licenses
listlengths 1
3
| version
stringclasses 677
values | tree_hash
stringlengths 40
40
| path
stringclasses 1
value | type
stringclasses 2
values | size
stringlengths 2
8
| text
stringlengths 25
67.1M
| package_name
stringlengths 2
41
| repo
stringlengths 33
86
|
---|---|---|---|---|---|---|---|---|
[
"MPL-2.0"
]
| 0.3.8 | 03a1e0d2d39b9ebc9510f2452c0adfbe887b9cb2 | code | 6947 | function test_stats()
show_statuses()
nlp = ADNLPModel(x -> dot(x, x), zeros(2))
stats = GenericExecutionStats(nlp)
set_status!(stats, :first_order)
set_objective!(stats, 1.0)
set_residuals!(stats, 0.0, 1e-12)
set_solution!(stats, ones(2))
set_iter!(stats, 10)
set_solver_specific!(stats, :matvec, 10)
set_solver_specific!(stats, :dot, 25)
set_solver_specific!(stats, :empty_vec, [])
broadcast_solver_specific!(stats, :empty_vec, [])
set_solver_specific!(stats, :axpy, 20)
set_solver_specific!(stats, :ray, -1 ./ (1:100))
show(stats)
print(stats)
println(stats)
open("teststats.out", "w") do f
println(f, stats)
end
println(stats, showvec = (io, x) -> print(io, x))
open("teststats.out", "a") do f
println(f, stats, showvec = (io, x) -> print(io, x))
end
line = [:status, :objective, :iter]
for field in line
value = statsgetfield(stats, field)
println("$field -> $value")
end
println(statshead(line))
println(statsline(stats, line))
@testset "Testing inference" begin
for T in (Float16, Float32, Float64, BigFloat)
nlp = ADNLPModel(x -> dot(x, x), ones(T, 2))
stats = GenericExecutionStats(nlp)
set_status!(stats, :first_order)
@test stats.status == :first_order
@test stats.status_reliable
@test typeof(stats.objective) == T
@test typeof(stats.dual_feas) == T
@test typeof(stats.primal_feas) == T
nlp = ADNLPModel(x -> dot(x, x), ones(T, 2), x -> [sum(x) - 1], T[0], T[0])
stats = GenericExecutionStats(nlp)
set_status!(stats, :first_order)
@test stats.status == :first_order
@test stats.status_reliable
@test typeof(stats.objective) == T
@test typeof(stats.dual_feas) == T
@test typeof(stats.primal_feas) == T
S = Vector{T}
stats = GenericExecutionStats{T, S, S, Any}()
set_status!(stats, :first_order)
@test stats.status == :first_order
@test stats.status_reliable
@test typeof(stats.objective) == T
@test typeof(stats.dual_feas) == T
@test typeof(stats.primal_feas) == T
end
end
@testset "Test throws" begin
stats = GenericExecutionStats(nlp)
@test_throws Exception set_status!(stats, :bad)
@test_throws Exception GenericExecutionStats(:unkwown, nlp, bad = true)
stats = GenericExecutionStats{Float64, Vector{Float64}, Vector{Float64}, Any}()
@test_throws Exception set_status!(stats, :bad)
end
@testset "Testing Dummy Solver with multi-precision" begin
for T in (Float16, Float32, Float64, BigFloat)
nlp = ADNLPModel(x -> dot(x, x), ones(T, 2))
solver = SolverCore.DummySolver(nlp)
stats = with_logger(NullLogger()) do
solve!(solver, nlp)
end
@test typeof(stats.objective) == T
@test typeof(stats.dual_feas) == T
@test typeof(stats.primal_feas) == T
@test eltype(stats.solution) == T
@test eltype(stats.multipliers) == T
@test eltype(stats.multipliers_L) == T
@test eltype(stats.multipliers_U) == T
nlp = ADNLPModel(x -> dot(x, x), ones(T, 2), x -> [sum(x) - 1], T[0], T[0])
solver = SolverCore.DummySolver(nlp)
stats = GenericExecutionStats(nlp)
with_logger(NullLogger()) do
solve!(solver, nlp, stats)
end
@test typeof(stats.objective) == T
@test typeof(stats.dual_feas) == T
@test typeof(stats.primal_feas) == T
@test eltype(stats.solution) == T
@test eltype(stats.multipliers) == T
@test eltype(stats.multipliers_L) == T
@test eltype(stats.multipliers_U) == T
stats = GenericExecutionStats{T, Vector{T}, Vector{T}, Any}()
reset!(stats, nlp)
with_logger(NullLogger()) do
solve!(solver, nlp, stats)
end
@test typeof(stats.objective) == T
@test typeof(stats.dual_feas) == T
@test typeof(stats.primal_feas) == T
@test eltype(stats.solution) == T
@test eltype(stats.multipliers) == T
@test eltype(stats.multipliers_L) == T
@test eltype(stats.multipliers_U) == T
end
end
@testset "Test stats setters" begin
T = Float64
nlp = ADNLPModel(x -> dot(x, x), ones(T, 2), x -> [sum(x) - 1], T[0], T[0])
stats = GenericExecutionStats(nlp)
fields = (
"status",
"solution",
"objective",
"primal_residual",
"dual_residual",
"multipliers",
"bounds_multipliers",
"iter",
"time",
"solver_specific",
)
for f ∈ fields
val = getfield(stats, Symbol("$(f)_reliable"))
@test val == false
end
n = 2
x = ones(T, n) / n
set_status!(stats, :first_order)
@test stats.status_reliable
set_solution!(stats, x)
@test stats.solution_reliable
set_objective!(stats, obj(nlp, x))
@test stats.objective_reliable
set_residuals!(stats, 1.0e-3, 1.0e-4)
@test stats.primal_residual_reliable
@test stats.dual_residual_reliable
set_multipliers!(stats, [2 / n], T[], T[])
@test stats.multipliers_reliable
@test stats.bounds_multipliers_reliable
set_iter!(stats, 2)
@test stats.iter_reliable
set_time!(stats, 0.1)
@test stats.time_reliable
set_solver_specific!(stats, :bla, "boo!")
@test stats.solver_specific_reliable
reset!(stats)
for f ∈ fields
val = getfield(stats, Symbol("$(f)_reliable"))
@test val == false
end
end
end
test_stats()
@testset "Test get_status" begin
nlp = ADNLPModel(x -> sum(x), ones(2))
@test get_status(nlp, optimal = true) == :first_order
@test get_status(nlp, small_residual = true) == :small_residual
@test get_status(nlp, infeasible = true) == :infeasible
@test get_status(nlp, unbounded = true) == :unbounded
@test get_status(nlp, stalled = true) == :stalled
@test get_status(nlp, iter = 8, max_iter = 5) == :max_iter
for i = 1:2
increment!(nlp, :neval_obj)
end
@test get_status(nlp, max_eval = 1) == :max_eval
@test get_status(nlp, elapsed_time = 60.0, max_time = 1.0) == :max_time
@test get_status(nlp, parameter_too_large = true) == :stalled
@test get_status(nlp, exception = true) == :exception
@test get_status(nlp) == :unknown
end
@testset "Test get_status for NLS" begin
nlp = ADNLSModel(x -> x, ones(2), 2)
@test get_status(nlp, optimal = true) == :first_order
@test get_status(nlp, small_residual = true) == :small_residual
@test get_status(nlp, infeasible = true) == :infeasible
@test get_status(nlp, unbounded = true) == :unbounded
@test get_status(nlp, stalled = true) == :stalled
@test get_status(nlp, iter = 8, max_iter = 5) == :max_iter
for i = 1:2
increment!(nlp, :neval_residual)
end
@test get_status(nlp, max_eval = 1) == :max_eval
@test get_status(nlp, elapsed_time = 60.0, max_time = 1.0) == :max_time
@test get_status(nlp, parameter_too_large = true) == :stalled
@test get_status(nlp, exception = true) == :exception
@test get_status(nlp) == :unknown
end
| SolverCore | https://github.com/JuliaSmoothOptimizers/SolverCore.jl.git |
|
[
"MPL-2.0"
]
| 0.3.8 | 03a1e0d2d39b9ebc9510f2452c0adfbe887b9cb2 | docs | 1736 | # SolverCore
[](https://doi.org/10.5281/zenodo.4758376)

[](https://cirrus-ci.com/github/JuliaSmoothOptimizers/SolverCore.jl)
[](https://JuliaSmoothOptimizers.github.io/SolverCore.jl/stable)
[](https://JuliaSmoothOptimizers.github.io/SolverCore.jl/dev)
[](https://codecov.io/gh/JuliaSmoothOptimizers/SolverCore.jl)
Core package to build novel optimization algorithms in Julia.
Please cite this repository if you use SolverCore.jl in your work: see [`CITATION.cff`](https://github.com/JuliaSmoothOptimizers/SolverCore.jl/blob/main/CITATION.cff).
# Installation
```
add SolverCore
```
# Bug reports and discussions
If you think you found a bug, feel free to open an [issue](https://github.com/JuliaSmoothOptimizers/SolverCore.jl/issues).
Focused suggestions and requests can also be opened as issues. Before opening a pull request, start an issue or a discussion on the topic, please.
If you want to ask a question not suited for a bug report, feel free to start a discussion [here](https://github.com/JuliaSmoothOptimizers/Organization/discussions). This forum is for general discussion about this repository and the [JuliaSmoothOptimizers](https://github.com/JuliaSmoothOptimizers) organization, so questions about any of our packages are welcome.
| SolverCore | https://github.com/JuliaSmoothOptimizers/SolverCore.jl.git |
|
[
"MPL-2.0"
]
| 0.3.8 | 03a1e0d2d39b9ebc9510f2452c0adfbe887b9cb2 | docs | 105 | # [SolverCore.jl documentation](@id Home)
Core package to build novel optimization algorithms in Julia.
| SolverCore | https://github.com/JuliaSmoothOptimizers/SolverCore.jl.git |
|
[
"MPL-2.0"
]
| 0.3.8 | 03a1e0d2d39b9ebc9510f2452c0adfbe887b9cb2 | docs | 2756 | # JSO-compliant solvers
The following are JSO-compliance expectations and recommendations that are implemented in JuliaSmoothOptimizers.
## Mandatory
- Create a function `solver_name(nlp::AbstractNLPModel; kwargs...)` that returns a `GenericExecutionStats`.
## Recommended
- Create a solver object `SolverName <: SolverCore.AbstractOptimizationSolver`.
- Store all memory-allocating things inside this object. This includes vectors, matrices, and possibly other objects.
- One of these objects should be `solver.x`, that stores the current iterate.
- Implement a constructor `SolverName(nlp; kwargs...)`.
- Implement `SolverCore.solve!(solver, nlp::AbstractNLPModel, stats::GenericExecutionStats)` and change `solver_name` to create a `SolverName` object and call `solve!`.
- Make sure that `solve!` is not allocating.
- Accept the following keyword arguments (`T` is float type and `V` is the container type):
- `x::V = nlp.meta.x0`: The starting point.
- `atol::T = sqrt(eps(T))`: Absolute tolerance for the gradient. Use in conjunction with the relative tolerance below to check $\Vert \nabla f(x_k)\Vert \leq \epsilon_a + \epsilon_r\Vert \nabla f(x_0)\Vert$.
- `rtol::T = sqrt(eps(T))`: Relative tolerance for the gradient. See `atol` above.
- `max_eval::Int = -1`: Maximum number of objective function evaluation plus constraint function evaluations. Negative number means unlimited.
- `max_iter::Int = typemax(Int)`: Maximum number of iterations.
- `max_time::Float64 = 30.0`: Maximum elapsed time.
- `verbose::Int = 0`: Verbosity level. `0` means nothing and `1` means something. There are no rules on the level of verbosity yet.
- `callback = (nlp, solver, stats) -> nothing`: A callback function to be called at the end of an iteration, before exit status are defined.
- Use `set_status!` and `get_status` to update `stats` before starting the method loop, and at the end of every iteration.
- Call the `callback` after running `set_status!` in both places.
- Define `done = stats.status != :unknown` and loop with `while !done`.
- To check for logic errors and stop the method use `set_status!(stats, ...)`, `done = true`, and `continue`, where the second argument of `set_status!` is one of the statuses available in SolverCore.STATUSES. You can call `SolverCore.show_statuses()` to see them. If you need more specific statuses, create an issue.
- Use the `set_...!(stats, ...)` functions from `SolverCore` to update the `stats`. For instance, `set_objective!(stats, f)`, `set_time!(stats, time() - start_time)`, and `set_dual_residual!(stats, gnorm)`.
- Don't log when `verbose == 0`. When logging, use `@info log_header(...)` and `@info log_row(...)`.
- Write docstrings for `SolverName`. The format is still a bit loose.
| SolverCore | https://github.com/JuliaSmoothOptimizers/SolverCore.jl.git |
|
[
"MPL-2.0"
]
| 0.3.8 | 03a1e0d2d39b9ebc9510f2452c0adfbe887b9cb2 | docs | 163 | # Reference
## Contents
```@contents
Pages = ["reference.md"]
```
## Index
```@index
Pages = ["reference.md"]
```
```@autodocs
Modules = [SolverCore]
``` | SolverCore | https://github.com/JuliaSmoothOptimizers/SolverCore.jl.git |
|
[
"MIT"
]
| 0.1.1 | 970a41b7bad791e91518f40c045b47eac7832d0e | code | 6853 | module TestReadme
# ---------------------------------------------------------------------------------------- #
using Test
export @test_readme, InputOutput, parse_readme
# ---------------------------------------------------------------------------------------- #
struct InputOutput
input :: Union{Symbol, Expr}
output :: String
# TODO: store line numbers of readme file?
end
function Base.show(io::IO, ::MIME"text/plain", inout::InputOutput)
Base.summary(io, inout)
println(io, ":")
printstyled(io, " julia> "; color=:green, bold=true)
for (i, l) in enumerate(eachsplit(string(inout.input), '\n'))
i≠1 && print(io, " ")
println(io, l)
end
if isempty(inout.output)
printstyled(io, " [no output]"; color=:light_black)
else
lines_output = count('\n', inout.output) + 1
for (i, l) in enumerate(eachsplit(inout.output, '\n'))
print(io, " ", l)
i ≠ lines_output && println(io)
end
end
end
function Base.show(io::IO, inout::InputOutput)
print(io, ":(")
str_input = string(inout.input)
lines_input = count('\n', str_input) + 1
for (i, l) in enumerate(eachsplit(str_input, '\n'))
print(io, strip(l))
i ≠ lines_input && print(io, "\\n ")
end
print(io, ") ⇒ ")
if isempty(inout.output)
print(io, "\"\"")
else
lines_output = count('\n', inout.output) + 1
for (i, l) in enumerate(eachsplit(inout.output, '\n'))
print(io, strip(l))
i ≠ lines_output && print(io, "\\n ")
end
end
end
# ---------------------------------------------------------------------------------------- #
# Parsing of README files: extracting input/output snippets for eventual testing
function parse_readme(path::AbstractString)
snippets = parse_readme_snippets(path)
inouts = InputOutput[]
for snippet in snippets
inout = extract_input_output(snippet)
append!(inouts, inout)
end
return inouts
end
function parse_readme_snippets(path::AbstractString)
readme = read(path, String)
idx = firstindex(readme)
snippets = Vector{String}()
while true
tmp = read_next_snippet(readme, idx)
isnothing(tmp) && break
s, idx = tmp
push!(snippets, s)
end
return snippets
end
function read_next_snippet(readme, idx)
# in principle, the same could be done with a regex - but it is a complicated regex
# (namely, rx =r"```jl\n((?:.*\n)*?)``", capture anything between ```jl and ```, incl.
# newlines) - but it is actually slower, so easier just to do it ourselves here
idxs′ = findnext(r"```(?:jl|julia)\r?\n", readme, idx)
isnothing(idxs′) && return nothing
idx₁ = nextind(readme, last(idxs′))
idxs′′ = findnext("\n```", readme, idx₁)
isnothing(idxs′′) && return nothing
idx₂ = prevind(readme, last(idxs′′), length("\n```"))
snippet = readme[idx₁:idx₂]
return snippet, nextind(readme, idx₂)
end
function extract_input_output(snippet::AbstractString)
inouts = InputOutput[]
idx′ = firstindex(snippet)
break_next = false
while !break_next
idxs = findnext("julia> ", snippet, idx′)
isnothing(idxs) && break
in, idx′ = Meta.parse(snippet, nextind(snippet, last(idxs)); greedy=true)
Base.remove_linenums!(in) # remove LineNumberNodes
# look for possible output string
if startswith((@view snippet[idx′:end]), "julia> ")
# we interpret this as a "no-output" input line, assigning `""` as output
out = ""
else
idxs′′ = findnext("julia> ", snippet, idx′)
if isnothing(idxs′′)
out = snippet[idx′:end]
break_next = true
else
idx′′ = prevind(snippet, first(idxs′′), 1)
out = snippet[idx′:idx′′]
idx′ = idx′′
end
out = replace(out, r"\s*#.*"=>"") # remove comments (TODO: deal w/ `#= ... =#`?)
out = rstrip(out, ['\r','\n'])
end
push!(inouts, InputOutput(in, out))
end
return inouts
end
# ---------------------------------------------------------------------------------------- #
# Macros
"""
@test_readme [path]
Test the code contents of a README file with file location `path`.
If omitted, `path` is set to `joinpath((@__DIR__), "..", "README.md")`.
Test results are associated with the `@testset` `"README tests"`.
"""
macro test_readme(path)
snippets = gensym()
snippet = gensym()
inouts = gensym()
inout = gensym()
quote
@testset "README tests" begin
$snippets = parse_readme_snippets($(esc(path)))
for $snippet in $snippets
$inouts = extract_input_output($snippet)
for $inout in $inouts
@test_repr_input_output $inout
end
end
end
end
end
macro test_readme()
quote
@test_readme joinpath((@__DIR__), "..", "README.md")
end
end
# this achieves two things: it evaluates the expression in `inout.input` while storing any
# variables created during this evaluates in the current scope (unlike `@test`); it then
# compares the `repr(MIME("text/plain", ...)` representation of this evaluated value against
# `output`. If the representations disagree, the test fails, and a nicely formatted
# expression is shown that actually shows what was evaluated
macro test_repr_input_output(inout)
input = gensym()
output = gensym()
r = gensym()
show_ex = gensym("show_ex")
quote
$input = $(esc(inout)).input
$output = $(esc(inout)).output
$r = eval(quote repr(MIME("text/plain"), $($input)) end)
$show_ex = :( ($($input)) |> Base.Fix1(repr, MIME("text/plain")) == $($output) )
#$show_ex = "(" *
# string(:( ($($input)) |> Base.Fix1(repr, MIME("text/plain")) )) *
# ") == \"$($output)\""
if !isempty($output)
@test_with_custom_expr_show(($r == $output), $show_ex)
else
@test true
end
end
end
# custom variant of `@test` that lets us pass our own expression to show (`show_ex`) if
# `ex` evaluates to `false`; the canabalization from `@test` unfortunately involves some
# code generation that introduces symbols that belong to Test.jl: if we don't manually
# import them ahead of time, they will be wrongly identified as belonging to this module
# (see imports marked by *)
using Test: get_test_result, do_test
using Test: eval_test, Threw # *) imported to avoid module confusion
macro test_with_custom_expr_show(ex, show_ex)
result = get_test_result(ex, __source__)
quote
do_test($result, $show_ex)
end
end
end # module | TestReadme | https://github.com/thchr/TestReadme.jl.git |
|
[
"MIT"
]
| 0.1.1 | 970a41b7bad791e91518f40c045b47eac7832d0e | code | 327 | using TestReadme
# NB: load any other packages that are needed to evaluate the code snippets contained in the
# README file _before_ calling `@test_readme`
@test_readme
# To inspect the parsed input-output pairs, use `parse_readme(path)`:
# path = joinpath((@__DIR__), "..", "README.md")
# inouts = parse_readme(path) | TestReadme | https://github.com/thchr/TestReadme.jl.git |
|
[
"MIT"
]
| 0.1.1 | 970a41b7bad791e91518f40c045b47eac7832d0e | docs | 2632 | # TestReadme.jl
[![Build status][ci-status-img]][ci-status-url] [![Coverage][coverage-img]][coverage-url]
This package provides a macro `@test_readme path` which extracts all Julia code snippets of the following form
~~~md
```jl
julia> input
output
```
~~~
from a file at `path`, comparing `repr(MIME(text/plain), input)` against `output` for each such input-output pair.
The purpose of TestReadme.jl is two-fold:
1. Automatically turn README examples into unit tests.
2. Ensure that README examples stay synced with package functionality.
Additional `@test_readme` details:
- If omitted, `path` defaults to `(@__DIR__)/../README.md` (i.e., default Julia project structure).
- If no `output` is featured in the code snippet, it is simply tested that `input` evaluates without error.
- If evaluation of the README code snippets requires specific packages, load them *before* calling `@test_readme`.
- Results are aggregated in a single `@testset`, named `"README tests"`.
## Example: README snippets tested by `@test_readme`
The following code snippets form part of the test suite of TestReadme.jl itself (see [`/test/runtests.jl`](https://github.com/thchr/TestReadme.jl/blob/main/test/runtests.jl)).
A basic test of math output:
```jl
julia> cos(π)
-1.0
julia> sum([1,2,3])
6
```
It is also possible to chain commands and define variables; a variable defined in one code snippet is in scope throughout a `@test_readme` call:
```jl
julia> x = 2
2
julia> x += 3
julia> x
5
```
Similarly, a single
```jl
julia> begin
y = 2
z = y+x
end
7
julia> exp(z)
1096.6331584284585
```
### Inspecting extracted code-snippets
The extracted input-output pairs can be obtained and inspected via `parse_readme(path)`:
```jl
julia> m = TestReadme # pick your module
julia> path = joinpath(pkgdir(m), "README.md")
julia> input_outputs = parse_readme(path)
```
With the last line here printing (not included as output above in order to avoid recursive madness):
```jl
10-element Vector{InputOutput}:
:(cos(π)) ⇒ -1.0
:(sum([1, 2, 3])) ⇒ 6
:(x = 2) ⇒ 2
:(x += 3) ⇒ ""
:(x) ⇒ 5
:(begin\n y = 2\n z = y + x\n end) ⇒ 7
:(exp(z)) ⇒ 1096.6331584284585
:(m = TestReadme) ⇒ ""
:(path = joinpath(pkgdir(TestReadme), "README.md")) ⇒ ""
:(input_outputs = parse_readme(path)) ⇒ ""
```
[ci-status-img]: https://github.com/thchr/TestReadme.jl/actions/workflows/CI.yml/badge.svg?branch=main
[ci-status-url]: https://github.com/thchr/TestReadme.jl/actions/workflows/CI.yml?query=branch%3Amain
[coverage-img]: https://codecov.io/gh/thchr/TestReadme.jl/branch/main/graph/badge.svg
[coverage-url]: https://codecov.io/gh/thchr/TestReadme.jl | TestReadme | https://github.com/thchr/TestReadme.jl.git |
|
[
"MIT"
]
| 0.2.1 | 2ea799f9bf723a03a31db09d8996fa99f0337396 | code | 10228 | module MzXML
using Base64
using LightXML, Unitful, ProgressMeter
using MzCore
using MzCore.IntervalSets, MzCore.AxisArrays
using CodecZlib
"""
MSscan(polarity::Char, msLevel::Int, retentionTime::typeof(1.0u"s"),
lowMz::Float64, highMz::Float64, basePeakMz::Float64,
totIonCurrent::Float64, mz::Vector, I::Vector, children::Vector{MSscan})
Represent mass spectrometry data at a single time point, scanning over all `m/z`.
`polarity` is `+` or `-` for positive-mode or negative-mode, respectively.
`msLevel` is 1 for MS, 2 for MS², and so on.
Retention time is represented using the Unitful package.
`mz` and `I` should be vectors of the same length, with an ion count of `I[i]` at `mz[i]`.
`children` refers to MS² or higher for particular ions in this scan.
"""
struct MSscan{T<:AbstractFloat,TI<:Real}
polarity::Char
msLevel::Int
retentionTime::typeof(1.0u"s")
lowMz::Float64
highMz::Float64
basePeakMz::Float64
totIonCurrent::Float64
mz::Vector{T}
I::Vector{TI}
children::Vector{MSscan{T,TI}}
end
MzCore.intensitytype(::Type{MSscan{T,TI}}) where {T<:AbstractFloat,TI<:Real} = TI
MzCore.mztype(::Type{MSscan{T,TI}}) where {T<:AbstractFloat,TI<:Real} = T
const empty32 = MSscan{Float32,Float32}[]
const empty64 = MSscan{Float64,Float64}[]
function Base.show(io::IO, scan::MSscan)
if scan.msLevel > 1
print(io, " "^(scan.msLevel-2), "└─basePeak ", scan.basePeakMz, ": ")
end
print(io, scan.retentionTime, ": ")
mzI = [mz=>I for (mz, I) in zip(scan.mz, scan.I)]
println(io, mzI)
end
"""
scanpos = MzXML.index(filename)
Return the vector of file positions corresponding to each scan.
"""
function index(filename)
scanpositions = open(filename) do file
# Find the indexOffset element
seekend(file)
p = position(file)
l = 200
if p < l
error("Too short to be mzXML")
end
skip(file, -l)
str = loadrest(file)
m = match(r"<indexOffset>([0-9].*)</indexOffset>", str)
if m == nothing
error("Cannot find indexOffset element")
end
length(m.captures) == 1 || error("indexOffset should contain a single number")
indexpos = parse(Int, m.captures[1])
length_tail = l - m.offset
# Read the index
seek(file, indexpos)
str = loadrest(file)
xindex = parse_string(str[1:end-length_tail-1])
xroot = root(xindex)
scanpositions = Int[]
for c in child_elements(xroot)
name(c) == "offset" || error("index could not be parsed")
push!(scanpositions, parse(Int, content(c)))
end
scanpositions
end
scanpositions
end
"""
scans, info = MzXML.load(filename; productlevels=0, timeinterval=0.0u"s" .. Inf*u"s", timeshift=0.0u"s")
Load a `*.mzXML` file. `scans` is a vector of scan structures, each at a particular scan time.
`productlevels` controls the number of product-ion levels that will be loaded in combined MS/MS² or similar runs.
The "base" MS level (the first encountered in the file) will always be loaded--`productlevels` controls the
number of additional levels.
By default no product-ion data will be loaded (`productlevels=0`), but you can request it by increasing `productlevels` to 1 or higher.
`scans` will include only those scans occuring within `timeinterval`, with the default to include all times.
The `..` is from the `IntervalSets` package.
"""
function load(filename; productlevels=0, kwargs...)
xdoc = parse_file(filename)
xroot = root(xdoc)
if name(xroot) != "mzXML"
error("Not an mzXML file")
end
# Find the msRun node
msRun = find_element(xroot, "msRun")
props = Dict{Symbol,Any}()
props[:startTime] = parse_time(attribute(msRun, "startTime"))
props[:endTime] = parse_time(attribute(msRun, "endTime"))
el = find_element(msRun, "msInstrument")
el = find_element(el, "msModel")
props[:msModel] = attribute(el, "value")
props[:productlevels] = productlevels
scans = load_scans(msRun, productlevels; kwargs...)
LightXML.free(xdoc)
return scans, props
end
function load_scans(elm, productlevels=0; kwargs...)
# First we discover the data type
local T
local TI
for c in child_elements(elm)
n = name(c)
if n != "scan"
continue
end
peak = find_element(c, "peaks")
TI, T, nochildren = precisiondict[attribute(peak, "precision")]
break
end
# Now load the data
scans = MSscan{T,TI}[]
load_scans!(scans, elm, productlevels; kwargs...)
end
@noinline function load_scans!(scans, elm, productlevels; kwargs...)
prog = ProgressUnknown("Number of scans read:")
for c in child_elements(elm)
n = name(c)
if n != "scan"
continue
end
scan = load_scan(c, productlevels; kwargs...)::Union{Nothing,eltype(scans)}
if scan !== nothing
push!(scans, scan)
next!(prog)
end
end
finish!(prog)
scans
end
polaritydict = Dict("+" => '+', "-" => '-')
precisiondict = Dict("32" => (Float32, Float32, empty32), "64" => (Float64, Float64, empty64))
function load_scan(elm, productlevels; timeinterval=0.0u"s" .. Inf*u"s", timeshift=0.0u"s")
polarity = polaritydict[attribute(elm, "polarity")]
retentionTime = parse_time(attribute(elm, "retentionTime")) + timeshift
retentionTime ∈ timeinterval || return nothing
lMza, hMza = attribute(elm, "lowMz"), attribute(elm, "highMz")
if lMza !== nothing && hMza !== nothing
lowMz, highMz = parse(Float64, lMza), parse(Float64, hMza)
else
lowMz = highMz = NaN
end
msLevela = attribute(elm, "msLevel")
msLevel = msLevela === nothing ? 1 : parse(Int, msLevela)
basePeakMz = parse(Float64, attribute(elm, "basePeakMz"))
totIonCurrent = parse(Float64, attribute(elm, "totIonCurrent"))
npeaks = parse(Int, attribute(elm, "peaksCount"))
peak = find_element(elm, "peaks")
data = base64decode(content(peak))
compression_type = attribute(peak, "compressionType")
if compression_type == "zlib"
data = transcode(ZlibDecompressor, data)
elseif compression_type != "none"
error("compressionType $compression_type not supported")
end
TI, T, nochildren = precisiondict[attribute(peak, "precision")]
A = reinterpret(TI, data)
bo = attribute(peak, "byteOrder")
if bo == "network"
ntoh!(A)
else
error("Don't know what to do with byteOrder $bo")
end
po = attribute(peak, "pairOrder")
if po === nothing
po = attribute(peak, "contentType")
end
po == "m/z-int" || error("Don't know what to do with pairOrder/contentType $po")
I = A[2:2:end]
mz = reinterpret(T, A[1:2:end])
if !issorted(mz) # it's unclear whether the standard requires these to be sorted, so better to check
perm = sortperm(mz)
I, mz = I[perm], mz[perm]
end
children = productlevels > 0 ? load_scans!(MSscan{T,TI}[], elm, productlevels-1) : nochildren
MSscan{T,TI}(polarity, msLevel, retentionTime, lowMz, highMz, basePeakMz, totIonCurrent, mz, I, children)
end
function loadrest(file::IOStream)
nb = filesize(file) - position(file)
b = read(file, nb)
return String(b)
end
function parse_time(tstr)
if startswith(tstr, "PT") && endswith(tstr, "S")
return parse(Float64, tstr[3:end-1])*u"s"
end
error("Time string $tstr not recognized")
end
function ntoh!(A)
for i = 1:length(A)
A[i] = ntoh(A[i])
end
end
Base.pairs(scan::MSscan) = zip(scan.mz, scan.I)
function val2index(ax::Axis, val)
f, s = first(ax.val), step(ax.val)
r = (val - f) / s
idx = floor(Int, r)
return idx + 1, r - idx
end
"""
copyto!(dest::AxisArray, src::Vector{<:MSscan})
Convert the "sparse-matrix" representation `src` of mass spectrometry
data into a dense 2d matrix `dest`. `dest` must have one axis named
`:mz` and the other `:time`; the `:time` axis must have physical units of
time.
Any previous contents of `dest` are erased. The values of `src` are
accumulated into `dest` using bilinear interpolation.
"""
function Base.copyto!(dest::AxisArray, src::Vector{<:MSscan})
axmz, axt = AxisArrays.axes(dest, Axis{:mz}), AxisArrays.axes(dest, Axis{:time})
fill!(dest, 0)
for scan in src
j, ft = val2index(axt, scan.retentionTime)
firstindex(axt.val) - 1 <= j <= lastindex(axt.val) || continue
for (mz, I) in pairs(scan)
i, fmz = val2index(axmz, mz)
# Accumulate into `dest`, using bilinear interpolation to
# avoid aliasing
if firstindex(axt.val) <= j <= lastindex(axt.val)
if firstindex(axmz.val) <= i <= lastindex(axmz.val)
dest[axmz(i),axt(j)] += (1-fmz)*(1-ft)*I
end
if firstindex(axmz.val) <= i+1 <= lastindex(axmz.val)
dest[axmz(i+1),axt(j)] += fmz*(1-ft)*I
end
end
if firstindex(axt.val) <= j+1 <= lastindex(axt.val)
if firstindex(axmz.val) <= i <= lastindex(axmz.val)
dest[axmz(i),axt(j+1)] += (1-fmz)*ft*I
end
if firstindex(axmz.val) <= i+1 <= lastindex(axmz.val)
dest[axmz(i+1),axt(j+1)] += fmz*ft*I
end
end
end
end
return dest
end
# An internal type for nested `extrema` calls (yielding the min of all mins, max of all maxs)
struct ExPair{T}
min::T
max::T
end
Base.min(a::ExPair, b::ExPair) = a.min <= b.min ? a : b
Base.max(a::ExPair, b::ExPair) = a.max >= b.max ? a : b
function MzCore.limits(scans::Vector{<:MSscan})
function extrema_mz(scan)
isfinite(scan.lowMz) && isfinite(scan.highMz) && return ExPair(scan.lowMz, scan.highMz)
return ExPair(first(scan.mz), last(scan.mz)) # safe because guaranteed to be sorted
end
tmin, tmax = extrema(scan->scan.retentionTime, scans)
mzmin, mzmax = extrema(extrema_mz, scans)
return Axis{:mz}(mzmin.min .. mzmax.max), Axis{:time}(tmin..tmax)
end
end
| MzXML | https://github.com/timholy/MzXML.jl.git |
|
[
"MIT"
]
| 0.2.1 | 2ea799f9bf723a03a31db09d8996fa99f0337396 | code | 1495 | using MzXML, Unitful
using MzXML.IntervalSets
using MzCore
using Test
@testset "MzXML" begin
scans, info = MzXML.load("test32.mzXML")
@test info[:msModel] == "API 3000"
@test eltype(scans) == MzXML.MSscan{Float32,Float32}
@test length(scans) == 2
scan = scans[1]
@test scan.polarity == '-'
@test scan.msLevel == 2
@test scan.basePeakMz == 321.4
@test scan.totIonCurrent == 1.637827475594e06
@test scan.retentionTime == 0.004*u"s"
@test scans[2].retentionTime == 0.809*u"s"
# MzCore traits
@test intensitytype(scans[1]) === Float32
@test mztype(scans[1]) === Float32
axmz, axt = limits(scans)
@test minimum(axmz.val) === 110.9f0
@test maximum(axmz.val) === 587.6f0
@test minimum(axt.val) === 0.004*u"s"
@test maximum(axt.val) === 0.809*u"s"
scans, info = MzXML.load("test32.mzXML"; timeinterval=0.2u"s" .. Inf*u"s")
@test length(scans) == 1 && scans[1].retentionTime == 0.809*u"s"
io = IOBuffer()
show(io, scan)
@test occursin("└─basePeak 321.4", String(take!(io)))
scans, info = MzXML.load("test32.mzXML"; timeinterval=10.2u"s" .. Inf*u"s", timeshift=10u"s")
@test length(scans) == 1 && scans[1].retentionTime == 10.809*u"s"
idx = MzXML.index("test32.mzXML")
@test idx == [1231, 4242]
scans, info = MzXML.load("test64.mzXML")
@test eltype(scans) == MzXML.MSscan{Float64,Float64}
@test intensitytype(scans[1]) === Float64
@test mztype(scans[1]) === Float64
end
| MzXML | https://github.com/timholy/MzXML.jl.git |
|
[
"MIT"
]
| 0.2.1 | 2ea799f9bf723a03a31db09d8996fa99f0337396 | docs | 1523 | # MzXML
[](http://codecov.io/github/timholy/MzXML.jl?branch=master)
A Julia package for reading mass spectrometry [mzXML files](https://en.wikipedia.org/wiki/Mass_spectrometry_data_format).
Example:
```julia
julia> using MzXML
julia> cd(joinpath(dirname(dirname(pathof(MzXML))), "test")) # location of a test file
julia> scans, info = MzXML.load("test32.mzXML");
julia> info
Dict{Symbol,Any} with 3 entries:
:endTime => 24.156 s
:msModel => "API 3000"
:startTime => 0.004 s
julia> scans
2-element Array{MzXML.MSscan{Float32,Float32},1}:
└─basePeak 321.4: 0.004 s: Pair{Float32,Float32}[111.3 => 6251.25, 111.4 => 0.0, 166.7 => 0.0, 166.8 => 18753.75, 166.9 => 0.0, 189.1 => 0.0, 189.2 => 12502.5, 189.3 => 0.0, 191.7 => 0.0, 191.8 => 37507.5 … 421.1 => 12502.5, 421.2 => 0.0, 437.9 => 0.0, 438.0 => 6251.25, 438.1 => 0.0, 438.5 => 0.0, 438.6 => 6251.25, 438.7 => 0.0, 481.0 => 0.0, 481.1 => 18753.75]
└─basePeak 321.0: 0.809 s: Pair{Float32,Float32}[110.9 => 12502.5, 111.0 => 0.0, 186.9 => 0.0, 187.0 => 6251.25, 187.1 => 0.0, 199.5 => 0.0, 199.6 => 12502.5, 199.7 => 18753.75, 199.8 => 50010.0, 199.9 => 6251.25 … 507.4 => 18753.75, 507.5 => 0.0, 526.4 => 0.0, 526.5 => 6251.25, 526.6 => 0.0, 545.6 => 0.0, 545.7 => 6251.25, 545.8 => 0.0, 587.5 => 0.0, 587.6 => 18753.75]
```
The "└─" indicates MS² or higher on the specified base peak. Also shown is the retention time (in seconds), and `mz=>ion count` pairs.
| MzXML | https://github.com/timholy/MzXML.jl.git |
|
[
"MIT"
]
| 0.1.0 | 9969c59e5023c476f491c6962cfeb785c47d1614 | code | 4894 | module ScalarKernelFunctions
using Reexport
@reexport using KernelFunctions
using SpecialFunctions: loggamma, besselk
using IrrationalConstants: logtwo
import KernelFunctions: Kernel
import KernelFunctions: kernelmatrix, kernelmatrix!, kernelmatrix_diag, kernelmatrix_diag!
import KernelFunctions: Transform, IdentityTransform, with_lengthscale
export ScalarKernel, ScalarSEKernel, ScalarLinearKernel, ScalarPeriodicKernel
export ScalarExponentialKernel
export ScalarMatern12Kernel, ScalarMatern32Kernel, ScalarMatern52Kernel, ScalarMaternKernel
export ScalarKernelSum, ScalarScaledKernel, with_lengthscale
export TransformedScalarKernel, ScalarScaleTransform
export gpu
gpu(k::Kernel) = k
gpu(t::Transform) = t
gpu(x::Real) = Float32(x)
abstract type ScalarKernel <: Kernel end
function kernelmatrix(
k::ScalarKernel,
x::AbstractVector{<:Real},
y::AbstractVector{<:Real}
)
return k.(x, y')
end
function kernelmatrix!(
K::AbstractMatrix{<:Real},
k::ScalarKernel,
x::AbstractVector{<:Real},
y::AbstractVector{<:Real}
)
K .= k.(x, y')
return K
end
function kernelmatrix_diag(
k::ScalarKernel,
x::AbstractVector{<:Real},
y::AbstractVector{<:Real}
)
l = min(length(x), length(y))
_x = view(x, firstindex(x):firstindex(x)+l-1)
_y = view(y, firstindex(y):firstindex(y)+l-1)
return k.(_x, _y)
end
function kernelmatrix_diag!(
K::AbstractVector{<:Real},
k::ScalarKernel,
x::AbstractVector{<:Real},
y::AbstractVector{<:Real}
)
l = min(length(x), length(y))
_x = view(x, firstindex(x):firstindex(x)+l-1)
_y = view(y, firstindex(y):firstindex(y)+l-1)
K .= k.(_x, _y)
return K
end
struct ScalarSEKernel <: ScalarKernel end
(k::ScalarSEKernel)(x, y) = exp(-abs2(x - y) / 2)
struct ScalarLinearKernel <: ScalarKernel end
(k::ScalarLinearKernel)(x, y) = x * y
struct ScalarPeriodicKernel{T<:Real} <: ScalarKernel
r::T
end
ScalarPeriodicKernel() = ScalarPeriodicKernel(1.)
(k::ScalarPeriodicKernel)(x, y) = exp(-abs2(sinpi(x - y) / k.r) / 2)
gpu(k::ScalarPeriodicKernel) = ScalarPeriodicKernel(gpu(k.r))
struct ScalarExponentialKernel <: ScalarKernel end
(k::ScalarExponentialKernel)(x, y) = exp(-abs(x - y))
const ScalarMatern12Kernel = ScalarExponentialKernel
struct ScalarMatern32Kernel <: ScalarKernel end
function (k::ScalarMatern32Kernel)(x::T, y::T) where T<:Real
sqrt3 = sqrt(T(3))
d = abs(x - y)
return (1 + sqrt3 * d) * exp(-sqrt3 * d)
end
struct ScalarMatern52Kernel <: ScalarKernel end
function (k::ScalarMatern52Kernel)(x::T, y::T) where T<:Real
sqrt5 = sqrt(T(5))
d = abs(x - y)
return (1 + sqrt5 * d + 5 * d^2 / 3) * exp(-sqrt5 * d)
end
struct ScalarMaternKernel{T<:Real} <: ScalarKernel
ν::T
end
ScalarMaternKernel() = ScalarMaternKernel(1.5)
function (k::ScalarMaternKernel)(x::T, y::T) where T<:Real
d = abs(x - y)
ν = k.ν
if iszero(d)
c = -ν / (ν - 1)
return one(d) + c * d^2 / 2
else
y = sqrt(2ν) * d
b = log(besselk(ν, y))
return exp((one(d) - ν) * oftype(y, logtwo) - loggamma(ν) + ν * log(y) + b)
end
end
gpu(k::ScalarMaternKernel) = ScalarMaternKernel(gpu(k.ν))
struct ScalarKernelSum{T1<:Kernel, T2<:Kernel} <: ScalarKernel
k1::T1
k2::T2
end
ScalarKernelSum(kernels::Tuple) = ScalarKernelSum(kernels...)
function ScalarKernelSum(k1::Kernel, k2::Kernel, k3::Kernel, ks...)
return ScalarKernelSum(k1, ScalarKernelSum(k2, k3, ks...))
end
(k::ScalarKernelSum)(x, y) = k.k1(x, y) + k.k2(x, y)
gpu(k::ScalarKernelSum) = ScalarKernelSum(gpu(k.k1), gpu(k.k2))
Base.:+(k1::ScalarKernel, k2::ScalarKernel) = ScalarKernelSum(k1, k2)
struct ScalarScaledKernel{Tk<:Kernel,Tσ²<:Real} <: ScalarKernel
kernel::Tk
σ²::Tσ²
end
(k::ScalarScaledKernel)(x, y) = k.σ² * k.kernel(x, y)
gpu(k::ScalarScaledKernel) = ScalarScaledKernel(gpu(k.kernel), gpu(k.σ²))
Base.:*(w::Real, k::ScalarKernel) = ScalarScaledKernel(k, w)
struct TransformedScalarKernel{Tk<:Kernel,Tr<:Transform} <: ScalarKernel
kernel::Tk
transform::Tr
end
(k::TransformedScalarKernel)(x, y) = k.kernel(k.transform(x), k.transform(y))
function gpu(k::TransformedScalarKernel)
return TransformedScalarKernel(gpu(k.kernel), gpu(k.transform))
end
Base.:∘(k::ScalarKernel, t::Transform) = TransformedScalarKernel(k, t)
function Base.:∘(k::TransformedScalarKernel, t::Transform)
return TransformedScalarKernel(k.kernel, k.transform ∘ t)
end
Base.:∘(k::TransformedScalarKernel, ::IdentityTransform) = k
struct ScalarScaleTransform{T<:Real} <: Transform
s::T
end
(t::ScalarScaleTransform)(x) = t.s * x
Base.:∘(t::ScalarScaleTransform, u::ScalarScaleTransform) = ScalarScaleTransform(t.s * u.s)
gpu(t::ScalarScaleTransform) = ScalarScaleTransform(gpu(t.s))
with_lengthscale(k::ScalarKernel, l::Real) = k ∘ ScalarScaleTransform(inv(l))
end # module ScalarKernelFunctions
| ScalarKernelFunctions | https://github.com/JuliaGaussianProcesses/ScalarKernelFunctions.jl.git |
|
[
"MIT"
]
| 0.1.0 | 9969c59e5023c476f491c6962cfeb785c47d1614 | code | 4045 | using ScalarKernelFunctions
using JLArrays: jl
using LinearAlgebra: diag
using Test
@testset "ScalarKernelFunctions" begin
@testset "Consistency with KernelFunctions.jl" begin
x0 = rand(100)
x1 = rand(100)
x2 = rand(50)
K1 = zeros(100, 100)
K2 = zeros(100, 100)
K3 = zeros(100, 50)
K4 = zeros(100, 50)
v1 = zeros(100)
v2 = zeros(100)
@testset "$kernel2" for (kernel1, kernel2) in (
# Simple kernels
SEKernel() => ScalarSEKernel(),
LinearKernel() => ScalarLinearKernel(),
PeriodicKernel() => ScalarPeriodicKernel(),
PeriodicKernel(; r = [2.0]) => ScalarPeriodicKernel(2.0),
Matern12Kernel() => ScalarMatern12Kernel(),
Matern32Kernel() => ScalarMatern32Kernel(),
Matern52Kernel() => ScalarMatern52Kernel(),
MaternKernel(; ν = 1.2) => ScalarMaternKernel(1.2),
MaternKernel(; ν = 3.0) => ScalarMaternKernel(3.0),
# Composite kernels
2.0 * SEKernel() + 3.0 * LinearKernel() =>
2.0 * ScalarSEKernel() + 3.0 * ScalarLinearKernel(),
SEKernel() * PeriodicKernel() => ScalarSEKernel() * ScalarPeriodicKernel()
)
@testset for (k1, k2) in (
(kernel1, kernel2),
with_lengthscale.((kernel1, kernel2), 2.0),
2.0 .* (kernel1, kernel2)
)
@test k1(1., 4.) ≈ k2(1., 4.)
@test kernelmatrix(k1, x0) ≈ kernelmatrix(k2, x0)
@test kernelmatrix(k1, x0, x1) ≈ kernelmatrix(k2, x0, x1)
@test kernelmatrix(k1, x0, x2) ≈ kernelmatrix(k2, x0, x2)
@test kernelmatrix(k1, x2, x0) ≈ kernelmatrix(k2, x2, x0)
@test kernelmatrix_diag(k1, x0) ≈ kernelmatrix_diag(k2, x0)
@test kernelmatrix_diag(k1, x0, x1) ≈ kernelmatrix_diag(k2, x0, x1)
kernelmatrix!(K1, k1, x0)
kernelmatrix!(K2, k2, x0)
@test K1 ≈ K2
kernelmatrix!(K1, k1, x0, x1)
kernelmatrix!(K2, k2, x0, x1)
@test K1 ≈ K2
kernelmatrix!(K3, k1, x0, x2)
kernelmatrix!(K4, k2, x0, x2)
@test K3 ≈ K4
kernelmatrix_diag!(v1, k1, x0, x1)
kernelmatrix_diag!(v2, k2, x0, x1)
@test v1 ≈ v2
end
end
end
@testset "GPU compatibility" begin
x0 = rand(Float32, 10) |> jl
x1 = rand(Float32, 10) |> jl
@testset "$k" for k in (
# Simple kernels
ScalarSEKernel(), ScalarLinearKernel(), ScalarPeriodicKernel(),
ScalarMatern12Kernel(), ScalarMatern32Kernel(), ScalarMatern52Kernel(),
ScalarMaternKernel(1.2),
# Composite kernels
with_lengthscale(ScalarSEKernel(), 2.0), 2.0 * ScalarLinearKernel(),
ScalarSEKernel() + ScalarPeriodicKernel(),
# ScalarSEKernel() * ScalarPeriodicKernel()
)
kgpu = gpu(k)
@test (@inferred kernelmatrix(kgpu, x0)) isa AbstractMatrix{Float32}
@test (@inferred kernelmatrix(kgpu, x0, x1)) isa AbstractMatrix{Float32}
@test (@inferred kernelmatrix_diag(kgpu, x0, x1)) isa AbstractVector{Float32}
@test (@inferred kernelmatrix_diag(kgpu, x0, x1)) isa AbstractVector{Float32}
end
end
@testset "`kernelmatrix_diag` with different input lengths" begin
x0 = rand(10)
x1 = rand(20)
v0 = zeros(10)
v1 = zeros(10)
@testset "$k" for k in (
ScalarSEKernel(), ScalarLinearKernel(), ScalarPeriodicKernel()
)
kernelmatrix_diag!(v0, k, x1, x0)
kernelmatrix_diag!(v1, k, x0, x1)
@test v0 ≈ kernelmatrix_diag(k, x0, x1) ≈ diag(kernelmatrix(k, x0, x1))
@test v1 ≈ kernelmatrix_diag(k, x1, x0) ≈ diag(kernelmatrix(k, x1, x0))
end
end
end
| ScalarKernelFunctions | https://github.com/JuliaGaussianProcesses/ScalarKernelFunctions.jl.git |
|
[
"MIT"
]
| 0.1.0 | 9969c59e5023c476f491c6962cfeb785c47d1614 | docs | 3796 | # ScalarKernelFunctions.jl
[](https://www.repostatus.org/#wip)
[](https://github.com/JuliaGaussianProcesses/ScalarKernelFunctions.jl/actions/workflows/CI.yml)
[](https://codecov.io/gh/JuliaGaussianProcesses/ScalarKernelFunctions.jl/tree/master)
This package implements kernel functions that are optimized for one-dimensional input and
that are GPU-compatible by default.
## Usage
This package expands the KernelFunctions.jl package (which is automatically loaded and
reexported) by a new abstract type `ScalarKernel`.
Its subtypes include a couple of base kernels with the `Scalar` prefix.
On 1-dimensional inputs, they give exactly the same output as their KernelFunctions.jl
counterparts, e.g.:
```julia
using ScalarKernelFunctions
k1 = ScalarSEKernel() # from this package
k2 = SEKernel() # from KernelFunctions.jl
x = rand(100)
kernelmatrix(k1, x) ≈ kernelmatrix(k2, x) # true
```
When combining subtypes of `ScalarKernel` using `+`, `with_lengthscale`, another subtype of
`ScalarKernel` will be produced, which means that specialized implementations will be used
for the composite kernel as well.
Mixing specialized and "normal" kernels will also work, but will no longer use the
specialized implementation.
Specializing on 1d input allows to achieve lower allocation counts and faster evaluation,
especially combined with AD packages such as Zygote or Enzyme. Parameter fields are also
scalar, which saves allocations with repeated construction of the kernel, e.g. when kernel
parameters are being optimized.
### GPU-compatibility by default
The kernels in this package are implemented using broadcast, which allows them to work on
the GPU by default. We also export a `gpu` function, which converts any kernel to use
`Float32` parameters (where needed), and calling `kernelmatrix` will preserve `Float32` to
be most efficient on GPUs. For example, this is how we use a kernel on `CuArray`s:
```julia
using CUDA
x = CUDA.rand(100)
k = ScalarPeriodicKernel() |> gpu # ScalarPeriodicKernel{Float32}(1.0f0)
kernelmatrix(k, x) # returns 100×100 CuArray{Float32, 2, CUDA.Mem.DeviceBuffer}
```
Omitting the `gpu` conversion will of course also work, but will be quite a bit slower.
## Supported kernels
### Base kernels
- [x] `ScalarSEKernel`
- [x] `ScalarLinearKernel`
- [x] `ScalarPeriodicKernel`
- [x] `ScalarMatern12Kernel` === `ScalarExponentialKernel`
- [x] `ScalarMatern32Kernel`
- [x] `ScalarMatern52Kernel`
- [x] `ScalarMaternKernel`
### Composite kernels
- [x] `ScalarKernelSum`, when doing `k1 + k2`, where `k1` and `k2` are `ScalarKernel`s
- [ ] `ScalarKernelProduct`
- [x] `ScalarTransformedKernel`, when doing `k ∘ t`, where `k` is a `ScalarKernel` and `t` is a `Transform`
- [x] `ScalarScaledKernel`, when doing `a * k`, where `k` is a `ScalarKernel` and `a` is a `Real`
### Transforms
- [x] `ScalarScaleTransform`
<!-- - [ ] `ScalarConstantKernel`
- [ ] `WhiteKernel`
- [ ] `EyeKernel`
- [ ] `ZeroKernel`
- [ ] `WienerKernel`
- [ ] `CosineKernel`
- [ ] `GaussianKernel`
- [ ] `LaplacianKernel`
- [ ] `ExponentialKernel`
- [ ] `GammaExponentialKernel`
- [ ] `ExponentiatedKernel`
- [ ] `FBMKernel`
- [ ] `MaternKernel`
- [ ] `PolynomialKernel`
- [ ] `RationalKernel`
- [ ] `RationalQuadraticKernel`
- [ ] `GammaRationalKernel`
- [ ] `PiecewisePolynomialKernel`
- [ ] `NeuralNetworkKernel`
- [ ] `KernelTensorProduct`
- [ ] `NormalizedKernel`
- [ ] `GibbsKernel` -->
| ScalarKernelFunctions | https://github.com/JuliaGaussianProcesses/ScalarKernelFunctions.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 1088 | # This script gets run once, on a developer's machine, to generate artifacts
using Pkg.Artifacts
using Downloads
function generate_artifacts(ver="2.3.0", repo="https://github.com/sglyon/PlotlyBase.jl")
artifacts_toml = joinpath(dirname(@__DIR__), "Artifacts.toml")
# if Artifacts.toml does not exist we also do not have to remove it
isfile(artifacts_toml) && rm(artifacts_toml)
plotschema_url = "https://raw.githubusercontent.com/plotly/plotly.js/v$(ver)/dist/plot-schema.json"
plotlyartifacts_hash = create_artifact() do artifact_dir
@show artifact_dir
Downloads.download(plotschema_url, joinpath(artifact_dir, "plot-schema.json"))
cp(joinpath(dirname(@__DIR__), "templates"), joinpath(artifact_dir, "templates"))
end
tarball_hash = archive_artifact(plotlyartifacts_hash, "plotly-base-artifacts-$ver.tar.gz")
bind_artifact!(artifacts_toml, "plotly-base-artifacts", plotlyartifacts_hash; download_info=[
("$repo/releases/download/plotly-base-artifacts-$ver/plotly-base-artifacts-$ver.tar.gz", tarball_hash)
])
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 129 | using Documenter, PlotlyBase
makedocs(
# options
modules=[PlotlyBase],
format=:html,
sitename="PlotlyBase.jl"
)
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 5055 | module PlotlyBase
using Base.Iterators
using JSON
using DocStringExtensions
using Requires
using UUIDs
using Dates
using Logging
using ColorSchemes
using Parameters
import Base: ==
using Statistics: mean
using DelimitedFiles: readdlm
# import LaTeXStrings and export the handy macros
using LaTeXStrings
export @L_mstr, @L_str
using Pkg.Artifacts
# export some names from JSON
export json
_symbol_dict(x) = x
_symbol_dict(d::AbstractDict) =
Dict{Symbol,Any}([(Symbol(k), _symbol_dict(v)) for (k, v) in d])
const _Maybe{T} = Union{Missing,T}
abstract type AbstractPlotlyAttribute end
mutable struct PlotlyAttribute{T <: AbstractDict{Symbol,Any}} <: AbstractPlotlyAttribute
fields::T
end
const _ATTR = PlotlyAttribute{Dict{Symbol,Any}}
struct Cycler
vals::Vector
end
Base.isempty(c::Cycler) = isempty(c.vals)
Base.length(c::Cycler) = length(c.vals)
Cycler(t::Tuple) = Cycler(collect(t))
Cycler(x::Union{String,Number,Date,Symbol}) = Cycler([x])
function Base.getindex(c::Cycler, ix::Integer)
n = length(c.vals)
@inbounds v = c.vals[mod1(ix, n)]
v
end
function Base.getindex(c::Cycler, ixs::AbstractVector{<:Integer})
[c[i] for i in ixs]
end
Base.iterate(c::Cycler, s::Int=1) = c[s], s + 1
Base.IteratorSize(::Cycler) = Base.IsInfinite()
# include these here because they are used below
include("plot_config.jl")
include("subplot_utils.jl")
include("traces_layouts.jl")
const PLOTSCHEMA = attr();
function get_plotschema()
if _isempty(PLOTSCHEMA)
out = JSON.parsefile(joinpath(artifact"plotly-base-artifacts", "plot-schema.json"))
PLOTSCHEMA.fields = _symbol_dict(out)
end
return PLOTSCHEMA
end
# core plot object
mutable struct Plot{TT<:AbstractVector{<:AbstractTrace},TL<:AbstractLayout,TF<:AbstractVector{<:PlotlyFrame}}
data::TT
layout::TL
frames::TF
divid::UUID
config::PlotConfig
end
# Default `convert` fallback constructor
Plot(p::Plot) = p
# include the rest of the core parts of the package
include("util.jl")
include("json.jl")
include("subplots.jl")
include("api.jl")
include("convenience_api.jl")
include("recession_bands.jl")
include("output.jl")
include("templates.jl")
include("colors.jl")
# Set some defaults for constructing `Plot`s
function Plot(;config::PlotConfig=PlotConfig())
Plot(GenericTrace{Dict{Symbol,Any}}[], Layout(), PlotlyFrame[], uuid4(), config)
end
function Plot(data::AbstractVector{<:AbstractTrace}, layout=Layout(), frames::AbstractVector{<:PlotlyFrame}=PlotlyFrame[];
config::PlotConfig=PlotConfig())
Plot(data, layout, frames, uuid4(), config)
end
function Plot(data::AbstractTrace, layout=Layout(), frames::AbstractVector{<:PlotlyFrame}=PlotlyFrame[];
config::PlotConfig=PlotConfig())
Plot([data], layout, frames; config=config)
end
# empty plot
function Plot(layout::Layout, frames::AbstractVector{<:PlotlyFrame}=PlotlyFrame[];
config::PlotConfig=PlotConfig())
Plot(GenericTrace[], layout, frames; config=config)
end
# NOTE: we export trace constructing types from inside api.jl
# NOTE: we export names of shapes from traces_layouts.jl
export
# core types
Plot, GenericTrace, PlotlyFrame, Layout, Shape, AbstractTrace, AbstractLayout,
PlotConfig, Spec, Subplots, Inset,
# plotly.js api methods
restyle!, relayout!, update!, addtraces!, deletetraces!, movetraces!,
redraw!, extendtraces!, prependtraces!, purge!, to_image, download_image,
react!,
# non-!-versions (forks, then applies, then returns fork)
restyle, relayout, update, addtraces, deletetraces, movetraces, redraw,
extendtraces, prependtraces, react,
# helper methods
plot, fork, vline, hline, attr, frame, add_trace!,
# templates
templates, Template,
# new trace types
stem,
# convenience stuff
add_recession_bands!, Cycler,
# other
savejson, colors
function __init__()
@require IJulia="7073ff75-c697-5162-941a-fcdaad2a7d2a" begin
function IJulia.display_dict(p::Plot)
Dict(
"application/vnd.plotly.v1+json" => JSON.lower(p),
"text/plain" => sprint(show, "text/plain", p),
"text/html" => let
buf = IOBuffer()
show(buf, MIME("text/html"), p, include_plotlyjs="require")
String(resize!(buf.data, buf.size))
end
)
end
end
@require DataFrames="a93c6f00-e57d-5684-b7b6-d8193f3e46c0" include("dataframes_api.jl")
@require Distributions="31c24e10-a181-5473-b8eb-7969acd0382f" include("distributions.jl")
@require Colors="5ae59095-9a9b-59fe-a467-6f913c188581" begin
_json_lower(a::Colors.Colorant) = string("#", Colors.hex(a))
end
@require JSON2="2535ab7d-5cd8-5a07-80ac-9b1792aadce3" JSON2.write(io::IO, p::Plot) = begin
data = JSON.lower(p)
pop!(data, :config, nothing)
JSON.print(io, data)
end
@require JSON3="0f8b85d8-7281-11e9-16c2-39a750bddbf1" include("json3.jl")
end
end # module
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 22446 | # -------------------------- #
# Standard Julia API methods #
# -------------------------- #
const ROW_COL_TYPE = Union{String,Int,Vector{Int},Colon}
_check_row_col_arg(_l::Layout, x::String, name, dim::Int=1) = x == "all" || @error "Unknown specifier $x for $name, must be an integer or `\"all\"`"
_check_row_col_arg(l::Layout, x::Int, name, dim::Int=1) = x <= size(l.subplots.grid_ref, dim) || error("$name index $x is too large for layout")
_check_row_col_arg(l::Layout, x::Vector{Int}, name, dim::Int=1) = foreach(_val -> _check_row_col_arg(l, _val, name, dim), x)
_check_row_col_arg(_l::Layout, x::Colon, name, dim::Int=1) = nothing
prep_kwarg(pair::Union{Pair,Tuple}) =
(Symbol(replace(string(pair[1]), "_" => ".")), pair[2])
prep_kwargs(pairs::AbstractVector) = Dict(map(prep_kwarg, pairs))
prep_kwargs(pairs::AbstractDict) = Dict(prep_kwarg((k, v)) for (k, v) in pairs)
"""
size(::PlotlyBase.Plot)
Return the size of the plot in pixels. Obtained from the `layout.width` and
`layout.height` fields.
"""
Base.size(p::Plot) = (get(p.layout.fields, :width, 800),
get(p.layout.fields, :height, 450))
const _TRACE_TYPES = [
:bar, :barpolar, :box, :candlestick, :carpet, :choropleth,
:choroplethmapbox, :cone, :contour, :contourcarpet, :densitymapbox,
:funnel, :funnelarea, :heatmap, :heatmapgl, :histogram, :histogram2d,
:histogram2dcontour, :icicle, :image, :indicator, :isosurface, :mesh3d, :ohlc,
:parcats, :parcoords, :pie, :pointcloud, :sankey, :scatter, :scatter3d,
:scattercarpet, :scattergeo, :scattergl, :scattermapbox, :scatterpolar,
:scatterpolargl, :scatterternary, :splom, :streamtube, :sunburst,
:surface, :table, :treemap, :violin, :volume, :waterfall
]
for t in _TRACE_TYPES
str_t = string(t)
code = quote
$t(;kwargs...) = GenericTrace($str_t; kwargs...)
$t(d::AbstractDict; kwargs...) = GenericTrace($str_t, _symbol_dict(d); kwargs...)
end
eval(code)
eval(Expr(:export, t))
end
Base.copy(hf::HF) where {HF <: HasFields} = HF(deepcopy(hf.fields))
Base.copy(p::Plot) = Plot(AbstractTrace[copy(t) for t in p.data], copy(p.layout))
fork(p::Plot) = Plot(deepcopy(p.data), copy(p.layout))
# -------------- #
# Javascript API #
# -------------- #
#=
this function is internal and allows us to match plotly.js semantics in
`resytle!`. The reason is that if you try to set an attribute on a trace with
and array, Plotly.restyle expects an array of arrays.
This means that to set the :x field with [1,2,3], the json should look
something like `[[1,2,3]]`. In Julia we can get this with a one row matrix `[1
2 3]'` or a tuple of arrays `([1, 2, 3], )`. This function applies that logic
and extracts the first element from an array or a tuple before calling
setindex.
All other field types are let through directly
NOTE that the argument `i` here is _not_ the same as the argument `ind` below.
`i` tracks which index we should use when extracting an element from
`v::Union{AbstractArray,Tuple}` whereas `ind` below specifies which trace to
apply the update to. =#
function _apply_restyle_setindex!(hf::Union{AbstractDict,HasFields}, k::Symbol,
v::Union{AbstractArray,Tuple}, i::Int)
setindex!(hf, v[i], k)
end
_apply_restyle_setindex!(hf::Union{AbstractDict,HasFields}, k::Symbol, v, i::Int) =
setindex!(hf, v, k)
#=
Wrap the vector so it repeats to be at least length N
This means
```julia
_prep_restyle_vec_setindex([1, 2], 2) --> [1, 2]
_prep_restyle_vec_setindex([1, 2], 3) --> [1, 2, 1]
_prep_restyle_vec_setindex([1, 2], 4) --> [1, 2, 1, 2]
_prep_restyle_vec_setindex((1, [42, 4]), 2) --> [1, [42, 4]]
_prep_restyle_vec_setindex((1, [42, 4]), 3) --> [1, [42, 4], 1]
_prep_restyle_vec_setindex((1, [42, 4]), 4) --> [1, [42, 4], 1, [42, 4]]
``` =#
_prep_restyle_vec_setindex(v::AbstractVector, N::Int) =
repeat(v, outer=[ceil(Int, N / length(v))])[1:N]
# treat tuples like vectors, just like JSON.json does
_prep_restyle_vec_setindex(v::Tuple, N::Int) =
_prep_restyle_vec_setindex(Any[i for i in v], N)
# everything else just goes through
_prep_restyle_vec_setindex(v, N::Int) = v
function _update_fields(hf::GenericTrace, i::Int, update::Dict=Dict(); kwargs...)
# apply updates in the dict w/out `_` processing
for (k, v) in update
_apply_restyle_setindex!(hf.fields, k, v, i)
end
for (k, v) in kwargs
_apply_restyle_setindex!(hf, k, v, i)
end
hf
end
"""
relayout!(l::Layout, update::AbstractDict=Dict(); kwargs...)
Update `l` using update dict and/or kwargs
"""
function relayout!(l::Layout, update::AbstractDict=Dict(); kwargs...)
merge!(l.fields, update) # apply updates in the dict w/out `_` processing
merge!(l, Layout(;kwargs...))
l
end
function relayout!(dest::Layout, src::Layout; kwargs...)
merge!(dest, src)
relayout!(dest; kwargs...)
end
"""
relayout!(p::Plot, update::AbstractDict=Dict(); kwargs...)
Update `p.layout` on using update dict and/or kwargs
"""
relayout!(p::Plot, args...; kwargs...) =
relayout!(p.layout, args...; kwargs...)
"""
restyle!(gt::GenericTrace, i::Int=1, update::AbstractDict=Dict(); kwargs...)
Update trace `gt` using dict/kwargs, assuming it was the `i`th ind in a call
to `restyle!(::Plot, ...)`
"""
restyle!(gt::GenericTrace, i::Int=1, update::AbstractDict=Dict(); kwargs...) =
_update_fields(gt, i, update; kwargs...)
"""
restyle!(p::Plot, ind::Int=1, update::AbstractDict=Dict(); kwargs...)
Update `p.data[ind]` using update dict and/or kwargs
"""
restyle!(p::Plot, ind::Int, update::AbstractDict=Dict(); kwargs...) =
restyle!(p.data[ind], 1, update; kwargs...)
"""
restyle!(::Plot, ::AbstractVector{Int}, ::AbstractDict=Dict(); kwargs...)
Update specific traces at `p.data[inds]` using update dict and/or kwargs
"""
function restyle!(p::Plot, inds::AbstractVector{Int},
update::AbstractDict=Dict(); kwargs...)
N = length(inds)
kw = Dict{Symbol,Any}(kwargs)
# prepare update and kw dicts for vectorized application
for d in (kw, update)
for (k, v) in d
d[k] = _prep_restyle_vec_setindex(v, N)
end
end
map((ind, i) -> restyle!(p.data[ind], i, update; kw...), inds, 1:N)
end
"""
restyle!(p::Plot, update::AbstractDict=Dict(); kwargs...)
Update all traces using update dict and/or kwargs
"""
restyle!(p::Plot, update::AbstractDict=Dict(); kwargs...) =
restyle!(p, 1:length(p.data), update; kwargs...)
"""
The `restyle!` method follows the semantics of the `Plotly.restyle` function in
plotly.js. Specifically the following rules are applied when trying to set
an attribute `k` to a value `v` on trace `ind`, which happens to be the `i`th
trace listed in the vector of `ind`s (if `ind` is a scalar then `i` is always
equal to 1)
- if `v` is an array or a tuple (both translated to javascript arrays when
`json(v)` is called) then `p.data[ind][k]` will be set to `v[i]`. See examples
below
- if `v` is any other type (any scalar type), then `k` is set directly to `v`.
**Examples**
```julia
# set marker color on first two traces to be red
restyle!(p, [1, 2], marker_color="red")
# set marker color on trace 1 to be green and trace 2 to be red
restyle!(p, [2, 1], marker_color=["red", "green"])
# set marker color on trace 1 to be red. green is not used
restyle!(p, 1, marker_color=["red", "green"])
# set the first marker on trace 1 to red, the second marker on trace 1 to green
restyle!(p, 1, marker_color=(["red", "green"],))
# suppose p has 3 traces.
# sets marker color on trace 1 to ["red", "green"]
# sets marker color on trace 2 to "blue"
# sets marker color on trace 3 to ["red", "green"]
restyle!(p, 1:3, marker_color=(["red", "green"], "blue"))
```
"""
restyle!
function update!(
p::Plot, ind::Union{AbstractVector{Int},Int},
update::AbstractDict=Dict(); layout::Layout=Layout(),
kwargs...
)
relayout!(p; layout.fields...)
restyle!(p, ind, update; kwargs...)
p
end
function update!(p::Plot, update=Dict(); layout::Layout=Layout(), kwargs...)
update!(p, 1:length(p.data), update; layout=layout, kwargs...)
end
"""
Apply both `restyle!` and `relayout!` to the plot. Layout arguments are
specified by passing an instance of `Layout` to the `layout` keyword argument.
The `update` Dict (optional) and all keyword arguments will be passed to
restyle
## Example
```jlcon
julia> p = Plot([scatter(y=[1, 2, 3])], Layout(yaxis_title="this is y"));
julia> print(json(p, 2))
{
"layout": {
"margin": {
"l": 50,
"b": 50,
"r": 50,
"t": 60
},
"yaxis": {
"title": "this is y"
}
},
"data": [
{
"y": [
1,
2,
3
],
"type": "scatter"
}
]
}
julia> update!(p, Dict(:marker => Dict(:color => "red")), layout=Layout(title="this is a title"), marker_symbol="star");
julia> print(json(p, 2))
{
"layout": {
"margin": {
"l": 50,
"b": 50,
"r": 50,
"t": 60
},
"yaxis": {
"title": "this is y"
},
"title": "this is a title"
},
"data": [
{
"y": [
1,
2,
3
],
"type": "scatter",
"marker": {
"color": "red",
"symbol": "star"
}
}
]
}
```
"""
update!
"""
addtraces!(p::Plot, traces::AbstractTrace...)
Add trace(s) to the end of the Plot's array of data
"""
addtraces!(p::Plot, traces::AbstractTrace...) = push!(p.data, traces...)
"""
addtraces!(p::Plot, i::Int, traces::AbstractTrace...)
Add trace(s) at a specified location in the Plot's array of data.
The new traces will start at index `p.data[i]`
"""
function addtraces!(p::Plot, i::Int, traces::AbstractTrace...)
new_data = vcat(p.data[1:i - 1], traces..., p.data[i:end])
p.data = new_data
end
"""
deletetraces!(p::Plot, inds::Int...) =
Remove the traces at the specified indices
"""
deletetraces!(p::Plot, inds::Int...) =
(p.data = p.data[setdiff(1:length(p.data), inds)])
"""
movetraces!(p::Plot, to_end::Int...)
Move one or more traces to the end of the data array"
"""
movetraces!(p::Plot, to_end::Int...) =
(p.data = p.data[vcat(setdiff(1:length(p.data), to_end), to_end...)])
function _move_one!(x::AbstractArray, from::Int, to::Int)
el = splice!(x, from) # extract the element
splice!(x, to:to - 1, (el,)) # put it back in the new position
x
end
"""
movetraces!(p::Plot, src::AbstractVector{Int}, dest::AbstractVector{Int})
Move traces from indices `src` to indices `dest`.
Both `src` and `dest` must be `Vector{Int}`
"""
movetraces!(p::Plot, src::AbstractVector{Int}, dest::AbstractVector{Int}) =
(map((i, j) -> _move_one!(p.data, i, j), src, dest); p)
function purge!(p::Plot)
empty!(p.data)
p.layout = Layout()
nothing
end
function react!(p::Plot, data::AbstractVector{<:AbstractTrace}, layout::Layout)
p.data = data
p.layout = layout
nothing
end
# no-op here
redraw!(p::Plot) = nothing
to_image(p::Plot; kwargs...) = nothing
download_image(p::Plot; kwargs...) = nothing
_tovec(v) = _tovec([v])
_tovec(v::Vector) = eltype(v) <: Vector ? v : Vector[v]
"""
extendtraces!(::Plot, ::Dict{Union{Symbol,AbstractString},AbstractVector{Vector{Any}}}), indices, maxpoints)
Extend one or more traces with more data. A few notes about the structure of the
update dict are important to remember:
- The keys of the dict should be of type `Symbol` or `AbstractString` specifying
the trace attribute to be updated. These attributes must already exist in the
trace
- The values of the dict _must be_ a `Vector` of `Vector` of data. The outer index
tells Plotly which trace to update, whereas the `Vector` at that index contains
the value to be appended to the trace attribute.
These concepts are best understood by example:
```julia
# adds the values [1, 3] to the end of the first trace's y attribute and doesn't
# remove any points
extendtraces!(p, Dict(:y=>Vector[[1, 3]]), [1], -1)
extendtraces!(p, Dict(:y=>Vector[[1, 3]])) # equivalent to above
```
```julia
# adds the values [1, 3] to the end of the third trace's marker.size attribute
# and [5,5,6] to the end of the 5th traces marker.size -- leaving at most 10
# points per marker.size attribute
extendtraces!(p, Dict("marker.size"=>Vector[[1, 3], [5, 5, 6]]), [3, 5], 10)
```
"""
function extendtraces!(p::Plot, update::AbstractDict, indices::AbstractVector{Int}=[1],
maxpoints=-1)
# TODO: maxpoints not handled here
for (ix, p_ix) in enumerate(indices)
tr = p.data[p_ix]
for k in keys(update)
v = update[k][ix]
tr[k] = push!(tr[k], v...)
end
end
end
"""
prependtraces!(p::Plot, update::AbstractDict, indices::AbstractVector{Int}=[1],
maxpoints=-1)
The API for `prependtraces` is equivalent to that for `extendtraces` except that
the data is added to the front of the traces attributes instead of the end. See
Those docstrings for more information
"""
function prependtraces!(p::Plot, update::AbstractDict, indices::AbstractVector{Int}=[1],
maxpoints=-1)
# TODO: maxpoints not handled here
for (ix, p_ix) in enumerate(indices)
tr = p.data[p_ix]
for k in keys(update)
v = update[k][ix]
tr[k] = vcat(v, tr[k])
end
end
end
for f in (:extendtraces!, :prependtraces!)
@eval begin
$(f)(p::Plot, inds::Vector{Int}=[1], maxpoints=-1; update...) =
($f)(p, Dict(map(x -> (x[1], _tovec(x[2])), update)), inds, maxpoints)
$(f)(p::Plot, ind::Int, maxpoints=-1; update...) =
($f)(p, [ind], maxpoints; update...)
$(f)(p::Plot, update::AbstractDict, ind::Int, maxpoints=-1) =
($f)(p, update, [ind], maxpoints)
end
end
for f in [:restyle, :relayout, :update, :addtraces, :deletetraces,
:movetraces, :redraw, :extendtraces, :prependtraces, :purge, :react]
f! = Symbol(f, "!")
@eval function $(f)(p::Plot, args...; kwargs...)
out = fork(p)
$(f!)(out, args...; kwargs...)
out
end
end
function _add_trace!(p::Plot, trace::GenericTrace, row::Int, col::Int, secondary_y::Bool)
refs = p.layout.subplots.grid_ref[row, col]
if secondary_y && length(refs) == 1
msg = "To use secondary_y, you must have created the Subplot with seconary_y=true"
error(msg)
end
ref = refs[secondary_y ? 2 : 1]
ref_trace = deepcopy(trace)
merge!(ref_trace, ref.trace_kwargs)
push!(p.data, ref_trace)
p
end
function _add_trace!(p::Plot, trace::GenericTrace, row::ROW_COL_TYPE, col::ROW_COL_TYPE, secondary_y::Bool)
for refs in p.layout.subplots.grid_ref[row, col]
if secondary_y && length(refs) == 1
msg = "To use secondary_y, you must have created the Subplot with seconary_y=true"
error(msg)
end
ref = refs[secondary_y ? 2 : 1]
ref_trace = deepcopy(trace)
merge!(ref_trace, ref.trace_kwargs)
push!(p.data, ref_trace)
end
p
end
function add_trace!(p::Plot, trace::GenericTrace; row::ROW_COL_TYPE=1, col::ROW_COL_TYPE=1, secondary_y::Bool=false)
# NOTE: This causes the domain to not be set for the first plot (row=1, col=1)
# if row == 1 && col == 1
# push!(p.data, trace)
# return p
# end
_check_row_col_arg(p.layout, row, "row", 1)
_check_row_col_arg(p.layout, col, "col", 2)
gridref_row_ix = row isa String ? Colon() : row
gridref_col_ix = col isa String ? Colon() : col
_add_trace!(p, trace, gridref_row_ix, gridref_col_ix, secondary_y)
p
end
## internal helpers
_get_colorway(p::Plot) = _get_colorway(p.layout)
function _get_colorway(l::Layout)
D3_colorway = [
"#1F77B4",
"#FF7F0E",
"#2CA02C",
"#D62728",
"#9467BD",
"#8C564B",
"#E377C2",
"#7F7F7F",
"#BCBD22",
"#17BECF",
]
Cycler(get(l.template.layout, :colorway, D3_colorway))
end
_get_seq_from_template_data(p::Plot, args...; kwargs...) = _get_seq_from_template_data(p.layout, args...; kwargs...)
function _get_seq_from_template_data(
l::Layout,
default::Cycler,
property_sub_path::Symbol,
root_template_path::Symbol=:scatter
)
template_specs = get(l.template.data, root_template_path, Dict())
if !isempty(template_specs)
seq = []
default_ix = 0
has_any_specs = false
for spec in template_specs
want = get(spec, property_sub_path, Dict())
if ismissing(want) || isempty(want)
push!(seq, default[default_ix += 1])
else
has_any_specs = true
push!(seq, want)
end
end
return has_any_specs ? Cycler(seq) : default
end
return default
end
function _get_line_dash_seq(l::Union{Layout,Plot})
default_line_dash = Cycler([
"solid",
"dot",
"dash",
"longdash",
"dashdot",
"longdashdot",
])
return _get_seq_from_template_data(l, default_line_dash, :line_dash, :scatter)
end
function _get_marker_symbol_seq(l::Union{Layout,Plot})
default = Cycler([
"circle"
"diamond"
"cross"
"triangle"
"square"
"x"
"pentagon"
"hexagon"
"hexagon2"
"octagon"
"star"
"hexagram"
"hourglass"
"bowtie"
"asterisk"
"hash"
"y"
"line"
])
return _get_seq_from_template_data(l, default, :marker_symbol, :scatter)
end
function _get_default_seq(l::Layout, attribute::Symbol)
_getter_funcs = Dict(
:line_dash => _get_line_dash_seq,
:symbol => _get_marker_symbol_seq,
:color => _get_colorway
)
if attribute in keys(_getter_funcs)
return _getter_funcs[attribute](l)
end
error("Don't know how to get defaults for $(attribute)")
end
function _update_all_layout_type!(l::Layout, layout_type::Symbol, with::PlotlyAttribute)
l[layout_type] = with
for (k, v) in pairs(l)
if startswith(string(k), string(layout_type))
l[k] = with
end
end
l
end
const _layout_obj_updaters = [:update_xaxes! => :xaxis, :update_yaxes! => :yaxis, :update_geos! => :geo, :update_mapboxes! => :mapbox, :update_polars! => :polar, :update_scenes! => :scene, :update_ternaries! => :ternary]
for (k1, k2) in _layout_obj_updaters
@eval $(k1)(l::Layout, with::PlotlyAttribute=attr(); kwargs...) = _update_all_layout_type!(l, $(Meta.quot(k2)), merge(with, attr(;kwargs...)))
@eval $(k1)(p::Plot, with::PlotlyAttribute=attr(); kwargs...) = $(k1)(p.layout, merge(with, attr(;kwargs...)))
@eval export $k1
end
const _layout_vector_updaters = [:update_annotations! => :(layout.annotations), :update_shapes! => :(layout.shapes), :update_images! => :(layout.images)]
for (k1, k2) in _layout_vector_updaters
@eval function $(k1)(layout::Layout, with::PlotlyAttribute; kwargs...)
final_with = merge(with, attr(;kwargs...))
for val in $(k2)
merge!(val, final_with)
end
end
@eval $(k1)(p::Plot, with::PlotlyAttribute=attr(); kwargs...) = $(k1)(p.layout, merge(with, attr(;kwargs...)))
@eval export $(k1)
end
function _get_subplot_grid_refs(l::Layout, row::ROW_COL_TYPE, col::ROW_COL_TYPE)
gridref_row_ix = row isa String ? Colon() : row
gridref_col_ix = col isa String ? Colon() : col
out = l.subplots.grid_ref[gridref_row_ix, gridref_col_ix]
(out[1] isa Array) ? out : [out]
end
function _add_many_layout_objects!(
l::Layout, base_val::HasFields, direction::Char, row::ROW_COL_TYPE, col::ROW_COL_TYPE, layout_key::Symbol
)
_check_row_col_arg(l, row, "row", 1)
_check_row_col_arg(l, col, "col", 2)
known_directions = ['h', 'v', 'X', 'I']
if !(direction in known_directions)
error("direction must be one of $(known_directions)")
end
layout_vec = get(l, layout_key, [])
for refs in _get_subplot_grid_refs(l, row, col)
# refs is always a vector...
ref = refs[1]
# find xref and yref
xax, yax = string.(ref.layout_keys)
xid = string("x", xax[6:end])
yid = string("y", yax[6:end])
new_val = deepcopy(base_val)
if direction == 'h'
new_val.xref = "$xid domain"
new_val.yref = yid
end
if direction == 'v'
new_val.xref = xid
new_val.yref = "$yid domain"
end
if direction == 'X'
new_val.xref = xid
new_val.yref = yid
end
if direction == 'I'
new_val.xref = "$xid domain"
new_val.yref = "$yid domain"
end
push!(layout_vec, new_val)
end
l[layout_key] = layout_vec
end
_add_many_layout_objects!(p::Plot, args...) = _add_many_layout_objects!(p.layout, args...)
_add_many_shapes!(l::Layout, args...) = _add_many_layout_objects!(l, args..., :shapes)
_add_many_shapes!(p::Plot, args...) = _add_many_shapes!(p.layout, args...)
function add_hrect!(l::Union{Plot,Layout}, y0, y1; row::ROW_COL_TYPE="all", col::ROW_COL_TYPE="all", kw...)
base_shape = rect(0, 1, y0, y1; kw...)
_add_many_shapes!(l, base_shape, 'h', row, col)
end
function add_hline!(l::Union{Plot,Layout}, y; row::ROW_COL_TYPE="all", col::ROW_COL_TYPE="all", kw...)
base_shape = hline(y; kw...)
_add_many_shapes!(l, base_shape, 'h', row, col)
end
function add_vrect!(l::Union{Plot,Layout}, x0, x1; row::ROW_COL_TYPE="all", col::ROW_COL_TYPE="all", kw...)
base_shape = rect(x0, x1, 0, 1; kw...)
_add_many_shapes!(l, base_shape, 'v', row, col)
end
function add_vline!(l::Union{Plot,Layout}, x; row::ROW_COL_TYPE="all", col::ROW_COL_TYPE="all", kw...)
base_shape = vline(x; kw...)
_add_many_shapes!(l, base_shape, 'v', row, col)
end
function add_shape!(l::Union{Plot,Layout}, base_shape; row::ROW_COL_TYPE="all", col::ROW_COL_TYPE="all")
_add_many_shapes!(l, base_shape, 'X', row, col)
end
function add_layout_image!(l::Union{Plot,Layout}, base_img; row::ROW_COL_TYPE="all", col::ROW_COL_TYPE="all")
_add_many_layout_objects!(l, base_img, 'I', row, col, :images)
end
export add_hrect!, add_hline!, add_vrect!, add_vline!, add_shape!, add_layout_image!
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 809 | @with_kw struct _color_types
sequential::Dict{Symbol,ColorScheme} = filter(kv -> occursin("sequential", kv[2].notes), pairs(colorschemes))
diverging::Dict{Symbol,ColorScheme} = filter(kv -> occursin("diver", kv[2].notes), pairs(colorschemes))
cyclical::Dict{Symbol,ColorScheme} = filter(kv -> occursin("cycl", kv[2].notes), pairs(colorschemes))
discrete::Dict{Symbol,ColorScheme} = filter(kv -> occursin("quali", kv[2].notes), pairs(colorschemes))
all::Dict{Symbol,ColorScheme} = colorschemes
end
Base.getindex(ct::_color_types, k::Symbol) = ct.all[k]
Base.getproperty(ct::_color_types, k::Symbol) = hasfield(_color_types, k) ? getfield(ct, :all) : ct[k]
Base.propertynames(ct::_color_types) = vcat(collect(fieldnames(typeof(ct))), collect(keys(ct.all)))
const colors = _color_types()
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 6515 | function GenericTrace(x::AbstractArray, y::AbstractArray;
kind="scatter", kwargs...)
GenericTrace(kind; x=x, y=y, kwargs...)
end
"""
$(SIGNATURES)
Build a plot of with one trace of type `kind`and set `x` to x and `y` to y. All
keyword arguments are passed directly as keyword arguments to the constructed
trace.
**NOTE**: If `y` is a matrix, one trace is constructed for each column of `y`
**NOTE**: If `x` and `y` are both matrices, they must have the same number of
columns (say `N`). Then `N` traces are constructed, where the `i`th column of
`x` is paired with the `i`th column of `y`.
"""
function Plot(
x::AbstractVector{T}, y::AbstractVector, l::Layout=Layout();
kind="scatter",
config::PlotConfig=PlotConfig(),
kwargs...
) where T <: _Scalar
Plot(GenericTrace(x, y; kind=kind, kwargs...), l, config=config)
end
function Plot(
x::AbstractVector{T}, y::AbstractMatrix, l::Layout=Layout();
config::PlotConfig=PlotConfig(),
kwargs...
) where T <: _Scalar
traces = GenericTrace[
GenericTrace(x, getindex(y, :, i); kwargs...)
for i in 1:size(y, 2)
]
Plot(traces, l, config=config)
end
function Plot(
x::AbstractVector{T}, y::AbstractMatrix, l::Layout=Layout();
config::PlotConfig=PlotConfig(),
kwargs...
) where T <: AbstractVector
size(x, 1) == size(y, 2) || error("x and y must have same number of cols")
traces = GenericTrace[
GenericTrace(x[i], getindex(y, :, i); kwargs...)
for i in 1:size(y, 2)
]
Plot(traces, l; config=config)
end
function Plot(
x::AbstractMatrix, y::AbstractMatrix, l::Layout=Layout();
config::PlotConfig=PlotConfig(),
kwargs...
)
if size(x, 2) == 1
# use method above
Plot(getindex(x, :, 1), y, l; config=config, kwargs...)
end
size(x, 2) == size(y, 2) || error("x and y must have same number of cols")
traces = GenericTrace[
GenericTrace(getindex(x, :, i), getindex(y, :, i); kwargs...)
for i in 1:size(y, 2)
]
Plot(traces, l; config=config)
end
# AbstractArray{T,N}
"""
$(SIGNATURES)
Build a scatter plot and set `y` to y. All keyword arguments are passed directly
as keyword arguments to the constructed scatter.
"""
function Plot(y::AbstractArray{T}, l::Layout=Layout(); kwargs...) where T <: _Scalar
# call methods above to get many traces if y is >1d
Plot(1:size(y, 1), y, l; kwargs...)
end
"""
$(SIGNATURES)
Construct a plot of `f` from `x0` to `x1`, using the layout `l`. All
keyword arguments are applied to the constructed trace.
"""
function Plot(
f::Function, x0::Number, x1::Number, l::Layout=Layout();
config::PlotConfig=PlotConfig(),
kwargs...
)
x = range(x0, stop=x1, length=50)
y = [f(_) for _ in x]
Plot(GenericTrace(x, y; name=Symbol(f), kwargs...), l, config=config)
end
"""
$(SIGNATURES)
For each function in `f` in `fs`, construct a scatter trace that plots `f` from
`x0` to `x1`, using the layout `l`. All keyword arguments are applied to all
constructed traces.
"""
function Plot(
fs::AbstractVector{Function}, x0::Number, x1::Number, l::Layout=Layout();
config::PlotConfig=PlotConfig(),
kwargs...
)
x = range(x0, stop=x1, length=50)
traces = GenericTrace[
GenericTrace(x, map(f, x); name=Symbol(f), kwargs...)
for f in fs
]
Plot(traces, l; config=config)
end
_make_attrable(x::Union{Number,Symbol,String,Missing,Nothing}) = x
_make_attrable(x::AbstractArray) = _make_attrable.(x)
function _make_attrable(x::Union{NamedTuple,_LikeAssociative})
out = attr()
for (k, v) in pairs(x)
out[k] = _make_attrable(v)
end
out
end
function attr(nt::NamedTuple)
out = attr()
for (k, v) in pairs(nt)
out[k] = _make_attrable(v)
end
out
end
# for reading from a namedtuple that Dash.jl
# generates when using `dcc_graph` as state
function Plot(fig::NamedTuple{(:layout, :frames, :data)})
traces = GenericTrace[]
for tr in fig.data
kind = hasproperty(tr, :kind) ? tr.kind : "scatter"
push!(traces, GenericTrace(kind; attr(tr)...))
end
layout_attr = attr(fig.layout)
if hasproperty(layout_attr, :template)
t_layout = Layout()
if hasproperty(layout_attr.template, :layout)
t_layout = Layout(;attr(fig.layout.template.layout)...)
end
pop!(t_layout, :template) # remove nested template
# now translate traces into _ATTR[]
t_data = Dict{Symbol,Vector{_ATTR}}()
if hasproperty(layout_attr, :data)
for (k, v) in pairs(fig.layout.template.data)
attrs = _ATTR[]
for trace_spec in v
push!(attrs, attr(trace_spec))
end
t_data[Symbol(k)] = attrs
end
end
layout_attr.fields[:template] = Template(t_data, t_layout)
end
layout = Layout(layout_attr.fields)
frames = PlotlyFrame[]
for f in fig.frames
push!(frames, frame(;f...))
end
Plot(traces, layout, frames)
end
Plot(fig::NamedTuple{(:layout, :data)}) = Plot((layout = fig.layout, frames = [], data = fig.data))
Plot(fig::NamedTuple{(:data, :layout)}) = Plot((layout = fig.layout, frames = [], data = fig.data))
for order in [(:data, :layout, :frames), (:data, :frames, :layout), (:frames, :data, :layout), (:frames, :layout, :data), (:layout, :data, :frames)]
@eval Plot(fig::NamedTuple{$(order)}) = Plot((layout = fig.layout, frames = fig.frames, data = fig.data))
end
"""
$(SIGNATURES)
Creates a "stem" or "lollipop" trace. It is implemented using plotly.js's
`scatter` type, using the error bars to draw the stem.
## Keyword Arguments:
* All properties accepted by `scatter` except `error_y`, which is used to draw
the stems
* stem_color - sets the color of the stems
* stem_thickness - sets the thickness of the stems
"""
function stem(;y=nothing, stem_color="grey", stem_thickness=1, kwargs...)
line_up = -min.(y, 0)
line_down = max.(y, 0)
trace = scatter(; y=y, text=y, marker_size=10, mode="markers", hoverinfo="text", kwargs...)
trace.fields[:error_y] = Dict(
:type => "data",
:symmetric => false,
:array => line_up,
:arrayminus => line_down,
:visible => true,
:color => stem_color,
:width => 0,
:thickness => stem_thickness)
trace
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 18663 | using Base:Symbol
# utilities
_has_group(df::DataFrames.AbstractDataFrame, group::Any) = false
_has_group(df::DataFrames.AbstractDataFrame, group::Symbol) = hasproperty(df, group)
function _has_group(df::DataFrames.AbstractDataFrame, group::Vector{Symbol})
all(x -> hasproperty(df, x), group)
end
_group_name(df::DataFrames.AbstractDataFrame, group::Symbol) = df[1, group]
function _group_name(df::DataFrames.AbstractDataFrame, groups::Vector{Symbol})
join([df[1, g] for g in groups], ", ")
end
function _obtain_setindex_val(container::DataFrames.AbstractDataFrame, val::Vector{Symbol}, key::Symbol)
if key == :dimensions
out = []
for v in val
if hasproperty(container, v)
push!(out, attr(label=v, values=container[!, v]))
else
push!(out, v)
end
end
return out
end
val
end
# hook into setindex!
function _obtain_setindex_val(container::DataFrames.AbstractDataFrame, val::Symbol, key::_Maybe{Symbol}=missing)
if hasproperty(container, val)
if isequal(key, :dimensions)
return [attr(label=val, values=container[!, val])]
end
return container[!, val]
end
val
end
"""
$(SIGNATURES)
Build a trace of kind `kind`, using the columns of `df` where possible. In
particular for all keyword arguments, if the value of the keyword argument is a
Symbol and matches one of the column names of `df`, replace the value of the
keyword argument with the column of `df`
If `group` is passed and is a Symbol that is one of the column names of `df`,
then call `by(df, group)` and construct one trace per SubDataFrame, passing
all other keyword arguments. This means all keyword arguments are passed
applied to all traces
Also, when using this routine you can pass a function as a value for any
keyword argument. This function will be replaced by calling the function on the
DataFrame. For example, if I were to pass `name=(df) -> "Wage (average =
\$(mean(df[!, :X1])))"` then the `name` attribute on the trace would be replaced by
the `Wage (average = XX)`, where `XX` is the average of the `X1` column in the
DataFrame.
The ability to pass functions as values for keyword arguments is particularly
useful when using the `group` keyword arugment, as the function will be applied
to each SubDataFrame. In the example above, the name attribute would set a
different mean for each group.
"""
function GenericTrace(df::DataFrames.AbstractDataFrame; group=missing, kind="scatter", kwargs...)
if _has_group(df, group)
_traces = []
for dfg in collect(DataFrames.groupby(df, group))
trace = GenericTrace(
dfg;
kind=kind,
name=_group_name(dfg, group),
legendgroup=_group_name(dfg, group),
kwargs...
)
push!(_traces, trace)
end
return GenericTrace[t for t in _traces]
else
if !ismissing(group)
@warn "Unknown group $(group), skipping"
end
end
attrs = attr()
for (key, value) in kwargs
# use `df` as `container` in setindex! for each property
attrs[df, key] = value
end
if Symbol(kind) == :splom && :dimensions in keys(kwargs)
attrs[:diagonal_visible] = false
@assert attrs.dimensions isa Vector
for d in attrs.dimensions
d.axis_matches = true
end
end
GenericTrace(kind; attrs...)
end
"""
$(SIGNATURES)
Pass the provided values of `x` and `y` as keyword arguments for constructing
the trace from `df`. See other method for more information
"""
function GenericTrace(df::DataFrames.AbstractDataFrame, x::Symbol, y::Symbol; kwargs...)
GenericTrace(df; x=x, y=y, kwargs...)
end
"""
$(SIGNATURES)
Pass the provided value `y` as keyword argument for constructing the trace from
`df`. See other method for more information
"""
function GenericTrace(df::DataFrames.AbstractDataFrame, y::Symbol; kwargs...)
GenericTrace(df; y=y, kwargs...)
end
"""
$(SIGNATURES)
lexographically sort obsvals to match orders, given keys into orders
"""
function _partial_val_sortperm(
obsvals::Vector{<:Vector}, orders::Dict{Symbol}, order_keys::Vector{Symbol}
)
if length(obsvals) == 0 && length(order_keys) > 0
error("obsvals is empty, but order_keys is not")
end
if length(obsvals) > 0 && (length(obsvals[1]) !== length(order_keys))
error("obsvals[i] and order_keys must be same length")
end
to_sort = deepcopy(obsvals)
for trace_i in 1:length(obsvals)
for (group_i, colname) in enumerate(order_keys)
if haskey(orders, Symbol(colname))
want_order = orders[Symbol(colname)]
group_val = obsvals[trace_i][group_i]
to_sort[trace_i][group_i] = findfirst(isequal(group_val), want_order)
end
end
end
return sortperm(to_sort)
end
@inline function _ind2sub_rowmajor(nr::Int, nc::Int, ix::Int)
out = CartesianIndices((nc, nr))[ix].I
CartesianIndex(out[2], out[1])
end
function _faceted_subplot_titles(nr::Int, nc::Int, titles::Vector{String})
subplot_titles = _Maybe{String}[missing for _ in 1:nr, _ in 1:nc]
for title_ix in 1:length(titles)
idx = _ind2sub_rowmajor(nr, nc, title_ix)
subplot_titles[idx] = titles[title_ix]
end
# we need to transpose b/c column vs row major...
permutedims(subplot_titles, (2, 1))
end
"""
$(SIGNATURES)
Construct a plot using the columns of `df` if possible. For each keyword
argument, if the value of the argument is a Symbol and the `df` has a column
whose name matches the value, replace the value with the column of the `df`.
If `group` is passed and is a Symbol that is one of the column names of `df`,
then call `by(df, group)` and construct one trace per SubDataFrame, passing
all other keyword arguments. This means all keyword arguments are passed
applied to all traces
All keyword arguments are passed directly to the trace constructor, with the
following exceptions:
- `category_orders`: A mapping from DataFrame column name to a vector of values that
appear in that column. Wherever the column is used in the chart (facets, x, y, etc.)
the chart elements tied to the column will be sorted according to the vector of values
specified here. This ordering can be partial, in which case all elements that appear
in the DataFrame, but not in the vector of values specified here, will be included
*after* values explicitly listed here.
- `labels`: A mapping from column names (symbols) to text that should appear on the chart.
This can be used to customize the title of axes, title of legend, etc.
- `facet_row`: A symbol denoting which DataFrame column should be used to create
facetted subplots in the vertical direction.
- `facet_col`: A symbol denoting which DataFrame column should be used to create
facetted subplots in the horizontal direction.
- `symbol`: A symbol noting which column should be used for the marker symbol. The values
of this column are not used directly, but rather a new trace will be created for each
unique value in this column. Each trace will have a different marker symbol, such
as circle, diamond, square, etc.
- `color`: Similar to `symbol`, but for the color of a trace
- `line_dash`: Similar to `symbol`, but for the line style of a trace.
"""
function Plot(
df::DataFrames.AbstractDataFrame,
l::Layout=Layout();
category_orders::_Maybe{Union{PlotlyAttribute,<:Dict{Symbol}}}=missing,
labels::_Maybe{Union{PlotlyAttribute,<: Dict}}=missing,
facet_row::_Maybe{Symbol}=missing,
facet_row_wrap::_Maybe{Int}=missing,
facet_col::_Maybe{Symbol}=missing,
facet_col_wrap::_Maybe{Int}=missing,
group::_Maybe{Union{Symbol,Vector{Symbol}}}=missing,
symbol::_Maybe{Symbol}=missing,
color::_Maybe{Symbol}=missing,
line_dash::_Maybe{Symbol}=missing,
kw...
)
groupby_cols = Symbol[]
facet_cols = Symbol[]
kwargs = Dict(kw)
kw_keys = keys(kwargs)
# prep groupings (symbol, color)
_group_args = (;symbol, color, line_dash)
_group_maps = Dict{Symbol,Dict{Any,String}}()
_group_cols = Dict{Symbol,Symbol}()
for (_group_arg, _group_col) in pairs(_group_args)
if !ismissing(_group_col)
if !_has_group(df, _group_col)
error("DataFrame is missing $(_group_col) column, cannot set as $(_group_arg)")
else
# mark that we have this group and add to list of groupby_cols
push!(groupby_cols, _group_col)
# make note of the column that we will use for the groupby
_group_cols[_group_arg] = _group_col
# prepare map from group observation in data to the value in trace
_group_map = Dict{Any,String}()
defaults = _get_default_seq(l, _group_arg)
for (i, val) in enumerate(unique(df[!, _group_col]))
_group_map[val] = defaults[i]
end
_group_maps[_group_arg] = _group_map
end
end
end
group_attr_pairs = [(:symbol, :marker_symbol), (:color, :marker_color), (:line_dash, :line_dash)]
if length(groupby_cols) > 0 && !ismissing(group)
@warn "One of color or symbol present AND group -- group will be ignored"
end
# handle labels
label_map = Dict{Symbol,Any}(pairs((;facet_row, facet_col, color, symbol, line_dash)))
for v in values(label_map)
!ismissing(v) && setindex!(label_map, v, v)
end
if !ismissing(labels)
for (k, v) in pairs(_symbol_dict(labels))
label_map[k] = v
end
end
# axis titles
for ax in [:x, :y, :z]
if ax in kw_keys
ax_val = kwargs[ax]
if !(ax_val isa Symbol)
continue
end
# use `get` below because if something was passed into labels it
# will already be set in `label_map` and we should use the Given
# label. Otherwise use hte value directly.
# e.g. in plot(x=:total_bill, labels=Dict(:total_bill=>"Total Bill"))
# label_map[:total_bill] will be "Total Bill", so we should set
# label_map[:x] = "Total Bill".
label_map[ax] = get(label_map, ax_val, ax_val)
label_map[ax_val] = ax_val
end
end
# Handle category orders
orders = Dict{Symbol,Any}()
if !ismissing(category_orders)
for (k, v) in pairs(category_orders)
if !_has_group(df, k)
@warn "Unknown category $k (dataframe has no column $k). Skipping"
continue
end
unique_vals = unique(df[!, k])
cat_order = deepcopy(v)
for v in unique_vals
if !(v in cat_order)
push!(cat_order, v)
end
end
orders[k] = cat_order
end
end
# handle facets
rows = []
cols = []
if !ismissing(facet_row)
if !_has_group(df, facet_row)
error("DataFrame is missing $(facet_row), cannot set as facet_row")
else
rows = get(orders, facet_row, unique(df[:, facet_row]))
end
end
if !ismissing(facet_col)
if !_has_group(df, facet_col)
error("DataFrame is missing $(facet_col), cannot set as facet_col")
else
cols = get(orders, facet_col, unique(df[:, facet_col]))
end
end
Nrows = max(1, length(rows))
Ncols = max(1, length(cols))
# handle facets here
if !ismissing(facet_row) && !ismissing(facet_col_wrap)
@warn "cannot set facet_row and facet_col_wrap -- setting facet_col_wrap to missing"
facet_col_wrap = missing
end
if !ismissing(facet_col) && !ismissing(facet_row_wrap)
@warn "cannot set facet_col and facet_row_wrap -- setting facet_row_wrap to missing"
facet_row_wrap = missing
end
out_layout = l
if Nrows > 1 || Ncols > 1
subplot_kw = Dict{Symbol,Any}()
if Ncols > 1
if !ismissing(facet_col_wrap) && Ncols > facet_col_wrap
nr = ceil(Int, Ncols / facet_col_wrap)
nc = facet_col_wrap
subplot_kw[:cols] = nc
subplot_kw[:rows] = nr
subplot_kw[:subplot_titles] = _faceted_subplot_titles(
nr, nc, map(x -> "$(label_map[facet_col])=$(x)", cols)
)
subplot_kw[:shared_yaxes] = "all"
else
subplot_kw[:cols] = Ncols
subplot_kw[:column_titles] = map(x -> "$(label_map[facet_col])=$(x)", cols)
subplot_kw[:shared_yaxes] = true
end
push!(facet_cols, facet_col)
end
if Nrows > 1
if !ismissing(facet_row_wrap) && Nrows > facet_row_wrap
nr, nc = facet_row_wrap, ceil(Int, Nrows / facet_row_wrap)
subplot_kw[:rows] = nr
subplot_kw[:cols] = nc
subplot_kw[:subplot_titles] = _faceted_subplot_titles(
nr, nc, map(x -> "$(label_map[facet_row])=$(x)", rows)
)
subplot_kw[:shared_xaxes] = "all"
else
subplot_kw[:rows] = Nrows
subplot_kw[:row_titles] = map(x -> "$(label_map[facet_row])=$(x)", rows)
subplot_kw[:shared_xaxes] = true
end
push!(facet_cols, facet_row)
end
subplots = Subplots(; subplot_kw...)
out_layout = Layout(subplots; out_layout.fields...)
end
out = Plot(out_layout)
legend_order = Vector{<:Any}[]
for dfg in collect(DataFrames.groupby(df, facet_cols))
row_ix = Nrows == 1 ? 1 : findfirst(isequal(dfg[1, facet_row]), rows)
col_ix = Ncols == 1 ? 1 : findfirst(isequal(dfg[1, facet_col]), cols)
# @show row_ix, col_ix, :before, dfg[1, facet_col]
if !ismissing(facet_col_wrap) || !ismissing(facet_row_wrap)
## ind2sub
# when making facets with wrap, we need to convert from the
# non-wrapped row_ix (=1 when facet_col_wrap) and col_ix (potentially
# greater than facet_col_wrap) to the wrapped row_ix and col_ix
# we do this by converting from a linear index in the faceted + wrapped
# dimenmsion only into a cartesian index in both dimensions
_facet_ix = ismissing(facet_col_wrap) ? row_ix : col_ix
row_ix, col_ix = _ind2sub_rowmajor(size(out.layout.subplots.grid_ref)..., _facet_ix).I
end
for sub_dfg in collect(DataFrames.groupby(dfg, groupby_cols))
all_kw = attr(;kwargs...)
group_obs = Any[sub_dfg[1, g] for g in groupby_cols]
group_name = join(string.(group_obs), ",")
if length(group_name) > 0
all_kw.name = group_name
all_kw.legendgroup = group_name
all_kw.showlegend = false
end
for (groupname, trace_attr) in group_attr_pairs
if !ismissing(getfield(_group_args, groupname))
obs = sub_dfg[1, _group_cols[groupname]]
all_kw[trace_attr] = _group_maps[groupname][obs]
end
end
traces = GenericTrace(sub_dfg; group=group, all_kw...,)
traces_vec = traces isa GenericTrace ? [traces] : traces
for trace in traces_vec
push!(legend_order, group_obs)
add_trace!(out, trace, row=row_ix, col=col_ix)
end
end
end
# turn on legend once for each
if length(groupby_cols) > 0
group_labels = getindex.(Ref(label_map), groupby_cols)
out.layout[:legend_title_text] = join(string.(group_labels), ", ")
seen = Set()
for trace in out.data
grp = trace.legendgroup
if !(grp in seen)
push!(seen, grp)
trace.showlegend = true
end
end
end
out.layout.legend_tracegroupgap = 0
# fix order of traces according to category_orders
trace_order = _partial_val_sortperm(legend_order, orders, groupby_cols)
out.data = out.data[trace_order]
# add x axis title to bottom row of subplots and y axis title to left column
for gr in out.layout.subplots.grid_ref[:, 1]
yname = gr[1].layout_keys[2]
if :y in keys(label_map)
setifempty!(out.layout, Symbol("$(yname)_title_text"), label_map[:y])
if haskey(orders, kwargs[:y])
# order the yticks
setifempty!(out.layout, Symbol("$(yname)_categoryorder"), "array")
setifempty!(out.layout, Symbol("$(yname)_categoryarray"), orders[kwargs[:y]])
end
end
end
for gr in out.layout.subplots.grid_ref[end, :]
xname = gr[1].layout_keys[1]
if :x in keys(label_map)
setifempty!(out.layout, Symbol("$(xname)_title_text"), label_map[:x])
if haskey(orders, kwargs[:x])
# order the xticks
setifempty!(out.layout, Symbol("$(xname)_categoryorder"), "array")
setifempty!(out.layout, Symbol("$(xname)_categoryarray"), orders[kwargs[:x]])
end
end
end
if Symbol(get(kwargs, :kind, "")) == :splom
out.layout.dragmode = "select"
end
# check dimensions for label maps
for trace in out.data
if !_isempty(trace.dimensions)
for dim in trace.dimensions
dim.label = get(label_map, dim.label, dim.label)
end
end
end
return out
end
"""
$(SIGNATURES)
Construct a plot from `df`, passing the provided values of x and y as keyword
arguments. See docstring for other method for more information.
"""
function Plot(d::DataFrames.AbstractDataFrame, x::Symbol, y::Symbol, l::Layout=Layout();
kwargs...)
Plot(d, l; x=x, y=y, kwargs...)
end
"""
$(SIGNATURES)
Construct a plot from `df`, passing the provided value y as a keyword argument.
See docstring for other method for more information.
"""
function Plot(d::DataFrames.AbstractDataFrame, y::Symbol, l::Layout=Layout();
kwargs...)
Plot(d, l; y=y, kwargs...)
end
for t in _TRACE_TYPES
str_t = string(t)
@eval $t(df::DataFrames.AbstractDataFrame; kwargs...) = GenericTrace(df; kind=$(str_t), kwargs...)
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 524 | _strip_module(s) = split(s, '.', limit=2)[end]
_strip_type_param(s) = replace(s, r"{.+?}" => "")
_clean_name(d::Distributions.Distribution) = _strip_module(_strip_type_param(repr(d)))
function scatter(d::Distributions.ContinuousUnivariateDistribution)
ls(a, b, c) = range(a, stop=b, length=c)
x = ls(Distributions.quantile.([d], [0.01, 0.99])..., 100)
trace = scatter(x=x, y=Distributions.pdf.([d], x), name=_clean_name(d))
end
Plot(d::Distributions.UnivariateDistribution...) = Plot(collect(map(scatter, d)))
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 2691 | # -------------------------------- #
# Custom JSON output for our types #
# -------------------------------- #
JSON.lower(a::HasFields) = a.fields
JSON.lower(c::Cycler) = c.vals
_json_lower(x) = JSON.lower(x)
_json_lower(x::Union{Bool,String,Number,Nothing,Missing}) = x
_json_lower(x::Union{Tuple,AbstractArray}) = _json_lower.(x)
_json_lower(d::Dict) = Dict{Any,Any}(k => _json_lower(v) for (k, v) in pairs(d))
_json_lower(a::HasFields) = Dict{Any,Any}(k => _json_lower(v) for (k, v) in pairs(a.fields))
_json_lower(c::Cycler) = c.vals
function _json_lower(c::ColorScheme)::Vector{Tuple{Float64,String}}
N = length(c.colors)
map(ic -> ((ic[1] - 1) / (N - 1), _json_lower(ic[2])), enumerate(c.colors))
end
_maybe_set_attr!(hf::HasFields, k::Symbol, v::Any) =
get(hf, k, nothing) == nothing && setindex!(hf, v, k)
# special case for associative to get nested application
function _maybe_set_attr!(hf::HasFields, k1::Symbol, v::AbstractDict)
for (k2, v2) in v
_maybe_set_attr!(hf, Symbol(k1, "_", k2), v2)
end
end
function _maybe_set_attr!(p::Plot, k1::Symbol, v::AbstractDict)
for (k2, v2) in v
_maybe_set_attr!(p, Symbol(k1, "_", k2), v2)
end
end
function _maybe_set_attr!(p::Plot, k::Symbol, v)
foreach(t -> _maybe_set_attr!(t, k, v), p.data)
end
function _maybe_set_attr!(p::Plot, k::Symbol, v::Cycler)
ix = 0
for t in p.data
if t[k] == Dict() # was empty
t[k] = v[ix += 1]
end
end
end
function JSON.lower(p::Plot)
out = Dict(
:data => _json_lower(p.data),
:layout => _json_lower(p.layout),
:frames => _json_lower(p.frames),
:config => _json_lower(p.config)
)
if templates.default !== "none" && _isempty(get(out[:layout], :template, Dict()))
out[:layout][:template] = _json_lower(templates[templates.default])
end
out
end
# Let string interpolation stringify to JSON format
Base.print(io::IO, a::Union{Shape,GenericTrace,PlotlyAttribute,Layout,Plot,PlotConfig}) = print(io, JSON.json(a))
Base.print(io::IO, a::Vector{T}) where {T <: GenericTrace} = print(io, JSON.json(a))
GenericTrace(d::AbstractDict{Symbol}) = GenericTrace(pop!(d, :type, "scatter"), d)
GenericTrace(d::AbstractDict{T}) where {T <: AbstractString} = GenericTrace(_symbol_dict(d))
Layout(d::AbstractDict{T}) where {T <: AbstractString} = Layout(_symbol_dict(d))
function JSON.parse(::Type{Plot}, str::AbstractString)
d = JSON.parse(str)
data = GenericTrace[GenericTrace(tr) for tr in d["data"]]
layout = Layout(d["layout"])
Plot(data, layout)
end
JSON.parsefile(::Type{Plot}, fn) =
open(fn, "r") do f; JSON.parse(Plot, String(read(f))) end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 761 | const StructTypes = JSON3.StructTypes
# StructTypes.StructType(::Type{T}) where T <: HasFields = StructTypes.DictType()
# StructTypes.construct(::Type{T}, x::AbstractDict; kw...) where T <: HasFields = T(_symbol_dict(x); kw...)
# StructTypes.StructType(::Type{T}) where T <: Union{Template} = StructTypes.Struct()
# StructTypes.StructType(::Type{T}) where T <: Union{Plot,PlotConfig} = StructTypes.Mutable()
# StructTypes.omitempties(::Type{PlotConfig}) = fieldnames(PlotConfig)
StructTypes.StructType(::Type{<:PlotlyBase.Plot}) = JSON3.RawType()
StructTypes.StructType(::Type{<:HasFields}) = JSON3.RawType()
StructTypes.StructType(::Type{PlotConfig}) = JSON3.RawType()
JSON3.rawbytes(x::Union{PlotlyBase.Plot,HasFields,PlotConfig}) = codeunits(JSON.json(x)) | PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 9522 | # ----------------------- #
# Display-esque functions #
# ----------------------- #
const _WINDOW_PLOTLY_CONFIG = """
<script type="text/javascript">
window.PlotlyConfig = {MathJaxConfig: 'local'};
</script>"""
const _MATHJAX_CONFIG = """
<script type="text/javascript">
if (window.MathJax) {MathJax.Hub.Config({SVG: {font: "STIX-Web"}});}
</script>"""
const PLOTLYJS_VERSION = "2.3.0"
const CDN_URL = "https://cdn.plot.ly/plotly-$(PLOTLYJS_VERSION).min.js"
function _requirejs_config()
"""
$(_WINDOW_PLOTLY_CONFIG)
$(_MATHJAX_CONFIG)
<script type="text/javascript">
if (typeof require !== 'undefined') {
require.undef("plotly");
requirejs.config({
paths: {
'plotly': ['$(rsplit(CDN_URL, '.', limit=2)[1])']
}
});
require(['plotly'], function(Plotly) {
window._Plotly = Plotly;
});
}
</script>
"""
end
"""
to_html(
io::IO,
p::Plot;
autoplay::Bool=true,
include_plotlyjs::Union{String,Missing}="cdn",
include_mathjax::Union{String,Missing}="cdn",
post_script::Union{String,Missing}=missing,
full_html::Bool=true,
animation_opts::Union{Dict,Missing}=missing,
default_width::String="100%",
default_height::String="100%"
)
- io: IO stream to write to
- p: Plot to save
- autoplay: Should animations start automatically
- include_plotlyjs: How to include plotly.js. Options are
- cdn: include a <script> tag to load plotly.js from cdn. Output will be standalone
- require: load using requirejs. Useful in Jupyter notebooks
- require-loaded: assume a `plotly` statement has already loaded
via requirejs (don't load it in context of this plot)
- directory: hardcode `<script src="plotly.min.js>` -- will
only work when the plotly.min.js file is in the same directory
as the output file
- anything ending in js: we assume you give us the path to the
plotly.js file. We will read it in and include it inline in the
output. Works best when points to a minified file (plotly.min.js)
- include_mathjax: How mathjax should be included. Options are
- string ending in .js: we load via
`<script src="\$(include_mathjax)">`. You are responsible for
making sure it resolves
- anything else: we load via cdn for you
- post_script: arbitrary javascript to run after plotly.js
finishes drawing the plot
- full_html: include all parts necessary for standalone html file
- animation_opts: extra options used to control animation. included
in `addFrames` call after the actual frames. See plotly.js docs for
more info on `addFrames`
- default_width: valid css specifier for width
- default_height: valid css specifier for height
"""
function to_html(
io::IO,
p::Plot;
autoplay::Bool=true,
include_plotlyjs::Union{String,Missing}="cdn",
include_mathjax::Union{String,Missing}="cdn",
post_script::Union{String,Missing}=missing,
full_html::Bool=true,
animation_opts::Union{Dict,Missing}=missing,
default_width::String="100%",
default_height::String="100%"
)
# get lowered form
js = JSON.lower(p)
jdata = js[:data]
jlayout = js[:layout]
jframes = js[:frames]
jconfig = js[:config]
get!(jconfig, :responsive, true)
# extract width and height from layout
div_width = get(p.layout, :width, default_width)
div_height = get(p.layout, :height, default_height)
# get platform url
base_url_line = ""
if p.config.showLink === true || p.config.showEditInChartStudio === true
url = ismissing(p.config.plotlyServerURL) ? "https://plot.ly" : p.config.plotlyServerURL
base_url_line = "window.PLOTLYENV.BASE_URL = '$url';\n"
else
pop!(jconfig, :plotlyServerURL, missing)
pop!(jconfig, :linkText, missing)
pop!(jconfig, :showLink, missing)
end
# build script body
then_post_script = ""
if !ismissing(post_script)
then_post_script = ".then(function() {$(replace(post_script, plot_id => p.divid))})"
end
then_addframes = ""
then_animate = ""
if !isempty(jframes)
then_addframes = """.then(function() {
Plotly.addFrames('$(p.divid)', $(JSON.json(jframes)));
})"""
if autoplay
animation_opts_arg = ""
if !ismissing(animation_opts)
animation_opts_arg = ", $(JSON.json(animation_opts))"
end
then_animate = """.then(function() {
Plotly.animate('$(p.divid)', null$(animation_opts_arg));
})"""
end
end # jframes
call_plotlyjs_script = """
if (document.getElementById('$(p.divid)')) {
Plotly.newPlot(
'$(p.divid)',
$(JSON.json(jdata)),
$(JSON.json(jlayout)),
$(JSON.json(jconfig)),
)$(then_addframes)$(then_animate)$(then_post_script)
}
"""
# handle loading/initializing plotly.js
# Start/end of requirejs block (if any)
require_start = ""
require_end = ""
load_plotlyjs = "" # Init and load
if !ismissing(include_plotlyjs)
including = lowercase(include_plotlyjs)
if including == "require"
load_plotlyjs = _requirejs_config()
require_start = "require([\"plotly\"], function(Plotly) {"
require_end = "});"
elseif including == "require-loaded"
require_start = "require([\"plotly\"], function(Plotly) {"
require_end = "});"
elseif including == "directory"
load_plotlyjs = """
$(_WINDOW_PLOTLY_CONFIG)
<script src="plotly.min.js"></script>
"""
elseif endswith(including, ".js")
# check if this is a file
if embed_plotlyjs
if !isfile(including)
error("cannot embed $(including) file not found")
end
# if so, read it and insert inline
load_plotlyjs = """
<script type="text/javascript">
$(read(including, String))
</script>
"""
else
# assume it is a url that can be found
load_plotlyjs = """
$(_WINDOW_PLOTLY_CONFIG)
<script src="$(including)"></script>
"""
end
else # assume cdn
load_plotlyjs = """
$(_WINDOW_PLOTLY_CONFIG)
<script src="$(CDN_URL)"></script>
"""
end
end
# handle mathjax
load_mathjax = ""
if isa(include_mathjax, String)
inmath = lowercase(include_mathjax)
math_url = ""
if endswith(inmath, ".js")
math_url = inmath
else
# assume cdn
math_url = "https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.5/MathJax.js"
end
load_mathjax = "<script src=\"$(math_url)?config=TeX-AMS-MML_SVG\"></script>"
end
html_div = """
<div>
$(load_mathjax)
$(load_plotlyjs)
<div
id=$(p.divid)
class="plotly-graph-div"
style="height:$(div_height); width:$(div_width);">
</div>
<script type="text/javascript">
$(require_start)
window.PLOTLYENV = window.PLOTLYENV || {}
$(base_url_line)
$(call_plotlyjs_script)
$(require_end)
</script>
</div>
"""
if full_html
return print(io, """<html>
<head><meta charset="utf-8" /></head>
<body>
$(html_div)
</body>
</html>""")
end
return print(io, html_div)
end # function
function savejson(p::Plot, fn::AbstractString)
ext = split(fn, ".")[end]
if ext == "json"
open(f -> print(f, json(p)), fn, "w")
return p
else
msg = "PlotlyBase can only save figures as JSON. For all other"
msg *= " file types, please use PlotlyJS.jl"
throw(ArgumentError(msg))
end
end
# jupyterlab/nteract integration
Base.Multimedia.istextmime(::MIME"application/vnd.plotly.v1+json") = true
function Base.show(io::IO, ::MIME"application/vnd.plotly.v1+json", p::Plot)
JSON.print(io, p)
end
function Base.show(io::IO, ::MIME"text/plain", p::Plot)
println(io, """
data: $(json(map(_describe, p.data), 2))
layout: "$(_describe(p.layout))"
""")
end
Base.show(io::IO, ::MIME"text/html", p::Plot; kwargs...) = to_html(io, p; kwargs...)
# integration with vscode and Juno
function Base.show(io::IO, ::MIME"application/prs.juno.plotpane+html", p::Plot)
show(io, MIME("text/html"), p; include_mathjax="cdn", include_plotlyjs="cdn")
end
Base.show(io::IO, p::Plot) = show(io, MIME("text/plain"), p)
import REPL
"""
opens a browser tab with the given html file
"""
function launch_browser(tmppath::String)
if Sys.isapple()
run(`open $tmppath`)
elseif Sys.iswindows()
run(`cmd /c start $tmppath`)
elseif Sys.islinux()
run(`xdg-open $tmppath`)
end
end
function Base.display(::REPL.REPLDisplay, p::Plot)
tmppath = string(tempname(), ".plotlyjs-jl.html")
open(tmppath, "w") do io
show(io, MIME("text/html"), p; include_mathjax="cdn", include_plotlyjs="cdn")
end
launch_browser(tmppath) # Open the browser
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 4512 | """
PlotConfig(;kwargs...)
Configuration options to be sent to the frontend to control aspects of how the plot is rendered. The acceptable keyword arguments are:
- `scrollZoom`: Determines whether mouse wheel or two-finger scroll zooms is enable. Turned on by default for gl3d, geo and mapbox subplots (as these subplot types do not have zoombox via pan), but turned off by default for cartesian subplots. Set `scrollZoom` to *false* to disable scrolling for all subplots.
- `editable`: Determines whether the graph is editable or not. Sets all pieces of `edits` unless a separate `edits` config item overrides individual parts.
- `staticPlot`: Determines whether the graphs are interactive or not. If *false*, no interactivity, for export or image generation.
- `toImageButtonOptions`: Statically override options for toImage modebar button allowed keys are format, filename, width, height
- `displayModeBar`: Determines the mode bar display mode. If *true*, the mode bar is always visible. If *false*, the mode bar is always hidden. If *hover*, the mode bar is visible while the mouse cursor is on the graph container.
- `modeBarButtonsToRemove`: Remove mode bar buttons by name
- `modeBarButtonsToAdd`: Add mode bar button using config objects. To enable predefined modebar buttons e.g. shape drawing, hover and spikelines, simply provide their string name(s). This could include: *v1hovermode*, *hoverclosest*, *hovercompare*, *togglehover*, *togglespikelines*, *drawline*, *drawopenpath*, *drawclosedpath*, *drawcircle*, *drawrect* and *eraseshape*. Please note that these predefined buttons will only be shown if they are compatible with all trace types used in a graph.
- `modeBarButtons`: Define fully custom mode bar buttons as nested array where the outer arrays represents button groups, and the inner arrays have buttons config objects or names of default buttons.
- `showLink`: Determines whether a link to Chart Studio Cloud is displayed at the bottom right corner of resulting graphs. Use with `sendData` and `linkText`.
- `plotlyServerURL`: When set it determines base URL for the 'Edit in Chart Studio' `showEditInChartStudio`/`showSendToCloud` mode bar button and the showLink/sendData on-graph link. To enable sending your data to Chart Studio Cloud, you need to set both `plotlyServerURL` to 'https://chart-studio.plotly.com' and also set `showSendToCloud` to true.
- `linkText`: Sets the text appearing in the `showLink` link.
- `showEditInChartStudio`: Same as `showSendToCloud`, but use a pencil icon instead of a floppy-disk. Note that if both `showSendToCloud` and `showEditInChartStudio` are turned, only `showEditInChartStudio` will be honored.
- `locale`: Which localization should we use? Should be a string like 'en' or 'en-US'.
- `displaylogo`: Determines whether or not the plotly logo is displayed on the end of the mode bar.
- `responsive`: Determines whether to change the layout size when window is resized.
- `doubleClickDelay`: Sets the delay for registering a double-click in ms. This is the time interval (in ms) between first mousedown and 2nd mouseup to constitute a double-click. This setting propagates to all on-subplot double clicks (except for geo and mapbox) and on-legend double clicks.
"""
@with_kw mutable struct PlotConfig
scrollZoom::Union{Nothing,Bool} = true
editable::Union{Nothing,Bool} = false
staticPlot::Union{Nothing,Bool} = false
toImageButtonOptions::Union{Nothing,Dict} = nothing
displayModeBar::Union{Nothing,Bool} = nothing
modeBarButtonsToRemove::Union{Nothing,Array} = nothing
modeBarButtonsToAdd::Union{Nothing,Array} = nothing
modeBarButtons::Union{Nothing,Array} = nothing
showLink::Union{Nothing,Bool} = false
plotlyServerURL::Union{Nothing,String} = nothing
linkText::Union{Nothing,String} = nothing
showEditInChartStudio::Union{Nothing,Bool} = nothing
locale::Union{Nothing,String} = nothing
displaylogo::Union{Nothing,Bool} = nothing
responsive::Union{Nothing,Bool} = true
doubleClickDelay::Union{Nothing,Int} = nothing
end
function JSON.lower(pc::PlotConfig)
out = Dict{Symbol,Any}()
for fn in fieldnames(PlotConfig)
field = getfield(pc, fn)
if !isnothing(field)
out[fn] = field
end
end
out
end
function Base.get(pc::PlotConfig, field::Symbol, default::Any)
if hasfield(pc, field)
val = getfield(pc, field)
if !isnothing(val)
return val
end
end
return default
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 6078 | # start with empty, it is easier to enumerate the true cases
to_date(x) = nothing
function to_date(x::AbstractString)
# maybe it is yyyy
if all(isdigit, x)
return to_date(parse(Int, x))
end
# maybe it is yyyy-mm or yyyy-mm-dd
parts = split(x, '-')
nparts = length(parts)
if nparts == 3
try
return Date(x, "y-m-d")
catch
return nothing
end
elseif nparts == 2
try
return Date(x, "y-m")
catch
return nothing
end
end
# don't know how to handle anything else.
return nothing
end
to_date(x::Union{Integer,Dates.TimeType}) = Date(x)
function to_date(x::AbstractArray{T,N}) where {T,N}
out_arr = Date[]
for i in x
maybe_date = to_date(i)
if maybe_date === nothing
return nothing
else
push!(out_arr, maybe_date)
end
end
reshape(out_arr, size(x))
end
to_date(x::AbstractArray{T,N}) where {T<:Dates.TimeType,N} = Date.(x)
#=
I populated recession_dates.tsv with the following (ugly!!) code
```
rec = get_data(f, "USREC", observation_start="1776-07-04").df[[:date, :value]]
did_start = fill(false, size(rec, 1))
did_end = copy(did_start)
did_start[1] = rec[1, :value] == 1.0
continuing_recession = did_start[1]
for i in 2:length(did_start)
if rec[i, :value] == 1.0 && !continuing_recession
did_start[i] = true
continuing_recession = true
end
if rec[i, :value] == 0.0 && continuing_recession
did_end[i] = true
continuing_recession = false
end
end
start_dates = rec[did_start, :date]
end_dates = rec[did_end, :date]
out_path = Pkg.dir("PlotlyBase", "recession_dates.tsv")
writedlm(out_path, [start_dates end_dates])
```
=#
function _recession_band_shapes(p::Plot; kwargs...)
# data is 2 column matrix, first column is start date, second column
# is end_date
recession_data = map(Date, readdlm(
joinpath(dirname(dirname(@__FILE__)), "recession_dates.tsv")
))::Matrix{Date}
# keep track of which traces have dates for x
n_trace = length(p.data)
x_has_dates = fill(false, n_trace)
# need to over the x attribute of each trace and get the most extreme dates
# for each trace
min_date = fill(Date(500_000), n_trace)
max_date = fill(Date(-500_000), n_trace)
dates_changed = fill(false, n_trace)
for (i, t) in enumerate(p.data)
t_x = t[:x]
if !isempty(t_x)
dates = to_date(t_x)
dates === nothing && continue
if typeof(dates) <: AbstractArray
min_date[i] = min(min_date[i], minimum(dates))
max_date[i] = max(max_date[i], maximum(dates))
dates_changed[i] = true
x_has_dates[i] = true
end
end
end
if !any(dates_changed)
return nothing
end
# map traces into xaxis and yaxis
xmap = trace_map(p, :x)
ymap = trace_map(p, :y)
# WANT: 1 set of shapes for each xaxis with dates
n_xaxis = length(unique(xmap))
band_kwargs = attr(
fillcolor="#E6E6E6", opacity=0.4, line_width=0, layer="below",
yref="paper", kwargs...
)
# if we only have one xaxis, only add one set of shapes
if n_xaxis == 1
ix1 = minimum(searchsortedfirst(recession_data[:, 1], m) for m in min_date)
ix2 = maximum(searchsortedlast(recession_data[:, 2], m) for m in max_date)
if isempty(ix1:ix2)
return nothing
end
out = rect(
recession_data[ix1:ix2, 1],
recession_data[ix1:ix2, 2],
0,
1,
band_kwargs.fields;
xref="x"
)
return out
end
# otherwise we need to loop over the traces and add one set of shapes
# for each x axis
out = Vector{Shape}()
# first determine the min_date and max_date for each axis, based on that
# info for each trace and the xmap.
min_ax_date = fill(Date(500_000), n_xaxis)
max_ax_date = fill(Date(-500_000), n_xaxis)
for i in 1:n_trace
ix = xmap[i]
min_ax_date[ix] = min(min_ax_date[ix], min_date[ix])
max_ax_date[ix] = max(max_ax_date[ix], max_date[ix])
end
# now loop through the traces and add one set of shapes per axis that
# appears. We loop over traces so that we can be sure to get the correct
# yaxis domain for each trace
already_added = fill(false, n_xaxis)
for i in 1:n_trace
if x_has_dates[i]
# move on if we have bands on this xax
ix = xmap[i]
already_added[ix] && continue
# get starting and ending date
ix1 = searchsortedfirst(recession_data[:, 1], min_ax_date[ix])
ix2 = searchsortedlast(recession_data[:, 2], max_ax_date[ix])
isempty(ix1:ix2) && continue # range was bogus, move on
# otherwise extract the yaxis details
yax = p.layout[Symbol("yaxis", ymap[i])]
isempty(yax) && continue # don't have this axis somehow... move on
# don't have info on where this axis lives ... move on
!haskey(yax, :domain) && continue
ybounds = yax[:domain]
# we are in business, build the shapes and append them
append!(out,
rect(recession_data[ix1:ix2, 1],
recession_data[ix1:ix2, 2],
ybounds[1],
ybounds[2],
band_kwargs.fields;
xref=string("x", xmap[i]))
)
already_added[xmap[i]] = true
end
end
out
end
function add_recession_bands!(p::Plot; kwargs...)
bands = _recession_band_shapes(p; kwargs...)
if bands === nothing
return
end
# now we have some bands that we need to apply to the layout
old_shapes = p.layout[:shapes]
new_shapes = isempty(old_shapes) ? bands : vcat(old_shapes, bands)
relayout!(p, shapes=new_shapes)
new_shapes
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 9401 | # implementation of algorithms from plotly.py/packages/python/plotly/plotly/subplots.py
# Constants
# ---------
# Subplot types that are each individually positioned with a domain
#
# Each of these subplot types has a `domain` property with `x`/`y`
# properties.
# Note that this set does not contain `xaxis`/`yaxis` because these behave a
# little differently.
const _single_subplot_types = Set(["scene", "geo", "polar", "ternary", "mapbox"])
const _subplot_types = union(_single_subplot_types, Set(["xy", "domain"]))
# For most subplot types, a trace is associated with a particular subplot
# using a trace property with a name that matches the subplot type. For
# example, a `scatter3d.scene` property set to `'scene2'` associates a
# scatter3d trace with the second `scene` subplot in the figure.
#
# There are a few subplot types that don't follow this pattern, and instead
# the trace property is just named `subplot`. For example setting
# the `scatterpolar.subplot` property to `polar3` associates the scatterpolar
# trace with the third polar subplot in the figure
const _subplot_prop_named_subplot = Set(["polar", "ternary", "mapbox"])
@with_kw struct SubplotRef
subplot_kind::String
layout_keys::Vector{Symbol}
trace_kwargs::PlotlyAttribute
end
const GridRef = Matrix{Vector{SubplotRef}}
function _get_initial_max_subplot_ids()
max_subplot_ids = Dict(subplot_kind => 0 for subplot_kind in _single_subplot_types)
max_subplot_ids["xaxis"] = 0
max_subplot_ids["yaxis"] = 0
return max_subplot_ids
end
"""
**Parameters**
- `kind` Subplot type. One of
- 'xy': 2D Cartesian subplot type for scatter, bar, etc.
- 'scene': 3D Cartesian subplot for scatter3d, cone, etc.
- 'polar': Polar subplot for scatterpolar, barpolar, etc.
- 'ternary': Ternary subplot for scatterternary
- 'mapbox': Mapbox subplot for scattermapbox
- 'domain': Subplot type for traces that are individually positioned. pie, parcoords, parcats, etc.
- Trace type: Put the name of the type of trace here and we will determine the appropriate kind of subplot
- `secondary_y`: If true, create a secondary y-axis positioned on the right side of the subplot. Only valid if kind="xy".
- `colspan`: number of subplot columns for this subplot to span.
- `rowspan`: number of subplot rows for this subplot to span.
- `l`: padding left of cell
- `r`: padding right of cell
- `t`: padding right of cell
- `b`: padding bottom of cell
"""
@with_kw struct Spec
kind::String = "xy"
secondary_y::Bool = false
colspan::Int = 1
rowspan::Int = 1
l::Float64 = 0.0
r::Float64 = 0.0
b::Float64 = 0.0
t::Float64 = 0.0
@assert !secondary_y || (kind == "xy")
end
"""
**Parameters**
- `cell`: index of the subplot cell to overlay inset axes onto.
- `kind`: Subplot kind (see `Spec` docs)
- `l`: padding left of inset in fraction of cell width
- `w`: inset width in fraction of cell width ('to_end': to cell right edge)
- `b`: padding bottom of inset in fraction of cell height
- `h`: inset height in fraction of cell height ('to_end': to cell top edge)
"""
@with_kw struct Inset
cell::Tuple{Int,Int} = (1, 1)
kind::String = "xy"
colspan::Int = 1
rowspan::Int = 1
l::Float64 = 0.0
w::Union{String,Float64} = "to_end"
b::Float64 = 0.0
h::Union{String,Float64} = "to_end"
end
function _check_hv_spacing(dimsize::Int, spacing::Float64, name::String, dimvarname::String)
spacing < 0 || spacing > 1 && error("$name spacing must be between 0 and 1")
if dimsize <= 1
return true
end
max_spacing = 1.0 / (dimsize - 1)
if spacing > max_spacing
error("$name spacing cannot be greater than 1/($dimvarname - 1) = $max_spacing")
end
return true
end
"""
**Parameters**
- `rows`: Number of rows in the subplot grid. Must be greater than zero.
- `cols`: Number of columns in the subplot grid. Must be greater than zero.
- `shared_xaxes`: Assign shared (linked) x-axes for 2D cartesian subplots
- true or "columns": Share axes among subplots in the same column
- "rows": Share axes among subplots in the same row
- "all": Share axes across all subplots in the grid
- `shared_yaxes`: Assign shared (linked) y-axes for 2D cartesian subplots
- "columns": Share axes among subplots in the same column
- true or "rows": Share axes among subplots in the same row
- "all": Share axes across all subplots in the grid.
- `start_cell`: Choose the starting cell in the subplot grid used to set the domains_grid of the subplots.
- "top-left": Subplots are numbered with (1, 1) in the top left corner
- "bottom-left": Subplots are numbererd with (1, 1) in the bottom left corner
- `horizontal_spacing`: Space between subplot columns in normalized plot coordinates. Must be a float between 0 and 1. Applies to all columns (use "specs" subplot-dependents spacing)
- `vertical_spacing`: Space between subplot rows in normalized plot coordinates. Must be a float between 0 and 1. Applies to all rows (use "specs" subplot-dependents spacing)
- `subplot_titles`: Title of each subplot as a list in row-major ordering. Empty strings ("") can be included in the list if no subplot title is desired in that space so that the titles are properly indexed.
- `specs`: Per subplot specifications of subplot type, row/column spanning, and spacing.
- The number of rows in "specs" must be equal to "rows".
- The number of columns in "specs"
- Each item in the "specs" list corresponds to one subplot
in a subplot grid. (N.B. The subplot grid has exactly "rows"
times "cols" cells.)
- Use missing for a blank a subplot cell (or to move past a col/row span).
- Each item in "specs" is an instance of `Spec`. See docs for `Spec` for more information
- Note: Use `horizontal_spacing` and `vertical_spacing` to adjust the spacing in between the subplots.
- `insets`: Inset specifications. Insets are subplots that overlay grid subplots
- Each item in "insets" is an instance of `Inset`. See docs for `Inset` for more info
- `column_widths`: Array of length `cols` of the relative widths of each column of suplots. Values are normalized internally and used to distribute overall width of the figure (excluding padding) among the columns.
- `row_heights`: Array of length `rows` of the relative heights of each row of subplots. Values are normalized internally and used to distribute overall height of the figure (excluding padding) among the rows
- `column_titles`: list of length `cols` of titles to place above the top subplot in each column.
- `row_titles`: list of length `rows` of titles to place on the right side of each row of subplots.
- `x_title`: Title to place below the bottom row of subplots, centered horizontally
- `y_title`: Title to place to the left of the left column of subplots, centered vertically
"""
@with_kw struct Subplots
rows::Int = 1
cols::Int = 1
shared_xaxes::_Maybe{Union{String,Bool}} = false
shared_yaxes::_Maybe{Union{String,Bool}} = false
start_cell::String = "top-left"
subplot_titles::_Maybe{Matrix{<:_Maybe{String}}} = missing
column_widths::_Maybe{Vector{Float64}} = missing
row_heights::_Maybe{Vector{Float64}} = missing
specs::Matrix{<:_Maybe{Spec}} = [Spec() for _ in 1:rows, __ in 1:cols]
insets::_Maybe{Union{Bool,Vector{Inset}}} = missing
column_titles::_Maybe{Vector{String}} = missing
row_titles::_Maybe{Vector{String}} = missing
x_title::_Maybe{String} = missing
y_title::_Maybe{String} = missing
grid_ref::GridRef = let
gr = GridRef(undef, (rows, cols))
if rows == 1 && cols == 1
gr[1, 1] = [SubplotRef(subplot_kind="xy", layout_keys=[:xaxis, :yaxis], trace_kwargs=attr(xaxis="x", yaxis="y"))]
end
gr
end
# computed
has_secondary_y::Bool = any(!ismissing(s) && getfield(s, :secondary_y) for s in specs)
horizontal_spacing::Float64 = has_secondary_y ? 0.4 / cols : 0.2 / cols
vertical_spacing::Float64 = ismissing(subplot_titles) ? 0.3 / rows : 0.5 / rows
max_width::Float64 = has_secondary_y ? 0.94 : (ismissing(row_titles) ? 1.0 : 0.98)
_widths::Vector{Float64} = let
if ismissing(column_widths)
fill((max_width - horizontal_spacing * (cols - 1)) / cols, cols)
else
w_sum = sum(column_widths)
[(max_width - horizontal_spacing * (cols - 1)) * (w / w_sum) for w in column_widths]
end
end
_heights::Vector{Float64} = let
if ismissing(row_heights)
fill((1.0 - vertical_spacing * (rows - 1)) / rows, rows)
else
h_sum = sum(row_heights)
[(1.0 - vertical_spacing * (rows - 1)) * (h / h_sum) for h in row_heights]
end
end
@assert start_cell in ["top-left", "bottom-left"]
@assert ismissing(shared_xaxes) || (shared_xaxes in [true, false, "rows", "columns", "all"])
@assert ismissing(shared_yaxes) || (shared_yaxes in [true, false, "rows", "columns", "all"])
@assert _check_hv_spacing(cols, horizontal_spacing, "Horizontal", "cols")
@assert _check_hv_spacing(rows, vertical_spacing, "Vertical", "rows")
@assert length(_widths) == cols
@assert length(_heights) == rows
@assert ismissing(column_titles) || (length(column_titles) == cols)
@assert ismissing(row_titles) || (length(row_titles) == rows)
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 18113 | """
Given the number of rows and columns, return an NTuple{4,Float64} containing
`(width, height, vspace, hspace)`, where `width` and `height` are the
width and height of each subplot and `vspace` and `hspace` are the vertical
and horizonal spacing between subplots, respectively.
"""
function sizes(nr::Int, nc::Int, subplot_titles::Bool=false)
# NOTE: the logic of this function was mostly borrowed from plotly.py
dx = 0.2 / nc
dy = subplot_titles ? 0.55 / nr : 0.3 / nr
width = (1. - dx * (nc - 1)) / nc
height = (1. - dy * (nr - 1)) / nr
vspace = nr == 1 ? 0.0 : (1 - height * nr) / (nr - 1)
hspace = nc == 1 ? 0.0 : (1 - width * nc) / (nc - 1)
width, height, vspace, hspace
end
function gen_layout(rows::Tuple{Vararg{Int}}, subplot_titles::Bool=false)
x = 0.0 # start from left
y = 1.0 # start from top
out = Layout()
subplot = 1
nr = length(rows)
for nc in rows
w, h, dy, dx = sizes(nr, nc, subplot_titles)
x = 0.0 # reset x as we start a new row
for col in 1:nc
out["xaxis$subplot"] = attr(domain=[x, x + w], anchor="y$subplot")
out["yaxis$subplot"] = attr(domain=[y - h, y], anchor="x$subplot")
x += nc == 1 ? 0.0 : w + dx
subplot += 1
end
y -= nr == 1 ? 0.0 : h + dy
end
out
end
gen_layout(nr::Int, nc::Int, subplot_titles::Bool=false) = gen_layout(tuple(fill(nc, nr)), subplot_titles)
function handle_titles!(big_layout, sub_layout, ix::Int)
# don't worry about it if the sub_layout doesn't have a title
if _isempty(sub_layout.title)
return big_layout
end
# check for title or title text
text = sub_layout.title
if text isa Dict
text = get(text, :text, missing)
if ismissing(text)
return big_layout
end
end
ann = attr(
font_size=16,
showarrow=false,
text=text,
x=mean(big_layout["xaxis$(ix).domain"]),
xanchor="center",
xref="paper",
y=big_layout["yaxis$(ix).domain"][2],
yanchor="bottom",
yref="paper"
)
anns = get(big_layout.fields, :annotations, [])
push!(anns, ann)
big_layout[:annotations] = anns
big_layout
end
# plots are 3d if any of their traces have a 3d type. This should flow down
# the methods as ordered here
_is3d(p::Plot) = any(_is3d, p.data)
_is3d(t::GenericTrace) = _is3d(t[:type])
_is3d(t::Symbol) = _is3d(string(t))
_is3d(s::AbstractString) = s in ["surface", "mesh3d", "scatter3d"]
# else (maybe if trace didn't have a :type field set)
_is3d(x::Any) = false
function _cat(rows::Tuple{Vararg{Int}}, ps::Plot...)
copied_plots = Plot[copy(p) for p in ps]
subplot_titles = any(map(x -> haskey(x.layout.fields, :title) ||
haskey(x.layout.fields, "title"), ps))
layout = gen_layout(rows, subplot_titles)
for ix in 1:sum(rows)
handle_titles!(layout, copied_plots[ix].layout, ix)
layout["xaxis$ix"] = merge(copied_plots[ix].layout["xaxis"], layout["xaxis$ix"])
layout["yaxis$ix"] = merge(copied_plots[ix].layout["yaxis"], layout["yaxis$ix"])
if _is3d(copied_plots[ix])
# need to remove (x|y)axis$ix and move their domains into scene$ix here
xaxis = pop!(layout, "xaxis$(ix)")
yaxis = pop!(layout, "yaxis$(ix)")
layout["scene$ix"] = attr(
domain = attr(
x = xaxis[:domain],
y = yaxis[:domain]
)
)
for trace in copied_plots[ix].data
trace["scene"] = "scene$ix"
end
else
for trace in copied_plots[ix].data
trace["xaxis"] = "x$ix"
trace["yaxis"] = "y$ix"
end
end
end
Plot(vcat([p.data for p in copied_plots]...), layout)
end
_cat(nr::Int, nc::Int, ps::Plot...) = _cat(Tuple(fill(nc, nr)), ps...)
Base.hcat(ps::Plot...) = _cat(1, length(ps), ps...)
Base.vcat(ps::Plot...) = _cat(length(ps), 1, ps...)
Base.hvcat(rows::Tuple{Vararg{Int}}, ps::Plot...) = _cat(rows, ps...)
# implementation of algorithms from plotly.py/packages/python/plotly/plotly/subplots.py
function _init_subplot_xy!(
layout::Layout, secondary_y::Bool, domain::NamedTuple{(:x, :y)}, max_subplot_ids::Dict
)
# Get axis label and anchor
x_count = max_subplot_ids["xaxis"] + 1
y_count = max_subplot_ids["xaxis"] + 1
# Compute x/y labels (the values of trace.xaxis/trace.yaxis
x_label = "x$(x_count > 1 ? x_count : "")"
y_label = "y$(y_count > 1 ? y_count : "")"
# Anchor x and y axes to each other
x_anchor, y_anchor = y_label, x_label
# Build layout.xaxis/layout.yaxis containers
xaxis_name = "xaxis$(x_count > 1 ? x_count : "")"
yaxis_name = "yaxis$(y_count > 1 ? y_count : "")"
# copy over existing xaxis/yaxis objects, but delete titles
x_axis = attr(;layout.xaxis...)
y_axis = attr(;layout.yaxis...)
x_axis.domain = domain.x
x_axis.anchor = x_anchor
y_axis.domain = domain.y
y_axis.anchor = y_anchor
pop!(x_axis, :title_text)
pop!(y_axis, :title_text)
layout[xaxis_name] = x_axis
layout[yaxis_name] = y_axis
subplot_refs = [
SubplotRef(
subplot_kind="xy",
layout_keys=Symbol.([xaxis_name, yaxis_name]),
trace_kwargs=attr(xaxis=x_label, yaxis=y_label)
)
]
if secondary_y
y_count += 1
secondary_yaxis_name = "yaxis$(y_count > 1 ? y_count : "")"
secondary_y_label = "y$(y_count > 1 ? y_count : "")"
push!(
subplot_refs,
SubplotRef(
subplot_kind="xy",
layout_keys=Symbol.([xaxis_name, secondary_yaxis_name]),
trace_kwargs=attr(xaxis=x_label, yaxis=secondary_y_label),
)
)
secondary_y_axis = attr(anchor=y_anchor, overlaying=y_label, side="right")
layout[secondary_yaxis_name] = secondary_y_axis
end
max_subplot_ids["xaxis"] = x_count
max_subplot_ids["yaxis"] = y_count
subplot_refs
end
function _init_subplot_single!(
layout::Layout, subplot_kind::String, domain::NamedTuple{(:x, :y)}, max_subplot_ids::Dict
)
count = max_subplot_ids[subplot_kind] + 1
label = "$(subplot_kind)$(count > 1 ? count : "")"
scene = attr(domain=attr(;domain...))
layout[label] = scene
trace_key = subplot_kind in _subplot_prop_named_subplot ? "subplot" : subplot_kind
subplot_ref = SubplotRef(
subplot_kind=subplot_kind,
layout_keys=[Symbol(label)],
trace_kwargs=attr(;Dict(Symbol(trace_key) => label)...)
)
max_subplot_ids[subplot_kind] = count
[subplot_ref]
end
function _init_subplot_domain!(domain::NamedTuple{(:x, :y)})
[SubplotRef(
subplot_kind="domain",
layout_keys=[],
trace_kwargs=attr(domain=attr(;domain...))
)]
end
function get_subplotkind_from_trace_type(k::Symbol)
schema = get_plotschema()
trace_attrs = schema.traces[k][:attributes]
trace_attr_names = keys(trace_attrs)
if :xaxis in trace_attr_names && :yaxis in trace_attr_names
return "xy"
elseif :domain in trace_attr_names
return "domain"
elseif :geo in trace_attr_names
return "geo"
elseif :scene in trace_attr_names
return "scene"
elseif k === :splom
return "xy"
elseif :subplot in trace_attr_names
return trace_attrs[:subplot][:dflt]
else
@error "Unknown subplot type for trace $k. Please open issue"
end
end
function _init_subplot!(
layout::Layout, subplot_kind::String, secondary_y::Bool,
domain::NamedTuple{(:x, :y)}, max_subplot_ids::Dict
)
sksymbol = Symbol(subplot_kind)
if sksymbol in _TRACE_TYPES
subplot_kind = get_subplotkind_from_trace_type(sksymbol)
end
if subplot_kind == "xy"
return _init_subplot_xy!(layout, secondary_y, domain, max_subplot_ids)
elseif subplot_kind in _single_subplot_types
return _init_subplot_single!(layout, subplot_kind, domain, max_subplot_ids)
elseif subplot_kind == "domain"
return _init_subplot_domain!(domain)
end
error("Unknown subplot_kind $subplot_kind")
end
function _configure_shared_axes!(layout::Layout, grid_ref::Matrix, specs::Matrix{<:_Maybe{Spec}}, x_or_y::String, shared::Union{Bool,String}, row_dir::Int)
rows, cols = size(grid_ref)
layout_key_ind = x_or_y == "x" ? 1 : 2
rows_iter = collect(1:rows)
row_dir < 0 && reverse!(rows_iter)
function update_axis_matches!(
first_axis_id, subplot_ref::SubplotRef, spec::Spec, remove_label::Bool
)
span = x_or_y == "x" ? spec.colspan : spec.rowspan
if subplot_ref.subplot_kind == "xy" && span == 1
if ismissing(first_axis_id)
first_axis_name = subplot_ref.layout_keys[layout_key_ind]
first_axis_id = replace(String(first_axis_name), "axis" => "")
else
axis_name = subplot_ref.layout_keys[layout_key_ind]
axis_to_match = layout[axis_name]
axis_to_match[:matches] = first_axis_id
if remove_label
axis_to_match[:showticklabels] = false
end
end
end
return first_axis_id
end
if shared == "columns" || (x_or_y == "x" && shared == true)
for c in 1:cols
first_axis_id = missing
ok_to_remove_label = x_or_y == "x"
for r in rows_iter
subplot_ref = grid_ref[r, c][1]
spec = specs[r, c]
first_axis_id = update_axis_matches!(
first_axis_id, subplot_ref, spec, ok_to_remove_label
)
end
end
elseif shared == "rows" || (x_or_y == "y" && shared == true)
for r in rows_iter
first_axis_id = missing
ok_to_remove_label = x_or_y == "y"
for c in 1:cols
subplot_ref = grid_ref[r, c][1]
spec = specs[r, c]
first_axis_id = update_axis_matches!(
first_axis_id, subplot_ref, spec, ok_to_remove_label
)
end
end
elseif shared == "all"
first_axis_id = missing
for c in 1:cols, (ri, r) in enumerate(rows_iter)
subplot_ref = grid_ref[r, c][1]
spec = specs[r, c]
ok_to_remove_label = let
if x_or_y == "y"
c > 0
else
row_dir > 0 ? ri > 0 : r < rows
end
end
first_axis_id = update_axis_matches!(
first_axis_id, subplot_ref, spec, ok_to_remove_label
)
end
end
end
_build_subplot_title_annotations(::Missing, list_of_domains; kw...) = []
function _build_subplot_title_annotations(
subplot_titles::Array{<:_Maybe{String}},
list_of_domains::Vector{<:NamedTuple{(:x, :y)}};
title_edge="top", offset=0
)
# If shared_axes is false (default) use list_of_domains
# This is used for insets and irregular layouts
# if not shared_xaxes and not shared_yaxes:
x_dom = [dom.x for dom in list_of_domains]
y_dom = [dom.y for dom in list_of_domains]
subtitle_pos_x = []
subtitle_pos_y = []
if title_edge == "top"
text_angle = 0
xanchor = "center"
yanchor = "bottom"
for x_domains in x_dom
push!(subtitle_pos_x, sum(x_domains) / 2)
end
for y_domains in y_dom
push!(subtitle_pos_y, y_domains[2])
end
yshift = offset
xshift = 0
elseif title_edge == "bottom"
text_angle = 0
xanchor = "center"
yanchor = "top"
for x_domains in x_dom
push!(subtitle_pos_x, sum(x_domains) / 2)
end
for y_domains in y_dom
push!(subtitle_pos_y, y_domains[1])
end
yshift = -offset
xshift = 0
elseif title_edge == "right"
text_angle = 90
xanchor = "left"
yanchor = "middle"
for x_domains in x_dom
push!(subtitle_pos_x, x_domains[2])
end
for y_domains in y_dom
push!(subtitle_pos_y, sum(y_domains) / 2.0)
end
yshift = 0
xshift = offset
elseif title_edge == "left"
text_angle = -90
xanchor = "right"
yanchor = "middle"
for x_domains in x_dom
push!(subtitle_pos_x, x_domains[1])
end
for y_domains in y_dom
push!(subtitle_pos_y, sum(y_domains) / 2.0)
end
yshift = 0
xshift = -offset
else
error("title_edge must be one of [top, bottom, left, right]")
end
plot_titles = []
for index in 1:length(subplot_titles)
title = subplot_titles[index]
should_continue = (
ismissing(title) || title == false || length(title) == 0 ||
index > length(subtitle_pos_y)
)
if should_continue
continue
end
annotation = attr(
y=subtitle_pos_y[index], yref="paper", yanchor=yanchor,
x=subtitle_pos_x[index], xref="paper", xanchor=xanchor,
text=title, font_size=16,
showarrow=false,
)
xshift != 0 && setindex!(annotation, xshift, :xshift)
yshift != 0 && setindex!(annotation, yshift, :yshift)
text_angle != 0 && setindex!(annotation, text_angle, :textangle)
push!(plot_titles, annotation)
end
plot_titles
end
function Layout(sp::Subplots; kw...)
layout = Layout(;kw...)
@unpack_Subplots sp
row_dir = start_cell == "top-left" ? -1 : 1
col_seq = collect(1:cols) .- 1
row_seq = collect(1:rows) .- 1
row_dir < 0 && reverse!(row_seq)
grid = [
(
sum(_widths[1:c]) + c * horizontal_spacing,
sum(_heights[1:r]) + r * vertical_spacing
)
for r in row_seq, c in col_seq
]
domains_grid = _Maybe{NamedTuple{(:x, :y)}}[missing for _ in 1:rows, __ in 1:cols]
list_of_domains = NamedTuple{(:x, :y)}[]
max_subplot_ids = _get_initial_max_subplot_ids()
for r in 1:rows, c in 1:cols
spec = specs[r, c]
if ismissing(spec)
continue
end
c_spanned = c + spec.colspan - 1
r_spanned = r + spec.rowspan - 1
c_spanned > cols && error("Some colspan value is too large for this subplot grid")
r_spanned > rows && error("Some rowspan value is too large for this subplot grid")
# grid x c_spanned -> x_domain
x_s = grid[r, c][1]
x_e = grid[r, c_spanned][1] + _widths[c_spanned] - spec.r
x_domain = (max(0.0, x_s), min(1.0, x_e))
# grid x r_spanned x row_dir -> yaxis_domain
y_domain = let
if row_dir > 1
(grid[r, c][2] + spec.b, grid[r_spanned, c][2] + _heights[r_spanned] - spec.t)
else
(grid[r_spanned, c][2], grid[r, c][2] + _heights[end - r + 1] - spec.t)
end
end
domain = (x = x_domain, y = y_domain)
domains_grid[r, c] = domain
push!(list_of_domains, domain)
grid_ref[r, c] = _init_subplot!(layout, spec.kind, spec.secondary_y, domain, max_subplot_ids)
end
_configure_shared_axes!(layout, grid_ref, specs, "x", shared_xaxes, row_dir)
_configure_shared_axes!(layout, grid_ref, specs, "y", shared_yaxes, row_dir)
# handle insets
insets_ref = ismissing(insets) ? missing : Any[missing for _ in 1:length(insets)]
if !ismissing(insets)
for (i_inset, inset) in enumerate(insets)
r = inset.cell[1]
c = inset.cell[2]
0 <= r <= rows || error("Some `cell` out of range")
0 <= c <= cols || error("Some `cell` out of range")
x_s = grid[r, c][1] + inset.l * _widths[c]
x_e = inset.w == "to_end" ? grid[r, c][1] + _widths[c] : (x_s + inset.w * _widths[c])
x_domain = (x_s, x_e)
y_s = grid[r, c][2] + inset.b * _heights[end - r + 1]
y_e = inset.h == "to_end" ? (grid[r, c][2] + _heights[end - r + 1]) : (y_s + inset.h * _heights[end - r + 1])
y_domain = (y_s, y_e)
domain = (x = x_domain, y = y_domain)
push!(list_of_domains, domain)
insets_ref[i_inset] = _init_subplot!(
layout, inset.kind, false, domain, max_subplot_ids
)
end
end
plot_title_annotations = _build_subplot_title_annotations(
subplot_titles, list_of_domains
)
layout[:annotations] = plot_title_annotations
if !ismissing(column_titles)
domains_list = let
if row_dir > 0
[domains_grid[end, c] for c in 1:cols]
else
[domains_grid[1, c] for c in 1:cols]
end
end
append!(
layout[:annotations],
_build_subplot_title_annotations(column_titles, domains_list)
)
end
if !ismissing(row_titles)
domains_list = [domains_grid[r, end] for r in 1:rows]
append!(
layout[:annotations],
_build_subplot_title_annotations(row_titles, domains_list, title_edge="right")
)
end
if !ismissing(x_title)
domains_list = [(x = (0, max_width), y = (0, 1))]
append!(
layout[:annotations],
_build_subplot_title_annotations(
[x_title], domains_list, title_edge="bottom", offset=30
)
)
end
if !ismissing(y_title)
domains_list = [(x = (0, 1), y = (0, 1))]
append!(
layout[:annotations],
_build_subplot_title_annotations(
[y_title], domains_list, title_edge="left", offset=40
)
)
end
layout.subplots = sp
layout
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 4805 | # key template data structure
@with_kw struct Template
data::Dict{Symbol,Vector{_ATTR}} = Dict()
layout::PlotlyAttribute = attr()
end
_json_lower(t::Template) = Dict(
:data => _json_lower(t.data),
:layout => _json_lower(t.layout)
)
==(t1::Template, t2::Template) = t1.data == t2.data && t1.layout == t2.layout
# to allow Template(data = attr(...))
function Base.convert(T::Type{Dict{Symbol,Vector{_ATTR}}}, x::PlotlyAttribute)
out = T()
for (k, v) in pairs(x)
if !(v isa Vector)
@error "Could not convert to Template.data. Expected argument at $k to be Vector. Found $v"
end
want = _ATTR[]
for item in v
push!(want, item)
end
out[k] = want
end
out
end
function Template(data, layout::Layout)
Template(data, attr(;layout.fields...))
end
_isempty(::Template) = false
Base.deepcopy(t::Template) = Template(deepcopy(t.data), deepcopy(t.layout))
Base.merge(t1::Template, _t2::Template) = merge!(deepcopy(t1), _t2)
function Base.merge!(out::Template, t2::Template)
for k in keys(t2.data)
t1_traces = Cycler(get(out.data, k, [attr()]))
t2_traces = Cycler(t2.data[k])
n_out = max(length(t1_traces), length(t2_traces))
out.data[k] = [merge(t1_traces[ix], t2_traces[ix]) for ix in 1:n_out]
end
# now update layout
merge!(out.layout, t2.layout)
return out
end
# loading templates from disk
_template_dir() = template_dir = joinpath(artifact"plotly-base-artifacts", "templates")
_available_templates() = [replace(x, ".json" => "") for x in readdir(_template_dir())]
function _load_template(name::String)
# first try to look it up
name = Symbol(replace(name, ".json" => ""))
if name in keys(templates) && !ismissing(templates.templates[name])
return templates.templates[name]
end
# otherwise try to load
template_dir = _template_dir()
to_load = joinpath(template_dir, string(name, ".json"))
# check if this template exists
exists = isfile(to_load)
if !exists
@error "Unknown template $(name). Known values are $(_available_templates())"
end
# if it does, load and process it
raw = _symbol_dict(open(JSON.parse, to_load))
layout = attr(;_symbol_dict(get(raw, :layout, Dict()))...)
data = Dict{Symbol,Vector{_ATTR}}()
# data will be object of arrays. Each array has trace attributes
for (trace_type, trace_val_array) in get(raw, :data, Dict())
want = _ATTR[]
for trace_data in trace_val_array
push!(want, attr(;_symbol_dict(trace_data)...))
end
data[Symbol(trace_type)] = want
end
out = Template(data, layout)
templates[name] = out
return out
end
# Singleton object for handling templates
mutable struct _TemplatesConfig
default::String
templates::Dict{Symbol,_Maybe{Template}}
end
# custom set property to allow setting default with validation
Base.setproperty!(tc::_TemplatesConfig, k::Symbol, x) = setproperty!(tc, Val{k}(), x)
function Base.setproperty!(tc::_TemplatesConfig, ::Val{k}, x) where k
if hasfield(_TemplatesConfig, k)
return setfield!(tc, k, x)
end
return setindex!(tc, x, k)
end
function Base.setproperty!(tc::_TemplatesConfig, ::Val{:default}, x::String)
# TODO: validation here
setfield!(tc, :default, x)
end
# custom getproperty to add available
Base.getproperty(tc::_TemplatesConfig, k::Symbol) = getproperty(tc, Val{k}())
function Base.getproperty(tc::_TemplatesConfig, ::Val{k}) where k
if hasfield(_TemplatesConfig, k)
return getfield(tc, k)
end
return getindex(tc, k)
end
Base.getproperty(tc::_TemplatesConfig, ::Val{:available}) = collect(keys(tc.templates))
Base.propertynames(tc::_TemplatesConfig) = Symbol[fieldnames(_TemplatesConfig)..., :available]
# setindex! and getindex, and keys work through the `.templates` dict
function Base.setindex!(tc::_TemplatesConfig, val::Template, k::Union{Symbol,String})
setindex!(tc.templates, val, Symbol(k))
end
function Base.getindex(tc::_TemplatesConfig, k::Symbol)
if haskey(tc.templates, k) && !ismissing(tc.templates[k])
return tc.templates[k]
end
return _load_template(String(k))
end
function Base.getindex(tc::_TemplatesConfig, k::String)
parts = strip.(split(k, "+"))
reduce(merge, getindex.(Ref(tc), Symbol.(parts)))
end
Base.keys(tc::_TemplatesConfig) = keys(tc.templates)
function Base.show(io::IO, ::MIME"text/plain", tc::_TemplatesConfig)
msg = """
Templates configuration
-----------------------
Default template: $(tc.default)
Available templates: $(tc.available)
"""
println(io, msg)
end
const templates = _TemplatesConfig("plotly", Dict(Symbol(k) => missing for k in _available_templates()))
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 15338 | abstract type AbstractTrace end
abstract type AbstractLayout end
mutable struct GenericTrace{T <: AbstractDict{Symbol,Any}} <: AbstractTrace
fields::T
end
function GenericTrace(kind::Union{AbstractString,Symbol},
fields=Dict{Symbol,Any}(); kwargs...)
# use setindex! methods below to handle `_` substitution
fields[:type] = kind
gt = GenericTrace(fields)
foreach(x -> setindex!(gt, x[2], x[1]), kwargs)
if Symbol(kind) in [:contour, :contourcarpet, :heatmap, :heatmapgl]
if !haskey(gt, :transpose)
gt.transpose = true
end
end
gt
end
function _layout_defaults()
Dict{Symbol,Any}(
:margin => Dict(:l => 50, :r => 50, :t => 60, :b => 50),
:template => templates[templates.default],
)
end
mutable struct Layout{T <: AbstractDict{Symbol,Any}} <: AbstractLayout
fields::T
subplots::Subplots
function Layout{T}(fields::T; kwargs...) where T
l = new{T}(merge(_layout_defaults(), fields), Subplots())
foreach(x -> setindex!(l, x[2], x[1]), kwargs)
l
end
end
Layout(fields::T=Dict{Symbol,Any}(); kwargs...) where {T <: AbstractDict{Symbol,Any}} =
Layout{T}(fields; kwargs...)
kind(gt::GenericTrace) = get(gt, :type, "scatter")
kind(l::Layout) = "layout"
# -------------------------------------------- #
# Specific types of trace or layout attributes #
# -------------------------------------------- #
function attr(fields::AbstractDict=Dict{Symbol,Any}(); kwargs...)
# use setindex! methods below to handle `_` substitution
s = PlotlyAttribute(fields)
for (k, v) in kwargs
s[k] = v
end
s
end
attr(x::PlotlyAttribute; kw...) = attr(;x..., kw...)
mutable struct PlotlyFrame{T <: AbstractDict{Symbol,Any}} <: AbstractPlotlyAttribute
fields::T
function PlotlyFrame{T}(fields::T) where T
!(Symbol("name") in keys(fields)) && @warn("Frame should have a :name field for expected behavior")
new{T}(fields)
end
end
function frame(fields=Dict{Symbol,Any}(); kwargs...)
for (k, v) in kwargs
fields[k] = v
end
PlotlyFrame{Dict{Symbol,Any}}(fields)
end
abstract type AbstractLayoutAttribute <: AbstractPlotlyAttribute end
abstract type AbstractShape <: AbstractLayoutAttribute end
kind(::AbstractPlotlyAttribute) = "PlotlyAttribute"
# TODO: maybe loosen some day
const _Scalar = Union{DateTime,Date,Number,AbstractString,Symbol}
# ------ #
# Shapes #
# ------ #
mutable struct Shape <: AbstractLayoutAttribute
fields::AbstractDict{Symbol}
end
function Shape(kind::AbstractString, fields=Dict{Symbol,Any}(); kwargs...)
# use setindex! methods below to handle `_` substitution
fields[:type] = kind
s = Shape(fields)
foreach(x -> setindex!(s, x[2], x[1]), kwargs)
s
end
# helper method needed below
_rep(x, n) = take(cycle(x), n)
# line, circle, and rect share same x0, x1, y0, y1 args. Define methods for
# them here
for t in [:line, :circle, :rect]
str_t = string(t)
@eval $t(d::AbstractDict=Dict{Symbol,Any}(), ;kwargs...) =
Shape($str_t, d; kwargs...)
eval(Expr(:export, t))
@eval function $(t)(x0::_Scalar, x1::_Scalar, y0::_Scalar, y1::_Scalar,
fields::AbstractDict=Dict{Symbol,Any}(); kwargs...)
$(t)(fields; x0=x0, x1=x1, y0=y0, y1=y1, kwargs...)
end
@eval function $(t)(x0::Union{AbstractVector,_Scalar},
x1::Union{AbstractVector,_Scalar},
y0::Union{AbstractVector,_Scalar},
y1::Union{AbstractVector,_Scalar},
fields::AbstractDict=Dict{Symbol,Any}(); kwargs...)
n = reduce(max, map(length, (x0, x1, y0, y1)))
f(_x0, _x1, _y0, _y1) = $(t)(_x0, _x1, _y0, _y1, copy(fields); kwargs...)
map(f, _rep(x0, n), _rep(x1, n), _rep(y0, n), _rep(y1, n))
end
end
@doc "Draw a line through the points (x0, y0) and (x1, y2)" line
@doc """
Draw a circle from ((`x0`+`x1`)/2, (`y0`+`y1`)/2)) with radius
(|(`x0`+`x1`)/2 - `x0`|, |(`y0`+`y1`)/2 -`y0`)|) """ circle
@doc """
Draw a rectangle linking (`x0`,`y0`), (`x1`,`y0`),
(`x1`,`y1`), (`x0`,`y1`), (`x0`,`y0`)""" rect
"Draw an arbitrary svg path"
path(p::AbstractString; kwargs...) = Shape("path"; path=p, kwargs...)
export path
# derived shapes
vline(x, ymin, ymax, fields::AbstractDict=Dict{Symbol,Any}(); kwargs...) =
line(x, x, ymin, ymax, fields; kwargs...)
"""
`vline(x, fields::AbstractDict=Dict{Symbol,Any}(); kwargs...)`
Draw vertical lines at each point in `x` that span the height of the plot
"""
vline(x, fields::AbstractDict=Dict{Symbol,Any}(); kwargs...) =
vline(x, 0, 1, fields; xref="x", yref="paper", kwargs...)
hline(y, xmin, xmax, fields::AbstractDict=Dict{Symbol,Any}(); kwargs...) =
line(xmin, xmax, y, y, fields; kwargs...)
"""
`hline(y, fields::AbstractDict=Dict{Symbol,Any}(); kwargs...)`
Draw horizontal lines at each point in `y` that span the width of the plot
"""
hline(y, fields::AbstractDict=Dict{Symbol,Any}(); kwargs...) =
hline(y, 0, 1, fields; xref="paper", yref="y", kwargs...)
# ---------------------------------------- #
# Implementation of getindex and setindex! #
# ---------------------------------------- #
const HasFields = Union{GenericTrace,Layout,Shape,PlotlyAttribute,PlotlyFrame}
const _LikeAssociative = Union{PlotlyAttribute,AbstractDict}
_symbol_dict(hf::HasFields) = _symbol_dict(hf.fields)
#= NOTE: Generate this list with the following code
using JSON, PlotlyJS, PlotlyBase
d = JSON.parsefile(Pkg.dir("PlotlyJS", "deps", "plotschema.json"))
d = PlotlyBase._symbol_dict(d)
nms = Set{Symbol}()
function add_to_names!(d::AbstractDict)
map(add_to_names!, collect(keys(d)))
map(add_to_names!, collect(values(d)))
nothing
end
add_to_names!(s::Symbol) = push!(nms, s)
add_to_names!(x) = nothing
add_to_names!(d[:schema][:layout][:layoutAttributes])
for (_, v) in d[:schema][:traces]
add_to_names!(v)
end
_UNDERSCORE_ATTRS = collect(
filter(
x-> contains(string(x), "_") && !startswith(string(x), "_"),
nms
)
) =#
const _UNDERSCORE_ATTRS = [:error_x, :copy_ystyle, :error_z, :plot_bgcolor,
:paper_bgcolor, :copy_zstyle, :error_y, :hr_name]
_isempty(x) = isempty(x)
_isempty(::Union{Nothing,Missing}) = true
_isempty(x::Bool) = x
_isempty(x::Union{Symbol,String}) = false
function Base.merge(hf::HasFields, d::Dict)
out = deepcopy(hf)
for (k, v) in d
out[k] = d
end
out
end
function Base.merge!(hf1::HasFields, hf2::HasFields)
for (k, v) in hf2.fields
hf1[k] = v
end
hf1
end
Base.merge(d::Dict{Symbol}, hf2::HasFields) = merge(d, hf2.fields)
Base.merge!(d::Dict{Symbol}, hf2::HasFields) = merge!(d, hf2.fields)
Base.pairs(hf::HasFields) = pairs(hf.fields)
Base.keys(hf::HasFields) = keys(hf.fields)
Base.values(hf::HasFields) = values(hf.fields)
function setifempty!(hf::HasFields, key::Symbol, value)
if _isempty(hf[key])
hf[key] = value
end
end
Base.haskey(hf::HasFields, k::Symbol) = haskey(hf.fields, k)
Base.merge(hf1::T, hf2::T) where {T <: HasFields} =
merge!(deepcopy(hf1), hf2)
Base.isempty(hf::HasFields) = isempty(hf.fields)
function Base.get(hf::HasFields, k::Symbol, default)
out = getindex(hf, k)
(out == Dict()) ? default : out
end
Base.iterate(hf::HasFields) = iterate(hf.fields)
Base.iterate(hf::HasFields, x) = iterate(hf.fields, x)
==(hf1::T, hf2::T) where {T <: HasFields} = hf1.fields == hf2.fields
# NOTE: there is another method in dataframes_api.jl
_obtain_setindex_val(container::Any, val::Any, key::_Maybe{Symbol}=missing) = val
_obtain_setindex_val(container::Dict, val::Any, key::_Maybe{Symbol}=missing) = haskey(container, val) ? container[val] : val
_obtain_setindex_val(container::Any, func::Function, key::_Maybe{Symbol}=missing) = func(container)
_obtain_setindex_val(container::Missing, func::Function, key::_Maybe{Symbol}=missing) = func
# no container
Base.setindex!(gt::HasFields, val, key::String...) = setindex!(gt, val, missing, key...)
# methods that allow you to do `obj["first.second.third"] = val`
function Base.setindex!(gt::HasFields, val, container, key::String)
if in(Symbol(key), _UNDERSCORE_ATTRS)
return gt.fields[Symbol(key)] = _obtain_setindex_val(container, val)
else
return setindex!(gt, val, container, map(Symbol, split(key, ['.', '_']))...)
end
end
Base.setindex!(gt::HasFields, val, container, keys::String...) =
setindex!(gt, val, container, map(Symbol, keys)...)
# Now for deep setindex. The deepest the json schema ever goes is 4 levels deep
# so we will simply write out the setindex calls for 4 levels by hand. If the
# schema gets deeper in the future we can @generate them with @nexpr
# no container
Base.setindex!(gt::HasFields, val, key::Symbol...) = setindex!(gt, val, missing, key...)
function Base.setindex!(gt::HasFields, val, container, key::Symbol)
# check if single key has underscores, if so split as str and call above
# unless it is one of the special attribute names with an underscore
if occursin("_", string(key))
# check for title
if key in [:xaxis_title, :yaxis_title, :zaxis_title, :title] && (typeof(val) in [String, Symbol])
key = Symbol(key, :_text)
end
if !in(key, _UNDERSCORE_ATTRS)
return setindex!(gt, val, container, string(key))
end
end
gt.fields[key] = _obtain_setindex_val(container, val, key)
end
function Base.setindex!(gt::HasFields, val, container, k1::Symbol, k2::Symbol)
d1 = get(gt.fields, k1, Dict())
si_val = _obtain_setindex_val(container, val)
d1[k2] = si_val
gt.fields[k1] = d1
si_val
end
function Base.setindex!(gt::HasFields, val, container, k1::Symbol, k2::Symbol, k3::Symbol)
d1 = get(gt.fields, k1, Dict())
d2 = get(d1, k2, Dict())
si_val = _obtain_setindex_val(container, val)
d2[k3] = si_val
d1[k2] = d2
gt.fields[k1] = d1
si_val
end
function Base.setindex!(gt::HasFields, val, container, k1::Symbol, k2::Symbol,
k3::Symbol, k4::Symbol)
d1 = get(gt.fields, k1, Dict())
d2 = get(d1, k2, Dict())
d3 = get(d2, k3, Dict())
si_val = _obtain_setindex_val(container, val)
d3[k4] = si_val
d2[k3] = d3
d1[k2] = d2
gt.fields[k1] = d1
si_val
end
function Base.setindex!(gt::HasFields, val, container, k1::Symbol, k2::Symbol,
k3::Symbol, k4::Symbol, k5::Symbol)
d1 = get(gt.fields, k1, Dict())
d2 = get(d1, k2, Dict())
d3 = get(d2, k3, Dict())
d4 = get(d3, k4, Dict())
si_val = _obtain_setindex_val(container, val)
d4[k5] = si_val
d3[k4] = d4
d2[k3] = d3
d1[k2] = d2
gt.fields[k1] = d1
si_val
end
#= NOTE: I need to special case instances when `val` is Associatve like so that
I can partially update something that already exists.
Example:
hf = Layout(font_size=10)
val = Layout(font_family="Helvetica") =#
Base.setindex!(gt::HasFields, val::_LikeAssociative, key::Symbol...) = setindex!(gt, val, missing, key...)
function Base.setindex!(gt::HasFields, val::_LikeAssociative, container, key::Symbol)
if occursin("_", string(key))
if !in(key, _UNDERSCORE_ATTRS)
return setindex!(gt, val, string(key))
end
end
if key === :geojson
gt.fields[key] = val
return
end
for (k, v) in val
setindex!(gt, v, container, key, k)
end
end
function Base.setindex!(gt::HasFields, val::_LikeAssociative, container, k1::Symbol,
k2::Symbol)
for (k, v) in val
setindex!(gt, v, container, k1, k2, k)
end
end
function Base.setindex!(gt::HasFields, val::_LikeAssociative, container, k1::Symbol,
k2::Symbol, k3::Symbol)
for (k, v) in val
setindex!(gt, v, container, k1, k2, k3, k)
end
end
function Base.setproperty!(hf::HF, p::Symbol, val) where HF <: HasFields
if hasfield(HF, p)
return setfield!(hf, p, val)
end
setindex!(hf, val, p)
end
# now on to the simpler getindex methods. They will try to get the desired
# key, but if it doesn't exist an empty dict is returned
function Base.getindex(gt::HasFields, key::String)
if in(Symbol(key), _UNDERSCORE_ATTRS)
gt.fields[Symbol(key)]
else
getindex(gt, map(Symbol, split(key, ['.', '_']))...)
end
end
Base.getindex(gt::HasFields, keys::String...) =
getindex(gt, map(Symbol, keys)...)
function Base.getindex(gt::HasFields, key::Symbol)
if occursin("_", string(key))
if !in(key, _UNDERSCORE_ATTRS)
return getindex(gt, string(key))
end
end
get(gt.fields, key, Dict())
end
function Base.getindex(gt::HasFields, k1::Symbol, k2::Symbol)
d1 = get(gt.fields, k1, Dict())
get(d1, k2, Dict())
end
function Base.getindex(gt::HasFields, k1::Symbol, k2::Symbol, k3::Symbol)
d1 = get(gt.fields, k1, Dict())
d2 = get(d1, k2, Dict())
get(d2, k3, Dict())
end
function Base.getindex(gt::HasFields, k1::Symbol, k2::Symbol,
k3::Symbol, k4::Symbol)
d1 = get(gt.fields, k1, Dict())
d2 = get(d1, k2, Dict())
d3 = get(d2, k3, Dict())
get(d3, k4, Dict())
end
function Base.getindex(gt::HasFields, k1::Symbol, k2::Symbol,
k3::Symbol, k4::Symbol, k5::Symbol)
d1 = get(gt.fields, k1, Dict())
d2 = get(d1, k2, Dict())
d3 = get(d2, k3, Dict())
d4 = get(d3, k4, Dict())
get(d4, k5, Dict())
end
function Base.getproperty(gt::HF, p::Symbol) where HF <: HasFields
if hasfield(HF, p)
return getfield(gt, p)
end
getindex(gt, p)
end
# Now to the pop! methods
function Base.pop!(gt::HasFields, key::String)
if in(Symbol(key), _UNDERSCORE_ATTRS)
pop!(gt.fields, Symbol(key))
else
pop!(gt, map(Symbol, split(key, ['.', '_']))...)
end
end
Base.pop!(gt::HasFields, keys::String...) =
pop!(gt, map(Symbol, keys)...)
function Base.pop!(gt::HasFields, key::Symbol)
if occursin("_", string(key))
if !in(key, _UNDERSCORE_ATTRS)
return pop!(gt, string(key))
end
end
pop!(gt.fields, key, Dict())
end
function Base.pop!(gt::HasFields, k1::Symbol, k2::Symbol)
d1 = get(gt.fields, k1, Dict())
pop!(d1, k2, Dict())
end
function Base.pop!(gt::HasFields, k1::Symbol, k2::Symbol, k3::Symbol)
d1 = get(gt.fields, k1, Dict())
d2 = get(d1, k2, Dict())
pop!(d2, k3, Dict())
end
function Base.pop!(gt::HasFields, k1::Symbol, k2::Symbol,
k3::Symbol, k4::Symbol)
d1 = get(gt.fields, k1, Dict())
d2 = get(d1, k2, Dict())
d3 = get(d2, k3, Dict())
pop!(d3, k4, Dict())
end
# Function used to have meaningful display of traces and layouts
function _describe(x::HasFields)
fields = sort(map(String, collect(keys(x.fields))))
n_fields = length(fields)
if n_fields == 0
return "$(kind(x)) with no fields"
elseif n_fields == 1
return "$(kind(x)) with field $(fields[1])"
elseif n_fields == 2
return "$(kind(x)) with fields $(fields[1]) and $(fields[2])"
else
return "$(kind(x)) with fields $(join(fields, ", ", ", and "))"
end
end
Base.show(io::IO, ::MIME"text/plain", g::HasFields) =
println(io, _describe(g))
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 580 | """
trace_map(p::Plot, axis::Symbol=:x)
Return an array of `length(p.data)` that maps each element of `p.data` into an
integer for which number axis of kind `axis` that trace belogs to. `axis` can
either be `x` or `y`. If `x` is given, return the integer for which x-axis the
trace belongs to. Similar for `y`.
"""
function trace_map(p::Plot, axis=:x)
out = fill(1, length(p.data))
ax_key = axis == :x ? :xaxis : :yaxis
for (i, t) in enumerate(p.data)
if haskey(t, ax_key)
out[i] = parse(Int, t[ax_key][2:end])
end
end
out
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 13507 | function fresh_data()
t1 = scatter(;y=[1, 2, 3])
t2 = scatter(;y=[10, 20, 30])
t3 = scatter(;y=[100, 200, 300])
l = Layout(;title="Foo")
p = Plot([copy(t1), copy(t2), copy(t3)], copy(l))
t1, t2, t3, l, p
end
@testset "Test api methods on Plot" begin
@testset "test helpers" begin
@test PlotlyBase._prep_restyle_vec_setindex([1, 2], 2) == [1, 2]
@test PlotlyBase._prep_restyle_vec_setindex([1, 2], 3) == [1, 2, 1]
@test PlotlyBase._prep_restyle_vec_setindex([1, 2], 4) == [1, 2, 1, 2]
@test PlotlyBase._prep_restyle_vec_setindex((1, [42, 4]), 2) == Any[1, [42, 4]]
@test PlotlyBase._prep_restyle_vec_setindex((1, [42, 4]), 3) == Any[1, [42, 4], 1]
@test PlotlyBase._prep_restyle_vec_setindex((1, [42, 4]), 4) == Any[1, [42, 4], 1, [42, 4]]
end
@testset "test _update_fields" begin
t1, t2, t3, l, p = fresh_data()
# test dict version
o = copy(t1)
PlotlyBase._update_fields(o, 1, Dict{Symbol,Any}(:foo => "Bar"))
@test o["foo"] == "Bar"
# kwarg version
PlotlyBase._update_fields(o, 1; foo="Foo")
@test o["foo"] == "Foo"
# dict + kwarg version. Make sure dict gets through w/out replacing _
PlotlyBase._update_fields(o, 1, Dict{Symbol,Any}(:fuzzy_wuzzy => "Bear");
fuzzy_wuzzy="?")
@test o.fields[:fuzzy_wuzzy] == "Bear"
@test isa(o.fields[:fuzzy], Dict)
@test o["fuzzy.wuzzy"] == "?"
end
@testset "test relayout!" begin
t1, t2, t3, l, p = fresh_data()
# test on plot object
relayout!(p, Dict{Symbol,Any}(:title => "Fuzzy"); xaxis_title="wuzzy")
@test p.layout["title"] == "Fuzzy"
@test p.layout["xaxis.title.text"] == "wuzzy"
# test on layout object
relayout!(l, Dict{Symbol,Any}(:title => "Fuzzy"); xaxis_title="wuzzy")
@test l["title"] == "Fuzzy"
@test l["xaxis.title.text"] == "wuzzy"
end
@testset "test react!" begin
t1, t2, t3, l, p = fresh_data()
t4 = bar(x=[1, 2, 3], y=[42, 242, 142])
l2 = Layout(xaxis_title="wuzzy")
react!(p, [t4], l2)
@test length(p.data) == 1
@test p.data[1] == t4
@test p.layout == l2
end
@testset "test purge!" begin
t1, t2, t3, l, p = fresh_data()
purge!(p)
@test p.data == []
@test p.layout == Layout()
end
@testset "test restyle!" begin
t1, t2, t3, l, p = fresh_data()
# test on trace object
restyle!(t1, 1, Dict{Symbol,Any}(:opacity => 0.4); marker_color="red")
@test t1["opacity"] == 0.4
@test t1["marker.color"] == "red"
# test for single trace in plot
restyle!(p, 2, Dict{Symbol,Any}(:opacity => 0.4); marker_color="red")
@test p.data[2]["opacity"] == 0.4
@test p.data[2]["marker.color"] == "red"
# test for multiple trace in plot
restyle!(p, [1, 3], Dict{Symbol,Any}(:opacity => 0.9); marker_color="blue")
@test p.data[1]["opacity"] == 0.9
@test p.data[1]["marker.color"] == "blue"
@test p.data[3]["opacity"] == 0.9
@test p.data[3]["marker.color"] == "blue"
# test for all traces in plot
restyle!(p, 1:3, Dict{Symbol,Any}(:opacity => 0.42); marker_color="white")
for i in 1:3
@test p.data[i]["opacity"] == 0.42
@test p.data[i]["marker.color"] == "white"
end
@testset "test restyle with vector attributes applied to all traces" begin
# test that short arrays repeat
restyle!(p, marker_color=["red", "green"])
@test p.data[1]["marker.color"] == "red"
@test p.data[2]["marker.color"] == "green"
@test p.data[3]["marker.color"] == "red"
# test that array of arrays is repeated and applied everywhere
restyle!(p, marker_color=(["red", "green"],))
@test p.data[1]["marker.color"] == ["red", "green"]
@test p.data[2]["marker.color"] == ["red", "green"]
@test p.data[3]["marker.color"] == ["red", "green"]
# test that array of arrays is repeated and applied everywhere
restyle!(p, marker_color=(["red", "green"], "blue"))
@test p.data[1]["marker.color"] == ["red", "green"]
@test p.data[2]["marker.color"] == "blue"
@test p.data[3]["marker.color"] == ["red", "green"]
end
@testset "test restyle with vector attributes applied to vector of traces" begin
# test that short arrays repeat
restyle!(p, 1:3, marker_color=["red", "green"])
@test p.data[1]["marker.color"] == "red"
@test p.data[2]["marker.color"] == "green"
@test p.data[3]["marker.color"] == "red"
# test that array of arrays is repeated and applied everywhere
restyle!(p, 1:3, marker_color=(["red", "green"],))
@test p.data[1]["marker.color"] == ["red", "green"]
@test p.data[2]["marker.color"] == ["red", "green"]
@test p.data[3]["marker.color"] == ["red", "green"]
# test that array of arrays is repeated and applied everywhere
restyle!(p, 1:3, marker_color=(["red", "green"], "blue"))
@test p.data[1]["marker.color"] == ["red", "green"]
@test p.data[2]["marker.color"] == "blue"
@test p.data[3]["marker.color"] == ["red", "green"]
end
@testset "test restyle with vector attributes applied to trace object" begin
restyle!(t1, 1, x=[1, 2, 3])
@test t1["x"] == 1
restyle!(t1, 1, x=([1, 2, 3],))
@test t1["x"] == [1, 2, 3]
end
@testset "test restyle with vector attributes applied to single trace " begin
restyle!(p, 2, x=[1, 2, 3])
@test p.data[2]["x"] == 1
restyle!(p, 2, x=([1, 2, 3],))
@test p.data[2]["x"] == [1, 2, 3]
end
end
@testset "test addtraces!" begin
t1, t2, t3, l, p = fresh_data()
p2 = Plot()
# test add one trace to end
addtraces!(p2, t1)
@test length(p2.data) == 1
@test p2.data[1] == t1
# test add two traces to end
addtraces!(p2, t2, t3)
@test length(p2.data) == 3
@test p2.data[2] == t2
@test p2.data[3] == t3
# test add one trace middle
t4 = scatter()
addtraces!(p2, 2, t4)
@test length(p2.data) == 4
@test p2.data[1] == t1
@test p2.data[2] == t4
@test p2.data[3] == t2
@test p2.data[4] == t3
# test add multiple trace middle
t5 = scatter()
t6 = scatter()
addtraces!(p2, 2, t5, t6)
@test length(p2.data) == 6
@test p2.data[1] == t1
@test p2.data[2] == t5
@test p2.data[3] == t6
@test p2.data[4] == t4
@test p2.data[5] == t2
@test p2.data[6] == t3
end
@testset "test deletetraces!" begin
t1, t2, t3, l, p = fresh_data()
# test delete one trace
deletetraces!(p, 2)
@test length(p.data) == 2
@test p.data[1]["y"] == t1["y"]
@test p.data[2]["y"] == t3["y"]
# test delete multiple traces
deletetraces!(p, 1, 2)
@test length(p.data) == 0
end
@testset "test movetraces!" begin
t1, t2, t3, l, p = fresh_data()
# test move one trace to end
movetraces!(p, 2) # now 1 3 2
@test p.data[1]["y"] == t1["y"]
@test p.data[2]["y"] == t3["y"]
@test p.data[3]["y"] == t2["y"]
# test move two traces to end
movetraces!(p, 1, 2) # now 2 1 3
@test p.data[1]["y"] == t2["y"]
@test p.data[2]["y"] == t1["y"]
@test p.data[3]["y"] == t3["y"]
# test move from/to
movetraces!(p, [1, 3], [2, 1]) # 213 -> 123 -> 312
@test p.data[1]["y"] == t3["y"]
@test p.data[2]["y"] == t1["y"]
@test p.data[3]["y"] == t2["y"]
end
@testset "test update_XXX! layout props" begin
t1, t2, t3, l, p = fresh_data()
p2 = [p p]
@test PlotlyBase._isempty(p2.layout.xaxis2_showticklabels)
@test PlotlyBase._isempty(p2.layout.xaxis_showticklabels)
update_xaxes!(p2, showticklabels=true)
@test p2.layout.xaxis2_showticklabels
@test p2.layout.xaxis_showticklabels
p3 = [p; p]
@test PlotlyBase._isempty(p3.layout.yaxis2_showticklabels)
@test PlotlyBase._isempty(p3.layout.yaxis_showticklabels)
update_yaxes!(p3, showticklabels=true)
@test p3.layout.yaxis2_showticklabels
@test p3.layout.yaxis_showticklabels
for ann in p2.layout.annotations
@test ann[:font][:size] != 1
end
update_annotations!(p2, font_size=1)
for ann in p2.layout.annotations
@test ann[:font][:size] == 1
end
end
@testset "add_trace!" begin
df = stack(DataFrame(x=1:10, one=1, two=2, three=3, four=4, five=5, six=6, seven=7), Not(:x))
p = Plot(df, x=:x, y=:value, facet_col=:variable, facet_col_wrap=2)
@test length(p.data) == 7
@test size(p.layout.subplots.grid_ref) == (4, 2)
add_trace!(p, scatter(x=1:4, y=(1:4).^2), row="all") # add to all rows in col 1
@test length(p.data) == 11
add_trace!(p, scatter(x=1:4, y=(1:4).^2), col="all") # add to all cols in row 1
@test length(p.data) == 13
add_trace!(p, scatter(x=1:4, y=(1:4).^2), col=Colon(), row=3) # add to all cols in row 3
@test length(p.data) == 15
add_trace!(p, scatter(x=1:4, y=(1:4).^2), row="all", col="all")
@test length(p.data) == 23 # adds 8 because we get full 4x2 grid
end
end
@testset "add_shape!" begin
p = Plot(Layout(Subplots(rows=2, cols=2)))
add_trace!(p, scatter(y=rand(4)), row="all", col="all")
add_shape!(p, rect(x0=2, x1=4, y0=0.2, y1=0.5, line_color="purple"), row="all", col="all")
@test length(p.layout.shapes) == 4
@test all(s -> s.type == "rect", p.layout.shapes)
@test all(s -> s.line_color == "purple", p.layout.shapes)
add_shape!(p, line(x0=1, x1=3, y0=.5, y1=.9, line_color="yellow"), row="all", col=2)
@test length(p.layout.shapes) == 6
@test all(s -> s.type == "line", p.layout.shapes[5:6])
@test all(s -> s.line_color == "yellow", p.layout.shapes[5:6])
add_shape!(p, circle(x0=4, y0=0.2, x1=5, y1=0.7, line_color="black"), row=2)
@test length(p.layout.shapes) == 8
@test all(s -> s.type == "circle", p.layout.shapes[7:8])
@test all(s -> s.line_color == "black", p.layout.shapes[7:8])
end
@testset "unpack namedtuple type figure" begin
fig = (
data = [
(type = "scatter", x = 1:4, y = rand(4), mode = "markers", marker = (line = (color = "red", width = 2), size = 8)),
(type = "scatter", x = 1:4, y = rand(4))
],
layout = (hovermode = "unified",)
)
plots = Plot[
Plot(fig)
Plot((layout = fig.layout, data = fig.data))
Plot((;frames=[], fig...))
Plot((;fig..., frames=[]))
Plot((layout = fig.layout, frames = [], data = fig.data))
Plot((data = fig.data, frames = [], layout = fig.layout))
]
p1 = plots[1];
for p2 in plots[2:end]
@test p1.layout == p2.layout
@test p1.data == p2.data
end
@test p1.data[1] isa GenericTrace
@test p1.data[1].marker isa Dict
@test p1.data[1].marker_line isa Dict
@test p1.data[1].marker_line_width isa Number
end
@testset "subplots" begin
labels = ["1st", "2nd", "3rd", "4th", "5th"]
# Define color sets of paintings
night_colors = ["rgb(56, 75, 126)", "rgb(18, 36, 37)", "rgb(34, 53, 101)",
"rgb(36, 55, 57)", "rgb(6, 4, 4)"]
sunflowers_colors = ["rgb(177, 127, 38)", "rgb(205, 152, 36)", "rgb(99, 79, 37)",
"rgb(129, 180, 179)", "rgb(124, 103, 37)"]
irises_colors = ["rgb(33, 75, 99)", "rgb(79, 129, 102)", "rgb(151, 179, 100)",
"rgb(175, 49, 35)", "rgb(36, 73, 147)"]
cafe_colors = ["rgb(146, 123, 21)", "rgb(177, 180, 34)", "rgb(206, 206, 40)",
"rgb(175, 51, 21)", "rgb(35, 36, 21)"]
# Create subplots, using "domain" type for pie charts
layout = Layout(Subplots(rows=2, cols=2, specs=fill(Spec(kind="domain"), 2, 2)))
fig = Plot(layout)
# Define pie charts
add_trace!(fig, pie(labels=labels, values=[38, 27, 18, 10, 7], name="Starry Night",
marker_colors=night_colors), row=1, col=1)
add_trace!(fig, pie(labels=labels, values=[28, 26, 21, 15, 10], name="Sunflowers",
marker_colors=sunflowers_colors), row=1, col=2)
add_trace!(fig, pie(labels=labels, values=[38, 19, 16, 14, 13], name="Irises",
marker_colors=irises_colors), row=2, col=1)
add_trace!(fig, pie(labels=labels, values=[31, 24, 19, 18, 8], name="The Night Café",
marker_colors=cafe_colors), row=2, col=2)
# Tune layout and hover info
restyle!(fig, hoverinfo="label+percent+name", textinfo="none")
relayout!(fig, title_text="Van Gogh: 5 Most Prominent Colors Shown Proportionally",
showlegend=false)
@test !isempty(fig.data[1].domain_y)
@test !isempty(fig.data[1].domain_x)
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 3159 | @testset "DataFrame constructor" begin
df = DataFrame(x=1:10, y=sin.(1:10))
p = Plot(df, x=:x, y=:y)
@test p isa Plot
@test length(p.data) == 1
@test p.layout.xaxis_title == Dict(:text => :x)
@test p.layout.yaxis_title == Dict(:text => :y)
end
@testset "overriding yaxis_title" begin
df = DataFrame(x=1:10, y=sin.(1:10))
p = Plot(df, x=:x, y=:y, Layout(yaxis_title="sin(x)"));
@test p.layout.xaxis_title == Dict(:text => :x)
@test p.layout.yaxis_title == Dict(:text => "sin(x)")
end
@testset "overriding yaxis_title_text" begin
df = DataFrame(x=1:10, y=sin.(1:10))
p = Plot(df, x=:x, y=:y, Layout(yaxis_title_text="sin(x)"));
@test p.layout.xaxis_title == Dict(:text => :x)
@test p.layout.yaxis_title == Dict(:text => "sin(x)")
end
@testset "overriding yaxis_title with attr(text=, x=)" begin
df = DataFrame(x=1:10, y=sin.(1:10))
p = Plot(df, x=:x, y=:y, Layout(yaxis_title=attr(x=0.5, text="sin(x)")));
@test p.layout.xaxis_title == Dict(:text => :x)
@test p.layout.yaxis_title_text == "sin(x)"
@test p.layout.yaxis_title == Dict(:text => "sin(x)", :x => 0.5)
end
@testset "facet_row_wrap and facet_col_wrap" begin
df = stack(DataFrame(x=1:10, one=1, two=2, three=3, four=4, five=5, six=6, seven=7), Not(:x))
# _43 means 4 subplots in row 1 and 3 in row 2
p_43 = Plot(df, x=:x, y=:value, facet_row=:variable, facet_row_wrap=2)
@test size(p_43.layout.subplots.grid_ref) == (2, 4)
p_2221 = Plot(df, x=:x, y=:value, facet_col=:variable, facet_col_wrap=2)
@test size(p_2221.layout.subplots.grid_ref) == (4, 2)
p_7x7 = @test_logs (:warn, "cannot set facet_row and facet_col_wrap -- setting facet_col_wrap to missing") Plot(df, x=:x, y=:value, facet_col=:variable, facet_col_wrap=2, facet_row=:value)
@test size(p_7x7.layout.subplots.grid_ref) == (7, 7)
end
@testset "splom and dimensions" begin
df = DataFrame(d1=rand(10), d2=rand(10), d3=rand(10), color_col=vcat(fill("c1", 5), fill("c2", 5)))
p1 = Plot(df, dimensions=[:d1, :d2], kind="splom")
@test length(p1.data) == 1
trace = p1.data[1]
@test !trace.diagonal_visible
@test length(trace.dimensions) == 2
@test trace.dimensions[1].label == :d1
@test trace.dimensions[1].values == df[!, :d1]
@test trace.dimensions[2].label == :d2
@test trace.dimensions[2].values == df[!, :d2]
@test all(x -> x.axis_matches, trace.dimensions)
p2 = Plot(df, dimensions=[:d1, :d2], kind="splom", color=:color_col)
@test length(p2.data) == 2
for (trace, inds) in zip(p2.data, [1:5, 6:10])
@test !trace.diagonal_visible
@test length(trace.dimensions) == 2
@test trace.dimensions[1].label == :d1
@test trace.dimensions[1].values == df[inds, :d1]
@test trace.dimensions[2].label == :d2
@test trace.dimensions[2].values == df[inds, :d2]
@test all(x -> x.axis_matches, trace.dimensions)
end
end
@testset "_obtain_setindex_val for marker_size" begin
df = DataFrame(d1=rand(10), d2=rand(10), d3=rand(10))
p = Plot(df, x=:d1, y=:d2, marker_size=:d3)
@test p.data[1].marker_size == df.d3
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 9636 | @testset "test frame constructors" begin
f = M.frame(;name="test")
@test isa(f, M.PlotlyFrame)
f = M.PlotlyFrame{Dict{Symbol,Any}}(Dict{Symbol,Any}(:name=>"test"))
@test isa(f, M.PlotlyFrame)
@test_logs (:warn, "Frame should have a :name field for expected behavior") frame()
@test_logs (:warn, "Frame should have a :name field for expected behavior") PlotlyFrame{Dict{Symbol,Any}}(Dict{Symbol,Any}())
end
@testset "_UNDERSCORE_ATTRS" begin
f = M.frame(;name="test")
# test setindex!
f[:paper_bgcolor] = "grey"
@test haskey(f.fields, :paper_bgcolor)
@test f.fields[:paper_bgcolor] == "grey"
# test getindex
@test f[:paper_bgcolor] == "grey"
@test f["paper_bgcolor"] == "grey"
# now do it again with the string form of setindex!
f = M.Layout()
# test setindex!
f["paper_bgcolor"] = "grey"
@test haskey(f.fields, :paper_bgcolor)
@test f.fields[:paper_bgcolor] == "grey"
# test getindex
@test f[:paper_bgcolor] == "grey"
@test f["paper_bgcolor"] == "grey"
end
@testset "test setting nested attr" begin
f = M.frame(;name="test")
times20 = attr(name="Times", size=20)
f[:xaxis_titlefont] = times20
@test isa(f[:xaxis], Dict)
@test f[:xaxis][:titlefont][:name] == "Times"
@test f[:xaxis][:titlefont][:size] == 20
end
@testset "test setindex!, getindex methods" begin
f = M.frame(;name="test", x=1:5, y=1:5, visible=false)
f[:visible] = true
@test length(f.fields) == 4
@test haskey(f.fields, :visible)
@test f.fields[:visible] == true
# now try with string. Make sure it updates inplace
f["visible"] = false
@test length(f.fields) == 4
@test haskey(f.fields, :visible)
@test f.fields[:visible] == false
# -------- #
# 2 levels #
# -------- #
f[:line, :color] = "red"
@test length(f.fields) == 5
@test haskey(f.fields, :line)
@test isa(f.fields[:line], Dict)
@test f.fields[:line][:color] == "red"
@test f["line.color"] == "red"
# now try string version
f["line", "color"] = "blue"
@test length(f.fields) == 5
@test haskey(f.fields, :line)
@test isa(f.fields[:line], Dict)
@test f.fields[:line][:color] == "blue"
@test f["line_color"] == "blue"
# now try convenience string dot notation
f["line.color"] = "green"
@test length(f.fields) == 5
@test haskey(f.fields, :line)
@test isa(f.fields[:line], Dict)
@test f.fields[:line][:color] == "green"
@test f[:line_color] == "green"
# now try symbol with underscore
f[:(line_color)] = "orange"
@test length(f.fields) == 5
@test haskey(f.fields, :line)
@test isa(f.fields[:line], Dict)
@test f.fields[:line][:color] == "orange"
@test f["line.color"] == "orange"
# now try string with underscore
f["line_color"] = "magenta"
@test length(f.fields) == 5
@test haskey(f.fields, :line)
@test isa(f.fields[:line], Dict)
@test f.fields[:line][:color] == "magenta"
@test f["line.color"] == "magenta"
# -------- #
# 3 levels #
# -------- #
f[:marker, :line, :color] = "red"
@test length(f.fields) == 6
@test haskey(f.fields, :marker)
@test isa(f.fields[:marker], Dict)
@test haskey(f.fields[:marker], :line)
@test isa(f.fields[:marker][:line], Dict)
@test haskey(f.fields[:marker][:line], :color)
@test f.fields[:marker][:line][:color] == "red"
@test f["marker.line.color"] == "red"
# now try string version
f["marker", "line", "color"] = "blue"
@test length(f.fields) == 6
@test haskey(f.fields, :marker)
@test isa(f.fields[:marker], Dict)
@test haskey(f.fields[:marker], :line)
@test isa(f.fields[:marker][:line], Dict)
@test haskey(f.fields[:marker][:line], :color)
@test f.fields[:marker][:line][:color] == "blue"
@test f["marker.line.color"] == "blue"
# now try convenience string dot notation
f["marker.line.color"] = "green"
@test length(f.fields) == 6
@test haskey(f.fields, :marker)
@test isa(f.fields[:marker], Dict)
@test haskey(f.fields[:marker], :line)
@test isa(f.fields[:marker][:line], Dict)
@test haskey(f.fields[:marker][:line], :color)
@test f.fields[:marker][:line][:color] == "green"
@test f["marker.line.color"] == "green"
# now string with underscore notation
f["marker_line_color"] = "orange"
@test length(f.fields) == 6
@test haskey(f.fields, :marker)
@test isa(f.fields[:marker], Dict)
@test haskey(f.fields[:marker], :line)
@test isa(f.fields[:marker][:line], Dict)
@test haskey(f.fields[:marker][:line], :color)
@test f.fields[:marker][:line][:color] == "orange"
@test f["marker.line.color"] == "orange"
# now symbol with underscore notation
f[:(marker_line_color)] = "magenta"
@test length(f.fields) == 6
@test haskey(f.fields, :marker)
@test isa(f.fields[:marker], Dict)
@test haskey(f.fields[:marker], :line)
@test isa(f.fields[:marker][:line], Dict)
@test haskey(f.fields[:marker][:line], :color)
@test f.fields[:marker][:line][:color] == "magenta"
@test f["marker.line.color"] == "magenta"
# -------- #
# 4 levels #
# -------- #
f[:marker, :colorbar, :tickfont, :family] = "Hasklig-ExtraLight"
@test length(f.fields) == 6 # notice we didn't add another top level key
@test haskey(f.fields, :marker)
@test isa(f.fields[:marker], Dict)
@test length(f.fields[:marker]) == 2 # but we did add a key at this level
@test haskey(f.fields[:marker], :colorbar)
@test isa(f.fields[:marker][:colorbar], Dict)
@test haskey(f.fields[:marker][:colorbar], :tickfont)
@test isa(f.fields[:marker][:colorbar][:tickfont], Dict)
@test haskey(f.fields[:marker][:colorbar][:tickfont], :family)
@test f.fields[:marker][:colorbar][:tickfont][:family] == "Hasklig-ExtraLight"
@test f["marker.colorbar.tickfont.family"] == "Hasklig-ExtraLight"
# now try string version
f["marker", "colorbar", "tickfont", "family"] = "Hasklig-Light"
@test length(f.fields) == 6
@test haskey(f.fields, :marker)
@test isa(f.fields[:marker], Dict)
@test length(f.fields[:marker]) == 2
@test haskey(f.fields[:marker], :colorbar)
@test isa(f.fields[:marker][:colorbar], Dict)
@test haskey(f.fields[:marker][:colorbar], :tickfont)
@test isa(f.fields[:marker][:colorbar][:tickfont], Dict)
@test haskey(f.fields[:marker][:colorbar][:tickfont], :family)
@test f.fields[:marker][:colorbar][:tickfont][:family] == "Hasklig-Light"
@test f["marker.colorbar.tickfont.family"] == "Hasklig-Light"
# now try convenience string dot notation
f["marker.colorbar.tickfont.family"] = "Hasklig-Medium"
@test length(f.fields) == 6 # notice we didn't add another top level key
@test haskey(f.fields, :marker)
@test isa(f.fields[:marker], Dict)
@test length(f.fields[:marker]) == 2 # but we did add a key at this level
@test haskey(f.fields[:marker], :colorbar)
@test isa(f.fields[:marker][:colorbar], Dict)
@test haskey(f.fields[:marker][:colorbar], :tickfont)
@test isa(f.fields[:marker][:colorbar][:tickfont], Dict)
@test haskey(f.fields[:marker][:colorbar][:tickfont], :family)
@test f.fields[:marker][:colorbar][:tickfont][:family] == "Hasklig-Medium"
@test f["marker.colorbar.tickfont.family"] == "Hasklig-Medium"
# now string with underscore notation
f["marker_colorbar_tickfont_family"] = "Webdings"
@test length(f.fields) == 6 # notice we didn't add another top level key
@test haskey(f.fields, :marker)
@test isa(f.fields[:marker], Dict)
@test length(f.fields[:marker]) == 2 # but we did add a key at this level
@test haskey(f.fields[:marker], :colorbar)
@test isa(f.fields[:marker][:colorbar], Dict)
@test haskey(f.fields[:marker][:colorbar], :tickfont)
@test isa(f.fields[:marker][:colorbar][:tickfont], Dict)
@test haskey(f.fields[:marker][:colorbar][:tickfont], :family)
@test f.fields[:marker][:colorbar][:tickfont][:family] == "Webdings"
@test f["marker.colorbar.tickfont.family"] == "Webdings"
# now symbol with underscore notation
f[:marker_colorbar_tickfont_family] = "Webdings42"
@test length(f.fields) == 6 # notice we didn't add another top level key
@test haskey(f.fields, :marker)
@test isa(f.fields[:marker], Dict)
@test length(f.fields[:marker]) == 2 # but we did add a key at this level
@test haskey(f.fields[:marker], :colorbar)
@test isa(f.fields[:marker][:colorbar], Dict)
@test haskey(f.fields[:marker][:colorbar], :tickfont)
@test isa(f.fields[:marker][:colorbar][:tickfont], Dict)
@test haskey(f.fields[:marker][:colorbar][:tickfont], :family)
@test f.fields[:marker][:colorbar][:tickfont][:family] == "Webdings42"
@test f["marker.colorbar.tickfont.family"] == "Webdings42"
# error on 5 levels
@test_throws MethodError f["marker.colorbar.tickfont.family.foo"] = :bar
end
@testset "create plot with frames" begin
# https://plotly.com/python/animations/
p = Plot(
[scatter(x=0:1, y=0:1)],
Layout(xaxis=attr(range=(0,5), autorange=false), yaxis=attr(range=(0,5), autorange=false), title="start title", updatemenus=[attr(type="buttons", buttons=[attr(label="Play", method="animate", args=[nothing])])]),
[
frame(name=:f1, data=[scatter(x=1:2, y=1:2)]),
frame(name=:f2, data=[scatter(x=1:4, y=1:4)]),
frame(name=:f3, data=[scatter(x=3:4, y=3:4)], layout=Layout(title_text="End Title")),
]
)
@test p isa Plot
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 451 |
gt = PlotlyBase.GenericTrace("scatter"; x=1:10, y=(1:10).^2)
pplot = PlotlyBase.Plot(gt)
layout = PlotlyBase.Layout()
using PlotlyBase.JSON, JSON3
@testset "Convert to json" begin
@test JSON.json(gt) == "{\"y\":[1,4,9,16,25,36,49,64,81,100],\"type\":\"scatter\",\"x\":[1,2,3,4,5,6,7,8,9,10]}"
@test JSON.json(gt) == JSON3.write(gt)
@test JSON.json(pplot) == JSON3.write(pplot)
@test JSON.json(layout) == JSON3.write(layout)
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 1393 | @testset "recession_bands" begin
p1 = Plot(scatter(x=1:10, y=rand(10)))
@test PlotlyBase._recession_band_shapes(p1) === nothing
_path = joinpath(@__DIR__, "data", "us_manu_unemp.csv")
_with_dates_raw = readdlm(_path, ',')
with_dates = _with_dates_raw[2:end, :]
with_dates[:, 1] = map(Date, with_dates[:, 1])
p2 = Plot(scatter(x=with_dates[:, 1], y=with_dates[:, 2]))
@test PlotlyBase._recession_band_shapes(p2) !== nothing
@test length(PlotlyBase._recession_band_shapes(p2)) == 7
p3 = Plot(scatter(x=with_dates[:, 1], y=with_dates[:, 3]))
@test PlotlyBase._recession_band_shapes(p3) !== nothing
@test length(PlotlyBase._recession_band_shapes(p3)) == 7
p12 = [p1; p2]
@test PlotlyBase._recession_band_shapes(p12) !== nothing
@test length(PlotlyBase._recession_band_shapes(p12)) == 7
p123 = [p1; p2; p3]
@test PlotlyBase._recession_band_shapes(p123) !== nothing
@test length(PlotlyBase._recession_band_shapes(p123)) == 14
add_recession_bands!(p1)
@test length(p1.layout["shapes"]) == 0
add_recession_bands!(p123)
@test length(p123.layout["shapes"]) == 14
p23 = [p2; p3]
china_WTO_date = Date(2001, 12, 11)
relayout!(
p23,
shapes=vline([china_WTO_date], line_color="red", opacity=0.4),
)
add_recession_bands!(p23)
@test length(p23.layout["shapes"]) == 15
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 968 | module PlotlyBaseTest
using Test
using Dates
using DataFrames
@static if VERSION >= v"0.7.0-alpha"
using DelimitedFiles:readdlm
end
using PlotlyBase
const M = PlotlyBase
# copied from TestSetExtensions.@includetests
macro includetests(testarg...)
if length(testarg) == 0
tests = []
elseif length(testarg) == 1
tests = testarg[1]
else
error("@includetests takes zero or one argument")
end
quote
tests = $tests
rootfile = @__FILE__
if length(tests) == 0
tests = readdir(dirname(rootfile))
tests = filter(f -> endswith(f, ".jl") && f != basename(rootfile), tests)
else
tests = map(f -> string(f, ".jl"), tests)
end
println();
for test in tests
print(splitext(test)[1], ": ")
include(test)
println()
end
end
end
@testset "PlotlyJS Tests" begin
@includetests ARGS
end
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 2283 | @testset "Subplots" begin
@testset "rows=1, cols=2" begin
have1 = Layout(Subplots(rows=1, cols=2))
# shared_xaxes does nothing with 1 column
have2 = Layout(Subplots(rows=1, cols=2, shared_xaxes=false))
for have in (have1, have2)
@test have[:xaxis] == attr(anchor="y", domain=(0.0, 0.45)).fields
@test have[:yaxis] == attr(anchor="x", domain=(0.0, 1.0)).fields
@test have[:xaxis2] == attr(anchor="y2", domain=(0.55, 1.0)).fields
@test have[:yaxis2] == attr(anchor="x2", domain=(0.0, 1.0)).fields
end
end
@testset "rows=1, cols=2, shared_yaxes=true" begin
have = Layout(Subplots(rows=1, cols=2, shared_yaxes=true))
@test have[:xaxis] == attr(anchor="y", domain=(0.0, 0.45)).fields
@test have[:yaxis] == attr(anchor="x", domain=(0.0, 1.0)).fields
@test have[:xaxis2] == attr(anchor="y2", domain=(0.55, 1.0)).fields
@test have[:yaxis2] == attr(anchor="x2", domain=(0.0, 1.0), matches="y", showticklabels=false).fields
end
@testset "rows=2, cols=1" begin
have1 = Layout(Subplots(rows=2, cols=1))
# shared_yaxes does nothing with 1 column
have2 = Layout(Subplots(rows=2, cols=1, shared_yaxes=true))
for have in (have1, have2)
@test have[:xaxis] == attr(anchor="y", domain=(0.0, 1.0)).fields
@test have[:yaxis] == attr(anchor="x", domain=(0.575, 1.0)).fields
@test have[:xaxis2] == attr(anchor="y2", domain=(0.0, 1.0)).fields
@test have[:yaxis2] == attr(anchor="x2", domain=(0.0, 0.425)).fields
end
end
@testset "rows=2, cols=1, shared_yaxes=true" begin
have = Layout(Subplots(rows=2, cols=1, shared_xaxes=true))
@test have[:xaxis] == attr(anchor="y", domain=(0.0, 1.0), matches="x2", showticklabels=false).fields
@test have[:yaxis] == attr(anchor="x", domain=(0.575, 1.0)).fields
@test have[:xaxis2] == attr(anchor="y2", domain=(0.0, 1.0)).fields
@test have[:yaxis2] == attr(anchor="x2", domain=(0.0, 0.425)).fields
end
@testset "can get subplot kind for trace type" begin
@test PlotlyBase.get_subplotkind_from_trace_type.(PlotlyBase._TRACE_TYPES) isa Vector{String}
end
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 1831 | make_t1() = Template(data=attr(
scatter=[attr(marker_color="red"), attr(marker_color="blue")]
))
make_t2() = Template(data=attr(
scatter=[attr(marker_line_color="black")],
bar=[attr(marker_color="cyan")]
))
make_t3() = merge(make_t1(), make_t2())
@testset "Templates" begin
@testset "constructor with data::PlotlyAttribute" begin
t1 = make_t1()
@test t1 isa Template
@test isempty(t1.layout)
@test length(t1.data[:scatter]) == 2
end
@testset "merge templates" begin
t1 = make_t1()
t2 = make_t2()
t3 = merge(t1, t2)
# test that neither t1 nor t2 were modified
@test length(t1.data[:scatter]) == 2
@test length(t2.data[:scatter]) == 1
@test !haskey(t1.data, :bar)
# test that updates were applied
@test length(t3.data[:scatter]) == 2
@test t3.data[:scatter][1] == attr(marker=attr(color="red", line_color="black"))
@test t3.data[:scatter][2] == attr(marker_color="blue", marker_line_color="black")
@test t3.data[:bar][1] == attr(marker_color="cyan")
end
@testset "Can save Template to `templates` object" begin
t1 = make_t1()
templates.foobar = t1
@test haskey(templates.templates, :foobar)
# clean up
pop!(templates.templates, :foobar)
end
@testset "Multiple templates via `+` syntax" begin
t1 = make_t1()
t2 = make_t2()
templates.t1 = t1
templates.t2 = t2
@test templates["t1+t2"] == merge(t1, t2)
# clean up
pop!(templates.templates, :t1)
pop!(templates.templates, :t2)
end
@testset "loading known templates from file" begin
@test templates["ggplot2"] isa Template
@test templates["plotly_dark"] isa Template
end
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | code | 11166 |
gt = M.GenericTrace("scatter"; x=1:10, y=sin.(1:10))
@testset "test constructors" begin
@test sort(collect(keys(gt.fields))) == [:type, :x, :y]
end
@testset "_UNDERSCORE_ATTRS" begin
l = M.Layout()
# test setindex!
l[:paper_bgcolor] = "grey"
@test haskey(l.fields, :paper_bgcolor)
@test l.fields[:paper_bgcolor] == "grey"
# test getindex
@test l[:paper_bgcolor] == "grey"
@test l["paper_bgcolor"] == "grey"
# now do it again with the string form of setindex!
l = M.Layout()
# test setindex!
l["paper_bgcolor"] = "grey"
@test haskey(l.fields, :paper_bgcolor)
@test l.fields[:paper_bgcolor] == "grey"
# test getindex
@test l[:paper_bgcolor] == "grey"
@test l["paper_bgcolor"] == "grey"
end
@testset "test setindex!, getindex methods" begin
gt[:visible] = true
@test length(gt.fields) == 4
@test haskey(gt.fields, :visible)
@test gt.fields[:visible] == true
# now try with string. Make sure it updates inplace
gt["visible"] = false
@test length(gt.fields) == 4
@test haskey(gt.fields, :visible)
@test gt.fields[:visible] == false
# -------- #
# 2 levels #
# -------- #
gt[:line, :color] = "red"
@test length(gt.fields) == 5
@test haskey(gt.fields, :line)
@test isa(gt.fields[:line], Dict)
@test gt.fields[:line][:color] == "red"
@test gt["line.color"] == "red"
# now try string version
gt["line", "color"] = "blue"
@test length(gt.fields) == 5
@test haskey(gt.fields, :line)
@test isa(gt.fields[:line], Dict)
@test gt.fields[:line][:color] == "blue"
@test gt["line_color"] == "blue"
# now try convenience string dot notation
gt["line.color"] = "green"
@test length(gt.fields) == 5
@test haskey(gt.fields, :line)
@test isa(gt.fields[:line], Dict)
@test gt.fields[:line][:color] == "green"
@test gt[:line_color] == "green"
# now try symbol with underscore
gt[:(line_color)] = "orange"
@test length(gt.fields) == 5
@test haskey(gt.fields, :line)
@test isa(gt.fields[:line], Dict)
@test gt.fields[:line][:color] == "orange"
@test gt["line.color"] == "orange"
# now try string with underscore
gt["line_color"] = "magenta"
@test length(gt.fields) == 5
@test haskey(gt.fields, :line)
@test isa(gt.fields[:line], Dict)
@test gt.fields[:line][:color] == "magenta"
@test gt["line.color"] == "magenta"
# -------- #
# 3 levels #
# -------- #
gt[:marker, :line, :color] = "red"
@test length(gt.fields) == 6
@test haskey(gt.fields, :marker)
@test isa(gt.fields[:marker], Dict)
@test haskey(gt.fields[:marker], :line)
@test isa(gt.fields[:marker][:line], Dict)
@test haskey(gt.fields[:marker][:line], :color)
@test gt.fields[:marker][:line][:color] == "red"
@test gt["marker.line.color"] == "red"
# now try string version
gt["marker", "line", "color"] = "blue"
@test length(gt.fields) == 6
@test haskey(gt.fields, :marker)
@test isa(gt.fields[:marker], Dict)
@test haskey(gt.fields[:marker], :line)
@test isa(gt.fields[:marker][:line], Dict)
@test haskey(gt.fields[:marker][:line], :color)
@test gt.fields[:marker][:line][:color] == "blue"
@test gt["marker.line.color"] == "blue"
# now try convenience string dot notation
gt["marker.line.color"] = "green"
@test length(gt.fields) == 6
@test haskey(gt.fields, :marker)
@test isa(gt.fields[:marker], Dict)
@test haskey(gt.fields[:marker], :line)
@test isa(gt.fields[:marker][:line], Dict)
@test haskey(gt.fields[:marker][:line], :color)
@test gt.fields[:marker][:line][:color] == "green"
@test gt["marker.line.color"] == "green"
# now string with underscore notation
gt["marker_line_color"] = "orange"
@test length(gt.fields) == 6
@test haskey(gt.fields, :marker)
@test isa(gt.fields[:marker], Dict)
@test haskey(gt.fields[:marker], :line)
@test isa(gt.fields[:marker][:line], Dict)
@test haskey(gt.fields[:marker][:line], :color)
@test gt.fields[:marker][:line][:color] == "orange"
@test gt["marker.line.color"] == "orange"
# now symbol with underscore notation
gt[:(marker_line_color)] = "magenta"
@test length(gt.fields) == 6
@test haskey(gt.fields, :marker)
@test isa(gt.fields[:marker], Dict)
@test haskey(gt.fields[:marker], :line)
@test isa(gt.fields[:marker][:line], Dict)
@test haskey(gt.fields[:marker][:line], :color)
@test gt.fields[:marker][:line][:color] == "magenta"
@test gt["marker.line.color"] == "magenta"
# -------- #
# 4 levels #
# -------- #
gt[:marker, :colorbar, :tickfont, :family] = "Hasklig-ExtraLight"
@test length(gt.fields) == 6 # notice we didn't add another top level key
@test haskey(gt.fields, :marker)
@test isa(gt.fields[:marker], Dict)
@test length(gt.fields[:marker]) == 2 # but we did add a key at this level
@test haskey(gt.fields[:marker], :colorbar)
@test isa(gt.fields[:marker][:colorbar], Dict)
@test haskey(gt.fields[:marker][:colorbar], :tickfont)
@test isa(gt.fields[:marker][:colorbar][:tickfont], Dict)
@test haskey(gt.fields[:marker][:colorbar][:tickfont], :family)
@test gt.fields[:marker][:colorbar][:tickfont][:family] == "Hasklig-ExtraLight"
@test gt["marker.colorbar.tickfont.family"] == "Hasklig-ExtraLight"
# now try string version
gt["marker", "colorbar", "tickfont", "family"] = "Hasklig-Light"
@test length(gt.fields) == 6
@test haskey(gt.fields, :marker)
@test isa(gt.fields[:marker], Dict)
@test length(gt.fields[:marker]) == 2
@test haskey(gt.fields[:marker], :colorbar)
@test isa(gt.fields[:marker][:colorbar], Dict)
@test haskey(gt.fields[:marker][:colorbar], :tickfont)
@test isa(gt.fields[:marker][:colorbar][:tickfont], Dict)
@test haskey(gt.fields[:marker][:colorbar][:tickfont], :family)
@test gt.fields[:marker][:colorbar][:tickfont][:family] == "Hasklig-Light"
@test gt["marker.colorbar.tickfont.family"] == "Hasklig-Light"
# now try convenience string dot notation
gt["marker.colorbar.tickfont.family"] = "Hasklig-Medium"
@test length(gt.fields) == 6 # notice we didn't add another top level key
@test haskey(gt.fields, :marker)
@test isa(gt.fields[:marker], Dict)
@test length(gt.fields[:marker]) == 2 # but we did add a key at this level
@test haskey(gt.fields[:marker], :colorbar)
@test isa(gt.fields[:marker][:colorbar], Dict)
@test haskey(gt.fields[:marker][:colorbar], :tickfont)
@test isa(gt.fields[:marker][:colorbar][:tickfont], Dict)
@test haskey(gt.fields[:marker][:colorbar][:tickfont], :family)
@test gt.fields[:marker][:colorbar][:tickfont][:family] == "Hasklig-Medium"
@test gt["marker.colorbar.tickfont.family"] == "Hasklig-Medium"
# now string with underscore notation
gt["marker_colorbar_tickfont_family"] = "Webdings"
@test length(gt.fields) == 6 # notice we didn't add another top level key
@test haskey(gt.fields, :marker)
@test isa(gt.fields[:marker], Dict)
@test length(gt.fields[:marker]) == 2 # but we did add a key at this level
@test haskey(gt.fields[:marker], :colorbar)
@test isa(gt.fields[:marker][:colorbar], Dict)
@test haskey(gt.fields[:marker][:colorbar], :tickfont)
@test isa(gt.fields[:marker][:colorbar][:tickfont], Dict)
@test haskey(gt.fields[:marker][:colorbar][:tickfont], :family)
@test gt.fields[:marker][:colorbar][:tickfont][:family] == "Webdings"
@test gt["marker.colorbar.tickfont.family"] == "Webdings"
# now symbol with underscore notation
gt[:marker_colorbar_tickfont_family] = "Webdings42"
@test length(gt.fields) == 6 # notice we didn't add another top level key
@test haskey(gt.fields, :marker)
@test isa(gt.fields[:marker], Dict)
@test length(gt.fields[:marker]) == 2 # but we did add a key at this level
@test haskey(gt.fields[:marker], :colorbar)
@test isa(gt.fields[:marker][:colorbar], Dict)
@test haskey(gt.fields[:marker][:colorbar], :tickfont)
@test isa(gt.fields[:marker][:colorbar][:tickfont], Dict)
@test haskey(gt.fields[:marker][:colorbar][:tickfont], :family)
@test gt.fields[:marker][:colorbar][:tickfont][:family] == "Webdings42"
@test gt["marker.colorbar.tickfont.family"] == "Webdings42"
# error on 5 levels
@test_throws MethodError gt["marker.colorbar.tickfont.family.foo"] = :bar
end
@testset "testing underscore constructor" begin
# now test underscore constructor and see if it matches gt
gt2 = M.scatter(;x=1:10, y=sin.(1:10),
marker_colorbar_tickfont_family="Webdings42",
marker_line_color="magenta",
line_color="magenta",
visible=false)
@test sort(collect(keys(gt.fields))) == sort(collect(keys(gt2.fields)))
for k in keys(gt.fields)
@test gt[k] == gt2[k]
end
end
@testset "testing vline hline" begin
vl = vline(0)
@test vl["x0"] == 0
@test vl["y0"] == 0
@test vl["y1"] == 1
@test vl["type"] == "line"
@test vl["xref"] == "x"
@test vl["yref"] == "paper"
hl = hline(0)
@test hl["y0"] == 0
@test hl["x0"] == 0
@test hl["x1"] == 1
@test hl["type"] == "line"
@test hl["yref"] == "y"
@test hl["xref"] == "paper"
av, b1v, b2v = 1:3, 5:7, 9:11
_want_i(x::Number, i) = x
_want_i(x::AbstractVector, i) = x[i]
for (a, b1, b2) in [(av, 1, 2),
(av, b1v, 2),
(av, b1v, b2v),
(av, 1, b2v),
(0, b1v, 2),
(0, b1v, b2v),
(0, 1, b2v)]
vl = vline(a, b1, b2)
for (i, v) in enumerate(vl)
@test v["x0"] == _want_i(a, i)
@test v["y0"] == _want_i(b1, i)
@test v["y1"] == _want_i(b2, i)
end
hl = hline(a, b1, b2)
for (i, h) in enumerate(hl)
@test h["y0"] == _want_i(a, i)
@test h["x0"] == _want_i(b1, i)
@test h["x1"] == _want_i(b2, i)
end
end
end
@testset "test other shapes" begin
# constructor logic was taken care of above. Just need to test
# rect/path/circle specfic things here
r = rect(1, 2, 3, 4)
@test r["type"] == "rect"
c = circle(1, 2, 3, 4)
@test c["type"] == "circle"
_path = "M 1 1 L 1 3 L 4 1 Z"
p = path(_path)
@test p["type"] == "path"
@test p["path"] == _path
end
@testset "test setting nested attr" begin
l = Layout()
times20 = attr(name="Times", size=20)
l[:xaxis_titlefont] = times20
@test isa(l[:xaxis], Dict)
@test l[:xaxis][:titlefont][:name] == "Times"
@test l[:xaxis][:titlefont][:size] == 20
end
@testset "test margin" begin
l1 = Layout(;margin=attr(l=120, t=120, b=120, r=120))
l2 = Layout(;margin=attr(l=60, t=60, b=60, r=60))
@test l1[:margin] == Dict(:l => 120,:b => 120,:r => 120,:t => 120)
@test l2[:margin] == Dict(:l => 60,:b => 60,:r => 60,:t => 60)
end
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | docs | 6615 | # Changelog
## [v0.4.1](https://github.com/sglyon/PlotlyBase.jl/tree/v0.4.1) (2020-08-19)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.4.0...v0.4.1)
**Merged pull requests:**
- TEST: add tests for kaleido. Also add mimetype support [\#30](https://github.com/sglyon/PlotlyBase.jl/pull/30) ([sglyon](https://github.com/sglyon))
- Julia Package Butler Updates [\#28](https://github.com/sglyon/PlotlyBase.jl/pull/28) ([github-actions[bot]](https://github.com/apps/github-actions))
- CompatHelper: bump compat for "JSON" to "0.21" [\#27](https://github.com/sglyon/PlotlyBase.jl/pull/27) ([github-actions[bot]](https://github.com/apps/github-actions))
- First pass at updating API for allowing animations [\#21](https://github.com/sglyon/PlotlyBase.jl/pull/21) ([nicrummel](https://github.com/nicrummel))
## [v0.4.0](https://github.com/sglyon/PlotlyBase.jl/tree/v0.4.0) (2020-08-12)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.3.1...v0.4.0)
**Merged pull requests:**
- CompatHelper: add new compat entry for "Requires" at version "1.0" [\#26](https://github.com/sglyon/PlotlyBase.jl/pull/26) ([github-actions[bot]](https://github.com/apps/github-actions))
- CompatHelper: add new compat entry for "DocStringExtensions" at version "0.8" [\#25](https://github.com/sglyon/PlotlyBase.jl/pull/25) ([github-actions[bot]](https://github.com/apps/github-actions))
- CompatHelper: add new compat entry for "LaTeXStrings" at version "1.1" [\#24](https://github.com/sglyon/PlotlyBase.jl/pull/24) ([github-actions[bot]](https://github.com/apps/github-actions))
- ENH: integrate kaleido to save/export figures [\#23](https://github.com/sglyon/PlotlyBase.jl/pull/23) ([sglyon](https://github.com/sglyon))
## [v0.3.1](https://github.com/sglyon/PlotlyBase.jl/tree/v0.3.1) (2020-05-25)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.3.0...v0.3.1)
**Merged pull requests:**
- Update api.jl [\#20](https://github.com/sglyon/PlotlyBase.jl/pull/20) ([jonas-kr](https://github.com/jonas-kr))
- Install TagBot as a GitHub Action [\#19](https://github.com/sglyon/PlotlyBase.jl/pull/19) ([JuliaTagBot](https://github.com/JuliaTagBot))
## [v0.3.0](https://github.com/sglyon/PlotlyBase.jl/tree/v0.3.0) (2019-10-03)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.2.7...v0.3.0)
## [v0.2.7](https://github.com/sglyon/PlotlyBase.jl/tree/v0.2.7) (2019-10-03)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.2.6...v0.2.7)
**Merged pull requests:**
- allow for datetime type in x axis [\#18](https://github.com/sglyon/PlotlyBase.jl/pull/18) ([kafisatz](https://github.com/kafisatz))
- Fix DataFrames v0.19 deprecations [\#17](https://github.com/sglyon/PlotlyBase.jl/pull/17) ([alyst](https://github.com/alyst))
## [v0.2.6](https://github.com/sglyon/PlotlyBase.jl/tree/v0.2.6) (2019-05-31)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.2.5...v0.2.6)
**Closed issues:**
- Inconsistencies in use of styles [\#15](https://github.com/sglyon/PlotlyBase.jl/issues/15)
- Configuration Options [\#2](https://github.com/sglyon/PlotlyBase.jl/issues/2)
**Merged pull requests:**
- add margin to each style and update \(gadfly\_dark\) [\#16](https://github.com/sglyon/PlotlyBase.jl/pull/16) ([asbisen](https://github.com/asbisen))
## [v0.2.5](https://github.com/sglyon/PlotlyBase.jl/tree/v0.2.5) (2018-11-03)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.2.4...v0.2.5)
**Closed issues:**
- Quick fix \(I promise\) [\#9](https://github.com/sglyon/PlotlyBase.jl/issues/9)
**Merged pull requests:**
- add STYLES to export for listing STYLES [\#14](https://github.com/sglyon/PlotlyBase.jl/pull/14) ([asbisen](https://github.com/asbisen))
- change min/max to min./max. in stem [\#13](https://github.com/sglyon/PlotlyBase.jl/pull/13) ([sam81](https://github.com/sam81))
- Remove `init\_notebook` export [\#12](https://github.com/sglyon/PlotlyBase.jl/pull/12) ([staticfloat](https://github.com/staticfloat))
- Updating the warn function for Julia v1.0 [\#11](https://github.com/sglyon/PlotlyBase.jl/pull/11) ([ghuba](https://github.com/ghuba))
## [v0.2.4](https://github.com/sglyon/PlotlyBase.jl/tree/v0.2.4) (2018-09-28)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.2.3...v0.2.4)
**Merged pull requests:**
- linspace -\> LinRange [\#10](https://github.com/sglyon/PlotlyBase.jl/pull/10) ([ghuba](https://github.com/ghuba))
## [v0.2.3](https://github.com/sglyon/PlotlyBase.jl/tree/v0.2.3) (2018-09-18)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.2.2...v0.2.3)
**Merged pull requests:**
- Remove deprecated usage of Void [\#7](https://github.com/sglyon/PlotlyBase.jl/pull/7) ([VPetukhov](https://github.com/VPetukhov))
## [v0.2.2](https://github.com/sglyon/PlotlyBase.jl/tree/v0.2.2) (2018-09-05)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.2.1...v0.2.2)
**Closed issues:**
- Clearing all traces [\#5](https://github.com/sglyon/PlotlyBase.jl/issues/5)
**Merged pull requests:**
- Fix require warnings [\#6](https://github.com/sglyon/PlotlyBase.jl/pull/6) ([cstjean](https://github.com/cstjean))
## [v0.2.1](https://github.com/sglyon/PlotlyBase.jl/tree/v0.2.1) (2018-08-28)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.2.0...v0.2.1)
**Closed issues:**
- Error tagging new release [\#4](https://github.com/sglyon/PlotlyBase.jl/issues/4)
## [v0.2.0](https://github.com/sglyon/PlotlyBase.jl/tree/v0.2.0) (2018-08-24)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.1.2...v0.2.0)
**Merged pull requests:**
- Julia 0.7 compatibility [\#3](https://github.com/sglyon/PlotlyBase.jl/pull/3) ([simonfxr](https://github.com/simonfxr))
## [v0.1.2](https://github.com/sglyon/PlotlyBase.jl/tree/v0.1.2) (2018-06-23)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.1.1...v0.1.2)
**Merged pull requests:**
- fix some docstrings [\#1](https://github.com/sglyon/PlotlyBase.jl/pull/1) ([CarloLucibello](https://github.com/CarloLucibello))
## [v0.1.1](https://github.com/sglyon/PlotlyBase.jl/tree/v0.1.1) (2018-02-05)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/v0.1.0...v0.1.1)
## [v0.1.0](https://github.com/sglyon/PlotlyBase.jl/tree/v0.1.0) (2018-01-29)
[Full Changelog](https://github.com/sglyon/PlotlyBase.jl/compare/aaa86b892a9789c2b8d3f492860156d99e84c5a0...v0.1.0)
\* *This Changelog was automatically generated by [github_changelog_generator](https://github.com/github-changelog-generator/github-changelog-generator)*
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | docs | 953 | # PlotlyBase
[](https://travis-ci.org/sglyon/PlotlyBase.jl)
[](https://coveralls.io/github/sglyon/PlotlyBase.jl?branch=master)
[](http://codecov.io/github/sglyon/PlotlyBase.jl?branch=master)
This is the plot making guts of [PlotlyJS.jl](https://github.com/JuliaPlots/PlotlyJS.jl)
Docs for this package are forthcoming, but check out the following sections of the PlotlyJS.jl docs (or just use PlotlyJS.jl):
- [Preliminaries](http://juliaplots.org/PlotlyJS.jl/stable/basics/)
- [Building Blocks](http://juliaplots.org/PlotlyJS.jl/stable/building_traces_layouts/)
- [Putting it together](http://juliaplots.org/PlotlyJS.jl/stable/syncplots/) up until the discussion of syncplots.
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.8.19 | 56baf69781fc5e61607c3e46227ab17f7040ffa2 | docs | 503 | ```@meta
CurrentModule = PlotlyBase
```
# PlotlyBase.jl API Documentation
Welcome to the PlotlyBase.jl API documentation. On this page we include all
docstrings found in PlotlyBase.jl.
## Navigation
The following types are documented:
```@index
Modules = [PlotlyBase]
Order = [:type]
```
The following functions are documented
```@index
Modules = [PlotlyBase]
Order = [:function]
```
## Types
```@autodocs
Modules = [PlotlyBase]
Order = [:module, :constant, :type, :function, :macro]
```
| PlotlyBase | https://github.com/sglyon/PlotlyBase.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 473 | #! julia --startup-file=no
using Pkg
Pkg.activate(@__DIR__)
Pkg.develop(PackageSpec(path=dirname(@__DIR__))) # adds the package this script is called from
Pkg.instantiate()
Pkg.update()
using LiveServer
@async serve(dir=joinpath(@__DIR__, "build"))
run = true
while run
try
include("make.jl")
catch e
@info "make.jl error" e
end
println("Run again? Enter! Exit with 'q'.")
if readline() == "q"
global run = false
end
end
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 2096 | using GraphMakie
using Documenter
using Literate
using CairoMakie
# preload the deps from the examples to supress precompilation output in docs
using JSServe
using NetworkDynamics
using LayeredLayouts
using Graphs
using RegistryInstances
DocMeta.setdocmeta!(GraphMakie, :DocTestSetup, :(using GraphMakie); recursive=true)
# generate examples
example_dir = joinpath(@__DIR__, "examples")
outdir = joinpath(@__DIR__, "src", "generated")
isdir(outdir) && rm(outdir, recursive=true)
mkpath(outdir)
for example in filter(contains(r".jl$"), readdir(example_dir, join=true))
Literate.markdown(example, outdir;
preprocess=c->replace(c, "@save_reference " => ""))
end
makedocs(; modules=[GraphMakie], authors="Simon Danisch, Hans Würfel",
repo="https://github.com/MakieOrg/GraphMakie.jl/blob/{commit}{path}#{line}",
sitename="GraphMakie.jl",
format=Documenter.HTML(; prettyurls=get(ENV, "CI", "false") == "true",
canonical="https://graph.makie.org", assets=String[],
size_threshold_ignore=["generated/plots.md"]),
pages=["Home" => "index.md",
"Examples" => [
"Feature Walkthrough" => "generated/plots.md",
"Interaction Examples" => "generated/interactions.md",
"Dependency Graph" => "generated/depgraph.md",
"Stress on Truss" => "generated/truss.md",
"Julia AST" => "generated/syntaxtree.md",
"Makie Scene tree" => "generated/scenegraph.md",
"Decision Tree" => "generated/decisiontree.md",
"Reference Tests" => "generated/reftests.md",
],
"🔗 Layouts (`NetworkLayout.jl`)" => "networklayout_forward.md",
],
warnonly=[:missing_docs])
# if gh_pages branch gets to big, check out
# https://juliadocs.github.io/Documenter.jl/stable/man/hosting/#gh-pages-Branch
deploydocs(;repo="github.com/MakieOrg/GraphMakie.jl",
push_preview=true)
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 4526 |
# # Visual representation of a decision tree
# In this example we are going to plot a decision tree of type [`DecisionTree.jl`](https://github.com/JuliaAI/DecisionTree.jl) using the
# Bucheim Layout from [`NetworkLayout.jl`](https://github.com/JuliaGraphs/NetworkLayout.jl).
using CairoMakie
using Graphs
using GraphMakie
import MLJ
using NetworkLayout
using DecisionTree
# This following code, which walks the tree and creates a `SimpleDiGraph` was taken and slightly modified from [`syntaxtree.jl`](https://github.com/MakieOrg/GraphMakie.jl/blob/master/docs/examples/syntaxtree.jl). Thanks!
# The model is a DecisionTree object.
# maxdepth defines the max Depth of the final tree generated.
import Base.convert
function Base.convert(::Type{SimpleDiGraph},model::DecisionTree.DecisionTreeClassifier; maxdepth=depth(model))
if maxdepth == -1
maxdepth = depth(model)
end
g = SimpleDiGraph()
properties = Any[]
walk_tree!(model.root.node,g,maxdepth,properties)
return g, properties
end
function walk_tree!(node::DecisionTree.Node, g, depthLeft, properties)
add_vertex!(g)
if depthLeft == 0
push!(properties,(Nothing,"..."))
return vertices(g)[end]
else
depthLeft -= 1
end
current_vertex = vertices(g)[end]
val = node.featval
featval = isa(val,AbstractString) ? val : round(val;sigdigits=2)
push!(properties,(Node,"Feature $(node.featid) < $featval ?"))
child = walk_tree!(node.left,g,depthLeft,properties)
add_edge!(g,current_vertex,child)
child = walk_tree!(node.right,g,depthLeft,properties)
add_edge!(g,current_vertex,child)
return current_vertex
end
function walk_tree!(leaf::DecisionTree.Leaf, g, depthLeft, properties)
add_vertex!(g)
n_matches = count(leaf.values .== leaf.majority)
#ratio = string(n_matches, "/", length(leaf.values))
push!(properties,(Leaf,"$(leaf.majority)"))# : $(ratio)"))
return vertices(g)[end]
end
nothing #hide
# Ooof, quite a bit of code!
# ## Makie @recipe
# Now let's define a MakieRecipe for the plot, to make plotting easy
@recipe(PlotDecisionTree) do scene
Attributes(
nodecolormap = :darktest,
textcolor = RGBf(0.5,0.5,0.5),
leafcolor = :darkgreen,
nodecolor = :white,
maxdepth = -1,
)
end
import GraphMakie.graphplot
import Makie.plot!
function GraphMakie.graphplot(model::DecisionTreeClassifier;kwargs...)
f,ax,h = plotdecisiontree(model;kwargs...)
hidedecorations!(ax); hidespines!(ax)
return f
end
function plot!(plt::PlotDecisionTree{<:Tuple{DecisionTreeClassifier}})
@extract plt leafcolor,textcolor,nodecolormap,nodecolor,maxdepth
model = plt[1]
## convert to graph
tmpObs = @lift convert(SimpleDiGraph,$model;maxdepth=$maxdepth)
graph = @lift $tmpObs[1]
properties = @lift $tmpObs[2]
## extract labels
labels = @lift [string(p[2]) for p in $properties]
## set the colors, first for nodes & cutoff-nodes, then for leaves
nlabels_color = map(properties, labels, leafcolor,textcolor,nodecolormap) do properties,labels,leafcolor,textcolor,nodecolormap
## set colors for the individual elements
leaf_ix = findall([p[1] == Leaf for p in properties])
leafValues = [p[1] for p in split.(labels[leaf_ix]," : ")]
## one color per category
uniqueLeafValues = unique(leafValues)
individual_leaf_colors = resample_cmap(nodecolormap,length(uniqueLeafValues))
nlabels_color = Any[p[1] == Node ? textcolor : leafcolor for p in properties]
for (ix,uLV) = enumerate(uniqueLeafValues)
ixV = leafValues .== uLV
nlabels_color[leaf_ix[ixV]] .= individual_leaf_colors[ix]
end
return nlabels_color
end
## plot :)
graphplot!(plt,graph;layout=Buchheim(),
nlabels=labels,
node_size = 100,
node_color=nodecolor,
nlabels_color=nlabels_color,
nlabels_align=(:center,:center),
##tangents=((0,-1),(0,-1))
)
return plt
end
nothing #hide
# ## Visualizing a DecisionTree
# Now finally we are ready to fit & visualize the tree
iris = MLJ.load_iris()
features = hcat(iris[1],iris[2],iris[3],iris[4])
labels = iris[5]
model = DecisionTreeClassifier(max_depth=4)
fit!(model, features, labels)
# now we are ready to plot
graphplot(model)
# you can also specify depth, or modify colors
graphplot(model;maxdepth=3,textcolor=:darkgreen)
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 4159 | #=
# Dependency Graph of a Package
In this example we'll plot a dependency graph of a package using
[`RegistryInstances.jl`](https://github.com/GunnarFarneback/RegistryInstances.jl) and
and a DAG layout from [`LayeredLayouts.jl`](https://github.com/oxinabox/LayeredLayouts.jl)
=#
using CairoMakie
CairoMakie.activate!(type="png") #hide
set_theme!(size=(800, 600)) #hide
using GraphMakie
using Graphs
using LayeredLayouts
using RegistryInstances
using Makie.GeometryBasics
using Makie.Colors
#=
First we need a small function which goes through the dependencies of a package and
builds a `SimpleDiGraph` object.
=#
function depgraph(root)
registries=RegistryInstances.reachable_registries()
general = registries[findfirst(x->x.name=="General", registries)]
packages = [root]
connections = Vector{Pair{Int,Int}}()
for pkg in packages
pkgidx = findfirst(isequal(pkg), packages)
uuids = uuids_from_name(general, pkg)
isempty(uuids) && continue
deps = String[]
pkginfo = registry_info(general[only(uuids)])
version = maximum(keys(pkginfo.version_info))
for (vrange, dep) ∈ pkginfo.deps
if version ∈ vrange
append!(deps, keys(pkginfo.deps[vrange]))
end
end
filter!(!isequal("julia"), deps)
for dep in deps
idx = findfirst(isequal(dep), packages)
if idx === nothing
push!(packages, dep)
idx = lastindex(packages)
end
push!(connections, idx => pkgidx)
end
end
g = SimpleDiGraph(length(packages))
for c in connections
add_edge!(g, c)
end
return (packages, g)
end
nothing #hide
#=
As an example we'll plot the dependency Graph of [`Revise.jl`](https://github.com/timholy/Revise.jl)
because it is one of the most important packages in the Julia ecosystem but does not have a huge
dependency tree.
=#
(packages, g) = depgraph("Revise")
N = length(packages)
xs, ys, paths = solve_positions(Zarate(), g)
## we scale the y coordinates so the plot looks nice in `DataAspect()`
ys .= 0.3 .* ys
foreach(v -> v[2] .= 0.3 .* v[2], values(paths))
nothing #hide
#=
In `GraphMakie` the layout always needs to be function. So we're creating a dummy function...
We will use the [Edge waypoints](@ref) attribute to get the graph with the least crossings.
=#
lay = Point.(zip(xs,ys))
## create a vector of Point2f per edge
wp = [Point2f.(zip(paths[e]...)) for e in edges(g)]
## manually tweak some of the label aligns
align = [(:right, :center) for i in 1:N]
align[findfirst(isequal("Revise"), packages)] = (:left, :center)
align[findfirst(isequal("LoweredCodeUtils"), packages)] = (:right, :top)
align[findfirst(isequal("CodeTracking"), packages)] = (:left, :bottom)
align[findfirst(isequal("JuliaInterpreter"), packages)] = (:left, :bottom)
align[findfirst(isequal("Requires"), packages)] = (:left, :bottom)
## shift "JuliaInterpreter" node in data space
offset = [Point2f(0,0) for i in 1:N]
offset[findfirst(isequal("JuliaInterpreter"), packages)] = Point(-0.1, 0.1)
f, ax, p = graphplot(g; layout=lay,
arrow_size=15,
edge_color=:gray,
nlabels=packages,
nlabels_align=align,
nlabels_distance=10,
nlabels_fontsize=15,
nlabels_offset=offset,
node_size=[9.0 for i in 1:N],
edge_width=[3 for i in 1:ne(g)],
waypoints=wp,
waypoint_radius=0.5)
ax.title = "Dependency Graph of Revise.jl"
xlims!(ax, -0.6, 5.6)
hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
f #hide
#=
If you run this example using `GLMakie` you can add this code to play
around with the interactive features.
```julia
deregister_interaction!(ax, :rectanglezoom)
register_interaction!(ax, :nodehover, NodeHoverHighlight(p))
register_interaction!(ax, :edgehover, EdgeHoverHighlight(p))
register_interaction!(ax, :edrag, EdgeDrag(p))
register_interaction!(ax, :ndrag, NodeDrag(p))
```
=#
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 5723 | #=
# Add interactions to your graph plot
In this example you will see, how to register interactions with your graph plot.
This tutorial will make use of the more basic [Interaction Interface](@ref).
If you just want to move nodes check out the [Predefined Interactions](@ref). The
implementation of those is quit similar to what is shown in this tutorial.
We star with a simple wheel graph again. This time we use arrays for some attributes
because we want to change them later in the interactions for individual nodes/edges.
=#
using CairoMakie
CairoMakie.activate!(type="png") # hide
set_theme!(size=(800, 400)) #hide
using GraphMakie
using Graphs
using CairoMakie.Colors
g = wheel_graph(10)
f, ax, p = graphplot(g,
edge_width = [2.0 for i in 1:ne(g)],
edge_color = [colorant"gray" for i in 1:ne(g)],
node_size = [10 for i in 1:nv(g)],
node_color = [colorant"red" for i in 1:nv(g)])
hidedecorations!(ax); hidespines!(ax)
ax.aspect = DataAspect()
@save_reference f # hide
# Later on we want to enable drag interactions, therefore we disable the default
# `:rectanglezoom` interaction
deregister_interaction!(ax, :rectanglezoom)
nothing #hide
# ## Hover interactions
# At first, let's add some hover interaction for our nodes using the [`NodeHoverHandler`](@ref)
# constructor. We need to define a action function with the signature `fun(state, idx, event, axis)`.
# We use the action to make the nodes bigger on hover events.
function node_hover_action(state, idx, event, axis)
@info idx #hide
p.node_size[][idx] = state ? 20 : 10
p.node_size[] = p.node_size[] # trigger observable
end
nhover = NodeHoverHandler(node_hover_action)
register_interaction!(ax, :nhover, nhover)
function set_cursor!(p) #hide
direction = Point2f(-0.1, 0.2) #hide
arrows!([p-direction], [direction], linewidth=3, arrowsize=20, lengthscale=0.7) #hide
end #hide
nodepos = copy(p[:node_pos][]) #hide
set_cursor!(nodepos[5] + Point2f(0.05, 0)) #hide
p.node_size[][5] = 20; p.node_size[] = p.node_size[] #hide
@save_reference f #hide
# Please run the script locally with `GLMakie.jl` if you want to play with the Graph 🙂
# The edge hover interaction is quite similar:
pop!(ax.scene.plots) #hide
p.node_size[][5] = 10; p.node_size[] = p.node_size[] #hide
function edge_hover_action(state, idx, event, axis)
@info idx #hide
p.edge_width[][idx]= state ? 5.0 : 2.0
p.edge_width[] = p.edge_width[] # trigger observable
end
ehover = EdgeHoverHandler(edge_hover_action)
register_interaction!(ax, :ehover, ehover)
set_cursor!((nodepos[4]+nodepos[1])/2) #hide
p.edge_width[][3] = 5.0; p.edge_width[] = p.edge_width[] #hide
@save_reference f #hide
# ## Click interactions
# In a similar fashion we might change the color of nodes and lines by click.
function node_click_action(idx, args...)
p.node_color[][idx] = rand(RGB)
p.node_color[] = p.node_color[]
end
nclick = NodeClickHandler(node_click_action)
register_interaction!(ax, :nclick, nclick)
function edge_click_action(idx, args...)
p.edge_color[][idx] = rand(RGB)
p.edge_color[] = p.edge_color[]
end
eclick = EdgeClickHandler(edge_click_action)
register_interaction!(ax, :eclick, eclick)
p.edge_color[][3] = colorant"blue"; p.edge_color[] = p.edge_color[] #hide
p.node_color[][7] = colorant"yellow" #hide
p.node_color[][2] = colorant"brown" #hide
p.node_color[][9] = colorant"pink" #hide
p.node_color[][6] = colorant"green" #hide
p.node_color[] = p.node_color[] #hide
@save_reference f #hide
# ## Drag interactions
pop!(ax.scene.plots) #hide
p.edge_width[][3] = 2.0; p.edge_width[] = p.edge_width[] #hide
function node_drag_action(state, idx, event, axis)
p[:node_pos][][idx] = event.data
p[:node_pos][] = p[:node_pos][]
end
ndrag = NodeDragHandler(node_drag_action)
register_interaction!(ax, :ndrag, ndrag)
p[:node_pos][][1] = nodepos[1] + Point2f(1.0,0.5) #hide
p[:node_pos][] = p[:node_pos][] #hide
set_cursor!(p[:node_pos][][1] + Point2f(0.05, 0)) #hide
p.node_size[][1] = 20; p.node_size[] = p.node_size[] #hide
@save_reference f # hide
# The last example is not as straight forward. By dragging an edge we want to
# change the positions of both attached nodes. Therefore we need some more state
# inside the action. We can achieve this with a callable struct.
pop!(ax.scene.plots) #hide
p.node_size[][1] = 10; p.node_size[] = p.node_size[] #hide
mutable struct EdgeDragAction
init::Union{Nothing, Point2f} # save click position
src::Union{Nothing, Point2f} # save src vertex position
dst::Union{Nothing, Point2f} # save dst vertex position
EdgeDragAction() = new(nothing, nothing, nothing)
end
function (action::EdgeDragAction)(state, idx, event, axis)
edge = collect(edges(g))[idx]
if state == true
if action.src===action.dst===action.init===nothing
action.init = event.data
action.src = p[:node_pos][][src(edge)]
action.dst = p[:node_pos][][dst(edge)]
end
offset = event.data - action.init
p[:node_pos][][src(edge)] = action.src + offset
p[:node_pos][][dst(edge)] = action.dst + offset
p[:node_pos][] = p[:node_pos][] # trigger change
elseif state == false
action.src = action.dst = action.init = nothing
end
end
edrag = EdgeDragHandler(EdgeDragAction())
register_interaction!(ax, :edrag, edrag)
p[:node_pos][][9] = nodepos[9] + Point2f(0.9,1.0) #hide
p[:node_pos][][10] = nodepos[10] + Point2f(0.9,1.0) #hide
p[:node_pos][] = p[:node_pos][] #hide
pm = (p[:node_pos][][9] + p[:node_pos][][10])/2 #hide
set_cursor!(pm) #hide
p.edge_width[][18] = 5.0; p.edge_width[] = p.edge_width[] #hide
@save_reference f # hide
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 11508 | #=
# Plotting Graphs with `GraphMakie.jl`
## The `graphplot` Command
Plotting your first `AbstractGraph` from [`Graphs.jl`](https://github.com/JuliaGraphs/Graphs.jl)
is as simple as
=#
using CairoMakie
CairoMakie.activate!(type="png") # hide
set_theme!(size=(800, 400)) #hide
using GraphMakie
using Graphs
g = wheel_graph(10)
f, ax, p = graphplot(g)
hidedecorations!(ax); hidespines!(ax)
ax.aspect = DataAspect()
@save_reference f #hide
#=
The `graphplot` command is a recipe which wraps several steps
- layout the graph in space using a layout function,
- create a `scatter` plot for the nodes and
- create a `linesegments` plot for the edges.
The default layout is `Spring()` from
[`NetworkLayout.jl`](https://github.com/JuliaGraphs/NetworkLayout.jl). The
layout attribute can be any function which takes an `AbstractGraph` and returns
a list of `Point{dim,Ptype}` (see [`GeometryBasics.jl`](https://github.com/JuliaGeometry/GeometryBasics.jl)
objects where `dim` determines the dimensionality of the plot.
Besides that there are some common attributes which are forwarded to the
underlying plot commands. See [`graphplot`](@ref).
=#
using GraphMakie.NetworkLayout
g = SimpleGraph(5)
add_edge!(g, 1, 2); add_edge!(g, 2, 4);
add_edge!(g, 4, 3); add_edge!(g, 3, 2);
add_edge!(g, 2, 5); add_edge!(g, 5, 4);
add_edge!(g, 4, 1); add_edge!(g, 1, 5);
## define some edge colors
edgecolors = [:black for i in 1:ne(g)]
edgecolors[4] = edgecolors[7] = :red
f, ax, p = graphplot(g, layout=Shell(),
node_color=[:black, :red, :red, :red, :black],
edge_color=edgecolors)
hidedecorations!(ax); hidespines!(ax)
ax.aspect = DataAspect()
@save_reference f #hide
#=
We can interactively change the attributes as usual with Makie.
=#
fixed_layout(_) = [(0,0), (0,1), (0.5, 1.5), (1,1), (1,0)]
## set new layout
p.layout = fixed_layout; autolimits!(ax)
## change edge width & color
p.edge_width = 5.0
p.edge_color[][3] = :green;
p.edge_color = p.edge_color[] # trigger observable
@save_reference f #hide
#=
## Adding Node Labels
=#
g = wheel_graph(10)
colors = [:black for i in 1:nv(g)]
colors[1] = :red
f, ax, p = graphplot(g,
nlabels=repr.(1:nv(g)),
nlabels_color=colors,
nlabels_align=(:center,:center))
hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
@save_reference f #hide
# This is not very nice, lets change the offsets based on the `node_positions`
offsets = 0.15 * (p[:node_pos][] .- p[:node_pos][][1])
offsets[1] = Point2f(0, 0.3)
p.nlabels_offset[] = offsets
autolimits!(ax)
@save_reference f #hide
#=
## Inner Node Labels
Sometimes it is prefered to show the labels inside the node. For that you can use the `ilabels` keyword arguments.
The Node sizes will be changed according to the size of the labels.
=#
g = cycle_digraph(3)
f, ax, p = graphplot(g;
ilabels=[1, L"\sum_{i=1}^n \alpha^i", "a label"],
arrow_shift=:end)
xlims!(ax, (-1.5, 1.3))
ylims!(ax, (-2.3, 0.7))
hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
f #hide
# ## Adding Edge Labels
g = barabasi_albert(6, 2; seed=42)
labels = repr.(1:ne(g))
f, ax, p = graphplot(g, elabels=labels,
elabels_color=[:black for i in 1:ne(g)],
edge_color=[:black for i in 1:ne(g)])
hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
@save_reference f #hide
#=
The position of the edge labels is determined by several plot arguments.
All possible arguments are described in the docs of the [`graphplot`](@ref) function.
By default, each label is placed in the middle of the edge and rotated to match the edge rotation.
The rotation for each label can be overwritten with the `elabels_rotation` argument.
=#
p.elabels_rotation[] = Dict(i => i == 5 ? 0.0 : Makie.automatic for i in 1:ne(g))
nothing #hide
#=
One can shift the label along the edge with the `elabels_shift` argument and determine the distance
in pixels using the `elabels_distance` argument.
=#
p.elabels_side[] = Dict(i => :right for i in [1,2,8,6])
p.elabels_offset[] = [Point2f(0.0, 0.0) for i in 1:ne(g)]
p.elabels_offset[][5] = Point2f(-0.4,0)
p.elabels_offset[] = p.elabels_offset[]
p.elabels_shift[] = [0.5 for i in 1:ne(g)]
p.elabels_shift[][1] = 0.6
p.elabels_shift[][7] = 0.4
p.elabels_shift[] = p.elabels_shift[]
p.elabels_distance[] = Dict(8 => 30)
@save_reference f #hide
#=
## Indicate Edge Direction
It is possible to put arrows on the edges using the `arrow_show` parameter. This parameter
is `true` for `SimpleDiGraph` by default. The position and size of each arrowhead can be
change using the `arrow_shift` and `arrow_size` parameters.
=#
g = wheel_digraph(10)
arrow_size = [10+i for i in 1:ne(g)]
arrow_shift = range(0.1, 0.8, length=ne(g))
f, ax, p = graphplot(g; arrow_size, arrow_shift)
hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
@save_reference f #hide
#=
There is a special case for `arrow_shift=:end` which moves the arrows close to the next node:
=#
g = cycle_digraph(3)
f, ax, p = graphplot(g; arrow_shift=:end, node_size=20, arrow_size=20)
hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
f #hide
#=
## Self edges
A self edge in a graph will be displayed as a loop.
!!! note
Selfe edges are not possible in 3D plots yet.
=#
g = complete_graph(3)
add_edge!(g, 1, 1)
add_edge!(g, 2, 2)
add_edge!(g, 3, 3)
f, ax, p = graphplot(g)
hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
@save_reference f #hide
# It is possible to change the appearance using the `selfedge_` attributes:
p.selfedge_size = Dict(1=>Makie.automatic, 4=>3.6, 6=>0.5) #idx as in edges(g)
p.selfedge_direction = Point2f(0.3, 1)
p.selfedge_width = Any[Makie.automatic for i in 1:ne(g)]
p.selfedge_width[][4] = 0.6*π; notify(p.selfedge_width)
autolimits!(ax)
@save_reference f #hide
#=
## Curvy edges
### `curve_distance` interface
The easiest way to enable curvy edges is to use the `curve_distance` parameter
which lets you add a "distance" parameter. The parameter changes the maximum
distance of the bent line to a straight line. Per default, only two way edges will
be drawn as a curve:
=#
g = SimpleDiGraph(3); add_edge!(g, 1, 2); add_edge!(g, 2, 3); add_edge!(g, 3, 1); add_edge!(g, 1, 3)
f, ax, p = graphplot(g)
hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
@save_reference f #hide
#=
This behaviour may be changed by using the `curve_distance_usage=Makie.automatic` parameter.
- `Makie.automatic`: Only apply `curve_distance` to double edges.
- `true`: Use on all edges.
- `false`: Don't use.
=#
f, ax, p = graphplot(g; curve_distance=-.5, curve_distance_usage=true)
hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
@save_reference f #hide
#=
It is also possible to specify the distance on a per edge base:
=#
g = complete_digraph(3)
distances = collect(0.05:0.05:ne(g)*0.05)
elabels = "d = ".* repr.(round.(distances, digits=2))
f, ax, p = graphplot(g; curve_distance=distances, elabels, arrow_size=20)
hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
@save_reference f #hide
#=
### `tangents` interface
Curvy edges are also possible using the low level interface of passing tangent
vectors and a `tfactor`. The tangent vectors can be `nothing` (straight line) or
two vectors per edge (one for src vertex, one for dst vertex). The `tfactor`
scales the distance of the bezier control point relative to the distance of src
and dst nodes. For real world usage see the [AST of a Julia function](@ref) example.
=#
using GraphMakie: plot_controlpoints!, SquareGrid
g = complete_graph(3)
tangents = Dict(1 => ((1,1),(0,-1)),
2 => ((0,1),(0,-1)),
3 => ((0,-1),(1,0)))
tfactor = [0.5, 0.75, (0.5, 0.25)]
f, ax, p = graphplot(g; layout=SquareGrid(cols=3), tangents, tfactor,
arrow_size=20, arrow_show=true, edge_color=[:red, :green, :blue],
elabels="Edge ".*repr.(1:ne(g)), elabels_distance=20)
hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
plot_controlpoints!(ax, p) # show control points for demonstration
@save_reference f #hide
#=
## Edge waypoints
It is possible to specify waypoints per edge which needs to be crossed. See the
[Dependency Graph of a Package](@ref) example.
If the attribute `waypoint_radius` is `nothing` or `:spline` the waypoints will be crossed
using natural cubic spline interpolation. If the supply a radius the waypoints won't be reached,
instead they will be connected with straight lines which bend in the given radius around the
waypoints.
=#
set_theme!(size=(800, 800)) #hide
g = SimpleGraph(8); add_edge!(g, 1, 2); add_edge!(g, 3, 4); add_edge!(g, 5, 6); add_edge!(g, 7, 8)
waypoints = Dict(1 => [(.25, 0.25), (.75, -0.25)],
2 => [(.25, -0.25), (.75, -0.75)],
3 => [(.25, -0.75), (.75, -1.25)],
4 => [(.25, -1.25), (.75, -1.75)])
waypoint_radius = Dict(1 => nothing,
2 => 0,
3 => 0.05,
4 => 0.15)
f = Figure(); f[1,1] = ax = Axis(f)
using Makie.Colors # hide
for i in 3:4 #hide
poly!(ax, Circle(Point2f(waypoints[i][1]), waypoint_radius[i]), color=RGBA(0.0,0.44705883,0.69803923,0.2)) #hide
poly!(ax, Circle(Point2f(waypoints[i][2]), waypoint_radius[i]), color=RGBA(0.0,0.44705883,0.69803923,0.2)) #hide
end #hide
p = graphplot!(ax, g; layout=SquareGrid(cols=2, dy=-0.5),
waypoints, waypoint_radius,
nlabels=["","r = nothing (equals :spline)",
"","r = 0 (straight lines)",
"","r = 0.05 (in data space)",
"","r = 0.1"],
nlabels_distance=30, nlabels_align=(:left,:center))
for i in 1:4 #hide
scatter!(ax, waypoints[i], color=RGBA(0.0,0.44705883,0.69803923,1.0)) #hide
end #hide
xlims!(ax, (-0.1, 2.25)), hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
@save_reference f #hide
#=
Specifying `waypoints` for self-edges will override any `selfedge_` attributes.
If `waypoints` are specified on an edge, `tangents` can also be added. However,
if `tangents` are given, but no `waypoints`, the `tangents` are ignored.
=#
g = SimpleDiGraph(1) #single node
add_edge!(g, 1, 1) #add self loop
f, ax, p = graphplot(g,
layout = [(0,0)],
waypoints = [[(1,-1),(1,1),(-1,1),(-1,-1)]])
hidedecorations!(ax); hidespines!(ax); ax.aspect = DataAspect()
@save_reference f #hide
#=
## Plot Graphs in 3D
If the layout returns points in 3 dimensions, the plot will be in 3D. However this is a bit
experimental. Feel free to file an issue if there are any problems.
=#
set_theme!(size=(800, 800)) #hide
g = smallgraph(:cubical)
elabels_shift = [0.5 for i in 1:ne(g)]
elabels_shift[[2,7,8,9]] .= 0.3
elabels_shift[10] = 0.25
f, ax, p = graphplot(g; layout=Spring(dim=3, seed=5),
elabels="Edge ".*repr.(1:ne(g)),
elabels_shift,
arrow_show=true,
arrow_shift=0.9,
arrow_size=15)
@save_reference f #hide
#=
Using [`JSServe.jl`](https://github.com/SimonDanisch/JSServe.jl) and [`WGLMakie.jl`](https://github.com/MakieOrg/Makie.jl/tree/master/WGLMakie)
we can also add some interactivity:
=#
using JSServe #md
Page(exportable=true, offline=true) #md
#
using WGLMakie #md
WGLMakie.activate!() #md
set_theme!(size=(800, 600)) #md
g = smallgraph(:dodecahedral) #md
graphplot(g, layout=Spring(dim=3)) #md
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 9493 | #=
# Images for Reference Testing
This is a more or less uncurated list of figures which are used for reference
testing. They might be interesting but they are most probably not.
=#
# ## Test different `nlabels_align` modes
using Graphs, GraphMakie, CairoMakie, NetworkLayout
import DataStructures: DefaultDict
CairoMakie.activate!(type="png") # hide
set_theme!(size=(400, 400)) #hide
g = SimpleGraph(9)
nlabels_align = [(:right, :bottom),
(:center, :bottom),
(:left, :bottom),
(:right, :center),
(:center, :center),
(:left, :center),
(:right, :top),
(:center, :top),
(:left, :top)]
nlabels = repr.(nlabels_align)
nlabels_fontsize = 10
fig, ax, p = graphplot(g; layout=SquareGrid(), nlabels, nlabels_align, nlabels_fontsize)
graphplot!(g; layout=SquareGrid(), nlabels, nlabels_align, nlabels_distance=30,
nlabels_color=:red, nlabels_fontsize)
hidedecorations!(ax); xlims!(-2,4); ylims!(-4,2)
@save_reference fig
# change the align
pop!(ax.scene.plots) # remove red plot
p[:nlabels_distance] = 10
p[:nlabels_align][] = [(:left, :bottom) for i in 1:nv(g)]
p[:nlabels][] = ["↙" for i in 1:nv(g)]
p[:nlabels_offset][] = Point2(0.1,0.2)
@save_reference fig
# variable offsets
p[:nlabels_distance] = 0
p[:nlabels_align][] = [(:center, :center) for i in 1:nv(g)]
p[:nlabels][] = ["×" for i in 1:nv(g)]
p[:nlabels_color][] = :red
p[:nlabels_offset][] = [Point2(.1*cos(-2π/9*i),.1*sin(-2π/9*i)) for i in 1:nv(g)]
@save_reference fig
# ## Edge label placement
g = path_graph(4)
elabels = ["a" for i in 1:ne(g)]
elabels_align = (:center, :center)
fig, ax, p = graphplot(g; layout=SquareGrid(), elabels, elabels_align,
elabels_color=:red)
hidedecorations!(ax)
@save_reference fig
# change the positioning of the edge labels
p[:elabels][] = repr.(edges(g))
p[:elabels_shift][] = [0.25, 0.5, 0.75]
p[:elabels_rotation][] = [π/8, 0, -π/8]
p[:elabels_offset][] = Point2(0.05,0.05)
p[:elabels_fontsize][] = 10
autolimits!(ax)
@save_reference fig
# ## Changes of node positions
# edge and node labels follow graph movement
g = complete_digraph(3)
elabels = repr.(edges(g))
nlabels = repr.(1:nv(g))
fig, ax, p = graphplot(g; elabels, nlabels, elabels_fontsize=10)
hidedecorations!(ax)
@save_reference fig
#
limits!(ax, ax.finallimits[]) # freeze the limits
p[:node_pos][] = Point2f.([(1., -.5), (-1.,0.), (-1.,-1.)])
hidedecorations!(ax)
@save_reference fig
# ## Change of the underlying graph
gn = Observable(SimpleDiGraph(3))
fig, ax, p = graphplot(gn)
hidedecorations!(ax)
@save_reference fig
add_edge!(gn[], 1, 2)
add_edge!(gn[], 1, 3)
add_edge!(gn[], 2, 3)
notify(gn)
autolimits!(ax)
@save_reference fig
# add another edge
add_edge!(gn[], 2, 1)
notify(gn)
@save_reference fig
# ## Different combinations of edge width and edgelabel distance
g = path_graph(3)
layout(y) = Point2f.([(0,-y),(1,-y),(2,-y)])
elabels = ["Edge 1", "Edge 2"]
node_color = :red
fig, ax, p = graphplot(g; layout=layout(0), elabels, node_color)
graphplot!(g; layout=layout(1), elabels, node_color, edge_width=10, elabels_fontsize=25)
graphplot!(g; layout=layout(2), elabels, node_color, edge_width=25, elabels_fontsize=15)
graphplot!(g; layout=layout(3), elabels, node_color, edge_width=25, elabels_fontsize=[15,25])
graphplot!(g; layout=layout(4), elabels, node_color, edge_width=[10,25], elabels_fontsize=25)
autolimits!(ax); hidedecorations!(ax); hidespines!(ax); ylims!(-5,1)
@save_reference fig
# ## Different linestyles per edge
fig = Figure()
graphplot(fig[1,1],
DiGraph([Edge(1 => 2), Edge(2 => 3)]),
edge_attr = (; linestyle = [:dot, :dash]),
edge_plottype = :beziersegments,
)
hidedecorations!(current_axis())
graphplot(fig[1,2],
DiGraph([Edge(1 => 2), Edge(2 => 1)]),
edge_attr = (; linestyle = [:dot, :dash]),
edge_plottype = :beziersegments,
)
hidedecorations!(current_axis())
graphplot(fig[2,1],
DiGraph([Edge(1 => 2), Edge(2 => 3), Edge(3=>4), Edge(4=>1)]),
edge_attr = (; linestyle = Linestyle([0.5, 1.0, 1.5, 2.5])),
edge_plottype = :beziersegments,
)
hidedecorations!(current_axis())
@save_reference fig
# ## Self loop with waypoints
g1 = SimpleDiGraph(1)
add_edge!(g1, 1, 1) #add self loop
fig, ax, p = graphplot(g1, layout = [(0,0)], waypoints = [[(1,-1),(1,1),(-1,1),(-1,-1)]])
hidedecorations!(ax)
@save_reference fig
# ## Shift arrows to nodes
fig, ax, p=graphplot(SimpleDiGraph(ones(2,2)),node_size=50,arrow_size=20,curve_distance=0.5,arrow_shift=:end)
hidedecorations!(ax)
@save_reference fig
# ### update shifts
g = SimpleDiGraph(3)
add_edge!(g, 1, 1); add_edge!(g, 1, 2); add_edge!(g, 2, 1); add_edge!(g, 2, 3); add_edge!(g, 3, 1);
# test update of node and arrow size, and node position
fig, ax, p = graphplot(g; arrow_shift=:end,
node_size=[20 for _ in 1:nv(g)],
arrow_size=[20 for _ in 1:ne(g)])
hidedecorations!(ax)
@save_reference fig
p.node_size[][1] = 40
notify(p.node_size)
@save_reference fig
p.arrow_size[][3] = 40
notify(p.arrow_size)
@save_reference fig
p.node_pos[][1] = (0,0)
notify(p.node_pos)
@save_reference fig
# test large nodes
using GraphMakie: SquareGrid
g = SimpleDiGraph(8)
add_edge!(g, 1, 2)
add_edge!(g, 3, 4)
add_edge!(g, 5, 6)
add_edge!(g, 7, 8)
fig, ax, p = graphplot(g; arrow_shift=:end, layout=SquareGrid(cols=2),
node_size=[10, 10, 10, 100, 10, 200, 10, 300],
arrow_size=[150,100,50,10],
arrow_attr=(color=:blue,),
edge_color=:red)
xlims!(-.5,1.5); ylims!(-3.5,.5)
hidedecorations!(ax)
@save_reference fig
# ## Inner node labels
fig, ax, p = graphplot(cycle_digraph(3), ilabels=[1, L"\sum_{i=1}^n \alpha^i", "a label"], node_marker=Circle)
hidedecorations!(ax)
@save_reference fig
# Interact with `arrow_shift=:end`
fig, ax, p = graphplot(cycle_digraph(3), ilabels=[1, L"\sum_{i=1}^n \alpha^i", "a label"], node_marker=Circle, arrow_shift=:end)
hidedecorations!(ax)
@save_reference fig
# Update observables
p[:ilabels][][1] = "1111"
p.node_size[] = DefaultDict(Makie.automatic, Dict{Int, Any}(2=>100))
notify(p[:ilabels])
@save_reference fig
p[:ilabels_fontsize][] = 10
@save_reference fig
p[:node_color][] = :red
@save_reference fig
# ## Changes of node and label sizes
gc = circular_ladder_graph(5);
ons = Observable(30);
onf = Observable(30);
fig,ax,p = graphplot(gc; nlabels=repr.(vertices(gc)), node_size=ons, nlabels_fontsize=onf)
hidedecorations!(ax)
@save_reference fig
# Change node size
ons[] = 10; # check changes
@save_reference fig
# Change label font size
onf[] = 10; # check changes
@save_reference fig
# Do the same with a `Dict`
onf = Observable(Dict(1=>30, 10=>30));
ons = Observable(Dict(1=>30, 10=>30));
fig,ax,p = graphplot(gc; nlabels=repr.(vertices(gc)), node_size=ons, nlabels_fontsize=onf)
hidedecorations!(ax)
@save_reference fig
# Change label font size
onf[] = Dict(7=>30); # check changes
@save_reference fig
# Change node size
ons[] = Dict(7=>30); # check changes
@save_reference fig
# Do the same with a `DefaultDict`
ons = Observable(DefaultDict(70, 1=>30, 10=>30));
onf = Observable(DefaultDict(70, 1=>30, 10=>30));
fig,ax,p = graphplot(gc; nlabels=repr.(vertices(gc)), node_size=ons, nlabels_fontsize=onf)
hidedecorations!(ax)
@save_reference fig
# Change node size
ons[] = DefaultDict(20, 10=>70); # check changes
@save_reference fig
# Change label font size
onf[] = DefaultDict(20, 10=>70); # check changes
@save_reference fig
# ## Dict and DefaultDict
# Test out argument functionality with `Dict` and `DefaultDict`
# First with a normal `Dict`
gc = circular_ladder_graph(5);
fig,ax,p = graphplot(gc, nlabels=Dict(1=>"One", 2 => "Two"))
hidedecorations!(ax)
@save_reference fig
# And also with a `DefaultDict`
fig,ax,p = graphplot(gc, nlabels=DefaultDict("Unknown", 1=>"One", 2 => "Two"))
hidedecorations!(ax)
@save_reference fig
# ## Use Dict{Edge} for edge arguments
fig,ax,p = graphplot(gc, edge_color=Dict(Edge(7,8)=>:blue))
hidedecorations!(ax)
@save_reference fig
# try out also the DefaultDict
fig,ax,p = graphplot(gc, edge_color=DefaultDict(:green, Edge(7,8)=>:blue))
hidedecorations!(ax)
@save_reference fig
# Of course you can still use integers labeling
ind = findfirst(==(Edge(7,8)) , collect(edges(gc)))
fig,ax,p = graphplot(gc, edge_color=DefaultDict(:green, ind=>:blue))
hidedecorations!(ax)
@save_reference fig
# The same can be done with all enumerations of edge arguments
fig,ax,p = graphplot(gc, elabels=DefaultDict("Unknown", Edge(1,2)=>"1-2", Edge(7,8) => "7-8"))
hidedecorations!(ax)
@save_reference fig
# directed and undirected graphs are handled appropriately.
# For example for directed graphs
gcd = SimpleDiGraph(gc)
fig,ax,p = graphplot(gcd, elabels=DefaultDict("Unknown", Edge(8,7)=>"8-7", Edge(2,7) => "2-7"), nlabels=repr.(vertices(gcd)))
hidedecorations!(ax)
@save_reference fig
# and non-directed graphs
fig,ax,p = graphplot(gc, elabels=DefaultDict("Unknown", Edge(8,7)=>"8-7", Edge(2,7) => "2-7"), nlabels=repr.(vertices(gc)))
hidedecorations!(ax)
@save_reference fig
# Test edge-specific updates
ec = Observable(Dict(Edge(8,7)=>:blue))
fig,ax,p = graphplot(gc, edge_color=ec, nlabels=repr.(vertices(gc)))
hidedecorations!(ax)
@save_reference fig
# update `Observable`
ec[] = Dict(Edge(7,2)=> :green)
@save_reference fig
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 3620 | #=
# Scene graph in Makie
In Makie, plots and scenes are stored as a tree. Scenes can hold child Scenes and Plots, and plots can hold other plots.
In this example, we create a simple plot (`streamplot`) and render the scene graph for it.
=#
using CairoMakie
CairoMakie.activate!(type="svg") #hide
using GraphMakie
using Graphs
# ## Extracting the scene graph
# First, we extract the scene graph by "walking" down the tree. This uses multiple dispatch to dispatch based on scenes and plots.
# Scenes and Plots can both hold plots, and the leaf nodes can be either Scenes with no plots (empty Scenes) or atomic plots, i.e.,
# plots which can be rendered directly by the backend.
# This function simply initializes the graph and labels, and begins the traversal.
function walk_tree(scene)
g = SimpleDiGraph()
labels = Any[]
walk_tree!(g, labels, scene)
return (g, labels)
end
nothing #hide
# Now, we can walk down the Scene tree. Scenes can have child Scenes as well as child Plots,
# but in terms of semantic order we walk down the Scene tree before looking at the Scene's attached
# plots.
function walk_tree!(g, labels, scene::Scene)
add_vertex!(g)
top_vertex = vertices(g)[end]
push!(labels, label_str(scene))
for child_scene in scene.children
child = walk_tree!(g, labels, child_scene)
add_edge!(g, top_vertex, child)
end
for child_plot in scene.plots
child = walk_tree!(g, labels, child_plot)
add_edge!(g, top_vertex, child)
end
return top_vertex
end
function walk_tree!(g, labels, plot)
add_vertex!(g)
top_vertex = vertices(g)[end]
push!(labels, label_str(plot))
for child_plot in plot.plots
child = walk_tree!(g, labels, child_plot)
add_edge!(g, top_vertex, child)
end
return top_vertex
end
## This is a utility function for the label, to avoid
## the cruft that comes from excessive type printing.
label_str(::Scene) = "Scene"
label_str(::Makie.Combined{F, T}) where {F, T} = string(F) # get only the plot func, not the argument type
nothing #hide
# ## Creating the plot
# This is a simple streamplot in an LScene, which has the simplest axis (Axis3 is more complex!)
fig, ax, plt = streamplot(-2..2, -2..2; axis = (type = LScene,),) do x::Point2
Point2(x[2], 4x[1])
end
# Let's walk down the tree with our previous `walk_tree` function:
newg, newl = walk_tree(fig.scene)
## This is for convenience later:
nlabels_align = [(:left, :center) for v in vertices(newg)]
nothing #hide
# We start out by plotting the graph itself.
f, a, p = graphplot(
newg;
layout=GraphMakie.Buchheim(),
nlabels=newl,
nlabels_distance=10,
nlabels_fontsize=30,
nlabels_align,
tangents=((0,-1),(0,-1)),
figure = (; size = (900, 600)),
axis = (limits = (-2.5, 2, -16, 2),)
)
hidedecorations!(a); hidespines!(a)
# Now, we add some improvements to the labels and positions (this is fairly minor):
_nlabels = deepcopy(newl)
_nlabels[1] = "Scene (root)"
_nlabels[2] = "Scene (lscene.blockscene)"
_nlabels[3] = "Scene (LScene)"
p.nlabels[] = _nlabels
fig
for v in vertices(newg)
if isempty(inneighbors(newg, v)) # root
nlabels_align[v] = (:center,:bottom)
elseif isempty(outneighbors(newg, v)) #leaf
nlabels_align[v] = (:center,:top)
else
self = p[:node_pos][][v]
parent = p[:node_pos][][inneighbors(newg, v)[1]]
if self[1] < parent[1] # left branch
nlabels_align[v] = (:right,:center)
end
end
end
p.nlabels_align = nlabels_align
nothing #hide
# ### Final figure
@save_reference f
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 2677 | #=
# AST of a Julia function
In this example we are going to plot an abstract syntax tree of a Julia function using the
Bucheim Layout from [`NetworkLayout.jl`](https://github.com/JuliaGraphs/NetworkLayout.jl).
=#
using CairoMakie
CairoMakie.activate!(type="png") #hide
set_theme!(size=(800, 600)) #hide
using Graphs
using GraphMakie
using NetworkLayout
using CairoMakie
#=
The following code, which walks the AST and creates a `SimpleDiGraph` was taken and slightly
modified from [`TreeView.jl`](https://github.com/JuliaTeX/TreeView.jl). Thanks!
=#
function walk_tree(ex; show_call=true)
g = SimpleDiGraph()
labels = Any[]
walk_tree!(g, labels, ex, show_call)
return (g, labels)
end
function walk_tree!(g, labels, ex, show_call)
add_vertex!(g)
top_vertex = vertices(g)[end]
where_start = 1 # which argument to start with
if !(show_call) && ex.head == :call
f = ex.args[1] # the function name
push!(labels, f)
where_start = 2 # drop "call" from tree
else
push!(labels, ex.head)
end
for i in where_start:length(ex.args)
if isa(ex.args[i], Expr)
child = walk_tree!(g, labels, ex.args[i], show_call)
add_edge!(g, top_vertex, child)
elseif !isa(ex.args[i], LineNumberNode)
add_vertex!(g)
n = vertices(g)[end]
add_edge!(g, top_vertex, n)
push!(labels, ex.args[i])
end
end
return top_vertex
end
nothing #hide
#=
The expression we want to look at is the recursive definition of the Fibonacci sequence.
=#
expr = quote
function fib(n)
if n > 1
return fib(n-1) + fib(n-2)
else
return n
end
end
end
g, labels = walk_tree(expr, show_call=true)
nlabels_align = [(:left, :bottom) for v in vertices(g)]
fig, ax, p = graphplot(g; layout=Buchheim(),
nlabels=repr.(labels),
nlabels_distance=5,
nlabels_align,
tangents=((0,-1),(0,-1)))
hidedecorations!(ax); hidespines!(ax)
@save_reference fig #hide
# This does not look nice yet! Lets tweak the `align` parameter of the nodes labels...
for v in vertices(g)
if isempty(inneighbors(g, v)) # root
nlabels_align[v] = (:center,:bottom)
elseif isempty(outneighbors(g, v)) #leaf
nlabels_align[v] = (:center,:top)
else
self = p[:node_pos][][v]
parent = p[:node_pos][][inneighbors(g, v)[1]]
if self[1] < parent[1] # left branch
nlabels_align[v] = (:right,:bottom)
end
end
end
p.nlabels_align = nlabels_align
@save_reference fig #hide
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 4902 | #=
# Stress on Truss
In this example we'll plot an animation of the stress on some truss structure
using `GaphMakie.jl` and [`NetworkDynamics.jl`](https://github.com/PIK-ICoN/NetworkDynamics.jl)

=#
using NetworkDynamics
using OrdinaryDiffEq
using Graphs
using GraphMakie
using LinearAlgebra: norm, ⋅
using Printf
using CairoMakie
CairoMakie.activate!()
#=
## Definition of the dynamical system
Define dynamic model for NetworkDynamics
=#
function edge_f!(e, v_s, v_d, (K, L), _)
Δd = view(v_d, 1:2) - view(v_s, 1:2)
nd = norm(Δd)
F = K * ( L - nd )
e[1:2] = F .* view(Δd, 1:2) ./ nd
end
function vertex_free!(du, u, edges, (M, γ, g), _)
F = sum(edges) - γ * view(u, 3:4) + [0, -M*g]
du[3:4] .= F ./ M
du[1:2] = view(u, 3:4)
end
function vertex_fixed!(du, u, edges, _, _)
du[1:2] .= 0.0
end
edge = StaticEdge(f = edge_f!, dim=2, coupling=:antisymmetric)
vertex_free = ODEVertex(f = vertex_free!, dim=4, sym=[:x, :y, :v, :w])
vertex_fix = ODEVertex(f = vertex_fixed!, dim=2, sym=[:x, :y])
nothing #hide
#=
Set up graph topology and initial positions.
=#
N = 5
dx = 1.0
shift = 0.2
g = SimpleGraph(2*N + 1)
for i in 1:N
add_edge!(g, i, i+N); add_edge!(g, i, i+N)
if i < N
add_edge!(g, i+1, i+N); add_edge!(g, i, i+1); add_edge!(g, i+N, i+N+1)
end
end
add_edge!(g, 2N, 2N+1)
pos0 = zeros(Point2f, 2N + 1)
pos0[1:N] = [Point((i-1)dx,0) for i in 1:N]
pos0[N+1:2*N] = [Point(i*dx + shift, 1) for i in 1:N]
pos0[2N+1] = Point(N*dx + 1, -1)
fixed = [1,4] # set fixed vertices
nothing #hide
# create networkdynamics object
verts = ODEVertex[vertex_free for i in 1:nv(g)]
for i in fixed
verts[i] = vertex_fix # use the fixed vertex for the fixed
end
nd = network_dynamics(verts, edge, g);
nothing #hide
#=
write some auxiliary functions to map between state vector of DGL `u` and graph data
=#
x_idxs = [findfirst(isequal(Symbol("x_$i")), nd.syms) for i in 1:nv(g)]
"Set positions `pos` inside dgl state `u`"
function set_pos!(u, pos)
for (i, idx) in enumerate(x_idxs)
u[idx] = pos[i][1]
u[idx+1] = pos[i][2]
end
end
"Extract vector of Points from dgl state `u`"
function to_pos(u)
pos = Vector{Point2f}(undef, nv(g))
for (i, idx) in enumerate(x_idxs)
pos[i] = Point(u[idx], u[idx+1])
end
return pos
end
"Calculate load on edge for given state."
function get_load(u, p, t=0.0)
gd_nd = nd(u, p, t, GetGD) # exposes underlying graph data struct
force = Vector{Float64}(undef, ne(g))
pos = to_pos(u)
for (i,e) in enumerate(edges(g))
edgeval = get_edge(gd_nd, i)
fvec = Point(edgeval[1], edgeval[2])
dir = pos[dst(e)] .- pos[src(e)]
force[i] = sign(fvec ⋅ dir) * norm(fvec)
end
return force
end
nothing #hide
#=
Set parameters.
=#
M = [10 for i in 1:nv(g)] # mass of the nodes
M[end] = 200 # heavy mass
gc = [9.81 for i in 1:nv(g)] # gravitational constant
γ = [200.0 for i in 1:nv(g)] # damping parameter
γ[end] = 100.0
L = [norm(pos0[src(e)] - pos0[dst(e)]) for e in edges(g)] # length of edges
K = [0.5e6 for i in 1:ne(g)] # spring constant of edges
## bundle parameters for NetworkDynamics
para = collect.((zip(M, γ, gc),
zip(K, L)))
nothing #hide
#=
Set initial state and solve the system
=#
u0 = zeros(length(nd.syms))
set_pos!(u0, pos0)
tspan = (0.0, 12.0)
prob = ODEProblem(nd, u0, tspan, para)
sol = solve(prob, Tsit5());
nothing #hide
#=
## Plot the solution
=#
fig = Figure(size=(1000,550))
fig[1,1] = title = Label(fig, "Stress on truss", fontsize=30)
title.tellwidth = false
fig[2,1] = ax = Axis(fig)
## calculate some values for colorscaling
(fmin, fmax) = 0.3 .* extrema([(map(u->get_load(u, para), sol)...)...])
nlabels = [" " for i in 1:nv(g)]
nlabels[fixed] .= "Δ"
elabels = ["edge $i" for i in 1:ne(g)]
p = graphplot!(ax, g;
edge_width=4.0,
node_size=sqrt.(M)*3,
nlabels=nlabels,
nlabels_align=(:center,:top),
nlabels_fontsize=30,
elabels=elabels,
elabels_side=Dict(ne(g) => :right),
edge_color=[0.0 for i in 1:ne(g)],
edge_attr=(colorrange=(fmin,fmax),
colormap=:diverging_bkr_55_10_c35_n256))
hidespines!(ax); hidedecorations!(ax); p[:node_pos][]=to_pos(u0); ax.aspect = DataAspect()
limits!(ax, -0.1, pos0[end][1]+0.3, pos0[end][2]-0.5, 1.15)
## draw colorbar
fig[3,1] = cb = Colorbar(fig, p.plots[1].plots[1], label = "Axial force", vertical=false)
T = tspan[2]
fps = 30
trange = range(0.0, sol.t[end], length=Int(T * fps))
record(fig, "truss.mp4", trange; framerate=fps) do t
title.text = @sprintf "Stress on truss (t = %.2f )" t
p[:node_pos][] = to_pos(sol(t))
load = get_load(sol(t), para)
p.elabels = [@sprintf("%.0f", l) for l in load]
p.edge_color[] = load
end
nothing #hide
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 316 | module GraphMakie
using NetworkLayout
using Graphs
using Makie
using LinearAlgebra
using SimpleTraits
import Makie: DocThemer, ATTRIBUTES, project, automatic
import DataStructures: DefaultDict, DefaultOrderedDict
include("beziercurves.jl")
include("recipes.jl")
include("interaction.jl")
include("utils.jl")
end
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 12733 | using GeometryBasics
using StaticArrays
using LinearAlgebra: normalize, ⋅
using PolynomialRoots
####
#### Type definitions
####
# type defs from Julius Krumbiegels PR
# https://github.com/MakieOrg/Makie.jl/pull/979
abstract type AbstractPath{PT<:AbstractPoint} end
# simple lines get there own type. This helps with type stability
# for graphs without curvy edges and improves performance
struct Line{PT} <: AbstractPath{PT}
p0::PT
p::PT
end
abstract type PathCommand{PT<:AbstractPoint} end
struct BezierPath{PT} <: AbstractPath{PT}
commands::Vector{PathCommand{PT}}
end
struct MoveTo{PT} <: PathCommand{PT}
p::PT
end
struct LineTo{PT} <: PathCommand{PT}
p::PT
end
struct CurveTo{PT} <: PathCommand{PT}
c1::PT
c2::PT
p::PT
end
ptype(::Union{AbstractPath{PT}, Type{<:AbstractPath{PT}}}) where {PT} = PT
straighten(l::Line) = l
straighten(p::BezierPath) = Line(interpolate(p,0.0), interpolate(p,1.0))
adjust_endpoint(l::Line, p) = Line(l.p0, p)
adjust_endpoint(::MoveTo, p) = MoveTo(p)
adjust_endpoint(::LineTo, p) = LineTo(p)
adjust_endpoint(c::CurveTo, p) = CurveTo(c.c1, c.c2, p)
function adjust_endpoint(path::BezierPath, p)
commands = copy(path.commands)
commands[end] = adjust_endpoint(commands[end], p)
BezierPath(commands)
end
####
#### Helper functions to work with bezier paths
####
"""
interpolate(p::AbstractPath, t)
Parametrize path `p` from `t ∈ [0, 1]`. Return position at `t`.
TODO: Points are not necessarily evenly spaced!
"""
function interpolate(p::BezierPath{PT}, t) where PT
@assert p.commands[begin] isa MoveTo
N = length(p.commands) - 1
tn = N*t
seg = max(0, min(floor(Int, tn), N-1))
tseg = tn - seg
p0 = p.commands[seg+1].p
return _interpolate(p.commands[seg+2], p0, tseg)
end
interpolate(l::Line{PT}, t) where PT = l.p0 + t*(l.p - l.p0) |> PT
"""
inverse_interpolate(p, pt)
Find interpolation point `t ∈ [0, 1]` for the point along `p` that is closest to `pt`.
Method: Calculates the square distance between `pt` and path `p` and minimizes (takes first derivative, equates to 0 and finds roots)
For BezierPath:
p = f(t)
D² = [f(t)[1] - pt[1]]^2 + [f(t)[2] - pt[2]]^2
d(D²)/dt = 2D*d(D)/dt = 2*([f(t)[1] - pt[1]] * d(f(t)[1])/dt + [f(t)[2] - pt[2]] * d(f(t)[2])/dt) = 0
[f(t)[1] - pt[1]] * d(f(t)[1])/dt + [f(t)[2] - pt[2]] * d(f(t)[2])/dt = 0
This is a 5th order polynomial for 3rd order Bezier curves.
For Lines:
D² = [p0[1] + t*p[1] - pt[1]]^2 + [p0[2] + t*p[2] - pt[2]]^2
Take derivative, equate to 0 and divide by 2:
[p0[1] + t*p[1] - pt[1]]*p[1] + [p0[2] + t*p[2] - pt[2]]*p[2] = 0
let a = p0 - pt, b = p - p0
t = -(a[1]*b[1] + a[2]*b[2]) / (b[1]^2 + b[2]^2)
"""
function inverse_interpolate(p::BezierPath{<:Point2}, pt)
p0 = p.commands[end-1].p
c = p.commands[end]
N = length(p.commands) - 1
tseg = _inverse_interpolate(c, p0, pt) #get interpolation value closest to pt
if isempty(tseg)
t = NaN
else
_, tloc = findmin((tseg .- 1).^2) #find the value closest to 1
t = ((N-1) + tseg[tloc]) / N
end
return t
end
function inverse_interpolate(l::Line{PT}, pt) where PT
a = l.p0 - pt
b = l.p - l.p0
t = -(a[1]*b[1] + a[2]*b[2]) / (b[1]^2 + b[2]^2)
return t
end
function inverse_interpolate(p, pt::Point3)
# TODO: is this the right place to throw an error when trying to shift arrows to destination nodes?
@warn "arrow_shift = :end will not display properly for 3D plots."
nothing
end
_inverse_interpolate(c::LineTo{<:Point2}, p0, pt) = inverse_interpolate(Line(p0, c.p), pt)
function _inverse_interpolate(c::CurveTo{<:Point2}, p0, pt)
p1, p2, p3 = c.c1, c.c2, c.p
poly0 = - p0[1]^2 + p0[1]*p1[1] + p0[1]*pt[1] - p0[2]^2 + p0[2]*p1[2] + p0[2]*pt[2] - p1[1]*pt[1] - p1[2]*pt[2]
poly1 = 5*p0[1]^2 - 10*p0[1]*p1[1] + 2*p0[1]*p2[1] - 2*p0[1]*pt[1] + 5*p0[2]^2 - 10*p0[2]*p1[2] + 2*p0[2]*p2[2] - 2*p0[2]*pt[2] + 3*p1[1]^2 + 4*p1[1]*pt[1] + 3*p1[2]^2 + 4*p1[2]*pt[2] - 2*p2[1]*pt[1] - 2*p2[2]*pt[2]
poly2 = -10*p0[1]^2 + 30*p0[1]*p1[1] - 12*p0[1]*p2[1] + p0[1]*p3[1] + p0[1]*pt[1] - 10*p0[2]^2 + 30*p0[2]*p1[2] - 12*p0[2]*p2[2] + p0[2]*p3[2] + p0[2]*pt[2] - 18*p1[1]^2 + 9*p1[1]*p2[1] - 3*p1[1]*pt[1] - 18*p1[2]^2 + 9*p1[2]*p2[2] - 3*p1[2]*pt[2] + 3*p2[1]*pt[1] + 3*p2[2]*pt[2] - p3[1]*pt[1] - p3[2]*pt[2]
poly3 = 10*p0[1]^2 - 40*p0[1]*p1[1] + 24*p0[1]*p2[1] - 4*p0[1]*p3[1] + 10*p0[2]^2 - 40*p0[2]*p1[2] + 24*p0[2]*p2[2] - 4*p0[2]*p3[2] + 36*p1[1]^2 - 36*p1[1]*p2[1] + 4*p1[1]*p3[1] + 36*p1[2]^2 - 36*p1[2]*p2[2] + 4*p1[2]*p3[2] + 6*p2[1]^2 + 6*p2[2]^2
poly4 = -5*p0[1]^2 + 25*p0[1]*p1[1] - 20*p0[1]*p2[1] + 5*p0[1]*p3[1] - 5*p0[2]^2 + 25*p0[2]*p1[2] - 20*p0[2]*p2[2] + 5*p0[2]*p3[2] - 30*p1[1]^2 + 45*p1[1]*p2[1] - 10*p1[1]*p3[1] - 30*p1[2]^2 + 45*p1[2]*p2[2] - 10*p1[2]*p3[2] - 15*p2[1]^2 + 5*p2[1]*p3[1] - 15*p2[2]^2 + 5*p2[2]*p3[2]
poly5 = p0[1]^2 - 6*p0[1]*p1[1] + 6*p0[1]*p2[1] - 2*p0[1]*p3[1] + p0[2]^2 - 6*p0[2]*p1[2] + 6*p0[2]*p2[2] - 2*p0[2]*p3[2] + 9*p1[1]^2 - 18*p1[1]*p2[1] + 6*p1[1]*p3[1] + 9*p1[2]^2 - 18*p1[2]*p2[2] + 6*p1[2]*p3[2] + 9*p2[1]^2 - 6*p2[1]*p3[1] + 9*p2[2]^2 - 6*p2[2]*p3[2] + p3[1]^2 + p3[2]^2
t_vals = roots5([poly0, poly1, poly2, poly3, poly4, poly5]) #get roots
t_reals = filter(i -> isreal(i), round.(t_vals, digits=6)) #get reals (round to 6 digits)
return real.(t_reals)
end
"""
tangent(p::AbstractPath, t)
Parametrize path `p` from `t ∈ [0, 1]`. Return tangent at `t`.
"""
function tangent(p::BezierPath, t)
@assert p.commands[begin] isa MoveTo
N = length(p.commands) - 1
tn = N*t
seg = max(0, min(floor(Int, tn), N-1))
tseg = tn - seg
p0 = p.commands[seg+1].p
return _tangent(p.commands[seg+2], p0, tseg)
end
tangent(l::Line, _) = normalize(l.p - l.p0)
"""
discretize(path::AbstractPath)
Return vector of points which represent the given `path`.
"""
function discretize(path::BezierPath{T}) where {T}
v = Vector{T}()
for c in path.commands
_discretize!(v, c)
end
return v
end
discretize(l::Line) = [l.p0, l.p]
"""
_interpolate(c::PathCommand, p0, t)
Returns positions along the path `c` starting from `p0` in range `t ∈ [0, 1]`.
"""
_interpolate(c::LineTo{PT}, p0, t) where {PT} = p0 + t*(c.p - p0) |> PT
function _interpolate(c::CurveTo{PT}, p0, t) where {PT}
p1, p2, p3 = c.c1, c.c2, c.p
(1 - t)^3 * p0 + 3(t - 2t^2 + t^3) * p1 + 3(t^2 -t^3) * p2 + t^3 * p3 |> PT
end
"""
_tangent(c::PathCommand, p0, t)
Returns tangent vector along the path `c` starting from `p0` in range `t ∈ [0, 1]`.
"""
_tangent(c::LineTo, p0, _) = normalize(c.p - p0)
function _tangent(c::CurveTo{PT}, p0, t) where PT
p1, p2, p3 = c.c1, c.c2, c.p
normalize(-3(1 - t)^2 * p0 + 3(1 - 4t + 3t^2) * p1 + 3(2t -3t^2) * p2 + 3t^2 * p3) |> PT
end
"""
_discretize!(v::Vector{AbstractPint}, c::PathCommand)
Append interpolated points of path `c` to pos vector `v`
"""
_discretize!(v::Vector{<:AbstractPoint}, c::Union{MoveTo, LineTo}) = push!(v, c.p)
function _discretize!(v::Vector{<:AbstractPoint}, c::CurveTo)
N0 = length(v)
p0 = v[end]
N = 60 # TODO: magic number of points for discretization
resize!(v, N0 + N)
dt = 1.0/N
for (i, t) in enumerate(dt:dt:1.0)
v[N0 + i] = _interpolate(c, p0, t)
end
end
"""
waypoints(p::BezierPath)
Returns all the characteristic points of the path. For debug reasons.
"""
function waypoints(p::BezierPath{PT}) where {PT}
v = PT[]
for c in p.commands
if c isa CurveTo
push!(v, c.c1)
push!(v, c.c2)
end
push!(v, c.p)
end
return v
end
"""
isline(p::AbstractPath)
True if the AbstractPath just represents a straight line.
"""
isline(p::Line) = true
isline(p::BezierPath) = length(p.commands)==2 && p.commands[1] isa MoveTo && p.commands[2] isa LineTo
####
#### Special constructors to create abstract paths
####
"""
Path(P::Vararg{PT, N}; tangents, tfactor=.5) where {PT<:AbstractPoint, N}
Create a bezier path by natural cubic spline interpolation of the points `P`.
If there are only two points and no tangents return a straight line.
The `tangents` kw allows you pass two vectors as tangents for the first and the
last point. The `tfactor` affects the curvature on the start and end given some
tangents.
"""
function Path(P::Vararg{PT, N}; tangents=nothing, tfactor=.5) where {PT<:AbstractPoint, N}
@assert N>2
# cubic_spline will work for each dimension separately
pxyz = _cubic_spline(map(p -> p[1], P)) # get first dimension
for i in 2:length(PT) # append all other dims
pxyz = hcat(pxyz, _cubic_spline(map(p -> p[i], P)))
end
# create waypoints from waypoints in separate dimensions
WP = SVector{length(P)-1, PT}(PT(p) for p in eachrow(pxyz))
commands = Vector{PathCommand{PT}}(undef, N)
commands[1] = MoveTo(P[1])
# first command, recalculate WP if tangent is given
first_wp = WP[1]
if tangents !== nothing
p1, p2, t = P[1], P[2], normalize(Pointf(tangents[1]))
dir = p2 - p1
d = tfactor * norm(dir ⋅ t)
first_wp = PT(p1+d*t)
end
commands[2] = CurveTo(first_wp,
2*P[2] - WP[2],
P[2])
# middle commands
for i in 3:(N-1)
commands[i] = CurveTo(WP[i-1],
2*P[i] - WP[i],
P[i])
end
# last command, recalculate last WP if tangent is given
last_wp = (P[N] + WP[N-1])/2
if tangents !== nothing
p1, p2, t = P[N-1], P[N], normalize(Pointf(tangents[2]))
dir = p2 - p1
d = tfactor * norm(dir ⋅ t)
last_wp = PT(p2-d*t)
end
commands[N] = CurveTo(WP[N-1],
last_wp,
P[N])
BezierPath(commands)
end
# same function as above but for just 2 points specifically
function Path(P::Vararg{PT, 2}; tangents=nothing, tfactor=.5) where {PT<:AbstractPoint}
if tfactor isa NTuple{2, <:Number}
tf1, tf2 = tfactor
else
tf1 = tf2 = tfactor
end
p1, p2 = P
if tangents === nothing
return Line(p1, p2)
else
t1 = normalize(Pointf(tangents[1]))
t2 = normalize(Pointf(tangents[2]))
len = norm(p2 - p1)
return BezierPath([MoveTo(p1),
CurveTo(PT(p1+len*tf1*t1),
PT(p2-len*tf2*t2),
p2)])
end
end
"""
Path(radius::Real, p::Vararg{PT, N}) where {PT<:AbstractPoint,N}
Draw straight lines through the points `p`. Within `radius` of each
point the line will be smoothly connected.
"""
function Path(radius::Real, p::Vararg{PT, N}) where {PT<:AbstractPoint,N}
if iszero(radius)
commands = PathCommand{PT}[MoveTo(PT(p[1]))]
for pos in p[2:end]
push!(commands, LineTo(PT(pos)))
end
return BezierPath(commands)
else
points = collect(reverse(p))
pos = pop!(points)
commands = PathCommand{PT}[MoveTo(PT(pos))]
while length(points) > 1
mid = pop!(points)
dst = points[end] # due to reverse...
dir1 = normalize(mid - pos)
dir2 = normalize(dst - mid)
r1 = mid - radius * dir1
r2 = mid + radius * dir2
c1 = mid - .25 * radius * dir1
c2 = mid + .25 * radius * dir2
push!(commands, LineTo(PT(r1)))
push!(commands, CurveTo(PT(c1),
PT(c2),
PT(r2)))
pos = r2
end
push!(commands, LineTo(points[end]))
return BezierPath(commands)
end
end
"""
_cubic_spline(p)
Given a number of points in one dimension calculate waypoints between them.
_cubic_spline([x1, x2, x3])
Will return the x coordinates of the waypoints `wp1` and `wp2`.
Those are the first waypoints between in the cubic bezier sense.
"""
function _cubic_spline(p)
N = length(p) - 1
M = SMatrix{N,N}(if i==j # diagonal
if i==1; 2; elseif i==N; 7; else 4 end
elseif i==j+1 # lower
if i==N; 2; else 1 end
elseif i==j-1 # upper
1
else
0
end for i in 1:N, j in 1:N)
b = SVector{N}(if i == 1
p[i] + 2p[i+1]
elseif i == N
8p[i] + p[i+1]
else
4p[i] + 2p[i+1]
end for i in 1:N)
return M \ b
end
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 13732 | using Makie: ScenePlot
import Makie: registration_setup!, process_interaction
export NodeHoverHandler, EdgeHoverHandler
export NodeHoverHighlight, EdgeHoverHighlight
export NodeClickHandler, EdgeClickHandler
export NodeDragHandler, EdgeDragHandler
export NodeDrag, EdgeDrag
"""
convert_selection(element, idx)
`pick` returns the basic plot type. In case of `Lines` check if it is
part of a `BezierSegments` and convert selection accordingly. In case of `LineSegments`
check if it is part of a `EdgePlot` and convert idx.
"""
convert_selection(element, idx) = (element, idx)
function convert_selection(element::LineSegments, idx)
if element.parent isa EdgePlot
return (element.parent, ceil(Int, idx / 2))
end
return (element, idx)
end
function convert_selection(element::Lines, idx)
if element.parent isa BezierSegments && element.parent.parent isa EdgePlot
bezier = element.parent
idx = findfirst(isequal(element), bezier.plots)
return (bezier.parent, idx)
end
return (element, idx)
end
"""
abstract type GraphInteraction
This abstract type supertypes other `GraphInteractions`. Some `GraphInteractions` need
references to the scatter and linesegments plot of the `GraphPlot`. By overloding
`set_nodeplot!(T<:GraphInteraction)` and `set_edgeplot!` those references will be set
during `registration_setup!`.
"""
abstract type GraphInteraction end;
set_nodeplot!(::GraphInteraction, plot) = nothing
set_edgeplot!(::GraphInteraction, plot) = nothing
function registration_setup!(parent, inter::GraphInteraction)
# in case of multiple graph plots in one axis this won't work
gplots = filter(p -> p isa GraphPlot, parent.scene.plots)
if length(gplots) !== 1
@warn "There are multiple GraphPlots, register interaction to first!"
end
gplot = gplots[1]
set_nodeplot!(inter, get_node_plot(gplot))
set_edgeplot!(inter, get_edge_plot(gplot))
end
####
#### Hover Interaction
####
"""
mutable struct HoverHandler{P<:ScenePlot, F} <: GraphInteraction
Object to handle hovers on `plot::P`. Calls `fun` on hover.
"""
mutable struct HoverHandler{P<:ScenePlot,F} <: GraphInteraction
idx::Union{Nothing,Int}
plot::Union{Nothing,P}
fun::F
end
set_nodeplot!(h::HoverHandler{Scatter}, plot) = h.plot = plot
set_edgeplot!(h::HoverHandler{EdgePlot}, plot) = h.plot = plot
function process_interaction(handler::HoverHandler, event::MouseEvent, axis)
if event.type === MouseEventTypes.over
(element, idx) = convert_selection(pick(axis.scene)...)
if element == handler.plot
if handler.idx === nothing
handler.idx = idx
ret = handler.fun(true, handler.idx, event, axis)
return ret isa Bool ? ret : false
end
else
if handler.idx !== nothing
ret = handler.fun(false, handler.idx, event, axis)
handler.idx = nothing
return ret isa Bool ? ret : false
end
end
end
return false
end
"""
NodeHoverHandler(fun)
Initializes `HoverHandler` for nodes. Calls function
fun(hoverstate, idx, event, axis)
with `hoverstate=true` on hover and `false` at the end of hover. `idx` is the node index.
# Example
```
julia> g = wheel_digraph(10)
julia> f, ax, p = graphplot(g, node_size = [20 for i in 1:nv(g)])
julia> function action(state, idx, event, axis)
p.node_size[][idx] = state ? 40 : 20
p.node_size[] = p.node_size[] #trigger observable
end
julia> register_interaction!(ax, :nodehover, NodeHoverHandler(action))
```
"""
NodeHoverHandler(fun::F) where {F} = HoverHandler{Scatter,F}(nothing, nothing, fun)
"""
NodeHoverHeighlight(p::GraphPlot, factor=2)
Magnifies the `node_size` of node under cursor by `factor`.
# Example
```
julia> g = wheel_graph(10)
julia> f, ax, p = graphplot(g, node_size = [20 for i in 1:nv(g)])
julia> register_interaction!(ax, :nodehover, NodeHoverHighlight(p))
```
"""
function NodeHoverHighlight(p::GraphPlot, factor=2)
@assert p.node_size[] isa Vector{<:Real} "`node_size` object needs to be an Vector{<:Real} for this interaction to work!"
action = (state, idx, _, _) -> begin
old = p.node_size[][idx]
p.node_size[][idx] = state ? old * factor : old / factor
p.node_size[] = p.node_size[] #trigger observable
end
return NodeHoverHandler(action)
end
"""
EdgeHoverHandler(fun)
Initializes `HoverHandler` for edges. Calls function
fun(hoverstate, idx, event, axis)
with `hoverstate=true` on hover and `false` at the end of hover. `idx` is the edge index.
# Example
```
julia> g = wheel_digraph(10)
julia> f, ax, p = graphplot(g, edge_width = [3.0 for i in 1:ne(g)])
julia> function action(state, idx, event, axis)
p.edge_width[][idx] = state ? 6.0 : 3.0
p.edge_width[] = p.edge_width[] #trigger observable
end
julia> register_interaction!(ax, :edgehover, EdgeHoverHandler(action))
```
"""
EdgeHoverHandler(fun::F) where {F} = HoverHandler{EdgePlot,F}(nothing, nothing, fun)
"""
EdgeHoverHeighlight(p::GraphPlot, factor=2)
Magnifies the `edge_width` of edge under cursor by `factor`.
If `arrow_size isa Vector{<:Real}` it also magnifies the arrow scatter.
# Example
```
julia> g = wheel_digraph(10)
julia> f, ax, p = graphplot(g, edge_width = [3 for i in 1:ne(g)],
arrow_size=[10 for i in 1:ne(g)])
julia> register_interaction!(ax, :nodehover, EdgeHoverHighlight(p))
```
"""
function EdgeHoverHighlight(p::GraphPlot, factor=2)
@assert p.edge_width[] isa Vector{<:Real} "`edge_width` object needs to be an Vector{<:Real} for this interaction to work!"
scale_arrows = p.arrow_size[] isa Vector{<:Real}
if length(p.edge_width[]) == ne(p[:graph][])
action = (state, idx, _, _) -> begin
old = p.edge_width[][idx]
p.edge_width[][idx] = state ? old * factor : old / factor
p.edge_width[] = p.edge_width[] #trigger observable
if scale_arrows
old = p.arrow_size[][idx]
p.arrow_size[][idx] = state ? old * factor : old / factor
p.arrow_size[] = p.arrow_size[] #trigger observable
end
end
elseif length(p.edge_width[]) == 2 * ne(p[:graph][])
action = (state, idx, _, _) -> begin
oldA = p.edge_width[][2 * idx - 1]
oldB = p.edge_width[][2 * idx]
p.edge_width[][2 * idx - 1] = state ? oldA * factor : oldA / factor
p.edge_width[][2 * idx] = state ? oldB * factor : oldB / factor
p.edge_width[] = p.edge_width[] #trigger observable
if scale_arrows
old = p.arrow_size[][idx]
p.arrow_size[][idx] = state ? old * factor : old / factor
p.arrow_size[] = p.arrow_size[] #trigger observable
end
end
else
error("Can not make sense of `length(p.edge_width)`")
end
return EdgeHoverHandler(action)
end
####
#### Drag Interaction
####
"""
mutable struct DragHandler{P<:ScenePlot, F} <: GraphInteraction
Object to handle left mous drags on `plot::P`.
"""
mutable struct DragHandler{P<:ScenePlot,F} <: GraphInteraction
dragstate::Bool
idx::Int
plot::Union{Nothing,P}
fun::F
end
set_nodeplot!(h::DragHandler{Scatter}, plot) = h.plot = plot
set_edgeplot!(h::DragHandler{EdgePlot}, plot) = h.plot = plot
function process_interaction(handler::DragHandler, event::MouseEvent, axis)
if handler.dragstate == false # not in drag state
if event.type === MouseEventTypes.leftdown
# on leftdown save idx if happens to be on right element/plot
(element, idx) = convert_selection(pick(axis.scene)...)
handler.idx = element === handler.plot ? idx : 0
elseif event.type === MouseEventTypes.leftdragstart && handler.idx != 0
# if idx!=0 the last leftdown was on right element!
handler.dragstate = true
end
elseif handler.dragstate == true # drag state
if event.type === MouseEventTypes.leftdrag
ret = handler.fun(true, handler.idx, event, axis)
return ret isa Bool ? ret : false
elseif event.type === MouseEventTypes.leftdragstop
ret = handler.fun(false, handler.idx, event, axis)
handler.idx = 0
handler.dragstate = false
return ret isa Bool ? ret : false
end
end
return false
end
"""
NodeDragHandler(fun)
Initializes `DragHandler` for Nodes. Calls function
fun(dragstate, idx, event, axis)
where `dragstate=true` during the drag and `false` at the end of the drag,
the last time `fun` is triggered. `idx` is the node index.
# Example
```
julia> g = wheel_digraph(10)
julia> f, ax, p = graphplot(g, node_size=20)
julia> deregister_interaction!(ax, :rectanglezoom)
julia> function action(state, idx, event, axis)
p[:node_pos][][idx] = event.data
p[:node_pos][] = p[:node_pos][]
end
julia> register_interaction!(ax, :nodedrag, NodeDragHandler(action))
```
"""
NodeDragHandler(fun::F) where {F} = DragHandler{Scatter,F}(false, 0, nothing, fun)
"""
NodeDrag(p::GraphPlot)
Allows drag and drop of Nodes. Please deregister the `:rectanglezoom` interaction.
# Example
```
julia> g = wheel_graph(10)
julia> f, ax, p = graphplot(g, node_size = [10 for i in 1:nv(g)])
julia> deregister_interaction!(ax, :rectanglezoom)
julia> register_interaction!(ax, :nodehover, NodeHoverHighlight(p))
julia> register_interaction!(ax, :nodedrag, NodeDrag(p))
```
"""
function NodeDrag(p)
action = (state, idx, event, _) -> begin
p[:node_pos][][idx] = event.data
p[:node_pos][] = p[:node_pos][]
end
return NodeDragHandler(action)
end
"""
EdgeDragHandler(fun)
Initializes `DragHandler` for Edges. Calls function
fun(dragstate, idx, event, axis)
where `dragstate=true` during the drag and `false` at the end of the drag,
the last time `fun` is triggered. `idx` is the edge index.
See [`EdgeDrag`](@ref) for a concrete implementation.
```
"""
EdgeDragHandler(fun::F) where {F} = DragHandler{EdgePlot,F}(false, 0, nothing, fun)
"""
EdgeDrag(p::GraphPlot)
Allows drag and drop of Edges. Please deregister the `:rectanglezoom` interaction.
# Example
```
julia> g = wheel_graph(10)
julia> f, ax, p = graphplot(g, edge_width = [3 for i in 1:ne(g)])
julia> deregister_interaction!(ax, :rectanglezoom)
julia> register_interaction!(ax, :edgehover, EdgeHoverHighlight(p))
julia> register_interaction!(ax, :edgedrag, EdgeDrag(p))
```
"""
function EdgeDrag(p)
action = EdgeDragAction(p)
return EdgeDragHandler(action)
end
mutable struct EdgeDragAction{PT<:GraphPlot}
p::PT
init::Union{Nothing,Point2f} # save click position
src::Union{Nothing,Point2f} # save src vertex position
dst::Union{Nothing,Point2f} # save dst vertex position
EdgeDragAction(p::T) where {T} = new{T}(p, nothing, nothing, nothing)
end
function (action::EdgeDragAction)(state, idx, event, _)
edge = collect(edges(action.p[:graph][]))[idx]
if state == true
if action.src === action.dst === action.init === nothing
action.init = event.data
action.src = action.p[:node_pos][][src(edge)]
action.dst = action.p[:node_pos][][dst(edge)]
end
offset = event.data - action.init
action.p[:node_pos][][src(edge)] = action.src + offset
action.p[:node_pos][][dst(edge)] = action.dst + offset
action.p[:node_pos][] = action.p[:node_pos][] # trigger change
elseif state == false
action.src = action.dst = action.init = nothing
end
end
####
#### Click Interaction
####
"""
mutable struct ClickHandler{P<:ScenePlot, F} <: GraphInteraction
Object to handle left mouse clicks on `plot::P`.
"""
mutable struct ClickHandler{P<:ScenePlot,F} <: GraphInteraction
plot::Union{Nothing,P}
fun::F
end
set_nodeplot!(h::ClickHandler{Scatter}, plot) = h.plot = plot
set_edgeplot!(h::ClickHandler{EdgePlot}, plot) = h.plot = plot
function process_interaction(handler::ClickHandler, event::MouseEvent, axis)
if event.type === MouseEventTypes.leftclick
(element, idx) = convert_selection(pick(axis.scene)...)
if element == handler.plot
ret = handler.fun(idx, event, axis)
return ret isa Bool ? ret : false
end
end
return false
end
"""
NodeClickHandler(fun)
Initializes `ClickHandler` for nodes. Calls function
fun(idx, event, axis)
on left-click events where `idx` is the node index.
# Example
```
julia> using Makie.Colors
julia> g = wheel_digraph(10)
julia> f, ax, p = graphplot(g, node_size=30, node_color=[colorant"red" for i in 1:nv(g)])
julia> function action(idx, event, axis)
p.node_color[][idx] = rand(RGB)
p.node_color[] = p.node_color[]
end
julia> register_interaction!(ax, :nodeclick, NodeClickHandler(action))
```
"""
NodeClickHandler(fun::F) where {F} = ClickHandler{Scatter,F}(nothing, fun)
"""
EdgeClickHandler(fun)
Initializes `ClickHandler` for edges. Calls function
fun(idx, event, axis)
on left-click events where `idx` is the edge index.
# Example
```
julia> using Makie.Colors
julia> g = wheel_digraph(10)
julia> f, ax, p = graphplot(g, edge_width=4, edge_color=[colorant"black" for i in 1:ne(g)])
julia> function action(idx, event, axis)
p.edge_color[][idx] = rand(RGB)
p.edge_color[] = p.edge_color[]
end
julia> register_interaction!(ax, :edgeclick, EdgeClickHandler(action))
```
"""
EdgeClickHandler(fun::F) where {F} = ClickHandler{EdgePlot,F}(nothing, fun)
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 31363 | using LinearAlgebra: normalize, ⋅, norm
using NetworkLayout: AbstractLayout, dim
export GraphPlot, graphplot, graphplot!, Arrow
const Arrow = Makie.Polygon(Point2f.([(-0.5,-0.5),(0.5,0),(-0.5,0.5),(-0.25,0)]))
"""
graphplot(graph::AbstractGraph)
graphplot!(ax, graph::AbstractGraph)
Creates a plot of the network `graph`. Consists of multiple steps:
- Layout the nodes: see `layout` attribute. The node position is accessible from outside
the plot object `p` as an observable using `p[:node_pos]`.
- plot edges as `edgeplot`-plot
- if `arrow_show` plot arrowheads as `scatter`-plot
- plot nodes as `scatter`-plot
- if `nlabels!=nothing` plot node labels as `text`-plot
- if `elabels!=nothing` plot edge labels as `text`-plot
The main attributes for the subplots are exposed as attributes for `graphplot`.
Additional attributes for the `scatter`, `edgeplot` and `text` plots can be provided
as a named tuples to `node_attr`, `edge_attr`, `nlabels_attr` and `elabels_attr`.
Most of the arguments can be either given as a vector of length of the
edges/nodes or as a single value. One might run into errors when changing the
underlying graph and therefore changing the number of Edges/Nodes.
## Attributes
### Main attributes
- `layout=Spring()`: function `AbstractGraph->Vector{Point}` or `Vector{Point}` determines the base layout
- `node_color=automatic`:
Defaults to `scatter_theme.color` in absence of `ilabels`.
- `node_size=automatic`:
Defaults to `scatter_theme.markersize` in absence of `ilabels`. Otherwise choses node size based on `ilabels` size.
- `node_marker=automatic`:
Defaults to `scatter_theme.marker` in absence of `ilabels`.
- `node_strokewidth=automatic`
Defaults to `scatter_theme.strokewidth` in absence of `ilabels`.
- `node_attr=(;)`: List of kw arguments which gets passed to the `scatter` command
- `edge_color=lineseg_theme.color`: Color for edges.
- `edge_width=lineseg_theme.linewidth`: Pass a vector with 2 width per edge to
get pointy edges.
- `edge_attr=(;)`: List of kw arguments which gets passed to the `linesegments` command
- `arrow_show=Makie.automatic`: `Bool`, indicate edge directions with arrowheads?
Defaults to `Graphs.is_directed(graph)`.
- `arrow_marker='➤'`
- `arrow_size=scatter_theme.markersize`: Size of arrowheads.
- `arrow_shift=0.5`: Shift arrow position from source (0) to dest (1) node.
If `arrow_shift=:end`, the arrowhead will be placed on the surface of the destination node
(assuming the destination node is circular).
- `arrow_attr=(;)`: List of kw arguments which gets passed to the `scatter` command
### Node labels
The position of each label is determined by the node position plus an offset in
data space.
- `nlabels=nothing`: `Vector{String}` with label for each node
- `nlabels_align=(:left, :bottom)`: Anchor of text field.
- `nlabels_distance=0.0`: Pixel distance from node in direction of align.
- `nlabels_color=labels_theme.color`
- `nlabels_offset=nothing`: `Point` or `Vector{Point}` (in data space)
- `nlabels_fontsize=labels_theme.fontsize`
- `nlabels_attr=(;)`: List of kw arguments which gets passed to the `text` command
### Inner node labels
Put labels inside the marker. If labels are provided, change default attributes to
`node_marker=Circle`, `node_strokewidth=1` and `node_color=:gray80`.
The `node_size` will match size of the `ilabels`.
- `ilabels=nothing`: `Vector` with label for each node
- `ilabels_color=labels_theme.color`
- `ilabels_fontsize=labels_theme.fontsize`
- `ilabels_attr=(;)`: List of kw arguments which gets passed to the `text` command
### Edge labels
The base position of each label is determined by `src + shift*(dst-src)`. The
additional `distance` parameter is given in pixels and shifts the text away from
the edge.
- `elabels=nothing`: `Vector{String}` with label for each edge
- `elabels_align=(:center, :center)`: Anchor of text field.
- `elabels_side = :left`: Side of the edge to put the edge label text
- `elabels_distance=Makie.automatic`: Pixel distance of anchor to edge. The direction is decided based on `elabels_side`
- `elabels_shift=0.5`: Position between src and dst of edge.
- `elabels_rotation=Makie.automatic`: Angle of text per label. If `nothing` this will be
determined by the edge angle. If `automatic` it will also point upwards making it easy to read.
- `elabels_offset=nothing`: Additional offset in data space
- `elabels_color=labels_theme.color`
- `elabels_fontsize=labels_theme.fontsize`
- `elabels_attr=(;)`: List of kw arguments which gets passed to the `text` command
### Curvy edges & self edges/loops
- `edge_plottype=Makie.automatic()`: Either `automatic`, `:linesegments` or
`:beziersegments`. `:beziersegments` are much slower for big graphs!
Self edges / loops:
- `selfedge_size=Makie.automatic()`: Size of selfloop (dict/vector possible).
- `selfedge_direction=Makie.automatic()`: Direction of center of the selfloop as `Point2` (dict/vector possible).
- `selfedge_width=Makie.automatic()`: Opening of selfloop in rad (dict/vector possible).
- Note: If valid waypoints are provided for selfloops, the selfedge attributes above will be ignored.
High level interface for curvy edges:
- `curve_distance=0.1`:
Specify a distance of the (now curved) line to the straight line *in data
space*. Can be single value, array or dict. User provided `tangents` or
`waypoints` will overrule this property.
- `curve_distance_usage=Makie.automatic()`:
If `Makie.automatic()`, only plot double edges in a curvy way. Other options
are `true` and `false`.
Tangents interface for curvy edges:
- `tangents=nothing`:
Specify a pair of tangent vectors per edge (for src and dst). If `nothing`
(or edge idx not in dict) draw a straight line.
- `tfactor=0.6`:
Factor is used to calculate the bezier waypoints from the (normalized) tangents.
Higher factor means bigger radius. Can be tuple per edge to specify different
factor for src and dst.
- Note: Tangents are ignored on selfloops if no waypoints are provided.
Waypoints along edges:
- `waypoints=nothing`
Specify waypoints for edges. This parameter should be given as a vector or
dict. Waypoints will be crossed using natural cubic splines. The waypoints may
or may not include the src/dst positions.
- `waypoint_radius=nothing`
If the attribute `waypoint_radius` is `nothing` or `:spline` the waypoints will
be crossed using natural cubic spline interpolation. If number (dict/vector
possible), the waypoints won't be reached, instead they will be connected with
straight lines which bend in the given radius around the waypoints.
"""
@recipe(GraphPlot, graph) do scene
# TODO: figure out this whole theme business
scatter_theme = default_theme(scene, Scatter)
lineseg_theme = default_theme(scene, LineSegments)
labels_theme = default_theme(scene, Makie.Text)
Attributes(
layout = Spring(),
# node attributes (Scatter)
node_color = automatic,
node_size = automatic,
node_marker = automatic,
node_strokewidth = automatic,
node_attr = (;),
# edge attributes (LineSegements)
edge_color = lineseg_theme.color,
edge_width = lineseg_theme.linewidth,
edge_attr = (;),
# arrow attributes (Scatter)
arrow_show = automatic,
arrow_marker = '➤',
arrow_size = scatter_theme.markersize,
arrow_shift = 0.5,
arrow_attr = (;),
# node label attributes (Text)
nlabels = nothing,
nlabels_align = (:left, :bottom),
nlabels_distance = 0.0,
nlabels_color = labels_theme.color,
nlabels_offset = nothing,
nlabels_fontsize = labels_theme.fontsize,
nlabels_attr = (;),
# inner node labels
ilabels = nothing,
ilabels_color = labels_theme.color,
ilabels_fontsize = labels_theme.fontsize,
ilabels_attr = (;),
# edge label attributes (Text)
elabels = nothing,
elabels_align = (:center, :center),
elabels_side = :left,
elabels_distance = automatic,
elabels_shift = 0.5,
elabels_rotation = automatic,
elabels_offset = nothing,
elabels_color = labels_theme.color,
elabels_fontsize = labels_theme.fontsize,
elabels_attr = (;),
# self edge attributes
edge_plottype = automatic,
selfedge_size = automatic,
selfedge_direction = automatic,
selfedge_width = automatic,
curve_distance = 0.1,
curve_distance_usage = automatic,
tangents=nothing,
tfactor=0.6,
waypoints=nothing,
waypoint_radius=nothing,
)
end
function Makie.plot!(gp::GraphPlot)
graph = gp[:graph]
dfth = default_theme(gp.parent, GraphPlot)
# create initial vertex positions, will be updated on changes to graph or layout
# make node_position-Observable available as named attribute from the outside
gp[:node_pos] = @lift if $(gp.layout) isa AbstractVector
if length($(gp.layout)) != nv($graph)
throw(ArgumentError("The length of the layout vector does not match the number of nodes in the graph!"))
else
Pointf.($(gp.layout))
end
else
[Pointf(p) for p in ($(gp.layout))($graph)]
end
sc = Makie.parent_scene(gp)
# function which projects the point in px space
to_px = lift(gp, sc.viewport, sc.camera.projectionview) do pxa, pv
# project should transform to 2d point in px space
(point) -> project(sc, point)
end
# get angle in px space from path p at point t
to_angle = @lift (path, p0, t) -> begin
# TODO: maybe shorter tangent? For some perspectives this might give wrong angles in 3d
p1 = p0 + tangent(path, t)
any(isnan, p1) && return 0.0 # lines with zero lengths might lead to NaN tangents
tpx = $to_px(p1) - $to_px(p0)
atan(tpx[2], tpx[1])
end
node_pos = gp[:node_pos]
# plot inside labels
scatter_theme = default_theme(sc, Scatter)
if gp[:ilabels][] !== nothing
positions = node_pos
ilabels_plot = text!(gp, positions;
text=@lift(string.($(gp.ilabels))),
align=(:center, :center),
color=gp.ilabels_color,
fontsize=gp.ilabels_fontsize,
gp.ilabels_attr...)
translate!(ilabels_plot, 0, 0, 1)
node_size_m = lift(ilabels_plot.plots[1][1], gp.ilabels_fontsize, gp.node_size) do glyphcollections, ilabels_fontsize, node_size
map(enumerate(glyphcollections)) do (i, gc)
_ns = getattr(node_size, i)
if _ns == automatic
rect = Rect2f(Makie.unchecked_boundingbox(gc, Quaternion((1,0,0,0))))
norm(rect.widths) + 0.1 * ilabels_fontsize
else
_ns
end
end
end
else
node_size_m = @lift $(gp.node_size) === automatic ? scatter_theme.markersize[] : $(gp.node_size)
end
node_color_m = @lift if $(gp.node_color) === automatic
gp.ilabels[] !== nothing ? :gray80 : scatter_theme.color[]
else
$(gp.node_color)
end
node_marker_m = @lift if $(gp.node_marker) === automatic
gp.ilabels[] !== nothing ? Circle : scatter_theme.marker[]
else
$(gp.node_marker)
end
node_strokewidth_m = @lift if $(gp.node_strokewidth) === automatic
gp.ilabels[] !== nothing ? 1.0 : scatter_theme.strokewidth[]
else
$(gp.node_strokewidth)
end
# compute initial edge paths; will be adjusted later if arrow_shift = :end
# create array of pathes triggered by node_pos changes
# in case of a graph change the node_position will change anyway
init_edge_paths = lift(node_pos, gp.selfedge_size,
gp.selfedge_direction, gp.selfedge_width, gp.curve_distance_usage, gp.curve_distance) do pos, s, d, w, cdu, cd
find_edge_paths(graph[], gp.attributes, pos)
end
# plot arrow heads
arrow_shift_m = lift(init_edge_paths, to_px, gp.arrow_shift, node_marker_m, node_size_m, gp.arrow_size) do paths, tpx, shift, nmarker, nsize, asize
update_arrow_shift(graph[], gp, paths, tpx, node_marker_m, node_size_m, shift)
end
arrow_pos = @lift if !isempty(init_edge_paths[])
broadcast(interpolate, init_edge_paths[], $arrow_shift_m)
else # if no edges return (empty) vector of points, broadcast yields Vector{Any} which can't be plotted
Vector{eltype(node_pos[])}()
end
arrow_rot = @lift if !isempty(init_edge_paths[])
Billboard(broadcast($to_angle, init_edge_paths[], $arrow_pos, $(arrow_shift_m)))
else
Billboard(Float32[])
end
# update edge paths to line up with arrow heads if arrow_shift = :end
edge_paths = gp[:edge_paths] = @lift map($init_edge_paths, $arrow_pos, eachindex($arrow_pos)) do ep, ap, i
if getattr($(gp.arrow_shift), i) == :end
adjust_endpoint(ep, ap)
else
ep
end
end
# actually plot edges
edge_plot = gp[:edge_plot] = edgeplot!(gp, edge_paths;
plottype=gp[:edge_plottype][],
color=prep_edge_attributes(gp.edge_color, graph, dfth.edge_color),
linewidth=prep_edge_attributes(gp.edge_width, graph, dfth.edge_width),
gp.edge_attr...)
# arrow plots
arrow_show_m = @lift $(gp.arrow_show) === automatic ? Graphs.is_directed($graph) : $(gp.arrow_show)
arrow_plot = gp[:arrow_plot] = scatter!(gp,
arrow_pos;
marker = prep_edge_attributes(gp.arrow_marker, graph, dfth.arrow_marker),
markersize = prep_edge_attributes(gp.arrow_size, graph, dfth.arrow_size),
color=prep_edge_attributes(gp.edge_color, graph, dfth.edge_color),
rotation = arrow_rot,
strokewidth = 0.0,
markerspace = :pixel,
visible = arrow_show_m,
gp.arrow_attr...)
# plot vertices
vertex_plot = gp[:node_plot] = scatter!(gp, node_pos;
color=prep_vertex_attributes(node_color_m, graph, scatter_theme.color),
marker=prep_vertex_attributes(node_marker_m, graph, scatter_theme.marker),
markersize=prep_vertex_attributes(node_size_m, graph, scatter_theme.markersize),
strokewidth=prep_vertex_attributes(node_strokewidth_m, graph, scatter_theme.strokewidth),
gp.node_attr...)
# plot node labels
if gp.nlabels[] !== nothing
positions = @lift begin
if $(gp.nlabels_offset) != nothing
$node_pos .+ $(gp.nlabels_offset)
else
copy($node_pos)
end
end
offset = @lift if $(gp.nlabels_align) isa Vector
$(gp.nlabels_distance) .* align_to_dir.($(gp.nlabels_align))
else
$(gp.nlabels_distance) .* align_to_dir($(gp.nlabels_align))
end
nlabels_plot = gp[:nlabels_plot] = text!(gp, positions;
text=prep_vertex_attributes(gp.nlabels, graph, Observable("")),
align=prep_vertex_attributes(gp.nlabels_align, graph, dfth.nlabels_align),
color=prep_vertex_attributes(gp.nlabels_color, graph, dfth.nlabels_color),
offset=offset,
fontsize=prep_vertex_attributes(gp.nlabels_fontsize, graph, dfth.nlabels_fontsize),
gp.nlabels_attr...)
end
# plot edge labels
if gp.elabels[] !== nothing
# positions: center point between nodes + offset + distance*normal + shift*edge direction
positions = @lift begin
pos = broadcast(interpolate, $edge_paths, $(gp.elabels_shift))
if $(gp.elabels_offset) !== nothing
pos .= pos .+ $(gp.elabels_offset)
end
pos
end
# rotations based on the edge_vec_px and opposite argument
rotation = lift(gp.elabels_rotation, to_angle, positions) do elabrots, tangle, pos
rot = broadcast(tangle, edge_paths[], pos, gp.elabels_shift[])
for i in 1:ne(graph[])
valrot = getattr(gp.elabels_rotation, i, nothing)
if valrot isa Real
# fix rotation to a single angle
rot[i] = valrot
elseif valrot == automatic
# point the labels up
if (rot[i] > π/2 || rot[i] < - π/2)
rot[i] += π
end
end
end
return rot
end
# calculate the offset in pixels in normal direction to the edge
offsets = lift(positions, to_px, gp.elabels_distance, gp.elabels_side) do pos, tpx, dist, side
tangent_px = broadcast(edge_paths[], pos, gp.elabels_shift[]) do path, p0, t
p1 = p0 + tangent(path, t)
tpx(p1) - tpx(p0)
end
offsets = map(p -> Point(-p.data[2], p.data[1])/norm(p), tangent_px)
offsets .= elabels_distance_offset(graph[], gp.attributes) .* offsets
end
elabels_plot = gp[:elabels_plot] = text!(gp, positions;
text=prep_edge_attributes(gp.elabels, graph, Observable("")),
rotation=rotation,
offset=offsets,
align=prep_edge_attributes(gp.elabels_align, graph, dfth.elabels_align),
color=prep_edge_attributes(gp.elabels_color, graph, dfth.elabels_color),
fontsize=prep_edge_attributes(gp.elabels_fontsize, graph, dfth.elabels_fontsize),
gp.elabels_attr...)
end
return gp
end
"""
elabels_distance_offset(g, attrs)
Returns the elabels_distance taking into consideration elabels_side
"""
function elabels_distance_offset(g, attrs)
offs = zeros(ne(g))
for i in 1:ne(g)
attrval = getattr(attrs.elabels_distance, i, automatic)
attrvalside = getattr(attrs.elabels_side, i, :left)
if attrval isa Real
if attrvalside == :left
offs[i] = attrval
elseif attrvalside == :right
offs[i] = -attrval
elseif attrvalside == :center
offs[i] = zero(attrval)
end
elseif attrval == automatic
offval = (getattr(attrs.elabels_fontsize, i) + getattr(attrs.edge_width, i))/2
if attrvalside == :left
offs[i] = offval
elseif attrvalside == :right
offs[i] = -offval
elseif attrvalside == :center
offs[i] = zero(offval)
end
end
end
return offs
end
"""
find_edge_paths(g, attr, pos::AbstractVector{PT}) where {PT}
Returns an `AbstractPath` for each edge in the graph. Based on the `edge_plotype` attribute
this returns either arbitrary bezier curves or just lines.
"""
function find_edge_paths(g, attr, pos::AbstractVector{PT}) where {PT}
paths = Vector{AbstractPath{PT}}(undef, ne(g))
for (i, e) in enumerate(edges(g))
p1, p2 = pos[src(e)], pos[dst(e)]
tangents = getattr(attr.tangents, i)
tfactor = getattr(attr.tfactor, i)
waypoints::Vector{PT} = getattr(attr.waypoints, i, PT[])
if !isnothing(waypoints) && !isempty(waypoints) #remove p1 and p2 from waypoints if these are given
waypoints[begin] == p1 && popfirst!(waypoints)
waypoints[end] == p2 && pop!(waypoints)
end
cdu = getattr(attr.curve_distance_usage, i)
if cdu === true
curve_distance = getattr(attr.curve_distance, i, 0.0)
elseif cdu === false
curve_distance = 0.0
elseif cdu === automatic
if is_directed(g) && has_edge(g, dst(e), src(e))
curve_distance = getattr(attr.curve_distance, i, 0.0)
else
curve_distance = 0.0
end
end
if !isnothing(waypoints) && !isempty(waypoints) #there are waypoints
radius = getattr(attr.waypoint_radius, i, nothing)
if radius === nothing || radius === :spline
paths[i] = Path(p1, waypoints..., p2; tangents, tfactor)
elseif radius isa Real
paths[i] = Path(radius, p1, waypoints..., p2)
else
throw(ArgumentError("Invalid radius $radius for edge $i!"))
end
elseif src(e) == dst(e) # selfedge
size = getattr(attr.selfedge_size, i)
direction = getattr(attr.selfedge_direction, i)
width = getattr(attr.selfedge_width, i)
paths[i] = selfedge_path(g, pos, src(e), size, direction, width)
elseif !isnothing(tangents)
paths[i] = Path(p1, p2; tangents, tfactor)
elseif PT<:Point2 && !iszero(curve_distance)
paths[i] = curved_path(p1, p2, curve_distance)
else # straight line
paths[i] = Path(p1, p2)
end
end
plottype = attr[:edge_plottype][]
if plottype === :beziersegments
# user explicitly specified beziersegments
return paths
elseif plottype === :linesegments
# user specified linesegments but there are non-lines
if !isempty(paths)
return straighten.(paths)
else
return Line{PT}[]
end
elseif plottype === automatic
ls = try
first(attr.edge_attr.linestyle[])
catch
nothing
end
if !isnothing(ls) && !isa(ls, Number)
throw(ArgumentError("Linestyles per edge are only supported when passing `edge_plottype=:beziersegments` to `graphplot` explicitly."))
end
# try to narrow down the typ, i.e. just `Line`s
# update :plottype in attr so this is persistent even if the graph changes
T = isempty(paths) ? Line{PT} : mapreduce(typeof, promote_type, paths)
if T <: Line
attr[:edge_plottype][] = :linesegments
return convert(Vector{T}, paths)
elseif ne(g) > 500
attr[:edge_plottype][] = :linesegments
@warn "Since there are a lot of edges ($(ne(g)) > 500), they will be drawn as straight lines "*
"even though they contain curvy edges. If you really want to plot them as "*
"bezier curves pass `edge_plottype=:beziersegments` explicitly. This will have "*
"much worse performance!"
return straighten.(paths)
else
attr[:edge_plottype][] = :beziersegments
return paths
end
else
throw(ArgumentError("Invalid argument for edge_plottype: $plottype"))
end
end
"""
selfedge_path(g, pos, v, size, direction, width)
Return a BezierPath for a selfedge.
"""
function selfedge_path(g, pos::AbstractVector{<:Point2}, v, size, direction, width)
vp = pos[v]
# get the vectors to all the neighbors
ndirs = [pos[n] - vp for n in all_neighbors(g, v) if n != v]
# angle and maximum width of loop
γ, Δ = 0.0, 0.0
if direction === automatic && !isempty(ndirs)
angles = SVector{length(ndirs)}(atan(p[2], p[1]) for p in ndirs)
angles = sort(angles)
for i in 1:length(angles)
α = angles[i]
β = get(angles, i+1, 2π + angles[1])
if β-α > Δ
Δ = β-α
γ = (β+α) / 2
end
end
# set width of selfloop
Δ = min(.7*Δ, π/2)
elseif direction === automatic && isempty(ndirs)
γ = π/2
Δ = π/2
else
@assert direction isa Point2 "Direction of selfedge should be 2 dim vector ($direction)"
γ = atan(direction[2], direction[1])
Δ = π/2
end
if width !== automatic
Δ = width
end
# the size (max distance to v) of loop
# if there are no neighbors set to 0.5. Else half dist to nearest neighbor
if size === automatic
size = isempty(ndirs) ? 0.5 : minimum(norm.(ndirs)) * 0.5
end
# the actual length of the tagent vectors, magic number from `CurveTo`
l = Float32( size/(cos(Δ/2) * 2*0.375) )
t1 = vp + l * Point2f(cos(γ-Δ/2), sin(γ-Δ/2))
t2 = vp + l * Point2f(cos(γ+Δ/2), sin(γ+Δ/2))
return BezierPath([MoveTo(vp),
CurveTo(t1, t2, vp)])
end
function selfedge_path(g, pos::AbstractVector{<:Point3}, v, size, direction, width)
error("Self edges in 3D not yet supported")
end
"""
curved_path(p1, p2, curve_distance)
Return a BezierPath for a curved edge (not selfedge).
"""
function curved_path(p1::PT, p2::PT, curve_distance) where {PT}
d = curve_distance
s = norm(p2 - p1)
γ = 2*atan(2 * d/s)
a = (p2 - p1)/s * (4*d^2 + s^2)/(3s)
m = @SMatrix[cos(γ) -sin(γ); sin(γ) cos(γ)]
c1 = PT(p1 + m*a)
c2 = PT(p2 - transpose(m)*a)
return BezierPath([MoveTo(p1), CurveTo(c1, c2, p2)])
end
"""
edgeplot(paths::Vector{AbstractPath})
edgeplot!(sc, paths::Vector{AbstractPath})
Recipe to draw the edges. Attribute `plottype` can be either.
If `eltype(pathes) <: Line` draw using `linesegments`. If `<:AbstractPath`
draw using bezier segments.
All attributes are passed down to the actual recipe.
"""
@recipe(EdgePlot, paths) do scene
Attributes(plottype=automatic)
end
function Makie.plot!(p::EdgePlot)
N = length(p[:paths][])
plottype = pop!(p.attributes, :plottype)[]
alllines = eltype(p[:paths][]) <: Line
if alllines && plottype != :beziersegments
PT = ptype(eltype(p[:paths][]))
segs = Observable(Vector{PT}(undef, 2*N))
update_segments!(segs, p[:paths][]) # first call to initialize
on(p[:paths]) do paths # update if pathes change
update_segments!(segs, paths)
end
linesegments!(p, segs; p.attributes...)
elseif plottype != :linesegments
beziersegments!(p, p[:paths]; p.attributes...)
else
error("Impossible combination of plottype=$plottype and alllines=$alllines.")
end
return p
end
function update_segments!(segs, paths)
N = length(paths)
if length(segs[]) != 2*N
resize!(segs[], 2*N)
end
for (i, p) in enumerate(paths)
segs[][2*i - 1] = interpolate(p, 0.0)
segs[][2*i] = interpolate(p, 1.0)
end
notify(segs)
end
"""
beziersegments(paths::Vector{AbstractPath})
beziersegments!(sc, paths::Vector{AbstractPath})
Recipe to draw bezier pathes. Each path will be descritized and ploted with a
separate `lines` plot. Scalar attributes will be used for all subplots. If you
provide vector attributs of same length als the pathes the i-th `lines` subplot
will see the i-th attribute.
TODO: Beziersegments plot won't work if the number of pathes changes.
"""
@recipe(BezierSegments, paths) do scene
Attributes(default_theme(scene, LineSegments)...)
end
function Makie.plot!(p::BezierSegments)
N = length(p[:paths][])
PT = ptype(eltype(p[:paths][]))
attr = p.attributes
if N == 0 # no edges, still need to plot some atomic element otherwise recipe does not work
lines!(p, PT[])
return p
end
# set colorange automaticially if needed
if attr[:color][] isa AbstractArray{<:Number}
numbers = attr[:color][]
colorrange = get(attr, :colorrange, nothing) |> to_value
if colorrange === Makie.automatic
attr[:colorrange] = extrema(numbers)
end
end
# array which holds observables for the discretized values
# this is needed so the `lines!` subplots will update
disc = [Observable{Vector{PT}}() for i in 1:N]
update_discretized!(disc, p[:paths][]) # first call to initialize
on(p[:paths]) do paths # update if pathes change
update_discretized!(disc, paths)
end
# plot all the lines
for i in 1:N
# each subplot will pick its specic arguments if there are vectors
specific_attr = Attributes()
for (k, v) in attr
if k === :color && v[] isa AbstractVector{<:Number} && length(v[]) == N
colormap = attr[:colormap][] |> to_colormap
colorrange = attr[:colorrange][]
specific_attr[k] = @lift Makie.interpolated_getindex(colormap,
Float64($v[i]),
colorrange)
elseif k === :linestyle && v[] isa AbstractVector && first(v[]) isa Number
# linestyle may be vector of numbers to specify pattern, in that case don't split
specific_attr[k] = v
elseif v[] isa AbstractVector && length(v[]) == N
specific_attr[k] = @lift $v[i]
else
specific_attr[k] = v
end
end
lines!(p, disc[i]; specific_attr...)
end
return p
end
function update_discretized!(disc, pathes)
for (i, p) in enumerate(pathes)
disc[i][] = discretize(p)
end
end
"""
update_arrow_shift(g, gp, edge_paths::Vector{<:AbstractPath{PT}}, to_px) where {PT}
Checks `arrow_shift` attr so that `arrow_shift = :end` gets transformed so that the arrowhead for that edge
lands on the surface of the destination node.
"""
function update_arrow_shift(g, gp, edge_paths::Vector{<:AbstractPath{PT}}, to_px, node_markers, node_sizes, shift) where {PT}
arrow_shift = Vector{Float32}(undef, ne(g))
for (i,e) in enumerate(edges(g))
t = getattr(shift, i, 0.5)
if t === :end
j = dst(e)
p0 = getattr(gp.node_pos, j)
node_marker = getattr(node_markers, j)
node_size = getattr(node_sizes, j)
arrow_marker = getattr(gp.arrow_marker, i)
arrow_size = getattr(gp.arrow_size, i)
d = distance_between_markers(node_marker, node_size, arrow_marker, arrow_size)
p1 = point_near_dst(edge_paths[i], p0, d, to_px)
t = inverse_interpolate(edge_paths[i], p1)
if isnan(t)
@warn """
Shifting arrowheads to destination nodes failed.
This can happen when the markers are inadequately scaled (e.g., when zooming out too far).
Arrow shift has been reset to 0.5.
"""
t = 0.5
end
end
arrow_shift[i] = t
end
return arrow_shift
end
function update_arrow_shift(g, gp, edge_paths::Vector{<:AbstractPath{<:Point3}}, to_px, shift)
arrow_shift = Vector{Float32}(undef, ne(g))
for (i,e) in enumerate(edges(g))
t = getattr(shift, i, 0.5)
if t === :end #not supported because to_px does not give pixels in 3D space (would need to map 3D coordinates to pixels...?)
error("`arrow_shift = :end` not supported for 3D plots.")
end
arrow_shift[i] = t
end
return arrow_shift
end
function Makie.preferred_axis_type(plot::Plot{GraphMakie.graphplot})
if haskey(plot.kw, :layout)
layout = plot.kw[:layout]
dim = _dimensionality(layout, plot[1][])
dim == 3 && return LScene
dim == 2 && return Axis
end
Axis
end
_dimensionality(layout::AbstractLayout, _) = dim(layout)
_dimensionality(layout::AbstractArray, _) = length(first(layout))
_dimensionality(layout, g) = length(first(layout(g)))
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 7919 | export get_edge_plot, get_arrow_plot, get_node_plot, get_nlabel_plot, get_elabel_plot
"Get the `EdgePlot` subplot from a `GraphPlot`."
get_edge_plot(gp::GraphPlot) = gp.edge_plot[]
"Get the scatter plot of the arrow heads from a `GraphPlot`."
get_arrow_plot(gp::GraphPlot) = gp.arrow_plot[]
"Get the scatter plot of the nodes from a `GraphPlot`."
get_node_plot(gp::GraphPlot) = gp.node_plot[]
"Get the text plot of the node labels from a `GraphPlot`."
get_nlabel_plot(gp::GraphPlot) = hasproperty(gp, :nlabels_plot) ? gp.nlabels_plot[] : nothing
"Get the text plot of the edge labels from a `GraphPlot`."
get_elabel_plot(gp::GraphPlot) = hasproperty(gp, :elabels_plot) ? gp.elabels_plot[] : nothing
"""
getedgekeys(gr::G, edgedat::D) where {G<:AbstractGraph, K<:AbstractEdge, D<:AbstractDict{K}, IsDirected{G}}
Return enumeration of edges for directed graph
"""
@traitfn function getedgekeys(gr::G, edgedat::D) where {G<:AbstractGraph, K<:AbstractEdge, D<:AbstractDict{K}; IsDirected{G}}
return edges(gr)
end
"""
getedgekeys(gr::G, edgedat::D) where {G<:AbstractGraph, K<:AbstractEdge, D<:AbstractDict{K}, IsDirected{G}}
Return enumeration of edges for undirected graph such that the user's keys are used
# Extended help
Wraps the `edges()` method such that the edges are referenced as the user defined them in the dictionary.
"""
@traitfn function getedgekeys(gr::G, edgedat::D) where {G<:AbstractGraph, K<:AbstractEdge, D<:AbstractDict{K}; !IsDirected{G}}
Iterators.map(e -> reverse(e) ∈ keys(edgedat) ? reverse(e) : e , edges(gr))
end
"""
getedgekeys(gr::AbstractGraph, <:AbstractDict{AbstractEdge})
Return enumeration of edge indices
"""
getedgekeys(gr::AbstractGraph, _) = 1:ne(gr)
"""
getattr(o::Observable, idx, default=nothing)
If observable wraps an AbstractVector or AbstractDict return
the value at idx. If dict has no key idx returns default.
Else return the one and only element.
"""
getattr(o::Observable, idx, default=nothing) = getattr(o[], idx, default)
"""
getattr(x, idx, default=nothing)
If `x` wraps an AbstractVector or AbstractDict return
the value at idx. If dict has no key idx return default.
Else return the one and only element.
"""
function getattr(x, idx, default=nothing)
if x isa AbstractVector && !isa(x, Point)
return x[idx]
elseif x isa DefaultDict || x isa DefaultOrderedDict
return getindex(x, idx)
elseif x isa AbstractDict
return get(x, idx, default)
else
return x === nothing ? default : x
end
end
"""
prep_vertex_attributes(oattr::Observable, ograph::Observable{<:AbstractGraph}, odefault::Observable)
Prepare the vertex attributes to be forwarded to the internal recipes.
If the attribute is a `Vector` or single value forward it as is (or the `odefault` value if isnothing).
If it is an `AbstractDict` expand it to a `Vector` using `indices`.
"""
function prep_vertex_attributes(oattr::Observable, ograph::Observable{<:AbstractGraph}, odefault::Observable=Observable(nothing))
@lift begin
if issingleattribute($oattr)
isnothing($oattr) ? $odefault : $oattr
elseif $oattr isa AbstractVector
$oattr
else
[getattr($oattr, i, $odefault) for i in vertices($ograph)]
end
end
end
"""
prep_edge_attributes(oattr::Observable, ograph::Observable{<:AbstractGraph}, odefault::Observable)
Prepare the edge attributes to be forwarded to the internal recipes.
If the attribute is a `Vector` or single value forward it as is (or the `odefault` value if isnothing).
If it is an `AbstractDict` expand it to a `Vector` using `indices`.
"""
function prep_edge_attributes(oattr::Observable, ograph::Observable{<:AbstractGraph}, odefault::Observable=Observable(nothing))
@lift begin
if issingleattribute($oattr)
isnothing($oattr) ? $odefault : $oattr
elseif $oattr isa AbstractVector
$oattr
else
[getattr($oattr, i, $odefault) for i in getedgekeys($ograph, $oattr)]
end
end
end
"""
issingleattribute(x)
Return `true` if `x` represents a single attribute value
"""
issingleattribute(x) = isa(x, Point) || (!isa(x, AbstractVector) && !isa(x, AbstractDict))
"""
Pointf(p::Point{N, T})
Convert Point{N, T} or NTuple{N, T} to Point{N, Float32}.
"""
Pointf(p::Union{Point{N,T}, NTuple{N,T}}) where {N,T} = Point{N, Float32}(p)
Pointf(p::StaticVector{N, T}) where {N,T} = Point{N, Float32}(p)
Pointf(p::Vararg{T,N}) where {N,T} = Point{N, Float32}(p)
Pointf(p::Vector{T}) where {T} = Point{length(p), Float32}(p)
"""
align_to_dir(align::Tuple{Symbol, Symbol})
Given a tuple of alignment (i.e. `(:left, :bottom)`) return a normalized
2d vector which points in the direction of the offset.
"""
function align_to_dir(align::Tuple{Symbol, Symbol})
halign, valign = align
x = 0.0
if halign === :left
x = 1.0
elseif halign === :right
x = -1.0
end
y = 0.0
if valign === :top
y = -1.0
elseif valign === :bottom
y = 1.0
end
norm = x==y==0.0 ? 1 : sqrt(x^2 + y^2)
return Point2f(x/norm, y/norm)
end
"""
plot_controlpoints!(ax::Axis, gp::GraphPlot)
plot_controlpoints!(ax::Axis, path::BezierPath)
Add all the bezier controlpoints of graph plot or a single
path to the axis `ax`.
"""
function plot_controlpoints!(ax::Axis, gp::GraphPlot)
ep = get_edge_plot(gp)
ep.plots[1] isa BezierSegments || return
ep = ep.plots[1]
paths = ep[:paths][]
for (i, p) in enumerate(paths)
p isa Line && continue
color = getattr(gp.edge_color, i)
plot_controlpoints!(ax, p; color)
end
end
function plot_controlpoints!(ax::Axis, p::BezierPath; color=:black)
for (j, c) in enumerate(p.commands)
if c isa CurveTo
segs = [p.commands[j-1].p, c.c1, c.p, c.c2]
linesegments!(ax, segs; color, linestyle=:dot)
scatter!(ax, [c.c1, c.c2]; color)
end
end
end
"""
scale_factor(marker)
Get base size (scaling) in pixels for `marker`.
"""
scale_factor(marker) = 1 #for 1x1 base sizes (Circle, Rect, Arrow)
function scale_factor(marker::Char)
if marker == '➤'
d = 0.675
else
d = 0.705 #set to the same value as :circle, but is really dependent on the Char
end
return d
end
function scale_factor(marker::Symbol)
size_factor = 0.75 #Makie.default_marker_map() has all markers scaled by 0.75
if marker == :circle #BezierCircle
r = 0.47
elseif marker in [:rect, :diamond, :vline, :hline] #BezierSquare
rmarker = 0.95*sqrt(pi)/2/2
r = sqrt(2*rmarker^2) #pithagoras to get radius of circle that circumscribes marker
elseif marker in [:utriangle, :dtriangle, :ltriangle, :rtriangle] #Bezier Triangles
r = 0.97/2
elseif marker in [:star4, :star5, :star6, :star8] #Bezier Stars
r = 0.6
else #Bezier Crosses/Xs and Ngons
r = 0.5
end
return 2*r*size_factor #get shape diameter
end
"""
distance_between_markers(marker1, size1, marker2, size2)
Calculate distance between 2 markers.
TODO: Implement for noncircular marker1.
(will require angle for line joining the 2 markers).
"""
function distance_between_markers(marker1, size1, marker2, size2)
marker1_scale = scale_factor(marker1)
marker2_scale = scale_factor(marker2)
d = marker1_scale*size1/2 + marker2_scale*size2/2
return d
end
"""
point_near_dst(edge_path, p0::PT, d, to_px) where {PT}
Find point near destination node along `edge_path` a
distance `d` pixels along the tangent line.
"""
function point_near_dst(edge_path, p0::PT, d, to_px) where {PT}
pt = tangent(edge_path, 1) #edge tangent at dst node
r = to_px(pt) - to_px(PT(0)) #direction vector in pixels
scale_px = 1 ./ (to_px(PT(1)) - to_px(PT(0)))
p1 = p0 - d*normalize(r)*scale_px
return p1
end
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 3976 | using Test
using GraphMakie
using GeometryBasics
using GraphMakie: BezierPath, MoveTo, LineTo, CurveTo, interpolate, discretize, tangent, Path, Line
@testset "interpolation and tangents" begin
path = BezierPath([
MoveTo(Point2f(0,0)),
LineTo(Point2f(0.5,0.5)),
CurveTo(Point2f(1,0),
Point2f(1,0),
Point2f(1,1)),
CurveTo(Point2f(1,2),
Point2f(0,2),
Point2f(0,1))])
lines(discretize(path))
ts = 0:0.1:1.0
pos = map(t->interpolate(path,t), ts)
tan = map(t->tangent(path,t), ts)
scatter!(pos)
arrows!(pos, tan; lengthscale=0.1)
path = BezierPath([
MoveTo(Point3f(0,0,0)),
LineTo(Point3f(1,0,0)),
LineTo(Point3f(1,1,1)),
LineTo(Point3f(0,1,1)),
LineTo(Point3f(0,0,1))])
ts = 0:0.1:1.0
pos = map(t->interpolate(path,t), ts)
tan = map(t->tangent(path,t), ts)
fig, ax, p = scatter(pos)
arrows!(pos, tan; lengthscale=0.1)
end
@testset "natural spline constructor" begin
path = Path(Point2f(0.0,0.0), Point2f(0.0,1.0))
@test path isa GraphMakie.Line
path = Path(Point2f(0.0,0.0),
Point2f(0.0,1.0),
Point2f(1.0,1.0))
lines(discretize(path))
scatter!(GraphMakie.waypoints(path))
path = Path(Point2f(0.0,0.0),
Point2f(-0.5,1.0),
Point2f(0.5,1.0),
Point2f(0.0,0.0))
lines(discretize(path))
scatter!(GraphMakie.waypoints(path))
path = Path(Point2f(0.0,0.0),
Point2f(-0.5,1.0),
Point2f(1.5,1.0),
Point2f(2.0,0.0))
lines(discretize(path))
scatter!(GraphMakie.waypoints(path))
path = Path(Point2f(0.0,0.0),
Point2f(-0.5,1.0),
Point2f(1.5,1.0),
Point2f(2.0,0.0);
tangents=(Point2f(-1,0),
Point2f(-1,0)))
lines(discretize(path), linewidth=10)
scatter!(GraphMakie.waypoints(path))
end
@testset "two points and tangents" begin
p1 = Point2f(0,0)
t1 = Point2f(0,1)
p2 = Point2f(1,1)
t2 = Point2f(0,1)
path = Path(p1, p2; tangents=(t1, t2))
lines(discretize(path))
scatter!(GraphMakie.waypoints(path))
p1 = Point2f(0,0)
t1 = Point2f(1,0)*10
p2 = Point2f(1,1)
t2 = Point2f(0,1)*5
path = Path(p1, p2; tangents=(t1, t2))
lines(discretize(path))
scatter!(GraphMakie.waypoints(path))
p1 = Point2f(0,0)
t1 = Point2f(-1,0)
p2 = Point2f(1,1)
t2 = Point2f(0,1)
path = Path(p1, p2; tangents=(t1, t2))
lines(discretize(path))
scatter!(GraphMakie.waypoints(path))
p1 = Point2f(0,0)
t1 = Point2f(-.5,.5)
p2 = Point2f(0,0)
t2 = Point2f(-.5,-.5)
path = Path(p1, p2; tangents=(t1, t2))
lines(discretize(path))
scatter!(GraphMakie.waypoints(path))
p1 = Point2f(0,0)
p2 = Point2f(1,0)
midp = Point2f[]
path = Path(p1, midp..., p2)
lines(discretize(path))
end
@testset "straight lines with radi" begin
using GraphMakie: plot_controlpoints!
p1 = Point2f(0,0)
p2 = Point2f(1,-.5)
p3 = Point2f(2,.5)
p4 = Point2f(3,0)
path = Path(0.5, p1, p2, p3, p4)
fig, ax, p = lines(discretize(path))
plot_controlpoints!(ax, path)
scatter!(ax, [p1,p2,p3,p4])
path = Path(0.0, p1, p2, p3, p4)
fig, ax, p = lines(discretize(path))
end
@testset "test beziersegments recipe" begin
using GraphMakie: beziersegments
paths = [Path(rand(Point2f, 4)...) for _ in 1:4]
fig, ax, p = beziersegments(paths; linewidth=[2,4,6,8], color=[1,2,3,4])
p.attributes
end
@testset "selfloop test" begin
using Graphs, GraphMakie
g = star_graph(10)
add_edge!(g, 1, 1)
add_edge!(g, 2, 2)
fig, ax, p = graphplot(g)
ax.aspect = DataAspect()
g = star_graph(10)
add_edge!(g, 1, 1)
@test_throws ErrorException graphplot(g; layout=_->rand(Point3f, 10))
end
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 3629 | using Graphs
using CairoMakie
using GraphMakie
using ReferenceTests
using Literate
using FileIO
const ASSETS = joinpath(@__DIR__, "..", "assets")
const EXAMPLE_BASEPATH = joinpath(@__DIR__, "..", "docs", "examples")
const TMPDIR = joinpath(ASSETS, "tmp")
isdir(TMPDIR) && rm(TMPDIR; recursive=true)
mkdir(TMPDIR)
const IMAGE_COUNTERS = Dict{String, Int}()
"""
@save_reference fig
Mark figs in example files as reference test image. Those images will
be saved as `filename-i.png` to the gobal temp dir defined in this script.
"""
macro save_reference(fig)
f = splitpath(string(__source__.file))[end]
postfix = if haskey(IMAGE_COUNTERS, f)
IMAGE_COUNTERS[f] += 1
else
IMAGE_COUNTERS[f] = 1
end
quote
path = joinpath(TMPDIR, $f*"-"*lpad($postfix, 2, "0")*".png")
save(path, $(esc(fig)), px_per_unit=1)
println(" saved fig $path")
end
end
@info "Generate reference images..."
# go through all examples in docs/examples
for exfile in filter(contains(r".jl$"), readdir(EXAMPLE_BASEPATH))
expath = joinpath(EXAMPLE_BASEPATH, exfile)
# check if `@save_reference` appears in the example
# if not, don't evaluate file
hasassets = false
for l in eachline(expath)
if contains(l, r"@save_reference")
hasassets = true
break
end
end
if !hasassets
@info "$exfile has no reference images!"
continue
end
# create script, include script (saves files), remove exported script
Literate.script(expath, TMPDIR)
script = joinpath(TMPDIR, exfile)
include(script)
rm(script)
end
oldassets = filter(contains(r".png$"), readdir(ASSETS))
newassets = filter(contains(r".png$"), readdir(TMPDIR))
function compare(ref, x)
if size(ref) != size(x)
return 0
end
return ReferenceTests._psnr(ref, x)
end
# now test all the generated graphics in the TMPDIR and compare against files in assets dir
@testset "Reference Tests" begin
for ass in oldassets
# skip unresolved conflicts
occursin(r"\+.png$", ass) && continue
old = joinpath(ASSETS, ass)
new = joinpath(TMPDIR, ass)
if !isfile(new)
@warn "New version for $ass missing! Delete file if not needed anymore."
@test false
continue
end
# equal = ReferenceTests.psnr_equality()(load(old), load(new))
score = compare(load(old), load(new))
MEH = 48
GOOD = 200
# basicially disable check on older julia versions
if VERSION < v"1.10"
MEH = 0;
end
if score > GOOD
printstyled(" ✓ [", repr(round(score, digits=1)), "] $ass\n"; color=:green)
@test true
rm(new)
else
if score > MEH
printstyled(" ? [", repr(round(score, digits=1)), "] $ass\n"; color=:yellow)
@test_broken false
else
printstyled(" × [", repr(round(score, digits=1)), "] $ass\n"; color=:red)
@test false
end
parts = rsplit(ass, "."; limit=2)
@assert length(parts) == 2
newname = parts[1] * "+." *parts[2]
mv(new, joinpath(ASSETS, newname), force=true)
@warn "There is a difference in $(ass)! New version moved to $newname. Resolve manually!"
end
end
for new in setdiff(newassets, oldassets)
printstyled(" × Move new asset $(new)!\n"; color=:red)
@test false
mv(joinpath(TMPDIR, new), joinpath(ASSETS, new))
end
rm(TMPDIR)
end
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | code | 9054 | using CairoMakie
using GraphMakie
using GraphMakie.Graphs
using GraphMakie.NetworkLayout
using Makie.Colors
using StaticArrays
using Test
include("beziercurves_test.jl")
@testset "GraphMakie.jl" begin
g = Observable(wheel_digraph(10))
f, ax, p = graphplot(g)
f, ax, p = graphplot(g, node_attr=Attributes(visible=false))
f, ax, p = graphplot(g, node_attr=(;visible=true))
# try to update graph
add_edge!(g[], 2, 4)
g[] = g[]
# try to update network
p.layout = SFDP()
# update node observables
p.node_color = :blue
p.node_size = 30
# update edge observables
p.edge_width = 5.0
p.edge_color = :green
# it should be also possible to pass multiple values
f, ax, p = graphplot(g, node_color=[rand([:blue,:red,:green]) for i in 1:nv(g[])])
end
@testset "graph without edges" begin
g = SimpleDiGraph(10)
graphplot(g)
graphplot(g; edge_plottype=:linesegments)
graphplot(g; edge_plottype=:beziersegments)
g = SimpleGraph(10)
graphplot(g)
graphplot(g; edge_plottype=:linesegments)
graphplot(g; edge_plottype=:beziersegments)
end
@testset "selfedge without neighbors" begin
g = SimpleGraph(10)
add_edge!(g, 1, 1)
graphplot(g)
end
@testset "small graphs" begin
g = SimpleGraph(1)
fig, ax, p = graphplot(g)
g = complete_graph(2)
graphplot(g)
end
@testset "single line width per edge" begin
g = complete_graph(3)
graphplot(g)
graphplot(g; edge_width=10)
graphplot(g; edge_width=[5, 10, 15])
graphplot(g; edge_width=[5, 10, 5, 10, 5, 10])
end
@testset "Hover, click and drag Interaction" begin
g = wheel_graph(10)
add_edge!(g, 1, 1)
f, ax, p = graphplot(g,
edge_width = [3.0 for i in 1:ne(g)],
edge_color = [colorant"black" for i in 1:ne(g)],
node_size = [20 for i in 1:nv(g)],
node_color = [colorant"red" for i in 1:nv(g)])
deregister_interaction!(ax, :rectanglezoom)
function edge_hover_action(s, idx, event, axis)
p.edge_width[][idx]= s ? 6.0 : 3.0
p.edge_width[] = p.edge_width[] # trigger observable
end
ehover = EdgeHoverHandler(edge_hover_action)
register_interaction!(ax, :ehover, ehover)
function node_hover_action(s, idx, event, axis)
p.node_size[][idx] = s ? 40 : 20
p.node_size[] = p.node_size[] # trigger observable
end
nhover = NodeHoverHandler(node_hover_action)
register_interaction!(ax, :nhover, nhover)
function node_click_action(idx, args...)
p.node_color[][idx] = rand(RGB)
p.node_color[] = p.node_color[]
end
nclick = NodeClickHandler(node_click_action)
register_interaction!(ax, :nclick, nclick)
function edge_click_action(idx, args...)
p.edge_color[][idx] = rand(RGB)
p.edge_color[] = p.edge_color[]
end
eclick = EdgeClickHandler(edge_click_action)
register_interaction!(ax, :eclick, eclick)
function node_drag_action(state, idx, event, axis)
p[:node_pos][][idx] = event.data
p[:node_pos][] = p[:node_pos][]
end
ndrag = NodeDragHandler(node_drag_action)
register_interaction!(ax, :ndrag, ndrag)
mutable struct EdgeDragAction
init::Union{Nothing, Point2f} # save click position
src::Union{Nothing, Point2f} # save src vertex position
dst::Union{Nothing, Point2f} # save dst vertex position
EdgeDragAction() = new(nothing, nothing, nothing)
end
function (action::EdgeDragAction)(state, idx, event, axis)
edge = collect(edges(g))[idx]
if state == true
if action.src===action.dst===action.init===nothing
action.init = event.data
action.src = p[:node_pos][][src(edge)]
action.dst = p[:node_pos][][dst(edge)]
end
offset = event.data - action.init
p[:node_pos][][src(edge)] = action.src + offset
p[:node_pos][][dst(edge)] = action.dst + offset
p[:node_pos][] = p[:node_pos][] # trigger change
elseif state == false
action.src = action.dst = action.init = nothing
end
end
edrag = EdgeDragHandler(EdgeDragAction())
register_interaction!(ax, :edrag, edrag)
end
@testset "align_to_direction" begin
using GraphMakie: align_to_dir
using LinearAlgebra: normalize
@test align_to_dir((:left, :center)) ≈ Point(1.0, 0)
@test align_to_dir((:right, :center)) ≈ Point(-1.0, 0)
@test align_to_dir((:center, :center)) ≈ Point(0.0, 0)
@test align_to_dir((:left, :top)) ≈ normalize(Point(1.0, -1.0))
@test align_to_dir((:right, :top)) ≈ normalize(Point(-1.0, -1))
@test align_to_dir((:center, :top)) ≈ normalize(Point(0.0, -1))
@test align_to_dir((:left, :bottom)) ≈ normalize(Point(1.0, 1.0))
@test align_to_dir((:right, :bottom)) ≈ normalize(Point(-1.0, 1.0))
@test align_to_dir((:center, :bottom)) ≈ normalize(Point(0.0, 1.0))
# g = complete_graph(9)
# nlabels_align = vec(collect(Iterators.product((:left,:center,:right),(:top,:center,:bottom))))
# nlabels= repr.(nlabels_align)
# graphplot(g; nlabels, nlabels_align, nlabels_distance=20)
end
@testset "test plot accessors" begin
g = complete_graph(10)
nlabels = repr.(1:nv(g))
elabels = repr.(1:ne(g))
fig, ax, p = graphplot(g)
@test get_edge_plot(p) isa EdgePlot
@test get_node_plot(p)[1][] == p[:node_pos][]
@test get_arrow_plot(p).visible[] == false
@test get_nlabel_plot(p) === nothing
@test get_elabel_plot(p) === nothing
fig, ax, p = graphplot(g; nlabels)
@test get_nlabel_plot(p)[:text][] == nlabels
@test get_elabel_plot(p) === nothing
fig, ax, p = graphplot(g; elabels)
@test get_nlabel_plot(p) === nothing
@test get_elabel_plot(p)[:text][] == elabels
fig, ax, p = graphplot(g; elabels, nlabels)
@test get_nlabel_plot(p)[:text][] == nlabels
@test get_elabel_plot(p)[:text][] == elabels
end
@testset "test Pointf" begin
using GraphMakie: Pointf
p = Point(0.0, 0.0)
@test typeof(Pointf(p)) == Point2f
@test Pointf(p) == Point2f(p)
p = Point(1, 0)
@test typeof(Pointf(p)) == Point2f
@test Pointf(p) == Point2f(p)
p = Point(0.0, 1.0, 2.0)
@test typeof(Pointf(p)) == Point3f
@test Pointf(p) == Point3f(p)
@test Pointf(0.0, 0.0, 0.0) isa Point3f
@test Pointf(1.0, 1.0) isa Point2f
@test Pointf((0.0, 0.0, 0.0 )) isa Point3f
@test Pointf((1.0, 1.0)) isa Point2f
@test Pointf([0.0, 0.0, 0.0]) isa Point3f
@test Pointf([1.0, 1.0]) isa Point2f
@test Pointf(SA[0.0, 0.0, 0.0]) isa Point3f
@test Pointf(SA[1.0, 1.0]) isa Point2f
g = complete_graph(3)
pos1 = [(0,0),
(1,1),
(0,1)]
pos2 = [[0,0],
[1,1],
[0,1]]
pos3 = [SA[0,0],
SA[1,1],
SA[0,1]]
@test isconcretetype(typeof(Pointf.(pos1)))
@test isconcretetype(typeof(Pointf.(pos2)))
@test isconcretetype(typeof(Pointf.(pos3)))
graphplot(g; layout=(x)->pos1)
graphplot(g; layout=(x)->pos2)
graphplot(g; layout=(x)->pos3)
end
@testset "test edges distance parameters" begin
g = SimpleDiGraph(3)
add_edge!(g, 1, 2)
add_edge!(g, 2, 3)
add_edge!(g, 3, 1)
graphplot(g)
add_edge!(g, 1, 3)
graphplot(g; curve_distance=0.2)
graphplot(g; curve_distance=0.2, curve_distance_usage=false)
graphplot(g; curve_distance=0.2, curve_distance_usage=true)
graphplot(g; curve_distance=collect(0.1:0.1:0.5))
graphplot(g; curve_distance=collect(0.1:0.1:0.5), curve_distance_usage=true)
end
@testset "separate linestyle per edge" begin
p = Point2f[(0,0), (0, 1), (0,0), (1,0)]
@test_broken display(linesegments(p; linestyle = [:dot, :dash]))
@test_throws ArgumentError graphplot(
DiGraph([Edge(1 => 2), Edge(2 => 1)]),
edge_attr = (; linestyle = [:dot, :dash]),
)
@test_throws ArgumentError graphplot(
DiGraph([Edge(1 => 2), Edge(2 => 3)]),
edge_attr = (; linestyle = [:dot, :dash]),
)
end
@testset "pan in scene after removal of plot" begin
g = wheel_graph(10)
fig, ax, p = graphplot(g)
delete!(ax, p)
rem_edge!(g, 1, 2)
ax.scene.camera.projectionview[] = ax.scene.camera.projectionview.val # Simlulate panning the screen
end
@testset "get correct axis type for arguments" begin
g = smallgraph(:petersen)
_, ax, _ = graphplot(g)
@test ax isa Axis
_, ax, _ = graphplot(g; layout=Stress(dim=3))
@test ax isa LScene
_, ax, _ = graphplot(g; layout=Stress(dim=2))
@test ax isa Axis
_, ax, _ = graphplot(g; layout=rand(Point2, nv(g)))
@test ax isa Axis
_, ax, _ = graphplot(g; layout=rand(Point3, nv(g)))
@test ax isa LScene
_, ax, _ = graphplot(g; layout=_->rand(Point2, nv(g)))
@test ax isa Axis
_, ax, _ = graphplot(g; layout=_->rand(Point3, nv(g)))
@test ax isa LScene
end
include("referencetests.jl")
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | docs | 1328 | # GraphMakie
[](http://graph.makie.org/stable)
[](http://graph.makie.org/dev/)
[](https://github.com/MakieOrg/GraphMakie.jl/actions)
[](https://codecov.io/gh/MakieOrg/GraphMakie.jl)
Plotting graphs¹... with [Makie](https://github.com/MakieOrg/Makie.jl)!
This package is just a plotting recipe, you still need to use one of the Makie backends.
`GraphMakie` is in an early development stage and might break often. Any
contribution such as suggesting features, raising issues and opening PRs are
welcome!
Starting from v0.3 `GraphMakie.jl` switches from `LightGraphs.jl` to `Graphs.jl` for the graph representation. See this [discourse post](https://discourse.julialang.org/t/lightgraphs-jl-transition/69526/17) for more information. If you want to use `LightGraphs.jl` please specifically `] add [email protected]`!
## Installation
``` julia
pkg> add GraphMakie
```
## Basic usage
```julia
using GLMakie
using GraphMakie
using Graphs
g = complete_graph(10)
graphplot(g)
```
----------------------------
¹the networky type with nodes and edges
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | docs | 3167 | ```@meta
CurrentModule = GraphMakie
```
# GraphMakie
This is the Documentation for [GraphMakie](https://github.com/MakieOrg/GraphMakie.jl).
This Package consists of two parts: a plot recipe for graphs types from
[Graphs.jl](https://github.com/JuliaGraphs/Graphs.jl) and some helper
functions to add interactions to those plots.
There are also [plot examples](generated/plots.md) and [interaction examples](generated/interactions.md) pages.
## The `graphplot` Recipe
```@docs
graphplot
```
## Passing arguments
The recipe can handle a range of argument types.
For all the arguments that support a collection of configurations per element, you pass-in a `Vector`.
However you can also pass in a `Dict` or a `DefaultDict` to only specify a configuration for a specific element of interest, while the rest get the default value.
The `keys` of the `Dict`ionaries are the `Int` index of the element or the `Edge` when reasonable.
See some demonstration on [Changes of node and label sizes](@ref), [Dict and DefaultDict](@ref) and [Use Dict{Edge} for edge arguments](@ref).
## Network Layouts
The layout algorithms are provided by [`NetworkLayout.jl`](https://github.com/JuliaGraphs/NetworkLayout.jl). See
the [docs](https://juliagraphs.org/NetworkLayout.jl/stable/) for a list of available layouts.
A layout has to be a function `f(g::AbstractGraph) -> pos::Vector{Point}`. You can also provide your
own layouts or use other packages like [`LayeredLayouts.jl`](https://github.com/oxinabox/LayeredLayouts.jl) for
DAG (see also the [Dependency Graph of a Package](@ref) example).
```julia
using LayeredLayouts
function mylayout(g::SimpleGraph)
xs, ys, _ = solve_positions(Zarate(), g)
return Point.(zip(xs, ys))
end
```
## Predefined Interactions
`GraphMakie.jl` provides some pre-built interactions to enable drag&drop of nodes and edges as well as highlight on hover.
To try them all use the following code in a `GLMakie` environment.
```julia
using GLMakie
using GraphMakie
using Graphs
g = wheel_graph(10)
f, ax, p = graphplot(g, edge_width=[3 for i in 1:ne(g)],
node_size=[10 for i in 1:nv(g)])
deregister_interaction!(ax, :rectanglezoom)
register_interaction!(ax, :nhover, NodeHoverHighlight(p))
register_interaction!(ax, :ehover, EdgeHoverHighlight(p))
register_interaction!(ax, :ndrag, NodeDrag(p))
register_interaction!(ax, :edrag, EdgeDrag(p))
```
```@docs
NodeHoverHighlight
EdgeHoverHighlight
NodeDrag
EdgeDrag
```
## Interaction Interface
`GraphMakie.jl` provides some helper functions to register interactions to your graph plot.
There are special interaction types for hovering, clicking and dragging nodes and edges.
For more information on the axis interaction please consult the [`Makie.jl` docs](https://docs.makie.org/stable/examples/blocks/axis/index.html#custom_interactions).
The general idea is to create some handler type, provide some action function and register it
as an interaction with the axes.
### Click Interactions
```@docs
NodeClickHandler
EdgeClickHandler
```
### Hover Interactions
```@docs
NodeHoverHandler
EdgeHoverHandler
```
### Drag Interactions
```@docs
NodeDragHandler
EdgeDragHandler
```
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.5.12 | c8c3ece1211905888da48e16f438af85e951ea55 | docs | 414 | ```@raw html
<meta http-equiv="refresh" content="0; url=https://juliagraphs.org/NetworkLayout.jl/stable/">
<script type="text/javascript">
window.location.href = "https://juliagraphs.org/NetworkLayout.jl/stable/"
</script>
If you are not redirected automatically, follow this <a href='https://juliagraphs.org/NetworkLayout.jl/stable/'>link to the documentation of NetworkLayout.jl</a>.
```
| GraphMakie | https://github.com/MakieOrg/GraphMakie.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | code | 956 | module Bplus
using BplusCore, BplusApp, BplusTools
@using_bplus_core
#TODO: Each sub-module should list its own important modules for B+ to automatically consume.
const SUB_MODULES = [
BplusCore => [:Utilities, :Math],
BplusApp => [ :GL, :GUI, :Input, :ModernGLbp ],
BplusTools => [ :ECS, :Fields, :SceneTree ]
]
for (sub_module, features) in SUB_MODULES
for feature_name in features
@eval const $feature_name = $sub_module.$feature_name
@eval export $feature_name
end
end
macro using_bplus()
# Import the modules as if they came directly from B+.
using_statements = [ ]
for (sub_module, features) in SUB_MODULES
push!(using_statements, :( using Bplus.$(Symbol(sub_module)) ))
for feature_name in features
push!(using_statements, :( using Bplus.$feature_name ))
end
end
return quote
$(using_statements...)
end
end
export @using_bplus
end # module
| Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | code | 627 | # Make sure the test is always running in the same directory and within the same project.
using Pkg
const MAIN_PROJECT_DIR = joinpath(@__DIR__, "..")
function reset_julia_env()
cd(MAIN_PROJECT_DIR)
Pkg.activate(".")
end
reset_julia_env()
# Run each sub-package's unit tests.
using Bplus
for (sub_package, modules) in Bplus.SUB_MODULES
sub_package_name = Symbol(sub_package)
@eval module $(Symbol(:Test_, sub_package_name))
using $sub_package_name
include(joinpath(
pathof($sub_package_name), "..", "..",
"test", "runtests.jl"
))
end
reset_julia_env()
end | Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 10669 | # B+
A game and 3D graphics framework for Julia, using OpenGL 4.6 + bindless textures.
Offers game math, wrappers for OpenGL/GLFW/Dear ImGUI, scene graph algorithms, and numerous other modules.
Add it to your project with `] add Bplus` or `using Pkg; Pkg.add("Bplus")`.
A number of example projects are provided in [the *BpExamples* repo](https://github.com/heyx3/BpExamples):
[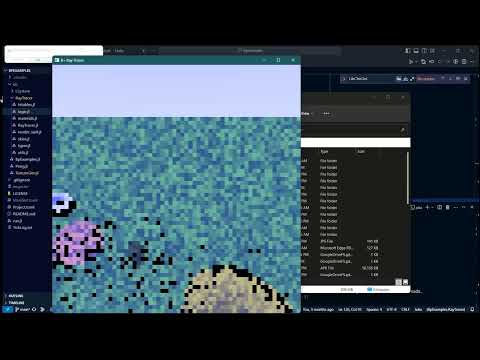](https://www.youtube.com/embed/Po3zGQRSRgE)
[Here is an open-source game project that heavily uses the ECS system](https://github.com/heyx3/Drill8):
[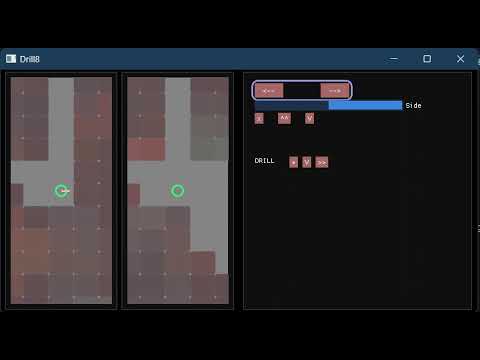](https://www.youtube.com/embed/H5IgtsJcJjw)
An ongoing [voxel toy project](https://github.com/heyx3/BpWorld) of mine:
[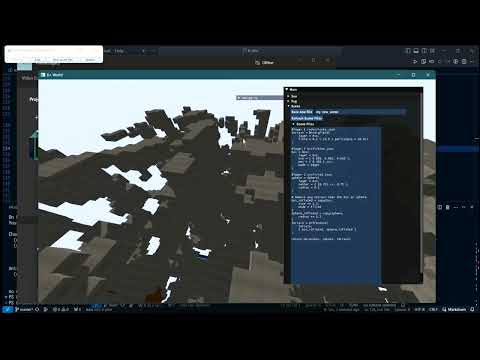](https://www.youtube.com/embed/fGxGdNAPTSY)
If you're wondering why Julia, [read about it here](docs/!why.md)!
## Structure
To import everything B+ has to offer, just do `using Bplus; @using_bplus`.
All documented modules are available directly inside (e.x. `Bplus.Math`),
but under the hood this library is segmented into several smaller packages:
* [BplusCore](https://github.com/heyx3/BplusCore) contains utilities and game math.
* [BplusApp](https://github.com/heyx3/BplusApp) provides convenient access to OpenGL, GLFW, and Dear ImGUI.
* [BplusTools](https://github.com/heyx3/BplusTools) provides a variety of other game-development tools, like a scene-graph algorithm, ECS algorithm, and more.
You can, for example, integrate `BplusCore` into your own project to get all the game math,
without having to use OpenGL, Dear ImGUI, or any of the other parts of the library.
## Tests
Unit tests follow Julia convention: kept in the *test* folder, and centralized in *test/runtests.jl*.
Each sub-package (`BplusCore`, `BplusApp`, and `BplusTools`) runs its own tests,
and this package runs each of those in sequence.
The test behavior is standardized by `BplusCore`, which provides the path
to a test-running Julia file through `BplusCore.TEST_RUNNER_PATH`.
I never liked how the official Julia Test package does not let you assign messages to tests,
so my tests are implemented with my own macros:
* `@bp_check(condition, msg...)` for normal checks
* `@bp_check_throws(statement, msg...)` to ensure something causes an error
* `@bp_test_no_allocations(expr, expected_value, msg...)` to test expressions that need to be completely static and type-stable (game math, scene graph operations, raycast math, etc).
* A slightly longer version is offered in case you need to set up the test with some operations that may allocate: `@bp_test_no_allocations_setup(setup_expr, test_expr, expected_value, msg...)`.
## Codebase
The three sub-packages are organized into a clear order.
Each one has a set of modules within it.
````
--------- BplusCore ----------
| |
| Utilities Math |
| |
--------------------------------
|
|
|
|
-------------- BplusApp ---------------
| |
| GL |
| / \ |
| / \ |
| / \ |
| GUI Input |
| |
-----------------------------------------
|
|
|
|
-------------- BplusTools ---------------
| |
| ECS Fields SceneTree |
| |
-------------------------------------------
````
To import all B+ sub-modules into your project, you can use this snippet: `using Bplus; @using_bplus`.
Below is a quick overview of the modules. [A thorough description is available here](docs/!home.md). You can also refer to the inline doc-strings, comments, and [sample projects](https://github.com/heyx3/BpExamples).
### `Bplus.Utilities`
A dumping ground for all sorts of convenience functions and macros.
Detailed info can be found [in this document](docs/Utilities.md), but here are the highlights:
* `PRNG`: An alternative to Julia's various `AbstractRNG`'s which is more suitable for aesthetic, short-term use-cases such as procedural generation.
* `@bp_check(condition, error_msg...)`, a run-time test that raises `error()` if the expression is false.
* `@make_toggleable_asserts()` sets up a "debug" vs "release" compile-time flag for your module.
* `@bp_enum` and `@bp_bitflag`: Much better alternatives to Julia's `@enum` and BitFlags' `@bitflag`.
* `SerializedUnion{Union{...}}`: Can serialize *and deserialize* a union of values, using the *StructTypes* package (which means it can be used in major IO packages like *JSON3*).
* `RandIterator{TIdx, TRng}`: Randomly and efficiently iterates through every value in some range `1:n`.
* `UpTo{N, T}`: A type-stable, immutable, stack-allocated container for up to N values of type T. Implements `AbstractVector{T}`.
### `Bplus.Math`
Typical game math stuff, but using Julia's full expressive power.
Detailed info can be found [in this document](docs/Math.md), but here is a high-level overview:
* Vectors are represented with `Vec{N, T}`.
* Quaternions are `Quaternion{F}`.
* Matrices are `@Mat{C, R, F}`.
* Various functions such as `lerp()` and `inv_lerp()`.
* `Box{N, T}` and `Interval{T}`, to represent contiguous spaces.
* `Ray{N, F}`, and a hierarchy of `AbstractShape`
* Noise functions:
* `perlin(pos)`
* `Contiguous{T}` is an alias for a wide variety of storage for some sequence of `T`. For example, `NTuple{10, Vec{3, Float32}}` is both a `Contiguous{Float32}` and a `Contiguous{Vec{3, Float32}}`.
### `Bplus.GL`
Wraps OpenGL constructs, and provides a centralized place for a graphics context and its accompanying window. This is the big central module of B+ along with `Math`.
Detailed information can be found [in this document](docs/GL.md), but here is a high-level overview:
* `Context` represents the global OpenGL context, uniquely associated with a specific thread and GLFW window.
* Services are singletons attached to the `Context`. Some are built into B+, but you can also define your own using the `@bp_service` macro.
* `AbstractResource` is the type hierarchy for OpenGL objects, like `Texture`, `Buffer`, or `Mesh`.
* Clear the screen with `clear_screen()`. Draw something with `render_mesh()`.
### `Bplus.Input`
Detailed explanation available [here](docs/Input.md).
Provides a greatly-simplified window into GLFW input, as a [GL Context Service](docs/GL.md#Services). Input bindings are defined in a data-driven way that is easily serialized through the `StructTypes` package.
Make sure to update this service every frame with `service_Input_update()`; this is already done for you if you build your game logic within [`@game_loop`](docs/Helpers.md#Game-Loop).
### `Bplus.GUI`
Helps you integrate B+ projects with the Dear ImGUI library, exposed in Julia through the *CImGui.jl* package.
Detailed information can be found in [this document](docs/GUI.md), but here is how you use it at a basic level:
1. Initialize the service with `service_GUI_init()`.
2. Start the frame with `service_GUI_start_frame()`.
3. After starting the frame, use *CImGui* as normal.
4. End the frame with `service_GUI_end_frame()`
If you use [`@game_loop`](docs/Helpers.md#Game-Loop), then this is all handled for you and you can use *CImGui* freely.
To send a texture (or texture View) to the GUI system, you must wrap it with `gui_tex_handle(tex_or_view)`
### `Bplus.ECS`
A very simple entity-component system that's easy to iterate on, loosely based on Unity3D's model. If you want a high-performance, use an external Julia ECS package instead such as *Overseer.jl*.
Detailed information can be found in [this document](docs/ECS.md), but here is a high-level overview:
* A `World` is a list of `Entity` (plus accelerated lookup structures)
* An `Entity` is a list of `AbstractComponent`
* You can add, remove, and query entities for components.
* You can also query the components across an entire `World`.
* You can define component types with the `@component` macro. Components have a OOP structure to them; abstract parent components can add fields, "promises" (a.k.a. abstract functions) and "configurables" (a.k.a. virtual functions).
* Refer to the `@component` doc-string for a very detailed explanation of the features and syntax.
* You can update an entire world with `tick_world(world, delta_seconds::Float32)`.
### Helpers
Things that don't neatly fit into the other modules are documented [here](docs/Helpers.md).
### `Bplus.SceneTree`
Provides a memory-layout-agnostic implementation of scene graph algorithms. **Still a work-in-progress**, but the architecture is pretty much done.
Read about this feature [here](docs/SceneTree.md).
### `Bplus.Fields`
A data representation of scalar or vector fields, i.e. functions of some `Vec{N1, F1}` to some `Vec{N2, F2}`. As well as a nifty little language for quickly specifying them.
This is the first of a series of planned modules that will help you play with procedural content generation. For example, you can use a Field to easily fill a texture with interesting noise.
I'm not going to write more about this module for now, but check out the unit tests for examples.
# License
Copyright William Manning 2019-2023. Released under the MIT license.
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
| Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 1522 | Detailed info on the B+ codebase. For high-level info, see [the root README.md file](../README.md).
* [BplusCore](https://github.com/heyx3/BplusCore)
* [Utilities](Utilities.md):
* [Asserts](Utilities.md#Asserts)
* [Optional](Utilities.md#Optional)
* [Enums](Utilities.md#Enums)
* [PRNG](Utilities.md#PRNG)
* [Collections and Iterators](Utilities.md#Collections-And-Iterators)
* [Macros](Utilities.md#Macros)
* [Functions](Utilities.md#Functions)
* [Interop](Utilities.md#Interop)
* [Metaprogramming](Utilities.md#Metaprogramming)
* [Math](Math.md):
* [Functions](Math.md#Functions)
* [`Vec{N, T}`](Vec.md)
* [`Quaternion{F}`](Quat.md)
* [`Mat{C, R, F}`](Matrix.md)
* [Rays](Math.md#Rays)
* [Box and Interval](Math.md#Box-and-Interval)
* [Shapes](Math.md#Shapes)
* [Random Generation](Math.md#Random-Generation)
* [BplusApp](https://github.com/heyx3/BplusApp)
* [GL module](GL.md):
* [Context](GL.md#Context)
* [Resources](Resources.md)
* [Services](GL.md#Services)
* [Clearing](GL.md#Clearing)
* [Drawing](GL.md#Drawing)
* [Debugging](GL.md#Debugging)
* [Input module](Input.md):
* [GUI module](GUI.md):
* [`BasicGraphics` service](Helpers.md#Basic-Graphics)
* [`@game_loop`](Helpers.md#Game-Loop)
* [BplusTools](https://github.com/heyx3/BplusTools)
* [Cam3D](Helpers.md#Cam3d)
* [File Cacher](Helpers.md#File-Cacher)
* [ECS module](ECS.md)
* [SceneTree module](SceneTree.md)
* [Fields module](Fields.md) | Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 9656 | Julia is a niche scientific computing language. What about it is interesting to game dev?
TL;DR: scientists and game developers are dealing with the same problem.
## The two-language problem
Scientists are not expert software engineers, yet they need high performance, so they end up splitting their time and attention between one language for performant libraries (usually C/C++) and another for high-level simplicity and usability (often Python). This isn't ideal.
If you're familiar at all with gamedev, you already know that we have the same issue! Balancing the needs of physics and rendering engineers with the needs of game designers and artists is difficult. Unity tries to go middle-of-the-road with C# (plus a C++ backend which you can't modify), while Unreal has the dichotomy of Blueprints and C++.
Julia manages to solve this problem very well IMHO, and it is just as useful for game developers as it is for PhD's.
## Syntax
Code is extremely terse, simple to write, and simple to read, yet the resulting programs can be heavily-optimized (the benchmarks I read have found that it's approaching the speed of C, and it's still a young language). Thanks to an extremely clever JIT engine, combined with aggressive Whole-Code-Optimization using inlining, constant-folding, and type inference, you can write complex abstractions which easily become zero-cost.
Compare this to C++, which achieves zero-cost abstractions at the price of legendary verbosity and inscrutable error messages. Julia has been a wonderful breath of fresh air for me.
Other high-performance scientific computing languages exist, like Matlab, but their syntax is much weirder. Julia's is quirky, yet readable.
It's also incredibly flexible. You can write extremely mathy code, like defining the cross product with `v₁ × v₂ = [blah blah])`, or you can write that same code with plain imperative syntax:
````
function ×(forward, up)
x = [blah]
y = [blah blah]
z = [blah blah blah]
return Vec(x, y, z)
end
````
## High-performance "Dynamic" Typing
Julia acts like a dynamically-typed language, which is great for high-level users. And unlike most high-performance languages, it *is* truly possible to have variables whose types are completely unknown at compile-time.
Additionally, the language offers (and **heavily** leans on) "multiple-dispatch", which is like runtime function overloading -- if you have a variable that is either `Int` or `Float32`, and you call a function that has different overloads for `Int` vs `Float32` parameters, the correct overload is figured out at runtime. Which, again, is super nice for high-level users, but if your data is dynamically-typed then any function calls with that data have a heavy performance cost.
So, why isn't this a fatal problem for high-peformance code?
In short, the dynamic typing does not propagate into other function calls, making dynamically-typed data the exception rather than the rule!
Nearly every Julia function gets re-compiled for each combination of specific parameter types. When calling a function with arguments of unknown type, you do pay the cost of dynamic dispatch, but the function you dispatch to knows the types of these parameters at compile-time. In other words, parameters are *always* strongly-typed unless you explicitly mark them otherwise.
Therefore, the only time you run into dynamically-typed data is when you create it within your own function, or if you call a function that returns it. Both of these cases can be caught with code introspection tools built into Julia, and easily mitigated. In fact, from what I've heard there is some new VSCode integration that highlights dynamically-typed variables for you, making it trivial.
## Metaprogramming Annihilates Boilerplate
On the rare occasion you have to write something that might be called "boilerplate", you can automate the task with metaprogramming features. Julia has syntactical macros (think Scheme, not C), with a built-in concept of code represented as data. Yet another breath of fresh air for C++ developers, whose choice for metaprogramming is between text-pasting macros and inscrutable, verbose templates.
For example, in order to upload data structures to the GPU, you must follow one of two onerous padding and alignment rules: `std140` or `std430`. In B+, this is completely automated by simply wrapping your struct in `@std140` or `@std430`! The bit pattern of your struct will then precisely match the bit pattern expected by the GPU. You can even query how many padding bytes were needed, helping you attempt to minimize its size.
But it's not just about macros. For example, let's say I'm implementing a vector type for game math (because I *am* doing that):
````
struct Vec{N, T}
components::NTuple{N, T}
end
# You can add and subtract vectors.
+(a::Vec, b::Vec) = Vec(map(+, a.components, b.components))
-(a::Vec, b::Vec) = Vec(map(-, a.components, b.components))
````
Already much less verbose than C++, for example I don't have to write the type parameters of `Vec` unless I really care about them (and as mentioned above, there's no performance penalty for omitting them). But it could still get annoying to rewrite this for every single operation and function. Plus, if I decide to change how they work (e.x. mark them all `@inline`), I have to fix each one by hand.
So instead, let's generate the code with a `for` loop.
````
# Name every built-in, two-parameter operator I want to overload for Vec.
# Symbols are like strings, but for metaprogramming.
const SUPPORTED_FUNCTIONS::Array{Symbol} = [
:+, :-, :*, :/,
:>, :<, :>=, :<=, :!=, :(==),
:min, :max, :pow
]
# Compile each of them.
for f in SUPPORTED_FUNCTIONS
@eval $f(a::Vec, b::Vec) = Vec(map($f, a.components, b.components))
end
````
This is also a good example of the fuzzy line between compile-time and run-time in Julia. You can think of it like `constexpr` in C++, but again, *far* less verbose, and also completely unlimited. You could define a compile-time constant by reading it in from a text file, or standard input! (Theoretically. In practice, the compiler suppresses `stdin` for some reason). You can similarly embed a file in your program merely by reading the file, and storing the contents in a `const` String or list of bytes. Anything you can do at run-time, you can also do at compile-time.
## Compile-time flags at Runtime!?
Because Julia is a JIT language, there's never a point where compile-time permanently stops. Any time you are running at the "global" level of code, outside of any function stack, you can change things. (You actually can change things from within an executing function too, but there be dragons).
For example, let's say you want to add a "debug or release?" flag for asserts. You might naively implement it as a const global:
````
const IS_DEBUG = false # Change to 'true' locally when debugging
macro assert(condition, messsage)
# I'm skipping over a few details, but this is basically how you make a macro
return quote
if IS_DEBUG && !($condition)
error($message)
end
end
end
````
Now you can do `@assert(some_slow_check(), "The check failed!")` and expect it to be compiled out when `IS_DEBUG` is false. But, there's actually a much more interesting way to do it. Define it as a *function* instead:
````
is_debug() = false
macro assert(condition, messsage)
return quote
if is_debug() && !($condition)
error($message)
end
end
end
````
A key feature of Julia is the ability to add new overloads to functions, potentially replacing old ones. So now, you can switch to "debug" mode without having to edit the core codebase. Instead, you can write the following in your test scripts, outside the main project:
````
using MyGame # Bring in the main codebase, including 'is_debug()'
MyGame.is_debug() = true # Recompile everything!
MyGame.run() # Run in debug mode!
````
The JIT will invalidate and eventually recompile any functions that invoked `is_debug()`, causing the asserts to suddenly appear!
My framework uses this technique to define a global up vector and coordinate handedness. If you prefer Dear ImGUI's coordinate system over OpenGL's, you can trivially recompile the engine for left-handed, Y-down coordinates, by adding two lines at the top of your own codebase.
# The downsides
Julia language devs will always prioritize scientific computing, so there are some things that it won't do much (or at all) that gamedev cares about.
The biggest issue is mobile. Nobody is running climate simulations on their Android phone, so it's very unlikely that Julia ever gets support for running on mobile. It's also based entirely around dynamic code compilation, which could be an outright deal-breaker on AOT platforms like iOS. I'm not bothered by this as I'm coding with and for my RTX2070-powered desktop, but plenty of you will reasonably consider this a deal-breaker.
Another issue is that compilation into a static executable was an after-thought. They've been working hard on *PackageCompiler.jl*, a package that deploys Julia code as a standalone executable binary, and it does work! But it's a weird process, and the binary is massive because you still need some dynamic compilation during play and therefore you need the entire Julia runtime, which includes things like LLVM and Clang.
Finally, the language uses 1-based indexing. This is not nearly as annoying as I feared when I started using the language, *but* once you interop with OpenGL which obviously uses 0-based indexing for buffer data, off-by-1 errors become more likely.
| Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 3834 | # ECS
A very simple and friendly entity-component system, loosely based on Unity3D's model of `GameObject`s and `MonoBehaviour`s.
If you prefer high-performance over simplicity, use an external Julia ECS package such as *Overseer.jl*.
## Overview
* A `World` is a list of `Entity` (plus accelerated lookup structures).
* An `Entity` is a list of `AbstractComponent`.
* A component has its own data and behavior, mostly in the form of a "TICK()" event that is invoked by the world.
You can update an entire world with `tick_world(world, delta_seconds::Float32)`. You can destroy all entities (and reset other fields) with `reset_world(world)`, which is useful if your components are managing resources and you want to make sure all of them are destructed properly.
A world stores timing data for easy access: `delta_seconds`, `elapsed_seconds`, and `time_scale`. You may set `time_scale` to change the speed of your world.
To enable extra error-checking around your use of the ECS, enable the module's [debug asserts flag](Utilities.md#asserts) by executing `BplusTools.ECS.bp_ecs_asserts_enabled() = true` while loading your game's code.
## Entity/component management
You can add and remove both entities and components:
* `add_entity(world)::Entity` and `remove_entity(world, entity)`
* `add_component(entity, T, constructor_args...; kw_constructor_args...)::T`
* All the extra arguments get passed into the component's constructor (refer to `CONSTRUCT()` in the `@component` macro)
* `remove_component(entity, c::AbstractComponent)`
You can query the components on an `Entity` or entire `World`:
* `has_component(entity_or_world, T)::Bool`
* `get_component(entity, T)::Optional{T}`
* Throws an error if there is more than one on the entity.
* `get_component(world, T)::Optional{Tuple{T, Entity}}`
* Throws an error if there is more than one in the world.
* Also returns the entity owning the component.
* `get_components(entity, T)::[iterator over T]`
* Returns all components of the given type within the given entity.
* `get_components(world, T)::[iterator over Tuple{T, Entity}]`
* Returns all components of the given type within the entire world, paired with their owning entities.
* `count_components(entity_or_world, T)::Int`
* `get_component_types(T)::Tuple{Vararg{Type}}`
* Returns a list of the type T, its parent type, grandparent type, etc. up to but not including the root type `AbstractComponent`.
## `@component`
You can define component types with the `@component` macro. Components have a OOP structure to them; abstract parent components can add fields, "promises" (a.k.a. abstract functions) and "configurables" (a.k.a. virtual functions) to their children. Components have a default constructor where each field is provided in order (parent fields before child ones), but you can override this behavior.
Refer to the `@component` doc-string for a very detailed explanation, with code samples, of the full features and syntax.
### Internal interface
If you wish, you can entirely ignore `@component` and manually implement your own component type through standard Julia syntax.
Simply define a mutable struct inheriting from`AbstractComponent` (or a child of it), and implement the interface described in *ECS/interface.jl*.
Note that your custom type could never be a parent of another `@component` (unless you implement all the interface functions at the top of *ECS/macros.jl*, but at that point just use the macro).
## Component printing
By default, when printing a component defined with `@component`, fields that reference an `Entity` `World` or `AbstractComponent` are shortened for brevity.
You can customize how a specific field of your `@component` displays itself, in `print()`, by overloading `component_field_print(component, Val{:my_field}, field_value, io::IO)`.
| Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 15375 | # GL
A thin wrapper over OpenGL 4.6 with the bindless textures extension, plus some helpers to manage a context/GLFW window.
**Important note**, all counting and indices in GL functions are 1-based, to stay consistent with the rest of Julia. They are converted to 0-based under the hood. For example, if doing instanced rendering with `N` instances, you want to pass the instancing range `IntervalU(min=1, max=N)`, not `IntervalU(min=0, max=N-1)`. Of course, GPU-side data such as a mesh's index buffer is still 0-based, so be careful when generating mesh data or indirect drawing data.
## Context
An OpenGL rendering context is a thread-local singleton, and is attached to a specific window. This is encapsulated in the mutable struct `Context`. Within your application code, you can retrieve the context for the current thread with `get_context()`.
Contexts manage several important things:
* A reference to the `GLFW.Window` and its current vsync setting.
* The current render state, in the field `state::RenderState`.
* For convenience, all the fields in `RenderState` are exposed as properties of `Context`. For example, instead of doing `get_context().state.viewport`, you can just do `get_context().viewport`.
* Which of your requested OpenGL extensions was actually available (by default, a few bindless-texture extensions are requested)
* Call `extension_supported("GL_ARB_my_extension_name")` to check
* Any services that are attached to this context (see [Services](#Services)).
* The specific GPU device limits as reported through OpenGL, in the field `device::Device`.
* Some of these statistics are not always reported reliably by every driver, so beware when using them.
* The currently-bound resources -- shader, mesh, UBO slots, SSBO slots.
* GLFW callbacks. The core GLFW library only allows one subscriber per event; Context allows you to add as many as you want.
* The [Input](Input.md) and [GUI](GUI.md) services already handle GLFW input, so you likely won't need these callbacks yourself.
* For more info on the callbacks and their signatures, see the relevant fields in `Context`.
### Initialization
Managing the life of a Context by yourself is not easy, due to Julia's threading model. For simple use-cases, you should just use the [`@game_loop` macro](Helpers.md#Game-Loop) to run all your code within the life of a `Context`.
If `@game_loop` doesn't provide enough flexibility, you can use the lower-level function `bp_gl_context()` to construct the context, run your custom application code, and then clean up the context automatically at the end. This function takes your code as the first parameter, which is an extremely common Julia idiom -- see the official docs about `do` blocks. The rest of the parameters go straight into the `Context` constructor, so refer to that for information on them.
### Render State
VSync (including adaptive sync) is normally managed by GLFW. In B+ it's represented by the `VsyncModes` enum. You can set it on Context creation. You can also change it afterwards with `set_vsync(mode::E_VsyncModes)`. You can get the current value with `get_context().vsync`.
You can easily get the GLFW window's size with `get_window_size()`.
The render state managed by the `Context` is specified by the `RenderState` struct. For any given piece of state defined in this struct, you can interact with it through the `Context` in several ways:
* Get its current value using properties: `get_context().cull_mode`
* Set its current value using properties: `get_context().cull_mode = FaceCullModes.off`
* Sets its current value using a more traditional function: `set_culling(FaceCullModes.off)`.
* Temporarily set its value, run some code, then set it back: `with_culling(FaceCullModes.off) do ... end`.
The following render state data is available:
* `color_write_mask::vRGBA{Bool}` toggles the ability of shaders to write to each individual color channel.
* `set_color_writes(...)` is the function.
* `with_color_writes(...)` is the wrapper.
* `depth_write_mask::Bool` toggles the ability of shaders to write to the depth buffer at all.
* `set_depth_writes(...)` is the function.
* `with_depth_writes(...)` is the wrapper.
* `cull_mode::E_FaceCullModes` changes back-face vs front-face culling.
* `set_cull(...)` is the function.
* `with_culling(...)` is the wrapper.
* `viewport::Box2Di` controls the range of pixels that can be rendered to.
* More precisely, it maps the NDC output from a vertex shader (positions in the range -1 to +1 along each axis, written to `gl_Position`), to the given range of pixels.
* `set_viewport(...)` is the function.
* `with_viewport(...)` is the wrapper.
* `scissor::Optional{Box2Di}` limits the range of pixels that can be affected by draw calls. Pixels outside this box are not affected.
* Unlike `viewport`, this setting does not change the coordinate math in mapping triangles to screen space. It just hides pixels.
* `set_scissor(...)` is the function.
* `with_scissor(...)` is the wrapper.
* `blend_mode::@NamedTuple{rgb::BlendStateRGB, alpha::BlendStateAlpha}` controls how newly-drawn pixels are added to the color already in the render target or framebuffer.
* See the `BlendState_` struct for info on how to make custom blend modes. There are some presets, for example:
* Opaque blending of color with `make_blend_opaque(BlendStateRGB)`
* Additive blending of alpha with `make_blend_additive(BlendStateAlpha)`
* Alpha blending of all 4 channels with `make_blend_alpha(BlendStateRGBA)`
* `set_blending(...)` is the function.
* `with_blending(...)` is the wrapper.
* `depth_test::E_ValueTests` controls the test used against the depth buffer to draw or occlude pixels.
* `set_depth_test(...)` is the function.
* `with_depth_test(...)` is the wrapper.
* `stencil_test::Union{StencilTest, @NamedTuple{front::StencilTest, back::StencilTest}}` controls the test used against the stencil buffer to draw or discard pixels. You may optionally use a different test for front-facing geometry vs back-facing geometry.
* `set_stencil_test(...)`, `set_stencil_test_front(...)`, and `set_stencil_test_back(...)` are the functions.
* `with_stencil_test(...)`, `with_stencil_test_front(...)`, and `with_stencil_test_back(...)` are the wrappers.
* `stencil_result::Union{StencilResult, @NamedTuple{front::StencilResult, back::StencilResult}}` controls the effect of a pixel on the stencil buffer. You may optionally use a different test for front-facing vs back-facing geometry.
* `set_stencil_result(...)`, `set_stencil_result_front(...)`, and `set_stencil_result_back(...)` are the functions.
* `with_stencil_result(...)`, `with_stencil_result_front(...)`, and `with_stencil_result_back(...)` are the wrappers.
* `stencil_write_mask::Union{GLuint, @NamedTuple{front::GLuint, back::GLuint}}` controls which bits of the stencil buffer can be affected by newly-drawn pixels. You may optionally use a different mask for front-facing vs back-facing geometry.
* `set_stencil_write_mask(...)`, `set_stencil_write_mask_front(...)`, and `set_stencil_write_mask_back(...)` are the functions.
* `with_stencil_write_mask(...)`, `with_stencil_write_mask_front(...)`, and `with_stencil_write_mask_back(...)` are the functions.
* `render_state::RenderState` controls the entire state at once. This is not more or less efficient than individually setting the states you care about; just different.
* `set_render_state(...)` is the function.
* `with_render_state(...)` is the wrapper.
### Refreshing the context
If external code makes OpenGL calls and modifies the driver state, you can call `refresh(context)` to force the Context to acknowledge these changes. This could be a very slow operation, so avoid using if at all possible!
## Resources
For low-level OpenGL work (which you usually don't need to do), OpenGL handle typedefs are provided in *GL/handles.jl*. These handles are all prefixed with `Ptr_`. For example, `Ptr_Program`, `Ptr_Texture`, `Ptr_Mesh`. These types aren't exported by default.
Managed OpenGL objects are instances of `AbstractResource`. All resource types implement the following interface (see doc-strings for more details):
* `get_ogl_handle(resource)`
* `is_destroyed(resource)::Bool`
* `Base.close(resource)` to clean it up
Resources cannot be copied and their fields cannot be directly set; instead you should use whatever interface that resource provides.
For more info on each type of resource, see [this document](Resources.md). The types of resources are as follows:
* [Program](Resources.md#Program) : A compiled shader
* [Texture](Resources.md#Texture) : A GPU texture (1D, 2D, 3D, cubemap)
* [Buffer](Resources.md#Buffer) : A GPU-side array of data
* [Mesh](Resources.md#Mesh) : What OpenGL calls a "Vertex Array Object".
* [Target](Resources.md#Target) : A render target, or what OpenGL calls a "FrameBuffer Object".
* [TargetBuffer](Resources.md#TargetBuffer) : Used internally when you don't need part of a Target to be sampleable (e.x. you need a depth buffer for depth-testing but not for custom effects).
## Sync
* To make sure that a specific future action can see the results of previous actions (for example, sampling from a texture after writing to it with a compute shader), call `gl_catch_up_before()`.
* This is only for "incoherent" actions, which OpenGL can't predict, as opposed to coherent actions like rendering into a target.
* The variant `gl_catch_up_renders_before()` is potentially more efficient when you are specifically considering actions taken in fragment shaders, concerning only a subset of the Target that you just rendered to.
* To force all submitted OpenGL commands to finish right now, call `gl_execute_everything()`. This isn't very useful, except in a few scenarios:
* You are sharing resources with another context (not natively supported in B+ anyway),
to ensure the shared resources are done being written to by the source context.
* You are tracking down a driver bug, and want to call this after every draw call
to track down the one that crashes.
* If you are ping-ponging within a single texture, by reading from and rendering to separate regions, you should call `gl_flush_texture_writes_in_place()` before reading from the region you just wrote to.
*
## Services
Sometimes you want your own little singleton associated with a window or rendering context, providing easy access to utilities and resources. These singletons are called "Services". You can define a new service using the `@bp_service()` macro. Its doc-string describes how to make and use it.
The following servces are built into B+:
* `Input`, from the [*BplusApp.Input module](Input.md), provides a high-level view into GLFW keyboard, mouse, and gamepad inputs. Automatically managed for you if you use the [`@game_loop`](Helpers.md#Game-Loop) macro.
* `GUI`, from the [*BplusApp.GUI module](GUI.md), takes care of Dear ImGUI integration. Automatically managed for you if you use the [`@game_loop`](Helpers.md#Game-Loop) macro.
* [`BasicGraphics`, from the *BplusApp module](Helpers.md#Basic-Graphics), provides simple shaders and meshes. Automatically managed for you if you use the [`@game_loop`](Helpers.md#Game-Loop) macro.
* `SamplerProvider` is an internal service that allocates sampler objects as needed. Separate textures with the same sampler settings will reference the same sampler.
* `ViewDebugging` helps catch *some* uses of bindless texture handles without properly activating them. It's only enabled if the *BplusApp.GL* module is in [debug mode](Utilities.md#Asserts), or the GL Context is started in debug mode.
## Clearing
The screen can be cleared with `clear_screen(values...)`:
* To clear color, pass a 4D vector of component type `GLfloat` (you can use the alias `vRGBAf`).
* If the back buffer is somehow in a non-normalized integer format, provide a component type of either `GLint` (`vRGBAi`) or `GLuint` (`vRGBAu`) as appropriate.
* To clear depth, pass a single `GLfloat` (a.k.a. `Float32`).
* To clear stencil, pass a single `GLuint` (a.k.a. `UInt32`).
* To clear a hybrid depth-stencil buffer, pass two arguments: the depth as `GLfloat` and the stencil as `GLuint`.
## Drawing
Draw a `Mesh` resource `m`, using a `Program` resource `p`, by calling:
`render_mesh(m, p; args...)`
There are numerous named parameters to customize the draw call. **Important note**, as with the rest of the *BplusApp.GL* module, all counting and indices in these paramters are 1-based, to stay consistent with the rest of Julia. They are converted to 0-based under the hood. For example, if doing instanced rendering with `N` instances, you want to pass the range `IntervalU(min=1, size=N)`, not `IntervalU(min=0, size=N)`.
* `shape::E_PrimitiveTypes` changes the type of primitive (line strip, triangle list, etc). By default, this information is taken from the `Mesh` being drawn.
* `indexed_params::Optional{DrawIndexed}` controls indexed drawing. By default, if the `Mesh` has indices, it will draw in indexed mode using all availble indices.
* `elements::Union{IntervalU, Vector{IntervalU}, ConstVector{IntervalU}}` controls which parts of the mesh are drawn. By default, the mesh's entire available data is drawn. You can pass a different contiguous range of elements to darw with. You can also do "multidraw", by providing a list of contiguous ranges.
* `instances::Optional{IntervalU}` allows you to optionally do instanced rendering, where the the mesh is re-drawn `N` times, providing a different "instance index" to the shader each time. Note that you can pass any contiguous interval of instances; it doesn't have to start at 1.
* `known_vertex_range::Optional{IntervalU}` can provide the driver with an optimization hint about the range of vertices being referenced by the indices you are drawing. This is mainly for if you are using indexed rendering *and* passing a custom range for `elements`.
**Important note**: Three of the parameters above are mutually-exclusive: multi-draw with `elements`, instancing with `instances`, and the optimization hint `known_vertex_range`. If you attempt to use more than one at once, you will get an error.
*NOTE*: There is one small, esoteric OpenGL feature that is not included, because adding it would probably require separating this function into 3 different overloads: indexed multi-draw is allowed to use different index offsets for each subset of elements.
*NOTE*: Indirect draw calls are not yet implemented. If you dispatch them yourself, remember to leave the OpenGL state unchanged, or else refresh the Context with `refresh(get_context())`.
## Compute Dispatch
Dispatch a compute shader with `dispatch_compute_threads()` or `dispatch_compute_groups()`.
## Debugging
For now, B+ uses the older form of OpenGL error catching. Call `pull_gl_logs()` to get a list, in chronological order, of all messages/errors that have occurred since the last time you called `pull_gl_logs()`. This list may drop messages if it's not checked for too long, so it's recommended to call it at least once a frame in [debug builds](Utilities.md#Asserts) of your game.
For convenience, you can use the macro `@check_gl_logs(msg...)` to pull log events and immediately print them along with the file and line number, and any information you provide. | Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 4773 | Provides a wrapper around the wonderful Dear ImGUI library (exposed in Julia as *CImGui.jl), plus many related helpers.
Note that you can freely convert between B+ vectors (`Vec{N, T}`) and CImGui vectors (`ImVec2`, `ImVec4`, `ImColor`).
## GUI service
The Dear ImGUI integration is provided as a [B+ Context service](GL#services), meaning it is a graphics-thread-singleton that you control with the following functions:
* `service_GUI_init()` to start the service
* `service_GUI_exists()` to check if the service is already running
* `service_GUI_shutdown()` to manually kill the service (you don't have to do this if the program is closing)
* `service_GUI_start_frame()` to set up before any GUI calls are made for the current frame
* `service_GUI_end_frame()` to finish up and render the GUI to the currently-bound target, presumably the screen.
* `service_GUI_rebuild_fonts()` to update the GUI library after you've added custom fonts.
* `service_GUI()` to get the current service instance
If you want to pass a texture (or texture view) to be drawn in the GUI, you must wrap it in a call to `gui_tex_handle()`. Do not just pass the OpenGL handle for the resource.
Note that the underlying library uses static variables, and does not manage multiple independent contexts, so you cannot run this service from more than one GL Context at a time.
## Text Editor
Text editing through a C library is tricky, due to the use of C-style strings, the need to integrate clipboard, and also the need to dynamically resize the string as the user writes larger and larger text.`BplusApp.GUI.GuiText` handles all of this for you.
* Create an instance with the initial string value, and optionally configuring some of its fields.
* For example, `GuiText("ab\ncdef\ngh", is_multiline=true)`
* Display the text widget with `gui_text!(my_text)`.
* Get the current value with `string(my_text)`.
* Change the text value with `update!(my_text, new_string)`.
## Scoped Helper Functions
Most of Dear ImGUI's state is static variables, which you configure as you draw things.
Often, it is useful to *temporarily* set some state, run GUI code, then undo your changes.
B+ offers many helper functions which manage this, by taking your GUI code as a lambda.
You should use Julia's `do` block syntax to pass the lambda, for example:
````
gui_with_indentation() do
# Your indented GUI code here
end
````
The following functions are availble (lambda parameter is omitted for brevity):
* `gui_window(args...; kw_args...)::Optional` nests your code inside a new window.
* All arguments are passed through to the `CImGui.Begin()` call.
* Returns the output of your code block, or `nothing` if the UI was culled
* `gui_with_item_width(width::Real)` changes the width of widgets.
* `gui_with_indentation(indent::Optional{Real} = nothing)` indents some GUI code.
* `gui_with_padding(padding...)` sets the padding used within a window.
* You can pass x and y values, or a tuple of X/Y values.
* `gui_with_clip_rect(rect::Box2Df, intersect_with_current_rect::Bool, draw_list = nothing)` sets the clip rectangle.
* `gui_with_font(font_or_idx::Union{Ptr, Int})` switches to one of the fonts you've already loaded into Dear ImGUI.
* `gui_with_unescaped_tabbing()` disables the ability to switch between widgets with Tab.
* Useful if you want Tab to be recognized in a text editor.
* `gui_with_nested_id(values::Union{AbstractString, Ptr, Integer}...)` pushes new data onto Dear ImGUI's ID stack. Dear ImGUI widgets must be uniquely identified by their label plus the state of the ID stack at the time they are called, so this helps you distinguish between different widgets that have the same label.
* `gui_within_fold(label)` nests some GUI within a collapsible region.
* Dear ImGUI calls this a "tree node".
* `gui_with_style_color(index::CImGui.ImGuiCol_, color::Union{Integer, CImGui.ImVec4})` configures a specific color within Dear ImGUI's style settings.
* `gui_within_group()` allows widgets to be referred to as an entire group. For example, you can make a vertical layout section within a horizontal layout section by calling `CImGui.SameLine()` after the entire vertical group.
* `gui_tab_views()` allows you to define multiple tabs, each with associated widgets inside it.
* `gui_tab_item()` defines one tab view within.
* `gui_within_child_window(size, flags=0)::Optional` nests a GUI within a sub-window. Returns the output of your code block, or `nothing` if the window is culled.
## Other Helper Functions
* `gui_spherical_vector(label, direction::v3f; settings...)::v3f` edits a vector using spherical coordinates (pitch and yaw).
* `gui_next_window_space(uv_space::Box2Df)` sets the size of the next GUI window in percentages of this program's window size. | Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 6267 | A grab-bag of B+ utilities you'll likely find useful.
# Game Loop
Handles all the boilerplate of a basic game loop for you. Refer to the doc-string `@game_loop`, and the file *Helpers/game_loop.jl*, for detailed info on usage.
The loop handles all built-in B+ services for you: [`Input`](Input.md), [`GUI`](GUI.md), and [`BasicGraphics`](Helpers.md#Basic-Graphics)
Within the loop you'll have access to the variable `LOOP::GameLoop`, which has the following fields which you can read but should not set:
* `delta_seconds::Float32` : the amount of time elapsed since the last loop iteration.
* `frame_idx::Int` : the number of elapsed frames so far, plus 1.
* `last_frame_time_ns::UInt64` : the most recent timestamp, from the end of the last frame.
* `context::Context` : the OpenGL/GLFW context
* `service_input` : the [Input service](Input.md).
* `service_basic_graphics` : the [Basic Graphics service](#Basic-Graphics).
* `service_gui` : the [GUI service](GUI.md).
`LOOP` also has some fields which you can set to configure the loop. You can set them both in the `SETUP` phase and the `LOOP` phase.
* `max_fps::Optional{Int} = 300` caps the game's framerate.
* `max_frame_duration::Float32 = 0.1` caps the `delta_seconds` field in the case of very slow frames. This prevents significant jumps in the game after a hang.
# Basic Graphics
A [B+ Context service](GL.md#Services) that provides lots of basic resources:
e which defines a bunch of useful GL resources:
* `screen_triangle` : A 1-triangle mesh with 2D positions in NDC-space.
When drawn, it will perfectly cover the entire screen,
making it easy to spin up post-processing effects.
The UV coordinates can be calculated from the XY positions
(or from gl_FragCoord).
* `screen_quad` : A 2-triangle mesh describing a square, with 2D coordinates
in NDC space (-1 to +1)
This _can_ be used for post-processing effects, but it's less efficient
than `screen_triangle` for technical reasons.
* `blit` : A simple shader to render a 2D texture (e.x. copy a Target to the screen).
Refer to `simple_blit()`.
* `empty_mesh` : A mesh with no vertex data, for dispatching entirely procedural geometry.
Uses `PrimitiveType.points`.
To draw a texture, call `simple_blit(tex_or_view; params...)`. It has the following named parmeters:
* `quad_transform::fmat3x3` transforms the 2D quad.
* Defaults to the identity transform.
* `color_transform::fmat4x4` transforms the sampled color into an output color.
* Defaults to the identity transform.
* `output_curve::Float32` is an exponent that can be applied to all 4 output channels.
* `disable_depth_test::Bool` is a flag for automtically disabling depth tests before drawing the quad, then reinstating the depth test state afterwards.
* By default it's true.
* `manage_tex_view::Bool` is a flag for automatically calling `view_activate()` and `view_deactivate()` on the blitted texture/view if it's not already activated.
* By default it's true.
# Cam3D
A representation of a movable, turnable 3D camera.
* `Cam3D{F}` represents the camera state, using `F` as the floating-point type (e.x. `Float32`).
* `Cam3D_Settings{F}` represents the camera's config.
* `Cam3D_Inputs{F}` represents the camera controls for a particular frame.
To update the camera, do `(cam, settings) = cam_update(cam, settings, input, delta_seconds)`. Note that the settings may change too; this is the case if you support the `speed_change` input.
* `cam_basis(cam)` and `cam_rightward(cam)` calculate the camera's facing vectors (the former gets all 3 axes, the latter gets just the rightward axis).
* Get the view matrix with `cam_view_mat(cam)`.
* Get the projection matrix with `cam_projection_mat(cam)`.
* The camera's projection settings are either a `PerspectiveProjection{F}` or an `OrthographicProjection{F}`.
# File Cacher
A system for reloading files from disk anytime they are changed. To use this system, do the following:
1. Define the type of data that is cached. We will refer to this type as `TCached`. For example, a texture cache could use `BplusApp.GL.Texture`.
2. Define a function which loads, or *re*-loads, an asset from disk. We will refer to this as `reload_response::Base.Callable`.
* The signature should be `(path::AbstractString[, old_data;:TCached]) -> new_data::TCached[, dependent_files]`.
* If your loaded asset depends on other files beyond its own path, add a second return value which is an iterator of those files. Then a reload can be triggered by any of these files changing.
* This function is allowed to throw if the file can't be loaded for any reason.
3. Define a function which acknowledges an error from calling `reload_response`. We will refer to this as `error_response::Base.Callable`.
* The signature should be `(path::AbstractString, exception, trace[, old_data::TCached]) -> [fallback_data::TCached]`.
* If you want to return a fallback instance (like an "error texture"), you can return it here. Otherwise, return anything other than a `TCached`, such as `nothing`.
* You may also want to log an error here, e.x. `@error "Failed to load $path" ex=(exception, trace)`
4. Create an instance of the cacher with the above parameters: `FileCacher{TCached}(reload_response=reload_response, error_response=error_response, other_args...)`.
* Pass `relative_path = p` to change the relative path for files.
* It defaults to `pwd()`, a.k.a. the location Julia is running from.
* Pass `check_interval_ms = a:b` to change the randomized time interval for checking each file for changes.
* The randomization prevents all cached files from being checked at the same time, which could cause a disk bottleneck.
* Default is `3000:5000`, i.e. 3-5 seconds.
5. Update the cacher with `check_disk_modifications!(cacher)::Bool`.
* It returns true if any files have been reloaded.
* The naive way to call this is once every frame, but you can probably get away with doing it much less often since files are not reloaded very frequently.
6. Get a file with `get_cached_data!(cacher, relative_or_absolute_path)::Optional{TCached}`.
* If your `error_response` provides a fallback instance, then this function *always* returns a `TCached`. | Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 1449 | # Input
Provides a greatly-simplified window into GLFW input, as a [GL Context Service](docs/GL.md#Services). Input bindings are defined in a data-driven way that is easily serialized through the `StructTypes` package.
Make sure to update this service every frame (with `service_Input_update()`); this is already handled for you if you build your game logic within [`@game_loop`](docs/Helpers.md#Game-Loop).
You can reset the service's state with `service_Input_reset()`.
## Buttons
Buttons are binary inputs. A single button can come from more than one source, in which case they're OR-ed together (e.x. you could bind "fire" to right-click, Enter, and Joystick1->Button4).
Create a button with `create_button(name::AbstractString, inputs::ButtonInput...)`. Refer to the `ButtonInput` class for more info on how to configure your buttons. A button can come from keyboard keys, mouse buttons, or joystick buttons.
Get the current value of a button with `get_button(name::AbstractString)::Bool`.
## Axes
Axes are continuous inputs, represented with `Float32`. Unlike buttons, they can only come from one source.
Create an axis with `create_axis(name::AbstractString, input::AxisInput)`. Refer to the `AxisInput` class for more info on how to configure your axis. An axis can come from mouse position, scroll wheel, joystick axes, or a list of `ButtonAsAxis`.
Get the current value of an axis with `get_axis(name::AbstractString)::Float32`. | Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 6727 | A wide variety of game math.
## Functions
* `lerp(a, b, t)`, `smoothstep([a, b, ]t)`, and `smootherstep([a, b, ]t)` implement the common game interpolation functions. `smoothstep` and `smootherstep` optionally take just a `t` value (implicitly using `a=0` and `b=1`).
* `inv_lerp(a, b, x)` performs the inverse of `lerp`: `lerp(a, b, inv_lerp(a, b, x) == x`.
* `fract(f)` gets the fractional part of `f`. Negative values wrap around; for example `fract(-0.1) == 0.9` within floating-point error.
* `saturate(x)` is equal to `clamp(x, 0, 1)`.
* `square(x)` is equal to `x*x`.
* `typemin_finite(T)` and `typemax_finite(T)` are an alternative to Julia's `typemin(T)` and `typemax(T)` that returns finite values for float types.
* `round_up_to_multiple(v, multiple)` rounds an integer (or `Vec{<:Integer}`) up to the next multiple of some other integer (or `Vec{<:Integer}`). For example, `round_up_to_multiple(7, 5) == 10`.
* `solve_quadratic(a, b, c)` returns either `nothing` or the two solutions to the quadratic equation `ax^2 + bx + c = 0`.
## Vectors, Matrices, Quaternions
These objects are described in separate documents.
* [`Vectors`](Vec.md)
* [`Quaternions`](Quat.md)
* [`Matrices`](Matrix.md)
## Rays
`Ray{N, F<:AbstractFloat}` defines an `N`-dimensional ray. You can construct it with a start position and direction vector.
Aliases exist for dimensionality: `Ray2D{F}`, `Ray3D{F}`, `Ray4D{F}`.
Aliases also exist assuming Float3: `Ray2`, `Ray3`, `Ray4`.
* `ray_at(ray, t)` gets the point on the ray at the given distance from the origin (proportional to the ray direction's magnitude).
* `closest_point(ray, pos; min_t=0, max_t=typemax_finite(F))::F` gets the point along the ray (as its `t` scalar value) which is closest to the given position. By default, limits the output to be >= 0 like a true ray, but you can change the allowed range of `t` values.
Ray intersection calculations can be found in the [Shapes](#Shapes) section of this document.
## Box and Interval
Contiguous spaces can be represented by `Box{N, T}`, and contiguous number ranges represented by `Interval{T}`. All `Box` functions are also implemented by `Interval`, using scalar data instead of vector data.
`Box` is part of the `AbstractShape` system (see the [Shapes](#Shapes) section), but this section focuses on the more general utility of `Box` (and `Interval`).
Like `Vec`, boxes have many aliases based on type and dimensionality. For example, `Box2Du`, `Box4Df`, `BoxT`. Intervals have aliases for common types: `IntervalI`, `IntervalU`, `IntervalF`, and `IntervalD`.
They can be constructed in several ways:
* Provide two named arguments from: `min`, `max` (inclusive), `size`, `center`. For example, `Box2Df(min=v2f(0, 0), max=v2f(1.5, 2.2))`.
* Construct the bounding box of some points and boxes (and other `AbstractShape` types) with `boundary(elements...)`.
* Create a box with the min and size set to 0 by constructing it with only its type arguments. For example, `Box2Di()`.
You can get the properties of a box with the following getters:
* `min_inclusive(box)`
* `max_exclusive(box)`
* `max_inclusive(box)` (only really useful for integer-type boxes)
* `min_exclusive(box)` (only really useful for integer-type boxes)
* `size(box)` (a new overload of the built-in function `Base.size()`)
* `center(box)`
* `corners(box)` calculates a tuple of all `2^N` corner points.
Some other box/interval functions (again, not including the `AbstractShape` interface) are:
* `is_empty(box)::Bool` returns whether the box's volume is <= 0
* `is_inside(box, point)::Bool` returns whether the point is inside the box, not touching its edges. This is only really useful for integer-type boxes.
* `contains(outer_box, inner_box)::Bool` checks whether the first box totally contains the second.
* `Base.intersect(boxes::Box...)::Box` gets the intersection of one or more boxes. You can call `is_empty(result)` to check if there is no intersection.
* `Base.reshape(box, new_dimensionality::Integer; ...)` removes or adds dimensions to the box. Added dimensions have a specific min and size (specified in named parameters).
Integer boxes and intervals can be used to slice into a multidimensional array.
For example, `myArr[Box(min=v3i(3, 4, 5), max=v3i(5, 5, 5))]` is equivalent to `myArr[3:5, 4:5, 5:5]`.
Boxes can be serialized and deserialized. Deserialization can come from any pair of constuctor parameters: `min`, `max`, `size`, `center`. For example, you can deserialize a Box2Df from this JSON string using the JSON3 package: `"{ "min": [ 1, 2 ], "size": [5, 5] }"`.
## Shapes
`AbstractShape{N, F}` is some N-dimensional shape using numbers of type `F`. Its interface is as follows:
* `volume(s)::F`
* `bounds(s)::Box{N, F}` (Box is a specific type of `AbstractShape`, described below)
* `center(s)::Vec{N, F}`
* `is_touching(s, p::Vec{N, F})::Bool`
* `closest_point(s, p::Vec{N, F})::Vec{N, F}`
* `intersections(s, r::Ray{N, F}; ...)::UpTo{M, F}`
* `collides(a::AbstractShape{N, F}, b::AbstractShape{N, F})::Bool`
* **Important note**: mostly unimplemented as of time of writing
**Important note**: shapes are still under development, so significant chunks of it are untested or unimplemented, and the API is a work-in-progress.
### Ray intersections
The interface for ray intersections is a bit wonky (and, as mentioned above, still under development).
By default, `intersections(shape, ray)` returns `UpTo{N, F}` intersections. If you also want the surface normal at the closest intersection, pass a third parameter `Val(true)`. In this case, it instead returns a `Tuple{UpTo{N, F}, Vec3{F}}`.
### Box
Box, [as mentioned above](#Box-And-Interval), is a particularly useful shape. For more info on the rest of its interface, unrelated to `AbstractShape`, see the linked section. Note that `Interval` does *not* implement `AbstractShape`.
## Contiguous
`Contiguous{T}` is an alias for any nested container of `T` that appears to be contiguous in memory. For example, a `Vector{v2i}` is both a `Contiguous{Int32}` and a `Contiguou{v2i}`.
The number of `T` in a `Contiguous{T}` is found with `contiguous_length(data, T)`.
Contiguous data can be turned into a `Ref` for interop using `contiguous_ref(values, T, idx=1)`. It can also be turned directly into a pointer with `coniguous_ptr(values, T, idx=1)`.
## Random Generation
* `rand_in_sphere(u, v, r)` generates a uniform-random `Vec3` within the unit sphere, given three uniform-random values.
* To generate the three values from an RNG, call `rand_in_sphere([rng][, F = Float32])`.
* `perlin(pos::Union{Real, Vec})` generates N-dimensional Perlin noise.
* This function is highly customizable; refer to its doc-string. | Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 4744 | Part of the [`Math` module](Math.md). The core game math types are: `Vec{N, T}`, [`Quaternion{F}`](Quat.md), and [`Mat{C, R, F}`](Matrix.md).
# `Mat{C, R, F}`
A statically-sized matrix, perfect for representing vector transformations.
## Aliases
Aliases are particularly important for `Mat` to avoid confusion (see the section [StaticArrays](#StaticArrays) below).
Matrices are named very similarly to HLSL, with the prefix `f` for Float32 and `d` for Float64, and the suffix `[columns]x[rows]`. If the row and column count is the same, you may abbreviate the suffix with just one number. For example:
* `fmat2x2` is a 2x2 Float32 matrix.
* `dmat3x4` is a 3-column, 4-row Float64 matrix.
* `fmat3` is a 3x3 Float32 matrix.
There are also aliases for specific matrix sizes:
* `Mat2{F}` is a 2x2 matrix.
* `Mat3{F}` is a 3x3 matrix.
* `Mat4{F}` is a 4x4 matrix.
If you need to manually specify a new matrix type, it's highly recommended to use the macro `@Mat(C, R, F)` because Julia's type system actually requires a fourth parameter which is `C * R`.
## Usage
In keeping with vectors and quaternions, matrix operations are prefixed with `m_`:
* To apply a matrix transform to a point, use one of the following:
* `m_apply_point(m, p)` for coordinates
* `m_apply_vector(m, v)` for vectors (ignores translation)
* If your matrix is affine (almost always true for world and view matrices, but not for a perspective projection matrix), you can use optimized versions:
* `m_apply_point_affine(m, p)` for coordinates and affine matrices.
* `m_apply_vector_affine(m, v)` for vectors and affine matrices.
* `m_combine(a, b...)` multiplies matrices together in chronological order, to produce a transformation of "a, then b, then ..."
* `m_invert(m)` inverts a matrix.
* `m_tranpose(m)` transposes a matrix.
* You can also use the `'` operator, for example `m = m'`.
* `m_identity(C, R, F)` creates an identity matrix.
* `m_identityf(C, R)` creates a Float32 matrix.
* `m_identityd(C, R)` creates a Float64 matrix.
* `m_to_mat4x4(m)` converts a 3x3 matrix to 4x4.
* `m_to_mat3x3(m)` drops the last row and column off a 4x4 matrix.
* Operator `*` performs multiplication with `Vec`, or other `Mat` instances. You can use it to transform a point/vector by a matrix, but the above explicit functions are preferred.
* B+ uses pre-multiplication, i.e. `M*v`. To make that unambiguous, `v*M` is not defined at all.
## Transforms
* `m3_translate(delta)` and `m4_translate(delta)` make 2D and 3D translation matrices, respectively.
* `m_scale(v)` and `m_scale(f, N)` creates a scale matrix using a vector, or a scalar spread over N dimensions, respectively.
* `m[N]_rotate[A](radians)` makes a rotation matrix around axis `A`, in 3x3 or 4x4 format `N`.
* For example, `m3_rotateX(radians)`.
* `m3_rotate(q)` and `m4_rotate(q)` make a rotation matrix for a quaternion.
* `m4_world(pos, rot, scale)` makes a typical World transform matrix.
* `m4_look_at(cam_pos, target_pos, up)` makes a typical View transform matrix.
* `m3_look_at(forward, up, right; [new basis])` makes a rotation matrix to turn the given basis into a typical View basis (by default: +X right, +Y up, -Z forward).
* You can also think of this as a camera View matrix without the translation.
* `m3_look_at(from::VBasis, to::VBasis)` makes a rotation matrix that turns the "from" vector basis into the "to" vector basis.
* `m4_projection(near_clip, far_clip, aspect_width_over_height, fov_degrees)` creates a typical OpenGL perspective matrix.
* `m4_ortho(range::Box3)` makes an orthographic projection matrix, mapping the given axis-aligned box to the range -1 => +1 along each axis.
## Component access
`Mat` behaves very similar to a Julia multidimensional array, and can be accessed in the same way.
As mentioned in the `Vec` docs, indexing a matrix with a 2D integer `Vec` is counter-intuitive:
the X component is the row, and the Y component is the column.
## StaticArrays
Under the hood, `Mat` is just an alias for `StaticMatrix`, or `SMatrix`, from the *StaticArrays* package.
However, it swaps the type parameters in order to be aligned with OpenGL --
Column then Row, whereas `SMatrix` does Row then Column.
Fortunately, the memory order of matrices is already in sync between *StaticArrays* and OpenGL.
They are column-major, meaning the second element in memory (`my_matrix[2]`) is column-1, row-2.
All `SMatrix` types come with a fourth type parameter, which is the total length (row count * column count).
Since this can be computed trivially from `C` and `R`, the convenience macro `@Mat(C, R, F)` is provided.
And of course there are [plenty of aliases](#Aliases) to cover 99% of the matrix types you will encounter in graphics. | Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 3378 | Part of the [`Math` module](Math.md). The core game math types are: `Vec{N, T}`, [`Quaternion{F}`](Quat.md), and [`Mat{C, R, F}`](Matrix.md).
# `Quaternion{F}`
Four numbers that together, efficiently and effectively represent 3D rotations.
Like `Vec`, this type is immutable and able to be allocated on the stack.
You can use `@set!` to create modified copies, but you shouldn't have to fiddle with a quaternion's individual components anyway.
## Aliases
* `fquat` is a Float32 quaternion.
* `dquat` is a Float64 quaternion.
## Construction
* You can provide the 4 components of the Quaternion directly as individual values, or a tuple, or a `Vec4`.
* You can provide nothing, to create an identity quaternion. For example, `fquat()`.
* You can provide an axis and angle (in radians). For example, `Quaternion(get_up_vector(), deg2rad(180))`
* You can provide a start and end vector, both normalized, to get the rotation from one to the other. For example, `Quaternion(get_up_vector(), vnorm(v3f(1, 1, 1)))`.
* You can provide a rotation matrix (see [`Mat{C, R, F}`](Matrix.md)) to convert it to a quaternion.
* You can provide a `VBasis` (the output of `vbasis(forward, up)`) to create a Quaternion representing the rotation that creates this basis.
* This constructor needs to know what an "un-rotated" basis is. For example, what direction is Up? By default, this comes from [the coordinate system configured for B+](Vec.md#Coordinate-System).
* You can provide a start and end `VBasis` to create a rotation transforming the former to the latter.
* You can provide a sequence of quaternions in chronological order, to make one quaternion representing all those rotations done in sequence.
## Operations
* `q_apply(q, v)` to rotate a `Vec`.
* `a >> b` and `a << b` to combine two quaternion rotations in the order specified by the arrow (respectively, "a then b" and "b then a").
* For a quick and cheap interpolation between quaternion rotations, `lerp(a, b, t)` works just fine.
* For higher-quality interpolation, such as an animation, `q_slerp(a, b, t)` is better.
* `q_basis()` gets the vector basis (as a `VBasis` instance) created by the given rotation. It assumes specific axes for "forward", "up", and "right", which you can configure with named parameters.
* `q_is_identity(q)` tests whether a Quaternion represents "no rotation".
* `q_slerp(a, b, t)` to interpolate between `a` and `b` using a nicer-quality interpolation that requires more math to compute.
* The standard `lerp()` function can also be used for Quaternions, but for animation it does not produce as nice an effect.
## Conversions
* `q_axisangle(q)::Tuple{Vec3, F}` returns the axis and radians of the rotation represented by this quaternion.
* `q_mat3x3(q)` and `q_mat4x4(q)` get the rotation matrix for this quaternion.
## Arithmetic
It's not recommended that you use these directly unless you know what you're doing.
* Multiplication (`a*b`) and negation (`-a`) are defined as you'd expect.
* `qnorm(q)` and `q_is_normalized(q)` help with normalization.
## Printing
Quaternions are printed as an axis and angle. The number of digits in each are controlled by `QUAT_AXIS_DIGITS` and `QUAT_ANGLE_DIGITS`, respectively.
To temporarily change the number of digits used, invoke `use_quat_digits()`.
To print a single quaternion with a specific number of digits, use `show_quat()`. | Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 34264 | Part of the [`GL` module](GL.md#Resources). Represents a managed OpenGL object.
All resource types implement the following interface (see doc-strings for more details):
* `get_ogl_handle(resource)`
* `is_destroyed(resource)::Bool`
* `Base.close(resource)` to clean it up
# Program
`Program` is a compiled shader, either for rendering or compute.
### From string literal
You can compile a shader from a static string literal with Julia's macro string syntax: `bp_glsl" [multiline shader code here] "`. Within the string, you can define mutiple sections:
* Code at the top of the string will be re-used for all shader stages.
* A specific token will be defined based on which shader stage you are in: `IN_VERTEX_SHADER`, `IN_FRAGMENT_SHADER`, `IN_GEOMETRY_SHADER`, `IN_COMPUTE_SHADER`.
* Code following the token `#START_VERTEX` is used as the vertex shader.
* Code following the token `#START_FRAGMENT` is used as the fragment shader.
* Code following the token `#START_GEOMETRY` (optional) is used as the geometry shader.
* Code following the token `#START_COMPUTE` makes this Program into a compute shader, forbidding all other shader types.
For dynamic strings, you can call `bp_glsl_str(shader_string)` to get the same result.
To get a printout of the final shaders, you can pass `debug_out = stderr`.
### From simple compilation
For a little more control, use the constructor `Program(vert_shader, frag_shader; geom_shader = nothing)`, or `Program(compute_shader)`.
It also takes an optional named parameter `flexible_mode::Bool` (defaulting to `true`), which indicates whether it should suppress errors when setting uniforms that have been optimized out.
### From advanced compilation
For more advanced features, you can use `ProgramCompiler`. Construct an instance of it, try compiling with `compile_program()`, then if that succeeds, construct the `Program` with the output of `compile_program()`. For more details, see the doc-strings for these types and functions.
The main purpose of `ProgramCompiler` is that it can keep a cached pre-compiled version of the shader (presumably loaded from disk). If the cache is given, it tries to use that to compile the program first, only falling back to string compilation if it fails. On the other side of things, after successfully compiling from a string, the binary cache is updated so that you can write it out to disk for the next time the program is started.
*NOTE*: Compilation from a cached binary fails very often, for example after a driver update, so you can never rely on it. You should always provide the string shader as a fallback.
## Uniforms
Uniforms are parameters given to the shader. Lots of data types can be given as uniforms.
You can see the uniforms in a compiled program with `program.uniforms`, `program.uniform_blocks`, and `program.storage_blocks`, but do not modify those collections directly!
### Basic data
Basic uniform data can be individual variables (e.x. `uniform vec3 myPos;`), or arrays of variables (e.x. `uniform vec4 lightPositions[10];`). Either way, the following types of data are supported:
* Scalars, including booleans (specified by the type alias `UniformScalar`).
* Vectors (specified by the type alias `UniformVector{1}` through `UniformVector{4}`).
* Matrices (specified by the type alias `UniformMatrix{2, 2}` through `UniformMatrix{4, 4}`).
* Textures, in several forms:
* The `Texture` resource itself (in which case its default sampler `View` is used).
* A `View` of a texture resource.
* A raw handle to a view, of type `Ptr_View`.
* For more info on texture views, see [Texture](Resources.md#Texture) below.
The type alias `Uniform` explicitly captures all of these.
Uniforms can be set with the following functions:
* `set_uniform(program, name, value::Uniform[, array_index])` sets a single uniform within a program. If that uniform is part of an array, you should provide the 1-based index.
* `set_uniforms(program, name, value_list::Vector{<:Uniform}[, dest_offset=0])` sets an array of uniforms given a `Vector` of their values. Optionally starts with a uniform partway through the array, using `dest_offset`.
* If the elements are instances of `Texture` or `View`, then the rules are relaxed bit: you can provide any iterator of them, not just a `Vector{Texture}` or `Vector{View}`.
* `set_uniforms(program, name, T::Type{<:Uniform}, contiguous_elements[, dest_offset=0])` sets an array of `T`-type uniforms to the data in some [`Contiguous{T}`](Math.md#Contiguous). This is useful if, for example, you want to set an array of floats using a `Vector{v4f}`. The previous overload of `set_uniforms()` would infer the uniform type to be `vec4` instead of `float`, causing problems.
### Buffer data
Additionally, you can provide [buffer data](Resources.md#Buffer) to the shader in one of two forms, explained below.
**Important note**: The packing of buffer data into one a shader block follows very specific layout rules, most commonly "std140" and "std430". See the [Buffer section](Resources.md#Buffer) for helper macros to pack data correctly.
#### Uniform Buffers
Called "Uniform Buffer Objects" or "UBO" in OpenGL. These are replacements for individually setting uniforms per-program. A Uniform Buffer is assigned to a global slot, then any and all shaders can reference that slot to set a block of uniforms using the buffer's data.
They are meant to be relatively small and read-only. For larger data, or data that can also be written to, use [Storage Buffers](#Storage-Buffers) instead.
Assign a buffer to a global uniform slot with `set_uniform_block(buffer, slot::Int[, byte_range])`. By default, the buffer's full set of bytes is used for the uniform block. You can pass a custom range if the uniform data starts at a certain byte offset, or should be limited to a subset of the full range.
Point a shader's uniform block to that global slot with `set_uniform_block(program, block_name, slot::Int)`.
Clear a Uniform Buffer global slot with `set_uniform_block(slot::Int)`.
#### Storage Buffers
Called "Shader Storage Buffer Objects" or "SSBO" in OpenGL. These are arbitrarily-large arrays which you can both read and write to. They are very important for compute shaders.
For small, read-only data, use [Uniform Buffers](#Uniform-Buffers) instead.
Assign a buffer to a global storage slot with `set_storage_block(buffer, slot::Int[, byte_range])`. By default, the buffer's full set of bytes is used for the uniform block. You can pass a custom range if the shader data starts at a certain byte offset, or should be limited to a subset of the full range.
Point a shader's storage block to that global slot with `set_storage_block(program, block_name, slot::Int)`.
Clear a Storage Buffer global slot with `set_storage_block(slot::Int)`.
# Buffer
A struct or array of data, stored on the GPU. It is often used for mesh data, but can also be used in shaders.
If using them in shaders, make sure to check the [Packing section](#Packing) below.
To create a buffer, just call one of its constructors.
The most basic constructor is `Buffer(byte_size, can_cpu_change_data_after_creation, recommend_storage_on_cpu=false)`.
Other constructors allow you to pass some initial data, or a bits Type whose byte-size is used.
The variety of data you can give to the constructor mostly matches [the data you can pass into `set_buffer_data()`](#Data).
You cannot resize a buffer after creating it.
## Data
To set a buffer's data (or a subset of it), call `set_buffer_data(buffer, ...)`.
There are several overloads for different kinds of data, including:
* `set_buffer_data(buf, ptr::Ref, buffer_byte_range::IntervalU = [all bytes])`
* `set_buffer_data(buf, array::AbstractArray, buffer_first_byte=1)`
* `set_buffer_data(buf, some_bits_data, buffer_first_byte=1)`
* `set_buffer_data(buf, block::AbstractOglBlock, buffer_first_byte=1)`
* `AbstractOglBlock` is any type created with [`@std140` or `@std430`](#Packing).
* `set_buffer_data(buf, block_array::BlockArray, buffer_first_byte=1)`
* `BlockArray` is a special kind of array that matches the [layout of the data on the GPU](#Packing)
To get a buffer's data (or a subset of it), call `get_buffer_data(buffer, ...)`.
There are several overloads for different kinds of data, including:
* `get_buffer_data(buf, ptr::Ref, buffer_byte_range::IntervalU = [all bytes])`
* `get_buffer_data(buf, array::AbstractArray, buffer_first_byte=1)`
* `get_buffer_data(buf, T, buffer_first_byte=1)::T`
* The type `T` must be one of the following:
* A bitstype
* Any type created with [`@std140` or `@std430`](#Packing)
* A GPU-layout array type inheriting from `BlockArray`, for example `StaticBlockArray{N, T, TMode<:Union{OglBlock_std140, OglBlock_std430}}`
* `get_buffer_data(buf, (T, size...), buffer_first_byte=1)::Array{T}`
* The type `T` must be a bitstype
To copy one buffer's data to another, call `copy_buffer(src, dest; ...)`. You can use the optional named parameters to pick subsets of the source or destination buffer.
## Packing
When using buffers in shaders (see [Buffer Data](#Buffer-Data) above), it's important to make sure the data is packed properly. OpenGL allows the "std140" packing standard for Uniform Blocks ("UBO"), and either "std140" or the more efficient "std430" for Storage Blocks ("SSBO").
These formats can be tricky to pack correctly. So B+ provides two macros, `@std140` and `@std430`, which set up a struct's data to be padded exactly the way it should be on the GPU! This helps you ensure the Julia version of a struct matches the shader version exactly.
See the doc-strings for these macros for help using them. Valid field types are:
* Any `Uniform` (see [Uniforms](#Uniforms) above)
* A nested struct that was also defined with the same macro (`@std140` or `@std430`).
* A static array, of type `StaticBlockArray{N, T}`.
# Texture
A 1D, 2D, 3D, or "Cubemap" grid of pixels. B+ uses the Bindless Textures extension, so textures are mostly passed to shaders as simple integer handles, associated with a particular `View` on that texture.
Texture arrays are not needed in a renderer with bindless textures, so they aren't supported.
MSAA textures are not yet implemented.
## Sampling
Textures can be associated with sampler objects, of type `TexSampler{N}`. `N` is the dimensionality of the texture; useful because wrapping behavior can be specified per-axis. Cubemap textures are special -- they don't have "wrapping" behavior or a border color, so B+ uses `TexSampler{1}` for them. All samplers are upscaled to `TexSampler{3}` when stored in the `Texture` resource for type-stability.
A texture is associated with a specific sampler setting on creation, but you can easily request a different sampler when passing the texture to a shader (see [Views](#Views) below).
The different parameters you can pass to a `TexSampler` are specified clearly in the struct's definition, so refer to it for more information.
`TexSampler` supports serialization with the *StructTypes.jl* package (and therefore the *JSON3* package).
### SamplerProvider service
Sampler objects can be re-used across textures, so there's no need for many of them. There is a built-in service that provides samplers, `SamplerProvider`, that textures use internally. You shouldn't have to use it, but if you're making bare OpenGL calls it may be useful to have.
## Creation
Create a 1D, 2D, or 3D texture with `Texture(format, size; args...)` or `Texture(format, data::PixelBuffer, data_bgr_ordering::Bool = false; args...)`.
* The dimensionality is inferred from the dimensionality of `size` or `data`.
Create a cubemap texture with `Texture_cube(format, square_length::Integer; args...)` or `Texture(format, six_faces_data::PixelBufferD{3}; args...)`. For more info on how cubemaps are specified, see [Cubemaps](#Cubemaps) below.
The optional arguments to the constructor are as follows:
* `sampler = TexSampler{N}()` is the default sampler associated with the N-dimensional texture.
* `n_mips::Integer = [maximum]` is the number of mips the texture has. Pass `1` to effectively disable mip-mapping.
* `depth_stencil_sampling::Optional{E_DepthStencilSources}` controls what can be sampled/read from a texture with a depth-stencil hybrid format. Only one of Depth or Stencil can be read at a time.
* This can be changed after construction with `set_tex_depthstencil_source(tex, new_value)`.
* `swizzling` can switch the components of the texture when it's being sampled or read from an Image view. Does *not* affect writes to the texture, or blending operations when rendering to the texture.
* This can be changed after construction with `set_tex_swizzling(tex, new_swizzle)`.
## Upload/Download Format
You can use almost any kind of basic scalar/vector data to upload or download a texture's pixels. The set of allowed pixel array types is captured by the type alias `PixelBuffer`, which is a Julia array of `N` dimensions and `T` elements. `N` is the texture dimensionality and `T` is the pixel data type. `T` must come from the type alias `PixelIOValue`, which is either a scalar `PixelIOComponent` or a vector of 1 to 4 such components.
If you're loading an image using the *FileIO* package, you can convert those image pixels to B+ data of a particular type `T` (such as `v3f`) using `convert_pixel(pixel, T)`.
For example, `using FileIO, ImageIO; bplus_pixel_array = convert_pixel.(FileIO.load("MyImage.png"), vRGBu8)`
For color textures, the components to work with are usually deduced from the type of the pixel data.
For example, an array of `v2f` is assumed to be working with the RG components.
If uploading/downloading a single channel from the texture, it defaults to the Red channel.
* Change deduced channels from *RGB* to *BGR* by passing `bgr_ordering=true` into texture operations.
* Change the single-channel from *Red* to *Green* or *Blue* by passing e.x. `single_component=PixelIOChannels.green` into texture operations.
**Important Note**: when setting fewer channels than actually exist in a color texture (e.x. only Green in an RG texture, or only RG in an RGBA texture), OpenGL sets missing color channels to 0 and the missing Alpha channel to 1. They are not left unchanged as you might expect.
For hybrid depth-stencil textures, you can use the special packed pixel types `Depth24uStencil8u` or `Depth32fStencil8u` depending on the specific format. These can be directly uploaded into their corresponding textures.
Cubemap textures are treated as 3D, where the Z coordinate represents the 6 faces.
## Operations
### Clearing
To generically clear a texture without knowing the format type, use `clear_tex_pixels(tex, value, ...)` and it will be inferred from the texture format and provided pixel value.
It's recommended to use one of the more specific functions when you know the format:
* `clear_tex_color(tex, color::PixelIOValue, ...)`
* `clear_tex_depth(tex, depth::PixelIOComponent, ...)`
* Can't be used for hybrid depth-stencil textures
* `clear_tex_stencil(tex, stencil::UInt8, ...)`
* Can't be used for hybrid depth-stencil textures
* `clear_tex_depthstencil(tex, depth::Float32, stencil::UInt8, ...)`
* `clear_tex_depthstencil(tex, value::Union{Depth24uStencil8u, Depth32fStencil8u}, ...)`
* The pixel format must precisely match the texture format.
These functions have the following optional arguments:
* `subset::TexSubset = [entire texture]`. For cubemap textures, this subset is 2D, and cleared on each desired face.
* `bgr_ordering::Bool = false` (only for 3- and 4-channel color) should be true if data is specified as BGR instead of RGB (faster for upload in many circumstances).
* `single_component::E_PixelIOChannels` (only for 1-component color) controls which color channel is being set, if only one channel is provided. Must be `red`, `green`, or `blue`, not any of the multi-channel enum values.
* `recompute_mips::Bool = true` if true, automatically computes mips after clearing.
### Setting pixels
When setting a texture's pixels, you must provide an array of the same dimensionality as the texture you're setting.
For more info on pixel data formats, see [*Upload/Download Format* above](#UploadDownload-Format).
To generically set a texture's pixels without knowing the format type, use `set_tex_pixels(tex, pixels::PixelBuffer; args...)` and Julia will infer it from the texture format and provided pixel format.
**Important Note**: when setting fewer channels than actually exist in a color texture (e.x. only Green in an RG texture, or only RG in an RGBA texture), OpenGL sets missing color channels to 0 and the missing Alpha channel to 1. They are not left unchanged as you might expect.
It's recommended to use one of the more specific functions when you know the format:
* `set_tex_color(tex, color::PixelBuffer, ...)`
* `set_tex_depth(tex, depth::PixelBuffer, ...)`
* Can't be used for hybrid depth-stencil textures
* `set_tex_stencil(tex, stencil::PixelBuffer{UInt8}, ...)`
* Can't be used for hybrid depth-stencil textures
* Also accepts a `Vector{Vec{1, UInt8}}`
* `set_tex_depthstencil(tex, value::PixelBuffer{<:Union{Depth24uStencil8u, Depth32fStencil8u}}, ...)`
* The pixel format must precisely match the texture format.
These functions have the following optional arguments:
* `subset::TexSubset = [entire texture]`. For cubemap textures, this subset is set on each desired face.
* `bgr_ordering::Bool = false` (only for 3- and 4-channel color) should be true if data is specified as BGR instead of RGB (faster for upload in many circumstances).
* `recompute_mips::Bool = true` if true, automatically computes mips afterwards.
* `single_component::E_PixelIOChannels = PixelIOChannels.red` : which color channel is getting set. Only relevant if you are passing scalar values to a color texture.
### Getting pixels
When getting a texture's pixels, you must provide an output array of the same dimensionality as the texture you're setting. Cubemap textures are considered 3D, with Z spanning the 6 faces.
For more info on pixel data formats, see [*Upload/Download Format* above](#UploadDownload-Format).
It's recommended to use one of the more specific functions when you know the format:
* `get_tex_color(tex, out_colors::PixelBuffer, ...)`
* `get_tex_depth(tex, out_depth::PixelBuffer, ...)`
* Can't be used for hybrid depth-stencil textures
* `get_tex_stencil(tex, out_stencil::PixelBuffer{UInt8}, ...)`
* Can't be used for hybrid depth-stencil textures
* Also accepts a `Vector{Vec{1, UInt8}}`
* `get_tex_depthstencil(tex, out_hybrid::PixelBuffer{<:Union{Depth24uStencil8u, Depth32fStencil8u}}, ...)`
* The pixel format must match the texture format.
These functions have the following optional named arguments:
* `subset::TexSubset = [entire texture]`. For cubemap textures, this subset is set on each desired face.
* `bgr_ordering::Bool = false` (only for 3- and 4-channel color) should be true if data is specified as BGR instead of RGB (faster for download in many circumstances).
### Copying
You can directly copy the bits of one texture's pixels to another texture with `copy_tex_pixels(src, dest, args...)`.
This is comparable to a `memcpy()`, meaning that the data is copied over without translation.
It is allowed if and only if the source and destination texture have the same bit size per-pixel.
When copying between a compressed and uncompressed texture, the requirement is a bit different: the bit size of one pixel of the uncompressed texture should match the bit size of one *block* of the compressed texture.
Optional arguments are as follows:
* `src_subset::TexSubset` : picks a subset of the source texture.
* `dest_min = 1` : picks a min corner of the destination texture to copy to.
* `dest_mip = 1` : picks a mip level of the destination texture to copy to.
### Other
Query a texture's metadata with:
* `get_mip_byte_size(tex, mip_level::Integer)`
* `get_gpu_byte_size(tex)`
Update a texture's global sampling settings (regardless of sampler object) with:
* `set_tex_swizzling(tex, new_swizzle)`
* `set_tex_depthstencil_source(tex, new_source)`
For information on how to deal with bindless texture views, see [Views](#Views) below.
## Cubemaps
The precise specification for cubemap pixel data in OpenGL is annoying to keep in mind. So B+ defines some data in *GL/textures/cube.jl* to encode this for you:
* The enum `E_CubeFaces` lists all 6 faces in their memory order. For example, `CubeFaces.from_index(1)` gets the first face, which is `CubeFaces.pos_x`.
* The six faces and their orientations are specified in `CUBEMAP_MEMORY_LAYOUT`, which is a tuple of 6 `CubeFaceOrientation`.
* `CubeFaceOrientation` has a lot of useful data, plus helper functions. For example:
* The UV.x axis on the +Y face is `CUBEMAP_MEMORY_LAYOUT[CubeFaces.to_index(CubeFaces.pos_y)].horz_axis`.
* The UV coordinate `v2f(0.85, 0.2)` on the -Z face corresponds to the cubemap vector `get_cube_dir(CUBEMAP_MEMORY_LAYOUT[CubeFaces.to_index(CubeFaces.neg_z)], v2f(0.85, 0.2))`.
* The first pixel on the +X face corresponds to the cubemap corner `CUBEMAP_MEMORY_LAYOUT[CubeFaces.to_index(CubeFaces.pos_x)].min_corner`.
## Format
Texture formats have a very clear and unambiguous specification in B+. A `TexFormat` is one of:
* `SimpleFormat`, a struct combining a `E_FormatTypes`, a `E_SimpleFormatComponents`, and a `E_SimpleFormatBitDepths`.
* For example, 4-bit RGBA is `SimpleFormat(FormatTypes.normalized_uint, SimpleFormatComponents.RGBA, SimpleFormatBitDepths.B4)`.
* Some combinations of these are not valid, for example any formats that amount to less than 8 bits per pixel.
* `E_CompressedFormats` for block-compressed formats like DXT5 or BC7.
* `E_SpecialFormats` for all the special cases:
* Asymmetrical bit depths, like `GL_RGB565`
* sRGB formats, like `GL_SRGB_ALPHA8`
* Esoteric formats, like `GL_RGB9_E5`
* `E_DepthStencilFormats` for depth, stencil, and depth-stencil textures.
`TexFormat` implements the following interface. In cases where there isn't an exact answer (e.x. getting the bits per pixel of a block-compressed format), an estimation is provided.
* `is_supported(format, tex_type)`
* `is_color(format)`
* `is_depth_only(format)`, `is_stencil_only(format)`, and `is_depth_and_stencil(format)`
* `is_integer(format)`, and `is_signed(format)`
* `stores_channel(format, channel)`
* `get_n_channels(format)`
* `get_pixel_bit_size(format)`
* `get_byte_size(format, tex_size)`
* `get_ogl_enum(format)`
* `get_native_ogl_enum(format, tex_type)`
## Views
*NOTE*: To better understand this topic, refer to [outside resources on OpenGL bindless textures](https://www.khronos.org/opengl/wiki/Bindless_Texture).
With the Bindless Textures extension to OpenGL, textures in shaders are accessed in a much simpler way from how they were in traditional OpenGL. A texture is now just a UInt64 handle representing the texture under a certain "View". This texture can be passed around like any other UInt64, for example inside a Uniform Block or Storage Block.
A View on a texture is either associated with a sampler, allowing you to sample from the texture (what OpenGL calls a "Texture View"), or it is a "simple view" associated with a `SimpleViewParams`. Simple Views (what OpenGL calls an "Image View") only allow for reading and/or writing individual pixels of a specific mip level. It can also make a 3D or cubemap texture appear 2D by focusing on a single Z-slice or face, respectively.
Views for a texture are retrieved with `get_view(tex, custom_sampler=nothing)` and `get_view(tex, p::SimpleViewParams)`. Most functions which accept a `View` will also accept a `Texture` and automatically call `get_view(tex)` as needed.
**Important Note**: The GPU driver cannot predict when bindless textures are used, since they can be hidden anywhere as a plain int64, so you must manually tell it to "activate" a view before you can use it, with `view_activate()`. Then when you are done, you should "deactivate" the view to make room for other textures, with `view_deactivate()`.
* Simple views take an extra parameter, which is the access mode of the view. By default it is both read and write, but you can create a read-only or write-only view.
### 64-bit Integers in Shaders
Normally GLSL doesn't have 64-bit int types and so you need to stitch together two 32-bit uints; however, B+ also tries to add the "shader int64" extension which adds int64 and uint64 as first-class data types. Unfortunately this extension apparently isn't supported well on integrated GPU's.
# Mesh
Called a "Vertex Array Object" or "VAO" in OpenGL. A `Mesh` is a configuration of buffers reprsenting vertex data and optionally index data. It also has a default "primitive type" such as `triangle_strip`, but this can easily be overridden when rendering the mesh.
## Vertex Data
Vertex data is specified in two parts:
1. "Sources" name a list of buffers, as instances of `VertexDataSource`. Each buffer represents an array of vertex data, with some byte offset and an element byte size.
2. "Fields" or "Attributes" pull specific data from the listed sources. Represented as an instance of `VertexAttribute`.
For example, if you have one buffer which lists each vertex as position+normal+uv, then you'll have one "source" and three "fields".
### Vertex Fields
Fields are more complex than sources, as there are many ways to pull data out of a buffer for use in the vertex shader. `VertexAttribute` contains the following data:
* The source buffer index
* The byte offset from each element of the buffer to the field being extracted
* The type of the buffer data, and the way it should be interpreted in the vertex shader (see below).
* A "per_instance" number, for instanced rendering. If greater than 0, this data is per every N instances rather than per-vertex.
The full specification of the vertex data type is in *GL/buffers/vertices.jl*, but here is the gist:
Whatever data format is in our buffer, the goal is to make it appear in the vertex shader as one of a few standard data types -- `vec2`, `ivec2`, `uvec3`, `dvec4`, etc.
1. If your buffer data is a simple 1D to 4D vector of `Float16`, `Float32`, `Float64`, `UInt8`, `UInt16`, `UInt32`, `Int8`, `Int16`, or `Int32`: start by picking the B+ vector type matching your buffer's data type; we'll call it `V`. For example, if you're specifying UV coordinates which come in as 2 32-bit floats, use `V=v2f`.
1. If the data should pass directly through to the shader (e.x. `v2i` showing up as `ivec2`, or `v4f` showing up as `vec4`), then simply use `VSInput(V)`.
2. If the data is integer and should be casted or normalized into float, then use `VSInput_FVector(V, normalized::Bool)`.
2. If your buffer data is a *matrix* of size 2x2 up to 4x4, with 32-bit or 64-bit components, then pick the matching B+ matrix type `M` and use `VSInput(M)`. For example, `VSInput(fmat2x3)`.
3. If your buffer data is one of these weird types, use the corresponding special field type:
1. For an `N`-dimensional vector of fixed-point decimals (16 bits for integer, and 16 for decimal), which should appear as `vecN` in the vertex shader, use `VSInput_FVector_Fixed(N).`
2. For RGB unsigned-float data packed into 10B11G11, which appears in the shader as `vec3`, use `VSInput_FVector_Packed_UF_B10_G11_R11()`.
3. For RGBA data packed into 2-bit Alpha and 10-bits each for RGB, which may or may not be signed, and which appears in the shader as `vec4` through either casting or normalization, use `VSInput_FVector_Packed_A2_BGR10(signed::Bool, normalized::Bool)`.
## Example
Putting it all together, here is an example of a mesh made of two triangles in a strip, using one buffer per field:
````
buffer_positions = Buffer(false, [ v4f(-0.75, -0.75, -0.75, 1.0),
v4f(-0.75, 0.75, 0.25, 1.0),
v4f(0.75, 0.75, 0.75, 1.0),
v4f(2.75, -0.75, -0.75, 1.0) ])
buffer_colors = Buffer(false, [ vRGBu8(255, 123, 10),
vRGBu8(0, 0, 0),
vRGBu8(34, 23, 80),
vRGBu8(255, 255, 255) ])
buffer_bones = Buffer(false, UInt8[ 1, 2, 1, 3 ])
mesh = Mesh(
PrimitiveTypes.triangle_strip,
[
VertexDataSource(buffer_positions, sizeof(v4f)),
VertexDataSource(buffer_colors, sizeof(vRGBu8)),
VertexDataSource(buffer_bones, sozeif(UInt8))
],
[
VertexAttribute(1, 0x0, VSInput(v4f)),
VertexAttribute(2, 0x0, VSInput(vRGBu8, true)),
VertexAttribute(3, 0x0, VSInput(UInt8))
]
)
...
// [in shader]
in vec4 pos;
in vec3 color;
in uint bone;
````
And here is that same example, using one buffer which keeps all fields together:
````
struct Vertex
pos::v4f
color::vRGBu8
bone::UInt8
end
...
buffer = Buffer(false, [
Vertex(v4f(-0.75, -0.75, -0.75, 1.0),
vRGBu8(255, 123, 10),
1),
Vertex(v4f(-0.75, 0.75, 0.25, 1.0),
vRGBu8(0, 0, 0),
2),
Vertex(v4f(0.75, 0.75, 0.75, 1.0),
vRGBu8(34, 23, 80),
1),
Vertex(v4f(2.75, -0.75, -0.75, 1.0),
vRGBu8(255, 255, 255),
3)
])
mesh = Mesh(
PrimitiveTypes.triangle_strip,
[ VertexDataSource(buffer, sizeof(Vertex)), ],
[
VertexAttribute(1, 0x0, VSInput(v4f)),
VertexAttribute(1, sizeof(v4f), VSInput(vRGBu8, true)),
VertexAttribute(1, sizeof(v4f) + sizeof(vRGBu8), VSInput(UInt8))
]
)
...
// [in shader]
in vec4 pos;
in vec3 color;
in uint bone;
````
## Index Data
If the mesh should be able to use indexed rendering, provide a `MeshIndexData` on construction. This struct points to a specific buffer and index type (`UInt8`, `UInt16`, or `UInt32`).
To enable or change a mesh's index data buffer after creation, use `set_index_data(mesh, buffer, type)`.
To remove a mesh's index data buffer, use `remove_index_data(mesh)`.
# Target
What OpenGL calls a "FrameBuffer Object" or "FBO". A collection of textures you can draw into instead of drawing into the screen.
For each color output, the fragment shader can write to one output variable. There can only be one depth, stencil, or depth-stencil texture. If you don't need the depth/stencil texture, you can omit it. If you only need depth/stencil for render operations and never for sampling, the `Target` can set up a limited `TargetBuffer` in place of a real `Texture`.
Targets can output to particular slices of a 3D texture or faces of a cubemap texture. The texture attachment can also be "layered", meaning ALL slices/faces are available and the geometry shader can emit different primitives to different layers as it pleases.
## Creation
There are several ways to construct a `Target`. Whenever you provide settings rather than an explicit texture, the `Target` will create a texture for you, and will remember to destroy it when the `Target` is destroyed.
Existing textures that you want to attach should be wrapped in the `TargetOutput` struct, which allows you to easily bind more exotic things to act like 2D attachments, such as slices of a 3D texture or faces of a cubemap texture. You can also bind an entire 3D texture or cubemap, and decide which slice/face to output to in the geometry shader. OpenGL refers to this as "layered" rendering.
* `Target(size::v2u, n_pretend_layes::Int)` creates an instance with no actual outputs, but which pretends to have the given number of outputs.
* `Target(color::Union{TargetOutput, Vector{TargetOutput}}, depth_stencil::TargetOutput)` creates an instance with zero or more color attachments, and the given depth/stencil attachment.
* `Target(depth_stencil::TargetOutput)` creates an instance with no color attachments, only a depth and/or stencil attachment.
* `Target(color::TargetOutput, depth_stencil::E_DepthStencilFormats, ds_no_sampling::Bool = true, ds_sampling_mode = DepthStencilSources.depth)` creates a target with one color output, and one depth/stencil output which by default is not sampleable (meaning, it's not a `Texture`). If you *do* mark it sampleable, the fourth parameter controls what can be sampled from it.
## Use
* `target_activate(target_or_nothing, reset_viewport=true, reset_scissor=true)`
* Sets all future rendering to go into the given target, or to the screen if `nothing` is passed.
* `target_clear(target, value, color_fragment_slot=1)` will clear one of the target's attachments.
* For color, pass a value of `vRGBAf` for float or normalized (u)int textures, `vRGBAu` for uint textures, and `vRGBAi` for int textures.
* When clearing color you must also specify the index. You can think of this as the color attachment index at first. However, if you call `target_configure_fragment_outputs()`, then note that the index here is really the fragment shader output index.
* For depth, pass any float value (`Float16` `Float32`, `Float64`).
* For stencil, pass a `UInt8`, or any other unsigned int which will get casted down.
* This operation is affected by the stencil write mask, and by default will try to catch unexpected behavior by throwing an error if any bits of the mask are turned off. This check can be disabled by passing `false`.
* For hybrid depth-stencil, pass a `Depth32fStencil8u`, *regardless* of the actual format used.
* `target_configure_fragment_outputs(target, slots)` routes each fragment shader output when rendering to this target, to one of the color attachments (or `nothing` to discard that output). For example, passing `[5, nothing, 1]` means that the fragment shader's first output goes to color attachment 5, its second output is discarded, its third output goes to color attachment 1, and any subsequent outputs are also discarded.
* When the `Target` is first created, all attachments are active and in order (as if you passed `[1, 2, 3, ...]`).
* When clearing a Target's color attachments, you provide an index in terms of these fragment shader outputs, *not* in terms of color attachment. For example, after passing `[5, nothing, 1]`, then clearing slot 1 actually means clearing attachment 5. | Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 5539 | # SceneTree
## Defining your memory layout
To use this module, you should first decide how you store your nodes. You can see examples of this in the unit test file *test/scene-tree.jl*. In particular, you need:
* A unique ID for each node. Call this data type `ID`.
* [Optional] a "context" that can retrieve the node for an ID. Call this type `TContext`. If you don't need a context to retrieve nodes, use `Nothing`.
* A custom node type which somehow owns an instance of the immutable struct `BplusTools.SceneTree.Node{ID, F}`. You should pick a specific `F` (and, of course, an `ID`). Call this node type `TNode`.
## Implementing the memory layout interface
Once you have an architecture in mind, you can integrate it with `SceneTree` by implementing the following interface with your types:
* `null_node_id(::Type{ID})::ID`: a special ID value representing 'null'.
* `is_null_id(id::ID)::Bool`: by default, compares with `null_node_id(ID)` using `===` (triple-equals, a.k.a. egality).
* `deref_node(id::ID[, context::TContext])::TNode`: gets the node with the given ID. Assume the ID is not null, and the node exists.
* `update_node(id::ID[, context::TContext], new_value::SceneTree.Node{ID})`: should replace your node's associated `SceneTree.Node` with the given new value.
* `on_rooted(id::ID[, context::TContext])` and/or `on_uprooted([same args])` to be notified when a node loses its parent (a.k.a. becomes a "root") or gains a parent (a.k.a. becomes "uprooted"). This is useful if you have a special way of storing root nodes separate from child nodes.
## Examples
### SceneTree as a flat list of nodes
A really simple and dumb memory layout would be to put each node in a list, assume nodes are never deleted, and reference each node using its index in the list. Let's also say the component type used is Float32. In this case, you can define the following architecture:
* `const MyID = Int`
* `const MyContext = Vector{SceneTree.Node{MyID, Float32}}`
* `null_node_id(::Type{MyID}) = 0`
* `deref_node(i::MyID, context::MyContext) = context[i]`
* `update_node(i::Int, context::Vector, value::SceneTree.Node) = (context[i] = value)`.
Now your list of nodes can participate in a scene graph!
NOTE: the use of `Int` and `Vector{SceneTree.Node}` in your custom SceneTree implementation is type piracy, and you should wrap one or both of them in a custom decorator type to avoid this.
### SceneTree as a group of garbage-collected nodes
Another way to layout memory for a scene tree would be to define a custom mutable struct, `MyEntity`, which has the field `node::SceneTree.Node{ID, Float32}`. Then `ID` is simply a reference to `MyEntity`, because mutable structs are reference types, and no context is needed! However, to handle null ID's, wrap the ID type in Julia's `Ref` type, which boxes it and allows for null values. So the final implementation looks like:
* `const MyID = Ref{MyEntity}`
* `deref_node(id::MyID) = id[].node`
* `update_node(id::MyID, data::SceneTree.Node) = (id[].node = data)`
* `null_node_id(::Type{MyID}) = MyID()`
* `is_null_id(id::MyID) = isassigned(id)`
NOTE: in practice, this is not easy to implement in Julia due to a circular reference. `MyID`'s definition references `MyEntity`, but `MyEntity` also has to reference `MyID` in its `node` field. This can be solved with an extra layer of indirection using type parameters; check out the unit tests to see how it's done.
## Usage
*Refer to function definitions for more info on the optional params within each function.*
Creating nodes and adding them to the scene graph is something you do yourself, since you chose the memory representation.
Once you have some nodes, you can parent them to each other with `set_parent(child::ID, parent::ID[, context]; ...)`. To unparent a node, pass a 'null' ID for the parent (the specific representation of 'null' depends on how you defined node ID's).
You can get the transform matrix of a node with `local_transform(id[, context])` or `world_transform(id[, context])`. The "world transform" means the transform in terms of the root of the tree.
Functions to *set* transform, or get individual position/rotation/scale values, are still to be implemented.
You can iterate over different parts of a tree:
* `siblings(id[, context], include_self=true)` gets an iterator over a node's siblings in order.
* `children(id[, context])` gets an iterator over a node's direct children (no grand-children).
* `parents(id[, context])` gets an iterator over a node's parent, grand-parent, etc. The last element will be a root node.
* `family(id[, context], include_self=true)` gets an iterator over the entire tree underneath a node. The iteration is Depth-First, and this is the most efficient iteration if you don't care about order.
* `family_breadth_first(id[, context], include_self=true)` gets a breadth-first iterator over the entire tree underneath a node. This implementation avoids any heap allocations, at the cost of some processing overhead. It's recommended for small trees.
* `family_breadth_first_deep(id[, context], include_self=true)` gets a breadth-first iterator over the entire tree underneath a node. This implementation makes heap allocations, but iterates more efficiently than the other breadth-first implementation. It's recommended for large trees.
Some other helper functions:
* `try_deref_node(id[, context])` gets a node, or `nothing` if the ID is null.
* `is_deep_child_of(parent_id, child_id[, context])` gets whether a node appears somewhere underneath another node.
| Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 19229 | Core utilities for B+.
## Asserts
You can do runtime checks with `@bp_check(condition, msg...)`. It throws an error if the condition is false, and provides the given message data (by converting each to `string()` and appending them together). You can also use `@bp_check_throws(statement, msg...)` in your tests to check that an expression throws.
To add asserts and a debug/release flag to your project, invoke `@make_toggleable_asserts(prefix)`. Invoking it adds a new compile-time flag, plus some helper macros. In B+ each sub-module has its own toggleable asserts; in your own program you probably just want one for the whole project.
For example, if you invoke `@make_toggleable_asserts(my_game_)`, then you'll get the following new definitions:
1. `@my_game_assert(condition, msg...)` is the debug-only version of `@bp_check`.
2. `@my_game_debug()` is a compile-time boolean constant for whether you're in debug mode.
3. `@my_game_debug(debug_code[, release_code])` runs some debug-only code, and alternatively some optional release-only code.
4. `my_game_asserts_enabled() = false` is the core function driving this feature. You can enable "debug mode" by explicitly redefining it, either within the module or after the module. For example, `MyGameModule.my_game_asserts_enabled() = true; MyGameModule.run_game()`.
* You can see an example of toggling debug flags in B+'s unit tests (*test/runtests.jl*).
* The way this works is that the implementation of `my_game_asserts_enabled()` is so simple that Julia will always inline it, causing the value (`true` or `false`) to propagate out and effectively remove debug statements from the codebase entirely when in release mode.
* Redefining the function forces Julia to recompile all functions which referenced it, causing debug code to suddenly appear.
**Important note**: constants do not get recompiled! For example, if you declare `const C = @my_game_debug(100, 1000)`, then toggle the debug flag, `C` will not change its value from 1000 to 100.
*Note*: `@make_toggleable_asserts` is a custom implementation of the *ToggleableAsserts.jl* package, which provides some of this functionality globally.
## Optional
* `Optional{T}` is an alias for `Union{T, Nothing}`.
* `exists(t)` is an alias for `!isnothing(t)`.
* `@optional(condition, outputs...)` helps you optionally pass parameters into a function call. It evaluates to `()...` if the condition is false, or `outputs...` if it is true.
* I'm guessing that the use of this macro is very type-unstable if the condition isn't known at compile-time, so don't use it in high-performance contexts unless you can check that it doesn't cause allocations/dynamic dispatch.
* `@optionalkw(condition, name, value)` is like `@optional` but for named parameters. It evalues to `NamedTuple()` if the condition is false, or `$name=$value` if the condition is true.
## Collections and Iterators
* `preallocated_vector(T, capacity::Int)` creates a vector with a specific initial capacity.
* `Base.append!` is now implemented for sets. This is type piracy, but it's also a very strange hole in Julia's API to not be able to append an iterator to a set.
* `UpTo{N, T}` is a type-stable, immutable container for 0 to N elements of type `T`. For example, calculating the intersection of a ray and sphere could return `UpTo{2, Float32}`.
* The type implements `AbstractVector{T}`, so it can be treated like a 1D array using all of Julia's built-in functions for array manipulation.
* `append(a::UpTo{N, T}, b::UpTo{M, T})` creates a new `UpTo{N+M, T}`.
* `SerializedUnion{U<:Union}` is a value that can be serialized with its type (using the ubiquitous *StructTypes* package for serialization, which also means it works with the *JSON3* package). The macro `@SerializedUnion(A, B, ...)` helps you specify the type more easily.
* For example, if you want to write and read a `Union{Int, String}` using *JSON3*, you can do it by reading and writing with the type `@SerializedUnion(Int, String)`.
* The order of priority for trying to parse the different types in a serialized union is controlled by the function `union_ordering(T)::Float64`. Lower values are tried first.
* `IterSome(lambda, T=Any)` creates an iterator that uses your lambda `idx::Int -> Union{Nothing, Some{T}}`, outputting each of your elements, until you return `nothing`. The `T` parameter helps the compiler in case it can't do type-inference with your lambda.
### Tuples
* `tuple_length(::Type{<:Tuple})::Int` gets the number of elements in a tuple type.
* For a tuple object you can use `length(t)`; this is for tuple *types*.
* `ConstVector{T, N}` is an alias for `NTuple{N, T}` which allows you to specify the element type without the count. For example, `ConstVector{Int64}` matches a tuple of all `Int64`, of any length.
### Functional programming
* `unzip(zipped)` takes the output of a `zip()` call (or something that looks like it) and splits them back out into the original iterators.
* If the input isn't from an actual call to `zip()`, then the unzipping will require iterating through the input several times (once for each output iterator).
* `reduce_some(f, predicate, iter; init=0)` works like the built-in `reduce()`, but also skips over elements that fail a certain predicate.
* `find_matching(element, collection, comparison=Base.:(==))` gets the index/key of the first element matching a desired one. Returns `nothing` if none matches. Optionally uses a comparison other than `==`.
* `iter(x)` provides a facade for iterators that don't work on functions like `map()`. For example, while you can't do `map(f, my_dictionary)`, you *can* do `map(f, iter(my_dictionary))`.
* `map_unordered(f, iters...)` uses `iter()` to run `map()` on unordered collections that don't normally support `map()`.
* `drop_last(iter)` removes the last element of an iteration.
* `iter_join(iter, delimiter)` inserts `delimiter` in-between elements `iter`, like `join()`.
## Enums
### Scoped enums
`@bp_enum(name, elements...)` is a more powerful version of Julia's `@enum`, with the same declaration syntax. It has the following improvements (assuming your enum is named `MyEnum`):
* The enum values are kept in their own scope, by turning `MyEnum` into a sub-module.
* The actual enum type inside the module is aliased to `E_MyEnum` outside the module, for convenience.
* The values can be parsed from a string (or `Val{S}`, where `S` is a `Symbol`) with `MyEnum.parse(s)`.
* The values can be converted from their integer value with `MyEnum.from(i)`, or from their index in the declaration order with `MyEnum.from_index(idx)`.
* The enum values can be converted to their integer index with `MyEnum.to_index(e)`.
* A tuple of all enum values can be gotten with `MyEnum.instances()`.
### Scoped bitflags
`@bp_bitflag` is for enums that reprsent bit-flags. Along with the above features of `@bp_enum`, `@bp_bitflag` adds the following (assuming your enum is named `MyBitflag`):
* Default values of elements increase by powers of two -- the first element is `1`, second is `2`, third is `4`, fourth is `8`, etc.
* To define a "none" element followed by default numbering, put it at the beginning. For example, `@bp_bitflag MyBitflag z=0 a b c`.
* For the full numbering rules, refer to the *BitFlags.jl* package which powers this macro.
* Combine two bitflags with `a | b`.
* Intersect two bitflags with `a & b`.
* Remove bitflags with `a - b` (equivalent to `a & (~b)`).
* Check for subsets with `a <= b` ("a is a subset of b?") and supersets with `a >= b` ("a is a superset of b?").
* `Base.contains` can be used to check if one bitflag is totally contained within another: `Base.contains(haystack::E_MyBitflag, needle::E_MyBitflag))`.
* The special value `MyBitflag.ALL` contains all the bitfield elements combined together. Equivalent to `reduce(|, MyBitflag.instances())`.
* Special aggregate values are defined with the `@` symbol. These do not show up in `instances()`, `to_index()`, or `from_index()`. For example:
````
@bp_bitflag(MyFlags,
a, b, c, d, e,
# Aggregates:
@ab(a|b), # Declares 'ab'
@abc(ab|c) # Declares 'abc'
@ace((abc - b) | e) # Declares 'ace'
)
````
*Note*: `@bp_bitflag` is meant to be a replacement for `@bitflag`, from the *BitFlags* package. `@bitflag` inherits a lot of the same problems as `@enum`, and also misses some big convenience features.
### Anonymous enums
If you want small-scale enums, for function parameters, you can use `@ano_enum(A, B, ...)` and `@ano_value(A)`. For example:
````
function do_stuff(i::Int, mode::@ano_enum(Mode1, Mode2, Mode3))
if mode isa @ano_enum(Mode1, Mode2)
i = -i
end
i *= 2
if mode isa @ano_enum(Mode1, Mode3)
i *= 10
end
return i
end
do_stuff(i, @ano_value(Mode2))
````
## Numbers
* `@f32(n)` is short-hand to cast a value to `Float32`.
* `Scalar8`, `Scalar16`, `Scalar32`, `Scalar64`, and `Scalar128` are aliases for built-in number types of the given bit sizes.
* Note that `Scalar8` does not include `Bool`.
* For example, `Scalar32` is `Union{UInt32, Int32, Float32}`.
* `ScalarBits` is a union of all the scalar types mentioned above.
* `type_str(::Type{<:Scalar})` gets a short-hand for a number or boolean type.
* For example, `type_str(UInt8)` returns `"u8"`.
* `Binary(x)` is a decorator for numbers that prints them as their binary representation.
* You can support this decorator for your own type by implementing the interface described in `Binary`'s doc-string.
## Random
* `rand_ntuple(rng, t::NTuple)` picks a random element from an `NTuple`.
* `RandIterator(n, rng)` efficiently iterates through `1:N` in a random order.
`PRNG` is a custom RNG struct which implements Julia's `AbstractRNG` interface. This means you can use it like any of the built-in RNG types. However, this one is designed for speed and quick spin-up time, which is important for procedural generation purposes.
You can construct it by passing 0 or more numbers to use as seeds. You can also construct it with an initial state (a `UInt32` followed by a `NTuple{3, UInt32}`).
### Mutable version
Like all RNG's offered by Julia, `PRNG` is mutable, which also means it's pass-by-reference and probably heap-allocated. This is OK for RNG's which are created once and last for a while.
### Immutable version
In some contexts, you want to quickly create and destroy thousands or millions of RNG's. For example, generating a random texture usually involves creating one RNG per pixel, using the pixel coordinates as the seed, and only generating a handful of numbers with each one.
For this use-case, use `ConstPRNG`. It is the same RNG as an immutable struct, which in Julia also means it's pass-by-value and not usually heap-allocated.
`ConstPRNG` implements many of the same functions as `PRNG`. However, its return type is different. It always returns a tuple of the usual random output and the next state of the RNG. Here is an example of how you can use it:
````
function generate_pixel(x, y)
rng = ConstPRNG(x, y)
(red, rng) = rand(rng, Float32)
(green, rng) = rand(rng, Float32)
(blue, rng) = rand(rng, Float32)
return (red, green, blue)
````
## Macros
* `@do_while code condition` implements a do-while loop. The syntax can pretty closely match do-while loops in C-like languages, for example:
````
i::Int = 0
@do_while begin
println(i)
i += 1
end i<10
````
* `@decentralized_module_init` allows you to add runtime initialization code in multiple different places within a single module. After invoking it, you make use of it by statically adding lambdas to the global list `RUN_ON_INIT`. All these lambdas will execute once at the start of each program run (or more precisely, the first time this module is loaded with `using` or `import`). You can find examples of this in the B+ codebase.
* This is valuable if you have a lot of disparate data to be computed at the beginning of each program run, rather than the more common `const` globals which are computed at compile-time. For example, a pointer to some C function or allocated memory will be different every time you run the program.
* You cannot add lambdas outside the module, or within a function running at run-time. If you try, that function will be ignored since the module has already finished initializing by the point your code is reached.
* Note the distinction between compile-time and run-time here: you are registering the lambdas with `RUN_ON_INIT` at compile-time, so that they can be later executed at run-time. The blurry line between write-time, compile-time, and run-time is key to Julia's expressive power, but it's tricky to get your head around at first.
* You shouldn't add lambdas within a submodule either, although I belive it works. You should just define `__init__()` or `@decentralized_module_init` for that sub-module.
* This macro is implemented by **a)** declaring the list `RUN_ON_INIT`, and **b)** implementing the special Julia function `__init__()` to call every lambda in the list.
* The order of lambda execution is always the order they were added to the list, a.k.a. the order of your `push!` calls.
## Functions
* `reinterpret_bytes(input, output)` converts between different bitstype data.
* Allowed inputs, for some bitstype `T`:
* An instance of a `T`
* `AbstractArray{T}` (if you want to read a subset, use `@view`)
* `Ref{T}`, pointing to one value
* `Tuple{Ref{T}, Integer}`, pointing to some number of `T` values
* `Tuple{Ptr{T}, Integer}`, pointing to some number of `T` values
* Allowed outputs:
* `AbstractArray{T}` for some bitstype `T`
* `Ref{T}` for some bitstype `T`
* `Ptr{T}` for some bitstype `T`
* The type `T` itself, causing the function to return the reinterpreted `T` rather than write it somewhere.
* `val_type(::Val{T})` and `val_type(::Type{Val{T}})` return `T`. For example, `val_type(Val(:hi))` returns `:hi`.
* For reference, `Val{T}` is a built-in Julia type that turns some bits data into a type parameter. It's an important part of building complex zero-cost abstractions.
## Interop
* `InteropString` juggles a string across two representations: a Julia `String` and a C-style, null-terminated `Vector{UInt8}`.
* Create a new instance with `i_str = InteropString(s::String[, buffer_capacity_multiplier])`.
* Get the Julia string's current value with `string(i_str)` (the same `string` function defined in `Base` and available everywhere).
* Set the Julia string with `update!(i_str, new_value::String)`.
* Note that `update!()` is also declared in the package *DataStructures*, so if you use that module you have to put the module name in front of each `update!` call, or pick the default one with an alias `const update! = BplusCore.Utilities.update!`.
* If the C version of the string was modified, regenerate the Julia string with `update!(i_str)`.
## Metaprogramming
### Unions
* `@unionspec(T{_}, A, B, C)` turns into `Union{T{A}, T{B}, T{C}}`
* You can get the same result with a generator expression, `Union{(T{t} for t in (A, B, C))...}`,
but it's a bit more verbose.
* `union_types(U)` calculates a tuple of the individual types inside a union.
* If you pass a single type, then you get a 1-tuple of that type.
### Expression manipulation
Much of this section requires some familiarity with Julia macros and metaprogramming, a.k.a. code that generates code. It's also heavily inspired by the *MacroTools* package. also builds off of the *MacroTools* package.
* `visit_exprs(to_do, root)` visits every expression, like `MacroTools.prewalk` but without modifying the expression and with more info that helps to pinpoint the location of each sub-expression.
* `FunctionMetadata(expr)` strips out top-level information from a function definition. In particular it checks for doc-strings, `@inline`, and `@generated`. For example, `FunctionMetadata(quote "Multiplies x by 5" @inline f(x) = x*5 end)` creates an instance of `FunctionMetadata("Multiplies x by 5", true, false, :( f(x) = x*5 ))`.
* If you pass some invalid syntax into `FunctionMetadata(expr)`, it outputs `nothing` rather than throwing an error. This lets you more easily check whether an expression is a valid function definition or not. However it doesn't check the core expression (e.x. `f(x) = x*5`); only the things surrounding it.
* You can turn an instance back into the original expression with `MacroTools.combinedef(fm::FunctionMetadata)::Expr`.
* `is_scopable_name(expr)::Bool` checks for a Symbol, possibly prefixed by one or more dot operators (e.x. `:( Base.iszero )`), and optionally allowing type parameters at the end (e.x. `:( A.B{C} )`).
* `is_function_call(expr)::Bool` checks for a function call/signature, like `:( MyGame.run(i::Int; j=5) )`.
* `is_function_decl(expr)::Bool` checks for a function definition, like `:( f() = 5 )`.
* `expr_deepcopy()` works like `deepcopy()`, but without copying certain things that may show up in an AST and which aren't supposed to be copied. For example, literal references to modules.
#### Expression Splitting
There are several data representations of important syntax structures. This is analogous to `MacroTools.splitdef()` and `MacroTools.splitarg()`, but deeper and broader.
All the below structures inherit from `AbstractSplitExpr`. They are created by constructing them with an expression (or another instance to be deep-copied with `expr_deepcopy()`). They can be turned back into the original AST with `combine_expr(s)`. Most of them can detect when the expression they're analyzing is wrapped in an `esc()` call.
For more precise info on each field's meaning and type, refer to comments in the definition.
* `SplitArg(expr)` gets all data about a function argument declaration, such as `:( i::Int=3 )`.
* `SplitType(expr)` gets all data about a type declaration, such as `:( A{X} <: B )`.
* By default the constructor will do deep error-checking to validate the individual elements of the expression (e.x. making sure the name is a Symbol), but you can disable this by passing a second parameter into the constructor, `false`.
* `SplitDef(expr)` gets all data about a function declaration (e.x. `quote "Returns 0." @inline f() = 0 end`).
* You can make it a function call/signature (e.x. `:( f(i::Int; j=5) )`) by setting the body to `nothing` (not to be confused with the expression `:nothing`, as in `:( f() = nothing )`).
* You can make it a lambda (e.x. `:( i -> i*2 )`) by setting the name to `nothing` (not to be confused with the expression `:nothing`).
* It is an error to set both the body and the name to `nothing`.
* `SplitMacro` gets all data about a macro invocation, such as `:( @m a b+c )`.
#### Assignment operators
* `ASSIGNMENT_INNER_OP` maps modifying assignment operators to the plain operator. For example, `ASSIGNMENT_INNER_OP[:+=]` maps to `:+`.
* `ASSIGNMENT_WITH_OP` is a map in the opposite direction: `ASSIGNMENT_WITH_OP[:+]` maps to `:+=`.
* `compute_op(s::Symbol, a, b)` performs one of the modifying assignments (e.x. `+=`) on `a` and `b`. For example, `compute_op(:+=, a, b)` computes `a + b`. | Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.1 | 7c7f44c209c1d68ce818bd280a43458e9b9ba953 | docs | 13775 | Part of the [`Math` module](Math.md). The core game math types are: `Vec{N, T}`, [`Quaternion{F}`](Quat.md), and [`Mat{C, R, F}`](Matrix.md).
# `Vec{N, T}`
An `N`-dimensional vector of `T`-type components. Unlike Julia's built-in `Vector`, this is static, immutable, and most importantly allocatable on the stack.
Under the hood, it holds a tuple `data::NTuple{N, T}`.
Obviously there are some similarities between this type and a static vector (see the *StaticArrays* package),
but if `Vec` were simply an alias for `SVector` then I would be committing a **lot** of type piracy,
so the use of a custom struct is warranted.
## Aliases
### By dimension
* `Vec2{T}`
* `Vec3{T}`
* `Vec4{T}`
### By component type
* `VecF{N}` : `Float32`
* `VecD{N}` : `Float64`
* `VecI{N}` : `Int32`
* `VecU{N}` : `UInt32`
* `VecB{N}` : `Bool`
* `VecT{T, N}` allows you to specify a vector's component type before its dimension. For example, `VecT{Float32} == VecF`.
### By dimension *and* component type
* Float32:
* `v2f`
* `v3f`
* `v4f`
* Float64:
* `v2d`
* `v3d`
* `v4d`
* Int32:
* `v2i`
* `v3i`
* `v4i`
* UInt32:
* `v2u`
* `v3u`
* `v4u`
* Bool:
* `v2b`
* `v3b`
* `v4b`
### By intent
* `vRGB{T} = Vec3{T}`, for 3-component data
* `vRGBu8` for UInt8 channels
* `vRGBi8` for Int8 channels
* `vRGBu` for UInt32 channels
* `vRGBi` for Int32 channels
* `vRGBf` for Float32 channels
* `vRGBA{T} = Vec4{T}`, for 4-component data
* `vRGBAu8` for UInt8 channels
* `vRGBAi8` for Int8 channels
* `vRGBAu` for UInt32 channels
* `vRGBAi` for Int32 channels
* `vRGBAf` for Float32 channels
## Construction
You can construct a vector in the following ways. Julia's type system is a bit tricky, so if you're having problems then come back to this cheat-sheet.
* `Vec{N, T}()` constructs an instance of specific size and component type, using all 0's. For example, `v3f()`.
* `Vec(components...)` pass in the individual components, which are all promoted to the same type. For example, `Vec(1.5, 2)` creates a `v2d`.
* `Vec{T}(components...)` casts all elements to `T`.
* `Vec{N, T}(components...)` casts all elements to `T` and throws an error if the wrong number of components is passed. For example, `v3f(3, 4, 5)` produces a `v3f` instead of a `Vec{3, Int64}`.
* `Vec(f::Callable, n::Int)` creates a vector of size `n` using the given lambda to map each component (1 through `n`) to a value. For example, `Vec(i->i*2, 3)` is equivalent to `Vec(2, 4, 6)`.
* `Vec(f::Callable, ::Val{N})` uses a compile-time constant for the size. For example, `Vec(i->i*2, Val(3))`. Note that this *doesn't* speed things up unless `N` is actually a compile-time constant.
* `Vec{N, T}(f::Callable)` infers the dimensionality and casts the components to `T`. For example, `v3f(i -> i*2)` makes a `v3f(2, 4, 6)`.
* `Vec(N, ::Val{F})` : constructs a vector with `N` components, of type `typeof(F)`, all set to the value `F`. For example, `Vec(4, Val(0.5))` is equivalent to `Vec4(0.5, 0.5, 0.5, 0.5)`.
* `Vec{N}(::Val{F})` is a variant that takes `N` as a type parameter. For example, `Vec4(::Val(0.5))`.
* `Vec{N, T}(::Val{F})` is a variant that also casts `F` to type `T`. For example, `v2f(Val(0.5))` makes a `v2f` instead of a `v2d`.
* `Vec(data::NTuple)` : directly pass in the ntuple of data.
* `Vec{T}(data::NTuple)` is a variant that will cast each element of the tuple to `T`.
* `Vec{N, T}(data::NTuple)` is a variant that will cast each element, and throw an error if the tuple has the wrong number of elements.
* `Vec{T}()` and `Vec{N, T}()` : constructs a 0-D vector of component type `T`.
* Another variant `Vec{T}(::Tuple{})` and `Vec{N, T}(::Tuple{})` is also provided.
* These constructors are useful for recursive functions.
`vappend()` lets you combine vectors and scalars into bigger vectors. For example, `vappend(Vec(1, 2), 3, Vec(4, 5, 6)) == Vec(1, 2, 3, 4, 5, 6)`.
`Vec` is serializable like an array, so it supports the *StructTypes* package and therefore packages like *JSON3*.
## Components/Swizzling
You can get a tuple of a vector's components by accessing its `data` field.
You can access the individual components of a vector in several ways:
* Naming its `x`, `y`, `z`, or `w` component, also named `r`, `g`, `b`, and `a`. For example, `Vec(1, 2, 3).x == 1`.
* Combining components in any order to "swizzle" a new vector. For example, `Vec(55, 66, 77).xxzy == Vec(55, 55, 77, 66)`.
* Getting a single component using its index (1-based, like everything else in Julia). For example, `v[2] == v.y`.
* Accessing a tuple of component indices. For example, `v[1, 1, 3, 2] == v.xxzy`.
Swizzling can also use some special characters to insert certain constant values:
* `0` gets the value 0. For example, `v2f(3, 10).x0y` turns a 2D vector into 3D one by inserting a Y value of 0.
* `1` gets the value 1. For example, `v3f(0.2, 0.8, 1.0).rgb1` appends an alpha of 1 to an RGB color.
* `Δ` (typed as '\Delta' followed by a Tab) gets the largest finite value for the component type. For example, `vRGBu8(20, 200, 63).rgbΔ` Adds an alpha of 255 to an RGB color.
* `∇` (typed as '\del' followed by a Tab) gets the smallest finite value for the component type. For example, `Vec2{Int8}(11, -23).xy∇` appends `-128` to the vector.
You can get a range of components by feeding a range into the vector, like `v[2:4]`, **but** it will be type-unstable as the size isn't known at compile time. If the range is a constant, you can feed it in as a `Val` to get a type-stable result: `v[Val(2:4)]`.
## Math
`Vec` implements many standard Julia functions for numbers:
* `zero()` and `one()`
* `typemin()` and `typemax()`
* `min()`, `max()`, and `minmax()`
* `abs()`, `round()`, `clamp()`, `floor()`, `ceil()`
It implements all the standard math operators (including bitwise ops and comparisons), component-wise with two exceptions: `==` and `!=` are done for the whole `Vec`, producing a single boolean.
* For component-wise equality use `map`, for example `map(==, v1, v2)`.
Vector math is implemented with functions prefixed by `v`:
* `vdot(a, b)` is the dot product.
* You can also use the operator `⋅` (typed as '\cdot' followed by a Tab): `a ⋅ b`
* `vcross(a, b)` is the cross product (only defined for `Vec3`).
* You can also use the operator `×` (typed as '\times' followed by a Tab): `a × b`
* Calculate distances/lengths with `vdist_sqr(a, b)`, `vdist(a, b)`, `vlength_sqr(a, b)`, and `vlength(a, b)`.
* `vnorm(v)`, `v_is_normalized(v)` for normalization
* `vreflect(v, normal)` and `vrefract(v, normal, IoR)`
* `vbasis(forward, up)` gets three orthogonal vectors, where the forward vector is exactly equal to `forward` and the up vector is as close as possible to `up`.
Vectors also interact nicely with Julia's multidimensional arrays:
* As an `AbstractVector`, `Vec` supports many standard array-processing functions (see [below](#Array-Like-Behavior)).
* Note that `Vector` is Julia's term for a 1D array; don't get them confused.
* You can get an element of a multidimensional array at an integer-vector index, with `arr[vec]`.
* Unfortunately, the indexing order of Julia arrays is reversed, so in a 2D array, a `Vec` index's X coordinate is the row, and Y is the column.
* To get the more intuitive indexing order, for example when working with arrays of pixels, you can `reverse()` the vector or wrap the index with `TrueOrdering` like so: `a[TrueOrdering(v)] == a[reverse(v)] == a[v.z, v.y, v.x]`.
* You can get the size of a multidimensional array as a vector, with `vsize(arr, I=Int32)`.
* As mentioned above, the indexing order of Julia arrays is reversed, so for example the `vsize` of a 2D array gives you the row count in the X component and the column count in the Y component.
* Pass `true_order=true` to swap the output's elements and get the more intuitive ordering, useful when working with pixel grids
Some other general utilities:
* `vselect(a, b, t)` lets you pick values component-wise using a `VecB`, sort of like a binary `lerp()`.
* Note that you can also use `lerp()` for this, although it may be a tiny bit less efficient.
* `vindex(p, size)` to convert a multidimensional coordinate into a flat index, for an array of some multidimensional size.
`vindex(i::Int, size)` can convert in the opposite direction -- a flat index to a multidimensional one.
* `Base.convert()` can convert the components of a `Vec`, for example `convert(v2f, Vec(3, 4))`. It can also convert a `StaticArrays.SVector` to a `Vec`.
* `Base.reinterpret()` can reinterpret the bits of a `Vec`'s components, for example `reinterpret(v2f, v2u(0xabcdef01, 0x12345678))`.
## Setfield
Because vectors are value-types, they are immutable. The only way to "modify" a vector is to replace it with a copy. Fortunately, Julia has *Setfield*, a built-in package which helps you do this. While it technically does lots of copying, use of *Setfield* will almost always optimize down to something equivalent to mutating your desired field.
You can use Setfield to "modify" a vector variable with the syntax `@set! v.x += 10`, or create a mutated copy with the expression `v2 = @set v1.x += 10`. **However**, it's important to note that if you try to `@set` a vector that belongs to something else, like `@set! my_class.v.x += 10`, *Setfield* will try to make a copy of that entire owning object (`my_class`, not just `my_class.v`).
## Coordinate System
B+ allows you to define the engine-wide up axis and handedness of vector math by redefining `BplusCore.Math.get_up_axis() = 2` (the default is `3` for the Z axis) and `BplusCore.Math.get_right_handed() = false` (the default is `true`) within your own project. For more info on how this works, see [How it works](#How-it-works) below.
An example of when this is useful is if you are primarily developing your project within `Dear ImGUI`'s 2D graphics system rather than 3D OpenGL. Dear ImGUI is left-handed, and arguably Y-up.
There are several built-in functions that respect this configuration:
* `v_rightward(forward, up)` does a cross-product based on the current handed-ness. In right-handed systems, it does `vcross(forward, up)`. In left-handed systems, it swaps them.
* `get_up_vector(F = Float32)` gets the 3D up vector. For example, if the up axis is `3`, then it returns `Vec3{F}(0, 0, 1)`.
* `get_horz_vector(i, F = Float32)` gets the first or second 3D horizontal vector, based on whether you pass in `1` or `2`.
* `get_horz_axes()` gets a tuple of the horizontal indices. For example, if the up-axis is `3` then it returns `(1, 2)`.
* `to_3d(v, f=0)` inserts a vertical component to a 2D horizontal vector.
* `get_vert(v)` grabs the vertical component of a 3D vector.
### How it works
Julia's blurry line between compile-time and run-time allows you to set up compile-time constants directly in the language. You can define a trivial function like `my_const() = 5`, which Julia will easily inline, and refer to `my_const()` like any other compile-time constant. Then specific projects can redefine it, e.x. `using ThePackage; ThePackage.my_const() = 10`. This forces Julia to recompile any functions that referenced `my_const()`. **However**, `const` global variables are never recalculated, so you can't do `const C = my_const()` and expect `C` to change when `my_const()` changes. Consts should never reference functions that are intended to be redefinable like this.
## Array-like behavior
`Vec{N, T}` implements `AbstractVector{T, 1}`, meaning Julia sees it as a kind of 1D array, and that lets you use all sorts of built-in array-processing functionality with it.
However, most built-in array-processing functions return an actual array (`Vector{T}`) as output, which is not ideal for vector math. We re-implement many of them to return a `Vec`. For example, calling `map()` on a `Vec` will return another `Vec`.
Unfortunately, one awesome Julia feature that `Vec` doesn't support well is broadcasting. Broadcasting operators will treat `Vec` as a 1D array, which is good, but the output will be an actual array (`Vector`), which is bad. This can hopefully be improved in the future.
An interesting note about Julia's multidimensional array literals: normally they would notice that `Vec` is an `AbstractVector` and treat it as an extra dimension. For example, a literal matrix of `v3f` would become a 3D array of Float32, with 3 Z-slices. However, the relevant functions (`hcat`, `vcat`, and `hvncat`) are overloaded to prevent this, so that a matrix literal of `Vec` stays a matrix of `Vec`.
## Ranges
Julia lets you specify number ranges with the colon syntax `begin : end`, for example `all_one_digit_numbers = 0:9`.
You can do the same with `Vec` to create multidimensional ranges.
For example, you can get every index of a 2D array `a` with the range `Vec(1, 1):Vec(size(a, 1), size(a, 2))`, or more succinctly `one(v2i):vsize(a)`. You can generalze it to work with any number of array dimensions by mixing numbers and vectors: `1:vsize(a)`.
You can add a step interval other than 1 using Julia's usual stepped range syntax `begin:step:end`, and again you can mix numbers and vectors. For example, you can skip every other column of a 2D array `a` with `1 : v2i(1, 2) : vsize(a)`.
This feature is mostly intended for integer vectors. While nothing stops you from making ranges with float vectors, it's highly recommended to use [`Box` and `Interval` instead](Math.md#Box-and-Interval).
## Printing
The number of digits that a vector is printed with is controlled by the global variable `BplusCore.Mat.VEC_N_DIGITS`. If you want to change this value only temporarily, call `use_vec_digits()`. If you want to print a single vector with a specific number of digits, use `show_vec`. | Bplus | https://github.com/heyx3/Bplus.jl.git |
|
[
"MIT"
]
| 0.2.0 | d57e066337ead58265044890809d1a1e9753d949 | code | 300 | using Documenter, Dimensionless
makedocs(sitename="Dimensionless.jl",
pages=["Home" => "index.md",
"example.md",
"library.md"
],
format=Documenter.HTML(prettyurls=get(ENV, "CI", nothing) == "true")
)
deploydocs(
repo="github.com/martinkosch/Dimensionless.jl.git",
)
| Dimensionless | https://github.com/martinkosch/Dimensionless.jl.git |
|
[
"MIT"
]
| 0.2.0 | d57e066337ead58265044890809d1a1e9753d949 | code | 309 | module Dimensionless
using Unitful
using LinearAlgebra
export DimBasis
export dim_matrix
export num_of_dims
export num_of_dimless
export fac_dimful
export dimless
export dimful
export change_basis
export print_dimless
include("dim_basis.jl")
include("dim_basis_ops.jl")
include("utils.jl")
end # module
| Dimensionless | https://github.com/martinkosch/Dimensionless.jl.git |
|
[
"MIT"
]
| 0.2.0 | d57e066337ead58265044890809d1a1e9753d949 | code | 3904 | # Helper Unions
QuantityOrUnits = Union{Unitful.AbstractQuantity,Unitful.Units}
QuantityOrUnitlike = Union{Unitful.AbstractQuantity,Unitful.Unitlike}
"""
DimBasis(basis_vectors...) -> DimBasis
Create a dimensional basis for a number of `basis_vectors` (quantities, units or dimensions).
A string identifier can optionally be added to each basis vector.
"""
struct DimBasis{T,N}
basis_vectors::T
basis_vector_names::N
basis_dims
dim_mat
function DimBasis(basis_vectors::T, basis_vector_names::N=nothing) where {
T<:AbstractVector{<:QuantityOrUnitlike}} where {
N<:Union{Nothing,AbstractVector{<:AbstractString}}}
basis_dims = unique_dims(basis_vectors...)
dim_mat = dim_matrix(basis_dims, basis_vectors...)
check_basis(dim_mat)
return new{T,N}(basis_vectors, basis_vector_names, basis_dims, dim_mat)
end
end
DimBasis(basis_vectors::Vararg{QuantityOrUnitlike}) =
DimBasis([basis_vectors...])
function DimBasis(basis_vectors::Vararg{Pair{<:AbstractString,<:QuantityOrUnitlike}})
to_quantity = any([isa(bv.second, Unitful.AbstractQuantity) for bv in basis_vectors])
new_basis_vectors = [to_quantity ? 1 * bv.second : bv.second for bv in basis_vectors]
new_basis_vector_names = [bv.first for bv in basis_vectors]
return DimBasis(new_basis_vectors, new_basis_vector_names)
end
# Do not broadcast DimBasis
Base.Broadcast.broadcastable(basis::DimBasis) = Ref(basis)
# Helper Types
QuantityDimBasis = DimBasis{<:AbstractVector{<:Unitful.AbstractQuantity}}
NamedDimBase = DimBasis{T,<:AbstractVector{<:AbstractString}} where {T}
"""
unique_dims(all_values...)
Return a vector of unique dimensions for `all_values`, a set of quantities, units or dimensions.
"""
function unique_dims(all_values::Vararg{Unitful.Dimensions})
basis_dims = Vector{Type{<:Unitful.Dimension}}()
for dims in all_values
union!(basis_dims, typeof.(typeof(dims).parameters[1]))
end
return basis_dims
end
unique_dims(all_values::Vararg{QuantityOrUnits}) =
unique_dims(dimension.(all_values)...)
"""
dim_matrix(basis_dims, all_values...)
Return the dimensional matrix for a set of basis dimensions `basis_dims` and `all_values`, a set of quantities, units or dimensions.
"""
function dim_matrix(basis_dims::Array{Type{<:Unitful.Dimension}}, all_values::Vararg{Unitful.Dimensions})
dim_mat = zeros(Rational, length(basis_dims), length(all_values))
for (dimension_ind, dims) in enumerate(all_values)
for dimension in typeof(dims).parameters[1]
basis_dim_ind = findfirst(x -> isa(dimension, x), basis_dims)
if isnothing(basis_dim_ind)
error("$(typeof(dimension)) is no basis dimension.")
end
dim_mat[basis_dim_ind, dimension_ind] = dimension.power
end
end
return dim_mat
end
dim_matrix(basis_dims::Array{Type{<:Unitful.Dimension}}, all_values::Vararg{QuantityOrUnits}) =
dim_matrix(basis_dims, dimension.(all_values)...)
"""
check_basis(dim_mat)
Use a dimensional matrix to check if a collection of dimensional vectors is a valid basis.
Throw errors if there are to few basis vectors or if the matrix does not have full rank.
"""
function check_basis(dim_mat)
if size(dim_mat, 2) < size(dim_mat, 1)
plr_sgl = (size(dim_mat, 2) == 1) ? "vector" : "vectors"
error("Invalid basis: There are $(size(dim_mat, 1)) dimensions but only $(size(dim_mat, 2)) basis $(plr_sgl).")
end
mat_rank = LinearAlgebra.rank(dim_mat)
if mat_rank < size(dim_mat, 2)
if mat_rank == 1
error("Invalid basis: There are $(size(dim_mat, 2)) basis vectors that are all linearly dependent.")
else
error("Invalid basis: There are $(size(dim_mat, 2)) basis vectors of which only $(mat_rank) are linearly independent.")
end
end
nothing
end
| Dimensionless | https://github.com/martinkosch/Dimensionless.jl.git |
|
[
"MIT"
]
| 0.2.0 | d57e066337ead58265044890809d1a1e9753d949 | code | 2939 | """
num_of_dims(all_vars...)
Return the number of unique dimensions for the given variables `all_vars`.
"""
function num_of_dims(all_vars::Vararg{Unitful.Dimensions})
return length(unique_dims(all_vars...))
end
num_of_dims(all_vars::Vararg{Pair{<:AbstractString,<:QuantityOrUnitlike}}) =
num_of_dims([var.second for var in all_vars]...)
num_of_dims(all_vars::Vararg{QuantityOrUnits}) =
num_of_dims(dimension.(all_vars)...)
"""
num_of_dimless(all_vars...)
Return the number of dimensionless numbers that can be constructed for a problem characterized by the variables `all_vars`.
"""
function num_of_dimless(all_vars::Vararg{QuantityOrUnitlike})
return length(all_vars) - num_of_dims(all_vars...)
end
num_of_dimless(all_vars::Vararg{Pair{<:AbstractString,<:QuantityOrUnitlike}}) =
num_of_dimless([var.second for var in all_vars]...)
"""
fac_dimful(unit, basis)
Return the scalar, dimensionless factor that a dimensionless value has to be multiplied with in order to translate it into the given `unit` in the specified `basis`.
"""
function fac_dimful(unit::Unitful.Units, basis::QuantityDimBasis)
dim_vec = dim_matrix(basis.basis_dims, dimension(unit))
fac = prod(basis.basis_vectors .^ (basis.dim_mat \ dim_vec))
return ustrip(uconvert(unit, fac))
end
"""
dimless(quantity, basis)
Make a `quantity` dimensionless using a dimensional `basis`.
"""
function dimless(quantity::Unitful.AbstractQuantity, basis::QuantityDimBasis)
fac = fac_dimful(unit(quantity), basis)
return ustrip(quantity) / fac
end
"""
dimful(value, unit, basis)
Restore the `unit`s of a dimensionless `value` using a dimensional `basis`.
"""
function dimful(value, unit::Unitful.Units, basis::QuantityDimBasis)
fac = fac_dimful(unit, basis)
return value * fac * unit
end
"""
current_to_new_fac(dims, basis, new_basis)
Return the factor that is needed to transform specified dimensions `dims` from a current `basis` to a `new_basis`.
"""
function current_to_new_fac(dims::Unitful.Dimensions, basis::QuantityDimBasis, new_basis::QuantityDimBasis)
dim_mat = dim_matrix(basis.basis_dims, dims)
new_dim_mat = dim_matrix(new_basis.basis_dims, dims)
basis_fac = prod(basis.basis_vectors .^ (basis.dim_mat \ dim_mat))
new_basis_multipler = prod(new_basis.basis_vectors .^ (new_basis.dim_mat \ new_dim_mat))
current_to_new_fac = new_basis_multipler / basis_fac
return ustrip(uconvert(Unitful.NoUnits, current_to_new_fac))
end
"""
change_basis(var, basis, new_basis)
Transform the specified quantity or unit `var` from a current `basis` to a `new_basis`.
"""
change_basis(var::Unitful.AbstractQuantity, basis::QuantityDimBasis, new_basis::QuantityDimBasis) =
var * current_to_new_fac(dimension(var), basis, new_basis)
change_basis(var::Unitful.Units, basis::QuantityDimBasis, new_basis::QuantityDimBasis) =
current_to_new_fac(dimension(var), basis, new_basis)
| Dimensionless | https://github.com/martinkosch/Dimensionless.jl.git |
|
[
"MIT"
]
| 0.2.0 | d57e066337ead58265044890809d1a1e9753d949 | code | 1610 | NamedDimVector = Pair{<:AbstractString,<:QuantityOrUnitlike}
"""
print_dimless([io, ]named_dim_vector, basis)
Print the dimensionless number that can be constructed using `named_dim_vector` in the specified dimensional `basis`.
"""
function print_dimless(io::IO, named_dim_vector::NamedDimVector, basis::NamedDimBase)
powers = -1 * (basis.dim_mat\dim_matrix(basis.basis_dims, named_dim_vector.second))[:]
perm = exps_perm(powers)
showoperators = get(io, :showoperators, false)
sep = showoperators ? "*" : " "
showrep(io, named_dim_vector.first, 1 // 1)
for i in perm
if powers[i] != 0
print(io, sep)
showrep(io, basis.basis_vector_names[i], powers[i])
end
end
nothing
end
print_dimless(named_dim_vector::NamedDimVector, basis::NamedDimBase) =
print_dimless(stdout, named_dim_vector, basis)
"""
showrep(io, identifier, exponent)
Print the `identifier` followed by the corresponding `exponent` to the specified stream `io`.
"""
function showrep(io::IO, identifier::String, exp::Rational)
print(io, identifier)
if exp != 1
exp_str = exp.den == 1 ? "^" * string(exp.num) : "^" * replace(string(exp), "//" => "/")
print(io, exp_str)
end
nothing
end
"""
exps_perm(powers)
Return the permutation of exponents so that large positive values are showed first followed by large negative values.
"""
function exps_perm(powers)
perm = sortperm(powers; rev=true)
first_negative = count(powers .> 0) + 1
perm[first_negative:end] .= reverse(perm[first_negative:end])
return perm
end
| Dimensionless | https://github.com/martinkosch/Dimensionless.jl.git |
|
[
"MIT"
]
| 0.2.0 | d57e066337ead58265044890809d1a1e9753d949 | code | 4912 | using Test
using Aqua
using Dimensionless
using Unitful
Aqua.test_all(Dimensionless; ambiguities=false)
@testset "DimBasis construction" begin
# Check invalid basis construction exceptions
@test_throws ErrorException DimBasis(9.81u"m/s^2", 1u"mm", 1u"s")
@test_throws ErrorException DimBasis(9.81u"m", 9.81u"m")
@test_throws ErrorException DimBasis(9.81u"m/s^2")
# Check exception in dim_matrix() in case of unvalid dimension
basis = DimBasis(9.81u"m/s^2", 6371u"km", 1420788u"kg", 1u"mol/g")
@test_throws ErrorException dim_matrix(basis.basis_dims, 1u"A")
@test basis.dim_mat == Rational.([1 1 0 0; -2 0 0 0; 0 0 1 -1; 0 0 0 1])
for dim in [:Mass, :Length, :Time, :Amount]
@test Unitful.Dimension{dim} in basis.basis_dims
end
for dim in [:Temperature, :Current, :Luminosity]
@test !(Unitful.Dimension{dim} in basis.basis_dims)
end
# Check all constructor combinations for DimBasis
basis_vector_names_template = ["α", "β", "γ"]
basis_vectors_template = [1u"s^2", 2u"mm^-1", 3u"bar^3"]
for (broadcast_fcn, isnamed) in Iterators.product((identity, unit, dimension), (false, true))
# Construct bases
input_vectors = broadcast(broadcast_fcn, basis_vectors_template)
basis = isnamed ?
DimBasis([Pair(basis_vector_names_template[i], input_vectors[i]) for i in eachindex(basis_vectors_template)]...) :
DimBasis(input_vectors...)
# Check validity
isnamed && @test basis.basis_vector_names == basis_vector_names_template
@test basis.dim_mat == Rational.([2 0 -6; 0 -1 -3; 0 0 3])
@test basis.basis_dims == [Unitful.Dimension{:Time}, Unitful.Dimension{:Length}, Unitful.Dimension{:Mass}]
@test basis.basis_vectors == input_vectors
end
end
@testset "Change of basis" begin
# Test change of basis and broadcast
dimless_dimful_basis = DimBasis(1u"mA", 2u"K", 3u"Pa*s", 4u"h", 5u"kg")
vars = [0u"A/m^2", 1u"nm", 2u"K"]
dim_less = dimless.(vars, dimless_dimful_basis)
@test dimful.(dim_less, [u"A/m^2", u"nm", u"K"], dimless_dimful_basis) == vars
# Test counting of dimensions and dimensionless variables for named basis
quantities_named = ["a" => 100.0u"m", "b" => 100u"kg", "c" => 0u"s"]
units_named = [Pair(var.first, unit(var.second)) for var in quantities_named]
dims_named = [Pair(var.first, dimension(var.second)) for var in quantities_named]
@test num_of_dims(quantities_named...) == 3
@test num_of_dims(units_named...) == 3
@test num_of_dims(dims_named...) == 3
@test num_of_dimless(quantities_named...) == 0
@test num_of_dimless(units_named...) == 0
@test num_of_dimless(dims_named...) == 0
# Test counting of dimensions and dimensionless variables for unnamed basis
quantities_unnamed = [var.second for var in quantities_named]
units_unnamed = [var.second for var in units_named]
dims_unnamed = [var.second for var in dims_named]
@test num_of_dims(quantities_unnamed...) == 3
@test num_of_dims(units_unnamed...) == 3
@test num_of_dims(dims_unnamed...) == 3
@test num_of_dimless(quantities_unnamed...) == 0
@test num_of_dimless(units_unnamed...) == 0
@test num_of_dimless(dims_unnamed...) == 0
# change_basis(quantity/unit, old_b, new_b) for named and unnamed quantity bases
basis_named = DimBasis("x" => 1u"m", "y" => 1u"kg", "z" => 1u"s")
new_basis_named = DimBasis("x_new" => 2u"m", "y_new" => 2u"kg", "z_new" => 2u"s")
@test Dimensionless.current_to_new_fac(Unitful.𝐋 * Unitful.𝐓 * Unitful.𝐌, basis_named, new_basis_named) == 2^3
@test change_basis(100u"cm*kg*s", basis_named, new_basis_named) == 8u"m*kg*s"
@test change_basis(u"m*kg*s", basis_named, new_basis_named) == 8
basis_unnamed = DimBasis(1u"m", 1u"kg", 1u"s")
new_basis_unnamed = DimBasis(2u"m", 2u"kg", 2u"s")
@test Dimensionless.current_to_new_fac(Unitful.𝐋 * Unitful.𝐓 * Unitful.𝐌, basis_unnamed, new_basis_unnamed) == 2^3
@test change_basis(100u"cm*kg*s", basis_unnamed, new_basis_unnamed) == 8u"m*kg*s"
@test change_basis(u"m*kg*s", basis_unnamed, new_basis_unnamed) == 8
end
@testset "Utils" begin
var = "L" => 0.2u"m"
quantities_named = ["ρ" => 1400.0u"kg/m^3", "v" => 0.5u"m/s", "η" => (10^4)u"Pa*s"]
units_named = [Pair(var.first, unit(var.second)) for var in quantities_named]
dims_named = [Pair(var.first, dimension(var.second)) for var in quantities_named]
res_desired = "L ρ v η^-1"
res_buf = IOBuffer()
# Test dimensionless string for named basis
print_dimless(res_buf, var, DimBasis(quantities_named...))
@test String(take!(res_buf)) == res_desired
print_dimless(res_buf, var, DimBasis(units_named...))
@test String(take!(res_buf)) == res_desired
print_dimless(res_buf, var, DimBasis(dims_named...))
@test String(take!(res_buf)) == res_desired
end
| Dimensionless | https://github.com/martinkosch/Dimensionless.jl.git |
|
[
"MIT"
]
| 0.2.0 | d57e066337ead58265044890809d1a1e9753d949 | docs | 2219 | [](https://github.com/martinkosch/Dimensionless.jl/actions/workflows/CI.yml)
[](https://codecov.io/github/martinkosch/Dimensionless.jl)
[](https://martinkosch.github.io/Dimensionless.jl/dev)
# Dimensionless.jl
Dimensionless is a package built on top of [Unitful.jl](https://github.com/PainterQubits/Unitful.jl). It contains tools to conduct dimensional analysis and solve similitude problems based on [the Buckingham Π theorem](https://en.wikipedia.org/wiki/Buckingham_%CF%80_theorem).
## Installation
Install the package using Julia's package manager:
```julia
pkg> add Dimensionless
```
Currently, Unitful's functionality is not exported from Dimensionless. To use Dimensionless, Unitful should be imported as well:
```julia
julia> using Dimensionless, Unitful
```
## Usage
The main functionality of this package is to find and make use of bases made of problem-specific variables and their units. Such bases can then be used to transform additional variables that describe a physical problem to corresponding dimensionless values and back to a dimensional form.
For example, if a problem is, among other variables, characterized by a mass ``m = 15 kg``, a length ``L = 75 cm``, and the gravitational constant ``g = 9.81 m/s^2``, a corresponding basis for the problem can be calculated:
```julia
julia> using Dimensionless, Unitful
julia> basis = DimBasis("m"=>15u"kg", "L"=>75u"cm", "g"=>9.81u"m/s^2")
DimBasis{...}
```
This basis can now be used to coherently tranform any variable within the spanned space of this basis to a dimensionless value and back to a dimensional value again:
```julia
julia> p_dimless = dimless(0.2u"bar", basis)
76.45259938837918
julia> p_dimful = dimful(p_dimless, u"bar", basis)
0.2 bar
```
This procedure enables reducing the number of simulations or experiments as well as streamlining problem formulations. A full [examplary use case](https://martinkosch.github.io/Dimensionless.jl/dev/example/) can be found in the documentation.
| Dimensionless | https://github.com/martinkosch/Dimensionless.jl.git |
|
[
"MIT"
]
| 0.2.0 | d57e066337ead58265044890809d1a1e9753d949 | docs | 7796 | # Example
The following example is taken from the [Similitude Wikipedia article](https://en.wikipedia.org/wiki/Similitude_(model)):
>Consider a submarine modeled at 1/40th scale. The application operates in sea water at 0.5 °C, moving at 5 m/s. The model will be tested in fresh water at 20 °C.
>
> The variables which describe the system are:
>
> Variable | Application | Scaled model |Units
>:-------------------------------|:---------------------|:----------------------|:-------------
> ``L`` (Diameter of submarine) | 1.0 | 1/40 | (m)
> ``V`` (Speed) | 5.0 | **To be calculated** | (m/s)
> ``ρ`` (Density) | 1028.0 | 998.0 | (kg/m^3)
> ``μ`` (Dynamic viscosity) | 1.88e−3 | 1.0e−3 | Pa·s (N s/m^2)
> ``F`` (Force) | **To be calculated** | **To be measured** | N (kg m/s^2)
>
> **Find the power required for the submarine to operate at the stated speed.**
Let's analyze this exemplary problem using Dimensionless.jl. The idea of similitude is that physical problems, despite appearing different in scale, geometry, or conditions, can exhibit similar behavior when analyzed using a dimensionless formulation of the underlying equations.
For the example at hand, the mechanical power `P` of the submarine can generally be expressed as the product of the propulsion force `F` and the velocity `V`.
This relationship applies regardless of the specific problem parameters and the units used. However, the numerical values of the underlying variables change depending on the scaling of the problem and the employed units. For example, the numerical values and the used units in this equation formulated for the submarine in the real application (``P_\mathrm{app} = F_\mathrm{app} \cdot V_\mathrm{app}``) will often be different from the values and units for the scaled submarine model (``P_\mathrm{model} = F_\mathrm{model} \cdot V_\mathrm{model}``). Similitude facilitates to systematically transform all variables in this physically meaningful equation to a dimensionless formulation without the constraints of specific units or scales: ``\tilde{P} = \tilde{F} \cdot \tilde{V}``.
In the following, it will be explained how such a transformation can be achieved and how it can be used to transfer knowledge between differently scaled versions of the same physical problem.
## Analyzing the number of independent variables
According to [the Buckingham Π theorem](https://en.wikipedia.org/wiki/Buckingham_%CF%80_theorem), a problem defined by `n` variables with corresponding units can be transformed into an equation with `n − m` dimensionless quantities, where `m` represents the number of independent base units used.
Translated to the exemplary submarine similitude problem stated above with `n = 5` variables, Dimensionless.jl can be used to calculate that there are `m = 3` independent base units in the present case:
```julia
julia> m = num_of_dims(u"m", u"m/s", u"kg/m^3", u"Pa*s", u"N")
3
```
Correspondingly, there are `n - m = 5 - 3 = 2` dimensionless variables, or Pi terms, that should be selected to effectively capture the essence of the physical phenomena under investigation:
```julia
julia> num_of_dimless(u"m", u"m/s", u"kg/m^3", u"Pa*s", u"N")
2
```
## Finding a dimensional basis
The first step to set up the similitude problem is to find a suitable set of `m` independent variables that serve as a dimensional basis. These variables must span the dimensional space of the studied problem.
In Dimensionless.jl, constructing such a dimensional basis is achieved by creating an instance of type `DimBasis`. While creating such a basis using the `DimBasis` function, it is helpful to name the used variables to allow pretty printing of the results later on:
```julia
julia> basis = DimBasis("L"=>u"m", "ρ"=>u"kg/m^3", "μ"=>u"Pa*s")
DimBasis{...}
```
In this case, a suitable basis could be found by selecting the `m = 3` variables `L`, `ρ`, and `μ` as basis variables. Using such a basis, every variable with a unit that lies inside the space spanned by this basis can be expressed as a linear combination of the basis variables. At the same time, each dimensioned variable can be transformed into a dimensionless representation using these basic variables.
For example, any velocity `V` can be made dimensionless by multiplying it with a factor `L ρ / μ` as can be determined using Dimensionless.jl:
```julia
julia> print_dimless("V"=>u"m/s", basis)
V L ρ μ^-1
```
The same is true for every force `F`:
```julia
julia> print_dimless("F"=>u"N", basis)
F ρ μ^-2
```
Note that the first found number is the Reynolds number ``\mathit{Re}`` and the second number can be identified as the product of the drag coefficient ``c_\mathrm{d}`` and ``\mathit{Re}^2``. In Dimensionless.jl, these numbers are mostly used under the hood to transform between formulations with and without dimensions.
## Calculating the missing entries in the table
Let's use the values in the data table to construct two bases describing the submarine model and the submarine in the real application, respectively:
```julia
julia> app_basis = DimBasis("L"=>1u"m", "ρ"=>1028u"kg/m^3", "μ"=>1.88e-3u"Pa*s")
DimBasis{...}
julia> model_basis = DimBasis("L"=>0.025u"m", "ρ"=>998u"kg/m^3", "μ"=>1e-3u"Pa*s")
DimBasis{...}
```
It is easy to transform values from one dimensional base to the other in order to fill the missing values in the table row by row. Starting with the submarine's velocity of `5 m/s` in the application case, the velocity of the scaled model should be selected to `109.6 m/s` in order to behave physically similar:
```julia
julia> V_model = change_basis(5u"m/s", app_basis, model_basis)
109.58 m s^-1
```
This model velocity is 21.9 times higher than the velocity of the submarine in the real application:
```julia
julia> change_basis(u"m/s", app_basis, model_basis)
21.916172771074063
```
Similarly, the factor needed to convert any given force in Newton from the case of the scaled submarine model to the real application can also be calculated:
```julia
julia> change_basis(u"N", model_basis, app_basis)
3.4313
```
A similar transformation can also be used to determine the power of the real submarine from experiments with the scaled submarine model. As stated above, the mechanical power of the submarine can generally be expressed as ``P = F \cdot V``. If an exemplary force of `1 N` is measured in the scaled experiment with a suitably scaled velocity of `109.6 m/s` as calculated above, this equates to a mechanical power of `1 N * 109.6 m/s = 109.6 W`. This value can be transformed back to obtain the mechanical power of the real submarine while traveling with `V = 5 m/s`:
```julia
julia> P_app_fac = change_basis(109.58u"W", model_basis, app_basis)
17.156144908079394 W
```
Of course, at a velocity of ``V = 109.6 \mathrm{m}/\mathrm{s}`` during the scaled experiment, the obtained force can easily be higher than `1 N`. Generally, the mechanical power of the real submarine scales proportional to the measured force with a factor ``17.156144908079394 \mathrm{W}/\mathrm{N}``.
## Removing and restoring dimensions
Arguably the two most important functions of this package are `dimless()` and `dimful()`. For a given dimensional basis, these two functions can be used to convert single or multiple variables from a formulation with dimensions to a corresponding dimensionless formulation and reverse:
```julia
julia> dim_vars = (1u"cm/g", 1u"mm/s")
(1 cm g^-1, 1 mm s^-1)
julia> dimless_vars = dimless.(dim_vars, model_basis)
(6.2375, 24.95)
julia> dimful.(dimless_vars, (u"cm/g", u"mm/s"), model_basis)
(1.0 cm g^-1, 1.0 mm s^-1)
```
| Dimensionless | https://github.com/martinkosch/Dimensionless.jl.git |
|
[
"MIT"
]
| 0.2.0 | d57e066337ead58265044890809d1a1e9753d949 | docs | 574 | # Introduction
Dimensionless is a package built on top of [Unitful.jl](https://github.com/PainterQubits/Unitful.jl). It contains tools to conduct dimensional analysis and solve similitude problems based on [the Buckingham Π theorem](https://en.wikipedia.org/wiki/Buckingham_%CF%80_theorem).
## Installation
Install the package using Julia's package manager:
```julia
pkg> add Dimensionless
```
Currently, Unitful's functionality is not exported from Dimensionless. To use Dimensionless, Unitful should be imported as well:
```julia
julia> using Dimensionless, Unitful
```
| Dimensionless | https://github.com/martinkosch/Dimensionless.jl.git |
|
[
"MIT"
]
| 0.2.0 | d57e066337ead58265044890809d1a1e9753d949 | docs | 257 | # Library
## Types
```@docs
DimBasis
```
## Functions
```@docs
dim_matrix
```
```@docs
num_of_dims
```
```@docs
num_of_dimless
```
```@docs
fac_dimful
```
```@docs
dimless
```
```@docs
dimful
```
```@docs
change_basis
```
```@docs
print_dimless
```
| Dimensionless | https://github.com/martinkosch/Dimensionless.jl.git |
|
[
"MIT"
]
| 0.1.1 | bf5a89a2be00fb057bcd2489d0b541e424fe3342 | code | 545 | using PaddedBlocks
using Documenter
makedocs(;
modules=[PaddedBlocks],
authors="Vilim Štih <[email protected]>",
repo="https://github.com/portugueslab/PaddedBlocks.jl/blob/{commit}{path}#L{line}",
sitename="PaddedBlocks.jl",
format=Documenter.HTML(;
prettyurls=get(ENV, "CI", "false") == "true",
canonical="https://portugueslab.github.io/PaddedBlocks.jl",
assets=String[],
),
pages=[
"Home" => "index.md",
],
)
deploydocs(;
repo="github.com/portugueslab/PaddedBlocks.jl",
)
| PaddedBlocks | https://github.com/portugueslab/PaddedBlocks.jl.git |
|
[
"MIT"
]
| 0.1.1 | bf5a89a2be00fb057bcd2489d0b541e424fe3342 | code | 109 | module PaddedBlocks
using DocStringExtensions
using JSON3
include("blocks.jl")
export Blocks, slices
end
| PaddedBlocks | https://github.com/portugueslab/PaddedBlocks.jl.git |
|
[
"MIT"
]
| 0.1.1 | bf5a89a2be00fb057bcd2489d0b541e424fe3342 | code | 4748 | struct Blocks{N}
full_size::NTuple{N,Int64}
block_size::NTuple{N,Int64}
crop_start::NTuple{N,Int64}
crop_end::NTuple{N,Int64}
padding::NTuple{N,Int64}
blocks_per_dim::NTuple{N,Int64}
end
function Base.:*(a::Blocks, b::Blocks)
return Blocks(
(a.full_size..., b.full_size...),
(a.block_size..., b.block_size...),
(a.crop_start..., b.crop_start...),
(a.crop_end..., b.crop_end...),
(a.padding..., b.padding...),
(a.blocks_per_dim..., b.blocks_per_dim...),
)
end
"Completes a partially-specified indexing expression"
function complete_index(s, full_size)
return I = tuple((
isa(se, Colon) ? (1:full_size[d]) : (isa(se, Int) ? (se:se) : se) for
(d, se) in enumerate(s)
)...)
end
"""
Constructs a structure helpful with splitting arrays into blocks with padding
$(SIGNATURES)
"""
function Blocks(
full_size::NTuple{N,Int64},
block_size::NTuple{N,Union{Int64,Colon}},
crop_start::NTuple{N,Int64},
crop_end::NTuple{N,Int64},
padding::NTuple{N,Int64},
) where {N}
block_size = tuple((isa(k, Colon) ? s : k for (k, s) in zip(block_size, full_size))...)
blocks_per_dim = tuple(ceil.(
Int64,
(full_size .- crop_start .- crop_end .- padding) ./ block_size,
)...)
return Blocks(full_size, block_size, crop_start, crop_end, padding, blocks_per_dim)
end
function Blocks(
full_size::NTuple{N,Int64},
block_size::NTuple{N,Union{Int64,Colon}};
crop_start::Union{Nothing,NTuple{N,Int64}} = nothing,
crop_end::Union{Nothing,NTuple{N,Int64}} = nothing,
padding::Union{Nothing,NTuple{N,Int64}} = nothing,
) where {N}
zt = tuple((0 for i in 1:N)...)
return Blocks(
full_size,
block_size,
crop_start === nothing ? zt : crop_start,
crop_end === nothing ? zt : crop_end,
padding === nothing ? zt : padding,
)
end
function Base.length(b::Blocks)
return reduce(*, b.blocks_per_dim)
end
function starts_ends(b::Blocks{N}) where {N}
block_starts = Array{Int64}(undef, b.blocks_per_dim..., N)
block_ends = similar(block_starts)
for idx_blocks in CartesianIndices(size(block_starts)[1:end-1])
block_starts[idx_blocks, :] .=
1 .+ b.crop_start .+ (idx_blocks.I .- 1) .* b.block_size
block_ends[idx_blocks, :] .=
min.(
b.full_size .- b.crop_end,
idx_blocks.I .* b.block_size .+ b.crop_start .+ b.padding,
)
end
return block_starts, block_ends
end
function slices(b::Blocks)
starts, ends = starts_ends(b)
i2s = CartesianIndices(b.blocks_per_dim)
return [
tuple(((s:e) for (s, e) in zip(starts[i2s[i], :], ends[i2s[i], :]))...)
for i in 1:length(b)
]
end
"""
Given an indexing expression, return which blocks to take and which parts of them to include
$(SIGNATURES)
"""
function blocks_to_take(
b::Blocks{N},
block_starts::Array{Int64},
block_ends::Array{Int64},
s::NTuple{N,Union{Int,Colon,AbstractRange}},
) where {N}
I = complete_index(s, b.full_size)
file_slices = Array{Int64,2}(undef, (2, N))
block_slices = Array{Int64,2}(undef, (2, N))
for d in 1:N
start = first(I[d])
stop = last(I[d])
axis_index = tuple(((i == d) ? Colon() : 1 for i in 1:N)..., d)
s = searchsortedlast(block_starts[axis_index...], start)
e = searchsortedlast(block_starts[axis_index...], stop)
block_start = start - block_starts[axis_index...][s] + 1
block_end = stop - block_starts[axis_index...][e] + 1
file_slices[:, d] .= [s, e]
block_slices[:, d] .= [block_start, block_end]
end
return file_slices, block_slices
end
function blocks_to_take(b::Blocks{N}, s::NTuple{N,Union{Int,Colon,AbstractRange}}) where {N}
return blocks_to_take(b, starts_ends(b)..., s)
end
function blocks_containing(
b::Blocks{N},
block_starts::Array{Int64},
block_ends::Array{Int64},
s::NTuple{N,Union{Int,Colon,AbstractRange}},
) where {N}
I = complete_index(s, b.full_size)
return tuple((
begin
start = I[d].start
stop = I[d].stop
axis_index = tuple(((i == d) ? Colon() : 1 for i in 1:N)..., d)
lo, up = searchsortedlast.(Ref(block_starts[axis_index...]), [start, stop])
lo:up
end for d in 1:N
)...)
end
# Define JSON serialization
JSON3.StructType(::Type{<:Blocks}) = JSON3.Struct()
function save(filename, b::Blocks)
return open(filename, "w") do f
return write(f, JSON3.write(b))
end
end
function load_blocks(filename)
return open(filename, "r") do f
return JSON3.read(f, Blocks)
end
end | PaddedBlocks | https://github.com/portugueslab/PaddedBlocks.jl.git |
|
[
"MIT"
]
| 0.1.1 | bf5a89a2be00fb057bcd2489d0b541e424fe3342 | code | 431 | @testset "Block operations" begin
n_t = 4
full_size = (n_t, 4, 8, 1)
block_size = (n_t, 4, 3, 1)
padding = (0, 0, 2, 0)
test_blocks = Blocks(full_size, block_size, (0, 0, 0, 0), (0, 0, 0, 0), padding);
test_block_starts, test_block_ends = PaddedBlocks.starts_ends(test_blocks);
@test all(size(test_block_starts) .== (1, 1, 2, 1, 4))
@test all(test_block_starts[1, 1, 2, 1, :] .== [1, 1, 4, 1])
end
| PaddedBlocks | https://github.com/portugueslab/PaddedBlocks.jl.git |
|
[
"MIT"
]
| 0.1.1 | bf5a89a2be00fb057bcd2489d0b541e424fe3342 | code | 51 | using PaddedBlocks
using Test
include("blocks.jl") | PaddedBlocks | https://github.com/portugueslab/PaddedBlocks.jl.git |
|
[
"MIT"
]
| 0.1.1 | bf5a89a2be00fb057bcd2489d0b541e424fe3342 | docs | 716 | # PaddedBlocks
[](https://github.com/portugueslab/PaddedBlocks.jl/actions)
[](https://codecov.io/gh/portugueslab/PaddedBlocks.jl)
[](https://portugueslab.github.io/PaddedBlocks.jl/stable)
[](https://portugueslab.github.io/PaddedBlocks.jl/dev)
Provides a strucuture, `Blocks` which helps splitting computation along continuous spatio-temporal subsets of multidimensional datasets.
## Example
```julia
b = Blocks()
``` | PaddedBlocks | https://github.com/portugueslab/PaddedBlocks.jl.git |
|
[
"MIT"
]
| 0.1.1 | bf5a89a2be00fb057bcd2489d0b541e424fe3342 | docs | 116 | ```@meta
CurrentModule = PaddedBlocks
```
# PaddedBlocks
```@index
```
```@autodocs
Modules = [PaddedBlocks]
```
| PaddedBlocks | https://github.com/portugueslab/PaddedBlocks.jl.git |
|
[
"MIT"
]
| 0.6.4 | b4ac59b6877535177e89e1fe1b0ff5d0c2e903de | code | 1301 | using SmithNormalForm
using BenchmarkTools
using SparseArrays
using Random
Random.seed!(2903872398473);
rows, cols = 64, 93
TMP = rand(1:10, rows*cols)
D = reshape(TMP, rows, cols)
i, j = 2, 3
a, b, c, d = 1, 2, 3, 4
suite = BenchmarkGroup()
suite["internal"] = BenchmarkGroup(["swap", "elimination"])
suite["internal"]["col_swap"] = @benchmarkable SmithNormalForm.cswap!(D, i, j) gctrial=true evals=1000 samples=100000
suite["internal"]["row_swap"] = @benchmarkable SmithNormalForm.rswap!(D, i, j) gctrial=true evals=1000 samples=100000
suite["internal"]["col_elim"] = @benchmarkable SmithNormalForm.colelimination(D, a, b, c, d, i, j) evals=500 gctrial=true
suite["internal"]["row_elim"] = @benchmarkable SmithNormalForm.rowelimination(D, a, b, c, d, i, j) evals=500 gctrial=true
suite["snf"] = BenchmarkGroup(["type"])
Z = rand(1:100, 100, 120)
Z[Z .> 66] .= 1
Z[Z .> 33] .= -1
Z[Z .> 0] .= 0
suite["snf"]["dense"] = @benchmarkable snf(Z)
Y = sprand(Int, 100, 120, 0.3)
Y[Y .< 0] .= -1
Y[Y .> 0] .= 1
suite["snf"]["sparse"] = @benchmarkable snf(Y)
# tune!(suite);
# BenchmarkTools.save("bmsetup.json", params(suite));
loadparams!(suite, BenchmarkTools.load("bmsetup.json")[1], :evals, :samples);
results = run(suite, verbose = true, seconds = 1)
BenchmarkTools.save("results.json", results)
| Crystalline | https://github.com/thchr/Crystalline.jl.git |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.