licenses
sequencelengths 1
3
| version
stringclasses 677
values | tree_hash
stringlengths 40
40
| path
stringclasses 1
value | type
stringclasses 2
values | size
stringlengths 2
8
| text
stringlengths 25
67.1M
| package_name
stringlengths 2
41
| repo
stringlengths 33
86
|
---|---|---|---|---|---|---|---|---|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 3056 | #! /usr/bin/julia
# comments are allowed before YAML frontmatter
# ---
# title: Write your demo in julia
# id: juliademocard_example
# cover: assets/literate.png
# date: 2020-09-13
# author: "[Johnny Chen](https://github.com/johnnychen94)"
# julia: 1.0.1
# description: This demo shows you how to write your demo in julia
# ---
# Different from markdown format demo, source demo files are preprocessed so that it generates:
#
# 1. assets such as cover image
# 2. julia source file
# 3. mardkown file
# 4. jupyter notebook file
#
# Links to nbviewer and source files are provided as well.
# !!! note
# This entails every source file being executed three times: 1) asset generation, 2) notebook
# generation, and 3) Documenter HTML generation. If the generation time is too long for your
# application, you could then choose to write your demo in markdown format. Or you can disable
# the notebook generation via the `notebook` keyword in your demos, this helps reduce the
# runtime to 2x.
#
# The conversions from source demo files to `.jl`, `.md` and `.ipynb` files are mainly handled by
# [`Literate.jl`](https://github.com/fredrikekre/Literate.jl). The syntax to control/filter the
# outputs can be found [here](https://fredrikekre.github.io/Literate.jl/stable/fileformat/)
x = 1//3
y = 2//5
x + y
#-
# Images can be loaded and displayed
using TestImages, ImageShow
img = testimage("lena")
# The frontmatter is also written in YAML with only a leading `#`, for example:
# ```
# # ---
# # title: <title>
# # cover: <cover>
# # id: <id>
# # date: 2020-09-13
# # author: Johnny Chen
# # julia: 1.3
# # description: <description>
# # ---
# ```
# In addition to the keywords supported by markdown files, Julia format demos accept one extra
# frontmatter keyword: `julia`. It allows you to specify the compat version of your demo. With this,
# `DemoCards` would:
#
# - throw a warning if your demos are generated using a lower version of Julia
# - insert a compat version badge.
#
# The warning is something like "The running Julia version `1.0.5` is older than the declared
# compatible version `1.3.0`."
# Another extra keyword is `notebook`, it allows you to control whether you needs to generate
# jupyter notebook `.ipynb` for the demo. The valid values are `true` and `false`. See also
# [Override default values with properties](@ref page_sec_properties) on how to disable all notebook
# generation via the properties entry.
# !!! warning
# You should be careful about the leading whitespaces after the first `#`. Frontmatter as weird as the
# following is not guaranteed to work and it is very likely to hit a YAML parsing error.
# ```yaml
# #---
# # title: <title>
# # cover: <cover>
# # id: <id>
# # description: <description>
# #---
# ```
# !!! tip
# Comments are allowed before frontmatter, but it would only be appeared in the julia source
# codes. Normally, you may only want to add magic comments and license information before the
# YAML frontmatter.
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 4340 | # ---
# title: Generate your cover on the fly
# cover: assets/lena_color_256.png
# description: this demo shows you how to generate cover on the fly
# julia: 1.0.0
# ---
# There're many reasons that you don't want to mannually manage the cover image.
# DemoCards.jl allows you to generate the card cover on the fly for demos written
# in julia.
# Let's do this with a simple example
using TestImages, FileIO, ImageShow
## ImageIO backend such as ImageMagick is required
cover = testimage("lena_color_256")
save("assets/lena_color_256.png", cover)
# You can use this image later with `cover`, `# `
# , or you can directly write it in your frontmatter
cover
# ## Advanced Literate & Documenter tricks
#
# The following tricks are what you'd probably need to work on a real-world demo.
#
# ### Hide asset generation
#
# There are cases that you want to hide the codes related to asset generation, e.g., the
# `save(...)` line. Fortunately, this is doable by combining the syntax of Documenter and Literate.
# For example, the following code can be inserted to whereever you need to generate the assets.
# The [`#src` flag](https://fredrikekre.github.io/Literate.jl/v2/fileformat/#Filtering-Lines) is
# used as a filter to tell Literate that this line is reserved only in the original source codes.
#
# ```julia
# save("assets/cover.png", img_noise) #src #md
# ```
#
# Gif image is also supported via `ImageMagick`:
#
# ```julia
# # --- save covers --- #src #md
# using ImageMagick #src #md
# imgs = map(10:5:50) do k #src #md
# colorview(RGB, rank_approx.(svdfactors, k)...) #src #md
# end #src #md
# ImageMagick.save("assets/color_separations_svd.gif", cat(imgs...; dims=3); fps=2) #src #md
# ```
#
# ### Hide the output result
#
# It does not look good to show the `0` result in the above code block as it does not provide
# anything that the reader cares. There are two ways to fix this.
#
# The first way is to insert a [`#hide`
# flag](https://juliadocs.github.io/Documenter.jl/stable/man/syntax/#@example-block) to the line you
# don't want the user see. For example, if you write your source file in this way
#
# ```julia
# cover = testimage("lena_color_256")
# save("assets/lena_color_256.png", cover) #hide
# ```
#
# it will show up in the generated HTML page as
cover = testimage("lena_color_256")
save("assets/lena_color_256.png", cover) #hide
# The return value is still `0`. Sometimes it is wanted, sometime it is not. To also hide the return
# value `0`, you could insert a trivial `nothing #hide #md` to work it around.
#
# ```julia
# cover = testimage("lena_color_256")
# save("assets/lena_color_256.png", cover) #hide #md
# nothing #hide #md #md
# ```
#
# generates
cover = testimage("lena_color_256")
save("assets/lena_color_256.png", cover) #hide
nothing #hide #md
# No output at all. You could, of course, insert `cover #hide #md` or others you would like readers
# to see:
#
#
# ```julia
# cover = testimage("lena_color_256")
# save("assets/lena_color_256.png", cover) #hide #md #md
# cover #hide #md #md
# ```
#
# generates
cover = testimage("lena_color_256")
save("assets/lena_color_256.png", cover) #hide #md
cover #hide #md
# The `#md` flag is used to keep a clean `.jl` file provided by the download-julia badge:
# 
# ### Be careful about the spaces and identation
#
# Both Documenter and Literate has specifications on spaces, so you'd probably hit this issue sooner
# or later.
#
# Let's say, you want to create a `warning` box using the `!!! warning` syntax, and you'd probably
# ends up with something like this:
#
# ```text
# # !!! warning
# # This is inside the warning box
# #
# # This is out side the warning box
# ```
#
# !!! warning
# This is inside the warning box
#
# This is out side the warning box
#
# ---
#
# The reason that contents are not shown correctly inside the warning box is that you need to insert
# *five* (not four) spaces to the following lines: `Literate` consumes one space, and `Documenter`
# consumes the other four.
#
# ```diff
# # !!! warning
# -# This is inside the warning box
# +# This is inside the warning box
# #
# # This is out side the warning box
# ```
#
# !!! warning
# This is inside the warning box
#
# This is out side the warning box
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 80 | # ---
# cover: ../../assets/logo.svg
# description: some description here
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 80 | # ---
# cover: ../../assets/logo.svg
# description: some description here
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 80 | # ---
# cover: ../../assets/logo.svg
# description: some description here
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 80 | # ---
# cover: ../../assets/logo.svg
# description: some description here
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 80 | # ---
# cover: ../../assets/logo.svg
# description: some description here
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 80 | # ---
# cover: ../../assets/logo.svg
# description: some description here
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 80 | # ---
# cover: ../../assets/logo.svg
# description: some description here
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 80 | # ---
# cover: ../../assets/logo.svg
# description: some description here
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 80 | # ---
# cover: ../../assets/logo.svg
# description: some description here
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 80 | # ---
# cover: ../../assets/logo.svg
# description: some description here
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 80 | # ---
# cover: ../../assets/logo.svg
# description: some description here
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 80 | # ---
# cover: ../../assets/logo.svg
# description: some description here
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 4013 | module PlutoNotebook
using Dates
using DemoCards: AbstractDemoCard, JULIA_COMPAT, load_config, download_badge, pluto_footer
import DemoCards: PlutoDemoCard, save_democards, make_badges, parse
isdefined(Base, :get_extension) ? (using PlutoStaticHTML) : (using ..PlutoStaticHTML)
function PlutoDemoCard(path::AbstractString)::PlutoDemoCard
# first consturct an incomplete democard, and then load the config
card = PlutoDemoCard(path, "", "", "", "", "", DateTime(0), JULIA_COMPAT, false)
config = parse(card)
card.cover = load_config(card, "cover"; config = config)
card.title = load_config(card, "title"; config = config)
card.date = load_config(card, "date"; config = config)
card.author = load_config(card, "author"; config = config)
card.julia = load_config(card, "julia"; config = config)
# default id requires a title
card.id = load_config(card, "id"; config = config)
# default description requires a title
card.description = load_config(card, "description"; config = config)
card.hidden = load_config(card, "hidden"; config = config)
return card
end
"""
save_democards(card_dir::AbstractString, card::PlutoDemoCard;
project_dir,
src,
credit,
nbviewer_root_url)
Process the original pluto notebook and saves it.
The processing pipeline is:
1. preprocess and copy source file
2. generate markdown file
3. copy the markdown file to cache folder
4. insert header and footer to generated markdown file
"""
function save_democards(
card_dir::AbstractString,
card::PlutoDemoCard;
credit,
nbviewer_root_url,
project_dir = Base.source_dir(),
src = "src",
throw_error = false,
properties = Dict{String,Any}(),
kwargs...,
)
if !isabspath(card_dir)
card_dir = abspath(card_dir)
end
isdir(card_dir) || mkpath(card_dir)
@debug card.path
# copy to card dir and do things
cardname = splitext(basename(card.path))[1]
# pluto outputs are expensive, we save the output to a cache dir
# these cache dir contains the render files from previous runs,
# saves time, while rendering
render_dir = joinpath(project_dir, "pluto_output") |> abspath
isdir(render_dir) || mkpath(render_dir)
nb_path = joinpath(card_dir, "$(cardname).jl")
md_path = joinpath(card_dir, "$(cardname).md")
cp(card.path, nb_path)
if VERSION < card.julia
# It may work, it may not work; I hope it would work.
@warn "The running Julia version `$(VERSION)` is older than the declared compatible version `$(card.julia)`. You might need to upgrade your Julia."
end
oopts = OutputOptions(; append_build_context = false)
output_format = documenter_output
bopts = BuildOptions(card_dir; previous_dir = render_dir, output_format = output_format)
# don't run notebooks in parallel
# TODO: User option to run it parallel or not
build_notebooks(bopts, ["$(cardname).jl"], oopts)
# move rendered files to cache
cache_path = joinpath(render_dir, basename(md_path))
cp(md_path, cache_path; force = true)
badges = make_badges(
card;
src = src,
card_dir = card_dir,
nbviewer_root_url = nbviewer_root_url,
project_dir = project_dir,
build_notebook = false,
)
header = "# [$(card.title)](@id $(card.id))\n"
footer = pluto_footer
body = join(readlines(md_path), "\n")
write(md_path, header, badges * "\n\n", body, footer)
return nothing
end
function make_badges(
card::PlutoDemoCard;
src,
card_dir,
nbviewer_root_url,
project_dir,
build_notebook,
)
cardname = splitext(basename(card.path))[1]
badges = []
push!(badges, "[]($(cardname).jl)")
push!(badges, invoke(make_badges, Tuple{AbstractDemoCard}, card))
join(badges, " ")
end
parse(card::PlutoDemoCard) = PlutoStaticHTML.Pluto.frontmatter(card.path)
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 1648 | module DemoCards
import Base: basename
using Dates
using Mustache
using Literate
using ImageCore
using FileIO, JSON, YAML
using Suppressor # suppress log generated by 3rd party tools, e.g., Literate
import HTTP
using Documenter
const JULIA_COMPAT = let regex=r"julia\s*=\s*\"([\d\.]*)\""
lines = filter(readlines(normpath(@__DIR__, "..", "Project.toml"))) do line
occursin(regex, line)
end
VersionNumber(match(regex, lines[1]).captures[1])
end
const config_filename = "config.json"
const template_filename = "index.md"
# directly copy these folders without processing
const ignored_dirnames = ["assets"]
function verbose_mode()
if haskey(ENV, "DOCUMENTER_DEBUG")
rst = ENV["DOCUMENTER_DEBUG"]
return lowercase(strip(rst)) == "true"
else
return false
end
end
include("compat.jl")
include("types/card.jl")
include("types/section.jl")
include("types/page.jl")
include("utils.jl")
include("show.jl")
include("Themes/Themes.jl")
using .CardThemes
include("generate.jl")
include("preview.jl")
export makedemos, cardtheme, preview_demos
if !isdefined(Base, :get_extension)
using Requires
end
function __init__()
@static if !isdefined(Base, :get_extension)
@require PlutoStaticHTML = "359b1769-a58e-495b-9770-312e911026ad" include("../ext/PlutoNotebook.jl")
end
end
"""
This package breaks the rendering of the whole demo page into three types:
* `DemoPage` contains serveral `DemoSection`s;
* `DemoSection` contains either serveral `DemoSection`s or serveral `DemoCard`s;
* `DemoCard` consists of cover image, title and other necessary information.
"""
DemoCards
end # module
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 132 | if v"1.0" <= VERSION < v"1.1"
isnothing(x) = x===nothing
end
if VERSION < v"1.4"
pkgdir(pkg) = abspath(@__DIR__, "..")
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 17328 | """
makedemos(source::String;
root = "<current-directory>",
src = "src",
build = "build",
branch = "gh-pages",
edit_branch = "master",
credit = true,
throw_error = false) -> page_path, postprocess_cb
Make a demo page for `source` and return the path to the generated index file.
Processing pipeline:
1. analyze the folder structure `source` and loading all available configs.
2. copy assets
3. preprocess demo files and save it
4. save/copy cover images
5. generate postprocess callback function, which includes url-redirection.
!!! warning
By default, `makedemos` generates all the necessary files to `docs/src/`, this means that the
data you pass to `makedemos` should not be placed at `docs/src/`. A recommendation is to
put folders in `docs/`. For example, `docs/quickstart` is a good choice.
# Inputs
* `source`: dir path to the root page of your demos; relative to `docs`.
# Outputs
* `page_path`: path to demo page's index. You can directly pass it to `makedocs`.
* `postprocess_cb`: callback function for postprocess. You can call `postprocess_cb()` _after_
`makedocs`.
* `theme_assets`: the stylesheet assets that you may need to pass to `Documenter.HTML`. When the
demo page has no theme, it will return `nothing`.
# Keywords
* `root::String`: should be equal to `Documenter`'s setting. Typically it is `"docs"` if this
function is called in `docs/make.jl` file.
* `src::String`: should be equal to `Documenter`'s setting. By default it's `"src"`.
* `build::String`: should be equal to `Documenter`'s setting. By default it's `"build"`.
* `edit_branch::String`: should be equal to `Documenter`'s setting. By default it's `"master"`.
* `branch::String`: should be equal to `Documenter`'s setting. By default it's `"gh-pages"`.
* `credit::Bool`: `true` to show a "This page is generated by ..." info. By default it's `true`.
* `throw_error::Bool`: `true` to throw an error when the julia demo build fails; otherwise it will
continue the build with warnings.
* `filter_function::Function`: the function is passed each potential `DemoCard`, and the
card is excluded if the function returns false. By default, all potential cards are included.
# Examples
The following is the simplest example for you to start with:
```julia
# 1. preprocess and generate demo files to docs/src
examples, postprocess_cb, demo_assets = makedemos("examples")
assets = []
isnothing(demo_assets) || (push!(assets, demo_assets))
# 2. do the standard Documenter pipeline
makedocs(format = Documenter.HTML(assets = assets),
pages = [
"Home" => "index.md",
examples
])
# 3. postprocess after makedocs
postprocess_cb()
```
By default, it won't generate the index page for your demos. To enable it and configure how index
page is generated, you need to create "examples/config.json", whose contents should looks like the
following:
```json
{
"theme": "grid",
"order": [
"basic",
"advanced"
]
}
```
For more details of how `config.json` is configured, please check [`DemoCards.DemoPage`](@ref).
"""
function makedemos(source::String, templates::Union{Dict, Nothing} = nothing;
root::String = Base.source_dir(),
src::String = "src",
build::String = "build",
branch::String = "gh-pages",
edit_branch::String = "master",
credit = true,
throw_error = false,
filter_function = x -> true)
if !(basename(pwd()) == "docs" || basename(root) == "docs" || root == preview_build_dir())
# special cases that warnings are not printed:
# 1. called from `docs/make.jl`
# 2. called from `preview_demos`
# 3. pwd() in `docs` -- REPL
@warn "Suspicious `root` setting: typically `makedemos` should be called from `docs/make.jl`. " pwd=pwd() root
end
root = abspath(root)
page_root = abspath(root, source)
if page_root == source
# reach here when source is absolute path
startswith(source, root) || throw(ArgumentError("invalid demo page source $page_root"))
end
page = DemoPage(page_root, filter_function)
relative_root = basename(page)
# Where files are generated by DemoCards for Documenter.makedocs
# e.g "$PROJECT_ROOT/docs/src/quickstart"
absolute_root = abspath(root, src, relative_root)
if page_root == absolute_root
throw(ArgumentError("Demo page $page_root should not be placed at \"docs/$src\" folder."))
end
config_file = joinpath(page.root, config_filename)
if !isnothing(templates)
Base.depwarn(
"It is not recommended to pass `templates`, please configure the index theme in the config file $(config_file). For example `\"theme\": \"grid\"`",
:makedemos)
end
if !isnothing(page.theme)
page_templates, theme_assets = cardtheme(page.theme; root = root, src = src)
theme_assets = something(page.stylesheet, theme_assets)
if templates != page_templates && templates != nothing
@warn "use configured theme from $(config_file)"
end
templates = page_templates
else
theme_assets = page.stylesheet
end
# we can directly pass it to Documenter.makedocs
if isnothing(templates)
out_path = walkpage(page; flatten=false) do item
splitext(joinpath(relative_root, relpath(item.path, page_root)))[1] * ".md"
end
else
out_path = joinpath(relative_root, "index.md")
end
@info "SetupDemoCardsDirectory: setting up \"$(source)\" directory."
if isdir(absolute_root)
# a typical and probably safe case -- that we're still in docs/ folder
trigger_prompt = isinteractive() && (
!endswith(dirname(absolute_root), joinpath("docs", "src")) &&
!(haskey(ENV, "GITLAB_CI") || haskey(ENV, "GITHUB_ACTIONS"))
)
@info "Deleting folder $absolute_root"
if trigger_prompt
@warn "DemoCards build dir $absolute_root already exists.\nThis should only happen when you are using DemoCards incorrectly."
# Should never reach this in CI environment
clean_builddir = input_bool("It might contain important data, do you still want to remove it?")
else
clean_builddir = true
end
if clean_builddir
@info "Remove DemoCards build dir: $absolute_root"
# Ref: https://discourse.julialang.org/t/find-what-has-locked-held-a-file/23278/2
Base.Sys.iswindows() && GC.gc()
rm(absolute_root; force=true, recursive=true)
else
error("Stopped demos build.")
end
end
mkpath(absolute_root)
try
# hard coded "covers" should be consistant to card template
isnothing(templates) || mkpath(joinpath(absolute_root, "covers"))
# make a copy before pipeline because `save_democards` modifies card path
source_files = [x.path for x in walkpage(page)[2]]
# pipeline
# for themeless version, we don't need to generate covers and index page
copy_assets(absolute_root, page)
# WARNING: julia cards are reconfigured here
save_democards(absolute_root, page;
project_root = root,
src = src,
credit = credit,
nbviewer_root_url = get_nbviewer_root_url(branch),
throw_error=throw_error)
isnothing(templates) || save_cover(joinpath(absolute_root, "covers"), page)
isnothing(templates) || generate(joinpath(absolute_root, "index.md"), page, templates)
# pipeline: generate postprocess callback function
postprocess_cb = ()->begin
@info "Clean up DemoCards build dir: \"$source\""
# Ref: https://discourse.julialang.org/t/find-what-has-locked-held-a-file/23278/2
Base.Sys.iswindows() && GC.gc()
rm(absolute_root; force=true, recursive=true)
if !isnothing(theme_assets)
assets_path = abspath(root, src, theme_assets)
# Ref: https://discourse.julialang.org/t/find-what-has-locked-held-a-file/23278/2
Base.Sys.iswindows() && GC.gc()
rm(assets_path, force=true)
if isdir(dirname(assets_path))
if(isempty(readdir(dirname(assets_path))))
# Ref: https://discourse.julialang.org/t/find-what-has-locked-held-a-file/23278/2
Base.Sys.iswindows() && GC.gc()
rm(dirname(assets_path))
end
end
end
end
return out_path, postprocess_cb, theme_assets
catch err
# clean up build dir if anything wrong happens here so that we _hopefully_ never trigger the
# user prompt logic before the build process.
# Ref: https://discourse.julialang.org/t/find-what-has-locked-held-a-file/23278/2
Base.Sys.iswindows() && GC.gc()
rm(absolute_root; force=true, recursive=true)
@error "Errors when building demo dir" pwd=pwd() source root src
rethrow(err)
end
end
function generate(file::String, page::DemoPage, args...)
check_ext(file, :markdown)
open(file, "w") do f
generate(f, page::DemoPage, args...)
end
end
generate(io::IO, page::DemoPage, args...) = write(io, generate(page, args...))
function generate(page::DemoPage, templates)
items = Dict("democards" => generate(page.sections, templates; properties=page.properties))
Mustache.render(page.template, items)
end
function generate(cards::AbstractVector{<:AbstractDemoCard}, template; properties=Dict{String, Any}())
# for those hidden cards, only generate the necessary assets and files, but don't add them into
# the index.md page
foreach(filter(x->x.hidden, cards)) do x
generate(x, template; properties=properties)
end
mapreduce(*, filter(x->!x.hidden, cards); init="") do x
generate(x, template; properties=properties)
end
end
function generate(secs::AbstractVector{DemoSection}, templates; level=1, properties=Dict{String, Any}())
mapreduce(*, secs; init="") do x
properties = merge(properties, x.properties) # sec.properties has higher priority
generate(x, templates; level=level, properties=properties)
end
end
function generate(sec::DemoSection, templates; level=1, properties=Dict{String, Any}())
header = if length(sec.title) > 0
repeat("#", level) * " " * sec.title
else
""
end * '\n'
footer = '\n'
properties = merge(properties, sec.properties) # sec.properties has higher priority
# either cards or subsections are empty
# recursively generate the page contents
if isempty(sec.cards)
body = generate(sec.subsections, templates; level=level+1, properties=properties)
else
items = Dict(
"cards" => generate(sec.cards, templates["card"], properties=properties),
"description" => sec.description
)
body = Mustache.render(templates["section"], items)
end
header * body * footer
end
function generate(card::AbstractDemoCard, template; properties=Dict{String, Any})
covername = get_covername(card)
if isnothing(covername)
# `generate` are called after `save_cover`, we assume that this default cover file is
# already generated
coverpath = "covers/" * basename(get_logopath())
else
coverpath = is_remote_url(card.cover) ? covername : "covers/" * covername
end
description = card.description
cut_idx = 500
if length(card.description) >= cut_idx
# cut descriptions into ~500 characters
offset = findfirst(' ', description[cut_idx:end])
offset === nothing && (offset = 0)
offset = cut_idx + offset - 2
description = description[1:cut_idx] * "..."
end
items = Dict(
"coverpath" => coverpath,
"id" => card.id,
"title" => card.title,
"description" => description,
)
Mustache.render(template, items)
end
### save demo card covers
save_cover(path::String, page::DemoPage) = save_cover.(path, page.sections)
function save_cover(path::String, sec::DemoSection)
save_cover.(path, sec.subsections)
save_cover.(path, sec.cards)
end
"""
save_cover(path::String, card::AbstractDemoCard)
process the cover image and save it.
"""
function save_cover(path::String, card::AbstractDemoCard)
covername = get_covername(card)
if isnothing(covername)
default_coverpath = get_logopath()
cover_path = joinpath(path, basename(default_coverpath))
!isfile(cover_path) && cp(default_coverpath, cover_path)
# now card uses default cover file as a fallback
card.cover = basename(default_coverpath)
return nothing
end
is_remote_url(card.cover) && return nothing
# only save cover image if it is a existing local file
src_path = joinpath(dirname(card.path), card.cover)
if !isfile(src_path)
@warn "cover file doesn't exist" cover_path=src_path
# reset it back to nothing and fallback to use default cover
card.cover = nothing
save_cover(path, card)
else
cover_path = joinpath(path, covername)
if isfile(cover_path)
@warn "cover file already exists, perhaps you have demos of the same filename" cover_path
end
!isfile(cover_path) && cp(src_path, cover_path)
end
end
function get_covername(card::AbstractDemoCard)
isnothing(card.cover) && return nothing
is_remote_url(card.cover) && return card.cover
default_covername = basename(get_logopath())
card.cover == default_covername && return default_covername
return splitext(basename(card))[1] * splitext(card.cover)[2]
end
get_logopath() = joinpath(pkgdir(DemoCards), "assets", "democards_logo.svg")
### save markdown files
"""
save_democards(root::String, page::DemoPage; credit, nbviewer_root_url)
recursively process and save source demo file
"""
function save_democards(root::String, page::DemoPage; kwargs...)
@debug page.root
save_democards.(root, page.sections; properties=page.properties, kwargs...)
end
function save_democards(root::String, sec::DemoSection; properties, kwargs...)
@debug sec.root
properties = merge(properties, sec.properties) # sec.properties has higher priority
save_democards.(joinpath(root, basename(sec.root)), sec.subsections;
properties=properties, kwargs...)
save_democards.(joinpath(root, basename(sec.root)), sec.cards;
properties=properties, kwargs...)
end
### copy assets
function copy_assets(path::String, page::DemoPage)
_copy_assets(dirname(path), page.root)
copy_assets.(path, page.sections)
end
function copy_assets(path::String, sec::DemoSection)
_copy_assets(path, sec.root)
copy_assets.(joinpath(path, basename(sec.root)), sec.subsections)
end
function _copy_assets(dest_root::String, src_root::String)
# copy assets of this section
assets_dirs = filter(x->isdir(x)&&(basename(x) in ignored_dirnames),
joinpath.(src_root, readdir(src_root)))
map(assets_dirs) do src
dest = joinpath(dest_root, basename(src_root), basename(src))
mkpath(dest)
cp(src, dest; force=true)
end
end
# modified from https://github.com/fredrikekre/Literate.jl to replace the use of @__NBVIEWER_ROOT_URL__
function get_nbviewer_root_url(branch)
if haskey(ENV, "HAS_JOSH_K_SEAL_OF_APPROVAL") # Travis CI
repo_slug = get(ENV, "TRAVIS_REPO_SLUG", "unknown-repository")
deploy_folder = if get(ENV, "TRAVIS_PULL_REQUEST", nothing) == "false"
tag = ENV["TRAVIS_TAG"]
isempty(tag) ? "dev" : tag
else
"previews/PR$(get(ENV, "TRAVIS_PULL_REQUEST", "##"))"
end
return "https://nbviewer.jupyter.org/github/$(repo_slug)/blob/$(branch)/$(deploy_folder)"
elseif haskey(ENV, "GITHUB_ACTIONS")
repo_slug = get(ENV, "GITHUB_REPOSITORY", "unknown-repository")
deploy_folder = if get(ENV, "GITHUB_EVENT_NAME", nothing) == "push"
if (m = match(r"^refs\/tags\/(.*)$", get(ENV, "GITHUB_REF", ""))) !== nothing
String(m.captures[1])
else
"dev"
end
elseif (m = match(r"refs\/pull\/(\d+)\/merge", get(ENV, "GITHUB_REF", ""))) !== nothing
"previews/PR$(m.captures[1])"
else
"dev"
end
return "https://nbviewer.jupyter.org/github/$(repo_slug)/blob/$(branch)/$(deploy_folder)"
elseif haskey(ENV, "GITLAB_CI")
if (url = get(ENV, "CI_PROJECT_URL", nothing)) !== nothing
cfg["repo_root_url"] = "$(url)/blob/$(devbranch)"
end
if (url = get(ENV, "CI_PAGES_URL", nothing)) !== nothing &&
(m = match(r"https://(.+)", url)) !== nothing
return "https://nbviewer.jupyter.org/urls/$(m[1])"
end
end
return ""
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 9025 | """
preview_demos(demo_path; kwargs...)
Generate a docs preview for a single demo card.
# Return values
* `index_file`: absolute path to the index file of the demo page. You can open this in your browser
and see the generated demos.
# Arguments
* `demo_path`: path to your demo file or folder. It can be path to the demo file, the folder of
multiple scripts. If standard demo page folder structure is detected, use it, and otherwise will
create a preview version of it.
# Parameters
* `theme`: the card theme you want to use in the preview. By default, it infers from your page
config file. To not generate index page, you could pass `nothing` to it. See also
[`cardtheme`](@ref DemoCards.cardtheme) for theme choices.
* `assets = String[]`: this is passed to `Documenter.HTML`
* `edit_branch = "master"`: same to that in `makedemos`
* `credit = true`: same to that in `makedemos`
* `throw_error = false`: same to that in `makedemos`
* `require_html = true`: if it needs to trigger the Documenter workflow and generate HTML preview.
If set to `false`, the return value is then the path to the generated `index.md` file. This is an
experimental keyword and should not be considered stable.
* `clean = true`: whether you need to first clean up the existing sandbox building dir.
"""
function preview_demos(demo_path::String;
theme = missing,
edit_branch = "master",
assets = String[],
credit = true,
throw_error = false,
require_html = true,
clean = true)
# hard code these args in a sandbox environment -- there's no need for customization
build = "build"
src = "src"
demo_path = abspath(rstrip(demo_path, ['/', '\\']))
ispath(demo_path) || throw(ArgumentError("demo path does not exists: $demo_path"))
build_dir = preview_build_dir()
if clean
# Ref: https://discourse.julialang.org/t/find-what-has-locked-held-a-file/23278/2
Base.Sys.iswindows() && GC.gc()
rm(build_dir; force=true, recursive=true)
mkpath(build_dir)
end
page_dir = generate_or_copy_pagedir(demo_path, build_dir)
copy_assets_and_configs(page_dir, build_dir)
cd(build_dir) do
page = @suppress_err DemoPage(page_dir)
if theme === missing
theme = page.theme
else
if page.theme != theme
@info "Detect themes in both page config file and keyword `theme`, use \"$(string(theme))\"" config=string(page.theme) keyword=string(theme)
end
end
if isnothing(theme)
card_templates = nothing
else
card_templates, card_theme = cardtheme(theme; root = build_dir, src = src)
push!(assets, something(page.stylesheet, card_theme))
end
page_index = joinpath(src, "index.md")
mkpath(src)
open(page_index, "w") do io
println(io, "# Index page")
end
demos, demos_cb = makedemos(
basename(page_dir), card_templates;
root = build_dir,
src = src,
build = build,
edit_branch = edit_branch,
credit = credit,
throw_error = throw_error,
)
# In cases that addtional Documenter pipeline is not needed
# This is mostly for test usage right now and might be changed if there is better solution
require_html || return abspath(build_dir, src, basename(page_dir))
format = Documenter.HTML(
edit_link = edit_branch,
prettyurls = get(ENV, "CI", nothing) == "true",
assets = assets
)
makedocs(
root=build_dir,
source=src,
format=format,
pages=[
"Index" => relpath(page_index, src),
demos
],
sitename="DemoCards Preview",
remotes=nothing
)
demos_cb()
return abspath(build, "index.html")
end
end
# A common workflow when previewing demos is to directly refresh the browser this requires us to use
# the same build dir, otherwise, user might be confused why the contents are not updated.
const _preview_build_dir = String[]
function preview_build_dir()
if isempty(_preview_build_dir)
build_dir = mktempdir()
push!(_preview_build_dir, build_dir)
end
return _preview_build_dir[1]
end
"""
generate_or_copy_pagedir(src_path, build_dir)
copy the page folder structure from `src_path` to `build_dir/\$(basename(src_path)`. If `src_path`
does not live in a standard demo page folder structure, generate a preview version.
"""
function generate_or_copy_pagedir(src_path, build_dir)
ispath(src_path) || throw(ArgumentError("$src_path does not exists"))
if is_democard(src_path)
card_path = src_path
page_dir = infer_pagedir(card_path)
if isnothing(page_dir)
page_dir = abspath(build_dir, "preview_page")
dst_card_path = abspath(page_dir, "preview_section", basename(card_path))
else
dst_card_path = abspath(build_dir, relpath(card_path, dirname(page_dir)))
end
mkpath(dirname(dst_card_path))
cp(card_path, dst_card_path; force=true)
elseif is_demosection(src_path)
sec_dir = src_path
page_dir = infer_pagedir(sec_dir)
if isnothing(page_dir)
page_dir = abspath(build_dir, "preview_page")
dst_sec_dir = abspath(page_dir, basename(sec_dir))
else
dst_sec_dir = abspath(build_dir, relpath(sec_dir, dirname(page_dir)))
end
mkpath(dirname(dst_sec_dir))
cp(sec_dir, dst_sec_dir; force=true)
elseif is_demopage(src_path)
page_dir = src_path
cp(page_dir, abspath(build_dir, basename(page_dir)); force=true)
else
throw(ArgumentError("failed to parse demo page structure from path: $src_path. There might be some invalid demo files."))
end
return page_dir
end
"""
copy_assets_and_configs(src_page_dir, dst_build_dir=pwd())
copy only assets, configs and templates from `src_page_dir` to `dst_build_dir`. The folder structure
is preserved under `dst_build_dir/\$(basename(src_page_dir))`
`order` in config files are modified accordingly.
"""
function copy_assets_and_configs(src_page_dir, dst_build_dir=pwd())
(isabspath(src_page_dir) && isdir(src_page_dir)) || throw(ArgumentError("src_page_dir is expected to be absolute folder path: $src_page_dir"))
for (root, dirs, files) in walkdir(src_page_dir)
assets_dirs = intersect(ignored_dirnames, dirs)
if !isempty(assets_dirs)
for assets in assets_dirs
src_assets = abspath(root, assets)
dst_assets_path = abspath(dst_build_dir, relpath(src_assets, dirname(src_page_dir)))
dst_sec_dir = dirname(dst_assets_path)
# only copy assets when there are section or card entries
if isdir(dst_sec_dir)
entry_names = filter(readdir(dst_sec_dir)) do x
x = joinpath(root, x)
is_democard(x) || is_demosection(x)
end
if !isempty(entry_names)
ispath(dst_assets_path) || cp(src_assets, dst_assets_path; force=true)
end
end
end
end
if template_filename in files
src_template_path = abspath(root, template_filename)
dst_template_path = abspath(dst_build_dir, relpath(src_template_path, dirname(src_page_dir)))
mkpath(dirname(dst_template_path))
ispath(dst_template_path) || cp(src_template_path, dst_template_path)
end
end
# modify and copy config.json after the folder structure is already set up
for (root, dirs, files) in walkdir(src_page_dir)
if config_filename in files
src_config_path = abspath(root, config_filename)
dst_config_path = abspath(dst_build_dir, relpath(src_config_path, dirname(src_page_dir)))
mkpath(dirname(dst_config_path))
config = JSON.parsefile(src_config_path)
config_dir = dirname(dst_config_path)
if haskey(config, "order")
order = [x for x in config["order"] if x in readdir(config_dir)]
if isempty(order)
delete!(config, "order")
else
config["order"] = order
end
end
if !isempty(config)
# Ref: https://discourse.julialang.org/t/find-what-has-locked-held-a-file/23278/2
Base.Sys.iswindows() && GC.gc()
open(dst_config_path, "w") do io
JSON.print(io, config)
end
end
end
end
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 780 | import Base: show
const indent_spaces = " "
function show(io::IO, card::AbstractDemoCard)
println(io, basename(card))
end
function show(io::IO, sec::DemoSection; level=1)
print(io, repeat(indent_spaces, level-1))
println(io, repeat("#", level), " ", sec.title)
# a section either holds cards or subsections
# here it's a mere coincident to show cards first
foreach(sec.cards) do x
print(io, repeat(indent_spaces, level))
show(io, x)
end
foreach(x->show(io, x; level=level+1), sec.subsections)
end
function show(io::IO, page::DemoPage)
page_root = replace(page.root, "\\" => "/")
println(io, "DemoPage(\"", page_root, "\"):\n")
println(io, "# ", page.title)
foreach(x->show(io, x; level=2), page.sections)
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 12448 | function validate_file(path, filetype = :text)
isfile(path) || throw(ArgumentError("$(path) is not a valid file"))
check_ext(path, filetype)
end
function check_ext(path, filetype = :text)
_, ext = splitext(path)
if filetype == :text
return true
elseif filetype == :markdown
return lowercase(ext) in markdown_exts || throw(ArgumentError("$(path) is not a valid markdown file"))
else
throw(ArgumentError("Unrecognized filetype $(filetype)"))
end
end
### common utils for DemoPage and DemoSection
function validate_order(order::AbstractArray, x::Union{DemoPage, DemoSection})
default_order = get_default_order(x)
if intersect(order, default_order) == union(order, default_order)
return true
else
config_filepath = joinpath(x.root, config_filename)
entries = join(string.(setdiff(order, default_order)), "\n")
isempty(entries) || @warn("The following entries in $(config_filepath) are not used anymore:\n$(entries)")
entries = join(string.(setdiff(default_order, order)), "\n")
isempty(entries) || @warn("The following entries in $(config_filepath) are missing:\n$(entries)")
throw(ArgumentError("incorrect order in $(config_filepath), please check the previous warning message."))
end
end
"""
walkpage([f=identity], page; flatten=true, max_depth=Inf)
Walk through the page structure and apply function f to each of the item.
By default, the page structure is not preserved in the result. To preserve that, you could pass
`flatten=false` to the function.
# Examples
This is particulaly useful to generate information for the page structure. For example:
```julia
julia> page = DemoPage("docs/quickstart/");
julia> walkpage(page; flatten=true) do item
basename(item.path)
end
"Quick Start" => [
"simple_markdown_demo.md",
"configure_card.md",
"configure_sec_and_page.md",
"hide_your_card_from_index.md",
"hidden_card.jl",
"1.julia_demo.jl",
"2.cover_on_the_fly.jl"
]
```
If `flatten=false`, then it gives you something like this:
```
"Quick Start" => [
"Usage example" => [
"Basics" => [
"simple_markdown_demo.md",
"configure_card.md",
"configure_sec_and_page.md",
"hide_your_card_from_index.md",
"hidden_card.jl"
],
"Julia demos" => [
"1.julia_demo.jl",
"2.cover_on_the_fly.jl"
]
]
]
```
"""
walkpage(page::DemoPage; kwargs...) = walkpage(identity, page; kwargs...)
function walkpage(f, page::DemoPage; flatten=true, kwargs...)
nodes = _walkpage.(f, page.sections, 1; flatten=flatten, kwargs...)
if flatten
nodes = reduce(vcat, nodes)
# compensate back to a vector when the first level section has only one section and one card
nodes isa AbstractArray || (nodes = [nodes])
end
page.title => nodes
end
function _walkpage(f, sec::DemoSection, depth; max_depth=Inf, flatten=true)
depth+1 > max_depth && return flatten ? f(sec) : [f(sec), ]
if !isempty(sec.cards)
return flatten ? mapreduce(f, vcat, sec.cards) : sec.title => f.(sec.cards)
else
map_fun(section) = _walkpage(f, section, depth+1; max_depth=max_depth, flatten=flatten)
return flatten ? mapreduce(map_fun, vcat, sec.subsections) : sec.title => map_fun.(sec.subsections)
end
end
### regexes and configuration parsers
raw"""
get_regex(card::AbstractDemoCard, regex_type)
Return regex used to parse `card`.
`regex_type` and capture fields:
* `:image`: $1=>title, $2=>path
* `:title`: $1=>title, (Optionally, $2=>id)
* `:content`: $1=>content
"""
function get_regex(::Val{:Markdown}, regex_type)
if regex_type == :image
# Example: 
return r"^\s*!\[(?<title>[^\]]*)\]\((?<path>[^\s]*)\)"
elseif regex_type == :title
# Example: # [title](@id id)
regex_title = r"\s*#+\s*\[(?<title>[^\]]+)\]\(\@id\s+(?<id>[^\s\)]+)\)"
# Example: # title
regex_simple_title = r"\s*#+\s*(?<title>[^#]+)"
# Note: return the complete one first
return (regex_title, regex_simple_title)
elseif regex_type == :content
# lines that are not title, image, link, list
# FIXME: list is also captured by this regex
return r"^\s*(?<content>[^#\-*!].*)"
else
error("Unrecognized regex type: $(regex_type)")
end
end
function get_regex(::Val{:Julia}, regex_type)
if regex_type == :image
# Example: #md 
return r"^#!?\w*\s+!\[(?<title>[^\]]*)\]\((?<path>[^\s]*)\)"
elseif regex_type == :title
# Example: # [title](@id id)
regex_title = r"\s*#!?\w*\s+#+\s*\[(?<title>[^\]]+)\]\(\@id\s+(?<id>[^\s\)]+)\)"
# Example: # title
regex_simple_title = r"\s*#!?\w*\s+#+\s*(?<title>[^#]+)"
# Note: return the complete one first
return (regex_title, regex_simple_title)
elseif regex_type == :content
# lines that are not title, image, link, list
# FIXME: list is also captured by this regex
return r"^\s*#!?\w*\s+(?<content>[^#\-\*!]+.*)"
else
error("Unrecognized regex type: $(regex_type)")
end
end
# YAML frontmatter
# 1. markdown: ---
# 2. julia: # ---
const regex_yaml = r"^#?\s*---"
# markdown URL: [text](url)
const regex_md_url = r"\[(?<text>[^\]]*)\]\((?<url>[^\)]*)\)"
"""
parse([T::Val], card::AbstractDemoCard)
parse(T::Val, contents)
parse(T::Val, path)
Parse the content of `card` and return the configuration.
Currently supported items are: `title`, `id`, `cover`, `description`.
!!! note
Users of this function need to use `haskey` to check if keys are existed.
They also need to validate the values.
"""
function parse(T::Val, card::AbstractDemoCard)
header, frontmatter, body = split_frontmatter(readlines(card.path))
config = parse(T, body)
# frontmatter has higher priority
if !isempty(frontmatter)
yaml_config = try
YAML.load(join(frontmatter, "\n"))
catch err
@warn "failed to parse YAML frontmatter, please double check the format"
println(stderr, frontmatter)
rethrow(err)
end
merge!(config, yaml_config)
end
return config
end
parse(card::JuliaDemoCard) = parse(Val(:Julia), card)
parse(card::MarkdownDemoCard) = parse(Val(:Markdown), card)
function parse(T::Val, contents::String)
# if we just check isfile then it's likely to complain that filename is too long
# compat: for Julia > 1.1 `endswith` supports regex as well
if match(r"\.(\w+)$", contents) isa RegexMatch && isfile(contents)
contents = readlines(contents)
else
contents = split(contents, "\n")
end
parse(T, contents)
end
function parse(T::Val, contents::AbstractArray{<:AbstractString})::Dict
config = Dict()
# only parse relevant information from the main contents body
_, _, contents = split_frontmatter(contents)
# it's too complicated to regex title correctly from body contents, so a simple
# strategy is to limit possible titles to lines before the contents
body_matches = map(contents) do line
re = get_regex(T, :content)
m = match(re, line)
end
body_lines = findall(map(x->x isa RegexMatch, body_matches))
start_of_body = isempty(body_lines) ? 1 : minimum(body_lines)
# The first title line in markdown format is parse out as the title
title_matches = map(contents[1:start_of_body-1]) do line
# try to match the most complete pattern first
for re in get_regex(T, :title)
m = match(re, line)
m isa RegexMatch && return m
end
return nothing
end
title_lines = findall(map(x->x isa RegexMatch, title_matches))
if !isempty(title_lines)
m = title_matches[title_lines[1]]
config["title"] = m["title"]
if length(m.captures) == 2
config["id"] = m["id"]
end
end
# The first valid image link is parsed out as card cover
image_matches = map(contents) do line
m = match(get_regex(T, :image), line)
m isa RegexMatch && return m
return nothing
end
image_lines = findall(map(x->x isa RegexMatch, image_matches))
if !isempty(image_lines)
config["cover"] = image_matches[image_lines[1]]["path"]
end
description = parse_description(contents, get_regex(T, :content))
if !isnothing(description)
config["description"] = description
end
return config
end
function parse_description(contents::AbstractArray{<:AbstractString}, regex)
# description as the first paragraph that is not a title, image, list or codes
content_lines = map(x->match(regex, x) isa RegexMatch, contents)
code_lines = map(contents) do line
match(r"#?!?\w*```", lstrip(line)) isa RegexMatch
end |> findall
for (i,j) in zip(code_lines[1:2:end], code_lines[2:2:end])
# mark code lines as non-content lines
content_lines[i:j] .= 0
end
m = findall(content_lines)
isempty(m) && return nothing
paragraph_line = findall(x->x!=1, diff(m))
offset = isempty(paragraph_line) ? length(m) : paragraph_line[1]
description = map(contents[m[1:offset]]) do line
m = match(regex, line)
m["content"]
end
description = join(description, " ")
return replace(description, regex_md_url => s"\g<text>")
end
"""
split_frontmatter(contents) -> frontmatter, body
splits the YAML frontmatter out from markdown and julia source code. Leading `# ` will be
stripped for julia codes.
`contents` can be `String` or vector of `String`. Outputs have the same type of `contents`.
"""
function split_frontmatter(contents::String)
header, frontmatter, body = split_frontmatter(split(contents, "\n"))
return join(header, "\n"), join(frontmatter, "\n"), join(body, "\n")
end
function split_frontmatter(contents::AbstractArray{<:AbstractString})
offsets = map(contents) do line
m = match(regex_yaml, line)
m isa RegexMatch
end
offsets = findall(offsets)
length(offsets) < 2 && return "", "", contents
if offsets[1] != 1 && !all(x->isempty(strip(x)) || startswith(strip(x), "#"), contents[1:offsets[1]-1])
# For julia demo, comments and empty lines are allowed before frontmatter
# For markdown demo, only empty lines are allowed before frontmatter
return "", "", contents
end
# infer how many spaces we need to trim from the start line. E.g., "# ---" => 1
start_line = contents[offsets[1]]
m = match(r"^#?(\s*)", start_line)
# make sure we strip the same amount of whitespaces -- preserve indentation
indent_spaces = isnothing(m) ? "" : m.captures[1]
frontmatter = map(contents[offsets[1]: offsets[2]]) do line
m = match(Regex("^#?$(indent_spaces)(.*)"), line)
if isnothing(m)
@warn "probably incorrect YAML syntax or indentation" line
# we don't know much about what `line` is, so do nothing here and let YAML complain about it
line
else
m.captures[1]
end
end
return contents[1:offsets[1]-1], frontmatter, contents[offsets[2]+1:end]
end
function get_default_title(x::Union{AbstractDemoCard, DemoSection, DemoPage})
name_without_ext = splitext(basename(x))[1]
strip(replace(uppercasefirst(name_without_ext), r"[_-]" => " "))
end
is_remote_url(path) = !isnothing(match(r"^https?://", path))
"""
input_bool(prompt)::Bool
Print `prompt` message and trigger user input for confirmation.
"""
function input_bool(prompt)
while true
println(prompt, " [y/n]")
response = readline()
length(response) == 0 && continue
reply = lowercase(first(strip(response)))
if reply == 'y'
return true
elseif reply =='n'
return false
end
# Otherwise loop and repeat the prompt
end
end
is_pluto_notebook(path::String) = any(occursin.(r"#\s?βββ‘\s?[0-9a-f\-]{36}", readlines(path)))
"""
meta_edit_url(path)
helper to add in corrected edit link for markdown files which will be processed by Documenter.jl later
"""
function meta_edit_url(path)
escaped_path = escape_string(path)
return """
```@meta
EditURL = "$escaped_path"
```
"""
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 2501 | module CardThemes
# Notes for adding new card theme
#
# Steps to write new card theme:
# 1. specialize `cardtheme`, it should return two items:
# * `templates`: a dict with following fields:
# * "card": a Mustache template
# * "section": a Mustache template
# * `stylesheet_path`: absolute filepath to the stylesheet.
# 2. add the new theme name to `themelists`
#
# available placeholders for section template:
# * `cards`: the card table/list/whatever contents
# * `description`: section description contents
#
# available placeholders for card template:
# * `description`: card description
# * `covername`: the name of the card cover image
# * `id`: reference id that reads by Documenter
# * `title`: card title
#
using Mustache
"""
A list of DemoCards theme presets
"""
const themelists = ["bulmagrid", "bokehlist", "grid", "list", "nocoverlist", "transitiongrid"]
# TODO: don't hardcode this
const max_coversize = (220, 200)
# add themes
include("bulmagrid/bulmagrid.jl")
include("grid/grid.jl")
include("transitiongrid/transitiongrid.jl")
include("list/list.jl")
include("nocoverlist/nocoverlist.jl")
include("bokehlist/bokehlist.jl")
"""
cardtheme(theme = "grid";
root = "<current-directory>",
src = "src",
destination = "democards") -> templates, stylesheet_path
For given theme, return the templates and path to stylesheet.
`root` and `destination` should have the same value to that passed to `makedemos`.
Available themes are:
\n - "$(join(themelists, "\"\n - \""))"
"""
function cardtheme(theme::AbstractString="grid";
root::AbstractString = Base.source_dir(),
src::AbstractString = "src",
destination::String = "democards")
templates, src_stylesheet_path = cardtheme(Val(Symbol(theme)))
# a copy is needed because Documenter only support relative path inside root
absolute_root = joinpath(root, src, destination)
filename = "$(theme)theme.css"
out_stylesheet_path = joinpath(absolute_root, filename)
isdir(absolute_root) || mkpath(absolute_root)
cp(src_stylesheet_path, out_stylesheet_path; force=true)
return templates, joinpath(destination, filename)
end
# fallback if the theme name doesn't exist
function cardtheme(theme::Val{T}) where T
themes_str = join(themelists, ", ")
error("unrecognized card theme: $T\nAvaiable theme options are: $themes_str")
end
export cardtheme, max_coversize
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 843 | const bokeh_list_section_template = mt"""
{{{description}}}
```@raw html
<div class="bokeh-list-card-section">
```
{{{cards}}}
```@raw html
</div>
```
"""
const bokeh_list_card_template = mt"""
```@raw html
<div class="bokeh-list-card">
<table>
<td valign="bottom"><div class="bokeh-list-card-cover">
```
[](@ref {{id}})
```@raw html
</div></td>
<td><div class="bokeh-list-card-text">
```
[{{{title}}}](@ref {{id}})
```@raw html
</div>
<div class="bokeh-list-card-description">
```
{{{description}}}
```@raw html
</div>
</td>
</tbody></table>
</div>
```
"""
function cardtheme(::Val{:bokehlist})
templates = Dict(
"card" => bokeh_list_card_template,
"section" => bokeh_list_section_template
)
return templates, abspath(@__DIR__, "style.css")
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 941 | const bulma_grid_section_template = mt"""
{{{description}}}
```@raw html
<div class="columns is-multiline bulma-grid-card-section">
```
{{{cards}}}
```@raw html
</div>
```
"""
const bulma_grid_card_template = mt"""
```@raw html
<div class="column is-half">
<div class="card bulma-grid-card">
<div class="card-content">
<h3 class="is-size-5 bulma-grid-card-text">
{{{title}}}
</h3>
<p class="is-size-6 bulma-grid-card-description">
{{{description}}}
</p>
</div>
<div class="card-image bulma-grid-card-cover">
```
[](@ref {{id}})
```@raw html
</div>
</div>
</div>
```
"""
function cardtheme(::Val{:bulmagrid})
templates = Dict(
"card" => bulma_grid_card_template,
"section" => bulma_grid_section_template
)
return templates, abspath(@__DIR__, "style.css")
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 700 | const grid_section_template = mt"""
{{{description}}}
```@raw html
<div class="grid-card-section">
```
{{{cards}}}
```@raw html
</div>
```
"""
const grid_card_template = mt"""
```@raw html
<div class="card grid-card">
<div class="grid-card-cover">
<div class="grid-card-description">
```
{{description}}
```@raw html
</div>
```
[](@ref {{id}})
```@raw html
</div>
<div class="grid-card-text">
```
[{{title}}](@ref {{id}})
```@raw html
</div>
</div>
```
"""
function cardtheme(::Val{:grid})
templates = Dict(
"card" => grid_card_template,
"section" => grid_section_template
)
return templates, abspath(@__DIR__, "style.css")
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 778 | const list_section_template = mt"""
{{{description}}}
```@raw html
<div class="list-card-section">
```
{{{cards}}}
```@raw html
</div>
```
"""
const list_card_template = mt"""
```@raw html
<div class="list-card">
<table>
<td valign="bottom"><div class="list-card-cover">
```
[](@ref {{id}})
```@raw html
</div></td>
<td><div class="list-card-text">
```
[{{{title}}}](@ref {{id}})
```@raw html
</div>
<div class="list-card-description">
```
{{{description}}}
```@raw html
</div>
</td>
</tbody></table>
</div>
```
"""
function cardtheme(::Val{:list})
templates = Dict(
"card" => list_card_template,
"section" => list_section_template
)
return templates, abspath(@__DIR__, "style.css")
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 673 | const nocoverlist_section_template = mt"""
{{{description}}}
```@raw html
<div class="list-card-section">
```
{{{cards}}}
```@raw html
</div>
```
"""
const nocoverlist_card_template = mt"""
```@raw html
<div class="list-card">
<table>
<td><div class="list-card-text">
```
[{{{title}}}](@ref {{id}})
```@raw html
</div>
<div class="list-card-description">
```
{{{description}}}
```@raw html
</div>
</td>
</tbody></table>
</div>
```
"""
function cardtheme(::Val{:nocoverlist})
templates = Dict(
"card" => nocoverlist_card_template,
"section" => nocoverlist_section_template
)
return templates, abspath(@__DIR__, "style.css")
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 820 | const transition_grid_section_template = mt"""
{{{description}}}
```@raw html
<div class="transition-grid-card-section">
```
{{{cards}}}
```@raw html
</div>
```
"""
const transition_grid_card_template = mt"""
```@raw html
<div class="card transition-grid-card">
<div class="transition-grid-card-cover" style="background-image: url({{{coverpath}}})">
<div class="transition-grid-card-description">
```
{{description}}
```@raw html
</div>
```
[](@ref {{id}})
```@raw html
</div>
<div class="transition-grid-card-text">
```
[{{title}}](@ref {{id}})
```@raw html
</div>
</div>
```
"""
function cardtheme(::Val{:transitiongrid})
templates = Dict(
"card" => transition_grid_card_template,
"section" => transition_grid_section_template
)
return templates, abspath(@__DIR__, "style.css")
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 4595 | abstract type AbstractDemoCard end
"""
struct MarkdownDemoCard <: AbstractDemoCard
MarkdownDemoCard(path::String)
Constructs a unknown-format demo card from a file at `path`.
"""
struct UnmatchedCard <: AbstractDemoCard
path::String
end
"""
democard(path::String)::T
Constructs a concrete AbstractDemoCard instance.
The return type `T` is determined by the extension of the path
to your demofile. Currently supported types are:
* [`MarkdownDemoCard`](@ref) for markdown files
* [`JuliaDemoCard`](@ref) for julia files
* [`PlutoDemoCard`](@ref) for pluto files
* [`UnmatchedCard`](@ref) for unmatched files
"""
function democard(path::String)::AbstractDemoCard
validate_file(path)
_, ext = splitext(path)
if ext in markdown_exts
return MarkdownDemoCard(path)
elseif ext in julia_exts
if is_pluto_notebook(path)
return try
PlutoDemoCard(path)
catch e
if isa(e, MethodError)
# method is not imported from PlutoNotebook
throw(
ErrorException(
"You need to load PlutoStaticHTML.jl for using pluto notebooks",
),
)
else
throw(e)
end
end
else
return JuliaDemoCard(path)
end
else
return UnmatchedCard(path)
end
end
basename(x::AbstractDemoCard) = basename(x.path)
function get_default_id(card::AbstractDemoCard)
# drop leading coutning numbers such as 1. 1_ 1-
m = match(r"\d*(?<name>.*)", card.title)
id = replace(strip(m["name"]), ' ' => '-')
end
function validate_id(id::AbstractString, card::AbstractDemoCard)
if occursin(' ', id)
throw(ArgumentError("invalid id in $(card.path), it should not contain spaces."))
end
end
function is_democard(file)
try
@suppress_err democard(file)
return true
catch err
@debug err
return false
end
end
function load_config(card::T, key; config=Dict()) where T <: AbstractDemoCard
isempty(config) && (config = parse(card))
if key == "cover"
haskey(config, key) || return nothing
cover_path = config[key]
if !is_remote_url(cover_path)
cover_path = replace(cover_path, r"[/\\]" => Base.Filesystem.path_separator) # windows compatibility
end
return cover_path
elseif key == "id"
haskey(config, key) || return get_default_id(card)
id = config[key]
validate_id(id, card)
return id
elseif key == "title"
return get(config, key, get_default_title(card))
elseif key == "description"
return get(config, key, card.title)
elseif key == "hidden"
hidden = get(config, key, false)
return hidden isa Bool ? hidden : parse(Bool, string(hidden))
elseif key == "author"
return get(config, key, "")
elseif key == "date"
return DateTime(get(config, key, DateTime(0)))
elseif key == "julia"
version = get(config, key, JULIA_COMPAT)
return version isa VersionNumber ? version : VersionNumber(string(version))
elseif key == "generate_cover"
return get(config, key, true)
elseif key == "execute"
return get(config, key, true)
else
throw(ArgumentError("Unrecognized key $(key) for $(T)"))
end
end
function make_badges(card::AbstractDemoCard)
badges = []
if !isempty(card.author)
for author in split(card.author, ';')
# TODO: also split on "and"
isempty(author) && continue
m = match(regex_md_url, author)
if isnothing(m)
author_str = HTTP.escapeuri(strip(author))
push!(badges, "-blue)")
else
# when markdown url is detected, create a link for it
# author: [Johnny Chen](https://github.com/johnnychen94)
name, url = strip.(m.captures)
name = HTTP.escapeuri(name)
badge_str = "[-blue)]($url)"
push!(badges, badge_str)
end
end
end
if card.date != DateTime(0)
date_str = string(round(Int, datetime2unix(card.date)))
push!(badges, ")")
end
isempty(badges) ? "" : join(badges, " ")
end
### load concrete implementations
include("markdown.jl")
include("julia.jl")
include("pluto.jl")
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 11603 | const julia_exts = [".jl",]
const nbviewer_badge = "https://img.shields.io/badge/show-nbviewer-579ACA.svg"
const download_badge = "https://img.shields.io/badge/download-julia-brightgreen.svg"
const julia_footer = raw"""
---
*This page was generated using [DemoCards.jl](https://github.com/JuliaDocs/DemoCards.jl) and [Literate.jl](https://github.com/fredrikekre/Literate.jl).*
"""
"""
struct JuliaDemoCard <: AbstractDemoCard
JuliaDemoCard(path::String)
Constructs a julia-format demo card from existing julia file `path`.
The julia file is written in [Literate syntax](https://fredrikekre.github.io/Literate.jl/stable/fileformat).
# Fields
Besides `path`, this struct has some other fields:
* `path`: path to the source julia file
* `cover`: path to the cover image
* `id`: cross-reference id
* `title`: one-line description of the demo card
* `author`: author(s) of this demo.
* `date`: the update date of this demo.
* `description`: multi-line description of the demo card
* `julia`: Julia version compatibility
* `hidden`: whether this card is shown in the generated index page
* `notebook`: enable or disable the jupyter notebook generation. Valid values are `true` or `false`.
* `generate_cover` whether to generate a cover image for this demo card by executing it
* `execute`: whether to execute the demo card when generating the notebook
# Configuration
You can pass additional information by adding a YAML front matter to the julia file.
Supported items are:
* `cover`: an URL or a relative path to the cover image. If not specified, it will use the first available image link, or all-white image if there's no image links.
* `description`: a multi-line description to this file, will be displayed when the demo card is hovered. By default it uses `title`.
* `id`: specify the `id` tag for cross-references. By default it's infered from the filename, e.g., `simple_demo` from `simple demo.md`.
* `title`: one-line description to this file, will be displayed under the cover image. By default, it's the name of the file (without extension).
* `author`: author name. If there are multiple authors, split them with semicolon `;`.
* `date`: any string contents that can be passed to `Dates.DateTime`. For example, `2020-09-13`.
* `julia`: Julia version compatibility. Any string that can be converted to `VersionNumber`
* `hidden`: whether this card is shown in the layout of index page. The default value is `false`.
* `generate_cover` whether to generate a cover image for this demo card by executing it. The default value is `true`. Note that `execute` must also be `true` for this to take effect.
* `execute`: whether to execute the demo card when generating the notebook. The default value is `true`.
An example of the front matter (note the leading `#`):
```julia
# ---
# title: passing extra information
# cover: cover.png
# id: non_ambiguious_id
# author: Jane Doe; John Roe
# date: 2020-01-31
# description: this demo shows how you can pass extra demo information to DemoCards package. All these are optional.
# julia: 1.0
# hidden: false
# ---
```
See also: [`MarkdownDemoCard`](@ref DemoCards.MarkdownDemoCard), [`PlutoDemoCard`](@ref DemoCards.PlutoDemoCard), [`DemoSection`](@ref DemoCards.DemoSection), [`DemoPage`](@ref DemoCards.DemoPage)
"""
mutable struct JuliaDemoCard <: AbstractDemoCard
path::String
cover::Union{String, Nothing}
id::String
title::String
description::String
author::String
date::DateTime
julia::VersionNumber
hidden::Bool
notebook::Union{Nothing, Bool}
generate_cover::Bool
execute::Bool
end
function JuliaDemoCard(path::String)::JuliaDemoCard
# first consturct an incomplete democard, and then load the config
card = JuliaDemoCard(path, "", "", "", "", "", DateTime(0), JULIA_COMPAT, false, nothing, true, true)
config = parse(card)
card.cover = load_config(card, "cover"; config=config)
card.title = load_config(card, "title"; config=config)
card.date = load_config(card, "date"; config=config)
card.author = load_config(card, "author"; config=config)
card.julia = load_config(card, "julia"; config=config)
# default id requires a title
card.id = load_config(card, "id"; config=config)
# default description requires a title
card.description = load_config(card, "description"; config=config)
card.hidden = load_config(card, "hidden"; config=config)
# Because we want bottom-level cards configuration to have higher priority, figuring
# out the default `notebook` option needs a reverse walk from top-level page to the
# bottom-level card.
if haskey(config, "notebook")
notebook = config["notebook"]
if notebook isa Bool
card.notebook = notebook
else
card.notebook = try
parse(Bool, lowercase(notebook))
catch err
@warn "`notebook` option should be either `\"true\"` or `\"false\"`, instead it is: $notebook. Fallback to unconfigured."
nothing
end
end
end
card.generate_cover = load_config(card, "generate_cover"; config=config)
card.execute = load_config(card, "execute"; config=config)
return card
end
"""
save_democards(card_dir::String, card::JuliaDemoCard;
project_dir,
src,
credit,
nbviewer_root_url)
process the original julia file and save it.
The processing pipeline is:
1. preprocess and copy source file
2. generate ipynb file
3. generate markdown file
4. insert header and footer to generated markdown file
"""
function save_democards(card_dir::String,
card::JuliaDemoCard;
credit,
nbviewer_root_url,
project_dir=Base.source_dir(),
src="src",
throw_error = false,
properties = Dict{String, Any}(),
kwargs...)
isdir(card_dir) || mkpath(card_dir)
@debug card.path
original_card_path = card.path
cardname = splitext(basename(card.path))[1]
md_path = joinpath(card_dir, "$(cardname).md")
nb_path = joinpath(card_dir, "$(cardname).ipynb")
src_path = joinpath(card_dir, "$(cardname).jl")
if VERSION < card.julia
# It may work, it may not work; I hope it would work.
@warn "The running Julia version `$(VERSION)` is older than the declared compatible version `$(card.julia)`. You might need to upgrade your Julia."
end
card.notebook = if isnothing(card.notebook)
# Backward compatibility: we used to generate notebooks for all jl files
op = get(properties, "notebook", "true")
op = isa(op, Bool) ? op : try
Base.parse(Bool, lowercase(op))
catch err
@warn "`notebook` option should be either `\"true\"` or `\"false\"`, instead it is: $op. Fallback to \"true\"."
true
end
else
card.notebook
end
@assert !isnothing(card.notebook)
# 1. generating assets
cp(card.path, src_path; force=true)
cd(card_dir) do
try
foreach(ignored_dirnames) do x
isdir(x) || mkdir(x)
end
if (card.generate_cover && card.execute)
# run scripts in a sandbox
m = Module(gensym())
# modules created with Module() does not have include defined
# abspath is needed since this will call `include_relative`
Core.eval(m, :(include(x) = Base.include($m, abspath(x))))
gen_assets() = Core.eval(m, :(include($cardname * ".jl")))
verbose_mode() ? gen_assets() : @suppress gen_assets()
end
# WARNING: card.path is modified here
card.path = cardname*".jl"
card.cover = load_config(card, "cover")
card.path = src_path
foreach(ignored_dirnames) do x
isempty(readdir(x)) && rm(x; recursive=true, force=true)
end
catch err
if err isa LoadError
# TODO: provide more informative debug message
@warn "something wrong during the assets generation for demo $cardname.jl"
if throw_error
rethrow(err)
else
@warn err
end
else
rethrow(err)
end
end
end
# remove YAML frontmatter
src_header, _, body = split_frontmatter(read(src_path, String))
isempty(strip(src_header)) || (src_header *= "\n\n")
# insert header badge
badges = make_badges(card;
src=src,
card_dir=card_dir,
nbviewer_root_url=nbviewer_root_url,
project_dir=project_dir,
build_notebook=card.notebook)
write(src_path, badges * "\n\n", body)
# 2. notebook
if card.notebook
try
@suppress Literate.notebook(src_path, card_dir; credit=credit, execute=card.execute)
catch err
nothing # There are already early warning in the assets generation
end
end
# 3. markdown
md_kwargs = Dict()
if card.execute
md_kwargs[:execute] = true
else
md_kwargs[:codefence] = ("````julia" => "````")
end
@suppress Literate.markdown(src_path, card_dir; credit=false, md_kwargs...) # manually add credit later
# remove meta info generated by Literate.jl
contents = readlines(md_path)
offsets = findall(x->startswith(x, "```"), contents)
body = join(contents[offsets[2]+1:end], "\n")
# 4. insert header and footer to generated markdown file
config = parse(Val(:Julia), body)
need_header = !haskey(config, "title")
# @ref syntax: https://juliadocs.github.io/Documenter.jl/stable/man/syntax/#@ref-link-1
header = need_header ? "# [$(card.title)](@id $(card.id))\n" : "\n"
footer = credit ? julia_footer : "\n"
write(md_path, meta_edit_url(original_card_path), header, body, footer)
# 5. filter out source file
mktempdir(card_dir) do tmpdir
@suppress Literate.script(src_path, tmpdir; credit=credit)
write(src_path, src_header, read(joinpath(tmpdir, basename(src_path)), String))
end
end
function make_badges(card::JuliaDemoCard; src, card_dir, nbviewer_root_url, project_dir, build_notebook)
cardname = splitext(basename(card.path))[1]
badges = ["#md #"]
push!(badges, "[]($(cardname).jl)")
if build_notebook
if !isempty(nbviewer_root_url)
# Note: this is only reachable in CI environment
nbviewer_folder = normpath(relpath(card_dir, "$project_dir/$src"))
nbviewer_url = replace("$(nbviewer_root_url)/$(nbviewer_folder)/$(cardname).ipynb", Base.Filesystem.path_separator=>'/')
else
nbviewer_url = "$(cardname).ipynb"
end
push!(badges, "[]($nbviewer_url)")
end
if card.julia != JULIA_COMPAT
# It might be over verbose to insert a compat badge for every julia card, only add one for
# cards that users should care about
# Question: Is this too conservative?
push!(badges, "-blue.svg)")
end
push!(badges, invoke(make_badges, Tuple{AbstractDemoCard}, card))
join(badges, " ")
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 4564 | # generally, Documenter.jl assumes markdown files ends with `.md`
const markdown_exts = [".md",]
const markdown_footer = raw"""
---
*This page was generated using [DemoCards.jl](https://github.com/JuliaDocs/DemoCards.jl).*
"""
"""
struct MarkdownDemoCard <: AbstractDemoCard
MarkdownDemoCard(path::String)
Constructs a markdown-format demo card from existing markdown file `path`.
# Fields
Besides `path`, this struct has some other fields:
* `path`: path to the source markdown file
* `cover`: path to the cover image
* `id`: cross-reference id
* `title`: one-line description of the demo card
* `author`: author(s) of this demo.
* `date`: the update date of this demo.
* `description`: multi-line description of the demo card
* `hidden`: whether this card is shown in the generated index page
# Configuration
You can pass additional information by adding a YAML front matter to the markdown file.
Supported items are:
* `cover`: an URL or a relative path to the cover image. If not specified, it will use the first available image link, or all-white image if there's no image links.
* `description`: a multi-line description to this file, will be displayed when the demo card is hovered. By default it uses `title`.
* `id`: specify the `id` tag for cross-references. By default it's infered from the filename, e.g., `simple_demo` from `simple demo.md`.
* `title`: one-line description to this file, will be displayed under the cover image. By default, it's the name of the file (without extension).
* `author`: author name. If there are multiple authors, split them with semicolon `;`.
* `date`: any string contents that can be passed to `Dates.DateTime`. For example, `2020-09-13`.
* `hidden`: whether this card is shown in the layout of index page. The default value is `false`.
An example of the front matter:
```text
---
title: passing extra information
cover: cover.png
id: non_ambiguious_id
author: Jane Doe; John Roe
date: 2020-01-31
description: this demo shows how you can pass extra demo information to DemoCards package. All these are optional.
hidden: false
---
```
See also: [`JuliaDemoCard`](@ref DemoCards.JuliaDemoCard), [`PlutoDemoCard`](@ref DemoCards.PlutoDemoCard), [`DemoSection`](@ref DemoCards.DemoSection), [`DemoPage`](@ref DemoCards.DemoPage)
"""
mutable struct MarkdownDemoCard <: AbstractDemoCard
path::String
cover::Union{String, Nothing}
id::String
title::String
description::String
author::String
date::DateTime
hidden::Bool
end
function MarkdownDemoCard(path::String)::MarkdownDemoCard
# first consturct an incomplete democard, and then load the config
card = MarkdownDemoCard(path, "", "", "", "", "", DateTime(0), false)
config = parse(card)
card.cover = load_config(card, "cover"; config=config)
card.title = load_config(card, "title"; config=config)
card.date = load_config(card, "date"; config=config)
card.author = load_config(card, "author"; config=config)
# Unlike JuliaDemoCard, Markdown card doesn't accept `julia` compat field. This is because we
# generally don't know the markdown processing backend. It might be Documenter, but who knows.
# More generally, any badges can just be manually added by demo writter, if they want.
# `date` and `author` fields are added just for convinience.
# default id requires a title
card.id = load_config(card, "id"; config=config)
# default description requires a title
card.description = load_config(card, "description"; config=config)
card.hidden = load_config(card, "hidden"; config=config)
return card
end
"""
save_democards(card_dir::String, card::MarkdownDemoCard)
process the original markdown file and save it.
The processing pipeline is:
1. strip the front matter
2. insert a level-1 title and id
"""
function save_democards(card_dir::String,
card::MarkdownDemoCard;
credit,
kwargs...)
isdir(card_dir) || mkpath(card_dir)
@debug card.path
markdown_path = joinpath(card_dir, basename(card))
_, _, body = split_frontmatter(read(card.path, String))
config = parse(Val(:Markdown), body)
need_header = !haskey(config, "title")
# @ref syntax: https://juliadocs.github.io/Documenter.jl/stable/man/syntax/#@ref-link-1
header = need_header ? "# [$(card.title)](@id $(card.id))\n" : "\n"
footer = credit ? markdown_footer : "\n"
write(markdown_path, meta_edit_url(card.path), header, make_badges(card)*"\n\n", body, footer)
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 9504 | """
struct DemoPage <: Any
DemoPage(root::String)
Constructs a demo page object.
# Fields
Besides the root path to the demo page folder `root`, this struct has some other fields:
* `title`: page title
* `template`: template content of the demo page.
* `sections`: demo sections found in `root`
# Configuration
You can manage an extra `config.json` file to customize rendering of a demo page.
Supported items are:
* `order`: specify the sections order. By default, it's case-insensitive alphabetic order.
* `template`: path to template filename. By default, it's `"index.md"`. The content of the template file should has one and only one `{{{democards}}}`.
* `theme`: specify which card theme should be used to generate the index page. If not specified, it
will default to `nothing`.
* `stylesheet`: relative path to the stylesheet file to override the default stylesheet provided by `"theme"`.
* `title`: specify the title of this demo page. By default, it's the folder name of `root`. Will be override by `template`.
* `properties`: a dictionary of properties that can be propagated to its children items. The same properties in
the children items, if exist, have higher priority.
The following is an example of `config.json`:
```json
{
"template": "template.md",
"theme": "grid",
"stylesheet": "assets/gridstyle.css",
"order": [
"basic",
"advanced"
],
"properties": {
"notebook": "false",
"julia": "1.6",
"author": "Johnny Chen"
}
}
```
# Examples
The following is the simplest folder structure of a `DemoPage`:
```text
demos
βββ basic
βββ demo_1.md
βββ demo_2.md
βββ demo_3.md
βββ demo_4.md
βββ demo_5.md
βββ demo_6.md
βββ demo_7.md
βββ demo_8.md
```
!!! note
A `DemoPage` doesn't manage demo files directly, so here you'll need
a DemoSection `basic` to manage them.
The following is a typical folder structure of a `DemoPage`:
```text
demos
βββ advanced
β βββ advanced_demo_1.md
β βββ advanced_demo_2.md
βββ basic
β βββ part1
β β βββ basic_demo_1_1.md
β β βββ basic_demo_1_2.md
β βββ part2
β βββ config.json
β βββ basic_demo_2_1.md
β βββ basic_demo_2_2.md
βββ config.json
βββ template.md
```
!!! warning
A section should only hold either subsections or demo files. A folder that has both subfolders and demo files (e.g., `*.md`) is invalid.
See also: [`MarkdownDemoCard`](@ref DemoCards.MarkdownDemoCard), [`DemoSection`](@ref DemoCards.DemoSection)
"""
mutable struct DemoPage
root::String
sections::Vector{DemoSection}
template::String
theme::Union{Nothing, String}
stylesheet::Union{Nothing, String}
title::String
# These properties will be shared by all children of it during build time
properties::Dict{String, Any}
end
basename(page::DemoPage) = basename(page.root)
function DemoPage(root::String, filter_func=x -> true)::DemoPage
root = replace(root, r"[/\\]" => Base.Filesystem.path_separator) # windows compatibility
isdir(root) || throw(ArgumentError("page root does not exist: $(root)"))
root = rstrip(root, '/') # otherwise basename(root) will returns `""`
path = joinpath.(root, filter(x->!startswith(x, "."), readdir(root))) # filter out hidden files
section_paths = filter(x->isdir(x)&&!(basename(x) in ignored_dirnames),
path)
if isempty(section_paths)
# This is an edge and trivial case that we need to disallow; we want the following
# folder structure be uniquely parsed as a demo section and not a demo page without
# ambiguity.
# ```
# demos/
# βββ demo1.jl
# ```
msg = "can not find a valid page structure in page dir \"$root\"\nit should have at least one folder as section inside it"
throw(ArgumentError(msg))
end
json_path = joinpath(root, config_filename)
json_config = isfile(json_path) ? JSON.parsefile(json_path) : Dict()
template_file = joinpath(root, get(json_config, "template", template_filename))
config = parse(Val(:Markdown), template_file)
config = merge(json_config, config) # template has higher priority over config file
sections = filter(map(p -> DemoSection(p, filter_func), section_paths)) do sec
empty_section = isempty(sec.cards) && isempty(sec.subsections)
if empty_section
@warn "Empty section detected, remove from the demo page tree." section=relpath(sec.root, root)
return false
else
return true
end
end
isempty(sections) && error("Empty demo page, you have to add something.")
page = DemoPage(root, sections, "", nothing, nothing, "", Dict{String, Any}())
page.theme = load_config(page, "theme"; config=config)
page.stylesheet = load_config(page, "stylesheet"; config=config)
section_orders = load_config(page, "order"; config=config)
section_orders = map(section_orders) do sec
findfirst(x-> sec == basename(x), sections)
end
ordered_sections = sections[section_orders]
title = load_config(page, "title"; config=config)
page.sections = ordered_sections
page.title = title
# default template requires a title
template = load_config(page, "template"; config=config)
page.template = meta_edit_url(template_file) * template
if haskey(config, "properties")
properties = config["properties"]::Dict
merge!(page.properties, properties)
end
return page
end
function load_config(page::DemoPage, key; config=Dict())
if isempty(config)
json_path = joinpath(page.root, config_filename)
json_config = isfile(json_path) ? JSON.parsefile(json_path) : Dict()
template_file = joinpath(page.root, get(json_config, "template", template_filename))
config = parse(Val(:Markdown), template_file)
config = merge(json_config, config) # template has higher priority over config file
end
# config could still be an empty dictionary
if key == "order"
haskey(config, key) || return get_default_order(page)
order = config[key]
validate_order(order, page)
return order
elseif key == "title"
return get(config, key, get_default_title(page))
elseif key == "template"
return load_template(page, config)
elseif key == "theme"
theme = get(config, key, nothing)
if !isnothing(theme) && lowercase(theme) == "nothing"
theme = nothing
end
return theme
elseif key == "stylesheet"
stylesheet = get(config, key, nothing)
return isnothing(stylesheet) ? nothing : abspath(page.root, stylesheet)
else
throw(ArgumentError("Unrecognized key $(key) for DemoPage"))
end
end
function load_template(page, config)
key = "template"
if haskey(config, key)
# windows compatibility
template_name = replace(config[key],
r"[/\\]" => Base.Filesystem.path_separator)
template_file = joinpath(page.root, template_name)
else
template_file = joinpath(page.root, template_filename)
end
isfile(template_file) || return get_default_template(page)
check_ext(template_file, :markdown)
if 1 != sum(occursin.("{{{democards}}}", readlines(template_file)))
throw(ArgumentError("invalid template file $(template_file): it should has one and only one {{{democards}}}"))
end
return read(template_file, String)
end
get_default_order(page::DemoPage) =
sort(basename.(page.sections), by = x->lowercase(x))
function get_default_template(page::DemoPage)
header = "# $(page.title)\n\n"
# TODO: by doing this we loss the control on section-level template
content = "{{{democards}}}" # render by Mustache
footer = ""
return header * content * footer
end
# page utils
"""
infer_pagedir(card_path; rootdir="")
Given a demo card path, infer the *outmost* dir path that makes it a valid demo page. If it fails to
find such dir path, return `nothing`.
The inference is done recursively, `rootdir` sets a stop condition for the inference process.
!!! warning
This inference may not be the exact page dir in trivial cases, for example:
```
testdir/
βββ examples
βββ sections
βββ demo1.md
```
Both `testdir` and `examples` can be valid dir path for a demo page, this function would
just return `testdir` as it is the outmost match.
"""
function infer_pagedir(path; rootdir::String=first(splitdrive(path)))
# the last root path that `DemoPage(root)` successfully parses
if is_demopage(path)
root, dir = splitdir(path) # we need the outmost folder, so not return right here
elseif is_demosection(path)
root, dir = splitdir(path)
elseif is_democard(path)
root, dir = splitdir(dirname(path))
else
return nothing
end
while true
if is_demopage(root)
root, dir = splitdir(root)
else
pagedir = joinpath(root, dir)
return is_demopage(pagedir) ? pagedir : nothing # in case it fails at the first try
end
root in push!(["/", ""], rootdir) && return nothing
end
end
function is_demopage(dir)
try
# if fails to parse, then it is not a valid demo page
@suppress_err DemoPage(dir)
return true
catch err
@debug err
return false
end
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 2746 | const pluto_footer = raw"""
---
*This page was generated using [DemoCards.jl](https://github.com/JuliaDocs/DemoCards.jl). and [PlutoStaticHTML.jl](https://github.com/rikhuijzer/PlutoStaticHTML.jl)*
"""
"""
struct PlutoDemoCard <: AbstractDemoCard
PlutoDemoCard(path::AbstractString)
Constructs a pluto-format demo card from a pluto notebook `path`.
# Fields
Besides `path`, this struct has some other fields:
* `path`: path to the source markdown file
* `cover`: path to the cover image
* `id`: cross-reference id
* `title`: one-line description of the demo card
* `author`: author(s) of this demo.
* `date`: the update date of this demo.
* `description`: multi-line description of the demo card
* `julia`: Julia version compatibility
* `hidden`: whether this card is shown in the generated index page
# Configuration
You can pass additional information by adding a pluto front-matter section to the notebook.
Supported items are:
* `cover`: an URL or a relative path to the cover image. If not specified, it will use the first available image link, or all-white image if there's no image links.
* `description`: a multi-line description to this file, will be displayed when the demo card is hovered. By default it uses `title`.
* `id`: specify the `id` tag for cross-references. By default it's infered from the filename, e.g., `simple_demo` from `simple demo.jl`.
* `title`: one-line description to this file, will be displayed under the cover image. By default, it's the name of the file (without extension).
* `author`: author name. If there are multiple authors, split them with semicolon `;`.
* `julia`: Julia version compatibility. Any string that can be converted to `VersionNumber`
* `date`: any string contents that can be passed to `Dates.DateTime`. For example, `2020-09-13`.
* `hidden`: whether this card is shown in the layout of index page. The default value is `false`.
An example of pluto front matter in the notebook:
```text
#> [frontmatter]
#> title = "passing extra information"
#> cover = "cover.png"
#> id = "non_ambiguious_id"
#> author = "Jane Doe; John Roe"
#> date = "2020-01-31"
#> description = "this demo shows how you can pass extra demo information to DemoCards package. All these are optional."
#> julia: "1.0"
#> hidden: "false"
```
See also: [`MarkdownDemoCard`](@ref DemoCards.MarkdownDemoCard), [`JuliaDemoCard`](@ref DemoCards.JuliaDemoCard), [`DemoSection`](@ref DemoCards.DemoSection), [`DemoPage`](@ref DemoCards.DemoPage)
"""
mutable struct PlutoDemoCard <: AbstractDemoCard
path::String
cover::Union{String,Nothing}
id::String
title::String
description::String
author::String
date::DateTime
julia::Union{Nothing,VersionNumber}
hidden::Bool
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 5552 | """
struct DemoSection <: Any
DemoSection(root::String)
Constructs a demo section that holds either nested subsections or demo cards.
# Fields
Besides the root path to the demo section folder `root`, this struct has some other fields:
* `cards`: demo cards found in `root`
* `subsections`: nested subsections found in `root`
# Configuration
You can manage an extra `config.json` file to customize rendering of a demo section.
Supported items are:
* `order`: specify the cards order or subsections order. By default, it's case-insensitive alphabetic order.
* `title`: specify the title of this demo section. By default, it's the folder name of `root`.
* `description`: some header description that you want to add before demo cards.
* `properties`: a dictionary of properties that can be propagated to its children items. The same properties in
the children items, if exist, have higher priority.
The following is an example of `config.json`:
```json
{
"title": "learn by examples",
"description": "some one-line description can be useful.",
"order": [
"quickstart.md",
"array.md"
],
"properties": {
"notebook": "false",
"julia": "1.6",
"author": "Johnny Chen"
}
}
```
# Examples
The following is the simplest folder structure of a `DemoSection`:
```text
section
βββ demo_1.md
βββ demo_2.md
βββ demo_3.md
βββ demo_4.md
βββ demo_5.md
βββ demo_6.md
βββ demo_7.md
βββ demo_8.md
```
The following is a typical nested folder structure of a `DemoSection`:
```text
section
βββ config.json
βββ part1
β βββ demo_21.md
β βββ demo_22.md
βββ part2
βββ config.json
βββ demo_23.md
βββ demo_24.md
```
!!! warning
A section should only hold either subsections or demo files. A folder that has both subfolders and demo files (e.g., `*.md`) is invalid.
See also: [`MarkdownDemoCard`](@ref DemoCards.MarkdownDemoCard), [`DemoPage`](@ref DemoCards.DemoPage)
"""
struct DemoSection
root::String
cards::Vector
subsections::Vector{DemoSection}
title::String
description::String
# These properties will be shared by all children of it during build time
properties::Dict{String, Any}
end
basename(sec::DemoSection) = basename(sec.root)
function DemoSection(root::String, filter_func=x -> true)::DemoSection
root = replace(root, r"[/\\]" => Base.Filesystem.path_separator) # windows compatibility
isdir(root) || throw(ArgumentError("section root should be a valid dir, instead it's $(root)"))
path = joinpath.(root, filter(x->!startswith(x, "."), readdir(root))) # filter out hidden files
card_paths = filter(x->isfile(x) && !endswith(x, config_filename), path)
section_paths = filter(x->isdir(x)&&!(basename(x) in ignored_dirnames), path)
if !isempty(card_paths) && !isempty(section_paths)
throw(ArgumentError("section folder $(root) should only hold either cards or subsections"))
end
config_file = joinpath(root, config_filename)
config = isfile(config_file) ? JSON.parsefile(config_file) : Dict()
# For files that `democard` fails to recognized, dummy
# `UnmatchedCard` will be generated. Currently, we only
# throw warnings for it.
cards = map(democard, card_paths)
unmatches = filter(cards) do x
x isa UnmatchedCard || !filter_func(x)
end
if !isempty(unmatches)
msg = join(map(basename, unmatches), "\", \"")
@warn "skip unmatched file: \"$msg\"" section_dir=root
end
cards = filter!(cards) do x
!(x isa UnmatchedCard || !filter_func(x))
end
section = DemoSection(root,
cards,
map(DemoSection, section_paths),
"",
"",
Dict{String, Any}())
ordered_paths = joinpath.(root, load_config(section, "order"; config=config))
if !isempty(section.cards)
cards = map(democard, ordered_paths)
subsections = []
else
cards = []
subsections = map(DemoSection, ordered_paths)
end
title = load_config(section, "title"; config=config)
description = load_config(section, "description"; config=config)
properties = if haskey(config, "properties")
Dict{String, Any}(config["properties"])
else
Dict{String, Any}()
end
DemoSection(root, cards, subsections, title, description, properties)
end
function load_config(sec::DemoSection, key; config=Dict())
if isempty(config)
config_file = joinpath(sec.root, config_filename)
config = isfile(config_file) ? JSON.parsefile(config_file) : Dict()
end
# config could still be an empty dictionary
if key == "order"
haskey(config, key) || return get_default_order(sec)
order = config[key]
validate_order(order, sec)
return order
elseif key == "title"
get(config, key, get_default_title(sec))
elseif key == "description"
get(config, key, "")
else
throw(ArgumentError("Unrecognized key $(key) for DemoSection"))
end
end
"""return case-insensitive alphabetic order"""
function get_default_order(sec::DemoSection)
order = isempty(sec.cards) ? basename.(sec.subsections) : basename.(sec.cards)
sort(order, by = x->lowercase(x))
end
function is_demosection(dir)
try
# if fails to parse, then it is not a valid demo page
@suppress_err DemoSection(dir)
return true
catch err
@debug err
return false
end
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 65 | if v"1.0" <= VERSION < v"1.1"
isnothing(x) = x===nothing
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 1994 | # test various page configuration
@testset "configurations" begin
root = joinpath("assets", "configurations")
@testset "notebook" begin
src_page_dir = joinpath(root, "without_notebook")
page = DemoPage(src_page_dir)
sec1 = page.sections[1]
@test sec1.title == "Sec1"
@test sec1.properties["notebook"] == "true"
sec2 = page.sections[2]
@test sec2.title == "Sec2"
@test isempty(sec2.properties)
@test isnothing(sec1.cards[1].notebook) # This might become `true` in the future
@test sec1.cards[2].notebook == false
page_dir = @suppress_err preview_demos(src_page_dir, require_html=false)
if basename(page_dir) == "without_notebook"
sec1_files = readdir(joinpath(page_dir, "sec1"))
@test Set(filter(x->startswith(x, "demo1"), sec1_files)) == Set(["demo1.ipynb", "demo1.jl", "demo1.md"])
@test Set(filter(x->startswith(x, "demo2"), sec1_files)) == Set(["demo2.jl", "demo2.md"])
@test filter(x->startswith(x, "demo3"), sec1_files) == ["demo3.md"]
sec2_files = readdir(joinpath(page_dir, "sec2"))
@test Set(filter(x->startswith(x, "demo4"), sec2_files)) == Set(["demo4.jl", "demo4.md"])
@test Set(filter(x->startswith(x, "demo5"), sec2_files)) == Set(["demo5.ipynb", "demo5.jl", "demo5.md"])
else
@warn "Inferred page dir for $src_page_dir is no longer \"without_notebook/\""
@test_broken false
end
end
@testset "stylesheet" begin
src_page_dir = joinpath(root, "stylesheet")
page = DemoPage(src_page_dir)
@test page.stylesheet == abspath(src_page_dir, "assets", "emptystyle.css")
html_file = @suppress_err preview_demos(src_page_dir, require_html=true)
@test occursin("emptystyle.css", read(html_file, String))
@test "emptystyle.css" in readdir(joinpath(dirname(html_file), "stylesheet", "assets"))
end
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 2671 | @testset "generate" begin
root = "assets"
for theme in ("grid", )
templates = DemoCards.cardtheme(Val(Symbol(theme)))[1]
sec = DemoSection(joinpath(root, "section", "default"))
@test_reference joinpath("references", "generate_section_$theme.txt") generate(sec, templates) by=ignore_CR
page = DemoPage(joinpath(root, "page", "default"))
@test_reference joinpath("references", "generate_page_$theme.txt") generate(page, templates) by=ignore_all
end
abs_root = joinpath(pwd(), root, "page", "default")
mktempdir() do root
tmp_root = joinpath(root, "page", "default")
mkpath(tmp_root)
cp(abs_root, tmp_root, force=true)
templates, theme = cardtheme(root=root)
path, post_process_cb = @suppress_err makedemos(tmp_root, templates, root=root)
@test @suppress_err path == joinpath("default", "index.md")
@test theme == joinpath("democards", "gridtheme.css")
end
@testset "hidden" begin
page_dir = @suppress_err preview_demos(joinpath(root, "page", "hidden"); theme="grid", require_html=false)
md_index = joinpath(page_dir, "index.md")
# test only one card is shown in index.md
@test_reference joinpath("references", "hidden_index.txt") read(md_index, String) by=ignore_all
# check that assets are still normally generated even if they are hidden from index.md
@test readdir(page_dir) == ["covers", "index.md", "sec"]
@test readdir(joinpath(page_dir, "covers")) == ["democards_logo.svg"]
@test readdir(joinpath(page_dir, "sec")) == ["hidden1.ipynb", "hidden1.jl", "hidden1.md", "hidden2.md", "normal.md"]
end
@testset "throw_error" begin
@suppress_err @test_throws LoadError preview_demos(joinpath(root, "broken_demo.jl"); throw_error=true)
end
@testset "badges" begin
@testset "author" begin
for (dir, file) in [
("julia", joinpath(root, "card", "julia", "author_with_md_url.jl")),
("markdown", joinpath(root, "card", "markdown", "author_with_md_url.md"))
]
page_dir = @suppress_err preview_demos(file; theme="grid", require_html=false)
md_file = joinpath(page_dir, dir, "author_with_md_url.md")
@test isfile(md_file)
line = read(md_file, String)
@test occursin("[](https://github.com/johnnychen94)", line)
@test occursin("", line)
end
end
end
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 5337 | @testset "preview" begin
root = "assets"
abs_root = abspath(pwd(), root)
@testset "page structure" begin
page_dir = @suppress_err preview_demos(joinpath(abs_root, "page", "template"), theme="grid", require_html=false)
md_index = joinpath(page_dir, "index.md")
@test_reference joinpath("references", "preview", "index_page.md") read(md_index, String) by=ignore_all
@test readdir(page_dir) == ["covers", "index.md", "subsection_1", "subsection_2"]
@test readdir(joinpath(page_dir, "subsection_2")) == ["card_3.md", "card_4.md"]
for demo_file in filter(DemoCards.is_democard, readdir(joinpath(page_dir, "subsection_2")))
demo_file = joinpath(joinpath(page_dir, "subsection_2"), demo_file)
@test_reference joinpath("references", "preview", basename(demo_file)) read(demo_file, String) by=ignore_CR
end
@test "gridtheme.css" in readdir(joinpath(dirname(page_dir), "democards"))
end
@testset "section with non page structure" begin
page_dir = @suppress_err preview_demos(joinpath(abs_root, "preview", "scripts"), theme="grid", require_html=false)
md_index = joinpath(page_dir, "index.md")
@test_reference joinpath("references", "preview", "index_sec_nopage.md") read(md_index, String) by=ignore_all
@test readdir(page_dir) == ["assets", "covers", "index.md", "scripts"]
@test readdir(joinpath(page_dir, "covers")) == ["demo2.svg", "democards_logo.svg"]
@test readdir(joinpath(page_dir, "scripts")) == ["assets", "demo1.ipynb", "demo1.jl", "demo1.md", "demo2.md"]
end
@testset "section with page structure" begin
page_dir = @suppress_err preview_demos(joinpath(abs_root, "page", "template", "subsection_2"), theme="grid", require_html=false)
md_index = joinpath(page_dir, "index.md")
@test_reference joinpath("references", "preview", "index_sec_page.md") read(md_index, String) by=ignore_all
@test readdir(page_dir) == ["covers", "index.md", "subsection_2"]
@test readdir(joinpath(page_dir, "subsection_2")) == ["card_3.md", "card_4.md"]
end
@testset "section with invalid page structure" begin
@test_throws ArgumentError @suppress_err preview_demos(joinpath(abs_root, "page"))
end
@testset "file with non page structure" begin
page_dir = @suppress_err preview_demos(joinpath(abs_root, "preview", "scripts", "demo2.md"), theme="grid", require_html=false)
md_index = joinpath(page_dir, "index.md")
@test_reference joinpath("references", "preview", "index_file.md") read(md_index, String) by=ignore_all
@test readdir(page_dir) == ["assets", "covers", "index.md", "scripts"]
@test readdir(joinpath(page_dir, "covers")) == ["demo2.svg"]
@test readdir(joinpath(page_dir, "scripts")) == ["assets", "demo2.md"]
@test readdir(joinpath(page_dir, "scripts", "assets")) == ["logo.svg"]
page_dir = @suppress_err preview_demos(joinpath(abs_root, "preview_demo1.jl"), theme="grid", require_html=false)
md_index = joinpath(page_dir, "index.md")
@test_reference joinpath("references", "preview", "index_file2.md") read(md_index, String) by=ignore_all
@test readdir(page_dir) == ["covers", "index.md", "preview_section"]
@test readdir(joinpath(page_dir, "covers")) == ["democards_logo.svg"]
@test readdir(joinpath(page_dir, "preview_section")) == ["preview_demo1.ipynb", "preview_demo1.jl", "preview_demo1.md"]
end
@testset "file with page structure" begin
page_dir = @suppress_err preview_demos(joinpath(abs_root, "page", "template", "subsection_2", "card_3.md"), theme="grid", require_html=false)
md_index = joinpath(page_dir, "index.md")
@test_reference joinpath("references", "preview", "index_file_page.md") read(md_index, String) by=ignore_all
@test readdir(page_dir) == ["covers", "index.md", "subsection_2"]
@test readdir(joinpath(page_dir, "subsection_2")) == ["card_3.md"]
page_dir = @suppress_err preview_demos(joinpath(abs_root, "page", "template", "subsection_2", "card_3.md"), theme=nothing, require_html=false)
@test !isfile(joinpath(page_dir, "index.md"))
@test readdir(page_dir) == ["subsection_2"]
@test readdir(joinpath(page_dir, "subsection_2")) == ["card_3.md"]
end
index_page = @suppress_err preview_demos(joinpath(abs_root, "preview"), theme="grid", require_html=true)
@test isfile(index_page)
index_page = @suppress_err preview_demos(joinpath(abs_root, "preview"), require_html=true)
@test isfile(index_page)
@testset "don't copy unrelated assets" begin
src_page_dir = joinpath(abs_root, "preview")
@test readdir(src_page_dir) == ["assets", "other_scripts", "scripts"]
page_dir = @suppress_err preview_demos(joinpath(src_page_dir, "other_scripts", "nested", "demo3.jl"), theme="grid", require_html=false)
# `assets` is copied, but `scripts` is not
@test readdir(page_dir) == ["assets", "covers", "index.md", "other_scripts"]
@test readdir(joinpath(page_dir, "other_scripts")) == ["assets", "nested"]
@test readdir(joinpath(page_dir, "other_scripts", "nested")) == ["assets", "demo3.ipynb", "demo3.jl", "demo3.md"]
end
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 672 | using DemoCards
using DemoCards: democard, MarkdownDemoCard, JuliaDemoCard, DemoSection, DemoPage
using DemoCards: generate
using HTTP
using Test, ReferenceTests, Suppressor
using Dates
include("compat.jl")
include("testutils.jl")
# support both `include("runtests.jl")` and `include("test/runtests.jl")`
proj_root = basename(pwd()) == "test" ? abspath(pwd(), "..") : pwd()
test_root = joinpath(proj_root, "test")
cd(test_root) do
include("types/card.jl")
include("types/section.jl")
include("types/page.jl")
include("generate.jl")
include("preview.jl")
include("show.jl")
include("utils.jl")
include("configurations.jl")
end
nothing
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 311 | @testset "show" begin
root = "assets"
sec = DemoSection(joinpath(root, "section", "default"))
@test_reference joinpath("references", "section.txt") sec by=ignore_CR
page = DemoPage(joinpath(root, "page", "default"))
@test_reference joinpath("references", "page.txt") page by=ignore_CR
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 1956 | """
This function combines `ignore_CR` with ignoring difference between `ref` and
`actual` resulting from blank lines.
"""
ignore_all(ref::AbstractString, actual::AbstractString) = isequal(_ignore_all(ref), _ignore_all(actual))
_ignore_all(x::AbstractString) = _ignore_tempdir(_ignore_blank_line_and_CR(_ignore_CR(x)))
const edit_url_re = r"EditURL = \"(.*)\""
function _ignore_tempdir(doc::AbstractString)
path_match = match(edit_url_re, doc)
if path_match === nothing
return doc
end
path = unescape_string(path_match[1])
if !startswith(path, "/") && match(r"^[A-Z]:", path) === nothing
# all we need to do is normalise the path separator
norm_dir = replace(path, "\\" => "/")
else
# we need to remove the tempdir part of the path
bits = split(replace(path, "\\" => "/"), "/")
tmp_dir = findlast(x -> startswith(x, "jl_"), bits)
if tmp_dir === nothing
error("Cannot find tempdir in $path")
end
norm_dir = "/TEMPDIR/" * join(bits[end+1:end], "/")
end
return replace(doc, edit_url_re => "EditURL = \"$norm_dir\"")
end
"""
This function combines `ignore_CR` with ignoring difference between `ref` and
`actual` resulting from blank lines.
"""
ignore_blank_line_and_CR(ref::AbstractString, actual::AbstractString) = isequal(_ignore_blank_line_and_CR(ref), _ignore_blank_line_and_CR(actual))
_ignore_blank_line_and_CR(x::AbstractString) = _ignore_blank_line(_ignore_CR(x))
_ignore_blank_line(x::AbstractString) = replace(x, r"\n+"=>"\n")
"""
ignore_CR(ref::AbstractString, actual::AbstractString)
Ignore the CRLF(`\\r\\n`) and LF(`\\n`) difference by removing `\\r` from the given string.
CRLF format is widely used by Windows while LF format is mainly used by Linux.
"""
ignore_CR(ref::AbstractString, actual::AbstractString) = isequal(_ignore_CR(ref), _ignore_CR(actual))
_ignore_CR(x::AbstractString) = replace(x, "\r\n"=>"\n")
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 3254 | using DemoCards: democard, walkpage, DemoPage
@testset "regexes" begin
root = joinpath("assets", "regexes")
@testset "title" begin
config_1_files = ("example_1.md",
"example_3.md",
"example_4.md",
"example_6.md",
"example_7.md",
"example_8.md",
"example_1.jl",
"example_4.jl",
"example_5.jl",
"example_6.jl",
"example_8.jl",
"example_9.jl",
"example_10.jl",
"example_11.jl",
"example_12.jl",
"example_13.jl")
foreach(config_1_files) do filename
card = democard(joinpath(root, filename))
@test card.title == "This is a title"
@test card.id == "This-is-a-title"
@test card.description == "This is a description"
end
config_2_files = ("example_2.md", "example_2.jl", "example_3.jl")
foreach(config_2_files) do filename
card = democard(joinpath(root, filename))
@test card.title == "This is a title"
@test card.id == "custom_id"
@test card.description == "This is a description"
end
config_3_files = ("example_5.md", "example_7.jl")
foreach(config_3_files) do filename
card = democard(joinpath(root, filename))
@test card.title == "This is a title"
@test card.id == "This-is-a-title"
@test card.description == "This is a description that spreads along multiple lines"
end
end
# only lines before body contents can be potential titles
config = DemoCards.parse(Val(:Julia), joinpath(root, "title_before_body.jl"))
@test haskey(config, "description")
@test config["description"] == "This is parsed as a description line --- [1]"
@test !haskey(config, "title")
@test !haskey(config, "id")
@test !haskey(config, "cover")
config = DemoCards.parse(Val(:Markdown), joinpath(root, "title_before_body.md"))
@test haskey(config, "description")
@test config["description"] == "This is parsed as a description line --- [1]"
@test !haskey(config, "title")
@test !haskey(config, "id")
@test !haskey(config, "cover")
end
@testset "walkpage" begin
page = DemoPage(joinpath("assets", "page", "hidden"))
reference = "Hidden" => ["hidden1.jl", "hidden2.md", "normal.md"]
@test reference == walkpage(page) do item
basename(item.path)
end
reference = "Hidden" => ["Sec" => ["hidden1.jl", "hidden2.md", "normal.md"]]
@test reference == walkpage(page; flatten=false) do item
basename(item.path)
end
page = DemoPage(joinpath("assets", "page", "one_card"))
reference = "One card" => ["card.md"]
@test reference == walkpage(page) do item
basename(item.path)
end
reference = "One card" => ["Section" => ["Subsection" => ["card.md"]]]
@test reference == walkpage(page; flatten=false) do item
basename(item.path)
end
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 109 | # ---
# author: "[Johnny Chen](https://github.com/johnnychen94); Jane Doe"
# ---
# author with markdown url
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 69 | # 
# First valid image link is the cover
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 190 | # 
# <!--  -->
# 
# <!--  -->
# First valid image link is the cover
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 68 | # ---
# cover: ../logo.png
# ---
# Configure cover in front matter
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 106 | # ---
# cover: nonexistence.jpg
# ---
# 
# front matter overrides image link
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 150 | # ---
# title: Custom Title
# description: >
# this is a single line
# destiption that spans
# over multiple lines
# ---
# This is the content
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 149 | # ---
# title: Custom Title
# description: |
# this is a multi line
# destiption that spans
# over multiple lines
# ---
# This is the content
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 29 | # ---
# notebook: true
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 30 | # ---
# notebook: false
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 30 | # ---
# notebook: False
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 32 | # ---
# notebook: nothing
# ---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 40 | # # Custom Title
# This is the content
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 61 | # # [Custom Title 2](@id custom_id_2)
# This is the content
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 110 | # # Custom Title 3-1
# This is the content
# # [Custom Title 3-2](@id custom_id_3-2)
# This is the content
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 57 | # ---
# title: Custom Title
# ---
# This is the content
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 91 | # ---
# title: Custom Title
# description: Custom Description
# ---
# This is the content
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 73 | # ---
# title: Custom Title
# id: custom_id
# ---
# This is the content
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 184 | #---
#title: Custom Title
#id: custom_id
#author: Jane Doe; John Roe
#date: 2020-01-31
#julia: 1.2.3
#cover: https://juliaimages.org/latest/assets/logo.png
#---
# This is the content
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 164 | #! /usr/local/bin
# comments are allowed before frontmatter
# empty lines are allowed, too
#---
#title: Custom Title
#id: custom_id
#---
# This is the content
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 39 | # notebook will be generated
x = 1 + 2
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 40 | # ---
# notebook: false
# ---
y = 1 + 2
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 43 | # notebook will not be generated
y = 1 + 2
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 39 | # ---
# notebook: true
# ---
x = 1 + 2
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 55 | #---
#hidden: true
#---
# This is a hidden julia card
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 45 | # # This is a title
# This is a description
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 128 | # # This is a title
#!jl This is a description
# # [This is another title](@id custom_id)
# This isn't a description
x = 1+1
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 98 | # # This is a title
# # [This is another title](@id custom_id)
# This is a description
x = 1+1
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 123 | # # This is a title
#
# ```
# x = 1+1
# ```
# # [This is another title](@id custom_id)
# This is a description
y = 2+2
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 147 | # # This is a title
## This is a comment
### This is also a comment
# # [This is another title](@id custom_id)
# This is a description
y = 2+2
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 62 | # # [This is a title](@id custom_id)
# This is a description
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 88 | # # [This is a title](@id custom_id)
# # This is another title
# This is a description
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 125 | # # This is a title
# This is a description
# # [This is another title](@id custom_id)
# This isn't a description
x = 1+1
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 90 | #md # This is a title
# # [This is another title](@id custom_id)
# This is a description
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 91 | #!jl # This is a title
# # [This is another title](@id custom_id)
# This is a description
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 135 | # # This is a title
# This is a description
# that spreads along
# multiple lines
# # [This is another title](@id custom_id)
x = 1+1
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 143 | # # This is a title
# [This is a description](@ref custom_id)
# # [This is another title](@id custom_id)
# This isn't a description
x = 1+1
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 127 | # # This is a title
#md This is a description
# # [This is another title](@id custom_id)
# This isn't a description
x = 1+1
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 267 | # ---
# title: this has higher priority than [2]
# description: this has higher priority than [1]
# ---
# This is parsed as a description line --- [1]
# # this is a section title --- [2]
# but won't be recognized as a card title because it's put after the contents
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 89 | # This file was generated using Literate.jl, https://github.com/fredrikekre/Literate.jl
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 190 | @testset "AbstractDemoCard" begin
for cardtype in ("markdown", "julia")
cd(joinpath("assets", "card", cardtype)) do
include("$(cardtype).jl")
end
end
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 6022 | @testset "JuliaDemoCard" begin
# default behavior
simplest = democard("simplest.jl")
@test simplest.cover === nothing
@test simplest.id == "Simplest"
@test simplest.path == "simplest.jl"
@test simplest.title == "Simplest"
@test simplest.description == "This is the content"
@testset "parse" begin
@testset "title, id and description" begin
# JuliaDemoCard doesn't parse title from the markdown contents
title_1 = JuliaDemoCard("title_1.jl")
@test title_1.id == "Custom-Title"
@test title_1.title == "Custom Title"
@test title_1.description == "This is the content"
@test title_1.hidden == false
@test title_1.author == ""
@test title_1.date == DateTime(0)
title_2 = JuliaDemoCard("title_2.jl")
@test title_2.id == "custom_id_2"
@test title_2.title == "Custom Title 2"
@test title_2.description == "This is the content"
title_3 = JuliaDemoCard("title_3.jl")
@test title_3.id == "Custom-Title-3-1"
@test title_3.title == "Custom Title 3-1"
@test title_3.description == "This is the content"
title_4 = JuliaDemoCard("title_4.jl")
@test title_4.id == "Custom-Title"
@test title_4.title == "Custom Title"
@test title_4.description == "This is the content"
title_5 = JuliaDemoCard("title_5.jl")
@test title_5.id == "Custom-Title"
@test title_5.title == "Custom Title"
@test title_5.description == "Custom Description"
title_6 = JuliaDemoCard("title_6.jl")
@test title_6.id == "custom_id"
@test title_6.title == "Custom Title"
@test title_6.description == "This is the content"
title_7 = JuliaDemoCard("title_7.jl")
@test title_7.id == "custom_id"
@test title_7.title == "Custom Title"
@test title_7.description == "This is the content"
@test title_7.hidden == false
@test title_7.author == "Jane Doe; John Roe"
@test title_7.date == DateTime("2020-01-31")
@test title_7.cover == "https://juliaimages.org/latest/assets/logo.png"
title_8 = JuliaDemoCard("title_8.jl")
@test title_8.id == "custom_id"
@test title_8.title == "Custom Title"
@test title_8.description == "This is the content"
description_1 = JuliaDemoCard("description_1.jl")
@test description_1.id == "Custom-Title"
@test description_1.title == "Custom Title"
@test description_1.description == "this is a single line destiption that spans over multiple lines\n"
description_2 = JuliaDemoCard("description_2.jl")
@test description_2.id == "Custom-Title"
@test description_2.title == "Custom Title"
@test description_2.description == "this is a multi line\ndestiption that spans\nover multiple lines\n"
end
@testset "cover" begin
cover_1 = JuliaDemoCard("cover_1.jl")
@test cover_1.cover == nothing
cover_2 = JuliaDemoCard("cover_2.jl")
@test cover_2.cover == joinpath("..", "logo.png")
cover_3 = JuliaDemoCard("cover_3.jl")
@test cover_3.cover == "nonexistence.jpg"
cover_4 = JuliaDemoCard("cover_4.jl")
@test cover_4.cover == joinpath("..", "logo.png")
end
@testset "version" begin
card = JuliaDemoCard("version_1.jl")
card.julia == v"1.2.3"
card = JuliaDemoCard("version_2.jl")
card.julia == v"999.0.0"
warn_msg = @capture_err preview_demos("version_2.jl"; require_html=false)
@test occursin("The running Julia version `$(VERSION)` is older than the declared compatible version `$(card.julia)`.", warn_msg)
end
@testset "notebook" begin
card = JuliaDemoCard("notebook_1.jl")
@test card.notebook == true
card = JuliaDemoCard("notebook_2.jl")
@test card.notebook == false
card = JuliaDemoCard("notebook_3.jl")
@test card.notebook == false
card = @suppress_err JuliaDemoCard("notebook_4.jl")
@test card.notebook == nothing
warn_msg = @capture_err JuliaDemoCard("notebook_4.jl")
@test occursin("`notebook` option should be either `\"true\"` or `\"false\"`, instead it is: nothing. Fallback to unconfigured.", warn_msg)
end
@testset "generate" begin
page_dir = @suppress_err preview_demos("title_7.jl", theme="grid", require_html=false)
card_dir = joinpath(page_dir, "julia")
function strip_notebook(contents)
# the notebook contents are different in CI and non-CI cases
# [](notebook_url)
replace(contents, r"\[!\[notebook\]\(.*\)\]\(.*\.ipynb\) " => "")
end
isempty(DemoCards.get_nbviewer_root_url("master")) || println(read(joinpath(card_dir, "title_7.md"), String)) # for debug usage
@test_reference joinpath(test_root, "references", "cards", "julia_md.md") strip_notebook(read(joinpath(card_dir, "title_7.md"), String)) by=ignore_all
@test_reference joinpath(test_root, "references", "cards", "julia_src.jl") read(joinpath(card_dir, "title_7.jl"), String) by=ignore_all
@test isfile(joinpath(card_dir, "title_7.ipynb"))
# check if notebook keyword actually affects the generation
page_dir = @suppress_err preview_demos("notebook_2.jl", theme="grid", require_html=false)
card_dir = joinpath(page_dir, "julia")
contents = read(joinpath(card_dir, "notebook_2.md"), String)
@test !occursin("[notebook]", contents)
end
end
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 3769 | @testset "MarkdownDemoCard" begin
# default behavior
simplest = democard("simplest.md")
@test simplest.cover === nothing
@test simplest.id == "Simplest"
@test simplest.path == "simplest.md"
@test simplest.title == "Simplest"
@test simplest.description == "This is the content"
@testset "parse" begin
@testset "title, id and description" begin
# MarkdownDemoCard doesn't parse title from the markdown contents
title_1 = MarkdownDemoCard("title_1.md")
@test title_1.id == ".-Custom-Title"
@test title_1.title == "1. Custom Title"
@test title_1.description == "This is the content"
@test title_1.hidden == false
@test title_1.author == ""
@test title_1.date == DateTime(0)
title_2 = MarkdownDemoCard("title_2.md")
@test title_2.id == "custom_id_2"
@test title_2.title == "Custom Title 2"
@test title_2.description == "This is the content"
title_3 = MarkdownDemoCard("title_3.md")
@test title_3.id == "Custom-Title-3-1"
@test title_3.title == "Custom Title 3-1"
@test title_3.description == "This is the content"
title_4 = MarkdownDemoCard("title_4.md")
@test title_4.id == "Custom-Title"
@test title_4.title == "Custom Title"
@test title_4.description == "This is the content"
title_5 = MarkdownDemoCard("title_5.md")
@test title_5.id == "Custom-Title"
@test title_5.title == "Custom Title"
@test title_5.description == "Custom Description"
title_6 = MarkdownDemoCard("title_6.md")
@test title_6.id == "custom_id"
@test title_6.title == "Custom Title"
@test title_6.description == "This is the content"
@test title_6.hidden == false
@test title_6.author == "Jane Doe; John Roe"
@test title_6.date == DateTime("2020-01-31")
@test title_6.cover == "https://juliaimages.org/latest/assets/logo.png"
title_7 = MarkdownDemoCard("title_7.md")
@test title_7.id == "custom_id"
@test title_7.title == "Custom Title"
@test title_7.description == "This is the content"
description_1 = MarkdownDemoCard("description_1.md")
@test description_1.id == "Custom-Title"
@test description_1.title == "Custom Title"
@test description_1.description == "this is a single line destiption that spans over multiple lines\n"
description_2 = MarkdownDemoCard("description_2.md")
@test description_2.id == "Custom-Title"
@test description_2.title == "Custom Title"
@test description_2.description == "this is a multi line\ndestiption that spans\nover multiple lines\n"
end
@testset "cover" begin
cover_1 = MarkdownDemoCard("cover_1.md")
@test cover_1.cover == nothing
cover_2 = MarkdownDemoCard("cover_2.md")
@test cover_2.cover == joinpath("..", "logo.png")
cover_3 = MarkdownDemoCard("cover_3.md")
@test cover_3.cover == "nonexistence.jpg"
cover_4 = MarkdownDemoCard("cover_4.md")
@test cover_4.cover == joinpath("..", "logo.png")
end
@testset "generate" begin
page_dir = @suppress_err preview_demos("title_6.md", theme="grid", require_html=false)
card_path = joinpath(page_dir, "markdown", "title_6.md")
@test_reference joinpath(test_root, "references", "cards", "markdown.md") read(card_path, String) by=ignore_all
end
end
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 2685 | using DemoCards: infer_pagedir, is_demosection, is_democard
@testset "DemoPage" begin
root = joinpath("assets", "page")
# default DemoPage behavior
page = DemoPage(joinpath(root, "default"))
@test page.title == "Default"
sec1, sec2 = page.sections
@test sec1.root == joinpath(page.root, "subsection_1")
@test sec2.root == joinpath(page.root, "subsection_2")
# title and order
page = DemoPage(joinpath(root, "title_and_order"))
@test page.title == "Custom Title"
sec1, sec2, sec3, sec4 = page.sections
@test sec1.root == joinpath(page.root, "subsection_4")
@test sec2.root == joinpath(page.root, "subsection_3")
@test sec3.root == joinpath(page.root, "subsection_1")
@test sec4.root == joinpath(page.root, "subsection_2")
# template
page = DemoPage(joinpath(root, "template"))
@test page.title == "Custom Title"
# template
page = DemoPage(joinpath(root, "template_2"))
@test page.title == "Custom Title"
# template has higher priority
page = DemoPage(joinpath(root, "suppressed_title"))
@test page.title == "Custom Title"
@testset "empty demo section" begin
page_dir = joinpath("assets", "section", "empty")
mkpath(joinpath(page_dir, "sec1")) # empty folder cannot be commited into git history
page = @suppress_err DemoPage(page_dir)
@test length(page.sections) == 1
warn_msg = @capture_err DemoPage(page_dir)
@test occursin("Empty section detected", warn_msg)
@test_throws ErrorException("Empty demo page, you have to add something.") @suppress_err begin
preview_demos(joinpath("assets", "section", "empty", "sec1"))
end
@test isempty(readdir(joinpath(page_dir, "sec1")))
rm(joinpath(page_dir, "sec1"))
end
end
@testset "infer_pagedir" begin
for pagename in ["default", "suppressed_title", "template", "template_2", "title_and_order"]
page_dir = joinpath("assets", "page", pagename)
for (root, dirs, files) in walkdir(page_dir)
for dir in dirs
secdir = joinpath(root, dir)
is_demosection(secdir) && @test infer_pagedir(secdir) == page_dir
end
for file in files
cardpath = joinpath(root, file)
is_democard(cardpath) && @test infer_pagedir(cardpath) == page_dir
end
end
end
# when no page structure is detected, return nothing
@test infer_pagedir("assets") |> isnothing
@test infer_pagedir(joinpath("assets", "regexes")) |> isnothing
@test infer_pagedir(joinpath("assets", "regexes", "example_1.jl")) |> isnothing
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | code | 1954 | @testset "DemoSection" begin
root = joinpath("assets", "section")
# default section behavior
sec = DemoSection(joinpath(root, "default"))
@test sec.title == "Default"
@test sec.cards == []
subsec1, subsec2 = sec.subsections
@test subsec1.title == "Subsection 1"
@test subsec2.title == "Subsection 2"
@test subsec1.subsections == []
@test subsec2.subsections == []
card1, card2 = subsec1.cards
card3, card4 = subsec2.cards
@test card1.path == joinpath(subsec1.root, "card_1.md")
@test card2.path == joinpath(subsec1.root, "card_2.md")
@test card3.path == joinpath(subsec2.root, "card_3.md")
@test card4.path == joinpath(subsec2.root, "card_4.md")
# only config subsection_1
sec = DemoSection(joinpath(root, "partial_config"))
@test sec.title == "Partial Config"
@test sec.cards == []
subsec1, subsec2 = sec.subsections
@test subsec1.title == "Subsection 1"
@test subsec2.title == "Subsection 2"
@test subsec1.subsections == []
@test subsec2.subsections == []
card1, card2 = subsec1.cards
card3, card4 = subsec2.cards
@test card1.path == joinpath(subsec1.root, "card_2.md")
@test card2.path == joinpath(subsec1.root, "card_1.md")
@test card3.path == joinpath(subsec2.root, "card_3.md")
@test card4.path == joinpath(subsec2.root, "card_4.md")
# invalid cases
@test_throws ArgumentError @suppress_err DemoSection(joinpath(root, "partial_order"))
@test_throws ArgumentError @suppress_err DemoSection(joinpath(root, "cards_and_subsections"))
@testset "unmatched dirty files" begin
msg = @capture_err DemoSection(joinpath(root, "dirty"))
@test occursin("skip unmatched file: \"data.csv\", \"script.py\"", msg)
sec = @suppress_err DemoSection(joinpath(root, "dirty"))
# unmatched files are excluded during the structure building stage.
@test length(sec.cards) == 1
end
end
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 5042 | # DemoCards
| **Documentation** | **Build Status** |
|:-------------------------------------------------------------------------------:|:-------------------------------------------------------------:|
| [![][docs-stable-img]][docs-stable-url] [![][docs-dev-img]][docs-dev-url] | [![][action-img]][action-url] [![][codecov-img]][codecov-url] |
This package is used to *dynamically* generate a demo page and integrate with [Documenter.jl](https://github.com/JuliaDocs/Documenter.jl).
_Let's focus on writing demos_
## Overview
* a plugin package to `Documenter.jl` to manage all your demos.
* folder structure is the demo structure.
* minimal configuration.
* CI friendly
* support demos in markdown and julia format.

The philosophy of DemoCards is "folder structure is the structure of demos"; organizing folders and files in
the a structural way, then `DemoCards.jl` will help manage how you navigate through the pages.
```text
examples
βββ part1
β βββ assets
β βββ demo_1.md
β βββ demo_2.md
β βββ demo_3.md
βββ part2
βββ demo_4.jl
βββ demo_5.jl
```
DemoCards would understand it in the following way:
```text
# Examples
## Part1
demo_1.md
demo_2.md
demo_3.md
## Part2
demo_4.jl
demo_5.jl
```
Read the [Quick Start](https://democards.juliadocs.org/stable/quickstart/) for more instructions.
## Examples
| repo | theme |
| :---- | :---- |
| [AlgebraOfGraphics.jl](https://aog.makie.org/stable/gallery/) | [![][theme-bulmagrid-img]][theme-bulmagrid] |
| [Augmentor.jl](https://evizero.github.io/Augmentor.jl/dev/operations/) | [![][theme-grid-img]][theme-grid] |
| [Bokeh.jl](https://cjdoris.github.io/Bokeh.jl/dev/gallery/) | [![][theme-bokehlist-img]][theme-bokehlist] |
| [Deneb.jl](https://brucala.github.io/Deneb.jl/dev/examples/) | [![][theme-transitiongrid-img]][theme-transitiongrid] |
| [FractionalDiffEq.jl](https://scifracx.org/FractionalDiffEq.jl/dev/ChaosGallery/) | [![][theme-bulmagrid-img]][theme-bulmagrid] |
| [LeetCode.jl](https://cn.julialang.org/LeetCode.jl/dev/) | [![][theme-none-img]][theme-none] |
| [Images.jl](https://juliaimages.org/latest/examples/) | [![][theme-grid-img]][theme-grid] |
| [ImageMorphology.jl](https://juliaimages.org/ImageMorphology.jl/stable/operators/) | [![][theme-list-img]][theme-list] |
| [ReinforcementLearning.jl](https://juliareinforcementlearning.org/docs/experiments/) | [![][theme-grid-img]][theme-grid] |
| [Plots.jl](https://docs.juliaplots.org/dev/user_gallery/) | [![][theme-bulmagrid-img]][theme-bulmagrid] |
| [PSSFSS.jl](https://simonp0420.github.io/PSSFSS.jl/stable/PSS_&_FSS_Element_Gallery/) | [![][theme-bulmagrid-img]][theme-bulmagrid] |
## Caveat Emptor
The use of this package heavily relies on [Documenter.jl](https://github.com/JuliaDocs/Documenter.jl),
[Literate.jl](https://github.com/fredrikekre/Literate.jl), [Mustache.jl](https://github.com/jverzani/Mustache.jl)
and others. Unfortunately, I'm not a contributor of any. This package also uses a lot of Regex, which I know little.
The initial purpose of this package is to set up the [demo page](https://juliaimages.org/latest/examples) of JuliaImages.
I'm not sure how broadly this package suits the need of others, but I'd like to accept any issues/PRs on improving the usage experience.
[docs-dev-img]: https://img.shields.io/badge/docs-dev-blue.svg
[docs-dev-url]: https://democards.juliadocs.org/dev
[docs-stable-img]: https://img.shields.io/badge/docs-stable-blue.svg
[docs-stable-url]: https://democards.juliadocs.org/stable
[action-img]: https://github.com/JuliaDocs/DemoCards.jl/workflows/Unit%20test/badge.svg
[action-url]: https://github.com/JuliaDocs/DemoCards.jl/actions
[codecov-img]: https://codecov.io/gh/JuliaDocs/DemoCards.jl/branch/master/graph/badge.svg
[codecov-url]: https://codecov.io/gh/JuliaDocs/DemoCards.jl
[pkgeval-img]: https://juliaci.github.io/NanosoldierReports/pkgeval_badges/D/DemoCards.svg
[pkgeval-url]: https://juliaci.github.io/NanosoldierReports/pkgeval_badges/report.html
[theme-bulmagrid-img]: https://img.shields.io/badge/theme-bulmagrid-blue.svg
[theme-transitiongrid-img]: https://img.shields.io/badge/theme-transitiongrid-blue.svg
[theme-grid-img]: https://img.shields.io/badge/theme-grid-blue.svg
[theme-list-img]: https://img.shields.io/badge/theme-list-blue.svg
[theme-bokehlist-img]: https://img.shields.io/badge/theme-bokehlist-blue.svg
[theme-none-img]: https://img.shields.io/badge/theme-none-blue.svg
[theme-bulmagrid]: https://democards.juliadocs.org/stable/bulmagrid/
[theme-transitiongrid]: https://democards.juliadocs.org/stable/transitiongrid/
[theme-grid]: https://democards.juliadocs.org/stable/grid/
[theme-list]: https://democards.juliadocs.org/stable/list/
[theme-bokehlist]: https://democards.juliadocs.org/stable/bokehlist/
[theme-none]: https://democards.juliadocs.org/stable/themeless/markdown/item1/
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 5617 | # [Quick Start](@id quickstart)
This section describes how you can set up your demos easily in _five lines of codes_.
## Manage your demo files
The rules of demo management are super simple:
* you need one demo page (folder) to hold all the demo files
* a demo page has several demo sections (subfolders)
* a demo section either
* has other demo sections as nested subsections, or,
* has the demo files (`.md`, `.jl`)
In the following example:
* we have one demo page: `"quickstart"` ---> The current page you're looking at
* `"quickstart"` has one demo section: `"usage example"`
* `"usage example"` has two demo subsections: `"basics"` and `"julia_demos"`
* `"basics"` section holds all markdown demos
* `"julia_demos"` section holds all julia demos
* `"assets"` folders are ignored by `DemoCards.jl`
* `"index.md"` is where all demo cards are organized in (aka page template)
* `"config.json"` are configuration files (there are many of them!)
```text
docs/quickstart
βββ config.json
βββ index.md
βββ usage_example
βββ basics
β βββ assets
β βββ config.json
β βββ configure_card.md
β βββ configure_sec_and_page.md
β βββ hidden_card.jl
β βββ hide_your_card_from_index.md
β βββ simple_markdown_demo.md
βββ config.json
βββ julia_demos
βββ 1.julia_demo.jl
βββ 2.cover_on_the_fly.jl
βββ assets
βββ config.json
```
This is the core idea, as long as you organize your folder in a structural way, DemoCards will read
and process your demos to map your folder structure.
```@repl simplest_demopage
using DemoCards
cd(pkgdir(DemoCards)) do
DemoCards.DemoPage("docs/quickstart")
end
```
## Deploy your demo page

The above image is the workflow of `DemoCards`. The deployment would be pretty easy:
1. pass your demos to `makedemos`, so that they're preprocessed before passing into `Documenter.jl`
2. generate the entire documentation using `Documenter.makedocs`
3. post process the generated demos using callbacks.
```julia
# 1. generate demo files
demopage, postprocess_cb, demo_assets = makedemos("demos") # this is the relative path to docs/
# if there are generated css assets, pass it to Documenter.HTML
assets = []
isnothing(demo_assets) || (push!(assets, demo_assets))
# 2. normal Documenter usage
format = Documenter.HTML(assets = assets)
makedocs(format = format,
pages = [
"Home" => "index.md",
demopage,
],
sitename = "Awesome demos")
# 3. postprocess after makedocs
postprocess_cb()
```
In this example, there are three returned objects from `makedemos`:
* `demopage`: this is the relative path to the generated demos (typically in `src`), you
can pass it to `makedocs`'s `pages`.
* `postprocess_cb`: this is the callback function that you'll need to call after `makedocs`.
* `demo_assets`: if available, it is the path to css file which you could pass to `Documenter.HTML`
as style assets. If no theme is configured for your page, it will be `nothing.
After it's set up, you can now focus on contributing more demos and leave
other works to `DemoCards.jl`.
π The following example grids are generated using `DemoCards`! You can read them one by one for
advanced configuration helps. Or you could read the [Concepts](@ref concepts) page first to get
better understanding about the DemoCards type system.
---
{{{democards}}}
---
## Page Themes
The page you are reading right now is the generated index page of demos. An index page always have
cover images for the demos. An index page is only generated if you configure the `theme` option in
your page config file (e.g., `docs/quickstart/config.json`):
```json
{
"theme": "grid"
}
```
There are three themes available now:
* `"nothing"`(default): index page and associated assets will not be generated, instead, it will use
the sidebar generated by Documenter.jl to navigate through pages. This is the default option when
you doesn't configure `"theme"`.
* `"grid"`, `"bulmagrid"` and `"list"`: an index page and associated cover images are generated
Please check the "Theme Gallery" part for a preview of these themes.
!!! note "custom stylesheet"
One could optionally override the default stylesheet with `"stylesheet"` entry in page config file:
```json
{
"theme": "grid",
"stylesheet": "relative/path/to/stylesheet.css"
}
```
## What DemoCards.jl does
The pipeline of [`makedemos`](@ref DemoCards.makedemos) is:
1. analyze the structure of your demo folder and extracts supplementary configuration information
2. copy "`assets`" folder without processing
3. preprocess demo files and save it
4. (optional) process and save cover images
5. (optional) generate index page
6. generate a postprocessing callback function
Since all files are generated to `docs/src`, the next step is to leave everything else
to `Documenter.jl` π―
!!! warning
By default, `makedemos` generates all the necessary files to `docs/src`, this means that the
data you pass to `makedemos` should not be placed at `docs/src`.
For advanced usage of `DemoCards.jl`, you need to understand the core [concepts](@ref concepts) of it.
!!! warning
Currently, there's no guarantee that this function works for untypical
documentation folder structure. By the word **typical**, it is:
```julia
.
βββ Project.toml
βββ docs/
β βββ demos/ # manage your demos outside docs/src
β βββ make.jl
β βββ Project.toml
β βββ src/
βββ src/
βββ test/
```
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 2950 | ---
title: Configure your card
cover: assets/logo.svg
id: configure_your_card
author: "[Johnny Chen](https://github.com/johnnychen94); Jane Doe"
date: 2020-09-13
description: This demo show you how to pass additional meta info of card to DemoCards.jl
---
This page is generated from a markdown file. In `DemoCards.jl`'s type system, it is called
[`MarkdownDemoCard`](@ref DemoCards.MarkdownDemoCard) whose contents are written in the markdown
format.
The markdown files are almost directly passed to `Documenter.jl`, check the
[syntax of Documenter.jl](https://juliadocs.github.io/Documenter.jl/stable/man/syntax/)
if you are unfamiliar with the Julia flavor markdown syntax.
DemoCards extracts potential information from the file to build the index page, e.g., name, title,
reference id, cover image file/URL. If that's not available, then you would need to configure them
by adding a [YAML format front matter](https://jekyllrb.com/docs/front-matter/).
Supported YAML keywords are list as follows:
* `cover`: an URL or a relative path to the cover image. If not configured, it would use the DemoCard logo.
* `description`: a multi-line description to this file, will be displayed when the demo card is hovered.
* `id`: specify the `id` tag for cross-references.
* `title`: one-line description to this file, will be displayed under the cover image.
* `author`: author name. If there are multiple authors, split them with semicolon `;`.
* `date`: any string contents that can be passed to `Dates.DateTime`.
* `hidden`: whether this card is shown in the layout of index page.
!!! tip
All these YAML configs are optional. If specified, it has higher priority over the meta info
extracted from the demo contents. For example, if you don't like the inferred demo title, then
specify one explicitly in the YAML frontmatter.
Of course, you have to make sure the `---` flag shows at the first line of the markdown file,
otherwise DemoCards would just read them as normal contents.
For example, the markdown file of this page uses the following frontmatter:
```markdown
---
title: Configure your card
cover: assets/logo.svg
id: configure_your_card
author: "[Johnny Chen](https://github.com/johnnychen94); Jane Doe"
date: 2020-09-13
description: This demo show you how to pass additional meta info of card to DemoCards.jl
---
```
As you can see, if configured, there will be badges for `author` and `date` info. If there are
multiple authors, they could be splitted by semicolon `;`. For example, `author: Jane Doe; John Roe`
would generate two author badges.
!!! tip
If `author` is configured as markdown url format, then the generated badge will be clickable.
!!! warning
A badly formatted YAML frontmatter will currently trigger a build failure with perhaps hard to
understand error. Sometimes, you need to assist YAML parser by explicitly quoting the content
with `""`. See the author field above as an instance.
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 2436 | ---
title: Configure your section and page
cover: assets/logo.svg
---
This demo shows you how to configure your demo page and sections.
By default, a demo section takes the folder name as its section title, and it
takes the file orders as its card orders, which can be unsatisfying in many cases.
Luckily, `DemoCards.jl` reads the `config.json` file in the section folder, for example:
```json
{
"title": "Basic Usage",
"order": [
"simple_markdown_demo.md",
"configure_with_yaml.md"
]
}
```
A demo page is maintained in a similar manner, except that it needs extra keys to configure the template
page. For example:
```json
{
"template": "template.md",
"theme": "grid",
"order": [
"basic",
"advanced"
]
}
```
If `template` is not specified, `"index.md"` will be used if available, otherwise, it will use a
blank template generated by DemoCards.
There are three possible options for `theme`:
* `"nothing"`: It won't generate the index page, instead, it will use the sidebar generated by
Documenter for navigation purpose. `template` option doesn't affect, neither.
* `"grid"`: a grid like index page.
* `"list"`: a list like index page.
* `"nocoverlist"`: a list like index page without cover images.
You can check the "Theme Gallery" to see how different themes look like.
## [Override default values with properties](@id page_sec_properties)
Another item that could be useful is `properties`, it is written as a dictionary-like format, and
provides a way to override some default values. Properties can be configured at any level and it
follows very simple rules:
- Properties at a higher level item can be propagated into lower level items
- Properties at lower level items are of higher priority
```text
example_page/
βββ config.json
βββ sec1
β βββ config.json
β βββ demo1.jl
β βββ demo2.jl
β βββ demo3.md
βββ sec2
βββ demo4.jl
βββ demo5.jl
```
For example, in the above folder structure, if we configure `example_page/config.json` with
```json
{
"properties":{
"notebook": "false"
}
}
```
Then all julia demos will not generate jupyter notebook unless it's override somewhere in the lower
level, e.g, `sec1/config.json` or `demo1.jl`.
Only a subset of demo entries support the property propagation, currently supported items are:
* Julia files: `notebook` allows you to enable/disable the jupyter notebook generation.
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 1327 | ---
title: Hide your card from page index layout
description: This demo shows you how to hide your card in page layout.
hidden: false
---
[](@ref hidden_card)
There are cases that you want to hide one card in the generated page layout and only provide the
entrance via reflink. For example, you have multiple version of demos and you only want to set the
latest one as the default and provide legacy versions as reflink in the latest page.
This can be done by setting `hidden: true` in the frontmatter, for example:
```markdown
---
hidden: true
id: hidden_card
---
```
By doing this, this page is not shown in the generated index page, the only way to visit it is
through URLs. Usually, you can use Documenter's [reflink
feature](https://juliadocs.github.io/Documenter.jl/dev/man/syntax/#@ref-link) to provide a reflink
to the hidden page.
For example, `[hidden card](@ref hidden_card)` generates a reflink to the [hidden page](@ref
hidden_card), note that it doesn't get displayed in [quickstart index page](@ref quickstart).
!!! note
If you don't pass a index template to `makedemos`, i.e., `makedemos(demodir)`, then it does not
generate an index page for you. In this case, `hidden` keyword does not change anything, for
obvious reasons.
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 795 | 
This demo show you what `DemoCards.jl` does to a markdown demo.
`DemoCards.jl` is a plugin package to [Documenter.jl](https://github.com/JuliaDocs/Documenter.jl).
Hence, in general, demos written in markdown format are directly passed to `Documenter.jl` without much
preprocessing.
`DemoCards.jl` extracts some meta information from your demos:
* The first title is extracted as the card title: the filename `simple_markdown_demo.md` gives the
title "Simple markdown demo"
* The first image link to `Documenter.jl`'s logo is extracted as the cover image
* The first paragraph is extracted as the description
When your mouse hover over the card, a description shows up, for example:

This becomes the simplest markdown demo!
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 4143 | # [Concepts](@id concepts)
This page is a brief introduction on the internal core types provided by `DemoCards.jl`. Generally,
you don't need to use them directly, but knowing them helps you to better organize and configure
your demo pages. For detailed description, please refer to the [Package References](@ref
package_references).
## [DemoPage](@id concepts_page)
[`DemoPage`](@ref DemoCards.DemoPage) is the root type in `DemoCards.jl`, everything
else is contained in it directly or indirectly.
You can configure your `DemoPage` by maintaining a `config.json` file, supported
configuration keys are:
* `"order"`: specify the section orders. By default, it's case-insensitive alphabetic order.
* `"title"`: specify the title of this demo page.
* `"theme"`: specify the index theme to use.
* `"template"`: filename to template that will be used to generate the index page. This option
doesn't work if `"theme"` is unconfigured or is `"nothing"`, in which case no index page will be
generated.
A valid template is a markdown file that contains one and only one `{{{democards}}}`. For example,
```markdown
# Examples
This page contains a set of examples for you to start with.
{{{democards}}}
Contributions are welcomed at [DemoCards.jl](https://github.com/JuliaDocs/DemoCards.jl) :D
```
Here's an example of `config.json` for `DemoPage`:
```json
{
"template": "index.md",
"theme": "grid",
"order": [
"basic",
"advanced"
]
}
```
!!! note
Key `template` has higher priority over other keys. For example, if you provide both
`template` and `title` in your `config.json` and the template file happens to have a title,
`title` in `config.json` will be suppressed.
## [DemoSection](@id concepts_section)
[`DemoSection`](@ref DemoCards.DemoSection) defines the structure of your demo page.
It has the following fields:
* `cards`: holds a list of demo cards.
* `subsections`: holds a list of demo subsections.
!!! warning
A `DemoSection` can't directly holds both cards and subsections; either of them
should be empty vector.
Similar to `DemoPage`, you can configure your `DemoSection` by maintaining a `config.json`
file, supported configuration keys are:
* `"order"`: specify the cards order or subsections order. By default, it's case-insensitive alphabetic order.
* `"title"`: specify the title of this demo section.
* `"description"`: words that would rendered under the section title.
The following is an example of `config.json`:
```json
{
"title": "learn by examples",
"description": "some one-line description can be useful.",
"order": [
"quickstart.md",
"array.md"
]
}
```
!!! note
π§ Unlike `DemoPage`, a `DemoSection` does not yet support `"theme"` and `"template"` keys. A
drawback of this design is that you have to put template contents into the `"description"` key,
even if it contains hundreds of words.
## [Demo card](@id concepts_card)
In simple words, a demo card consists of a link to its content and other relavent informations. In
`"grid"` theme, it looks just like a card.
Depending on how your demos are written, there are two types of demo cards:
* [`MarkdownDemoCard`](@ref configure_your_card) for markdown files, and
* [`JuliaDemoCard`](@ref juliademocard_example) for julia files.
`JuliaDemoCard`s are julia files that are specially handled by DemoCards to generate associated
assets, for example, markdown files (.md) and jupyter notebooks (.ipynb).
## Remarks
Currently, there're two special names used in `DemoCards.jl`:
* `assets` is a preserved name for `DemoCards.jl` to escape preprocessing
* `config.json` is used to tell `DemoCards.jl` more informations of current folder.
To free you from managing/re-organizing demo structure, some decisions are made here:
* maintain a `config.json` in each folder
* always use relative path
* all information is supplementary and hence optional
!!! note
If you've specified orders in `config.json`, then for every new demos, you need to add
its filename to `order`. `DemoCards.jl` isn't smart enough to guess what you really want.
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 592 | # DemoCards.jl
_Let's focus on writing good demos._
A plugin package to [`Documenter.jl`](https://github.com/JuliaDocs/Documenter.jl) that dynamically
generate demos and associated assets for you.
!!! note
Please read the [`Documenter.jl` documentation](https://juliadocs.github.io/Documenter.jl/stable/) first if you're unfamiliar with the julia documentation system.
## Package Features
* a plugin package to `Documenter.jl` to manage all your demos.
* folder structure is the demo structure.
* minimal configuration.
* CI friendly
* support demos in markdown and julia format.
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 4007 | # [Pluto.jl notebooks support](@id pluto)
[Pluto.jl](https://plutojl.org/) is a modern interactive notebook for Julia.
The Pluto notebook content is saved as a Julia script with special syntax decorations to support features like built-in dependency management and reactive cell execution.
In this section, we will discuss how we can add demos written using Pluto as DemoCards.
DemoCards.jl natively supports only Julia and markdown files.
Pluto notebooks are supported via an [extension](https://pkgdocs.julialang.org/dev/creating-packages/#Conditional-loading-of-code-in-packages-%28Extensions%29) to the existing package.
PlutoStaticHTML.jl is used for rendering pluto notebooks to desired markdown format.
The functionality for rendering pluto notebooks is automatically loaded when PlutoStaticHTML is imported into the current environment.
!!! note
Julia versions before julia 1.9 doesn't support the extension functionality.
Requires.jl is used for conditional loading of code in older julia versions.
The set of functionalities supported for Julia and Markdown files is also supported for Pluto.
We can compose any number of Pluto, Julia or Markdown files together for a demo.
If one of the demos contains a pluto notebook, we just need to add PlutoStaticHTML to the list of imports.
```julia
using Documenter, DemoCards # Load functionality for markdown and julia
using PlutoStaticHTML # Import the pluto notebook functionality to DemoCards
# The tutorials folders/sub-folders can contain pluto, julia and markdown
tutorials, tutorials_cb, tutorial_assets = makedemos("tutorials")
```
!!! warning
The pluto notebooks in DemoCards are evaluated sequentially and not in parallel.
This was done primarily to avoid repetitive logs in CI.
We recommend using the cache functionality with the pluto notebooks in case of heavy workloads.
## Adding frontmatter to pluto notebooks
Pluto.jl has its own GUI to manipulate the front matter of a notebook.
This makes it easier for users to create and edit frontmatter.
The pluto frontmatter is not saved in YAML format.
See this [PR](https://github.com/fonsp/Pluto.jl/pull/2104) for more details.
!!! warning
Frontmatter support for Pluto demoCards are currently limited to the frontmatter support for pluto.
It doesn't support the inference of `title`, `description`, `id` or `cover` from the content of the notebook.
Make sure to explicitly mention custom configs in the frontmatter to avoid surprises.
## Cache computationally expensive notebooks
Rendering a Pluto notebook is sometimes time and resource-consuming, especially in a CI environment.
Fortunately, PlutoStaticHTML.jl has a cache functionality that uses outputs of previous runs as a cache.
If the source pluto notebook (.jl file) or the Julia environment didn't change from the last run, the output md file of the last run is used instead of re-rendering the source.
DemoCards stores all the rendered pluto notebooks in a `pluto_output` folder under the `docs` folder.
During the demo creation process, DemoCards.jl checks for a file with a cache (filename with .md extension) in `docs/pluto_output` for each pluto notebook. For example: if the pluto notebook file name is `example_demo.jl`, it searches for cache with filename `example_demo.md`. If the cache exists and the input hash and the Julia version matches, rendering is skipped, and the cache is used as output.
For more insight into the cache mechanism, visit the [cache documentation](https://huijzer.xyz/PlutoStaticHTML.jl/dev/#Caching) on PlutoStaticHTML.
## Examples
The usage of pluto notebooks as DemoCards can be found in [GraphNeuralNetworks.jl](https://github.com/CarloLucibello/GraphNeuralNetworks.jl).
## References
```@docs; canonical=false
DemoCards.PlutoDemoCard
DemoCards.save_democards(card_dir::AbstractString, card::DemoCards.PlutoDemoCard;
project_dir,
src,
credit,
nbviewer_root_url)
```
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 6543 | # [Partial build](@id preview)
## The REPL way
Typically, when you write up examples and demos using `DemoCards`, you'll need to do
`include("docs/make.jl)` to trigger a rebuild for many times. This is okay, just not that fast as
you need to rebuild the whole documentation even if you are just making some trivial typo checks.
This page shows how you could make this experience smoother, especially for julia demos.
`DemoCards` uses [`Literate.jl`](https://github.com/fredrikekre/Literate.jl) as the backend to
transform your julia files to markdown and jupyter notebook. This means that you can code up your
example file with whatever programming environment you like. For example, you can use `Ctrl-Enter`
to execute your codes line by line in Juno/VSCode, check the output, add comments, and so on; all
these can be done without the need to build the documentation.
For example, assume that you are writing a `demos/sec1/demo1.jl`
```julia
# demos/sec1/demo1.jl
using Images # you can `Ctrl-Enter` in VSCode to execute this line
img = testimage("camera") # `Ctrl-Enter` again, and see the output in the plot panel
# and add more example codes in a REPL way
```
## Beyond REPL
This REPL workflow works pretty well and responsive until you start to building the documentation.
For example, There are a lot of demos in [JuliaImages
demos](https://juliaimages.org/latest/democards/examples/) and it takes several minutes to build the
whole documentation. If we're only to make many some small modifications to one of the demo, then it
would be pretty painful to wait the whole build pipeline ends. After all, why should we build demos
we don't care about? Fortunately, `DemoCards` provides a sandbox environment for you to preview your
demos without doing so, which is [`preview_demos`](@ref).
Assume that your `docs/make.jl` is written up like the following:
```julia
# 1. generate demo files
demopage, postprocess_cb, demo_assets = makedemos("demos") # this is the relative path to docs/
# if there are generated css assets, pass it to Documenter.HTML
assets = []
isnothing(demo_assets) || (push!(assets, demo_assets))
# 2. normal Documenter usage
format = Documenter.HTML(assets = assets)
makedocs(format = format,
pages = [
"Home" => "index.md",
demopage,
],
sitename = "Awesome demos")
# 3. postprocess after makedocs
postprocess_cb()
```
To preview only one demo in `demos` folder, say, `demos/sec1/demo1.jl`, what you need to do is:
```julia
preview_demos("docs/demos/sec1/demo1.jl")
```
It will output the path to the generated `index.html` file, for example, it might be something like
this:
```julia
"/var/folders/c0/p23z1x6x3jg_qtqsy_r421y40000gn/T/jl_5sc8fM/build/democards/demos/index.html"
```
Open this file in your favorite browser, and you'll see a reduced version of the demo page, which
only contains one demo file. Other unrelated contents are not included in this page.
This `preview_demos` function is not reserved for single file, it works for folders, too. For
example, you could use it with `preview_demos("demos/sec1")`, or just `preview_demos("demos")`.
`preview_demos` does not do anything magic, it simply copies the single demo file and its assets
into a sandbox folder, and trigger the `Documenter.makedocs` in that folder. Because it doesn't
contain any other files, this process becomes much faster than `include("docs/make.jl")` workflow.
Compared to rebuild the whole documentation, building a preview version only adds about 1 extra
second to `include("demo1.jl")`.
If the file is placed in a typical demo page folder structure, `preview_demos(file)` will preserve
that structure. In cases that demo page folder structure is not detected, it will instead create
such a folder structure. You can check the following JuliaImages example to see how it looks like.
!!! tip
`prewview_demos` accepts an additional `theme` keyword, that you can use this to override the
page theme specified in `config.json`. For example, if `"grid"` theme is specified in
`config.json` file, calling `preview_demos("demos", theme="list")` would just get you to the
list style look without any changes.
!!! note
`preview_demos` does not include any other part of the documentation in the preview, hence
reflinks are very likely to be broken and you will receieve warnings about that. In this case,
you still need to `include("docs/make.jl")` to rebuild the whole documentation if reflinks are
what you concern about.
## Example -- JuliaImages
Let's take the docs of JuliaImages as a live example, and illustrate how `preview_demos` can be used
in practice.
First, let's clone the JuliaImages docs repo:
```bash
git clone https://github.com/JuliaImages/juliaimages.github.io.git
cd juliaimages.github.io.git
```
The result might change, but you can still get the idea.
### preview the whole demo page
The following page is generated by `preview_demos("docs/examples")`, note that all other
documentation contents are not generated in this page. It only contains the contents in
`"docs/examples"`.
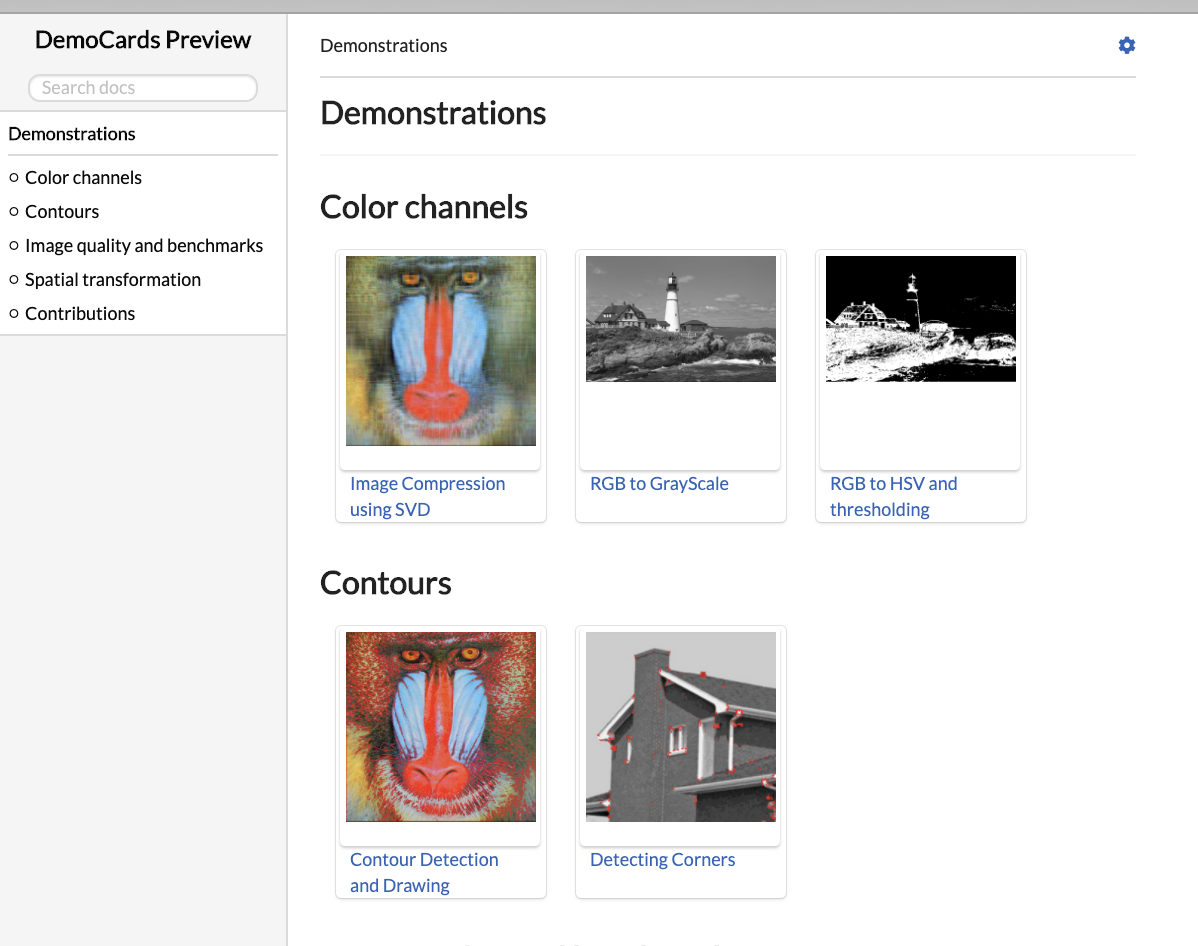
### Preview one section in the demo page
This is the page generated by `preview_demos("docs/examples/color_channels")`, it only generates the
"Color Channels" section:
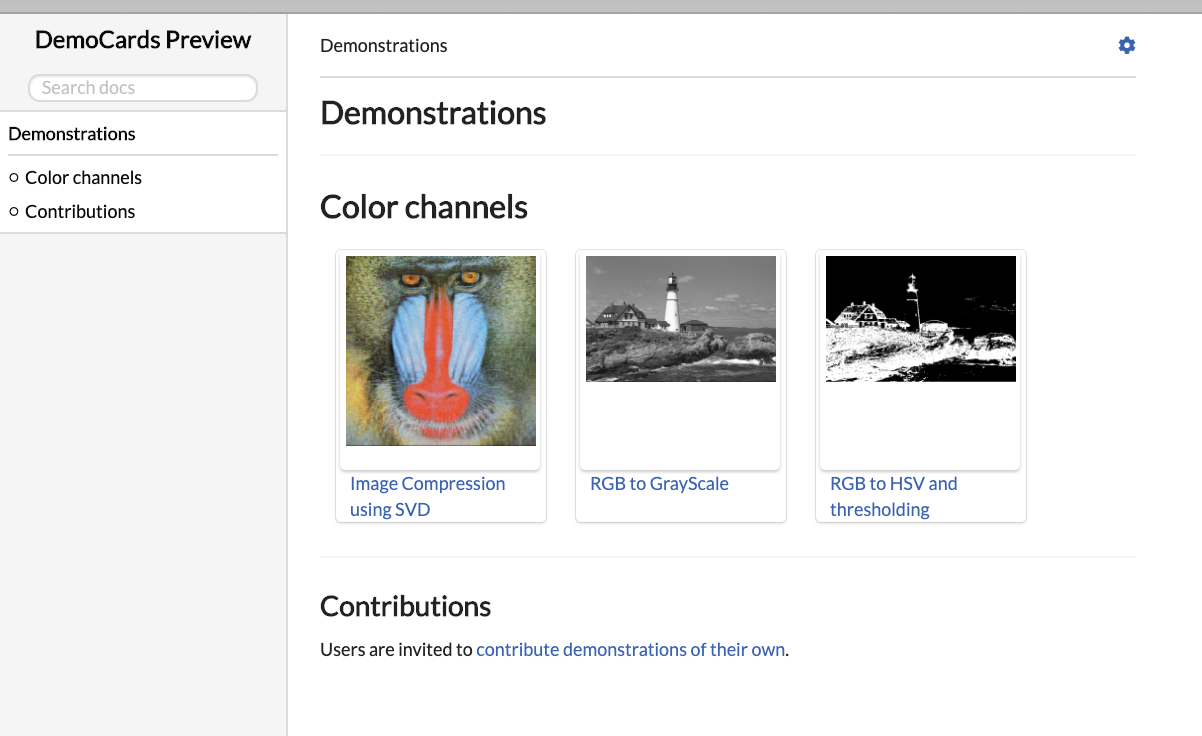
### Preview only one file in the demo page
This is the page generated by `preview_demos("docs/examples/color_separations_svd.jl")` with only one
demo generated.
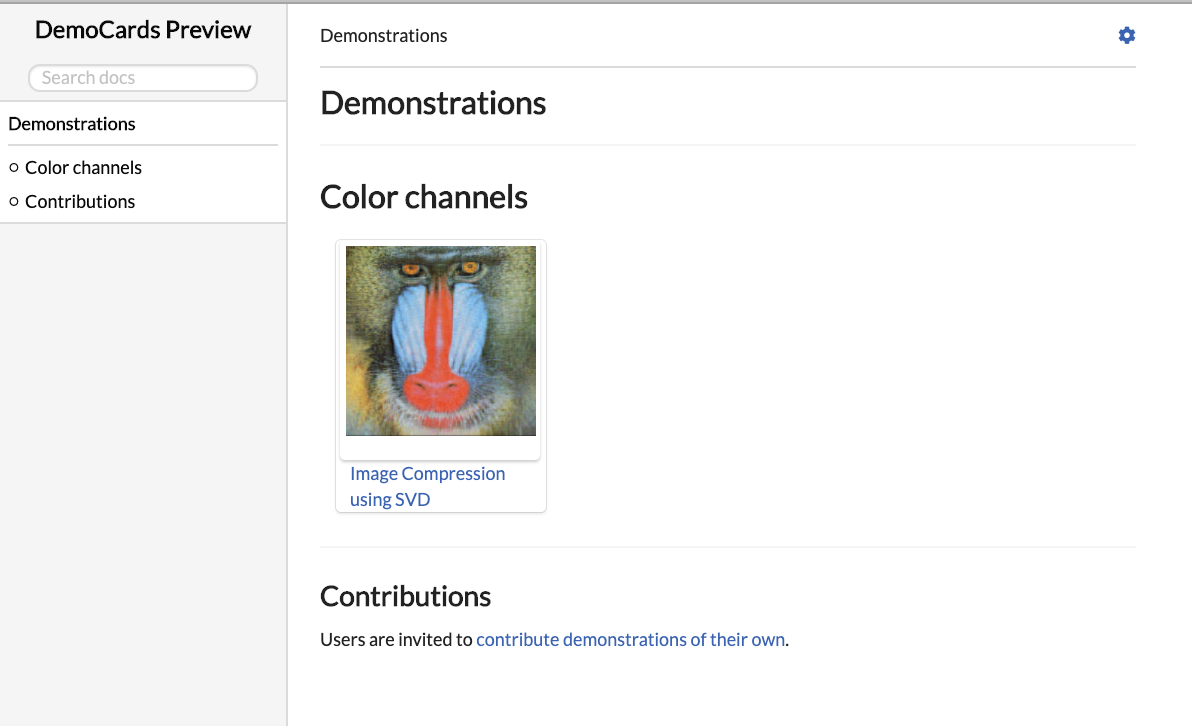
### Preview files without demo page structure
Assume that you have the following folder structure:
```text
testimages/
βββ cameraman.jl
βββ mandril.jl
```
with each file written in this way:
```julia
# This shows how you can load the mandril test image
using Images, TestImages
img = testimage("mandril")
save("assets/mandril.png", img); #hide #md
# 
```
Doing this with `make_demos("testimages")` would just fails because it is not a recognizable folder
structure, but `preview_demos("testimages")` works nicely with this by creating a wrapper folder
preview page:
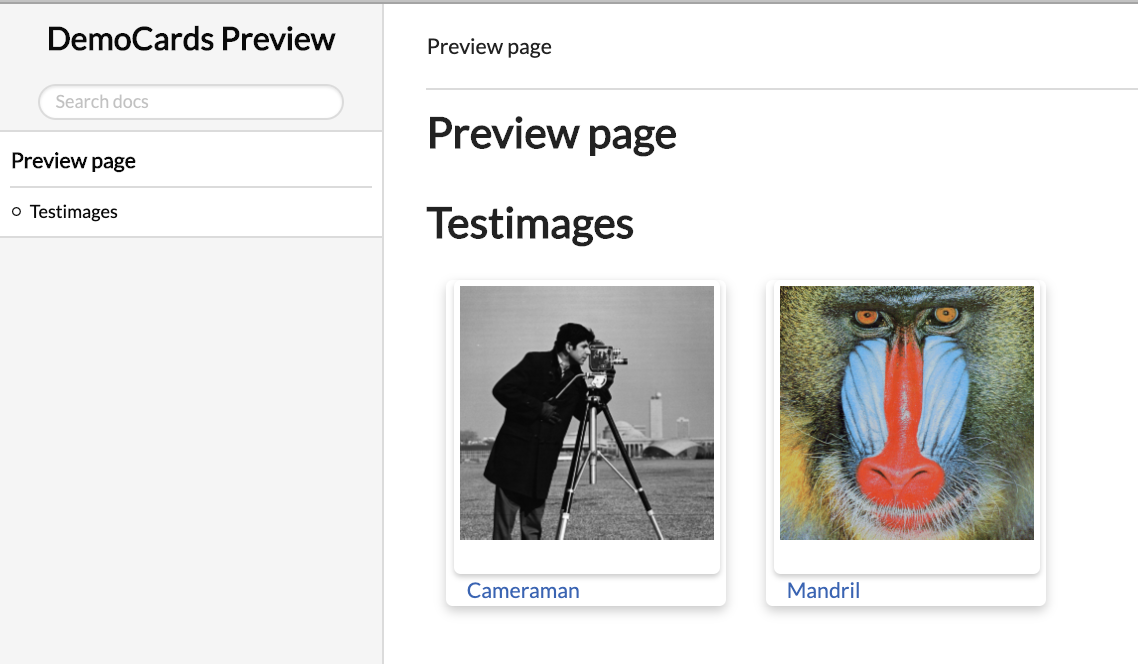
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 139 | # [Package References](@id package_references)
```@autodocs
Modules = [DemoCards, DemoCards.CardThemes]
Order = [:type, :function]
```
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 3139 | # [Structure manipulation](@id manipulation)
It is not uncommon that you'd need to manipulate the folder structure of your demos. For example,
when you are creating new demos, or when you need to reorder the demos and sections. The core design
of `DemoCards.jl` is to make such operation as trivial as possible; the less you modify `make.jl`,
the safer you'll be.
I'll explain it case by case, hopefully you'd get how things work out with `DemoCards.jl`.
Throughout this page, I'll use the following folder structure as an example:
```text
examples
βββ part1
β βββ assets
β βββ config.json
β βββ demo_1.md
β βββ demo_2.md
β βββ demo_3.md
βββ part2
βββ demo_4.jl
βββ demo_5.jl
```
## To add/remove/rename a demo file
Suppose we need to add one `demo_6.jl` in section `"part2"`, just add the file in `examples/part2`
folder. Everything just works without the need to touch `make.jl`.
Adding `demo_6.jl` in section `"part1"` would be a little bit different, because there's a config
file for this section: `examples/part1/config.json`. If `"order"` is not defined in the config file,
it would be the same story. If `"order"` is defined in the config file, then you'll do one more step
to specify the exact order you want `demo_5.jl` be placed at.
Removing/renaming the demo file is similar that, if needed, you'll need to remove/rename the demo
from `"order"` in the config file.
You have to do so because `DemoCards` is not smart enough to guess a partial order for you.
Otherwise `DemoCards` would throw an error on it, for example, this is the error message if I change
`demo_1.md` to `demo1.md`.
```text
β Warning: The following entries in examples/part1/config.json are not used anymore:
β demo_1.md
β @ DemoCards ~/Documents/Julia/DemoCards/src/utils.jl:29
β Warning: The following entries in examples/part1/config.json are missing:
β demo1.md
β @ DemoCards ~/Documents/Julia/DemoCards/src/utils.jl:32
ERROR: LoadError: ArgumentError: incorrect order in examples/part1/config.json, please check the previous warning message.
```
The error message says you have to modify the "orders" item in `examples/part1/config.json` accordingly.
## To rename a section
Suppose we want to rename `"Part1"` to something more meaningful, say `"Markdown demos"`. There are
two ways to do so:
* rename `"examples/part1"` to `"examples/markdown demos"`
* add `"title" = "Markdown demos"` item in `examples/part1/config.json`.
## To move section around
If everything `demo_1.md`, `demo_2.md`, `demo_3.md` needed are placed in `examples/part1/assets`,
then it would be just a piece of cake to move the entire `examples/part1` folder around. Say, move
`examples/part1` to `examples/part1/markdown`.
To minimize the changes you need to do when you organize your demos, here's some general advice:
* Keep an `assets` folder for each of your section.
* Try to avoid specifying path in other folders.
This is just some advice and you don't have to follow it. After all, we don't really change the
folder structure that often.
## To change the page theme
Change the `"theme"` item in `examples/config.json` and that's all.
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 786 | # Theme: BokehList
BokehList is a list theme used in [Bokeh.jl](https://cjdoris.github.io/Bokeh.jl/dev/gallery/).
Given demos organized as following, you can make them displayed as a list of charts
```text
bokehlist
βββ assets
βΒ Β βββ logo.svg
βββ config.json
βββ bokehlist_section_1
βΒ Β βββ bokehlist_subsection_1
βΒ Β βΒ Β βββ bokehlist_card_1.md
βΒ Β βΒ Β βββ bokehlist_card_2.md
βΒ Β βββ bokehlist_subsection_2
βΒ Β βββ bokehlist_card_3.md
βΒ Β βββ bokehlist_card_4.md
βββ index.md
```
```julia
bokehlist_demopage, bokehlist_cb, bokehlist_assets = makedemos("theme_gallery/bokehlist", bokehlist_templates)
```
The page configuration file `bokehlist/config.json` should contain an entry `theme = "bokehlist"`, e.g.,
```json
{
"theme": "bokehlist"
}
```
{{{democards}}}
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
|
[
"MIT"
] | 0.5.5 | bb21a5a9b9f6234f367ba3d47d3b3098b3911788 | docs | 72 | ---
cover: ../../assets/logo.svg
description: some description here
---
| DemoCards | https://github.com/JuliaDocs/DemoCards.jl.git |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.