title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
⏳ [VIDEO] O(m log k) Maximizing Computer Run Time with Binary Search | maximum-running-time-of-n-computers | 1 | 1 | # Intuition\nWhen I first read this problem, I realized that it\'s not just about using each battery to its full capacity before moving on to the next. The goal here is to maximize the running time of all computers, and a simple linear approach would not achieve that. The challenge here lies in striking a balance between using batteries that have the most power and ensuring that no energy is wasted.\n\n## Video Explenation & Live Coding\nhttps://youtu.be/_qFXyY5vBIY\n\n# Approach\nThe approach I took for this problem is to find a balance between using batteries that have the most power and ensuring that no energy is wasted. I employed a binary search strategy to locate the maximum possible running time.\n\nInitially, I set 1 as the left boundary and the total power of all batteries divided by \'n\' as the right boundary. This is because 1 is the minimum time a computer can run (given the constraints that battery power is at least 1) and `sum(batteries) / n` is the maximum time all computers can run if we could distribute the power of all batteries evenly among the computers.\n\nIn the binary search, I set a target running time and checked if we could reach this target with the available batteries. This check is done using the line of code `if sum(min(battery, target) for battery in batteries) >= target * n:`. \n\nThe expression `min(battery, target) for battery in batteries` calculates how much of each battery\'s power we can use to achieve the target running time. If a battery\'s power is more than the target, we can only use power equal to the target from it. If a battery\'s power is less than the target, we can use all of its power. The sum of these amounts is the total power we can use to achieve the target running time.\n\nIf this total power is greater than or equal to `target * n` (the total power needed to run all `n` computers for `target` time), it means we can achieve the target running time with the available batteries, so I update the left boundary to the target.\n\nIf we couldn\'t achieve the target running time (i.e., the total power is less than `target * n`), I update the right boundary to one less than the target. I continue this process until the left and right boundaries meet, at which point we\'ve found the maximum possible running time.\n\n# Complexity\n- Time complexity: The time complexity for this problem is \\(O(m log k)\\), where \\(m\\) is the length of the input array batteries and \\(k\\) is the maximum power of one battery.\n\n- Space complexity: The space complexity for this problem is \\(O(1)\\). During the binary search, we only need to record the boundaries of the searching space and the power extra, and the accumulative sum of extra, which only takes constant space.\n\n# Code\n```Python []\nclass Solution:\n def maxRunTime(self, n: int, batteries: List[int]) -> int:\n left, right = 1, sum(batteries) // n \n while left < right: \n target = right - (right - left) // 2 \n if sum(min(battery, target) for battery in batteries) >= target * n: \n left = target \n else: \n right = target - 1 \n return left \n```\n``` C++ []\nclass Solution {\npublic:\n long long maxRunTime(int n, std::vector<int>& batteries) {\n std::sort(batteries.begin(), batteries.end());\n long long left = 1, right = std::accumulate(batteries.begin(), batteries.end(), 0LL) / n;\n while (left < right) {\n long long target = right - (right - left) / 2;\n long long total = std::accumulate(batteries.begin(), batteries.end(), 0LL, [&target](long long a, int b) { return a + std::min(static_cast<long long>(b), target); });\n if (total >= target * n) {\n left = target;\n } else {\n right = target - 1;\n }\n }\n return left;\n }\n};\n```\n``` Java []\npublic class Solution {\n public long maxRunTime(int n, int[] batteries) {\n Arrays.sort(batteries);\n long left = 1, right = (long)Arrays.stream(batteries).asLongStream().sum() / n;\n while (left < right) {\n long target = right - (right - left) / 2;\n long total = Arrays.stream(batteries).asLongStream().map(battery -> Math.min(battery, target)).sum();\n if (total >= target * n) {\n left = target;\n } else {\n right = target - 1;\n }\n }\n return left;\n }\n}\n```\n``` C# []\npublic class Solution {\n public long MaxRunTime(int n, int[] batteries) {\n Array.Sort(batteries);\n long left = 1, right = (long)batteries.Sum(b => (long)b) / n;\n while (left < right) {\n long target = right - (right - left) / 2;\n long total = batteries.Sum(battery => Math.Min((long)battery, target));\n if (total >= target * n) {\n left = target;\n } else {\n right = target - 1;\n }\n }\n return left;\n }\n}\n```\n``` JavaScript []\n/**\n * @param {number} n\n * @param {number[]} batteries\n * @return {number}\n */\nvar maxRunTime = function(n, batteries) {\n batteries.sort((a, b) => a - b);\n let left = 1, right = Math.floor(batteries.reduce((a, b) => a + b) / n);\n while (left < right) {\n let target = right - Math.floor((right - left) / 2);\n let total = batteries.reduce((a, b) => a + Math.min(b, target), 0);\n if (total >= target * n) {\n left = target;\n } else {\n right = target - 1;\n }\n }\n return left;\n};\n``` \n## Video Python & JavaScript\n\nhttps://youtu.be/10BC-5zKrb0 \nhttps://youtu.be/xnkXF1Yed94 \n | 31 | You have `n` computers. You are given the integer `n` and a **0-indexed** integer array `batteries` where the `ith` battery can **run** a computer for `batteries[i]` minutes. You are interested in running **all** `n` computers **simultaneously** using the given batteries.
Initially, you can insert **at most one battery** into each computer. After that and at any integer time moment, you can remove a battery from a computer and insert another battery **any number of times**. The inserted battery can be a totally new battery or a battery from another computer. You may assume that the removing and inserting processes take no time.
Note that the batteries cannot be recharged.
Return _the **maximum** number of minutes you can run all the_ `n` _computers simultaneously._
**Example 1:**
**Input:** n = 2, batteries = \[3,3,3\]
**Output:** 4
**Explanation:**
Initially, insert battery 0 into the first computer and battery 1 into the second computer.
After two minutes, remove battery 1 from the second computer and insert battery 2 instead. Note that battery 1 can still run for one minute.
At the end of the third minute, battery 0 is drained, and you need to remove it from the first computer and insert battery 1 instead.
By the end of the fourth minute, battery 1 is also drained, and the first computer is no longer running.
We can run the two computers simultaneously for at most 4 minutes, so we return 4.
**Example 2:**
**Input:** n = 2, batteries = \[1,1,1,1\]
**Output:** 2
**Explanation:**
Initially, insert battery 0 into the first computer and battery 2 into the second computer.
After one minute, battery 0 and battery 2 are drained so you need to remove them and insert battery 1 into the first computer and battery 3 into the second computer.
After another minute, battery 1 and battery 3 are also drained so the first and second computers are no longer running.
We can run the two computers simultaneously for at most 2 minutes, so we return 2.
**Constraints:**
* `1 <= n <= batteries.length <= 105`
* `1 <= batteries[i] <= 109` | Could you generate all the different numbers comprised of only digit1 and digit2 with the constraints? Going from least to greatest, check if the number you generated is greater than k and a multiple of k. |
Python3 Solution | maximum-running-time-of-n-computers | 0 | 1 | \n```\nclass Solution:\n def maxRunTime(self, n: int, batteries: List[int]) -> int:\n left=0\n right=sum(batteries)//n+1\n def check(time):\n return sum(min(time,b) for b in batteries)>=n*time\n\n while left<=right:\n mid=(left+right)//2\n if check(mid):\n left=mid+1\n\n else:\n right=mid-1\n\n return right \n``` | 6 | You have `n` computers. You are given the integer `n` and a **0-indexed** integer array `batteries` where the `ith` battery can **run** a computer for `batteries[i]` minutes. You are interested in running **all** `n` computers **simultaneously** using the given batteries.
Initially, you can insert **at most one battery** into each computer. After that and at any integer time moment, you can remove a battery from a computer and insert another battery **any number of times**. The inserted battery can be a totally new battery or a battery from another computer. You may assume that the removing and inserting processes take no time.
Note that the batteries cannot be recharged.
Return _the **maximum** number of minutes you can run all the_ `n` _computers simultaneously._
**Example 1:**
**Input:** n = 2, batteries = \[3,3,3\]
**Output:** 4
**Explanation:**
Initially, insert battery 0 into the first computer and battery 1 into the second computer.
After two minutes, remove battery 1 from the second computer and insert battery 2 instead. Note that battery 1 can still run for one minute.
At the end of the third minute, battery 0 is drained, and you need to remove it from the first computer and insert battery 1 instead.
By the end of the fourth minute, battery 1 is also drained, and the first computer is no longer running.
We can run the two computers simultaneously for at most 4 minutes, so we return 4.
**Example 2:**
**Input:** n = 2, batteries = \[1,1,1,1\]
**Output:** 2
**Explanation:**
Initially, insert battery 0 into the first computer and battery 2 into the second computer.
After one minute, battery 0 and battery 2 are drained so you need to remove them and insert battery 1 into the first computer and battery 3 into the second computer.
After another minute, battery 1 and battery 3 are also drained so the first and second computers are no longer running.
We can run the two computers simultaneously for at most 2 minutes, so we return 2.
**Constraints:**
* `1 <= n <= batteries.length <= 105`
* `1 <= batteries[i] <= 109` | Could you generate all the different numbers comprised of only digit1 and digit2 with the constraints? Going from least to greatest, check if the number you generated is greater than k and a multiple of k. |
Binary_Search.py | maximum-running-time-of-n-computers | 0 | 1 | # Code\n```\nclass Solution:\n def maxRunTime(self, n: int, b: List[int]) -> int:\n def check(n,mid):\n return sum(mid if i>mid else i for i in b)/n>=mid\n i,j=0,10**20\n while i<j:\n mid=(i+j)//2\n if check(n,mid):\n i=mid+1\n else:\n j=mid\n return i-1\n```\n\n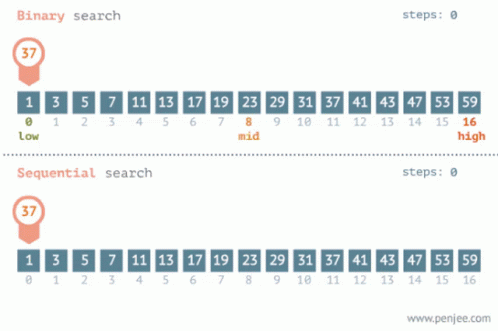 | 3 | You have `n` computers. You are given the integer `n` and a **0-indexed** integer array `batteries` where the `ith` battery can **run** a computer for `batteries[i]` minutes. You are interested in running **all** `n` computers **simultaneously** using the given batteries.
Initially, you can insert **at most one battery** into each computer. After that and at any integer time moment, you can remove a battery from a computer and insert another battery **any number of times**. The inserted battery can be a totally new battery or a battery from another computer. You may assume that the removing and inserting processes take no time.
Note that the batteries cannot be recharged.
Return _the **maximum** number of minutes you can run all the_ `n` _computers simultaneously._
**Example 1:**
**Input:** n = 2, batteries = \[3,3,3\]
**Output:** 4
**Explanation:**
Initially, insert battery 0 into the first computer and battery 1 into the second computer.
After two minutes, remove battery 1 from the second computer and insert battery 2 instead. Note that battery 1 can still run for one minute.
At the end of the third minute, battery 0 is drained, and you need to remove it from the first computer and insert battery 1 instead.
By the end of the fourth minute, battery 1 is also drained, and the first computer is no longer running.
We can run the two computers simultaneously for at most 4 minutes, so we return 4.
**Example 2:**
**Input:** n = 2, batteries = \[1,1,1,1\]
**Output:** 2
**Explanation:**
Initially, insert battery 0 into the first computer and battery 2 into the second computer.
After one minute, battery 0 and battery 2 are drained so you need to remove them and insert battery 1 into the first computer and battery 3 into the second computer.
After another minute, battery 1 and battery 3 are also drained so the first and second computers are no longer running.
We can run the two computers simultaneously for at most 2 minutes, so we return 2.
**Constraints:**
* `1 <= n <= batteries.length <= 105`
* `1 <= batteries[i] <= 109` | Could you generate all the different numbers comprised of only digit1 and digit2 with the constraints? Going from least to greatest, check if the number you generated is greater than k and a multiple of k. |
Beats 100% || Clear and concise explanation || No Binary Search No heap || | maximum-running-time-of-n-computers | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nTry to reach a state where all computers have same runtime by interchanging batteries, or it is not possible to Reach such state because of certain bottleneck batteries in your store.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n----------------------------------------------------------------------\n**If you do not want to Read this big of a thought provoking approach, please Look at the code it has necessary comments for all lines.**\n\n----------------------------------------------------------------------\nSort the Batteris as only the lower value batteries can stop you from getting more runtime, so use your higher value batteries first.\nnow declare the **higher n batteries** as your current active batteries(say **inuse** =list of **n** higher value batteries.).\nWe also have the rest of the batteries sum of whose capacity is say **Bank_capacity.**\nso the **bottleneck in your current inuse is the lowest value battery in your inuse list.**\nI have sorted the batteries in reverse order so my lowest value battery is my index no n-1;\nso my current bottleneck is at index no **i=n-1**\nI will take a variavle c(stands for count) that would store how many such bottleneck batteries I have in my active batteries list currently.**After counting the number of such batteries , find the nearest battery that is bigger than this bottleneck battery(or batteries).** To equalise or get over this current bottleneck **we must \ngive all such bottleneck batteries a power equal to the difference between this nearest higher capacity battery and the bottleneck batteries**(say this total is called Total power needed). Repeat this process untill all active batteries have the **same capacity**(say all n have x capacity), or it is not possible to give all of the bottleneck batteries a power equal to this Total power needed. in this case distibute whatever avilavle capacity is left in our Bank_capacity by making a **integer divison** of this capacity by number of bottleneck_batteries ,now return the bottleneck capacity+this distributed value.\n\n\nIf all batteries have the same capacity distribute whatever power is left in my Bank_capacity in all n of the batteries.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nSay we have M number of Batteris and N number of computers.\nSorting takes O(MlogM) time by conventional sorting methods.\nthe while loops together can run only n times till Bank_capacity is available so this has complexity of O(N).\nTotal time complexity is O(MlogM)+O(N)==O(MlogM)\n\n**This part I am unsure about, if you find any mistake in this calculation, please comment your approach.**\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nRequires O(n) of extra space but can easily be modified to run with \nO(1) by not storing the inuse list of computers but using the batteries array instead.\n\n# Code\n```\nclass Solution:\n def maxRunTime(self, n: int, batteries: List[int]) -> int:\n batteries.sort(reverse=True)\n inuse=batteries[:n] #Batties in use by active computers\n bank=sum(batteries[n:]) #stores total resedue power\n i=n-1 #index of my current bottleneck before start\n c=0 #Total number of bottleneck batteries\n current_power=sum(inuse) #Stores the amount of power inuse by all active computers currently\n while bank>0 and current_power!=n*inuse[0]:#stop when bank is empty or all computers have same batteries\n bottle_neck=inuse[i] #the current ith battery is our bottleneck\n while i>=0 and inuse[i]==bottle_neck: #count the number of bottlenecks\n c+=1\n i-=1\n power_per_battery=inuse[i]-bottle_neck #calculate the amount of power our current bottleneck- \n power_needed=power_per_battery*(c) #-batteries need\n if power_needed>bank: # if bank does not have thst much power distribute the\n return inuse[i+1]+bank//c # leftover power equally to all bottleneck batteries\n else:\n bank-=power_needed #Else Battery has the Required power so \n current_power+=power_needed #decrese the power in the bank and increase the current consumption\n return inuse[i]+bank//n \n\n\n\n\n\n \n\n``` | 1 | You have `n` computers. You are given the integer `n` and a **0-indexed** integer array `batteries` where the `ith` battery can **run** a computer for `batteries[i]` minutes. You are interested in running **all** `n` computers **simultaneously** using the given batteries.
Initially, you can insert **at most one battery** into each computer. After that and at any integer time moment, you can remove a battery from a computer and insert another battery **any number of times**. The inserted battery can be a totally new battery or a battery from another computer. You may assume that the removing and inserting processes take no time.
Note that the batteries cannot be recharged.
Return _the **maximum** number of minutes you can run all the_ `n` _computers simultaneously._
**Example 1:**
**Input:** n = 2, batteries = \[3,3,3\]
**Output:** 4
**Explanation:**
Initially, insert battery 0 into the first computer and battery 1 into the second computer.
After two minutes, remove battery 1 from the second computer and insert battery 2 instead. Note that battery 1 can still run for one minute.
At the end of the third minute, battery 0 is drained, and you need to remove it from the first computer and insert battery 1 instead.
By the end of the fourth minute, battery 1 is also drained, and the first computer is no longer running.
We can run the two computers simultaneously for at most 4 minutes, so we return 4.
**Example 2:**
**Input:** n = 2, batteries = \[1,1,1,1\]
**Output:** 2
**Explanation:**
Initially, insert battery 0 into the first computer and battery 2 into the second computer.
After one minute, battery 0 and battery 2 are drained so you need to remove them and insert battery 1 into the first computer and battery 3 into the second computer.
After another minute, battery 1 and battery 3 are also drained so the first and second computers are no longer running.
We can run the two computers simultaneously for at most 2 minutes, so we return 2.
**Constraints:**
* `1 <= n <= batteries.length <= 105`
* `1 <= batteries[i] <= 109` | Could you generate all the different numbers comprised of only digit1 and digit2 with the constraints? Going from least to greatest, check if the number you generated is greater than k and a multiple of k. |
python 3 - binary search, greedy | maximum-running-time-of-n-computers | 0 | 1 | # Intuition\nObviously you need to try different time t. This part requires binary search. Nothing special. For each binary search iteration, you need to check "if a certain t can be achieved". This is the hardest part.\n\nReverse sort the array first. Sum up bat[n:]. If sum > 0, you can rearrange this sum to any battery in bat[:n]. You don\'t need to know exactly how to arrange. If the sum can distribute to bat[:n] to achieve time t, return True. Else, return False. This part is greedy.\n\n# Approach\nBinary search + greedy\n\n# Complexity\n- Time complexity:\nO(nlogk) -> Do bs operation within a time range.\n\n- Space complexity:\nO(n) -> the sorted array\n\nn = given n\nk = sum(bat) // n -> right boundary\n\n# Code\n```\nclass Solution:\n def maxRunTime(self, n: int, bat: List[int]) -> int:\n\n def ok(time): # return bool, ok?\n if bat[n-1] >= time:\n return True\n \n remain = sum(bat[n:])\n for i in range(n-1, -1, -1):\n if bat[i] < time:\n remain -= time - bat[i]\n return remain >= 0\n\n bat.sort(reverse = True)\n \n l, r = 1, sum(bat) // n\n\n while l < r:\n m = (l + r) // 2\n if ok(m):\n l = m + 1\n else:\n r = m\n\n return l if ok(l) else l - 1\n``` | 1 | You have `n` computers. You are given the integer `n` and a **0-indexed** integer array `batteries` where the `ith` battery can **run** a computer for `batteries[i]` minutes. You are interested in running **all** `n` computers **simultaneously** using the given batteries.
Initially, you can insert **at most one battery** into each computer. After that and at any integer time moment, you can remove a battery from a computer and insert another battery **any number of times**. The inserted battery can be a totally new battery or a battery from another computer. You may assume that the removing and inserting processes take no time.
Note that the batteries cannot be recharged.
Return _the **maximum** number of minutes you can run all the_ `n` _computers simultaneously._
**Example 1:**
**Input:** n = 2, batteries = \[3,3,3\]
**Output:** 4
**Explanation:**
Initially, insert battery 0 into the first computer and battery 1 into the second computer.
After two minutes, remove battery 1 from the second computer and insert battery 2 instead. Note that battery 1 can still run for one minute.
At the end of the third minute, battery 0 is drained, and you need to remove it from the first computer and insert battery 1 instead.
By the end of the fourth minute, battery 1 is also drained, and the first computer is no longer running.
We can run the two computers simultaneously for at most 4 minutes, so we return 4.
**Example 2:**
**Input:** n = 2, batteries = \[1,1,1,1\]
**Output:** 2
**Explanation:**
Initially, insert battery 0 into the first computer and battery 2 into the second computer.
After one minute, battery 0 and battery 2 are drained so you need to remove them and insert battery 1 into the first computer and battery 3 into the second computer.
After another minute, battery 1 and battery 3 are also drained so the first and second computers are no longer running.
We can run the two computers simultaneously for at most 2 minutes, so we return 2.
**Constraints:**
* `1 <= n <= batteries.length <= 105`
* `1 <= batteries[i] <= 109` | Could you generate all the different numbers comprised of only digit1 and digit2 with the constraints? Going from least to greatest, check if the number you generated is greater than k and a multiple of k. |
Beats 100% in runtime and 98% in memory | maximum-running-time-of-n-computers | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution(object):\n def maxRunTime(self, n, batteries):\n m = len(batteries)\n if n==m:\n return min(batteries)\n if m < n:\n return 0\n batteries.sort()\n S = sum(batteries)\n T = S//n\n for i in range(1,n):\n S -= batteries[-i]\n T = min(T, S//(n-i))\n return T \n``` | 1 | You have `n` computers. You are given the integer `n` and a **0-indexed** integer array `batteries` where the `ith` battery can **run** a computer for `batteries[i]` minutes. You are interested in running **all** `n` computers **simultaneously** using the given batteries.
Initially, you can insert **at most one battery** into each computer. After that and at any integer time moment, you can remove a battery from a computer and insert another battery **any number of times**. The inserted battery can be a totally new battery or a battery from another computer. You may assume that the removing and inserting processes take no time.
Note that the batteries cannot be recharged.
Return _the **maximum** number of minutes you can run all the_ `n` _computers simultaneously._
**Example 1:**
**Input:** n = 2, batteries = \[3,3,3\]
**Output:** 4
**Explanation:**
Initially, insert battery 0 into the first computer and battery 1 into the second computer.
After two minutes, remove battery 1 from the second computer and insert battery 2 instead. Note that battery 1 can still run for one minute.
At the end of the third minute, battery 0 is drained, and you need to remove it from the first computer and insert battery 1 instead.
By the end of the fourth minute, battery 1 is also drained, and the first computer is no longer running.
We can run the two computers simultaneously for at most 4 minutes, so we return 4.
**Example 2:**
**Input:** n = 2, batteries = \[1,1,1,1\]
**Output:** 2
**Explanation:**
Initially, insert battery 0 into the first computer and battery 2 into the second computer.
After one minute, battery 0 and battery 2 are drained so you need to remove them and insert battery 1 into the first computer and battery 3 into the second computer.
After another minute, battery 1 and battery 3 are also drained so the first and second computers are no longer running.
We can run the two computers simultaneously for at most 2 minutes, so we return 2.
**Constraints:**
* `1 <= n <= batteries.length <= 105`
* `1 <= batteries[i] <= 109` | Could you generate all the different numbers comprised of only digit1 and digit2 with the constraints? Going from least to greatest, check if the number you generated is greater than k and a multiple of k. |
Python solution. Array sorting. Beats 100% | maximum-running-time-of-n-computers | 0 | 1 | 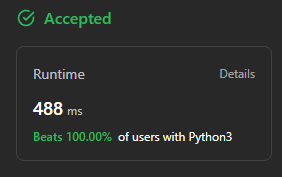\n# Complexity\n- Time complexity:\n$$O(m\u22C5log\u2061m)$$\n\n- Space complexity:\n$$O(m)$$\n\n\n# Code\n```\nclass Solution:\n def maxRunTime(self, n: int, batteries: List[int]) -> int:\n\n # array sorting\n batteries.sort()\n\n # getting the energy of all extra batteries\n extra = sum(batteries[:-n])\n\n # earlier we sorted an array to get n the largest batteries that our computers will use\n live = batteries[-n:]\n\n # iterate over an array live\n for i in range(n - 1):\n \n # if extra has enough energy for our batteries (indexed from 0 to i), we take energy from extra\n # if hasn\'t, return the value of smallest battery + all energy that can be provided for one battery\n if extra // (i + 1) > live[i + 1] - live[i]: \n extra -= (i + 1) * (live[i + 1] - live[i])\n else:\n return live[i] + extra // (i + 1) \n \n # if we got there, all batteries have equal energy, so we return the last battery with remaining energy, provided for all batteries\n return live[-1] + extra // n\n\n\n\n\n``` | 1 | You have `n` computers. You are given the integer `n` and a **0-indexed** integer array `batteries` where the `ith` battery can **run** a computer for `batteries[i]` minutes. You are interested in running **all** `n` computers **simultaneously** using the given batteries.
Initially, you can insert **at most one battery** into each computer. After that and at any integer time moment, you can remove a battery from a computer and insert another battery **any number of times**. The inserted battery can be a totally new battery or a battery from another computer. You may assume that the removing and inserting processes take no time.
Note that the batteries cannot be recharged.
Return _the **maximum** number of minutes you can run all the_ `n` _computers simultaneously._
**Example 1:**
**Input:** n = 2, batteries = \[3,3,3\]
**Output:** 4
**Explanation:**
Initially, insert battery 0 into the first computer and battery 1 into the second computer.
After two minutes, remove battery 1 from the second computer and insert battery 2 instead. Note that battery 1 can still run for one minute.
At the end of the third minute, battery 0 is drained, and you need to remove it from the first computer and insert battery 1 instead.
By the end of the fourth minute, battery 1 is also drained, and the first computer is no longer running.
We can run the two computers simultaneously for at most 4 minutes, so we return 4.
**Example 2:**
**Input:** n = 2, batteries = \[1,1,1,1\]
**Output:** 2
**Explanation:**
Initially, insert battery 0 into the first computer and battery 2 into the second computer.
After one minute, battery 0 and battery 2 are drained so you need to remove them and insert battery 1 into the first computer and battery 3 into the second computer.
After another minute, battery 1 and battery 3 are also drained so the first and second computers are no longer running.
We can run the two computers simultaneously for at most 2 minutes, so we return 2.
**Constraints:**
* `1 <= n <= batteries.length <= 105`
* `1 <= batteries[i] <= 109` | Could you generate all the different numbers comprised of only digit1 and digit2 with the constraints? Going from least to greatest, check if the number you generated is greater than k and a multiple of k. |
JavaScript && Python [Clean, Short and Simple solution] (With Explanation) | maximum-running-time-of-n-computers | 0 | 1 | # JavaScript\n```js\nvar maxRunTime = function(n, batteries) {\n batteries.sort((a, b) => a - b); // Sort\n let total = batteries.reduce((a, b) => a + b, 0); // Sum of all\n\n // If last battery is very high\n // (So that if remaining battery is shared by remaining computers still less than last battery)\n while (batteries.at(-1) > total / n)\n --n, total -= batteries.pop();\n\n return Math.floor(total / n);\n};\n```\n\n# Python\n```py\nclass Solution:\n def maxRunTime(self, n, batteries):\n batteries.sort() # Sort\n total = sum(batteries) # Sum of all\n\n # If last battery is very high\n # (So that if remaining battery is shared by remaining computers still less than last battery)\n while batteries[-1] > total / n:\n n -= 1\n total -= batteries.pop()\n\n return total // n\n``` | 1 | You have `n` computers. You are given the integer `n` and a **0-indexed** integer array `batteries` where the `ith` battery can **run** a computer for `batteries[i]` minutes. You are interested in running **all** `n` computers **simultaneously** using the given batteries.
Initially, you can insert **at most one battery** into each computer. After that and at any integer time moment, you can remove a battery from a computer and insert another battery **any number of times**. The inserted battery can be a totally new battery or a battery from another computer. You may assume that the removing and inserting processes take no time.
Note that the batteries cannot be recharged.
Return _the **maximum** number of minutes you can run all the_ `n` _computers simultaneously._
**Example 1:**
**Input:** n = 2, batteries = \[3,3,3\]
**Output:** 4
**Explanation:**
Initially, insert battery 0 into the first computer and battery 1 into the second computer.
After two minutes, remove battery 1 from the second computer and insert battery 2 instead. Note that battery 1 can still run for one minute.
At the end of the third minute, battery 0 is drained, and you need to remove it from the first computer and insert battery 1 instead.
By the end of the fourth minute, battery 1 is also drained, and the first computer is no longer running.
We can run the two computers simultaneously for at most 4 minutes, so we return 4.
**Example 2:**
**Input:** n = 2, batteries = \[1,1,1,1\]
**Output:** 2
**Explanation:**
Initially, insert battery 0 into the first computer and battery 2 into the second computer.
After one minute, battery 0 and battery 2 are drained so you need to remove them and insert battery 1 into the first computer and battery 3 into the second computer.
After another minute, battery 1 and battery 3 are also drained so the first and second computers are no longer running.
We can run the two computers simultaneously for at most 2 minutes, so we return 2.
**Constraints:**
* `1 <= n <= batteries.length <= 105`
* `1 <= batteries[i] <= 109` | Could you generate all the different numbers comprised of only digit1 and digit2 with the constraints? Going from least to greatest, check if the number you generated is greater than k and a multiple of k. |
100% time complexity, 85% space complexity. m log(m) sorting solution. | maximum-running-time-of-n-computers | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nYou want to allocate batteries in the most efficient way possible to get the maximum usage. \n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe key concept is that by using the n largest batteries, you can ensure that rest of the batteries can be used to its maximum without having to charge multiple computers at the same time. For example, if our batteries is [10, 10, 8] with n = 2. We start with [10, 10] as our starting batteries. half of the 8 can be used in the first 4 seconds then rest can be used in the later 4 seconds. Since the third battery can never exceed the two batteries, we can distribute all of 8. If there are more than one excess batteries, the amount that needs to be allocated is the largest difference between any excess batteries. Again, this cannot exceed any of the starting batteries, so we can be 100% sure all of the excess batteries can be used.\n\nCase 1: There are more computers than batteries\nNot all computers can run simulataneously, return 0\n\nCase 2: There are same amount of batteries as computers\nReturn the minimum battery as once that battery runs out, one of the computer cannot run anymore.\n\nCase 3: There are more batteries than computers\nThis is when the distribution needs to occur. We pick the n largest batteries and set them as our starting batteries. Since we know that the rest of the batteries can be used to its maximum, we can simply sum them. Starting from the first start battery, check if we have enough batteries left so that we can reach the next smallest starting battery. Remember that we need to multiply the difference between the next start battery and current start battery with amount of start batteries we have visited.\nIn the case where amount of batteries you need to get to the next starting battery exceeds the amount of batteries you have left, return the current starting battery + maximum ammount of batteries you can distribute to the starting batteries you have visited. For example, if you have [10, 7, 5, 4] and n = 3, you can use 2 out of 4 to get 5 to 7, making the starting batteries [10, 7, 7] and left = 2. Since left is not enough to make both 7 go upto 10, we distribute the 2 to the 7s and return 7+1 = 8.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nSorting requires $$O(m log(m))$$ where m = amount of batteries and iteration of the starting batteries require $$O(n)$$. Since n < m, time complexity is $$O(m log(m))$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(m)$$ needed for sorting\n# Code\n```\nclass Solution:\n def maxRunTime(self, n: int, batteries: List[int]) -> int:\n l = len(batteries)\n if n > l:\n return 0\n if n == l:\n return min(batteries)\n batteries.sort()\n curbatteries = []\n for _ in range (n):\n curbatteries.append(batteries.pop())\n left = sum(batteries)\n curbatteries.reverse()\n for i in range(n-1):\n need = (curbatteries[i+1]-curbatteries[i])*(i+1)\n if left < need:\n return curbatteries[i]+left//(i+1)\n left-=need\n return curbatteries[-1] + left//n\n \n\n\n``` | 3 | You have `n` computers. You are given the integer `n` and a **0-indexed** integer array `batteries` where the `ith` battery can **run** a computer for `batteries[i]` minutes. You are interested in running **all** `n` computers **simultaneously** using the given batteries.
Initially, you can insert **at most one battery** into each computer. After that and at any integer time moment, you can remove a battery from a computer and insert another battery **any number of times**. The inserted battery can be a totally new battery or a battery from another computer. You may assume that the removing and inserting processes take no time.
Note that the batteries cannot be recharged.
Return _the **maximum** number of minutes you can run all the_ `n` _computers simultaneously._
**Example 1:**
**Input:** n = 2, batteries = \[3,3,3\]
**Output:** 4
**Explanation:**
Initially, insert battery 0 into the first computer and battery 1 into the second computer.
After two minutes, remove battery 1 from the second computer and insert battery 2 instead. Note that battery 1 can still run for one minute.
At the end of the third minute, battery 0 is drained, and you need to remove it from the first computer and insert battery 1 instead.
By the end of the fourth minute, battery 1 is also drained, and the first computer is no longer running.
We can run the two computers simultaneously for at most 4 minutes, so we return 4.
**Example 2:**
**Input:** n = 2, batteries = \[1,1,1,1\]
**Output:** 2
**Explanation:**
Initially, insert battery 0 into the first computer and battery 2 into the second computer.
After one minute, battery 0 and battery 2 are drained so you need to remove them and insert battery 1 into the first computer and battery 3 into the second computer.
After another minute, battery 1 and battery 3 are also drained so the first and second computers are no longer running.
We can run the two computers simultaneously for at most 2 minutes, so we return 2.
**Constraints:**
* `1 <= n <= batteries.length <= 105`
* `1 <= batteries[i] <= 109` | Could you generate all the different numbers comprised of only digit1 and digit2 with the constraints? Going from least to greatest, check if the number you generated is greater than k and a multiple of k. |
Python | 6 Lines of Code | Easy to Understand | Hard Problem | Maximum Running Time of N Computers | maximum-running-time-of-n-computers | 0 | 1 | # Python | 6 Lines of Code | Easy to Understand | Hard Problem | 2141. Maximum Running Time of N Computers\n```\nclass Solution:\n def maxRunTime(self, n: int, batteries: List[int]) -> int:\n batteries.sort()\n total = sum(batteries)\n while batteries[-1] > total//n:\n n -= 1\n total -= batteries.pop()\n return total//n\n``` | 16 | You have `n` computers. You are given the integer `n` and a **0-indexed** integer array `batteries` where the `ith` battery can **run** a computer for `batteries[i]` minutes. You are interested in running **all** `n` computers **simultaneously** using the given batteries.
Initially, you can insert **at most one battery** into each computer. After that and at any integer time moment, you can remove a battery from a computer and insert another battery **any number of times**. The inserted battery can be a totally new battery or a battery from another computer. You may assume that the removing and inserting processes take no time.
Note that the batteries cannot be recharged.
Return _the **maximum** number of minutes you can run all the_ `n` _computers simultaneously._
**Example 1:**
**Input:** n = 2, batteries = \[3,3,3\]
**Output:** 4
**Explanation:**
Initially, insert battery 0 into the first computer and battery 1 into the second computer.
After two minutes, remove battery 1 from the second computer and insert battery 2 instead. Note that battery 1 can still run for one minute.
At the end of the third minute, battery 0 is drained, and you need to remove it from the first computer and insert battery 1 instead.
By the end of the fourth minute, battery 1 is also drained, and the first computer is no longer running.
We can run the two computers simultaneously for at most 4 minutes, so we return 4.
**Example 2:**
**Input:** n = 2, batteries = \[1,1,1,1\]
**Output:** 2
**Explanation:**
Initially, insert battery 0 into the first computer and battery 2 into the second computer.
After one minute, battery 0 and battery 2 are drained so you need to remove them and insert battery 1 into the first computer and battery 3 into the second computer.
After another minute, battery 1 and battery 3 are also drained so the first and second computers are no longer running.
We can run the two computers simultaneously for at most 2 minutes, so we return 2.
**Constraints:**
* `1 <= n <= batteries.length <= 105`
* `1 <= batteries[i] <= 109` | Could you generate all the different numbers comprised of only digit1 and digit2 with the constraints? Going from least to greatest, check if the number you generated is greater than k and a multiple of k. |
Simple python solution | minimum-cost-of-buying-candies-with-discount | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimumCost(self, cost: List[int]) -> int:\n sum=0\n cost.sort(reverse=True)\n print(cost)\n for i in range(len(cost)):\n if (i+1)%3==0 and i!=0:\n pass\n else:\n sum=sum+cost[i]\n return sum\n\n``` | 5 | A shop is selling candies at a discount. For **every two** candies sold, the shop gives a **third** candy for **free**.
The customer can choose **any** candy to take away for free as long as the cost of the chosen candy is less than or equal to the **minimum** cost of the two candies bought.
* For example, if there are `4` candies with costs `1`, `2`, `3`, and `4`, and the customer buys candies with costs `2` and `3`, they can take the candy with cost `1` for free, but not the candy with cost `4`.
Given a **0-indexed** integer array `cost`, where `cost[i]` denotes the cost of the `ith` candy, return _the **minimum cost** of buying **all** the candies_.
**Example 1:**
**Input:** cost = \[1,2,3\]
**Output:** 5
**Explanation:** We buy the candies with costs 2 and 3, and take the candy with cost 1 for free.
The total cost of buying all candies is 2 + 3 = 5. This is the **only** way we can buy the candies.
Note that we cannot buy candies with costs 1 and 3, and then take the candy with cost 2 for free.
The cost of the free candy has to be less than or equal to the minimum cost of the purchased candies.
**Example 2:**
**Input:** cost = \[6,5,7,9,2,2\]
**Output:** 23
**Explanation:** The way in which we can get the minimum cost is described below:
- Buy candies with costs 9 and 7
- Take the candy with cost 6 for free
- We buy candies with costs 5 and 2
- Take the last remaining candy with cost 2 for free
Hence, the minimum cost to buy all candies is 9 + 7 + 5 + 2 = 23.
**Example 3:**
**Input:** cost = \[5,5\]
**Output:** 10
**Explanation:** Since there are only 2 candies, we buy both of them. There is not a third candy we can take for free.
Hence, the minimum cost to buy all candies is 5 + 5 = 10.
**Constraints:**
* `1 <= cost.length <= 100`
* `1 <= cost[i] <= 100` | Could you keep track of the minimum element visited while traversing? We have a potential candidate for the answer if the prefix min is lesser than nums[i]. |
Beats 100% | 0ms | Greedy 🚀🔥 | minimum-cost-of-buying-candies-with-discount | 1 | 1 | # Intuition\n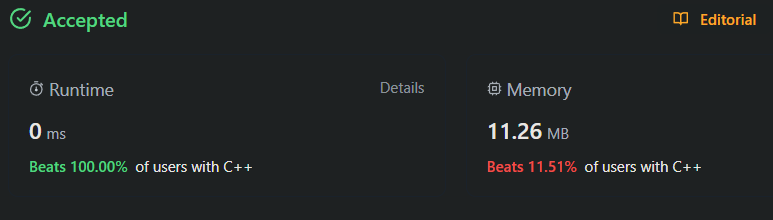\n\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(NlogN)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minimumCost(vector<int>& cost) {\n ios_base::sync_with_stdio(false);\n cin.tie(0);\n cout.tie(0);\n \n sort(cost.begin(),cost.end(),greater<int>());\n int amount = 0;\n\n int j = 2;\n for(int i = 0; i < cost.size(); i++)\n {\n // We buy first two candies and take the third candy for free\n // 3rd candy = 2nd index\n // Next free candy will be at index -> 2 + 3 = 5\n if(i == j)\n {\n j += 3;\n continue;\n }\n amount += cost[i];\n }\n \n return amount;\n }\n};\n``` | 2 | A shop is selling candies at a discount. For **every two** candies sold, the shop gives a **third** candy for **free**.
The customer can choose **any** candy to take away for free as long as the cost of the chosen candy is less than or equal to the **minimum** cost of the two candies bought.
* For example, if there are `4` candies with costs `1`, `2`, `3`, and `4`, and the customer buys candies with costs `2` and `3`, they can take the candy with cost `1` for free, but not the candy with cost `4`.
Given a **0-indexed** integer array `cost`, where `cost[i]` denotes the cost of the `ith` candy, return _the **minimum cost** of buying **all** the candies_.
**Example 1:**
**Input:** cost = \[1,2,3\]
**Output:** 5
**Explanation:** We buy the candies with costs 2 and 3, and take the candy with cost 1 for free.
The total cost of buying all candies is 2 + 3 = 5. This is the **only** way we can buy the candies.
Note that we cannot buy candies with costs 1 and 3, and then take the candy with cost 2 for free.
The cost of the free candy has to be less than or equal to the minimum cost of the purchased candies.
**Example 2:**
**Input:** cost = \[6,5,7,9,2,2\]
**Output:** 23
**Explanation:** The way in which we can get the minimum cost is described below:
- Buy candies with costs 9 and 7
- Take the candy with cost 6 for free
- We buy candies with costs 5 and 2
- Take the last remaining candy with cost 2 for free
Hence, the minimum cost to buy all candies is 9 + 7 + 5 + 2 = 23.
**Example 3:**
**Input:** cost = \[5,5\]
**Output:** 10
**Explanation:** Since there are only 2 candies, we buy both of them. There is not a third candy we can take for free.
Hence, the minimum cost to buy all candies is 5 + 5 = 10.
**Constraints:**
* `1 <= cost.length <= 100`
* `1 <= cost[i] <= 100` | Could you keep track of the minimum element visited while traversing? We have a potential candidate for the answer if the prefix min is lesser than nums[i]. |
4 lines. Beats 99% runtime | minimum-cost-of-buying-candies-with-discount | 0 | 1 | # Intuition\nFor the max value, we have to pay for it.\nFor the second max value, we still have to pay for it.\nFor the third max value, we can get it free one as bonus.\nAnd continuely do this for the rest.\n\n\n# Complexity\n- Time complexity:\nO(nlogn)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimumCost(self, cost: List[int]) -> int:\n cost.sort()\n res = 0\n for i in range(len(cost)):\n if i%3 != len(cost)%3: res+=cost[i]\n return res\n\n\n\n``` | 1 | A shop is selling candies at a discount. For **every two** candies sold, the shop gives a **third** candy for **free**.
The customer can choose **any** candy to take away for free as long as the cost of the chosen candy is less than or equal to the **minimum** cost of the two candies bought.
* For example, if there are `4` candies with costs `1`, `2`, `3`, and `4`, and the customer buys candies with costs `2` and `3`, they can take the candy with cost `1` for free, but not the candy with cost `4`.
Given a **0-indexed** integer array `cost`, where `cost[i]` denotes the cost of the `ith` candy, return _the **minimum cost** of buying **all** the candies_.
**Example 1:**
**Input:** cost = \[1,2,3\]
**Output:** 5
**Explanation:** We buy the candies with costs 2 and 3, and take the candy with cost 1 for free.
The total cost of buying all candies is 2 + 3 = 5. This is the **only** way we can buy the candies.
Note that we cannot buy candies with costs 1 and 3, and then take the candy with cost 2 for free.
The cost of the free candy has to be less than or equal to the minimum cost of the purchased candies.
**Example 2:**
**Input:** cost = \[6,5,7,9,2,2\]
**Output:** 23
**Explanation:** The way in which we can get the minimum cost is described below:
- Buy candies with costs 9 and 7
- Take the candy with cost 6 for free
- We buy candies with costs 5 and 2
- Take the last remaining candy with cost 2 for free
Hence, the minimum cost to buy all candies is 9 + 7 + 5 + 2 = 23.
**Example 3:**
**Input:** cost = \[5,5\]
**Output:** 10
**Explanation:** Since there are only 2 candies, we buy both of them. There is not a third candy we can take for free.
Hence, the minimum cost to buy all candies is 5 + 5 = 10.
**Constraints:**
* `1 <= cost.length <= 100`
* `1 <= cost[i] <= 100` | Could you keep track of the minimum element visited while traversing? We have a potential candidate for the answer if the prefix min is lesser than nums[i]. |
Python | 100% Faster | Easy Solution✅ | minimum-cost-of-buying-candies-with-discount | 0 | 1 | # Code\u2705\n```\nclass Solution:\n def minimumCost(self, cost: List[int]) -> int:\n cost = sorted(cost,reverse=True)\n i =0 \n output = 0\n while i<len(cost):\n output += cost[i] if i == len(cost)-1 else cost[i] + cost[i+1]\n i +=3\n return output\n\n\n```\n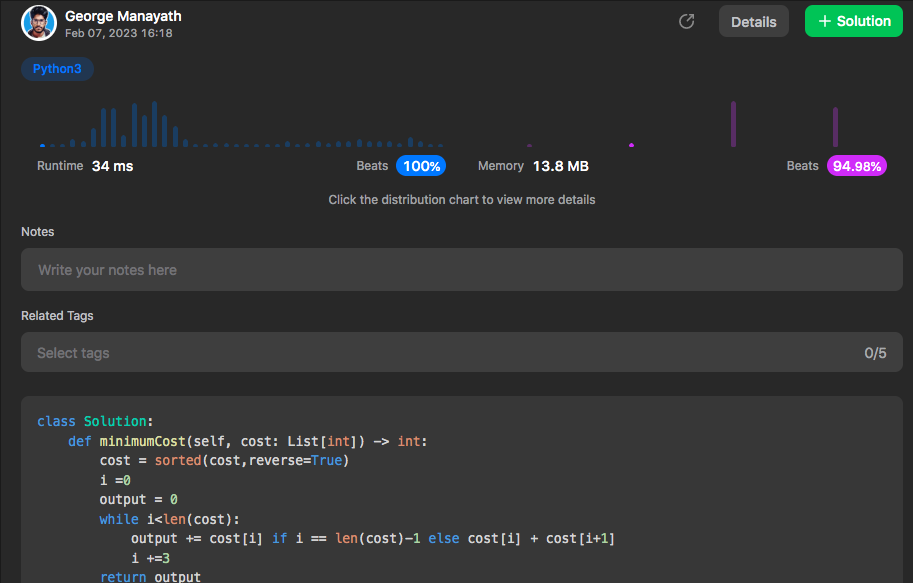\n | 6 | A shop is selling candies at a discount. For **every two** candies sold, the shop gives a **third** candy for **free**.
The customer can choose **any** candy to take away for free as long as the cost of the chosen candy is less than or equal to the **minimum** cost of the two candies bought.
* For example, if there are `4` candies with costs `1`, `2`, `3`, and `4`, and the customer buys candies with costs `2` and `3`, they can take the candy with cost `1` for free, but not the candy with cost `4`.
Given a **0-indexed** integer array `cost`, where `cost[i]` denotes the cost of the `ith` candy, return _the **minimum cost** of buying **all** the candies_.
**Example 1:**
**Input:** cost = \[1,2,3\]
**Output:** 5
**Explanation:** We buy the candies with costs 2 and 3, and take the candy with cost 1 for free.
The total cost of buying all candies is 2 + 3 = 5. This is the **only** way we can buy the candies.
Note that we cannot buy candies with costs 1 and 3, and then take the candy with cost 2 for free.
The cost of the free candy has to be less than or equal to the minimum cost of the purchased candies.
**Example 2:**
**Input:** cost = \[6,5,7,9,2,2\]
**Output:** 23
**Explanation:** The way in which we can get the minimum cost is described below:
- Buy candies with costs 9 and 7
- Take the candy with cost 6 for free
- We buy candies with costs 5 and 2
- Take the last remaining candy with cost 2 for free
Hence, the minimum cost to buy all candies is 9 + 7 + 5 + 2 = 23.
**Example 3:**
**Input:** cost = \[5,5\]
**Output:** 10
**Explanation:** Since there are only 2 candies, we buy both of them. There is not a third candy we can take for free.
Hence, the minimum cost to buy all candies is 5 + 5 = 10.
**Constraints:**
* `1 <= cost.length <= 100`
* `1 <= cost[i] <= 100` | Could you keep track of the minimum element visited while traversing? We have a potential candidate for the answer if the prefix min is lesser than nums[i]. |
One-liner | minimum-cost-of-buying-candies-with-discount | 0 | 1 | Sort cost descending, and then sum, skipping every third cost.\n\n**Python 3**\n```python\nclass Solution:\n minimumCost = lambda self, cost: sum(c for i, c in enumerate(sorted(cost, reverse=True)) if i % 3 != 2)\n```\n\n**C++**\nSame logic in C++.\n```cpp\nint minimumCost(vector<int>& cost) {\n int res = 0;\n sort(rbegin(cost), rend(cost));\n for (int i = 0; i < cost.size(); ++i)\n res += i % 3 == 2 ? 0 : cost[i];\n return res;\n}\n``` | 17 | A shop is selling candies at a discount. For **every two** candies sold, the shop gives a **third** candy for **free**.
The customer can choose **any** candy to take away for free as long as the cost of the chosen candy is less than or equal to the **minimum** cost of the two candies bought.
* For example, if there are `4` candies with costs `1`, `2`, `3`, and `4`, and the customer buys candies with costs `2` and `3`, they can take the candy with cost `1` for free, but not the candy with cost `4`.
Given a **0-indexed** integer array `cost`, where `cost[i]` denotes the cost of the `ith` candy, return _the **minimum cost** of buying **all** the candies_.
**Example 1:**
**Input:** cost = \[1,2,3\]
**Output:** 5
**Explanation:** We buy the candies with costs 2 and 3, and take the candy with cost 1 for free.
The total cost of buying all candies is 2 + 3 = 5. This is the **only** way we can buy the candies.
Note that we cannot buy candies with costs 1 and 3, and then take the candy with cost 2 for free.
The cost of the free candy has to be less than or equal to the minimum cost of the purchased candies.
**Example 2:**
**Input:** cost = \[6,5,7,9,2,2\]
**Output:** 23
**Explanation:** The way in which we can get the minimum cost is described below:
- Buy candies with costs 9 and 7
- Take the candy with cost 6 for free
- We buy candies with costs 5 and 2
- Take the last remaining candy with cost 2 for free
Hence, the minimum cost to buy all candies is 9 + 7 + 5 + 2 = 23.
**Example 3:**
**Input:** cost = \[5,5\]
**Output:** 10
**Explanation:** Since there are only 2 candies, we buy both of them. There is not a third candy we can take for free.
Hence, the minimum cost to buy all candies is 5 + 5 = 10.
**Constraints:**
* `1 <= cost.length <= 100`
* `1 <= cost[i] <= 100` | Could you keep track of the minimum element visited while traversing? We have a potential candidate for the answer if the prefix min is lesser than nums[i]. |
Greedy solution in Python, beats 100% (36ms) | minimum-cost-of-buying-candies-with-discount | 0 | 1 | Your goal is: skip the costly items as many as possible.\n\nTo do so, you choose the two largest items in the list, and then skip the next largest item. Repeat this process greedily.\n\nAlgorithm is to sort the item in an descending order, and take the first 2 items and move the index by 3, and repeat this until you reach the end.\n\nTime complexity: sort dominates and O(NlogN)\nSpace complexity: O(1)\n\n```\nclass Solution:\n def minimumCost(self, cost: List[int]) -> int:\n cost.sort(reverse=True)\n res, idx, N = 0, 0, len(cost)\n while idx < N:\n res += sum(cost[idx : idx + 2])\n idx += 3\n return res\n``` | 6 | A shop is selling candies at a discount. For **every two** candies sold, the shop gives a **third** candy for **free**.
The customer can choose **any** candy to take away for free as long as the cost of the chosen candy is less than or equal to the **minimum** cost of the two candies bought.
* For example, if there are `4` candies with costs `1`, `2`, `3`, and `4`, and the customer buys candies with costs `2` and `3`, they can take the candy with cost `1` for free, but not the candy with cost `4`.
Given a **0-indexed** integer array `cost`, where `cost[i]` denotes the cost of the `ith` candy, return _the **minimum cost** of buying **all** the candies_.
**Example 1:**
**Input:** cost = \[1,2,3\]
**Output:** 5
**Explanation:** We buy the candies with costs 2 and 3, and take the candy with cost 1 for free.
The total cost of buying all candies is 2 + 3 = 5. This is the **only** way we can buy the candies.
Note that we cannot buy candies with costs 1 and 3, and then take the candy with cost 2 for free.
The cost of the free candy has to be less than or equal to the minimum cost of the purchased candies.
**Example 2:**
**Input:** cost = \[6,5,7,9,2,2\]
**Output:** 23
**Explanation:** The way in which we can get the minimum cost is described below:
- Buy candies with costs 9 and 7
- Take the candy with cost 6 for free
- We buy candies with costs 5 and 2
- Take the last remaining candy with cost 2 for free
Hence, the minimum cost to buy all candies is 9 + 7 + 5 + 2 = 23.
**Example 3:**
**Input:** cost = \[5,5\]
**Output:** 10
**Explanation:** Since there are only 2 candies, we buy both of them. There is not a third candy we can take for free.
Hence, the minimum cost to buy all candies is 5 + 5 = 10.
**Constraints:**
* `1 <= cost.length <= 100`
* `1 <= cost[i] <= 100` | Could you keep track of the minimum element visited while traversing? We have a potential candidate for the answer if the prefix min is lesser than nums[i]. |
[Python] Simple solution | 100% faster | O(N logN) Time | O(1) Space | minimum-cost-of-buying-candies-with-discount | 0 | 1 | Understanding:\nReverse sort the array of costs. Now the first two will be highest costs which can help us get the third one for free and so on. \n\nAlgorithm:\n- Reverse sort the array\n- Initialize index i at 0, resultant cost as 0 and N as length of array cost\n- Add the cost of 2 candies to result, i.e., the cost at index and the next one\n- Increment index by 3, continue this step and above one till index reaches length of array\n- Return the result\n\nPython code :\n```\nclass Solution:\n def minimumCost(self, cost: List[int]) -> int:\n cost.sort(reverse=True)\n res, i, N = 0, 0, len(cost)\n while i < N:\n res += sum(cost[i : i + 2])\n i += 3\n return res\n``` | 10 | A shop is selling candies at a discount. For **every two** candies sold, the shop gives a **third** candy for **free**.
The customer can choose **any** candy to take away for free as long as the cost of the chosen candy is less than or equal to the **minimum** cost of the two candies bought.
* For example, if there are `4` candies with costs `1`, `2`, `3`, and `4`, and the customer buys candies with costs `2` and `3`, they can take the candy with cost `1` for free, but not the candy with cost `4`.
Given a **0-indexed** integer array `cost`, where `cost[i]` denotes the cost of the `ith` candy, return _the **minimum cost** of buying **all** the candies_.
**Example 1:**
**Input:** cost = \[1,2,3\]
**Output:** 5
**Explanation:** We buy the candies with costs 2 and 3, and take the candy with cost 1 for free.
The total cost of buying all candies is 2 + 3 = 5. This is the **only** way we can buy the candies.
Note that we cannot buy candies with costs 1 and 3, and then take the candy with cost 2 for free.
The cost of the free candy has to be less than or equal to the minimum cost of the purchased candies.
**Example 2:**
**Input:** cost = \[6,5,7,9,2,2\]
**Output:** 23
**Explanation:** The way in which we can get the minimum cost is described below:
- Buy candies with costs 9 and 7
- Take the candy with cost 6 for free
- We buy candies with costs 5 and 2
- Take the last remaining candy with cost 2 for free
Hence, the minimum cost to buy all candies is 9 + 7 + 5 + 2 = 23.
**Example 3:**
**Input:** cost = \[5,5\]
**Output:** 10
**Explanation:** Since there are only 2 candies, we buy both of them. There is not a third candy we can take for free.
Hence, the minimum cost to buy all candies is 5 + 5 = 10.
**Constraints:**
* `1 <= cost.length <= 100`
* `1 <= cost[i] <= 100` | Could you keep track of the minimum element visited while traversing? We have a potential candidate for the answer if the prefix min is lesser than nums[i]. |
[Python3] Sort + One Pass + Greedy | O(n) Time | O(1) Space | minimum-cost-of-buying-candies-with-discount | 0 | 1 | Below is the code, please let me know if you have any questions!\n```\nclass Solution:\n def minimumCost(self, cost: List[int]) -> int:\n cost.sort(reverse=True)\n bought = res = 0\n for p in cost:\n if bought < 2:\n res += p\n bought += 1\n else:\n bought = 0\n return res\n``` | 2 | A shop is selling candies at a discount. For **every two** candies sold, the shop gives a **third** candy for **free**.
The customer can choose **any** candy to take away for free as long as the cost of the chosen candy is less than or equal to the **minimum** cost of the two candies bought.
* For example, if there are `4` candies with costs `1`, `2`, `3`, and `4`, and the customer buys candies with costs `2` and `3`, they can take the candy with cost `1` for free, but not the candy with cost `4`.
Given a **0-indexed** integer array `cost`, where `cost[i]` denotes the cost of the `ith` candy, return _the **minimum cost** of buying **all** the candies_.
**Example 1:**
**Input:** cost = \[1,2,3\]
**Output:** 5
**Explanation:** We buy the candies with costs 2 and 3, and take the candy with cost 1 for free.
The total cost of buying all candies is 2 + 3 = 5. This is the **only** way we can buy the candies.
Note that we cannot buy candies with costs 1 and 3, and then take the candy with cost 2 for free.
The cost of the free candy has to be less than or equal to the minimum cost of the purchased candies.
**Example 2:**
**Input:** cost = \[6,5,7,9,2,2\]
**Output:** 23
**Explanation:** The way in which we can get the minimum cost is described below:
- Buy candies with costs 9 and 7
- Take the candy with cost 6 for free
- We buy candies with costs 5 and 2
- Take the last remaining candy with cost 2 for free
Hence, the minimum cost to buy all candies is 9 + 7 + 5 + 2 = 23.
**Example 3:**
**Input:** cost = \[5,5\]
**Output:** 10
**Explanation:** Since there are only 2 candies, we buy both of them. There is not a third candy we can take for free.
Hence, the minimum cost to buy all candies is 5 + 5 = 10.
**Constraints:**
* `1 <= cost.length <= 100`
* `1 <= cost[i] <= 100` | Could you keep track of the minimum element visited while traversing? We have a potential candidate for the answer if the prefix min is lesser than nums[i]. |
[Python3] greedy 1-line | minimum-cost-of-buying-candies-with-discount | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/8fc8469e823a229654fc858172dfa9383d805e39) for solutions of biweekly 70. \n\n**Intuition**\nSort the candies in descending order and group them by every 3 candies. Among each group, add the cost of largest 2 to the final answer. \n\n```\nclass Solution:\n def minimumCost(self, cost: List[int]) -> int:\n return sum(x for i, x in enumerate(sorted(cost, reverse=True)) if (i+1)%3)\n```\n\n**AofA**\ntime complexity `O(NlogN)` \nspace complexity `O(N)` | 5 | A shop is selling candies at a discount. For **every two** candies sold, the shop gives a **third** candy for **free**.
The customer can choose **any** candy to take away for free as long as the cost of the chosen candy is less than or equal to the **minimum** cost of the two candies bought.
* For example, if there are `4` candies with costs `1`, `2`, `3`, and `4`, and the customer buys candies with costs `2` and `3`, they can take the candy with cost `1` for free, but not the candy with cost `4`.
Given a **0-indexed** integer array `cost`, where `cost[i]` denotes the cost of the `ith` candy, return _the **minimum cost** of buying **all** the candies_.
**Example 1:**
**Input:** cost = \[1,2,3\]
**Output:** 5
**Explanation:** We buy the candies with costs 2 and 3, and take the candy with cost 1 for free.
The total cost of buying all candies is 2 + 3 = 5. This is the **only** way we can buy the candies.
Note that we cannot buy candies with costs 1 and 3, and then take the candy with cost 2 for free.
The cost of the free candy has to be less than or equal to the minimum cost of the purchased candies.
**Example 2:**
**Input:** cost = \[6,5,7,9,2,2\]
**Output:** 23
**Explanation:** The way in which we can get the minimum cost is described below:
- Buy candies with costs 9 and 7
- Take the candy with cost 6 for free
- We buy candies with costs 5 and 2
- Take the last remaining candy with cost 2 for free
Hence, the minimum cost to buy all candies is 9 + 7 + 5 + 2 = 23.
**Example 3:**
**Input:** cost = \[5,5\]
**Output:** 10
**Explanation:** Since there are only 2 candies, we buy both of them. There is not a third candy we can take for free.
Hence, the minimum cost to buy all candies is 5 + 5 = 10.
**Constraints:**
* `1 <= cost.length <= 100`
* `1 <= cost[i] <= 100` | Could you keep track of the minimum element visited while traversing? We have a potential candidate for the answer if the prefix min is lesser than nums[i]. |
Right - Left | count-the-hidden-sequences | 0 | 1 | Assuming that the starting point is zero, we determine the range of the hidden sequence ([left, right]).\n\nIf this range is smaller than [lower, upper] - we can form a valid sequence. The difference between those two ranges tells us how many valid sequences we can form: `upper - lower - (right - left) + 1`.\n\n**Python 3**\n```python\nclass Solution:\n def numberOfArrays(self, diff: List[int], lower: int, upper: int) -> int:\n diff = list(accumulate(diff, initial = 0))\n return max(0, upper - lower - (max(diff) - min(diff)) + 1)\n```\n**C++**\n```cpp\nint numberOfArrays(vector<int>& diff, int lower, int upper) {\n long long left = 0, right = 0, cur = 0;\n for (int d : diff) {\n cur += d;\n left = min(left, cur);\n right = max(right, cur);\n }\n return max(0LL, upper - lower - (right - left) + 1);\n}\n``` | 5 | You are given a **0-indexed** array of `n` integers `differences`, which describes the **differences** between each pair of **consecutive** integers of a **hidden** sequence of length `(n + 1)`. More formally, call the hidden sequence `hidden`, then we have that `differences[i] = hidden[i + 1] - hidden[i]`.
You are further given two integers `lower` and `upper` that describe the **inclusive** range of values `[lower, upper]` that the hidden sequence can contain.
* For example, given `differences = [1, -3, 4]`, `lower = 1`, `upper = 6`, the hidden sequence is a sequence of length `4` whose elements are in between `1` and `6` (**inclusive**).
* `[3, 4, 1, 5]` and `[4, 5, 2, 6]` are possible hidden sequences.
* `[5, 6, 3, 7]` is not possible since it contains an element greater than `6`.
* `[1, 2, 3, 4]` is not possible since the differences are not correct.
Return _the number of **possible** hidden sequences there are._ If there are no possible sequences, return `0`.
**Example 1:**
**Input:** differences = \[1,-3,4\], lower = 1, upper = 6
**Output:** 2
**Explanation:** The possible hidden sequences are:
- \[3, 4, 1, 5\]
- \[4, 5, 2, 6\]
Thus, we return 2.
**Example 2:**
**Input:** differences = \[3,-4,5,1,-2\], lower = -4, upper = 5
**Output:** 4
**Explanation:** The possible hidden sequences are:
- \[-3, 0, -4, 1, 2, 0\]
- \[-2, 1, -3, 2, 3, 1\]
- \[-1, 2, -2, 3, 4, 2\]
- \[0, 3, -1, 4, 5, 3\]
Thus, we return 4.
**Example 3:**
**Input:** differences = \[4,-7,2\], lower = 3, upper = 6
**Output:** 0
**Explanation:** There are no possible hidden sequences. Thus, we return 0.
**Constraints:**
* `n == differences.length`
* `1 <= n <= 105`
* `-105 <= differences[i] <= 105`
* `-105 <= lower <= upper <= 105` | There are n choices for when the first robot moves to the second row. Can we use prefix sums to help solve this problem? |
✔️ [Python3] FLOWING CONSTRAINT ♪♪ ヽ(ˇ∀ˇ )ゞ, Explained | count-the-hidden-sequences | 0 | 1 | **UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.**\n\nThe idea is to calculate constraints for every element of the hidden sequence starting from the beginning. The last constraint will be equal to the range that the last element of the sequence can take. Considering that the value of elements in the sequence depends on each other, the number of values that the last element can take is equal to the number of possible sequences, i.e. this is our answer. \n\nExample: `differences = [1,-3,4], lower = 1, upper = 6`. Let the hidden sequence be `[a, b, c, d]`. Thus:\n```\nb - a = 1\nc - b = -3\nd - c = 4\n```\nLet\'s isolate every element:\n```\na = b - 1\nb = c + 3\nc = d - 4\n```\nCalculate constraints for every element:\n```\nlower <= a <= upper\n\nlower <= b - 1 <= upper\nlower + 1 <= b <= upper + 1\n\nlower + 1 <= c + 3 <= upper + 1\nlower + 1 - 3 <= c <= upper + 1 - 3\n\nlower + 1 - 3 <= d - 4 <= upper + 1 - 3\nlower + 1 - 3 + 4 <= d <= upper + 1 - 3 + 4\n```\nOn every step, we clip the resulting boundaries to the range `lower .. upper`, and also check if we got out of the range and return 0.\n\nTime: **O(n)** - linear\nSpace: **O(1)** - nothing stored \n\nRuntime: 1288 ms, faster than **89.22%** of Python3 online submissions for Count the Hidden Sequences.\nMemory Usage: 28.5 MB, less than **95.81%** of Python3 online submissions for Count the Hidden Sequences.\n\n```\ndef numberOfArrays(self, differences: List[int], lower: int, upper: int) -> int:\n\tlo, up = lower, upper\n\tfor diff in differences:\n\t\tlo, up = max(lower, lo + diff), min(upper, up + diff)\n\t\tif lo > upper or up < lower: return 0\n\n\treturn up - lo + 1\n```\n\n**UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.** | 7 | You are given a **0-indexed** array of `n` integers `differences`, which describes the **differences** between each pair of **consecutive** integers of a **hidden** sequence of length `(n + 1)`. More formally, call the hidden sequence `hidden`, then we have that `differences[i] = hidden[i + 1] - hidden[i]`.
You are further given two integers `lower` and `upper` that describe the **inclusive** range of values `[lower, upper]` that the hidden sequence can contain.
* For example, given `differences = [1, -3, 4]`, `lower = 1`, `upper = 6`, the hidden sequence is a sequence of length `4` whose elements are in between `1` and `6` (**inclusive**).
* `[3, 4, 1, 5]` and `[4, 5, 2, 6]` are possible hidden sequences.
* `[5, 6, 3, 7]` is not possible since it contains an element greater than `6`.
* `[1, 2, 3, 4]` is not possible since the differences are not correct.
Return _the number of **possible** hidden sequences there are._ If there are no possible sequences, return `0`.
**Example 1:**
**Input:** differences = \[1,-3,4\], lower = 1, upper = 6
**Output:** 2
**Explanation:** The possible hidden sequences are:
- \[3, 4, 1, 5\]
- \[4, 5, 2, 6\]
Thus, we return 2.
**Example 2:**
**Input:** differences = \[3,-4,5,1,-2\], lower = -4, upper = 5
**Output:** 4
**Explanation:** The possible hidden sequences are:
- \[-3, 0, -4, 1, 2, 0\]
- \[-2, 1, -3, 2, 3, 1\]
- \[-1, 2, -2, 3, 4, 2\]
- \[0, 3, -1, 4, 5, 3\]
Thus, we return 4.
**Example 3:**
**Input:** differences = \[4,-7,2\], lower = 3, upper = 6
**Output:** 0
**Explanation:** There are no possible hidden sequences. Thus, we return 0.
**Constraints:**
* `n == differences.length`
* `1 <= n <= 105`
* `-105 <= differences[i] <= 105`
* `-105 <= lower <= upper <= 105` | There are n choices for when the first robot moves to the second row. Can we use prefix sums to help solve this problem? |
[Python3] compare range | count-the-hidden-sequences | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/8fc8469e823a229654fc858172dfa9383d805e39) for solutions of biweekly 70. \n\n**Intuition**\nCompute the range of variation from `differences`. Compuare this range with allowed range `upper - lower`. If it is within this range, then a solutions exists i.e. `upper - lower - range` otherwise return 0. \n\n```\nclass Solution:\n def numberOfArrays(self, differences: List[int], lower: int, upper: int) -> int:\n prefix = mn = mx = 0 \n for x in differences: \n prefix += x\n mn = min(mn, prefix)\n mx = max(mx, prefix)\n return max(0, (upper-lower) - (mx-mn) + 1)\n```\n\n**AofA**\ntime complexity `O(N)`\nspace complexity `O(1)` | 2 | You are given a **0-indexed** array of `n` integers `differences`, which describes the **differences** between each pair of **consecutive** integers of a **hidden** sequence of length `(n + 1)`. More formally, call the hidden sequence `hidden`, then we have that `differences[i] = hidden[i + 1] - hidden[i]`.
You are further given two integers `lower` and `upper` that describe the **inclusive** range of values `[lower, upper]` that the hidden sequence can contain.
* For example, given `differences = [1, -3, 4]`, `lower = 1`, `upper = 6`, the hidden sequence is a sequence of length `4` whose elements are in between `1` and `6` (**inclusive**).
* `[3, 4, 1, 5]` and `[4, 5, 2, 6]` are possible hidden sequences.
* `[5, 6, 3, 7]` is not possible since it contains an element greater than `6`.
* `[1, 2, 3, 4]` is not possible since the differences are not correct.
Return _the number of **possible** hidden sequences there are._ If there are no possible sequences, return `0`.
**Example 1:**
**Input:** differences = \[1,-3,4\], lower = 1, upper = 6
**Output:** 2
**Explanation:** The possible hidden sequences are:
- \[3, 4, 1, 5\]
- \[4, 5, 2, 6\]
Thus, we return 2.
**Example 2:**
**Input:** differences = \[3,-4,5,1,-2\], lower = -4, upper = 5
**Output:** 4
**Explanation:** The possible hidden sequences are:
- \[-3, 0, -4, 1, 2, 0\]
- \[-2, 1, -3, 2, 3, 1\]
- \[-1, 2, -2, 3, 4, 2\]
- \[0, 3, -1, 4, 5, 3\]
Thus, we return 4.
**Example 3:**
**Input:** differences = \[4,-7,2\], lower = 3, upper = 6
**Output:** 0
**Explanation:** There are no possible hidden sequences. Thus, we return 0.
**Constraints:**
* `n == differences.length`
* `1 <= n <= 105`
* `-105 <= differences[i] <= 105`
* `-105 <= lower <= upper <= 105` | There are n choices for when the first robot moves to the second row. Can we use prefix sums to help solve this problem? |
✔️ Python3 Solution | Heap | k-highest-ranked-items-within-a-price-range | 0 | 1 | # Complexity\n- Time complexity: $$O(n*m)$$\n- Space complexity: $$O(n*m)$$\n\n# Code\n```\nclass Solution:\n def highestRankedKItems(self, grid, pricing, start, k):\n\n res = []\n m, n = len(grid), len(grid[0])\n low, high = pricing\n row, col = start\n heap = deque([(0, row, col)])\n grid[row][col] = -grid[row][col]\n\n while heap:\n dis, row, col = heap.popleft()\n if low <= -grid[row][col] <= high:\n res.append((dis, -grid[row][col], row, col))\n for x, y in ((1, 0), (-1, 0), (0, 1), (0, -1)):\n if 0 <= row + x < m and 0 <= col + y < n and grid[row + x][col + y] > 0:\n heap.append((dis + 1, row + x, col + y))\n grid[row + x][col + y] = -grid[row + x][col + y]\n \n res.sort()\n return [(x, y) for _, _, x, y in res[:k]]\n``` | 1 | You are given a **0-indexed** 2D integer array `grid` of size `m x n` that represents a map of the items in a shop. The integers in the grid represent the following:
* `0` represents a wall that you cannot pass through.
* `1` represents an empty cell that you can freely move to and from.
* All other positive integers represent the price of an item in that cell. You may also freely move to and from these item cells.
It takes `1` step to travel between adjacent grid cells.
You are also given integer arrays `pricing` and `start` where `pricing = [low, high]` and `start = [row, col]` indicates that you start at the position `(row, col)` and are interested only in items with a price in the range of `[low, high]` (**inclusive**). You are further given an integer `k`.
You are interested in the **positions** of the `k` **highest-ranked** items whose prices are **within** the given price range. The rank is determined by the **first** of these criteria that is different:
1. Distance, defined as the length of the shortest path from the `start` (**shorter** distance has a higher rank).
2. Price (**lower** price has a higher rank, but it must be **in the price range**).
3. The row number (**smaller** row number has a higher rank).
4. The column number (**smaller** column number has a higher rank).
Return _the_ `k` _highest-ranked items within the price range **sorted** by their rank (highest to lowest)_. If there are fewer than `k` reachable items within the price range, return _**all** of them_.
**Example 1:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,0,1\],\[0,2,5,1\]\], pricing = \[2,5\], start = \[0,0\], k = 3
**Output:** \[\[0,1\],\[1,1\],\[2,1\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,5\], we can take items from (0,1), (1,1), (2,1) and (2,2).
The ranks of these items are:
- (0,1) with distance 1
- (1,1) with distance 2
- (2,1) with distance 3
- (2,2) with distance 4
Thus, the 3 highest ranked items in the price range are (0,1), (1,1), and (2,1).
**Example 2:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,3,1\],\[0,2,5,1\]\], pricing = \[2,3\], start = \[2,3\], k = 2
**Output:** \[\[2,1\],\[1,2\]\]
**Explanation:** You start at (2,3).
With a price range of \[2,3\], we can take items from (0,1), (1,1), (1,2) and (2,1).
The ranks of these items are:
- (2,1) with distance 2, price 2
- (1,2) with distance 2, price 3
- (1,1) with distance 3
- (0,1) with distance 4
Thus, the 2 highest ranked items in the price range are (2,1) and (1,2).
**Example 3:**
**Input:** grid = \[\[1,1,1\],\[0,0,1\],\[2,3,4\]\], pricing = \[2,3\], start = \[0,0\], k = 3
**Output:** \[\[2,1\],\[2,0\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,3\], we can take items from (2,0) and (2,1).
The ranks of these items are:
- (2,1) with distance 5
- (2,0) with distance 6
Thus, the 2 highest ranked items in the price range are (2,1) and (2,0).
Note that k = 3 but there are only 2 reachable items within the price range.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 105`
* `1 <= m * n <= 105`
* `0 <= grid[i][j] <= 105`
* `pricing.length == 2`
* `2 <= low <= high <= 105`
* `start.length == 2`
* `0 <= row <= m - 1`
* `0 <= col <= n - 1`
* `grid[row][col] > 0`
* `1 <= k <= m * n` | Check all possible placements for the word. There is a limited number of places where a word can start. |
[Python 3] Dijkstra Algorithm - Easy to Understand | k-highest-ranked-items-within-a-price-range | 0 | 1 | # Intuition\n- First we can recognize that we should find the shortest path from start to all the cells -> Dijkstra\n- then we after we have the shortest path from the start cell to all other cells, we may sort it and take k highest one with value in range pricing (we can do this better utilize the properites in dijkstra)\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(m * n log(m * n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(m * n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def highestRankedKItems(self, grid: List[List[int]], pricing: List[int], start: List[int], k: int) -> List[List[int]]:\n m, n = len(grid), len(grid[0])\n sx, sy = start\n pl, ph = pricing\n q = [(0, grid[sx][sy], sx, sy)]\n visited = set()\n res = []\n\n while q:\n dis, price, x, y = heapq.heappop(q)\n if (x, y) in visited: continue\n \n visited.add((x, y))\n \n if price != 1 and pl <= price <= ph: res.append([x, y])\n if len(res) == k: return res\n\n for dx, dy in [(1, 0), (-1, 0), (0, 1), (0, -1)]:\n new_x, new_y = x + dx, y + dy\n if 0 <= new_x < m and 0 <= new_y < n:\n val = grid[new_x][new_y]\n if val == 0: continue\n else: heapq.heappush(q, (dis + 1, val, new_x, new_y))\n return res\n``` | 3 | You are given a **0-indexed** 2D integer array `grid` of size `m x n` that represents a map of the items in a shop. The integers in the grid represent the following:
* `0` represents a wall that you cannot pass through.
* `1` represents an empty cell that you can freely move to and from.
* All other positive integers represent the price of an item in that cell. You may also freely move to and from these item cells.
It takes `1` step to travel between adjacent grid cells.
You are also given integer arrays `pricing` and `start` where `pricing = [low, high]` and `start = [row, col]` indicates that you start at the position `(row, col)` and are interested only in items with a price in the range of `[low, high]` (**inclusive**). You are further given an integer `k`.
You are interested in the **positions** of the `k` **highest-ranked** items whose prices are **within** the given price range. The rank is determined by the **first** of these criteria that is different:
1. Distance, defined as the length of the shortest path from the `start` (**shorter** distance has a higher rank).
2. Price (**lower** price has a higher rank, but it must be **in the price range**).
3. The row number (**smaller** row number has a higher rank).
4. The column number (**smaller** column number has a higher rank).
Return _the_ `k` _highest-ranked items within the price range **sorted** by their rank (highest to lowest)_. If there are fewer than `k` reachable items within the price range, return _**all** of them_.
**Example 1:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,0,1\],\[0,2,5,1\]\], pricing = \[2,5\], start = \[0,0\], k = 3
**Output:** \[\[0,1\],\[1,1\],\[2,1\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,5\], we can take items from (0,1), (1,1), (2,1) and (2,2).
The ranks of these items are:
- (0,1) with distance 1
- (1,1) with distance 2
- (2,1) with distance 3
- (2,2) with distance 4
Thus, the 3 highest ranked items in the price range are (0,1), (1,1), and (2,1).
**Example 2:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,3,1\],\[0,2,5,1\]\], pricing = \[2,3\], start = \[2,3\], k = 2
**Output:** \[\[2,1\],\[1,2\]\]
**Explanation:** You start at (2,3).
With a price range of \[2,3\], we can take items from (0,1), (1,1), (1,2) and (2,1).
The ranks of these items are:
- (2,1) with distance 2, price 2
- (1,2) with distance 2, price 3
- (1,1) with distance 3
- (0,1) with distance 4
Thus, the 2 highest ranked items in the price range are (2,1) and (1,2).
**Example 3:**
**Input:** grid = \[\[1,1,1\],\[0,0,1\],\[2,3,4\]\], pricing = \[2,3\], start = \[0,0\], k = 3
**Output:** \[\[2,1\],\[2,0\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,3\], we can take items from (2,0) and (2,1).
The ranks of these items are:
- (2,1) with distance 5
- (2,0) with distance 6
Thus, the 2 highest ranked items in the price range are (2,1) and (2,0).
Note that k = 3 but there are only 2 reachable items within the price range.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 105`
* `1 <= m * n <= 105`
* `0 <= grid[i][j] <= 105`
* `pricing.length == 2`
* `2 <= low <= high <= 105`
* `start.length == 2`
* `0 <= row <= m - 1`
* `0 <= col <= n - 1`
* `grid[row][col] > 0`
* `1 <= k <= m * n` | Check all possible placements for the word. There is a limited number of places where a word can start. |
[Java/Python 3] BFS using PriorityQueue/heap w/ brief explanation and analysis. | k-highest-ranked-items-within-a-price-range | 1 | 1 | **Q & A**\n\nQ1: Why do you have `ans.size() < k` in the `while` condition?\nA1: The `PriorityQueue/heap` is sorted according to the rank specified in the problem. Therefore, the `ans` always holds highest ranked items within the given price range, and once it reaches the size of `k`, the loop does NOT need to iterate any more.\n\n**End of Q & A**\n\n----\n\nUse a `PriorityQueue/heap` to store (distance, price, row, col) and a HashSet to prune duplicates.\n```java\n private static final int[] d = {0, 1, 0, -1, 0};\n public List<List<Integer>> highestRankedKItems(int[][] grid, int[] pricing, int[] start, int k) {\n int R = grid.length, C = grid[0].length;\n int x = start[0], y = start[1], low = pricing[0], high = pricing[1];\n Set<String> seen = new HashSet<>(); // Set used to prune duplicates.\n seen.add(x + "," + y);\n List<List<Integer>> ans = new ArrayList<>();\n // PriorityQueue sorted by (distance, price, row, col).\n PriorityQueue<int[]> pq = new PriorityQueue<>((a, b) -> a[0] == b[0] ? a[1] == b[1] ? a[2] == b[2] ? a[3] - b[3] : a[2] - b[2] : a[1] - b[1] : a[0] - b[0]);\n pq.offer(new int[]{0, grid[x][y], x, y}); // BFS starting point.\n while (!pq.isEmpty() && ans.size() < k) {\n int[] cur = pq.poll();\n int distance = cur[0], price = cur[1], r = cur[2], c = cur[3]; // distance, price, row & column.\n if (low <= price && price <= high) { // price in range?\n ans.add(Arrays.asList(r, c));\n }\n for (int m = 0; m < 4; ++m) { // traverse 4 neighbors.\n int i = r + d[m], j = c + d[m + 1];\n // in boundary, not wall, and not visited yet?\n if (0 <= i && i < R && 0 <= j && j < C && grid[i][j] > 0 && seen.add(i + "," + j)) {\n pq.offer(new int[]{distance + 1, grid[i][j], i, j});\n }\n }\n }\n return ans;\n }\n```\n```python\n def highestRankedKItems(self, grid: List[List[int]], pricing: List[int], start: List[int], k: int) -> List[List[int]]:\n R, C = map(len, (grid, grid[0]))\n ans, (x, y), (low, high) = [], start, pricing\n heap = [(0, grid[x][y], x, y)]\n seen = {(x, y)}\n while heap and len(ans) < k:\n distance, price, r, c = heapq.heappop(heap)\n if low <= price <= high:\n ans.append([r, c])\n for i, j in (r, c + 1), (r, c - 1), (r + 1, c), (r - 1, c):\n if R > i >= 0 <= j < C and grid[i][j] > 0 and (i, j) not in seen: \n seen.add((i, j))\n heapq.heappush(heap, (distance + 1, grid[i][j], i, j))\n return ans\n```\n\nIn case you prefer negating the `grid` values to using HashSet, please refer to the following codes.\nOf course, the input is modified.\n\n```java\n private static final int[] d = {0, 1, 0, -1, 0};\n public List<List<Integer>> highestRankedKItems(int[][] grid, int[] pricing, int[] start, int k) {\n int R = grid.length, C = grid[0].length;\n int x = start[0], y = start[1], low = pricing[0], high = pricing[1];\n List<List<Integer>> ans = new ArrayList<>();\n // PriorityQueue sorted by (distance, price, row, col).\n PriorityQueue<int[]> pq = new PriorityQueue<>((a, b) -> a[0] == b[0] ? a[1] == b[1] ? a[2] == b[2] ? a[3] - b[3] : a[2] - b[2] : a[1] - b[1] : a[0] - b[0]);\n pq.offer(new int[]{0, grid[x][y], x, y}); // BFS starting point.\n grid[x][y] *= -1;\n while (!pq.isEmpty() && ans.size() < k) {\n int[] cur = pq.poll();\n int distance = cur[0], price = cur[1], r = cur[2], c = cur[3]; // distance, price, row & column.\n if (low <= price && price <= high) { // price in range?\n ans.add(Arrays.asList(r, c));\n }\n for (int m = 0; m < 4; ++m) { // traverse 4 neighbors.\n int i = r + d[m], j = c + d[m + 1];\n // in boundary, not wall, and not visited yet?\n if (0 <= i && i < R && 0 <= j && j < C && grid[i][j] > 0) {\n pq.offer(new int[]{distance + 1, grid[i][j], i, j});\n grid[i][j] *= -1;\n }\n }\n }\n return ans;\n }\n```\n```python\n def highestRankedKItems(self, grid: List[List[int]], pricing: List[int], start: List[int], k: int) -> List[List[int]]:\n R, C = map(len, (grid, grid[0]))\n ans, (x, y), (low, high) = [], start, pricing\n heap = [(0, grid[x][y], x, y)]\n grid[x][y] *= -1\n while heap and len(ans) < k:\n distance, price, r, c = heapq.heappop(heap)\n if low <= price <= high:\n ans.append([r, c])\n for i, j in (r, c + 1), (r, c - 1), (r + 1, c), (r - 1, c):\n if R > i >= 0 <= j < C and grid[i][j] > 0: \n heapq.heappush(heap, (distance + 1, grid[i][j], i, j))\n grid[i][j] *= -1\n return ans\n```\n**Analysis:**\n\nTime: `O(R * C * log(R * C))`, space: `O(R * C)`, where `R = grid.length, C = grid[0].length`. | 16 | You are given a **0-indexed** 2D integer array `grid` of size `m x n` that represents a map of the items in a shop. The integers in the grid represent the following:
* `0` represents a wall that you cannot pass through.
* `1` represents an empty cell that you can freely move to and from.
* All other positive integers represent the price of an item in that cell. You may also freely move to and from these item cells.
It takes `1` step to travel between adjacent grid cells.
You are also given integer arrays `pricing` and `start` where `pricing = [low, high]` and `start = [row, col]` indicates that you start at the position `(row, col)` and are interested only in items with a price in the range of `[low, high]` (**inclusive**). You are further given an integer `k`.
You are interested in the **positions** of the `k` **highest-ranked** items whose prices are **within** the given price range. The rank is determined by the **first** of these criteria that is different:
1. Distance, defined as the length of the shortest path from the `start` (**shorter** distance has a higher rank).
2. Price (**lower** price has a higher rank, but it must be **in the price range**).
3. The row number (**smaller** row number has a higher rank).
4. The column number (**smaller** column number has a higher rank).
Return _the_ `k` _highest-ranked items within the price range **sorted** by their rank (highest to lowest)_. If there are fewer than `k` reachable items within the price range, return _**all** of them_.
**Example 1:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,0,1\],\[0,2,5,1\]\], pricing = \[2,5\], start = \[0,0\], k = 3
**Output:** \[\[0,1\],\[1,1\],\[2,1\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,5\], we can take items from (0,1), (1,1), (2,1) and (2,2).
The ranks of these items are:
- (0,1) with distance 1
- (1,1) with distance 2
- (2,1) with distance 3
- (2,2) with distance 4
Thus, the 3 highest ranked items in the price range are (0,1), (1,1), and (2,1).
**Example 2:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,3,1\],\[0,2,5,1\]\], pricing = \[2,3\], start = \[2,3\], k = 2
**Output:** \[\[2,1\],\[1,2\]\]
**Explanation:** You start at (2,3).
With a price range of \[2,3\], we can take items from (0,1), (1,1), (1,2) and (2,1).
The ranks of these items are:
- (2,1) with distance 2, price 2
- (1,2) with distance 2, price 3
- (1,1) with distance 3
- (0,1) with distance 4
Thus, the 2 highest ranked items in the price range are (2,1) and (1,2).
**Example 3:**
**Input:** grid = \[\[1,1,1\],\[0,0,1\],\[2,3,4\]\], pricing = \[2,3\], start = \[0,0\], k = 3
**Output:** \[\[2,1\],\[2,0\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,3\], we can take items from (2,0) and (2,1).
The ranks of these items are:
- (2,1) with distance 5
- (2,0) with distance 6
Thus, the 2 highest ranked items in the price range are (2,1) and (2,0).
Note that k = 3 but there are only 2 reachable items within the price range.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 105`
* `1 <= m * n <= 105`
* `0 <= grid[i][j] <= 105`
* `pricing.length == 2`
* `2 <= low <= high <= 105`
* `start.length == 2`
* `0 <= row <= m - 1`
* `0 <= col <= n - 1`
* `grid[row][col] > 0`
* `1 <= k <= m * n` | Check all possible placements for the word. There is a limited number of places where a word can start. |
Dijkstra Algorithm, and a list of similar questions | k-highest-ranked-items-within-a-price-range | 0 | 1 | If you are new to Dijkstra, see my other posts with graph explanations. https://leetcode.com/problems/the-maze-ii/discuss/1377876/improved-dijkstra-based-on-the-most-voted-solution/1208402\n\nFor such matrix-like problem, can always think about coverting it to a graph problem: each cell represents a node in graph and is connected to its for different neighbors. The number of the cell represent the the weight.\n\nThen the question is then translated to: starting from the start point, find k nodes (meaing that the cell\'s value should not be 0 according to the question) according to the **rule** provided.\n\nThinking about the Dijkstra Algorithm, it can rank the nodes accoding a **rule** of measuing the accumulated weights of edges from the starting point to the nodes. In this sense, we can just use the Dikstra Algorithm and replace its classical rule with the rule provided here. Bingo!\n\nSimilar Leetcode problems can be solved with the same paradigm:\nhttps://leetcode.com/problems/minimum-cost-to-make-at-least-one-valid-path-in-a-grid/\nhttps://leetcode.com/problems/swim-in-rising-water/\n\n```\nimport heapq\nclass Solution:\n def highestRankedKItems(self, grid: List[List[int]], pricing: List[int], start: List[int], k: int) -> List[List[int]]:\n row, col = len(grid), len(grid[0])\n q = [(0, grid[start[0]][start[1]], start[0], start[1])]\n visit = set()\n result = []\n while q:\n dis, price, x, y = heapq.heappop(q)\n if (x, y) in visit:\n continue\n visit.add((x, y))\n if price != 1 and price >= pricing[0] and price <= pricing[1]:\n result.append([x, y])\n if len(result) == k:\n return result\n for dx, dy in (0, 1), (0, -1), (1, 0), (-1, 0):\n new_x, new_y = x + dx, y + dy\n if 0 <= new_x < row and 0 <= new_y < col and (new_x, new_y) not in visit:\n temp = grid[new_x][new_y]\n if temp == 0:\n continue\n elif temp == 1:\n heapq.heappush(q, (dis + 1, 1, new_x, new_y))\n else:\n heapq.heappush(q, (dis + 1, temp, new_x, new_y))\n \n return result\n``` | 6 | You are given a **0-indexed** 2D integer array `grid` of size `m x n` that represents a map of the items in a shop. The integers in the grid represent the following:
* `0` represents a wall that you cannot pass through.
* `1` represents an empty cell that you can freely move to and from.
* All other positive integers represent the price of an item in that cell. You may also freely move to and from these item cells.
It takes `1` step to travel between adjacent grid cells.
You are also given integer arrays `pricing` and `start` where `pricing = [low, high]` and `start = [row, col]` indicates that you start at the position `(row, col)` and are interested only in items with a price in the range of `[low, high]` (**inclusive**). You are further given an integer `k`.
You are interested in the **positions** of the `k` **highest-ranked** items whose prices are **within** the given price range. The rank is determined by the **first** of these criteria that is different:
1. Distance, defined as the length of the shortest path from the `start` (**shorter** distance has a higher rank).
2. Price (**lower** price has a higher rank, but it must be **in the price range**).
3. The row number (**smaller** row number has a higher rank).
4. The column number (**smaller** column number has a higher rank).
Return _the_ `k` _highest-ranked items within the price range **sorted** by their rank (highest to lowest)_. If there are fewer than `k` reachable items within the price range, return _**all** of them_.
**Example 1:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,0,1\],\[0,2,5,1\]\], pricing = \[2,5\], start = \[0,0\], k = 3
**Output:** \[\[0,1\],\[1,1\],\[2,1\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,5\], we can take items from (0,1), (1,1), (2,1) and (2,2).
The ranks of these items are:
- (0,1) with distance 1
- (1,1) with distance 2
- (2,1) with distance 3
- (2,2) with distance 4
Thus, the 3 highest ranked items in the price range are (0,1), (1,1), and (2,1).
**Example 2:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,3,1\],\[0,2,5,1\]\], pricing = \[2,3\], start = \[2,3\], k = 2
**Output:** \[\[2,1\],\[1,2\]\]
**Explanation:** You start at (2,3).
With a price range of \[2,3\], we can take items from (0,1), (1,1), (1,2) and (2,1).
The ranks of these items are:
- (2,1) with distance 2, price 2
- (1,2) with distance 2, price 3
- (1,1) with distance 3
- (0,1) with distance 4
Thus, the 2 highest ranked items in the price range are (2,1) and (1,2).
**Example 3:**
**Input:** grid = \[\[1,1,1\],\[0,0,1\],\[2,3,4\]\], pricing = \[2,3\], start = \[0,0\], k = 3
**Output:** \[\[2,1\],\[2,0\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,3\], we can take items from (2,0) and (2,1).
The ranks of these items are:
- (2,1) with distance 5
- (2,0) with distance 6
Thus, the 2 highest ranked items in the price range are (2,1) and (2,0).
Note that k = 3 but there are only 2 reachable items within the price range.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 105`
* `1 <= m * n <= 105`
* `0 <= grid[i][j] <= 105`
* `pricing.length == 2`
* `2 <= low <= high <= 105`
* `start.length == 2`
* `0 <= row <= m - 1`
* `0 <= col <= n - 1`
* `grid[row][col] > 0`
* `1 <= k <= m * n` | Check all possible placements for the word. There is a limited number of places where a word can start. |
Python || Clean and Simple solution using BFS and Priority Queue | k-highest-ranked-items-within-a-price-range | 0 | 1 | ```python\nfrom collections import deque\nfrom heapq import heappush, heappushpop\n\nMOVES = (\n (1, 0),\n (-1, 0),\n (0, 1),\n (0, -1),\n)\nWALL = 0\n\n\nclass Solution:\n def highestRankedKItems(self, M: list[list[int]], P: list[int],\n start: list[int], k: int) -> list[list[int]]:\n m, n = len(M), len(M[0])\n\n q = deque()\n pq = []\n\n def add_to_q(r: int, c: int, d: int):\n q.append((r, c))\n\n if P[0] <= M[r][c] <= P[1]:\n key = (-d, -M[r][c], -r, -c)\n\n if len(pq) < k:\n heappush(pq, key)\n else:\n heappushpop(pq, key)\n\n M[r][c] = WALL\n\n distance = 0\n add_to_q(start[0], start[1], distance)\n\n while q:\n distance += 1\n\n for _ in range(len(q)):\n i, j = q.popleft()\n\n for dx, dy in MOVES:\n if 0 <= (x := i + dx) < m and 0 <= (y := j + dy) < n and M[x][y] != WALL:\n add_to_q(x, y, distance)\n\n # we can also pop heap using heappop (instead of sorting pq directly as used below) which will\n # provide items in sorted order and then reverse the list of items popped and extract negative\n # of coordinates\n pq.sort(reverse=True)\n return [[-x, -y] for _, _, x, y in pq]\n``` | 0 | You are given a **0-indexed** 2D integer array `grid` of size `m x n` that represents a map of the items in a shop. The integers in the grid represent the following:
* `0` represents a wall that you cannot pass through.
* `1` represents an empty cell that you can freely move to and from.
* All other positive integers represent the price of an item in that cell. You may also freely move to and from these item cells.
It takes `1` step to travel between adjacent grid cells.
You are also given integer arrays `pricing` and `start` where `pricing = [low, high]` and `start = [row, col]` indicates that you start at the position `(row, col)` and are interested only in items with a price in the range of `[low, high]` (**inclusive**). You are further given an integer `k`.
You are interested in the **positions** of the `k` **highest-ranked** items whose prices are **within** the given price range. The rank is determined by the **first** of these criteria that is different:
1. Distance, defined as the length of the shortest path from the `start` (**shorter** distance has a higher rank).
2. Price (**lower** price has a higher rank, but it must be **in the price range**).
3. The row number (**smaller** row number has a higher rank).
4. The column number (**smaller** column number has a higher rank).
Return _the_ `k` _highest-ranked items within the price range **sorted** by their rank (highest to lowest)_. If there are fewer than `k` reachable items within the price range, return _**all** of them_.
**Example 1:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,0,1\],\[0,2,5,1\]\], pricing = \[2,5\], start = \[0,0\], k = 3
**Output:** \[\[0,1\],\[1,1\],\[2,1\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,5\], we can take items from (0,1), (1,1), (2,1) and (2,2).
The ranks of these items are:
- (0,1) with distance 1
- (1,1) with distance 2
- (2,1) with distance 3
- (2,2) with distance 4
Thus, the 3 highest ranked items in the price range are (0,1), (1,1), and (2,1).
**Example 2:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,3,1\],\[0,2,5,1\]\], pricing = \[2,3\], start = \[2,3\], k = 2
**Output:** \[\[2,1\],\[1,2\]\]
**Explanation:** You start at (2,3).
With a price range of \[2,3\], we can take items from (0,1), (1,1), (1,2) and (2,1).
The ranks of these items are:
- (2,1) with distance 2, price 2
- (1,2) with distance 2, price 3
- (1,1) with distance 3
- (0,1) with distance 4
Thus, the 2 highest ranked items in the price range are (2,1) and (1,2).
**Example 3:**
**Input:** grid = \[\[1,1,1\],\[0,0,1\],\[2,3,4\]\], pricing = \[2,3\], start = \[0,0\], k = 3
**Output:** \[\[2,1\],\[2,0\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,3\], we can take items from (2,0) and (2,1).
The ranks of these items are:
- (2,1) with distance 5
- (2,0) with distance 6
Thus, the 2 highest ranked items in the price range are (2,1) and (2,0).
Note that k = 3 but there are only 2 reachable items within the price range.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 105`
* `1 <= m * n <= 105`
* `0 <= grid[i][j] <= 105`
* `pricing.length == 2`
* `2 <= low <= high <= 105`
* `start.length == 2`
* `0 <= row <= m - 1`
* `0 <= col <= n - 1`
* `grid[row][col] > 0`
* `1 <= k <= m * n` | Check all possible placements for the word. There is a limited number of places where a word can start. |
Quite Ordinary Approach | k-highest-ranked-items-within-a-price-range | 0 | 1 | # Complexity\n- Time complexity: $$O(n*log(n))$$, n - number of elements in the grid.\n\n- Space complexity: $$O(n)$$.\n\n# Code\n```\nclass Solution:\n def highestRankedKItems(self, grid: List[List[int]], pricing: List[int], start: List[int], k: int) -> List[List[int]]:\n m, n = len(grid), len(grid[0])\n result = []\n seen = {tuple(start)}\n q = deque([start])\n distance = 0\n while q and len(result) < k:\n for _ in range(len(q)):\n r, c = q.pop()\n if pricing[0] <= grid[r][c] <= pricing[1]:\n result.append((distance, grid[r][c], r, c))\n \n for rr, cc in ((r-1, c), (r+1, c), (r, c-1), (r, c+1)):\n if (0 <= rr < m) and (0 <= cc < n) and (rr, cc) not in seen and grid[rr][cc]:\n q.appendleft((rr, cc))\n seen.add((rr, cc))\n \n distance += 1\n\n result.sort()\n\n return [(r, c) for _, _, r, c in result[:k]]\n``` | 0 | You are given a **0-indexed** 2D integer array `grid` of size `m x n` that represents a map of the items in a shop. The integers in the grid represent the following:
* `0` represents a wall that you cannot pass through.
* `1` represents an empty cell that you can freely move to and from.
* All other positive integers represent the price of an item in that cell. You may also freely move to and from these item cells.
It takes `1` step to travel between adjacent grid cells.
You are also given integer arrays `pricing` and `start` where `pricing = [low, high]` and `start = [row, col]` indicates that you start at the position `(row, col)` and are interested only in items with a price in the range of `[low, high]` (**inclusive**). You are further given an integer `k`.
You are interested in the **positions** of the `k` **highest-ranked** items whose prices are **within** the given price range. The rank is determined by the **first** of these criteria that is different:
1. Distance, defined as the length of the shortest path from the `start` (**shorter** distance has a higher rank).
2. Price (**lower** price has a higher rank, but it must be **in the price range**).
3. The row number (**smaller** row number has a higher rank).
4. The column number (**smaller** column number has a higher rank).
Return _the_ `k` _highest-ranked items within the price range **sorted** by their rank (highest to lowest)_. If there are fewer than `k` reachable items within the price range, return _**all** of them_.
**Example 1:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,0,1\],\[0,2,5,1\]\], pricing = \[2,5\], start = \[0,0\], k = 3
**Output:** \[\[0,1\],\[1,1\],\[2,1\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,5\], we can take items from (0,1), (1,1), (2,1) and (2,2).
The ranks of these items are:
- (0,1) with distance 1
- (1,1) with distance 2
- (2,1) with distance 3
- (2,2) with distance 4
Thus, the 3 highest ranked items in the price range are (0,1), (1,1), and (2,1).
**Example 2:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,3,1\],\[0,2,5,1\]\], pricing = \[2,3\], start = \[2,3\], k = 2
**Output:** \[\[2,1\],\[1,2\]\]
**Explanation:** You start at (2,3).
With a price range of \[2,3\], we can take items from (0,1), (1,1), (1,2) and (2,1).
The ranks of these items are:
- (2,1) with distance 2, price 2
- (1,2) with distance 2, price 3
- (1,1) with distance 3
- (0,1) with distance 4
Thus, the 2 highest ranked items in the price range are (2,1) and (1,2).
**Example 3:**
**Input:** grid = \[\[1,1,1\],\[0,0,1\],\[2,3,4\]\], pricing = \[2,3\], start = \[0,0\], k = 3
**Output:** \[\[2,1\],\[2,0\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,3\], we can take items from (2,0) and (2,1).
The ranks of these items are:
- (2,1) with distance 5
- (2,0) with distance 6
Thus, the 2 highest ranked items in the price range are (2,1) and (2,0).
Note that k = 3 but there are only 2 reachable items within the price range.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 105`
* `1 <= m * n <= 105`
* `0 <= grid[i][j] <= 105`
* `pricing.length == 2`
* `2 <= low <= high <= 105`
* `start.length == 2`
* `0 <= row <= m - 1`
* `0 <= col <= n - 1`
* `grid[row][col] > 0`
* `1 <= k <= m * n` | Check all possible placements for the word. There is a limited number of places where a word can start. |
Python BFSb | k-highest-ranked-items-within-a-price-range | 0 | 1 | # Code\n```\nclass Solution:\n def highestRankedKItems(self, grid: List[List[int]], pricing: List[int], start: List[int], k: int) -> List[List[int]]:\n if not grid:\n return []\n \n m, n = len(grid), len(grid[0])\n d = [[0,1], [0,-1], [1,0], [-1,0]]\n h = []\n queue = [[start[0], start[1]]]\n step = 0\n\n while queue:\n nextQ = []\n\n for [i, j] in queue:\n if 0 <= i < m and 0 <= j < n and grid[i][j]:\n p = grid[i][j]\n grid[i][j] = 0\n\n if p >= pricing[0] and p <= pricing[1]:\n heapq.heappush(h, (step, p, i, j))\n\n for [a, b] in d:\n x, y = i+a, j+b\n\n if x >= 0 and x < m and y >= 0 and y < n and grid[x][y] != 0:\n nextQ.append([x, y])\n\n queue = nextQ\n step += 1\n\n if len(h) >= k:\n break\n\n res = heapq.nsmallest(k, h)\n return [[i, j] for (step, p, i, j) in res]\n``` | 0 | You are given a **0-indexed** 2D integer array `grid` of size `m x n` that represents a map of the items in a shop. The integers in the grid represent the following:
* `0` represents a wall that you cannot pass through.
* `1` represents an empty cell that you can freely move to and from.
* All other positive integers represent the price of an item in that cell. You may also freely move to and from these item cells.
It takes `1` step to travel between adjacent grid cells.
You are also given integer arrays `pricing` and `start` where `pricing = [low, high]` and `start = [row, col]` indicates that you start at the position `(row, col)` and are interested only in items with a price in the range of `[low, high]` (**inclusive**). You are further given an integer `k`.
You are interested in the **positions** of the `k` **highest-ranked** items whose prices are **within** the given price range. The rank is determined by the **first** of these criteria that is different:
1. Distance, defined as the length of the shortest path from the `start` (**shorter** distance has a higher rank).
2. Price (**lower** price has a higher rank, but it must be **in the price range**).
3. The row number (**smaller** row number has a higher rank).
4. The column number (**smaller** column number has a higher rank).
Return _the_ `k` _highest-ranked items within the price range **sorted** by their rank (highest to lowest)_. If there are fewer than `k` reachable items within the price range, return _**all** of them_.
**Example 1:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,0,1\],\[0,2,5,1\]\], pricing = \[2,5\], start = \[0,0\], k = 3
**Output:** \[\[0,1\],\[1,1\],\[2,1\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,5\], we can take items from (0,1), (1,1), (2,1) and (2,2).
The ranks of these items are:
- (0,1) with distance 1
- (1,1) with distance 2
- (2,1) with distance 3
- (2,2) with distance 4
Thus, the 3 highest ranked items in the price range are (0,1), (1,1), and (2,1).
**Example 2:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,3,1\],\[0,2,5,1\]\], pricing = \[2,3\], start = \[2,3\], k = 2
**Output:** \[\[2,1\],\[1,2\]\]
**Explanation:** You start at (2,3).
With a price range of \[2,3\], we can take items from (0,1), (1,1), (1,2) and (2,1).
The ranks of these items are:
- (2,1) with distance 2, price 2
- (1,2) with distance 2, price 3
- (1,1) with distance 3
- (0,1) with distance 4
Thus, the 2 highest ranked items in the price range are (2,1) and (1,2).
**Example 3:**
**Input:** grid = \[\[1,1,1\],\[0,0,1\],\[2,3,4\]\], pricing = \[2,3\], start = \[0,0\], k = 3
**Output:** \[\[2,1\],\[2,0\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,3\], we can take items from (2,0) and (2,1).
The ranks of these items are:
- (2,1) with distance 5
- (2,0) with distance 6
Thus, the 2 highest ranked items in the price range are (2,1) and (2,0).
Note that k = 3 but there are only 2 reachable items within the price range.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 105`
* `1 <= m * n <= 105`
* `0 <= grid[i][j] <= 105`
* `pricing.length == 2`
* `2 <= low <= high <= 105`
* `start.length == 2`
* `0 <= row <= m - 1`
* `0 <= col <= n - 1`
* `grid[row][col] > 0`
* `1 <= k <= m * n` | Check all possible placements for the word. There is a limited number of places where a word can start. |
Level wise BFS for each cell #Python3 || 86% beats | k-highest-ranked-items-within-a-price-range | 0 | 1 | # Intuition\nSince we want to return k highest ranked items, and for determining rank we need to use (level, price, row, col). The shortest the distance better. Because of this I decided to use BFS to track the shortest distance starting from start point. So we will explore neighbors level by level and add them to the heap in this tuple format (level,price, row, col).\nhere the level is the distance from the starting point\nwe will take Top K prices based on distances.\n# Approach\nApproach\nInitiate visited set, queue for BFS, and items array keeping items explored\nAdd the starting point values to visited, queue, and items\nExplore from the starting point with BFS level by level. \nAdd them to the heap in this tuple format (level,price, row, col).\nhere the level is the distance from the starting point\nwe will take Top K prices based on distances\n\n\n\n# Complexity\n\nTime Complexity: O(MNlogK)\nFor exploring each cell with BFS: O(MN)\n\n\n- Space complexity:\nSpace complexity: O(MN)\nFor visited set and queue: O(MN)\n\n\n# Code\n```\nclass Solution:\n def highestRankedKItems(self, grid: List[List[int]], pricing: List[int], start: List[int], k: int) -> List[List[int]]:\n direc=[(0,1),(1,0),(-1,0),(0,-1)]\n n=len(grid)\n m=len(grid[0])\n\n\n que=[(start[0],start[1])]\n visit=set()\n visit.add((start[0],start[1]))\n\n heap=[]\n if pricing[0]<=grid[start[0]][start[1]]<=pricing[1]:\n heapq.heappush(heap,(0,grid[start[0]][start[1]],start[0],start[1]))\n stage=0\n while que:\n level=[]\n stage+=1\n \n\n for _ in range(len(que)):\n i,j= que.pop(0)\n for a,b in direc:\n r=i+a\n c=j+b\n if 0<=r<n and 0<=c<m and grid[r][c]!=0 and (r,c) not in visit:\n\n que.append((r,c))\n visit.add((r,c))\n if pricing[0]<=grid[r][c]<=pricing[1]:\n heapq.heappush(heap,((stage,grid[r][c],r,c)))\n \n\n \n # print(heap)\n if heap:\n res=[]\n for i in range(k):\n if heap:\n dis,val,r,c =heapq.heappop(heap)\n res.append([r,c])\n return res\n return []\n\n\n\n\n\n\n``` | 0 | You are given a **0-indexed** 2D integer array `grid` of size `m x n` that represents a map of the items in a shop. The integers in the grid represent the following:
* `0` represents a wall that you cannot pass through.
* `1` represents an empty cell that you can freely move to and from.
* All other positive integers represent the price of an item in that cell. You may also freely move to and from these item cells.
It takes `1` step to travel between adjacent grid cells.
You are also given integer arrays `pricing` and `start` where `pricing = [low, high]` and `start = [row, col]` indicates that you start at the position `(row, col)` and are interested only in items with a price in the range of `[low, high]` (**inclusive**). You are further given an integer `k`.
You are interested in the **positions** of the `k` **highest-ranked** items whose prices are **within** the given price range. The rank is determined by the **first** of these criteria that is different:
1. Distance, defined as the length of the shortest path from the `start` (**shorter** distance has a higher rank).
2. Price (**lower** price has a higher rank, but it must be **in the price range**).
3. The row number (**smaller** row number has a higher rank).
4. The column number (**smaller** column number has a higher rank).
Return _the_ `k` _highest-ranked items within the price range **sorted** by their rank (highest to lowest)_. If there are fewer than `k` reachable items within the price range, return _**all** of them_.
**Example 1:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,0,1\],\[0,2,5,1\]\], pricing = \[2,5\], start = \[0,0\], k = 3
**Output:** \[\[0,1\],\[1,1\],\[2,1\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,5\], we can take items from (0,1), (1,1), (2,1) and (2,2).
The ranks of these items are:
- (0,1) with distance 1
- (1,1) with distance 2
- (2,1) with distance 3
- (2,2) with distance 4
Thus, the 3 highest ranked items in the price range are (0,1), (1,1), and (2,1).
**Example 2:**
**Input:** grid = \[\[1,2,0,1\],\[1,3,3,1\],\[0,2,5,1\]\], pricing = \[2,3\], start = \[2,3\], k = 2
**Output:** \[\[2,1\],\[1,2\]\]
**Explanation:** You start at (2,3).
With a price range of \[2,3\], we can take items from (0,1), (1,1), (1,2) and (2,1).
The ranks of these items are:
- (2,1) with distance 2, price 2
- (1,2) with distance 2, price 3
- (1,1) with distance 3
- (0,1) with distance 4
Thus, the 2 highest ranked items in the price range are (2,1) and (1,2).
**Example 3:**
**Input:** grid = \[\[1,1,1\],\[0,0,1\],\[2,3,4\]\], pricing = \[2,3\], start = \[0,0\], k = 3
**Output:** \[\[2,1\],\[2,0\]\]
**Explanation:** You start at (0,0).
With a price range of \[2,3\], we can take items from (2,0) and (2,1).
The ranks of these items are:
- (2,1) with distance 5
- (2,0) with distance 6
Thus, the 2 highest ranked items in the price range are (2,1) and (2,0).
Note that k = 3 but there are only 2 reachable items within the price range.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 105`
* `1 <= m * n <= 105`
* `0 <= grid[i][j] <= 105`
* `pricing.length == 2`
* `2 <= low <= high <= 105`
* `start.length == 2`
* `0 <= row <= m - 1`
* `0 <= col <= n - 1`
* `grid[row][col] > 0`
* `1 <= k <= m * n` | Check all possible placements for the word. There is a limited number of places where a word can start. |
【Video】Give me 7 minutes - How we think about a solution | number-of-ways-to-divide-a-long-corridor | 1 | 1 | # Intuition\nConsider two seats as a pair.\n\n---\n\n# Solution Video\n\nhttps://youtu.be/DF9NXNW0G6E\n\n\u25A0 Timeline of the video\n\n`0:05` Return 0\n`0:38` Think about a case where we can put dividers\n`4:44` Coding\n`7:18` Time Complexity and Space Complexity\n`7:33` Summary of the algorithm with my solution code\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 3,258\nMy first goal is 10,000 (It\'s far from done \uD83D\uDE05)\nThank you for your support!\n\n**My channel reached 3,000 subscribers these days. Thank you so much for your support!**\n\n---\n\n# Approach\n## How we think about a solution\n\n\nLet\'s think about simple cases\n```\nInput: corridor = "SSPS"\n```\nIn this case, we can\'t create division with `2` seats because we have `3` seats in the corridor.\n\nHow about this?\n```\nInput: corridor = "SSPSPSS"\n```\nIn this case, we can\'t create division with `2` seats because we have `5` seats in the corridor.\n\n---\n\n\u2B50\uFE0F Points\n\nIf we have odd number of seats, return 0\n\n---\n\nLet\'s think about this case.\n```\nInput: corridor = "SSPPSPS"\n```\nWe need to have `2` seats in each division. In other words the first `2` seats should be a pair and third seat and fourth seat also should be pair.\n\nThat\'s why we can put dividers only between pairs.\n```\n"SS|PPSPS"\n"SSP|PSPS"\n"SSPP|SPS"\n```\n```\nOutput: 3\n```\n- How can you calculate the output?\n\nWe can put divider between pairs. In the above example, we have `2` plants between pairs and output is `3`. What if we have `1` plant between pairs\n```\nInput: corridor = "SSPSPS"\n```\n```\n"SS|PSPS"\n"SSP|SPS"\n```\n```\nOutput: 2\n```\nSo, number of divider should be `number of plants + 1`. More precisely, we can say `index of thrid seat - index of second seat` is the same as `number of plants + 1`.\n\n```\n 0123456\n"SSPPSPS"\n \u2191 \u2191\n\n4 - 1 = 3\n\n 012345\n"SSPSPS"\n \u2191 \u2191\n\n3 - 1 = 2\n```\nLet\'s say we have `2` ways(x and y) to put dividers so far and find `3` new ways(a,b and c) to put dividers, in that case, we have `6` ways to put dividers.\n\n```\nx + a\ny + a\nx + b\ny + b\nx + c\ny + c\n= 6 ways\n```\n\n\n---\n\n\u2B50\uFE0F Points\n\nEvery time we find new ways, we should multiply number of new ways with current number of ways. That happens when we have more than 2 seats and find odd number of seats (= between pairs).\n\n---\n\nI think we talk about main points.\nLet\'s see a real algorithm!\n\n\n---\n\n\n\n### 1. Initialization:\n\n#### Code:\n```python\nmod = 10**9 + 7 \nres = 1\nprev_seat = 0\nnum_seats = 0\n```\n#### Explanation:\n- Set `mod` to `10**9 + 7` as the modulo value for the final result.\n- Initialize `res` to 1. This variable will be updated during iteration and represents the result.\n- Initialize `prev_seat` to 0. This variable tracks the index of the previous seat encountered.\n- Initialize `num_seats` to 0. This variable counts the number of seats encountered.\n\n### 2. Iteration through the Corridor:\n\n#### Code:\n```python\nfor i, c in enumerate(corridor):\n if c == \'S\':\n num_seats += 1\n\n if num_seats > 2 and num_seats % 2 == 1:\n res *= i - prev_seat\n\n prev_seat = i\n```\n#### Explanation:\n- Use a for loop to iterate through each character `c` and its index `i` in the corridor.\n- Check if the character `c` is equal to \'S\', indicating the presence of a seat.\n - Increment `num_seats` by 1, as a seat is encountered.\n - Check if there are more than 2 consecutive seats and an odd number of seats.\n - If the condition is met, update `res` by multiplying it with the distance between the current seat and the previous seat (`i - prev_seat`).\n - Update `prev_seat` to the current index `i`.\n\n### 3. Final Result:\n\n#### Code:\n```python\nreturn res % mod if num_seats > 1 and num_seats % 2 == 0 else 0\n```\n\n#### Explanation:\n- Return the final result, calculated as `res % mod`, only if there are more than 1 seat and an even number of seats. Otherwise, return 0.\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n```python []\nclass Solution:\n def numberOfWays(self, corridor: str) -> int:\n mod = 10**9 + 7 # Define the modulo value for the final result\n res = 1 # Initialize the result variable to 1 (will be updated during iteration)\n prev_seat = 0 # Initialize the variable to track the index of the previous seat\n num_seats = 0 # Initialize the variable to count the number of seats encountered\n\n for i, c in enumerate(corridor):\n if c == \'S\':\n num_seats += 1 # Increment the seat count when \'S\' is encountered\n # Check if there are more than 2 consecutive seats and an odd number of seats\n if num_seats > 2 and num_seats % 2 == 1:\n # Update the answer using the distance between the current seat and the previous seat\n res *= i - prev_seat\n prev_seat = i # Update the previous seat index to the current index\n\n # Return the answer only if there are more than 1 seat and an even number of seats\n return res % mod if num_seats > 1 and num_seats % 2 == 0 else 0\n```\n```javascript []\n/**\n * @param {string} corridor\n * @return {number}\n */\nvar numberOfWays = function(corridor) {\n const mod = 1000000007; // Define the modulo value for the final result\n let res = 1; // Initialize the result variable to 1 (will be updated during iteration)\n let prevSeat = 0; // Initialize the variable to track the index of the previous seat\n let numSeats = 0; // Initialize the variable to count the number of seats encountered\n\n for (let i = 0; i < corridor.length; i++) {\n const c = corridor.charAt(i);\n if (c === \'S\') {\n numSeats += 1; // Increment the seat count when \'S\' is encountered\n // Check if there are more than 2 consecutive seats and an odd number of seats\n if (numSeats > 2 && numSeats % 2 === 1) {\n // Update the answer using the distance between the current seat and the previous seat\n res = res * (i - prevSeat) % mod;\n }\n prevSeat = i; // Update the previous seat index to the current index\n }\n }\n\n // Return the answer only if there are more than 1 seat and an even number of seats\n return numSeats > 1 && numSeats % 2 === 0 ? res : 0; \n};\n```\n```java []\npublic class Solution {\n public int numberOfWays(String corridor) {\n final int mod = 1000000007; // Define the modulo value for the final result\n long res = 1; // Initialize the result variable to 1 (will be updated during iteration)\n int prevSeat = 0; // Initialize the variable to track the index of the previous seat\n int numSeats = 0; // Initialize the variable to count the number of seats encountered\n\n for (int i = 0; i < corridor.length(); i++) {\n char c = corridor.charAt(i);\n if (c == \'S\') {\n numSeats += 1; // Increment the seat count when \'S\' is encountered\n // Check if there are more than 2 consecutive seats and an odd number of seats\n if (numSeats > 2 && numSeats % 2 == 1) {\n // Update the answer using the distance between the current seat and the previous seat\n res = res * (i - prevSeat) % mod;\n }\n prevSeat = i; // Update the previous seat index to the current index\n }\n }\n\n // Return the answer only if there are more than 1 seat and an even number of seats\n return numSeats > 1 && numSeats % 2 == 0 ? (int)res : 0;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int numberOfWays(string corridor) {\n const int mod = 1000000007; // Define the modulo value for the final result\n long res = 1; // Initialize the result variable to 1 (will be updated during iteration)\n int prevSeat = 0; // Initialize the variable to track the index of the previous seat\n int numSeats = 0; // Initialize the variable to count the number of seats encountered\n\n for (int i = 0; i < corridor.length(); i++) {\n char c = corridor[i];\n if (c == \'S\') {\n numSeats += 1; // Increment the seat count when \'S\' is encountered\n // Check if there are more than 2 consecutive seats and an odd number of seats\n if (numSeats > 2 && numSeats % 2 == 1) {\n // Update the answer using the distance between the current seat and the previous seat\n res = res * (i - prevSeat) % mod; \n }\n prevSeat = i; // Update the previous seat index to the current index\n }\n }\n\n // Return the answer only if there are more than 1 seat and an even number of seats\n return numSeats > 1 && numSeats % 2 == 0 ? res : 0; \n }\n};\n```\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u25A0 Subscribe URL\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\n\u25A0 Twitter\nhttps://twitter.com/CodingNinjaAZ\n\n### My next daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/number-of-1-bits/solutions/4343578/video-give-me-5-minutes-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/3Vv1jvWc6eg\n\n\u25A0 Timeline of the video\n\n`0:03` Right shift\n`1:36` Bitwise AND\n`3:09` Demonstrate how it works\n`6:03` Coding\n`6:38` Time Complexity and Space Complexity\n\n### My previous daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/largest-submatrix-with-rearrangements/solutions/4331142/video-give-me-10-minutes-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/odAv92zWKqs\n\n\u25A0 Timeline of the video\n\n`0:05` How we can move columns\n`1:09` Calculate height\n`2:48` Calculate width\n`4:10` Let\'s make sure it works\n`5:40` Demonstrate how it works\n`7:44` Coding\n`9:59` Time Complexity and Space Complexity\n`10:35` Summary of the algorithm with my solution code\n | 15 | Along a long library corridor, there is a line of seats and decorative plants. You are given a **0-indexed** string `corridor` of length `n` consisting of letters `'S'` and `'P'` where each `'S'` represents a seat and each `'P'` represents a plant.
One room divider has **already** been installed to the left of index `0`, and **another** to the right of index `n - 1`. Additional room dividers can be installed. For each position between indices `i - 1` and `i` (`1 <= i <= n - 1`), at most one divider can be installed.
Divide the corridor into non-overlapping sections, where each section has **exactly two seats** with any number of plants. There may be multiple ways to perform the division. Two ways are **different** if there is a position with a room divider installed in the first way but not in the second way.
Return _the number of ways to divide the corridor_. Since the answer may be very large, return it **modulo** `109 + 7`. If there is no way, return `0`.
**Example 1:**
**Input:** corridor = "SSPPSPS "
**Output:** 3
**Explanation:** There are 3 different ways to divide the corridor.
The black bars in the above image indicate the two room dividers already installed.
Note that in each of the ways, **each** section has exactly **two** seats.
**Example 2:**
**Input:** corridor = "PPSPSP "
**Output:** 1
**Explanation:** There is only 1 way to divide the corridor, by not installing any additional dividers.
Installing any would create some section that does not have exactly two seats.
**Example 3:**
**Input:** corridor = "S "
**Output:** 0
**Explanation:** There is no way to divide the corridor because there will always be a section that does not have exactly two seats.
**Constraints:**
* `n == corridor.length`
* `1 <= n <= 105`
* `corridor[i]` is either `'S'` or `'P'`. | The number of operators in the equation is less. Could you find the right answer then generate all possible answers using different orders of operations? Divide the equation into blocks separated by the operators, and use memoization on the results of blocks for optimization. Use set and the max limit of the answer for further optimization. |
multiply plant counts | number-of-ways-to-divide-a-long-corridor | 0 | 1 | One possible solution can use the fact that the ans is the multiplication of - no of consecutive plants between seats (when numbered 1 indexed ) n and n +1 ( where n = 2,4,6 ... )\n\nS P S **P** S P S **P** S P S\n\nThe two bold Ps above has 2 possible solutions, ans would be 2*2 = 4\n\n\n```\nclass Solution:\n def numberOfWays(self, corridor: str) -> int:\n seatsToLeft = 0 \n consecutivePlants = 0\n ans = 1\n \n for c in corridor:\n if c == "P":\n if seatsToLeft and seatsToLeft % 2 == 0:\n consecutivePlants += 1\n else:\n seatsToLeft += 1\n \n if seatsToLeft % 2 == 1 and consecutivePlants != 0:\n ans *= (consecutivePlants+1)\n consecutivePlants = 0\n \n return ans%(10**9+7) if (seatsToLeft and seatsToLeft%2 == 0) else 0\n \n | 4 | Along a long library corridor, there is a line of seats and decorative plants. You are given a **0-indexed** string `corridor` of length `n` consisting of letters `'S'` and `'P'` where each `'S'` represents a seat and each `'P'` represents a plant.
One room divider has **already** been installed to the left of index `0`, and **another** to the right of index `n - 1`. Additional room dividers can be installed. For each position between indices `i - 1` and `i` (`1 <= i <= n - 1`), at most one divider can be installed.
Divide the corridor into non-overlapping sections, where each section has **exactly two seats** with any number of plants. There may be multiple ways to perform the division. Two ways are **different** if there is a position with a room divider installed in the first way but not in the second way.
Return _the number of ways to divide the corridor_. Since the answer may be very large, return it **modulo** `109 + 7`. If there is no way, return `0`.
**Example 1:**
**Input:** corridor = "SSPPSPS "
**Output:** 3
**Explanation:** There are 3 different ways to divide the corridor.
The black bars in the above image indicate the two room dividers already installed.
Note that in each of the ways, **each** section has exactly **two** seats.
**Example 2:**
**Input:** corridor = "PPSPSP "
**Output:** 1
**Explanation:** There is only 1 way to divide the corridor, by not installing any additional dividers.
Installing any would create some section that does not have exactly two seats.
**Example 3:**
**Input:** corridor = "S "
**Output:** 0
**Explanation:** There is no way to divide the corridor because there will always be a section that does not have exactly two seats.
**Constraints:**
* `n == corridor.length`
* `1 <= n <= 105`
* `corridor[i]` is either `'S'` or `'P'`. | The number of operators in the equation is less. Could you find the right answer then generate all possible answers using different orders of operations? Divide the equation into blocks separated by the operators, and use memoization on the results of blocks for optimization. Use set and the max limit of the answer for further optimization. |
✅☑[C++/Java/Python/JavaScript] || 5 Approaches || DP || EXPLAINED🔥 | number-of-ways-to-divide-a-long-corridor | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n**(Also explained in the code)**\n\n#### ***Approach 1(Top Dowm DP)***\n1. **count function:**\n\n - This function is responsible for counting the number of valid ways to divide the corridor string into sections with exactly two \'S\'s.\n - It uses dynamic programming (DP) with memoization to avoid recomputing results for already solved sub-problems.\n - Parameters:\n - **index:** Tracks the current position in the corridor string.\n - **seats:** Tracks the number of \'S\'s encountered in the current section (limited to 2 for a valid section).\n - **corridor:** The input corridor string.\n - **cache:** A 2D array storing previously computed results for sub-problems.\n1. **Base Cases:**\n\n - If the function reaches the end of the corridor string, it returns 1 if `seats` is 2 (indicating a valid section) and 0 otherwise.\n1. **Memoization:**\n\n - It checks the cache to see if the result for the current sub-problem (identified by `index` and `seats`) has already been computed. If so, it returns the cached result.\n1. **Calculating the result:**\n\n - Depending on the current character (\'S\' or \'P\') and the count of \'S\'s encountered (`seats`), the function computes the number of valid ways to proceed further.\n - If there are already 2 \'S\'s in the current section:\n - If the current character is \'S\', it closes the section and starts a new section with one \'S\' from the next index.\n - If the current character is \'P\', it has two options: close the section or continue growing it.\n - If there are less than 2 \'S\'s in the current section:\n - If the current character is \'S\', it increments the `seats` count and continues growing the section.\n - If the current character is \'P\', it continues growing the section without changing the count of \'S\'s.\n1. **Memoization of result:**\n\n - Once the result for the sub-problem is calculated, it is stored in the cache for future reference to avoid redundant calculations.\n1. **numberOfWays function:**\n\n - Initializes the cache array and calls the `count` function with initial parameters.\n\n\n\n# Complexity\n- *Time complexity:*\n $$O(n)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n // Store 1000000007 in a variable for convenience\n const int MOD = 1e9 + 7;\n\n // Count the number of ways to divide from "index" to the last index\n // with "seats" number of "S" in the current section\n int count(int index, int seats, string& corridor, int cache[][3]) {\n // If we have reached the end of the corridor,\n // the current section is valid only if "seats" is 2\n if (index == corridor.length()) {\n return seats == 2 ? 1 : 0; // If seats is 2, return 1; otherwise, return 0\n }\n\n // If we have already computed the result of this sub-problem,\n // then return the cached result\n if (cache[index][seats] != -1) {\n return cache[index][seats]; // Return the previously calculated result\n }\n\n // Result of the sub-problem\n int result = 0;\n\n // If the current section has exactly 2 "S"\n if (seats == 2) {\n // If the current element is "S", then close the section and start a new section from this index.\n // Next index will have one "S" in the current section\n if (corridor[index] == \'S\') {\n result = count(index + 1, 1, corridor, cache); // Start a new section with one "S"\n } else {\n // If the current element is "P", then we have two options:\n // 1. Close the section and start a new section from this index\n // 2. Keep growing the section\n result = (count(index + 1, 0, corridor, cache) + count(index + 1, 2, corridor, cache)) % MOD; \n // Add results of starting new section or extending the current one\n // Use MOD to handle large numbers and avoid overflow\n }\n } else {\n // Keep growing the section. Increment "seats" if the present element is "S"\n if (corridor[index] == \'S\') {\n result = count(index + 1, seats + 1, corridor, cache); // Extend the current section\n } else {\n result = count(index + 1, seats, corridor, cache); // Continue the current section\n }\n }\n\n // Memoize the result for future use and return it\n cache[index][seats] = result; // Store the result for future use\n return cache[index][seats]; // Return the result\n }\n\n int numberOfWays(string corridor) {\n // Cache the result of each sub-problem\n int cache[corridor.length()][3];\n memset(cache, -1, sizeof(cache)); // Initialize cache with -1 values\n\n // Call the count function\n return count(0, 0, corridor, cache); // Start counting from the beginning of the corridor\n }\n};\n\n\n\n```\n```C []\n\n// Count the number of ways to divide from "index" to the last index\n// with "seats" number of "S" in the current section\nint count(int index, int seats, char * corridor, int cache[][3]) {\n // If we have reached the end of the corridor, then\n // the current section is valid only if "seats" is 2\n if (index == strlen(corridor)) {\n return seats == 2 ? 1 : 0;\n }\n\n // If we have already computed the result of this sub-problem,\n // then return the cached result\n if (cache[index][seats] != -1) {\n return cache[index][seats];\n }\n\n // Result of the sub-problem\n int result = 0;\n\n // If the current section has exactly 2 "S"\n if (seats == 2) {\n // If the current element is "S", then we have to close the\n // section and start a new section from this index. Next index\n // will have one "S" in the current section\n if (corridor[index] == \'S\') {\n result = count(index + 1, 1, corridor, cache);\n } else {\n // If the current element is "P", then we have two options\n // 1. Close the section and start a new section from this index\n // 2. Keep growing the section\n result = (count(index + 1, 0, corridor, cache) + count(index + 1, 2, corridor, cache)) % 1000000007; \n }\n } else {\n // Keep growing the section. Increment "seats" if present\n // element is "S"\n if (corridor[index] == \'S\') {\n result = count(index + 1, seats + 1, corridor, cache);\n } else {\n result = count(index + 1, seats, corridor, cache);\n }\n }\n\n // Memoize the result, and return it\n cache[index][seats] = result;\n return cache[index][seats];\n}\n\nint numberOfWays(char * corridor){\n // Cache the result of each sub-problem\n int cache[strlen(corridor)][3];\n memset(cache, -1, sizeof(cache));\n\n // Call the count function\n return count(0, 0, corridor, cache);\n}\n\n\n```\n```Java []\n\nclass Solution {\n // Store 1000000007 in a variable for convenience\n private static final int MOD = 1_000_000_007;\n\n // Count the number of ways to divide from "index" to the last index\n // with "seats" number of "S" in the current section\n private int count(int index, int seats, String corridor, Map<Pair<Integer, Integer>, Integer> cache) {\n // If we have reached the end of the corridor, then\n // the current section is valid only if "seats" is 2\n if (index == corridor.length()) {\n return seats == 2 ? 1 : 0;\n }\n\n // If we have already computed the result of this sub-problem,\n // then return the cached result\n if (cache.containsKey(new Pair<>(index, seats))) {\n return cache.get(new Pair<>(index, seats));\n }\n\n // Result of the sub-problem\n int result = 0;\n\n // If the current section has exactly 2 "S"\n if (seats == 2) {\n // If the current element is "S", then we have to close the\n // section and start a new section from this index. Next index\n // will have one "S" in the current section\n if (corridor.charAt(index) == \'S\') {\n result = count(index + 1, 1, corridor, cache);\n } else {\n // If the current element is "P", then we have two options\n // 1. Close the section and start a new section from this index\n // 2. Keep growing the section\n result = (count(index + 1, 0, corridor, cache) + count(index + 1, 2, corridor, cache)) % MOD; \n }\n } else {\n // Keep growing the section. Increment "seats" if present\n // element is "S"\n if (corridor.charAt(index) == \'S\') {\n result = count(index + 1, seats + 1, corridor, cache);\n } else {\n result = count(index + 1, seats, corridor, cache);\n }\n }\n\n // Memoize the result, and return it\n cache.put(new Pair<>(index, seats), result);\n return result;\n }\n\n\n public int numberOfWays(String corridor) {\n // Cache the result of each sub-problem\n Map<Pair<Integer, Integer>, Integer> cache = new HashMap<>();\n\n // Call the count function\n return count(0, 0, corridor, cache);\n }\n}\n\n```\n```python3 []\n\nclass Solution:\n def numberOfWays(self, corridor: str) -> int:\n # Store 1000000007 in a variable for convenience\n MOD = 1_000_000_007\n\n # Cache the result of each sub-problem\n cache = [[-1] * 3 for _ in range(len(corridor))]\n\n # Count the number of ways to divide from "index" to the last index\n # with "seats" number of "S" in the current section\n def count(index, seats):\n # If we have reached the end of the corridor, then\n # the current section is valid only if "seats" is 2\n if index == len(corridor):\n return 1 if seats == 2 else 0\n\n # If we have already computed the result of this sub-problem,\n # then return the cached result\n if cache[index][seats] != -1:\n return cache[index][seats]\n \n # If the current section has exactly 2 "S"\n if seats == 2:\n # If the current element is "S", then we have to close the\n # section and start a new section from this index. Next index\n # will have one "S" in the current section\n if corridor[index] == "S":\n result = count(index + 1, 1)\n else:\n # If the current element is "P", then we have two options\n # 1. Close the section and start a new section from this index\n # 2. Keep growing the section\n result = (count(index + 1, 0) + count(index + 1, 2)) % MOD\n else:\n # Keep growing the section. Increment "seats" if present\n # element is "S"\n if corridor[index] == "S":\n result = count(index + 1, seats + 1)\n else:\n result = count(index + 1, seats)\n \n # Memoize the result, and return it\n cache[index][seats] = result\n return cache[index][seats]\n \n # Call the count function\n return count(0, 0)\n\n```\n```javascript []\nvar numberOfWays = function(corridor) {\n // Store 1000000007 in a variable for convenience\n const MOD = 1e9 + 7;\n \n // Cache the result of each sub-problem\n const cache = new Map();\n\n // Count the number of ways to divide from "index" to the last index\n // with "seats" number of "S" in the current section\n const count = (index, seats) => {\n // If we have reached the end of the corridor, then\n // the current section is valid only if "seats" is 2\n if (index == corridor.length) {\n return seats == 2 ? 1 : 0;\n }\n\n // If we have already computed the result of this sub-problem,\n // then return the cached result\n if (cache.has(`${index}-${seats}`)) {\n return cache.get(`${index}-${seats}`);\n }\n\n // Result of the sub-problem\n let result = 0;\n\n // If the current section has exactly 2 "S"\n if (seats == 2) {\n // If the current element is "S", then we have to close the\n // section and start a new section from this index. Next index\n // will have one "S" in the current section\n if (corridor[index] == \'S\') {\n result = count(index + 1, 1);\n } else {\n // If the current element is "P", then we have two options\n // 1. Close the section and start a new section from this index\n // 2. Keep growing the section\n result = (count(index + 1, 0) + count(index + 1, 2)) % MOD; \n }\n } else {\n // Keep growing the section. Increment "seats" if present\n // element is "S"\n if (corridor[index] == \'S\') {\n result = count(index + 1, seats + 1);\n } else {\n result = count(index + 1, seats);\n }\n }\n\n // Memoize the result, and return it\n cache.set(`${index}-${seats}`, result);\n return result;\n }\n\n // Call the count function\n return count(0, 0);\n};\n\n```\n\n---\n\n#### ***Approach 2(Bottom Up DP)***\n\n1. **Initialization and Constants:**\n\n - The code initializes a constant `MOD` as 1e9 + 7 for convenience in handling modulo operations.\n - It sets up a 2D array `count` to store the results of sub-problems. The array has a size of `corridor.length() + 1` rows and 3 columns.\n1. **Base Cases:**\n\n - The base cases are set for the end of the corridor (`corridor.length()`) representing no more elements to process.\n - At the end, when there are no more elements, the array is initialized with values:\n - `count[corridor.length()][0] = 0`\n - `count[corridor.length()][1] = 0`\n - `count[corridor.length()][2] = 1`\n1. **Bottom-up DP Approach:**\n\n - The loop iterates through the corridor string from right to left (from the end towards the beginning).\n - For each position in the corridor:\n - If the current element is \'S\':\n - `count[index][0]` (represents sections without \'S\'s) is updated to the value of `count[index + 1][1]`.\n - `count[index][1]`(represents sections with one \'S\') is updated to the value of `count[index + 1][2]`.\n - `count[index][2]`(represents sections with two \'S\'s) is updated to the value of `count[index + 1][1]`.\n - If the current element is \'P\':\n - `count[index][0]` remains the same as `count[index + 1][0]`.\n - `count[index][1]` remains the same as `count[index + 1][1]`.\n - `count[index][2]` (sections with two \'S\'s) is updated to the sum of `count[index + 1][0]` and `count[index + 1][2]`, then modulo `MOD`.\n1. **Return Result:**\n\n - Finally, the function returns the value stored in `count[0][0]`, representing the number of ways to divide the corridor from the beginning (index 0) with no \'S\'s in the section.\n\n\n# Complexity\n- *Time complexity:*\n $$O(n)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int numberOfWays(string corridor) {\n // Store 1000000007 in a variable for convenience\n const int MOD = 1e9 + 7;\n\n // Initialize the array to store the result of each sub-problem\n int count[corridor.length() + 1][3];\n\n // Base cases\n count[corridor.length()][0] = 0;\n count[corridor.length()][1] = 0;\n count[corridor.length()][2] = 1;\n\n // Fill the array in a bottom-up fashion\n for (int index = corridor.length() - 1; index >= 0; index--) {\n if (corridor[index] == \'S\') {\n count[index][0] = count[index + 1][1];\n count[index][1] = count[index + 1][2];\n count[index][2] = count[index + 1][1];\n } else {\n count[index][0] = count[index + 1][0];\n count[index][1] = count[index + 1][1];\n count[index][2] = (count[index + 1][0] + count[index + 1][2]) % MOD;\n }\n }\n\n // Return the result\n return count[0][0];\n }\n};\n\n\n```\n```C []\nint numberOfWays(char * corridor){\n // Store 1000000007 in a variable for convenience\n const int MOD = 1e9 + 7;\n\n // Initialize the array to store the result of each sub-problem\n int count[strlen(corridor) + 1][3];\n\n // Base cases\n count[strlen(corridor)][0] = 0;\n count[strlen(corridor)][1] = 0;\n count[strlen(corridor)][2] = 1;\n\n // Fill the array in a bottom-up fashion\n for (int index = strlen(corridor) - 1; index >= 0; index--) {\n if (corridor[index] == \'S\') {\n count[index][0] = count[index + 1][1];\n count[index][1] = count[index + 1][2];\n count[index][2] = count[index + 1][1];\n } else {\n count[index][0] = count[index + 1][0];\n count[index][1] = count[index + 1][1];\n count[index][2] = (count[index + 1][0] + count[index + 1][2]) % MOD;\n }\n }\n\n // Return the result\n return count[0][0];\n}\n\n\n\n```\n```Java []\nclass Solution {\n public int numberOfWays(String corridor) {\n // Store 1000000007 in a variable for convenience\n final int MOD = 1_000_000_007;\n\n // Initialize the array to store the result of each sub-problem\n int[][] count = new int[corridor.length() + 1][3];\n\n // Base cases\n count[corridor.length()][0] = 0;\n count[corridor.length()][1] = 0;\n count[corridor.length()][2] = 1;\n\n // Fill the array in a bottom-up fashion\n for (int index = corridor.length() - 1; index >= 0; index--) {\n if (corridor.charAt(index) == \'S\') {\n count[index][0] = count[index + 1][1];\n count[index][1] = count[index + 1][2];\n count[index][2] = count[index + 1][1];\n } else {\n count[index][0] = count[index + 1][0];\n count[index][1] = count[index + 1][1];\n count[index][2] = (count[index + 1][0] + count[index + 1][2]) % MOD;\n }\n }\n\n // Return the result\n return count[0][0];\n }\n}\n\n\n```\n```python3 []\n\nclass Solution:\n def numberOfWays(self, corridor: str) -> int:\n # Store 1000000007 in a variable for convenience\n MOD = 1_000_000_007\n\n # Initialize the array to store the result of each sub-problem\n count = [[-1] * 3 for _ in range(len(corridor) + 1)]\n\n # Base cases\n count[len(corridor)][0] = 0\n count[len(corridor)][1] = 0\n count[len(corridor)][2] = 1\n\n # Fill the array in a bottom-up fashion\n for index in range(len(corridor) - 1, -1, -1):\n if corridor[index] == "S":\n count[index][0] = count[index + 1][1]\n count[index][1] = count[index + 1][2]\n count[index][2] = count[index + 1][1]\n else:\n count[index][0] = count[index + 1][0]\n count[index][1] = count[index + 1][1]\n count[index][2] = (count[index + 1][0] + count[index + 1][2]) % MOD\n\n # Return the result\n return count[0][0]\n\n```\n```javascript []\nvar numberOfWays = function(corridor) {\n // Store 1000000007 in a variable for convenience\n const MOD = 1e9 + 7;\n \n // Initialize the array to store the result of each sub-problem\n const count = new Array(corridor.length + 1).fill(0).map(() => new Array(3).fill(0));\n\n // Base cases\n count[corridor.length][0] = 0;\n count[corridor.length][1] = 0;\n count[corridor.length][2] = 1;\n\n // Fill the array in a bottom-up fashion\n for (let index = corridor.length - 1; index >= 0; index--) {\n if (corridor[index] == \'S\') {\n count[index][0] = count[index + 1][1];\n count[index][1] = count[index + 1][2];\n count[index][2] = count[index + 1][1];\n } else {\n count[index][0] = count[index + 1][0];\n count[index][1] = count[index + 1][1];\n count[index][2] = (count[index + 1][0] + count[index + 1][2]) % MOD;\n }\n }\n\n // Return the result\n return count[0][0];\n};\n\n```\n\n---\n#### ***Approach 3(Space Optimized Bottom Up DP)***\n1. **Initialization and Constants:**\n\n - The code initializes a constant `MOD` as 1e9 + 7 for convenience in handling modulo operations.\n - It initializes three variables: `zero`, `one`, and `two` to keep track of counts for different scenarios within the corridor string.\n1. **Looping through the Corridor:**\n\n - The code uses a for-each loop to iterate through each character (`thing`) in the `corridor` string.\n1. **Updating Counts based on Characters (\'S\' or \'P\'):**\n\n - When encountering an \'S\' in the corridor:\n - `zero` (representing sections without \'S\'s) is updated to the value of `one`.\n - The `swap` function is used between `one` (representing sections with one \'S\') and `two` (representing sections with two \'S\'s).\n - When encountering a \'P\' (passage) in the corridor:\n - `two` (representing sections with two \'S\'s) is updated to `(two + zero) % MOD`, effectively accumulating valid section counts.\n1. **Return Result:**\n\n - Finally, the function returns the value stored in `zero`, which represents the count of ways to divide the corridor string from the beginning (index 0) without any \'S\'s in the section.\n\n# Complexity\n- *Time complexity:*\n $$O(n)$$\n \n\n- *Space complexity:*\n $$O(1)$$\n \n\n\n# Code\n```C++ []\n\nclass Solution {\npublic:\n int numberOfWays(string corridor) {\n // Store 1000000007 in a variable for convenience\n const int MOD = 1e9 + 7;\n\n // Initial values of three variables\n int zero = 0;\n int one = 0;\n int two = 1;\n\n // Compute using derived equations\n for (char thing : corridor) {\n if (thing == \'S\') {\n zero = one;\n swap(one, two);\n } else {\n two = (two + zero) % MOD;\n }\n }\n\n // Return the result\n return zero;\n }\n};\n\n```\n```C []\nvoid swap(int * a, int * b) {\n int temp = *a;\n *a = *b;\n *b = temp;\n}\n\nint numberOfWays(char * corridor) { \n // Initial values of three variables\n int zero = 0;\n int one = 0;\n int two = 1;\n\n // Compute using derived equations\n for (int index = 0; index < strlen(corridor); index++) {\n if (corridor[index] == \'S\') {\n zero = one;\n swap(&one, &two);\n } else {\n two = (two + zero) % 1000000007;\n }\n }\n\n // Return the result\n return zero;\n}\n\n\n\n```\n```Java []\nclass Solution {\n public int numberOfWays(String corridor) {\n // Store 1000000007 in a variable for convenience\n final int MOD = 1_000_000_007;\n\n // Initial values of three variables\n int zero = 0;\n int one = 0;\n int two = 1;\n\n // Compute using derived equations\n for (char thing : corridor.toCharArray()) {\n if (thing == \'S\') {\n zero = one;\n int temp = one;\n one = two;\n two = temp;\n } else {\n two = (two + zero) % MOD;\n }\n }\n\n // Return the result\n return zero;\n }\n}\n\n\n```\n```python3 []\nclass Solution:\n def numberOfWays(self, corridor: str) -> int:\n # Store 1000000007 in a variable for convenience\n MOD = 1_000_000_007\n\n # Initial values of three variables\n zero = 0\n one = 0\n two = 1\n\n # Compute using derived equations\n for thing in corridor:\n if thing == "S":\n zero = one\n one, two = two, one\n else:\n two = (two + zero) % MOD\n\n # Return the result\n return zero\n\n\n```\n```javascript []\nvar numberOfWays = function(corridor) {\n // Store 1000000007 in a variable for convenience\n const MOD = 1e9 + 7;\n \n // Initial values of three variables\n let zero = 0;\n let one = 0;\n let two = 1;\n\n // Compute using derived equations\n for (thing of corridor) {\n if (thing == \'S\') {\n zero = one;\n [one, two] = [two, one];\n } else {\n two = (two + zero) % MOD;\n }\n }\n\n // Return the result\n return zero;\n};\n\n```\n\n---\n#### ***Approach 4(Combinatorics)***\n1. **Initialization and Constants:**\n\n - The code initializes a constant `MOD` as 1e9 + 7 for convenience in handling modulo operations.\n1. **Finding Indices of \'S\' Characters:**\n\n - The code uses a loop to iterate through the `corridor` string, storing the indices of \'S\' characters in a vector named `indices`.\n1. **Checking Possibility of Division:**\n\n - If the total count of \'S\' characters (`indices.size()`) is zero or an odd number (indicating an odd count of \'S\'s), which implies that pairing for sections is not possible, the function returns 0 immediately.\n1. **Calculating the Number of Ways:**\n\n - The code initializes a `long` variable `count` to store the total number of ways to divide the corridor.\n - It then iterates through the indices of \'S\' characters and calculates the product of distances between non-paired neighboring \'S\'s.\n - It starts by taking the product of the distances between the first non-paired \'S\' (index 0) and the second non-paired \'S\' (index 1) and continues in pairs.\n - The loop calculates the product by multiplying the distance between two \'S\' indices and updating the count while taking the modulo operation with `MOD` to prevent integer overflow.\n1. **Returning the Result:**\n\n - Finally, the function returns the calculated `count` as an integer, representing the total number of valid ways to divide the corridor into sections with two consecutive \'S\' characters.\n\n# Complexity\n- *Time complexity:*\n $$O(n)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int numberOfWays(string corridor) {\n // Store 1000000007 in a variable for convenience\n const int MOD = 1e9 + 7;\n\n // Store indices of S in an array\n vector<int> indices;\n for (int index = 0; index < corridor.length(); index++) {\n if (corridor[index] == \'S\') {\n indices.push_back(index);\n }\n }\n\n // When division is not possible\n if (indices.size() == 0 || indices.size() % 2 == 1) {\n return 0;\n }\n\n // Total number of ways\n long count = 1;\n\n // Take product of non-paired neighbors\n int previousPairLast = 1;\n int currentPairFirst = 2;\n while (currentPairFirst < indices.size()) {\n count *= (indices[currentPairFirst] - indices[previousPairLast]);\n count %= MOD;\n previousPairLast += 2;\n currentPairFirst += 2;\n }\n\n // Return the number of ways\n return (int) count;\n }\n};\n\n\n```\n```C []\n\nint numberOfWays(char * corridor) {\n // Store 1000000007 in a variable for convenience\n const int MOD = 1e9 + 7;\n\n // Store indices of S in an array\n int indices[strlen(corridor)];\n int indicesLength = 0;\n for (int index = 0; index < strlen(corridor); index++) {\n if (corridor[index] == \'S\') {\n indices[indicesLength++] = index;\n }\n }\n\n // When division is not possible\n if (indicesLength == 0 || indicesLength % 2 == 1) {\n return 0;\n }\n\n // Total number of ways\n long count = 1;\n\n // Take product of non-paired neighbors\n int previousPairLast = 1;\n int currentPairFirst = 2;\n while (currentPairFirst < indicesLength) {\n count *= (indices[currentPairFirst] - indices[previousPairLast]);\n count %= MOD;\n previousPairLast += 2;\n currentPairFirst += 2;\n }\n\n // Return the number of ways\n return (int) count;\n}\n\n\n```\n```Java []\nclass Solution {\n public int numberOfWays(String corridor) {\n // Store 1000000007 in a variable for convenience\n final int MOD = 1_000_000_007;\n\n // Store indices of S in an array\n List<Integer> indices = new ArrayList<>();\n for (int index = 0; index < corridor.length(); index++) {\n if (corridor.charAt(index) == \'S\') {\n indices.add(index);\n }\n }\n\n // When division is not possible\n if (indices.size() == 0 || indices.size() % 2 == 1) {\n return 0;\n }\n\n // Total number of ways\n long count = 1;\n\n // Take product of non-paired neighbors\n int previousPairLast = 1;\n int currentPairFirst = 2;\n while (currentPairFirst < indices.size()) {\n count *= (indices.get(currentPairFirst) - indices.get(previousPairLast));\n count %= MOD;\n previousPairLast += 2;\n currentPairFirst += 2;\n }\n\n // Return the number of ways\n return (int) count;\n }\n}\n\n\n```\n```python3 []\nclass Solution:\n def numberOfWays(self, corridor: str) -> int:\n # Store 1000000007 in a variable for convenience\n MOD = 1_000_000_007\n\n # Store indices of S in an array\n indices = []\n for i, thing in enumerate(corridor):\n if thing == "S":\n indices.append(i)\n \n # When division is not possible\n if indices == [] or len(indices) % 2 == 1:\n return 0\n \n # Total number of ways\n count = 1\n\n # Take the product of non-paired neighbors\n previous_pair_last = 1\n current_pair_first = 2\n while current_pair_first < len(indices):\n count *= (indices[current_pair_first] - indices[previous_pair_last])\n count %= MOD\n previous_pair_last += 2\n current_pair_first += 2\n\n # Return the number of ways\n return count\n\n\n```\n```javascript []\nvar numberOfWays = function(corridor) {\n // Store 1000000007 in a variable for convenience\n const MOD = 1e9 + 7;\n \n // Store indices of S in an array\n const indices = [];\n for (let index = 0; index < corridor.length; index++) {\n if (corridor[index] == \'S\') {\n indices.push(index);\n }\n }\n\n // When division is not possible\n if (indices.length == 0 || indices.length % 2 == 1) {\n return 0;\n }\n\n // Total number of ways\n let count = 1;\n\n // Take product of non-paired neighbors\n let previousPairLast = 1;\n let currentPairFirst = 2;\n while (currentPairFirst < indices.length) {\n count *= (indices[currentPairFirst] - indices[previousPairLast]);\n count %= MOD;\n previousPairLast += 2;\n currentPairFirst += 2;\n }\n\n // Return the number of ways\n return count;\n};\n\n```\n\n---\n#### ***Approach 5(Combinatorics, Space Optimized)***\n1. **Initialization and Constants:**\n\n - The code initializes a constant `MOD` as 1e9 + 7 for convenience in handling modulo operations.\n1. **Variables Initialization:**\n\n - It initializes a `long` variable `count` to store the total number of ways to divide the corridor.\n - `seats` variable is used to keep track of the number of \'S\'s encountered in the current section.\n - `previousPairLast` tracks the index of the last \'S\' in the previous section. It starts with -1 to indicate no previous section initially.\n1. **Looping through the Corridor:**\n\n - The code uses a loop to iterate through each character in the `corridor` string.\n1. **Tracking \'S\'s and Calculating Ways:**\n\n - When encountering an \'S\' in the corridor:\n - `seats` is incremented by 1 to count the \'S\' encountered.\n - If two \'S\'s are encountered consecutively, indicating the end of a section:\n - It updates `previousPairLast` with the index of the last \'S\' in the section.\n - Resets `seats` to 0 to start counting for the next section.\n - If one \'S\' is encountered and a previous section existed (`previousPairLast != -1`):\n - It calculates the product of the distance between the current \'S\' and the last \'S\' in the previous section and updates the `count`.\n1. **Handling Edge Cases:**\n\n - If the loop ends and there\'s an odd number of \'S\'s remaining (`seats == 1`) or no sections were found (`previousPairLast == -1`), indicating invalid or incomplete sections, the function returns 0.\n1. **Returning the Result:**\n\n - Finally, the function returns the calculated `count` as an integer, representing the total number of valid ways to divide the corridor into sections with two consecutive \'S\' characters.\n\n# Complexity\n- *Time complexity:*\n $$O(n)$$\n \n\n- *Space complexity:*\n $$O(1)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int numberOfWays(string corridor) {\n // Store 1000000007 in a variable for convenience\n const int MOD = 1e9 + 7;\n\n // Total number of ways\n long count = 1;\n\n // Number of seats in the current section\n int seats = 0;\n\n // Tracking Index of last S in the previous section\n int previousPairLast = -1;\n\n // Keep track of seats in corridor\n for (int index = 0; index < corridor.length(); index++) {\n if (corridor[index] == \'S\') {\n seats += 1;\n\n // If two seats, then this is the last S in the section\n // Update seats for the next section\n if (seats == 2) {\n previousPairLast = index;\n seats = 0;\n }\n\n // If one seat, then this is the first S in the section\n // Compute product of non-paired neighbors\n else if (seats == 1 && previousPairLast != -1) {\n count *= (index - previousPairLast);\n count %= MOD;\n }\n }\n }\n\n // If odd seats, or zero seats\n if (seats == 1 || previousPairLast == -1) {\n return 0;\n }\n\n // Return the number of ways\n return (int) count;\n }\n};\n\n\n```\n```C []\nint numberOfWays(char * corridor){\n // Store 1000000007 in a variable for convenience\n const int MOD = 1e9 + 7;\n\n // Total number of ways\n long count = 1;\n\n // Number of seats in the current section\n int seats = 0;\n\n // Tracking Index of last S in the previous section\n int previousPairLast = -1;\n\n // Keep track of seats in the corridor\n for (int index = 0; index < strlen(corridor); index++) {\n if (corridor[index] == \'S\') {\n seats += 1;\n\n // If two seats, then this is the last S in the section\n // Update seats for the next section\n if (seats == 2) {\n previousPairLast = index;\n seats = 0;\n }\n\n // If one seat, then this is the first S in the section\n // Compute product of non-paired neighbors\n else if (seats == 1 && previousPairLast != -1) {\n count *= (index - previousPairLast);\n count %= MOD;\n }\n }\n }\n\n // If odd seats, or zero seats\n if (seats == 1 || previousPairLast == -1) {\n return 0;\n }\n\n // Return the number of ways\n return (int) count;\n}\n\n\n\n```\n```Java []\nclass Solution {\n public int numberOfWays(String corridor) {\n // Store 1000000007 in a variable for convenience\n final int MOD = 1_000_000_007;\n\n // Total number of ways\n long count = 1;\n\n // Number of seats in the current section\n int seats = 0;\n\n // Tracking Index of last S in the previous section\n Integer previousPairLast = null;\n\n // Keep track of seats in corridor\n for (int index = 0; index < corridor.length(); index++) {\n if (corridor.charAt(index) == \'S\') {\n seats += 1;\n\n // If two seats, then this is the last S in the section\n // Update seats for the next section\n if (seats == 2) {\n previousPairLast = index;\n seats = 0;\n }\n\n // If one seat, then this is the first S in the section\n // Compute product of non-paired neighbors\n else if (seats == 1 && previousPairLast != null) {\n count *= (index - previousPairLast);\n count %= MOD;\n }\n }\n }\n\n // If odd seats, or zero seats\n if (seats == 1 || previousPairLast == null) {\n return 0;\n }\n\n // Return the number of ways\n return (int) count;\n }\n}\n\n\n```\n```python3 []\nclass Solution:\n def numberOfWays(self, corridor: str) -> int:\n # Store 1000000007 in a variable for convenience\n MOD = 1_000_000_007\n\n # Total number of ways\n count = 1\n\n # Number of seats in current section\n seats = 0\n\n # Tracking Index of last S in the previous section\n previous_pair_last = None\n\n # Keep track of seats in the corridor\n for index, thing in enumerate(corridor):\n if thing == "S":\n seats += 1\n\n # If two seats, then this is the last S in the section\n # Update seats for the next section\n if seats == 2:\n previous_pair_last = index\n seats = 0\n \n # If one seat, then this is the first S in the section\n # Compute the product of non-paired neighbors\n elif seats == 1 and previous_pair_last is not None:\n count *= (index - previous_pair_last)\n count %= MOD\n \n # If odd seats, or zero seats\n if seats == 1 or previous_pair_last is None:\n return 0\n\n # Return the number of ways\n return count\n\n\n```\n```javascript []\nvar numberOfWays = function(corridor) {\n // Store 1000000007 in a variable for convenience\n const MOD = 1e9 + 7;\n\n // Total number of ways\n let count = 1;\n\n // Number of seats in the current section\n let seats = 0;\n\n // Tracking Index of last S in the previous section\n let previousPairLast = -1;\n\n // Keep track of seats in corridor\n for (let index = 0; index < corridor.length; index++) {\n if (corridor[index] == \'S\') {\n seats += 1;\n\n // If two seats, then this is the last S in the section\n // Update seats for the next section\n if (seats == 2) {\n previousPairLast = index;\n seats = 0;\n }\n\n // If one seat, then this is the first S in the section\n // Compute product of non-paired neighbors\n else if (seats == 1 && previousPairLast != -1) {\n count *= (index - previousPairLast);\n count %= MOD;\n }\n }\n }\n\n // If odd seats, or zero seats\n if (seats == 1 || previousPairLast == -1) {\n return 0;\n }\n\n // Return the number of ways\n return count;\n};\n\n```\n\n---\n\n\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n---\n | 4 | Along a long library corridor, there is a line of seats and decorative plants. You are given a **0-indexed** string `corridor` of length `n` consisting of letters `'S'` and `'P'` where each `'S'` represents a seat and each `'P'` represents a plant.
One room divider has **already** been installed to the left of index `0`, and **another** to the right of index `n - 1`. Additional room dividers can be installed. For each position between indices `i - 1` and `i` (`1 <= i <= n - 1`), at most one divider can be installed.
Divide the corridor into non-overlapping sections, where each section has **exactly two seats** with any number of plants. There may be multiple ways to perform the division. Two ways are **different** if there is a position with a room divider installed in the first way but not in the second way.
Return _the number of ways to divide the corridor_. Since the answer may be very large, return it **modulo** `109 + 7`. If there is no way, return `0`.
**Example 1:**
**Input:** corridor = "SSPPSPS "
**Output:** 3
**Explanation:** There are 3 different ways to divide the corridor.
The black bars in the above image indicate the two room dividers already installed.
Note that in each of the ways, **each** section has exactly **two** seats.
**Example 2:**
**Input:** corridor = "PPSPSP "
**Output:** 1
**Explanation:** There is only 1 way to divide the corridor, by not installing any additional dividers.
Installing any would create some section that does not have exactly two seats.
**Example 3:**
**Input:** corridor = "S "
**Output:** 0
**Explanation:** There is no way to divide the corridor because there will always be a section that does not have exactly two seats.
**Constraints:**
* `n == corridor.length`
* `1 <= n <= 105`
* `corridor[i]` is either `'S'` or `'P'`. | The number of operators in the equation is less. Could you find the right answer then generate all possible answers using different orders of operations? Divide the equation into blocks separated by the operators, and use memoization on the results of blocks for optimization. Use set and the max limit of the answer for further optimization. |
✔️🚀Beats 99% | 102ms | Dynamic Programming Bottom Up Approach with Space Optimisation | number-of-ways-to-divide-a-long-corridor | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nwe can say that to compute three values at index indexindexindex, we need only three values at index index+1index + 1index+1. Hence, we can save only three variables, namely zero, one, and two that represent the values at the most recent computed index index+1index + 1index+1.\n\n- Initially\n\n zero = 0\n one = 0\n two = 1\n- If corridor[index] is S, then\n\n new_zero = one\n new_one = two\n new_two = one\nThen we can update the values of zero, one, and two as zero = new_zero, one = new_one, and two = new_two.\n\nHowever, it is worth noting that zero is not used in the computation of any new variables. Thus, zero = one will work, and we are not overwriting the value of zero before using it.\nAdding to it, next we just need to swap the values of one and two. Hence, the final update is as\n\n zero = one\n swap(one, two). For this, we can do an XOR swap or can use a temporary variable.\n- If corridor[index] is P, then\n\n new_zero = zero\n new_one = one\n new_two = (zero + two) % MOD\nThen we can update the values of zero, one, and two as zero = new_zero, one = new_one, and two = new_two.\n\nHowever, it is worth noting that zero and one remain unchanged in all of these assignments. Thus, updating zero and one is redundant.\nRegarding two, we just need to add zero in it, and then take modulo MOD. Hence, the final update is as\n\n two = (two + zero) % MOD\nDue to the symmetry of count, the corridor traversal is possible from left to right as well as from right to left. We will traverse the corridor from left to right this time.\n\nAt last, our answer will be stored in variable zero, as initially, we have zero number of S.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1.Store 1000000007 in the variable MOD for convenience. It is a good practice to store constants.\n2.Initialize three variables zero, one, and two to 0, 0, and 1 respectively.\n\n3.Traverse corridor from left to right, for index from 0 to n - 1\n\n- if corridor[index] is S, then\nzero = one\nswap(one, two)\n- if corridor[index] is P, then\ntwo = (two + zero) % MOD\n4. Return zero as we have a zero number of S initially.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nWe are linearly traversing the corridor. In each iteration, we are updating three variables, which will take constant time. Thus, the time complexity will be O(N)\u22C5O(1)O(N) \\cdot O(1)O(N)\u22C5O(1), which is O(N)O(N)O(N).\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nWe are using a handful of variables, which will take constant extra space. Thus, the space complexity will be O(1)O(1)O(1).\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int numberOfWays(string corridor) {\n // Store 1000000007 in a variable for convenience\n const int MOD = 1e9 + 7;\n\n // Initial values of three variables\n int zero = 0;\n int one = 0;\n int two = 1;\n\n // Compute using derived equations\n for (char thing : corridor) {\n if (thing == \'S\') {\n zero = one;\n swap(one, two);\n } else {\n two = (two + zero) % MOD;\n }\n }\n\n // Return the result\n return zero;\n }\n};\n```\n```javascript []\nclass Solution {\n numberOfWays(corridor) {\n const MOD = 1e9 + 7;\n let zero = 0;\n let one = 0;\n let two = 1;\n\n for (const thing of corridor) {\n if (thing === \'S\') {\n zero = one;\n [one, two] = [two, one];\n } else {\n two = (two + zero) % MOD;\n }\n }\n\n return zero;\n }\n}\n```\n```python []\nclass Solution:\n def numberOfWays(self, corridor):\n MOD = 10**9 + 7\n zero = 0\n one = 0\n two = 1\n\n for thing in corridor:\n if thing == \'S\':\n zero = one\n one, two = two, one\n else:\n two = (two + zero) % MOD\n\n return zero\n```\n```Java []\npublic class Solution {\n public int numberOfWays(String corridor) {\n final int MOD = 1000000007;\n int zero = 0;\n int one = 0;\n int two = 1;\n\n for (char thing : corridor.toCharArray()) {\n if (thing == \'S\') {\n zero = one;\n int temp = one;\n one = two;\n two = temp;\n } else {\n two = (two + zero) % MOD;\n }\n }\n\n return zero;\n }\n}\n\n``` | 15 | Along a long library corridor, there is a line of seats and decorative plants. You are given a **0-indexed** string `corridor` of length `n` consisting of letters `'S'` and `'P'` where each `'S'` represents a seat and each `'P'` represents a plant.
One room divider has **already** been installed to the left of index `0`, and **another** to the right of index `n - 1`. Additional room dividers can be installed. For each position between indices `i - 1` and `i` (`1 <= i <= n - 1`), at most one divider can be installed.
Divide the corridor into non-overlapping sections, where each section has **exactly two seats** with any number of plants. There may be multiple ways to perform the division. Two ways are **different** if there is a position with a room divider installed in the first way but not in the second way.
Return _the number of ways to divide the corridor_. Since the answer may be very large, return it **modulo** `109 + 7`. If there is no way, return `0`.
**Example 1:**
**Input:** corridor = "SSPPSPS "
**Output:** 3
**Explanation:** There are 3 different ways to divide the corridor.
The black bars in the above image indicate the two room dividers already installed.
Note that in each of the ways, **each** section has exactly **two** seats.
**Example 2:**
**Input:** corridor = "PPSPSP "
**Output:** 1
**Explanation:** There is only 1 way to divide the corridor, by not installing any additional dividers.
Installing any would create some section that does not have exactly two seats.
**Example 3:**
**Input:** corridor = "S "
**Output:** 0
**Explanation:** There is no way to divide the corridor because there will always be a section that does not have exactly two seats.
**Constraints:**
* `n == corridor.length`
* `1 <= n <= 105`
* `corridor[i]` is either `'S'` or `'P'`. | The number of operators in the equation is less. Could you find the right answer then generate all possible answers using different orders of operations? Divide the equation into blocks separated by the operators, and use memoization on the results of blocks for optimization. Use set and the max limit of the answer for further optimization. |
Easy Full Explanation 💯 || Beginner Friendly🔥🔥|| 100% Fast | number-of-ways-to-divide-a-long-corridor | 1 | 1 | \n\n# Intuition\n- Iterate through the corridor, counting consecutive seats and the number of divisions between them.\n- For each section of consecutive seats, calculate the number of ways to insert dividers based on the number of divisions.\n- Accumulate the result, considering the constraints and modulo.\n\n# Approach\n1. Initialize counters for consecutive seats (chairs) and the result (result).\n2. Iterate through the corridor:\n- Count consecutive seats and divisions.\n- For each section, calculate the number of ways to insert dividers based on the number of divisions.\n- Update the result accordingly.\n3. Return the final result if the number of chairs is even; otherwise, return 0.\n\n# Code\n```c++ []\nclass Solution {\npublic:\n int numberOfWays(string corridor) {\n int chairs = 0;\n long long result = 1;\n\n for (int i = 0; i < corridor.size(); i++) {\n if (corridor[i] == \'S\') {\n chairs++;\n\n while (++i < corridor.size() && corridor[i] != \'S\');\n if (i < corridor.size() && corridor[i] == \'S\') {\n chairs++;\n }\n\n int divisions = 1;\n while (++i < corridor.size() && corridor[i] != \'S\') {\n divisions++;\n }\n\n if (divisions > 1 && i < corridor.size()) {\n result = (result * divisions) % 1000000007;\n }\n i--;\n }\n }\n\n return (chairs != 0 && chairs % 2 == 0) ? static_cast<int>(result) : 0;\n \n }\n};\n```\n```java []\nclass Solution {\n\n public int numberOfWays(String corridor) {\n char[] array = corridor.toCharArray();\n int chairs = 0;\n long result = 1;\n\n for (int i = 0; i < array.length; i++) {\n // Count consecutive seats\n if (array[i] == \'S\') {\n chairs++;\n\n // Move to the next non-seat character or the end of the array\n while (++i < array.length && array[i] != \'S\');\n\n // Check if there is another seat after the consecutive seats\n if (i < array.length && array[i] == \'S\') {\n chairs++;\n }\n\n // Count the number of divisions between seats\n int divisions = 1;\n while (++i < array.length && array[i] != \'S\') {\n divisions++;\n }\n\n // If there are more than one division and another seat follows, update the result\n if (divisions > 1 && i < array.length) {\n result = (result * divisions) % 1000000007;\n }\n\n // Move the index back by 1 to handle the next character in the next iteration\n i--;\n }\n }\n\n // Return the result if the number of chairs is even, otherwise, return 0\n return (chairs != 0 && chairs % 2 == 0) ? (int) result : 0;\n }\n}\n\n```\n```python []\nclass Solution:\n def numberOfWays(self, corridor: str) -> int:\n array = list(corridor)\n chairs = 0\n result = 1\n\n i = 0\n while i < len(array):\n # Count consecutive seats\n if array[i] == \'S\':\n chairs += 1\n\n # Move to the next non-seat character or the end of the array\n while i + 1 < len(array) and array[i + 1] != \'S\':\n i += 1\n\n # Check if there is another seat after the consecutive seats\n if i + 1 < len(array) and array[i + 1] == \'S\':\n chairs += 1\n\n # Count the number of divisions between seats\n divisions = 1\n while i + 1 < len(array) and array[i + 1] != \'S\':\n i += 1\n divisions += 1\n\n # If there are more than one division and another seat follows, update the result\n if divisions > 1 and i + 1 < len(array):\n result = (result * divisions) % (10**9 + 7)\n\n # Move the index to handle the next character in the next iteration\n i += 1\n\n # Return the result if the number of chairs is even, otherwise, return 0\n return result if chairs != 0 and chairs % 2 == 0 else 0\n\n```\n\n# Explanation\n- The algorithm counts consecutive seats and divisions between seats.\n- For each section of consecutive seats, it calculates the number of ways to insert dividers based on the number of divisions.\n- The result is accumulated, and the final answer is returned if the number of chairs is even.\n- The code uses a modular approach to handle large numbers and avoid overflow.\n\nThis approach ensures that each section has exactly two seats, meeting the requirements of the problem.\n\n---\n\n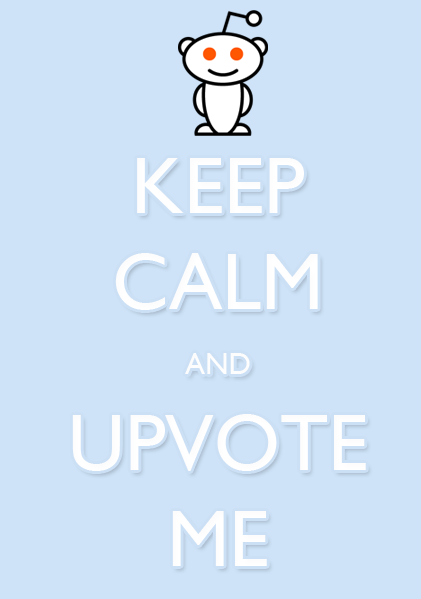\n\n\n\n\n\n\n | 3 | Along a long library corridor, there is a line of seats and decorative plants. You are given a **0-indexed** string `corridor` of length `n` consisting of letters `'S'` and `'P'` where each `'S'` represents a seat and each `'P'` represents a plant.
One room divider has **already** been installed to the left of index `0`, and **another** to the right of index `n - 1`. Additional room dividers can be installed. For each position between indices `i - 1` and `i` (`1 <= i <= n - 1`), at most one divider can be installed.
Divide the corridor into non-overlapping sections, where each section has **exactly two seats** with any number of plants. There may be multiple ways to perform the division. Two ways are **different** if there is a position with a room divider installed in the first way but not in the second way.
Return _the number of ways to divide the corridor_. Since the answer may be very large, return it **modulo** `109 + 7`. If there is no way, return `0`.
**Example 1:**
**Input:** corridor = "SSPPSPS "
**Output:** 3
**Explanation:** There are 3 different ways to divide the corridor.
The black bars in the above image indicate the two room dividers already installed.
Note that in each of the ways, **each** section has exactly **two** seats.
**Example 2:**
**Input:** corridor = "PPSPSP "
**Output:** 1
**Explanation:** There is only 1 way to divide the corridor, by not installing any additional dividers.
Installing any would create some section that does not have exactly two seats.
**Example 3:**
**Input:** corridor = "S "
**Output:** 0
**Explanation:** There is no way to divide the corridor because there will always be a section that does not have exactly two seats.
**Constraints:**
* `n == corridor.length`
* `1 <= n <= 105`
* `corridor[i]` is either `'S'` or `'P'`. | The number of operators in the equation is less. Could you find the right answer then generate all possible answers using different orders of operations? Divide the equation into blocks separated by the operators, and use memoization on the results of blocks for optimization. Use set and the max limit of the answer for further optimization. |
Easy Python Solution|| O(N) with O(1) Space complexity|| :) | number-of-ways-to-divide-a-long-corridor | 0 | 1 | # Generalizing the problem\n\n\n## - If Seats < 2,then\nNo division possible\n\n---\n\n\n## - If Seats == 2,then\nonly 1 division possible\n\n---\n\n\n## - If Seats are odd,then\nNo division is possible since the question requires each division to have exactly 2 seats\n\n---\n\n\n## - If Seats >2,then\n#### Explaination:\n**S P S P P P S P P S** P P S S\n\nFrom the above problem we can observe taking the first sub problem:\n\n**S P S** P P P **S P P S**\nThe divisions possible are:\nS P S **|** P P P S P P S\nS P S P **|** P P S P P S\nS P S P P **|** P S P P S\nS P S P P P **|** S P P S\n\n1. Therefore number of possible divisions =\n (1st seat of next div - 2nd seat of previous div)\n2. for the next sub problem all the previous solutions can be applied thus we multiply the outcomes together\nS P S P P P **S P P S P P S S**\nFor example in this section of the above problem,\n\n**S P P S**| P P **S S** => 4 previous divison\n**S P P S** P| P **S S** => 4 previous divison\n**S P P S** P P| **S S** => 4 previous divison\n3. Finally, we arrive at the conclusion,\nans=ans*(1st seat of next div - 2nd seat of previous div)\n\n\n\n# Code\n```\nclass Solution:\n def numberOfWays(self, corridor: str) -> int:\n seat=0\n prev=0\n ans=1\n for x in range(len(corridor)):\n if(corridor[x]==\'S\'):\n if(seat<2):\n seat+=1\n else:\n seat=1\n ans*= (x-prev)\n prev=x\n return 0 if seat<2 else ans%(10**9+7)\n```\n# Complexity\n- Time complexity:\nO(N)\n\n- Space complexity:\nO(1)\n | 2 | Along a long library corridor, there is a line of seats and decorative plants. You are given a **0-indexed** string `corridor` of length `n` consisting of letters `'S'` and `'P'` where each `'S'` represents a seat and each `'P'` represents a plant.
One room divider has **already** been installed to the left of index `0`, and **another** to the right of index `n - 1`. Additional room dividers can be installed. For each position between indices `i - 1` and `i` (`1 <= i <= n - 1`), at most one divider can be installed.
Divide the corridor into non-overlapping sections, where each section has **exactly two seats** with any number of plants. There may be multiple ways to perform the division. Two ways are **different** if there is a position with a room divider installed in the first way but not in the second way.
Return _the number of ways to divide the corridor_. Since the answer may be very large, return it **modulo** `109 + 7`. If there is no way, return `0`.
**Example 1:**
**Input:** corridor = "SSPPSPS "
**Output:** 3
**Explanation:** There are 3 different ways to divide the corridor.
The black bars in the above image indicate the two room dividers already installed.
Note that in each of the ways, **each** section has exactly **two** seats.
**Example 2:**
**Input:** corridor = "PPSPSP "
**Output:** 1
**Explanation:** There is only 1 way to divide the corridor, by not installing any additional dividers.
Installing any would create some section that does not have exactly two seats.
**Example 3:**
**Input:** corridor = "S "
**Output:** 0
**Explanation:** There is no way to divide the corridor because there will always be a section that does not have exactly two seats.
**Constraints:**
* `n == corridor.length`
* `1 <= n <= 105`
* `corridor[i]` is either `'S'` or `'P'`. | The number of operators in the equation is less. Could you find the right answer then generate all possible answers using different orders of operations? Divide the equation into blocks separated by the operators, and use memoization on the results of blocks for optimization. Use set and the max limit of the answer for further optimization. |
Count only needed plants. O(n) (beats 100% time) | number-of-ways-to-divide-a-long-corridor | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n1) We don\'t need to consider plants before first seat and after last seat\n2) We can only place dividers between odd and even numbered seats (0-indexed)\n3) Special cases:\n Odd number of seats -> return 0\n No seats -> return 0\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1) Strip `corridor` of \'P\'\n2) Make a boolean variable `seats` that shows whether we are between odd and even numbered seats\n3) If after iteration `seats` remains `True` -> we have odd number of seats -> `return 0`\n4) After each group of plants of length `plants` multiply `answer` by `plants`\n# Complexity\n- Time complexity: $$O(n)$$ (iterate through `corridor` once and strip it)\n\n- Space complexity: $$O(1)$$ \n\n# Code\n```\nclass Solution:\n def numberOfWays(self, corridor: str) -> int:\n seats = False\n plants = 0\n answer = 1\n corridor = corridor.strip(\'P\')\n if not corridor: # no seats\n return 0\n for sp in corridor:\n if sp == \'S\':\n seats = not seats\n if plants:\n answer = answer * (plants + 1) % 1000000007\n plants = 0\n elif not seats: # between odd and even numbered seats\n plants += 1\n \n if seats: # odd number of seats\n return 0\n return answer % 1000000007\n``` | 2 | Along a long library corridor, there is a line of seats and decorative plants. You are given a **0-indexed** string `corridor` of length `n` consisting of letters `'S'` and `'P'` where each `'S'` represents a seat and each `'P'` represents a plant.
One room divider has **already** been installed to the left of index `0`, and **another** to the right of index `n - 1`. Additional room dividers can be installed. For each position between indices `i - 1` and `i` (`1 <= i <= n - 1`), at most one divider can be installed.
Divide the corridor into non-overlapping sections, where each section has **exactly two seats** with any number of plants. There may be multiple ways to perform the division. Two ways are **different** if there is a position with a room divider installed in the first way but not in the second way.
Return _the number of ways to divide the corridor_. Since the answer may be very large, return it **modulo** `109 + 7`. If there is no way, return `0`.
**Example 1:**
**Input:** corridor = "SSPPSPS "
**Output:** 3
**Explanation:** There are 3 different ways to divide the corridor.
The black bars in the above image indicate the two room dividers already installed.
Note that in each of the ways, **each** section has exactly **two** seats.
**Example 2:**
**Input:** corridor = "PPSPSP "
**Output:** 1
**Explanation:** There is only 1 way to divide the corridor, by not installing any additional dividers.
Installing any would create some section that does not have exactly two seats.
**Example 3:**
**Input:** corridor = "S "
**Output:** 0
**Explanation:** There is no way to divide the corridor because there will always be a section that does not have exactly two seats.
**Constraints:**
* `n == corridor.length`
* `1 <= n <= 105`
* `corridor[i]` is either `'S'` or `'P'`. | The number of operators in the equation is less. Could you find the right answer then generate all possible answers using different orders of operations? Divide the equation into blocks separated by the operators, and use memoization on the results of blocks for optimization. Use set and the max limit of the answer for further optimization. |
Python3 Solution | number-of-ways-to-divide-a-long-corridor | 0 | 1 | \n```\nclass Solution:\n def numberOfWays(self, corridor: str) -> int:\n mod=10**9+7\n a,b,c=1,0,0\n for ch in corridor:\n if ch=="S":\n a,b,c=0,a+c,b\n else:\n a,b,c=a+c,b,c\n return c%mod \n``` | 2 | Along a long library corridor, there is a line of seats and decorative plants. You are given a **0-indexed** string `corridor` of length `n` consisting of letters `'S'` and `'P'` where each `'S'` represents a seat and each `'P'` represents a plant.
One room divider has **already** been installed to the left of index `0`, and **another** to the right of index `n - 1`. Additional room dividers can be installed. For each position between indices `i - 1` and `i` (`1 <= i <= n - 1`), at most one divider can be installed.
Divide the corridor into non-overlapping sections, where each section has **exactly two seats** with any number of plants. There may be multiple ways to perform the division. Two ways are **different** if there is a position with a room divider installed in the first way but not in the second way.
Return _the number of ways to divide the corridor_. Since the answer may be very large, return it **modulo** `109 + 7`. If there is no way, return `0`.
**Example 1:**
**Input:** corridor = "SSPPSPS "
**Output:** 3
**Explanation:** There are 3 different ways to divide the corridor.
The black bars in the above image indicate the two room dividers already installed.
Note that in each of the ways, **each** section has exactly **two** seats.
**Example 2:**
**Input:** corridor = "PPSPSP "
**Output:** 1
**Explanation:** There is only 1 way to divide the corridor, by not installing any additional dividers.
Installing any would create some section that does not have exactly two seats.
**Example 3:**
**Input:** corridor = "S "
**Output:** 0
**Explanation:** There is no way to divide the corridor because there will always be a section that does not have exactly two seats.
**Constraints:**
* `n == corridor.length`
* `1 <= n <= 105`
* `corridor[i]` is either `'S'` or `'P'`. | The number of operators in the equation is less. Could you find the right answer then generate all possible answers using different orders of operations? Divide the equation into blocks separated by the operators, and use memoization on the results of blocks for optimization. Use set and the max limit of the answer for further optimization. |
🥇 C++ | PYTHON | JAVA || O( N ) EXPLAINED || ; ] ✅ | number-of-ways-to-divide-a-long-corridor | 1 | 1 | \n# Solution 1\n\n- We need to place a dividor after 2 seats.\n- When a dividor is placed between any 2 seats : \n- * `Number of ways to do so = (Number of plants in between seats + 1)`\n\nSo we iterate through the String\n1. After every two seats : Count the number of plants till next seat\n2. Number of ways to put dividor = `(Number of plants in between seats + 1)`\n3. Multiply all the number of ways to get the answer.\nReturn modulo `10^9 + 7`\n\n``` Python []\nclass Solution:\n def numberOfWays(self, corridor):\n seat, res, plant = 0, 1, 0\n for i in corridor:\n if i==\'S\':\n seat += 1\n else:\n if seat == 2:\n plant += 1\n if seat == 3:\n res = res*(plant+1) % (10**9 + 7)\n seat , plant = 1 , 0\n if seat != 2:\n return 0\n return res\n```\n``` C++ []\nnt numberOfWays(std::string corridor) {\n int seat = 0, res =1, plant = 0;\n\n for (char i : corridor) {\n if (i == \'S\')\n seat += 1;\n else\n if (seat == 2)\n plant += 1;\n\n if (seat == 3) {\n res = (res * (plant + 1)) % (1000000007);\n seat = 1;\n plant = 0;\n }\n }\n\n if (seat != 2)\n return 0;\n\n return res;\n }\n```\n\n\n# Solution 2\nIf seats == odd , then ```answer -> 0```\nIf seats == even, then there is always a possible division.\n\nWe can only put a DIVIDER after every two seats\n\n# Explanation\n\n`x` -> number of way to put divider betweem seats, current number of seats. It mean, when x > 0 it mean we have counted 1 or 2 seats.\n\n`y` -> state variable, if y > 0 it means we have counted 1 seat.\n`z` -> total ways to put divider.\n\nThe flow of the algo:\n- `x` start with 1, due to we counted no seat. y, z = 0 hence we counted 0 seats and 0 ways to put divider.\n- When we counted first seat, x = 0, y = 1, z = 0.\n- When we counted second seat, x = 0, y = 0, z = 1. After the second seat, x is increase by z every time we meet a plant.\n- When we meet a seat again, the swap happen -> `z=y, y = x, x = 0`. When we meet the second seat, the swap become -> `z = y, y = x, x = 0`. so, this basically update number of way we counted to z when we have countered 2 more seat again, if not, z stay 0.\n\nCreating variables\n```\nx -> 0 seats\ny -> 1 seat\nz -> 2 seats\n```\n\nFor each ``"s"`` in string :\n\n``x -> x + z``\n``z -> y``\n``y -> x``\n``x -> 0``\nElse\n``\nx -> x + z\n``\n\n\n\n- Time complexity: O(N)\n\n- Space complexity: O(1)\n\n``` C++ []\nclass Solution {\npublic:\n int numberOfWays(string corridor) {\n int x = 1; // 0 seat\n int y = 0; // 1 seat\n int z = 0; // 2 seats\n for (char& ch: corridor)\n if (ch == \'S\')\n x = (x + z) % int(1e9 + 7), z = y, y = x, x = 0;\n else\n x = (x + z) % int(1e9 + 7);\n return z;\n }\n};\n```\n``` Python []\nclass Solution:\n def numberOfWays(self, corridor):\n x = 1 # 0 seat\n y = 0 # 1 seat\n z = 0 # 2 seats\n for char in corridor:\n if char == \'S\':\n x, y, z = 0, x + z, y\n else:\n x, y, z = x + z, y, z\n return z % (10**9+7) \n```\n```JAVA []\nclass Solution {\n public int numberOfWays(String corridor) {\n int x = 1; // 0 seat\n int y = 0; // 1 seat\n int z = 0; // 2 seats\n int mod = (int)1e9 + 7;\n for (int i = 0; i < corridor.length(); ++i)\n if (corridor.charAt(i) == \'S\') {\n x = (x + z) % mod;\n z = y; \n y = x;\n x = 0;\n } else {\n x = (x + z) % mod;\n }\n return z;\n }\n}\n\n```\n\n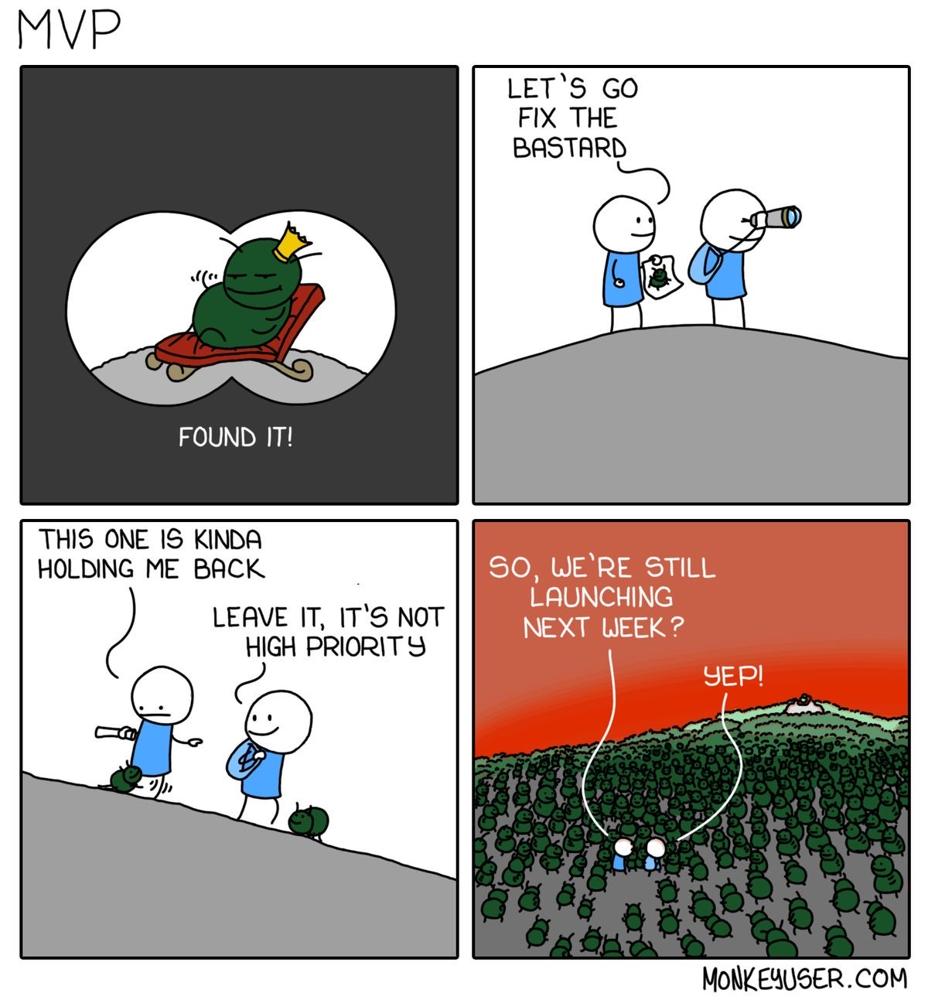\n | 42 | Along a long library corridor, there is a line of seats and decorative plants. You are given a **0-indexed** string `corridor` of length `n` consisting of letters `'S'` and `'P'` where each `'S'` represents a seat and each `'P'` represents a plant.
One room divider has **already** been installed to the left of index `0`, and **another** to the right of index `n - 1`. Additional room dividers can be installed. For each position between indices `i - 1` and `i` (`1 <= i <= n - 1`), at most one divider can be installed.
Divide the corridor into non-overlapping sections, where each section has **exactly two seats** with any number of plants. There may be multiple ways to perform the division. Two ways are **different** if there is a position with a room divider installed in the first way but not in the second way.
Return _the number of ways to divide the corridor_. Since the answer may be very large, return it **modulo** `109 + 7`. If there is no way, return `0`.
**Example 1:**
**Input:** corridor = "SSPPSPS "
**Output:** 3
**Explanation:** There are 3 different ways to divide the corridor.
The black bars in the above image indicate the two room dividers already installed.
Note that in each of the ways, **each** section has exactly **two** seats.
**Example 2:**
**Input:** corridor = "PPSPSP "
**Output:** 1
**Explanation:** There is only 1 way to divide the corridor, by not installing any additional dividers.
Installing any would create some section that does not have exactly two seats.
**Example 3:**
**Input:** corridor = "S "
**Output:** 0
**Explanation:** There is no way to divide the corridor because there will always be a section that does not have exactly two seats.
**Constraints:**
* `n == corridor.length`
* `1 <= n <= 105`
* `corridor[i]` is either `'S'` or `'P'`. | The number of operators in the equation is less. Could you find the right answer then generate all possible answers using different orders of operations? Divide the equation into blocks separated by the operators, and use memoization on the results of blocks for optimization. Use set and the max limit of the answer for further optimization. |
Python 7lines / Rust a little more. Linear solution, constant time with explanation | number-of-ways-to-divide-a-long-corridor | 0 | 1 | # Intuition\n\nFirst of all it is clear that if the number of seats you have is odd or there are no seats, you can\'t really create any divisions and the answer is zero. So lets focus on another situation and lets change seat to 1 and plant to 0 (as it is just easy for me to distinguish between them). Also it is clear that all the tralinig and leading zeros are irrelevant.\n\nSo lets look at this array:\n\n [1, 1, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1]\n\nAnd start working from left to right trying to create a divisions to not have more than two element.\n\n```\n[\n 1, 1, 0, 0, \n 1, 0, 1,\n 1, 1, 0,\n 1, 0, 0, 0, 1\n]\n```\n\nSo basically you have something like this\n\n g, 0, 0, g, g, 0, g\n\nwhere a group starts with 1 any number of zeros and ends with 1. Now you see that groups are indivisible and you can put divisions everywhere between two groups. For example: `g, 0, 0, g` can be divided into `g | 0, 0, g` or `g, 0 | 0, g` or `g, 0, 0 | g`.\n\nBasically if you have a group, `k` zeros and another group, you can divide them in `k + 1` ways. As all of them are independent the solution is just a product of all those values.\n\nThis can give us an easy linear solution.\n\n\n\n# Complexity\n- Time complexity: $O(n)$\n- Space complexity: $O(1)$\n\n# Code\n```Rust []\nimpl Solution {\n pub fn number_of_ways(s: String) -> i32 {\n let (mut res, mut m, mut num_seats, mut num_gaps) = (1u64, 1000000007u64, 0u64, 1u64);\n let s: Vec<char> = s.chars().collect();\n\n let (mut p1, mut p2) = (0, s.len() - 1);\n while p1 < s.len() && s[p1] != \'S\' {\n p1 += 1;\n }\n while p2 < s.len() && s[p2] != \'S\' {\n p2 -= 1;\n }\n\n if p2 >= s.len() {\n return 0;\n }\n\n for i in p1 ..=p2 {\n if s[i] == \'S\' {\n res = (res * num_gaps) % m;\n num_seats += 1;\n num_gaps = 1;\n } else if num_seats % 2 == 0 {\n num_gaps += 1;\n }\n }\n\n if num_seats % 2 == 1 || num_seats == 0 {\n return 0;\n }\n return res as i32;\n }\n}\n```\n```python []\nclass Solution:\n def numberOfWays(self, s: str) -> int:\n res, mod, num_seats, s, num_gap = 1, 10**9 + 7, 0, s.strip(\'P\'), 1\n for c in s:\n if c == \'S\':\n res, num_seats, num_gap = (res * num_gap) % mod, num_seats + 1, 1\n elif num_seats % 2 == 0:\n num_gap += 1\n \n return 0 if num_seats % 2 or not num_seats else res\n\n``` | 1 | Along a long library corridor, there is a line of seats and decorative plants. You are given a **0-indexed** string `corridor` of length `n` consisting of letters `'S'` and `'P'` where each `'S'` represents a seat and each `'P'` represents a plant.
One room divider has **already** been installed to the left of index `0`, and **another** to the right of index `n - 1`. Additional room dividers can be installed. For each position between indices `i - 1` and `i` (`1 <= i <= n - 1`), at most one divider can be installed.
Divide the corridor into non-overlapping sections, where each section has **exactly two seats** with any number of plants. There may be multiple ways to perform the division. Two ways are **different** if there is a position with a room divider installed in the first way but not in the second way.
Return _the number of ways to divide the corridor_. Since the answer may be very large, return it **modulo** `109 + 7`. If there is no way, return `0`.
**Example 1:**
**Input:** corridor = "SSPPSPS "
**Output:** 3
**Explanation:** There are 3 different ways to divide the corridor.
The black bars in the above image indicate the two room dividers already installed.
Note that in each of the ways, **each** section has exactly **two** seats.
**Example 2:**
**Input:** corridor = "PPSPSP "
**Output:** 1
**Explanation:** There is only 1 way to divide the corridor, by not installing any additional dividers.
Installing any would create some section that does not have exactly two seats.
**Example 3:**
**Input:** corridor = "S "
**Output:** 0
**Explanation:** There is no way to divide the corridor because there will always be a section that does not have exactly two seats.
**Constraints:**
* `n == corridor.length`
* `1 <= n <= 105`
* `corridor[i]` is either `'S'` or `'P'`. | The number of operators in the equation is less. Could you find the right answer then generate all possible answers using different orders of operations? Divide the equation into blocks separated by the operators, and use memoization on the results of blocks for optimization. Use set and the max limit of the answer for further optimization. |
✅ One Line Solution | number-of-ways-to-divide-a-long-corridor | 0 | 1 | # Code #1 - Oneliner\n```\nclass Solution:\n def numberOfWays(self, c: str) -> int:\n return prod(len(m.group(1))+1 for m in re.finditer(r\'S(P*)S\', c[c.find(\'S\')+1:]))%(10**9+7) if (s:=c.count(\'S\')) and s%2 == 0 else 0\n```\n\n# Code #1.1 - Oneliner Unwrapped\n```\nclass Solution:\n def numberOfWays(self, c: str) -> int:\n MOD = 10**9 + 7\n\n seatsTotal = c.count(\'S\')\n if not (seatsTotal and seatsTotal%2 == 0):\n return 0\n\n result = 1\n for m in re.finditer(r\'S(P*)S\', c[c.find(\'S\')+1:]):\n result *= len(m.group(1)) + 1\n result %= MOD\n\n return result\n```\n\n# Code #2 - Not Oneliner\n```\nclass Solution:\n def numberOfWays(self, corridor: str) -> int:\n MOD = 10**9 + 7\n\n seatsTotal = corridor.count(\'S\')\n if seatsTotal == 0 or seatsTotal%2:\n return 0\n\n result = 1\n i = 0\n while i < len(corridor):\n seats = 0\n while i < len(corridor) and seats < 2:\n if corridor[i] == \'S\':\n seats += 1\n i += 1\n \n iPrev = i\n while i < len(corridor):\n if corridor[i] == \'S\':\n break\n i += 1\n \n if i < len(corridor) and i-iPrev:\n result *= i-iPrev+1\n result %= MOD\n\n return result\n``` | 4 | Along a long library corridor, there is a line of seats and decorative plants. You are given a **0-indexed** string `corridor` of length `n` consisting of letters `'S'` and `'P'` where each `'S'` represents a seat and each `'P'` represents a plant.
One room divider has **already** been installed to the left of index `0`, and **another** to the right of index `n - 1`. Additional room dividers can be installed. For each position between indices `i - 1` and `i` (`1 <= i <= n - 1`), at most one divider can be installed.
Divide the corridor into non-overlapping sections, where each section has **exactly two seats** with any number of plants. There may be multiple ways to perform the division. Two ways are **different** if there is a position with a room divider installed in the first way but not in the second way.
Return _the number of ways to divide the corridor_. Since the answer may be very large, return it **modulo** `109 + 7`. If there is no way, return `0`.
**Example 1:**
**Input:** corridor = "SSPPSPS "
**Output:** 3
**Explanation:** There are 3 different ways to divide the corridor.
The black bars in the above image indicate the two room dividers already installed.
Note that in each of the ways, **each** section has exactly **two** seats.
**Example 2:**
**Input:** corridor = "PPSPSP "
**Output:** 1
**Explanation:** There is only 1 way to divide the corridor, by not installing any additional dividers.
Installing any would create some section that does not have exactly two seats.
**Example 3:**
**Input:** corridor = "S "
**Output:** 0
**Explanation:** There is no way to divide the corridor because there will always be a section that does not have exactly two seats.
**Constraints:**
* `n == corridor.length`
* `1 <= n <= 105`
* `corridor[i]` is either `'S'` or `'P'`. | The number of operators in the equation is less. Could you find the right answer then generate all possible answers using different orders of operations? Divide the equation into blocks separated by the operators, and use memoization on the results of blocks for optimization. Use set and the max limit of the answer for further optimization. |
Sliding Window | O(1) Space | number-of-ways-to-divide-a-long-corridor | 0 | 1 | Simple Sliding Window approach\n# C++\n```\nint numberOfWays(string corridor) {\n long mod = 1e9 + 7;\n int ans = 1;\n int seats = 0;\n for (int l = 0, r = 0; r < corridor.size(); r++) {\n if (corridor[r] == \'P\') continue;\n seats++;\n if (seats < 2) continue;\n if (seats % 2 == 0) l = r;\n else ans = (ans * (r - l)) % mod;\n }\n if (seats < 2 || seats % 2 == 1) return 0;\n return ans;\n}\n```\n# Python\n```\ndef numberOfWays(self, corridor: str) -> int:\n mod = 1000000007\n ans = 1\n seats, l = 0, 0\n for r in range(len(corridor)):\n if corridor[r] == \'P\': continue\n seats += 1\n if seats < 2: continue\n if seats % 2 == 0: l = r\n else: ans = (ans * (r - l)) % mod\n if seats < 2 or seats % 2 == 1: return 0\n return ans\n```\n# Dart\n```\nclass Solution {\n int numberOfWays(String corridor) {\n final mod = 1000000007;\n int ans = 1, seats = 0;\n for(int l = 0, r = 0; r < corridor.length; r++) {\n if(corridor[r] == \'P\') continue;\n seats++;\n if(seats < 2) continue;\n if(seats % 2 == 0) l = r;\n else ans = (ans * (r - l)) % mod;\n }\n if(seats < 2 || seats % 2 == 1) return 0;\n return ans;\n }\n}\n``` | 1 | Along a long library corridor, there is a line of seats and decorative plants. You are given a **0-indexed** string `corridor` of length `n` consisting of letters `'S'` and `'P'` where each `'S'` represents a seat and each `'P'` represents a plant.
One room divider has **already** been installed to the left of index `0`, and **another** to the right of index `n - 1`. Additional room dividers can be installed. For each position between indices `i - 1` and `i` (`1 <= i <= n - 1`), at most one divider can be installed.
Divide the corridor into non-overlapping sections, where each section has **exactly two seats** with any number of plants. There may be multiple ways to perform the division. Two ways are **different** if there is a position with a room divider installed in the first way but not in the second way.
Return _the number of ways to divide the corridor_. Since the answer may be very large, return it **modulo** `109 + 7`. If there is no way, return `0`.
**Example 1:**
**Input:** corridor = "SSPPSPS "
**Output:** 3
**Explanation:** There are 3 different ways to divide the corridor.
The black bars in the above image indicate the two room dividers already installed.
Note that in each of the ways, **each** section has exactly **two** seats.
**Example 2:**
**Input:** corridor = "PPSPSP "
**Output:** 1
**Explanation:** There is only 1 way to divide the corridor, by not installing any additional dividers.
Installing any would create some section that does not have exactly two seats.
**Example 3:**
**Input:** corridor = "S "
**Output:** 0
**Explanation:** There is no way to divide the corridor because there will always be a section that does not have exactly two seats.
**Constraints:**
* `n == corridor.length`
* `1 <= n <= 105`
* `corridor[i]` is either `'S'` or `'P'`. | The number of operators in the equation is less. Could you find the right answer then generate all possible answers using different orders of operations? Divide the equation into blocks separated by the operators, and use memoization on the results of blocks for optimization. Use set and the max limit of the answer for further optimization. |
simple.py | count-elements-with-strictly-smaller-and-greater-elements | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countElements(self, nums: List[int]) -> int:\n k=len(nums) - nums.count(min(nums)) - nums.count(max(nums))\n if k<0:\n return 0\n return k\n``` | 3 | Given an integer array `nums`, return _the number of elements that have **both** a strictly smaller and a strictly greater element appear in_ `nums`.
**Example 1:**
**Input:** nums = \[11,7,2,15\]
**Output:** 2
**Explanation:** The element 7 has the element 2 strictly smaller than it and the element 11 strictly greater than it.
Element 11 has element 7 strictly smaller than it and element 15 strictly greater than it.
In total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Example 2:**
**Input:** nums = \[-3,3,3,90\]
**Output:** 2
**Explanation:** The element 3 has the element -3 strictly smaller than it and the element 90 strictly greater than it.
Since there are two elements with the value 3, in total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Constraints:**
* `1 <= nums.length <= 100`
* `-105 <= nums[i] <= 105` | Can we sort the arrays to help solve the problem? Can we greedily match each student to a seat? The smallest positioned student will go to the smallest positioned chair, and then the next smallest positioned student will go to the next smallest positioned chair, and so on. |
Simple python method | count-elements-with-strictly-smaller-and-greater-elements | 0 | 1 | \n\n```\nclass Solution:\n def countElements(self, nums: List[int]) -> int:\n min_num = min(nums)\n max_num = max(nums)\n count = 0\n for i in nums:\n if i > min_num and i < max_num:\n count += 1\n return count\n \n``` | 0 | Given an integer array `nums`, return _the number of elements that have **both** a strictly smaller and a strictly greater element appear in_ `nums`.
**Example 1:**
**Input:** nums = \[11,7,2,15\]
**Output:** 2
**Explanation:** The element 7 has the element 2 strictly smaller than it and the element 11 strictly greater than it.
Element 11 has element 7 strictly smaller than it and element 15 strictly greater than it.
In total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Example 2:**
**Input:** nums = \[-3,3,3,90\]
**Output:** 2
**Explanation:** The element 3 has the element -3 strictly smaller than it and the element 90 strictly greater than it.
Since there are two elements with the value 3, in total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Constraints:**
* `1 <= nums.length <= 100`
* `-105 <= nums[i] <= 105` | Can we sort the arrays to help solve the problem? Can we greedily match each student to a seat? The smallest positioned student will go to the smallest positioned chair, and then the next smallest positioned student will go to the next smallest positioned chair, and so on. |
[C++] [Python] Brute Force with Optimized Approach || Too Easy || Fully Explained | count-elements-with-strictly-smaller-and-greater-elements | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nSuppose we have the following input vector:\n```\nvector<int> nums = {3, 1, 7, 4, 2, 6};\n```\n\nNow, let\'s go through the function step by step:\n\n1. Initialize two boolean variables, `mx` (for "maximum") and `mn` (for "minimum"), to false.\n2. Initialize `cnt` (count of elements that satisfy the condition) to 0.\n3. Start a loop to iterate through the `nums` vector.\n - First iteration: `i` is 0, `nums[i]` is 3.\n - Initialize `mx` and `mn` to false.\n - Start another loop to compare the current element (`nums[i]`) with all the elements in the `nums` vector.\n - First comparison: `nums[i]` (which is 3) is not greater than `nums[j]` (which is 3), so no change in `mx`.\n - First comparison: `nums[i]` (which is 3) is not smaller than `nums[j]` (which is 3), so no change in `mn`.\n - Second comparison: `nums[i]` (which is 3) is not greater than `nums[j]` (which is 1), so no change in `mx`.\n - Second comparison: `nums[i]` (which is 3) is greater than `nums[j]` (which is 1). Set `mn` to true.\n - Third comparison: `nums[i]` (which is 3) is greater than `nums[j]` (which is 7). Set `mn` to true.\n - Fourth comparison: `nums[i]` (which is 3) is not greater than `nums[j]` (which is 4), so no change in `mx`.\n - Fourth comparison: `nums[i]` (which is 3) is not smaller than `nums[j]` (which is 4), so no change in `mn`.\n - Fifth comparison: `nums[i]` (which is 3) is not greater than `nums[j]` (which is 2), so no change in `mx`.\n - Fifth comparison: `nums[i]` (which is 3) is greater than `nums[j]` (which is 2). Set `mn` to true.\n - Sixth comparison: `nums[i]` (which is 3) is greater than `nums[j]` (which is 6). Set `mn` to true.\n - Since both `mx` and `mn` are true, increment `cnt` to 1.\n - The loop continues for the remaining elements in the `nums` vector.\n\n4. The loop ends, and `cnt` contains the count of elements in the `nums` vector that have both greater and smaller elements.\n\nIn this example, only the element 4 satisfies the condition of having both greater and smaller elements in the given `nums` vector.\n\n# Complexity\n- Time complexity:$$O(n^2)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n``` C++ []\nclass Solution {\npublic:\n int countElements(vector<int>& nums) {\n bool mx = false;\n bool mn = false;\n int cnt = 0;\n for(int i = 0; i < nums.size(); i++){\n mx = false;\n mn = false;\n for(int j = 0; j < nums.size(); j++){\n if(nums[i] > nums[j])\n mn = true;\n if(nums[i] < nums[j])\n mx = true;\n }\n if(mn == true && mx == true)\n cnt++;\n }\n return cnt;\n }\n};\n```\n``` Python []\nclass Solution:\n def countElements(self, nums: List[int]) -> int:\n cnt = 0\n for i in range(len(nums)):\n mn = min(nums)\n mx = max(nums)\n\n if(mn < nums[i] and mx > nums[i] ):\n cnt += 1\n return cnt\n```\n# Approach\nSuppose we have the following input vector:\n```\nvector<int> nums = {3, 1, 7, 4, 2, 6};\n```\n\nNow, let\'s go through the function step by step:\n\n1. Initialize `mx` (maximum value) to INT_MIN (the minimum value that can be represented by the `int` data type) and `mn` (minimum value) to INT_MAX (the maximum value that can be represented by the `int` data type).\n2. Start a loop to iterate through the `nums` vector using the range-based for loop.\n - First iteration: `it` is 3.\n - Update `mx` to the maximum of `mx` (which is INT_MIN) and `it` (which is 3), so `mx` becomes 3.\n - Update `mn` to the minimum of `mn` (which is INT_MAX) and `it` (which is 3), so `mn` becomes 3.\n - Second iteration: `it` is 1.\n - Update `mx` to the maximum of `mx` (which is 3) and `it` (which is 1), so `mx` remains 3.\n - Update `mn` to the minimum of `mn` (which is 3) and `it` (which is 1), so `mn` becomes 1.\n - Third iteration: `it` is 7.\n - Update `mx` to the maximum of `mx` (which is 3) and `it` (which is 7), so `mx` becomes 7.\n - Update `mn` to the minimum of `mn` (which is 1) and `it` (which is 7), so `mn` remains 1.\n - Fourth iteration: `it` is 4.\n - Update `mx` to the maximum of `mx` (which is 7) and `it` (which is 4), so `mx` remains 7.\n - Update `mn` to the minimum of `mn` (which is 1) and `it` (which is 4), so `mn` remains 1.\n - Fifth iteration: `it` is 2.\n - Update `mx` to the maximum of `mx` (which is 7) and `it` (which is 2), so `mx` remains 7.\n - Update `mn` to the minimum of `mn` (which is 1) and `it` (which is 2), so `mn` remains 1.\n - Sixth iteration: `it` is 6.\n - Update `mx` to the maximum of `mx` (which is 7) and `it` (which is 6), so `mx` remains 7.\n - Update `mn` to the minimum of `mn` (which is 1) and `it` (which is 6), so `mn` remains 1.\n\n3. After the first loop, `mx` is 7, and `mn` is 1, which represent the maximum and minimum values in the `nums` vector, respectively.\n4. Initialize `cnt` (count of elements that satisfy the condition) to 0.\n5. Start a loop to iterate through the `nums` vector using a traditional for loop.\n - First iteration: `i` is 0, `nums[i]` is 3.\n - Check if `nums[i]` (which is 3) is greater than `mn` (which is 1) and less than `mx` (which is 7). It satisfies the condition, so increment `cnt` to 1.\n - Second iteration: `i` is 1, `nums[i]` is 1.\n - Check if `nums[i]` (which is 1) is greater than `mn` (which is 1) and less than `mx` (which is 7). It does not satisfy the condition, so no change in `cnt`.\n - The loop continues for the remaining elements in the `nums` vector.\n\n\n# Complexity\n- Time complexity:$$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int countElements(vector<int>& nums) {\n int mx = INT_MIN;\n int mn = INT_MAX;\n for(auto it:nums){\n mx = max(mx, it);\n mn = min(mn, it);\n }\n int cnt = 0;\n for(int i = 0; i < nums.size(); i++){\n if(nums[i] > mn && nums[i] < mx)\n cnt++;\n }\n return cnt;\n }\n};\n``` | 3 | Given an integer array `nums`, return _the number of elements that have **both** a strictly smaller and a strictly greater element appear in_ `nums`.
**Example 1:**
**Input:** nums = \[11,7,2,15\]
**Output:** 2
**Explanation:** The element 7 has the element 2 strictly smaller than it and the element 11 strictly greater than it.
Element 11 has element 7 strictly smaller than it and element 15 strictly greater than it.
In total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Example 2:**
**Input:** nums = \[-3,3,3,90\]
**Output:** 2
**Explanation:** The element 3 has the element -3 strictly smaller than it and the element 90 strictly greater than it.
Since there are two elements with the value 3, in total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Constraints:**
* `1 <= nums.length <= 100`
* `-105 <= nums[i] <= 105` | Can we sort the arrays to help solve the problem? Can we greedily match each student to a seat? The smallest positioned student will go to the smallest positioned chair, and then the next smallest positioned student will go to the next smallest positioned chair, and so on. |
easiest 7 liner code in python | count-elements-with-strictly-smaller-and-greater-elements | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n## Code\n```\nclass Solution:\n def countElements(self, nums: List[int]) -> int:\n maxval=max(nums)\n minval=min(nums)\n while maxval in nums:\n nums.remove(maxval)\n while minval in nums:\n nums.remove(minval)\n return len(nums)\n #please do upvote it would be encouraging to post more solutions.\n\n```\n## Consider upvoting if found helpful\n \n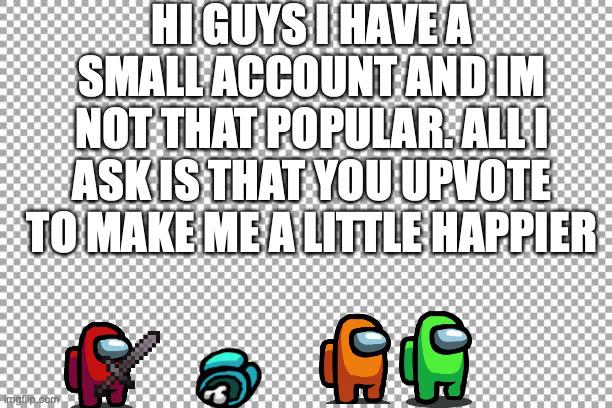\n\n | 5 | Given an integer array `nums`, return _the number of elements that have **both** a strictly smaller and a strictly greater element appear in_ `nums`.
**Example 1:**
**Input:** nums = \[11,7,2,15\]
**Output:** 2
**Explanation:** The element 7 has the element 2 strictly smaller than it and the element 11 strictly greater than it.
Element 11 has element 7 strictly smaller than it and element 15 strictly greater than it.
In total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Example 2:**
**Input:** nums = \[-3,3,3,90\]
**Output:** 2
**Explanation:** The element 3 has the element -3 strictly smaller than it and the element 90 strictly greater than it.
Since there are two elements with the value 3, in total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Constraints:**
* `1 <= nums.length <= 100`
* `-105 <= nums[i] <= 105` | Can we sort the arrays to help solve the problem? Can we greedily match each student to a seat? The smallest positioned student will go to the smallest positioned chair, and then the next smallest positioned student will go to the next smallest positioned chair, and so on. |
Very very easy code in just 3 lines using Python | count-elements-with-strictly-smaller-and-greater-elements | 0 | 1 | ```\nclass Solution:\n def countElements(self, nums: List[int]) -> int:\n res = 0\n mn = min(nums)\n mx = max(nums)\n for i in nums:\n if i > mn and i < mx:\n res += 1\n return res\n``` | 13 | Given an integer array `nums`, return _the number of elements that have **both** a strictly smaller and a strictly greater element appear in_ `nums`.
**Example 1:**
**Input:** nums = \[11,7,2,15\]
**Output:** 2
**Explanation:** The element 7 has the element 2 strictly smaller than it and the element 11 strictly greater than it.
Element 11 has element 7 strictly smaller than it and element 15 strictly greater than it.
In total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Example 2:**
**Input:** nums = \[-3,3,3,90\]
**Output:** 2
**Explanation:** The element 3 has the element -3 strictly smaller than it and the element 90 strictly greater than it.
Since there are two elements with the value 3, in total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Constraints:**
* `1 <= nums.length <= 100`
* `-105 <= nums[i] <= 105` | Can we sort the arrays to help solve the problem? Can we greedily match each student to a seat? The smallest positioned student will go to the smallest positioned chair, and then the next smallest positioned student will go to the next smallest positioned chair, and so on. |
Python Min and Max solution | count-elements-with-strictly-smaller-and-greater-elements | 0 | 1 | **Python :**\n\n```\ndef countElements(self, nums: List[int]) -> int:\n\tmin_ = min(nums)\n\tmax_ = max(nums)\n\n\treturn sum(1 for i in nums if min_ < i < max_)\n```\n\n**Like it ? please upvote !** | 8 | Given an integer array `nums`, return _the number of elements that have **both** a strictly smaller and a strictly greater element appear in_ `nums`.
**Example 1:**
**Input:** nums = \[11,7,2,15\]
**Output:** 2
**Explanation:** The element 7 has the element 2 strictly smaller than it and the element 11 strictly greater than it.
Element 11 has element 7 strictly smaller than it and element 15 strictly greater than it.
In total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Example 2:**
**Input:** nums = \[-3,3,3,90\]
**Output:** 2
**Explanation:** The element 3 has the element -3 strictly smaller than it and the element 90 strictly greater than it.
Since there are two elements with the value 3, in total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Constraints:**
* `1 <= nums.length <= 100`
* `-105 <= nums[i] <= 105` | Can we sort the arrays to help solve the problem? Can we greedily match each student to a seat? The smallest positioned student will go to the smallest positioned chair, and then the next smallest positioned student will go to the next smallest positioned chair, and so on. |
Simple Python one-liner | count-elements-with-strictly-smaller-and-greater-elements | 0 | 1 | \n# Code\n```\nclass Solution:\n def countElements(self, nums: List[int]) -> int:\n return len(nums) - nums.count(max(nums)) - nums.count(min(nums)) if max(nums) != min(nums) else 0\n``` | 3 | Given an integer array `nums`, return _the number of elements that have **both** a strictly smaller and a strictly greater element appear in_ `nums`.
**Example 1:**
**Input:** nums = \[11,7,2,15\]
**Output:** 2
**Explanation:** The element 7 has the element 2 strictly smaller than it and the element 11 strictly greater than it.
Element 11 has element 7 strictly smaller than it and element 15 strictly greater than it.
In total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Example 2:**
**Input:** nums = \[-3,3,3,90\]
**Output:** 2
**Explanation:** The element 3 has the element -3 strictly smaller than it and the element 90 strictly greater than it.
Since there are two elements with the value 3, in total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Constraints:**
* `1 <= nums.length <= 100`
* `-105 <= nums[i] <= 105` | Can we sort the arrays to help solve the problem? Can we greedily match each student to a seat? The smallest positioned student will go to the smallest positioned chair, and then the next smallest positioned student will go to the next smallest positioned chair, and so on. |
Easy python solution || 45 ms Beats 97.88% | count-elements-with-strictly-smaller-and-greater-elements | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def countElements(self, nums: List[int]) -> int:\n from collections import Counter\n if len(Counter(nums))<=1:\n return 0\n nums=sorted(nums)\n return len(nums)-nums.count(nums[0])-nums.count(nums[-1])\n``` | 2 | Given an integer array `nums`, return _the number of elements that have **both** a strictly smaller and a strictly greater element appear in_ `nums`.
**Example 1:**
**Input:** nums = \[11,7,2,15\]
**Output:** 2
**Explanation:** The element 7 has the element 2 strictly smaller than it and the element 11 strictly greater than it.
Element 11 has element 7 strictly smaller than it and element 15 strictly greater than it.
In total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Example 2:**
**Input:** nums = \[-3,3,3,90\]
**Output:** 2
**Explanation:** The element 3 has the element -3 strictly smaller than it and the element 90 strictly greater than it.
Since there are two elements with the value 3, in total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Constraints:**
* `1 <= nums.length <= 100`
* `-105 <= nums[i] <= 105` | Can we sort the arrays to help solve the problem? Can we greedily match each student to a seat? The smallest positioned student will go to the smallest positioned chair, and then the next smallest positioned student will go to the next smallest positioned chair, and so on. |
Python Easy Solution | count-elements-with-strictly-smaller-and-greater-elements | 0 | 1 | # Code\n```\nclass Solution:\n def countElements(self, nums: List[int]) -> int:\n d=dict()\n cnt=0\n for i in nums:\n if i in d:\n d[i]+=1\n else:\n d[i]=1\n nums=list(set(nums))\n nums.sort()\n if len(nums)>=3:\n for i in range(1,len(nums)-1):\n cnt+=d[nums[i]]\n return cnt\n``` | 1 | Given an integer array `nums`, return _the number of elements that have **both** a strictly smaller and a strictly greater element appear in_ `nums`.
**Example 1:**
**Input:** nums = \[11,7,2,15\]
**Output:** 2
**Explanation:** The element 7 has the element 2 strictly smaller than it and the element 11 strictly greater than it.
Element 11 has element 7 strictly smaller than it and element 15 strictly greater than it.
In total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Example 2:**
**Input:** nums = \[-3,3,3,90\]
**Output:** 2
**Explanation:** The element 3 has the element -3 strictly smaller than it and the element 90 strictly greater than it.
Since there are two elements with the value 3, in total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Constraints:**
* `1 <= nums.length <= 100`
* `-105 <= nums[i] <= 105` | Can we sort the arrays to help solve the problem? Can we greedily match each student to a seat? The smallest positioned student will go to the smallest positioned chair, and then the next smallest positioned student will go to the next smallest positioned chair, and so on. |
Very easy [Python] | count-elements-with-strictly-smaller-and-greater-elements | 0 | 1 | ```\nclass Solution:\n def countElements(self, nums: List[int]) -> int:\n min_=min(nums)\n max_=max(nums)\n c=0\n for i in nums:\n if min_<i<max_:\n c+=1\n return c\n``` | 1 | Given an integer array `nums`, return _the number of elements that have **both** a strictly smaller and a strictly greater element appear in_ `nums`.
**Example 1:**
**Input:** nums = \[11,7,2,15\]
**Output:** 2
**Explanation:** The element 7 has the element 2 strictly smaller than it and the element 11 strictly greater than it.
Element 11 has element 7 strictly smaller than it and element 15 strictly greater than it.
In total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Example 2:**
**Input:** nums = \[-3,3,3,90\]
**Output:** 2
**Explanation:** The element 3 has the element -3 strictly smaller than it and the element 90 strictly greater than it.
Since there are two elements with the value 3, in total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Constraints:**
* `1 <= nums.length <= 100`
* `-105 <= nums[i] <= 105` | Can we sort the arrays to help solve the problem? Can we greedily match each student to a seat? The smallest positioned student will go to the smallest positioned chair, and then the next smallest positioned student will go to the next smallest positioned chair, and so on. |
Python Solution Using Sorting and Counter | count-elements-with-strictly-smaller-and-greater-elements | 0 | 1 | ```\nclass Solution:\n def countElements(self, nums: List[int]) -> int:\n nums.sort()\n freq_table = Counter(nums)\n arr = list(freq_table.keys())\n arr.sort()\n ans = len(nums)\n ans -= freq_table[arr[0]]\n ans -= freq_table[arr[-1]]\n return max(ans,0)\n``` | 3 | Given an integer array `nums`, return _the number of elements that have **both** a strictly smaller and a strictly greater element appear in_ `nums`.
**Example 1:**
**Input:** nums = \[11,7,2,15\]
**Output:** 2
**Explanation:** The element 7 has the element 2 strictly smaller than it and the element 11 strictly greater than it.
Element 11 has element 7 strictly smaller than it and element 15 strictly greater than it.
In total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Example 2:**
**Input:** nums = \[-3,3,3,90\]
**Output:** 2
**Explanation:** The element 3 has the element -3 strictly smaller than it and the element 90 strictly greater than it.
Since there are two elements with the value 3, in total there are 2 elements having both a strictly smaller and a strictly greater element appear in `nums`.
**Constraints:**
* `1 <= nums.length <= 100`
* `-105 <= nums[i] <= 105` | Can we sort the arrays to help solve the problem? Can we greedily match each student to a seat? The smallest positioned student will go to the smallest positioned chair, and then the next smallest positioned student will go to the next smallest positioned chair, and so on. |
Simplest Beginner friendly Python solution | rearrange-array-elements-by-sign | 0 | 1 | \n# Code\n```\nclass Solution:\n def rearrangeArray(self, nums: List[int]) -> List[int]:\n p, n = [x for x in nums if x > 0], [x for x in nums if x < 0]\n a = []\n for i in range(len(p)):\n a.append(p[i])\n a.append(n[i])\n return a \n``` | 1 | You are given a **0-indexed** integer array `nums` of **even** length consisting of an **equal** number of positive and negative integers.
You should **rearrange** the elements of `nums` such that the modified array follows the given conditions:
1. Every **consecutive pair** of integers have **opposite signs**.
2. For all integers with the same sign, the **order** in which they were present in `nums` is **preserved**.
3. The rearranged array begins with a positive integer.
Return _the modified array after rearranging the elements to satisfy the aforementioned conditions_.
**Example 1:**
**Input:** nums = \[3,1,-2,-5,2,-4\]
**Output:** \[3,-2,1,-5,2,-4\]
**Explanation:**
The positive integers in nums are \[3,1,2\]. The negative integers are \[-2,-5,-4\].
The only possible way to rearrange them such that they satisfy all conditions is \[3,-2,1,-5,2,-4\].
Other ways such as \[1,-2,2,-5,3,-4\], \[3,1,2,-2,-5,-4\], \[-2,3,-5,1,-4,2\] are incorrect because they do not satisfy one or more conditions.
**Example 2:**
**Input:** nums = \[-1,1\]
**Output:** \[1,-1\]
**Explanation:**
1 is the only positive integer and -1 the only negative integer in nums.
So nums is rearranged to \[1,-1\].
**Constraints:**
* `2 <= nums.length <= 2 * 105`
* `nums.length` is **even**
* `1 <= |nums[i]| <= 105`
* `nums` consists of **equal** number of positive and negative integers. | Does the number of moves a player can make depend on what the other player does? No How many moves can Alice make if colors == "AAAAAA" If a group of n consecutive pieces has the same color, the player can take n - 2 of those pieces if n is greater than or equal to 3 |
2 lists | rearrange-array-elements-by-sign | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def rearrangeArray(self, nums: List[int]) -> List[int]:\n pos = []\n neg = []\n for i in nums:\n if i > 0:\n pos.append(i)\n else:\n neg.append(i)\n for k in range(len(pos)):\n nums[2*k] = pos[k]\n nums[2*k+1] = neg[k]\n return nums \n``` | 1 | You are given a **0-indexed** integer array `nums` of **even** length consisting of an **equal** number of positive and negative integers.
You should **rearrange** the elements of `nums` such that the modified array follows the given conditions:
1. Every **consecutive pair** of integers have **opposite signs**.
2. For all integers with the same sign, the **order** in which they were present in `nums` is **preserved**.
3. The rearranged array begins with a positive integer.
Return _the modified array after rearranging the elements to satisfy the aforementioned conditions_.
**Example 1:**
**Input:** nums = \[3,1,-2,-5,2,-4\]
**Output:** \[3,-2,1,-5,2,-4\]
**Explanation:**
The positive integers in nums are \[3,1,2\]. The negative integers are \[-2,-5,-4\].
The only possible way to rearrange them such that they satisfy all conditions is \[3,-2,1,-5,2,-4\].
Other ways such as \[1,-2,2,-5,3,-4\], \[3,1,2,-2,-5,-4\], \[-2,3,-5,1,-4,2\] are incorrect because they do not satisfy one or more conditions.
**Example 2:**
**Input:** nums = \[-1,1\]
**Output:** \[1,-1\]
**Explanation:**
1 is the only positive integer and -1 the only negative integer in nums.
So nums is rearranged to \[1,-1\].
**Constraints:**
* `2 <= nums.length <= 2 * 105`
* `nums.length` is **even**
* `1 <= |nums[i]| <= 105`
* `nums` consists of **equal** number of positive and negative integers. | Does the number of moves a player can make depend on what the other player does? No How many moves can Alice make if colors == "AAAAAA" If a group of n consecutive pieces has the same color, the player can take n - 2 of those pieces if n is greater than or equal to 3 |
# 3 Down to Up stage Solution | rearrange-array-elements-by-sign | 0 | 1 | @LEE BOSS\n After Solving qustions i watch @Lee submittion...Everytime i got something new and i tried to implement this in my code gives me a lot of confidence. Thanks @Lee Sir\n\n\n \'\'\' 1st ONE\n pos=[po for po in nums if po > 0]\n neg=[ne for ne in nums if ne < 0]\n res=[]\n for p, n in zip_longest(pos, neg):\n res += [p, n]\n return res\n \'\'\'\n ZIP_LONGEST:-\n Res stores the value of iterables alternatively in sequence. If one of the iterables is stored fully, the remaining values are filled by the values assigned to fillvalue parameter.\n \n\'\'\'\n@Coder_Harshit \n...2nd One...\n res=[0]*len(nums)\n pos,neg=0,1\n for i in nums:\n if i > 0:\n res[pos]=i\n pos+=2\n else:\n res[neg]=i\n neg+=2\n return res\n \'\'\'\n \'\'\'\n...3rd One.....\n neg,pos,res=[],[],[]\n for i in nums:\n if i < 0:\n neg.append(i)\n else:\n pos.append(i)\n i=0\n while i < len(neg) and i < len(pos):\n res.append(pos[i])\n res.append(neg[i])\n i+=1\n while i < len(pos):\n res.append(pos[i])\n i+=1\n while(i < len(neg)):\n res.append(neg[i])\n i+=1\n return res\n \'\'\' | 2 | You are given a **0-indexed** integer array `nums` of **even** length consisting of an **equal** number of positive and negative integers.
You should **rearrange** the elements of `nums` such that the modified array follows the given conditions:
1. Every **consecutive pair** of integers have **opposite signs**.
2. For all integers with the same sign, the **order** in which they were present in `nums` is **preserved**.
3. The rearranged array begins with a positive integer.
Return _the modified array after rearranging the elements to satisfy the aforementioned conditions_.
**Example 1:**
**Input:** nums = \[3,1,-2,-5,2,-4\]
**Output:** \[3,-2,1,-5,2,-4\]
**Explanation:**
The positive integers in nums are \[3,1,2\]. The negative integers are \[-2,-5,-4\].
The only possible way to rearrange them such that they satisfy all conditions is \[3,-2,1,-5,2,-4\].
Other ways such as \[1,-2,2,-5,3,-4\], \[3,1,2,-2,-5,-4\], \[-2,3,-5,1,-4,2\] are incorrect because they do not satisfy one or more conditions.
**Example 2:**
**Input:** nums = \[-1,1\]
**Output:** \[1,-1\]
**Explanation:**
1 is the only positive integer and -1 the only negative integer in nums.
So nums is rearranged to \[1,-1\].
**Constraints:**
* `2 <= nums.length <= 2 * 105`
* `nums.length` is **even**
* `1 <= |nums[i]| <= 105`
* `nums` consists of **equal** number of positive and negative integers. | Does the number of moves a player can make depend on what the other player does? No How many moves can Alice make if colors == "AAAAAA" If a group of n consecutive pieces has the same color, the player can take n - 2 of those pieces if n is greater than or equal to 3 |
Python Easy Solution || Hash Table | find-all-lonely-numbers-in-the-array | 0 | 1 | # Code\n```\nclass Solution:\n def findLonely(self, nums: List[int]) -> List[int]:\n dic={}\n res=[]\n for i in nums:\n if i in dic:\n dic[i]+=1\n else:\n dic[i]=1\n for i in nums:\n if dic[i]==1:\n if (i-1 not in dic) and (i+1 not in dic):\n res.append(i)\n return res\n``` | 1 | You are given an integer array `nums`. A number `x` is **lonely** when it appears only **once**, and no **adjacent** numbers (i.e. `x + 1` and `x - 1)` appear in the array.
Return _**all** lonely numbers in_ `nums`. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[10,6,5,8\]
**Output:** \[10,8\]
**Explanation:**
- 10 is a lonely number since it appears exactly once and 9 and 11 does not appear in nums.
- 8 is a lonely number since it appears exactly once and 7 and 9 does not appear in nums.
- 5 is not a lonely number since 6 appears in nums and vice versa.
Hence, the lonely numbers in nums are \[10, 8\].
Note that \[8, 10\] may also be returned.
**Example 2:**
**Input:** nums = \[1,3,5,3\]
**Output:** \[1,5\]
**Explanation:**
- 1 is a lonely number since it appears exactly once and 0 and 2 does not appear in nums.
- 5 is a lonely number since it appears exactly once and 4 and 6 does not appear in nums.
- 3 is not a lonely number since it appears twice.
Hence, the lonely numbers in nums are \[1, 5\].
Note that \[5, 1\] may also be returned.
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 106` | Can we split this problem into four cases depending on the sign of the numbers? Can we binary search the value? |
Python Elegant & Short | Hash Map | Counter | find-all-lonely-numbers-in-the-array | 0 | 1 | # Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(n)$$\n\n# Code\n```\nclass Solution:\n def findLonely(self, nums: List[int]) -> List[int]:\n count = Counter(nums)\n return [num for num, cnt in count.items() if cnt == 1 and count[num - 1] == count[num + 1] == 0]\n``` | 1 | You are given an integer array `nums`. A number `x` is **lonely** when it appears only **once**, and no **adjacent** numbers (i.e. `x + 1` and `x - 1)` appear in the array.
Return _**all** lonely numbers in_ `nums`. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[10,6,5,8\]
**Output:** \[10,8\]
**Explanation:**
- 10 is a lonely number since it appears exactly once and 9 and 11 does not appear in nums.
- 8 is a lonely number since it appears exactly once and 7 and 9 does not appear in nums.
- 5 is not a lonely number since 6 appears in nums and vice versa.
Hence, the lonely numbers in nums are \[10, 8\].
Note that \[8, 10\] may also be returned.
**Example 2:**
**Input:** nums = \[1,3,5,3\]
**Output:** \[1,5\]
**Explanation:**
- 1 is a lonely number since it appears exactly once and 0 and 2 does not appear in nums.
- 5 is a lonely number since it appears exactly once and 4 and 6 does not appear in nums.
- 3 is not a lonely number since it appears twice.
Hence, the lonely numbers in nums are \[1, 5\].
Note that \[5, 1\] may also be returned.
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 106` | Can we split this problem into four cases depending on the sign of the numbers? Can we binary search the value? |
Easy Python Solution | find-all-lonely-numbers-in-the-array | 0 | 1 | # Intuition\nThe code counts the frequency of integers in the input list using the collections.Counter() function. It then checks if an integer occurs only once in the input list and does not have adjacent integers. The integers satisfying these conditions are added to the output list.\n\n# Approach\nThe approach taken in the code is to first count the frequency of integers in the input list, and then iterate over the list to check for the required conditions. The check involves looking up the frequency of each integer and verifying that it appears only once and that its neighboring integers do not appear in the list. If the integer satisfies these conditions, it is added to the output list.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution:\n def findLonely(self, nums: List[int]) -> List[int]:\n numCount = collections.Counter(nums)\n ans = []\n\n for i in nums:\n if numCount[i] == 1 and i - 1 not in numCount and i + 1 not in numCount:\n ans.append(i)\n \n return ans\n``` | 1 | You are given an integer array `nums`. A number `x` is **lonely** when it appears only **once**, and no **adjacent** numbers (i.e. `x + 1` and `x - 1)` appear in the array.
Return _**all** lonely numbers in_ `nums`. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[10,6,5,8\]
**Output:** \[10,8\]
**Explanation:**
- 10 is a lonely number since it appears exactly once and 9 and 11 does not appear in nums.
- 8 is a lonely number since it appears exactly once and 7 and 9 does not appear in nums.
- 5 is not a lonely number since 6 appears in nums and vice versa.
Hence, the lonely numbers in nums are \[10, 8\].
Note that \[8, 10\] may also be returned.
**Example 2:**
**Input:** nums = \[1,3,5,3\]
**Output:** \[1,5\]
**Explanation:**
- 1 is a lonely number since it appears exactly once and 0 and 2 does not appear in nums.
- 5 is a lonely number since it appears exactly once and 4 and 6 does not appear in nums.
- 3 is not a lonely number since it appears twice.
Hence, the lonely numbers in nums are \[1, 5\].
Note that \[5, 1\] may also be returned.
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 106` | Can we split this problem into four cases depending on the sign of the numbers? Can we binary search the value? |
Counter | find-all-lonely-numbers-in-the-array | 0 | 1 | **Python 3**\n```python\nclass Solution:\n def findLonely(self, nums: List[int]) -> List[int]:\n m = Counter(nums)\n return [n for n in nums if m[n] == 1 and m[n - 1] + m[n + 1] == 0]\n```\n\n**C++**\n```cpp\nvector<int> findLonely(vector<int>& nums) {\n unordered_map<int, int> m;\n vector<int> res;\n for (int n : nums)\n ++m[n];\n for (const auto [n, cnt] : m)\n if (cnt == 1 && m.count(n + 1) == 0 && m.count(n - 1) == 0)\n res.push_back(n);\n return res;\n}\n``` | 49 | You are given an integer array `nums`. A number `x` is **lonely** when it appears only **once**, and no **adjacent** numbers (i.e. `x + 1` and `x - 1)` appear in the array.
Return _**all** lonely numbers in_ `nums`. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[10,6,5,8\]
**Output:** \[10,8\]
**Explanation:**
- 10 is a lonely number since it appears exactly once and 9 and 11 does not appear in nums.
- 8 is a lonely number since it appears exactly once and 7 and 9 does not appear in nums.
- 5 is not a lonely number since 6 appears in nums and vice versa.
Hence, the lonely numbers in nums are \[10, 8\].
Note that \[8, 10\] may also be returned.
**Example 2:**
**Input:** nums = \[1,3,5,3\]
**Output:** \[1,5\]
**Explanation:**
- 1 is a lonely number since it appears exactly once and 0 and 2 does not appear in nums.
- 5 is a lonely number since it appears exactly once and 4 and 6 does not appear in nums.
- 3 is not a lonely number since it appears twice.
Hence, the lonely numbers in nums are \[1, 5\].
Note that \[5, 1\] may also be returned.
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 106` | Can we split this problem into four cases depending on the sign of the numbers? Can we binary search the value? |
Python Dictionary | find-all-lonely-numbers-in-the-array | 0 | 1 | ```\nclass Solution:\n def findLonely(self, nums: List[int]) -> List[int]:\n dict1=dict()\n l=[]\n for i in nums:\n if(i in dict1.keys()):\n dict1[i]=-1\n else: \n dict1[i]=1\n dict1[i-1]=-1\n dict1[i+1]=-1 \n for i in nums:\n if(dict1[i]==1):\n l.append(i)\n return l | 2 | You are given an integer array `nums`. A number `x` is **lonely** when it appears only **once**, and no **adjacent** numbers (i.e. `x + 1` and `x - 1)` appear in the array.
Return _**all** lonely numbers in_ `nums`. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[10,6,5,8\]
**Output:** \[10,8\]
**Explanation:**
- 10 is a lonely number since it appears exactly once and 9 and 11 does not appear in nums.
- 8 is a lonely number since it appears exactly once and 7 and 9 does not appear in nums.
- 5 is not a lonely number since 6 appears in nums and vice versa.
Hence, the lonely numbers in nums are \[10, 8\].
Note that \[8, 10\] may also be returned.
**Example 2:**
**Input:** nums = \[1,3,5,3\]
**Output:** \[1,5\]
**Explanation:**
- 1 is a lonely number since it appears exactly once and 0 and 2 does not appear in nums.
- 5 is a lonely number since it appears exactly once and 4 and 6 does not appear in nums.
- 3 is not a lonely number since it appears twice.
Hence, the lonely numbers in nums are \[1, 5\].
Note that \[5, 1\] may also be returned.
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 106` | Can we split this problem into four cases depending on the sign of the numbers? Can we binary search the value? |
Python Solution Using Sorting & Counter | find-all-lonely-numbers-in-the-array | 0 | 1 | ```\nclass Solution:\n def findLonely(self, nums: List[int]) -> List[int]:\n ans = []\n freq_count = Counter(nums)\n nums.sort()\n n = len(nums)\n for i in range(n):\n val = nums[i]\n if freq_count[val] == 1 and (i == 0 or nums[i-1] != val-1) and (i == n-1 or nums[i+1] != val+1):\n ans.append(val)\n return ans\n``` | 2 | You are given an integer array `nums`. A number `x` is **lonely** when it appears only **once**, and no **adjacent** numbers (i.e. `x + 1` and `x - 1)` appear in the array.
Return _**all** lonely numbers in_ `nums`. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[10,6,5,8\]
**Output:** \[10,8\]
**Explanation:**
- 10 is a lonely number since it appears exactly once and 9 and 11 does not appear in nums.
- 8 is a lonely number since it appears exactly once and 7 and 9 does not appear in nums.
- 5 is not a lonely number since 6 appears in nums and vice versa.
Hence, the lonely numbers in nums are \[10, 8\].
Note that \[8, 10\] may also be returned.
**Example 2:**
**Input:** nums = \[1,3,5,3\]
**Output:** \[1,5\]
**Explanation:**
- 1 is a lonely number since it appears exactly once and 0 and 2 does not appear in nums.
- 5 is a lonely number since it appears exactly once and 4 and 6 does not appear in nums.
- 3 is not a lonely number since it appears twice.
Hence, the lonely numbers in nums are \[1, 5\].
Note that \[5, 1\] may also be returned.
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 106` | Can we split this problem into four cases depending on the sign of the numbers? Can we binary search the value? |
[Python] Easy to undestand hash map | find-all-lonely-numbers-in-the-array | 0 | 1 | ```\nclass Solution:\n def findLonely(self, nums: List[int]) -> List[int]:\n if not nums:\n return []\n count = {}\n for num in nums:\n if num in count:\n count[num] += 1\n else:\n count[num] = 1\n \n result = []\n for num in nums:\n if not count.get(num-1) and not count.get(num+1) and count.get(num, 0) < 2:\n result.append(num)\n return result\n``` | 1 | You are given an integer array `nums`. A number `x` is **lonely** when it appears only **once**, and no **adjacent** numbers (i.e. `x + 1` and `x - 1)` appear in the array.
Return _**all** lonely numbers in_ `nums`. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[10,6,5,8\]
**Output:** \[10,8\]
**Explanation:**
- 10 is a lonely number since it appears exactly once and 9 and 11 does not appear in nums.
- 8 is a lonely number since it appears exactly once and 7 and 9 does not appear in nums.
- 5 is not a lonely number since 6 appears in nums and vice versa.
Hence, the lonely numbers in nums are \[10, 8\].
Note that \[8, 10\] may also be returned.
**Example 2:**
**Input:** nums = \[1,3,5,3\]
**Output:** \[1,5\]
**Explanation:**
- 1 is a lonely number since it appears exactly once and 0 and 2 does not appear in nums.
- 5 is a lonely number since it appears exactly once and 4 and 6 does not appear in nums.
- 3 is not a lonely number since it appears twice.
Hence, the lonely numbers in nums are \[1, 5\].
Note that \[5, 1\] may also be returned.
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 106` | Can we split this problem into four cases depending on the sign of the numbers? Can we binary search the value? |
C++ & Python | Easy to understand | O(n) | Simple, Clean, Explained | find-all-lonely-numbers-in-the-array | 0 | 1 | ### Approach:\nHashmaps are the most convenient way since we are dealing with frequencies of more than one element. The definition of a lonely number `x` requires us to check:\n1. the presence of `x - 1` and `x + 1` -> frequency of `x - 1` and `x + 1` = 0\n2. whether frequency of `x` in the array to be **exactly 1**.\n\nHence, the steps are as follows:\n- Record frequencies of all the elements in the array\n- Iterate through the array and check for the conditions 1 and 2 above.\n- If condition is met, we append it to the result array\n\n#### C++ Solution\n```\nclass Solution {\npublic:\n vector<int> findLonely(vector<int>& nums) {\n unordered_map<int, int> freq;\n vector<int> lonelies;\n\t\t\n for (int i = 0; i < nums.size(); i++) {\n freq[nums[i]]++;\n }\n \n for (int i = 0; i < nums.size(); i++) {\n if (freq[nums[i] - 1] == 0 && freq[nums[i]] == 1 && freq[nums[i] + 1] == 0) {\n lonelies.push_back(nums[i]);\n }\n }\n \n return lonelies;\n }\n};\n```\n\n#### Python Solution\n```\nclass Solution:\n def findLonely(self, nums: List[int]) -> List[int]:\n freq = dict()\n result = []\n \n for n in nums:\n freq[n] = freq.get(n, 0) + 1\n \n for n in nums:\n if freq.get(n - 1, 0) == 0 and freq.get(n, 0) == 1 and freq.get(n + 1, 0) == 0:\n result.append(n)\n \n return result\n``` | 2 | You are given an integer array `nums`. A number `x` is **lonely** when it appears only **once**, and no **adjacent** numbers (i.e. `x + 1` and `x - 1)` appear in the array.
Return _**all** lonely numbers in_ `nums`. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[10,6,5,8\]
**Output:** \[10,8\]
**Explanation:**
- 10 is a lonely number since it appears exactly once and 9 and 11 does not appear in nums.
- 8 is a lonely number since it appears exactly once and 7 and 9 does not appear in nums.
- 5 is not a lonely number since 6 appears in nums and vice versa.
Hence, the lonely numbers in nums are \[10, 8\].
Note that \[8, 10\] may also be returned.
**Example 2:**
**Input:** nums = \[1,3,5,3\]
**Output:** \[1,5\]
**Explanation:**
- 1 is a lonely number since it appears exactly once and 0 and 2 does not appear in nums.
- 5 is a lonely number since it appears exactly once and 4 and 6 does not appear in nums.
- 3 is not a lonely number since it appears twice.
Hence, the lonely numbers in nums are \[1, 5\].
Note that \[5, 1\] may also be returned.
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 106` | Can we split this problem into four cases depending on the sign of the numbers? Can we binary search the value? |
Bitmasking Video Solution | maximum-good-people-based-on-statements | 0 | 1 | For the full video, you can check out this [Youtube video]( https://youtu.be/v7sr-1Zbh6k) \n\nSince `n<=15` we can brute force our solution and generate all possibilities of good / bad people of our group.\nThen for each of those arrangement we will verify if there are no contradictions. \nIf there are no contradictions then we will count the `1` bit. \nThat is the number of good people. \n\n```\nclass Solution: \n def maximumGood(self, statements: List[List[int]]) -> int:\n \n n = len(statements)\n res = 0\n \n def checkGood(person, mask):\n if (mask>>person) & 1:\n return True\n return False\n \n def validateStatement(mask):\n for i in range(n):\n if checkGood(i, mask):\n for j in range(n):\n statement = statements[i][j]\n \n if (statement==0 and checkGood(j, mask)) or (statement==1 and not checkGood(j, mask)):\n return False\n return True\n \n for i in range(1,1<<n): \n if validateStatement(i):\n res = max(res, bin(i).count(\'1\'))\n return res\n``` | 4 | There are two types of persons:
* The **good person**: The person who always tells the truth.
* The **bad person**: The person who might tell the truth and might lie.
You are given a **0-indexed** 2D integer array `statements` of size `n x n` that represents the statements made by `n` people about each other. More specifically, `statements[i][j]` could be one of the following:
* `0` which represents a statement made by person `i` that person `j` is a **bad** person.
* `1` which represents a statement made by person `i` that person `j` is a **good** person.
* `2` represents that **no statement** is made by person `i` about person `j`.
Additionally, no person ever makes a statement about themselves. Formally, we have that `statements[i][i] = 2` for all `0 <= i < n`.
Return _the **maximum** number of people who can be **good** based on the statements made by the_ `n` _people_.
**Example 1:**
**Input:** statements = \[\[2,1,2\],\[1,2,2\],\[2,0,2\]\]
**Output:** 2
**Explanation:** Each person makes a single statement.
- Person 0 states that person 1 is good.
- Person 1 states that person 0 is good.
- Person 2 states that person 1 is bad.
Let's take person 2 as the key.
- Assuming that person 2 is a good person:
- Based on the statement made by person 2, person 1 is a bad person.
- Now we know for sure that person 1 is bad and person 2 is good.
- Based on the statement made by person 1, and since person 1 is bad, they could be:
- telling the truth. There will be a contradiction in this case and this assumption is invalid.
- lying. In this case, person 0 is also a bad person and lied in their statement.
- **Following that person 2 is a good person, there will be only one good person in the group**.
- Assuming that person 2 is a bad person:
- Based on the statement made by person 2, and since person 2 is bad, they could be:
- telling the truth. Following this scenario, person 0 and 1 are both bad as explained before.
- **Following that person 2 is bad but told the truth, there will be no good persons in the group**.
- lying. In this case person 1 is a good person.
- Since person 1 is a good person, person 0 is also a good person.
- **Following that person 2 is bad and lied, there will be two good persons in the group**.
We can see that at most 2 persons are good in the best case, so we return 2.
Note that there is more than one way to arrive at this conclusion.
**Example 2:**
**Input:** statements = \[\[2,0\],\[0,2\]\]
**Output:** 1
**Explanation:** Each person makes a single statement.
- Person 0 states that person 1 is bad.
- Person 1 states that person 0 is bad.
Let's take person 0 as the key.
- Assuming that person 0 is a good person:
- Based on the statement made by person 0, person 1 is a bad person and was lying.
- **Following that person 0 is a good person, there will be only one good person in the group**.
- Assuming that person 0 is a bad person:
- Based on the statement made by person 0, and since person 0 is bad, they could be:
- telling the truth. Following this scenario, person 0 and 1 are both bad.
- **Following that person 0 is bad but told the truth, there will be no good persons in the group**.
- lying. In this case person 1 is a good person.
- **Following that person 0 is bad and lied, there will be only one good person in the group**.
We can see that at most, one person is good in the best case, so we return 1.
Note that there is more than one way to arrive at this conclusion.
**Constraints:**
* `n == statements.length == statements[i].length`
* `2 <= n <= 15`
* `statements[i][j]` is either `0`, `1`, or `2`.
* `statements[i][i] == 2` | What method can you use to find the shortest time taken for a message from a data server to reach the master server? How can you use this value and the server's patience value to determine the time at which the server sends its last message? What is the time when the last message sent from a server gets back to the server? For each data server, by the time the server receives the first returned messages, how many messages has the server sent? |
Python 3 | Itertools & Bitmask | Explanation | maximum-good-people-based-on-statements | 0 | 1 | ### Explanation\n- The idea is super simple: Test out possibilities and take the one has most `good` persons\n\t- There are at most `2^15 = 32768` possibilities\n\t- The `n*n` matrix `statements` will have at most `15 * 15 = 225` cells\n\t- Total will be `2 ^ 15 * 15 * 15 = 7372800 ~= 7 * 10^3 * 10^3`\n\t\t- The general acceptance time complexity for LeetCode is around `10^6`\n\t\t- With some optimization, we can make the time complexity of the idea much faster than `7 * 10^3 * 10^3`\n### Approach \\#1. Use Python `itertools.product` to create mask\n- Use `itertools.product` to make a list only contains `0` or `1` with a length of `n = len(statements)`\n- Take this list and test it against the `statements` (i.e. `Proof by contradiction`)\n\t- Assuming the list is the correct `good` & `bad` identity for each person\n\t- If a `good` person\'s statement on someone else is different from what the list says, then there is a **contradiction**\n\t- We will discard this list and continue to test the next one\n- See below comments for more explanation\n```python\nclass Solution:\n def maximumGood(self, statements: List[List[int]]) -> int:\n ans, n = 0, len(statements)\n for person in itertools.product([0, 1], repeat=n): # use itertools to create a list only contains 0 or 1\n valid = True # initially, we think the `person` list is valid\n for i in range(n):\n if not person[i]: continue # only `good` person\'s statement can lead to a contradiction, we don\'t care what `bad` person says\n for j in range(n):\n if statements[i][j] == 2: continue # ignore is no statement was made\n if statements[i][j] != person[j]: # if there is a contradiction, then valid = False\n valid = False\n break # optimization: break the loop when not valid\n if not valid: # optimization: break the loop when not valid\n break \n if valid: \n ans = max(ans, sum(person)) # count sum only when valid == True\n return ans\n```\n### Approach \\#2. Bitmask\n- Below is a bitmask version of the above approach. I think for most starters, **Approach #1** is easier to understand. If you are not familiar with bitmask or bit operation, that\'s totally fine. It takes time to build that mindset.\n- See comments below for more explanation\n```python\nclass Solution:\n def maximumGood(self, statements: List[List[int]]) -> int:\n ans, n = 0, len(statements)\n for mask in range(1 << n): # 2**n possibilities\n valid = True\n for i in range(n):\n if not (mask >> i) & 1: continue # check if the `i`th person is a `good` person\n for j in range(n):\n if statements[i][j] == 2: continue\n elif statements[i][j] != (mask >> j) & 1:# check if `i`th person\'s statement about `j` matches what `mask` says\n valid = False\n break # optimization: break the loop when not valid\n if not valid: \n break # optimization: break the loop when not valid\n if valid: \n ans = max(ans, bin(mask).count(\'1\')) # count `1` in mask, take the largest\n return ans\n``` | 2 | There are two types of persons:
* The **good person**: The person who always tells the truth.
* The **bad person**: The person who might tell the truth and might lie.
You are given a **0-indexed** 2D integer array `statements` of size `n x n` that represents the statements made by `n` people about each other. More specifically, `statements[i][j]` could be one of the following:
* `0` which represents a statement made by person `i` that person `j` is a **bad** person.
* `1` which represents a statement made by person `i` that person `j` is a **good** person.
* `2` represents that **no statement** is made by person `i` about person `j`.
Additionally, no person ever makes a statement about themselves. Formally, we have that `statements[i][i] = 2` for all `0 <= i < n`.
Return _the **maximum** number of people who can be **good** based on the statements made by the_ `n` _people_.
**Example 1:**
**Input:** statements = \[\[2,1,2\],\[1,2,2\],\[2,0,2\]\]
**Output:** 2
**Explanation:** Each person makes a single statement.
- Person 0 states that person 1 is good.
- Person 1 states that person 0 is good.
- Person 2 states that person 1 is bad.
Let's take person 2 as the key.
- Assuming that person 2 is a good person:
- Based on the statement made by person 2, person 1 is a bad person.
- Now we know for sure that person 1 is bad and person 2 is good.
- Based on the statement made by person 1, and since person 1 is bad, they could be:
- telling the truth. There will be a contradiction in this case and this assumption is invalid.
- lying. In this case, person 0 is also a bad person and lied in their statement.
- **Following that person 2 is a good person, there will be only one good person in the group**.
- Assuming that person 2 is a bad person:
- Based on the statement made by person 2, and since person 2 is bad, they could be:
- telling the truth. Following this scenario, person 0 and 1 are both bad as explained before.
- **Following that person 2 is bad but told the truth, there will be no good persons in the group**.
- lying. In this case person 1 is a good person.
- Since person 1 is a good person, person 0 is also a good person.
- **Following that person 2 is bad and lied, there will be two good persons in the group**.
We can see that at most 2 persons are good in the best case, so we return 2.
Note that there is more than one way to arrive at this conclusion.
**Example 2:**
**Input:** statements = \[\[2,0\],\[0,2\]\]
**Output:** 1
**Explanation:** Each person makes a single statement.
- Person 0 states that person 1 is bad.
- Person 1 states that person 0 is bad.
Let's take person 0 as the key.
- Assuming that person 0 is a good person:
- Based on the statement made by person 0, person 1 is a bad person and was lying.
- **Following that person 0 is a good person, there will be only one good person in the group**.
- Assuming that person 0 is a bad person:
- Based on the statement made by person 0, and since person 0 is bad, they could be:
- telling the truth. Following this scenario, person 0 and 1 are both bad.
- **Following that person 0 is bad but told the truth, there will be no good persons in the group**.
- lying. In this case person 1 is a good person.
- **Following that person 0 is bad and lied, there will be only one good person in the group**.
We can see that at most, one person is good in the best case, so we return 1.
Note that there is more than one way to arrive at this conclusion.
**Constraints:**
* `n == statements.length == statements[i].length`
* `2 <= n <= 15`
* `statements[i][j]` is either `0`, `1`, or `2`.
* `statements[i][i] == 2` | What method can you use to find the shortest time taken for a message from a data server to reach the master server? How can you use this value and the server's patience value to determine the time at which the server sends its last message? What is the time when the last message sent from a server gets back to the server? For each data server, by the time the server receives the first returned messages, how many messages has the server sent? |
Bitmask Iteration | Python | maximum-good-people-based-on-statements | 0 | 1 | \n# Code\n```\nfrom typing import List\n\n\nclass Solution:\n\tdef maximumGood(self, statements: List[List[int]]) -> int:\n\t\tn = len(statements)\n\n\t\tdef correct(mask):\n\t\t\tfor p in range(n):\n\t\t\t\tfor q in range(n):\n\t\t\t\t\tif not (\n\t\t\t\t\t\t\tstatements[p][q] == 2 or\n\t\t\t\t\t\t\t(mask >> p & 1) == 0 or\n\t\t\t\t\t\t\t(mask >> q & 1) == statements[p][q]):\n\t\t\t\t\t\treturn False\n\t\t\treturn True\n\n\t\tans = 0\n\t\tfor mask in range(1 << n):\n\t\t\tif correct(mask):\n\t\t\t\tans = max(ans, mask.bit_count())\n\t\treturn ans\n\n``` | 0 | There are two types of persons:
* The **good person**: The person who always tells the truth.
* The **bad person**: The person who might tell the truth and might lie.
You are given a **0-indexed** 2D integer array `statements` of size `n x n` that represents the statements made by `n` people about each other. More specifically, `statements[i][j]` could be one of the following:
* `0` which represents a statement made by person `i` that person `j` is a **bad** person.
* `1` which represents a statement made by person `i` that person `j` is a **good** person.
* `2` represents that **no statement** is made by person `i` about person `j`.
Additionally, no person ever makes a statement about themselves. Formally, we have that `statements[i][i] = 2` for all `0 <= i < n`.
Return _the **maximum** number of people who can be **good** based on the statements made by the_ `n` _people_.
**Example 1:**
**Input:** statements = \[\[2,1,2\],\[1,2,2\],\[2,0,2\]\]
**Output:** 2
**Explanation:** Each person makes a single statement.
- Person 0 states that person 1 is good.
- Person 1 states that person 0 is good.
- Person 2 states that person 1 is bad.
Let's take person 2 as the key.
- Assuming that person 2 is a good person:
- Based on the statement made by person 2, person 1 is a bad person.
- Now we know for sure that person 1 is bad and person 2 is good.
- Based on the statement made by person 1, and since person 1 is bad, they could be:
- telling the truth. There will be a contradiction in this case and this assumption is invalid.
- lying. In this case, person 0 is also a bad person and lied in their statement.
- **Following that person 2 is a good person, there will be only one good person in the group**.
- Assuming that person 2 is a bad person:
- Based on the statement made by person 2, and since person 2 is bad, they could be:
- telling the truth. Following this scenario, person 0 and 1 are both bad as explained before.
- **Following that person 2 is bad but told the truth, there will be no good persons in the group**.
- lying. In this case person 1 is a good person.
- Since person 1 is a good person, person 0 is also a good person.
- **Following that person 2 is bad and lied, there will be two good persons in the group**.
We can see that at most 2 persons are good in the best case, so we return 2.
Note that there is more than one way to arrive at this conclusion.
**Example 2:**
**Input:** statements = \[\[2,0\],\[0,2\]\]
**Output:** 1
**Explanation:** Each person makes a single statement.
- Person 0 states that person 1 is bad.
- Person 1 states that person 0 is bad.
Let's take person 0 as the key.
- Assuming that person 0 is a good person:
- Based on the statement made by person 0, person 1 is a bad person and was lying.
- **Following that person 0 is a good person, there will be only one good person in the group**.
- Assuming that person 0 is a bad person:
- Based on the statement made by person 0, and since person 0 is bad, they could be:
- telling the truth. Following this scenario, person 0 and 1 are both bad.
- **Following that person 0 is bad but told the truth, there will be no good persons in the group**.
- lying. In this case person 1 is a good person.
- **Following that person 0 is bad and lied, there will be only one good person in the group**.
We can see that at most, one person is good in the best case, so we return 1.
Note that there is more than one way to arrive at this conclusion.
**Constraints:**
* `n == statements.length == statements[i].length`
* `2 <= n <= 15`
* `statements[i][j]` is either `0`, `1`, or `2`.
* `statements[i][i] == 2` | What method can you use to find the shortest time taken for a message from a data server to reach the master server? How can you use this value and the server's patience value to determine the time at which the server sends its last message? What is the time when the last message sent from a server gets back to the server? For each data server, by the time the server receives the first returned messages, how many messages has the server sent? |
Top python Solution | keep-multiplying-found-values-by-two | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findFinalValue(self, nums: List[int], original: int) -> int:\n while original in nums:\n original = original*2\n return original\n``` | 2 | You are given an array of integers `nums`. You are also given an integer `original` which is the first number that needs to be searched for in `nums`.
You then do the following steps:
1. If `original` is found in `nums`, **multiply** it by two (i.e., set `original = 2 * original`).
2. Otherwise, **stop** the process.
3. **Repeat** this process with the new number as long as you keep finding the number.
Return _the **final** value of_ `original`.
**Example 1:**
**Input:** nums = \[5,3,6,1,12\], original = 3
**Output:** 24
**Explanation:**
- 3 is found in nums. 3 is multiplied by 2 to obtain 6.
- 6 is found in nums. 6 is multiplied by 2 to obtain 12.
- 12 is found in nums. 12 is multiplied by 2 to obtain 24.
- 24 is not found in nums. Thus, 24 is returned.
**Example 2:**
**Input:** nums = \[2,7,9\], original = 4
**Output:** 4
**Explanation:**
- 4 is not found in nums. Thus, 4 is returned.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i], original <= 1000` | Find the smallest substring you need to consider at a time. Try delaying a move as long as possible. |
Python solution simple faster than 80% less memory than 99% | keep-multiplying-found-values-by-two | 0 | 1 | \tclass Solution:\n\n\t def findFinalValue(self, nums: List[int], original: int) -> int:\n\t\n while original in nums:\n\t\t\n original *= 2\n\t\t\t\n return original\n\t\t\n\t\t\n | 2 | You are given an array of integers `nums`. You are also given an integer `original` which is the first number that needs to be searched for in `nums`.
You then do the following steps:
1. If `original` is found in `nums`, **multiply** it by two (i.e., set `original = 2 * original`).
2. Otherwise, **stop** the process.
3. **Repeat** this process with the new number as long as you keep finding the number.
Return _the **final** value of_ `original`.
**Example 1:**
**Input:** nums = \[5,3,6,1,12\], original = 3
**Output:** 24
**Explanation:**
- 3 is found in nums. 3 is multiplied by 2 to obtain 6.
- 6 is found in nums. 6 is multiplied by 2 to obtain 12.
- 12 is found in nums. 12 is multiplied by 2 to obtain 24.
- 24 is not found in nums. Thus, 24 is returned.
**Example 2:**
**Input:** nums = \[2,7,9\], original = 4
**Output:** 4
**Explanation:**
- 4 is not found in nums. Thus, 4 is returned.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i], original <= 1000` | Find the smallest substring you need to consider at a time. Try delaying a move as long as possible. |
very easy cod uzbeksila | keep-multiplying-found-values-by-two | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findFinalValue(self, nums: List[int], original: int) -> int:\n while original in nums:\n original*=2\n return original\n\n | 0 | You are given an array of integers `nums`. You are also given an integer `original` which is the first number that needs to be searched for in `nums`.
You then do the following steps:
1. If `original` is found in `nums`, **multiply** it by two (i.e., set `original = 2 * original`).
2. Otherwise, **stop** the process.
3. **Repeat** this process with the new number as long as you keep finding the number.
Return _the **final** value of_ `original`.
**Example 1:**
**Input:** nums = \[5,3,6,1,12\], original = 3
**Output:** 24
**Explanation:**
- 3 is found in nums. 3 is multiplied by 2 to obtain 6.
- 6 is found in nums. 6 is multiplied by 2 to obtain 12.
- 12 is found in nums. 12 is multiplied by 2 to obtain 24.
- 24 is not found in nums. Thus, 24 is returned.
**Example 2:**
**Input:** nums = \[2,7,9\], original = 4
**Output:** 4
**Explanation:**
- 4 is not found in nums. Thus, 4 is returned.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i], original <= 1000` | Find the smallest substring you need to consider at a time. Try delaying a move as long as possible. |
[Python 3] Prefix Sum + Hash Table - Easy to Understand | all-divisions-with-the-highest-score-of-a-binary-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(N)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(N)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n cnt_1 = 0\n for num in nums:\n if num == 1: cnt_1 += 1\n \n cnt_0, max_division = 0, cnt_1\n d = collections.defaultdict(list)\n d[cnt_1].append(0)\n for i, num in enumerate(nums):\n cnt_0 += int(num == 0)\n cnt_1 -= int(num == 1)\n d[cnt_0 + cnt_1].append(i + 1)\n max_division = max(max_division, cnt_0 + cnt_1)\n return d[max_division]\n``` | 2 | You are given a **0-indexed** binary array `nums` of length `n`. `nums` can be divided at index `i` (where `0 <= i <= n)` into two arrays (possibly empty) `numsleft` and `numsright`:
* `numsleft` has all the elements of `nums` between index `0` and `i - 1` **(inclusive)**, while `numsright` has all the elements of nums between index `i` and `n - 1` **(inclusive)**.
* If `i == 0`, `numsleft` is **empty**, while `numsright` has all the elements of `nums`.
* If `i == n`, `numsleft` has all the elements of nums, while `numsright` is **empty**.
The **division score** of an index `i` is the **sum** of the number of `0`'s in `numsleft` and the number of `1`'s in `numsright`.
Return _**all distinct indices** that have the **highest** possible **division score**_. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[0,0,1,0\]
**Output:** \[2,4\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,**1**,0\]. The score is 0 + 1 = 1.
- 1: numsleft is \[**0**\]. numsright is \[0,**1**,0\]. The score is 1 + 1 = 2.
- 2: numsleft is \[**0**,**0**\]. numsright is \[**1**,0\]. The score is 2 + 1 = 3.
- 3: numsleft is \[**0**,**0**,1\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 4: numsleft is \[**0**,**0**,1,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Indices 2 and 4 both have the highest possible division score 3.
Note the answer \[4,2\] would also be accepted.
**Example 2:**
**Input:** nums = \[0,0,0\]
**Output:** \[3\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,0\]. The score is 0 + 0 = 0.
- 1: numsleft is \[**0**\]. numsright is \[0,0\]. The score is 1 + 0 = 1.
- 2: numsleft is \[**0**,**0**\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 3: numsleft is \[**0**,**0**,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Only index 3 has the highest possible division score 3.
**Example 3:**
**Input:** nums = \[1,1\]
**Output:** \[0\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[**1**,**1**\]. The score is 0 + 2 = 2.
- 1: numsleft is \[1\]. numsright is \[**1**\]. The score is 0 + 1 = 1.
- 2: numsleft is \[1,1\]. numsright is \[\]. The score is 0 + 0 = 0.
Only index 0 has the highest possible division score 2.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 105`
* `nums[i]` is either `0` or `1`. | What should the sum of the n rolls be? Could you generate an array of size n such that each element is between 1 and 6? |
Prefix and Suffix arrays + HashMap | all-divisions-with-the-highest-score-of-a-binary-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nusing prefix, suffix arrays and storing running count of 0\'s and 1\'s\n# Approach\n<!-- Describe your approach to solving the problem. -->\nUsing prefix,suffix arrays by storing running count of 1\'s and 0\'s\nand then using a hashmap to map each index with its division score.\nThen finding the maximum divisionScore and returning those indices which are having max divisionScore.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->O(n)\n3 passes whole array\n\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(3n) for 2 Lists and 1 HashMap\n\n# Code\n```\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n l1=[]\n l2=[0]*len(nums)\n for i in range(len(nums)):\n if i==0:\n if nums[i]==0:\n l1.append((1,0))\n else:\n l1.append((0,1))\n else:\n if nums[i]==0:\n l1.append((l1[-1][0]+1,l1[-1][1]))\n else:\n l1.append((l1[-1][0],l1[-1][1]+1))\n for i in range(len(nums)-1,-1,-1):\n if i==len(nums)-1:\n if nums[i]==0:\n l2[i]=(1,0)\n else:\n l2[i]=(0,1)\n else:\n if nums[i]==0:\n l2[i]=(l2[i+1][0]+1,l2[i+1][1])\n else:\n l2[i]=(l2[i+1][0],l2[i+1][1]+1)\n d={}\n for i in range(len(nums)):\n if i==0:\n d[i]=l2[i][1]\n elif i==len(nums)-1:\n d[i]=l1[i-1][0]+l2[i][1]\n else:\n d[i]=l1[i-1][0]+l2[i][1]\n d[len(nums)]=l1[len(nums)-1][0]\n k=max(d.values())\n ans=[]\n for i in d:\n if d[i]==k:\n ans.append(i)\n return ans\n``` | 2 | You are given a **0-indexed** binary array `nums` of length `n`. `nums` can be divided at index `i` (where `0 <= i <= n)` into two arrays (possibly empty) `numsleft` and `numsright`:
* `numsleft` has all the elements of `nums` between index `0` and `i - 1` **(inclusive)**, while `numsright` has all the elements of nums between index `i` and `n - 1` **(inclusive)**.
* If `i == 0`, `numsleft` is **empty**, while `numsright` has all the elements of `nums`.
* If `i == n`, `numsleft` has all the elements of nums, while `numsright` is **empty**.
The **division score** of an index `i` is the **sum** of the number of `0`'s in `numsleft` and the number of `1`'s in `numsright`.
Return _**all distinct indices** that have the **highest** possible **division score**_. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[0,0,1,0\]
**Output:** \[2,4\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,**1**,0\]. The score is 0 + 1 = 1.
- 1: numsleft is \[**0**\]. numsright is \[0,**1**,0\]. The score is 1 + 1 = 2.
- 2: numsleft is \[**0**,**0**\]. numsright is \[**1**,0\]. The score is 2 + 1 = 3.
- 3: numsleft is \[**0**,**0**,1\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 4: numsleft is \[**0**,**0**,1,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Indices 2 and 4 both have the highest possible division score 3.
Note the answer \[4,2\] would also be accepted.
**Example 2:**
**Input:** nums = \[0,0,0\]
**Output:** \[3\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,0\]. The score is 0 + 0 = 0.
- 1: numsleft is \[**0**\]. numsright is \[0,0\]. The score is 1 + 0 = 1.
- 2: numsleft is \[**0**,**0**\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 3: numsleft is \[**0**,**0**,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Only index 3 has the highest possible division score 3.
**Example 3:**
**Input:** nums = \[1,1\]
**Output:** \[0\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[**1**,**1**\]. The score is 0 + 2 = 2.
- 1: numsleft is \[1\]. numsright is \[**1**\]. The score is 0 + 1 = 1.
- 2: numsleft is \[1,1\]. numsright is \[\]. The score is 0 + 0 = 0.
Only index 0 has the highest possible division score 2.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 105`
* `nums[i]` is either `0` or `1`. | What should the sum of the n rolls be? Could you generate an array of size n such that each element is between 1 and 6? |
Python | O(n) step by step solution | all-divisions-with-the-highest-score-of-a-binary-array | 0 | 1 | Starting with `i=0`, the left array will be empty, so the division score is the number of ones in `nums`, which we can easily get by summing its elements. Let\'s initialize `max_score` to be this score as well:\n```python\nscore = max_score = sum(nums)\n```\nWe may create a `highest_scores` list which will hold the indices of the maximum score as we calculate them. For now, it will hold the zeroth index since we are assuming at the start that its score is the maximum score:\n```python\nhighest_scores = [0]\n```\nThen we may loop over `nums` to calculate the division score of each index. To avoid any redundant calculations, we\'ll use the score of the previous index to calculate the score of the current index as follows:\nwhen we move a zero from the right array to the left array, the number of ones in the right array won\'t change, while the number of zeros on the left will increase by one. Thus, the total score for this index will equal the score of the previous index plus 1. \nSimilarly, moving a one from the right array to the left array will reduce the total score of the previous index by one.\n```python\nfor i, v in enumerate(nums, 1):\n\tscore += 1 if v == 0 else -1\n\tif score > max_score:\n\t\thighest_scores = [i]\n\t\tmax_score = score\n\telif score == max_score:\n\t\thighest_scores.append(i)\n```\nwhen `score > max_score`, we set `highest_score` to a list containing the index of the new `max_score`, and when `score == max_score`, we append this index to `highest_score` \n\nHere\'s the full code:\n```python\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n score = max_score = sum(nums)\n highest_scores = [0]\n \n for i, v in enumerate(nums, 1):\n score += 1 if v == 0 else -1\n if score > max_score:\n highest_scores = [i]\n max_score = score\n elif score == max_score:\n highest_scores.append(i)\n \n return highest_scores\n```\n\nAnother solution is to calculate the division scores first and then find the indices that correspond to the highest score:\n```python\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n dev_scores = [0] * (len(nums) + 1)\n dev_scores[0] = max_score = sum(nums)\n \n for i, v in enumerate(nums, 1):\n dev_scores[i] = dev_scores[i - 1] + (1 if v == 0 else -1)\n max_score = max(max_score, dev_scores[i])\n \n return [i for i, score in enumerate(dev_scores) if score == max_score]\n```\nBoth solutions have `O(n)` time complexity and `O(n)` space complexity, but the first one is slightly more efficient; As it doesn\'t create a separate list to track the scores, and it builds the `highest_scores` list while looping over `nums`. | 1 | You are given a **0-indexed** binary array `nums` of length `n`. `nums` can be divided at index `i` (where `0 <= i <= n)` into two arrays (possibly empty) `numsleft` and `numsright`:
* `numsleft` has all the elements of `nums` between index `0` and `i - 1` **(inclusive)**, while `numsright` has all the elements of nums between index `i` and `n - 1` **(inclusive)**.
* If `i == 0`, `numsleft` is **empty**, while `numsright` has all the elements of `nums`.
* If `i == n`, `numsleft` has all the elements of nums, while `numsright` is **empty**.
The **division score** of an index `i` is the **sum** of the number of `0`'s in `numsleft` and the number of `1`'s in `numsright`.
Return _**all distinct indices** that have the **highest** possible **division score**_. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[0,0,1,0\]
**Output:** \[2,4\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,**1**,0\]. The score is 0 + 1 = 1.
- 1: numsleft is \[**0**\]. numsright is \[0,**1**,0\]. The score is 1 + 1 = 2.
- 2: numsleft is \[**0**,**0**\]. numsright is \[**1**,0\]. The score is 2 + 1 = 3.
- 3: numsleft is \[**0**,**0**,1\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 4: numsleft is \[**0**,**0**,1,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Indices 2 and 4 both have the highest possible division score 3.
Note the answer \[4,2\] would also be accepted.
**Example 2:**
**Input:** nums = \[0,0,0\]
**Output:** \[3\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,0\]. The score is 0 + 0 = 0.
- 1: numsleft is \[**0**\]. numsright is \[0,0\]. The score is 1 + 0 = 1.
- 2: numsleft is \[**0**,**0**\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 3: numsleft is \[**0**,**0**,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Only index 3 has the highest possible division score 3.
**Example 3:**
**Input:** nums = \[1,1\]
**Output:** \[0\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[**1**,**1**\]. The score is 0 + 2 = 2.
- 1: numsleft is \[1\]. numsright is \[**1**\]. The score is 0 + 1 = 1.
- 2: numsleft is \[1,1\]. numsright is \[\]. The score is 0 + 0 = 0.
Only index 0 has the highest possible division score 2.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 105`
* `nums[i]` is either `0` or `1`. | What should the sum of the n rolls be? Could you generate an array of size n such that each element is between 1 and 6? |
Python || Easy || O(n) Solution || Prefix Sum | all-divisions-with-the-highest-score-of-a-binary-array | 0 | 1 | ```\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n l,r=0,sum(nums)\n n=len(nums)\n ans=[0]\n m=r\n for i in range(n):\n if nums[i]==0:\n l+=1\n else:\n r-=1\n s=l+r\n if s==m:\n ans.append(i+1)\n elif s>m:\n ans=[]\n ans.append(i+1)\n m=s\n return ans \n```\n\n**An upvote will be encouraging** | 3 | You are given a **0-indexed** binary array `nums` of length `n`. `nums` can be divided at index `i` (where `0 <= i <= n)` into two arrays (possibly empty) `numsleft` and `numsright`:
* `numsleft` has all the elements of `nums` between index `0` and `i - 1` **(inclusive)**, while `numsright` has all the elements of nums between index `i` and `n - 1` **(inclusive)**.
* If `i == 0`, `numsleft` is **empty**, while `numsright` has all the elements of `nums`.
* If `i == n`, `numsleft` has all the elements of nums, while `numsright` is **empty**.
The **division score** of an index `i` is the **sum** of the number of `0`'s in `numsleft` and the number of `1`'s in `numsright`.
Return _**all distinct indices** that have the **highest** possible **division score**_. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[0,0,1,0\]
**Output:** \[2,4\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,**1**,0\]. The score is 0 + 1 = 1.
- 1: numsleft is \[**0**\]. numsright is \[0,**1**,0\]. The score is 1 + 1 = 2.
- 2: numsleft is \[**0**,**0**\]. numsright is \[**1**,0\]. The score is 2 + 1 = 3.
- 3: numsleft is \[**0**,**0**,1\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 4: numsleft is \[**0**,**0**,1,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Indices 2 and 4 both have the highest possible division score 3.
Note the answer \[4,2\] would also be accepted.
**Example 2:**
**Input:** nums = \[0,0,0\]
**Output:** \[3\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,0\]. The score is 0 + 0 = 0.
- 1: numsleft is \[**0**\]. numsright is \[0,0\]. The score is 1 + 0 = 1.
- 2: numsleft is \[**0**,**0**\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 3: numsleft is \[**0**,**0**,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Only index 3 has the highest possible division score 3.
**Example 3:**
**Input:** nums = \[1,1\]
**Output:** \[0\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[**1**,**1**\]. The score is 0 + 2 = 2.
- 1: numsleft is \[1\]. numsright is \[**1**\]. The score is 0 + 1 = 1.
- 2: numsleft is \[1,1\]. numsright is \[\]. The score is 0 + 0 = 0.
Only index 0 has the highest possible division score 2.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 105`
* `nums[i]` is either `0` or `1`. | What should the sum of the n rolls be? Could you generate an array of size n such that each element is between 1 and 6? |
[Python3] scan | all-divisions-with-the-highest-score-of-a-binary-array | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/badfbf601ede2f43ba191b567d6f5c7e0d046286) for solutions of weekly 278. \n\n```\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n ans = [0]\n cand = most = nums.count(1)\n for i, x in enumerate(nums): \n if x == 0: cand += 1\n elif x == 1: cand -= 1\n if cand > most: ans, most = [i+1], cand\n elif cand == most: ans.append(i+1)\n return ans \n``` | 2 | You are given a **0-indexed** binary array `nums` of length `n`. `nums` can be divided at index `i` (where `0 <= i <= n)` into two arrays (possibly empty) `numsleft` and `numsright`:
* `numsleft` has all the elements of `nums` between index `0` and `i - 1` **(inclusive)**, while `numsright` has all the elements of nums between index `i` and `n - 1` **(inclusive)**.
* If `i == 0`, `numsleft` is **empty**, while `numsright` has all the elements of `nums`.
* If `i == n`, `numsleft` has all the elements of nums, while `numsright` is **empty**.
The **division score** of an index `i` is the **sum** of the number of `0`'s in `numsleft` and the number of `1`'s in `numsright`.
Return _**all distinct indices** that have the **highest** possible **division score**_. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[0,0,1,0\]
**Output:** \[2,4\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,**1**,0\]. The score is 0 + 1 = 1.
- 1: numsleft is \[**0**\]. numsright is \[0,**1**,0\]. The score is 1 + 1 = 2.
- 2: numsleft is \[**0**,**0**\]. numsright is \[**1**,0\]. The score is 2 + 1 = 3.
- 3: numsleft is \[**0**,**0**,1\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 4: numsleft is \[**0**,**0**,1,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Indices 2 and 4 both have the highest possible division score 3.
Note the answer \[4,2\] would also be accepted.
**Example 2:**
**Input:** nums = \[0,0,0\]
**Output:** \[3\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,0\]. The score is 0 + 0 = 0.
- 1: numsleft is \[**0**\]. numsright is \[0,0\]. The score is 1 + 0 = 1.
- 2: numsleft is \[**0**,**0**\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 3: numsleft is \[**0**,**0**,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Only index 3 has the highest possible division score 3.
**Example 3:**
**Input:** nums = \[1,1\]
**Output:** \[0\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[**1**,**1**\]. The score is 0 + 2 = 2.
- 1: numsleft is \[1\]. numsright is \[**1**\]. The score is 0 + 1 = 1.
- 2: numsleft is \[1,1\]. numsright is \[\]. The score is 0 + 0 = 0.
Only index 0 has the highest possible division score 2.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 105`
* `nums[i]` is either `0` or `1`. | What should the sum of the n rolls be? Could you generate an array of size n such that each element is between 1 and 6? |
Python 3 Solutions | all-divisions-with-the-highest-score-of-a-binary-array | 0 | 1 | # Approach 1:\nCounted the zeros from left(NumsLeft) and ones from the right(NumsRight).\nFound the total sum for each index i and if it is the maximum sum, appended the index in a list.\n# Solution 1:\n```\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n\t\tn=len(nums)\n\t\tzero=[0]*(n+1)\n\t\tone=[0]*(n+1)\n\t\tfor i in range(n):\n\t\t\tzero[i+1]=zero[i]+(nums[i]==0)\n\t\tfor i in range(n-1,-1,-1):\n\t\t\tone[i]=one[i+1]+(nums[i]==1)\n\t\ttotal = [0]*(n+1)\n\t\tm=0\n\t\tres=[]\n\t\tfor i in range(n+1):\n\t\t\ttotal[i]=zero[i]+one[i]\n\t\t\tif total[i]>m:\n\t\t\t\tres=[]\n\t\t\t\tm=total[i]\n\t\t\tif total[i]==m:\n\t\t\t\tres+=[i]\n\t\treturn res\n```\n\nTime Complexity: O(n)\nSpace Complexity: O(n)\n# Approach 2:\nAnother approach was to use PREFIX sum.\nWe use a prefix sum which calculates the total prefix sum at each index i.\nThis combines the first two loops from previous solution into one.\n# Solution 2:\n```\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n n=len(nums)\n res=[]\n pref=[0]*(n+1)\n for i in range(n):\n pref[i+1]=pref[i]+nums[i]\n zero,total,one=0,0,0\n m=-1\n for i in range(n+1):\n one=pref[n]-pref[i]\n zero=i-pref[i]\n total=zero+one\n if total>m:\n m=total\n res=[]\n if total==m:\n res+=[i]\n return res\n```\nTime Complexity: O(n)\nSpace Complexity: O(n)\n# Approach 3:\nInstead of using a prefix array, we can just count the number of ones and use it to calculate prefix sum at each index i.\nThis reduces the Space Complexity to O(1).\n# Solution 3:\n```\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n n=len(nums)\n res=[0]\n onecount=0\n for i in range(n):\n onecount+=(nums[i]==1)\n m=onecount\n for i in range(n):\n onecount+=(nums[i]==0)-(nums[i]==1)\n if onecount>=m:\n if onecount!=m:\n m=onecount\n res=[]\n res+=[i+1]\n return res\n```\nTime Complexity: O(n)\nSpace Complexity: O(1) | 2 | You are given a **0-indexed** binary array `nums` of length `n`. `nums` can be divided at index `i` (where `0 <= i <= n)` into two arrays (possibly empty) `numsleft` and `numsright`:
* `numsleft` has all the elements of `nums` between index `0` and `i - 1` **(inclusive)**, while `numsright` has all the elements of nums between index `i` and `n - 1` **(inclusive)**.
* If `i == 0`, `numsleft` is **empty**, while `numsright` has all the elements of `nums`.
* If `i == n`, `numsleft` has all the elements of nums, while `numsright` is **empty**.
The **division score** of an index `i` is the **sum** of the number of `0`'s in `numsleft` and the number of `1`'s in `numsright`.
Return _**all distinct indices** that have the **highest** possible **division score**_. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[0,0,1,0\]
**Output:** \[2,4\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,**1**,0\]. The score is 0 + 1 = 1.
- 1: numsleft is \[**0**\]. numsright is \[0,**1**,0\]. The score is 1 + 1 = 2.
- 2: numsleft is \[**0**,**0**\]. numsright is \[**1**,0\]. The score is 2 + 1 = 3.
- 3: numsleft is \[**0**,**0**,1\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 4: numsleft is \[**0**,**0**,1,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Indices 2 and 4 both have the highest possible division score 3.
Note the answer \[4,2\] would also be accepted.
**Example 2:**
**Input:** nums = \[0,0,0\]
**Output:** \[3\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,0\]. The score is 0 + 0 = 0.
- 1: numsleft is \[**0**\]. numsright is \[0,0\]. The score is 1 + 0 = 1.
- 2: numsleft is \[**0**,**0**\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 3: numsleft is \[**0**,**0**,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Only index 3 has the highest possible division score 3.
**Example 3:**
**Input:** nums = \[1,1\]
**Output:** \[0\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[**1**,**1**\]. The score is 0 + 2 = 2.
- 1: numsleft is \[1\]. numsright is \[**1**\]. The score is 0 + 1 = 1.
- 2: numsleft is \[1,1\]. numsright is \[\]. The score is 0 + 0 = 0.
Only index 0 has the highest possible division score 2.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 105`
* `nums[i]` is either `0` or `1`. | What should the sum of the n rolls be? Could you generate an array of size n such that each element is between 1 and 6? |
python | O(n) | Beats 100.00% | all-divisions-with-the-highest-score-of-a-binary-array | 0 | 1 | \n# Approach\n<!-- Describe your approach to solving the problem. -->\n- we first count the number of zeros and ones\n- initalize an array to store the score \n- add the value of ones at first\n- create another variable to keep count of the zeros that are to the left\n- iterate starting from index 1 to len(nums)+1\n- with each iteration if nums[i-1] is zero i increment curzero variable else we decrement the ones count because it went to the left subarray\n- we append the sum of curzero and ones to score\n- maxi----max(score)\n- create a list to store the indices where highest score is located\n- iterate through the score and append those indices whose value are equal to max and return ans\n\n# Complexity\n- Time complexity:O(N)\n\n- Space complexity:O(N)\n\n\n# Code\n```\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n ones=nums.count(1)\n zeros=nums.count(0)\n\n score=[ones]\n curzero=0\n\n for i in range(1,len(nums)+1):\n if nums[i-1]==0:\n curzero+=1\n else:\n ones-=1\n score.append(curzero+ones)\n\n ans=[]\n maxi=max(score)\n\n for i in range(len(score)):\n if score[i]==maxi:\n ans.append(i)\n return ans\n``` | 0 | You are given a **0-indexed** binary array `nums` of length `n`. `nums` can be divided at index `i` (where `0 <= i <= n)` into two arrays (possibly empty) `numsleft` and `numsright`:
* `numsleft` has all the elements of `nums` between index `0` and `i - 1` **(inclusive)**, while `numsright` has all the elements of nums between index `i` and `n - 1` **(inclusive)**.
* If `i == 0`, `numsleft` is **empty**, while `numsright` has all the elements of `nums`.
* If `i == n`, `numsleft` has all the elements of nums, while `numsright` is **empty**.
The **division score** of an index `i` is the **sum** of the number of `0`'s in `numsleft` and the number of `1`'s in `numsright`.
Return _**all distinct indices** that have the **highest** possible **division score**_. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[0,0,1,0\]
**Output:** \[2,4\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,**1**,0\]. The score is 0 + 1 = 1.
- 1: numsleft is \[**0**\]. numsright is \[0,**1**,0\]. The score is 1 + 1 = 2.
- 2: numsleft is \[**0**,**0**\]. numsright is \[**1**,0\]. The score is 2 + 1 = 3.
- 3: numsleft is \[**0**,**0**,1\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 4: numsleft is \[**0**,**0**,1,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Indices 2 and 4 both have the highest possible division score 3.
Note the answer \[4,2\] would also be accepted.
**Example 2:**
**Input:** nums = \[0,0,0\]
**Output:** \[3\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,0\]. The score is 0 + 0 = 0.
- 1: numsleft is \[**0**\]. numsright is \[0,0\]. The score is 1 + 0 = 1.
- 2: numsleft is \[**0**,**0**\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 3: numsleft is \[**0**,**0**,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Only index 3 has the highest possible division score 3.
**Example 3:**
**Input:** nums = \[1,1\]
**Output:** \[0\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[**1**,**1**\]. The score is 0 + 2 = 2.
- 1: numsleft is \[1\]. numsright is \[**1**\]. The score is 0 + 1 = 1.
- 2: numsleft is \[1,1\]. numsright is \[\]. The score is 0 + 0 = 0.
Only index 0 has the highest possible division score 2.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 105`
* `nums[i]` is either `0` or `1`. | What should the sum of the n rolls be? Could you generate an array of size n such that each element is between 1 and 6? |
95%, 95%, 7 Line O(n) | all-divisions-with-the-highest-score-of-a-binary-array | 0 | 1 | # Code\n```\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n mx = nums.count(1); cnt = [0]; cur = mx\n for i, num in enumerate(nums):\n if num == 0: cur += 1\n else: cur -=1\n if cur > mx: mx = cur; cnt = [i+1]\n elif cur == mx: cnt.append(i+1)\n return cnt\n``` | 0 | You are given a **0-indexed** binary array `nums` of length `n`. `nums` can be divided at index `i` (where `0 <= i <= n)` into two arrays (possibly empty) `numsleft` and `numsright`:
* `numsleft` has all the elements of `nums` between index `0` and `i - 1` **(inclusive)**, while `numsright` has all the elements of nums between index `i` and `n - 1` **(inclusive)**.
* If `i == 0`, `numsleft` is **empty**, while `numsright` has all the elements of `nums`.
* If `i == n`, `numsleft` has all the elements of nums, while `numsright` is **empty**.
The **division score** of an index `i` is the **sum** of the number of `0`'s in `numsleft` and the number of `1`'s in `numsright`.
Return _**all distinct indices** that have the **highest** possible **division score**_. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[0,0,1,0\]
**Output:** \[2,4\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,**1**,0\]. The score is 0 + 1 = 1.
- 1: numsleft is \[**0**\]. numsright is \[0,**1**,0\]. The score is 1 + 1 = 2.
- 2: numsleft is \[**0**,**0**\]. numsright is \[**1**,0\]. The score is 2 + 1 = 3.
- 3: numsleft is \[**0**,**0**,1\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 4: numsleft is \[**0**,**0**,1,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Indices 2 and 4 both have the highest possible division score 3.
Note the answer \[4,2\] would also be accepted.
**Example 2:**
**Input:** nums = \[0,0,0\]
**Output:** \[3\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,0\]. The score is 0 + 0 = 0.
- 1: numsleft is \[**0**\]. numsright is \[0,0\]. The score is 1 + 0 = 1.
- 2: numsleft is \[**0**,**0**\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 3: numsleft is \[**0**,**0**,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Only index 3 has the highest possible division score 3.
**Example 3:**
**Input:** nums = \[1,1\]
**Output:** \[0\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[**1**,**1**\]. The score is 0 + 2 = 2.
- 1: numsleft is \[1\]. numsright is \[**1**\]. The score is 0 + 1 = 1.
- 2: numsleft is \[1,1\]. numsright is \[\]. The score is 0 + 0 = 0.
Only index 0 has the highest possible division score 2.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 105`
* `nums[i]` is either `0` or `1`. | What should the sum of the n rolls be? Could you generate an array of size n such that each element is between 1 and 6? |
Easy to understand Python3 solution | all-divisions-with-the-highest-score-of-a-binary-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n leftsum, rightsum = 0, nums.count(1)\n\n d = {}\n\n i = 0\n while i <= len(nums):\n score = leftsum + rightsum\n d[i] = score\n\n if i >= len(nums):\n break\n\n if nums[i] == 0:\n leftsum += 1\n if nums[i] == 1:\n rightsum -= 1\n \n i += 1\n \n d = list(sorted(d.items(), key= lambda x: -x[1]))\n m = d[0][1]\n\n res = []\n\n for k,v in d:\n if v == m:\n res.append(k)\n else:\n break\n\n return res\n \n``` | 0 | You are given a **0-indexed** binary array `nums` of length `n`. `nums` can be divided at index `i` (where `0 <= i <= n)` into two arrays (possibly empty) `numsleft` and `numsright`:
* `numsleft` has all the elements of `nums` between index `0` and `i - 1` **(inclusive)**, while `numsright` has all the elements of nums between index `i` and `n - 1` **(inclusive)**.
* If `i == 0`, `numsleft` is **empty**, while `numsright` has all the elements of `nums`.
* If `i == n`, `numsleft` has all the elements of nums, while `numsright` is **empty**.
The **division score** of an index `i` is the **sum** of the number of `0`'s in `numsleft` and the number of `1`'s in `numsright`.
Return _**all distinct indices** that have the **highest** possible **division score**_. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[0,0,1,0\]
**Output:** \[2,4\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,**1**,0\]. The score is 0 + 1 = 1.
- 1: numsleft is \[**0**\]. numsright is \[0,**1**,0\]. The score is 1 + 1 = 2.
- 2: numsleft is \[**0**,**0**\]. numsright is \[**1**,0\]. The score is 2 + 1 = 3.
- 3: numsleft is \[**0**,**0**,1\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 4: numsleft is \[**0**,**0**,1,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Indices 2 and 4 both have the highest possible division score 3.
Note the answer \[4,2\] would also be accepted.
**Example 2:**
**Input:** nums = \[0,0,0\]
**Output:** \[3\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,0\]. The score is 0 + 0 = 0.
- 1: numsleft is \[**0**\]. numsright is \[0,0\]. The score is 1 + 0 = 1.
- 2: numsleft is \[**0**,**0**\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 3: numsleft is \[**0**,**0**,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Only index 3 has the highest possible division score 3.
**Example 3:**
**Input:** nums = \[1,1\]
**Output:** \[0\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[**1**,**1**\]. The score is 0 + 2 = 2.
- 1: numsleft is \[1\]. numsright is \[**1**\]. The score is 0 + 1 = 1.
- 2: numsleft is \[1,1\]. numsright is \[\]. The score is 0 + 0 = 0.
Only index 0 has the highest possible division score 2.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 105`
* `nums[i]` is either `0` or `1`. | What should the sum of the n rolls be? Could you generate an array of size n such that each element is between 1 and 6? |
A clear O(n) solution | all-divisions-with-the-highest-score-of-a-binary-array | 0 | 1 | # Intuition\nIf we know the score for division at index $$i$$, then calculating the score for $$i + 1$$ is easy.\n\nWhen we move from $$i$$ to $$i + 1$$ state, we remove $$nums[i]$$ from $$nums_{right}$$ and then add it to $$nums_{left}$$.\n\n- If $$nums[i]$$ is $$0$$, then it is a gain for $$nums_{left}$$. $$nums_{right}$$ isn\'t affected. So, we increment previous score.\n- If $$nums[i]$$ is $$1$$, then it is a loss for $$nums_{right}$$. $$nums_{left}$$ isn\'t affected. So, we decrement previous score.\n\nIf we can come up with the score for $$i = 0$$, then we can compute the rest of the scores in one pass.\n\n# Approach\nWhat is the score for $$i = 0$$?\n\nIn $$i = 0$$ state, $$nums_{left}$$ is empty. $$nums_{right}$$ is the entire array. So, the score is equal to number of $$1$$s in the entire array:\n\n```python\nscore = sum(nums)\n```\n\nWe also need to keep track of the maximum score found so far and the indices that can give this maximum score. Initially, the maximum score is the score for $$i = 0$$ and the only index that gives this score is index $$0$$:\n\n```python\nmax_score, indices = score, [0]\n```\n\nWe go through the entire array and at each step we compute a new `score`:\n\n```python\nfor i, num in enumerate(nums):\n if num == 0:\n score += 1\n else:\n score -= 1\n```\n\nNow with that new score, we may have 3 outcomes:\n\n1. New `score` is less than the `max_score`. Nothing needs to be updated.\n2. New `score` is more than the `max_score`. `max_score` is updated. We just reached this `max_score` and only index that gives this `max_score` is the current one. So, `indices` becomes `[i + 1]`.\n3. New `score` is equal to the `max_score`. We found a new index that gives this `max_score`. So, we append `i + 1` to `indices`.\n\nLet\'s add this logic:\n\n```python\nfor i, num in enumerate(nums):\n .\n .\n .\n if score > max_score:\n max_score, indices = score, [i + 1]\n elif score == max_score:\n indices.append(i + 1)\n```\n\nAt the end, we return `indices`:\n\n```python\nreturn indices\n```\n\n# Complexity\n- Time complexity: $$O(n)$$\nWe pass through the array twice. Once for counting $$1$$s and once for figuring out the `max_score` and `indices`.\n\n- Space complexity: $$O(n)$$\nWe only allocate for `indices` and when $$nums$$ has a pattern of $$0, 1, 0, 1, 0, 1$$, `indices` may have $$O(\\frac{n}{2})$$ elements.\n\n# Code\n```python\nclass Solution:\n def maxScoreIndices(self, nums: List[int]) -> List[int]:\n score = sum(nums)\n max_score, result = score, [0]\n for i, num in enumerate(nums):\n if num == 0:\n score += 1\n else:\n score -= 1\n\n if score > max_score:\n max_score, result = score, [i + 1]\n elif score == max_score:\n result.append(i + 1)\n return result\n``` | 0 | You are given a **0-indexed** binary array `nums` of length `n`. `nums` can be divided at index `i` (where `0 <= i <= n)` into two arrays (possibly empty) `numsleft` and `numsright`:
* `numsleft` has all the elements of `nums` between index `0` and `i - 1` **(inclusive)**, while `numsright` has all the elements of nums between index `i` and `n - 1` **(inclusive)**.
* If `i == 0`, `numsleft` is **empty**, while `numsright` has all the elements of `nums`.
* If `i == n`, `numsleft` has all the elements of nums, while `numsright` is **empty**.
The **division score** of an index `i` is the **sum** of the number of `0`'s in `numsleft` and the number of `1`'s in `numsright`.
Return _**all distinct indices** that have the **highest** possible **division score**_. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[0,0,1,0\]
**Output:** \[2,4\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,**1**,0\]. The score is 0 + 1 = 1.
- 1: numsleft is \[**0**\]. numsright is \[0,**1**,0\]. The score is 1 + 1 = 2.
- 2: numsleft is \[**0**,**0**\]. numsright is \[**1**,0\]. The score is 2 + 1 = 3.
- 3: numsleft is \[**0**,**0**,1\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 4: numsleft is \[**0**,**0**,1,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Indices 2 and 4 both have the highest possible division score 3.
Note the answer \[4,2\] would also be accepted.
**Example 2:**
**Input:** nums = \[0,0,0\]
**Output:** \[3\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,0\]. The score is 0 + 0 = 0.
- 1: numsleft is \[**0**\]. numsright is \[0,0\]. The score is 1 + 0 = 1.
- 2: numsleft is \[**0**,**0**\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 3: numsleft is \[**0**,**0**,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Only index 3 has the highest possible division score 3.
**Example 3:**
**Input:** nums = \[1,1\]
**Output:** \[0\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[**1**,**1**\]. The score is 0 + 2 = 2.
- 1: numsleft is \[1\]. numsright is \[**1**\]. The score is 0 + 1 = 1.
- 2: numsleft is \[1,1\]. numsright is \[\]. The score is 0 + 0 = 0.
Only index 0 has the highest possible division score 2.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 105`
* `nums[i]` is either `0` or `1`. | What should the sum of the n rolls be? Could you generate an array of size n such that each element is between 1 and 6? |
Python || Clean and Simple | all-divisions-with-the-highest-score-of-a-binary-array | 0 | 1 | ```\nclass Solution:\n def maxScoreIndices(self, A: list[int]) -> list[int]:\n output, s = [], -1\n\n for i, score in enumerate(self.generate_scores(A)):\n if s < score:\n output, s = [i], score\n elif s == score:\n output.append(i)\n\n return output\n\n @staticmethod\n def generate_scores(A: list[int]):\n zeros, ones = 0, sum(A)\n yield zeros + ones\n\n for a in A:\n if a == 0:\n zeros += 1\n elif a == 1:\n ones -= 1\n\n yield zeros + ones\n``` | 0 | You are given a **0-indexed** binary array `nums` of length `n`. `nums` can be divided at index `i` (where `0 <= i <= n)` into two arrays (possibly empty) `numsleft` and `numsright`:
* `numsleft` has all the elements of `nums` between index `0` and `i - 1` **(inclusive)**, while `numsright` has all the elements of nums between index `i` and `n - 1` **(inclusive)**.
* If `i == 0`, `numsleft` is **empty**, while `numsright` has all the elements of `nums`.
* If `i == n`, `numsleft` has all the elements of nums, while `numsright` is **empty**.
The **division score** of an index `i` is the **sum** of the number of `0`'s in `numsleft` and the number of `1`'s in `numsright`.
Return _**all distinct indices** that have the **highest** possible **division score**_. You may return the answer in **any order**.
**Example 1:**
**Input:** nums = \[0,0,1,0\]
**Output:** \[2,4\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,**1**,0\]. The score is 0 + 1 = 1.
- 1: numsleft is \[**0**\]. numsright is \[0,**1**,0\]. The score is 1 + 1 = 2.
- 2: numsleft is \[**0**,**0**\]. numsright is \[**1**,0\]. The score is 2 + 1 = 3.
- 3: numsleft is \[**0**,**0**,1\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 4: numsleft is \[**0**,**0**,1,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Indices 2 and 4 both have the highest possible division score 3.
Note the answer \[4,2\] would also be accepted.
**Example 2:**
**Input:** nums = \[0,0,0\]
**Output:** \[3\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[0,0,0\]. The score is 0 + 0 = 0.
- 1: numsleft is \[**0**\]. numsright is \[0,0\]. The score is 1 + 0 = 1.
- 2: numsleft is \[**0**,**0**\]. numsright is \[0\]. The score is 2 + 0 = 2.
- 3: numsleft is \[**0**,**0**,**0**\]. numsright is \[\]. The score is 3 + 0 = 3.
Only index 3 has the highest possible division score 3.
**Example 3:**
**Input:** nums = \[1,1\]
**Output:** \[0\]
**Explanation:** Division at index
- 0: numsleft is \[\]. numsright is \[**1**,**1**\]. The score is 0 + 2 = 2.
- 1: numsleft is \[1\]. numsright is \[**1**\]. The score is 0 + 1 = 1.
- 2: numsleft is \[1,1\]. numsright is \[\]. The score is 0 + 0 = 0.
Only index 0 has the highest possible division score 2.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 105`
* `nums[i]` is either `0` or `1`. | What should the sum of the n rolls be? Could you generate an array of size n such that each element is between 1 and 6? |
[Python3] rolling hash | find-substring-with-given-hash-value | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/badfbf601ede2f43ba191b567d6f5c7e0d046286) for solutions of weekly 278. \n\n```\nclass Solution:\n def subStrHash(self, s: str, power: int, modulo: int, k: int, hashValue: int) -> str:\n pp = pow(power, k-1, modulo)\n hs = ii = 0 \n for i, ch in enumerate(reversed(s)): \n if i >= k: hs -= (ord(s[~(i-k)]) - 96)*pp\n hs = (hs * power + (ord(ch) - 96)) % modulo\n if i >= k-1 and hs == hashValue: ii = i \n return s[~ii:~ii+k or None]\n``` | 2 | The hash of a **0-indexed** string `s` of length `k`, given integers `p` and `m`, is computed using the following function:
* `hash(s, p, m) = (val(s[0]) * p0 + val(s[1]) * p1 + ... + val(s[k-1]) * pk-1) mod m`.
Where `val(s[i])` represents the index of `s[i]` in the alphabet from `val('a') = 1` to `val('z') = 26`.
You are given a string `s` and the integers `power`, `modulo`, `k`, and `hashValue.` Return `sub`, _the **first** **substring** of_ `s` _of length_ `k` _such that_ `hash(sub, power, modulo) == hashValue`.
The test cases will be generated such that an answer always **exists**.
A **substring** is a contiguous non-empty sequence of characters within a string.
**Example 1:**
**Input:** s = "leetcode ", power = 7, modulo = 20, k = 2, hashValue = 0
**Output:** "ee "
**Explanation:** The hash of "ee " can be computed to be hash( "ee ", 7, 20) = (5 \* 1 + 5 \* 7) mod 20 = 40 mod 20 = 0.
"ee " is the first substring of length 2 with hashValue 0. Hence, we return "ee ".
**Example 2:**
**Input:** s = "fbxzaad ", power = 31, modulo = 100, k = 3, hashValue = 32
**Output:** "fbx "
**Explanation:** The hash of "fbx " can be computed to be hash( "fbx ", 31, 100) = (6 \* 1 + 2 \* 31 + 24 \* 312) mod 100 = 23132 mod 100 = 32.
The hash of "bxz " can be computed to be hash( "bxz ", 31, 100) = (2 \* 1 + 24 \* 31 + 26 \* 312) mod 100 = 25732 mod 100 = 32.
"fbx " is the first substring of length 3 with hashValue 32. Hence, we return "fbx ".
Note that "bxz " also has a hash of 32 but it appears later than "fbx ".
**Constraints:**
* `1 <= k <= s.length <= 2 * 104`
* `1 <= power, modulo <= 109`
* `0 <= hashValue < modulo`
* `s` consists of lowercase English letters only.
* The test cases are generated such that an answer always **exists**. | There are limited outcomes given the current sum and the stones remaining. Can we greedily simulate starting with taking a stone with remainder 1 or 2 divided by 3? |
Rolling Hash Back to Front in python3 | find-substring-with-given-hash-value | 0 | 1 | ```\nclass Solution:\n def subStrHash(self, s: str, power: int, modulo: int, k: int, hashValue: int) -> str:\n ords = [ord(l) - ord(\'a\') + 1 for l in s]\n pows = [pow(power,i,modulo) for i in range(k)]\n n = len(s) \n hash0 = sum(pows[i]*ords[n-k+i] for i in range(k)) % (modulo)\n res = \'\' \n if hash0 == hashValue:\n res = s[n-k:]\n\n for i in range(n-2,k-2,-1):\n sa = ords[i+1]\n sb = ords[i-k+1]\n hash0 -= sa*pows[-1]\n hash0 *= power\n hash0 += sb*pows[0]\n hash0 = hash0 % modulo\n \n if hash0 == hashValue:\n res = s[i-k+1:i+1]\n return res\n``` | 0 | The hash of a **0-indexed** string `s` of length `k`, given integers `p` and `m`, is computed using the following function:
* `hash(s, p, m) = (val(s[0]) * p0 + val(s[1]) * p1 + ... + val(s[k-1]) * pk-1) mod m`.
Where `val(s[i])` represents the index of `s[i]` in the alphabet from `val('a') = 1` to `val('z') = 26`.
You are given a string `s` and the integers `power`, `modulo`, `k`, and `hashValue.` Return `sub`, _the **first** **substring** of_ `s` _of length_ `k` _such that_ `hash(sub, power, modulo) == hashValue`.
The test cases will be generated such that an answer always **exists**.
A **substring** is a contiguous non-empty sequence of characters within a string.
**Example 1:**
**Input:** s = "leetcode ", power = 7, modulo = 20, k = 2, hashValue = 0
**Output:** "ee "
**Explanation:** The hash of "ee " can be computed to be hash( "ee ", 7, 20) = (5 \* 1 + 5 \* 7) mod 20 = 40 mod 20 = 0.
"ee " is the first substring of length 2 with hashValue 0. Hence, we return "ee ".
**Example 2:**
**Input:** s = "fbxzaad ", power = 31, modulo = 100, k = 3, hashValue = 32
**Output:** "fbx "
**Explanation:** The hash of "fbx " can be computed to be hash( "fbx ", 31, 100) = (6 \* 1 + 2 \* 31 + 24 \* 312) mod 100 = 23132 mod 100 = 32.
The hash of "bxz " can be computed to be hash( "bxz ", 31, 100) = (2 \* 1 + 24 \* 31 + 26 \* 312) mod 100 = 25732 mod 100 = 32.
"fbx " is the first substring of length 3 with hashValue 32. Hence, we return "fbx ".
Note that "bxz " also has a hash of 32 but it appears later than "fbx ".
**Constraints:**
* `1 <= k <= s.length <= 2 * 104`
* `1 <= power, modulo <= 109`
* `0 <= hashValue < modulo`
* `s` consists of lowercase English letters only.
* The test cases are generated such that an answer always **exists**. | There are limited outcomes given the current sum and the stones remaining. Can we greedily simulate starting with taking a stone with remainder 1 or 2 divided by 3? |
Time: O(n)O(n) Space: O(1)O(1) | find-substring-with-given-hash-value | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def subStrHash(self, s: str, power: int, modulo: int, k: int, hashValue: int) -> str:\n maxPower = pow(power, k, modulo)\n hashed = 0\n\n def val(c: chr) -> int:\n return ord(c) - ord(\'a\') + 1\n\n for i, c in reversed(list(enumerate(s))):\n hashed = (hashed * power + val(c)) % modulo\n if i + k < len(s):\n hashed = (hashed - val(s[i + k]) * maxPower) % modulo\n if hashed == hashValue:\n bestLeft = i\n\n return s[bestLeft:bestLeft + k]\n\n``` | 0 | The hash of a **0-indexed** string `s` of length `k`, given integers `p` and `m`, is computed using the following function:
* `hash(s, p, m) = (val(s[0]) * p0 + val(s[1]) * p1 + ... + val(s[k-1]) * pk-1) mod m`.
Where `val(s[i])` represents the index of `s[i]` in the alphabet from `val('a') = 1` to `val('z') = 26`.
You are given a string `s` and the integers `power`, `modulo`, `k`, and `hashValue.` Return `sub`, _the **first** **substring** of_ `s` _of length_ `k` _such that_ `hash(sub, power, modulo) == hashValue`.
The test cases will be generated such that an answer always **exists**.
A **substring** is a contiguous non-empty sequence of characters within a string.
**Example 1:**
**Input:** s = "leetcode ", power = 7, modulo = 20, k = 2, hashValue = 0
**Output:** "ee "
**Explanation:** The hash of "ee " can be computed to be hash( "ee ", 7, 20) = (5 \* 1 + 5 \* 7) mod 20 = 40 mod 20 = 0.
"ee " is the first substring of length 2 with hashValue 0. Hence, we return "ee ".
**Example 2:**
**Input:** s = "fbxzaad ", power = 31, modulo = 100, k = 3, hashValue = 32
**Output:** "fbx "
**Explanation:** The hash of "fbx " can be computed to be hash( "fbx ", 31, 100) = (6 \* 1 + 2 \* 31 + 24 \* 312) mod 100 = 23132 mod 100 = 32.
The hash of "bxz " can be computed to be hash( "bxz ", 31, 100) = (2 \* 1 + 24 \* 31 + 26 \* 312) mod 100 = 25732 mod 100 = 32.
"fbx " is the first substring of length 3 with hashValue 32. Hence, we return "fbx ".
Note that "bxz " also has a hash of 32 but it appears later than "fbx ".
**Constraints:**
* `1 <= k <= s.length <= 2 * 104`
* `1 <= power, modulo <= 109`
* `0 <= hashValue < modulo`
* `s` consists of lowercase English letters only.
* The test cases are generated such that an answer always **exists**. | There are limited outcomes given the current sum and the stones remaining. Can we greedily simulate starting with taking a stone with remainder 1 or 2 divided by 3? |
[Python3] union-find | groups-of-strings | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/badfbf601ede2f43ba191b567d6f5c7e0d046286) for solutions of weekly 278. \n\n```\nclass UnionFind: \n def __init__(self, n): \n self.parent = list(range(n))\n self.rank = [1] * n \n \n def find(self, p): \n if p != self.parent[p]: \n self.parent[p] = self.find(self.parent[p])\n return self.parent[p]\n \n def union(self, p, q): \n prt, qrt = self.find(p), self.find(q)\n if prt == qrt: return False \n if self.rank[prt] > self.rank[qrt]: prt, qrt = qrt, prt\n self.parent[prt] = self.parent[qrt]\n self.rank[qrt] += self.rank[prt]\n return True \n\n\nclass Solution:\n def groupStrings(self, words: List[str]) -> List[int]:\n n = len(words)\n uf = UnionFind(n)\n seen = {}\n for i, word in enumerate(words): \n m = reduce(or_, (1<<ord(ch)-97 for ch in word))\n if m in seen: uf.union(i, seen[m])\n for k in range(26): \n if m ^ 1<<k in seen: uf.union(i, seen[m ^ 1<<k])\n if m & 1<<k: \n mm = m ^ 1<<k ^ 1<<26\n if mm in seen: uf.union(i, seen[mm])\n seen[mm] = i\n seen[m] = i \n freq = Counter(uf.find(i) for i in range(n))\n return [len(freq), max(freq.values())]\n``` | 6 | You are given a **0-indexed** array of strings `words`. Each string consists of **lowercase English letters** only. No letter occurs more than once in any string of `words`.
Two strings `s1` and `s2` are said to be **connected** if the set of letters of `s2` can be obtained from the set of letters of `s1` by any **one** of the following operations:
* Adding exactly one letter to the set of the letters of `s1`.
* Deleting exactly one letter from the set of the letters of `s1`.
* Replacing exactly one letter from the set of the letters of `s1` with any letter, **including** itself.
The array `words` can be divided into one or more non-intersecting **groups**. A string belongs to a group if any **one** of the following is true:
* It is connected to **at least one** other string of the group.
* It is the **only** string present in the group.
Note that the strings in `words` should be grouped in such a manner that a string belonging to a group cannot be connected to a string present in any other group. It can be proved that such an arrangement is always unique.
Return _an array_ `ans` _of size_ `2` _where:_
* `ans[0]` _is the **maximum number** of groups_ `words` _can be divided into, and_
* `ans[1]` _is the **size of the largest** group_.
**Example 1:**
**Input:** words = \[ "a ", "b ", "ab ", "cde "\]
**Output:** \[2,3\]
**Explanation:**
- words\[0\] can be used to obtain words\[1\] (by replacing 'a' with 'b'), and words\[2\] (by adding 'b'). So words\[0\] is connected to words\[1\] and words\[2\].
- words\[1\] can be used to obtain words\[0\] (by replacing 'b' with 'a'), and words\[2\] (by adding 'a'). So words\[1\] is connected to words\[0\] and words\[2\].
- words\[2\] can be used to obtain words\[0\] (by deleting 'b'), and words\[1\] (by deleting 'a'). So words\[2\] is connected to words\[0\] and words\[1\].
- words\[3\] is not connected to any string in words.
Thus, words can be divided into 2 groups \[ "a ", "b ", "ab "\] and \[ "cde "\]. The size of the largest group is 3.
**Example 2:**
**Input:** words = \[ "a ", "ab ", "abc "\]
**Output:** \[1,3\]
**Explanation:**
- words\[0\] is connected to words\[1\].
- words\[1\] is connected to words\[0\] and words\[2\].
- words\[2\] is connected to words\[1\].
Since all strings are connected to each other, they should be grouped together.
Thus, the size of the largest group is 3.
**Constraints:**
* `1 <= words.length <= 2 * 104`
* `1 <= words[i].length <= 26`
* `words[i]` consists of lowercase English letters only.
* No letter occurs more than once in `words[i]`. | Use stack. For every character to be appended, decide how many character(s) from the stack needs to get popped based on the stack length and the count of the required character. Pop the extra characters out from the stack and return the characters in the stack (reversed). |
(Python3) Union find/ Bitmask - Beats 97.83% | groups-of-strings | 0 | 1 | # Intuition\nUsing an out of range mask (`SIMILAR_MASK`) to detect when we can replace one word to get another is copied from https://leetcode.com/problems/groups-of-strings/solutions/1732959/python3-union-find/\n\n# Complexity\n- Time complexity:\n $$O(n*s*alphabetSize*Ackermann(n))$$\nwhere `n = len(words)`, `s = max(len(s) for s in words)`, `alphabetSize = 26`\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nSIMILAR_MASK = 1 << 26\nCHAR_MASKS = {char: 1 << i for i, char in enumerate(string.ascii_lowercase)}\nget_bits = lambda word: functools.reduce(\n operator.or_, (CHAR_MASKS[char] for char in word)\n)\n\n\nclass Solution:\n def groupStrings(self, words: List[str]) -> List[int]:\n max_size = 1\n num_groups = len(words)\n disjoint_set = list(range(num_groups + 1))\n sizes = [1] * (num_groups + 1)\n\n def find(i):\n while (parent := disjoint_set[i]) != i:\n disjoint_set[i] = disjoint_set[parent]\n i = parent\n return parent\n\n def union(i, j):\n nonlocal max_size, num_groups\n if (i := find(i)) == (j := find(j)):\n return\n\n if sizes[i] < sizes[j]:\n i, j = j, i\n\n disjoint_set[j] = i\n sizes[i] += sizes[j]\n max_size = max(max_size, sizes[i])\n num_groups -= 1\n\n seen = {}\n for i, word in enumerate(words, start=1):\n bits = get_bits(word)\n if peer := seen.get(bits):\n union(peer, i)\n continue\n\n for mask in CHAR_MASKS.values():\n complement = bits ^ mask\n if peer := seen.get(complement):\n union(peer, i)\n\n if bits & mask:\n similar = complement ^ SIMILAR_MASK\n if peer := seen.get(similar):\n union(peer, i)\n else:\n seen[similar] = i\n\n seen[bits] = i\n\n return num_groups, max_size\n``` | 1 | You are given a **0-indexed** array of strings `words`. Each string consists of **lowercase English letters** only. No letter occurs more than once in any string of `words`.
Two strings `s1` and `s2` are said to be **connected** if the set of letters of `s2` can be obtained from the set of letters of `s1` by any **one** of the following operations:
* Adding exactly one letter to the set of the letters of `s1`.
* Deleting exactly one letter from the set of the letters of `s1`.
* Replacing exactly one letter from the set of the letters of `s1` with any letter, **including** itself.
The array `words` can be divided into one or more non-intersecting **groups**. A string belongs to a group if any **one** of the following is true:
* It is connected to **at least one** other string of the group.
* It is the **only** string present in the group.
Note that the strings in `words` should be grouped in such a manner that a string belonging to a group cannot be connected to a string present in any other group. It can be proved that such an arrangement is always unique.
Return _an array_ `ans` _of size_ `2` _where:_
* `ans[0]` _is the **maximum number** of groups_ `words` _can be divided into, and_
* `ans[1]` _is the **size of the largest** group_.
**Example 1:**
**Input:** words = \[ "a ", "b ", "ab ", "cde "\]
**Output:** \[2,3\]
**Explanation:**
- words\[0\] can be used to obtain words\[1\] (by replacing 'a' with 'b'), and words\[2\] (by adding 'b'). So words\[0\] is connected to words\[1\] and words\[2\].
- words\[1\] can be used to obtain words\[0\] (by replacing 'b' with 'a'), and words\[2\] (by adding 'a'). So words\[1\] is connected to words\[0\] and words\[2\].
- words\[2\] can be used to obtain words\[0\] (by deleting 'b'), and words\[1\] (by deleting 'a'). So words\[2\] is connected to words\[0\] and words\[1\].
- words\[3\] is not connected to any string in words.
Thus, words can be divided into 2 groups \[ "a ", "b ", "ab "\] and \[ "cde "\]. The size of the largest group is 3.
**Example 2:**
**Input:** words = \[ "a ", "ab ", "abc "\]
**Output:** \[1,3\]
**Explanation:**
- words\[0\] is connected to words\[1\].
- words\[1\] is connected to words\[0\] and words\[2\].
- words\[2\] is connected to words\[1\].
Since all strings are connected to each other, they should be grouped together.
Thus, the size of the largest group is 3.
**Constraints:**
* `1 <= words.length <= 2 * 104`
* `1 <= words[i].length <= 26`
* `words[i]` consists of lowercase English letters only.
* No letter occurs more than once in `words[i]`. | Use stack. For every character to be appended, decide how many character(s) from the stack needs to get popped based on the stack length and the count of the required character. Pop the extra characters out from the stack and return the characters in the stack (reversed). |
[Python] Bitmask & UnionFind | groups-of-strings | 0 | 1 | Caution: this code gets accepted, but sometimes gets TLE.\n\n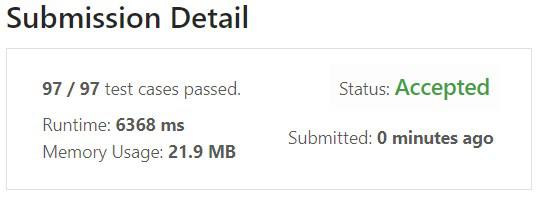\n\n\nFirst, we just need to think about the set of characters. This means that we can handle each word as a bit mask.\nFor example, \'a\' can be written as `10000........0` (following 25 0\'s). \'xyz\' can be written as `000.......0111`. And so on.\n\nFor each word, we can do three operations:\n1. **Addition**: change 0 to 1 at some place\n2. **Deletion**: change 1 to 0 at some place\n3. **Replace**: change 0 to 1 and 1 to 0 at some place\n\nWe can write three operations in bit manipulation.\nNote that there could be multiple same words, so I used `Counter` to consider the frequency.\n\n**Time complexity**: O(N) * O(13 * 13)\n**Space complexity**: O(N)\n\n```\nclass UnionFind:\n def __init__(self, num):\n self.parents = list(range(num))\n self.ranks = [0] * num\n \n def find_set(self, u):\n if u != self.parents[u]:\n self.parents[u] = self.find_set(self.parents[u])\n return self.parents[u]\n \n def union(self, set1, set2):\n if set1 == set2:\n return\n rank1, rank2 = self.ranks[set1], self.ranks[set2]\n if rank1 > rank2:\n self.parents[set2] = set1\n else:\n self.parents[set1] = set2\n if rank1 == rank2:\n self.ranks[set2] += 1\n\nfrom collections import defaultdict, Counter\nclass Solution:\n def groupStrings(self, words: List[str]) -> List[int]:\n def get_mask(word):\n res = 0\n for char in word:\n res |= 1 << (ord(\'z\') - ord(char))\n return res\n \n words = [get_mask(word) for word in words]\n counter = Counter(words)\n words_set = set(words)\n word_idx_dict = {word : idx for idx, word in enumerate(words_set)}\n N = len(words_set)\n uf = UnionFind(N)\n \n for idx, word in enumerate(words):\n word_idx = word_idx_dict[word]\n zero_idxes, one_idxes = [], []\n for i in range(26):\n if word & (1 << i):\n one_idxes.append(i)\n else:\n zero_idxes.append(i)\n \n #addition\n for i in zero_idxes:\n new_word = word | (1 << i)\n if new_word in word_idx_dict:\n new_word_idx = word_idx_dict[new_word]\n uf.union(uf.find_set(word_idx), uf.find_set(new_word_idx))\n \n #delete\n for i in one_idxes:\n new_word = word & ~(1 << i)\n if new_word in word_idx_dict:\n new_word_idx = word_idx_dict[new_word]\n uf.union(uf.find_set(word_idx), uf.find_set(new_word_idx))\n \n #replace\n for i in zero_idxes:\n for j in one_idxes:\n new_word = word & ~(1 << j) | (1 << i)\n if new_word in word_idx_dict:\n new_word_idx = word_idx_dict[new_word]\n uf.union(uf.find_set(word_idx), uf.find_set(new_word_idx))\n\t\t\t\t\t\tbreak\n \n union_dict = defaultdict(int)\n \n for i, word in enumerate(words_set):\n idx = uf.find_set(i)\n union_dict[idx] += counter[word]\n return [len(union_dict), max(union_dict.values())]\n``` | 3 | You are given a **0-indexed** array of strings `words`. Each string consists of **lowercase English letters** only. No letter occurs more than once in any string of `words`.
Two strings `s1` and `s2` are said to be **connected** if the set of letters of `s2` can be obtained from the set of letters of `s1` by any **one** of the following operations:
* Adding exactly one letter to the set of the letters of `s1`.
* Deleting exactly one letter from the set of the letters of `s1`.
* Replacing exactly one letter from the set of the letters of `s1` with any letter, **including** itself.
The array `words` can be divided into one or more non-intersecting **groups**. A string belongs to a group if any **one** of the following is true:
* It is connected to **at least one** other string of the group.
* It is the **only** string present in the group.
Note that the strings in `words` should be grouped in such a manner that a string belonging to a group cannot be connected to a string present in any other group. It can be proved that such an arrangement is always unique.
Return _an array_ `ans` _of size_ `2` _where:_
* `ans[0]` _is the **maximum number** of groups_ `words` _can be divided into, and_
* `ans[1]` _is the **size of the largest** group_.
**Example 1:**
**Input:** words = \[ "a ", "b ", "ab ", "cde "\]
**Output:** \[2,3\]
**Explanation:**
- words\[0\] can be used to obtain words\[1\] (by replacing 'a' with 'b'), and words\[2\] (by adding 'b'). So words\[0\] is connected to words\[1\] and words\[2\].
- words\[1\] can be used to obtain words\[0\] (by replacing 'b' with 'a'), and words\[2\] (by adding 'a'). So words\[1\] is connected to words\[0\] and words\[2\].
- words\[2\] can be used to obtain words\[0\] (by deleting 'b'), and words\[1\] (by deleting 'a'). So words\[2\] is connected to words\[0\] and words\[1\].
- words\[3\] is not connected to any string in words.
Thus, words can be divided into 2 groups \[ "a ", "b ", "ab "\] and \[ "cde "\]. The size of the largest group is 3.
**Example 2:**
**Input:** words = \[ "a ", "ab ", "abc "\]
**Output:** \[1,3\]
**Explanation:**
- words\[0\] is connected to words\[1\].
- words\[1\] is connected to words\[0\] and words\[2\].
- words\[2\] is connected to words\[1\].
Since all strings are connected to each other, they should be grouped together.
Thus, the size of the largest group is 3.
**Constraints:**
* `1 <= words.length <= 2 * 104`
* `1 <= words[i].length <= 26`
* `words[i]` consists of lowercase English letters only.
* No letter occurs more than once in `words[i]`. | Use stack. For every character to be appended, decide how many character(s) from the stack needs to get popped based on the stack length and the count of the required character. Pop the extra characters out from the stack and return the characters in the stack (reversed). |
Python3 with explanation | groups-of-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom collections import defaultdict\nfrom typing import List\n\nclass Solution:\n def __init__(self):\n self.parent = {}\n self.rank = defaultdict(int)\n self.count = defaultdict(int)\n\n def find(self, x):\n """Find the root of x in the union-find structure."""\n root = x\n while self.parent[root] != root:\n root = self.parent[root]\n while self.parent[x] != x: # path compression\n x, self.parent[x] = self.parent[x], root\n return root\n\n def union(self, x, y):\n """Unite the sets that x and y belong to."""\n xr = self.find(x)\n yr = self.find(y)\n if xr != yr:\n if self.rank[xr] < self.rank[yr]:\n xr, yr = yr, xr # Ensure xr has a greater or equal rank\n self.parent[yr] = xr\n self.count[xr] += self.count[yr]\n if self.rank[xr] == self.rank[yr]:\n self.rank[xr] += 1\n\n def groupStrings(self, words: List[str]) -> List[int]:\n """Group strings that can be transformed into each other by swapping 0 or 1 characters."""\n for word in words:\n bit = sum(1 << (ord(c) - ord(\'a\')) for c in word) # convert word to bit representation\n if bit not in self.parent:\n self.parent[bit] = bit\n self.count[bit] += 1\n\n for bit in list(self.parent.keys()):\n # Generate all possible transformations\n for i in range(26):\n mask = 1 << i\n # Adding a letter\n if not bit & mask:\n nbit = bit | mask\n if nbit in self.parent:\n self.union(bit, nbit)\n # Removing a letter or replacing a letter\n elif bit & mask:\n # Removing a letter\n nbit = bit & ~mask\n if nbit in self.parent:\n self.union(bit, nbit)\n # Replacing a letter\n for j in range(26):\n if i != j:\n n2bit = (bit & ~mask) | (1 << j)\n if n2bit in self.parent:\n self.union(bit, n2bit)\n\n roots = {self.find(key) for key in self.parent.keys()}\n return [len(roots), max(self.count[root] for root in roots)]\n\n``` | 0 | You are given a **0-indexed** array of strings `words`. Each string consists of **lowercase English letters** only. No letter occurs more than once in any string of `words`.
Two strings `s1` and `s2` are said to be **connected** if the set of letters of `s2` can be obtained from the set of letters of `s1` by any **one** of the following operations:
* Adding exactly one letter to the set of the letters of `s1`.
* Deleting exactly one letter from the set of the letters of `s1`.
* Replacing exactly one letter from the set of the letters of `s1` with any letter, **including** itself.
The array `words` can be divided into one or more non-intersecting **groups**. A string belongs to a group if any **one** of the following is true:
* It is connected to **at least one** other string of the group.
* It is the **only** string present in the group.
Note that the strings in `words` should be grouped in such a manner that a string belonging to a group cannot be connected to a string present in any other group. It can be proved that such an arrangement is always unique.
Return _an array_ `ans` _of size_ `2` _where:_
* `ans[0]` _is the **maximum number** of groups_ `words` _can be divided into, and_
* `ans[1]` _is the **size of the largest** group_.
**Example 1:**
**Input:** words = \[ "a ", "b ", "ab ", "cde "\]
**Output:** \[2,3\]
**Explanation:**
- words\[0\] can be used to obtain words\[1\] (by replacing 'a' with 'b'), and words\[2\] (by adding 'b'). So words\[0\] is connected to words\[1\] and words\[2\].
- words\[1\] can be used to obtain words\[0\] (by replacing 'b' with 'a'), and words\[2\] (by adding 'a'). So words\[1\] is connected to words\[0\] and words\[2\].
- words\[2\] can be used to obtain words\[0\] (by deleting 'b'), and words\[1\] (by deleting 'a'). So words\[2\] is connected to words\[0\] and words\[1\].
- words\[3\] is not connected to any string in words.
Thus, words can be divided into 2 groups \[ "a ", "b ", "ab "\] and \[ "cde "\]. The size of the largest group is 3.
**Example 2:**
**Input:** words = \[ "a ", "ab ", "abc "\]
**Output:** \[1,3\]
**Explanation:**
- words\[0\] is connected to words\[1\].
- words\[1\] is connected to words\[0\] and words\[2\].
- words\[2\] is connected to words\[1\].
Since all strings are connected to each other, they should be grouped together.
Thus, the size of the largest group is 3.
**Constraints:**
* `1 <= words.length <= 2 * 104`
* `1 <= words[i].length <= 26`
* `words[i]` consists of lowercase English letters only.
* No letter occurs more than once in `words[i]`. | Use stack. For every character to be appended, decide how many character(s) from the stack needs to get popped based on the stack length and the count of the required character. Pop the extra characters out from the stack and return the characters in the stack (reversed). |
[Python] AC Union Find O(N*26) | Pre-Processing | groups-of-strings | 0 | 1 | # Code\n```\nclass Solution:\n def groupStrings(self, words: List[str]) -> List[int]:\n def gm(w):\n mk =0\n for x in w:\n mk |= 1<<(ord(x)-ord(\'a\'))\n return mk\n masks = [gm(w) for w in words]\n d = dict([(w,i) for i,w in enumerate(masks)])\n r_d = dict()\n for k,x in enumerate(masks):\n for i in range(26):\n if((x&(1<<i))==0): continue\n r_d[x^(1<<i)] = k\n ds = DSU(len(words))\n for u,m in enumerate(masks):\n for i in range(26): # add remove\n if((m^(1<<i)) in d): \n ds.union(u,d[(m^(1<<i))])\n ds.union(u,d[m])\n for i in range(26): # replace \n if((m&(1<<i))==0): continue\n nm = (m^(1<<i))\n if(nm in r_d): ds.union(u,r_d[nm])\n return [ds.cnt,ds.mx]\n\n\n\n\n \n\n\nclass DSU:\n def __init__(self, N):\n self.par = list(range(N))\n self.sz = [1] * N\n self.mx = 1\n self.cnt = N\n\n def find(self, x):\n if self.par[x] != x:\n self.par[x] = self.find(self.par[x])\n return self.par[x]\n\n def union(self, x, y):\n xr, yr = self.find(x), self.find(y)\n if xr == yr:\n return False\n if self.sz[xr] < self.sz[yr]:\n xr, yr = yr, xr\n self.par[yr] = xr\n self.sz[xr] += self.sz[yr]\n self.mx = max(self.mx,self.sz[xr])\n self.cnt -=1\n return True\n``` | 0 | You are given a **0-indexed** array of strings `words`. Each string consists of **lowercase English letters** only. No letter occurs more than once in any string of `words`.
Two strings `s1` and `s2` are said to be **connected** if the set of letters of `s2` can be obtained from the set of letters of `s1` by any **one** of the following operations:
* Adding exactly one letter to the set of the letters of `s1`.
* Deleting exactly one letter from the set of the letters of `s1`.
* Replacing exactly one letter from the set of the letters of `s1` with any letter, **including** itself.
The array `words` can be divided into one or more non-intersecting **groups**. A string belongs to a group if any **one** of the following is true:
* It is connected to **at least one** other string of the group.
* It is the **only** string present in the group.
Note that the strings in `words` should be grouped in such a manner that a string belonging to a group cannot be connected to a string present in any other group. It can be proved that such an arrangement is always unique.
Return _an array_ `ans` _of size_ `2` _where:_
* `ans[0]` _is the **maximum number** of groups_ `words` _can be divided into, and_
* `ans[1]` _is the **size of the largest** group_.
**Example 1:**
**Input:** words = \[ "a ", "b ", "ab ", "cde "\]
**Output:** \[2,3\]
**Explanation:**
- words\[0\] can be used to obtain words\[1\] (by replacing 'a' with 'b'), and words\[2\] (by adding 'b'). So words\[0\] is connected to words\[1\] and words\[2\].
- words\[1\] can be used to obtain words\[0\] (by replacing 'b' with 'a'), and words\[2\] (by adding 'a'). So words\[1\] is connected to words\[0\] and words\[2\].
- words\[2\] can be used to obtain words\[0\] (by deleting 'b'), and words\[1\] (by deleting 'a'). So words\[2\] is connected to words\[0\] and words\[1\].
- words\[3\] is not connected to any string in words.
Thus, words can be divided into 2 groups \[ "a ", "b ", "ab "\] and \[ "cde "\]. The size of the largest group is 3.
**Example 2:**
**Input:** words = \[ "a ", "ab ", "abc "\]
**Output:** \[1,3\]
**Explanation:**
- words\[0\] is connected to words\[1\].
- words\[1\] is connected to words\[0\] and words\[2\].
- words\[2\] is connected to words\[1\].
Since all strings are connected to each other, they should be grouped together.
Thus, the size of the largest group is 3.
**Constraints:**
* `1 <= words.length <= 2 * 104`
* `1 <= words[i].length <= 26`
* `words[i]` consists of lowercase English letters only.
* No letter occurs more than once in `words[i]`. | Use stack. For every character to be appended, decide how many character(s) from the stack needs to get popped based on the stack length and the count of the required character. Pop the extra characters out from the stack and return the characters in the stack (reversed). |
Python (Simple DFS + Bitmask) | groups-of-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def groupStrings(self, words):\n def convert(w): return sum(1<<(ord(c)-ord("a")) for c in w)\n\n ans = [convert(i) for i in words]\n\n dict1 = collections.Counter(ans)\n\n def dfs(n):\n if n in visited: return 0\n total = dict1[n]\n visited.add(n)\n total += _add(n)\n total += _remove(n)\n total += _replace(n)\n return total\n\n def _add(n):\n total = 0\n\n for i in range(26):\n if (1<<i)&n == 0:\n m = n + (1<<i)\n if m in dict1:\n total += dfs(m)\n\n return total\n\n \n def _remove(n):\n total = 0\n\n for i in range(26):\n if (1<<i)&n != 0:\n m = n - (1<<i)\n if m in dict1:\n total += dfs(m)\n\n return total\n\n \n def _replace(n):\n total = 0\n\n pos = [i for i in range(26) if (1<<i)&n != 0]\n\n for p in pos:\n m = n - (1<<p)\n for j in range(26):\n if j not in pos and m + (1<<j) in dict1:\n total += dfs(m + (1<<j))\n\n return total \n\n number_of_groups, largest_size, visited = 0, 0, set()\n\n for key,val in dict1.items():\n x = dfs(key)\n if x > 0:\n largest_size = max(largest_size,x)\n number_of_groups += 1\n\n return [number_of_groups,largest_size]\n\n\n\n\n\n\n\n\n\n\n \n\n \n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n \n\n\n\n\n\n\n\n\n\n\n \n\n\n \n\n\n``` | 0 | You are given a **0-indexed** array of strings `words`. Each string consists of **lowercase English letters** only. No letter occurs more than once in any string of `words`.
Two strings `s1` and `s2` are said to be **connected** if the set of letters of `s2` can be obtained from the set of letters of `s1` by any **one** of the following operations:
* Adding exactly one letter to the set of the letters of `s1`.
* Deleting exactly one letter from the set of the letters of `s1`.
* Replacing exactly one letter from the set of the letters of `s1` with any letter, **including** itself.
The array `words` can be divided into one or more non-intersecting **groups**. A string belongs to a group if any **one** of the following is true:
* It is connected to **at least one** other string of the group.
* It is the **only** string present in the group.
Note that the strings in `words` should be grouped in such a manner that a string belonging to a group cannot be connected to a string present in any other group. It can be proved that such an arrangement is always unique.
Return _an array_ `ans` _of size_ `2` _where:_
* `ans[0]` _is the **maximum number** of groups_ `words` _can be divided into, and_
* `ans[1]` _is the **size of the largest** group_.
**Example 1:**
**Input:** words = \[ "a ", "b ", "ab ", "cde "\]
**Output:** \[2,3\]
**Explanation:**
- words\[0\] can be used to obtain words\[1\] (by replacing 'a' with 'b'), and words\[2\] (by adding 'b'). So words\[0\] is connected to words\[1\] and words\[2\].
- words\[1\] can be used to obtain words\[0\] (by replacing 'b' with 'a'), and words\[2\] (by adding 'a'). So words\[1\] is connected to words\[0\] and words\[2\].
- words\[2\] can be used to obtain words\[0\] (by deleting 'b'), and words\[1\] (by deleting 'a'). So words\[2\] is connected to words\[0\] and words\[1\].
- words\[3\] is not connected to any string in words.
Thus, words can be divided into 2 groups \[ "a ", "b ", "ab "\] and \[ "cde "\]. The size of the largest group is 3.
**Example 2:**
**Input:** words = \[ "a ", "ab ", "abc "\]
**Output:** \[1,3\]
**Explanation:**
- words\[0\] is connected to words\[1\].
- words\[1\] is connected to words\[0\] and words\[2\].
- words\[2\] is connected to words\[1\].
Since all strings are connected to each other, they should be grouped together.
Thus, the size of the largest group is 3.
**Constraints:**
* `1 <= words.length <= 2 * 104`
* `1 <= words[i].length <= 26`
* `words[i]` consists of lowercase English letters only.
* No letter occurs more than once in `words[i]`. | Use stack. For every character to be appended, decide how many character(s) from the stack needs to get popped based on the stack length and the count of the required character. Pop the extra characters out from the stack and return the characters in the stack (reversed). |
Python - simple & fast with explanation - no permutation | minimum-sum-of-four-digit-number-after-splitting-digits | 0 | 1 | Please upvote if you like my solution. Let me know in the comments if you have any suggestions to increase performance or readability. \n\n**Notice** that:\n1. We do not need to generate all possible combinations of spliting the input into two numbers. In the ideal solution, num1 and num2 will always be of equal length.\n2. We will step by step build num1 and num2 as we go through the algorithm. It is not necessary to generate all combinations of num1 and num2 (with both numbers having equal length).\n3. We do not need to save num1 and num2 in a variable. We can keep adding to the total sum as we go through the algorithm. \n4. The algorithm below works for any input length. If you want you can simplify it, since you know the input length is 4. \n\n**My algorithm** goes as follows:\n1. convert the input into a list of digits\n2. sort the list of digits in descending order\n3. iterate through the list of digits\n3.1 with each iteration, multiply the current digit with 10*current position in num1/num2. \n3.2 add the current digit to the result\n3.3 if you already added an even number of digits to the total of results, then increase the current position by one\n4. return result\n\n```\nclass Solution:\n def minimumSum(self, num: int) -> int:\n num = sorted(str(num),reverse=True)\n n = len(num) \n res = 0\n even_iteration = False\n position = 0\n for i in range(n):\n res += int(num[i])*(10**position)\n if even_iteration:\n position += 1\n even_iteration = False\n else:\n even_iteration = True\n return res\n```\n\nif you know that the input is going to be exaclty 4 digits, you can simplify it:\n\n```\nclass Solution:\n def minimumSum(self, num: int) -> int:\n num = sorted(str(num),reverse=True)\n return int(num[0]) + int(num[1]) + int(num[2])*10 + int(num[3])*10\n```\n | 61 | You are given a **positive** integer `num` consisting of exactly four digits. Split `num` into two new integers `new1` and `new2` by using the **digits** found in `num`. **Leading zeros** are allowed in `new1` and `new2`, and **all** the digits found in `num` must be used.
* For example, given `num = 2932`, you have the following digits: two `2`'s, one `9` and one `3`. Some of the possible pairs `[new1, new2]` are `[22, 93]`, `[23, 92]`, `[223, 9]` and `[2, 329]`.
Return _the **minimum** possible sum of_ `new1` _and_ `new2`.
**Example 1:**
**Input:** num = 2932
**Output:** 52
**Explanation:** Some possible pairs \[new1, new2\] are \[29, 23\], \[223, 9\], etc.
The minimum sum can be obtained by the pair \[29, 23\]: 29 + 23 = 52.
**Example 2:**
**Input:** num = 4009
**Output:** 13
**Explanation:** Some possible pairs \[new1, new2\] are \[0, 49\], \[490, 0\], etc.
The minimum sum can be obtained by the pair \[4, 9\]: 4 + 9 = 13.
**Constraints:**
* `1000 <= num <= 9999` | Is it possible to make two integers a and b equal if they have different remainders dividing by x? If it is possible, which number should you select to minimize the number of operations? What if the elements are sorted? |
🔥 [Python3] 2 line, simple sort and make pairs | minimum-sum-of-four-digit-number-after-splitting-digits | 0 | 1 | ```\nclass Solution:\n def minimumSum(self, num: int) -> int:\n d = sorted(str(num))\n return int(d[0] + d[2]) + int(d[1] + d[3])\n\n``` | 14 | You are given a **positive** integer `num` consisting of exactly four digits. Split `num` into two new integers `new1` and `new2` by using the **digits** found in `num`. **Leading zeros** are allowed in `new1` and `new2`, and **all** the digits found in `num` must be used.
* For example, given `num = 2932`, you have the following digits: two `2`'s, one `9` and one `3`. Some of the possible pairs `[new1, new2]` are `[22, 93]`, `[23, 92]`, `[223, 9]` and `[2, 329]`.
Return _the **minimum** possible sum of_ `new1` _and_ `new2`.
**Example 1:**
**Input:** num = 2932
**Output:** 52
**Explanation:** Some possible pairs \[new1, new2\] are \[29, 23\], \[223, 9\], etc.
The minimum sum can be obtained by the pair \[29, 23\]: 29 + 23 = 52.
**Example 2:**
**Input:** num = 4009
**Output:** 13
**Explanation:** Some possible pairs \[new1, new2\] are \[0, 49\], \[490, 0\], etc.
The minimum sum can be obtained by the pair \[4, 9\]: 4 + 9 = 13.
**Constraints:**
* `1000 <= num <= 9999` | Is it possible to make two integers a and b equal if they have different remainders dividing by x? If it is possible, which number should you select to minimize the number of operations? What if the elements are sorted? |
Easy Python3 Solution | minimum-sum-of-four-digit-number-after-splitting-digits | 0 | 1 | \n# Approach\n<!-- Describe your approach to solving the problem. -->\nConvert the integer into a sorted string. After sorting,ascending order is obtained.\nreturn sum of the lowest sum pairs after converting pairs backs to integers.\n# Code\n```\nclass Solution:\n def minimumSum(self, num: int) -> int:\n l=sorted(str(num))\n return int(l[0] + l[3]) + int(l[1] + l[2])\n \n\n\n``` | 1 | You are given a **positive** integer `num` consisting of exactly four digits. Split `num` into two new integers `new1` and `new2` by using the **digits** found in `num`. **Leading zeros** are allowed in `new1` and `new2`, and **all** the digits found in `num` must be used.
* For example, given `num = 2932`, you have the following digits: two `2`'s, one `9` and one `3`. Some of the possible pairs `[new1, new2]` are `[22, 93]`, `[23, 92]`, `[223, 9]` and `[2, 329]`.
Return _the **minimum** possible sum of_ `new1` _and_ `new2`.
**Example 1:**
**Input:** num = 2932
**Output:** 52
**Explanation:** Some possible pairs \[new1, new2\] are \[29, 23\], \[223, 9\], etc.
The minimum sum can be obtained by the pair \[29, 23\]: 29 + 23 = 52.
**Example 2:**
**Input:** num = 4009
**Output:** 13
**Explanation:** Some possible pairs \[new1, new2\] are \[0, 49\], \[490, 0\], etc.
The minimum sum can be obtained by the pair \[4, 9\]: 4 + 9 = 13.
**Constraints:**
* `1000 <= num <= 9999` | Is it possible to make two integers a and b equal if they have different remainders dividing by x? If it is possible, which number should you select to minimize the number of operations? What if the elements are sorted? |
Easy Python Solution - minSum | minimum-sum-of-four-digit-number-after-splitting-digits | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def minimumSum(self, num: int) -> int:\n l=[]\n while num != 0:\n l.append(num % 10)\n num = num // 10\n \n l.sort()\n res = (l[1] * 10 + l[2]) + (l[0] * 10 + l[3])\n return res\n``` | 5 | You are given a **positive** integer `num` consisting of exactly four digits. Split `num` into two new integers `new1` and `new2` by using the **digits** found in `num`. **Leading zeros** are allowed in `new1` and `new2`, and **all** the digits found in `num` must be used.
* For example, given `num = 2932`, you have the following digits: two `2`'s, one `9` and one `3`. Some of the possible pairs `[new1, new2]` are `[22, 93]`, `[23, 92]`, `[223, 9]` and `[2, 329]`.
Return _the **minimum** possible sum of_ `new1` _and_ `new2`.
**Example 1:**
**Input:** num = 2932
**Output:** 52
**Explanation:** Some possible pairs \[new1, new2\] are \[29, 23\], \[223, 9\], etc.
The minimum sum can be obtained by the pair \[29, 23\]: 29 + 23 = 52.
**Example 2:**
**Input:** num = 4009
**Output:** 13
**Explanation:** Some possible pairs \[new1, new2\] are \[0, 49\], \[490, 0\], etc.
The minimum sum can be obtained by the pair \[4, 9\]: 4 + 9 = 13.
**Constraints:**
* `1000 <= num <= 9999` | Is it possible to make two integers a and b equal if they have different remainders dividing by x? If it is possible, which number should you select to minimize the number of operations? What if the elements are sorted? |
Python || Easy || 3Lines || Sorting || | minimum-sum-of-four-digit-number-after-splitting-digits | 0 | 1 | ```\nclass Solution:\n def minimumSum(self, num: int) -> int:\n n=[num//1000,(num//100)%10,(num//10)%10,num%10] #This line will convert the four digit no. into array \n n.sort() #It will sort the digits in ascending order\n return (n[0]*10+n[3])+(n[1]*10+n[2]) # Combination of first and last and the remaining two digits will give us the minimum value\n```\n\n**Upvote if you like the solution or ask if there is any query** | 4 | You are given a **positive** integer `num` consisting of exactly four digits. Split `num` into two new integers `new1` and `new2` by using the **digits** found in `num`. **Leading zeros** are allowed in `new1` and `new2`, and **all** the digits found in `num` must be used.
* For example, given `num = 2932`, you have the following digits: two `2`'s, one `9` and one `3`. Some of the possible pairs `[new1, new2]` are `[22, 93]`, `[23, 92]`, `[223, 9]` and `[2, 329]`.
Return _the **minimum** possible sum of_ `new1` _and_ `new2`.
**Example 1:**
**Input:** num = 2932
**Output:** 52
**Explanation:** Some possible pairs \[new1, new2\] are \[29, 23\], \[223, 9\], etc.
The minimum sum can be obtained by the pair \[29, 23\]: 29 + 23 = 52.
**Example 2:**
**Input:** num = 4009
**Output:** 13
**Explanation:** Some possible pairs \[new1, new2\] are \[0, 49\], \[490, 0\], etc.
The minimum sum can be obtained by the pair \[4, 9\]: 4 + 9 = 13.
**Constraints:**
* `1000 <= num <= 9999` | Is it possible to make two integers a and b equal if they have different remainders dividing by x? If it is possible, which number should you select to minimize the number of operations? What if the elements are sorted? |
Magic Logic Marvellous Python3 | minimum-sum-of-four-digit-number-after-splitting-digits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimumSum(self, num: int) -> int:\n string=str(num)\n s=sorted(string)\n m=s[0]+s[2]\n n=s[1]+s[3]\n return (int(m)+int(n))\n#please upvote me it would encourage me alot\n \n``` | 8 | You are given a **positive** integer `num` consisting of exactly four digits. Split `num` into two new integers `new1` and `new2` by using the **digits** found in `num`. **Leading zeros** are allowed in `new1` and `new2`, and **all** the digits found in `num` must be used.
* For example, given `num = 2932`, you have the following digits: two `2`'s, one `9` and one `3`. Some of the possible pairs `[new1, new2]` are `[22, 93]`, `[23, 92]`, `[223, 9]` and `[2, 329]`.
Return _the **minimum** possible sum of_ `new1` _and_ `new2`.
**Example 1:**
**Input:** num = 2932
**Output:** 52
**Explanation:** Some possible pairs \[new1, new2\] are \[29, 23\], \[223, 9\], etc.
The minimum sum can be obtained by the pair \[29, 23\]: 29 + 23 = 52.
**Example 2:**
**Input:** num = 4009
**Output:** 13
**Explanation:** Some possible pairs \[new1, new2\] are \[0, 49\], \[490, 0\], etc.
The minimum sum can be obtained by the pair \[4, 9\]: 4 + 9 = 13.
**Constraints:**
* `1000 <= num <= 9999` | Is it possible to make two integers a and b equal if they have different remainders dividing by x? If it is possible, which number should you select to minimize the number of operations? What if the elements are sorted? |
Python || 2 Approach (Math, String) || 99.70%beats | minimum-sum-of-four-digit-number-after-splitting-digits | 0 | 1 | \n# Code\n> # Full Math Approach\n```\nclass Solution:\n def minimumSum(self, num: int) -> int:\n n = []\n while num > 0:\n n.append(num%10)\n num = num // 10\n \n n.sort()\n\n n1 = n[0] * 10 + n[-1]\n n2 = n[1] * 10 + n[-2]\n return n1+ n2\n\n\n\n```\n\n> # String Approach\n```\nclass Solution:\n def minimumSum(self, num: int) -> int:\n num = \'\'.join(sorted(str(num)))\n return int(num[0]+num[3]) + int(num[1]+num[2])\n\n\n#\n``` | 3 | You are given a **positive** integer `num` consisting of exactly four digits. Split `num` into two new integers `new1` and `new2` by using the **digits** found in `num`. **Leading zeros** are allowed in `new1` and `new2`, and **all** the digits found in `num` must be used.
* For example, given `num = 2932`, you have the following digits: two `2`'s, one `9` and one `3`. Some of the possible pairs `[new1, new2]` are `[22, 93]`, `[23, 92]`, `[223, 9]` and `[2, 329]`.
Return _the **minimum** possible sum of_ `new1` _and_ `new2`.
**Example 1:**
**Input:** num = 2932
**Output:** 52
**Explanation:** Some possible pairs \[new1, new2\] are \[29, 23\], \[223, 9\], etc.
The minimum sum can be obtained by the pair \[29, 23\]: 29 + 23 = 52.
**Example 2:**
**Input:** num = 4009
**Output:** 13
**Explanation:** Some possible pairs \[new1, new2\] are \[0, 49\], \[490, 0\], etc.
The minimum sum can be obtained by the pair \[4, 9\]: 4 + 9 = 13.
**Constraints:**
* `1000 <= num <= 9999` | Is it possible to make two integers a and b equal if they have different remainders dividing by x? If it is possible, which number should you select to minimize the number of operations? What if the elements are sorted? |
Convert into the list and then store the value.. | minimum-sum-of-four-digit-number-after-splitting-digits | 0 | 1 | # Code\n```\nclass Solution:\n def minimumSum(self, num: int) -> int:\n nums=list(str(num))\n nums.sort()\n return int(nums[0]+nums[-1])+int(nums[1]+nums[2])\n \n``` | 5 | You are given a **positive** integer `num` consisting of exactly four digits. Split `num` into two new integers `new1` and `new2` by using the **digits** found in `num`. **Leading zeros** are allowed in `new1` and `new2`, and **all** the digits found in `num` must be used.
* For example, given `num = 2932`, you have the following digits: two `2`'s, one `9` and one `3`. Some of the possible pairs `[new1, new2]` are `[22, 93]`, `[23, 92]`, `[223, 9]` and `[2, 329]`.
Return _the **minimum** possible sum of_ `new1` _and_ `new2`.
**Example 1:**
**Input:** num = 2932
**Output:** 52
**Explanation:** Some possible pairs \[new1, new2\] are \[29, 23\], \[223, 9\], etc.
The minimum sum can be obtained by the pair \[29, 23\]: 29 + 23 = 52.
**Example 2:**
**Input:** num = 4009
**Output:** 13
**Explanation:** Some possible pairs \[new1, new2\] are \[0, 49\], \[490, 0\], etc.
The minimum sum can be obtained by the pair \[4, 9\]: 4 + 9 = 13.
**Constraints:**
* `1000 <= num <= 9999` | Is it possible to make two integers a and b equal if they have different remainders dividing by x? If it is possible, which number should you select to minimize the number of operations? What if the elements are sorted? |
Simple Solution || Minimum sum of four digit number after splitting digits | minimum-sum-of-four-digit-number-after-splitting-digits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimumSum(self, num: int) -> int:\n l=[]\n while(num!=0):\n l.append(num%10)\n num//=10\n l.sort()\n result=(l[1]*10+l[2])+(l[0]*10+l[3])\n return result\n \n``` | 3 | You are given a **positive** integer `num` consisting of exactly four digits. Split `num` into two new integers `new1` and `new2` by using the **digits** found in `num`. **Leading zeros** are allowed in `new1` and `new2`, and **all** the digits found in `num` must be used.
* For example, given `num = 2932`, you have the following digits: two `2`'s, one `9` and one `3`. Some of the possible pairs `[new1, new2]` are `[22, 93]`, `[23, 92]`, `[223, 9]` and `[2, 329]`.
Return _the **minimum** possible sum of_ `new1` _and_ `new2`.
**Example 1:**
**Input:** num = 2932
**Output:** 52
**Explanation:** Some possible pairs \[new1, new2\] are \[29, 23\], \[223, 9\], etc.
The minimum sum can be obtained by the pair \[29, 23\]: 29 + 23 = 52.
**Example 2:**
**Input:** num = 4009
**Output:** 13
**Explanation:** Some possible pairs \[new1, new2\] are \[0, 49\], \[490, 0\], etc.
The minimum sum can be obtained by the pair \[4, 9\]: 4 + 9 = 13.
**Constraints:**
* `1000 <= num <= 9999` | Is it possible to make two integers a and b equal if they have different remainders dividing by x? If it is possible, which number should you select to minimize the number of operations? What if the elements are sorted? |
Simple and Logic code in python | minimum-sum-of-four-digit-number-after-splitting-digits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimumSum(self, num: int) -> int:\n s=list(str(num))\n s.sort()\n ans=int(s[0]+s[2])+int(s[1]+s[3])\n return ans\n \n\n \n\n``` | 1 | You are given a **positive** integer `num` consisting of exactly four digits. Split `num` into two new integers `new1` and `new2` by using the **digits** found in `num`. **Leading zeros** are allowed in `new1` and `new2`, and **all** the digits found in `num` must be used.
* For example, given `num = 2932`, you have the following digits: two `2`'s, one `9` and one `3`. Some of the possible pairs `[new1, new2]` are `[22, 93]`, `[23, 92]`, `[223, 9]` and `[2, 329]`.
Return _the **minimum** possible sum of_ `new1` _and_ `new2`.
**Example 1:**
**Input:** num = 2932
**Output:** 52
**Explanation:** Some possible pairs \[new1, new2\] are \[29, 23\], \[223, 9\], etc.
The minimum sum can be obtained by the pair \[29, 23\]: 29 + 23 = 52.
**Example 2:**
**Input:** num = 4009
**Output:** 13
**Explanation:** Some possible pairs \[new1, new2\] are \[0, 49\], \[490, 0\], etc.
The minimum sum can be obtained by the pair \[4, 9\]: 4 + 9 = 13.
**Constraints:**
* `1000 <= num <= 9999` | Is it possible to make two integers a and b equal if they have different remainders dividing by x? If it is possible, which number should you select to minimize the number of operations? What if the elements are sorted? |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.