licenses
sequencelengths 1
3
| version
stringclasses 677
values | tree_hash
stringlengths 40
40
| path
stringclasses 1
value | type
stringclasses 2
values | size
stringlengths 2
8
| text
stringlengths 25
67.1M
| package_name
stringlengths 2
41
| repo
stringlengths 33
86
|
---|---|---|---|---|---|---|---|---|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 363 | using Clang.Generators
using Lua_jll
cd(@__DIR__)
const INCLUDE_DIR = normpath(Lua_jll.artifact_dir, "include")
options = load_options(joinpath(@__DIR__, "generator.toml"))
args = get_default_args()
push!(args, "-I$INCLUDE_DIR")
headers = joinpath.(INCLUDE_DIR, ["lua.h", "lauxlib.h", "lualib.h"])
ctx = create_context(headers, args, options)
build!(ctx)
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 192 | const UINT_MAX = typemax(UInt)
const LLONG_MIN = typemin(Clonglong)
const LLONG_MAX = typemax(Clonglong)
const ULLONG_MAX = typemax(Culonglong)
const ptrdiff_t = Cptrdiff_t
const NULL = C_NULL | LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 30310 | module LibLua
using Lua_jll
export Lua_jll
const UINT_MAX = typemax(UInt)
const LLONG_MIN = typemin(Clonglong)
const LLONG_MAX = typemax(Clonglong)
const ULLONG_MAX = typemax(Culonglong)
const ptrdiff_t = Cptrdiff_t
const NULL = C_NULL
const lua_Number = Cdouble
const lua_Integer = Clonglong
mutable struct lua_State end
const lua_KContext = Cptrdiff_t
# typedef int ( * lua_KFunction ) ( lua_State * L , int status , lua_KContext ctx )
const lua_KFunction = Ptr{Cvoid}
function lua_callk(L, nargs, nresults, ctx, k)
ccall((:lua_callk, liblua), Cvoid, (Ptr{lua_State}, Cint, Cint, lua_KContext, lua_KFunction), L, nargs, nresults, ctx, k)
end
function lua_pcallk(L, nargs, nresults, errfunc, ctx, k)
ccall((:lua_pcallk, liblua), Cint, (Ptr{lua_State}, Cint, Cint, Cint, lua_KContext, lua_KFunction), L, nargs, nresults, errfunc, ctx, k)
end
function lua_yieldk(L, nresults, ctx, k)
ccall((:lua_yieldk, liblua), Cint, (Ptr{lua_State}, Cint, lua_KContext, lua_KFunction), L, nresults, ctx, k)
end
function lua_tonumberx(L, idx, isnum)
ccall((:lua_tonumberx, liblua), lua_Number, (Ptr{lua_State}, Cint, Ptr{Cint}), L, idx, isnum)
end
function lua_tointegerx(L, idx, isnum)
ccall((:lua_tointegerx, liblua), lua_Integer, (Ptr{lua_State}, Cint, Ptr{Cint}), L, idx, isnum)
end
function lua_settop(L, idx)
ccall((:lua_settop, liblua), Cvoid, (Ptr{lua_State}, Cint), L, idx)
end
function lua_createtable(L, narr, nrec)
ccall((:lua_createtable, liblua), Cvoid, (Ptr{lua_State}, Cint, Cint), L, narr, nrec)
end
# typedef int ( * lua_CFunction ) ( lua_State * L )
const lua_CFunction = Ptr{Cvoid}
function lua_pushcclosure(L, fn, n)
ccall((:lua_pushcclosure, liblua), Cvoid, (Ptr{lua_State}, lua_CFunction, Cint), L, fn, n)
end
function lua_setglobal(L, name)
ccall((:lua_setglobal, liblua), Cvoid, (Ptr{lua_State}, Ptr{Cchar}), L, name)
end
function lua_type(L, idx)
ccall((:lua_type, liblua), Cint, (Ptr{lua_State}, Cint), L, idx)
end
function lua_pushstring(L, s)
ccall((:lua_pushstring, liblua), Ptr{Cchar}, (Ptr{lua_State}, Ptr{Cchar}), L, s)
end
function lua_rawgeti(L, idx, n)
ccall((:lua_rawgeti, liblua), Cint, (Ptr{lua_State}, Cint, lua_Integer), L, idx, n)
end
function lua_tolstring(L, idx, len)
ccall((:lua_tolstring, liblua), Ptr{Cchar}, (Ptr{lua_State}, Cint, Ptr{Csize_t}), L, idx, len)
end
function lua_rotate(L, idx, n)
ccall((:lua_rotate, liblua), Cvoid, (Ptr{lua_State}, Cint, Cint), L, idx, n)
end
function lua_copy(L, fromidx, toidx)
ccall((:lua_copy, liblua), Cvoid, (Ptr{lua_State}, Cint, Cint), L, fromidx, toidx)
end
function lua_newuserdatauv(L, sz, nuvalue)
ccall((:lua_newuserdatauv, liblua), Ptr{Cvoid}, (Ptr{lua_State}, Csize_t, Cint), L, sz, nuvalue)
end
function lua_getiuservalue(L, idx, n)
ccall((:lua_getiuservalue, liblua), Cint, (Ptr{lua_State}, Cint, Cint), L, idx, n)
end
function lua_setiuservalue(L, idx, n)
ccall((:lua_setiuservalue, liblua), Cint, (Ptr{lua_State}, Cint, Cint), L, idx, n)
end
const lua_Unsigned = Culonglong
# typedef const char * ( * lua_Reader ) ( lua_State * L , void * ud , size_t * sz )
const lua_Reader = Ptr{Cvoid}
# typedef int ( * lua_Writer ) ( lua_State * L , const void * p , size_t sz , void * ud )
const lua_Writer = Ptr{Cvoid}
# typedef void * ( * lua_Alloc ) ( void * ud , void * ptr , size_t osize , size_t nsize )
const lua_Alloc = Ptr{Cvoid}
# typedef void ( * lua_WarnFunction ) ( void * ud , const char * msg , int tocont )
const lua_WarnFunction = Ptr{Cvoid}
function lua_newstate(f, ud)
ccall((:lua_newstate, liblua), Ptr{lua_State}, (lua_Alloc, Ptr{Cvoid}), f, ud)
end
function lua_close(L)
ccall((:lua_close, liblua), Cvoid, (Ptr{lua_State},), L)
end
function lua_newthread(L)
ccall((:lua_newthread, liblua), Ptr{lua_State}, (Ptr{lua_State},), L)
end
function lua_resetthread(L)
ccall((:lua_resetthread, liblua), Cint, (Ptr{lua_State},), L)
end
function lua_atpanic(L, panicf)
ccall((:lua_atpanic, liblua), lua_CFunction, (Ptr{lua_State}, lua_CFunction), L, panicf)
end
function lua_version(L)
ccall((:lua_version, liblua), lua_Number, (Ptr{lua_State},), L)
end
function lua_absindex(L, idx)
ccall((:lua_absindex, liblua), Cint, (Ptr{lua_State}, Cint), L, idx)
end
function lua_gettop(L)
ccall((:lua_gettop, liblua), Cint, (Ptr{lua_State},), L)
end
function lua_pushvalue(L, idx)
ccall((:lua_pushvalue, liblua), Cvoid, (Ptr{lua_State}, Cint), L, idx)
end
function lua_checkstack(L, n)
ccall((:lua_checkstack, liblua), Cint, (Ptr{lua_State}, Cint), L, n)
end
function lua_xmove(from, to, n)
ccall((:lua_xmove, liblua), Cvoid, (Ptr{lua_State}, Ptr{lua_State}, Cint), from, to, n)
end
function lua_isnumber(L, idx)
ccall((:lua_isnumber, liblua), Cint, (Ptr{lua_State}, Cint), L, idx)
end
function lua_isstring(L, idx)
ccall((:lua_isstring, liblua), Cint, (Ptr{lua_State}, Cint), L, idx)
end
function lua_iscfunction(L, idx)
ccall((:lua_iscfunction, liblua), Cint, (Ptr{lua_State}, Cint), L, idx)
end
function lua_isinteger(L, idx)
ccall((:lua_isinteger, liblua), Cint, (Ptr{lua_State}, Cint), L, idx)
end
function lua_isuserdata(L, idx)
ccall((:lua_isuserdata, liblua), Cint, (Ptr{lua_State}, Cint), L, idx)
end
function lua_typename(L, tp)
ccall((:lua_typename, liblua), Ptr{Cchar}, (Ptr{lua_State}, Cint), L, tp)
end
function lua_toboolean(L, idx)
ccall((:lua_toboolean, liblua), Cint, (Ptr{lua_State}, Cint), L, idx)
end
function lua_rawlen(L, idx)
ccall((:lua_rawlen, liblua), lua_Unsigned, (Ptr{lua_State}, Cint), L, idx)
end
function lua_tocfunction(L, idx)
ccall((:lua_tocfunction, liblua), lua_CFunction, (Ptr{lua_State}, Cint), L, idx)
end
function lua_touserdata(L, idx)
ccall((:lua_touserdata, liblua), Ptr{Cvoid}, (Ptr{lua_State}, Cint), L, idx)
end
function lua_tothread(L, idx)
ccall((:lua_tothread, liblua), Ptr{lua_State}, (Ptr{lua_State}, Cint), L, idx)
end
function lua_topointer(L, idx)
ccall((:lua_topointer, liblua), Ptr{Cvoid}, (Ptr{lua_State}, Cint), L, idx)
end
function lua_arith(L, op)
ccall((:lua_arith, liblua), Cvoid, (Ptr{lua_State}, Cint), L, op)
end
function lua_rawequal(L, idx1, idx2)
ccall((:lua_rawequal, liblua), Cint, (Ptr{lua_State}, Cint, Cint), L, idx1, idx2)
end
function lua_compare(L, idx1, idx2, op)
ccall((:lua_compare, liblua), Cint, (Ptr{lua_State}, Cint, Cint, Cint), L, idx1, idx2, op)
end
function lua_pushnil(L)
ccall((:lua_pushnil, liblua), Cvoid, (Ptr{lua_State},), L)
end
function lua_pushnumber(L, n)
ccall((:lua_pushnumber, liblua), Cvoid, (Ptr{lua_State}, lua_Number), L, n)
end
function lua_pushinteger(L, n)
ccall((:lua_pushinteger, liblua), Cvoid, (Ptr{lua_State}, lua_Integer), L, n)
end
function lua_pushlstring(L, s, len)
ccall((:lua_pushlstring, liblua), Ptr{Cchar}, (Ptr{lua_State}, Ptr{Cchar}, Csize_t), L, s, len)
end
function lua_pushboolean(L, b)
ccall((:lua_pushboolean, liblua), Cvoid, (Ptr{lua_State}, Cint), L, b)
end
function lua_pushlightuserdata(L, p)
ccall((:lua_pushlightuserdata, liblua), Cvoid, (Ptr{lua_State}, Ptr{Cvoid}), L, p)
end
function lua_pushthread(L)
ccall((:lua_pushthread, liblua), Cint, (Ptr{lua_State},), L)
end
function lua_getglobal(L, name)
ccall((:lua_getglobal, liblua), Cint, (Ptr{lua_State}, Ptr{Cchar}), L, name)
end
function lua_gettable(L, idx)
ccall((:lua_gettable, liblua), Cint, (Ptr{lua_State}, Cint), L, idx)
end
function lua_getfield(L, idx, k)
ccall((:lua_getfield, liblua), Cint, (Ptr{lua_State}, Cint, Ptr{Cchar}), L, idx, k)
end
function lua_geti(L, idx, n)
ccall((:lua_geti, liblua), Cint, (Ptr{lua_State}, Cint, lua_Integer), L, idx, n)
end
function lua_rawget(L, idx)
ccall((:lua_rawget, liblua), Cint, (Ptr{lua_State}, Cint), L, idx)
end
function lua_rawgetp(L, idx, p)
ccall((:lua_rawgetp, liblua), Cint, (Ptr{lua_State}, Cint, Ptr{Cvoid}), L, idx, p)
end
function lua_getmetatable(L, objindex)
ccall((:lua_getmetatable, liblua), Cint, (Ptr{lua_State}, Cint), L, objindex)
end
function lua_settable(L, idx)
ccall((:lua_settable, liblua), Cvoid, (Ptr{lua_State}, Cint), L, idx)
end
function lua_setfield(L, idx, k)
ccall((:lua_setfield, liblua), Cvoid, (Ptr{lua_State}, Cint, Ptr{Cchar}), L, idx, k)
end
function lua_seti(L, idx, n)
ccall((:lua_seti, liblua), Cvoid, (Ptr{lua_State}, Cint, lua_Integer), L, idx, n)
end
function lua_rawset(L, idx)
ccall((:lua_rawset, liblua), Cvoid, (Ptr{lua_State}, Cint), L, idx)
end
function lua_rawseti(L, idx, n)
ccall((:lua_rawseti, liblua), Cvoid, (Ptr{lua_State}, Cint, lua_Integer), L, idx, n)
end
function lua_rawsetp(L, idx, p)
ccall((:lua_rawsetp, liblua), Cvoid, (Ptr{lua_State}, Cint, Ptr{Cvoid}), L, idx, p)
end
function lua_setmetatable(L, objindex)
ccall((:lua_setmetatable, liblua), Cint, (Ptr{lua_State}, Cint), L, objindex)
end
function lua_load(L, reader, dt, chunkname, mode)
ccall((:lua_load, liblua), Cint, (Ptr{lua_State}, lua_Reader, Ptr{Cvoid}, Ptr{Cchar}, Ptr{Cchar}), L, reader, dt, chunkname, mode)
end
function lua_dump(L, writer, data, strip)
ccall((:lua_dump, liblua), Cint, (Ptr{lua_State}, lua_Writer, Ptr{Cvoid}, Cint), L, writer, data, strip)
end
function lua_resume(L, from, narg, nres)
ccall((:lua_resume, liblua), Cint, (Ptr{lua_State}, Ptr{lua_State}, Cint, Ptr{Cint}), L, from, narg, nres)
end
function lua_status(L)
ccall((:lua_status, liblua), Cint, (Ptr{lua_State},), L)
end
function lua_isyieldable(L)
ccall((:lua_isyieldable, liblua), Cint, (Ptr{lua_State},), L)
end
function lua_setwarnf(L, f, ud)
ccall((:lua_setwarnf, liblua), Cvoid, (Ptr{lua_State}, lua_WarnFunction, Ptr{Cvoid}), L, f, ud)
end
function lua_warning(L, msg, tocont)
ccall((:lua_warning, liblua), Cvoid, (Ptr{lua_State}, Ptr{Cchar}, Cint), L, msg, tocont)
end
function lua_error(L)
ccall((:lua_error, liblua), Cint, (Ptr{lua_State},), L)
end
function lua_next(L, idx)
ccall((:lua_next, liblua), Cint, (Ptr{lua_State}, Cint), L, idx)
end
function lua_concat(L, n)
ccall((:lua_concat, liblua), Cvoid, (Ptr{lua_State}, Cint), L, n)
end
function lua_len(L, idx)
ccall((:lua_len, liblua), Cvoid, (Ptr{lua_State}, Cint), L, idx)
end
function lua_stringtonumber(L, s)
ccall((:lua_stringtonumber, liblua), Csize_t, (Ptr{lua_State}, Ptr{Cchar}), L, s)
end
function lua_getallocf(L, ud)
ccall((:lua_getallocf, liblua), lua_Alloc, (Ptr{lua_State}, Ptr{Ptr{Cvoid}}), L, ud)
end
function lua_setallocf(L, f, ud)
ccall((:lua_setallocf, liblua), Cvoid, (Ptr{lua_State}, lua_Alloc, Ptr{Cvoid}), L, f, ud)
end
function lua_toclose(L, idx)
ccall((:lua_toclose, liblua), Cvoid, (Ptr{lua_State}, Cint), L, idx)
end
function lua_closeslot(L, idx)
ccall((:lua_closeslot, liblua), Cvoid, (Ptr{lua_State}, Cint), L, idx)
end
mutable struct CallInfo end
struct lua_Debug
event::Cint
name::Ptr{Cchar}
namewhat::Ptr{Cchar}
what::Ptr{Cchar}
source::Ptr{Cchar}
srclen::Csize_t
currentline::Cint
linedefined::Cint
lastlinedefined::Cint
nups::Cuchar
nparams::Cuchar
isvararg::Cchar
istailcall::Cchar
ftransfer::Cushort
ntransfer::Cushort
short_src::NTuple{60, Cchar}
i_ci::Ptr{CallInfo}
end
# typedef void ( * lua_Hook ) ( lua_State * L , lua_Debug * ar )
const lua_Hook = Ptr{Cvoid}
function lua_getstack(L, level, ar)
ccall((:lua_getstack, liblua), Cint, (Ptr{lua_State}, Cint, Ptr{lua_Debug}), L, level, ar)
end
function lua_getinfo(L, what, ar)
ccall((:lua_getinfo, liblua), Cint, (Ptr{lua_State}, Ptr{Cchar}, Ptr{lua_Debug}), L, what, ar)
end
function lua_getlocal(L, ar, n)
ccall((:lua_getlocal, liblua), Ptr{Cchar}, (Ptr{lua_State}, Ptr{lua_Debug}, Cint), L, ar, n)
end
function lua_setlocal(L, ar, n)
ccall((:lua_setlocal, liblua), Ptr{Cchar}, (Ptr{lua_State}, Ptr{lua_Debug}, Cint), L, ar, n)
end
function lua_getupvalue(L, funcindex, n)
ccall((:lua_getupvalue, liblua), Ptr{Cchar}, (Ptr{lua_State}, Cint, Cint), L, funcindex, n)
end
function lua_setupvalue(L, funcindex, n)
ccall((:lua_setupvalue, liblua), Ptr{Cchar}, (Ptr{lua_State}, Cint, Cint), L, funcindex, n)
end
function lua_upvalueid(L, fidx, n)
ccall((:lua_upvalueid, liblua), Ptr{Cvoid}, (Ptr{lua_State}, Cint, Cint), L, fidx, n)
end
function lua_upvaluejoin(L, fidx1, n1, fidx2, n2)
ccall((:lua_upvaluejoin, liblua), Cvoid, (Ptr{lua_State}, Cint, Cint, Cint, Cint), L, fidx1, n1, fidx2, n2)
end
function lua_sethook(L, func, mask, count)
ccall((:lua_sethook, liblua), Cvoid, (Ptr{lua_State}, lua_Hook, Cint, Cint), L, func, mask, count)
end
function lua_gethook(L)
ccall((:lua_gethook, liblua), lua_Hook, (Ptr{lua_State},), L)
end
function lua_gethookmask(L)
ccall((:lua_gethookmask, liblua), Cint, (Ptr{lua_State},), L)
end
function lua_gethookcount(L)
ccall((:lua_gethookcount, liblua), Cint, (Ptr{lua_State},), L)
end
function lua_setcstacklimit(L, limit)
ccall((:lua_setcstacklimit, liblua), Cint, (Ptr{lua_State}, Cuint), L, limit)
end
function luaL_checkversion_(L, ver, sz)
ccall((:luaL_checkversion_, liblua), Cvoid, (Ptr{lua_State}, lua_Number, Csize_t), L, ver, sz)
end
function luaL_loadfilex(L, filename, mode)
ccall((:luaL_loadfilex, liblua), Cint, (Ptr{lua_State}, Ptr{Cchar}, Ptr{Cchar}), L, filename, mode)
end
struct luaL_Reg
name::Ptr{Cchar}
func::lua_CFunction
end
function luaL_setfuncs(L, l, nup)
ccall((:luaL_setfuncs, liblua), Cvoid, (Ptr{lua_State}, Ptr{luaL_Reg}, Cint), L, l, nup)
end
function luaL_argerror(L, arg, extramsg)
ccall((:luaL_argerror, liblua), Cint, (Ptr{lua_State}, Cint, Ptr{Cchar}), L, arg, extramsg)
end
function luaL_typeerror(L, arg, tname)
ccall((:luaL_typeerror, liblua), Cint, (Ptr{lua_State}, Cint, Ptr{Cchar}), L, arg, tname)
end
function luaL_checklstring(L, arg, l)
ccall((:luaL_checklstring, liblua), Ptr{Cchar}, (Ptr{lua_State}, Cint, Ptr{Csize_t}), L, arg, l)
end
function luaL_optlstring(L, arg, def, l)
ccall((:luaL_optlstring, liblua), Ptr{Cchar}, (Ptr{lua_State}, Cint, Ptr{Cchar}, Ptr{Csize_t}), L, arg, def, l)
end
function luaL_loadstring(L, s)
ccall((:luaL_loadstring, liblua), Cint, (Ptr{lua_State}, Ptr{Cchar}), L, s)
end
function luaL_loadbufferx(L, buff, sz, name, mode)
ccall((:luaL_loadbufferx, liblua), Cint, (Ptr{lua_State}, Ptr{Cchar}, Csize_t, Ptr{Cchar}, Ptr{Cchar}), L, buff, sz, name, mode)
end
struct var"##Ctag#294"
data::NTuple{1024, UInt8}
end
function Base.getproperty(x::Ptr{var"##Ctag#294"}, f::Symbol)
f === :n && return Ptr{lua_Number}(x + 0)
f === :u && return Ptr{Cdouble}(x + 0)
f === :s && return Ptr{Ptr{Cvoid}}(x + 0)
f === :i && return Ptr{lua_Integer}(x + 0)
f === :l && return Ptr{Clong}(x + 0)
f === :b && return Ptr{NTuple{1024, Cchar}}(x + 0)
return getfield(x, f)
end
function Base.getproperty(x::var"##Ctag#294", f::Symbol)
r = Ref{var"##Ctag#294"}(x)
ptr = Base.unsafe_convert(Ptr{var"##Ctag#294"}, r)
fptr = getproperty(ptr, f)
GC.@preserve r unsafe_load(fptr)
end
function Base.setproperty!(x::Ptr{var"##Ctag#294"}, f::Symbol, v)
unsafe_store!(getproperty(x, f), v)
end
struct luaL_Buffer
data::NTuple{1056, UInt8}
end
function Base.getproperty(x::Ptr{luaL_Buffer}, f::Symbol)
f === :b && return Ptr{Ptr{Cchar}}(x + 0)
f === :size && return Ptr{Csize_t}(x + 8)
f === :n && return Ptr{Csize_t}(x + 16)
f === :L && return Ptr{Ptr{lua_State}}(x + 24)
f === :init && return Ptr{var"##Ctag#294"}(x + 32)
return getfield(x, f)
end
function Base.getproperty(x::luaL_Buffer, f::Symbol)
r = Ref{luaL_Buffer}(x)
ptr = Base.unsafe_convert(Ptr{luaL_Buffer}, r)
fptr = getproperty(ptr, f)
GC.@preserve r unsafe_load(fptr)
end
function Base.setproperty!(x::Ptr{luaL_Buffer}, f::Symbol, v)
unsafe_store!(getproperty(x, f), v)
end
function luaL_prepbuffsize(B, sz)
ccall((:luaL_prepbuffsize, liblua), Ptr{Cchar}, (Ptr{luaL_Buffer}, Csize_t), B, sz)
end
function luaL_getmetafield(L, obj, e)
ccall((:luaL_getmetafield, liblua), Cint, (Ptr{lua_State}, Cint, Ptr{Cchar}), L, obj, e)
end
function luaL_callmeta(L, obj, e)
ccall((:luaL_callmeta, liblua), Cint, (Ptr{lua_State}, Cint, Ptr{Cchar}), L, obj, e)
end
function luaL_tolstring(L, idx, len)
ccall((:luaL_tolstring, liblua), Ptr{Cchar}, (Ptr{lua_State}, Cint, Ptr{Csize_t}), L, idx, len)
end
function luaL_checknumber(L, arg)
ccall((:luaL_checknumber, liblua), lua_Number, (Ptr{lua_State}, Cint), L, arg)
end
function luaL_optnumber(L, arg, def)
ccall((:luaL_optnumber, liblua), lua_Number, (Ptr{lua_State}, Cint, lua_Number), L, arg, def)
end
function luaL_checkinteger(L, arg)
ccall((:luaL_checkinteger, liblua), lua_Integer, (Ptr{lua_State}, Cint), L, arg)
end
function luaL_optinteger(L, arg, def)
ccall((:luaL_optinteger, liblua), lua_Integer, (Ptr{lua_State}, Cint, lua_Integer), L, arg, def)
end
function luaL_checkstack(L, sz, msg)
ccall((:luaL_checkstack, liblua), Cvoid, (Ptr{lua_State}, Cint, Ptr{Cchar}), L, sz, msg)
end
function luaL_checktype(L, arg, t)
ccall((:luaL_checktype, liblua), Cvoid, (Ptr{lua_State}, Cint, Cint), L, arg, t)
end
function luaL_checkany(L, arg)
ccall((:luaL_checkany, liblua), Cvoid, (Ptr{lua_State}, Cint), L, arg)
end
function luaL_newmetatable(L, tname)
ccall((:luaL_newmetatable, liblua), Cint, (Ptr{lua_State}, Ptr{Cchar}), L, tname)
end
function luaL_setmetatable(L, tname)
ccall((:luaL_setmetatable, liblua), Cvoid, (Ptr{lua_State}, Ptr{Cchar}), L, tname)
end
function luaL_testudata(L, ud, tname)
ccall((:luaL_testudata, liblua), Ptr{Cvoid}, (Ptr{lua_State}, Cint, Ptr{Cchar}), L, ud, tname)
end
function luaL_checkudata(L, ud, tname)
ccall((:luaL_checkudata, liblua), Ptr{Cvoid}, (Ptr{lua_State}, Cint, Ptr{Cchar}), L, ud, tname)
end
function luaL_where(L, lvl)
ccall((:luaL_where, liblua), Cvoid, (Ptr{lua_State}, Cint), L, lvl)
end
function luaL_checkoption(L, arg, def, lst)
ccall((:luaL_checkoption, liblua), Cint, (Ptr{lua_State}, Cint, Ptr{Cchar}, Ptr{Ptr{Cchar}}), L, arg, def, lst)
end
function luaL_fileresult(L, stat, fname)
ccall((:luaL_fileresult, liblua), Cint, (Ptr{lua_State}, Cint, Ptr{Cchar}), L, stat, fname)
end
function luaL_execresult(L, stat)
ccall((:luaL_execresult, liblua), Cint, (Ptr{lua_State}, Cint), L, stat)
end
function luaL_ref(L, t)
ccall((:luaL_ref, liblua), Cint, (Ptr{lua_State}, Cint), L, t)
end
function luaL_unref(L, t, ref)
ccall((:luaL_unref, liblua), Cvoid, (Ptr{lua_State}, Cint, Cint), L, t, ref)
end
function luaL_newstate()
ccall((:luaL_newstate, liblua), Ptr{lua_State}, ())
end
function luaL_len(L, idx)
ccall((:luaL_len, liblua), lua_Integer, (Ptr{lua_State}, Cint), L, idx)
end
function luaL_addgsub(b, s, p, r)
ccall((:luaL_addgsub, liblua), Cvoid, (Ptr{luaL_Buffer}, Ptr{Cchar}, Ptr{Cchar}, Ptr{Cchar}), b, s, p, r)
end
function luaL_gsub(L, s, p, r)
ccall((:luaL_gsub, liblua), Ptr{Cchar}, (Ptr{lua_State}, Ptr{Cchar}, Ptr{Cchar}, Ptr{Cchar}), L, s, p, r)
end
function luaL_getsubtable(L, idx, fname)
ccall((:luaL_getsubtable, liblua), Cint, (Ptr{lua_State}, Cint, Ptr{Cchar}), L, idx, fname)
end
function luaL_traceback(L, L1, msg, level)
ccall((:luaL_traceback, liblua), Cvoid, (Ptr{lua_State}, Ptr{lua_State}, Ptr{Cchar}, Cint), L, L1, msg, level)
end
function luaL_requiref(L, modname, openf, glb)
ccall((:luaL_requiref, liblua), Cvoid, (Ptr{lua_State}, Ptr{Cchar}, lua_CFunction, Cint), L, modname, openf, glb)
end
function luaL_buffinit(L, B)
ccall((:luaL_buffinit, liblua), Cvoid, (Ptr{lua_State}, Ptr{luaL_Buffer}), L, B)
end
function luaL_addlstring(B, s, l)
ccall((:luaL_addlstring, liblua), Cvoid, (Ptr{luaL_Buffer}, Ptr{Cchar}, Csize_t), B, s, l)
end
function luaL_addstring(B, s)
ccall((:luaL_addstring, liblua), Cvoid, (Ptr{luaL_Buffer}, Ptr{Cchar}), B, s)
end
function luaL_addvalue(B)
ccall((:luaL_addvalue, liblua), Cvoid, (Ptr{luaL_Buffer},), B)
end
function luaL_pushresult(B)
ccall((:luaL_pushresult, liblua), Cvoid, (Ptr{luaL_Buffer},), B)
end
function luaL_pushresultsize(B, sz)
ccall((:luaL_pushresultsize, liblua), Cvoid, (Ptr{luaL_Buffer}, Csize_t), B, sz)
end
function luaL_buffinitsize(L, B, sz)
ccall((:luaL_buffinitsize, liblua), Ptr{Cchar}, (Ptr{lua_State}, Ptr{luaL_Buffer}, Csize_t), L, B, sz)
end
struct luaL_Stream
f::Ptr{Libc.FILE}
closef::lua_CFunction
end
function jl_lua_int_type()
ccall((:jl_lua_int_type, liblua), Cint, ())
end
function jl_lua_float_type()
ccall((:jl_lua_float_type, liblua), Cint, ())
end
function jl_lua_function_wrapper(L)
ccall((:jl_lua_function_wrapper, liblua), Cint, (Ptr{lua_State},), L)
end
function luaopen_base(L)
ccall((:luaopen_base, liblua), Cint, (Ptr{lua_State},), L)
end
function luaopen_coroutine(L)
ccall((:luaopen_coroutine, liblua), Cint, (Ptr{lua_State},), L)
end
function luaopen_table(L)
ccall((:luaopen_table, liblua), Cint, (Ptr{lua_State},), L)
end
function luaopen_io(L)
ccall((:luaopen_io, liblua), Cint, (Ptr{lua_State},), L)
end
function luaopen_os(L)
ccall((:luaopen_os, liblua), Cint, (Ptr{lua_State},), L)
end
function luaopen_string(L)
ccall((:luaopen_string, liblua), Cint, (Ptr{lua_State},), L)
end
function luaopen_utf8(L)
ccall((:luaopen_utf8, liblua), Cint, (Ptr{lua_State},), L)
end
function luaopen_math(L)
ccall((:luaopen_math, liblua), Cint, (Ptr{lua_State},), L)
end
function luaopen_debug(L)
ccall((:luaopen_debug, liblua), Cint, (Ptr{lua_State},), L)
end
function luaopen_package(L)
ccall((:luaopen_package, liblua), Cint, (Ptr{lua_State},), L)
end
function luaL_openlibs(L)
ccall((:luaL_openlibs, liblua), Cvoid, (Ptr{lua_State},), L)
end
const LUAI_IS32INT = UINT_MAX >> 30 >= 3
const LUA_INT_INT = 1
const LUA_INT_LONG = 2
const LUA_INT_LONGLONG = 3
const LUA_FLOAT_FLOAT = 1
const LUA_FLOAT_DOUBLE = 2
const LUA_FLOAT_LONGDOUBLE = 3
const LUA_INT_DEFAULT = LUA_INT_LONGLONG
const LUA_FLOAT_DEFAULT = LUA_FLOAT_DOUBLE
const LUA_32BITS = 0
const LUA_C89_NUMBERS = 0
const LUA_INT_TYPE = LUA_INT_DEFAULT
const LUA_FLOAT_TYPE = LUA_FLOAT_DEFAULT
const LUA_PATH_SEP = ";"
const LUA_PATH_MARK = "?"
const LUA_EXEC_DIR = "!"
const LUA_VERSION_MAJOR = "5"
const LUA_VERSION_MINOR = "4"
const LUA_LDIR = "!\\lua\\"
const LUA_CDIR = "!\\"
# Skipping MacroDefinition: LUA_PATH_DEFAULT LUA_LDIR "?.lua;" LUA_LDIR "?\\init.lua;" LUA_CDIR "?.lua;" LUA_CDIR "?\\init.lua;" LUA_SHRDIR "?.lua;" LUA_SHRDIR "?\\init.lua;" ".\\?.lua;" ".\\?\\init.lua"
# Skipping MacroDefinition: LUA_CPATH_DEFAULT LUA_CDIR "?.dll;" LUA_CDIR "..\\lib\\lua\\" LUA_VDIR "\\?.dll;" LUA_CDIR "loadall.dll;" ".\\?.dll"
const LUA_DIRSEP = "\\"
# Skipping MacroDefinition: LUA_API extern
# Skipping MacroDefinition: LUAI_FUNC extern
LUAI_DDEC(dec) = LUAI_FUNC(dec)
l_mathop(op) = op
l_floor(x) = (l_mathop(floor))(x)
l_sprintf(s, sz, f, i) = (Cvoid(sz), sprintf(s, f, i))
const LUA_NUMBER_FMT = "%.14g"
const LUAI_UACNUMBER = Float64
lua_number2str(s, sz, n) = l_sprintf(s, sz, LUA_NUMBER_FMT, LUAI_UACNUMBER(n))
const LUA_NUMBER = Float64
const LUA_MININTEGER = LLONG_MIN
const LUA_INTEGER = Clonglong
const LUA_NUMBER_FRMLEN = ""
lua_str2number(s, p) = strtod(s, p)
const LUA_INTEGER_FRMLEN = "ll"
const LUAI_UACINT = LUA_INTEGER
lua_integer2str(s, sz, n) = l_sprintf(s, sz, LUA_INTEGER_FMT, LUAI_UACINT(n))
const LUA_UNSIGNED = unsigned(LUAI_UACINT)
const LUA_MAXINTEGER = LLONG_MAX
const LUA_MAXUNSIGNED = ULLONG_MAX
lua_pointer2str(buff, sz, p) = l_sprintf(buff, sz, "%p", p)
const LUA_KCONTEXT = ptrdiff_t
# Skipping MacroDefinition: lua_getlocaledecpoint ( ) ( localeconv ( ) -> decimal_point [ 0 ] )
luai_likely(x) = __builtin_expect(x != 0, 1)
luai_unlikely(x) = __builtin_expect(x != 0, 0)
const LUAI_MAXSTACK = 1000000
# Skipping MacroDefinition: LUA_EXTRASPACE ( sizeof ( void * ) )
const LUA_IDSIZE = 60
# Skipping MacroDefinition: LUAL_BUFFERSIZE ( ( int ) ( 16 * sizeof ( void * ) * sizeof ( lua_Number ) ) )
const LUA_VERSION_RELEASE = "4"
const LUA_VERSION_NUM = 504
const LUA_VERSION_RELEASE_NUM = LUA_VERSION_NUM * 100 + 4
const LUA_AUTHORS = "R. Ierusalimschy, L. H. de Figueiredo, W. Celes"
const LUA_SIGNATURE = "\eLua"
const LUA_MULTRET = -1
const LUA_REGISTRYINDEX = -LUAI_MAXSTACK - 1000
lua_upvalueindex(i) = LUA_REGISTRYINDEX - i
const LUA_OK = 0
const LUA_YIELD = 1
const LUA_ERRRUN = 2
const LUA_ERRSYNTAX = 3
const LUA_ERRMEM = 4
const LUA_ERRERR = 5
const LUA_TNONE = -1
const LUA_TNIL = 0
const LUA_TBOOLEAN = 1
const LUA_TLIGHTUSERDATA = 2
const LUA_TNUMBER = 3
const LUA_TSTRING = 4
const LUA_TTABLE = 5
const LUA_TFUNCTION = 6
const LUA_TUSERDATA = 7
const LUA_TTHREAD = 8
const LUA_NUMTYPES = 9
const LUA_MINSTACK = 20
const LUA_RIDX_MAINTHREAD = 1
const LUA_RIDX_GLOBALS = 2
const LUA_RIDX_LAST = LUA_RIDX_GLOBALS
const LUA_OPADD = 0
const LUA_OPSUB = 1
const LUA_OPMUL = 2
const LUA_OPMOD = 3
const LUA_OPPOW = 4
const LUA_OPDIV = 5
const LUA_OPIDIV = 6
const LUA_OPBAND = 7
const LUA_OPBOR = 8
const LUA_OPBXOR = 9
const LUA_OPSHL = 10
const LUA_OPSHR = 11
const LUA_OPUNM = 12
const LUA_OPBNOT = 13
const LUA_OPEQ = 0
const LUA_OPLT = 1
const LUA_OPLE = 2
lua_call(L, n, r) = lua_callk(L, n, r, 0, NULL)
lua_pcall(L, n, r, f) = lua_pcallk(L, n, r, f, 0, NULL)
lua_yield(L, n) = lua_yieldk(L, n, 0, NULL)
const LUA_GCSTOP = 0
const LUA_GCRESTART = 1
const LUA_GCCOLLECT = 2
const LUA_GCCOUNT = 3
const LUA_GCCOUNTB = 4
const LUA_GCSTEP = 5
const LUA_GCSETPAUSE = 6
const LUA_GCSETSTEPMUL = 7
const LUA_GCISRUNNING = 9
const LUA_GCGEN = 10
const LUA_GCINC = 11
# Skipping MacroDefinition: lua_getextraspace ( L ) ( ( void * ) ( ( char * ) ( L ) - LUA_EXTRASPACE ) )
lua_tonumber(L, i) = lua_tonumberx(L, i, NULL)
lua_tointeger(L, i) = lua_tointegerx(L, i, NULL)
lua_pop(L, n) = lua_settop(L, -n - 1)
lua_newtable(L) = lua_createtable(L, 0, 0)
lua_pushcfunction(L, f) = lua_pushcclosure(L, f, 0)
lua_register(L, n, f) = (lua_pushcfunction(L, f), lua_setglobal(L, n))
lua_isfunction(L, n) = lua_type(L, n) == LUA_TFUNCTION
lua_istable(L, n) = lua_type(L, n) == LUA_TTABLE
lua_islightuserdata(L, n) = lua_type(L, n) == LUA_TLIGHTUSERDATA
lua_isnil(L, n) = lua_type(L, n) == LUA_TNIL
lua_isboolean(L, n) = lua_type(L, n) == LUA_TBOOLEAN
lua_isthread(L, n) = lua_type(L, n) == LUA_TTHREAD
lua_isnone(L, n) = lua_type(L, n) == LUA_TNONE
lua_isnoneornil(L, n) = lua_type(L, n) <= 0
lua_pushliteral(L, s) = lua_pushstring(L, ("")(s))
lua_pushglobaltable(L) = (Cvoid(lua_rawgeti))(L, LUA_REGISTRYINDEX, LUA_RIDX_GLOBALS)
lua_tostring(L, i) = lua_tolstring(L, i, NULL)
lua_insert(L, idx) = lua_rotate(L, idx, 1)
lua_remove(L, idx) = (lua_rotate(L, idx, -1), lua_pop(L, 1))
lua_replace(L, idx) = (lua_copy(L, -1, idx), lua_pop(L, 1))
lua_newuserdata(L, s) = lua_newuserdatauv(L, s, 1)
lua_getuservalue(L, idx) = lua_getiuservalue(L, idx, 1)
lua_setuservalue(L, idx) = lua_setiuservalue(L, idx, 1)
const LUA_NUMTAGS = LUA_NUMTYPES
const LUA_HOOKCALL = 0
const LUA_HOOKRET = 1
const LUA_HOOKLINE = 2
const LUA_HOOKCOUNT = 3
const LUA_HOOKTAILCALL = 4
const LUA_MASKCALL = 1 << LUA_HOOKCALL
const LUA_MASKRET = 1 << LUA_HOOKRET
const LUA_MASKLINE = 1 << LUA_HOOKLINE
const LUA_MASKCOUNT = 1 << LUA_HOOKCOUNT
const LUA_GNAME = "_G"
const LUA_ERRFILE = LUA_ERRERR + 1
const LUA_LOADED_TABLE = "_LOADED"
const LUA_PRELOAD_TABLE = "_PRELOAD"
# Skipping MacroDefinition: LUAL_NUMSIZES ( sizeof ( lua_Integer ) * 16 + sizeof ( lua_Number ) )
luaL_checkversion(L) = luaL_checkversion_(L, LUA_VERSION_NUM, LUAL_NUMSIZES)
const LUA_NOREF = -2
const LUA_REFNIL = -1
luaL_loadfile(L, f) = luaL_loadfilex(L, f, NULL)
# Skipping MacroDefinition: luaL_newlibtable ( L , l ) lua_createtable ( L , 0 , sizeof ( l ) / sizeof ( ( l ) [ 0 ] ) - 1 )
luaL_newlib(L, l) = (luaL_checkversion(L), luaL_newlibtable(L, l), luaL_setfuncs(L, l, 0))
luaL_argcheck(L, cond, arg, extramsg) = Cvoid(luai_likely(cond) || luaL_argerror(L, arg, extramsg))
luaL_argexpected(L, cond, arg, tname) = Cvoid(luai_likely(cond) || luaL_typeerror(L, arg, tname))
luaL_checkstring(L, n) = luaL_checklstring(L, n, NULL)
luaL_optstring(L, n, d) = luaL_optlstring(L, n, d, NULL)
luaL_typename(L, i) = lua_typename(L, lua_type(L, i))
luaL_dofile(L, fn) = luaL_loadfile(L, fn) || lua_pcall(L, 0, LUA_MULTRET, 0)
luaL_getmetatable(L, n) = lua_getfield(L, LUA_REGISTRYINDEX, n)
luaL_opt(L, f, n, d) = if lua_isnoneornil(L, n)
d
else
f(L, n)
end
luaL_loadbuffer(L, s, sz, n) = luaL_loadbufferx(L, s, sz, n, NULL)
luaL_intop(op, v1, v2) = lua_Integer((((lua_Unsigned(v1))(op))(lua_Unsigned))(v2))
luaL_pushfail(L) = lua_pushnil(L)
lua_assert(c) = Cvoid(0)
luaL_bufflen(bf) = (bf->begin
#= none:1 =#
n
end)
luaL_buffaddr(bf) = (bf->begin
#= none:1 =#
b
end)
# Skipping MacroDefinition: luaL_addchar ( B , c ) ( ( void ) ( ( B ) -> n < ( B ) -> size || luaL_prepbuffsize ( ( B ) , 1 ) ) , ( ( B ) -> b [ ( B ) -> n ++ ] = ( c ) ) )
luaL_addsize(B, s) = (B->begin
#= none:1 =#
n += s
end)
luaL_buffsub(B, s) = (B->begin
#= none:1 =#
n -= s
end)
luaL_prepbuffer(B) = luaL_prepbuffsize(B, LUAL_BUFFERSIZE)
const LUA_FILEHANDLE = "FILE*"
lua_writestring(s, l) = fwrite(s, sizeof(Cchar), l, stdout)
lua_writeline() = (lua_writestring("\n", 1), fflush(stdout))
lua_writestringerror(s, p) = (fprintf(stderr, s, p), fflush(stderr))
const LUA_FUNCTION_WRAPPER_STATUS_NORMAL = 0
const LUA_FUNCTION_WRAPPER_STATUS_ERROR = 1
const LUA_FUNCTION_WRAPPER_STATUS_YIELD = 2
const LUA_COLIBNAME = "coroutine"
const LUA_TABLIBNAME = "table"
const LUA_IOLIBNAME = "io"
const LUA_OSLIBNAME = "os"
const LUA_STRLIBNAME = "string"
const LUA_UTF8LIBNAME = "utf8"
const LUA_MATHLIBNAME = "math"
const LUA_DBLIBNAME = "debug"
const LUA_LOADLIBNAME = "package"
# exports
const PREFIXES = ["lua_", "luaL_", "LUA_"]
for name in names(@__MODULE__; all=true), prefix in PREFIXES
if startswith(string(name), prefix)
@eval export $name
end
end
end # module
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 1986 | module LuaCall
include("../lib/LibLua.jl")
using .LibLua
export lualoadstring, luacall, LUA_STATE, top, @luascope, @luareturn
export LuaState, LuaTable, LuaUserData, LuaThread, LuaFunction
export get_julia, new_table!, get_uservalue, set_uservalue!
export new_userdata!, set_metatable!, get_metatable, pushstack!, getstack
export get_global, set_global!, get_globaltable, new_cfunction!
export iscfunction, mainthread, lualoadfile, registry, status, resume
export start
include("types.jl")
include("luascope.jl")
include("luastate.jl")
include("luadebug.jl")
include("luatable.jl")
include("luauserdata.jl")
include("luafunction.jl")
include("luathread.jl")
include("luastack.jl")
include("juliavalue.jl")
"""
lualoadstring([LS::LuaState,] str)
Evaluate the given Lua code, return the resulting function.
Lua treats individual chunk as body of an anonymous function,
you can pass arguments and get return values accordingly.
Example:
```julia
@luascope LS begin
f = lualoadstring(LS, s)
ret = f(args...; multiret=true)
@luareturn ret...
end
```
"""
function lualoadstring(LS::LuaState, str)
rc = luaL_loadstring(LS, str)
rc == LUA_OK || throw(LuaError(LS))
PopStack(LuaFunction(LS, -1), LS, 1)
end
lualoadstring(str) = lualoadstring(LUA_STATE, str)
"""
lualoadfile(LS::LuaState, filename, mode="bt")
Load a Lua script, return the parsed chunk.
"""
function lualoadfile(LS::LuaState, filename, mode="bt")
rc = luaL_loadfilex(LS, filename, mode)
rc == LUA_OK || throw(LuaError(LS))
PopStack(LuaFunction(LS, -1), LS, -1)
end
lualoadfile(filename, mode="bt") = lualoadfile(LUA_STATE, filename, mode)
function luacall(LS::LuaState, f::Symbol, args...; ka...)
g = get_global(LS, f) |> unwrap_popstack
@assert g isa LuaCallable
g(args...; ka...)
end
luacall(f::Symbol, args...; ka...) = luacall(LUA_STATE, f, args..., ka...)
const LUA_STATE = LuaStateWrapper(C_NULL)
function __init__()
init(LUA_STATE)
end
end
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 6239 |
const GC_ROOT = []
const GC_ROOT_FREE_LIST = Ref(0)
function add_object(@nospecialize x)
if GC_ROOT_FREE_LIST[] == 0
push!(GC_ROOT, x)
return length(GC_ROOT)
else
i = GC_ROOT_FREE_LIST[]
GC_ROOT_FREE_LIST[] = GC_ROOT[i]
GC_ROOT[i] = x
return i
end
end
function get_object(i)
GC_ROOT[i]
end
function del_object(i)
GC_ROOT[i] = GC_ROOT_FREE_LIST[]
GC_ROOT_FREE_LIST[] = i
end
# Return number of objects remaining
function check_gc_root()
free = 0
node = GC_ROOT_FREE_LIST[]
while node != 0
node = GC_ROOT[node]
free += 1
end
length(GC_ROOT) - free
end
function lua_gc(L::LuaState)::Cint
ptr = lua_touserdata(L, -1)
idx = unsafe_load_gc_index(ptr)
del_object(idx)
return 0
end
"""
const JULIA_IDENTITY = "__julia"
This field identifies a Julia object from other Lua userdata.
Call `get_julia` to convert such Lua userdata to a Julia object.
Implementation detail:
LuaCall place all Julia objects that are passed to Lua in a global
array, and Lua userdata store an index into the array. The index
is freed by `__gc` callback when Lua frees the userdata.
"""
const JULIA_IDENTITY = "__julia"
const JULIA_IDENTITY_OBJECT = 1
const JULIA_IDENTITY_MODULE = 2
#=
Lua `for key, other1, other2 in exp do ...body... end` is implemented as:
```lua
iterate_func, iterable, key, to_be_closed = exp
while true
key, other1, other2 = iterate_func(iterable, key)
if key == nil then
break
end
...body...
end
```
=#
function lua_iterate(iter, state)
ret = if isnothing(state)
iterate(iter)
else
iterate(iter, state)
end
if isnothing(ret)
nothing
else
MultipleReturn((ret[2], ret[1]))
end
end
lua_index(iter, key) = iter[key]
lua_newindex(table, key, value) = setindex!(table, value, key)
lua_call(f, args...) = f(args...)
lua_length(x) = length(x)
lua_tostring(x) = string(x)
lua_idiv(x, y) = x ÷ y
lua_concat(x, y) = error("Lua concat operator `..` is not defined for $(typeof(x)) and $(typeof(y))")
# @luacall does not work behind macros
macro mirror_method(lua_name, julia_name, nargs=2)
esc(quote
f = new_cfunction!(LS, @lua_CFunction LuaFunctionWrapper($julia_name, $nargs)) |> unwrap_popstack
t[$(string(lua_name))] = wrapper(f) |> unwrap_popstack
end)
end
const JULIA_METATABLE_OBJECT = "julia_metatable_object"
function init_julia_value_metatable(LS::LuaState)
@luascope LS begin
t = new_table!(LS)
registry(LS)[JULIA_METATABLE_OBJECT] = t
t[JULIA_IDENTITY] = JULIA_IDENTITY_OBJECT
# Not wrapped
f = new_cfunction!(LS, @lua_CFunction lua_gc)
t["__gc"] = f
wrapper = lualoadstring(LS, get_julia_function_wrapper(1))
@mirror_method __add (+)
@mirror_method __sub (-)
@mirror_method __mul (*)
@mirror_method __div (/)
@mirror_method __mod (%)
@mirror_method __pow (^)
@mirror_method __unm (-) 1
@mirror_method __idiv lua_idiv
@mirror_method __band (&)
@mirror_method __bor (|)
@mirror_method __bxor (⊻)
@mirror_method __bnot (~) 1
@mirror_method __shl (<<)
@mirror_method __shr (>>)
@mirror_method __concat lua_concat
@mirror_method __len lua_length 1
@mirror_method __eq (==)
@mirror_method __lt (<)
@mirror_method __le (<=)
@mirror_method __index lua_index
@mirror_method __newindex lua_newindex 3
@mirror_method __call lua_call -1
@mirror_method __tostring lua_tostring 1
pairs_wrapper_code = """
local iter, state = ...
return function (self)
return iter, self --, nil, nil
end
"""
pairs_wrapper = lualoadstring(LS, pairs_wrapper_code)
f = new_cfunction!(LS, @lua_CFunction LuaFunctionWrapper(pairs, 1))
julia_pairs = wrapper(f)
wrapper3 = lualoadstring(LS, get_julia_function_wrapper(3))
f = new_cfunction!(LS, @lua_CFunction LuaFunctionWrapper(lua_iterate, 2))
julia_next = wrapper3(f)
pairs_wrapped = pairs_wrapper(julia_next, julia_pairs)
t["__pairs"] = pairs_wrapped
end
end
const JULIA_METATABLE_MODULE = "julia_metatable_module"
julia_getproperty(x, f) = getproperty(x, Symbol(f))
julia_setproperty(x, f, v) = setproperty!(x, Symbol(f), v)
# I don't like so many mapped methods for modules
function init_julia_module_metatable(LS::LuaState)
@luascope LS begin
t = new_table!(LS; ndict=4)
registry(LS)[JULIA_METATABLE_MODULE] = t
t[JULIA_IDENTITY] = JULIA_IDENTITY_MODULE
t["__gc"] = new_cfunction!(LS, @lua_CFunction lua_gc)
wrapper = lualoadstring(LS, get_julia_function_wrapper(1))
f = new_cfunction!(LS, @lua_CFunction LuaFunctionWrapper(julia_getproperty, 2))
t.__index = wrapper(f)
f = new_cfunction!(LS, @lua_CFunction LuaFunctionWrapper(julia_setproperty, 3))
t.__newindex = wrapper(f)
f = new_cfunction!(LS, @lua_CFunction LuaFunctionWrapper(string, 1))
t.__str = wrapper(f)
end
end
unsafe_store_gc_index!(ptr, idx) = unsafe_store!(Ptr{Int}(ptr), idx)
unsafe_load_gc_index(ptr) = unsafe_load(Ptr{Int}(ptr))
function push_julia_object!(LS::LuaState, obj)
idx = add_object(obj)
ptr = lua_newuserdatauv(LS, sizeof(Int), 0)
unsafe_store_gc_index!(ptr, idx)
luaL_setmetatable(LS, JULIA_METATABLE_OBJECT)
PopStack(LuaUserData(LS, -1), LS, 1)
end
function pushstack!(LS::LuaState, mod::Module)
push_julia_object!(LS, mod)
luaL_setmetatable(LS, JULIA_METATABLE_MODULE)
PopStack(LuaUserData(LS, -1), LS, 1)
end
function pushstack!(LS::LuaState, obj)
push_julia_object!(LS, obj)
end
get_julia(x) = x
"""
get_julia(ud::LuaUserData)
Try to identify and convert a Lua userdata value to its Julia value.
Return `ud` if failed.
"""
function get_julia(ud::LuaUserData)
t = luaL_getmetafield(LS(ud), idx(ud), JULIA_IDENTITY)
t == LUA_TNIL && return ud
pop!(LS(ud), 1)
i = unsafe_load_gc_index(get_userdata(ud))
get_object(i)
end
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 2856 |
function LuaError(msg::AbstractString)
LuaError(msg, [])
end
function LuaError(LS::LuaState)
msg = LS[] |> get_julia
pop!(LS, 1)
stack = get_stacktrace(LS)
set_stacktrace!(LS, [])
LuaError(msg, stack)
end
function Base.showerror(io::IO, ex::LuaError)
println(io, "LuaError: ", ex.msg)
if ex.stacktrace |> !isempty
for frame in ex.stacktrace
println(io, frame)
end
end
end
@noinline function get_julia_stacktrace(offset)
anchor_frame = stacktrace()[offset]
full_julia_stack = catch_backtrace()
anchor = findfirst(full_julia_stack) do x
for f in StackTraces.lookup(x)
f == anchor_frame && return true
end
false
end
if isnothing(anchor)
StackTraces.StackTrace()
else
julia_stack = full_julia_stack[1:anchor-1]
stacktrace(julia_stack)
end
end
Base.@kwdef struct LuaStackFrame
name::String
namewhat::String
what::String
source::String # short source
currentline::Int
istailcall::Bool
# parameter names or "..." for vararg
params::Vector{String}
end
function Base.show(io::IO, f::LuaStackFrame)
print(io, '[', f.what, "] ")
if f.namewhat != ""
print(io, f.namewhat, ' ')
end
print(io, f.name, '(', join(f.params, ", "), ')')
print(io, " at ", f.source, ':', f.currentline)
if f.istailcall
print(io, " [tailcall]")
end
end
function get_param_names(LS::LuaState, ar::Ref{lua_Debug})
nparams = ar[].nparams
[unsafe_string(lua_getlocal(LS, ar, i)) for i in 1:nparams]
end
function get_lua_stacktrace(LS::LuaState)
level = 0
info = Ref{lua_Debug}()
lua_stack = LuaStackFrame[]
while lua_getstack(LS, level, info) |> !iszero
level += 1
lua_getinfo(LS, "nSlut", info)
name_ptr = info[].name
name = name_ptr == C_NULL ? "(anonymous)" : unsafe_string(name_ptr)
namewhat = unsafe_string(info[].namewhat)
what = unsafe_string(info[].what)
buf = collect(mod.(info[].short_src, UInt8))
GC.@preserve buf source = unsafe_string(pointer(buf))
params = get_param_names(LS, info)
if info[].isvararg |> !iszero
push!(params, "...")
end
frame = LuaStackFrame(;
name,
namewhat,
what,
source,
info[].currentline,
istailcall = !iszero(info[].istailcall),
params)
push!(lua_stack, frame)
end
lua_stack
end
@noinline function store_stacktrace(LS::LuaState)
# We may be unwinding a stack from a coroutine
wrapper = get_julia_wrapper(LS)
get_debug(wrapper) || return
julia_stack = get_julia_stacktrace(5)
lua_stack = get_lua_stacktrace(LS)
set_stacktrace!(wrapper, [julia_stack; lua_stack])
end
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 4470 |
const LuaCallable = Union{LuaFunction,LuaTable,LuaUserData}
iscfunction(f::LuaFunction) = lua_iscfunction(LS(f), idx(f)) |> !iszero
"""
(f::LuaCallable)(args...; multiret=false, stacktrace=nothing)
Call the Lua function.
By default only the first return value is used,
pass `multiret=true` to collect all return values.
If `stacktrace` is not `nothing`, specify stacktrace to be collected
or not in case of error for the current call. Otherwise the setting on
the Lua state wrapper will be used.
"""
function (F::LuaCallable)(args...; multiret=false, stacktrace=nothing)
call(F, args...; multiret, stacktrace)
end
function call(F::LuaCallable, args...; multiret=false, stacktrace=nothing)
push!(LS(F), F)
pcall(LS(F), args...; multiret, stacktrace)
end
"Pop and call the function at the top of the stack with `args`."
function pcall(LS::LuaState, args...; multiret=false, stacktrace::Union{Nothing,Bool}=nothing)
@assert lua_isfunction(LS, -1)
old_top = top(LS) - 1
push!(LS, args...)
isnothing(stacktrace) || (old_debug = set_debug!(LS, stacktrace))
rc = lua_pcall(LS, length(args), multiret ? -1 : 1, 0)
isnothing(stacktrace) || set_debug!(LS, old_debug)
rc == LUA_OK || throw(LuaError(LS))
nret = top(LS) - old_top
return_on_lua_stack(LS, multiret ? (-nret:-1) : -1)
end
"""
new_cfunction!(LS::LuaState, f::Ptr{Cvoid}, upvalues...)
Push a C function to the Lua stack and return it.
You may optionally associate upvalues with it, which can be accessed
in the function with pseudo-index `lua_upvalueindex`.
"""
function new_cfunction!(LS::LuaState, f::Ptr{Cvoid}, upvalues...)
checkstack(LS, length(upvalues) + 1)
@inbounds push!(LS, upvalues...)
lua_pushcclosure(LS, f, length(upvalues))
PopStack(LuaFunction(LS, -1), LS, 1)
end
struct MultipleReturn
ret::Any
end
"""
LuaFunctionWrapper{func,narg}
Wrap a Julia `@cfunction` into a Lua function which handles error and yield.
Return a single value unless the return values is wrapped in `MultipleReturn`.
To avoid jumping out of `@cfunction`, this function returns a status code
(0 for normal return, 1 for error, 2 for yield), and the real return is handled
by Lua code. See `get_julia_function_wrapper`.
TODO: Multiple return need an C wrapper.
"""
struct LuaFunctionWrapper{func,narg} end
LuaFunctionWrapper(f, narg) = LuaFunctionWrapper{f,narg}()
function (::LuaFunctionWrapper{func,narg})(LS::Ptr{lua_State})::Cint where {func,narg}
cur_top = top(LS)
if narg > 0 && cur_top < narg
push!(LS, 1, "Not enough arguments for $func: expect $narg, got $cur_top")
return 2
end
try
args = (get_julia(LS[i]) for i in 1:cur_top)
ret = func(args...)
if ret isa MultipleReturn
ret = ret.ret
push!(LS, 0, ret...)
return 1 + length(ret)
else
push!(LS, 0, ret)
return 2
end
catch e
store_stacktrace(LS)
@debug "Error occurred when Lua calls Julia function `$func`" exception = (e, catch_backtrace())
# `lua_error` is implemented as longjmp that jump out of this @cfunction
# which is not allowed. So we simply return nothing and store the error
msg = sprint(showerror, e)
push!(LS, 1, msg)
return 2
end
end
"""
@lua_CFunction
Shorthand for `@cfunction \$func Cint (Ptr{lua_State},)`.
The Lua API requires that this function has single argument `LS::Ptr{lua_State}`.
Arguments are everything on the stack, upvalues can be accessed with `lua_upvalueindex`
pseudo index on the stack. Return values are simply pushed to the stack, and this function
return the number of return values.
"""
macro lua_CFunction(func)
quote
@cfunction $func Cint (Ptr{lua_State},)
end
end
function get_julia_function_wrapper(nret=1)
rets = join(("ret$i" for i in 1:nret), ", ")
"""
-- lua wrapper for $nret-return julia function
local f = ...
return function (...)
status, $rets, k = f(...)
if status == 0 then
return $rets
elseif status == 1 then
error(ret1, 0)
elseif status == 2 then
arg = coroutine.yield($rets)
-- Lua implements proper tail calls
return julia_call_handler(k, arg)
end
end
"""
end
function kw(f, kw, args...)
kw = (Symbol(k) => v for (k,v) in kw)
f(args...; kw...)
end
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 2804 |
unwrap_popstack(x) = x
unwrap_popstack(x::PopStack) = x.data
function luareturn_impl(LS::LuaState, old_base, vars...)
new_base = Ref(old_base)
moved = map(vars) do x
if x isa OnLuaStack
new_base[] += 1
typeof(x)(LS, new_base[])
else
x
end
end
lua_rotate(LS, old_base + 1, new_base[] - old_base)
new_base[], moved
end
function luascope_impl(LS_expr, code::Expr)
@assert code.head == :block
LS = gensym(:LS)
base = gensym(:base)
body = []
for expr in code.args
if expr isa Expr && expr.head == :(=)
left, right = expr.args
if left isa Expr && left.head == :(::)
right = :($right::$PopStack{$(left.args[2])})
left = left.args[1]
end
push!(body, :($left = $unwrap_popstack($right)))
elseif expr isa Expr && expr.head == :macrocall &&
expr.args[1] == Symbol("@luareturn")
vars = expr.args[3:end]
nvars = length(vars)
ret = gensym(:ret)
ret_expr = nvars == 1 ? :(only($ret)) : ret
push!(body, quote
$base, $ret = $luareturn_impl($LS, $base, $(vars...))
$PopStack($ret_expr, $LS, $nvars)
end)
else
push!(body, expr)
end
end
quote
$LS = $LS_expr
$base = $top($LS)
try
$(body...)
finally
$lua_settop($LS, $base)
end
end |> esc
end
"""
@luascope
Manage Lua stack for all top-level expressions in this block. Usage:
```julia
LS = LUA_STATE
@luascope LS begin
t::LuaTable = new_table!(LS)
t[1] = 2
@luareturn t
end
```
In the above example, `getglobal` and `j["Base"]` both push value to the Lua stack and return `PopStack` values.
To account for this, `@luascope` detects top-level assignment `var = expr`, where `expr` evaluates to a `PopStack` value
to unwrap the value.
After control leaves the block, the Lua stack is reset, destroying all lua variables.
If you want to return Lua values, call `@luareturn` at the end of the block.
!!! warning
This macro does not free Lua stack inside the block, so a loop that repetitively pushes Lua value may run out of stack space.
"""
macro luascope(LS, code::Expr)
luascope_impl(LS, code)
end
"""
@luareturn v1 v2...
Mark `v1, v2...` as Julia return value, they will be kept on the Lua stack.
Assume each value occupy one Lua stack slot.
Manage Lua stack yourself if you want to use multiple return values.
!!! warning
This macro will corrupt the current Lua stack, thus invalidating any existing values.
"""
macro luareturn(args...)
error("`@luareturn` is only a placeholder, see `@luascope.`")
end
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 3304 | """
checkstack(LS::LuaState, n::Integer)
Ensure the stack has additional space for `n` elements.
You should call this function before you call any `lua[L]_` function that may push elements to the stack.
"""
function checkstack(LS::LuaState, n::Integer=1)
rc = lua_checkstack(LS, n)
iszero(rc) && throw(LuaError("insufficient space on the Lua stack for $n items"))
nothing
end
function to_julia_type(LS, idx, typeind)
if typeind == LUA_TNONE
Missing
elseif typeind == LUA_TNIL
Nothing
elseif typeind == LUA_TBOOLEAN
Bool
elseif typeind == LUA_TLIGHTUSERDATA
Ptr{Cvoid}
elseif typeind == LUA_TNUMBER
!iszero(lua_isinteger(LS, idx)) ? LuaInt : LuaFloat
elseif typeind == LUA_TSTRING
String
elseif typeind == LUA_TTABLE
LuaTable
elseif typeind == LUA_TFUNCTION
LuaFunction
elseif typeind == LUA_TUSERDATA
LuaUserData
elseif typeind == LUA_TTHREAD
LuaThread
else
error("Unsupported Lua type: ", unsafe_string(lua_typename(LS, typeind)))
end
end
getstack(LS::LuaState, idx, ::Type{Missing}) = missing
getstack(LS::LuaState, idx, ::Type{Nothing}) = nothing
getstack(LS::LuaState, idx, ::Type{Bool}) = lua_toboolean(LS, idx)
getstack(LS::LuaState, idx, ::Type{<:Integer}) = lua_tointeger(LS, idx)
getstack(LS::LuaState, idx, ::Type{<:AbstractFloat}) = lua_tonumber(LS, idx)
getstack(LS::LuaState, idx, ::Type{Ptr{Cvoid}}) = lua_touserdata(LS, idx)
getstack(LS::LuaState, idx, T::Type{<:OnLuaStack}) = T(LS, idx)
function getstack(LS::LuaState, idx, ::Type{<:AbstractString})
len = Ref{Csize_t}()
ptr = lua_tolstring(LS, idx, len)
unsafe_string(ptr, len[])
end
"""
getstack(LS::LuaState, idx=-1, typeind)
Get the value at the given Lua stack index. Does not modify the Lua stack.
"""
getstack(LS::LuaState, idx=-1, typeind::Integer=lua_type(LS, idx)) = getstack(LS, idx, to_julia_type(LS, idx, typeind))
"""
pushstack!(LS::LuaState, x)
Push a value to the Lua stack and return it.
"""
function pushstack! end
pushstack!(LS::LuaState, ::Nothing) = lua_pushnil(LS)
pushstack!(LS::LuaState, x::Bool) = lua_pushboolean(LS, x)
pushstack!(LS::LuaState, x::AbstractFloat) = lua_pushnumber(LS, x)
pushstack!(LS::LuaState, x::Integer) = lua_pushinteger(LS, x)
pushstack!(LS::LuaState, x::AbstractString) = lua_pushlstring(LS, x, length(x))
pushstack!(LS::LuaState, x::Ptr) = lua_pushlightuserdata(LS, x)
pushstack!(LS::LuaState, x::Symbol) = pushstack!(LS, string(x))
pushstack!(_::LuaState, _::PopStack) = error("You should not push PopStack")
function stackdump(LS::LuaState)
[LS[i] for i in 1:top(LS)]
end
function pushstack!(to::LuaState, x::OnLuaStack)
if to == LS(x)
lua_pushvalue(to, idx(x))
else
lua_pushvalue(LS(x), idx(x))
lua_xmove(LS(x), to, 1)
end
end
function return_on_lua_stack(LS::LuaState, idx::Integer, typeind=lua_type(LS, idx))
x = getstack(LS, idx, typeind)
if x isa OnLuaStack
PopStack(x, LS, 1)
else
pop!(LS, 1)
PopStack(x, LS, 0)
end
end
function return_on_lua_stack(LS::LuaState, range::AbstractVector)
PopStack([getstack(LS, i) for i in range], LS, length(range))
end
Base.getindex(pop::PopStack) = unwrap_popstack(pop)
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 3741 |
function LuaStateWrapper(L::Ptr, debug=true)
return LuaStateWrapper(LuaThread(L), debug, [])
end
"""
LuaState(julia_module=Main, debug=true)
Create a new Lua state, with `julia_module` set to Lua global varaible `julia`
or not set if `nothing` is passed.
If `debug` is true, stacktrace will be stored for every exception.
You can also set debug on a per-call basis.
"""
function LuaStateWrapper(julia_module::Union{Nothing,Module}, debug=true)
LS = LuaStateWrapper(LuaThread(C_NULL), debug, [])
init(LS; julia_module)
LS
end
LuaState(julia_module::Union{Nothing,Module}=Main, debug=true) = LuaStateWrapper(julia_module, debug)
"Push values to the stack. This function also calls `lua_checkstack` as `@boundscheck`."
@inline function Base.push!(LS::LuaState, xs...)
@boundscheck checkstack(LS, length(xs))
for x in xs
pushstack!(LS, x)
end
end
Base.pop!(LS::LuaState, n) = lua_pop(LS, n)
top(LS::LuaState) = lua_gettop(LS)
Base.getproperty(LS::LuaState, f::Symbol) = get_global(LS, f)
Base.setproperty!(LS::LuaState, f::Symbol, x) = set_global!(LS, f, x)
Base.getindex(LS::LuaState, i=-1) = getstack(LS, i)
function get_global(LS::LuaState, f)
checkstack(LS, 1)
lua_getglobal(LS, f)
return_on_lua_stack(LS, -1)
end
function set_global!(LS::LuaState, f, v)
push!(LS, v)
lua_setglobal(LS, f)
end
function get_globaltable(LS::LuaState)
registry(LS)[LUA_RIDX_GLOBALS]
end
function mainthread(LS::LuaState)
@luascope LS begin
t::LuaThread = registry(LS)[LUA_RIDX_MAINTHREAD]
t
end
end
mainthread(LS::LuaStateWrapper) = getfield(LS, :L)
"""
registry(LS::LuaState)
Get the Lua registry.
"""
registry(LS::LuaState) = LuaTable(LS, LUA_REGISTRYINDEX)
function get_metatable(obj::Union{LuaTable,LuaUserData})
@assert lua_getmetatable(LS(obj), idx(obj)) == 1
PopStack(LuaTable(LS(obj), -1), LS(obj), 1)
end
"""
set_metatable!(obj::Union{LuaTable,LuaUserData}, table)
Set metatable for `obj`, if `table` is `nothing`, unset the metatable.
"""
function set_metatable!(obj::Union{LuaTable,LuaUserData}, table::LuaTable)
push!(LS(obj), table)
lua_setmetatable(LS(obj), idx(obj))
end
function lua_getextraspace(L::LuaState)
L = Base.unsafe_convert(Ptr{lua_State}, L)
Ptr{Cvoid}(L - sizeof(Ptr{Cvoid}))
end
function set_julia_wrapper!(LS::LuaStateWrapper)
space = lua_getextraspace(LS)
unsafe_store!(Ptr{Any}(space), LS)
end
function get_julia_wrapper(L::Ptr{lua_State})
space = lua_getextraspace(L)
unsafe_load(Ptr{Any}(space))::LuaStateWrapper
end
get_julia_wrapper(LS::LuaThread) = get_julia_wrapper(Base.unsafe_convert(Ptr{lua_State}, LS))
get_julia_wrapper(LS::LuaStateWrapper) = LS
get_debug(LS::LuaStateWrapper) = getfield(LS, :debug)
function set_debug!(LS::LuaStateWrapper, enable::Bool)
old_debug = get_debug(LS)
setfield!(LS, :debug, enable)
old_debug
end
get_stacktrace(LS::LuaStateWrapper) = getfield(LS, :stacktrace)
set_stacktrace!(LS::LuaStateWrapper, stacktrace) = setfield!(LS, :stacktrace, stacktrace)
for func in [:get_debug, :set_debug!, :get_stacktrace, :set_stacktrace!]
@eval $func(L::LuaState, args...) = $func(get_julia_wrapper(L), args...)
end
function Base.close(LS::LuaStateWrapper)
lua_close(LS)
setfield!(LS, :L, LuaThread(C_NULL))
end
function init(LS::LuaStateWrapper; julia_module=Main)
L = luaL_newstate()
L == C_NULL && error("Failed to initialize Lua")
setfield!(LS, :L, LuaThread(L))
set_julia_wrapper!(LS)
finalizer(close, LS)
luaL_openlibs(LS)
init_julia_value_metatable(LS)
init_julia_module_metatable(LS)
@luascope LS begin
set_global!(LS, "julia", julia_module)
end
end
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 3379 |
function Base.getindex(T::LuaTable, k)
push!(LS(T), k)
t = lua_gettable(LS(T), idx(T))
return_on_lua_stack(LS(T), -1, t)
end
function Base.getindex(T::LuaTable, k::AbstractString)
t = lua_getfield(LS(T), idx(T), k)
return_on_lua_stack(LS(T), -1, t)
end
function Base.getindex(T::LuaTable, k::Integer)
t = lua_geti(LS(T), idx(T), k)
return_on_lua_stack(LS(T), -1, t)
end
# Map property to index, what Lua `T.f` does
Base.getproperty(T::LuaTable, f::Symbol) = T[string(f)]
Base.setproperty!(T::LuaTable, f::Symbol, v) = T[string(f)] = v
"""
rawget(T::LuaTable, k)
Get table data with raw access.
"""
function rawget(T::LuaTable, k)
push!(LS(T), k)
t = lua_rawget(LS(T), idx(T))
return_on_lua_stack(LS(T), -1, t)
end
function rawget(T::LuaTable, k::Integer)
t = lua_rawgeti(LS(T), idx(T), k)
return_on_lua_stack(LS(T), -1, t)
end
function rawget(T::LuaTable, k::Ptr)
t = lua_rawgetp(LS(T), idx(T), k)
return_on_lua_stack(LS(T), -1, t)
end
function Base.setindex!(T::LuaTable, v, k)
push!(LS(T), k, v)
lua_settable(LS(T), idx(T))
end
function Base.setindex!(T::LuaTable, v, k::AbstractString)
# lua_setfield may implicitly push a new field, what???
checkstack(LS(T), 2)
@inbounds push!(LS(T), v)
lua_setfield(LS(T), idx(T), k)
end
function Base.setindex!(T::LuaTable, v, k::Integer)
push!(LS(T), v)
lua_seti(LS(T), idx(T), k)
end
"""
rawset(T::LuaTable, k, v)
Set table data with raw access.
"""
function rawset!(T::LuaTable, k, v)
push!(LS(T), k, v)
lua_rawset(LS(T), idx(T))
end
function rawset!(T::LuaTable, k::Integer, v)
push!(LS(T), v)
lua_rawseti(LS(T), idx(T), k)
end
function rawset!(T::LuaTable, k::Ptr, v)
push!(LS(T), v)
lua_rawsetp(LS(T), idx(T), k)
end
"""
new_table!(LS::LuaState; narr=0, ndict=0)
new_table!(LS::LuaState, dict::AbstractDict; narr=0, ndict=0)
new_table(LS::LuaState, arr::AbstractArray; narr=0, ndict=0)
Create a Lua table and push it to the stack. Also opy data from `dict` or `arr` into the table.
`narr` and `ndict` are hint for number of sequential elements and other elements.
"""
function new_table!(LS::LuaState; narr=0, ndict=0)
checkstack(LS, 1)
lua_createtable(LS, narr, ndict)
PopStack(LuaTable(LS, -1), LS, 1)
end
function new_table!(LS::LuaState, dict::AbstractDict; narr=0, ndict=0)
t = new_table!(LS; narr, ndict=ndict != 0 ? ndict : length(dict)) |> unwrap_popstack
for (k, v) in pairs(dict)
t[k] = v
end
PopStack(t, LS, 1)
end
function new_table!(LS::LuaState, arr::AbstractArray; narr=0, ndict=0)
t = new_table!(LS; narr=narr != 0 ? narr : length(arr), ndict) |> unwrap_popstack
for (k, v) in enumerate(arr)
t[k] = v
end
PopStack(t, LS, 1)
end
const LuaIterable = Union{LuaTable,LuaUserData}
function Base.iterate(t::LuaTable)
checkstack(LS(t), 2)
@inbounds push!(LS(t), nothing)
if !iszero(lua_next(LS(t), idx(t)))
(getstack(LS(t), -2), getstack(LS(t), -1)), top(LS(t))
end
end
function Base.iterate(t::LuaTable, last_top::Cint)
@assert top(LS(t)) == last_top
pop!(LS(t), 1)
if !iszero(lua_next(LS(t), idx(t)))
(getstack(LS(t), -2), getstack(LS(t), -1)), top(LS(t))
end
end
function Base.length(t::Union{LuaTable,LuaUserData})
lua_rawlen(LS(t), idx(t))
end
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 1469 | """
new_thread!(LS::LuaState, main::Union{LuaCallable,Nothing}=nothing)
Create a Lua thread(coroutine).
The main function must be pushed before resuming, call `start` if you
did not set `main` on invokation.
"""
function new_thread!(LS::LuaState, main::Union{LuaCallable,Nothing}=nothing)
t = LuaThread(lua_newthread(LS))
isnothing(main) || start(t, main)
t
end
function status(t::LuaThread)
lua_status(t)
end
function start(t::LuaThread, main::LuaCallable)
push!(t, main)
end
"""
resume(host::LuaState, guest::LuaThread, args...; multiret=false, stacktrace=nothing)
Resume a thread `guest` with arguments `args`. The results will be moved to `host`. Keyword arguments
are similar to `(::LuaFunction)(...)`.
"""
function resume(host::LuaState, guest::LuaThread, args...; multiret=false, stacktrace=nothing)
host = get_julia_wrapper(host)
push!(guest, args...)
nresults = Ref{Cint}()
isnothing(stacktrace) || (old_debug = set_debug!(host, stacktrace))
status = lua_resume(guest, host, length(args), nresults)
isnothing(stacktrace) || set_debug!(host, old_debug)
if status == LUA_OK || status == LUA_YIELD
checkstack(host, nresults[])
lua_xmove(guest, host, nresults[])
return_on_lua_stack(host, multiret ? (-nresults[]:-1) : -1)
else
throw(LuaError(guest))
end
end
function getstack(LS::LuaState, idx, ::Type{LuaThread})
LuaThread(lua_tothread(LS, idx))
end
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 625 |
function get_userdata(UD::LuaUserData)
lua_touserdata(LS(UD), idx(UD))
end
function get_uservalue(UD::LuaUserData, i)
t = lua_getiuservalue(LS(UD), idx(UD), i)
return_on_lua_stack(LS(UD), -1, t)
end
function set_uservalue!(UD::LuaUserData, i, v)
push!(LS(UD), v)
lua_setiuservalue(LS(UD), idx(UD), i)
end
"""
new_userdata!(LS::LuaState, size, nuservalue=0)
Create a userdata with given number of bytes and given number of uservalue.
"""
function new_userdata!(LS::LuaState, size, nuservalue=0)
lua_newuserdatauv(LS, size, nuservalue)
UD = LuaUserData(LS, -1)
PopStack(UD, LS, 1)
end
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 3455 | # Directly added to the artifact
@assert LibLua.jl_lua_int_type() == LUA_INT_TYPE
@assert LibLua.jl_lua_float_type() == LUA_FLOAT_TYPE
const LuaInt = let t = LUA_INT_TYPE
t == 1 ? Cint :
t == 2 ? Clong :
t == 3 ? Clonglong :
error("unknown Lua integer type")
end
const LuaFloat = let t = LUA_FLOAT_TYPE
t == 1 ? Cfloat :
t == 2 ? Cdouble :
t == 3 ? error("Julia does not natively support long doubles") :
error("unknown Lua float type")
end
"""
OnStackType
Values of this type are valid only for the current Lua stack frame. For safety, they are always wrapped in a `PopStack` wrapper.
"""
abstract type OnLuaStack end
struct LuaThread <: OnLuaStack
L::Ptr{lua_State}
end
Base.unsafe_convert(::Type{Ptr{lua_State}}, LS::LuaThread) = getfield(LS, :L)
mutable struct LuaStateWrapper
L::LuaThread
debug::Bool
stacktrace::Vector{Any}
end
Base.unsafe_convert(::Type{Ptr{lua_State}}, LS::LuaStateWrapper) = Base.unsafe_convert(Ptr{lua_State}, getfield(LS, :L))
"""
LuaState
Julia interface for `lua_State` (or `LuaStateWrapper`). Supports access to the Lua stack and Lua global variabls.
Low-level stack interface:
- `pop!(LS, n)`: pop `n` elements from the Lua stack.
- `top(LS)`: maximum index for the stack.
High-level stack interface:
- `LS[idx]` or `getstack(LS, idx)`: get Julia wrapper for element at stack position `idx`.positive `idx` if count from the bottom (1),
negative `idx` if count from the top(-1). This function does not mutate any Lua state.
- `pushstack(LS, x)` push `x` to the Lua stack and return its Julia wrapper.
- `push!(LS, args...)`: push `args...` to the Lua stack.
Global variable interface:
- `LS.var` or `get_global(LS, :var)`: push global variable `var` to the Lua stack.
- `LS.var = x` or `set_gloabl!(LS, :var, x)`: set global variable `var` to `x`.
"""
const LuaState = Union{Ptr{lua_State},LuaStateWrapper,LuaThread}
struct LuaError <: Exception
msg::String
stacktrace::Vector{Any}
end
LS(x::OnLuaStack) = getfield(x, :LS)
idx(x::OnLuaStack) = getfield(x, :idx)
"""
PopStack{T}
A data type to protect from passing Values on the Lua stack around carelessly.
You should either take care to restore Lua stack, or use `@luascope`
"""
struct PopStack{T,StateT<:LuaState}
data::T
LS::StateT
npop::Cint
end
PopStack(data, LS, npop) = PopStack{typeof(data),typeof(LS)}(data, LS, npop)
struct LuaFunction{StateT<:LuaState} <: OnLuaStack
LS::StateT
idx::Cint
LuaFunction{StateT}(LS::StateT, idx) where {StateT<:LuaState} = new{StateT}(LS, idx > 0 ? idx : lua_absindex(LS, idx))
end
LuaFunction(LS::LuaState, idx) = LuaFunction{typeof(LS)}(LS, idx)
struct LuaTable{StateT<:LuaState} <: OnLuaStack
LS::StateT
idx::Cint
LuaTable{StateT}(LS::StateT, idx) where {StateT<:LuaState} = new{StateT}(LS, idx > 0 ? idx : lua_absindex(LS, idx))
end
LuaTable(LS::LuaState, idx) = LuaTable{typeof(LS)}(LS, idx)
struct LuaUserData{StateT<:LuaState} <: OnLuaStack
LS::StateT
idx::Cint
LuaUserData{StateT}(LS::StateT, idx) where {StateT<:LuaState} = new{StateT}(LS, idx > 0 ? idx : lua_absindex(LS, idx))
end
LuaUserData(LS::LuaState, idx) = LuaUserData{typeof(LS)}(LS, idx)
function Base.:(==)(obj1::OnLuaStack, obj2::OnLuaStack)
LS(obj1) == LS(obj2) || return false
lua_rawequal(LS(obj1), idx(obj1), idx(obj2)) |> !iszero
end
function Base.:(==)(t1::LuaThread, t2::LuaThread)
t1 === t2
end
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | code | 7959 | using LuaCall
using Test
@testset "JuliaModule" begin
@luascope LUA_STATE begin
A = [2 1; 8 9]
B1 = inv(A)
lua_inv = lualoadstring("return julia.inv(...)")
B2 = lua_inv(A)
@test B1 == get_julia(B2)
C1 = A + A
lua_add = lualoadstring("local A = ...; return A + A")
C2 = lua_add(A)
@test C1 == get_julia(C2)
D1 = A^2
lua_square = lualoadstring("local A = ...; return A ^ 2")
D2 = lua_square(A)
@test D1 == get_julia(D2)
E1 = A[3]
lua_index = lualoadstring("local A, i = ...; return A[i]")
E2 = lua_index(A, 3)
@test E1 == get_julia(E2)
A = [1, 3, 5]
keysum1, valsum1 = 0, 0
for (k, v) in pairs(A)
keysum1 += k
valsum1 += v
end
lua_iter = lualoadstring("""
local A = ...
local keysum, valsum = 0, 0
for _, value in pairs(julia.pairs(A)) do
keysum, valsum = keysum + value[1], valsum + value[2]
end
return keysum, valsum
""")
keysum2, valsum2 = lua_iter(A; multiret=true)
@test keysum1 == keysum2
@test valsum1 == valsum2
end
@test top(LUA_STATE) == 0
end
@testset "LuaTable" begin
@luascope LUA_STATE begin
d = Dict((1 => "hello", "str" => 1234, Ptr{Cvoid}(4321) => 234))
t1 = new_table!(LUA_STATE, d)
for (k, v) in d
@luascope LUA_STATE begin
v2 = t1[k]
@test v2 == v
end
end
t1.field = 4321
f = t1.field
@test f == 4321
t2 = new_table!(LUA_STATE)
for (k, v) in d
LuaCall.rawset!(t2, k, v)
end
for (k, v) in d
@luascope LUA_STATE begin
v2 = LuaCall.rawget(t2, k)
@test v2 == v
end
end
a = [4 3 2 1]
t3 = new_table!(LUA_STATE, a)
for (k, v) in t3
@test a[k] == v
end
foreach(t3) do (k, v)
@test a[k] == v
end
mt = new_table!(LUA_STATE, Dict(["__name" => "hello"]))
obj = new_table!(LUA_STATE)
set_metatable!(obj, mt)
mt1 = get_metatable(obj)
@test mt1 == mt
f = lualoadstring("return tostring(getmetatable(...).__name)")
name = f(obj)
@test name == "hello"
end
end
@testset "LuaUserdata" begin
struct IntAndFloat
i::Int
f::Float64
end
@luascope LUA_STATE begin
ud = new_userdata!(LUA_STATE, sizeof(IntAndFloat), 1)
set_uservalue!(ud, 1, "hello")
uv = get_uservalue(ud, 1)
@test uv == "hello"
end
end
@testset "getstack" begin
@luascope LUA_STATE begin
values = [nothing, false, 1234, 12.34, "hello", Ptr{Cvoid}(123456)]
for v in values
push!(LUA_STATE, v)
@test LUA_STATE[] == v
end
push!(LUA_STATE, :sym)
@test LUA_STATE[] == "sym"
new_table!(LUA_STATE)
@test LUA_STATE[] isa LuaTable
ud = new_userdata!(LUA_STATE, 0)
@test LUA_STATE[] isa LuaUserData
end
ud = new_userdata!(LUA_STATE, 0)
@test_throws ErrorException push!(LUA_STATE, ud)
pop!(LUA_STATE, 1)
end
@testset "LuaFunction" begin
@luascope LUA_STATE begin
f = new_cfunction!(LUA_STATE, LuaCall.@lua_CFunction LuaCall.LuaFunctionWrapper(+, 2))
@test iscfunction(f)
status, x = f(1, 2; multiret=true)
@test status == 0 && x == 3
f = lualoadstring("return 1")
@test !iscfunction(f)
sum_abc(a, b... ; c) = a + sum(b) + c
caller = lualoadstring("""
local f = ...
return julia.LuaCall.kw(f, {c=3}, 1, 2)
""")
sum1 = caller(sum_abc)
sum2 = sum_abc(1, 2; c=3)
@test sum1 == sum2
end
end
@testset "LuaThread" begin
LS = LuaState()
@luascope LS begin
main_thread1 = mainthread(LS)
main_thread2 = mainthread(main_thread1)
@test main_thread1 == main_thread2
code = lualoadstring(LS, """
return coroutine.create(function (a, b)
local c, d = coroutine.yield(a + b)
return a + b - c - d
end)""")
thread = code()
main_thread3 = mainthread(thread)
@test main_thread3 == main_thread1
sum = resume(LS, thread, 1, 2)
@test status(thread) == LuaCall.LUA_YIELD
@test sum == 1 + 2
diff = resume(LS, thread, 3, 4)
@test status(thread) == LuaCall.LUA_OK
@test diff == 1 + 2 - 3 - 4
@test_throws LuaCall.LuaError resume(LS, thread)
thread2 = LuaCall.new_thread!(LS)
main_fn = lualoadstring(LS, """
return function (a, b)
local c, d = coroutine.yield(a + b)
return a + b - c - d
end""")[]()
start(thread2, main_fn)
sum2 = resume(LS, thread2, 1, 2)
@test sum2 == 1 + 2
diff2 = resume(LS, thread2, 3, 4)
@test diff2 == 1 + 2 - 3 - 4
end
end
@testset "Thread data exchange" begin
LS = LuaState()
@luascope LS begin
code = lualoadstring(LS, """
return coroutine.create(function (t, b)
coroutine.yield(t.a)
return b
end)""")
thread = code()
t = new_table!(LS, Dict(("a"=>1)))
b = [1,2,3]
@luascope thread begin
a = resume(LS, thread, t, b)
b2 = resume(LS, thread)
@test status(thread) == LuaCall.LUA_OK
@test a == 1
@test b == get_julia(b2)
end
end
end
@testset "globals" begin
LS = LuaState()
@luascope LS begin
main = LS.julia
@test get_julia(main) == Main
LS.foo = "bar"
field1 = LS.foo
@test get_julia(field1) == "bar"
gt = get_globaltable(LS)
field2 = gt.foo
@test field2 == "bar"
LS.julia = nothing
LS.foo = nothing
@test length(gt) == 0
end
@test LS |> top == 0
end
@testset "@luascope" begin
@luascope LUA_STATE begin
t = @luascope LUA_STATE begin
t = new_table!(LUA_STATE)
t[1] = 2
@luareturn t
end
v = t[1]
@test v == 2
t, num = @luascope LUA_STATE begin
t = new_table!(LUA_STATE)
t[1] = 2
@luareturn t 1234
end
v = t[1]
@test v == 2
@test num == 1234
end
end
@testset "Errors" begin
@luascope LUA_STATE begin
f = lualoadfile(joinpath(@__DIR__, "test.lua"))
f()
@test_throws LuaCall.LuaError luacall(:add, 1, "str")
try
LuaCall.set_debug!(LUA_STATE, true)
luacall(:add, [], [1])
catch e
@test e isa LuaCall.LuaError
@test !isempty(e.stacktrace)
@test occursin("test.lua", sprint(showerror, e))
end
try
LuaCall.set_debug!(LUA_STATE, false)
luacall(:add, [], [1])
catch e
@test e isa LuaCall.LuaError
@test isempty(e.stacktrace)
end
try
LuaCall.set_debug!(LUA_STATE, false)
luacall(:add, [], [1]; stacktrace=true)
catch e
@test e isa LuaCall.LuaError
@test isempty(e.stacktrace)
end
end
end
@testset "GC" begin
old_roots = LuaCall.check_gc_root()
LS = LuaState(Main, false)
@luascope LS begin
luacall(LS, :collectgarbage, "collect")
f = lualoadstring(LS, "local x; for _, v in pairs(...) do x = julia.Base end")
f(1:100)
LS.julia = nothing
luacall(LS, :collectgarbage, "collect")
end
@test LuaCall.check_gc_root() - old_roots== 0
@test LS |> top == 0
end
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 0.1.0 | a601793dad9a4f5380baefa4d0031fc3993ed9ca | docs | 4178 | # LuaCall.jl
A Julia package for interoperability with [Lua](https://www.lua.org/).
Functionality:
- Calling any Julia function from Lua
- Sharing any Julia object with Lua
- Accessing any Lua data from Julia
- Resuming Lua coroutines from Julia
## Showcase
### Call Julia from Lua
When LuaCall initializes a Lua state, a global variables `julia` represents the Julia module `Main`,
where you can access any Julia functions. Any Julia value involved is shared with Lua as opaque userdata.
For example, you can do linear algebra from Lua
```julia
using LuaCall
LS = LuaState()
# Guard whatever Lua scope with @luascope
@luascope LS begin
lua_inv = lualoadstring(LS, """
a = ...
return julia.inv(a)
""")
inv = lua_inv([1 2; 3 4])
get_julia(inv) # => [-2 -1; 1.5 -0.5]
end
```
### Calling Lua from Julia
```julia
using LuaCall
LS = LuaState()
@luascope LS begin
code = lualoadstring(LS, """
return {a=1, [2]="hello"}, coroutine.create(function (a)
b = coroutine.yield(a + 1)
return a + b
end)""")
table, thread = code(; multiret=true)
a = table["a"]
b = table[2]
c = resume(LS, thread, 3)
d = resume(LS, thread, 4)
a, b, c, d # => (1, "hello", 4, 7)
end
```
## Lua API overview
Each Lua session is contained in a Lua state, `lua_State` in C or `LuaState` in Julia. While Lua sessions do not
share data among each other, any access to a Lua state may affect its internal state. Of most concern is the
**stack** of one state, where the Lua state stores its execution context. To hide implimentation details for Lua
users, we can not use pointers to access Lua objects. Instead, we must reference it as one specific index into the
stack, which has substantial impact on the design of this package:
- The Lua API itself uses the stack to transfer data, may reference the data on the stack, or pop/push any object.
- LuaCall duplicates the poped elements so that no objects will be removed.
- However, new objects must be located on the stack, potentially leaking memory.
- `@luascope` resets the top of the stack to remove all elements pushed in the block.
- `@luareturn` can be used to return elements on the Lua stack, that is, remove any other elements but leave the returned
object.
- Any object on the Lua stack is wrapped in `PopStack`, which is automatically unwrapped if appear on the right-hand-side
of assignment in a `@luascope` block. You can manually unwrap it with `[]`, e.g. `table[key][]` returns the value unwrapped.
- You can also manage stack manually with `push!` and `pop!`.
Example:
```julia
@luascope LUA_STATE begin
array = [1,2,3]
array2 = @luascope LUA_STATE begin
table = new_table!(LUA_STATE)
# stack: [table1]
table[1] = array
# stack: [table] (unmodified)
userdata = table[1]
# stack: [table userdata(array)]
@luareturn userdata
end
# stack: [userdata(array)]
get_julia(array2[]) == array
end
# stack: []
```
## Type mapping
| Lua type | To Julia type | From Julia type | On Lua stack |
| -------------- | --------------------- | ---------------- | ------------- |
| `nil` | `nothing`(value) | `nothing` | ❌ |
| integer | `LuaInt`(`Int64`) | `AbstractInt` | ❌ |
| floating point | `LuaFloat`(`Float64`) | `AbstractFloat` | ❌ |
| string | `String` | `AbstractString` | ❌ |
| table | `LuaTable` | * | ✔ |
| function | `LuaFunction` | * | ✔ |
| userdata | `LuaUserData`, `Any` | `Any` | ✔ |
| thread | `LuaThread` | * | ✔ |
You can retrive any value on the Lua stack with `LUA_STATE[index]` or `getstack(LUA_STATE, index)`.
To retrive the Julia value contained in a Lua userdata, use `get_julia`.
To push value to the stack with default conversion, use `push!(LUA_STATE, values...)`.
To construct mutable object from Julia, check `new_table!`, `new_cfunction!`, `new_userdata` and `new_thread!`.
| LuaCall | https://github.com/melonedo/LuaCall.jl.git |
|
[
"MIT"
] | 1.0.0 | e62ad97646f585a03255bbbb4a4788204700f635 | code | 1029 | # This script can be used to quickly instantiate the docs/Project.toml environment.
using Pkg
documenter_directory = joinpath(@__DIR__, "..")
project_directory = isempty(ARGS) ? @__DIR__() : joinpath(pwd(), ARGS[1])
cd(project_directory) do
Pkg.activate(project_directory)
# Install a DocumenterTools version that declares compatibility with Documenter 0.28,
# but from an unreleased tag.
# https://github.com/JuliaDocs/DocumenterTools.jl/releases/tag/documenter-v0.1.14%2B0.28.0-DEV
if isdir("dev/DocumenterTools")
@info "DocumenterTools already cloned to dev/DocumenterTools"
run(`git -C dev/DocumenterTools fetch origin`)
else
run(`git clone -n https://github.com/JuliaDocs/DocumenterTools.jl.git dev/DocumenterTools`)
end
run(`git -C dev/DocumenterTools checkout documenter-v0.1.14+0.28.0-DEV`)
Pkg.develop([
PackageSpec(path = documenter_directory),
PackageSpec(path = "dev/DocumenterTools"),
])
Pkg.instantiate()
end
| PublishOrPerish | https://github.com/ParallelGSReg/PublishOrPerish.jl.git |
|
[
"MIT"
] | 1.0.0 | e62ad97646f585a03255bbbb4a4788204700f635 | code | 1052 | using Documenter, DocumenterTools
using PublishOrPerish
# The DOCSARGS environment variable can be used to pass additional arguments to make.jl.
# This is useful on CI, if you need to change the behavior of the build slightly but you
# can not change the .travis.yml or make.jl scripts any more (e.g. for a tag build).
if haskey(ENV, "DOCSARGS")
for arg in split(ENV["DOCSARGS"])
(arg in ARGS) || push!(ARGS, arg)
end
end
makedocs(
format = Documenter.HTML(
prettyurls = true,
assets = ["assets/favicon.ico"],
),
source = "src",
build = "build",
clean = true,
modules = [PublishOrPerish],
sitename = "PublishOrPerish.jl",
pages = [
"Home" => "index.md",
"Getting Started" => "start.md",
"Usage" => "usage.md",
"Contributing" => "contributing.md",
"News" => "news.md",
"Todo" => "todo.md",
"License" => "license.md",
],
)
deploydocs(
repo = "github.com/ParallelGSReg/PublishOrPerish.jl.git",
versions = nothing,
)
| PublishOrPerish | https://github.com/ParallelGSReg/PublishOrPerish.jl.git |
|
[
"MIT"
] | 1.0.0 | e62ad97646f585a03255bbbb4a4788204700f635 | code | 1634 | module PublishOrPerish
sources = ["crossref", "gsauthor", "gscholar", "gsciting", "gsprofile",
"masv2", "openalex", "pubmed", "scopus", "semscholar", "wos"]
methods = ["author", "affiliation", "citedid", "field", "issn", "journal", "title", "keywords", "years", "raw"]
function search(
query::Union{String, Vector{String}};
max::Int = 100,
source::String = "pubmed",
method::String = "keywords",
outfile::String = "output.csv",
username::String = "default",
password::String = "default"
)::Bool
if !(source in sources)
# FIXME throw exception
print("Invalid source. Given: $source, available sources $sources")
return false
end
if !(method in methods)
# FIXME throw exception
print("Invalid method. Given: $method, available methods $methods")
return false
end
source = "--$source"
method = "--$method"
#=
--author authorspec
--affiliation affiliation
--citedid id
--field fieldofstudy
--issn issn
--journal journalname
--title title
--keywords words
--years from-to
or:
--raw syntax use native 'syntax' directly
=#
cmd_salida = `bin/pop8query --max=$max $source $method=\"$query\" $outfile`
cmd_bibtex= `bin/pop8query --max=$max $source $method=\"$query\" --format=bibtex $outfile.bib`
try
output = read(run(cmd_salida), String)
output = read(run(cmd_bibtex), String)
catch y
print("Error: $y")
if(isa(y, ProcessFailedException))
return false
end
# run(::Base.AbstractCmd, ::Any...; wait) at process.jl:477
end
return true
end
export search
end
| PublishOrPerish | https://github.com/ParallelGSReg/PublishOrPerish.jl.git |
|
[
"MIT"
] | 1.0.0 | e62ad97646f585a03255bbbb4a4788204700f635 | docs | 5522 | [](https://github.com/SciML/ColPrac)
We follow the ColPrac guide for collaborative practices. New contributor should make sure to read that guide.
Following here are some additional clarifications and practices we follow.
Thanks for taking the plunge!
## Reporting Issues
* It's always good to start with a quick search for an existing issue to post on,
or related issues for context, before opening a new issue
* Including minimal examples is greatly appreciated
* If it's a bug, or unexpected behaviour, reproducing on the latest development version
(`Pkg.add(name="ModelSelectionGUI", rev="main")`) is a good gut check and can streamline the process,
along with including the first two lines of output from `versioninfo()`
## Contributing
* If you are a new contributor and would like to get a guidance on what area
you could focus your first PR please do not hesitate to ask and the developers
will help you with picking a topic matching your experience.
* Feel free to open, or comment on, an issue and solicit feedback early on,
especially if you're unsure about aligning with design goals and direction,
or if relevant historical comments are ambiguous.
* Aim for atomic commits, if possible, e.g. `change 'foo' behavior like so` &
`'bar' handles such and such corner case`,
rather than `update 'foo' and 'bar'` & `fix typo` & `fix 'bar' better`.
* The style guidelines outlined below are not the personal style of most contributors,
but for consistency throughout the project, we've adopted them.
* It is recommended to disable GitHub Actions on your fork; check Settings > Actions.
* A PR with breaking changes should have `[BREAKING]` as a first part of its name.
* If a PR changes or adds functionality please update NEWS.md file accordingly as
a part of the PR (along with the link to the PR); please do not add entries
to NEWS.md for changes that are bug fixes or are not user visible, such as
adding tests, updating documentation or improving code layout.
* If you make a PR please try to avoid pushing many small commits to GitHub in
a sequence as each such commit triggers a separate CI job, which takes over
an hour. This has a consequence of making other PRs in packages from the JuliaData
ecosystem wait for such CI jobs to finish as they share a common pool of CI resources.
## Style Guidelines
* Include spaces
+ After commas
+ Around operators: `=`, `<:`, comparison operators, and generally around others
+ But not after opening parentheses or before closing parentheses
* Use four spaces for indentation (test data files and Makefiles excepted)
* Don't leave trailing whitespace at the end of lines
* Don't go over the 79 per-line character limit
* Avoid squashing code blocks onto one line, e.g. `for foo in bar; baz += qux(foo); end`
* Don't explicitly parameterize types unless it's necessary
* Never leave things without type qualifications. Use an explicit `::Any`.
* Order method definitions from most specific to least specific type constraints
* Always include a digit after decimal when writing a float, e.g. `[1.0, 2.0]`
rather than `[1., 2.]`
* In docstrings, optional arguments, including separators and spaces, are surrounded by brackets,
e.g. `mymethod(required[, optional1[, optional2] ]; kwargs...)`
## Git Recommendations For Pull Requests
* Avoid working from the `main` branch of your fork, creating a new branch will make it
easier if DataFrames.jl `main` branch changes and you need to update your pull request;
* All PRs and issues should be opened against the `main` branch not against the current release;
* If any conflicts arise due to changes in DataFrames.jl `main` branch, prefer updating your pull
request branch with `git rebase` (rather than `git merge`), since the latter will introduce a merge
commit that might confuse GitHub when displaying the diff of your PR, which makes your changes more
difficult to review. Alternatively use conflict resolution tool available at GitHub;
* Please try to use descriptive commit messages to simplify the review process;
* Using `git add -p` or `git add -i` can be useful to avoid accidentally committing unrelated changes;
* Maintainers get notified of all changes made on GitHub. However, what is useful is writing a short
message after a sequence of changes is made summarizing what has changed and that the PR is ready
for a review;
* When linking to specific lines of code in discussion of an issue or pull request, hit the `y` key
while viewing code on GitHub to reload the page with a URL that includes the specific commit that
you're viewing. That way any lines of code that you refer to will still be correct in the future, even
if additional commits are pushed to the branch you are reviewing;
* Please make sure you follow the code formatting guidelines when submitting a PR;
Also preferably do not modify parts of code that you are not editing as this makes
reviewing the PR harder (it is better to open a separate maintenance PR
if e.g. code layout can be improved);
* If a PR is not finished yet and should not be reviewed yet then it should be opened as DRAFT
(in this way maintainers will know that they can ignore such PR until it is made non-draft or the author
asks for a review).
| PublishOrPerish | https://github.com/ParallelGSReg/PublishOrPerish.jl.git |
|
[
"MIT"
] | 1.0.0 | e62ad97646f585a03255bbbb4a4788204700f635 | docs | 2148 | # PublishOrPerish.jl
[![][documentation-main-img]][documentation-main-url] [![][build-main-img]][build-main-url]
PublishOrPerish.jl is a Julia package that serves as a wrapper for the powerful Publish or Perish software. The package aims to streamline the process of accessing and utilizing Publish or Perish functionalities directly from Julia.
## Features
- Seamless Integration: Easily integrate with Publish or Perish, a leading citation analysis tool for academic and scientific research.
- Simplified API: Abstracts away complexities, providing a straightforward API to access and leverage Publish or Perish's extensive capabilities.
- Automated Retrieval: Retrieve publication metrics, citation data, and more with ease, all directly from your Julia environment.
## Installation
You can install the package by running the following command in the Julia REPL:
```julia
using Pkg
Pkg.add("PublishOrPerish")
```
## Usage
```julia
using PublishOrPerish
data = search(["machine learning", "ai", "julia"])
```
## Documentation
For more detailed information about this package, its functionalities, and usage instructions, please refer to our [documentation page](https://parallelgsreg.github.io/PublishOrPerish.jl).
## Contributing
Contributions are welcome! If you encounter any issues or have suggestions for improvements, please open an issue or submit a pull request on the repository. Make sure to follow the guidelines outlined in the [CONTRIBUTING.md](CONTRIBUTING.md) file.
## TODO List
For an overview of pending tasks, improvements, and future plans for the ModelSelectionGUI package, please refer to the [TODO.md](TODO.md) file.
## License
This package is licensed under the [MIT License](LICENSE).
[build-main-img]: https://github.com/ParallelGSReg/PublishOrPerish.jl/actions/workflows/build.yaml/badge.svg?branch=main
[build-main-url]: https://github.com/ParallelGSReg/PublishOrPerish.jl/pkgs/container/publishorperish.jl
[documentation-main-img]: https://github.com/ParallelGSReg/PublishOrPerish.jl/actions/workflows/docs.yaml/badge.svg
[documentation-main-url]: https://parallelgsreg.github.io/PublishOrPerish.jl
| PublishOrPerish | https://github.com/ParallelGSReg/PublishOrPerish.jl.git |
|
[
"MIT"
] | 1.0.0 | e62ad97646f585a03255bbbb4a4788204700f635 | docs | 441 | - Pasar a BERT para summarization: https://huggingface.co/philschmid/bart-large-cnn-samsum
- Clusterizar.
- Ver poner la búsqueda en la distancia de BERT
- Luego poner los papers buscados y también ordenarlos según su distancia a la búsqueda (ahí que pueda poner un pequeño abstract)
- Hacer similarity matrix de clusterización
- Mostrar tablas
Más a futuro
- Ver de leer por python directo los sitions donde están los papers para gscholar
| PublishOrPerish | https://github.com/ParallelGSReg/PublishOrPerish.jl.git |
|
[
"MIT"
] | 1.0.0 | e62ad97646f585a03255bbbb4a4788204700f635 | docs | 141 | # Contributing
```@eval
using Markdown, PublishOrPerish
Markdown.parse_file(joinpath(pkgdir(PublishOrPerish), "CONTRIBUTING.md"))
```
| PublishOrPerish | https://github.com/ParallelGSReg/PublishOrPerish.jl.git |
|
[
"MIT"
] | 1.0.0 | e62ad97646f585a03255bbbb4a4788204700f635 | docs | 1181 | # PublishOrPerish.jl
PublishOrPerish.jl is a Julia package that serves as a wrapper for the powerful Publish or Perish software. The package aims to streamline the process of accessing and utilizing Publish or Perish functionalities directly from Julia.
## Features
- Seamless Integration: Easily integrate with Publish or Perish, a leading citation analysis tool for academic and scientific research.
- Simplified API: Abstracts away complexities, providing a straightforward API to access and leverage Publish or Perish's extensive capabilities.
- Automated Retrieval: Retrieve publication metrics, citation data, and more with ease, all directly from your Julia environment.
## Contributing
Contributions are welcome! If you encounter any issues or have suggestions for improvements, please open an issue or submit a pull request on the repository. Make sure to follow the guidelines outlined in the [CONTRIBUTING.md](CONTRIBUTING.md) file.
## TODO List
For an overview of pending tasks, improvements, and future plans for the ModelSelectionGUI package, please refer to the [TODO.md](TODO.md) file.
## License
This package is licensed under the [MIT License](LICENSE).
| PublishOrPerish | https://github.com/ParallelGSReg/PublishOrPerish.jl.git |
|
[
"MIT"
] | 1.0.0 | e62ad97646f585a03255bbbb4a4788204700f635 | docs | 125 | # News
```@eval
using Markdown, PublishOrPerish
Markdown.parse_file(joinpath(pkgdir(PublishOrPerish), "NEWS.md"))
```
| PublishOrPerish | https://github.com/ParallelGSReg/PublishOrPerish.jl.git |
|
[
"MIT"
] | 1.0.0 | e62ad97646f585a03255bbbb4a4788204700f635 | docs | 607 | # Getting started
This basic example demonstrates how to use the package in its simplest way. However, for a more in-depth understanding of the various options and features, please navigate to the [Usage section](usage.md) where all available functionalities and usage scenarios are thoroughly explained.
## Installation
PublishOrPerish.jl can be installed using the Julia package manager. From the Julia REPL, type ] to enter the Pkg REPL mode and run
```
pkg> add PublishOrPerish
```
## Usage
```julia
using PublishOrPerish
data = search(["machine learning", "ai", "julia"])
```
| PublishOrPerish | https://github.com/ParallelGSReg/PublishOrPerish.jl.git |
|
[
"MIT"
] | 1.0.0 | e62ad97646f585a03255bbbb4a4788204700f635 | docs | 125 | # News
```@eval
using Markdown, PublishOrPerish
Markdown.parse_file(joinpath(pkgdir(PublishOrPerish), "TODO.md"))
```
| PublishOrPerish | https://github.com/ParallelGSReg/PublishOrPerish.jl.git |
|
[
"MIT"
] | 1.0.0 | e62ad97646f585a03255bbbb4a4788204700f635 | docs | 1601 | # Usage
## Index
```@contents
Pages = ["usage.md"]
```
## Advanced usage
```julia
using PublishOrPerish
search(
query = ["machine learning", "ai", "julia"],
max = 50,
source = "pubmed",
method = "keywords",
outfile = "output.csv",
username = "a_username",
password = "a_password"
)
```
## search() function
```julia
search(
query::Union{String, Vector{String}};
max::Int = 100,
source::String = "pubmed",
method::String = "keywords",
outfile::String = "output.csv",
username::String = "default",
password::String = "default"
)
```
### Parameters
- `query::Union{String, Vector{String}}`: The search query, which can be a single string or a vector of strings for advanced queries.
### Optional parameters
- `max::Int64`: The maximum number of results to retrieve (default: 100).
- `source::String`: The source from which to perform the search. Possible values are `crossref,` `gsauthor,` `gscholar,` `gsciting,` `gsprofile,` `masv2,` `openalex,` `pubmed,` `scopus,` `semscholar,` and `wos` (default: `pubmed`).
- `method::String`: The search method to use. Possible values are `author,` `affiliation,` `citedid,` `field,` `issn,` `journal,` `title,` `keywords,` `years,` and `raw` (default: `keywords`).
- `outfile::String`: The name of the output file to store the search results in CSV format (default: `salida.csv`).
- `username::String`: The username for authentication (default: `default`).
- `password::String`: The password for authentication (default: `default`).
| PublishOrPerish | https://github.com/ParallelGSReg/PublishOrPerish.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 1020 | using Documenter, Muscade
repo = normpath((@__DIR__)*"/..")
push!(LOAD_PATH,joinpath(repo,"src"))
cp(joinpath(repo,"LICENSE.md"),joinpath(repo,"docs/src/LICENSE.md"),force=true)
makedocs(sitename ="Muscade.jl",
format = Documenter.HTML(prettyurls = false,sidebar_sitename = false),
pages = ["index.md",
"Theory.md",
"Modelling.md",
"Elements.md",
"Solvers.md",
"Builtin_els.md",
"Builtin_sol.md",
"TypeStable.md",
"Memory.md",
"Adiff.md",
"reference.md",
"LICENSE.md"],
source = "src",
build = "build"
)
deploydocs(repo = "github.com/SINTEF/Muscade.jl.git",target="build",devbranch="philippe")
# https://sintef.github.io/Muscade.jl/v0.3.6/index.html
# https://sintef.github.io/Muscade.jl/stable/index.html
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 1096 | using Test,StaticArrays,MacroTools
include("../src/Dialect.jl")
include("../src/Espy.jl")
include("../src/Exceptions.jl")
#using Muscade
# @espydbg function residual(x::Vector{R},y) where{R<:Real}
# ngp = 2
# α,β = 1,2
# va = 1,2,3
# tu = (a=1.,b=2)
# e,f = foo()
# accum = ntuple(ngp) do igp
# ☼z = x[igp]+y[igp]
# ☼s,☼t = ☼material(z)
# @named(s)
# end
# r = sum(i->accum[i].s,ngp)
# return r,nothing,nothing
# end
# @espydbg function material(z)
# ☼a = z+1
# ☼b = a*z
# return b,3.
# end
# @espydbg lagrangian(o::Vector{R}, δX,X,U,A, t,γ,dbg) where{R} = o.cost(∂n(X,R)[1],t),nothing,nothing
exbar= @espydbg function bar(x,y,z)
ngp = 4
vec = SVector{2}
☼p = 3
for i ∈ 1:2
j = i^2
k = i+j
end
t = ntuple(ngp) do igp
a = vec(igp,igp^2)
b = vec(1.,1.)
☼c = b*b'
♢square = c^2
χ = randn()
r = vcat(a,reshape(c,4))
@named(χ,r)
end
χ = ntuple(i->t[i].χ,ngp)
r = sum( i->t[i].r,ngp)
return r,χ,nothing
end
;
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 1949 | using Profile,ProfileView,BenchmarkTools
using Muscade
include("../Test/SomeElements.jl")
model = Model(:TestModel)
n1 = addnode!(model,𝕣[ 0, 0]) # moving node
n2 = addnode!(model,𝕣[10, 0]) # anchor 1
n3 = addnode!(model,𝕣[ 0,10]) # anchor 2
n4 = addnode!(model,𝕣[ ]) # A-nod for springs
e1 = addelement!(model,Spring{2},[n1,n2,n4], EI=1)
e2 = addelement!(model,Spring{2},[n1,n3,n4], EI=1)
@once f1(t) = t
e3 = addelement!(model,DofLoad ,[n1], field=:tx1 ,value=f1)
e4 = addelement!(model,Hold ,[n2], field=:tx1)
e5 = addelement!(model,Hold ,[n2], field=:tx2)
e6 = addelement!(model,Hold ,[n3], field=:tx1)
e7 = addelement!(model,Hold ,[n3], field=:tx2)
@once f2(x,t) = 1x^2
@once f3(a) = 0.11a^2
e8 = addelement!(model,SingleDofCost ,[n1], class=:X ,field=:tx1 ,cost=f2)
e9 = addelement!(model,SingleDofCost ,[n1], class=:X ,field=:tx2 ,cost=f2)
e10 = addelement!(model,SingleDofCost ,[n4], class=:A ,field=:ΞL₀ ,cost=f3)
e10 = addelement!(model,SingleDofCost ,[n4], class=:A ,field=:ΞEI ,cost=f3)
initialstate = initialize!(model)
stateX = solve(StaticX; initialstate,time=[.5,1.],verbose=false)
state = solve(StaticXUA;initialstate=stateX,maxYiter= 50,verbose=false)
#@btime state = solve(StaticXUA;initialstate=stateX,maxYiter= 0,verbose=false)
Profile.clear()
Profile.@profile for i=1:1000#25000
# local stateX = solve(StaticX; initialstate,time=[.5,1.],verbose=false)
local state = solve(StaticXUA;initialstate=stateX,maxYiter= 50,verbose=false);
end
ProfileView.view(fontsize=30);
# After clicking on a bar in the flame diagram, you can type warntype_last() and see the result of
# code_warntype for the call represented by that bar.
;
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 4034 | using Profile,ProfileView,BenchmarkTools
using Muscade
using StaticArrays,GLMakie,Random,Printf#nλfloor
#------------- custom element code
struct Pipe <: AbstractElement
K :: SMatrix{4,4,𝕣,16}
W :: SVector{4,𝕣}
x :: SVector{2,𝕣}
end
function Pipe(nod::Vector{Node};EI,w)
c = coord(nod)
x1,x2 = c[1][1],c[2][1]
L = abs(x2-x1)
L² = L^2
K = EI/L^3*SMatrix{4,4,𝕣}(12,6L,-12,6L, 6L,4L²,-6L,2L², -12,-6L,12,-6L, 6L,2L²,-6L,4L²)
W = w*L*SVector{4,𝕣}(1,0,1,0)
return Pipe(K,W,SVector(x1,x2))
end
Muscade.doflist(::Type{Pipe}) =(inod=(1,1,2,2), class=(:X,:X,:X,:X), field=(:z,:r,:z,:r))
@espy function Muscade.residual(o::Pipe, X,U,A, t,χ,χcv,SP,dbg)
return o.K*∂0(X)+o.W,nothing,nothing
end
function Muscade.draw(axe,key,out, o::Pipe, δX,X,U,A, t,χ,χcv,SP,dbg;kwargs...)
z = ∂0(X)[SVector(1,3)]
lines!(axe,collect(o.x),collect(z) ;kwargs...)
end
#---------------- analysis and result extraction
nel = 2000
δx = 1.
δz = 0.1
w = 0#100*9.81
EI = 5000000
Δz = 0.25 # 0.25 causes a rattle if not interior point
x = δx*(0:nel)
z = zeros(nel+1)
rng = MersenneTwister(0)
zfloor = cumsum(0.1*randn(rng,nel+1))
zfloor .-= range(0,zfloor[end],nel+1) .- .01
@once floorgap(z,t,zfloor)=z[1]-zfloor
@once ceilgap(z,t,zceil)=zceil-z[1]
# zceil = zfloor.+Δz
# floorgap = [(z,t)->z[1]-zfloor[i] for i=1:nel+1] # comment to avoid recompilation
# ceilgap = [(z,t)->zceil[i]-z[1] for i=1:nel+1] # comment to avoid recompilation
coords = cat(x,z,dims=2)
gₛ = 0.1
λₛ = 4000.
model = Model(:Pipe)
node = addnode!(model,coords)
pipe = [addelement!(model,Pipe,node[i:i+1];EI,w) for i=1:nel]
floorctct = [addelement!(model,Constraint,[node[i]];λₛ,gₛ,xinod=(1,),xfield=(:z,),
λinod=1, λclass=:X, λfield=:λfloor, g=floorgap,gargs=(zfloor[i],), mode=inequal) for i=1:nel+1]
ceilctct = [addelement!(model,Constraint,[node[i]];λₛ,gₛ,xinod=(1,),xfield=(:z,),
λinod=1, λclass=:X, λfield=:λceil, g=ceilgap,gargs=(zfloor[i]+Δz,), mode=inequal) for i=1:nel+1]
initialstate = initialize!(model)
mission = :profile
if mission == :report
state = solve(StaticX;initialstate,time=[0.],maxiter=200,saveiter=true,γ0=10.,γfac1=.5,γfac2=10.,verbose=true)
elseif mission == :time
state = solve(StaticX;initialstate,time=[0.],maxiter=200,saveiter=true,γ0=10.,γfac1=.5,γfac2=10.,verbose=false)
@btime state = solve(StaticX;initialstate,time=[0.],maxiter=200,saveiter=true,γ0=10.,γfac1=.5,γfac2=10.,verbose=false)
elseif mission == :profile
Profile.clear()
Profile.@profile for i=1:10
local state = solve(StaticX;initialstate,time=[0.],maxiter=200,saveiter=true,γ0=10.,γfac1=.5,γfac2=10.,verbose=false)
end
ProfileView.view(fontsize=30);
end
if false # sandwich plot
state = cat(dims=1,initialstate,state[1:findlastassigned(state)])
niter = length(state)
out,key = getresult(state,@request((λ,g)),floorctct)
λfloor = out[key.λ,:,:] # out[ikey,iele,istep]
gfloor = out[key.g,:,:] # out[ikey,iele,istep]
out,key = getresult(state,@request((λ,g)),ceilctct)
λceil = out[key.λ,:,:] # out[ikey,iele,istep]
gceil = out[key.g,:,:] # out[ikey,iele,istep]
z,dofid = getdof(state,field=:z) #x[iel,idir,iiter]
fig1 = Figure(resolution = (1000,250))
with_theme(Theme(fontsize = 30,font="Arial")) do
axe = Axis(fig1[1,1])
hidespines!(axe)
hidedecorations!(axe)
for i=1:niter-1
lines!(axe,x,z[:,1,i];linewidth=1,color=:grey)
end
draw(axe,state[niter];linewidth=2,color=:black)
lines!(axe,x,zfloor,color=:red, linewidth=2)
lines!(axe,x,zceil,color=:red, linewidth=2)
end
display(fig1)
end
; | Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 4557 | using Muscade,GLMakie
f1(x) = .15*x^2-.2x-1 +2 # start outside feasible domain 😃
f2(x) = .05*x^2+x +2 +2
g1(x,t) = x[2]-f1(x[1])
g2(x,t) = x[2]-f2(x[1])
gravity(t) = -2.
gravity2(t) = 0
model = Model(:TestModel)
n1 = addnode!(model,𝕣[0,0])
e1 = addelement!(model,Constraint,[n1],xinod=(1,1),xfield=(:t1,:t2),λinod=1, λclass=:X, λfield=:λ1,g=g1,mode=inequal)
e2 = addelement!(model,Constraint,[n1],xinod=(1,1),xfield=(:t1,:t2),λinod=1, λclass=:X, λfield=:λ2,g=g2,mode=inequal)
e3 = addelement!(model,DofLoad ,[n1],field=:t1,value=gravity2)
e4 = addelement!(model,DofLoad ,[n1],field=:t2,value=gravity)
e5 = addelement!(model,QuickFix ,[n1],inod=(1,1),field=(:t1,:t2),res=(x,u,a,t)->0.1x)
initialstate = initialize!(model)
state = solve(StaticX;initialstate,time=[0.],verbose=false,γ0=.5,γfac1=.5,γfac2=10.,saveiter=true,maxiter=1000) # because there is zero physical stiffness in this model, setting γ0=0 gives singularity if one or more constraint is inactive
last = findlastassigned(state)
println("Converged in ",last, " iterations.")
X = state[last].X[1][1:2]
x1 = [0,[state[i].X[1][1] for i ∈1:last]...]
x2 = [0,[state[i].X[1][2] for i ∈1:last]...]
fig = Figure(resolution = (800,800))
display(fig) # open interactive window (gets closed down by "save")
axe = Axis(fig[1,1],title="Modified interior point")
a1 = -4:.01:0
a2 = -4:.01:0
b = f1.(a1)
c = f2.(a2)
scatter!(axe,x1,x2)
lines!( axe,x1,x2,color = :black, linewidth = 1)
lines!( axe,a1,b ,color = :red, linewidth = 1)
lines!( axe,a2,c ,color = :blue, linewidth = 1)
#-----------------------------------------------------------------
# @once g g(x,t) = x[1]
# @once f f(x) = 0.4x.+1+.5x.^2
# γ0 = .3
# model = Model(:TestModel)
# n1 = addnode!(model,𝕣[0])
# e1 = addelement!(model,Constraint,[n1],xinod=(1,),xfield=(:t1,),λinod=1, λclass=:X, λfield=:λ1,g=g,mode=inequal)
# e2 = addelement!(model,QuickFix ,[n1],inod=(1,),field=(:t1,),res=(x,u,a,t)->f(x))
# TODO update call syntax
# state = solve(staticX;model,time=[0.],verbose=false,γ0=γ0,γfac=1.,saveiter=true,maxiter=1000) # because there is zero physical stiffness in this model, setting γ0=0 gives singularity if one or more constraint is inactive
# last = findlastassigned(state)
# println("Converged in ",last, " iterations.")
# x = [0,[state[i].X[1][1] for i ∈1:last]...]
# λ = [0,[state[i].X[1][2] for i ∈1:last]...]
# fig = Figure(resolution = (800,800))
# display(fig) # open interactive window (gets closed down by "save")
# axe = Axis(fig[1,1],title="Modified interior point")
# scatter!(axe,x,λ)
# lines!( axe,x,λ,color = :black, linewidth = 1)
# X = -1:.1:1
# Λ = -1:.1:2
# S = [Muscade.S(a,b,γ0) for a∈X, b∈Λ] # meshgrid...
# contour!(axe,X,Λ,S,levels=-1:.2:1,color=:grey)
# contour!(axe,X,Λ,S,levels=0:0,color=:black)
# lines!( axe,X,f.(X),color=:red)
#-----------------------------------------------------------------
# @once g g(x,t) = x[1]+.1
# @once f f(x) = 0.4x.+.08+.5x.^2
# γ0 = 1.
# γfac = .5
# model = Model(:TestModel)
# n1 = addnode!(model,𝕣[0])
# e1 = addelement!(model,Constraint,[n1],xinod=(1,),xfield=(:t1,),λinod=1, λclass=:X, λfield=:λ1,g=g,mode=inequal)
# e2 = addelement!(model,QuickFix ,[n1],inod=(1,),field=(:t1,),res=(x,u,a,t)->f(x))
# TODO update call syntax
# state = solve(staticX;model,time=[0.],verbose=false,γ0=γ0,γfac=γfac,saveiter=true,maxiter=1000) # because there is zero physical stiffness in this model, setting γ0=0 gives singularity if one or more constraint is inactive
# last = findlastassigned(state)
# println("Converged in ",last, " iterations.")
# x = [[state[i].X[1][1] for i ∈1:last]...]
# λ = [[state[i].X[1][2] for i ∈1:last]...]
# fig = Figure(resolution = (800,800))
# display(fig) # open interactive window (gets closed down by "save")
# axe = Axis(fig[1,1],title="Modified interior point")
# scatter!(axe,x,λ)
# lines!( axe,x,λ,color = :black, linewidth = 1)
# X = -1:.01:1
# Λ = -1:.01:1
# for i = 1:last
# γ=γ0*γfac.^(i-1)
# slack = [Muscade.S(a+ .1,b,γ) for a∈X, b∈Λ] # meshgrid...
# contour!(axe,X,Λ,slack,levels=0:0,color=:black)
# end
# lines!( axe,X,f.(X),color=:red)
; | Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 13962 | using StaticArrays
using SpecialFunctions
using Printf
## Type and construction
const SV = SVector
const SA = SArray
const SM = SMatrix
# Types
# P precedence
# N number of partials
# R type of the variable (and partials)
struct ∂ℝ{P,N,R} <:ℝ where{R<:ℝ} # P for precedence, N number of partials, R type of the variable (∂ℝ can be nested)
x :: R
dx :: SV{N,R}
end
# Constructors
∂ℝ{P,N }(x::R ,dx::SV{N,R}) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}(x ,SV{N,R}(dx))
∂ℝ{P,N }(x::R ) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}(x ,SV{N,R}(zero(R) for j=1:N))
∂ℝ{P,N }(x::R,i::ℤ ) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}(x ,SV{N,R}(i==j ? one(R) : zero(R) for j=1:N))
∂ℝ{P,N,R}(x::𝕣 ) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}(R(x),SV{N,R}(zero(R) for j=1:N))
function ∂ℝ{P,N}(x::Rx,dx::SV{N,Rdx}) where{P,N,Rx<:ℝ,Rdx<:ℝ}
R = promote_type(Rx,Rdx)
return ∂ℝ{P,N}(convert(R,x),convert.(R,dx))
end
# zeros, ones
Base.zero(::Type{∂ℝ{P,N,R}}) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}(zero(R), SV{N,R}(zero(R) for j=1:N))
Base.one( ::Type{∂ℝ{P,N,R}}) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}(one( R), SV{N,R}(zero(R) for j=1:N))
Base.isnan( a::∂ℝ) = isnan( VALUE(a))
Base.isone( a::∂ℝ) = isone( VALUE(a))
Base.iszero( a::∂ℝ) = iszero( VALUE(a))
Base.isinf( a::∂ℝ) = isinf( VALUE(a))
Base.isfinite(a::∂ℝ) = isfinite(VALUE(a))
Base.typemax( ::Type{∂ℝ{P,N,R}}) where{P,N,R<:ℝ} = typemax(R)
Base.typemin( ::Type{∂ℝ{P,N,R}}) where{P,N,R<:ℝ} = typemin(R)
Base.floatmax(::Type{∂ℝ{P,N,R}}) where{P,N,R<:ℝ} = floatmax(R)
Base.floatmin(::Type{∂ℝ{P,N,R}}) where{P,N,R<:ℝ} = floatmin(R)
Base.floatmax(:: ∂ℝ{P,N,R} ) where{P,N,R<:ℝ} = floatmax(R) # because ℝ is Real, not AbstractFloat
Base.floatmin(:: ∂ℝ{P,N,R} ) where{P,N,R<:ℝ} = floatmin(R) # because ℝ is Real, not AbstractFloat
Base.eps( ::Type{∂ℝ{P,N,R}}) where{P,N,R<:ℝ} = eps(R)
Base.float(a::∂ℝ) = a
# promote rules
Base.promote_rule(::Type{∂ℝ{P ,N ,Ra}},::Type{∂ℝ{P,N,Rb}}) where{P ,N ,Ra<:ℝ,Rb<:ℝ} = ∂ℝ{P ,N ,promote_type(Ra,Rb)}
Base.promote_rule(::Type{∂ℝ{Pa,Na,Ra}},::Type{ Rb }) where{Pa,Na,Ra<:ℝ,Rb<:ℝ} = ∂ℝ{Pa,Na,promote_type(Ra,Rb)}
function Base.promote_rule(::Type{∂ℝ{Pa,Na,Ra}},::Type{∂ℝ{Pb,Nb,Rb}}) where{Pa,Pb,Na,Nb,Ra<:ℝ,Rb<:ℝ}
if Pa>Pb ∂ℝ{Pa,Nb,promote_type( Ra ,∂ℝ{Pb,Nb,Rb})}
else ∂ℝ{Pb,Nb,promote_type(∂ℝ{Pa,Na,Ra}, Rb )}
end
end
# conversions
Base.convert(::Type{∂ℝ{P,N,Ra}},b::∂ℝ{P,N,Rb}) where{P,N,Ra<:ℝ,Rb<:ℝ} = ∂ℝ{P ,N }(convert(Ra,b.x) ,convert.(Ra,b.dx))
Base.convert(::Type{∂ℝ{P,N,Ra}},b::ℝ ) where{P,N,Ra<:ℝ } = ∂ℝ{P ,N }(convert(Ra,b ) ,SV{N,Ra}(zero(Ra) for j=1:N))
function Base.convert(::Type{∂ℝ{Pa,Na,Ra}},b::∂ℝ{Pb,Nb,Rb}) where{Pa,Pb,Na,Nb,Ra<:ℝ,Rb<:ℝ}
if Pa> Pb return ∂ℝ{Pa,Na}(convert(Ra,b.x) ,convert.(Ra,b.dx))
else muscadeerror(printf("Cannot convert precedence ",Pb," to ",Pa))
end
end
# Pack and unpack
precedence( ::Type{<:∂ℝ{P,N,R}}) where{P,N,R<:ℝ} = P
npartial( ::Type{<:∂ℝ{P,N,R}}) where{P,N,R<:ℝ} = N
precedence( ::Type{<:ℝ}) = 0
npartial( ::Type{<:ℝ}) = 0
precedence(a::SA) = precedence(eltype(a))
npartial( a::SA) = npartial(eltype(a))
precedence(a::ℝ) = precedence(typeof(a))
npartial( a::ℝ) = npartial(typeof(a))
constants( a) = 1+precedence(a)
constants( a,args...) = max(1+precedence(a),constants(args...))
# variate
struct δ{P,N,R} end # need dum, because syntax δ{P,N,R}() collides with default constructor
struct variate{P,N} end
struct directional{P,N} end
δ{P,N,R}( ) where{P,N,R<:ℝ} = SV{N,∂ℝ{P,N,R}}(∂ℝ{P,N }(zero(R),i ) for i=1:N)
δ{P,N,R}( δa::SV{N,𝕣}) where{P,N,R<:ℝ} = SV{N,∂ℝ{P,N,R}}(∂ℝ{P,N,R}(zero(R),SV{N,R}(i==j ? δa[i] : zero(R) for i=1:N)) for j=1:N)
variate{P,N}(a::SV{N,R} ) where{P,N,R<:ℝ} = SV{N,∂ℝ{P,N,R}}(∂ℝ{P,N }(a[i] ,i ) for i=1:N)
variate{P,N}(a::SV{N,R},δa::SV{N,𝕣}) where{P,N,R<:ℝ} = SV{N,∂ℝ{P,N,R}}(∂ℝ{P,N,R}(a[j] ,SV{N,R}(i==j ? R(δa[i]) : zero(R) for i=1:N)) for j=1:N)
variate{P}(a::R) where{P,R<:ℝ} = ∂ℝ{P,1}(a,SV{1,R}(one(R)))
directional{P}(a::SV{N,R},δa::SV{N,R}) where{P,N,R<:ℝ} = SV{N,∂ℝ{P,1,R}}(∂ℝ{P,1}(a[i],SV{1,R}(δa[i])) for i=1:N)
# Analyse
VALUE(a::ℝ ) = a
VALUE(a::∂ℝ) = VALUE( a.x)
VALUE(a::SA) = VALUE.(a)
struct ∂{P,N} end
struct value{P,N} end
struct value_∂{P,N} end
value{P}(a::∂ℝ{P,N,R}) where{P,N,R } = a.x
value{P}(a::R ) where{P ,R<:ℝ} = a
value{P}(a::SA ) where{P } = value{P}.(a)
# ∂{P}(a) is handled as ∂{P,1}(a) and returns a scalar
∂{P,N}(a:: ∂ℝ{P,N,R} ) where{ P,N,R } = a.dx
∂{P,N}(a:: R ) where{ P,N,R<:ℝ} = SV{ N,R}(zero(R) for i=1:N )
∂{P,N}(a::SV{M,∂ℝ{P,N,R}}) where{M,P,N,R } = SM{M,N,R}(a[i].dx[j] for i=1:M,j∈1:N) # ∂(a,x)[i,j] = ∂a[i]/∂x[j]
∂{P,N}(a::SV{M, R }) where{M,P,N,R } = SM{M,N,R}(zero(R) for i=1:M,j=1:N)
∂{P }(a:: R ) where{ P, R<:ℝ} = zero(R)
∂{P }(a:: ∂ℝ{P,1,R} ) where{ P, R } = a.dx[1]
∂{P }(a::SV{N,∂ℝ{P,1,R}}) where{ P,N,R } = SV{ N,R}(a[i].dx[1] for i=1:N ) # ∂(a,x)[i] = ∂a[i]/∂x
#∂{P,N}(a::SA{M,∂ℝ{P,N,R}}) where{M,P,N,R} = SA{(M...,N),R}(a[i].dx[j] for i∈eachindex(a),j∈1:N) # ∂(a,x)[i,...,j] = ∂a[i,...]/∂x[j]
#∂{P,N}(a::SA{M, R }) where{M,P,N,R} = SA{(M...,N),R}(zero(R) for i∈eachindex(a),j∈1:N)
value_∂{P,N}(a) where{ P,N}= value{P}(a),∂{P,N}(a)
value_∂{P }(a) where{ P }= value{P}(a),∂{P }(a)
## Binary operations
for OP∈(:(>),:(<),:(==),:(>=),:(<=),:(!=))
@eval Base.$OP(a::∂ℝ,b::∂ℝ) = $OP(VALUE(a),VALUE(b))
@eval Base.$OP(a:: ℝ,b::∂ℝ) = $OP( a ,VALUE(b))
@eval Base.$OP(a::∂ℝ,b:: ℝ) = $OP(VALUE(a), b )
end
macro Op2(OP,AB,A,B)
return esc(quote
@inline $OP(a::∂ℝ{P,N,R},b::∂ℝ{P,N,R}) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}($OP(a.x,b.x),$AB)
@inline $OP(a::∂ℝ{P,N,R},b::ℝ ) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}($OP(a.x,b ),$A )
@inline $OP(a::ℝ ,b::∂ℝ{P,N,R}) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}($OP(a ,b.x),$B )
@inline function $OP(a::∂ℝ{Pa,Na,Ra},b::∂ℝ{Pb,Nb,Rb}) where{Pa,Pb,Na,Nb,Ra<:ℝ,Rb<:ℝ}
if Pa==Pb
R = promote_type(Ra,Rb)
return ∂ℝ{Pa,Na}(convert(R,$OP(a.x,b.x)),convert.(R,$AB))
elseif Pa> Pb
R = promote_type(Ra,typeof(b))
return ∂ℝ{Pa,Na}(convert(R,$OP(a.x,b )),convert.(R,$A ))
else
R = promote_type(typeof(a),Rb)
return ∂ℝ{Pb,Nb}(convert(R,$OP(a ,b.x)),convert.(R,$B ))
end
end
end)
end
@Op2(Base.hypot,(a.dx*a.x+b.dx*b.x)/hypot(a.x,b.x), a.dx*a.x/hypot(a.x,b), b.dx*b.x/hypot(a,b.x))
@Op2(Base.:(+), a.dx+b.dx, a.dx, b.dx )
@Op2(Base.:(-), a.dx-b.dx, a.dx, -b.dx )
@Op2(Base.:(*), a.dx*b.x+a.x*b.dx, a.dx*b, a*b.dx )
@Op2(Base.:(/), a.dx/b.x-a.x/b.x^2*b.dx, a.dx/b, -a/b.x^2*b.dx )
@Op2(Base.:(^), a.dx*b.x*a.x^(b.x-1)+log(a.x)*a.x^b.x*b.dx, a.dx*b*a.x^(b -1), log(a)*a ^b.x*b.dx )
@inline Base.:(^)(a::∂ℝ{P,N,R},b::Integer) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}(a.x^b ,a.dx*b*a.x^(b-1) )
## Functions
macro Op1(OP,A)
return esc(:(@inline $OP(a::∂ℝ{P,N}) where{P,N} = ∂ℝ{P,N}($OP(a.x),$A)))
end
@Op1(Base.:(+), a.dx )
@Op1(Base.:(-), -a.dx )
@Op1(Base.abs ,a.x==0.0 ? zero(a.dx) : (a.x>0.0 ? a.dx : -a.dx) )
@Op1(Base.conj , a.dx )
@Op1(Base.sqrt, a.dx / 2. / sqrt(a.x) )
@Op1(Base.cbrt, a.dx / 3. / cbrt(a.x)^2 )
@Op1(Base.abs2, a.dx*2. * a.x )
@Op1(Base.inv, -a.dx * abs2(inv(a.x)) )
@Op1(Base.log, a.dx / a.x )
@Op1(Base.log10, a.dx / a.x / log(10.) )
@Op1(Base.log2, a.dx / a.x / log(2.) )
@Op1(Base.log1p, a.dx / (a.x + 1.) )
@Op1(Base.exp, exp(a.x) * a.dx )
@Op1(Base.exp2, log(2. ) * exp2( a.x) * a.dx )
@Op1(Base.exp10, log(10.) * exp10(a.x) * a.dx )
@Op1(Base.expm1, exp(a.x) * a.dx )
@Op1(Base.sin, cos(a.x) * a.dx )
@Op1(Base.cos, -sin(a.x) * a.dx )
@Op1(Base.tan, (1. + tan(a.x)^2) * a.dx )
@Op1(Base.sec, sec(a.x) * tan(a.x) * a.dx )
@Op1(Base.csc, -csc(a.x) * cot(a.x) * a.dx )
@Op1(Base.cot, -(1. + cot(a.x)^2) * a.dx )
@Op1(Base.sind, π / 180. * cosd(a.x) * a.dx )
@Op1(Base.cosd, -π / 180. * sind(a.x) * a.dx )
@Op1(Base.tand, π / 180. * (1. + tand(a.x)^2) * a.dx )
@Op1(Base.secd, π / 180. * secd(a.x) * tand(a.x) * a.dx )
@Op1(Base.cscd, -π / 180. * cscd(a.x) * cotd(a.x) * a.dx )
@Op1(Base.cotd, -π / 180. * (1. + cotd(a.x)^2) * a.dx )
@Op1(Base.asin, a.dx / sqrt(1. - a.x^2) )
@Op1(Base.acos, -a.dx / sqrt(1. - a.x^2) )
@Op1(Base.atan, a.dx / (1. + a.x^2) )
@Op1(Base.asec, a.dx / abs(a.x) / sqrt(a.x^2 - 1.) )
@Op1(Base.acsc, -a.dx / abs(a.x) / sqrt(a.x^2 - 1.) )
@Op1(Base.acot, -a.dx / (1. + a.x^2) )
@Op1(Base.asind, 180. / π / sqrt(1. - a.x^2) * a.dx )
@Op1(Base.acosd, -180. / π / sqrt(1. - a.x^2) * a.dx )
@Op1(Base.atand, 180. / π / (1. + a.x^2) * a.dx )
@Op1(Base.asecd, 180. / π / abs(a.x) / sqrt(a.x^2- 1.) * a.dx )
@Op1(Base.acscd, -180. / π / abs(a.x) / sqrt(a.x^2- 1.) * a.dx )
@Op1(Base.acotd, -180. / π / (1. + a.x^2) * a.dx )
@Op1(Base.sinh, cosh(a.x) * a.dx )
@Op1(Base.cosh, sinh(a.x) * a.dx )
@Op1(Base.tanh, sech(a.x)^2 * a.dx )
@Op1(Base.sech, -tanh(a.x) * sech(a.x) * a.dx )
@Op1(Base.csch, -coth(a.x) * csch(a.x) * a.dx )
@Op1(Base.coth, -csch(a.x)^2 )
@Op1(Base.asinh, a.dx / sqrt(a.x^2 + 1.) )
@Op1(Base.acosh, a.dx / sqrt(a.x^2 - 1.) )
@Op1(Base.atanh, a.dx / (1. - a.x^2) )
@Op1(Base.asech, -a.dx / a.x / sqrt(1. - a.x^2) )
@Op1(Base.acsch, -a.dx / abs(a.x) / sqrt(1. + a.x^2) )
@Op1(Base.acoth, a.dx / (1. - a.x^2) )
@Op1(SpecialFunctions.erf, 2. * exp(-a.x^2) / sqrt(π) * a.dx )
@Op1(SpecialFunctions.erfc, -2. * exp(-a.x^2) / sqrt(π) * a.dx )
@Op1(SpecialFunctions.erfi, 2. * exp( a.x^2) / sqrt(π) * a.dx )
@Op1(SpecialFunctions.gamma, digamma(a.x) * gamma(a.x) * a.dx )
@Op1(SpecialFunctions.lgamma, digamma(a.x) * a.dx )
@Op1(SpecialFunctions.airy, airyprime(a.x) * a.dx ) # note: only covers the 1-arg version
@Op1(SpecialFunctions.airyprime, airy(2., a.x) * a.dx )
@Op1(SpecialFunctions.airyai, airyaiprime(a.x) * a.dx )
@Op1(SpecialFunctions.airybi, airybiprime(a.x) * a.dx )
@Op1(SpecialFunctions.airyaiprime, a.x * airyai(a.x) * a.dx )
@Op1(SpecialFunctions.airybiprime, a.x * airybi(a.x) * a.dx )
@Op1(SpecialFunctions.besselj0, -besselj1(a.x) * a.dx )
@Op1(SpecialFunctions.besselj1, (besselj0(a.x) - besselj(2., a.x))/2. * a.dx )
@Op1(SpecialFunctions.bessely0, -bessely1(a.x) * a.dx )
@Op1(SpecialFunctions.bessely1, (bessely0(a.x) - bessely(2., a.x))/2. * a.dx )
## Comparison for debug purposes
≗(a::ℝ,b::ℝ) = (typeof(a)==typeof(b)) && ((a-b) < 1e-10*max(1,a+b))
≗(a::SA,b::SA) = (size(a)==size(b)) && all(a .≗ b)
≗(a::∂ℝ,b::∂ℝ) = (typeof(a)==typeof(b)) && (a.x ≗ b.x) && (a.dx ≗ b.dx)
## Find NaN in derivatives
hasnan(a::∂ℝ ) = hasnan(a.x) || hasnan(a.dx)
hasnan(a::ℝ ) = isnan(a)
function hasnan(a::SV{N,R}) where{N,R<:ℝ}
for el∈a
if hasnan(el)
return true
end
end
return false
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 18489 | ######## The disassembler
struct XUA{T,nX,nU,nA}
X::SVector{nX,T}
U::SVector{nU,T}
A::SVector{nA,T}
end
struct ΛXUA{T,nX,nU,nA}
Λ::SVector{nX,T}
X::SVector{nX,T}
U::SVector{nU,T}
A::SVector{nA,T}
end
struct EletypDisassembler{nX,nU,nA}
index :: Vector{XUA{𝕫,nX,nU,nA}}
scale :: ΛXUA{𝕣,nX,nU,nA}
end
# dis.dis[ieletyp].index.[iele].X|U|A[ieledof]
# dis.dis[ieletyp].scale.Λ|X|U|A[ieledof]
# dis.scaleΛ|X|U|A[imoddof]
struct Disassembler
dis :: Vector{EletypDisassembler}
scaleΛ :: 𝕣1
scaleX :: 𝕣1
scaleU :: 𝕣1
scaleA :: 𝕣1
end
function Disassembler(model::Model)
neletyp = length(model.eleobj)
dis = Vector{EletypDisassembler}(undef,neletyp)
NX,NU,NA = getndof(model,(:X,:U,:A))
scaleΛ = Vector{𝕣}(undef,NX) # scale for state
scaleX = Vector{𝕣}(undef,NX)
scaleU = Vector{𝕣}(undef,NU)
scaleA = Vector{𝕣}(undef,NA)
for ieletyp = 1:neletyp
nele = length(model.eleobj[ieletyp])
E = eltype(model.eleobj[ieletyp])
nX,nU,nA = getndof(E,(:X,:U,:A))
sΛ,sX,sU,sA = 𝕣1(undef,nX),𝕣1(undef,nX),𝕣1(undef,nU),𝕣1(undef,nA) # scale for element
ixdof,iudof,iadof = 0,0,0
for dofID ∈ model.ele[ieletyp][begin].dofID
doftyp = getdoftyp(model,dofID)
class,scale = doftyp.class,doftyp.scale
if class == :X
ixdof += 1
sX[ixdof] = scale
sΛ[ixdof] = scale * model.scaleΛ
elseif class == :U
iudof += 1
sU[iudof] = scale
elseif class == :A
iadof += 1
sA[iadof] = scale
end
end
scale = ΛXUA{𝕣,nX,nU,nA}(sΛ,sX,sU,sA) # scale for element type
iX,iU,iA = 𝕫1(undef,nX),𝕫1(undef,nU),𝕫1(undef,nA) # tmp arrays fof index into state of eledofs
index = Vector{XUA{𝕫,nX,nU,nA}}(undef,nele) # such indexes, for all elements in type
for iele = 1:nele
ixdof,iudof,iadof = 0,0,0
for dofID ∈ model.ele[ieletyp][iele].dofID
doftyp = getdoftyp(model,dofID)
class = doftyp.class
idof = dofID.idof
if class == :X
ixdof += 1
iX[ixdof] = idof
elseif class == :U
iudof += 1
iU[iudof] = idof
elseif class == :A
iadof += 1
iA[iadof] = idof
else
muscadeerror("element dof class must be :X,:U or :A")
end
end
index[iele] = XUA{𝕫,nX,nU,nA}(iX,iU,iA)
scaleΛ[iX] = scale.Λ # "assemble" element scales into state scales
scaleX[iX] = scale.X
scaleU[iU] = scale.U
scaleA[iA] = scale.A
end # for iele
dis[ieletyp] = EletypDisassembler{nX,nU,nA}(index,scale)
end # for ieletyp
return Disassembler(dis,scaleΛ,scaleX,scaleU,scaleA)
end
######## state and initstate
# at each step, contains the complete, unscaled state of the system
mutable struct State{Nxder,Nuder,TSP}
Λ :: 𝕣1
X :: NTuple{Nxder,𝕣1}
U :: NTuple{Nuder,𝕣1}
A :: 𝕣1
time :: 𝕣
SP :: TSP # solver parameter
model :: Model
dis :: Disassembler
end
# a constructor that provides an initial state
State(model::Model,dis;time=-∞) = State(zeros(getndof(model,:X)),(zeros(getndof(model,:X)),),(zeros(getndof(model,:U)),),zeros(getndof(model,:A)),time,nothing,model,dis)
function State{nXder,nUder}(s::State) where{nXder,nUder}
X = ntuple(i->copy(∂n(s.X,i)),nXder)
U = ntuple(i->copy(∂n(s.U,i)),nUder)
State{nXder,nUder}(copy(s.Λ),X,U,copy(s.A),s.time,s.SP,s.model,s.dis)
end
#### DofGroup
struct DofGroup{T1,T2,T3,T4,T5,T6,T7,T8}
nX :: 𝕫 # of the _model_
nU :: 𝕫
nA :: 𝕫
iΛ :: T1 # state.Λ[iΛ] <-> y[jΛ]*Λscale
iX :: T2
iU :: T3
iA :: T4
jΛ :: T5
jX :: T6
jU :: T7
jA :: T8
scaleΛ :: 𝕣1
scaleX :: 𝕣1
scaleU :: 𝕣1
scaleA :: 𝕣1
end
function DofGroup(dis::Disassembler,iΛ,iX,iU,iA)
# constructor for dofgroup with permutation within classe. The datastructure of DofGroup supports dofgroups with arbitrary permutations - write another constructor
nX,nU,nA = length(dis.scaleX),length(dis.scaleU),length(dis.scaleA) # number of dofs in _model_
nλ,nx,nu,na = length(iΛ),length(iX),length(iU),length(iA) # number of dofs of each class in group
jΛ,jX,jU,jA = gradientpartition(nλ,nx,nu,na) # we stack classes on top of each other in group vectors
Λs,Xs,Us,As = dis.scaleΛ[iΛ],dis.scaleX[iX],dis.scaleU[iU],dis.scaleA[iA]
return DofGroup(nX,nU,nA, iΛ,iX,iU,iA, jΛ,jX,jU,jA, Λs,Xs,Us,As)
end
function decrement!(s::State,der::𝕫,y::𝕣1,gr::DofGroup)
for i ∈ eachindex(gr.iΛ); s.Λ[ gr.iΛ[i]] -= y[gr.jΛ[i]] * gr.scaleΛ[i]; end
for i ∈ eachindex(gr.iX); s.X[der+1][gr.iX[i]] -= y[gr.jX[i]] * gr.scaleX[i]; end
for i ∈ eachindex(gr.iU); s.U[der+1][gr.iU[i]] -= y[gr.jU[i]] * gr.scaleU[i]; end
for i ∈ eachindex(gr.iA); s.A[ gr.iA[i]] -= y[gr.jA[i]] * gr.scaleA[i]; end
end
getndof(gr::DofGroup) = length(gr.iΛ)+length(gr.iX)+length(gr.iU)+length(gr.iA)
allΛdofs( model::Model,dis) = DofGroup(dis, 1:getndof(model,:X),𝕫[],𝕫[],𝕫[])
allXdofs( model::Model,dis) = DofGroup(dis, 𝕫[],1:getndof(model,:X),𝕫[],𝕫[])
allUdofs( model::Model,dis) = DofGroup(dis, 𝕫[],𝕫[],1:getndof(model,:U),𝕫[])
allAdofs( model::Model,dis) = DofGroup(dis, 𝕫[],𝕫[],𝕫[],1:getndof(model,:A))
allΛXUdofs(model::Model,dis) = DofGroup(dis, 1:getndof(model,:X),1:getndof(model,:X),1:getndof(model,:U),𝕫[])
######## Prepare assemblers
# asm[iarray,ieletyp][ieledof/ientry,iele] has value zero for terms from element gradient/hessian that are not to be added in. Otherwise, the value they
# have is where in the matrix/vector/nzval to put the values
function indexedstate(gr::DofGroup)
# create a "state" (Λ,X,U,A) of indices into the group - with zeros for modeldofs not in group
Λ = zeros(𝕫,gr.nX)
X = zeros(𝕫,gr.nX)
U = zeros(𝕫,gr.nU)
A = zeros(𝕫,gr.nA)
Λ[gr.iΛ] = gr.jΛ
X[gr.iX] = gr.jX
U[gr.iU] = gr.jU
A[gr.iA] = gr.jA
return Λ,X,U,A
end
function gradientstructure(dofgr,dis::EletypDisassembler)
# number of dofs of each class in the gradient returned by an element
# because adiff is what it is, the gradient contains either all or no dofs in any given class
nΛ = length(dofgr.iΛ)==0 ? 0 : length(dis.scale.Λ)
nX = length(dofgr.iX)==0 ? 0 : length(dis.scale.X)
nU = length(dofgr.iU)==0 ? 0 : length(dis.scale.U)
nA = length(dofgr.iA)==0 ? 0 : length(dis.scale.A)
return nΛ,nX,nU,nA
end
function gradientpartition(nΛ,nX,nU,nA)
# indices into the class partitions of the gradient returned by an element
iΛ = (1:nΛ)
iX = nΛ .+(1:nX)
iU = nΛ+nX .+(1:nU)
iA = nΛ+nX+nU.+(1:nA)
return iΛ,iX,iU,iA
end
nonzeros(v) = v[v.≠0]
function asmvec!(asm,dofgr,dis)
# asm[ieletyp] == undef, please fill
Λ,X,U,A = indexedstate(dofgr) # create a state of indices into the group - with zeros for modeldofs not in group
for ieletyp ∈ eachindex(dis.dis)
asmvec_kernel!(asm,ieletyp,dofgr,dis.dis[ieletyp],Λ,X,U,A)
end
return 𝕣1(undef,getndof(dofgr))
end
function asmvec_kernel!(asm,ieletyp,dofgr,dis,Λ,X,U,A)
nΛ,nX,nU,nA = gradientstructure(dofgr,dis) # number of dofs of each class in the gradient returned by an element
iΛ,iX,iU,iA = gradientpartition(nΛ,nX,nU,nA) # indices into said gradient TODO type unstable, barrier function
asm[ieletyp] = zeros(𝕫,nΛ+nX+nU+nA,length(dis.index)) # asm[ieletyp][idof,iele] (its a view)
for (iele,index) ∈ enumerate(dis.index)
asm[ieletyp][iΛ,iele] .= nonzeros(Λ[index.X])
asm[ieletyp][iX,iele] .= nonzeros(X[index.X])
asm[ieletyp][iU,iele] .= nonzeros(U[index.U])
asm[ieletyp][iA,iele] .= nonzeros(A[index.A])
end
end
function asmfullmat!(asm,iasm,jasm,nimoddof,njmoddof)
for ieletyp ∈ eachindex(iasm)
nieledof,nele = size(iasm[ieletyp])
njeledof = size(jasm[ieletyp],1)
asm[ieletyp] = zeros(𝕫,nieledof*njeledof,nele)
for iele=1:nele, jeledof=1:njeledof, ieledof=1:nieledof
imoddof,jmoddof = iasm[ieletyp][ieledof,iele], jasm[ieletyp][jeledof,iele]
if (imoddof≠0) && (jmoddof≠0)
ientry = ieledof+nieledof*(jeledof-1)
asm[ieletyp][ientry,iele] = imoddof+nimoddof*(jmoddof-1)
end
end
end
return 𝕣2(undef,nimoddof,njmoddof)
end
function asmmat!(asm,iasm,jasm,nimoddof,njmoddof)
# 1) traverse all eletyp
# compute number npairs of contribution
npair = 0
for ieletyp ∈ eachindex(iasm)
for iele = 1:size(iasm[ieletyp],2)
npair += sum(iasm[ieletyp][:,iele].≠0)*sum(jasm[ieletyp][:,iele].≠0)
end
end
# 2) traverse all elements
# prepare a Vector A of all (jmoddof,imoddof) (in that order, for sort to work!) pairs of model dofs ::Vector{Tuple{Int64, Int64}}(undef,N)
A = Vector{Tuple{𝕫,𝕫}}(undef,npair)
ipair = 0
for ieletyp ∈ eachindex(iasm)
nieledof,nele = size(iasm[ieletyp])
njeledof = size(jasm[ieletyp],1)
for iele=1:nele, jeledof=1:njeledof, ieledof=1:nieledof
if (iasm[ieletyp][ieledof,iele]≠0) && (jasm[ieletyp][jeledof,iele]≠0)
ipair += 1
A[ipair] = (jasm[ieletyp][jeledof,iele] , iasm[ieletyp][ieledof,iele]) # NB: (j,i), not (i,j), because of lexicographic sortperm
end
end
end
# 3) sortperm(A)
I = sortperm(A)
# 4) traverse A[I]
# count nnz
# create a list J that to each element of A[I] associates an entry 1≤inz≤nnz into nzval
# prepare sparse
nnz = 0
for ipair = 1:npair
if (ipair==1) || (A[I[ipair]]≠A[I[ipair-1]])
nnz +=1
end
end
J = 𝕫1(undef,npair) # to each pair in A[I] associate a unique entry number
K = 𝕫1(undef,npair) # to each pair in A associate a unique entry number
nzval = ones(𝕣,nnz) # could this be left undef and still get past the sparse constructor?
colptr = 𝕫1(undef,njmoddof+1) # Column icol is in colptr[icol]:(colptr[icol+1]-1)
colptr[njmoddof+1] = nnz+1
rowval = 𝕫1(undef,nnz)
inz = 0
icol = 1
colptr[icol] = inz+1
for ipair = 1:npair
if (ipair==1) || (A[I[ipair]]≠A[I[ipair-1]])
inz +=1
(j,i) = A[I[ipair]] # NB: (j,i), not (i,j)
rowval[inz] = i
while j>icol
icol +=1
colptr[icol] = inz
end
end
J[ipair] = inz
end
K[I] = J
# 5) traverse all elements again to distribute J into asm
ipair = 0
for ieletyp ∈ eachindex(iasm)
nieledof,nele = size(iasm[ieletyp] )
njeledof = size(jasm[ieletyp],1)
asm[ieletyp] = zeros(𝕫,nieledof*njeledof,nele)
for iele=1:nele, jeledof=1:njeledof, ieledof=1:nieledof
if (iasm[ieletyp][ieledof,iele]≠0) && (jasm[ieletyp][jeledof,iele]≠0)
ipair += 1
ientry = ieledof+nieledof*(jeledof-1)
asm[ieletyp][ientry,iele] = K[ipair]
end
end
end
# 6)
return SparseMatrixCSC(nimoddof,njmoddof,colptr,rowval,nzval)
end
######## Generic assembler
abstract type Assembly end
using Base.Threads
# sequential
function assemble!(out::Assembly,asm,dis,model,state,dbg)
zero!(out)
for ieletyp = 1:lastindex(model.eleobj)
eleobj = model.eleobj[ieletyp]
assemble_!(out,view(asm,:,ieletyp),dis.dis[ieletyp],eleobj,state,state.SP,(dbg...,ieletyp=ieletyp))
end
end
function assemble_!(out::Assembly,asm,dis,eleobj,state::State{Nxder,Nuder},SP,dbg) where{Nxder,Nuder}
scale = dis.scale
for iele = 1:lastindex(eleobj)
index = dis.index[iele]
Λe = state.Λ[index.X]
Xe = NTuple{Nxder}(x[index.X] for x∈state.X)
Ue = NTuple{Nuder}(u[index.U] for u∈state.U)
Ae = state.A[index.A]
addin!(out,asm,iele,scale,eleobj[iele],Λe,Xe,Ue,Ae, state.time,SP,(dbg...,iele=iele))
end
end
# multithreaded
one_for_each_thread(x) = SVector{nthreads()}(deepcopy(x) for i=1:nthreads())
firstelement(x::AbstractVector) = x[1]
firstelement(x ) = x
function add!(a::Array,b::Array)
for i∈eachindex(a)
a[i] += b[i]
end
end
function add!(a::SparseMatrixCSC,b::SparseMatrixCSC) # assumes identical sparsity structure
for i∈eachindex(a.nzval)
a.nzval[i] += b.nzval[i]
end
end
function assemble!(out::AbstractVector{A},asm,dis,model,state,dbg) where{A<:Assembly}
for i = 1:nthreads()
zero!(out[i])
end
for ieletyp = 1:lastindex(model.eleobj)
eleobj = model.eleobj[ieletyp]
assemble_!(out,view(asm,:,ieletyp),dis.dis[ieletyp], eleobj,state,state.SP,(dbg...,ieletyp=ieletyp))
end
for i = 2:nthreads()
add!(out[1],out[i])
end
end
function assemble_!(out::AbstractVector{A},asm,dis,eleobj,state::State{Nxder,Nuder},SP,dbg) where{Nxder,Nuder,A<:Assembly}
scale = dis.scale
@threads for iele = 1:lastindex(eleobj)
index = dis.index[iele]
Λe = state.Λ[index.X]
Xe = NTuple{Nxder}(x[index.X] for x∈state.X)
Ue = NTuple{Nuder}(u[index.U] for u∈state.U)
Ae = state.A[index.A]
addin!(out[threadid()],asm,iele,scale,eleobj[iele],Λe,Xe,Ue,Ae, state.time,SP,(dbg...,iele=iele))
end
end
#######
# assemble! calls MySolver/addin!, which calls getresidual or getlagrangian
####### Lagrangian from residual and residual from Lagrangian
const True,False = Val{true},Val{false}
@generated function implemented(eleobj)
# δX,X, U, A, t,χ, χcv, SP, dbg
r = hasmethod(residual ,(eleobj, NTuple,NTuple,𝕣1,𝕣,Any,Function,NamedTuple,NamedTuple))
l = hasmethod(lagrangian,(eleobj,𝕣1,NTuple,NTuple,𝕣1,𝕣,Any,Function,NamedTuple,NamedTuple))
return :(Val{$r},Val{$l})
end
function checkresidual(eleobj::AbstractElement,X,U,A,t,χ,χcv,SP,dbg,req...)
res = residual(eleobj,X,U,A,t,χ,χcv,SP,dbg,req...)
hasnan(res[1]) && muscadeerror((dbg...,t=t,SP=SP),
"NaN in a residual or its partial derivatives")
return res
end
function checklagrangian(eleobj::AbstractElement,Λ,X,U,A,t,χ,χcv,SP,dbg,req...)
res = lagrangian(eleobj,Λ,X,U,A,t,χ,χcv,SP,dbg,req...)
hasnan(res[1]) && muscadeerror((dbg...,t=t,SP=SP),
"NaN in a lagrangian or its partial derivatives")
return res
end
# has residual has lagrangian
getresidual( ::Type{False},::Type{False},eleobj::AbstractElement, X,U,A,t,χ,χcv,SP,dbg,req...) = muscadeerror(dbg,@sprintf("Element %s must have method 'Muscade.lagrangian' or/and 'Muscade.residual' with correct interface",typeof(eleobj)))
getlagrangian(::Type{False},::Type{False},eleobj::AbstractElement,Λ,X,U,A,t,χ,χcv,SP,dbg,req...) = muscadeerror(dbg,@sprintf("Element %s must have method 'Muscade.lagrangian' or/and 'Muscade.residual' with correct interface",typeof(eleobj)))
getresidual( ::Type{True },::Type{<:Val},eleobj::AbstractElement, X,U,A,t,χ,χcv,SP,dbg,req...) = checkresidual( eleobj, X,U,A,t,χ,χcv,SP,dbg,req...)
getlagrangian(::Type{<:Val},::Type{True },eleobj::AbstractElement,Λ,X,U,A,t,χ,χcv,SP,dbg,req...) = checklagrangian(eleobj,Λ,X,U,A,t,χ,χcv,SP,dbg,req...)
# want residual, lagrangian implemented
function getresidual(::Type{False},::Type{True} ,eleobj::AbstractElement,X,U,A,t,χ,χcv,SP,dbg,req...)
P = constants(∂0(X),∂0(U),A,t)
Nx = length(∂0(X)) # TODO this does no generalize to dynamics
Λ = δ{P,Nx,𝕣}()
L,χn,FB,eleres... = checklagrangian(eleobj,Λ,X,U,A,t,χ,χcv,SP,dbg,req...)
return ∂{P,Nx}(L),χn,FB,eleres...
end
# want lagrangian, residual implemented
function getlagrangian(::Type{True} ,::Type{False},eleobj::AbstractElement,Λ,X,U,A,t,χ,χcv,SP,dbg,req...)
R,χn,FB,eleres... = checkresidual( eleobj, X,U,A,t,χ,χcv,SP,dbg,req...)
return Λ ∘₁ R ,χn,FB,eleres...
end
#### zero!
function zero!(out::DenseArray)
for i∈eachindex(out)
out[i] = 0
end
end
function zero!(out::AbstractSparseArray)
for i∈eachindex(out.nzval)
out.nzval[i] = 0
end
end
#### extract value or derivatives from a SVector 'a' of adiffs, and add it directly into vector, full matrix or sparse matrix 'out'.
function add_value!(out::𝕣1,asm,iele,a::SVector{M,∂ℝ{P,N,𝕣}},ias) where{P,N,M}
for (iasm,ia) ∈ enumerate(ias)
iout = asm[iasm,iele]
if iout≠0
out[iout]+=a[ia].x
end
end
end
function add_value!(out::𝕣1,asm,iele,a::SVector{M,𝕣},ias) where{M}
for (iasm,ia) ∈ enumerate(ias)
iout = asm[iasm,iele]
if iout≠0
out[iout]+=a[ia]
end
end
end
add_value!(out,asm,iele,a) = add_value!(out,asm,iele,a,eachindex(a))
struct add_∂!{P} end
function add_∂!{P}(out::Array,asm,iele,a::SVector{M,∂ℝ{P,N,R}},i1as,i2as) where{P,N,R,M}
for (i1asm,i1a) ∈ enumerate(i1as), (i2asm,i2a) ∈ enumerate(i2as)
iasm = i1asm+length(i1as)*(i2asm-1)
iout = asm[iasm,iele]
if iout≠0
out[iout]+=a[i1a].dx[i2a]
end
end
end
add_∂!{P}(out::SparseMatrixCSC,args...) where{P} = add_∂!{P}(out.nzval,args...)
add_∂!{P}(out::Array,asm,iele,a::SVector{M,R},args...) where{P,M,R} = nothing
add_∂!{P}(out::Array,asm,iele,a::SVector{M,∂ℝ{P,N,R}}) where{P,N,R,M} = add_∂!{P}(out,asm,iele,a,1:M,1:N)
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 25072 | """
DofCost{Class,Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,
afield,Tcost,Tcostargs} <: AbstractElement
An element to apply costs on combinations of dofs.
# Named arguments to the constructor
- `xinod::NTuple{Nx,𝕫}=()` For each X-dof to enter `cost`, its element-node number.
- `xfield::NTuple{Nx,Symbol}=()` For each X-dof to enter `cost`, its field.
- `uinod::NTuple{Nu,𝕫}=()` For each U-dof to enter `cost`, its element-node number.
- `ufield::NTuple{Nu,Symbol}=()` For each U-dof to enter `cost`, its field.
- `ainod::NTuple{Na,𝕫}=()` For each A-dof to enter `cost`, its element-node number.
- `afield::NTuple{Na,Symbol}=()` For each A-dof to enter `cost`, its field.
- `class:Symbol` `:A` for cost on A-dofs only, `:I` ("instant") otherwise.
- `cost::Function` if `class==:I`, `cost(X,U,A,t,costargs...)→ℝ`
if `class==:A`, `cost(A,costargs...)→ℝ`
`X` and `U` are tuples (derivates of dofs...), and `∂0(X)`,`∂1(X)`,`∂2(X)`
must be used by `cost` to access the value and derivatives of `X` (resp. `U`)
- `costargs::NTuple`
# Requestable internal variables
- `cost`, the value of the cost.
# Example
```
ele1 = addelement!(model,DofCost,[nod1],xinod=(1,),field=(:tx1,),
class=:I,cost=(X,U,A,t)->X[1]^2
```
See also: [`SingleDofCost`](@ref), [`ElementCost`](@ref), [`addelement!`](@ref)
"""
struct DofCost{Class,Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield,Tcost,Tcostargs} <: AbstractElement
cost :: Tcost
costargs :: Tcostargs
end
function DofCost(nod::Vector{Node};xinod::NTuple{Nx,𝕫}=(),xfield::NTuple{Nx,Symbol}=(),
uinod::NTuple{Nu,𝕫}=(),ufield::NTuple{Nu,Symbol}=(),
ainod::NTuple{Na,𝕫}=(),afield::NTuple{Na,Symbol}=(),
class::Symbol=:I,cost::Function ,costargs=()) where{Nx,Nu,Na} # :I for "instantaneous" or "integrand" cost.
(class==:A && (Nx>0||Nu>0)) && muscadeerror("Cost with Class==:A must have zero X-dofs and zero U-dofs")
return DofCost{class,Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield,typeof(cost),typeof(costargs)}(cost,costargs)
end
doflist(::Type{<:DofCost{Class,Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield}}) where
{Class,Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield} =
(inod =(xinod... ,uinod... ,ainod... ),
class=(ntuple(i->:X,Nx)...,ntuple(i->:U,Nu)...,ntuple(i->:A,Na)...),
field=(xfield... ,ufield... ,afield... ) )
@espy function lagrangian(o::DofCost{:I,Nx,Nu,Na},Λ,X,U,A,t,χ,χcv,SP,dbg) where{Nx,Nu,Na}
☼cost = o.cost(X,U,A,t,o.costargs...)
return cost,noχ,noFB
end
@espy function lagrangian(o::DofCost{:A,Nx,Nu,Na},Λ,X,U,A,t,χ,χcv,SP,dbg) where{Nx,Nu,Na}
☼cost = o.cost( A ,o.costargs...)
return cost,noχ,noFB
end
"""
ElementCost{Teleobj,Treq,Tcost,Tcostargs} <: AbstractElement
An element to apply costs on another "target" element's dofs and element-results.
The target element must *not* be added separatly to the model. Instead, the
`ElementType`, and the named arguments to the target element are provided
as input to the `ElementCost` constructor.
# Named arguments to the constructor
- `req` A request for element-results to be extracted from the target element, see [`@request`](@ref).
The request is formulated as if adressed directly to the target element (no "prefixing" with
`eleres`, see "Requestable internal variables")
- `cost` a cost function `cost(eleres,X,U,A,t,costargs...)→ℝ`
`X` and `U` are tuples (derivates of dofs...), and `∂0(X)`,`∂1(X)`,`∂2(X)`
must be used by `cost` to access the value and derivatives of `X` (resp. `U`).
`X`, `U` and `A` are the degrees of freedom of the element `ElementType`.
- `costargs=(;)` A named tuple of additional arguments to the cost function
- `ElementType` The named of the constructor for the relevant element
- `elementkwargs...` Additional named arguments to the `ElementCost` constructor are passed on to the `ElementType` constructor.
# Requestable internal variables
From `ElementConstraint` one can request
- `cost` The value of the cost
From the target element on can request
- `eleres(...)` where `...` is the list of requestables from the target element. It must be "prefixed" by
`eleres` to prevent possible confusion with variables requestable from `ElementConstraint`.
# Example
```
@once cost(eleres,X,U,A,t) = eleres.Fh^2
ele1 = addelement!(model,ElementCost,[nod1];req=@request(Fh),
cost=cost,ElementType=AnchorLine,
Λₘtop=[5.,0,0], xₘbot=[250.,0], L=290., buoyancy=-5e3)
```
See also: [`SingleDofCost`](@ref), [`DofCost`](@ref), [`@request`](@ref)
"""
struct ElementCost{Teleobj,Treq,Tcost,Tcostargs} <: AbstractElement
eleobj :: Teleobj
req :: Treq
cost :: Tcost
costargs :: Tcostargs
end
function ElementCost(nod::Vector{Node};req,cost,costargs=(;),ElementType,elementkwargs...)
eleobj = ElementType(nod;elementkwargs...)
return ElementCost(eleobj,(eleres=req,),cost,costargs)
end
doflist( ::Type{<:ElementCost{Teleobj}}) where{Teleobj} = doflist(Teleobj)
@espy function lagrangian(o::ElementCost, Λ,X,U,A,t,χ,χcv,SP,dbg)
req = merge(o.req)
L,χ,FB,☼eleres = getlagrangian(implemented(o.eleobj)...,o.eleobj,Λ,X,U,A,t,χ,χcv,SP,(dbg...,via=ElementCost),req.eleres)
☼cost = o.cost(eleres,X,U,A,t,o.costargs...)
return L+cost,χ,FB
end
"""
SingleDofCost{Derivative,Class,Field,Tcost} <: AbstractElement
An element with a single node, for adding a cost to a given dof.
# Named arguments to the constructor
- `class::Symbol`, either `:X`, `:U` or `:A`.
- `field::Symbol`.
- `cost::Function`, where `cost(x::ℝ,t::ℝ[,costargs...]) → ℝ` if `class` is `:X` or
`:U`, and `cost(x::ℝ,[,costargs...]) → ℝ` if `class` is `:A`.
- `costargs::NTuple`
- `derivative::Int` 0, 1 or 2 - which derivative of the dof enters the cost
# Requestable internal variables
- `cost`, the value of the cost.
# Example
```
using Muscade
model = Model(:TestModel)
node = addnode!(model,𝕣[0,0])
e = addelement!(model,SingleDofCost,[node];class=:X,field=:tx,
costargs=(3.,),cost=(x,t,three)->(x/three)^2)
```
See also: [`DofCost`](@ref), [`ElementCost`](@ref)
"""
struct SingleDofCost <: AbstractElement end
function SingleDofCost(nod::Vector{Node};class::Symbol,field::Symbol,cost::Function,derivative=0::𝕫,costargs=())
∂=∂n(derivative)
if class==:X; DofCost(nod;xinod=(1,),xfield=(field,),class=:I,cost=(X,U,A,t,args...)->cost(∂(X)[1],t,args...),costargs)
elseif class==:U; DofCost(nod;uinod=(1,),ufield=(field,),class=:I,cost=(X,U,A,t,args...)->cost(∂(U)[1],t,args...),costargs)
elseif class==:A; DofCost(nod;ainod=(1,),afield=(field,),class=:A,cost=( A, args...)->cost(A[1] ,args...),costargs)
else muscadeerror("'class' must be :X,:U or :A")
end
end
#-------------------------------------------------
"""
DofLoad{Tvalue,Field} <: AbstractElement
An element to apply a loading term to a single X-dof.
# Named arguments to the constructor
- `field::Symbol`.
- `value::Function`, where `value(t::ℝ) → ℝ`.
# Requestable internal variables
- `F`, the value of the load.
# Examples
```
using Muscade
model = Model(:TestModel)
node = addnode!(model,𝕣[0,0])
e = addelement!(model,DofLoad,[node];field=:tx,value=t->3t-1)
```
See also: [`Hold`](@ref), [`DofCost`](@ref)
"""
struct DofLoad{Field,Tvalue,Targs} <: AbstractElement
value :: Tvalue # Function
args :: Targs
end
DofLoad(nod::Vector{Node};field::Symbol,value::Tvalue,args...) where{Tvalue<:Function} = DofLoad{field,Tvalue,typeof(args)}(value,args)
doflist(::Type{<:DofLoad{Field}}) where{Field}=(inod=(1,), class=(:X,), field=(Field,))
@espy function residual(o::DofLoad, X,U,A,t,χ,χcv,SP,dbg)
☼F = o.value(t,o.args...)
return SVector{1}(-F),noχ,noFB
end
#-------------------------------------------------
#McCormick(a,b)= α->a*exp(-(α/b)^2) # provided as input to solvers, used by their Addin
decided(λ,g,γ) = abs(VALUE(λ)-VALUE(g))/γ # used by constraint elements
S(λ,g,γ) = (g+λ-hypot(g-λ,2γ))/2 # Modified interior point method's take on KKT's-complementary slackness
KKT(λ::𝕣 ,g::𝕣 ,γ::𝕣,λₛ,gₛ) = 0 # A pseudo-potential with strange derivatives
KKT(λ::∂ℝ{P,N,R},g::∂ℝ{P,N,R},γ::𝕣,λₛ,gₛ) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}(0, λ.x*g.dx + gₛ*S(λ.x/λₛ,g.x/gₛ,γ)*λ.dx)
KKT(λ:: ℝ ,g::∂ℝ{P,N,R},γ::𝕣,λₛ,gₛ) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}(0, λ.x*g.dx )
KKT(λ:: 𝕣 ,g::∂ℝ{P,N,R},γ::𝕣,λₛ,gₛ) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}(0, λ *g.dx )
KKT(λ::∂ℝ{P,N,R},g:: ℝ ,γ::𝕣,λₛ,gₛ) where{P,N,R<:ℝ} = ∂ℝ{P,N,R}(0, gₛ*S(λ.x/λₛ,g.x/gₛ,γ)*λ.dx)
function KKT(λ::∂ℝ{Pλ,Nλ,Rλ},g::∂ℝ{Pg,Ng,Rg},γ::𝕣,λₛ,gₛ) where{Pλ,Pg,Nλ,Ng,Rλ<:ℝ,Rg<:ℝ}
if Pλ==Pg
R = promote_type(Rλ,Rg)
return ∂ℝ{Pλ,Nλ}(convert(R,KKT(λ.x,g.x,γ,λₛ,gₛ)),convert.(R, λ.x*g.dx + gₛ*S(λ.x/λₛ,g.x/gₛ,γ)*λ.dx))
elseif Pλ> Pg
R = promote_type(Rλ,typeof(b))
return ∂ℝ{Pλ,Nλ}(convert(R,KKT(λ ,g.x,γ,λₛ,gₛ)),convert.(R, λ.x*g.dx ))
else
R = promote_type(typeof(a),Rg)
return ∂ℝ{Pg,Ng}(convert(R,KKT(λ.x,g ,γ,λₛ,gₛ)),convert.(R, gₛ*S(λ.x/λₛ,g.x/gₛ,γ)*λ.dx))
end
end
#-------------------------------------------------
"""
off(t) → :off
A function which for any value `t` returns the symbol `off`. Usefull for specifying
the keyword argument `mode=off` in adding an element of type ``DofConstraint` to
a `Model`.
See also: [`DofConstraint`](@ref), [`ElementConstraint`](@ref), [`equal`](@ref), [`positive`](@ref)
"""
off(t) = :off
"""
equal(t) → :equal
A function which for any value `t` returns the symbol `equal`. Usefull for specifying
the keyword argument `mode=equal` in adding an element of type ``DofConstraint` to
a `Model`.
See also: [`DofConstraint`](@ref), [`ElementConstraint`](@ref), [`off`](@ref), [`positive`](@ref)
"""
equal(t) = :equal
"""
positive(t) → :positive
A function which for any value `t` returns the symbol `positive`. Usefull for specifying
the keyword argument `mode=positive` in adding an element of type ``DofConstraint` to
a `Model`.
See also: [`DofConstraint`](@ref), [`ElementConstraint`](@ref), [`off`](@ref), [`equal`](@ref)
"""
positive(t) = :positive
"""
DofConstraint{λclass,Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,
afield,λinod,λfield,Tg,Tmode} <: AbstractElement
An element to apply physical/optimisation equality/inequality constraints on dofs.
The constraints are holonomic, i.e. they apply to the values, not the time derivatives, of the involved dofs.
This element is very general but not very user-friendly to construct, factory functions are provided for better useability.
The sign convention is that the gap `g≥0` and the Lagrange multiplier `λ≥0`.
This element can generate three classes of constraints, depending on the input argument `λclass`.
- `λclass=:X` Physical constraint. In mechanics, the Lagrange multiplier dof is a
generalized force, dual of the gap. The gap function must be of the form `gap(x,t,gargs...)`.
- `λclass=:U` Time varying optimisation constraint. For example: find `A`-parameters so that
at all times, the response does not exceed a given criteria. The gap function must be of the form
`gap(x,u,a,t,gargs...)`.
- `λclass=:A` Time invariant optimisation constraint. For example: find `A`-parameters such that
`A[1]+A[2]=gargs.somevalue`. The gap function must be of the form `gap(a,gargs...)`.
# Named arguments to the constructor
- `xinod::NTuple{Nx,𝕫}=()` For each X-dof to be constrained, its element-node number.
- `xfield::NTuple{Nx,Symbol}=()` For each X-dof to be constrained, its field.
- `uinod::NTuple{Nu,𝕫}=()` For each U-dof to be constrained, its element-node number.
- `ufield::NTuple{Nu,Symbol}=()` For each U-dof to be constrained, its field.
- `ainod::NTuple{Na,𝕫}=()` For each A-dof to be constrained, its element-node number.
- `afield::NTuple{Na,Symbol}=()` For each A-dof to be constrained, its field.
- `λinod::𝕫` The element-node number of the Lagrange multiplier.
- `λclass::Symbol` The class (`:X`,`:U` or `:A`) of the Lagrange multiplier.
See the explanation above for classes of constraints
- `λfield::Symbol` The field of the Lagrange multiplier.
- `gₛ::𝕣=1.` A scale for the gap.
- `λₛ::𝕣=1.` A scale for the Lagrange multiplier.
- `gap::Function` The gap function.
- `gargs::NTuple` Additional inputs to the gap function.
- `mode::Function` where `mode(t::ℝ) -> Symbol`, with value `:equal`,
`:positive` or `:off` at any time. An `:off` constraint
will set the Lagrange multiplier to zero.
# Example
```jldoctest; output = false
using Muscade
model = Model(:TestModel)
n1 = addnode!(model,𝕣[0])
e1 = addelement!(model,DofConstraint,[n1],xinod=(1,),xfield=(:t1,),
λinod=1, λclass=:X, λfield=:λ1,gap=(x,t)->x[1]+.1,
mode=positive)
e2 = addelement!(model,QuickFix ,[n1],inod=(1,),field=(:t1,),
res=(x,u,a,t)->0.4x.+.08+.5x.^2)
initialstate = initialize!(model)
state = solve(StaticX;initialstate,time=[0.],verbose=false)
X = state[1].X[1]
# output
2-element Vector{Float64}:
-0.09999997108612142
0.04500000867027695
```
See also: [`Hold`](@ref), [`ElementConstraint`](@ref), [`off`](@ref), [`equal`](@ref), [`positive`](@ref)
"""
struct DofConstraint{λclass,Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield,λinod,λfield,Tg,Tgargs,Tmode} <: AbstractElement
gap :: Tg # Class==:X gap(x,t,gargs...) ,Class==:U gap(x,u,a,t,gargs...), Class==:A gap(a,gargs...)
gargs :: Tgargs
mode :: Tmode # mode(t)->symbol, or Symbol for Aconstraints
gₛ :: 𝕣
λₛ :: 𝕣
end
function DofConstraint(nod::Vector{Node};xinod::NTuple{Nx,𝕫}=(),xfield::NTuple{Nx,Symbol}=(),
uinod::NTuple{Nu,𝕫}=(),ufield::NTuple{Nu,Symbol}=(),
ainod::NTuple{Na,𝕫}=(),afield::NTuple{Na,Symbol}=(),
λinod::𝕫, λclass::Symbol, λfield::Symbol,
gₛ::𝕣=1.,λₛ::𝕣=1.,
gap::Function ,gargs=(),mode::Function) where{Nx,Nu,Na}
(λclass==:X && (Nu>0||Na>0)) && muscadeerror("Constraints with λclass=:X must have zero U-dofs and zero A-dofs")
(λclass==:A && (Nx>0||Nu>0)) && muscadeerror("Constraints with λclass=:A must have zero X-dofs and zero U-dofs")
return DofConstraint{λclass,Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield,λinod,λfield,
typeof(gap),typeof(gargs),typeof(mode)}(gap,gargs,mode,gₛ,λₛ)
end
doflist(::Type{<:DofConstraint{λclass,Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield,λinod,λfield}}) where
{λclass,Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield,λinod,λfield} =
(inod =(xinod... ,uinod... ,ainod... ,λinod ),
class=(ntuple(i->:X,Nx)...,ntuple(i->:U,Nu)...,ntuple(i->:A,Na)...,λclass),
field=(xfield... ,ufield... ,afield... ,λfield))
@espy function residual(o::DofConstraint{:X,Nx}, X,U,A,t,χ,χcv,SP,dbg) where{Nx}
γ = default{:γ}(SP,0.)
P,gₛ,λₛ = constants(∂0(X)),o.gₛ,o.λₛ
x,☼λ = ∂0(X)[SVector{Nx}(1:Nx)], ∂0(X)[Nx+1]
x∂ = variate{P,Nx}(x)
☼gap,g∂x = value_∂{P,Nx}(o.gap(x∂,t,o.gargs...))
if o.mode(t)==:equal; return SVector{Nx+1}(( -g∂x*λ)...,-gap ) ,noχ,(α=∞ ,)
elseif o.mode(t)==:positive; return SVector{Nx+1}(( -g∂x*λ)...,-gₛ*S(λ/λₛ,gap/gₛ,γ)) ,noχ,(α=decided(λ/λₛ,gap/gₛ,γ),)
elseif o.mode(t)==:off; return SVector{Nx+1}(ntuple(i->0,Nx)...,-gₛ/λₛ*λ ) ,noχ,(α=∞ ,)
end
end
@espy function lagrangian(o::DofConstraint{:U,Nx,Nu,Na}, Λ,X,U,A,t,χ,χcv,SP,dbg) where{Nx,Nu,Na}
γ = default{:γ}(SP,0.)
x,u,a,☼λ = ∂0(X),∂0(U)[SVector{Nu}(1:Nu)],A,∂0(U)[Nu+1]
☼gap = o.gap(x,u,a,t,o.gargs...)
if o.mode(t)==:equal; return -gap*λ ,noχ,(α=∞ ,)
elseif o.mode(t)==:positive; return -KKT(λ,gap,γ,o.λₛ,o.gₛ) ,noχ,(α=decided(λ/o.λₛ,gap/o.gₛ,γ),)
elseif o.mode(t)==:off; return -o.gₛ/(2o.λₛ)*λ^2 ,noχ,(α=∞ ,)
end
end
@espy function lagrangian(o::DofConstraint{:A,Nx,Nu,Na}, Λ,X,U,A,t,χ,χcv,SP,dbg) where{Nx,Nu,Na}
γ = default{:γ}(SP,0.)
a,☼λ = A[SVector{Na}(1:Na)],A[ Na+1]
☼gap = o.gap(a,o.gargs...)
if o.mode(t)==:equal; return -gap*λ ,noχ,(α=∞ ,)
elseif o.mode(t)==:positive; return -KKT(λ,gap,γ,o.λₛ,o.gₛ) ,noχ,(α=decided(λ/o.λₛ,gap/o.gₛ,γ),)
elseif o.mode(t)==:off; return -o.gₛ/(2o.λₛ)*λ^2 ,noχ,(α=∞ ,)
end
end
#-------------------------------------------------
"""
Hold <: AbstractElement
An element to set a single X-dof to zero.
# Named arguments to the constructor
- `field::Symbol`. The field of the X-dof to constraint.
- `λfield::Symbol=Symbol(:λ,field)`. The field of the Lagrange multiplier.
# Example
```
using Muscade
model = Model(:TestModel)
node = addnode!(model,𝕣[0,0])
e = addelement!(model,Hold,[node];field=:tx)
```
See also: [`DofConstraint`](@ref), [`DofLoad`](@ref), [`DofCost`](@ref)
"""
struct Hold <: AbstractElement end
function Hold(nod::Vector{Node};field::Symbol,λfield::Symbol=Symbol(:λ,field))
gap(v,t)=v[1]
return DofConstraint{:X ,1, 0, 0, (1,),(field,),(), (), (), (), 1, λfield, typeof(gap),typeof(()),typeof(equal)}(gap,(),equal,1.,1.)
end
#-------------------------------------------------
"""
ElementConstraint{Teleobj,λinod,λfield,Nu,Treq,Tg,Tgargs,Tmode} <: AbstractElement
An element to apply optimisation equality/inequality constraints on the element-results of
another "target" element. The target element must *not* be added separatly to the model. Instead, the
`ElementType`, and the named arguments to the target element are provided as input to the
`ElementConstraint` constructor.
This element generates a time varying optimisation constraint. For example: find `A`-parameters so that
at all times, the element-result von-Mises stress does not exceed a given value.
The Lagrangian multiplier introduced by this optimisation constraint is of class :U
# Named arguments to the constructor
- `λinod::𝕫` The element-node number of the Lagrange multiplier.
- `λfield::Symbol` The field of the Lagrange multiplier.
- `req` A request for element-results to be extracted from the target element, see [`@request`](@ref).
The request is formulated as if adressed directly to the target element (no "prefixing" with
`eleres`, see "Requestable internal variables")
- `gₛ::𝕣=1.` A scale for the gap.
- `λₛ::𝕣=1.` A scale for the Lagrange multiplier.
- `gap` a gap function `gap(eleres,X,U,A,t,gargs...)→ℝ`
`X` and `U` are tuples (derivates of dofs...), and `∂0(X)`,`∂1(X)`,`∂2(X)`
must be used by `cost` to access the value and derivatives of `X` (resp. `U`).
`X`, `U` and `A` are the degrees of freedom of the element `ElementType`.
- `gargs::NTuple` Additional inputs to the gap function.
- `mode::Function` where `mode(t::ℝ) -> Symbol`, with value `:equal`,
`:positive` or `:off` at any time. An `:off` constraint
will set the Lagrange multiplier to zero.
- `ElementType` The named of the constructor for the relevant element
- `elementkwargs...` Additional named arguments to the `ElementCost` constructor are passed on to the `ElementType` constructor.
# Requestable internal variables
From `ElementConstraint` one can request
- `λ` The constraints Lagrange multiplier
- `gap` The constraints gap function
From the target element on can request
- `eleres(...)` where `...` is the list of requestables from the target element. It must be "prefixed" by
`eleres` to prevent possible confusion with variables requestable from `ElementConstraint`.
# Example
```
@once gap(eleres,X,U,A,t) = eleres.Fh^2
ele1 = addelement!(model,ElementCoonstraint,[nod1];req=@request(Fh),
gap,λinod=1,λfield=:λ,mode=equal,
ElementType=AnchorLine,Δxₘtop=[5.,0,0], xₘbot=[250.,0],
L=290., buoyancy=-5e3)
```
See also: [`Hold`](@ref), [`DofConstraint`](@ref), [`off`](@ref), [`equal`](@ref), [`positive`](@ref), [`@request`](@ref)
"""
struct ElementConstraint{Teleobj,λinod,λfield,Nu,Treq,Tg,Tgargs,Tmode} <: AbstractElement
eleobj :: Teleobj
req :: Treq
gap :: Tg
gargs :: Tgargs
mode :: Tmode
gₛ :: 𝕣
λₛ :: 𝕣
end
function ElementConstraint(nod::Vector{Node};λinod::𝕫, λfield::Symbol,
req,gap::Function,gargs=(;),mode::Function,gₛ::𝕣=1.,λₛ::𝕣=1.,ElementType,elementkwargs...)
eleobj = ElementType(nod;elementkwargs...)
Nu = getndof(typeof(eleobj),:U)
return ElementConstraint{typeof(eleobj),λinod,λfield,Nu,typeof((eleres=req,)),typeof(gap),typeof(gargs),typeof(mode)}(eleobj,(eleres=req,),gap,gargs,mode,gₛ,λₛ)
end
doflist( ::Type{<:ElementConstraint{Teleobj,λinod,λfield}}) where{Teleobj,λinod,λfield} =
(inod =(doflist(Teleobj).inod... ,λinod),
class=(doflist(Teleobj).class...,:U),
field=(doflist(Teleobj).field...,λfield))
@espy function lagrangian(o::ElementConstraint{Teleobj,λinod,λfield,Nu}, Λ,X,U,A,t,χ,χcv,SP,dbg) where{Teleobj,λinod,λfield,Nu}
req = merge(o.req)
γ = default{:γ}(SP,0.)
u = getsomedofs(U,SVector{Nu}(1:Nu))
☼λ = ∂0(U)[Nu+1]
L,χn,FB,☼eleres = getlagrangian(implemented(o.eleobj)...,o.eleobj,Λ,X,u,A,t,χ,χcv,SP,(dbg...,via=ElementConstraint),req.eleres)
☼gap = o.gap(eleres,X,u,A,t,o.gargs...)
if o.mode(t)==:equal; return L-gap*λ ,noχ,(α=∞ ,)
elseif o.mode(t)==:positive; return L-KKT(λ,gap,γ,o.λₛ,o.gₛ) ,noχ,(α=decided(λ/o.λₛ,gap/o.gₛ,γ),)
elseif o.mode(t)==:off; return L-o.gₛ/(2o.λₛ)*λ^2 ,noχ,(α=∞ ,)
end
end
#-------------------------------------------------
"""
QuickFix <: AbstractElement
An element for creating simple elements with "one line" of code.
Elements thus created have several limitations:
- no internal state.
- no initialisation.
- physical elements with only X-dofs.
- only `R` can be espied.
The element is intended for testing. Muscade-based applications should not include this in their API.
# Named arguments to the constructor
- `inod::NTuple{Nx,𝕫}`. The element-node numbers of the X-dofs.
- `field::NTuple{Nx,Symbol}`. The fields of the X-dofs.
- `res::Function`, where `res(X::ℝ1,X′::ℝ1,X″::ℝ1,t::ℝ) → ℝ1`, the residual.
# Examples
A one-dimensional linear elastic spring with stiffness 2.
```jldoctest; output = false
using Muscade
model = Model(:TestModel)
node1 = addnode!(model,𝕣[0])
node2 = addnode!(model,𝕣[1])
e = addelement!(model,QuickFix,[node1,node2];inod=(1,2),field=(:tx1,:tx1),
res=(X,X′,X″,t)->Svector{2}(2*(X[1]-X[2]),2*(X[2]-X[1])) )
# output
Muscade.EleID(1, 1)
```
"""
struct QuickFix{Nx,inod,field,Tres} <: AbstractElement
res :: Tres # R = res(X,X′,X″,t)
end
QuickFix(nod::Vector{Node};inod::NTuple{Nx,𝕫},field::NTuple{Nx,Symbol},res::Function) where{Nx} = QuickFix{Nx,inod,field,typeof(res)}(res)
doflist(::Type{<:QuickFix{Nx,inod,field}}) where{Nx,inod,field} = (inod =inod,class=ntuple(i->:X,Nx),field=(field))
@espy function residual(o::QuickFix, X,U,A, t,χ,χcv,SP,dbg)
☼R = o.res(∂0(X),∂1(X),∂2(X),t)
return R,noχ,noFB
end
#-------------------------------------------------
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 5671 |
# by Philippe Mainçon
#
# In VScode, use the "Fast Unicode math characters" plugging
# To interrupt Julia, CTRL-j,k
using Printf,StaticArrays
import Base.Threads.@spawn, Base.Threads.nthreads
## Basic types
# abstract types for dispatch
"""
𝔹 (\\bbB)
an alias for `Bool`. For use in dispatching.
`𝔹1`... `𝔹4` are `AbstractArrays` of dimensions 1 to 4.
"""
const 𝔹 = Bool # \bbB
"""
ℕ (\\bbN)
an alias for `UInt64`. For use in dispatching.
`ℕ1`... `ℕ4` are `AbstractArrays` of dimensions 1 to 4.
"""
const ℕ = UInt64
"""
ℤ (\\bbZ)
an alias for abstract type `Integer`. For use in dispatching.
`ℤ1`... `ℤ4` are `AbstractArrays` of dimensions 1 to 4.
`ℤ11` is an `AbstractVector` of `AbstractVector`.
"""
const ℤ = Integer
"""
ℝ (\\bbR)
an alias for abstract type `Real`. For use in dispatching.
`ℝ1`... `ℝ4` are `AbstractArrays` of dimensions 1 to 4.
`ℝ11` is an `AbstractVector` of `AbstractVector`.
"""
const ℝ = Real
# concrete types for allocation
"""
𝕓 (\\bbb)
an alias for `Bool`. For use in `struct` definitions.
`𝕓1`... `𝕓4` are `Arrays` of dimensions 1 to 4.
"""
const 𝕓 = Bool
"""
𝕟 (\\bbn)
an alias for `UInt64`. For use in `struct` definitions.
`𝕟1`... `𝕟4` are `Arrays` of dimensions 1 to 4.
"""
const 𝕟 = UInt64
"""
𝕫 (\\bbz)
an alias for `Int64`. For use in `struct` definitions.
`𝕫1`... `𝕫4` are `Arrays` of dimensions 1 to 4.
`𝕫11` is a `Vector` of `Vector`.
"""
const 𝕫 = Int64
"""
𝕣 (\\bbr)
an alias for `Float64`. For use in `struct` definitions.
`𝕣1`... `𝕣4` are `Arrays` of dimensions 1 to 4.
`𝕣11` is a `Vector` of `Vector`.
"""
const 𝕣 = Float64
"""
ϵ (\\epsilon)
an alias for `Base.eps(𝕣)`.
"""
const ϵ = Base.eps(𝕣)
"""
∞ (\\infty)
an alias for `Base.inf`.
"""
const ∞ = Base.Inf
# define arrays of these
for T in (:𝔹,:ℕ,:ℤ,:ℝ)
#@eval export $T
@eval const $(Symbol(T,:x)) = AbstractArray{t} where {t<: $T}
#@eval export $(Symbol(T,:x))
for N in (:1,:2,:3,:4)
TN = Symbol(T,N)
@eval const $TN{t} = AbstractArray{t,$N} where {t<: $T}
#@eval export $TN
end
end
for T in (:𝕓,:𝕟,:𝕫,:𝕣)
#@eval export $T
Ts = Symbol(T,:s)
for N in (:1,:2,:3,:4)
TN = Symbol(T,N)
@eval const $TN = Array{$T,$N}
#@eval export $TN
end
end
const ℝ11 = AbstractVector{A} where {A<:ℝ1}
const ℤ11 = AbstractVector{A} where {A<:ℤ1}
const 𝕣11 = Vector{Vector{𝕣}}
const 𝕫11 = Vector{Vector{𝕫}}
## Miscellaneous
subtypeof(a::AbstractVector,b::AbstractVector) = a[a .<: Union{b...}]
# Given a variable, or its type, e.g. SMatrix{S,T}, get the name of the constructor, e.g. SMatrix
constructor(T::DataType) = T.name.wrapper
constructor(x::T) where{T} = T.name.wrapper
"""
toggle(condition,a,b)
Typestable equivalent of `condition ? a : b`.
Returns a value converted to `promote_type(typeof(a),typeof(b))`
"""
toggle(cond::Bool,a::Ta,b::Tb) where{Ta,Tb} = convert(promote_type(Ta,Tb), cond ? a : b)
# macro toggle(cond,a,b) # evaluate only a or only b
# return :(convert(promote_type(typeof($a),typeof($b)), $cond ? $a : $b))
# end
getval(::Val{v}) where{v} = v
## Array handling
flat(a) = reshape(a,length(a))
# flatten a vector of vectors of identical size, but lead a matrix as-is
consolidate(a) = a
consolidate(a::AbstractVector{E}) where{E<:AbstractVector{T}} where{T} = reduce(hcat,a)
# same as 'unique', but returns also idx, a vector of vectors, index into v of the uniques
function uniques(v::AbstractVector{T}) where{T}
u = Vector{T }()
idx = Vector{Vector{𝕫}}()
for (i,x) ∈ enumerate(v)
if x ∉ u
push!(u ,x )
push!(idx,findall([x==w for w∈v]))
end
end
return u,idx
end
function showtime(t)
return if t<1e-6
@sprintf " %3d [ns]" round(Int,t*1e9)
elseif t<1e-3
@sprintf " %3d [μs]" round(Int,t*1e6)
elseif t<9
@sprintf " %3d [ms]" round(Int,t*1e3)
elseif t<9*3600
@sprintf "%4d [s] " round(Int,t)
else
@sprintf "%4d [h] " round(Int,t/3600)
end
end
# if a function f is given the argument pointer= Ref{SomeType}()
# the function can then do e.g. vec=allocate(pointer,Vector...) and write to vec.
# and the caller retrievs the data with vec = pointer[]
# advantage over "return vec" is if f throws, then vec still contains some data.
const Pointer = Base.RefValue
#function allocate(pointer::Pointer{T},target::T) where{T}
function allocate(pointer::Pointer,target) # TODO use line above
pointer[]=target
return target
end
copies(n,a::T) where{T} = NTuple{n,T}(deepcopy(a) for i∈1:n)
using MacroTools: postwalk,gensym_ids,rmlines,unblock
"""
@once f(x)= x^2
do not parse the definition of function `f` again if not modified.
Using in a script, this prevents recompilations in `Muscade` or applications
based on it when they receive such functions as argument
"""
macro once(ex)
ex = postwalk(rmlines,ex)
ex = postwalk(unblock,ex)
qex = QuoteNode(ex)
ex = esc(ex)
tag = gensym("tag")
return quote
if ~@isdefined($tag) || $tag≠$qex
$tag = $qex
$ex
end
end
end
"""
default{:fieldname}(namedtuple,defval)
attempt to get a field `fieldname` from a `NamedTuple`. If `namedtuple` does not have
such a field - or is not a `NamedTuple`, return `defval`.
"""
struct default{S} end
default{S}(t::T,d=nothing) where{S,T<:NamedTuple} = hasfield(T,S) ? getfield(t,S) : d
default{S}(t::T,d=nothing) where{S,T } = d
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 2012 | using Base.Cartesian
#\circ \otimes
∘₀(a,b) = dots(a,b,Val(0))
∘₁(a,b) = dots(a,b,Val(1))
∘₂(a,b) = dots(a,b,Val(2))
Base. ∘(a::AbstractArray,b::AbstractArray) = dots(a,b,Val(1))
⊗(a,b) = dots(a,b,Val(0))
@generated function dots(a::AbstractArray{Ta,Na},b::AbstractArray{Tb,Nb},::Val{ndot}) where {Ta,Tb,Na,Nb,ndot}
# "Elrod", on Discourse/Julia, is acknowledged for the forerunner to this code
Nar = Na - ndot
Nbr = Nb - ndot
Nc = Nar+ Nbr
Nloop = Nc + ndot
Tc = promote_type(Ta,Tb)
if Nc > 0
return quote
sc = Base.@ntuple $Nc i -> begin
i <= $Nar ? size(a)[i] : size(b)[i - $Nar + $ndot]
end
c = zeros($Tc, sc)
@nloops $Nloop i j -> begin
j <= $Na ? axes(a,j) : axes(b,j - $Na + $ndot)
end begin
(@nref $Nc c j -> j <= $Nar ? i_{j} : i_{j+$ndot} ) += (@nref $Na a i) * (@nref $Nb b j -> i_{j+$Nar})
end
return c
end
else # scalar products
Tc = promote_type(Ta,Tb)
return quote
c = zero($Tc)
@nloops $Na i a begin
c += (@nref $Na a i) * (@nref $Na b i)
end
return c
end
end
end
dots(a:: Ta ,b::AbstractArray{Tb,Nb},::Val{0}) where {Ta<:Number,Tb<:Number ,Nb} = a*b
dots(a::AbstractArray{Ta,Na},b:: Tb ,::Val{0}) where {Ta<:Number,Tb<:Number,Na } = a*b
dots(a:: Ta ,b:: Tb ,::Val{0}) where {Ta<:Number,Tb<:Number } = a*b
# Accelerators
dots(a::AbstractMatrix{Ta},b::AbstractMatrix{Tb},::Val{1}) where {Ta<:Number,Tb<:Number } = a*b
dots(a::AbstractVector{Ta},b::AbstractMatrix{Tb},::Val{1}) where {Ta<:Number,Tb<:Number } = b'*a
dots(a::AbstractMatrix{Ta},b::AbstractVector{Tb},::Val{1}) where {Ta<:Number,Tb<:Number } = a*b
dots(a::AbstractVector{Ta},b::AbstractVector{Tb},::Val{0}) where {Ta<:Number,Tb<:Number } = a*b'
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 2699 | using Printf
"""
L,Lλ,Lx,Lu,La,χn = gradient(eleobj,Λ,X,U,A,t,χ,χcv,SP,dbg)
Compute the Lagrangian, its gradients, and the memory of an element.
For element debugging and testing.
See also: [`residual`](@ref),[`lagrangian`](@ref)
"""
function gradient(eleobj,Λ,X,U,A,t,χ,χcv,SP,dbg)
P = constants(Λ,∂0(X),∂0(U),A,t)
nX,nU,nA = length(Λ),length(∂0(U)),length(A)
N = 2nX+nU+nA
iΛ,iX,iU,iA = (1:nX) , (1:nX) .+ nX , (1:nU) .+ 2nX , (1:nA) .+ (2nX+nU)
ΔY = δ{P,N,𝕣}()
L,χn,FB = Muscade.getlagrangian(Muscade.implemented(eleobj)...,eleobj,Λ+ΔY[iΛ],(∂0(X)+ΔY[iX],),(∂0(U)+ΔY[iU],),A+ΔY[iA],t,χ,χcv,SP,dbg)
Ly = ∂{P,N}(L)
return (L=value{P}(L), Lλ=Ly[iΛ], Lx=Ly[iX], Lu=Ly[iU], La=Ly[iA],χn=χn)
end
"""
test_static_element(eleobj,δX,X,U,A,t=0,χ=nothing,χcv=identity,SP=nothing,verbose=true,dbg=(;))
Compute the Lagrangian, its gradients, and the memory of an element.
For element debugging and testing.
See also: [`residual`](@ref),[`lagrangian`](@ref),[`gradient`](@ref)
"""
function test_static_element(ele::eletyp; δX,X,U,A, t::Float64=0.,χ=nothing,
χcv::Function=identity,SP=nothing,verbose::Bool=true,dbg = NamedTuple()) where{eletyp<:AbstractElement}
inod,class,field = Muscade.getdoflist(eletyp)
iXdof = Muscade.getidof(eletyp,:X)
iUdof = Muscade.getidof(eletyp,:U)
iAdof = Muscade.getidof(eletyp,:A)
nX,nU,nA = Muscade.getndof(eletyp,(:X,:U,:A))
L,Lδx,Lx,Lu,La,χn = gradient(ele,δX,[X],[U],A, t,χ,χcv,SP,dbg)
if verbose
@printf "\nElement type: %s\n" typeof(el)
if nX > 0
@printf "\n idof doftyp inod δX X Lδx Lx \n"
for iX = 1:nX
idof = iXdof[iX]
@printf " %4d %16s %5d %10.3g %10.3g %10.3g %10.3g\n" idof field[idof] inod[idof] δX[idof] X[idof] Lδx[idof] Lx[idof]
end
end
if nU > 0
@printf "\n idof doftyp inod U Lu \n"
for iU = 1:nU
idof = iUdof[iU]
@printf " %4d %16s %5d %10.3g %10.3g\n" idof field[idof] inod[idof] U[idof] Lu[idof]
end
end
if nA > 0
@printf "\n idof doftyp inod A La \n"
for iA = 1:nA
idof = iAdof[iA]
@printf " %4d %16s %5d %10.3g %10.3g\n" idof field[idof] inod[idof] A[idof] La[idof]
end
end
end
return Lδx,Lx,Lu,La,χn
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 5429 |
"""
noχ
A constant, used by elements' `residual` or `lagrangian` as their 2nd output if they do not export
any material memory (for example, plastic strain).
Example:
`return L,noχ,noFB`
See also: [`noFB`](@ref)
"""
const noχ =nothing
"""
noFB
A constant, used by elements' `residual` or `lagrangian` as their 3rd output if they do provide
any feedback to the solver (for example, on the reduction of the barrier parameter in interior point method).
Example:
`return L,noχ,noFB`
See also: [`noFB`](@ref)
"""
const noFB=nothing
"""
position = ∂0(X)
Used by elements' `residual` or `lagrangian` to extract the zero-th order time derivative
from the variables `X` and `U`.
See also: [`∂1`](@ref),[`∂2`](@ref),[`getsomedofs`](@ref)
"""
∂0(y) = y[1]
"""
velocity = ∂1(X)
Used by elements' `residual` or `lagrangian` to extract the first order time derivative
from the variables `X` and `U`. Where the solver does not provide this derivative (e.g.
a static solver), the output is a vector of zeros.
See also: [`∂0`](@ref),[`∂2`](@ref),[`getsomedofs`](@ref)
"""
∂1(y) = length(y) ≥2 ? y[2] : zeros(SVector{length(y[1])})
"""
position = ∂2(X)
Used by elements' `residual` or `lagrangian` to extract the zero-th order time derivative
from the variables `X` and `U`. Where the solver does not provide this derivative (e.g.
a static solver), the output is a vector of zeros.
See also: [`∂0`](@ref),[`∂1`](@ref),[`getsomedofs`](@ref)
"""
∂2(y) = length(y) ≥3 ? y[2] : zeros(SVector{length(y[1])})
∂n(n) = (∂0,∂1,∂2)[n+1]
"""
rotations = getsomedofs(X,[3,6])
Used by elements' `residual` or `lagrangian` to some degrees of freedom, and their
time derivatives, from the variables `X` and `U`.
See also: [`∂0`](@ref),[`∂1`](@ref),[`∂2`](@ref)
"""
getsomedofs(A::NTuple{Nder,SVector},ind) where{Nder} = ntuple(i->A[i][ind],Nder)
#const Dof{Ndof,Nder} = NTuple{Nder,SVector{Ndof}}
"""
c = coord(node)
Used by element constructors to obtain the coordinates of a vector of Nodes handed by
Muscade to the constructor. `c` is accessed as
```
c[inod][icoord]
```
where `inod` is the element-node number and `icoord` an index into a vector of coordinates.
See also: [`addnode!`](@ref), [`addelement!`](@ref), [`describe`](@ref), [`solve`](@ref)
"""
coord(nod::AbstractVector{Node}) = [n.coord for n∈nod]
"""
Muscade.doflist(::Type{E<:AbstractElement})
Elements must overload Muscade's `doflist` function.
The method must take the element type as only input, and return
a `NamedTuple` with fieldnames `inod`,`class` and `field`. The tuple-fields
are `NTuple`s of the same length. For example
```
Muscade.doflist( ::Type{<:Turbine}) = (inod =(1 ,1 ,2 ,2 ),
class=(:X ,:X ,:A ,:A ),
field=(:tx1,:tx2,:Δseadrag,:Δskydrag))
```
In `Λ`, `X`, `U` and `A` handed by Muscade to `residual` or `lagrangian`,
the dofs in the vectors will follow the order in the doflist. Element developers
are free to number their dofs by node, by field, or in any other way.
See also: [`lagrangian`](@ref), [`residual`](@ref)
"""
doflist( ::Type{E}) where{E<:AbstractElement} = muscadeerror(@sprintf("method 'Muscade.doflist' must be provided for elements of type '%s'\n",E))
"""
@espy function Muscade.lagrangian(eleobj::MyElement,Λ,X,U,A,t,χ,χcv,SP,dbg)
...
return L,χ,FB
end
# Inputs
- `eleobj` an element object
- `Λ` a `SVector{nXdof,R} where{R<:Real}`, Lagrange multipliers (aka `δX` virtual displacements).
- `X` a `NTuple` of `SVector{nXdof,R} where{R<:Real}`, containing the Xdofs and, depending on the solver,
their time derivatives. Use `x=∂0(X)`, `v=∂1(X)` and `a=∂2(X)` to safely obtain vectors of zeros
where the solver leaves time derivatives undefined.
- `U` a `NTuple` of `SVector{nUdof,R} where{R<:Real}`, containing the Udofs and, depending on the solver,
their time derivatives. Use `u=∂0(U)`, `̇u=∂1(U)` and `̈u=∂2(U)` to safely obtain vectors of zeros
where the solver leaves time derivatives undefined.
- `A` a `SVector{nAdof,R} where{R<:Real}`.
- `t` a ``Real` containing the time.
- `χ` the element memory
- `χcv` a function used to built the updated element memory.
- `SP` solver parameters (for example: the barrier parameter `γ` for
interior point methods).
- `dbg` a `NamedTuple` to be used _only_ for debugging purposes.
# Outputs
- `L` the lagrangian
- `χ` an updated element memory. Return `noχ` if the element has no memory.
- `FB` feedback from the element to the solver (for example: can `γ` be
reduced?). Return `noFB` of the element has no feedback to provide.
See also: [`residual`](@ref), [`doflist`](@ref), [`@espy`](@ref), [`∂0`](@ref), [`∂1`](@ref), [`∂2`](@ref), [`noχ`](@ref), [`noFB`](@ref)
"""
lagrangian()=nothing
"""
@espy function Muscade.residual(eleobj::MyElement,X,U,A,t,χ,χcv,SP,dbg)
...
return R,χ,FB
end
The inputs and outputs to `residual` are the same as for `lagrangian` with two exceptions:
- `residual` does no have input `Λ`.
- the first output argument of `residual` is not a Lagrangian `L` but `R`,
a `SVector{nXdof,R} where{R<:Real}`, containing the element's contribution
to the residual of the equations to be solved.
See [`lagrangian`](@ref) for the rest of the inputs and outputs.
"""
residual()=nothing
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 15842 | using Printf,MacroTools
######################## Helper functions
☼tag(s::Symbol) = string(s)[1]=='☼' # \sun
☼tag(s) = false
♢tag(s::Symbol) = string(s)[1]=='♢' # \diamond
♢tag(s) = false
☼tail(s::Symbol) = Symbol(string(s)[4:end])
tail(s::Symbol) = Symbol(string(s)[2:end])
code_tuple(e...) = Expr(:tuple,e...)
const digits = Set("0123456789")
function newsym(name)
s = string(name)
if s[end]∈digits && s[end-1]∈digits && s[end-2]∈digits && s[end-3]=='_'
i = parse(Int64,s[end-2:end])
s = s[1:end-4]
return Symbol(s,"_",@sprintf("%03i",i+1))
else
return Symbol(s,"_001")
end
end
######################## Request definition
"""
req = @request expr
Create a request of internal results wanted from a function. Considering the function
presented as example for [`@espy`](@ref), examples of possible syntax include
```
req = @request gp(s,z,material(a,b))
req = @request gp(s)
req = @request gp(material(a))
```
The first expression can be read as follows: "In the function, there is a `do` loop over variable `igp`, and within this
loop a call to a function `material`. Results `s` and `z` are wanted from within the loop, and results `a` and `b`
from within `material`.
The corresponding datastructure containing the results for each element is a nesting of `NTuples` and `NamedTuples`,
and can be accessed as `out.gp[igp].material.a` and so forth.
See also: [`@espy`](@ref), [`@espydbg`](@ref)
"""
macro request(ex)
if @capture(ex,(args__,)); prettify(code_tuple(request_.(args)...))
elseif @capture(ex,name_(args__)); prettify(code_tuple(request_(ex)))
elseif @capture(ex,symbol_); prettify(code_tuple(request_(ex)))
else; muscadeerror("Not a valid request")
end
end
request_(ex::Symbol) = quote $ex=nothing end
request_(ex ) = @capture(ex,name_(args__)) ? quote $name=$(code_tuple(request_.(args)...)) end : muscadeerror("Not a valid request")
instantiate(::Type{Nothing} ) = nothing
instantiate(::Type{NamedTuple{k,F}}) where{k,F} = NamedTuple{k}(instantiate.(fieldtypes(F)))
"""
req = Muscade.merge(o.req)
Elements like [`ElementCost`](@ref) and [`ElementConstraint`](@ref) use requests to apply a cost or a constraint to
requestables from another "target" element. These cost/constraint elements must be coded carefully so that `getresult` can be used to extracted
both requestable internal results from the cost/constraint and from the target element.
`merge` (which is *not exported by Muscade*) is used to merge the requests for the request needed to enforce a cost or constraint, and the user's
request for element to be obtained from the analysis. The call to `merge`, to be inserted in the code of `lagrange` for the cost/constraint element
will be modified by `@espy`.
See the code of `ElementCost`'s constructor and `lagrange` method for an example.
See also: [`ElementCost`](@ref),[`@request`](@ref),[`getresult`](@ref)
"""
@inline merge(x) = x
merge(x , ::Nothing) = x
merge(::Nothing, x ) = x
merge(::Nothing, ::Nothing) = nothing
@generated function merge(NT1::NamedTuple, NT2::NamedTuple)
nt1 = instantiate(NT1)
nt2 = instantiate(NT2)
kout = union(keys(nt1), keys(nt2))
t = ntuple(length(kout)) do ikey
key = kout[ikey]
v1 = get(nt1, key, nothing)
v2 = get(nt2, key, nothing)
v = merge(v1, v2)
:($key = $v)
end
return code_tuple(t...)
return c
end
######################## Generate new function code
# ✓check,✔Check,∎QED,⋆star,♢diamond,☼sun,☐Box
spaces = "| | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | | "
function printtrace(trace::𝕫,s::String)
if trace < 0;return end
println(spaces[1:3trace],s)
end
## Clean code
function code_clean_function(ex::Expr )
if @capture(ex,@named(res__,))
Expr(:tuple,(:($s=$s) for s∈res)...)
elseif @capture(ex,left_=right_) && ♢tag(left)
:(nothing)
else
Expr(ex.head,[code_clean_function(a) for a ∈ ex.args]...)
end
end
code_clean_function(ex::Symbol) = ☼tag(ex) ? ☼tail(ex) : ex
code_clean_function(ex ) = ex
## Extractor code
# should such a function itself generate the new "out" variable?
function code_write_to_out(out,req,var,trace)
printtrace(trace,"code_write_to_out")
newout = newsym(out)
code = quote
$newout = haskey($req,$(QuoteNode(var))) ? ($out..., $var=$var) : $out
end
printtrace(trace,"done")
return code,newout
end
function code_merge_req(left,oreq,req,out,trace=-999999) # req = merge(o.req)
printtrace(trace,"code_merge_req")
code = quote
$left = merge($req,$oreq)
end
printtrace(trace,"done")
return code,out
end
function code_call(left,foo,args,req,out,trace=-999999) # left,... = foo(args)
printtrace(trace,"code_call")
out_new = newsym(out)
out_foo = newsym(out)
if left isa Symbol
leftout = code_tuple(left,out_foo)
elseif left isa Expr && left.head==:tuple
leftout = code_tuple(left.args...,out_foo)
else
muscadeerror("Espy breakdown")
end
code = quote
if haskey($req,$(QuoteNode(foo)))
$leftout = $foo($(args...),$req.$foo,)
$out_new = ($out...,$foo=$out_foo)
else
$left = $foo($(args...))
$out_new = $out
end
end
printtrace(trace,"done")
return code,out_new
end
function code_ntupledoloop(left,igp,ngp,body,req,out,trace=-999999) # foo(args) do var body end
printtrace(trace,"code_ntupledoloop")
gp = tail(igp)
req_gp = Symbol(req,"_",gp)
out_gp = Symbol(out,"_",gp)
out_new = newsym(out)
gpsym = QuoteNode(gp)
out_gp_new = out_gp
for i=1:length(body)-1
body[i],out_gp_new = code_recursion(body[i],out_gp_new,req_gp,trace+1)
end
if @capture(body[end],@named(res__,))
tup = Expr(:tuple,(:($s=$s) for s∈res)...) #@named(a,b) → (a=a,b=b)
push!(tup.args,:(out=$out_gp_new)) # → (a=a,b=b,out=out_gp_new)
code = quote
$req_gp = haskey($req,$gpsym) ? $req.$gp : nothing
$left = ntuple($ngp) do $igp
$out_gp = (;)
$(body[1:end-1]...)
$tup
end
$out_new = haskey($req,$gpsym) ? ($out..., $gp=NTuple{$ngp}($left[$igp].out for $igp=1:$ngp)) : $out
end
else muscadeerror("body of ntuple-do-loop must end with a NamedTuple containing the outputs of the iteration (;a=a) or (a=a,b=b)")
end
printtrace(trace,"done")
return code,out_new
end
function code_assigment_rhs(left,right,out,req,trace=-999999) # work with the rhs of an assigment, return the whole assigment
printtrace(trace,"code_assigment_rhs")
trace += 1
if @capture(right, merge(oreq_) )
printtrace(trace,"req = merge(o.req)")
code,out_new = code_merge_req(left,oreq,req,out,trace+1)
printtrace(trace,"done")
elseif @capture(right, foo_(args__) ) && ☼tag(foo)
printtrace(trace,"left = ☼foo(args)")
code,out_new = code_call(left,☼tail(foo),args,req,out,trace+1)
printtrace(trace,"done")
elseif @capture(right, mod_.foo_(args__) ) && ☼tag(foo)
printtrace(trace,"left = mod.☼foo(args)")
code,out_new = code_call(left,quote $mod.$(☼tail(foo)) end,args,req,out,trace+1)
printtrace(trace,"done")
elseif @capture(right, ntuple(ngp_) do igp_ body__ end)
printtrace(trace,"left = ntuple(ngp) do igp body end")
code,out_new = code_ntupledoloop(left,igp,ngp,body,req,out,trace+1)
printtrace(trace,"done")
else
printtrace(trace,"left = right")
code = quote
$left = $right
end
out_new = out
printtrace(trace,"done")
end
trace -= 1
printtrace(trace,"done")
return code,out_new
end
function code_recursion(ex::Expr,out,req,trace=-999999)
printtrace(trace,"code_recursion")
trace += 1
if @capture(ex, for i_=it_ body_ end) || # syntaxes which contain an assigment must not recurse the assigment because
@capture(ex, [a_ for i_=it_])
out_new = out # 1) anotation is forbidden in this context
code = ex # 2) processing an equality introduces a LineNumber, at an illegal place within the syntax
elseif @capture(ex, left_ = right_ )
printtrace(trace,"assign")
trace += 1
if ☼tag(left) # ☼a = ...
printtrace(trace,"☼a = ...")
left = ☼tail(left)
assigment,out_new = code_assigment_rhs(left,right,out,req,trace+1)
write,out_new = code_write_to_out(out_new,req,left,trace+1)
code = quote
$assigment
$write
$left
end
printtrace(trace,"done")
elseif @capture(left, (args__,) ) # (a,☼b) = ...
printtrace(trace,"(a,☼b) = ...")
write = Vector{Expr}(undef,0) # will contain the macros to insert
@capture(left, (args__,) )
left = code_clean_function(left)
assignement,out_new = code_assigment_rhs(left,right,out,req,trace+1)
for arg ∈ args
if ☼tag(arg)
arg = ☼tail(arg)
w,out_new = code_write_to_out(out_new,req,arg,trace+1)
push!(write,w) # @espy_record out req b
end
end
if length(write)==0
code = assignement
else
code = quote
$assignement
$(write...)
$left
end
end
printtrace(trace,"done")
elseif ♢tag(left) # ♢a = ...
printtrace(trace,"♢a = ...")
left = ☼tail(left)
varsym = QuoteNode(left)
assigment,out_tmp = code_assigment_rhs(left,right,out,req,trace+1)
out_new = newsym(out_tmp)
code = quote
if haskey($req,$varsym) # if haskey(req,:x)
$assigment
$out_new = ($out...,$left = $left)
else
$out_new = $out
end
end
printtrace(trace,"done")
else
printtrace(trace,"a = ...")
code,out_new = code_assigment_rhs(left,right,out,req,trace+1)
printtrace(trace,"done")
end
trace -= 1
printtrace(trace,"done assign")
elseif @capture(ex, return (args__,))
code = quote
return $(args...),$out
end
out_new = out
else
printtrace(trace,"Expr recursion")
args = ()
for a ∈ ex.args
codea,out = code_recursion(a,out,req,trace+1)
args = (args...,codea)
end
code = Expr(ex.head,args...)
out_new = out
printtrace(trace,"done Expr recursion")
end
trace -= 1
printtrace(trace,"done code_recursion")
return code,out_new
end
function code_recursion(ex,out,req,trace=-999999) # to handle line-number nodes, Symbols... etc.
printtrace(trace,"code_recursion (default)")
printtrace(trace,"done")
return ex,out
end
function code_espying_function(ex::Expr,out,req,trace=-999999) # ex must be a function declaration
printtrace(trace,"code_espying_function")
if @capture(ex, foo_(args__) = (body__,)) # oneliner functions can not have ☼ or ♢
return quote
$foo($(args...),req) = $(body...),nothing
end
elseif @capture(ex, foo_(args__) where T_= (body__,))
return quote
$foo($(args...),req) where $T = $(body...),nothing
end
else
dict = splitdef(ex)
dict[:body] = quote
$out = (;)
$(dict[:body])
end
push!(dict[:args],req)
dict[:body],out = code_recursion(dict[:body],out,req,trace+1)
printtrace(trace,"done")
return combinedef(dict)
end
end
"""
@espy function ... end
From an anotated function code, generate
- "clean" code, in which the anotations have been deleted, and with
the call syntax `argout... = foo(argin...)`
- "espying" code, with added input and ouput arguments
`argout...,res = foo(argin...,req)` where `req` has been
generated using `@request` and `res` is a nested structure
of `NamedTuple`s and `NTuple`s containing the requested data.
The macro is not general: it is designed for `residual` and `lagrangian`,
which for performance have to be programmed in "immutable" style: they must
never mutate variables (this implies in particular, no adding into
an array in a loop over Gauss points). So @espy only supports the specific
programming constructs needed in this style.
The following is an example of anotated code:
```
@espy function residual(x::Vector{R},y) where{R<:Real}
ngp=2
accum = ntuple(ngp) do igp
☼z = x[igp]+y[igp]
☼s,☼t = ☼material(z)
♢square = s^2
@named(s)
end
r = sum(i->accum[i].s,ngp)
return r,nothing,nothing
end
```
- The keyword `function` is preceded by the macro-call `@espy`.
- The name of requestable variables is preceded by `☼` (`\\sun`).
Such anotation must always appear on the left of an assigment.
- If the name of a variable is preceded by `♢` (`\\diamond`), then
the variable is evaluated only if requested. Such a notation can only
be used if there is only one variable left of the assignement.
- The name of a function being called must be preceded by `☼` if the
definition of the function is itself preceeded by the macro-call `@espy`.
- `for`-loops are not supported. `do`-loops must be used: to be efficient,
`residual` and `lagrangian` must not allocate and thus use immutables.
- The keyword `return` must be explicitly used, and if must be followed
the a comma separated list of output variables. Syntaxes like
`return if...` are not supported.
See also: [`@request`](@ref), [`@espydbg`](@ref), [`getresult`](@ref)
"""
macro espy(ex)
cntr = 0
esc(quote
$(code_clean_function(ex))
$(code_espying_function(ex,newsym(:out),newsym(:req)))
end)
end
"""
@espydbg function ... end
Generate the same code as [`@espy`](@ref) and print it (for debug purposes).
See also: [`@espy`](@ref), [`@request`](@ref)
"""
macro espydbg(ex)
cntr = 0
bold = true
printstyled("@espydbg: ";bold,color=:cyan)
printstyled("Clean code\n";bold,color=:green)
clean = code_clean_function(ex)
println(prettify(clean))
printstyled("\n@espydbg: ";bold,color=:cyan)
printstyled("Espying code\n";bold,color=:yellow)
espying = code_espying_function(ex,newsym(:out),newsym(:req))#,0) #trace
println(prettify(espying))
printstyled("\n@espydbg: ";bold,color=:cyan)
printstyled("Done\n\n";bold,color=:cyan)
esc(quote
$clean
$espying
end)
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 1327 |
struct MuscadeException <:Exception
msg::String
dbg::NamedTuple
end
"""
muscadeerror([[dbg,]msg])
Throw a `MuscadeException`, where
- `dbg` is a `NamedTuple` that contains "location information"
(for example: solver, step, iteration, element, quadrature point) that will be displayed with the error message.
- `msg` is a `String` describing the problem.
"""
muscadeerror(dbg::NamedTuple,msg) = throw(MuscadeException(msg,dbg))
muscadeerror(msg) = throw(MuscadeException(msg,(;)))
muscadeerror() = throw(MuscadeException("" ,(;)))
# relativebacktrace() = setdiff(catch_backtrace(),backtrace())[2:end-1]
function Base.showerror(io::IO, e::MuscadeException)
printstyled(io,"MuscadeException: ",color=:red,bold=true)
println(io, e.msg)
if e.dbg≠(;)
println(io,e.dbg)
end
end
report(::Exception) = rethrow()
function report(::MuscadeException)
print("\n\n")
cs = Base.catch_stack()
nex = length(cs)
for iex = 1:nex-1
showerror(stderr,cs[iex][1])
Base.show_backtrace(stdout,setdiff(cs[iex][2],cs[iex+1][2])[2:end-1])
print("\n\nthen caused ")
end
showerror(stderr,cs[nex][1])
Base.show_backtrace(stdout,setdiff(cs[nex][2],backtrace())[2:end-1])
print("\n\n")
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 17988 | using StaticArrays,Printf
# model datastructure - private, structure may change, use accessor functions
"""
AbstractElement
An abstract data type. An element type `MyElement` must be
declared as a subtype of `AbstractElement`.
`MyELement`must provide a constructor with interface
`eleobj = MyElement(nod::Vector{Node}; kwargs...)`
See also: [`coord`](@ref), [`Node`](@ref), [`lagrangian`](@ref)
"""
abstract type AbstractElement end
abstract type ID end
struct DofID <: ID
class :: Symbol # either :X,:U or :A
idof :: 𝕫 # index into model X,U or A
end
struct EleID <: ID
ieletyp :: 𝕫
iele :: 𝕫
end
struct NodID <: ID
inod :: 𝕫
end
struct Dof
ID :: DofID # global dof number, unique ID
nodID :: NodID # global number of associated node within class
idoftyp :: 𝕫
eleID :: Vector{EleID}
end
Dof1 = Vector{Dof}
"""
Node
The eltype of vectors handed by Muscade as first argument to element constructors.
Example:
`function SingleDofCost(nod::Vector{Node};class::Symbol, ... )`
See also: [`coord`](@ref)
"""
struct Node
ID :: NodID # global node number, unique ID
coord :: 𝕣1 # all nodes in a model have coordinates in same space
dofID :: Vector{DofID} # list of dofs on this node
eleID :: Vector{EleID} # list of elements connected to the node
end
struct Element
ID :: EleID # global element number, unique ID
ieletyp :: 𝕫
iele :: 𝕫 # number of element within type
nodID :: Vector{NodID}
dofID :: Vector{DofID}
end
mutable struct DofTyp
class :: Symbol # either :X,:U or :A
field :: Symbol # user defined. e.g. :rx1 :tx1
scale :: 𝕣
dofID :: Vector{DofID}
end
mutable struct Model
ID :: Symbol # analyses could have multiple models
nod :: Vector{Node} # model.nod[nodID]
ele :: Vector{Vector{Element}} # model.ele[eleID] or model.ele[ieletyp][iele]
dof :: @NamedTuple begin # model.dof[dofID] or model.dof.X[idof]
X::Dof1
U::Dof1
A::Dof1
end
eleobj :: Vector{Vector{E} where{E<:AbstractElement}} # model.ele[eleID]or model.eleobj[ieletyp][iele]
doftyp :: Vector{DofTyp} # model.doftyp[idoftyp]
scaleΛ :: 𝕣
locked :: 𝕓
end
# Model construction - private
firstindex(x) = any(x) ? findfirst(x) : 0
getidoftyp(model,class,field) = firstindex(doftyp.class==class && doftyp.field==field for doftyp∈model.doftyp)
getidoftyp(model,dofID::DofID) = model.dof[dofID].idoftyp
getieletyp(model,E) = firstindex(eltype(eleobj) == E for eleobj∈model.eleobj)
getdoftyp(model,args...) = model.doftyp[getidoftyp(model,args...)]
getneletyp(model) = length(model.ele)
Base.getindex(nod::AbstractArray,nodID::NodID) = nod[nodID.inod ]
Base.getindex(dof::NamedTuple{(:X,:U,:A), Tuple{Dof1, Dof1, Dof1}},dofID::DofID) = dof[dofID.class ][dofID.idof]
Base.getindex(ele::AbstractArray,eleID::EleID) = ele[eleID.ieletyp][eleID.iele]
Base.getindex(A ::AbstractArray,id::AbstractArray{ID}) = [A[i] for i ∈ id]
getidof(E::DataType,class) = findall(doflist(E).class.==class)
"""
getndof(model|Element)
getndof(model|Element,class)
getndof(model|Element,(class1,class2,[,...]))
where `class` can be any of `:X`, `:U`, `:A`: get the number of dofs of the
specified dof-classes (default: all classes) for the variable `model` or the type
`Element`.
See also: [`describe`](@ref)
"""
getndof(E::DataType) = length(doflist(E).inod)
getndof(E::DataType,class) = length(getidof(E,class))
getndof(E::DataType,class::Tuple) = ntuple(i->getndof(E,class[i]),length(class))
getndof(model::Model,class::Symbol) = length(model.dof[class])
getndof(model::Model,class::Tuple) = ntuple(i->getndof(model,class[i]),length(class))
getndof(model::Model) = sum(length(d) for d∈model.dof)
getnele(model::Model,ieletyp) = length(model.ele[ieletyp])
getnele(model::Model) = sum(length(e) for e∈model.ele)
newdofID(model::Model,class) = DofID(class ,getndof(model,class)+1)
neweleID(model::Model,ieletyp) = EleID(ieletyp,getndof(model,class)+1)
getnnod(E::DataType) = maximum(doflist(E).inod)
getdoflist(E::DataType) = doflist(E).inod, doflist(E).class, doflist(E).field
function getdoftyp(model::Model,class::Symbol,field::Symbol)
idoftyp = findfirst(doftyp.class==class && doftyp.field==field for doftyp∈model.doftyp)
isnothing(idoftyp) && muscadeerror(@sprintf("The model has no dof of class %s and field %s.",class,field))
return model.doftyp[idoftyp]
end
getdofID(model::Model,class::Symbol,field::Symbol) = getdoftyp(model,class,field).dofID
function getdofID(model::Model,class::Symbol,field::Symbol,nodID::AbstractVector{NodID})
dofID = getdofID(model,class,field)
i = [model.dof[d].nodID ∈ nodID for d ∈ dofID]
return dofID[i]
end
# Model construction - API
"""
model = Model([ID=:my_model])
Construct a blank `model`, which will be mutated to create a finite element [optimization] problem.
See also: [`addnode!`](@ref), [`addelement!`](@ref), [`describe`](@ref), [`solve`](@ref)
"""
Model(ID=:muscade_model::Symbol) = Model(ID, Vector{Node}(),Vector{Vector{Element}}(),(X=Dof1(),U=Dof1(),A=Dof1()),Vector{Any}(),Vector{DofTyp}(),1.,false)
"""
nodid = addnode!(model,coord)
If `coord` is an `AbstractVector` of `Real`: add a single node to the model.
Muscade does not prescribe what coordinate system to use. Muscade will handle
to each element the `coord` of the nodes of the element, and the element
constructor must be able to make sense of it. `nodid` is a node identifier, that
is used as input to `addelement!`.
If `coord` is an `AbstractMatrix`, its rows are treated as vectors of coordinates.
`nodid` is then a vector of node identifiers.
See also: [`addelement!`](@ref), [`coord`](@ref) , [`describe`](@ref)
"""
function addnode!(model::Model,coord::ℝ2)
assert_unlocked(model)
Δnnod = size(coord,1)
nodID = [NodID(length(model.nod)+inod) for inod ∈ 1:Δnnod]
append!(model.nod, [Node(nodID[inod],coord[inod,:],Vector{DofID}(),Vector{EleID}()) for inod = 1:Δnnod] )
return nodID
end
addnode!(model::Model,coord::ℝ1) = addnode!(model,reshape(coord,(1,length(coord))))[1]
Base.adjoint(nodid::NodID)=nodid
"""
eleid = addelement!(model,ElType,nodid;kwargs...)
Add one or several elements to `model`, connecting them to the nodes specified
by `nodid`.
If `nodid` is an `AbstractVector`: add a single element to the model. `eleid`
is then a single element identifier.
If `nodid` is an `AbstractMatrix`: add multiple elements to the model. Each
row of `nodid` identifies the node of a single element. `eleid` is then
a vector of element identifiers.
For each element, `addelement!` will call `eleobj = ElType(nodes;kwargs...)` where
`nodes` is a vector of nodes of the element.
See also: [`addnode!`](@ref), [`describe`](@ref), [`coord`](@ref)
"""
function addelement!(model::Model,::Type{T},nodID::AbstractMatrix{NodID};kwargs...) where{T<:AbstractElement}
assert_unlocked(model)
# new element type? make space in model.eletyp and model.eleobj for that
nod = [model.nod[nodID[1,i]] for i∈eachindex(nodID[1,:])]
ele1 = T(nod;kwargs...)
E = typeof(ele1)
# add eletyp to model (if new)
ieletyp = getieletyp(model,E)
if ieletyp == 0 # new element type!
ieletyp = length(model.ele)+1
push!(model.eleobj, Vector{E }() )
push!(model.ele , Vector{Element}() )
end
iele_sofar = length(model.ele[ieletyp])
nele_new,nnod = size(nodID)
inod,class,field = getdoflist(E)
neledof = getndof(E)
nnod == getnnod(E) || muscadeerror(@sprintf "Connecting element of type %s: Second dimension of inod (%i) must be equal to element's nnod (%i)" T nnod getnnod(E) )
dofID = Vector{DofID }(undef,neledof ) # work array
eleID = Vector{EleID }(undef,nele_new) # allocate return variable
ele = Vector{Element}(undef,nele_new) # work array - will be appended to model.ele
eleobj = Vector{E }(undef,nele_new) # work array - will be appended to model.eleobj
for iele_new = 1:nele_new
# add eleID to nodes
eleID[iele_new] = EleID(ieletyp,iele_new+iele_sofar)
for nod ∈ [model.nod[i] for i∈unique(nodID[iele_new,:])] # unique: if several nodes of an element are connected to the same model node, mention element only once
push!(nod.eleID,eleID[iele_new])
end
# for all dofs of the element, make sure they are represented in the nod object and get an ID
for ieledof = 1:neledof
nodid = nodID[iele_new,inod[ieledof]] # nodID of current eledof
idoftyp = getidoftyp(model,class[ieledof],field[ieledof])
# add doftyp to model (if new)
if idoftyp == 0 # new dof type
push!(model.doftyp, DofTyp(class[ieledof],field[ieledof],1.,DofID[])) # DofID[]: do not add dof to doftyp, though
idoftyp = length(model.doftyp)
end
# add dof to model (if new)
idofID = firstindex(model.dof[dofID].idoftyp == idoftyp for dofID ∈ model.nod[nodid].dofID)
if idofID == 0 # new dof
dofID[ieledof] = newdofID(model,class[ieledof])
push!(model.dof[class[ieledof]], Dof(dofID[ieledof],nodid,idoftyp,𝕫[]) ) # do not add element to dof, though
push!(model.doftyp[idoftyp].dofID, dofID[ieledof])
else
dofID[ieledof] = model.nod[nodid].dofID[idofID]
end
# add element to dof (always)
push!(model.dof[dofID[ieledof]].eleID, eleID[iele_new])
# add dof to node (if new)
if dofID[ieledof] ∉ model.nod[nodid].dofID
push!(model.nod[nodid].dofID, dofID[ieledof])
end
end
# add element to model (always)
ele[ iele_new] = Element(eleID[iele_new], ieletyp, iele_sofar+iele_new, nodID[iele_new,:],deepcopy(dofID))
if iele_new==1
eleobj[iele_new] = ele1
else
nod = [model.nod[nodID[iele_new,i]] for i∈eachindex(nodID[iele_new,:])]
eleobj[iele_new] = T(nod;kwargs...)
end
end
append!(model.ele[ ieletyp],ele )
append!(model.eleobj[ieletyp],eleobj)
return eleID
end
addelement!(model::Model,::Type{E},nodID::AbstractVector{NodID};kwargs...) where{E<:AbstractElement} = addelement!(model,E,reshape(nodID,(1,length(nodID)));kwargs...)[1]
"""
setscale!(model;scale=nothing,Λscale=nothing)
Provides an order of magnitude for each type of dof in the model.
This is usued to improve the conditioning of the incremental problems and
for convergence criteria. `scale` is a `NamedTuple` with fieldnames within
`X`, `U` and `A`. Each field is itself a `NamedTuple` with fieldnames being
dof fields, and value being the expected order of magnitude.
For example `scale = (X=(tx=10,rx=1),A=(drag=3.))` should be read as: X-dofs
of field `:tx` are in tens of meters, `:rx` are in radians, and A-dofs of
field `drag` are of the order of 3. All other degrees of freedom are expected
to be roughly of the order of 1.
`Λscale` is a scalar. The scale of a Λ-dof will be deemed to be the scale of the
corresponding X-dof, times `Λscale`.
See also: [`addnode!`](@ref), [`describe`](@ref), [`coord`](@ref)
"""
function setscale!(model::Model;scale=nothing,Λscale=nothing) # scale = (X=(tx=10,rx=1),A=(drag=3.))
assert_unlocked(model)
if ~isnothing(scale)
for doftyp ∈ model.doftyp
if doftyp.class ∈ keys(scale) && doftyp.field ∈ keys(scale[doftyp.class])
doftyp.scale = scale[doftyp.class][doftyp.field] # otherwise leave untouched
end
end
end
if ~isnothing(Λscale)
model.scaleΛ = Λscale
end
end
assert_unlocked(model::Model) = model.locked && muscadeerror(@sprintf("model %s is initialized and can no longer be edited",model.ID))
"""
initialstate = initialize!(model)
Finalize a model (invoquing `addnode!` and `addelement!` after `initialize!` will result in an error)
and return an initial `State` (with all dofs set to zero, as starting point for an analysis.)
See also: [`addnode!`](@ref), [`addelement!`](@ref), [`solve`](@ref)
"""
function initialize!(model::Model)
assert_unlocked(model)
model.locked = true
return State(model,Disassembler(model))
end
function unlock(model::Model,ID::Symbol)
ID == model.ID && muscadeerror("ID must be distinct from model.ID")
newmodel = deepcopy(model)
newmodel.locked = false
newmodel.ID = ID
return newmodel
end
### extracting data from the model
eletyp(model::Model) = eltype.(model.eleobj)
### getting printout of the model
using Printf
"""
describe(model,spec)
Print out information about `model`.
`spec` can be
- an `EleID` to describe an element,
- a `DofID` to describe a dof.
- a `NodID` to describe a node,
- `:doftyp` to obtain a list of doftypes,
- `:dof` to obtain a list of dofs or
- `:eletyp` for a list of element types.
See also: [`addelement!`](@ref), [`addnode!`](@ref)
"""
function describe(model::Model,eleID::EleID)
try
dof = model.ele[eleID]
catch
printstyled("Not a valid EleID\n",color=:red,bold=true)
return
end
e = model.ele[eleID]
eo = model.eleobj[eleID]
@printf "Element with EleID(%i,%i)\n" eleID.ieletyp eleID.iele
@printf " model.eleobj[%i][%i]::" eleID.ieletyp eleID.iele
printstyled(@sprintf("%s\n",typeof(eo)),color=:cyan)
@printf " model.ele[%i][%i]:\n" eleID.ieletyp eleID.iele
for dofid ∈ e.dofID
dof = model.dof[dofid]
nod = model.nod[dof.nodID]
doftyp = model.doftyp[dof.idoftyp]
@printf " NodID(%i), class=:%s, field=:%-12s\n" dof.nodID.inod doftyp.class doftyp.field
end
end
function describe(model::Model,dofID::DofID)
try
dof = model.dof[dofID]
catch
printstyled("Not a valid DofID\n",color=:red,bold=true)
if dofID.class==:Λ
@printf "Optimisation solvers introduce a one-to-one correspondance between :Λ-dofs and :X-dofs, \nbut :Λ-dofs are not part of the model description: try DofID(:X,...)\n"
end
return
end
dof = model.dof[dofID]
doftyp = model.doftyp[dof.idoftyp]
@printf "Degree of freedom with DofID(:%s,%i)\n" dofID.class dofID.idof
@printf " model.dof.%s[%i]:\n" dofID.class dofID.idof
@printf " NodID(%i), class=:%s, field=:%-12s\n" dof.nodID.inod dofID.class doftyp.field
@printf " elements:\n"
for eleid ∈ dof.eleID
@printf " EleID(%i,%i), " eleid.ieletyp eleid.iele
printstyled(@sprintf("%s\n",eltype(model.eleobj[eleid.ieletyp])),color=:cyan)
end
if dofID.class == :X
@printf " Output in state[istep].X[ider+1][%i] and state[istep].Λ[%i]\n" dofID.idof dofID.idof
elseif dofID.class ==:U
@printf " Output in state[istep].U[ider][%i]\n" dofID.idof
elseif dofID.class == :A
@printf " Output in state[istep].A[%i]\n" dofID.idof
end
end
function describe(model::Model,nodID::NodID)
try
nod = model.nod[nodID]
catch
printstyled("Not a valid NodID\n",color=:red,bold=true)
return
end
nod = model.nod[nodID]
@printf "Node with NodID(%i)\n" nodID.inod
@printf " model.nod[%i]:\n" nodID.inod
nc = length(nod.coord)
@printf " coord=["
for ic=1:nc-1
@printf "%g," nod.coord[ic]
end
if nc>0
@printf "%g" nod.coord[nc]
end
@printf "]\n"
@printf " dof (degrees of freedom):\n"
for dofid ∈ nod.dofID
dof = model.dof[dofid]
doftyp = model.doftyp[dof.idoftyp]
@printf " DofID(:%s,%i), class=:%s, inod=%i, field=:%-12s\n" dofid.class dofid.idof dofid.class nodID.inod doftyp.field
end
@printf " elements:\n"
for eleID ∈ nod.eleID
@printf " EleID(%i,%i), " eleID.ieletyp eleID.iele
printstyled(@sprintf("%s\n",typeof(model.eleobj[eleID])),color=:cyan)
end
end
function describe(model::Model,s::Symbol)
@printf "\nModel '%s'\n" model.ID
if s==:dof
for class ∈ (:X,:U,:A)
ndof = getndof(model,class)
ndof>0 && @printf "\n Dofs of class :%s\n" class
for idof = 1:ndof
dof = model.dof[class][idof]
doftyp = model.doftyp[dof.idoftyp]
@printf " %6d. field= :%-15s NodID(%i)\n" idof doftyp.field dof.nodID.inod
end
end
elseif s==:doftyp
for doftyp ∈ model.doftyp
doftyp.class==:X && @printf " class= :%-5s field= :%-15s\n" doftyp.class doftyp.field
end
for doftyp ∈ model.doftyp
doftyp.class==:U && @printf " class= :%-5s field= :%-15s\n" doftyp.class doftyp.field
end
for doftyp ∈ model.doftyp
doftyp.class==:A && @printf " class= :%-5s field= :%-15s\n" doftyp.class doftyp.field
end
elseif s==:eletyp
et = eletyp(model)
for i∈eachindex(et)
@printf " %6d elements of type %s\n" length(model.eleobj[i]) et[i]
end
end
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 1660 | module Muscade
using Printf,SparseArrays,StaticArrays,LinearAlgebra
include("Dialect.jl")
export ℝ,ℤ,𝕣,𝕫,𝔹,𝕓
export ℝ1,ℤ1,𝕣1,𝕫1,𝔹1,𝕓1
export ℝ2,ℤ2,𝕣2,𝕫2,𝔹2,𝕓2
export ℝ11,ℤ11,𝕣11,𝕫11,𝔹11,𝕓11
export toggle,@once,default
include("Adiff.jl")
export ∂ℝ #\partial \bbR
export variate,δ # \delta
export value,VALUE,∂ # \partial, \nabla
export constants,precedence,npartial,norm
export ≗ #\circeq
include("Dots.jl")
export dots,∘₀,∘₁,∘₂,⊗
include("Espy.jl")
export @request
export @espy,@espydbg
include("Exceptions.jl")
export muscadeerror
include("ModelDescription.jl")
export AbstractElement
export Model,addnode!,addelement!,setscale!,initialize!
export Node
export describe,getndof
include("ElementAPI.jl")
export lagrangian,residual,espyable
export coord,∂0,∂1,∂2,getsomedofs
export doflist
export noχ,noFB
include("BasicElements.jl")
export off,equal,positive
export DofCost,SingleDofCost,ElementCost
export DofConstraint,Hold,ElementConstraint
export QuickFix,DofLoad
include("Assemble.jl")
export Assembly
include("Solve.jl")
export solve
include("StaticX.jl")
export StaticX
include("StaticXUA.jl")
export StaticXUA
include("Output.jl")
export getdof,getresult,findlastassigned
include("SelfDraw.jl")
export draw,request2draw
module Unit
include("Unit.jl")
export unit,←,→,convert
end
module ElTest
using Muscade
include("ElTest.jl")
export test_static_element,gradient
end
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 6967 | ## Nodal results
"""
dofres,dofID = getdof(state;[class=:X],field=:somefield,nodID=[nodids...],[iders=0|1|2])
Obtain the value of dofs of the same class and field, at various nodes and for various states.
If `state` is a vector, the output `dofres` has size `(ndof,nder+1,nstate)`.
If `state` is a scalar, the output `dofres` has size `(ndof,nder+1)`.
See also: [`getresult`](@ref), [`addnode!`](@ref), [`solve`](@ref)
"""
function getdof(state::State;kwargs...)
dofres,dofID = getdof([state];kwargs...)
return reshape(dofres,size(dofres)[1:2]),dofID
end
function getdof(state::Vector{S};class::Symbol=:X,field::Symbol,nodID::Vector{NodID}=NodID[],iders::ℤ1=[0])where {S<:State}
class ∈ [:Λ,:X,:U,:A] || muscadeerror(sprintf("Unknown dof class %s",class))
c = class==:Λ ? :X : class
dofID = nodID==NodID[] ? getdofID(state[begin].model,c,field) : getdofID(state[begin].model,c,field,nodID)
iders = class∈[:Λ,:A] ? [0] : iders
dofres = Array{𝕣,3}(undef,length(dofID),length(iders),length(state)) # dofres[inod,ider+1]
for istate ∈ eachindex(state)
for ider∈iders
s = if class==:Λ; state[istate].Λ
elseif class==:X; state[istate].X[ider+1]
elseif class==:U; state[istate].U[ider+1]
elseif class==:A; state[istate].A
end
for (idof,d) ∈ enumerate(dofID)
dofres[idof,ider+1,istate] = s[d.idof]
end
end
end
return dofres,dofID
end
# Elemental results
function extractkernel!(iele::AbstractVector{𝕫},eleobj::Vector{E},dis::EletypDisassembler,state::Vector{S},dbg,req) where{E,S<:State}# typestable kernel
return [begin
index = dis.index[i]
Λ = s.Λ[index.X]
X = Tuple(x[index.X] for x∈s.X)
U = Tuple(u[index.U] for u∈s.U)
A = s.A[index.A]
L,χn,FB,e = getlagrangian(implemented(eleobj[i])...,eleobj[i],Λ,X,U,A,s.time,nothing,nothing,s.SP,(dbg...,istep=istep,iele=i),req)
e
end for i∈iele, (istep,s)∈enumerate(state)]
end
"""
eleres = getresult(state,req,eleids)
Obtain an array of nested NamedTuples and NTuples of element results.
`req` is a request defined using `@request`.
`state` a vector of `State`s or a single `State`.
`eleids` can be either
- a vector of `EleID`s (obtained from `addelement!`) all corresponding
to the same concrete element type
- a concrete element type.
If `state` is a vector, the output `dofres` has size `(nele,nstate)`.
If `state` is a scalar, the output `dofres` has size `(nele)`.
See also: [`getdof`](@ref), [`@request`](@ref), [`@espy`](@ref), [`addelement!`](@ref), [`solve`](@ref)
"""
function getresult(state::Vector{S},req,eleID::Vector{EleID})where {S<:State}
# Some elements all of same type, multisteps
# eleres[iele,istep].gp[3].σ
ieletyp = eleID[begin].ieletyp
all(e.ieletyp== ieletyp for e∈eleID) || muscadeerror("All elements must be of the same element type")
eleobj = state[begin].model.eleobj[ieletyp]
dis = state[begin].dis.dis[ieletyp]
iele = [e.iele for e∈eleID]
return extractkernel!(iele,eleobj,dis,state,(func=:getresult,ieletyp=ieletyp),req)
end
function getresult(state::Vector{S},req,::Type{E}) where{S<:State,E<:AbstractElement}
# All elements within the type, multisteps
# eleres[iele,istep].gp[3].σ
ieletyp = findfirst(E.==eletyp(state[begin].model))
isnothing(ieletyp) && muscadeerror("This type of element is not in the model. See 'eletyp(model)'")
eleobj = state[begin].model.eleobj[ieletyp]
dis = state[begin].dis.dis[ieletyp]
iele = eachindex(eleobj)
return extractkernel!(iele,eleobj,dis,state,(func=:getresult,eletyp=E),req)
end
# single step
# eleres[iele].gp[3].σ
getresult(state::State,req,args...) = flat(getresult([state],req,args...))
############## describe state to the user
function describeX(state::State)
model = state.model
nX = getndof(model,:X)
nder = length(state.X)
for iX = 1:nX
dofID = DofID(:X,iX)
dof = model.dof[dofID]
doftyp = model.doftyp[dof.idoftyp]
@printf "NodID(%i), class=:%s, field=:%-15s " dof.nodID.inod dofID.class doftyp.field
for ider = 1:nder
@printf "%15g " state.X[ider][iX]
end
@printf "\n"
end
end
function describeΛX(state::State)
model = state.model
nX = getndof(model,:X)
nder = length(state.X)
for iX = 1:nX
dofID = DofID(:X,iX)
dof = model.dof[dofID]
doftyp = model.doftyp[dof.idoftyp]
@printf "NodID(%i), class=:%s, field=:%-15s %15g " dof.nodID.inod dofID.class doftyp.field state.Λ[iX]
for ider = 1:nder
@printf "%15g " state.X[ider][iX]
end
@printf "\n"
end
end
function describeU(state::State)
model = state.model
nU = getndof(model,:U)
nder = length(state.U)
for iU = 1:nU
dofID = DofID(:U,iU)
dof = model.dof[dofID]
doftyp = model.doftyp[dof.idoftyp]
@printf "NodID(%i), class=:%s, field=:%-15s " dof.nodID.inod dofID.class doftyp.field
for ider = 1:nder
@printf "%15g " state.U[ider][iU]
end
@printf "\n"
end
end
function describeA(state::State)
model = state.model
nA = getndof(model,:A)
for iA = 1:nA
dofID = DofID(:A,iA)
dof = model.dof[dofID]
doftyp = model.doftyp[dof.idoftyp]
@printf "NodID(%i), class=:%s, field=:%-15s %15g\n" dof.nodID.inod dofID.class doftyp.field state.A[iA]
end
end
"""
describe(state;class=:all)
Provide a description of the dofs stored in `state`.
`class` can be either `:all`, `:Λ`, `:ΛX`, `:X`, `:U` or `:A`
See also: [`solve`](@ref)
"""
function describe(state::State;class::Symbol=:all)
if class ==:all
describeΛX(state)
describeU(state)
describeA(state)
elseif class==:Λ || class==:ΛX
describeΛX(state)
elseif class ==:X
describeX(state)
elseif class ==:U
describeU(state)
elseif class ==:A
describeA(state)
else
printstyled("Not a valid class\n",color=:red,bold=true)
end
end
"""
ilast = findlastassigned(state)
Find the index `ilast` of the element before the first non assigment element in a vector `state`.
In multistep analyses, `solve` returns a vector `state` of length equal to the number of steps
requested by the user. If the analysis is aborted, `solve` still returns any available results
at the begining of `state`, and the vector `state[1:ilast]` is fully assigned.
See also: [`solve`](@ref)
"""
findlastassigned(v::Vector) = findlast([isassigned(v,i) for i=1:length(v)])
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 1207 | draw(axe,::AbstractElement,args...;kwargs...) = nothing # by default, an element draws nothing
function draw_(axe,dis::EletypDisassembler,eleobj,iele,state,dbg;kwargs...)
# typestable kernel
for ie ∈ iele
index = dis.index[ie]
Λe = state.Λ[index.X]
Xe = Tuple(x[index.X] for x∈state.X)
Ue = Tuple(u[index.U] for u∈state.U)
Ae = state.A[index.A]
eo = eleobj[ie]
draw(axe, eo, Λe,Xe,Ue,Ae, state.time,nothing,nothing,state.SP,(dbg...,iele=ie);kwargs...)
end
return
end
function draw(axe,state::State,ieletyp::𝕫; iele::ℤ1=1:length(state.model.ele[ieletyp]),kwargs...)
# User syntax 2: One element type, some or all elements within the types
eleobj = state.model.eleobj[ieletyp]
dis = state.dis.dis[ieletyp]
draw_(axe,dis,eleobj,iele,state,(ieletyp=ieletyp,);kwargs...) # call kernel
return
end
function draw(axe,state::State;ieletyp::ℤ1=1:length(state.model.ele),kwargs...)
# User syntax 1: Draw several element types - cannot specify iele
for et ∈ ieletyp
draw(axe,state,et;kwargs...) # call for one element type
end
return
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 1650 | using Printf
"""
solve(Solver;dbg=(;),verbose=true,silenterror=false,kwargs...)
Execute an analysis using `Solver`, and safeguard partial results in the
case of error.
# Named arguments
- `dbg=(;)` a named tuple to trace the call tree (for debugging)
- `verbose=true` set to false to suppress printed output (for testing)
- `silenterror=false` set to true to suppress print out of error (for testing)
- `kwargs...` Further arguments passed on to the method `solve` provided by the solver
This will call the method `solve` provided by the solver with
`solve(Solver,pstate,verbose,(dbg...,solver=Symbol(Solver));kwargs...)`
See also: [`StaticX`](@ref), [`StaticXUA`](@ref), [`initialize!`](@ref)
"""
function solve(Solver;dbg=NamedTuple(),verbose::𝕓=true,silenterror::𝕓=false,kwargs...)
verbose && printstyled("\n\n\nMuscade:",bold=true,color=:cyan)
verbose && printstyled(@sprintf(" %s solver\n\n",Symbol(Solver)),color=:cyan)
Tstate = getStateType(Solver)
pstate = Base.RefValue{Vector{Tstate}}() # state is not a return argument of the solver, so that partial results are not lost on error
try
t = @elapsed solve(Solver,pstate,verbose,(dbg...,solver=Symbol(Solver));kwargs...)
verbose && @printf(" %s time: %s\n",Symbol(Solver),showtime(t))
catch exn
silenterror || report(exn)
silenterror || printstyled("\nAborting the analysis.",color=:red)
silenterror || println(" Function 'solve' should still be returning results obtained so far.")
end
verbose && printstyled("Muscade done.\n\n\n",bold=true,color=:cyan)
return pstate[]
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 6240 |
###--------------------- ASMstaticX: for good old static FEM
mutable struct AssemblyStaticX{Tλ,Tλx} <:Assembly
Lλ :: Tλ
Lλx :: Tλx
α :: 𝕣
end
function prepare(::Type{AssemblyStaticX},model,dis)
dofgr = allXdofs(model,dis)
ndof = getndof(dofgr)
narray,neletyp = 2,getneletyp(model)
asm = Matrix{𝕫2}(undef,narray,neletyp)
Lλ = asmvec!(view(asm,1,:),dofgr,dis)
Lλx = asmmat!(view(asm,2,:),view(asm,1,:),view(asm,1,:),ndof,ndof)
# out = one_for_each_thread(AssemblyStaticX(Lλ,Lλx,∞)) # KEEP - parallel
out = AssemblyStaticX(Lλ,Lλx,∞) # sequential
return out,asm,dofgr
end
function zero!(out::AssemblyStaticX)
zero!(out.Lλ)
zero!(out.Lλx)
out.α = ∞
end
function add!(out1::AssemblyStaticX,out2::AssemblyStaticX)
add!(out1.Lλ,out2.Lλ)
add!(out1.Lλx,out2.Lλx)
out1.α = min(out1.α,out2.α)
end
function addin!(out::AssemblyStaticX,asm,iele,scale,eleobj::E,Λ,X::NTuple{Nxder,<:SVector{Nx}},U,A,t,SP,dbg) where{E,Nxder,Nx}
if Nx==0; return end # don't waste time on Acost elements...
ΔX = δ{1,Nx,𝕣}(scale.X) # NB: precedence==1, input must not be Adiff
Lλ,χ,FB = getresidual(implemented(eleobj)...,eleobj,(∂0(X)+ΔX,),U,A,t,nothing,nothing,SP,dbg)
Lλ = Lλ .* scale.X
add_value!(out.Lλ ,asm[1],iele,Lλ)
add_∂!{1}( out.Lλx,asm[2],iele,Lλ)
out.α = min(out.α,default{:α}(FB,∞))
end
"""
StaticX
A non-linear static solver for forward (not inverse, optimisation) FEM.
The current implementation does not handle element memory.
An analysis is carried out by a call with the following syntax:
```
initialstate = initialize!(model)
state = solve(StaticX;initialstate=initialstate,time=[0.,1.])
```
# Named arguments
- `dbg=(;)` a named tuple to trace the call tree (for debugging)
- `verbose=true` set to false to suppress printed output (for testing)
- `silenterror=false` set to true to suppress print out of error (for testing)
- `initialstate` a single `state` - obtained from a call to `initialize!`, or
from a previous analysis
- `time` an `AbstractVector` vector of the times at which to compute
equilibrium. While this solver does not account for dynamic
effect, the model will typicaly describe some loads as time
dependent.
- `maxiter=50` maximum number of Newton-Raphson iteration at any given step
- `maxΔx=1e-5` convergence criteria: a norm on the scaled `X` increment
- `maxincrement=∞` convergence criteria: a norm on the scaled residual
- `saveiter=false` set to true so that the output `state` is a vector describing
the states of the model at the last iteration (for debugging
non-convergence)
- `γ0=1.` an initial value of the barrier coefficient for the handling of contact
using an interior point method
- `γfac1=0.5` at each iteration, the barrier parameter γ is multiplied
- `γfac2=100.` by γfac1*exp(-min(αᵢ)/γfac2)^2), where αᵢ is computed by the i-th
interior point savvy element as αᵢ=abs(λ-g)/γ
# Output
A vector of length equal to that of `time` containing the state of the model at each of these steps
See also: [`solve`](@ref), [`StaticXUA`](@ref), [`initialize!`](@ref)
"""
struct StaticX end
getStateType(::Type{StaticX}) = State{1,1,typeof((γ=0.,))} # nXder,nUder,TSP
function solve(::Type{StaticX},pstate,verbose,dbg;
time::AbstractVector{𝕣},
initialstate::State,
maxiter::ℤ=50,maxΔx::ℝ=1e-5,maxresidual::ℝ=∞,
saveiter::𝔹=false,γ0::𝕣=1.,γfac1::𝕣=.5,γfac2::𝕣=100.)
# important: this code assumes that there is no χ in state.
model,dis = initialstate.model,initialstate.dis
out,asm,dofgr = prepare(AssemblyStaticX,model,dis)
citer = 0
cΔx²,cLλ² = maxΔx^2,maxresidual^2
Tstate,i = getStateType(StaticX),initialstate
s = Tstate(i.Λ,deepcopy(i.X),i.U,i.A,i.time,(γ=0.,),i.model,i.dis)
state = allocate(pstate,Vector{Tstate}(undef,saveiter ? maxiter : length(time))) # state is not a return argument of this function. Hence it is not lost in case of exception
local facLλx
for (step,t) ∈ enumerate(time)
s.time = t
s.SP = (γ=γ0,)
for iiter = 1:maxiter
citer += 1
assemble!(out,asm,dis,model,s,(dbg...,solver=:StaticX,step=step,iiter=iiter))
try if step==1 && iiter==1
facLλx = lu(firstelement(out).Lλx)
else
lu!(facLλx,firstelement(out).Lλx)
end catch; muscadeerror(@sprintf("matrix factorization failed at step=%i, iiter=%i",step,iiter)) end
Δx = facLλx\firstelement(out).Lλ
Δx²,Lλ² = sum(Δx.^2),sum(firstelement(out).Lλ.^2)
decrement!(s,0,Δx,dofgr)
verbose && saveiter && @printf(" iteration %3d, γ= %7.1e\n",iiter,γ)
saveiter && (state[iiter]=State(s.Λ,deepcopy(s.X),s.U,s.A,s.time,s.SP,model,dis))
if Δx²≤cΔx² && Lλ²≤cLλ²
verbose && @printf " step %3d converged in %3d iterations. |Δx|=%7.1e |Lλ|=%7.1e\n" step iiter √(Δx²) √(Lλ²)
~saveiter && (state[step]=State(s.Λ,deepcopy(s.X),s.U,s.A,s.time,s.SP,model,dis))
break#out of the iiter loop
end
iiter==maxiter && muscadeerror(@sprintf("no convergence in step %3d after %3d iterations |Δx|=%g / %g, |Lλ|=%g / %g",step,iiter,√(Δx²),maxΔx,√(Lλ²)^2,maxresidual))
Δγ = γfac1*exp(-(firstelement(out).α/γfac2)^2)
s.SP = (γ=s.SP.γ*Δγ,)
end
end
verbose && @printf "\n nel=%d, ndof=%d, nstep=%d, niter=%d, niter/nstep=%5.2f\n" getnele(model) getndof(dofgr) length(time) citer citer/length(time)
return
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 10865 |
mutable struct AssemblyStaticΛXU_A{Ty,Ta,Tyy,Tya,Taa} <:Assembly
Ly :: Ty
La :: Ta
Lyy :: Tyy
Lya :: Tya
Laa :: Taa
α :: 𝕣
end
function prepare(::Type{AssemblyStaticΛXU_A},model,dis)
Ydofgr = allΛXUdofs(model,dis)
Adofgr = allAdofs( model,dis)
nY,nA = getndof(Ydofgr),getndof(Adofgr)
narray,neletyp = 5,getneletyp(model)
asm = Matrix{𝕫2}(undef,narray,neletyp)
Ly = asmvec!(view(asm,1,:),Ydofgr,dis)
La = asmvec!(view(asm,2,:),Adofgr,dis)
Lyy = asmmat!(view(asm,3,:),view(asm,1,:),view(asm,1,:),nY,nY)
Lya = asmfullmat!(view(asm,4,:),view(asm,1,:),view(asm,2,:),nY,nA)
Laa = asmfullmat!(view(asm,5,:),view(asm,2,:),view(asm,2,:),nA,nA)
out = AssemblyStaticΛXU_A(Ly,La,Lyy,Lya,Laa,0.)
return out,asm,Adofgr,Ydofgr
end
function zero!(out::AssemblyStaticΛXU_A)
zero!(out.Ly )
zero!(out.La )
zero!(out.Lyy)
zero!(out.Lya)
zero!(out.Laa)
out.α = ∞
end
function add!(out1::AssemblyStaticΛXU_A,out2::AssemblyStaticΛXU_A)
add!(out1.Ly,out2.Ly)
add!(out1.La,out2.La)
add!(out1.Lyy,out2.Lyy)
add!(out1.Lya,out2.Lya)
add!(out1.Laa,out2.Laa)
out1.α = min(out1.α,out2.α)
end
function addin!(out::AssemblyStaticΛXU_A,asm,iele,scale,eleobj::E,Λ,X::NTuple{Nxder,<:SVector{Nx}},
U::NTuple{Nuder,<:SVector{Nu}},A::SVector{Na},t,SP,dbg) where{E,Nxder,Nx,Nuder,Nu,Na} # TODO make Nx,Nu,Na types
Ny = 2Nx+Nu # Y=[Λ;X;U]
Nz = 2Nx+Nu+Na # Z = [Y;A]=[Λ;X;U;A]
scaleZ = SVector(scale.Λ...,scale.X...,scale.U...,scale.A...)
ΔZ = variate{2,Nz}(δ{1,Nz,𝕣}(scaleZ),scaleZ)
iλ,ix,iu,ia = gradientpartition(Nx,Nx,Nu,Na) # index into element vectors ΔZ and Lz
iy = 1:Ny
ΔΛ,ΔX,ΔU,ΔA = view(ΔZ,iλ),view(ΔZ,ix),view(ΔZ,iu),view(ΔZ,ia) # TODO Static?
L,χn,FB = getlagrangian(implemented(eleobj)...,eleobj, Λ+ΔΛ, (∂0(X)+ΔX,),(∂0(U)+ΔU,),A+ΔA,t,nothing,nothing,SP,dbg)
∇L = ∂{2,Nz}(L)
add_value!(out.Ly ,asm[1],iele,∇L,iy )
add_value!(out.La ,asm[2],iele,∇L,ia )
add_∂!{1}( out.Lyy,asm[3],iele,∇L,iy,iy)
add_∂!{1}( out.Lya,asm[4],iele,∇L,iy,ia)
add_∂!{1}( out.Laa,asm[5],iele,∇L,ia,ia)
out.α = min(out.α,default{:α}(FB,∞))
end
#------------------------------------
mutable struct AssemblyStaticΛXU{Ty,Tyy} <:Assembly
Ly :: Ty
Lyy :: Tyy
α :: 𝕣
end
function prepare(::Type{AssemblyStaticΛXU},model,dis)
Ydofgr = allΛXUdofs(model,dis)
nY = getndof(Ydofgr)
narray,neletyp = 2,getneletyp(model)
asm = Matrix{𝕫2}(undef,narray,neletyp)
Ly = asmvec!(view(asm,1,:),Ydofgr,dis)
Lyy = asmmat!(view(asm,2,:),view(asm,1,:),view(asm,1,:),nY,nY)
out = AssemblyStaticΛXU(Ly,Lyy,0.)
return out,asm,Ydofgr
end
function zero!(out::AssemblyStaticΛXU)
zero!(out.Ly )
zero!(out.Lyy)
out.α = ∞
end
function add!(out1::AssemblyStaticΛXU,out2::AssemblyStaticΛXU)
add!(out1.Ly,out2.Ly)
add!(out1.Lyy,out2.Lyy)
out1.α = min(out1.α,out2.α)
end
function addin!(out::AssemblyStaticΛXU,asm,iele,scale,eleobj::E,Λ,X::NTuple{Nxdir,<:SVector{Nx}},
U::NTuple{Nudir,<:SVector{Nu}},A, t,SP,dbg) where{E,Nxdir,Nx,Nudir,Nu}
Ny = 2Nx+Nu # Y=[Λ;X;U]
if Ny==0; return end # don't waste time on Acost elements...
scaleY = SVector(scale.Λ...,scale.X...,scale.U...)
ΔY = variate{2,Ny}(δ{1,Ny,𝕣}(scaleY),scaleY)
iλ,ix,iu,_ = gradientpartition(Nx,Nx,Nu,0) # index into element vectors ΔY and Ly
ΔΛ,ΔX,ΔU = view(ΔY,iλ),view(ΔY,ix),view(ΔY,iu)
L,χn,FB = getlagrangian(implemented(eleobj)...,eleobj, Λ+ΔΛ, (∂0(X)+ΔX,),(∂0(U)+ΔU,),A, t,nothing,nothing,SP,dbg)
∇L = ∂{2,Ny}(L)
add_value!(out.Ly ,asm[1],iele,∇L)
add_∂!{1}( out.Lyy,asm[2],iele,∇L)
out.α = min(out.α,default{:α}(FB,∞))
end
"""
StaticXUA
A non-linear static solver for optimisation FEM.
The current algorithm does not handle element memory.
An analysis is carried out by a call with the following syntax:
```
initialstate = initialize!(model)
stateX = solve(StaticX ;initialstate=initialstate,time=[0.,1.])
stateXUA = solve(StaticXUA;initialstate=stateX)
```
# Named arguments
- `dbg=(;)` a named tuple to trace the call tree (for debugging)
- `verbose=true` set to false to suppress printed output (for testing)
- `silenterror=false` set to true to suppress print out of error (for testing)
- `initialstate` a vector of `state`s, one for each load case in the optimization problem,
obtained from one or several previous `StaticX` analyses
- `maxAiter=50` maximum number of "outer" Newton-Raphson iterations over `A`
- `maxΔa=1e-5` "outer" convergence criteria: a norm on the scaled `A` increment
- `maxLa=∞` "outer" convergence criteria: a norm on the scaled `La` residual
- `maxYiter=0` maximum number of "inner" Newton-Raphson iterations over `X`
and `U` for every value of `A`. Experience so far is that these inner
iterations do not increase performance, so the default is "no inner
iterations".
- `maxΔy=1e-5` "inner" convergence criteria: a norm on the scaled `Y=[XU]` increment
- `maxLy=∞` "inner" convergence criteria: a norm on the scaled `Ly=[Lx,Lu]` residual
- `γ0=1.` an initial value of the barrier coefficient for the handling of contact
using an interior point method
- `γfac1=0.5` at each iteration, the barrier parameter γ is multiplied
- `γfac2=100.` by γfac1*exp(-min(αᵢ)/γfac2)^2), where αᵢ is computed by the i-th
interior point savvy element as αᵢ=abs(λ-g)/γ
# Output
A vector of length equal to that of `initialstate` containing the state of the optimized model at each of these steps
See also: [`solve`](@ref), [`StaticX`](@ref)
"""
struct StaticXUA end
getStateType(::Type{StaticXUA}) = State{1,1,typeof((γ=0.,))} # nXder,nUder
function solve(::Type{StaticXUA},pstate,verbose::𝕓,dbg;initialstate::Vector{<:State},
maxAiter::ℤ=50,maxYiter::ℤ=0,maxΔy::ℝ=1e-5,maxLy::ℝ=∞,maxΔa::ℝ=1e-5,maxLa::ℝ=∞,γ0::𝕣=1.,γfac1::𝕣=.5,γfac2::𝕣=100.)
model,dis = initialstate[begin].model,initialstate[begin].dis
out1,asm1,Ydofgr = prepare(AssemblyStaticΛXU ,model,dis)
out2,asm2,Adofgr,_ = prepare(AssemblyStaticΛXU_A,model,dis)
Tstate = getStateType(StaticX)
state = allocate(pstate,[Tstate(i.Λ,deepcopy(i.X),deepcopy(i.U),deepcopy(i.A),i.time,(γ=γ0,),i.model,i.dis) for i ∈ initialstate])
cΔy²,cLy²,cΔa²,cLa²= maxΔy^2,maxLy^2,maxΔa^2,maxLa^2
nA,nStep = getndof(model,:A),length(state)
La = Vector{𝕣 }(undef,nA )
Laa = Matrix{𝕣 }(undef,nA,nA)
Δy = Vector{𝕣1}(undef,nStep)
y∂a = Vector{𝕣2}(undef,nStep)
Δy²,Ly² = Vector{𝕣 }(undef,nStep),Vector{𝕣}(undef,nStep)
cAiter,cYiter = 0,0
local facLyy, facLyys, Δa
for iAiter = 1:maxAiter
verbose && @printf " A-iteration %3d\n" iAiter
La .= 0
Laa .= 0
for step ∈ eachindex(state)
for iYiter = 1:maxYiter
cYiter+=1
assemble!(out1,asm1,dis,model,state[step],(dbg...,solver=:StaticXUA,step=step,iYiter=iYiter))
try if iAiter==1 && step==1 && iYiter==1
facLyys = lu(out1.Lyy)
else
lu!(facLyys,out1.Lyy)
end catch; muscadeerror(@sprintf("Incremental Y-solution failed at step=%i, iAiter=%i, iYiter=%i",step,iAiter,iYiter)) end
Δy[ step] = facLyys\out1.Ly
decrement!(state[step],0,Δy[ step],Ydofgr)
Δy²s,Ly²s = sum(Δy[step].^2),sum(out2.Ly.^2)
if Δy²s≤cΔy² && Ly²s≤cLy²
verbose && @printf " step % i Y-converged in %3d Y-iterations: |ΔY|=%7.1e |∇L/∂Y|=%7.1e\n" step iYiter √(Δy²s) √(Ly²s)
break#out of iYiter
end
iYiter==maxYiter && muscadeerror(@sprintf("no Y-convergence after %3d Y-iterations. |ΔY|=%7.1e |Ly|=%7.1e\n",iYiter,√(Δy²s),√(Ly²s)))
end
assemble!(out2,asm2,dis,model,state[step],(dbg...,solver=:StaticXUA,step=step,iAiter=iAiter))
try if iAiter==1 && step==1
facLyy = lu(out2.Lyy)
else
lu!(facLyy,out2.Lyy)
end catch; muscadeerror(@sprintf("Lyy matrix factorization failed at step=%i, iAiter=%i",step,iAiter));end
Δy[ step] = facLyy\out2.Ly
y∂a[step] = facLyy\out2.Lya
La .+= out2.La - out2.Lya' * Δy[ step]
Laa .+= out2.Laa - out2.Lya' * y∂a[step]
Δy²[step],Ly²[step] = sum(Δy[step].^2),sum(out2.Ly.^2)
end
try
Δa = Laa\La
catch; muscadeerror(@sprintf("Laa\\La solution failed at iAiter=%i",iAiter));end
Δa²,La² = sum(Δa.^2),sum(La.^2)
for (step,s) ∈ enumerate(state)
ΔY = Δy[step] - y∂a[step] * Δa
decrement!(s,0,ΔY,Ydofgr)
decrement!(s,0,Δa,Adofgr)
s.SP = (γ= s.SP.γ* γfac1*exp(-(out2.α/γfac2)^2),)
end
if all(Δy².≤cΔy²) && all(Ly².≤cLy²) && Δa².≤cΔa² && La².≤cLa²
cAiter = iAiter
verbose && @printf "\n StaticXUA converged in %3d A-iterations.\n" iAiter
verbose && @printf " maxₜ(|ΔY|)=%7.1e maxₜ(|∇L/∂Y|)=%7.1e |ΔA|=%7.1e |∇L/∂A|=%7.1e\n" √(maximum(Δy²)) √(maximum(Ly²)) √(Δa²) √(La²)
break#out of iAiter
end
iAiter==maxAiter && muscadeerror(@sprintf("no convergence after %3d A-iterations. |ΔY|=%7.1e |Ly|=%7.1e |ΔA|=%7.1e |La|=%7.1e\n",iAiter,√(maximum(Δy²)),√(maximum(Ly²)),√(Δa²),√(La²)))
end
verbose && @printf "\n nel=%d, ndof=%d, nstep=%d, nAiter=%d\n" getnele(model) getndof(Adofgr) nStep cAiter
verbose && @printf "\n nYiter=%d, nYiter/(nstep*nAiter)=%5.2f\n" cYiter cYiter/nStep/cAiter
return
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 7579 | """
Muscade provides `unit` to transform quantities to and from basic SI units.
This allows to develop Muscade-based applications with a coherent unit system.
```
using Muscade
using Muscade: m, kg, pound, foot
rho = 3←pound/foot^3 # convert to SI
vieuxquintal = 1000*pound # define new unit
printf("Density [pound/foot^3] %f",rho→pound/foot^3) # convert from SI
```
Whole arrays can be converted in the same way.
In the above, `rho` would be of type `Float64`. This contrasts with
`Unitful.jl` which would make ``rho` be of a type containing data about
dimensionality thus providing check against operations like `length+surface`.
The drawback is that this would in many cases cause arrays to appear
that contain heterogenous data. In Julia, such arrays with abstract element
have severely lower performance.
The typical usage is
- Application developers assume inputs with consistent units.
- Application developers require any constants (acceleration of gravity,
gas constant...) as user input.
- Users convert all their input values as they define it `rho = 3 ← pound/foot^3`
- Users convert Muscade outputs before printing them out
`printf("stress [MPa] %f",stress → MPa)`
Inspect the file `espy.jl` for an overview of the available units.
"""
struct unit
phydim :: Vector{Float64}
ct :: Float64
end
unit(phydim :: Vector{Float64}) = unit(phydim,1.)
unit(ct :: Float64 ) = unit([0.,0.,0.,0.,0.,0.,0.,0.],ct)
Base.:(*)(a::unit ,b::unit) = unit( a.phydim+b.phydim,a.ct*b.ct)
Base.:(*)(a::Number,b::unit) = unit( b.phydim,a *b.ct)
Base.:(*)(a::unit ,b::Number) = unit( a.phydim ,a.ct*b )
Base.:(/)(a::unit ,b::unit) = unit( a.phydim-b.phydim,a.ct/b.ct)
Base.:(/)(a::Number,b::unit) = unit( -b.phydim,a /b.ct)
Base.:(/)(a::unit ,b::Number) = unit( a.phydim ,a.ct/b )
Base.:(^)(a::unit ,b::Integer) = unit( a.phydim*b ,a.ct^b )
Base.:(^)(a::unit ,b::Real) = unit( a.phydim*b ,a.ct^b )
Base.inv(a::unit) = unit(-a.phydim ,1. /a.ct)
←(a::Number,b::unit) = a *b.ct
←(a ,b::unit) = a .*b.ct
→(a::Number,b::unit) = a /b.ct
→(a ,b::unit) = a ./b.ct
function string(a::unit)
fundamental = ["m","kg","s","A","K","cd","mol","bit"]
dsc = a.ct == 1 ? "" : "$(a.ct) "
for (i,d) in enumerate(a.phydim)
if d==0. continue end
if d==1. dsc = "$dsc$(fundamental[i])*"
else dsc = "$dsc$(fundamental[i])^$(d)*"
end
end
if length(dsc)==0 dsc = "." end
if dsc[end]=='*' dsc = dsc[1:end-1] end
return dsc
end
show(io::IO,x::unit) = write(io,string(x))
# Basic units
const m = metre = unit([1.,0.,0.,0.,0.,0.,0.,0.])
const kg = kilogram = unit([0.,1.,0.,0.,0.,0.,0.,0.])
const s = second = unit([0.,0.,1.,0.,0.,0.,0.,0.])
const A = Ampere = unit([0.,0.,0.,1.,0.,0.,0.,0.])
const K = Kelvin = unit([0.,0.,0.,0.,1.,0.,0.,0.])
const Cd = candela = unit([0.,0.,0.,0.,0.,1.,0.,0.])
const mol = mole = unit([0.,0.,0.,0.,0.,0.,1.,0.])
const nat = nit = unit([0.,0.,0.,0.,0.,0.,0.,1.])
# Prefixes
const yocto = unit(1e-24)
const zepto = unit(1e-21)
const atto = unit(1e-18)
const femto = unit(1e-15)
const pico = unit(1e-12)
const nano = unit(1e-9)
const micro = unit(1e-6)
const milli = unit(1e-3)
const centi = unit(1e-2)
const deci = unit(1e-1)
const dimensionless = ena = unit(1e0)
const deca = unit(1e1)
const hecto = unit(1e2)
const kilo = unit(1e3)
const mega = unit(1e6)
const giga = unit(1e9)
const tera = unit(1e12)
const peta = unit(1e15)
const exa = unit(1e18)
const zetta = unit(1e21)
const yotta = unit(1e24)
# Engineering
const Å = Angstrom = 1e-10metre
const μm = micrometre = micro*metre
const mm = milli*metre
const cm = centi*metre
const dm = deci*metre
const km = kilo*metre
const are = hecto*metre^2
const ha = hectare = hecto*are
const l = litre = milli*metre^3
const g = gram = milli*kilogram
const Mg = tonne = mega*gram
const N = Newton = m*kg/s^2
const kN = kilo*Newton
const MN = mega*Newton
const GN = giga*Newton
const Pa = Pascal = N/m^2
const kPa = kilo*Pascal
const MPa = mega*Pascal
const GPa = giga*Pascal
const J = Joule = N*m
const W = Watt = J/s
const V = Volt = W/A #m^2*kg/s^3/A
const mV = milli*Volt
const ε = strain = dimensionless
const με = microstrain = micro
const event = dimensionless
const Hz = Hertz = 1/s
const rad = radian = dimensionless
const period = turn = 2π*dimensionless
const deg = degree = period/360
const sr = steradian = dimensionless
const sphere = 4π*sr
const Coulomb = C = A*s
const Ohm = Ω = V/A
# Physical constants
const c = 299792458m/s
const G = 9.80665m/s^2
const elementarycharge = e = 1.602176620898e-19C
const Avogadro = Nₐ = 6.02214086e23/mol
const Faraday = F = e*Nₐ
const gasconst = R = 8.314459848J/mol/K
const Boltzman = kᵦ = R/Nₐ
const Planck = h = 6.62601015e-35J*s
const rPlanck = ħ = h/2π
# data/probability
const bit = Shannon = log( 2)nit
const ban = dit = Hartley = log(10)nit
const octet = byte = 8bit
# Calendar
const minute = 60s
const hour = 60minute
const day = 24hour
const week = 7day
const year = 365.25day
const month = year/12
# Prehistoric stuff. The imperials strike back. We can even do Donald Duck units!!!
const eV = elementarycharge*V
const inch = 25.4mm
const foot = 12inch
const yard = 3foot
const fathom = 2yard
const furlong = 110fathom
const cable = 120fathom
const mils = thou = milli*inch
const nautical = 1852m
const mile = 1760yard
const USacre = 43560foot^2
const mål = 1000m^2
const ounce = 28.349523125g
const pound = 16ounce
const shortton = 2kilo*pound
const poundforce = pound*G
const kip = 1e3poundforce
const cmil = pi/4*mils^2
const kcmil = MCM = kilo*cmil
const kgf = kilogram*G
const horsepower = 75kgf*m/s
const knot = nautical/hour
const USpint = 28.875*inch^3
const USgallon = 8USpint
const USfloz = USpint/16
const barrel = 42USgallon
const hogshead = 63USgallon
const psi = poundforce/inch^2
const ksi = kilo*psi
const bar = 1e5Pa
const atm = 101325Pa
const mmHg = 133.322387415Pa
const torr = (1/760)atm
const BTU = 1.0545e3J
const calorie = 4.184J
const kgTNT = 1e6calorie
const alen = 622.77cm
const kWh = 1e3W*hour
const denier = 1g/9000m | Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 242 | struct SpyAxe
call::Vector{Any}
end
SpyAxe() = SpyAxe(Any[])
lines!(axe,args...;kwargs...) = push!(axe.call,(fun=:lines!,args=args,kwargs=kwargs))
scatter!(axe,args...;kwargs...) = push!(axe.call,(fun=:scatter!,args=args,kwargs=kwargs)) | Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 5285 | using Muscade
using StaticArrays,LinearAlgebra #,GLMakie
function horner(p::AbstractVector,x::Number) # avoiding to use e.g. Polynomials.jl just for test code
y = zero(x) # not typestable if eltype(p)≠typeof(x)
for i ∈ reverse(p)
y = i + x*y
end
return y
end
### Turbine
struct Turbine{Tsea,Tsky} <: AbstractElement
xₘ :: SVector{2,𝕣} # tx1,tx2
z :: 𝕣
seadrag :: 𝕣
sea :: Tsea # function
skydrag :: 𝕣
sky :: Tsky # function
end
Turbine(nod::Vector{Node};seadrag,sea,skydrag,sky) = Turbine(SVector(coord(nod)[1][1],coord(nod)[1][2]),coord(nod)[1][3],seadrag,sea,skydrag,sky)
@espy function Muscade.residual(o::Turbine, X,U,A, t,χ,χcv,SP,dbg)
☼x = ∂0(X)+o.xₘ
R = -o.sea(t,x)*o.seadrag*(1+A[1]) - o.sky(t,x)*o.skydrag*(1+A[2])
return R,noχ,noFB
end
function Muscade.draw(axe,o::Turbine, Λ,X,U,A, t,χ,χcv,SP,dbg)
x = ∂0(X)+o.xₘ
lines!(axe,SMatrix{2,3}(x[1],x[1],x[2],x[2],o.z-10,o.z+10)' ,color=:orange, linewidth=5)
end
Muscade.doflist( ::Type{<:Turbine}) = (inod =(1 ,1 ,2 ,2 ),
class=(:X ,:X ,:A ,:A ),
field=(:tx1,:tx2,:Δseadrag,:Δskydrag))
### AnchorLine
struct AnchorLine <: AbstractElement
xₘtop :: SVector{3,𝕣} # x1,x2,x3
Δxₘtop :: SVector{3,𝕣} # as meshed, node to fairlead
xₘbot :: SVector{2,𝕣} # x1,x2 (x3=0)
L :: 𝕣
buoyancy:: 𝕣
end
AnchorLine(nod::Vector{Node};Δxₘtop,xₘbot,L,buoyancy) = AnchorLine(coord(nod)[1],Δxₘtop,xₘbot,L,buoyancy)
p = SVector( 2.82040487827, -24.86027164695, 153.69500343165, -729.52107422849, 2458.11921356871,
-5856.85610233072, 9769.49700812681,-11141.12651712473, 8260.66447746395,-3582.36704093187,
687.83550335374)
@espy function Muscade.lagrangian(o::AnchorLine, δX,X,U,A,t,χ,χcv,SP,dbg)
xₘtop,Δxₘtop,xₘbot,L,buoyancy = o.xₘtop,o.Δxₘtop,o.xₘbot,o.L*(1+A[1]),o.buoyancy*(1+A[2]) # a for anchor, t for TDP, f for fairlead
x = ∂0(X)
☼Xtop = SVector(x[1],x[2],0.) + xₘtop
α = x[3] # azimut from COG to fairlead
c,s = cos(α),sin(α)
☼ΔXtop = SMatrix{3,3}(c,s,0,-s,c,0,0,0,1)*Δxₘtop # arm of the fairlead
☼ΔXchain = Xtop[1:2]+ΔXtop[1:2]-xₘbot # vector from anchor to fairlead
☼xaf = norm(ΔXchain) # horizontal distance from anchor to fairlead
☼cr = exp10(horner(p,(L-xaf)/Xtop[3]))*Xtop[3] # curvature radius at TDP
☼Fh = -cr*buoyancy # horizontal force
☼ltf = √(Xtop[3]^2+2Xtop[3]*cr) # horizontal distance from fairlead to TDP
Fd = ΔXchain/xaf.*Fh
m3 = ΔXtop[1]*Fd[2]-ΔXtop[2]*Fd[1]
δW = δX[1:2] ∘₁ Fd
δW += δX[3 ] * m3
return δW,noχ,noFB
end
function Muscade.draw(axe,o::AnchorLine, δX,X,U,A, t,χ,χcv,SP,dbg)
req = @request (Xtop,ΔXtop,ΔXchain,cr,xaf,ltf)
L,χn,FB,out = Muscade.lagrangian(o, δX,X,U,A, t,χ,χcv,SP,(dbg...,espy2draw=true),req)
Laf,Xbot,Xtop,ΔXtop,ΔXchain,cr,xaf,Ltf = o.L, o.xₘbot, out.Xtop,out.ΔXtop,out.ΔXchain, out.cr, out.xaf, out.ltf
n = ΔXchain./xaf # horizontal normal vector from anchor to fairlead
xat = Laf-Ltf
xtf = xaf-xat
Xtdp = Xbot + n*xat
x = range(max(0,-xat),xtf,11)
X = n[1].*x.+Xtdp[1]
Y = n[2].*x.+Xtdp[2]
Z = cr.*(cosh.(x./cr).-1)
lines!(axe,hcat(X,Y,Z) ,color=:blue, linewidth=2) # line
scatter!(axe,Xtdp ,markersize=10,color=:blue)
scatter!(axe,Xbot ,markersize=20,color=:red)
if xat>0
lines!(axe,hcat(Xbot,Xtdp) ,color=:green, linewidth=2) # seafloor
else
x = range(0,-xat,11)
X = n[1].*x.+Xtdp[1]
Y = n[2].*x.+Xtdp[2]
Z = cr.*(cosh.(x./cr).-1)
lines!(axe,hcat(X,Y,Z) ,color=:red, linewidth=5) # line
end
lines!(axe,hcat(Xtop,Xtop+ΔXtop) ,color=:red , linewidth=2) # excentricity
end
Muscade.doflist( ::Type{<:AnchorLine}) = (inod =(1 ,1 ,1 ,2 ,2 ),
class=(:X ,:X ,:X ,:A ,:A ),
field=(:tx1,:tx2,:rx3,:ΔL,:Δbuoyancy))
#### Spring
struct Spring{D} <: AbstractElement
x₁ :: SVector{D,𝕣} # x1,x2,x3
x₂ :: SVector{D,𝕣}
EI :: 𝕣
L :: 𝕣
end
Spring{D}(nod::Vector{Node};EI) where{D}= Spring{D}(coord(nod)[1],coord(nod)[2],EI,norm(coord(nod)[1]-coord(nod)[2]))
@espy function Muscade.residual(o::Spring{D}, X,U,A, t,χ,χcv,SP,dbg) where{D}
x₁ = ∂0(X)[SVector{D}(i for i∈1:D)]+o.x₁
x₂ = ∂0(X)[SVector{D}(i+D for i∈1:D)]+o.x₂
☼L₀ = o.L *exp10(A[1])
☼EI = o.EI*exp10(A[2])
Δx = x₁-x₂
☼L = norm(Δx)
☼T = EI*(L-L₀)
F₁ = Δx/L*T # external force on node 1
R = vcat(F₁,-F₁)
return R,noχ,noFB
end
Muscade.doflist( ::Type{Spring{D}}) where{D}=(
inod = (( 1 for i=1: D)...,(2 for i=1:D)...,3,3),
class = ((:X for i=1:2D)...,:A,:A),
field = ((Symbol(:tx,i) for i=1: D)...,(Symbol(:tx,i) for i=1: D)...,:ΞL₀,:ΞEI)) # \Xi
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 4369 | module TestAdiff
using Muscade
using Test,StaticArrays
const ∂𝕣11= ∂ℝ{1,1,𝕣}
const ∂𝕣12= ∂ℝ{1,2,𝕣}
const ∂𝕣22= ∂ℝ{2,2,𝕣}
## Constructors and promotion
dx1= ∂ℝ{1,2}(3.,SVector(.1,.2))
dx2= ∂ℝ{1,2}(2.,SVector(.3,.4))
dx3= ∂ℝ{1,2}(4.,SVector(.5,.6))
x = ∂ℝ{2,2}(dx1,SVector(dx2,dx3))
a = ∂ℝ{2,2}(dx1,SVector(1,2))
b = ∂ℝ{2,4}(dx1)
c = ∂ℝ{2,4}(dx1,3)
t1 = promote_rule(typeof(dx1),typeof(3))
t2 = promote_rule(typeof(dx1),typeof(x))
(d,e)=promote(dx1,7)
(g,h)=promote(dx1,x)
## Extraction
dscaled=δ{1,3,𝕣}(SVector(1.,2.,3.))
vscaled=variate{1,3}(SVector(4.,4.,4.),SVector(1.,2.,3.))
Δ = δ{1,2,𝕣}()
C1 = variate{constants(Δ),2}(SVector(3.,4.))
C = variate{constants(C1),2}(C1)
PC = precedence(C)
PC1 = precedence(C1)
vC = value{PC}(C)
∂C = ∂{PC,2}( C)
vvC = value{PC1}(vC)
v∂C = value{PC1}(∂C)
∂vC = ∂{PC1,2}( vC)
#∂∂C = ∂{PC1,2}( ∂C)
dX1 = toggle(false,dx1,3.)
## Operations
oa = variate{1}(1.)
ob = variate{constants(oa )}(2.)
oc = variate{constants(oa,ob)}(3.)
od = oa+oc
oe = od+ob
oj = od^2
og = 2^od
oh = oa^oc
ok = oc*oa/oc
ox = SVector(1.,2.,3.)
oX = variate{1,3}(ox)
## norm
using LinearAlgebra
nrm = norm(oX)
##
@testset "Adiff" begin
@testset "Adiff construct and promote" begin
@test dscaled[2] ≗ ∂ℝ{1, 3, Float64}(0.0, SVector(0.0, 2.0, 0.0))
@test vscaled[2] ≗ ∂ℝ{1, 3, Float64}(4.0, SVector(0.0, 2.0, 0.0))
@test dx1 ≗ ∂𝕣12(3.0, SVector(0.1, 0.2))
@test x ≗ ∂ℝ{2,2,∂𝕣12}(∂𝕣12(3.0, SVector(0.1, 0.2)), SVector(∂𝕣12(2.0, SVector(0.3, 0.4)), ∂𝕣12(4.0, SVector(0.5, 0.6))))
@test a ≗ ∂ℝ{2,2,∂𝕣12}(∂𝕣12(3.0, SVector(0.1, 0.2)), SVector(∂𝕣12(1.0, SVector(0.0, 0.0)), ∂𝕣12(2.0, SVector(0.0, 0.0))))
@test b ≗ ∂ℝ{2,4,∂𝕣12}(∂𝕣12(3.0, SVector(0.1, 0.2)), SVector(∂𝕣12(0.0, SVector(0.0, 0.0)), ∂𝕣12(0.0, SVector(0.0, 0.0)), ∂𝕣12(0.0, SVector(0.0, 0.0)), ∂𝕣12(0.0, SVector(0.0, 0.0))))
@test c ≗ ∂ℝ{2,4,∂𝕣12}(∂𝕣12(3.0, SVector(0.1, 0.2)), SVector(∂𝕣12(0.0, SVector(0.0, 0.0)), ∂𝕣12(0.0, SVector(0.0, 0.0)), ∂𝕣12(1.0, SVector(0.0, 0.0)), ∂𝕣12(0.0, SVector(0.0, 0.0))))
@test t1 == ∂𝕣12
@test t2 ==∂ℝ{2,2,∂𝕣12}
@test d ≗ dx1
@test e ≗ ∂𝕣12(7.0, SVector(0.0, 0.0))
@test typeof(g) == ∂ℝ{2,2,∂𝕣12}
@test g ≗ ∂ℝ{2,2,∂𝕣12}(∂𝕣12(3.0, SVector(0.0, 0.0)), SVector(∂𝕣12(0.1, SVector(0.0, 0.0)), ∂𝕣12(0.2, SVector(0.0, 0.0))))
@test h ≗ x
end
@testset "Adiff extraction" begin
@test Δ ≗ SVector(∂𝕣12(0.0, SVector(1.0, 0.0)),∂𝕣12(0.0, SVector(0.0, 1.0)))
@test constants(Δ) ≗ 2
@test typeof(C) == SVector{2, ∂ℝ{3, 2, ∂ℝ{2, 2, Float64}}}#Array{∂ℝ{3,2,∂𝕣22},1}
@test C[1] ≗ ∂ℝ{3,2,∂𝕣22}(∂𝕣22(3.0, SVector(1.0, 0.0)), SVector(∂𝕣22(1.0, SVector(0.0, 0.0)), ∂𝕣22(0.0, SVector(0.0, 0.0))))
@test vC[1] ≗ ∂𝕣22(3.0, SVector(1.0, 0.0))
@test ∂C[1] ≗ ∂𝕣22(1.0, SVector(0.0, 0.0))
@test vvC ≗ SVector(3.0,4.0)
@test v∂C ≗ SMatrix{2,2}(1.0,0.0,0.0,1.0)
@test ∂vC ≗ ∂vC
# @test ∂∂C ≗ zeros(2,2,2) broken=true # extract partial derivatives of an SMatrix or higher order
@test typeof(dX1) == ∂𝕣12
end
@testset "Adiff operations" begin
@test oa ≗ ∂𝕣11(1.0, SVector(1.0))
@test ob ≗ ∂ℝ{2,1,𝕣}(2, SVector(1))
@test oc ≗ ∂ℝ{3,1,𝕣}(3.0, SVector(1.0))
@test od ≗ ∂ℝ{3,1,∂𝕣11}(∂𝕣11(4.0, SVector(1.0)), SVector(∂𝕣11(1.0, SVector(0.0))))
@test oe ≗ ∂ℝ{3,1,∂ℝ{2,1,∂𝕣11}}(∂ℝ{2,1,∂𝕣11}(∂𝕣11(6.0, SVector(1.0)), SVector(∂𝕣11(1.0, SVector(0.0)))), SVector( ∂ℝ{2,1,∂𝕣11}(∂𝕣11(1.0, SVector(0.0)), SVector(∂𝕣11(0.0, SVector(0.0))))))
@test od ≗ ∂ℝ{3,1,∂𝕣11}(∂𝕣11(4.0, SVector(1.0)), SVector(∂𝕣11(1.0, SVector(0.0))))
@test og ≗ ∂ℝ{3,1,∂𝕣11}(∂𝕣11(16.0, SVector(11.090354888959125)), SVector(∂𝕣11(11.090354888959125, SVector(7.687248222691222))))
@test oj ≗ od*od
@test og ≗ ∂ℝ{3,1,∂𝕣11}(∂𝕣11(16.0, SVector(11.090354888959125)), SVector(∂𝕣11(11.090354888959125, SVector(7.687248222691222))))
@test ok ≗ ∂ℝ{3,1,∂𝕣11}(∂𝕣11(1.0, SVector(1.0)), SVector(∂𝕣11(0.0, SVector(0.0))))
@test value{1}(2*oX)==2*ox
end
@testset " norm" begin
@test nrm ≗ sqrt(sum(oX.^2))
end
end # testset Adiff
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 5180 | module TestAssemble
using Test,StaticArrays,SparseArrays
using Muscade
using Muscade.ElTest
include("SomeElements.jl")
### Turbine
sea(t,x) = SVector(1.,0.)
sky(t,x) = SVector(0.,1.)
turbine = Turbine(SVector(0.,0.),-10., 2.,sea, 3.,sky)
δX = @SVector [1.,1.]
X = @SVector [1.,2.]
U = @SVector 𝕣[]
A = @SVector [0.,0.] # [Δseadrag,Δskydrag]
# eleobj, Λ, X, U, A, t, χ, χcv, SP, dbg
L,Lδx,Lx,Lu,La,χn = gradient(turbine,δX,[X],[U],A, 0.,nothing,identity,nothing,(;))
@testset "Turbine gradient" begin
@test Lδx ≈ [-2, -3]
@test Lx ≈ [0, 0]
@test length(Lu) == 0
@test La ≈ [-2, -3]
end
Lδx,Lx,Lu,La,χn = test_static_element(turbine;δX,X,U,A,verbose=false)
@testset "test_static_element" begin
@test Lδx ≈ [-2, -3]
@test Lx ≈ [0, 0]
@test length(Lu) == 0
@test La ≈ [-2, -3]
end
# ### AnchorLine
anchorline = AnchorLine(SVector(0.,0.,100.), SVector(0,2.,0), SVector(94.,0.), 170., -1.)
δX = @SVector [1.,1.,1.]
X = @SVector [0.,0.,0.]
U = @SVector 𝕣[]
A = @SVector [0.,0.] # [Δseadrag,Δskydrag]
# eleobj, Λ, X, U, A, t, χ, χcv, SP, dbg
L,Lδx,Lx,Lu,La = gradient(anchorline,δX,[X],[U],A, 0.,nothing,identity,nothing,(;))
@testset "anchorline1" begin
@test Lδx ≈ [-12.25628901693551, 0.2607721067433087, 24.51257803387102]
@test Lx ≈ [-0.91509745608786, 0.14708204066349, 1.3086506986891027]
@test length(Lu) == 0
@test La ≈ [-156.06324599170992, 12.517061123678818]
end
model = Model(:TestModel)
n1 = addnode!(model,𝕣[0,0,+100])
n2 = addnode!(model,𝕣[])
n3 = addnode!(model,𝕣[])
sea(t,x) = SVector(1.,0.)
sky(t,x) = SVector(0.,1.)
e1 = addelement!(model,Turbine ,[n1,n2], seadrag=2., sea=sea, skydrag=3., sky=sky)
e2 = addelement!(model,AnchorLine,[n1,n3], Δxₘtop=SVector(5.,0.,0), xₘbot=SVector(150.,0.), L=180., buoyancy=-1e3)
dis = Muscade.Disassembler(model)
@testset "Disassembler" begin
@test dis.dis[1].index[1].X == [1,2]
@test dis.dis[1].index[1].U == []
@test dis.dis[1].index[1].A == [1,2]
@test dis.dis[2].index[1].X == [1,2,3]
@test dis.dis[2].index[1].U == []
@test dis.dis[2].index[1].A == [3,4]
@test dis.scaleX ≈ [1,1,1]
@test dis.scaleU ≈ 𝕫[]
@test dis.scaleA ≈ [1,1,1,1]
end
dofgr = Muscade.allXdofs(model,dis)
Λ,X,U,A = Muscade.indexedstate(dofgr)
nΛ,nX,nU,nA = Muscade.gradientstructure(dofgr,dis.dis[1]) # number of dofs of each class in the gradient returned by an element
iΛ,iX,iU,iA = Muscade.gradientpartition(nΛ,nX,nU,nA) # indices into said gradient
@testset "dofgr" begin
@test dofgr.nX == 3
@test dofgr.nU == 0
@test dofgr.nA == 4
@test dofgr.iΛ == Int64[]
@test dofgr.iX == 1:3
@test dofgr.iU == Int64[]
@test dofgr.iA == Int64[]
@test dofgr.jΛ == 1:0
@test dofgr.jX == 1:3
@test dofgr.jU == 4:3
@test dofgr.jA == 4:3
@test dofgr.scaleΛ == Float64[]
@test dofgr.scaleX ≈ [1,1,1]
@test dofgr.scaleU == Float64[]
@test dofgr.scaleA == Float64[]
end
@testset "state" begin
@test Λ == [0, 0 ,0]
@test X == [1, 2, 3]
@test U == Int64[]
@test A == [0, 0, 0, 0]
@test nΛ == 0
@test nX == 2
@test nU == 0
@test nA == 0
@test iΛ == 1:0
@test iX == 1:2
@test iU == 3:2
@test iA == 3:2
end
neletyp = 2
asmvec = Vector{𝕫2}(undef,neletyp)
Lλ = Muscade.asmvec!(asmvec,dofgr,dis)
@testset "asmvec" begin
@test typeof(Lλ)== Vector{Float64}
@test length(Lλ)== 3
@test asmvec[1] == [1; 2;;]
@test asmvec[2] == [1; 2; 3;;]
end
out,asm,dofgr = Muscade.prepare(Muscade.AssemblyStaticX,model,dis)
Muscade.zero!(out)
@testset "prepare" begin
@test Muscade.firstelement(out).Lλ ≈ [0,0,0]
@test Muscade.firstelement(out).Lλx ≈ sparse([1,2,3,2,3], [1,2,2,3,3], [0,0,0,0,0], 3, 3)
@test asm[1,1] == [1; 2;;]
@test asm[1,2] == [1; 2; 3;;]
@test asm[2,1] == [1; 2; 4; 5;;]
@test asm[2,2] == [1; 2; 3; 4; 5; 6; 7; 8; 9;;]
end
@testset "dofgr again" begin
@test dofgr.nX == 3
@test dofgr.nU == 0
@test dofgr.nA == 4
@test dofgr.iΛ == Int64[]
@test dofgr.iX == 1:3
@test dofgr.iU == Int64[]
@test dofgr.iA == Int64[]
@test dofgr.jΛ == 1:0
@test dofgr.jX == 1:3
@test dofgr.jU == 4:3
@test dofgr.jA == 4:3
@test dofgr.scaleΛ == Float64[]
@test dofgr.scaleX ≈ [1,1,1]
@test dofgr.scaleU == Float64[]
@test dofgr.scaleA == Float64[]
end
state = Muscade.State(model,dis)
Muscade.assemble!(out,asm,dis,model,state,(someunittest=true,))
@testset "assemble" begin
@test Muscade.firstelement(out).Lλ ≈ [-152130.71199858442, -3.0, 0.0]
@test Muscade.firstelement(out).Lλx ≈ sparse([1, 2, 3, 1, 2, 3, 1, 2, 3], [1, 1, 1, 2, 2, 2, 3, 3, 3], [10323.069597975566, 0.0, 0.0, 0.0, 1049.1635310247202, 5245.817655123601, 0.0, 5245.8176551236, 786872.6482685402], 3, 3)
end
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 7410 | module TestConstraints
using Test,StaticArrays
using Muscade
Muscade.DofConstraint{:X, Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield,λinod,λfield }(g,mode,gₛ,λₛ) where
{Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield,λinod,λfield} =
Muscade.DofConstraint{:X,Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield,λinod,λfield,typeof(g),typeof(()),typeof(mode)}(g,(),mode,gₛ,λₛ)
Muscade.DofConstraint{:U, Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield,λinod,λfield }(g,mode,gₛ,λₛ) where
{Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield,λinod,λfield} =
Muscade.DofConstraint{:U,Nx,Nu,Na,xinod,xfield,uinod,ufield,ainod,afield,λinod,λfield,typeof(g),typeof(()),typeof(mode)}(g,(),mode,gₛ,λₛ)
#t,γ,dbg = 0.,1.,(status=:testing,)
t,χ,χcv,dbg = 0.,nothing,identity,(status=:testing,)
SP1 = (γ=1.,)
SP0 = (γ=0.,)
#---------------------------------------------------------
g(x,t) = .3x[1] + .4x[2]
Xctc = Muscade.variate{1,3}(SVector{3}(4,-3,10.)) # contact
Xgap = Muscade.variate{1,3}(SVector{3}(4,3,10.)) # gap
U = SVector{0,𝕣}()
A = SVector{0,𝕣}()
C = Muscade.DofConstraint{:X, 2 ,0 ,0 ,(1,1),(:t1,:t2),() ,() ,() ,() ,1 ,:λ }
# DofConstraint{λclass,Nx,Nu,Na,xinod,xfield ,uinod,ufield,ainod,afield,λinod,λfield}(g,mode,gₛ,λₛ)
@testset "X equal contact" begin
c = C(g,equal,1,1)
r,χn,FB = residual(c, (Xctc,),(U,),A, t,χ,χcv,SP1,dbg)
R,R∂X = Muscade.value_∂{1,3}(r)
@test doflist(typeof(c)) == (inod=(1,1,1),class=(:X,:X,:X),field=(:t1,:t2,:λ))
@test R ≈ [-3,-4,0]
@test R∂X ≈ [0 0 -.3;
0 0 -.4;
-.3 -.4 0 ]
end
@testset "X equal gap" begin
c = C(g,equal,1,1)
r,χn,FB = residual(c, (Xgap,),(U,),A, t,χ,χcv,SP1,dbg)
R,R∂X = Muscade.value_∂{1,3}(r)
@test R ≈ [-3, -4, -2.4]
@test R∂X ≈ [0 0 -.3;
0 0 -.4;
-.3 -.4 0 ]
end
@testset "X off" begin
c = C(g,off,1,1)
r,χn,FB = residual(c, (Xctc,),(U,),A, t,χ,χcv,SP1,dbg)
R,R∂X = Muscade.value_∂{1,3}(r)
@test R ≈ [0,0,-10]
@test R∂X ≈ [0 0 0; 0 0 0; 0 0 -1]
end
@testset "X positive contact" begin
c = C(g,positive,1,1)
r,χn,FB = residual(c, (Xctc,),(U,),A, t,χ,χcv,SP1,dbg)
R,R∂X = Muscade.value_∂{1,3}(r)
@test R ≈ [-3.0, -4.0, 0.09901951359278449]
@test R∂X ≈ [-0.0 -0.0 -0.3; -0.0 -0.0 -0.4; -0.29708710135363803 -0.39611613513818406 -0.009709662154539889]
end
@testset "X positive gap" begin
c = C(g,positive,1,1)
r,χn,FB = residual(c, (Xgap,),(U,),A, t,χ,χcv,SP1,dbg)
R,R∂X = Muscade.value_∂{1,3}(r)
@test R ≈ [-3.0, -4.0, -2.2706234591223002]
@test R∂X ≈ [-0.0 -0.0 -0.3; -0.0 -0.0 -0.4; -0.29506118058939695 -0.39341490745252927 -0.01646273136867682]
end
@testset "X positive contact γ==0" begin
c = C(g,positive,1,1)
r,χn,FB = residual(c, (Xctc,),(U,),A, t,χ,χcv,SP0,dbg)
R,R∂X = Muscade.value_∂{1,3}(r)
@test R ≈ [-3,-4,0]
@test R∂X ≈ [0 0 -.3;
0 0 -.4;
-.3 -.4 0 ]
end
@testset "X positive gap γ==0" begin
c = C(g,positive,1,1)
r,χn,FB = residual(c, (Xgap,),(U,),A, t,χ,χcv,SP0,dbg)
R,R∂X = Muscade.value_∂{1,3}(r)
@test R ≈ [-3, -4, -2.4]
@test R∂X ≈ [0 0 -.3;
0 0 -.4;
-.3 -.4 0 ]
end
#---------------------------------------------------------
g(x,u,a,t) = .3u[1] + .4u[2]
Uctc = Muscade.variate{2,3}(Muscade.variate{1,3}(SVector{3}(4,-3,10.))) # contact
Ugap = Muscade.variate{2,3}(Muscade.variate{1,3}(SVector{3}(4, 3,10.))) # gap
Λ = SVector{0,𝕣}()
X = SVector{0,𝕣}()
A = SVector{0,𝕣}()
C = Muscade.DofConstraint{:U, 0 ,2 ,0 ,() ,() ,(1,1),(:t1,:t2),() ,() ,1 ,:λ }
# DofConstraint{λclass,Nx,Nu,Na,xinod,xfield ,uinod,ufield ,ainod,afield,λinod,λfield}(g,mode,gₛ,λₛ)
@testset "U equal contact" begin
c = C(g,equal,1,1)
r,χn,FB = lagrangian(c, Λ,(X,),(Uctc,),A, t,χ,χcv,SP1,dbg)
R,R∂U = Muscade.value_∂{1,3}(Muscade.∂{2,3}(r))
@test doflist(typeof(c)) == (inod=(1,1,1),class=(:U,:U,:U),field=(:t1,:t2,:λ))
@test R ≈ [-3,-4,0]
@test R∂U ≈ [0 0 -.3;
0 0 -.4;
-.3 -.4 0 ]
end
@testset "U equal gap" begin
c = C(g,equal,1,1)
r,χn,FB = lagrangian(c, Λ,(X,),(Ugap,),A, t,χ,χcv,SP1,dbg)
R,R∂U = Muscade.value_∂{1,3}(Muscade.∂{2,3}(r))
@test R ≈ [-3, -4, -2.4]
@test R∂U ≈ [0 0 -.3;
0 0 -.4;
-.3 -.4 0 ]
end
@testset "U off" begin
c = C(g,off,1,1)
r,χn,FB = lagrangian(c, Λ,(X,),(Uctc,),A, t,χ,χcv,SP1,dbg)
R,R∂U = Muscade.value_∂{1,3}(Muscade.∂{2,3}(r))
@test R ≈ [0,0,-10]
@test R∂U ≈ [0 0 0; 0 0 0; 0 0 -1]
end
@testset "U positive contact" begin
c = C(g,positive,1,1)
r,χn,FB = lagrangian(c, Λ,(X,),(Uctc,),A, t,χ,χcv,SP1,dbg)
R,R∂U = Muscade.value_∂{1,3}(Muscade.∂{2,3}(r))
@test R ≈ [-3.0, -4.0, 0.09901951359278449]
@test R∂U ≈ [-0.0 -0.0 -0.3; -0.0 -0.0 -0.4; -0.29708710135363803 -0.39611613513818406 -0.009709662154539889]
end
@testset "U positive gap" begin
c = C(g,positive,1,1)
r,χn,FB = lagrangian(c, Λ,(X,),(Ugap,),A, t,χ,χcv,SP1,dbg)
R,R∂U = Muscade.value_∂{1,3}(Muscade.∂{2,3}(r))
@test R ≈ [-3.0, -4.0, -2.2706234591223002]
@test R∂U ≈ [-0.0 -0.0 -0.3; -0.0 -0.0 -0.4; -0.29506118058939695 -0.39341490745252927 -0.01646273136867682]
end
@testset "U positive contact γ==0" begin
c = C(g,positive,1,1)
r,χn,FB = lagrangian(c, Λ,(X,),(Uctc,),A, t,χ,χcv,SP0,dbg)
R,R∂U = Muscade.value_∂{1,3}(Muscade.∂{2,3}(r))
@test R ≈ [-3,-4,0]
@test R∂U ≈ [0 0 -.3;
0 0 -.4;
-.3 -.4 0 ]
end
@testset "U positive gap γ==0" begin
c = C(g,positive,1,1)
r,χn,FB = lagrangian(c, Λ,(X,),(Ugap,),A, t,χ,χcv,SP0,dbg)
R,R∂U = Muscade.value_∂{1,3}(Muscade.∂{2,3}(r))
@test R ≈ [-3, -4, -2.4]
@test R∂U ≈ [0 0 -.3;
0 0 -.4;
-.3 -.4 0 ]
end
#---------------------------------------------------------
g1(x,t) = -0.1*sin(1.2*x[1])+.2x[1]+x[2]-1 # start outside feasible domain 😃
g2(x,t) = -.4x[1] + .3x[2]+.1
f1(x) = -(-0.1*sin(1.2*x)+.2x-1)
f2(x) = (-1/.3)*(-.4x + .1)
gravity(t) = -2.
model = Model(:TestModel)
n1 = addnode!(model,𝕣[0,0])
e1 = addelement!(model,DofConstraint,[n1],xinod=(1,1),xfield=(:t1,:t2),λinod=1, λclass=:X, λfield=:λ1,gap=g1,mode=positive)
e2 = addelement!(model,DofConstraint,[n1],xinod=(1,1),xfield=(:t1,:t2),λinod=1, λclass=:X, λfield=:λ2,gap=g2,mode=positive)
e3 = addelement!(model,DofLoad ,[n1],field=:t2,value=gravity)
initialstate = initialize!(model)
state = solve(StaticX;initialstate,time=[0.],verbose=false) # because there is zero physical stiffness in this model, setting γ0=0 gives singularity if one or more constraint is inactive
@testset "interior point" begin
X = state[findlastassigned(state)].X[1][1:2]
@test g1(X,0) ≈ 0.0
@test abs(g2(X,0)) < 1e-16
end
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 1970 | module TestElementAPI
using Test,StaticArrays
using Muscade
include("SomeElements.jl")
### Turbine
sea(t,x) = SVector(1.,0.)
sky(t,x) = SVector(0.,1.)
turbine = Turbine(SVector(0.,0.),-10., 2.,sea, 3.,sky)
X = @SVector [1.,2.]
U = @SVector []
A = @SVector [0.,0.] # [Δseadrag,Δskydrag]
R,χ,FB =residual(turbine, [X],[U],A, 0.,nothing,identity,nothing,(dbg=true,))
@testset "Turbine" begin
@test R ≈ [-2, -3]
end
T = typeof(turbine)
@testset "Element utility functions" begin
@test Muscade.getdoflist(T) == ((1, 1, 2, 2),(:X, :X, :A, :A),(:tx1, :tx2, :Δseadrag, :Δskydrag))
@test Muscade.getidof(T,:X) == [1,2]
@test Muscade.getidof(T,:U) == []
@test Muscade.getidof(T,:A) == [3,4]
@test Muscade.getndof(T) == 4
@test Muscade.getndof(T,:X) == 2
@test Muscade.getndof(T,(:X,:U,:A)) == (2,0,2)
@test Muscade.getnnod(T) == 2
end
# ### AnchorLine
anchorline = AnchorLine(SVector(0.,0.,100.), SVector(0,2.,0), SVector(94.,0.), 170., -1.)
δX = @SVector [1.,1.,1.]
X = @SVector [0.,0.,0.]
U = @SVector []
A = @SVector [0.,0.] # [Δseadrag,Δskydrag]
L1,χ,FB = lagrangian(anchorline, δX,[X],[U],A, 0.,nothing,identity,nothing,(dbg=true,))
X = [0,-1,45/180*π]
L2,χ,FB = lagrangian(anchorline, δX,[X],[U],A, 0.,nothing,identity,nothing,(dbg=true,))
@testset "Lagrangian" begin
@test L1 ≈ 12.517061123678818
@test L2 ≈ 5.590087401683872
end
### Spring
T = Spring{2}
@testset "Element utility functions" begin
@test Muscade.getdoflist(T) == ((1, 1, 2, 2, 3, 3), (:X, :X, :X, :X, :A, :A), (:tx1, :tx2, :tx1, :tx2, :ΞL₀, :ΞEI))
@test Muscade.getidof(T,:X) == [1,2,3,4]
@test Muscade.getidof(T,:U) == []
@test Muscade.getidof(T,:A) == [5,6]
@test Muscade.getndof(T) == 6
@test Muscade.getndof(T,:X) == 4
@test Muscade.getndof(T,(:X,:U,:A)) == (4,0,2)
@test Muscade.getnnod(T) == 3
end
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 2764 | module TestElementCost
using Test,StaticArrays
using Muscade
include("SomeElements.jl")
model = Model(:TestModel)
n1 = addnode!(model,𝕣[0,0,+100]) # turbine
n3 = addnode!(model,𝕣[]) # Anod for anchor
@once cost(eleres,X,U,A,t) = eleres.Fh^2
el = ElementCost(model.nod;req=@request(Fh),cost,ElementType=AnchorLine,
Δxₘtop=[5.,0,0], xₘbot=[250.,0], L=290., buoyancy=-5e3)
d = doflist(typeof(el))
Nx,Nu,Na = 3,0,2
Nz = 2Nx+Nu+Na
iλ,ix,iu,ia = Muscade.gradientpartition(Nx,Nx,Nu,Na)
ΔZ = δ{1,Nz,𝕣}()
ΔΛ,ΔX,ΔU,ΔA = view(ΔZ,iλ),view(ΔZ,ix),view(ΔZ,iu),view(ΔZ,ia)
Λ = zeros(Nx)
X = (ones(Nx),)
U = (zeros(Nu),)
A = zeros(Na)
L,χ,FB = lagrangian(el, Λ+ΔΛ, (∂0(X)+ΔX,),(∂0(U)+ΔU,),A+ΔA, 0.,nothing,identity,nothing,(testall=true,))
@testset "ElementCost" begin
@test d == (inod = (1, 1, 1, 2, 2), class = (:X, :X, :X, :A, :A), field = (:tx1, :tx2, :rx3, :ΔL, :Δbuoyancy))
@test value{1}(L) ≈ 1.926851845351649e11
@test ∂{1,Nz}(L) ≈ [-438861.1307445675,9278.602091074139,1.8715107899328927e6,-2.322235123921358e10,4.9097753633879846e8,9.903105530914653e10,-6.735986859485705e12,3.853703690703298e11]
end
###
@once gap(eleres,X,U,A,t) = eleres.Fh^2
el = ElementConstraint(model.nod;req=@request(Fh),gap,ElementType=AnchorLine,λinod=1,λfield=:λ,mode=equal,
Δxₘtop=[5.,0,0], xₘbot=[250.,0], L=290., buoyancy=-5e3)
d = doflist(typeof(el))
Nx,Nu,Na = 3,0+1,2
Nz = 2Nx+Nu+Na
iλ,ix,iu,ia = Muscade.gradientpartition(Nx,Nx,Nu,Na)
ΔZ = δ{1,Nz,𝕣}()
ΔΛ,ΔX,ΔU,ΔA = view(ΔZ,iλ),view(ΔZ,ix),view(ΔZ,iu),view(ΔZ,ia)
Λ = SVector{Nx}(0. for i=1:Nx)
X = (SVector{Nx}(1. for i=1:Nx),)
U = (SVector{Nu}(1. for i=1:Nu),)
A = SVector{Na}(0. for i=1:Na)
L,χ,FB = lagrangian(el, Λ+ΔΛ, (∂0(X)+ΔX,),(∂0(U)+ΔU,),A+ΔA, 0.,nothing,identity,nothing,(testall=true,))
@testset "ElementConstraint" begin
@test d == (inod = (1, 1, 1, 2, 2, 1), class = (:X, :X, :X, :A, :A, :U), field = (:tx1, :tx2, :rx3, :ΔL, :Δbuoyancy, :λ))
@test value{1}(L) ≈ -1.926851845351649e11
@test ∂{1,Nz}(L) ≈ [-438861.1307445675, 9278.602091074139, 1.8715107899328927e6, 2.322235123921358e10, -4.9097753633879846e8, -9.903105530914653e10, -1.926851845351649e11, 6.735986859485705e12, -3.853703690703298e11]
end
req = @request λ,eleres(cr)
L,χ,FB,eleres = lagrangian(el, Λ+ΔΛ, X,U,A, 0.,nothing,identity,nothing,(testall=true,),req)
@testset "ElementConstraintResult" begin
@test eleres.λ ≈ 1.
@test eleres.eleres.cr ≈ 87.79184120068672
@test eleres.eleres.Fh ≈ 438959.2060034336
end
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 10175 | module TestEspy
using Test,StaticArrays,MacroTools
include("../src/Dialect.jl")
include("../src/Espy.jl")
include("../src/Exceptions.jl")
macro expression(ex) return QuoteNode(ex) end
pretty(ex) = println(prettify(ex))
####
exresidual = @macroexpand @espy function residual(x::Vector{R},y) where{R<:Real}
ngp=2
accum = ntuple(ngp) do igp
☼z = x[igp]+y[igp]
☼s,☼t = ☼material(z)
@named(s)
end
r = sum(i->accum[i].s,ngp)
return r,nothing,nothing
end
exmaterial = @macroexpand @espy function material(z)
☼a = z+1
☼b = a*z
return b,3.
end
exlagrangian = @macroexpand @espy lagrangian(o::Vector{R}, δX,X,U,A, t,γ,dbg) where{R} = o.cost(∂n(X,R)[1],t),nothing,nothing
exbar = @macroexpand @espy function bar(x,y,z)
ngp = 4
vec = SVector{2}
☼p = 3
for i ∈ 1:2
j = i^2
k = i+j
end
t = ntuple(ngp) do igp
a = vec(igp,igp^2)
b = vec(1.,1.)
☼c = b*b'
♢square = c^2
χ = randn()
r = vcat(a,reshape(c,4))
@named(χ,r)
end
χ = ntuple(i->t[i].χ,ngp)
r = sum( i->t[i].r,ngp)
return r,χ,nothing
end
exmerge = @macroexpand @espy function lagrangian(o::ElementConstraint{Teleobj,λinod,λfield,Nu}, Λ,X,U,A,t,χ,χcv,SP,dbg) where{Teleobj,λinod,λfield,Nu}
req = merge(o.req)
γ = default{:γ}(SP,0.)
u = getsomedofs(U,SVector{Nu}(1:Nu))
☼λ = ∂0(U)[Nu+1]
L,χn,FB,☼eleres = getlagrangian(implemented(o.eleobj)...,o.eleobj,Λ,X,u,A,t,χ,χcv,SP,(dbg...,via=ElementConstraint),req)
☼gap = o.gap(eleres,X,u,A,t,o.gargs...)
if o.mode(t)==:equal; return L-gap*λ ,noχ,(α=∞ ,)
elseif o.mode(t)==:positive; return L-KKT(λ,gap,γ,o.λₛ,o.gₛ) ,noχ,(α=decided(λ/o.λₛ,gap/o.gₛ,γ),)
elseif o.mode(t)==:off; return L-o.gₛ/(2o.λₛ)*λ^2 ,noχ,(α=∞ ,)
end
end
############# @espy outputs
exresidual_ = quote
function residual(x::Vector{R}, y) where R <: Real
ngp = 2
accum = ntuple(ngp) do igp
z = x[igp] + y[igp]
(s, t) = material(z)
(s = s,)
end
r = sum((i->(accum[i]).s), ngp)
return (r, nothing, nothing)
end
function residual(x::Vector{R}, y, req_001; ) where R <: Real
out_001 = (;)
ngp = 2
req_001_gp = if haskey(req_001, :gp)
req_001.gp
else
nothing
end
accum = ntuple(ngp) do igp
out_001_gp = (;)
z = x[igp] + y[igp]
out_001_gp_001 = if haskey(req_001_gp, :z)
(out_001_gp..., z = z)
else
out_001_gp
end
z
if haskey(req_001_gp, :material)
(s, t, out_001_gp_002) = material(z, req_001_gp.material)
out_001_gp_002 = (out_001_gp_001..., material = out_001_gp_002)
else
(s, t) = material(z)
out_001_gp_002 = out_001_gp_001
end
out_001_gp_003 = if haskey(req_001_gp, :s)
(out_001_gp_002..., s = s)
else
out_001_gp_002
end
out_001_gp_004 = if haskey(req_001_gp, :t)
(out_001_gp_003..., t = t)
else
out_001_gp_003
end
(s, t)
(s = s, out = out_001_gp_004)
end
out_002 = if haskey(req_001, :gp)
(out_001..., gp = NTuple{ngp}(((accum[igp]).out for igp = 1:ngp)))
else
out_001
end
r = sum((i->(accum[i]).s), ngp)
return (r, nothing, nothing, out_002)
end
end
exmaterial_ = quote
function material(z)
a = z + 1
b = a * z
return (b, 3.0)
end
function material(z, req_001; )
out_001 = (;)
a = z + 1
out_002 = if haskey(req_001, :a)
(out_001..., a = a)
else
out_001
end
a
b = a * z
out_003 = if haskey(req_001, :b)
(out_002..., b = b)
else
out_002
end
b
return (b, 3.0, out_003)
end
end
exlagrangian_ = quote
(lagrangian(o::Vector{R}, δX, X, U, A, t, γ, dbg) where R) = (o.cost((∂n(X, R))[1], t), nothing, nothing)
(lagrangian(o::Vector{R}, δX, X, U, A, t, γ, dbg, req) where R) = (o.cost((∂n(X, R))[1], t), nothing, nothing, nothing)
end
exbar_ = quote
function bar(x, y, z)
ngp = 4
vec = SVector{2}
p = 3
for i = 1:2
j = i ^ 2
k = i + j
end
t = ntuple(ngp) do igp
a = vec(igp, igp ^ 2)
b = vec(1.0, 1.0)
c = b * b'
nothing
χ = randn()
r = vcat(a, reshape(c, 4))
(χ = χ, r = r)
end
χ = ntuple((i->(t[i]).χ), ngp)
r = sum((i->(t[i]).r), ngp)
return (r, χ, nothing)
end
function bar(x, y, z, req_001; )
out_001 = (;)
ngp = 4
vec = SVector{2}
p = 3
out_002 = if haskey(req_001, :p)
(out_001..., p = p)
else
out_001
end
p
for i = 1:2
j = i ^ 2
k = i + j
end
req_001_gp = if haskey(req_001, :gp)
req_001.gp
else
nothing
end
t = ntuple(ngp) do igp
out_002_gp = (;)
a = vec(igp, igp ^ 2)
b = vec(1.0, 1.0)
c = b * b'
out_002_gp_001 = if haskey(req_001_gp, :c)
(out_002_gp..., c = c)
else
out_002_gp
end
c
if haskey(req_001_gp, :square)
square = c ^ 2
out_002_gp_002 = (out_002_gp_001..., square = square)
else
out_002_gp_002 = out_002_gp_001
end
χ = randn()
r = vcat(a, reshape(c, 4))
(χ = χ, r = r, out = out_002_gp_002)
end
out_003 = if haskey(req_001, :gp)
(out_002..., gp = NTuple{ngp}(((t[igp]).out for igp = 1:ngp)))
else
out_002
end
χ = ntuple((i->(t[i]).χ), ngp)
r = sum((i->(t[i]).r), ngp)
return (r, χ, nothing, out_003)
end
end
exmerge_ = quote
function lagrangian(o::ElementConstraint{Teleobj, λinod, λfield, Nu}, Λ, X, U, A, t, χ, χcv, SP, dbg) where {Teleobj, λinod, λfield, Nu}
req = merge(o.req)
γ = default{:γ}(SP, 0.0)
u = getsomedofs(U, SVector{Nu}(1:Nu))
λ = (∂0(U))[Nu + 1]
(L, χn, FB, eleres) = getlagrangian(implemented(o.eleobj)..., o.eleobj, Λ, X, u, A, t, χ, χcv, SP, (dbg..., via = ElementConstraint), req)
gap = o.gap(eleres, X, u, A, t, o.gargs...)
if o.mode(t) == :equal
return (L - gap * λ, noχ, (α = ∞,))
elseif o.mode(t) == :positive
return (L - KKT(λ, gap, γ, o.λₛ, o.gₛ), noχ, (α = decided(λ / o.λₛ, gap / o.gₛ, γ),))
elseif o.mode(t) == :off
return (L - (o.gₛ / (2 * o.λₛ)) * λ ^ 2, noχ, (α = ∞,))
end
end
function lagrangian(o::ElementConstraint{Teleobj, λinod, λfield, Nu}, Λ, X, U, A, t, χ, χcv, SP, dbg, req_001; ) where {Teleobj, λinod, λfield, Nu}
out_001 = (;)
req = merge(req_001, o.req)
γ = default{:γ}(SP, 0.0)
u = getsomedofs(U, SVector{Nu}(1:Nu))
λ = (∂0(U))[Nu + 1]
out_002 = if haskey(req_001, :λ)
(out_001..., λ = λ)
else
out_001
end
λ
(L, χn, FB, eleres) = getlagrangian(implemented(o.eleobj)..., o.eleobj, Λ, X, u, A, t, χ, χcv, SP, (dbg..., via = ElementConstraint), req)
out_003 = if haskey(req_001, :eleres)
(out_002..., eleres = eleres)
else
out_002
end
(L, χn, FB, eleres)
gap = o.gap(eleres, X, u, A, t, o.gargs...)
out_004 = if haskey(req_001, :gap)
(out_003..., gap = gap)
else
out_003
end
gap
if o.mode(t) == :equal
return (L - gap * λ, noχ, (α = ∞,), out_004)
elseif o.mode(t) == :positive
return (L - KKT(λ, gap, γ, o.λₛ, o.gₛ), noχ, (α = decided(λ / o.λₛ, gap / o.gₛ, γ),), out_004)
elseif o.mode(t) == :off
return (L - (o.gₛ / (2 * o.λₛ)) * λ ^ 2, noχ, (α = ∞,), out_004)
end
end
end
@testset "Transformed codes" begin
@test prettify(exresidual) == prettify(exresidual_)
@test prettify(exmaterial) == prettify(exmaterial_)
@test prettify(exlagrangian) == prettify(exlagrangian_)
@test prettify(exbar) == prettify(exbar_)
@test prettify(exmerge) == prettify(exmerge_)
end
r1 = @request (a,b,c)
r2 = @request (a,b,c(x,y))
@testset "Request" begin
@test r1 == (a = nothing, b = nothing, c = nothing)
@test r2 == (a = nothing, b = nothing, c = (x = nothing, y = nothing))
end
r1 = @request a(x(α),y),b,e
r2 = @request a(x(α),z),c(x,y),d(f),e
r = merge(r1,r2)
@testset "MergeRequest" begin
@test r == (a=(x=(α=nothing,),y=nothing,z=nothing),b=nothing,e=nothing,c=(x=nothing,y=nothing),d=(f=nothing,))
end
eval(exresidual)
eval(exmaterial)
eval(exlagrangian)
eval(exbar)
req = @request gp(z,s,t,material(a,b))
r,χ,SFB,out = residual([1.,2.],[3.,4.],req)
@testset "Get output" begin
@test out == (gp = ((z = 4.0, material = (a = 5.0, b = 20.0), s = 20.0, t = 3.0), (z = 6.0, material = (a = 7.0, b = 42.0), s = 42.0, t = 3.0)),)
end
end # Module
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 4058 | module TestModelDescription
using Test
using Muscade
using Muscade: DofID,EleID,NodID
include("SomeElements.jl")
model = Model(:TestModel)
n1 = addnode!(model,𝕣[0,0,-10])
n2 = addnode!(model,𝕣[])
n3 = addnode!(model,𝕣[])
@testset "Nodes" begin
@test n1 == Muscade.NodID(1)
@test n2 == Muscade.NodID(2)
end
# the arguments to element constructors are irrelevant - elements are not tested here
sea(t,x) = SVector(1.,0.)
sky(t,x) = SVector(0.,1.)
e1 = addelement!(model,Turbine ,[n1,n2], seadrag=2., sea=sea, skydrag=3., sky=sky)
e2 = addelement!(model,AnchorLine,[n1,n3], Δxₘtop=SVector(0,2.,0), xₘbot=SVector(94.,0.), L=170., buoyancy=-1.)
@testset "Receipts" begin
@test n1 == NodID(1)
@test n2 == NodID(2)
@test e1 == EleID(1,1)
@test e2 == EleID(2,1)
end
@testset "model.nod" begin
@test model.nod[1].ID == NodID(1)
@test model.nod[1].coord ≈ [0.0, 0.0, -10.0]
@test model.nod[1].dofID == [DofID(:X,1), DofID(:X,2), DofID(:X,3)]
@test model.nod[1].eleID == [EleID(1,1), EleID(2,1)]
@test model.nod[2].ID == NodID(2)
@test model.nod[2].coord == 𝕣[]
@test model.nod[2].dofID == [DofID(:A,1), DofID(:A,2)]#[3,4]
@test model.nod[2].eleID == [EleID(1,1)]
@test model.nod[3].ID == NodID(3)
@test model.nod[3].coord == 𝕣[]
@test model.nod[3].dofID == [DofID(:A,3), DofID(:A,4)]
@test model.nod[3].eleID == [EleID(2,1)]
end
@testset "model.ele" begin
@test model.ele[1][1].ID == EleID(1,1)
@test model.ele[1][1].ieletyp == 1
@test model.ele[1][1].iele == 1
@test model.ele[1][1].nodID == [NodID(1),NodID(2)]
@test model.ele[1][1].dofID == [DofID(:X, 1), DofID(:X, 2), DofID(:A, 1), DofID(:A, 2)]
@test model.ele[2][1].ID == EleID(2,1)
@test model.ele[2][1].ieletyp == 2
@test model.ele[2][1].iele == 1
@test model.ele[2][1].nodID == [NodID(1),NodID(3)]
@test model.ele[2][1].dofID == [DofID(:X, 1), DofID(:X, 2), DofID(:X, 3), DofID(:A, 3), DofID(:A, 4)]
end
@testset "model.dof" begin
@test model.dof[DofID(:X,1)].ID == DofID(:X,1)
@test model.dof[DofID(:X,1)].nodID == NodID(1)
@test model.dof[DofID(:X,1)].idoftyp == 1
@test model.dof[DofID(:X,1)].eleID == [EleID(1,1),EleID(2,1)]
@test model.dof[DofID(:A,1)].ID == DofID(:A,1)
@test model.dof[DofID(:A,1)].nodID == NodID(2)
@test model.dof[DofID(:A,1)].idoftyp == 3
@test model.dof[DofID(:A,1)].eleID == [EleID(1,1)]
@test model.dof[DofID(:X,3)].ID == DofID(:X,3)
@test model.dof[DofID(:X,3)].nodID == NodID(1)
@test model.dof[DofID(:X,3)].idoftyp == 5
@test model.dof[DofID(:X,3)].eleID == [EleID(2,1)]
@test model.dof[DofID(:A,3)].ID == DofID(:A,3)
@test model.dof[DofID(:A,3)].nodID == NodID(3)
@test model.dof[DofID(:A,3)].idoftyp == 6
@test model.dof[DofID(:A,3)].eleID == [EleID(2,1)]
end
setscale!(model;scale=(X=(tx1=1.,tx2=1.,rx3=2.),A=(Δseadrag=3.,Δskydrag=4.,ΔL=5)),Λscale=2) # scale = (X=(tx=10,rx=1),A=(drag=3.))
@testset "model.doftyp" begin
@test model.doftyp[1].class == :X
@test model.doftyp[1].field == :tx1
@test model.doftyp[1].scale ≈ 1.
@test model.doftyp[1].dofID == [DofID(:X, 1)]
@test model.doftyp[3].class == :A
@test model.doftyp[3].field == :Δseadrag
@test model.doftyp[3].scale ≈ 3.
@test model.doftyp[3].dofID == [DofID(:A, 1)]
@test model.doftyp[5].class == :X
@test model.doftyp[5].field == :rx3
@test model.doftyp[5].scale ≈ 2.
@test model.doftyp[5].dofID == [DofID(:X, 3)]
@test model.scaleΛ ≈ 2.
end
@testset "model inspection" begin
@test model.eleobj[e1] == Turbine{typeof(sea), typeof(sky)}([0.0, 0.0], -10.0, 2.0, sea, 3.0, sky)
@test model.nod[n1].coord ≈ [0.0, 0.0, -10.0]
getndof(model,:X) == 3
getndof(model,(:X,:A)) == (3,2)
Muscade.getnele(model) == 2
Muscade.getnele(model,1) == 1
end
end # module
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 2066 | module TestScale
using Test,StaticArrays,SparseArrays
using Muscade
include("SomeElements.jl")
model1 = Model(:TestModel)
n1 = addnode!(model1,𝕣[ 0, 0]) # moving node
n2 = addnode!(model1,𝕣[10, 0]) # anchor 1
n3 = addnode!(model1,𝕣[ 0,10]) # anchor 2
n4 = addnode!(model1,𝕣[ ]) # A-nod for springs
e1 = addelement!(model1,Spring{2},[n1,n2,n4], EI=1)
e2 = addelement!(model1,Spring{2},[n1,n3,n4], EI=1)
@once f1(t) = t
e3 = addelement!(model1,DofLoad ,[n1], field=:tx1 ,value=f1)
e4 = addelement!(model1,Hold ,[n2], field=:tx1)
e5 = addelement!(model1,Hold ,[n2], field=:tx2)
e6 = addelement!(model1,Hold ,[n3], field=:tx1)
e7 = addelement!(model1,Hold ,[n3], field=:tx2)
@once f2(x,t) = 1x^2
@once f3(a) = 0.1a^2
e8 = addelement!(model1,SingleDofCost ,class=:X, field=:tx1,[n1] ,cost=f2)
e9 = addelement!(model1,SingleDofCost ,class=:X, field=:tx2,[n1] ,cost=f2)
e10 = addelement!(model1,SingleDofCost ,class=:A, field=:ΞL₀,[n4] ,cost=f3)
e11 = addelement!(model1,SingleDofCost ,class=:A, field=:ΞEI,[n4] ,cost=f3)
model2 = deepcopy(model1)
initialstate1 = initialize!(model1)
stateX1 = solve(StaticX; initialstate=initialstate1,time=[.5,1.],verbose=false)
stateXUA1 = solve(StaticXUA;initialstate=stateX1,maxYiter= 50,verbose=false)
setscale!(model2;scale=(X=(tx1=1.,tx2=10.,rx3=2.),A=(Δseadrag=3.,Δskydrag=4.,ΔL=5)),Λscale=2)
initialstate2 = initialize!(model2)
stateX2 = solve(StaticX; initialstate=initialstate2,time=[.5,1.],verbose=false)
stateXUA2 = solve(StaticXUA;initialstate=stateX2,maxYiter= 50,verbose=false)
step = 1
@testset "ScaleStaticX" begin
@test stateX1[step].X[1] ≈ stateX2[step].X[1]
end
@testset "ScaleStaticXUA" begin
@test stateXUA1[step].X[1] ≈ stateXUA2[step].X[1]
@test stateXUA1[step].A ≈ stateXUA2[step].A
end
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 4277 | module TestStaticX
using Test,StaticArrays,SparseArrays
using Muscade
using Muscade: DofID,EleID,NodID
include("SomeElements.jl")
model = Model(:TestModel)
n1 = addnode!(model,𝕣[0,0,+100]) # turbine
n2 = addnode!(model,𝕣[]) # Anod for turbine
n3 = addnode!(model,𝕣[]) # Anod for anchor
@once sea(t,x) = SVector(1.,0.)*t
@once sky(t,x) = SVector(0.,10.)
α(i) = SVector(cos(i*2π/3),sin(i*2π/3))
e1 = addelement!(model,Turbine ,[n1,n2], seadrag=1e6, sea=sea, skydrag=1e5, sky=sky)
e2 = [addelement!(model,AnchorLine,[n1,n3], Δxₘtop=vcat(5*α(i),[0.]), xₘbot=250*α(i), L=290., buoyancy=-5e3) for i∈0:2]
initialstate = initialize!(model)
state = solve(StaticX;initialstate,time=[0.,1.],verbose=false)
step = 1
@testset "StaticX" begin
@test state[step].Λ ≈ [0.0, 0.0, 0.0]
@test state[step].X[1] ≈ [-5.332268523655259, 21.09778288272267, 0.011304253608808651]
@test state[step].U[1] ≈ Float64[]
@test state[step].A ≈ [0.0, 0.0, 0.0, 0.0]
@test state[step].time ≈ 0.
@test model.locked == true
end
dis = initialstate.dis #Muscade.Disassembler(model)
dofgr = Muscade.allXdofs(model,dis)
s = deepcopy(state[step])
Muscade.decrement!(s,0,[1.,1.,-1.],dofgr)
#s[dofgr] = [1.,1.,1.]
# @testset "AllXdofs construction" begin
# # @test dofgr.scale ≈ [1., 1., 1.]
# # @test state[step][dofgr] ≈ [-5.332268523655259, 21.09778288272267, 0.011304253608808651]
# # @test s[dofgr] ≈ [-6.332268523655259, 20.09778288272267, 1.011304253608808651]
# end
#using GLMakie
#fig = Figure(resolution = (2000,1500))
#display(fig) # open interactive window (gets closed down by "save")
#axe = Axis3(fig[1,1],title="Muscade made this drawing",xlabel="X",ylabel="Y",zlabel="Z",aspect=:data,viewmode=:fit,perspectiveness=.5)
#draw(axe,state[step])
#save("C:\\Users\\philippem\\C home\\GIT\\Muscade.jl\\test\\first_light.jpg",fig)
include("GLMakieTester.jl")
axe = SpyAxe()
draw(axe,state[step],ieletyp=[1,2])
@testset "drawing" begin
@test axe.call[1].fun == :lines!
@test axe.call[1].args[1] ≈ [-5.332268523655259 -5.332268523655259; 21.09778288272267 21.09778288272267; 90.0 110.0]
@test axe.call[1].kwargs[:color] == :orange
@test axe.call[1].kwargs[:linewidth] == 5
@test axe.call[2].fun == :lines!
@test axe.call[2].args[1][1:5]≈[144.05988106384137, 129.6206341588945, 115.1813872539476, 100.74214034900072, 86.30289344405384]
@test axe.call[2].kwargs[:color] == :blue
@test axe.call[2].kwargs[:linewidth] == 2
end
out1,dofid1 = getdof(state[1],field=:tx1)
out2,dofid2 = getdof(state ,field=:tx1)
out3,dofid3 = getdof(state[1],class=:A,field=:ΔL)
out4,dofid4 = getdof(state[1],field=:tx1,nodID=[n1])
out5,dofid5 = getdof(state ,field=:tx1,nodID=[n1])
@testset "getdof" begin
@test out1 ≈ [-5.332268523655259;;]
@test dofid1 == DofID[DofID(:X, 1)]
@test out2 ≈ [-5.332268523655259;;;20.184170880401076]
@test dofid2 == DofID[DofID(:X, 1)]
@test out3 ≈ [0.0;;]
@test dofid3 == DofID[DofID(:A, 3)]
@test out4 ≈ [-5.332268523655259;;]
@test dofid4 == DofID[DofID(:X, 1)]
@test out5 ≈ [-5.332268523655259;;; 20.18417088040054]
@test dofid5 == DofID[DofID(:X, 1)]
end
req = @request cr,ltf
eleres = getresult(state,req,e2) # eleres[iele,istep].cr
cr = [e.cr for e∈eleres[:,1]]
ltf = [e.ltf for e∈eleres[:,1]]
@testset "getdof2" begin
@test cr ≈ [118.69592125130082, 23.961941539907585, 235.51441727552435]
@test ltf ≈ [183.68229160771097, 121.62396272109176, 238.96209627282917]
end
eleres = getresult(state[1],req,e2) # eleres[iele].cr
cr = [e.cr for e∈eleres]
ltf = [e.ltf for e∈eleres]
@testset "getdof3" begin
@test cr ≈ [118.69592125130082, 23.961941539907585, 235.51441727552435]
@test ltf ≈ [183.68229160771097, 121.62396272109176, 238.96209627282917]
end
eleres = getresult(state[1],req,AnchorLine) # eleres[iele].cr
cr = [e.cr for e∈eleres]
ltf = [e.ltf for e∈eleres]
@testset "getdof4" begin
@test cr ≈ [118.69592125130082, 23.961941539907585, 235.51441727552435]
@test ltf ≈ [183.68229160771097, 121.62396272109176, 238.96209627282917]
end
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 2098 | module TestStaticXUA
using Test
using Muscade
include("SomeElements.jl")
model = Model(:TestModel)
n1 = addnode!(model,𝕣[ 0, 0]) # moving node
n2 = addnode!(model,𝕣[10, 0]) # anchor 1
n3 = addnode!(model,𝕣[ 0,10]) # anchor 2
n4 = addnode!(model,𝕣[ ]) # A-nod for springs
e1 = addelement!(model,Spring{2},[n1,n2,n4], EI=1)
e2 = addelement!(model,Spring{2},[n1,n3,n4], EI=1)
@once f1(t) = t
e3 = addelement!(model,DofLoad ,[n1], field=:tx1 ,value=f1)
e4 = addelement!(model,Hold ,[n2], field=:tx1)
e5 = addelement!(model,Hold ,[n2], field=:tx2)
e6 = addelement!(model,Hold ,[n3], field=:tx1)
e7 = addelement!(model,Hold ,[n3], field=:tx2)
@once f2(x,t) = 1x^2
@once f3(a) = 0.1a^2
e8 = addelement!(model,SingleDofCost ,class=:X, field=:tx1,[n1] ,cost=f2)
e9 = addelement!(model,SingleDofCost ,class=:X, field=:tx2,[n1] ,cost=f2)
e10 = addelement!(model,SingleDofCost ,class=:A, field=:ΞL₀,[n4] ,cost=f3)
e11 = addelement!(model,SingleDofCost ,class=:A, field=:ΞEI,[n4] ,cost=f3)
initialstate = initialize!(model)
@testset "StaticX" begin
stateX = solve(StaticX;initialstate,time=[0.,1.],verbose=false)
@test stateX[2].X[1] ≈ [1.0008305423582147, 0.056562064402881376, 0.0, 0.0, 0.0, 0.0,
-1.0006330261310146, 0.006289232571302629,
0.0006330261310144907, -0.006289232571302629]
end
stateX = solve(StaticX; initialstate,time=[.5,1.],verbose=false)
stateXUA = solve(StaticXUA;initialstate=stateX,maxYiter= 50,verbose=false)
@testset "StaticXUA" begin
@test stateXUA[2].X[1] ≈ [ 0.16947517267111387, -0.09872147216175686, 0.0, 0.0, 0.0, 0.0, -0.9998314994105624, -0.01004064780561606, -0.00016850058943765545, 0.01004064780561606]
@test stateXUA[2].A ≈ [0.004212461115295247, 0.5743380076037062]
@test stateXUA[2].A == stateXUA[1].A
end
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 616 | module TestUnit
include("../src/Unit.jl")
using Test
imp = pound/foot^3
dou = 2m
@testset "Unit" begin
@test ( 4. ← pound/foot^3 )≈ 64.07385349584058
@test ( 4. ← imp )≈ 64.07385349584058
@test ( 2^2←imp )≈ 64.07385349584058
@test ( 2+2←imp )≈ 64.07385349584058
@test ( [1,2,3]←dou )≈ [2.,4.,6.]
@test ( (1,2,3)←dou )== (2.,4.,6.)
@test ( ([1,2],3)←dou )== ([2.,4.],6.)
@test ( (1:3)←dou) ≈ [2.,4.,6.]
@test ( [[1,2],3]←dou)≈ [[2.,4.],6.]
@test ( (2←imp)→pound/foot^3 )≈ 2.
@test (2+2→imp )≈ (4→imp)
@test string(imp )== "16.018463373960145 m^-3.0*kg"
end
end
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | code | 1124 | using Muscade
module Runtest
using Test,Documenter,Muscade
@testset "Muscade.jl package" begin
@testset "TestEspy" begin
include("TestEspy.jl")
end
@testset "TestElementAPI" begin
include("TestElementAPI.jl")
end
@testset "TestAdiff" begin
include("TestAdiff.jl")
end
@testset "TestModelDescription" begin
include("TestModelDescription.jl")
end
@testset "TestAssemble" begin
include("TestAssemble.jl")
end
@testset "TestStaticX" begin
include("TestStaticX.jl")
end
@testset "TestStaticXUA" begin
include("TestStaticXUA.jl")
end
@testset "TestScale" begin
include("TestScale.jl")
end
@testset "TestDofConstraints" begin
include("TestDofConstraints.jl")
end
@testset "ElementCost" begin
include("TestElementCost.jl")
end
@testset "TestUnit" begin
include("TestUnit.jl")
end
doctest(Muscade)
end
end | Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | docs | 2384 | # Muscade.jl
**Create and solve optimization-FEM models.**
## Purpose
Optimization-FEM consists in optimising a target function (here called the cost function) under the constraint that a FEM model be exactly verified. This in turn implies that the problem has more unknowns than the model has equation (or at least: more unknowns than linearly independent equations, as would be the case with “insufficient” boundary conditions).
The unknowns that are dual to the FEM equations are noted X-dofs (the *response* of the model). The rest of the unknowns can be separated into U-dofs (varying with time, generaly *unknown loads*) and A-dofs (constant over time, generaly *unknown model parameters*). The conditions for such a constrained optimization problem to be well-posed are the Babushka-Brezzi conditions, which say, in essence “if you do not restrain, then at least measure”.
## Applications of optimization-FEM
Besides solving well-posed FEM problems (obtaining the response of a system, given adequate boundary conditions and known loading terms), many applications can be, or should be, possible within `Muscade`.
**Reliability analysis**: Finding a design point. What is the most probable combination of external loads `U` and strength of the structure `A` that may cause the response `X` to exceed, in one of many ways, the acceptable?
**Design optimization**: What is the cheapest way to engineer a system (for example a structure) that will survive a set of loading conditions?
**Load identification and monitoring**: Given incomplete and noisy measurements of the response of a system, what are the loads that are most likely to cause a response close to what has been measured?
**Optimal control**: how to steer a system with many dofs into a wanted behaviour?
**Model identification**: given enough measurements on the response of a system responding to at least partly unknown load, is it possible to adjust the model of the system (model calibration, damage detection)?
**Sensor array optimization**: how best to place sensors in a system in order to support the above applications?
## Documentation
See the [documentation](https://sintef.github.io/Muscade.jl/stable/index.html).
## Installing Muscade
In the REPL, type `]` (to go into package management mode), followed by
- `add Muscade`.
- Press the `backspace` key to leave the package manager. | Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | docs | 33 | # Automatic differentiation
TODO | Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | docs | 1679 | # Built-in elements
`Muscade` does not physical elements, implementing the solution of some differential equation: this is left to the apps built on `Muscade`. Rather, it provide provides some general purpose elements which are practical in some setting in order to introduce loads, costs or constraints.
[`DofLoad`](@ref) adds a time-varying load on a single X-dof. Elements for more general loads, in particular, consistent loads on element boundaries or domain, or follower loads, need to be provided by apps if required.
[`DofCost`](@ref) adds a cost as a function of either X-dofs ,U-dofs (and/or their derivatives), A-dofs and time, or as a function of A-dofs alone. Elements for costs on unknwn distributed load *fields* (over boundary or domain) must be provided by apps if required.
[`SingleDofCost`](@ref) provides a simplified syntax for costs on a single dof.
[`ElementCost`](@ref) adds a cost on a combination of one element's dofs and element-results.
[`DofConstraint`](@ref) adds a constraint to a combination of *values* (no time derivatives) of dofs. The constraints can switch over time between equality, inequality and "off". Inequality constraints are handled using an interior point method without feasibility step.
[`ElementConstraint`](@ref) adds a constraint to a function of internal results from one element. The constraints can switch over time between equality, inequality and "off". Inequality constraints are handled using an interior point method without feasibility step.
[`Hold`](@ref) provides a simplified syntax to set a single X-dof to zero.
[`QuickFix`](@ref) allows to rapidly create a simple element. Apps should not use this.
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | docs | 1695 | # Built-in solvers
## `StaticX` solver
!!! warning
At this time, the handling of element-memory (see [Creating an element](@ref)) is not implemented.
`StaticX` is a non-linear, static, explicit solver for "normal" FEM (not optimisation-FEM): At a succession of times ``t``, it will solve ``R(X(t),t)=0`` (see [Theory](@ref)).
`StaticX` can be applied to models that have U and A-dofs. This is handled as follows: One input to `StaticX` is a `State`, which can come from [`initialize!`](@ref) or from the output of another solver. `StaticX` will keep the U- and A-dofs to the value in the input `State`. `initialize!` sets all dofs to zero, so when `StaticX` is given a `State` produced by `initialize!` the analysis starts with X-dofs equal to zero, and U- and A-dofs are kept zero throughout the analysis.
`StaticX` handles inequality constraints using a simplified interior point method, without a feasibility step. This works (reasonnably) well in concert with the built-in [`DofConstraint`](@ref) element.
See [`StaticX`](@ref).
## `StaticXUA` solver
!!! warning
At this time, the handling of element-memory (see [Creating an element](@ref)) is not implemented.
`StaticXUA` is a non-linear, static, optimisation-FEM solver, with load and model parameter identification. Given a vector of static equilibrium configurations, obtained for example using `StaticX`, on a model with costs (and possibly constraints) on U- and A-dofs, the solver will determine response (X-dofs) and unknown loads for each step (Udofs) as well as model parameters for the whole history (Adofs).
The solvers handles inequality constraints in the same way as `StaticX`.
See [`StaticXUA`](@ref). | Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | docs | 10033 | # Creating an element
## Introducton
New element types, providing new element formulations or adressing new domains in physics are implemented by implementing a datatype and methods for function defined by `Muscade`:
- A **constructor** which is called when the user adds an element to the model.
- **`Muscade.doflist`** specifies the degrees of freedom (dofs) of the element.
- **`Muscade.residual`** takes element dofs as input and returns the element's additive contribution to a non-linear system of equations to be solved (a residual aka. a right hand side),
- **`Muscade.lagrangian`** takes element dofs as input and returns the element's additive contribution to a function to be extremized (Lagrangian aka. cost function, surprisal...)
Each element must implement a constructor following a format specified by `Muscade`, as well as `Muscade.doflist`. Each element must also implement *either* `Muscade.lagrangian` *or* `Muscade.residual`, depending on what is more natural.
## DataType
For a new element type `MyELement`, the datatype is defined as
```julia
struct MyElement <: AbstractElement
...
end
```
`MyElement` *must* be a subtype of `AbstractElement`.
## Constructor
The element must provide a constructor of the form
```julia
function MyElement(nod::Vector{Node};kwargs...)
...
return eleobj
end
```
which will then call the default constructor provided by Julia.
`nod` can be used to access the coordinates of the nodes:
```julia
x = nod[inod].coord[icoord]
```
where `inod` is the element-node number and `icoord` the index into a vector of coordinates. `coord` is
provided by the user when adding a `Node` to the `Model`. `Muscade` has no opinion about, and provides no
check of, the length of `coord` provided by the user. In this way elements can define what coordinate system
(how many coordinates, and their interpretation) is to be used. Coordinate systems can even differ from one
node to the next. See also [`coord`](@ref).
`kwargs...` is any number of named arguments, typicaly defining the material properties of the element.
The user does not call the above-defined element directly. Instead, an element is added to the model by
a call of the form
```julia
e1 = addelement!(model,MyElement,nodid,kwargs...)
```
See [`addelement!`](@ref).
## `Muscade.doflist`
The element must provide a method of the form
```julia
function Muscade.doflist(::Type{MyElement})
return (inod =(...),
class=(...),
field=(...))
end
```
The syntax `::Type{MyElement}` is because `doflist` will be called by `Muscade` with a `DataType` (the type `MyElement`). The function name must begin
with `Muscade.` to make it possible to overload a function defined in the module `Muscade`.
The return value of the function is a `NamedTuple` with the fields `inod`, `class` and `field`.
- `inod` is a `NTuple` of `Int64`: for each dof, its element-node number.
- `class` is a `NTuple` of `Symbol`: for each dof, its class (must be `:X`, `:U` or `:A`).
- `field` is a `NTuple` of `Symbol`: for each dof, its field.
Importantly, `doflist` does not mention dofs of class `:Λ`: if the element implements `lagrangian`, there is automaticaly a one-to-one correspondance between Λ-dofs and X-dofs.
For example (using Julia's syntax for one-liner functions):
```julia
Muscade.doflist( ::Type{Turbine}) = (inod =(1 ,1 ,2 ,2 ),
class=(:X ,:X ,:A ,:A ),
field=(:tx1,:tx2,:Δseadrag,:Δskydrag))
```
See [`doflist`](@ref).
## `Muscade.lagrangian`
Elements that implement a cost on the degrees of freedom must implement a method of the form
See [`lagrangian`](@ref) for the list of arguments and outputs.
### Automatic differentiation
The gradients and Hessians of `L` do not need to be implemented, because `Muscade` uses automatic
differentiation. Because of this, it is important not to over-specify the inputs. For example,
implementing a function header with
```julia
@espy function Muscade.lagrangian(o::MyElement,Λ::Vector{Float64},X,U,A,t,χ,χcv,SP,dbg)
# ____bad_idea____
```
would cause a `MethodError`, because `Muscade` will attempt to call with a `SVector` instead of `Vector`, and a special
datatype supporting automatic diffeentiation instead of `Float64`.
### Extraction of element-results
The function definition must be anotated with the macro call `@espy`. Variables within the body of `lagrange`, which
the user may want to obtain must be anotated with `☼` (by typing `\sun` they pressing `TAB`) at the place where they
are calculated. An example would be
```julia
☼σ = E*ε
```
The macro will generate two versions of `lagrange`. One in which the anotations `☼` are taken away, which is used to
solve the numerical problem. Another with additional input and output variables, and code inserted into the body
of the function to extract results wanted by the user.
See [`@espy`](@ref) for a complete guide on code anotations.
## `Muscade.residual`
Elements that implement "physics" will typicaly implement `residual` (they could implement the same using `lagrangian`, but the resulting code would be less performant).
The interface is mostly the same as for `lagrangian` with the differences that
- `residual` returns a vector `R`
- there is no argument `Λ`
```julia
@espy function Muscade.residual(o::MyElement,X,U,A,t,χ,χcv,SP,dbg)
...
return R,noχ,noFB
end
```
### Immutables and Gauss quadrature
`residual` is called many times and it is critical to obtain high performance. Thus, allocating the vector `R` on the heap
within the function must be avoided. A design option would be to have `Muscade` pass a preallocated vector `R` and have
residual mutate its argument. For "forward" automatic differentiation, it can be difficult to predict the element type of `R`,
and other techniques of automatic differentiaton do not accomodate mutations.
For this reason, `residual` and `lagrange` must be writen in a functional style, using only immutable variables, and in particular
immutable arrays. This can be done using `StaticArrays.jl` (tested) or `Tensorial.jl` (not tested with `Muscade`), and generaly
results in very readable code that directly expresses concepts of linear algebra.
One difficulty arises with Gauss quadrature. Typical implementations would rely on setting `R` to zero, then adding the
contributions from quadrature points to `R` within a `for` loop over the Gauss points. The pseudo code (not valid in `Muscade`):
```julia
R .= 0
for igp = 1:ngp
F = ...
Σ = ...
R += F ∘ Σ ∘ ∇N * dV
end
```
shows that `R` is mutated. A pseudocode in immutable style would be
```julia
@espy function residual(x,χ)
t = ntuple(ngp) do igp
☼F = ...
☼Σ = ...
r = F ∘ Σ ∘ ∇N * dV
@named(χ,r)
end
χ = ntuple(igp->t[igp].χ,ngp)
R = sum( igp->t[igp].r,ngp)
return R,χ
end
```
`r` are the contributions to `R` at each quadrature point. The operation `t = ntuple ...` returns a datastructure `t` such that `t[igp].χ` are the memory
variable and ``t[igp].r` the contribution to the residual from the `igp`-th quadrature point. This is because
```julia
t = ntuple(ngp) do igp
expr(igp)
end
```
is equivalent to
```julia
t = ntuple(expr for igp=1:ngp)
```
which returns
```julia
t = (expr(1),expr(2),...,expr(ngp))
```
where the value of `expr` is that of its last line `@named(χ,r)` which is a macro provided by `Muscade` that inserts the code `(χ=χ,r=r)`.
The code
```julia
χ = ntuple(igp->t[igp].χ,ngp)
R = sum( igp->t[igp].r,ngp)
```
gathers the memories of all quadrature points into a `Tuple` and adds together the contributions `r` into the residual `R`.
See [`residual`](@ref).
## Help functions
`Muscade` provides functions and constants to make it easier to comply with the API:
- Element constructors can use function [`coord`](@ref) to extract the coordinates fron the `Vector{Node}` they get as first argument.
- `residual` and `lagrangian` **must** use [`∂0`](@ref), [`∂1`](@ref) and [`∂2`](@ref) when extracting the zeroth, first and second time derivatives from arguments `X` and `U`.
- Constants [`noχ`](@ref) and [`noFB`](@ref) (which have value `nothing`) can be used by elements that do not have memory or no feedback to the solving procedure.
## Performance
For a given element formulation, the performance of `residual` and `lagrangian` can vary with a factor up to 100 between a good and a bad implementation.
**Type stable code** allows the compiler to know the type of every variable in a function given the type of its parameters. Code that is type unstable is significantly slower. See the page on [type stability](TypeStable.md).
**Allocation**, and the corresponding deallocation of memory *on the heap* takes time. By contrast, allocation and deallocation *on the stack* is fast. In Julia, only immutable variables can be allocated on the stack. See the page on [memory management](Memory.md)
**Automatic differentiation** generaly does not affect how `residual` and `lagrangian` are writen. There are two performance-related exceptions to this:
1. If a complex sub function in `residual` and `lagrangian` (typicaly a material model or other closure) operates on an array (for example, the strain) that is smaller than the number of degrees of freedom of the system, computing time can be saved by computing the derivative of the output (in the example, the stress) with respect to the input to the subfunction, and then compose the derivatives.
2. Iterative precedures are sometimes used within `residual` and `lagrangian`, a typical example being in plastic material formulations. There is no need to propagate automatic differentiation through all the iterations - doing so with the result of the iteration provides the same result.
See the page on [automatic differentiation](Adiff.md)
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | docs | 11524 | # Memory management
## Introduction
Mutable structs, arguments passes by value to functions, garbage collection, variable names as tags. A variety of concept in Julia become easier to understand by considering how Julia manages memory, and how this affect performance.
In line with programming languages of the past decades, Julia uses two approaches to store variables, namely the *heap*, and the *stack*. Julia's designer made a few important choices on how they use heap and stack, and these strongly shape how we use the language, in particular when writing high-performance code.
## The heap
The heap is a swath of memory made available to a program. When a variable is *allocated*, a preferably contiguous amount of available memory of the right size is found on the heap, and its adress (a pointer) is returned. Values within that segment of memory can be written to or read, and the variable is *deallocated* when it is no longer in use.
This is very flexible, but this flexibilty comes at a cost: To allocate a variable one must find available memory, thus there must be a heap-ledger describing what memory is available. Browsing the heap-ledger takes time.
Early in the execution of a program, the heap is unused, and finding space for new variables is easy. But as variables are allocated and deallocated in arbitrary order, large contiguous area of free memory become rarer: the heap is fragmented, and there might be a need to swap things around (defragmentation).
Further, language designers must make a choice. Option one is to let the programmer explicitely deallocate a variable when it is no longer needed. Unfortunately, bugs in which a variable is not deallocate easily occur. If this happens in some loop, memory is allocated but not deallocated (a memory leak) and these bugs are nasty to track down.
The safer option 2, adopted by Julia, is to automaticaly deallocate memory on the heap when nothing anymore points to it. That implies that there must be a pointer-ledger of all pointers into the heap, an the language must periodicaly go through the pointer-ledger to find orphaned heap-memory, update the heap-ledger, and possibly defragment the heap (move the variables in order to create large contiguous unallocated memory). This process is called garbage collection. While invisible to the programmer, the user sees it: it takes time.
When a profiler reports the number of allocations, it actualy refers to allocation on the heap, not counting variables created on the stack.
## The stack
The stack is a limited amount of memory managed on a "last in first out" basis: as a picture, the stack is vertical and when the stack start empty, the stack pointer points tot he base of the stack. If a new variable needs to be stored, it is added to the top of the stack and the pointer is updated. When the variable no longer needs to be stored, the pointer is just updated back to its previous value (pop the stack).
The mechanism for creating or destroying a variable is simple, and thus extremely fast. The drawback of course is that variables are destroyed in reverse order of their creation, which is not always convenient. However this works perfectly for:
1. input arguments to a function, in languages where these are passed by values, and
2. local variables for the function.
All these variables are created when entering the function, and destroyed when leaving it. The (memory) stack thus fills as the code goes deeper into the call stack. Recursions gone beserk typicaly result in a (memory) stack overflow.
That, at least, is how things looked like in the late pre-internet age. Since then, CPUs have been equipped with a nested system of fast access caches. An outer cache, smaller and fast than RAM, a inner cache, yet smaller and faster, and the CPU's registers are, in a sense, the innermost caches. It makes sense to store the data currently being processed into one of these inner caches. But this comes at the cost of complexity to the language and compiler designers: *moving* data to the cache means to free its original location (which is awkward in the context of a stack). *Copying* data introduces the classic problem of keeping all copies of the data up to date.
Thus the designers of the Julia language made one important choice: *once a variable on the stack has been assigned a value, that value can never be changed*. The variable is said to be *immutable*. This allows Julia copy any part of the stack to the CPU caches to optimize performance, without worrying about out-of-date copies. "Immutables are easier to reason about" says the doc: this may not be true when learning Julia, but applies when writing the compiler.
Now we see why variable names in Julia are best viualised as tags on a value (you copy the tag if you copy the value, and there can be multiple tags for the same value) as opposed to the parable of a variable as a box in which you can store different value (valid in other languages).
Julia's designers made another important choice: the size of all variables stored the stack must be known at compile time. This drasticaly simplifies the process of creating or destroying space on the stack when entering a function: just increment or decrement to stack pointer by a value determined for the function (actualy the method instance) at compile time. Accessing a local variable from inside the function is likewise very fast: add a compile-time constant to the stack pointer, and that's were your data is.
As we will see, the fact that variables must be
1. Immutable
2. Of size known at compile time
significantly affects how one programs "on the stack" in Julia.
## An `Array`
In Julia, an `Array{2,Float64}` is "copied", for example by passing it as an argument to a function. What happens?
The array comes in several parts
1. Heap-memory enough to store all the values in the array, in column major order.
2. A smaller amount of heap-memory containing.
a. the sizes of the first and second indices of the array.
b. a pointer to the storage 1. of the values.
3. On the stack, a pointer to 2.
4. Machine code that is written under the assumption that this array has two indices, that the size of an array element is 64 bits, that array elements can be copied directly to the CPU register for algebraic operations, etc.
When the array is passed to a function, only 3., the pointer is actually passed to the function, as a copy placed at the right spot on the stack. Being on the stack, the pointer is immutable: the function cannot reallocate 2. (as then the pointer to 2. would change value, pointing to a new spot on th heap). But one can change the content of the array, and its sizes (`push!`). Upon return from the function, the caller still has the pointer to a place on same spot on the heap: any change made by the function to values inside the array is visible by the caller.
If function overwrites the array as a whole however, the interpretation is different
```julia
function foo(a)
a = [0,0]
end
A = [1,2]
foo(A)
@show A
2-element Vector{Int64}:
1
2
```
Here `foo` was given a pointer to an array. By writing `a =`, `foo` discards its knowledge of the pointer, and uses the same tag-name to refer to a new spot of memory on the heap containing a pair of zeros.
## Strategies for performance
Allocating and deallocating memory on the heap takes time. The amount of time for each allocation is tiny, but an ocean is just many drops.
The time required is not proportional to the amount of memory allocated, but to the amount of individual allocations made. So let us consider as an example a function inside of a hot loop, that needs internal memory for its local computations, and to return the results it produces. For the function to be fast it must not allocate/deallocate memory on the heap. Two different strategies can be used to this effect, which we could call "procedural" and "functional".
## Procedural strategy
In the procedural strategy, memory (say `Array`s) is preallocated "once and for all" on the heap, and passed to the function: arrays or slices of arrays are passed to the function. The function uses this as work arrays and/or to return outputs: it is said to work "in place". In that way there is one (or several) allocation[s] before the hot loop, and many calls to the function. In Julia, the naming convention for functions that thus modify their input arguments is to end the function name with `!`.
```julia
function double!(a)
for i∈eachindex(a)
a[i] *= 2
end
end
a = randn(10)
for i = 1:10 # "hot" loop
double!(a)
end
```
This strategy is sometimes difficult to implement:
1. If the function requires some working space, passing arrays to it is a breach of separation of concern (a new algorithm might require less, or more memory).
2. The return type of some functions might be hard to predict: even for type-stable functions, evaluating the types of an algorithm's output given the types of its inputs is sometimes best left to the compiler.
3. Algebraic operations, for example, are built into larger expression. In an implementation of `*`, to be used in `a*b+c`, operating in place is not an option (see however syntaxes like `d .= a.*b.+c` that *are* operating in place).
## Functional strategy
In the functional strategy, the function creates new variables, both for intermediate results and for return value[s]. For performance, these variables are created on the stack, and must thus be immutable and of size known at compile time.
```julia
function double(a)
b = 2 .*a
return b
end
using StaticArrays
a = SVector{10}(randn() for i=1:10)
for i = 1:10 # "hot" loop
a = double(a)
end
```
Note that `double` is called with a `SVector`. `SVector`s' size is a parameter to the type, and is thus known at compile time. `SVector`s' are immutable (`a[2] = 0` will throw an error), and thus `SVector`s live on the stack. If `double` was called with a `Vector` (which size is part of the value, and which is mutable), the operation `b = 2 .*a` would result in an allocation.
This strategy may also be difficult to implement:
1. If many array sizes may be used (leading to the compilation of many method instances).
2. If array sizes depend on input *values*, using types in which size is part of the type leads to type-unstable code.
3. If large arrays are used, compile times will become excessively high.
## Working with immutables
`SArray`s, `ntuple`s and `NamedTuple`s are useful immutable datastructures.
If a for example an `SArray` can not be modified after it is created, how can we fill it with values? Julia provides two syntaxs to do this, comprehensions and `do`-loops. A comprehension looks like this:
```julia
using StaticArrays
const N=3
f(i) = i^2
a = SVector{N}(f(i) for i=1:N)
```
Importantly, `N` must be a compile-time constant.
A `do`-loop allows more complicated operations. We assume that the function `material` returns a `StaticArray` `σ` and a variable `χgp` of type difficult to predict. The `do`-loop creates the `ntuple` `accum`, which is then unpacked (using comprehensions) to sum the `σ` values into `r` and stack the `χgp` values into `χ`.
```julia
function residual(x,y)
ngp = 4
accum = ntuple(ngp) do igp
z = x[igp] + y[igp]
σ, χgp = material(z)
(σ=σ, χ=χgp)
end # do igp
r = sum( accum[igp].σ for igp=1:ngp)
χ = NTuple{ngp}(accum[igp].χ for igp=1:ngp)
return r,χ
end
```
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | docs | 3829 | # Creating a model
## Script as input
`Muscade` being a framework for the development of optimization-FEM applications, it only provides a limited number of generic modeling capabilities, like fixing degrees of freedom (dofs) to describe boundary conditions, introducing holonomic constraints or costs on either dofs, or element-results (see [Built-in elements](@ref)). `Muscade` does not provide the elements needed to treat any specific application. Hence to create a model, one will typicaly be `using` both `Muscade` and another package that provide a `Muscade`-based application (app). The app provides specific elements for domains like continuum mechanics, marine structures, hydrogen diffusion etc.
Input to such an app is provided in the form of a Julia script containing instructions (calls to `Muscade`, using elements and possibly solvers provided by the app) to define the model, execute analyses, and extract and process analysis results. This has two advantages:
1. Scripting a series of analyses, or some specific pre or postprocessing is simply done in the same script.
2. App developpers do not need to write code pertaining to a user interface.
That said, an app could introduce a GUI that would itself do the calls to `Muscade`.
Here is a simple example of analysis:
```julia
using Muscade
using StaticArrays
model = Model(:TestModel)
n1 = addnode!(model,[0.])
n2 = addnode!(model,[1.])
e1 = addelement!(model,Hold,[n1];field=:tx1)
e2 = addelement!(model,DofLoad,[n2];field=:tx1,value=t->3t)
e3 = addelement!(model,QuickFix,[n1,n2];inod=(1,2),field=(:tx1,:tx1),
res=(X,X′,X″,t)->12SVector(X[1]-X[2],X[2]-X[1]))
initialstate = initialize!(model)
state = solve(StaticX;initialstate,time=[0.,1.],verbose=false)
tx1,_ = getdof(state[2],field=:tx1,nodID=[n2])
req = @request F
eleres = getresult(state,req,[e2])
iele,istep = 1,2
force = eleres[iele,istep].F
```
## Model definition
The definition of a model is done in three phases:
1. Creating a blank model.
2. Adding nodes.
3. Adding elements.
One can actually add nodes to the model after elements have been added. One can however only add an element to the model *after* all the nodes of the element have been added to the model.
`Muscade` does not provide a mesher. There are some general purposes meshers with Julia API that could be used.
## Running the analysis
`initialize!` is used to create an as-meshed state of the system. Typicaly (but this depends on the app), all dofs are set to zero. The resulting variable, here called `initialstate` contains a pointer to the model. Once a model is thus initialized, one can no longer add nodes or elements to it. This is to ensure that a model can not be modified during a sequence of analyses.
`solve` is then called with the name of the solver to be used (here `StaticX`), and any named parameters required by the solver. The return value `state` is *for this solver* a vector (over the time steps) of `State`s.
## Extracting results
`State`s (returned by `initialize!` and `solve`). are variables which contents are private (not part of the API, and subject to change), but can be accessed using functions like `getdof` and `getresult`.
`getdof` allows to obtain dofs which are directly stored in `state`, by specifying class, field and node.
`getresult` (used in combination with `@request`) allows to obtain element-results which have been marked as requestable inside the function `lagrangian` or `residual` of an element. These element-results are not stored in the `State`: `getresult` will call a modified version of `lagrangian` or `residual` to obtain the `@request`ed results.
TODO Plotting results
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | docs | 26 | # Creating a solver
TO DO | Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | docs | 6785 | # Theory
## Classes of degrees of freedom
`Muscade` introduces 3 *classes* of degrees of freedom (dofs).
**Xdofs** are the dofs normaly encountered in normal "forward" FEM analysis. They provide a discrete representation of the response of the system. There is a one-to-one relation (a "duality") between the Xdofs ``X`` and the *residuals* ``R``, which are the discretized form of he differential equations we seek to solve. In forward FEM, we seek to solve a problem of the form
```math
\forall t, R(X(t),t)=0
```
**Udofs** are additional dofs that can be used to represent additional loads on the system. Like Xdofs, Udofs are time-dependant. Unlike Xdofs, there str no residuald (no new equations) corresponding to them.
**Adofs** are additional dofs that can be used to parameterise the model. Adofs are *not* time-dependant,and there are no residuals corresponing to them.
Equilibrium now requires
```math
\forall t, R(X(t),U(t),A,t)=0
```
which is ill-posed (unknowns ``U`` and ``A`` have been added, but there are no new equations).
To simplify the presentations in the following, we drop time ``t`` from the notations. This can be interpreted in two ways:
1. We are looking at a problem at a single instant (static).
2. We are solving an evolution problem (dynamic), and ``X`` and ``U`` are now vectors-valued functions of time, and ``R``represents a vector of ordinary differential equations (over time).
## Target function
To make the problem well-posed again, we introduce a target function ``Q(X,U,A)``. We then seek to make ``Q`` extremal (finding a local minimum, maximum), under the constraint ``R(X,U,A)=0``. Depending on the relevant application, ``Q`` can represent different concepts.
In design optimisation, one can associate a monetary value to ``A`` (building stronger costs more money), and to ``X`` (some system responses, including failure, would cost money). The objective is to find the design with the lowest associated cost.
In an load estimation problem where part of the response is measured, we wish to find the most probable unknown load ``U`` (large loads are not likely) and response (a computed response ``X`` that drasticaly disagrees with the actual measurements is not likely) - under the constraint that load and response verify equilibrium. In this case, we minimize the *surprisal* ``s = -\log(P)`` instead of maximizing ``P``. Making probabilities or surprisal extremal is equivalent. However, since probabilities multiply (the joint probability density of independant variables is equal to the product of the marginal probability densities), the corresponding surprisals add, which fits into the system of having elements *add* contribution to the system of equations to be solved. Further, the surprisals are typicaly numericaly "kinder".
## Lagrangian
Making ``Q`` extremal under the constraint ``R(X,U,A)=0`` is equivalent to finding an extremal (a saddle point) of the *Lagrangian* ``L(\Lambda,X,U,A)`` (not to be confused with the potential in Lagrangian mechanics)
```math
L(\Lambda,X,U,A) = Q(X,U,A) + R(X,U,A) \cdot \Lambda
```
where ``Λ`` are Lagrange multipliers. There is again a one-to-one correspondance between Λdofs and residuals, and this between Λdofs and Xdofs. One result of this correspondance is that when implmenting a new element, the method `doflist` does not list the Λdofs (this would otherwise just have been a compulsory repetition of the list of Xdofs).
For evolution problems, the dot product ``R(X,U,A) \cdot \Lambda`` includes an integral over time: the Lagrangian is a *functional*, and the gradients of ``L`` are ordinary (or integral) differential equations (in time), found using Frechet derivatives.
Additional constraints may be introduced. *Physical constraints* (like contact or boundary conditions) are added by augmenting ``X`` and ``R``. *Optimisation constraints* may have to be verified at every step (stresses to remain below a critical level, at any time). They are handled by augmenting ``U`` (introducing a Udof Lagrange multiplier). Optimisation constraints that act only on Adofs (there is a limit to the strength of steel we can order) are handled by augmenting ``A``. With the additional constraints, the Lagrangian is of the form
```math
\begin{split}
L(\Lambda,X,U,A) = Q(X,U,A) &+ \left[\begin{array}{c}R(X,U,A) - ∇g_x(X,U,A) \cdot X_λ \\ g_x(X,U,A)\end{array}\right] \cdot Λ \\ &+ g_u(X,U,A) \cdot U_\lambda \\ &+ g_a(A) \cdot A_\lambda
\end{split}
```
## Elements
In the original finite element analysis, the *elements* in a model form a partition of a domain over which differential equations are to be solved. We will call these "physical elements". These elements provide additive contributions to the system of equations ``R(X,U,A)=0``. Further contributions can come from external loads.
When doing optimization-FEM in `Muscade`, the elements provide additive contributions to the Lagrangian scalar ``L``, instead of to the residual vector ``R``. Actualy when creating a new element in `Muscade` to create an application, one can either implement a contribution to ``R`` by implementing a method `Muscade.residual` for the element, or a contribution to ``L`` by implementing `Muscade.lagrangian`. For performance, whenever possible (for example when implementing a physical element), prefer `Muscade.residual`.
In `Muscade`, the broad view is taken that anything that contributes to ``L`` is an element (even if no part of the domain is actualy associated to the element). This more general definition of "elements" includes a variety of types:
- Physical element, disretizing differential equations over a part of the domain
- Known external loads on the boundary (non-essential boundary conditions)
- Known external loads in the domain
- Constrained dofs (essential boundary conditions)
- Holonomic equality and inequality constraints (contact)
- Optimisation constraints (e.g. stresses shal not exceed some limit at any point within part of the domain)
- Response measurements (surprisal on Xdofs)
- Unknown external loads (surprisal on Udofs)
- Damage (surprisal on Adofs)
- Cost of unfavorable response (cost on Xdofs)
- Cost of actuators (cost on Udofs)
- Cost of building a system (cost on Adofs)
Because "everything" is an element, app developers can express a wide range of ideas through `Muscade`'s element API.
## Solvers
Solvers in `Muscade` solve problems of different forms including forward `X` problems
```math
R(X,0,0)=0
```
optimisation `XUA` problems
```math
\begin{aligned}
\nabla_\Lambda L(\Lambda,X,U,A) &= 0 \\
\nabla_X L(\Lambda,X,U,A) &= 0 \\
\nabla_U L(\Lambda,X,U,A) &= 0 \\
\nabla_A L(\Lambda,X,U,A) &= 0
\end{aligned}
```
as well as corresponding `XU` and `XA` problems.
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | docs | 20212 | # Type-stability
## Introduction
This text presents *type stability*, which is one of the important concepts that one needs to understand in order to write high-performance Julia code.
This text is aimed at Julia users that are familiar with composite types, abstract types, functions, methods and multiple dispatch. At the same time, as little advanced Julia syntax as possible is used, to make the text accessible.
## To type, or not to type
The developers of Julia wanted to solve the two-language problem. They have achieved this and produced a language that "walks like Python and runs like C". Julia "walks like Python", because it is not necessary to systematically define the type of every variable that appears in the code. It "runs like C" because it is a compiled language, and produces (or rather, *can* produce) highly efficient machine code.
Python and MATLAB are examples of interpreted language. In a pure interpreted language, the type of the variables is computed at run time, at the same time as the value of the variables. As long as the values of the inputs to the code are known at the top level (in the REPL or the top script), the interpretation infers, step by step the type of the variables, all the way down the call stack. This allows to write functions without specifying types, and this in turn allows to write generic code (for example an iterative solver that works just as well with `Float64` and `Float32` variables). The disadvantage is that inferring the type of variables on the fly introduces significant overhead at run time.
At the other end of the scale C and Fortran are examples of strictly typed compiled languages. Because the source code specifies the type of every variable in the function (both variables in the function interface, and local variables), the compiler can create efficient machine code for each function, just by considering the code of that function alone. The disadvantage is that type declaration takes time to write and clutters the source code, and (unless the language offers "templates", as C++ does), it may be necessary to write several methods, identical in all but types of variables, to make an algorithm available to various data types.
## Julia's approach to type specifications
Julia takes the sweet spot in between, not requiring to specify the type of each variable, yet producing fast machine code. The trick is as follows: every time a method is called (so, at run time), with a combination of *concrete types* of arguments that has not yet been encountered for this method, the compiler quicks in. A "concrete type" is the information returned by `typeof()` when called on a variable. One example is `Float64`. This is as opposed to an abstract type, like `Real`, which is a set of concrete types, and includes `Float64` and `Float32`. *In the rest of this text "type" will refer to "concrete type"*.
The compiler now has the source code of the method, and the types of all the arguments. The compiler will produce a *method instance* (or instance, for short), which is machine code for this combination. One interesting implication is that writing strictly typed method interfaces in Julia does not provide any improvement of machine code performance: the compiler takes the type of the arguments from the calling context anyway. A strictly typed interface has the disadvantage of offering no flexibility. A method that only accepts a `Vector` will not accept other vector-like things like a `SubArray` (an array view), a `Adjoint` (a transposed array), a `SparseMatrix` or a `StaticArray`, even thought the method probably implements an algorithm that would compile perfectly well for all of these.
However, providing partial specification of the type of the arguments of a method serves important purposes in Julia:
1. If a function has several methods, it allows to specify which method should be executed (multiple dispatch). This is where abstract types like `Real`, `AbstractVector` and `AbstractVector{<:Real}` come into their own.
2. It improves code readability, stating for example "this method expects some vector of some real numbers - but not a string".
3. It provides more graceful failures: "function `foo` has no method that takes in a string" is more informative that some esoteric failure down the line when attempting to add two strings.
## What is type stability?
If the source code of the method is well written, the source code and the concrete type of all arguments is enough information for the compiler to infer the concrete type of every variable and expression within the method. The method is then said to be "typestable", and the Julia compiler will produce efficient code.
If, for a variety of reasons that will be studied in the following, the type of a local variable cannot be inferred from the types of the arguments, the compiler will produce machine code full of "if"s, covering all options of what the type of each variable could be. The loss in performance is often significant, easily by a factor of 10.
**If you are yourself able to infer the type of every local variable, and every expression in a method (or script) from the types (*not* the values) of the arguments or from constants in the code, the function will be typestable.** Actually, as will be seen below, this inference of types is also allowed access to `struct` declarations, and to the types of the return values of functions called by the function you are studying.
The rest of this text will examine a variety of situations, ranging from obvious to more tricky tricky, in which it is not possible to infer the types of local variables from the types of the arguments, resulting in type instability.
For this purpose, it will be useful to write down the information available to the compiler. So for example, if the method
```julia
function add(a::Number,b::Number)
c = a+b
return c
end
```
is called with `a` of type `Float64` and `b` of type `Int32`, then we will write the information available to the compiler to create an instance as
```julia
instance add(a::Float64,b::Int32)
c = a+b
return c
end
```
`instance` is not Julia syntax, it is just a notation introduced in this text to describe an instance. In such `instance` description, a *concrete* type must be associated with every argument.
## If, then
Consider the following method instance
```julia
instance largest(a::Float64,b::Int64)
if a > b
c = a
else
c = b
end
return c
end
```
The variable `c` will be set to either `a` or `b`. `c` will take the value *and the type* of either *a* or *b*. The type of `c` depends on an operation `a > b` on the *values* of `a` and `b`: the type of `c` cannot be inferred from the type of arguments alone, and this code is not typestable.
Several approaches might be relevant to prevent type instability. The simplest is to code `largest` so that it only accepts two arguments of the same type.
```julia
function largest(a::R,b::R) where{R<:Real}
if a > b
c = a
else
c = b
end
return c
end
```
The method is general, it can result in the generation of method instances like `instance largest(a::Float64,b::Float64)`, `instance largest(a::Int64,b::Int64)` and many others. It cannot result in the generation of machine code for `instance largest(a::Float64,b::Int64)` (because `R` cannot be both `Int64` and `Float64`). If we need to be able to handle variables of different types, yet want type stability, a solution is to use *promotion* to ensure that `c` is always of the same type.
```julia
function largest(a,b)
pa,pb = promote(a,b)
if a > b
c = pa
else
c = pb
end
return c
end
```
`promote` is defined so that `pa` and `pb` have the same type, and this type is inferred from the types of `a` and `b`. For example, for a call `instance largest(a::Float64,b::Int64)`, the types of `pa`, `pb` and `c` will be `Float64`, to which one can convert a `Int64` variable without loss of information (well, mostly).
**Do not allow an if-then construct to return a variable which type depends on the branch taken.**
## Method return value
A method `foo` that would call the above first, not typestable, version of the method instance `largest` would receive as output a variable of a type that is value dependent: `foo` itself would not be typestable. The workaround here is to create typestable methods for `largest`, as suggested above.
One example is the method `Base.findfirst(A)`, which given a `Vector{Boolean}` returns the index of the first `true` element of the vector. The catch is that if all the vector's elements are `false`, the method returns `nothing`. `nothing` is of type `Nothing`, while the index is of type `Int64`. Using this method will make the calling method not typestable.
**Avoid methods that return variables of value-dependant types.**
## Array of abstract element type
Consider the following code
```julia
v = [3.,1,"Hello world!"]
function showall(v)
for e ∈ v
@show e
end
end
showall(v)
```
The above call `showall(v)` generates a method instance
```julia
instance showall(v::Array{Any,1})
for e ∈ v
@show e
end
end
```
The concrete type of `e` cannot be inferred from `Array{Any,1}`, because `Any` is not a concrete type. More specifically, the type of `e` changes from one iteration to the next: the code is not typestable. If `v` is of type `Array{Any,1}`, even if `V` has elements that are all of the same type, this does not help:
```julia
v = Vector{Any}(undef,3)
v[1] = 3.
v[2] = 1.
v[3] = 3.14
showall(v)
```
`e` may have the same type at each iteration, but this type still cannot be inferred from the type `Array{Any,1}` of the argument.
If we define `w = randn(3)`, `w` has type `Array{Float64,1}`. This is much more informative: every element of `w` is known to have the same concrete type `Float64`. Hence the call `showall(w)` generates a method instance
```julia
instance showall(v::Array{Float64,1})
for e ∈ v
@show e
end
end
```
and the compiler can infer that `e` is a `Float64`.
**Wherever possible use arrays with a concrete element type.**
Sometimes, the use of array with abstract element type is deliberate. One may really wish to iterate over a heterogeneous collection of elements and apply various methods of the same function to them: we design for dynamic dispatch, and must accept that the process of deciding which method to call takes time. Two techniques can be used to limit the performance penalty.
The first is the use of a "function barrier": The loop over the heterogenous array should contain as little code as possible, ideally only the access to the arrays element, and the call to a method.
```julia
for e ∈ v
foo(e)
end
```
If `v` contains elements of different type, the loop is not typestable and hence slow. Yet each value of `e` at each iteration has its unique concrete type, for which an instance of `foo` will be generated: `foo` can be made typestable and fast.
The second, a further improvement of the first, is to group elements by concrete type, for example, using a heterogenous arrays of homogeneous arrays.
```julia
vv = [[1.,2.,3.],[1,2]]
for v ∈ vv # outerloop
innerloop(v)
end
function innerloop(v)
for e ∈ v
foo(e)
end
end
```
Here `vv` is an `Array{Any,1}`, containing two vectors of different types. `vv[1]` is a `Array{Float64,1}` and `vv[2]` is a `Array{Int64,1}`.
Function `innerloop` is called twice and two instances are generated
```julia
instance innerloop(v::Array{Float64,1})
for e ∈ v # e is Float64
foo(e)
end
end
instance innerloop(v::Array{Int64,1})
for e ∈ v # e is Int64
foo(e)
end
end
```
and in both instances, the type of `e` is clearly defined: the instances are typestable.
The with this second approach is that the loop `for v ∈ vv` has few iterations (if the number of types is small compared to the number of elements in each types).
## Structure of abstract field type
A similar loss of type stability arises when reading data from structures that have a field of abstract type:
```julia
struct SlowType
a
end
struct JustAsBad
a::Real
end
struct MuchBetter
a::Float64
end
function show_a(s)
@show s.a
end
show_a(SlowType(3.))
show_a(JustAsBad(3.))
show_a(MuchBetter(3.))
```
The first call to `show_a` generates
```julia
instance show_a(s::SlowType)
@show s.a # The concrete type of field a of type SlowType cannot be
# inferred from the definition of SlowType
end
```
The second call to `show_a` has the same problem. The third call generates a typestable instance
```julia
instance show_a(s::Better)
@show s.a # That's a Float64
end
```
It is often interesting to create structures with fields that can have various types. A classic example is Julia's `Complex` type, which can have real and imaginary components which are either both `Float64`, both `Float32` or other more exotic choices. This can be done without losing type stability by using parametric types:
```julia
struct FlexibleAndFast{R}
a::R
end
show_a(FlexibleAndFast(3.))
show_a(FlexibleAndFast(3 ))
```
The above calls generate two typestable instances of `show_a`
```julia
instance show_a(s::FlexibleAndFast{Float64})
@show s.a # That's a Float64
end
instance show_a(s::FlexibleAndFast{Int64})
@show s.a # That's an Int64
end
```
**Always use `struct` with fields of concrete types. Use parametric structure where necessary**.
## A note on constructors for parametric types
Consider a `struct` definition without inner constructor:
```julia
struct MyType{A,B}
a::A
b::B
end
```
Julia will automatically generate a constructor *method* with signature
```julia
MyType{A,B}(a::A,b::B)
```
Julia will also produce another method with signature
```julia
MyType(a::A,b::B)
```
because for `MyType`, it is possible to infer all type parameters from the types of the inputs to the constructor. Other constructors like
```julia
MyType{A}(a::A,b::B)
```
have to be defined explicitly (how should the compiler decide whether to interpret a single type-parameter input as `A` or `B`...).
Consider another example:
```julia
struct MyType{A,B,C}
a::A
b::B
end
```
Julia will automatically generate a constructor method with signature
```julia
MyType{A,B,C}(a::A,b::B)
```
but will not generate other methods. A method like
```julia
MyType{C}(a::A,b::B)
```
would have to be defined explicitly.
## StaticArrays
Julia `Array`s are an example of parametric type, where the parameters are the type of elements, and the dimension (the number of indices). Importantly, the *size* of the array is not part of the *type*, it is a part of the *value* of the array.
The package `StaticArrays.jl` provides the type `StaticArray`, useful for avoiding another performance problem: garbage collection that follows the allocation of `Array`s on the heap. This is because `StaticArray` are allocated on the stack, simplifying runtime memory management.
```julia
using StaticArrays
SA = SVector{3,Float64}([1.,2.,3.])
SA = SVector(1.,2.,3.)
SA = SVector([1.,2.,3.])
```
The first call to `SVector` is typestable: all the information needed to infer the type of `SA` is provided in curly braces. The second call is typestable too, because the compiler can deduce the same information from the type and number of inputs. The third call is problematic: while the type of the elements of `SA` can be inferred by the compiler, the length of `[1.,2.,3.]` is part of this array's value, not type. The type of `SA` has a parameter that depends on the value (the size) of the argument passed to the constructor. Not only does this generate an instance of the constructor that is not type stable, but the non-inferable type of `SA` "contaminates" the calling code with type instability.
## Val
What if we want to write a function that takes an `Vector` as an input, processes it (for example just keeps it as it is), and returns a `SVector` of the same shape. Of course we want this function to be general and not be limited to a given array size *and* we want this function to be typestable, for good performance.
First attempt:
```julia
function static(v::Vector)
return SVector{length(v),eltype(v)}(v)
end
```
This function is not typestable. It constructs a variable of type `StaticArray{(3,),Float64}`, where `3` is obtained as the `length` of `v`, and the length is part of the value of an `Array`. Value-to-type alarm!
One possible solution is to use `Val`. Let us say that `static` is called by a function `foo` within which the length of `v` can be inferred at compile time. We could create the following code
```julia
function static(v,::Val{L}) where{L}
return SVector{L,Float64}(v)
end
function foo()
Val3 = Val(3)
Val4 = Val(4)
@show static([1.,2.,3.] ,Val3)
@show static([1.,2.,3.,4.],Val4)
end
```
The call `Val(3)` generates a variable, of type `Val{3}`. Clearly, `Val` as a function is not typestable, since it creates a variable of a type depending on the value of its argument.
However, function `foo` is typestable. This may come as a surprise, but two things conspire to allow this:
1. The source code of `foo` explicitly mentions the *constants* `3` and `4`, and the compiler has access to it.
2. The compiler is greedy - it evaluates at compile time whenever possible. Hence the call `Val(3)` is evaluated during compilation, and `Val3` is known to the compiler to be a a value-empty variable of type `Val{3}`.
In `foo`, the method `static` is called twice, leading to the generation of two typestable instances
```julia
instance static(v,::Val{3})
return SVector{3,Float64}(v)
end
instance static(v,::Val{4})
return SVector{4,Float64}(v)
end
```
What if the length of the vectors is not defined as a constant in `foo`? If this length is the result of some computation, the call to `Val` with not be typestable. If `foo` is high enough in the call hierarchy, and outside any time-critical loop, this is not an issue: only `foo` will not be typestable, but functions that it calls can still be typestable (cf. the function barrier pattern).
**`Val` allows to move type instability up the call hierarchy, or eliminate it altogether.**
## Functions
The type `Function` is an *abstract* datatype, and every function in Julia has its own type. Here we refer not to the type of the variables returned by the function, but to the function being a variable in itself.
The implication is that if we have a scalar-valued function `energy` that takes a function `signal` as an input, and computes the energy of the signal over some interval, then a new instance of `energy` will be compiled every time it is called with an new argument `signal`.
This also has implications on how to store functions in a `struct`. This is not typestable
```julia
struct MyType
foo::Function
end
```
but this is
```julia
struct MyType{Tfoo}
foo::Tfoo
end
```
## @code_warntype
One important tool to check that an instance is typestable is the macro `@code_warntype`. For example
```julia
v = randn(3)
@code_warntype Val(length(v))
@code_warntype static(v,Val(length(v)))
```
The first invocation of `@code_warntype` outputs a semi-compiled code, and highlights some of the types in red: the call `Val(3)` is not typestable. The second invocation of `@code_warntype` produces an output in which all types are highlighted in blue: the call to `static` is typestable. Note that `@code_warntype` only analyses the compilation of the outermost function `static` - given the arguments `v` and `Val(length(v))`.
## Profile.jl
`Profile.jl` and `ProfileView.jl` together provide a "flame graph", a graphical representation of where processor time goes, in which code that is not typestable is highlighted. Output from the profiler often shows how type instability propagates: a single variable that is not typestable makes "anything it touches" type unstable.
Particularly useful, one can click on a function, and then type `warntype_last()` in the REPL to get to see a `@code_warntype` output for that function. | Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | docs | 4766 | ```@meta
CurrentModule = Muscade
```
!!! info
`Muscade.jl` is still under development, and not ready for general usage:
- New versions may introduce breaking changes in user and element APIs.
- Graphics generation will be revised.
- New solvers will be added.
# [Purpose](@id purpose)
**[Muscade.jl](https://github.com/SINTEF/Muscade.jl): Create and solve optimization-FEM models.**
**Framework**: `Muscade` provides an API to create new elements and new solvers. It also provides the "piping" - facilities to create models, assemble matrices and vectors and extract and export results. As a framework, `Muscade` does not provide finite element types modeling any specific domain of physics. It does however provide some domain-agnostic element types for doing things like introducing boundary conditions and introducing costs.
The API to create new elements is quite classic (given degrees of freedom, compute their duals, aka. residuals): as such Muscade is not a *modelling language* like for example UFL which provides a formalism in which a "well posed problem" can be defined with domain, differential equations and boundary conditions.
In order to obtain high performance, elements have to be implemented using a specific programming style: functional programming, using immutables.
**Rapid development**: `Muscade` lets application developer focus on the interesting parts: formulating new element types and creating new solvers. `Muscade` takes care of the tedious parts.
It further accelerates the rapid development of new element types by using automatic differentiation and automatic extraction of element-results (think of stresses and strains, in an element that given nodal displacements computes nodal forces). This results in shorter and more readable element code.
The idea is thus to be able to produce specialised FEM applications, at low cost, to create highly efficient solution to niche problems. It is also hope that `Muscade` will help students of the finite element method to put theory into practice.
**Multiphysics**: When creating a new element, one must define a function `doflist` to describe the `class` (see below) `field` and `node` of each degree of freedom (dof). Element developers can introduce new `field`s (node rotation, hydrogen concentration, temperature, components of the magnetic field...). The user can then associate a `scale` to each field stating that "displacements are the order of `10^-3 m`" or "potentials are of the order of `kV`. This is used to improve the conditioning of the problem.
**Optimization**: Muscade handles optimization-FEM problems, that is, optimization problems constrained by equilibrium of the FEM model.
Optimization-FEM consists in optimising a target function (here called the cost function) under the constraint that a FEM model be exactly verified. This in turn implies that the problem has more unknowns than the model has equation (or at least: more unknowns than linearly independent equations, as would be the case with “insufficient” boundary conditions).
The unknowns that are dual to the FEM equations are noted X-dofs, and sometimes refered to as “response dofs”. The rest of the unknowns can be separated into U-dofs (varying with time, generaly unknown loads) and A-dofs (comstant over time, generaly unknown model parameters). The conditions for such a constrained optimization problem to be well-posed are the Babushka-Brezzi conditions, which say, in essence “if you do not restrain, then at least measure”.
## Applications of optimization-FEM
Besides solving well-posed FEM problems (obtaining the repsonse of a system, given adequate boundary conditions and known loading terms), many applications can be, or should be possible within `Muscade`.
**Reliability analysis**: Finding a design point. What is the most probable combination of external loads `U` and strength of the structure `A` that may cause the response `X` to exceed, in one of many ways, the acceptable?
**Design optimization**: What is the cheapest way to engineer a system (for example a structure) that will survive a set of loading conditions?
**Load identification and monitoring**: Given incomplete and noisy measurements of the response of a system, what are the loads that are most likely to cause a response close to what has been measured?
**Optimal control**: how to steer a system with many dofs into a wanted behaviour?
**Model identification**: given enough measurements on the response of a system responding to at least partly unknown load, is it possible to adjust the model of the system (model calibration, damage detection)?
**Sensor array optimization**: how best to place sensors in a system in order to support the above applications?
| Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.3.8 | 5b0c7355fedce808d74a42cb773fbed686adff3e | docs | 342 | # [Reference manual](@id reference)
## Constants
```@autodocs
Modules = [Muscade]
Order = [:constant]
```
## Types
```@autodocs
Modules = [Muscade]
Order = [:type]
```
## Functions
```@autodocs
Modules = [Muscade]
Order = [:function]
```
## Macros
```@autodocs
Modules = [Muscade]
Order = [:macro]
```
## Index
```@index
``` | Muscade | https://github.com/SINTEF/Muscade.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | code | 676 | module LVServer
using ZMQ, JSON3, ImageCore, Colors, PkgVersion
# version of the communication protocoll: First, and, presumably, the last as well
const PROTOC_V = 0x01
# update these constants on update of any of the packages!
const LabVIEW0_LOCAL_CURRENT = v"0.1.1"
const LabVIEW0_LOCAL_MIN = v"0.1.0"
include("./Julia/ZMQ-server.jl")
# see setglobals() and get_script_path() using these globals
scriptexists = false
scriptOK = false
scriptexcep = nothing
# see setreorderbytes()
reorderbytes = :undefined
export server_0mq4lv, get_script_path, setglobals, setreorderbytes, get_LVlib_path # functions
export scriptexists, scriptOK, scriptexcep # global variables
end
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | code | 4209 | include("./version_check.jl")
include("./ZMQ_utils.jl")
# include("./async.jl")
include("./test_functions.jl")
builtin_fns = merge(utilfunctions, testfunctions, versionfunctions) #, asyncfunctions)
"""
server_0mq4lv(fns=(;); initOK=false)
`server_0mq4lv` (meaning zero MQ for LabView) is the top-level function of the package.
User functions must be supplied as a NamedTuple or Dict. Start ZMQ socket, listen to
requests, parse them, execute the requested user function, send response. Repeat.
# Arguments
- `initOK`: set it to `true` to skip check of the user script
# Examples
```julia-repl
julia> server_0mq4lv((;foo=foo, bar, baz))
```
"""
function server_0mq4lv(fns=(;); initOK=false)
function available_fns()
return (;ext_fns=keys(fns), all_fns = keys(fns_all))
end
context = Context()
socket = Socket(context, REP)
ZMQ.bind(socket, "tcp://*:5555")
version = string(PkgVersion.Version(LVServer))
fns_all = merge(builtin_fns, (;available_fns), fns)
fnlist = keys(fns_all)
global scriptexists
global scriptOK
if ! initOK
if isempty(fns)
initOK = true # package-internal functions only
else
initOK = scriptexists && scriptOK
end
end
if initOK
println("starting ZMQ server, Julia for LabVIEW: LVServer v=$version")
println("available functions:")
println(fnlist)
else
println("server initialisation failed")
@show scriptexists scriptOK
end
try
while true
# Wait for next request from client
bytesreceived = ZMQ.recv(socket)
cmnd = parse_cmnd(bytesreceived)
response = UInt8[]
if cmnd.command == :stop
response = UInt8.([1, PROTOC_V])
elseif !initOK
if !isnothing(scriptexcep)
excep, stack_trace = scriptexcep
else
stack_trace = []
excep = "Julia error on initialisation script"
end
err = nothing
try
err = build_err_info(;
err = true,
errcode = 5235817,
source = @__FILE__,
stack_trace = err_stack_trace,
excep = excep,
)
catch
err = build_err_info(;
err = true,
errcode = 5235817,
source = @__FILE__,
excep = "Julia error on initialisation script",
)
end
response = puttogether(; err = err, returncode = 3)
elseif cmnd.command == :ping
response = UInt8.([2, PROTOC_V])
elseif cmnd.command in (:callfun, :async)
try
pr = parse_REQ(bytesreceived)
fn = pr.fun2call
f = fns_all[fn]
if cmnd.command == :callfun
y = f(; pr.args...)
else
y = asyncall(f; pr.args...)
end
response = puttogether(; y = y)
catch excep
err_stack_trace = stacktrace(catch_backtrace())
# # https://docs.julialang.org/en/v1/manual/stacktraces/#Error-handling-1
err = build_err_info(;
err = true,
errcode = 5235805,
source = @__FILE__,
stack_trace = err_stack_trace,
excep = excep,
)
response = puttogether(; err = err, returncode = 3)
end
else
response = UInt8.([255, PROTOC_V])
end
# Send reply back to client
ZMQ.send(socket, response)
cmnd.command == :stop && break
end
finally
# clean up
ZMQ.close(socket)
ZMQ.close(context)
end # try
end
# server_0mq4lv()
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | code | 6644 | include("./typedefs.jl")
include("./conversions.jl")
"""
setglobals(;isOK, extant=scriptexists, excpn = nothing)
Set the globals of the `LVServer` module. By default do not change the value of `scriptexists`.
Use this function from the top-level scripts, e.g. as executing from LabVIEW.
# Examples
```julia-repl
julia> setglobals(;isOK=true, extant=true);
```
"""
function setglobals(; isOK, extant=scriptexists, excpn = nothing)
global scriptOK = isOK
global scriptexists = extant
global scriptexcep = excpn
return nothing
end
function setreorderbytes(swp::Bool)
global reorderbytes
if swp
reorderbytes = :reorder
else
reorderbytes = :noreorder
end
return nothing
end
"""
get_script_path(p)
Accept a Julia script (with user defined functions) as full path for user functions of just
file name for examples delivered with this package. If the file not found, return the path
to the empty file "dummy.jl". If `p` is empty string, return path to "Examples-UserFn.jl",
which in turn includes some example scripts. Set the global `scriptexists` to `true`
if everything OK.
# Examples
```julia-repl
julia> include(get_script_path(raw"C:\\Users\\eben\\Desktop\\Julia\\my_functions.jl"))
julia> server_0mq4lv((;foo=foo, bar, baz))
```
"""
function get_script_path(p="")
global scriptexists
srcdir = @__DIR__
dummy = joinpath(srcdir, "dummy.jl")
_, suffix = splitext(p)
if p == ""
scriptexists = true
p1 = joinpath(srcdir, "Examples-UserFn.jl")
return p1
elseif suffix != ".jl"
scriptexists = false
return dummy
elseif isfile(p)
scriptexists = true
return realpath(p)
end
p1 = joinpath(srcdir, p) # used for example scripts residing in the package
if isfile(p1)
scriptexists = true
return p1
else
scriptexists = false
return dummy
end
end
"""
StackFrame_to_NamedTuple(fm)
Convert Error Stack Frame.
"""
function StackFrame_to_NamedTuple(fm)
#= StackFrame, see:
https://docs.julialang.org/en/v1/base/stacktraces/
func::Symbol
The name of the function containing the execution context.
linfo::Union{Core.MethodInstance, CodeInfo, Nothing}
The MethodInstance containing the execution context (if it could be found).
file::Symbol
The path to the file containing the execution context.
line::Int
The line number in the file containing the execution context.
from_c::Bool
True if the code is from C.
inlined::Bool
True if the code is from an inlined frame.
pointer::UInt64
=#
return (
frame_summary = string(fm),
func = fm.func,
linfo = string(fm.linfo),
file = fm.file,
line = fm.line,
from_c = fm.from_c,
inlined = fm.inlined,
pointer = fm.pointer,
)
end
"""
build_err_info(;err, errcode, source, stack_trace, excep)
Combine data for LV-style error cluster (the first three arguments), and detailed
exception information.
"""
function build_err_info(;
err::Bool = false,
errcode::Int = 0,
source::String = "",
stack_trace = [],
excep = "",
)
if err
# default values on error
if errcode == 0
errcode = 5235805
end
end
if !isempty(stack_trace)
stack_trace = [StackFrame_to_NamedTuple(e) for e in stack_trace]
end
return (;
status = err,
code = errcode,
source = source,
detailed_info = (exception_or_info = string(excep), trace = stack_trace),
)
end
"""
puttogether(;y, err, opt_header, returncode)
Combine data to be sent to LabVIEW into a byte vector.
# Arguments
- `y`: data returned by the user function. Can be Dict, NamedTuple or other data type
convertible to pairs with `key::T where T<: Union{Symbol, AbstractString}`
- other arguments are self-explaining
"""
function puttogether(;
y = Dict{Symbol,Any}(),
err = build_err_info(),
opt_header::ByteArr = UInt8[],
returncode::Int = 0,
)
returncode = UInt8(returncode)
y = Dict{Symbol,Any}(pairs(y)) # y could be Dict or named tuple
@assert haskey(err, :status) &
haskey(err, :code) &
haskey(err, :source) &
haskey(err, :detailed_info)
if haskey(y, :bigarrs)
arrs = pop!(y, :bigarrs)
bin_data, bindata_descr = nums2bin(arrs)
push!(y, :bindata_descr => bindata_descr)
elseif haskey(y, :bin_data)
bin_data = y[:bin_data]
else
bin_data = UInt8[]
end
push!(y, :errorinfo => err)
jsonstring = ByteArr(JSON3.write(y))
o_h_lng = int2bytar(length(opt_header))
bin_lng = int2bytar(length(bin_data))
js_lng = int2bytar(length(jsonstring))
r = vcat(returncode, PROTOC_V, o_h_lng, bin_lng, opt_header, bin_data, jsonstring)
return r
end
function parse_cmnd(b)
c = b[1]
prot_v = b[2]
prot_OK = prot_v <= PROTOC_V
if c == UInt8('p')
command = :ping
elseif c == UInt8('s')
command = :stop
elseif c == UInt8('c')
command = :callfun
elseif c == UInt8('a')
command = :async
else
command = :undef
end
return (; command, prot_OK, prot_v)
end
# # # # # # #
"""
parse_REQ(b)
Parse data received from LabVIEW, first pass. If there are any binary data, these will be
further parsed downstream.
# Arguments
- `b::UInt8[]`: data received
"""
function parse_REQ(b)
opt_header = fun2call = json_data = json_dict = args = bin_data = bytearr_lng = nothing
o_h_lng_start = 3
bin_lng_start = o_h_lng_start + 4
o_h_lng = bytar2int(b[o_h_lng_start:3+o_h_lng_start])
bin_lng = bytar2int(b[bin_lng_start:3+bin_lng_start])
o_h_start = bin_lng_start + 4
if o_h_lng > 0
opt_header = b[o_h_start:o_h_start+o_h_lng-1]
end
bin_start = o_h_start + o_h_lng
if bin_lng > 0
bin_data = b[bin_start:bin_start+bin_lng-1]
end
json_start = bin_start + bin_lng
json_data = String(b[json_start:end])
json_dict = Dict(JSON3.read(json_data))
fun2call = Symbol(pop!(json_dict, :fun2call))
args = json_dict # the rest
if haskey(args, :bindata_descr)
bindata_descr = pop!(args, :bindata_descr)
numarrs = bin2nums(bin_data = bin_data, bindata_descr = bindata_descr) #TODO add semicolon before kwargs?
args = merge(args, numarrs)
elseif !isnothing(bin_data)
push!(args, :bin_data => bin_data)
end
return (; opt_header, fun2call, args)
end
utilfunctions = (; setreorderbytes)
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | code | 625 | import Base.Threads.@spawn
mutable struct Tasks
d::Dict{Int, Any}
c::Int # counter
end
const tasks = Tasks(Dict(), 1)
function getticket(tasks)
return tasks.c += 1
end
function asyncall(f; kwargs...)
global tasks
ticket = getticket(tasks)
id = @spawn f(; kwargs...)
push!(tasks.d, ticket=>id)
return (;ticket)
end
function checkjob(; ticket)
global tasks
id = tasks.d[ticket]
done = istaskdone(id)
if !done
return (; done)
else
result = fetch(id)
pop!(tasks.d, ticket)
return (; done, result)
end
end
asyncfunctions = (; checkjob)
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | code | 6714 | include("./reorder_bytes.jl")
const SUPPORTED_REALS = (
Float32, Float64,
Int8, Int16, Int32, Int64,
UInt8, UInt16, UInt32, UInt64,
Bool,
)
const SUPPORTED_COMPLEX = (ComplexF32, ComplexF64)
function int2bytar(i::Int; i_type = UInt32)
if i_type == Bool
return UInt8(Bool)
else
return reinterpret(UInt8, [i_type(i)])
end
end
function bytar2int(b::ByteArr; i_type = UInt32)
if i_type != Bool
return reinterpret(i_type, b)[1]
else
return Bool(b[1])
end
end
function numtypestring(ar)
t = eltype(ar)
global SUPPORTED_REALS
if t in SUPPORTED_REALS
return string(t)
elseif t == ComplexF32 # special cases, as string(ComplexF32) is "Complex{Float32}"
return "ComplexF32"
elseif t == ComplexF64
return "ComplexF64"
else
return throw(
DomainError("$(string(t)) is not a supported numeric type for exchange with LabVIEW: consider converting array first."))
end
end
function fromrowmajor(v, arrdims)
revdims = Tuple(reverse(arrdims))
neworder = length(revdims):-1:1
arr = permutedims(reshape(v, revdims), neworder)
return arr
end
function fromcolmajor(v, arrdims)
revdims = Tuple(reverse(arrdims))
neworder = length(revdims):-1:1
arr = permutedims(reshape(v, revdims), neworder)
return arr
end
function cmplxswap(c::Complex)
c = imag(c) + real(c)im
return c
end
function emptyarr(arrdims, numtype)
Array{numtype}(undef, zeros(Int, length(arrdims))...)
end
function bin2num(; bin_data, nofbytes, start, arrdims, numtype)
nt = Symbol(numtype)
numtype = eval(nt)
if nofbytes == 0 || any(arrdims.==0)
return emptyarr(arrdims, numtype)
end
bin_data = bin_data[start:start+nofbytes-1]
if numtype != Bool
global SUPPORTED_REALS
global SUPPORTED_COMPLEX
global reorderbytes
if reorderbytes == :noreorder
nums = collect(reinterpret(numtype, bin_data))
elseif reorderbytes == :reorder
if numtype in SUPPORTED_REALS
sz = sizeof(numtype)
elseif numtype in SUPPORTED_COMPLEX
sz = sizeof(numtype)÷2
end
bin_data = reorder_bytes(bin_data, sz)
nums = collect(reinterpret(numtype, bin_data)) # TODO is collect necessary here??
else
error("bytes order must be set before starting further communication")
end
else
nums = Bool.(bin_data)
end
if length(arrdims) > 1
nums = fromrowmajor(nums, arrdims)
end
return nums
end
function isimage(a)
return (typeof(a) <: AbstractArray{C,2} where C <: Color)
end
function bin2img(; bin_data, nofbytes, start, arrdims, numtype)
if nofbytes == 0 || any(arrdims.==0)
T = typeof(RGB(0,0,0)) # type by example
return emptyarr(arrdims, T)
end
bin_data = bin_data[start:start+nofbytes-1]
if numtype == "img24bit"
arrdims = (3, arrdims[2], arrdims[1])
nums = bin_data
nums = reshape(bin_data, arrdims)
nums = permutedims(nums, [1, 3, 2])
nums = colorview(RGB, normedview(nums))
else
throw(DomainError("image data type ($numtype) not supported"))
end
return nums
end
function fn_by_category(ctg)
fs = Dict("images" => bin2img, "numarrays" => bin2num)
return fs[ctg]
end
"""
bin2nums(; bin_data, bindata_descr)
Get data as received from LabVIEW (binary + array of decriptions) and return a
dictionary of de-serialized arrays (or other supported data types, e.g. images).
The dictionary items meant to be supplied as kwargs to user functions.
"""
function bin2nums(; bin_data, bindata_descr)
arrs = [
fn_by_category(bdd.category)(
bin_data = bin_data,
nofbytes = bdd.nofbytes,
start = bdd.start,
arrdims = bdd.arrdims,
numtype = bdd.numtype,
) for bdd in bindata_descr
]
kwarg_names = [Symbol(bdd.kwarg_name) for bdd in bindata_descr]
darrs = Dict(Pair.(kwarg_names, arrs))
return darrs
end
# # # # # # # #
function tocolmajor(arr)
arrdims = size(arr)
lng = length(arrdims)
if lng > 1
neworder = lng:-1:1
arr = permutedims(arr, neworder)
end
return vec(arr)
end
function nums2ByteArr(nums)
nums = tocolmajor(nums)
if eltype(nums) == Bool
return UInt8.(nums)
else
return reorder_bytes(nums)
end
end
function data2bin(img::I, kwarg_name) where I <: AbstractArray{C,2} where C <: Colorant
# in most cases, image is already RGB{N0f8} anyway, but who knows:
img = convert.(RGB{N0f8}, img)
cnv = channelview(img)
rwv = collect(rawview(cnv))
prw = collect(permutedims(rwv, [1,3,2]))
bd = vec(prw)
bdd = BinDescr()
bdd.kwarg_name = kwarg_name
bdd.numtype = "img24bit"
bdd.category = "images"
bdd.arrdims = collect(size(img))
bdd.nofbytes = length(bd)
return (bd, bdd)
end
function data2bin(arrdata, kwarg_name)
bdd = BinDescr()
bdd.kwarg_name = kwarg_name
bdd.numtype = numtypestring(arrdata)
if eltype(arrdata) <: Complex
arrdata .= cmplxswap.(arrdata)
end
bdd.arrdims = collect(size(arrdata))
bd = nums2ByteArr(arrdata)
bdd.nofbytes = length(bd)
return (bd, bdd)
end
"""
data2bin!!(bin_data::ByteArr, bindata_descr::Vector{BinDescr}; arrdata, kwarg_name)
Serialize for exchange with LabVIEW: Compute binary representation and
description of data (currently supported types are numeric arrays and images).
Append the binary representation to `bin_data`, and description to
`bindata_descr` array (if the first two arguments are provided, mutate them,
otherwise create).
"""
function data2bin!!(
bin_data::ByteArr = ByteArr(),
bindata_descr::Vector{BinDescr} = BinDescr[];
arrdata,
kwarg_name,
)
@assert isempty(bin_data) == isempty(bindata_descr)
bd, bdd = data2bin(arrdata, kwarg_name)
bdd.start = length(bin_data) + 1
bin_data = vcat(bin_data, bd)
push!(bindata_descr, bdd)
return (; bin_data, bindata_descr)
end
"""
nums2bin(arrs)
Accept an iterable of array-like data (numeric arrays, images, anything to come...). Compute
binary representation and description for them and return binary data and array of
descriptions, ready to be sent to LabVIEW.
"""
function nums2bin(arrs)
bin_data = ByteArr()
bindata_descr = BinDescr[]
for arrname in keys(arrs)
bin_data, bindata_descr =
data2bin!!(bin_data, bindata_descr; arrdata = arrs[arrname], kwarg_name = arrname)
end
return (; bin_data, bindata_descr)
end
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | code | 543 | function reorder_bytes(bvec::T, elsize) where T<:AbstractVector{I} where I<:Union{UInt8,Int8}
l = length(bvec)
h = Int(l//elsize)
m = reshape(bvec, elsize, h)
m1 = m[end:-1:begin, :] # having @views result in a slow collect with a lot of allocations downstream
return reshape(m1, l)
end
function reorder_bytes(vc::T) where T<:AbstractArray{N} where N<:Number
elsize = sizeof(eltype(vc))
l = length(vc)
v = vec(vc)
s = elsize * l
bvec = reinterpret(UInt8, v)
return reorder_bytes(bvec, elsize)
end
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | code | 936 | """
loopback(; showversion=false, idx=-1, kwargs...)
Return all "binary-encoded" arrays without change. For a positive `idx` also return
a (JSON-encoded) element of one of these arrays. Can be used for testing.
"""
function loopback(; idx=-1, kwargs...)
bigarrs = Dict(pairs(kwargs))
if idx < 0
return (; bigarrs)
end
# otherwise return an element
if :testarr in keys(bigarrs)
a=kwargs[:testarr]
else
first_arrkey = sort([(keys(bigarrs))...])[1] # first by alphabet
a=kwargs[first_arrkey]
end
elem = -1
if ! isimage(a)
try
elem = a[idx...]
if typeof(elem) in (ComplexF32, ComplexF64)
elem = (re = real.(elem), im = imag.(elem))
elseif elem isa Bool
elem = UInt8(elem)
end
catch
end
end
return (; elem, bigarrs)
end
testfunctions = (; loopback)
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | code | 528 | ByteArr = Vector{UInt8} # byte array
"""
BinDescr
Describe (a chunk) of binary data, e.g. numeric array or image in the LVServer format used
for exchange between Julia and LabVIEW. Gets converted from/to JSON for transport.
"""
mutable struct BinDescr
nofbytes::Int
numtype::String
start::Int
arrdims::Vector{Int}
kwarg_name::Union{String,Symbol}
category::String
end
BinDescr() = BinDescr(0, "", 1, [], "", "numarrays")
JSON3.StructTypes.StructType(::Type{BinDescr}) = JSON3.StructTypes.Struct()
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | code | 3944 |
function version_this_pkg()
v = PkgVersion.Version(LVServer)
return (; major=v.major, minor=v.minor, patch=v.patch)
end
function trunc_v(v)
return VersionNumber(v.major, v.minor, v.patch)
end
"""
check_pkg_versions(;LV_v, LVServer_current, LVServer_min)
Compare the (LV) client and (Julia) server package software versions. \
Error on incompatible versions, warn if not the most recent version known to the opposite side.
# Arguments
- `LV_v`: version of `LabVIEW0` on the client as reported by `LV2Julia_core.lvlib`
- `LVServer_current`: most current `LVServer` version known to `LabVIEW0`/`LV2Julia_core.lvlib`
- `LVServer_min`: the least `LVServer` version compatible with the `LabVIEW0`/`LV2Julia_core.lvlib` client
# Examples
```julia-repl
julia> lvnt = (; LV_v=(major=0, minor=2, patch=1), LVServer_current=(major=1, minor=5, patch=6), LVServer_min=(major=0, minor=2, patch=0));
julia> check_pkg_versions(; lvnt...)
```
"""
function check_pkg_versions(;LV_v, LVServer_current, LVServer_min)
LV_v = VersionNumber(NamedTuple(LV_v)...)
LVServer_min = VersionNumber(NamedTuple(LVServer_min)...)
LVServer_remote_current = VersionNumber(NamedTuple(LVServer_current)...)
LVServer_localv = PkgVersion.Version(LVServer)
# @show LV_v LVServer_localv LVServer_min LVServer_remote_current
LVServer_incompat = LVServer_localv < LVServer_min
LV_incompat = LV_v < LabVIEW0_LOCAL_MIN
LVServer_OK = trunc_v(LVServer_localv) >= LVServer_remote_current
LV_OK = LV_v >= trunc_v(LabVIEW0_LOCAL_CURRENT)
if LVServer_incompat
err_msg = "The LVServer package version on this Julia server is $LVServer_localv. \
At least $LVServer_min is expected by LabVIEW0 client."
end
if LV_incompat
err_msg = "The version of LabVIEW0 client (or of the currently used LV2Julia_core.lvlib) is $LV_v. \
At least $LabVIEW0_LOCAL_MIN is expected by LVServer package version on this Julia server"
end
if (LVServer_incompat | LV_incompat)
@warn err_msg # for local display on the server
throw(ErrorException(err_msg)) # returned to the LV client
end
if !LVServer_OK
warn_msg = "The LVServer package version on this Julia server is $LVServer_localv. \
According to LabVIEW0 client, $LVServer_remote_current is available, please consider LVServer update."
end
if !LV_OK
warn_msg = "The version of LabVIEW0 client (or of the currently used LV2Julia_core.lvlib) is $LV_v. \
According to LVServer package on this Julia server, $LabVIEW0_LOCAL_CURRENT is available, \
please consider LabVIEW0 update"
end
all_current = (LVServer_OK & LV_OK)
if !all_current
@warn warn_msg # for local display on the server
end
v = LVServer_localv
return (;all_current, LVServer_v=(; major=v.major, minor=v.minor, patch=v.patch))
end
versionfunctions = (; version_this_pkg, check_pkg_versions,)
# const LabVIEW0_LOCAL_CURRENT = v"0.1.1"
# const LabVIEW0_LOCAL_MIN = v"0.1.0"
# version = "0.2.2"
#
# lvnt_e1 = (; LV_v=(major=0, minor=1, patch=1), LVServer_current=(major=4, minor=5, patch=6), LVServer_min=(major=1, minor=3, patch=0));
# LVServer below min
#
# lvnt_e2 = (; LV_v=(major=0, minor=0, patch=3), LVServer_current=(major=0, minor=2, patch=2), LVServer_min=(major=0, minor=2, patch=0));
# LabVIEW0 client below min
#
# lvnt_w1 = (; LV_v=(major=0, minor=1, patch=0), LVServer_current=(major=0, minor=2, patch=1), LVServer_min=(major=0, minor=2, patch=0));
# LabVIEW0 client not the most current
#
# lvnt_w2 = (; LV_v=(major=0, minor=1, patch=1), LVServer_current=(major=0, minor=2, patch=5), LVServer_min=(major=0, minor=2, patch=0));
# LVServer not the most current
#
# lvnt_ok = (; LV_v=(major=0, minor=1, patch=1), LVServer_current=(major=0, minor=2, patch=2), LVServer_min=(major=0, minor=2, patch=0));
# lvjs = JSON3.write(lvnt);
# lvnt1 = lvnt1=JSON3.read(lvjs);
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | code | 1116 | include("../ZMQ_utils.jl")
function n2b2n(ar)
bin_data, bdds = data2bin!!(; nums = ar, kwarg_name = "nn")
@show bdds[1]
# bin2nums(;bin_data=bin_data, bindata_descr=bdds)
bin2nums(; bin_data = UInt8.(collect(1:24)), bindata_descr = bdds)
end
function buildbin(sz = [24], tp = UInt8; kwn = "nn")
lng = prod(sz)
ar1d = tp.(1:lng)
if tp in (ComplexF32, ComplexF64)
ar1d = cmplxswap.(ar1d)
end
bin_data = reinterpret(UInt8, ar1d)
if tp == ComplexF32
tpstr = "ComplexF32"
elseif tp == ComplexF64
tpstr = "ComplexF64"
else
tpstr = string(tp)
end
bdd = BinDescr(1, length(bin_data), sz, tpstr, kwn, "numarrays")
return (; bin_data, bdd)
end
function b2n2b(sz = [24], tp = UInt8; kwn = "nn")
bdin, bddin = buildbin(sz, tp; kwn = kwn)
@show bddin
nums = bin2nums(; bin_data = bdin, bindata_descr = [bddin])[Symbol(kwn)]
bdout, bdds = data2bin!!(; arrdata = nums, kwarg_name = kwn)
ok = bdin == bdout
@show ok
return (; ok, bdin, bdout, bddin, bddout = bdds[1], nums, numsint = Int.(abs.(nums)))
end
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | code | 224 | using LVServer
using Test
@testset "LVServer.jl" begin
# Write your tests here.
# Here are the tests:
@test LVServer.tmp_test(0) == 0
@test LVServer.tmp_test(3) == 3
@test LVServer.tmp_test(5) == 5
end
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | docs | 239 | # LVServer
Auxillary package for [LabVIEW0](https://github.com/Eben60/LabVIEW0.jl): Julia server for calling functions from LabVIEW (TM) using ZMQ.
For documentation, see the main package [LabVIEW0](https://github.com/Eben60/LabVIEW0.jl)
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.2.2 | 035706457e85a5d9a374a535e1a0c20de712e6d5 | docs | 922 | # Request message structure
Bytes | format | description | values
------------ | ------------- | ------------- | -------------
**1** | Byte | Command | "p": ping, "s": stop server, "c": call function
**1** | UInt8 | Prot. version | 0x1
**4** | UInt32 | Opt. header length | m
**4** | UInt32 | Binary data length | n
**m** | Vector{UInt8} | Optional header | ..
**n** | Vector{UInt8} | Binary data | ..
**p** | Vector{UInt8} | JSON | see below
## Optional header
A message containing an optional header will be processed as correct, however the content of the optional header ignored. In future versions of protocoll an optional header could be used e.g. for a message ID.
## JSON structure
Field | format | required? | decription
------------ | ------------- | ------------- | -------------
fun2call | string | required | Name of the Julia function
kwargs | any | optional | Keyword args to be passed to that function
| LVServer | https://github.com/Eben60/LVServer.jl.git |
|
[
"MIT"
] | 0.1.1 | 6b6ed2992ac44fd72259b02b3c9f2711dce75ab4 | code | 90 | using Documenter, Example
push!(LOAD_PATH,"../src/")
makedocs(sitename="My Documentation") | CSSMakieLayout | https://github.com/QuantumSavory/CSSMakieLayout.jl.git |
|
[
"MIT"
] | 0.1.1 | 6b6ed2992ac44fd72259b02b3c9f2711dce75ab4 | code | 1820 | using WGLMakie
WGLMakie.activate!()
using JSServe
using Markdown
# 1. LOAD LIBRARY
using CSSMakieLayout
config = Dict(
:resolution => (1400, 700), #used for the main figures
:colorscheme => ["rgb(242, 242, 247)", "black", "#000529", "white"]
)
landing = App() do session::Session
# Active index: 1 2 or 3
# 1: the first a.k.a 'a' figure is active
# 2: the second a.k.a 'b' figure is active
# 3: the third a.k.a 'c' figure is active
activeidx = Observable(1)
# Create the buttons and the mainfigures
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
buttons = [modifier(wrap(DOM.h1("〈")); action=:decreasecap, parameter=activeidx, cap=3),
modifier(wrap(DOM.h1("〉")); action=:increasecap, parameter=activeidx, cap=3)]
axii = [Axis(mainfigures[i][1, 1]) for i in 1:3]
# Plot each of the 3 figures using your own plots!
scatter!(axii[1], 0:0.1:10, x -> sin(x))
scatter!(axii[2], 0:0.1:10, x -> tan(x))
scatter!(axii[3], 0:0.1:10, x -> log(x))
# Obtain the reactive layout using a zstack controlled by the activeidx observable
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
activeidx=activeidx,
style="width: $(config[:resolution][1])px")
layout = hstack(buttons[1], activefig, buttons[2])
return hstack(CSSMakieLayout.formatstyle, CSSMakieLayout.Themes[:elegant](config), layout)
t
end
isdefined(Main, :server) && close(server);
port = 8888
interface = "127.0.0.1"
proxy_url = ""
server = JSServe.Server(interface, port; proxy_url);
JSServe.HTTPServer.start(server)
JSServe.route!(server, "/" => landing);
wait(server) | CSSMakieLayout | https://github.com/QuantumSavory/CSSMakieLayout.jl.git |
|
[
"MIT"
] | 0.1.1 | 6b6ed2992ac44fd72259b02b3c9f2711dce75ab4 | code | 3665 |
using WGLMakie
WGLMakie.activate!()
using JSServe
using Markdown
# 1. LOAD LIBRARY
using CSSMakieLayout
config = Dict(
:resolution => (1400, 700), #used for the main figures
:colorscheme => ["rgb(242, 242, 247)", "black", "#000529", "white"],
:smallresolution => (280, 160), #used for the menufigures
)
# define some style for the menufigures' container
menufigs_style = """
display:flex;
flex-direction: row;
justify-content: space-around;
background-color: $(config[:colorscheme][1]);
padding-top: 20px;
width: $(config[:resolution][1])px;
"""
landing = App() do session::Session
# Create the menufigures and the mainfigures
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
menufigures = [Figure(backgroundcolor=:white, resolution=config[:smallresolution]) for _ in 1:3]
# Figure titles
titles= ["Figure a: sin(x)",
"Figure b: tan(x)",
"Figure c: cos(x)"]
# Active index/ hovered index: 1 2 or 3
# 1: the first a.k.a 'a' figure is active / hovered respectively
# 2: the second a.k.a 'b' figure is active / hovered respectively
# 3: the third a.k.a 'c' figure is active / hovered respectively
activeidx = Observable(1)
hoveredidx = Observable(0)
# Add custom click event listeners
for i in 1:3
on(events(menufigures[i]).mousebutton) do event
activeidx[]=i
notify(activeidx)
end
on(events(menufigures[i]).mouseposition) do event
hoveredidx[]=i
notify(hoveredidx)
end
end
# Axii of each of the 6 figures
main_axii = [Axis(mainfigures[i][1, 1]) for i in 1:3]
menu_axii = [Axis(menufigures[i][1, 1]) for i in 1:3]
# Plot each of the 3 figures using your own plots!
scatter!(main_axii[1], 0:0.1:10, x -> sin(x))
scatter!(main_axii[2], 0:0.1:10, x -> tan(x))
scatter!(main_axii[3], 0:0.1:10, x -> log(x))
scatter!(menu_axii[1], 0:0.1:10, x -> sin(x))
scatter!(menu_axii[2], 0:0.1:10, x -> tan(x))
scatter!(menu_axii[3], 0:0.1:10, x -> log(x))
# Create ZStacks displaying titles below the menu graphs
titles_zstack = [zstack(wrap(DOM.h4(titles[i], class="upper")),
wrap("");
activeidx=@lift(($hoveredidx == i || $activeidx == i)),
anim=[:opacity], style="""color: $(config[:colorscheme][2]);""") for i in 1:3]
# Wrap each of the menu figures and its corresponing title zstack in a div
menufigs_andtitles = wrap([
vstack(
hoverable(menufigures[i], anim=[:border];
stayactiveif=@lift($activeidx == i)),
titles_zstack[i];
class="justify-center align-center "
) for i in 1:3];
class="menufigs",
style=menufigs_style
)
# Create the active figure zstack and add the :whoop (zoom in) animation to it
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
activeidx=activeidx,
anim=[:whoop])
# Obtain reactive layout of the figures
return wrap(menufigs_andtitles, activefig, CSSMakieLayout.formatstyle)
end
isdefined(Main, :server) && close(server);
port = 8888
interface = "127.0.0.1"
proxy_url = ""
server = JSServe.Server(interface, port; proxy_url);
JSServe.HTTPServer.start(server)
JSServe.route!(server, "/" => landing);
# the app will run on localhost at port 8888
wait(server) | CSSMakieLayout | https://github.com/QuantumSavory/CSSMakieLayout.jl.git |
|
[
"MIT"
] | 0.1.1 | 6b6ed2992ac44fd72259b02b3c9f2711dce75ab4 | code | 9946 | using WGLMakie
WGLMakie.activate!()
using JSServe
using Markdown
# 1. LOAD LAYOUT HELPER FUNCTION AND UTILSm
using CSSMakieLayout
## config sizes TODO: make linear w.r.t screen size
# Change between color schemes by uncommentinh lines 17-18
config = Dict(
:resolution => (1400, 700), #used for the main figures
:smallresolution => (280, 160), #used for the menufigures
:colorscheme => ["rgb(242, 242, 247)", "black", "#000529", "white"]
#:colorscheme => ["rgb(242, 242, 247)", "black", "rgb(242, 242, 247)", "black"]
)
###################### 2. LAYOUT ######################
# Returns the reactive (click events handled by zstack)
# layout of the activefigure (mainfigure)
# and menufigures (the small figures at the top which get
# clicked)
function layout_content(DOM, mainfigures #TODO: remove DOM param
, menufigures, title_zstack, session, active_index)
menufigs_style = """
display:flex;
flex-direction: row;
justify-content: space-around;
background-color: $(config[:colorscheme][1]);
padding-top: 20px;
width: $(config[:resolution][1])px;
"""
menufigs_andtitles = wrap([
vstack(
hoverable(menufigures[i], anim=[:border], class="$(config[:colorscheme][2])";
stayactiveif=@lift($active_index == i)),
title_zstack[i];
class="justify-center align-center "
)
for i in 1:3]; class="menufigs", style=menufigs_style)
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
activeidx=active_index,
anim=[:whoop],
style="width: $(config[:resolution][1])px")
content = Dict(
:activefig => activefig,
:menufigs => menufigs_andtitles
)
return DOM.div(menufigs_andtitles, CSSMakieLayout.formatstyle, activefig), content
end
###################### 3. PLOT FUNCTIONS ######################
# These are used to configure each figure from the layout,
# meaning both the menufigures and the mainfigures.
# One can use either on whatever figure, but for the purpose
# of this project, they will be used as such
# | plot_alphafig - for the first figure (Entanglement Generation)
# | plot_betafig - for the second figure (Entanglement Swapping)
# | plot_gammafig - for the third figure (Entanglement Purification)
# , as one can see in the plot(figure_array, metas) function.
function plot_alphafig(f, meta=""; hidedecor=false)
# This is where we will do the receipe for the first figure (Entanglement Gen)
# for now this plot is taken from the tutorial
ax = Axis(f[1, 1], limits = (0, 1, 0, 1))
rs_h = IntervalSlider(f[2, 1], range = LinRange(0, 1, 1000),
startvalues = (0.2, 0.8))
rs_v = IntervalSlider(f[1, 2], range = LinRange(0, 1, 1000),
startvalues = (0.4, 0.9), horizontal = false)
labeltext1 = lift(rs_h.interval) do int
string(round.(int, digits = 2))
end
Label(f[3, 1], labeltext1, tellwidth = false)
labeltext2 = lift(rs_v.interval) do int
string(round.(int, digits = 2))
end
Label(f[1, 3], labeltext2,
tellheight = false, rotation = pi/2)
points = rand(Point2f, 300)
# color points differently if they are within the two intervals
colors = lift(rs_h.interval, rs_v.interval) do h_int, v_int
map(points) do p
(h_int[1] < p[1] < h_int[2]) && (v_int[1] < p[2] < v_int[2])
end
end
scatter!(ax, points, color = colors, colormap = [:black, :orange], strokewidth = 0)
if hidedecor
hidedecorations!(ax)
end
end
function plot_betafig(figure, meta=""; hidedecor=false)
# This is where we will do the receipe for the second figure (Entanglement Swap)
ax = Axis(figure[1, 1])
scatter!(ax, [1,2], [2,3], color=(:black, 0.2))
axx = Axis(figure[1, 2])
scatter!(axx, [1,2], [2,3], color=(:black, 0.2))
axxx = Axis(figure[2, 1:2])
scatter!(axxx, [1,2], [2,3], color=(:black, 0.2))
if hidedecor
hidedecorations!(ax)
hidedecorations!(axx)
hidedecorations!(axxx)
end
end
function plot_gammafig(figure, meta=""; hidedecor=false)
# This is where we will do the receipe for the third figure (Entanglement Purif)
ax = Axis(figure[1, 1])
scatter!(ax, [1,2], [2,3], color=(:black, 0.2))
if hidedecor
hidedecorations!(ax)
end
end
# The plot function is used to prepare the receipe (plots) for
# the mainfigures which get toggled by the identical figures in
# the menu (the menufigures), as well as for the menufigures themselves
function plot(figure_array, metas=["", "", ""]; hidedecor=false)
plot_alphafig(figure_array[1], metas[1]; hidedecor=hidedecor)
plot_betafig( figure_array[2], metas[2]; hidedecor=hidedecor)
plot_gammafig(figure_array[3], metas[3]; hidedecor=hidedecor)
end
###################### 4. LANDING PAGE OF THE APP ######################
landing = App() do session::Session
# Create the menufigures and the mainfigures
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
menufigures = [Figure(backgroundcolor=:white, resolution=config[:smallresolution]) for _ in 1:3]
titles= ["Entanglement Generation",
"Entanglement Swapping",
"Entanglement Purification"]
# Active index: 1 2 or 3
# 1: the first a.k.a alpha (Entanglement Generation) figure is active
# 2: the second a.k.a beta (Entanglement Swapping) figure is active
# 3: the third a.k.a gamma (Entanglement Purification) figure is active
activeidx = Observable(1)
hoveredidx = Observable(0)
# CLICK EVENT LISTENERS
for i in 1:3
on(events(menufigures[i]).mousebutton) do event
activeidx[]=i
notify(activeidx)
end
on(events(menufigures[i]).mouseposition) do event
hoveredidx[]=i
notify(hoveredidx)
end
# TODO: figure out when mouse leaves and set hoverableidx[] to 0
end
# Using the aforementioned plot function to plot for each figure array
plot(mainfigures)
plot(menufigures; hidedecor=true)
# Create ZStacks displayong titles below the menu graphs
titles_zstack = [zstack(wrap(DOM.h4(titles[i], class="upper")),
wrap("");
activeidx=@lift(($hoveredidx == i || $activeidx == i)),
anim=[:opacity], style="""color: $(config[:colorscheme][2]);""") for i in 1:3]
# Obtain reactive layout of the figures
layout, content = layout_content(DOM, mainfigures, menufigures, titles_zstack, session, activeidx)
# Add title to the right in the form of a ZStack
titles_div = [DOM.h1(t) for t in titles]
titles_div[1] = active(titles_div[1])
titles_div = zstack(titles_div; activeidx=activeidx, anim=[:static]
, style="""color: $(config[:colorscheme][4]);""") # static = no animation
return hstack(layout, hstack(titles_div; style="padding: 20px; margin-left: 10px;
background-color: $(config[:colorscheme][3]);"); style="width: 100%;")
end
landing2 = App() do session::Session
# Active index: 1 2 or 3
# 1: the first a.k.a alpha (Entanglement Generation) figure is active
# 2: the second a.k.a beta (Entanglement Swapping) figure is active
# 3: the third a.k.a gamma (Entanglement Purification) figure is active
activeidx = Observable(1)
hoveredidx = Observable(0)
# Create the buttons and the mainfigures
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
buttonstyle = """
background-color: $(config[:colorscheme][1]);
color: $(config[:colorscheme][2]);
border: none !important;
"""
buttons = [modifier(wrap(DOM.h1("〈")); action=:decreasecap, parameter=activeidx, cap=3, style=buttonstyle),
modifier(wrap(DOM.h1("〉")); action=:increasecap, parameter=activeidx, cap=3, style=buttonstyle)]
# Titles of the plots
titles= ["Entanglement Generation",
"Entanglement Swapping",
"Entanglement Purification"]
# Using the aforementioned plot function to plot for each figure array
plot(mainfigures)
# Obtain the reactive layout
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
activeidx=activeidx,
style="width: $(config[:resolution][1])px")
layout = hstack(buttons[1], activefig, buttons[2])
# Add title to the right in the form of a ZStack
titles_div = [DOM.h1(t) for t in titles]
titles_div[1] = active(titles_div[1])
titles_div = zstack(titles_div; activeidx=activeidx, anim=[:static],
style="""color: $(config[:colorscheme][4]);""") # static = no animation
return hstack(CSSMakieLayout.formatstyle, layout, hstack(titles_div; style="padding: 20px; margin-left: 10px;
background-color: $(config[:colorscheme][3]);"); style="width: 100%;")
end
nav = App() do session::Session
return vstack(DOM.a("LANDING", href="/1"), DOM.a("LANDING2", href="/2"))
end
##
# Serve the Makie app
isdefined(Main, :server) && close(server);
port = parse(Int, get(ENV, "QS_COLORCENTERMODCLUSTER_PORT", "8888"))
interface = get(ENV, "QS_COLORCENTERMODCLUSTER_IP", "127.0.0.1")
proxy_url = get(ENV, "QS_COLORCENTERMODCLUSTER_PROXY", "")
server = JSServe.Server(interface, port; proxy_url);
JSServe.HTTPServer.start(server)
JSServe.route!(server, "/" => nav);
JSServe.route!(server, "/1" => landing);
JSServe.route!(server, "/2" => landing2);
##
wait(server) | CSSMakieLayout | https://github.com/QuantumSavory/CSSMakieLayout.jl.git |
|
[
"MIT"
] | 0.1.1 | 6b6ed2992ac44fd72259b02b3c9f2711dce75ab4 | code | 25175 | module CSSMakieLayout
using Base.Threads
using WGLMakie
WGLMakie.activate!()
using JSServe
using Markdown
import JSServe.TailwindDashboard as D
export hstack, vstack, wrap, zstack, active,
modifier, hoverable, tie
animtoclass(anim) = join(pushfirst!([String(s) for s in anim], ""), " anim-")
cml(class) = join(["CSSMakieLayout_", class])
###################### 1. Helper functions for UX ######################
# Functions that add css classes to DOM.div elements in order to
# createa a nice UX experience and also cleaner code
"""
markdowned(figure)
Markdown wrapper that displays `figure`'s scene content. Use it when you want simpler layouts created with Markdown.
Use:
It is optional, meaning you can also wrap the figure itself in a `wrap` function. Tipically used
with Markdown pages.
"""
markdowned(figure) = md"""$(figure.scene)"""
"""
wrap(content...; class, style, md=false)
Wraps the content in a div element and sets the position of the div to `relative`.
Use it to nest elements together.
# Arguments
- `class`: classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them
"""
wrap(content...; class="", style="", md=false) = DOM.div(JSServe.MarkdownCSS,
JSServe.Styling,
md ? [markdowned(i) for i in content] : content,
style=style*"; position: relative;", class=class)
"""
_hoverable(item...; class="", style="", anim=[:default], md=false)
Wraps content in a div and adds the hoverable class to it
# Arguments
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
- `anim`: Choose which animation to perform on hover: can be set to [:default] or [:border]
"""
_hoverable(item...; class="", style="", anim=[:default], md=false) = wrap(item; class="CSSMakieLayout_hoverable "*class*" "*animtoclass(anim), style=style, md=md)
"""
hoverable(item...; stayactiveif::Observable{Bool}=Observable(false), anim=[:default], class="", style="", md=false)
Hoverable element which also stays active if the `stayactiveif` observable is set to 1. By active in the hoverable context, we mean
"to remain in the same state as when hovered".
# Arguments
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
- `anim::Array`: Choose which animations to perform on hover: can be set to [:default] or [:border] or a combination of the 2
- `stayactiveif::Observable`: If the observable set as parameter is one, the element will remain in the hovered state weather hovered or not,
otherwise it will not be in the hovered state unless hovered
"""
struct Hoverable
items::Array
attributes::Dict{Symbol, Any}
end
function attr(h::Hoverable, attribute::Symbol)
if haskey(h.attributes, attribute)
return h.attributes[attribute]
else
defaultvals = Dict(
:anim => [:default],
:class => "",
:style => "",
:md => false,
:stayactiveif => Observable(false)
)
return defaultvals[attribute]
end
end
attr(h::Hoverable) = h.attributes
hoverable(items...; kw...) = Hoverable(collect(items), Dict{Symbol, Any}(kw))
hoverable(items::Array; kw...) = Hoverable(items, Dict{Symbol, Any}(kw))
function JSServe.jsrender(session::Session, h::Hoverable)
item = [JSServe.jsrender(session, l) for l in h.items][1]
stayactiveif = attr(h, :stayactiveif)
if stayactiveif === nothing
return JSServe.jsrender(session ,_hoverable(item; class=class, style=style, md=md))
end
return JSServe.jsrender(session, selectclass(_hoverable(item; anim=attr(h, :anim),
class=attr(h, :class), style=attr(h, :style), md=attr(h, :md));
selector=stayactiveif,
toggleclasses=["CSSMakieLayout_stay", "_"]))
end
# function hoverable(item...; stayactiveif::Observable{Bool}=Observable(false), session::Session=CurrentSession, anim=[:default], class="", style="", md=false)
# if stayactiveif === nothing
# return _hoverable(item; class=class, style=style, md=md)
# end
# return selectclass(_hoverable(item; anim=anim, class=class, style=style, md=md);
# selector=stayactiveif, session=session,
# toggleclasses=["CSSMakieLayout_stay", "_"])
# end
"""
_zstack(item...; class="", style="", md=false)
A zstack receives an array/a tuple of elements, and displays just one of them based on the
`activeidx` given as parameter. _zstack is a static version of the zstack, which is used in the main [`zstack`](@ref)
implementation.
It can also be used as scaffolding for user defined behaviours.
# Arguments
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
"""
_zstack(item...; class="", style="", md=false) = wrap(item; class="CSSMakieLayout_zstack "*class, style=style)
"""
active(item...; class="", style="", md=false)
When constructing a layout, this function marks an element as 'active', i.e. topmost in a zstack.
# Arguments
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
"""
active(item...; class="", style="", md=false) = wrap(item; class="CSSMakieLayout_active "*class, style=style)
"""
struct ZStack
items::Array
attributes::Dict{Symbol, Any}
end
default attributes: activeidx::Observable=nothing,
class="", anim=[:default], style="", md=false
A zstack receives an array/a tuple of elements, and displays just one of them based on the
`activeidx` given as parameter. The displayed (active in the context of the zstack) element can be thought of as the top of the zstack
# Arguments
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
- `activeidx::Observable`: This selects the element which is displayed. For example if observable is 4,
the zstack will display the 4th element of the `item` array/tuple.
- `anim::Array`: Choose which animations to perform on transition (when `observable` is changed). Can be set to [:default], [:whoop], [:static], [:opacity] or a non-conflicting combination of them
# Example
```julia
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
activeidx=activeidx)
```
"""
struct ZStack
items::Array
attributes::Dict{Symbol, Any}
end
function attr(zstack::ZStack, attribute::Symbol)
if haskey(zstack.attributes, attribute)
return zstack.attributes[attribute]
else
defaultvals = Dict(
:anim => [:default],
:class => "",
:style => "",
:md => false,
:activeidx => nothing
)
return defaultvals[attribute]
end
end
attr(zstack::ZStack) = zstack.attributes
"""
zstack(item...; kw...)
kw... : activeidx::Observable=nothing,
class="", anim=[:default], style="", md=false
A zstack receives an array/a tuple of elements, and displays just one of them based on the
`activeidx` given as parameter (it will desplay the `activeindex`'th element). Think of it as a carousel.
The displayed (active in the context of the zstack) will represent top of the zstack
# Arguments
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
- `activeidx::Observable`: This selects the element which is displayed. For example if observable is 4,
the zstack will display the 4th element of the `item` array/tuple.
- `anim::Array`: Choose which animations to perform on transition (when `activeidx` is changed). Can be set to [:default], [:whoop], [:static], [:opacity] or a non-conflicting combination of them
# Example
```
activeidx = Observable(1)
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
activeidx=activeidx)
```
"""
zstack(items...; kw...) = ZStack(collect(items), Dict{Symbol, Any}(kw))
zstack(items::Array; kw...) = ZStack(items, Dict{Symbol, Any}(kw))
function JSServe.jsrender(session::Session, zstack::ZStack)
item = [JSServe.jsrender(session, l) for l in zstack.items]
height = size(zstack.items)
# static zstack
item_div = wrap(item...; class="CSSMakieLayout_zstack "*attr(zstack, :class)*" "*animtoclass(attr(zstack, :anim)),
style=attr(zstack, :style))
item_div = JSServe.jsrender(session, item_div)
# add on(activeidx) event
onjs(session, attr(zstack, :activeidx), js"""function on_update(new_value) {
const activefig_stack = $(item_div)
for(i = 1; i <= $(height); ++i) {
const element = activefig_stack.children.item(i-1)
element.classList.remove("CSSMakieLayout_active");
if(i == new_value) {
element.classList.add("CSSMakieLayout_active");
}
}
}
""")
return item_div
end
"""
Tie observable to divider, escaping HTML.
If no target is provided, a new element is created and returned.
"""
struct Tie
observable::Observable
target
end
tie(observable::Observable, target=nothing) = Tie(observable::Observable, target)
function JSServe.jsrender(session::Session, tie::Tie)
div = wrap("")
!isnothing(tie.target) && (div = tie.target)
onjs(session, tie.observable, js"""function on_update(new_value) {
const divider = $(div)
console.log(divider, new_value)
divider.innerHTML = new_value
}""")
JSServe.jsrender(session, div)
return div
end
# function zstack(item...; height=nothing, activeidx::Observable=nothing, session::Session=CurrentSession, class="", anim=[:default], style="", md=false)
# if activeidx === nothing
# return _zstack(item; class=class*" "*animtoclass(anim), style=style, md=md)
# else
# if height===nothing
# height=length(item)
# end
# # static zstack
# item_div = wrap(item; class="CSSMakieLayout_zstack "*class*" "*animtoclass(anim), style=style)
# # add on(activeidx) event
# onjs(session, activeidx, js"""function on_update(new_value) {
# const activefig_stack = $(item_div)
# for(i = 1; i <= $(height); ++i) {
# const element = activefig_stack.querySelector(":nth-child(" + i +")")
# element.classList.remove("CSSMakieLayout_active");
# if(i == new_value) {
# element.classList.add("CSSMakieLayout_active");
# }
# }
# }
# """)
# end
# return item_div
# end
"""
hstack(item...; class="", style="", md=false)
Displays the given elements in a flex row.
# Arguments
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them
"""
hstack(item...; class="", style="", md=false) = wrap(item; class="CSSMakieLayout_hstack "*class, style=style)
"""
vstack(item...; class="", style="", md=false)
Displays the given elements in a flex column.
# Arguments
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them
"""
vstack(item...; class="", style="", md=false) = wrap(item; class="CSSMakieLayout_vstack "*class, style=style)
"""
selectclass(item; toggleclasses=[], selector::Observable=nothing,
class="", style="", md=false)
Ads a class from the `toggleclasses` Array to the `item` element based on the value of the `selector` Observable.
Returns the modified item.
Use it when an element needs to quickly toggle between two or more classes based on the value of an observable.
A simple example would be light/dark mode selection based on an observable. This can also be used hand in hand with with a
`modifier` element.
# Arguments
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
- `toggleclasses::Array` : Array of classes to select from
- `selector::Observable`: Selects which class is added to the element.
"""
struct SelectClass
items::Array
attributes::Dict{Symbol, Any}
end
function attr(sc::SelectClass, attribute::Symbol)
if haskey(sc.attributes, attribute)
return sc.attributes[attribute]
else
defaultvals = Dict(
:toggleclasses => [],
:class => "",
:style => "",
:md => false,
:selector => nothing
)
return defaultvals[attribute]
end
end
attr(sc::SelectClass) = sc.attributes
selectclass(items...; kw...) = SelectClass(collect(items), Dict{Symbol, Any}(kw))
selectclass(items::Array; kw...) = SelectClass(items, Dict{Symbol, Any}(kw))
function JSServe.jsrender(session::Session, sc::SelectClass)
item = [JSServe.jsrender(session, l) for l in sc.items][1]
toggleclasses = attr(sc, :toggleclasses)
height = size(toggleclasses)
onjs(session, attr(sc, :selector), js"""function on_update(new_value) {
const element = $(item)
const cllist = $(toggleclasses)
cllist.forEach((el) => {
element.classList.remove(el);
})
element.classList.add(cllist[new_value-1]);
}
""")
return JSServe.jsrender(session, item)
end
# function selectclass(item; toggleclasses=[], selector::Observable=nothing, session::Session=CurrentSession, class="", style="", md=false)
# if selector === nothing
# return item
# else
# height = size(toggleclasses)
# # add on(observable) event
# onjs(session, selector, js"""function on_update(new_value) {
# const element = $(item)
# const cllist = $(toggleclasses)
# cllist.forEach((el) => {
# element.classList.remove(el);
# })
# element.classList.add(cllist[new_value-1]);
# }
# """)
# end
# return item
# end
"""
_button(item; class="", style="")
Static button with no click events added (equivalent to hoverable).
"""
_button(item; class="", style="") = hoverable(item; class=class, style=style)
"""
modifier(item; action=:toggle, parameter::Observable=nothing, class="", style="", cap=3, step=1, md=false)
Wrap an item in a clickable div (modifier element/button) and bind it to an observable. When clicked, it modifies the `parameter` Observable taken as parameter based on the button's `action`, `cap` and `step`.
`action` can be: :toggle, :increase, :decrease, :increasemod, :decreasemod
:increasecap, :decreasecap
Use it when you need to modify the value of an observale based on the number of click events on an element.
Examples could range from
- play/pause, dark/light mode (togglers)
- previous/next (decreasecap/increasecap or decreasemod/increasemod for loopback)
# Arguments
- `class`: additional classes of the modifier element in a string separated with space
- `style`: string containing the additional css style of the modifier
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
- `parameter::Observable`: Observable that is modified when a click event is triggered on the modifier.
- `action`: The way that the modifier button modifies it's `parameter` when clicked:
- `:toggle`: toggles the observable from 0 to 1, or from 1 to 0 (for example 1 - play, 0 - pause)
- `:increase`, `decrease`: increase or decrease the observable by `step`
- `:increasemod`, `decreasemod`: increase or decrease the observable by `step` nd then take the modulo w.r.t `cap` and add 1, to keep the number in the [1, cap] interval
- `:increasecap`, `:decreasecap`: increase or decrease the observable by `step`, keep it in the [1, cap] interval, but do not increase/decrease when increasing and decreasing would make the observable exit the interval (as oposed to the mod option which loops back, the cap option stays there).
- `step`, `cap`: the step of the increase/decrease steps and the maximum cappacity
# Example
The modifier element can be used hand in hand with a zstack element to create reactive layouts as such:
```julia
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
buttons = [modifier(wrap(DOM.h1("〈")); action=:decreasecap, parameter=activeidx, cap=3, style=buttonstyle),
modifier(wrap(DOM.h1("〉")); action=:increasecap, parameter=activeidx, cap=3, style=buttonstyle)]
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
observable=activeidx)
layout = hstack(buttons[1], activefig, buttons[2])
```
"""
function modifier(item; action=:toggle, parameter::Observable=nothing, class="", style="", cap=3, step=1, md=false)
if parameter === nothing
return _button(item; class=class, style=style)
end
t = D.Button(item; class=class, style=style)
on(t) do event
if action == :toggle
parameter[] = !parameter[]
elseif action == :increase
parameter[] = parameter[] + step
elseif action == :decrease
parameter[] = parameter[] - step
elseif action == :increasemod
parameter[] = parameter[] + step
if parameter[] >= cap + 1
parameter[] = 1
end
elseif action == :decreasemod
parameter[] = parameter[] - step
if parameter[] <= 0
parameter[] = cap
end
elseif action == :increasecap
parameter[] = parameter[] + step
if parameter[] >= cap + 1
parameter[] = parameter[] - step
end
elseif action == :decreasecap
parameter[] = parameter[] - step
if parameter[] <= 0
parameter[] = parameter[] + step
end
end
# notify(parameter)
end
return wrap(t; class="CSSMakieLayout_btn")
end
"""
CSSMakieLayout.formatstyle
CSS code used by the library for styling
Include it in your layout when returning the final element as such:
```julia
return hstack(CSSMakieLayout.formatstyle, layout)
```
"""
const formatstyle=DOM.style("""
.CSSMakieLayout_hoverable.anim-default{
transition: all 0.1s ease;
}
.CSSMakieLayout_hoverable.anim-default:hover, .CSSMakieLayout_stay.anim-default{
transform: scale(1.1);
}
.CSSMakieLayout_hoverable.anim-border{
transition: all 0.1s ease;
border: 2px solid transparent;
}
.CSSMakieLayout_hoverable.anim-border:hover, .CSSMakieLayout_stay.anim-border{
border: 2px solid black;
}
.CSSMakieLayout_hoverable.anim-border.white{
transition: all 0.1s ease;
border: 2px solid transparent;
padding: 4px;
padding-bottom: 0px;
}
.CSSMakieLayout_hoverable.anim-border.white:hover, .CSSMakieLayout_stay.white.anim-border{
border: 2px solid white;
}
.CSSMakieLayout_hstack{
display:flex;
flex-direction: row;
}
.CSSMakieLayout_vstack{
display: flex;
flex-direction: column;
}
.align-center{
align-items: center;
}
.justify-center{
justify-content: center;
}
.CSSMakieLayout_zstack{
display:flex;
flex-direction: row;
}
.CSSMakieLayout_zstack, .CSSMakieLayout_zstack > *{
transition: all 0.3s ease;
}
.CSSMakieLayout_zstack.anim-default .CSSMakieLayout_active{
transition: all 0.3s ease;
width: 100%;
overflow: hidden;
}
.CSSMakieLayout_zstack.anim-default > :not(.CSSMakieLayout_active){
transition: all 0.3s ease;
width: 0%;
overflow: hidden;
}
.CSSMakieLayout_zstack.anim-whoop{
display: grid;
}
.CSSMakieLayout_zstack.anim-whoop > *{
grid-area: 1/1/1/1;
}
.CSSMakieLayout_zstack.anim-whoop .CSSMakieLayout_active{
z-index: 4;
position: absolute;
transition: all 0.3s ease;
transform: scale(1);
overflow: hidden;
}
.CSSMakieLayout_zstack.anim-whoop > :not(.CSSMakieLayout_active){
position: absolute;
z-index: 1;
transition: all 0.3s ease;
transform: scale(0);
overflow: hidden;
}
.CSSMakieLayout_zstack.anim-static .CSSMakieLayout_active{
overflow: hidden;
}
.CSSMakieLayout_zstack.anim-static > :not(.CSSMakieLayout_active){
width: 0px;
overflow: hidden;
}
.CSSMakieLayout_zstack.anim-opacity .CSSMakieLayout_active{
transition: all 0.1s ease;
opacity: 1;
}
.CSSMakieLayout_zstack.anim-opacity > :not(.CSSMakieLayout_active){
transition: all 0.1s ease;
opacity: 0;
}
.upper{
text-transform: uppercase;
}
.CSSMakieLayout_btn button{
height: 100%;
width: 100%;
}
.CSSMakieLayout_btn button:hover{
box-shadow: rgba(50, 50, 93, 0.25) 0px 30px 60px -12px inset, rgba(0, 0, 0, 0.3) 0px 18px 36px -18px inset !important;
}
""")
const Themes = Dict(
:elegant => function fct(config)
return DOM.style("""
.CSSMakieLayout_btn button{
background-color: $(config[:colorscheme][1]);
color: $(config[:colorscheme][2]);
border: none !important;
}""")
end,
)
end # module CSSMakieLayout
| CSSMakieLayout | https://github.com/QuantumSavory/CSSMakieLayout.jl.git |
|
[
"MIT"
] | 0.1.1 | 6b6ed2992ac44fd72259b02b3c9f2711dce75ab4 | docs | 9874 | # CSSMakieLayout.jl
This library helps in the development of reactive frontends and can be
used alongside **WGLMakie** and **JSServe**.
## The functions you care about
Most frequently you will be using the `hstack` (row of items), `vstack` (column of items), and `zstack` functions to **create your HTML/CSS layout**. You will be wrapping your figures in HTML div tags with `wrap`.
When stacking things with `zstack` you will want to select which one is currently viewable with the `active` function and the `activeidx` keyword argument. **Transitions** between the states can also be enabled with the `anim` keyword argument. One can select `[:default]`, `[:whoop]`, `[:static]` , `[:opacity]` or a valid combination of the four.
**Hover animations** are available with the `hoverable` function with the specified `anim` keyword. One can select `[:default]`, `[:border]` or a combination of the two.
And for convenience you can create **clickable buttons** that navigate the layout with `modifier`.
### The workflow can be defined as such:
- Reactiveness centers around the `observable` objects.
- There are three kinds of CSSMakieLayout elements: **static**, **modifiers** and **modifiable**
- The **static** elements are purely for styling, with no reactive component. For example `hstack`, `vstack`, `wrap` and `hoverable` if no observable is set for the *stayactiveif* parameter
- The **modifiers** are the ones that modify the observables that in turn modity the **modifiable** elements. For now there exists only one **modifier element** that is luckily called **modifier**. It takes the observable to be modified as the `parameter` keyword, and the way in which to modify it as the `action` keyword (which can be `:toggle`, `:increase`, `:decrease`, `:increasecap`, `:decreasecap`, `:increasemod`, `:decreasemod`)
- The **modifiable** elements are the ones that get modified by an observable: `zstack`, `hoverable` with the `stayactiveif` observable set and `selectclass`
## Focus on the styling and let us handle the reactive part!
Let's go through two examples on how to use this library, the first one will be a simple one, and the second, more complex.
Example 1 | Example 2
:-------------------------:|:-------------------------:
 | 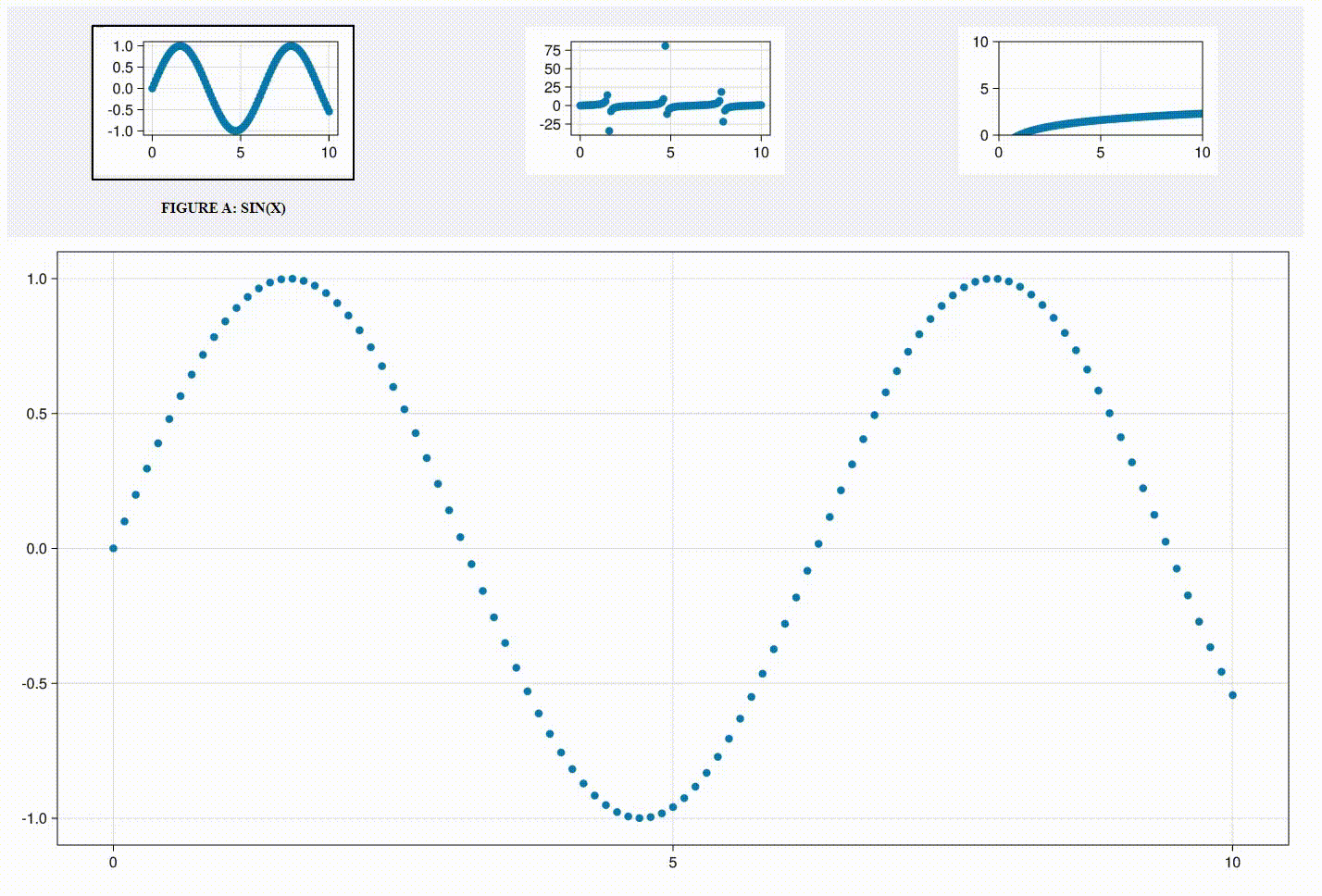
## Example 1
For example let's say we want to create a view in which we can visualize
one of three figures (**a**, **b** and **c**) in a slider manner.
We also want to control the slider with two buttons: `LEFT` and `RIGHT`. The
`RIGHT` button slided to the next figure and the `LEFT` one slides to the
figure before.
The layout would look something like this:

By acting on the buttons, one moves from one figure to the other.
### This can be easily implemented using **CSSMakieLayout.jl**
1. First of all include the library in your project
```julia
using WGLMakie
WGLMakie.activate!()
using JSServe
using Markdown
# 1. LOAD LIBRARY
using CSSMakieLayout
```
2. Then define your layout using CSSMakieLayout.jl,
```julia
config = Dict(
:resolution => (1400, 700), #used for the main figures
)
landing = App() do session::Session
CSSMakieLayout.CurrentSession = session
# Active index: 1 2 or 3
# 1: the first a.k.a 'a' figure is active
# 2: the second a.k.a 'b' figure is active
# 3: the third a.k.a 'c' figure is active
# This observable is used to communicate between the zstack and the selection menu/buttons as such: the selection buttons modify the observable which in turn, modifies the active figure zstack.
activeidx = Observable(1)
# Create the buttons and the mainfigures
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
buttons = [modifier(wrap(DOM.h1("〈")); action=:decreasecap, parameter=activeidx, cap=3),
modifier(wrap(DOM.h1("〉")); action=:increasecap, parameter=activeidx, cap=3)]
axii = [Axis(mainfigures[i][1, 1]) for i in 1:3]
# Plot each of the 3 figures using your own plots!
scatter!(axii[1], 0:0.1:10, x -> sin(x))
scatter!(axii[2], 0:0.1:10, x -> tan(x))
scatter!(axii[3], 0:0.1:10, x -> log(x))
# Obtain the reactive layout using a zstack controlled by the activeidx observable
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
activeidx=activeidx,
style="width: $(config[:resolution][1])px")
layout = hstack(buttons[1], activefig, buttons[2])
return hstack(CSSMakieLayout.formatstyle, layout)
end
```
3. And finally Serve the app
```julia
isdefined(Main, :server) && close(server);
port = 8888
interface = "127.0.0.1"
server = JSServe.Server(interface, port);
JSServe.HTTPServer.start(server)
JSServe.route!(server, "/" => landing);
# the app will run on localhost at port 8888
wait(server)
```
This code can be visualized at [./examples/example_readme](./examples/example_readme), or at [https://github.com/adrianariton/QuantumFristGenRepeater](https://github.com/adrianariton/QuantumFristGenRepeater) (this will be updated shortly with the plots of the first gen repeater)
# Example 2
This time we are going to create a selectable layout with a menu, that will look like this:
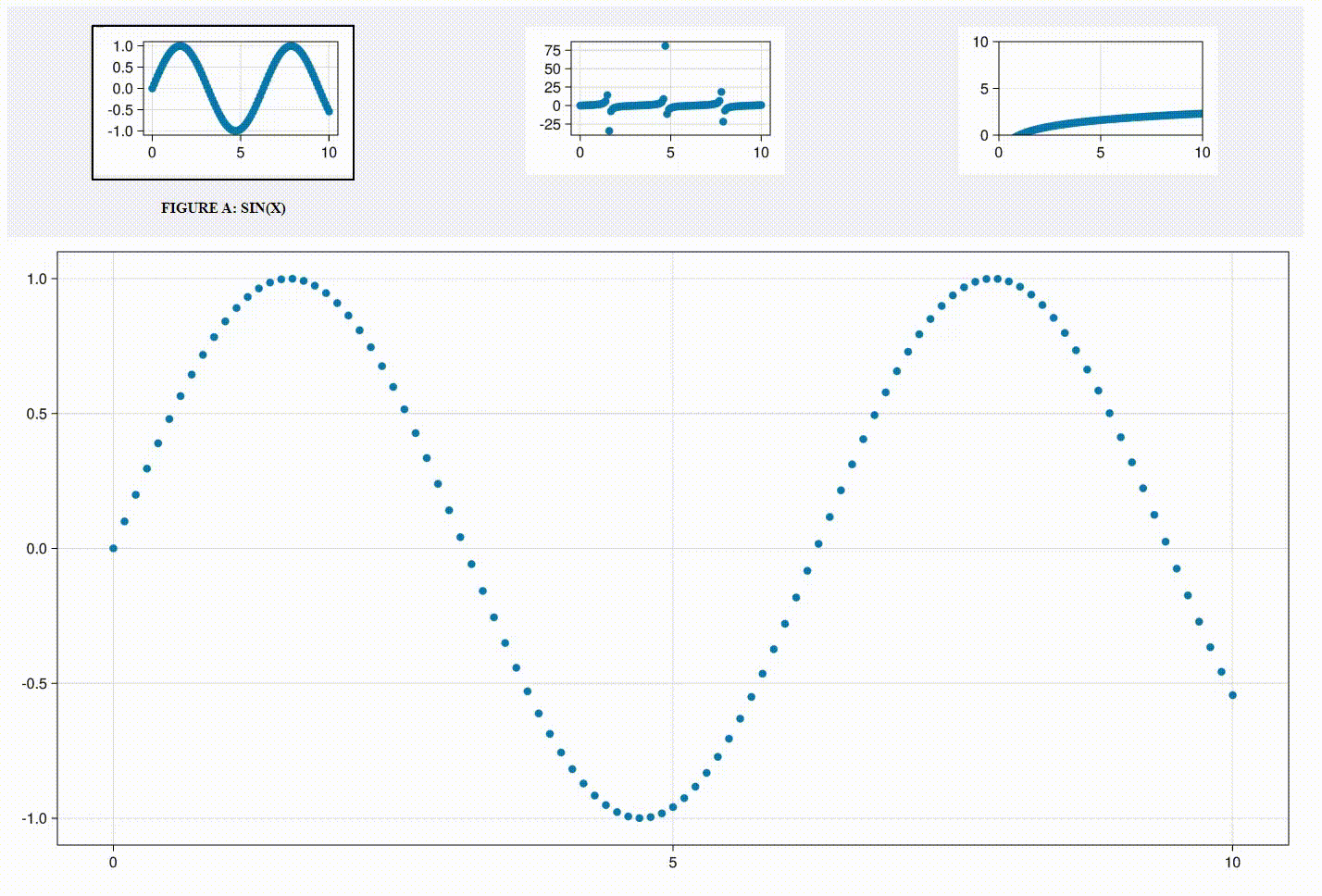
To do this we will follow the same stept, with a modified layout function:
1. First of all include the library in your project
```julia
using WGLMakie
WGLMakie.activate!()
using JSServe
using Markdown
# 1. LOAD LIBRARY
using CSSMakieLayout
```
2. Create the layout
```julia
config = Dict(
:resolution => (1400, 700), #used for the main figures
:smallresolution => (280, 160), #used for the menufigures
)
# define some additional style for the menufigures' container
menufigs_style = """
display:flex;
flex-direction: row;
justify-content: space-around;
background-color: rgb(242, 242, 247);
padding-top: 20px;
width: $(config[:resolution][1])px;
"""
landing = App() do session::Session
CSSMakieLayout.CurrentSession = session
# Create the menufigures and the mainfigures
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
menufigures = [Figure(backgroundcolor=:white, resolution=config[:smallresolution]) for _ in 1:3]
# Figure titles
titles= ["Figure a: sin(x)",
"Figure b: tan(x)",
"Figure c: cos(x)"]
# Active index/ hovered index: 1 2 or 3
# 1: the first a.k.a 'a' figure is active / hovered respectively
# 2: the second a.k.a 'b' figure is active / hovered respectively
# 3: the third a.k.a 'c' figure is active / hovered respectively
# These two observables are used to communicate between the zstack and the selection menu/buttons as such: the selection buttons modify the observables which in turn, modify the active figure zstack.
activeidx = Observable(1)
hoveredidx = Observable(0)
# Add custom click event listeners
for i in 1:3
on(events(menufigures[i]).mousebutton) do event
activeidx[]=i
notify(activeidx)
end
on(events(menufigures[i]).mouseposition) do event
hoveredidx[]=i
notify(hoveredidx)
end
end
# Axii of each of the 6 figures
main_axii = [Axis(mainfigures[i][1, 1]) for i in 1:3]
menu_axii = [Axis(menufigures[i][1, 1]) for i in 1:3]
# Plot each of the 3 figures using your own plots!
scatter!(main_axii[1], 0:0.1:10, x -> sin(x))
scatter!(main_axii[2], 0:0.1:10, x -> tan(x))
scatter!(main_axii[3], 0:0.1:10, x -> log(x))
scatter!(menu_axii[1], 0:0.1:10, x -> sin(x))
scatter!(menu_axii[2], 0:0.1:10, x -> tan(x))
scatter!(menu_axii[3], 0:0.1:10, x -> log(x))
# Create ZStacks displaying titles below the menu graphs
titles_zstack = [zstack(wrap(DOM.h4(titles[i], class="upper")),
wrap("");
activeidx=@lift(($hoveredidx == i || $activeidx == i)),
anim=[:opacity], style="""color: $(config[:colorscheme][2]);""") for i in 1:3]
# Wrap each of the menu figures and its corresponing title zstack in a div
menufigs_andtitles = wrap([
vstack(
hoverable(menufigures[i], anim=[:border];
stayactiveif=@lift($activeidx == i)),
titles_zstack[i];
class="justify-center align-center "
) for i in 1:3];
class="menufigs",
style=menufigs_style
)
# Create the active figure zstack and add the :whoop (zoom in) animation to it
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
activeidx=activeidx,
anim=[:whoop])
# Obtain reactive layout of the figures
return wrap(menufigs_andtitles, activefig, CSSMakieLayout.formatstyle)
end
```
3. And finally Serve the app
```julia
isdefined(Main, :server) && close(server);
port = 8888
interface = "127.0.0.1"
server = JSServe.Server(interface, port);
JSServe.HTTPServer.start(server)
JSServe.route!(server, "/" => landing);
# the app will run on localhost at port 8888
wait(server)
``` | CSSMakieLayout | https://github.com/QuantumSavory/CSSMakieLayout.jl.git |
|
[
"MIT"
] | 0.1.1 | 6b6ed2992ac44fd72259b02b3c9f2711dce75ab4 | docs | 8011 | # CSSMakieLayout.jl
This library helps in the development of reactive frontends and can be
used alongside **WGLMakie** and **JSServe**.
## Focus on the styling and let us handle the reactive part!
Let's go through two examples on how to use this library, the first one will be a simple one, and the second, more complex.
Example 1 | Example 2
:-------------------------:|:-------------------------:
 | 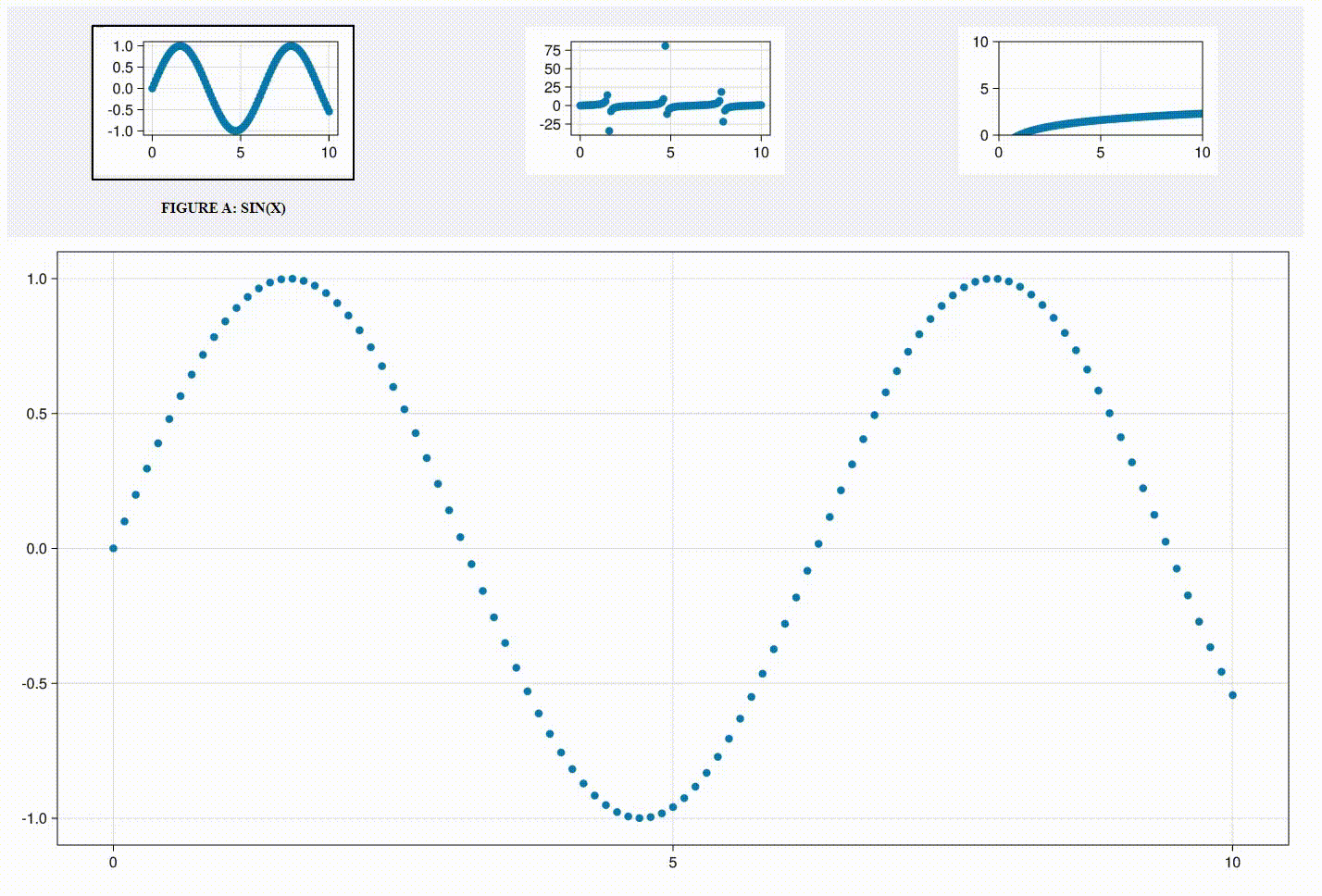
## Example 1
For example let's say we want to create a view in which we can visualize
one of three figures (**a**, **b** and **c**) in a slider manner.
We also want to control the slider with two buttons: `LEFT` and `RIGHT`. The
`RIGHT` button slided to the next figure and the `LEFT` one slides to the
figure before.
The layout would look something like this:

By acting on the buttons, one moves from one figure to the other.
### This can be easily implemented using **CSSMakieLayout.jl**
1. First of all include the library in your project
```julia
using WGLMakie
WGLMakie.activate!()
using JSServe
using Markdown
# 1. LOAD LIBRARY
using CSSMakieLayout
```
2. Then define your layout using CSSMakieLayout.jl,
```julia
config = Dict(
:resolution => (1400, 700), #used for the main figures
)
landing = App() do session::Session
CSSMakieLayout.CurrentSession = session
# Active index: 1 2 or 3
# 1: the first a.k.a 'a' figure is active
# 2: the second a.k.a 'b' figure is active
# 3: the third a.k.a 'c' figure is active
# This observable is used to communicate between the zstack and the selection menu/buttons as such: the selection buttons modify the observable which in turn, modifies the active figure zstack.
activeidx = Observable(1)
# Create the buttons and the mainfigures
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
buttons = [modifier(wrap(DOM.h1("〈")); action=:decreasecap, parameter=activeidx, cap=3),
modifier(wrap(DOM.h1("〉")); action=:increasecap, parameter=activeidx, cap=3)]
axii = [Axis(mainfigures[i][1, 1]) for i in 1:3]
# Plot each of the 3 figures using your own plots!
scatter!(axii[1], 0:0.1:10, x -> sin(x))
scatter!(axii[2], 0:0.1:10, x -> tan(x))
scatter!(axii[3], 0:0.1:10, x -> log(x))
# Obtain the reactive layout using a zstack controlled by the activeidx observable
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
activeidx=activeidx,
style="width: $(config[:resolution][1])px")
layout = hstack(buttons[1], activefig, buttons[2])
return hstack(CSSMakieLayout.formatstyle, layout)
end
```
3. And finally Serve the app
```julia
isdefined(Main, :server) && close(server);
port = 8888
interface = "127.0.0.1"
server = JSServe.Server(interface, port);
JSServe.HTTPServer.start(server)
JSServe.route!(server, "/" => landing);
# the app will run on localhost at port 8888
wait(server)
```
This code can be visualized at [./examples/example_readme](./examples/example_readme), or at [https://github.com/adrianariton/QuantumFristGenRepeater](https://github.com/adrianariton/QuantumFristGenRepeater) (this will be updated shortly with the plots of the first gen repeater)
# Example 2
This time we are going to create a selectable layout with a menu, that will look like this:
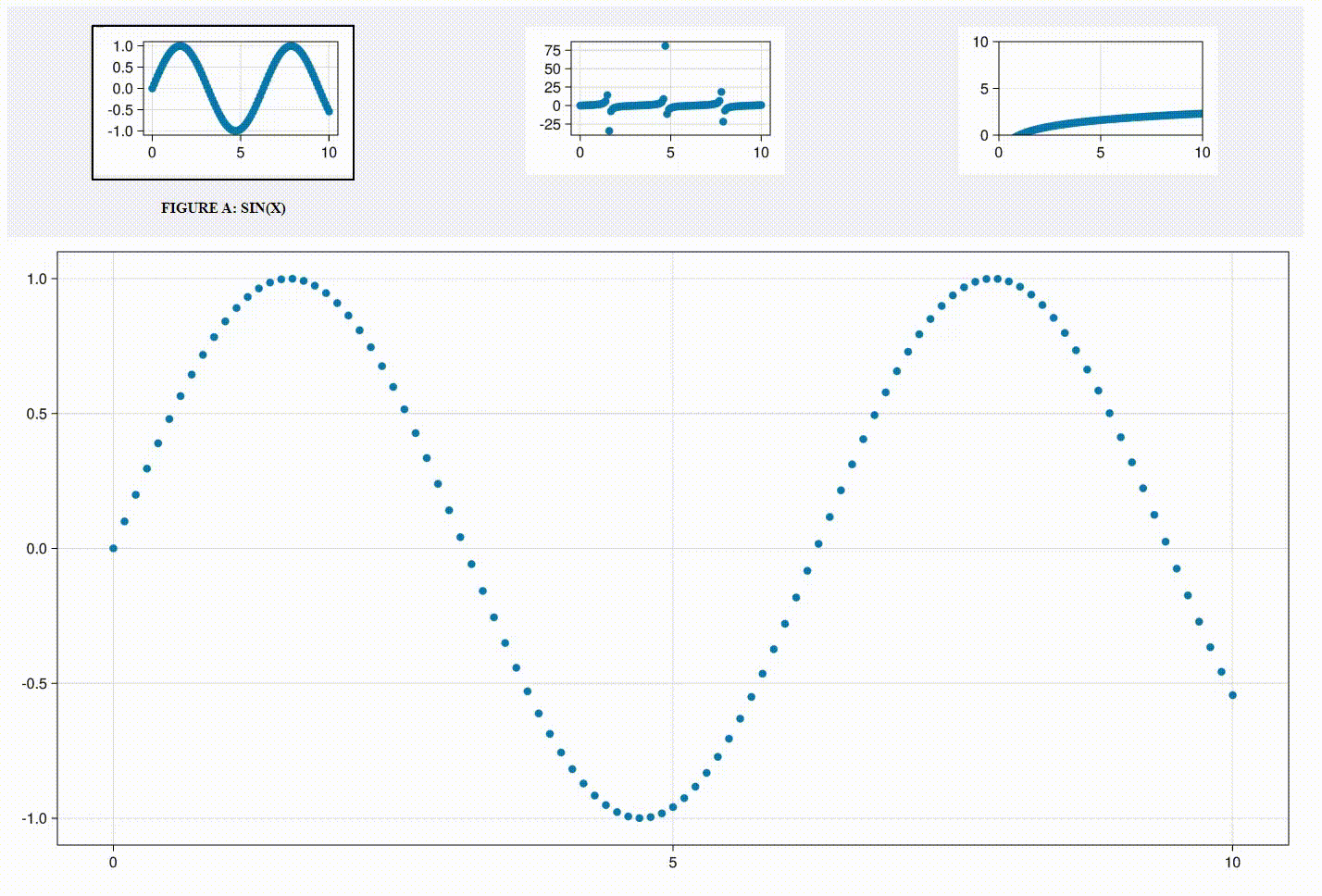
To do this we will follow the same stept, with a modified layout function:
1. First of all include the library in your project
```julia
using WGLMakie
WGLMakie.activate!()
using JSServe
using Markdown
# 1. LOAD LIBRARY
using CSSMakieLayout
```
2. Create the layout
```julia
config = Dict(
:resolution => (1400, 700), #used for the main figures
:smallresolution => (280, 160), #used for the menufigures
)
# define some additional style for the menufigures' container
menufigs_style = """
display:flex;
flex-direction: row;
justify-content: space-around;
background-color: rgb(242, 242, 247);
padding-top: 20px;
width: $(config[:resolution][1])px;
"""
landing = App() do session::Session
CSSMakieLayout.CurrentSession = session
# Create the menufigures and the mainfigures
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
menufigures = [Figure(backgroundcolor=:white, resolution=config[:smallresolution]) for _ in 1:3]
# Figure titles
titles= ["Figure a: sin(x)",
"Figure b: tan(x)",
"Figure c: cos(x)"]
# Active index/ hovered index: 1 2 or 3
# 1: the first a.k.a 'a' figure is active / hovered respectively
# 2: the second a.k.a 'b' figure is active / hovered respectively
# 3: the third a.k.a 'c' figure is active / hovered respectively
# These two observables are used to communicate between the zstack and the selection menu/buttons as such: the selection buttons modify the observables which in turn, modify the active figure zstack.
activeidx = Observable(1)
hoveredidx = Observable(0)
# Add custom click event listeners
for i in 1:3
on(events(menufigures[i]).mousebutton) do event
activeidx[]=i
notify(activeidx)
end
on(events(menufigures[i]).mouseposition) do event
hoveredidx[]=i
notify(hoveredidx)
end
end
# Axii of each of the 6 figures
main_axii = [Axis(mainfigures[i][1, 1]) for i in 1:3]
menu_axii = [Axis(menufigures[i][1, 1]) for i in 1:3]
# Plot each of the 3 figures using your own plots!
scatter!(main_axii[1], 0:0.1:10, x -> sin(x))
scatter!(main_axii[2], 0:0.1:10, x -> tan(x))
scatter!(main_axii[3], 0:0.1:10, x -> log(x))
scatter!(menu_axii[1], 0:0.1:10, x -> sin(x))
scatter!(menu_axii[2], 0:0.1:10, x -> tan(x))
scatter!(menu_axii[3], 0:0.1:10, x -> log(x))
# Create ZStacks displaying titles below the menu graphs
titles_zstack = [DOM.h4(t, class="upper") for t in titles]
for i in 1:3
titles_zstack[i] = zstack(titles_zstack[i], wrap("");
activeidx=@lift(($hoveredidx == i || $activeidx == i)),
anim=[:opacity])
end
# Wrap each of the menu figures and its corresponing title zstack in a div
menufigs_andtitles = wrap([
vstack(
hoverable(menufigures[i], anim=[:border];
stayactiveif=@lift($activeidx == i)),
titles_zstack[i];
class="justify-center align-center "
) for i in 1:3];
class="menufigs",
style=menufigs_style
)
# Create the active figure zstack and add the :whoop (zoom in) animation to it
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
activeidx=activeidx,
anim=[:whoop])
# Obtain reactive layout of the figures
return wrap(menufigs_andtitles, activefig, CSSMakieLayout.formatstyle)
end
```
3. And finally Serve the app
```julia
isdefined(Main, :server) && close(server);
port = 8888
interface = "127.0.0.1"
server = JSServe.Server(interface, port);
JSServe.HTTPServer.start(server)
JSServe.route!(server, "/" => landing);
# the app will run on localhost at port 8888
wait(server)
``` | CSSMakieLayout | https://github.com/QuantumSavory/CSSMakieLayout.jl.git |
|
[
"MIT"
] | 0.1.1 | 6b6ed2992ac44fd72259b02b3c9f2711dce75ab4 | docs | 11874 | # Reactive and static elements
## CSSMakieLayout.CurrentSession
Session used as default for all session::Session params of the following functions. Set it at the begining of your code as such:
```julia
landing2 = App() do session::Session
CSSMakieLayout.CurrentSession = session
...
end
```
## markdowned
```julia
markdowned(figure)
```
Markdown wrapper that displays `figure`'s scene content.
Use:
It is optional,
meaning you can also wrap the figure itself in a `wrap` function. Tipically used
with Markdown pages.
# wrap
```julia
wrap(content...; class, style, md=false)
```
Wraps the content in a div element and sets the position of the div to `relative`.
**Arguments**
- `class`: classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them
## _hoverable
```julia
_hoverable(item...; class="", style="", anim=[:default], md=false)
```
Wraps content in a div and adds the hoverable class to it
**Arguments**
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
- `anim`: Choose which animation to perform on hover: can be set to [:default] or [:border]
## hoverable
```julia
hoverable(item...; stayactiveif::Observable=nothing, session::Session=CurrentSession, anim=[:default], class="", style="", md=false)
```
Hoverable element which also stays active if the `stayactiveif` observable is set to 1. By active, we mean
"to remain in the same state as when hovered".
**Arguments**
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
- `anim::Array`: Choose which animations to perform on hover: can be set to [:default] or [:border] or a combination of the 2
- `stayactiveif::Observable`: If the observable set as parameter is one, the element will be active weather hovered or not,
otherwise it will not be active unless hovered
- `session::Session=CurrentSession`: App session (defaults to CSSMakieLayout.CurrentSession which can be set at the begining. See [`CurrentSession`](@ref))
## _zstack
```julia
_zstack(item...; class="", style="", md=false)
```
A zstack receives an array/a tuple of elements, and displays just one of them based on the
`activeidx` given as parameter. _zstack is a static version of the zstack, which is used in the main [`zstack`](@ref)
implementation.
It can also be used as scaffolding for user defined behaviours.
**Arguments**
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
## active
```julia
active(item...; class="", style="", md=false)
```
When constructing a layout, this function marks an element as 'active', i.e. topmost in a zstack.
**Arguments**
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
## zstack
```julia
zstack(item::Array; activeidx::Observable=nothing, session::Session=CurrentSession, class="", anim=[:default], style="", md=false)
```
A zstack receives an array/a tuple of elements, and displays just one of them based on the
`activeidx` given as parameter. The displayed (active in the context of the zstack) element can be thought of as the top of the zstack
# Arguments
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
- `activeidx::Observable`: This selects the element which is displayed. For example if observable is 4,
the zstack will display the 4th element of the `item` array/tuple.
- `anim::Array`: Choose which animations to perform on transition (when `observable` is changed). Can be set to [:default], [:whoop], [:static], [:opacity] or a non-conflicting combination of them
- `session::Session=CurrentSession`: App session (defaults to CSSMakieLayout.CurrentSession which can be set at the begining. See [`CurrentSession`](@ref))
# Example
```julia
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
activeidx=activeidx)
```
## zstack
```julia
zstack(item...; activeidx::Observable=nothing, session::Session=CurrentSession, class="", anim=[:default], style="", md=false)
```
A zstack receives an array/a tuple of elements, and displays just one of them based on the
`activeidx` given as parameter (it will desplay the `activeindex`'th element). Think of it as a carousel.
The displayed (active in the context of the zstack) will represent top of the zstack
# Arguments
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
- `activeidx::Observable`: This selects the element which is displayed. For example if observable is 4,
the zstack will display the 4th element of the `item` array/tuple.
- `anim::Array`: Choose which animations to perform on transition (when `activeidx` is changed). Can be set to [:default], [:whoop], [:static], [:opacity] or a non-conflicting combination of them
- `session::Session=CurrentSession`: App session (defaults to CSSMakieLayout.CurrentSession which can be set at the begining. See [`CurrentSession`](@ref))
# Example
```julia
activeidx = Observable(1)
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
activeidx=activeidx)
```
## hstack
```julia
hstack(item...; class="", style="", md=false)
```
Displays the given elements in a flex row.
**Arguments**
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them
## vstack
```julia
vstack(item...; class="", style="", md=false)
```
Displays the given elements in a flex column.
**Arguments**
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them
## selectclass
```julia
selectclass(item; toggleclasses=[], selector::Observable=nothing, session::Session=CurrentSession, class="", style="", md=false)
```
Ads a class from the `toggleclasses` Array to the `item` element based on the value of the `selector` Observable.
Returns the modified item.
**Arguments**
- `class`: additional classes of the element in a string separated with space
- `style`: string containing the additional css style of the wrapper div
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
- `toggleclasses::Array` : Array of classes to select from
- `selector::Observable`: Selects which class is added to the element.
- `session::Session=CurrentSession`: App session (defaults to CSSMakieLayout.CurrentSession which can be set at the begining. See [`CurrentSession`](@ref))
## _button
```julia
_button(item; class="", style="")
```
Static button with no click events added (equivalent to hoverable).
## modifier
```julia
modifier(item; action=:toggle, parameter::Observable=nothing, class="", style="", cap=3, step=1, md=false)
```
Wrap an item in a clickable div (modifier element/button) and bind it to an observable. When clicked, it modifies the `parameter` Observable taken as parameter based on the button's `action`, `cap` and `step`.
`action` can be: :toggle, :increase, :decrease, :increasemod, :decreasemod
:increasecap, :decreasecap
**Arguments**
- `class`: additional classes of the modifier element in a string separated with space
- `style`: string containing the additional css style of the modifier
- `md`: Set to false unless specified otherwise. Specifies weather to aply the [`markdowned`](@ref)
function to each element of the content parameter before wrapping them.
- `parameter::Observable`: Observable that is modified when a click event is triggered on the modifier.
- `action`: The way that the modifier button modifies it's `parameter` when clicked:
- `:toggle`: toggles the observable from 0 to 1, or from 1 to 0 (for example 1 - play, 0 - pause)
- `:increase`, `decrease`: increase or decrease the observable by `step`
- `:increasemod`, `decreasemod`: increase or decrease the observable by `step` nd then take the modulo w.r.t `cap` and add 1, to keep the number in the [1, cap] interval
- `:increasecap`, `:decreasecap`: increase or decrease the observable by `step`, keep it in the [1, cap] interval, but do not increase/decrease when increasing and decreasing would make the observable exit the interval (as oposed to the mod option which loops back, the cap option stays there).
- `step`, `cap`: the step of the increase/decrease steps and the maximum cappacity
### Example
The modifier element can be used hand in hand with a zstack element to create reactive layouts as such:
```julia
mainfigures = [Figure(backgroundcolor=:white, resolution=config[:resolution]) for _ in 1:3]
buttons = [modifier(wrap(DOM.h1("〈")); action=:decreasecap, parameter=activeidx, cap=3, style=buttonstyle),
modifier(wrap(DOM.h1("〉")); action=:increasecap, parameter=activeidx, cap=3, style=buttonstyle)]
activefig = zstack(
active(mainfigures[1]),
wrap(mainfigures[2]),
wrap(mainfigures[3]);
observable=activeidx)
layout = hstack(buttons[1], activefig, buttons[2])
```
## CSSMakieLayout.formatstyle
CSS code used by the library for styling
Include it in your layout when returning the final element as such:
```julia
return hstack(CSSMakieLayout.formatstyle, layout)
```
## CSSMakieLayout.Themes
Some basic themes for abstract styling that are still in development
| CSSMakieLayout | https://github.com/QuantumSavory/CSSMakieLayout.jl.git |
|
[
"MIT"
] | 0.10.2 | 592e97807d90904fd94a681a4bd1976edc41c0df | code | 1782 | using PostNewtonian
using Symbolics # To document the extension
using Documenter
DocMeta.setdocmeta!(
PostNewtonian, :DocTestSetup, :(using PostNewtonian); recursive=true, warn=false
)
const page_rename = Dict("developer.md" => "Developer docs") # Without the numbers
makedocs(;
modules=[
PostNewtonian, PostNewtonian.FundamentalVariables, PostNewtonian.DerivedVariables
],
authors="Michael Boyle <[email protected]> and contributors",
repo=Remotes.GitHub("moble", "PostNewtonian.jl"),
#repo = "https://github.com/moble/PostNewtonian.jl/blob/{commit}{path}#{line}",
sitename="PostNewtonian.jl",
format=Documenter.HTML(;
prettyurls=get(ENV, "CI", "false") == "true",
canonical="https://moble.github.io/PostNewtonian.jl/stable/",
assets=String[],
),
pages=[
"Introduction" => "index.md",
"Interface" => [
"interface/high-level.md",
"Units" => "interface/units.md",
#"interface/symbolics.md",
"interface/differentiation.md",
"interface/assorted_binaries.md",
"Python" => "interface/python.md",
"GWFrames" => "interface/gwframes.md",
],
"Internals" => [
"Code structure" => "internals/code_structure.md",
"internals/pn_systems.md",
"internals/fundamental_variables.md",
"internals/derived_variables.md",
"internals/pn_expressions.md",
"internals/dynamics.md",
"Waveforms" => "internals/waveforms.md",
"internals/utilities.md",
],
"Adding terms" => "adding_terms.md",
],
)
deploydocs(; repo="github.com/moble/PostNewtonian.jl", devbranch="main", push_preview=true)
| PostNewtonian | https://github.com/moble/PostNewtonian.jl.git |
|
[
"MIT"
] | 0.10.2 | 592e97807d90904fd94a681a4bd1976edc41c0df | code | 10016 | module PostNewtonianSymbolicsExt
# See ../../src/predefinitions_Symbolics.jl for a few predefinitions of things that really
# only exist here, but will be needed elsewhere. The documentation evidently needs to
# occur there as well.
using MacroTools: MacroTools
using SymbolicUtils: SymbolicUtils
isdefined(Base, :get_extension) ? (using Symbolics: Symbolics) : (import ..Symbolics)
using PostNewtonian
import PostNewtonian:
hold,
unhold,
SymbolicPNSystem,
type_converter,
fundamental_quaternionic_variables,
derived_variables,
causes_domain_error!,
prepare_pn_order,
order_index,
𝓔′,
apply_to_first_add!,
flatten_add!,
flatten_mul!,
pn_expression,
pn_expansion,
@pn_expansion,
M₁,
M₂,
χ⃗₁,
χ⃗₂,
v,
Φ,
Λ₁,
Λ₂,
R,
M,
μ,
ν,
δ,
q,
ℳ,
X₁,
X₂,
ln,
ln2,
ln3,
ln5,
ζ3,
γₑ,
_efficient_vector
#apply_to_first_add!, flatten_add!, pn_expression,
using RuntimeGeneratedFunctions: init, @RuntimeGeneratedFunction
init(@__MODULE__)
function _efficient_vector(N, ::Type{Symbolics.Num})
return Symbolics.variables(string(gensym()), 1:N)
end
### Moved from src/utilities/macros.jl
hold(x) = x
Symbolics.@register_symbolic hold(x)
Symbolics.derivative(::typeof(hold), args::NTuple{1,Any}, ::Val{1}) = 1
function unhold(expr)
MacroTools.postwalk(expr) do x
m = MacroTools.trymatch(:(f_(i_)), x)
m === nothing || m[:f] !== hold ? x : Symbol(m[:i])
end
end
function type_converter(::PNSystem{T}, x) where {T<:Vector{Symbolics.Num}}
return Symbolics.Num(SymbolicUtils.Term(hold, [x]))
end
function type_converter(::PNSystem{T}, x::Symbolics.Num) where {T<:Vector{Symbolics.Num}}
return x
end
# Add symbolic capabilities to all derived variables (fundamental variables already work)
for method ∈ [fundamental_quaternionic_variables; derived_variables]
name = method.name
@eval begin
function PostNewtonian.$name(v::PNSystem{T}) where {T<:Vector{Symbolics.Num}}
return Symbolics.Num(SymbolicUtils.Sym{Real}(Symbol($name)))
end
function PostNewtonian.$name(v::Vector{T}) where {T<:Symbolics.Num}
return Symbolics.Num(SymbolicUtils.Sym{Real}(Symbol($name)))
end
end
end
"""
extract_var_factor(term, var)
Extract a factor of `var` from the product `term`.
This is a helper function for [`var_collect`](@ref).
"""
function extract_var_factor(term, var)
if MacroTools.isexpr(term, :call) && term.args[1] ∈ ((/), :/)
k₂, term₂ = extract_var_factor(term.args[2], var)
k₃, term₃ = extract_var_factor(term.args[3], var)
return k₂ - k₃, Expr(:call, term.args[1], term₂, term₃)
#return k₂-k₃, :($(term.args[1]), $term₂, $term₃)
end
if !MacroTools.isexpr(term, :call) || term.args[1] ∉ ((*), :*)
if term == var
return 1, 1
end
m = MacroTools.trymatch(:((^)(v_, k_)), term)
m = !isnothing(m) ? m : MacroTools.trymatch(:($(^)(v_, k_)), term)
if !isnothing(m) && m[:v] == var
return m[:k], 1
end
return 0, term
end
term = flatten_mul!(deepcopy(term))
k = 0
indices = Int[]
for (i, factor) ∈ enumerate(term.args)
if i == 1
continue # Skip the :*
end
if MacroTools.isexpr(factor, :call)
k′, term′ = extract_var_factor(factor, var)
# if term′ isa Expr
# term′ = Expr(:call, term′.args...)
# end
if k′ > 0
k += k′
term.args[i] = term′
end
else
if factor == var
k += 1
push!(indices, i)
continue
end
m = MacroTools.trymatch(:((^)(v_, k_)), factor)
m = !isnothing(m) ? m : MacroTools.trymatch(:($(^)(v_, k_)), factor)
if !isnothing(m) && m[:v] == var
k += m[:k]
push!(indices, i)
end
end
end
if !isempty(indices)
splice!(term.args, indices)
end
# TODO: If there's just 1 arg left over, return it alone
if length(term.args) == 2
k, term.args[2]
else
k, term
end
end
"""
var_collect(expr, var)
Collect coefficients in `expr` of various powers of the variable `var`.
The inputs should be an
[`Expr`](https://docs.julialang.org/en/v1/manual/metaprogramming/#Program-representation)
and a single `Symbol` to be found in that `Expr`.
The return value is an `Int` representing the highest power of `v` in the expression, and an
`Expr` representing a `Tuple` of values corresponding to the coefficients of `var` to
various powers. For example,
```jl-doctest
julia> PostNewtonian.var_collect(:(1 + a*v + b*v^2 + c*v^4), :v)
4, :((1, a, b, 0, c))
```
(Note that there was *no* factor in `v^3`.) This result is convenient for passing to
`evalpoly`, for example.
"""
function var_collect(expr, var)
if !MacroTools.isexpr(expr, :call)
error("Input expression is not a call at its highest level: $expr")
end
terms = Dict{Int,Any}()
if expr.args[1] ∉ ((+), :+, (-), :-)
k, term = extract_var_factor(expr, var)
terms[k] = term
else
for (i, term) ∈ enumerate(expr.args[2:end])
k, term = extract_var_factor(term, var)
if expr.args[1] ∈ ((-), :-) && i == 2
if k ∈ keys(terms)
terms[k] = :($(terms[k]) - $(term))
else
terms[k] = :(-$(term))
end
else
if k ∈ keys(terms)
terms[k] = :($(terms[k]) + $(term))
else
terms[k] = term
end
end
end
end
max_k = maximum(keys(terms))
term_exprs = [get(terms, k, 0) for k ∈ 0:max_k]
return max_k, :(($(term_exprs...),))
end
function var_collect(expr::Symbolics.Num, var; max_power=100, max_gap=4)
expr = SymbolicUtils.expand(expr)
dict = Dict(var^j => 0 for j ∈ 1:max_power)
c = SymbolicUtils.substitute(expr, dict; fold=false)
expr = expr - c
coefficients = [c]
gap = 0
for i ∈ 1:max_power
dict[var^i] = 1
if i > 1
dict[var^(i - 1)] = 0
end
push!(coefficients, Symbolics.substitute(expr, dict; fold=false))
if iszero(coefficients[end])
gap += 1
if gap ≥ max_gap
return coefficients[1:(end - gap)]
end
else
gap = 0
end
end
return coefficients
end
macro pn_expansion(offset, pnsystem, expr)
return esc(pn_expansion(offset, pnsystem, expr))
end
function pn_expansion(offset, pnsystem, expr)
max_k, coefficients = var_collect(expr, :v)
max_index = max_k + 1
if offset != 0
max_index_var = gensym("max_index")
quote
$max_index_var = min($max_index, order_index($pnsystem) - $offset)
if $max_index_var < 1
zero(v)
else
evalpoly(v, $coefficients[1:($max_index_var)])
end
end
else
:(evalpoly(v, $coefficients[1:min($max_index, order_index($pnsystem))]))
end
end
## Moved from src/pn_systems.jl
causes_domain_error!(u̇, ::PNSystem{VT}) where {VT<:Vector{Symbolics.Num}} = false
function SymbolicPNSystem(PNOrder=typemax(Int))
Symbolics.@variables M₁ M₂ χ⃗₁ˣ χ⃗₁ʸ χ⃗₁ᶻ χ⃗₂ˣ χ⃗₂ʸ χ⃗₂ᶻ Rʷ Rˣ Rʸ Rᶻ v Φ Λ₁ Λ₂
ET = typeof(M₁)
return SymbolicPNSystem{Vector{ET},prepare_pn_order(PNOrder),ET}(
[M₁, M₂, χ⃗₁ˣ, χ⃗₁ʸ, χ⃗₁ᶻ, χ⃗₂ˣ, χ⃗₂ʸ, χ⃗₂ᶻ, Rʷ, Rˣ, Rʸ, Rᶻ, v, Φ], Λ₁, Λ₂
)
end
"""
symbolic_pnsystem
A symbolic `PNSystem` that contains symbolic information for all types of `PNSystem`s.
In particular, note that this object has an (essentially) infinite `PNOrder` and has nonzero
values for quantities like `Λ₁` and `Λ₂`. If you want different choices, you may need to
call [`SymbolicPNSystem`](@ref) yourself, or even construct a different specialized subtype
of `PNSystem` (it's not hard).
# Examples
```jldoctest
julia> using PostNewtonian: M₁, M₂, χ⃗₁, χ⃗₂
julia> M₁(symbolic_pnsystem), M₂(symbolic_pnsystem)
(M₁, M₂)
julia> χ⃗₁(symbolic_pnsystem)
χ⃗₁
julia> χ⃗₂(symbolic_pnsystem)
χ⃗₂
```
"""
const symbolic_pnsystem = SymbolicPNSystem()
## Moved from src/fundamental_variables.jl
Λ₁(pn::SymbolicPNSystem) = pn.Λ₁
Λ₂(pn::SymbolicPNSystem) = pn.Λ₂
## Moved from src/pn_expressions/binding_energy.jl with a little padding to distinguish it
## from the new FastDifferentiation-based version
const 𝓔′Symbolics = let 𝓔 = 𝓔(symbolic_pnsystem), v = v(symbolic_pnsystem)
∂ᵥ = Symbolics.Differential(v)
# Evaluate derivative symbolically
𝓔′ = SymbolicUtils.simplify(Symbolics.expand_derivatives(∂ᵥ(𝓔)); expand=true)#, simplify_fractions=false)
# Turn it into (an Expr of) a function taking one argument: `pnsystem`
𝓔′ = Symbolics.build_function(𝓔′, :pnsystem; nanmath=false)
# Remove `hold` (which we needed for Symbolics.jl to not collapse to Float64)
𝓔′ = unhold(𝓔′)
# "Flatten" the main sum, because Symbolics nests sums for some reason
𝓔′ = apply_to_first_add!(𝓔′, flatten_add!)
# Apply `@pn_expansion` to the main sum
splitfunc = MacroTools.splitdef(𝓔′)
splitfunc[:body] = apply_to_first_add!(splitfunc[:body], x -> :(@pn_expansion(-1, $x)))
𝓔′ = MacroTools.combinedef(splitfunc)
# Finally, apply the "macro" to it and get a full function out
@RuntimeGeneratedFunction(pn_expression(1, 𝓔′))
end
function 𝓔′(
pnsystem::PNSystem{ST,PNOrder},
::Val{:Symbolics};
pn_expansion_reducer::Val{PNExpansionReducer}=Val(sum),
) where {ST,PNOrder,PNExpansionReducer}
if PNExpansionReducer != sum
error("Symbolic 𝓔′ is not implemented for PNExpansionReducer other than `sum`")
else
𝓔′Symbolics(pnsystem)
end
end
end #module
| PostNewtonian | https://github.com/moble/PostNewtonian.jl.git |
|
[
"MIT"
] | 0.10.2 | 592e97807d90904fd94a681a4bd1976edc41c0df | code | 545 | # Run this script from any directory as
#
# julia -t 4 scripts/docs.jl
#
# The docs will build and the browser should open automatically. `LiveServer`
# will monitor the docs for any changes, then rebuild them and refresh the browser
# until this script is stopped.
using Dates: Dates
println("Building docs starting at ", Dates.format(Dates.now(), "HH:MM:SS"), ".")
using Pkg
#using Revise # Doesn't enable updates of docstrings in the output.
cd((@__DIR__) * "/..")
Pkg.activate("docs")
using LiveServer
servedocs(; launch_browser=true)
| PostNewtonian | https://github.com/moble/PostNewtonian.jl.git |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.