0 0 0 0 0 0 0; 2 2 2 2 2 2 2]
@testset "normalizemean" begin
ans = Float64[6.285714285714286 -0.7142857142857142 -1.7142857142857142 -0.7142857142857142 -4.714285714285714 -0.7142857142857142 2.2857142857142856; -8.428571428571429 4.571428571428571 2.571428571428571 -4.428571428571429 3.571428571428571 1.5714285714285714 0.5714285714285714; 0.8571428571428571 0.8571428571428571 2.857142857142857 0.8571428571428571 1.8571428571428572 -6.142857142857143 -1.1428571428571428; 0 0 0 0 0 0 0; 0 0 0 0 0 0 0]
B = normalizemean(A)
@test mean(B,dims=2) ≈ zeros(5,1) atol=1e-9
@test B ≈ ans
C = Float64.(A)
normalizemean!(C)
@test mean(C,dims=2) ≈ zeros(5,1) atol=1e-9
@test C ≈ ans
end
@testset "normalizemeanstd" begin
ans = Float64[1.8217730766638152 -0.20701966780270625 -0.496847202726495 -0.20701966780270625 -1.3663298074978614 -0.20701966780270625 0.6624629369686601; -1.785421221372967 0.9683640522700837 0.5447047794019221 -0.9381026756366437 0.7565344158360029 0.33287514296784126 0.12104550653376046; 0.28878657066713365 0.28878657066713365 0.962621902223779 0.28878657066713365 0.6257042364454564 -2.069637089781125 -0.38504876088951157; 0 0 0 0 0 0 0; 0 0 0 0 0 0 0]
B = normalizemeanstd(A)
@test mean(B,dims=2) ≈ zeros(5,1) atol=1e-9
@test std(B,dims=2).-[1,1,1,0,0] ≈ zeros(5,1) atol=1e-9
@test B ≈ ans
C = Float64.(A)
normalizemeanstd!(C)
@test mean(C,dims=2) ≈ zeros(5,1) atol=1e-9
@test std(C,dims=2).-[1,1,1,0,0] ≈ zeros(5,1) atol=1e-9
@test C ≈ ans
end
end
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | docs | 1755 | # PrincipalMomentAnalysis
[](https://principalmomentanalysis.github.io/PrincipalMomentAnalysis.jl/stable)
[](https://principalmomentanalysis.github.io/PrincipalMomentAnalysis.jl/dev)
[](https://travis-ci.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl)
[](https://ci.appveyor.com/project/PrincipalMomentAnalysis/PrincipalMomentAnalysis-jl)
[](https://codecov.io/gh/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl)
[](https://coveralls.io/github/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl?branch=master)
This is a Julia implementation of (Simplex) Principal Moment Analysis.
See also:
* [Principal Moment Analysis home page](https://principalmomentanalysis.github.io/).
* [Package documentation](https://principalmomentanalysis.github.io/PrincipalMomentAnalysis.jl/stable).
* [The Principal Moment Analysis App](https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysisApp.jl/).
## Installation
To install PrincipalMomentAnalysis.jl, start Julia and type:
```julia
using Pkg
Pkg.add("PrincipalMomentAnalysis")
```
Please refer to the [documentation](https://principalmomentanalysis.github.io/PrincipalMomentAnalysis.jl/stable) for more information.
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | docs | 1140 | # PrincipalMomentAnalysis.jl
PrincipalMomentAnalysis.jl provides an implementation of (Simplex) Principal Moment Analysis (PMA) as described [here](https://arxiv.org/abs/2003.04208).
If you want to cite this work, please use:
> [Fontes, M., & Henningsson, R. (2020). Principal Moment Analysis. arXiv arXiv:2003.04208.](https://arxiv.org/abs/2003.04208)
* Corresponding author: Magnus Fontes ([email protected])
* Julia implementation: Rasmus Henningsson ([email protected])
The Principal Moment Analysis source code is available at [GitHub](https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl).
## Principal Moment Analysis App
If you prefer to run Principal Moment Analysis using a GUI, check out the [Principal Moment Analysis App](https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysisApp.jl/).
## Installation
To install PrincipalMomentAnalysis.jl, start Julia and type:
```julia
using Pkg
Pkg.add("PrincipalMomentAnalysis")
```
## Source code
[The PrincipalMomentAnalysis.jl source code can be found here](https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl/).
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | docs | 183 | # Reference
```@docs
pma
PMA
normalizemean!
normalizemean
normalizemeanstd!
normalizemeanstd
SimplexGraph
groupsimplices
timeseriessimplices
neighborsimplices
neighborsimplices2
```
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.2.2 | 64fb1fa6b716299cd85513f0ed36e8612eee7827 | docs | 25 | # Tutorial
Coming soon!
| PrincipalMomentAnalysis | https://github.com/PrincipalMomentAnalysis/PrincipalMomentAnalysis.jl.git |
|
[
"MIT"
] | 0.1.0 | 65ed29fdaeaa926169e7d7a8be0b74dec60680db | code | 278 | using Documenter
using LiteHF
makedocs(
sitename = "LiteHF",
format = Documenter.HTML(),
modules = [LiteHF],
pages=[
"APIs" => "index.md",
"Tips & Recommendations" => "tips.md",
],
)
deploydocs(;
repo="github.com/JuliaHEP/LiteHF.jl",
)
| LiteHF | https://github.com/JuliaHEP/LiteHF.jl.git |
|
[
"MIT"
] | 0.1.0 | 65ed29fdaeaa926169e7d7a8be0b74dec60680db | code | 1009 | module LiteHF
using Distributions
import Random, Optim
# interpolations
# export InterpCode0, InterpCode1, InterpCode2, InterpCode4
# modifiers
export ExpCounts
export Normsys, Histosys, Normfactor, Lumi, Staterror, nmodifiers
# pyhf
export pyhf_loglikelihoodof, pyhf_logpriorof, pyhf_logjointof
export load_pyhfjson, build_pyhf, free_maximize, cond_maximize, PyHFModel,
AsimovModel, inits, observed, expected, priors, prior_names
# Test Statistics & Test Statistics Distributions
export T_tmu, T_tmutilde, T_q0, T_qmu, T_qmutilde
export asymptotic_dists, expected_pvalue
include("./interpolations.jl")
include("./modifiers.jl")
include("./pyhfparser.jl")
abstract type AbstractModel end
include("./pyhfmodel.jl")
include("./teststatistics.jl")
include("./asymptotictests.jl")
function _precompile_()
ccall(:jl_generating_output, Cint, ()) == 1 || return nothing
r = load_pyhfjson(joinpath(@__DIR__, "../test/pyhfjson/sample_lumi.json"))
M = build_pyhf(r)
end
_precompile_()
end
| LiteHF | https://github.com/JuliaHEP/LiteHF.jl.git |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.