title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
Z algorithm | sum-of-scores-of-built-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def sumScores(self, s: str) -> int:\n z_arr = [0 for i in range(len(s))]\n left, right = 0,0\n n = len(s)\n for i in range(1,len(s)):\n if i > right:\n left, right = i, i\n while right < n and s[right-left]==s[right]:\n right += 1\n z_arr[i] = right-left\n right -= 1\n else:\n k = i - left\n if z_arr[k] < (right-i+1):\n z_arr[i] = z_arr[k]\n else:\n left = i \n while right < n and s[right-left] == s[right]:\n right += 1\n z_arr[i] = right - left\n right -= 1\n print(z_arr)\n return sum(z_arr) + len(s)\n\n\n``` | 0 | You are **building** a string `s` of length `n` **one** character at a time, **prepending** each new character to the **front** of the string. The strings are labeled from `1` to `n`, where the string with length `i` is labeled `si`.
* For example, for `s = "abaca "`, `s1 == "a "`, `s2 == "ca "`, `s3 == "aca "`, etc.
The **score** of `si` is the length of the **longest common prefix** between `si` and `sn` (Note that `s == sn`).
Given the final string `s`, return _the **sum** of the **score** of every_ `si`.
**Example 1:**
**Input:** s = "babab "
**Output:** 9
**Explanation:**
For s1 == "b ", the longest common prefix is "b " which has a score of 1.
For s2 == "ab ", there is no common prefix so the score is 0.
For s3 == "bab ", the longest common prefix is "bab " which has a score of 3.
For s4 == "abab ", there is no common prefix so the score is 0.
For s5 == "babab ", the longest common prefix is "babab " which has a score of 5.
The sum of the scores is 1 + 0 + 3 + 0 + 5 = 9, so we return 9.
**Example 2:**
**Input:** s = "azbazbzaz "
**Output:** 14
**Explanation:**
For s2 == "az ", the longest common prefix is "az " which has a score of 2.
For s6 == "azbzaz ", the longest common prefix is "azb " which has a score of 3.
For s9 == "azbazbzaz ", the longest common prefix is "azbazbzaz " which has a score of 9.
For all other si, the score is 0.
The sum of the scores is 2 + 3 + 9 = 14, so we return 14.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of lowercase English letters. | null |
prefix arr and binary search with no additional spaces | sum-of-scores-of-built-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def sumScores(self, A: str) -> int:\n m = len(A)\n\n def bisect_strs(t):\n lo, hi = 0 , len(t)\n if A[0]!= t[0]:\n return 0\n if t == A[:hi]:\n return hi\n \n while lo < hi:\n mid = (lo+hi) >>1\n if A[:mid+1] == t[:mid+1]:\n lo = mid +1\n else:\n hi = mid\n\n return lo\n res = 0\n for i in range(m):\n res += bisect_strs(A[i:])\n return res\n``` | 0 | You are **building** a string `s` of length `n` **one** character at a time, **prepending** each new character to the **front** of the string. The strings are labeled from `1` to `n`, where the string with length `i` is labeled `si`.
* For example, for `s = "abaca "`, `s1 == "a "`, `s2 == "ca "`, `s3 == "aca "`, etc.
The **score** of `si` is the length of the **longest common prefix** between `si` and `sn` (Note that `s == sn`).
Given the final string `s`, return _the **sum** of the **score** of every_ `si`.
**Example 1:**
**Input:** s = "babab "
**Output:** 9
**Explanation:**
For s1 == "b ", the longest common prefix is "b " which has a score of 1.
For s2 == "ab ", there is no common prefix so the score is 0.
For s3 == "bab ", the longest common prefix is "bab " which has a score of 3.
For s4 == "abab ", there is no common prefix so the score is 0.
For s5 == "babab ", the longest common prefix is "babab " which has a score of 5.
The sum of the scores is 1 + 0 + 3 + 0 + 5 = 9, so we return 9.
**Example 2:**
**Input:** s = "azbazbzaz "
**Output:** 14
**Explanation:**
For s2 == "az ", the longest common prefix is "az " which has a score of 2.
For s6 == "azbzaz ", the longest common prefix is "azb " which has a score of 3.
For s9 == "azbazbzaz ", the longest common prefix is "azbazbzaz " which has a score of 9.
For all other si, the score is 0.
The sum of the scores is 2 + 3 + 9 = 14, so we return 14.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of lowercase English letters. | null |
Python (Simple KMP) | sum-of-scores-of-built-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def sumScores(self, s):\n n = len(s)\n\n dp, ans, j = [1]*n, [0]*n, 0 \n\n for i in range(1,n):\n while s[i] != s[j] and j > 0:\n j = ans[j-1]\n\n if s[i] == s[j]:\n dp[i] += dp[j]\n ans[i] = j+1\n j += 1 \n\n return sum(dp)\n\n\n\n\n\n\n\n \n``` | 0 | You are **building** a string `s` of length `n` **one** character at a time, **prepending** each new character to the **front** of the string. The strings are labeled from `1` to `n`, where the string with length `i` is labeled `si`.
* For example, for `s = "abaca "`, `s1 == "a "`, `s2 == "ca "`, `s3 == "aca "`, etc.
The **score** of `si` is the length of the **longest common prefix** between `si` and `sn` (Note that `s == sn`).
Given the final string `s`, return _the **sum** of the **score** of every_ `si`.
**Example 1:**
**Input:** s = "babab "
**Output:** 9
**Explanation:**
For s1 == "b ", the longest common prefix is "b " which has a score of 1.
For s2 == "ab ", there is no common prefix so the score is 0.
For s3 == "bab ", the longest common prefix is "bab " which has a score of 3.
For s4 == "abab ", there is no common prefix so the score is 0.
For s5 == "babab ", the longest common prefix is "babab " which has a score of 5.
The sum of the scores is 1 + 0 + 3 + 0 + 5 = 9, so we return 9.
**Example 2:**
**Input:** s = "azbazbzaz "
**Output:** 14
**Explanation:**
For s2 == "az ", the longest common prefix is "az " which has a score of 2.
For s6 == "azbzaz ", the longest common prefix is "azb " which has a score of 3.
For s9 == "azbazbzaz ", the longest common prefix is "azbazbzaz " which has a score of 9.
For all other si, the score is 0.
The sum of the scores is 2 + 3 + 9 = 14, so we return 14.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of lowercase English letters. | null |
Fully annotated Z algorithm Python Solution | sum-of-scores-of-built-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def sumScores(self, s: str) -> int:\n # Initialize the Z array with zeros\n n = len(s)\n z = [0] * n\n\n # The Z algorithm works by comparing the current character with the characters\n # that come before it in the string. To do this, we need to keep track of\n # two indices: l and r. l is the start of the current Z block, and r is the\n # end of the current Z block.\n l, r = 0, 0\n\n # The first element of the Z array is always equal to the length of the string\n z[0] = n\n\n # Iterate through the string, starting from the second character\n for i in range(1, n):\n # If the current index is outside the current Z block, we need to\n # recalculate the Z value for this index from scratch.\n if i > r:\n # Set the start and end of the Z block to the current index\n l, r = i, i\n\n # Keep advancing the end of the Z block until we find a mismatch\n # between the characters at the start and end of the block\n while r < n and s[r - l] == s[r]:\n r += 1\n\n # The Z value for the current index is the length of the Z block\n z[i] = r - l\n\n # Move the end of the Z block back by one character, so that it\n # is ready to be compared with the next character in the string\n r -= 1\n\n # If the current index is inside the current Z block, we can use the\n # previously calculated Z values to determine the Z value for this index\n # more efficiently.\n else:\n # k is the number of characters that have been matched so far\n # between the current index and the start of the Z block\n k = i - l\n\n # If the Z value for the kth character is less than the distance\n # between the current index and the end of the Z block, we can\n # simply copy the Z value from the kth character.\n if z[k] < r - i + 1:\n z[i] = z[k]\n\n # Otherwise, we need to recalculate the Z value for the current\n # index from scratch, just like we did before.\n else:\n # Set the start of the Z block to the current index\n l = i\n\n # Keep advancing the end of the Z block until we find a mismatch\n while r < n and s[r - l] == s[r]:\n r += 1\n\n # The Z value for the current index is the length of the Z block\n z[i] = r - l\n\n # Move the end of the Z block back by one character, so that it\n # is ready to be compared with the next character in the string\n r -= 1\n\n # Return the final Z array\n return sum(z)\n``` | 0 | You are **building** a string `s` of length `n` **one** character at a time, **prepending** each new character to the **front** of the string. The strings are labeled from `1` to `n`, where the string with length `i` is labeled `si`.
* For example, for `s = "abaca "`, `s1 == "a "`, `s2 == "ca "`, `s3 == "aca "`, etc.
The **score** of `si` is the length of the **longest common prefix** between `si` and `sn` (Note that `s == sn`).
Given the final string `s`, return _the **sum** of the **score** of every_ `si`.
**Example 1:**
**Input:** s = "babab "
**Output:** 9
**Explanation:**
For s1 == "b ", the longest common prefix is "b " which has a score of 1.
For s2 == "ab ", there is no common prefix so the score is 0.
For s3 == "bab ", the longest common prefix is "bab " which has a score of 3.
For s4 == "abab ", there is no common prefix so the score is 0.
For s5 == "babab ", the longest common prefix is "babab " which has a score of 5.
The sum of the scores is 1 + 0 + 3 + 0 + 5 = 9, so we return 9.
**Example 2:**
**Input:** s = "azbazbzaz "
**Output:** 14
**Explanation:**
For s2 == "az ", the longest common prefix is "az " which has a score of 2.
For s6 == "azbzaz ", the longest common prefix is "azb " which has a score of 3.
For s9 == "azbazbzaz ", the longest common prefix is "azbazbzaz " which has a score of 9.
For all other si, the score is 0.
The sum of the scores is 2 + 3 + 9 = 14, so we return 14.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of lowercase English letters. | null |
Python solution | KMP-prefix | Z-function | sum-of-scores-of-built-strings | 0 | 1 | # Approach 1\n<!-- Describe your approach to solving the problem. -->\nKMP-prefix computation\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def sumScores(self, s: str) -> int:\n n = len(s)\n dp, p = [1]*n, [0]*n\n for i in range(1, n):\n j = p[i-1]\n while(j and s[i]!=s[j]):\n j = p[j-1]\n if s[i]==s[j]:\n dp[i] += dp[j]\n j += 1\n p[i] = j\n return sum(dp)\n```\n\n# Approach 2\n<!-- Describe your approach to solving the problem. -->\nZ-function computation\n\nthe references link of z-function algorithm.\n[Video explanation: highly recommended!](https://www.youtube.com/watch?v=CpZh4eF8QBw&ab_channel=TusharRoy-CodingMadeSimple)\n[Text explanation](https://cp-algorithms.com/string/z-function.html)\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n\n# Code\n```\nclass Solution:\n def sumScores(self, s: str) -> int:\n n, l, r = len(s), 0, 0\n z = [0] * n\n for i in range(1, n):\n if i <= r:\n z[i] = min(r - i + 1, z[i - l])\n while i + z[i] < n and s[z[i]] == s[i + z[i]]:\n z[i] += 1\n if i + z[i] - 1 > r:\n l, r = i, i + z[i] - 1\n return sum(z)+len(s)\n```\n\n | 0 | You are **building** a string `s` of length `n` **one** character at a time, **prepending** each new character to the **front** of the string. The strings are labeled from `1` to `n`, where the string with length `i` is labeled `si`.
* For example, for `s = "abaca "`, `s1 == "a "`, `s2 == "ca "`, `s3 == "aca "`, etc.
The **score** of `si` is the length of the **longest common prefix** between `si` and `sn` (Note that `s == sn`).
Given the final string `s`, return _the **sum** of the **score** of every_ `si`.
**Example 1:**
**Input:** s = "babab "
**Output:** 9
**Explanation:**
For s1 == "b ", the longest common prefix is "b " which has a score of 1.
For s2 == "ab ", there is no common prefix so the score is 0.
For s3 == "bab ", the longest common prefix is "bab " which has a score of 3.
For s4 == "abab ", there is no common prefix so the score is 0.
For s5 == "babab ", the longest common prefix is "babab " which has a score of 5.
The sum of the scores is 1 + 0 + 3 + 0 + 5 = 9, so we return 9.
**Example 2:**
**Input:** s = "azbazbzaz "
**Output:** 14
**Explanation:**
For s2 == "az ", the longest common prefix is "az " which has a score of 2.
For s6 == "azbzaz ", the longest common prefix is "azb " which has a score of 3.
For s9 == "azbazbzaz ", the longest common prefix is "azbazbzaz " which has a score of 9.
For all other si, the score is 0.
The sum of the scores is 2 + 3 + 9 = 14, so we return 14.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of lowercase English letters. | null |
⭐Easy Python Solution | Convert time to minutes | minimum-number-of-operations-to-convert-time | 0 | 1 | 1. Convert times into minutes and then the problem becomes simpler. \n2. To minimize the number of total operations we try to use the largest possible change from `[60,15,5,1]` till possible.\n```\nclass Solution:\n def convertTime(self, current: str, correct: str) -> int:\n current_time = 60 * int(current[0:2]) + int(current[3:5]) # Current time in minutes\n target_time = 60 * int(correct[0:2]) + int(correct[3:5]) # Target time in minutes\n diff = target_time - current_time # Difference b/w current and target times in minutes\n count = 0 # Required number of operations\n\t\t# Use GREEDY APPROACH to calculate number of operations\n for i in [60, 15, 5, 1]:\n count += diff // i # add number of operations needed with i to count\n diff %= i # Diff becomes modulo of diff with i\n return count\n```\n | 35 | You are given two strings `current` and `correct` representing two **24-hour times**.
24-hour times are formatted as `"HH:MM "`, where `HH` is between `00` and `23`, and `MM` is between `00` and `59`. The earliest 24-hour time is `00:00`, and the latest is `23:59`.
In one operation you can increase the time `current` by `1`, `5`, `15`, or `60` minutes. You can perform this operation **any** number of times.
Return _the **minimum number of operations** needed to convert_ `current` _to_ `correct`.
**Example 1:**
**Input:** current = "02:30 ", correct = "04:35 "
**Output:** 3
**Explanation:**
We can convert current to correct in 3 operations as follows:
- Add 60 minutes to current. current becomes "03:30 ".
- Add 60 minutes to current. current becomes "04:30 ".
- Add 5 minutes to current. current becomes "04:35 ".
It can be proven that it is not possible to convert current to correct in fewer than 3 operations.
**Example 2:**
**Input:** current = "11:00 ", correct = "11:01 "
**Output:** 1
**Explanation:** We only have to add one minute to current, so the minimum number of operations needed is 1.
**Constraints:**
* `current` and `correct` are in the format `"HH:MM "`
* `current <= correct` | null |
🔥[Python 3] Convert to minutes and find parts using divmod, beats 85% | minimum-number-of-operations-to-convert-time | 0 | 1 | ```\nclass Solution:\n def convertTime(self, current: str, correct: str) -> int:\n def toMinutes(s):\n h, m = s.split(\':\')\n return 60 * int(h) + int(m)\n \n minutes = toMinutes(correct) - toMinutes(current)\n hours, minutes = divmod(minutes, 60)\n quaters, minutes = divmod(minutes, 15)\n fives, minutes = divmod(minutes, 5)\n\n return hours + quaters + fives + minutes\n``` | 5 | You are given two strings `current` and `correct` representing two **24-hour times**.
24-hour times are formatted as `"HH:MM "`, where `HH` is between `00` and `23`, and `MM` is between `00` and `59`. The earliest 24-hour time is `00:00`, and the latest is `23:59`.
In one operation you can increase the time `current` by `1`, `5`, `15`, or `60` minutes. You can perform this operation **any** number of times.
Return _the **minimum number of operations** needed to convert_ `current` _to_ `correct`.
**Example 1:**
**Input:** current = "02:30 ", correct = "04:35 "
**Output:** 3
**Explanation:**
We can convert current to correct in 3 operations as follows:
- Add 60 minutes to current. current becomes "03:30 ".
- Add 60 minutes to current. current becomes "04:30 ".
- Add 5 minutes to current. current becomes "04:35 ".
It can be proven that it is not possible to convert current to correct in fewer than 3 operations.
**Example 2:**
**Input:** current = "11:00 ", correct = "11:01 "
**Output:** 1
**Explanation:** We only have to add one minute to current, so the minimum number of operations needed is 1.
**Constraints:**
* `current` and `correct` are in the format `"HH:MM "`
* `current <= correct` | null |
Python convert to minutes | minimum-number-of-operations-to-convert-time | 0 | 1 | ```\ndef convertTime(self, current: str, correct: str) -> int:\n\tans = 0\n\th, m = list(map(int, current.split(\':\')))\n\tnewH, newM = list(map(int, correct.split(\':\')))\n\td = 60*(newH - h) + newM - m\n\tfor x in [60, 15, 5, 1]:\n\t\tans += d//x\n\t\td %= x\n\treturn ans \n``` | 4 | You are given two strings `current` and `correct` representing two **24-hour times**.
24-hour times are formatted as `"HH:MM "`, where `HH` is between `00` and `23`, and `MM` is between `00` and `59`. The earliest 24-hour time is `00:00`, and the latest is `23:59`.
In one operation you can increase the time `current` by `1`, `5`, `15`, or `60` minutes. You can perform this operation **any** number of times.
Return _the **minimum number of operations** needed to convert_ `current` _to_ `correct`.
**Example 1:**
**Input:** current = "02:30 ", correct = "04:35 "
**Output:** 3
**Explanation:**
We can convert current to correct in 3 operations as follows:
- Add 60 minutes to current. current becomes "03:30 ".
- Add 60 minutes to current. current becomes "04:30 ".
- Add 5 minutes to current. current becomes "04:35 ".
It can be proven that it is not possible to convert current to correct in fewer than 3 operations.
**Example 2:**
**Input:** current = "11:00 ", correct = "11:01 "
**Output:** 1
**Explanation:** We only have to add one minute to current, so the minimum number of operations needed is 1.
**Constraints:**
* `current` and `correct` are in the format `"HH:MM "`
* `current <= correct` | null |
Beat 99.29% 22ms Python3 easy solution | minimum-number-of-operations-to-convert-time | 0 | 1 | \n# Code\n```\nclass Solution:\n def convertTime(self, current: str, correct: str) -> int:\n current, correct = current.replace(\':\',\'\'), correct.replace(\':\',\'\')\n a, count = (int(correct[:2]) * 60 + int(correct[2:])) - (int(current[:2]) * 60 + int(current[2:])), 0\n for i in [60, 15, 5, 1]:\n count += a // i\n a %= i\n return count \n\n #before for loop\n # while a != 0:\n # if a >= 60:\n # count += (a // 60)\n # a %= 60\n # elif 15 <= a < 60:\n # count += (a // 15)\n # a %= 15\n # elif 5 <= a < 15:\n # count += (a // 5)\n # a %= 5\n # elif 1 <= a < 5:\n # count += (a // 1)\n # a %= 1\n``` | 2 | You are given two strings `current` and `correct` representing two **24-hour times**.
24-hour times are formatted as `"HH:MM "`, where `HH` is between `00` and `23`, and `MM` is between `00` and `59`. The earliest 24-hour time is `00:00`, and the latest is `23:59`.
In one operation you can increase the time `current` by `1`, `5`, `15`, or `60` minutes. You can perform this operation **any** number of times.
Return _the **minimum number of operations** needed to convert_ `current` _to_ `correct`.
**Example 1:**
**Input:** current = "02:30 ", correct = "04:35 "
**Output:** 3
**Explanation:**
We can convert current to correct in 3 operations as follows:
- Add 60 minutes to current. current becomes "03:30 ".
- Add 60 minutes to current. current becomes "04:30 ".
- Add 5 minutes to current. current becomes "04:35 ".
It can be proven that it is not possible to convert current to correct in fewer than 3 operations.
**Example 2:**
**Input:** current = "11:00 ", correct = "11:01 "
**Output:** 1
**Explanation:** We only have to add one minute to current, so the minimum number of operations needed is 1.
**Constraints:**
* `current` and `correct` are in the format `"HH:MM "`
* `current <= correct` | null |
Python O(1) Time | minimum-number-of-operations-to-convert-time | 0 | 1 | ```\nclass Solution:\n def convertTime(self, s: str, c: str) -> int:\n dif=(int(c[:2])*60+int(c[3:]))-(int(s[:2])*60+int(s[3:]))\n count=0\n print(dif)\n arr=[60,15,5,1]\n for x in arr:\n count+=dif//x\n dif=dif%x\n return count | 3 | You are given two strings `current` and `correct` representing two **24-hour times**.
24-hour times are formatted as `"HH:MM "`, where `HH` is between `00` and `23`, and `MM` is between `00` and `59`. The earliest 24-hour time is `00:00`, and the latest is `23:59`.
In one operation you can increase the time `current` by `1`, `5`, `15`, or `60` minutes. You can perform this operation **any** number of times.
Return _the **minimum number of operations** needed to convert_ `current` _to_ `correct`.
**Example 1:**
**Input:** current = "02:30 ", correct = "04:35 "
**Output:** 3
**Explanation:**
We can convert current to correct in 3 operations as follows:
- Add 60 minutes to current. current becomes "03:30 ".
- Add 60 minutes to current. current becomes "04:30 ".
- Add 5 minutes to current. current becomes "04:35 ".
It can be proven that it is not possible to convert current to correct in fewer than 3 operations.
**Example 2:**
**Input:** current = "11:00 ", correct = "11:01 "
**Output:** 1
**Explanation:** We only have to add one minute to current, so the minimum number of operations needed is 1.
**Constraints:**
* `current` and `correct` are in the format `"HH:MM "`
* `current <= correct` | null |
dictionary does the job | find-players-with-zero-or-one-losses | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n dc=defaultdict(lambda:[0,0])\n for a in matches:\n dc[a[0]][0]+=1\n dc[a[1]][1]+=1\n ans=[[],[]]\n for a in dc:\n if(dc[a][1]==0):\n ans[0].append(a)\n if(dc[a][1]==1):\n ans[1].append(a)\n ans[0].sort()\n ans[1].sort()\n return ans\n``` | 6 | You are given an integer array `matches` where `matches[i] = [winneri, loseri]` indicates that the player `winneri` defeated player `loseri` in a match.
Return _a list_ `answer` _of size_ `2` _where:_
* `answer[0]` is a list of all players that have **not** lost any matches.
* `answer[1]` is a list of all players that have lost exactly **one** match.
The values in the two lists should be returned in **increasing** order.
**Note:**
* You should only consider the players that have played **at least one** match.
* The testcases will be generated such that **no** two matches will have the **same** outcome.
**Example 1:**
**Input:** matches = \[\[1,3\],\[2,3\],\[3,6\],\[5,6\],\[5,7\],\[4,5\],\[4,8\],\[4,9\],\[10,4\],\[10,9\]\]
**Output:** \[\[1,2,10\],\[4,5,7,8\]\]
**Explanation:**
Players 1, 2, and 10 have not lost any matches.
Players 4, 5, 7, and 8 each have lost one match.
Players 3, 6, and 9 each have lost two matches.
Thus, answer\[0\] = \[1,2,10\] and answer\[1\] = \[4,5,7,8\].
**Example 2:**
**Input:** matches = \[\[2,3\],\[1,3\],\[5,4\],\[6,4\]\]
**Output:** \[\[1,2,5,6\],\[\]\]
**Explanation:**
Players 1, 2, 5, and 6 have not lost any matches.
Players 3 and 4 each have lost two matches.
Thus, answer\[0\] = \[1,2,5,6\] and answer\[1\] = \[\].
**Constraints:**
* `1 <= matches.length <= 105`
* `matches[i].length == 2`
* `1 <= winneri, loseri <= 105`
* `winneri != loseri`
* All `matches[i]` are **unique**. | In the string "abacad", the letter "a" appears 3 times. How many substrings begin with the first "a" and end with any "a"? There are 3 substrings ("a", "aba", and "abaca"). How many substrings begin with the second "a" and end with any "a"? How about the third? 2 substrings begin with the second "a" ("a", and "aca") and 1 substring begins with the third "a" ("a"). There is a total of 3 + 2 + 1 = 6 substrings that begin and end with "a". If a character appears i times in the string, there are i * (i + 1) / 2 substrings that begin and end with that character. |
dictionary does the job | find-players-with-zero-or-one-losses | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n dc=defaultdict(lambda:[0,0])\n for a in matches:\n dc[a[0]][0]+=1\n dc[a[1]][1]+=1\n ans=[[],[]]\n for a in dc:\n if(dc[a][1]==0):\n ans[0].append(a)\n if(dc[a][1]==1):\n ans[1].append(a)\n ans[0].sort()\n ans[1].sort()\n return ans\n``` | 6 | You are given an array `target` of n integers. From a starting array `arr` consisting of `n` 1's, you may perform the following procedure :
* let `x` be the sum of all elements currently in your array.
* choose index `i`, such that `0 <= i < n` and set the value of `arr` at index `i` to `x`.
* You may repeat this procedure as many times as needed.
Return `true` _if it is possible to construct the_ `target` _array from_ `arr`_, otherwise, return_ `false`.
**Example 1:**
**Input:** target = \[9,3,5\]
**Output:** true
**Explanation:** Start with arr = \[1, 1, 1\]
\[1, 1, 1\], sum = 3 choose index 1
\[1, 3, 1\], sum = 5 choose index 2
\[1, 3, 5\], sum = 9 choose index 0
\[9, 3, 5\] Done
**Example 2:**
**Input:** target = \[1,1,1,2\]
**Output:** false
**Explanation:** Impossible to create target array from \[1,1,1,1\].
**Example 3:**
**Input:** target = \[8,5\]
**Output:** true
**Constraints:**
* `n == target.length`
* `1 <= n <= 5 * 104`
* `1 <= target[i] <= 109` | Count the number of times a player loses while iterating through the matches. |
Most space optimized python solution | find-players-with-zero-or-one-losses | 0 | 1 | ```\nfrom collections import defaultdict\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n dct=defaultdict(lambda :[0,0])\n for p1,p2 in matches:\n dct[p1][0]+=1\n dct[p1][1]+=1\n dct[p2][0]+=1\n wonAll=[]\n lossOne=[]\n for i in dct:\n if dct[i][0]==dct[i][1]:\n wonAll.append(i)\n elif dct[i][0]-dct[i][1]==1:\n lossOne.append(i)\n wonAll.sort()\n lossOne.sort()\n return [wonAll,lossOne]\n``` | 5 | You are given an integer array `matches` where `matches[i] = [winneri, loseri]` indicates that the player `winneri` defeated player `loseri` in a match.
Return _a list_ `answer` _of size_ `2` _where:_
* `answer[0]` is a list of all players that have **not** lost any matches.
* `answer[1]` is a list of all players that have lost exactly **one** match.
The values in the two lists should be returned in **increasing** order.
**Note:**
* You should only consider the players that have played **at least one** match.
* The testcases will be generated such that **no** two matches will have the **same** outcome.
**Example 1:**
**Input:** matches = \[\[1,3\],\[2,3\],\[3,6\],\[5,6\],\[5,7\],\[4,5\],\[4,8\],\[4,9\],\[10,4\],\[10,9\]\]
**Output:** \[\[1,2,10\],\[4,5,7,8\]\]
**Explanation:**
Players 1, 2, and 10 have not lost any matches.
Players 4, 5, 7, and 8 each have lost one match.
Players 3, 6, and 9 each have lost two matches.
Thus, answer\[0\] = \[1,2,10\] and answer\[1\] = \[4,5,7,8\].
**Example 2:**
**Input:** matches = \[\[2,3\],\[1,3\],\[5,4\],\[6,4\]\]
**Output:** \[\[1,2,5,6\],\[\]\]
**Explanation:**
Players 1, 2, 5, and 6 have not lost any matches.
Players 3 and 4 each have lost two matches.
Thus, answer\[0\] = \[1,2,5,6\] and answer\[1\] = \[\].
**Constraints:**
* `1 <= matches.length <= 105`
* `matches[i].length == 2`
* `1 <= winneri, loseri <= 105`
* `winneri != loseri`
* All `matches[i]` are **unique**. | In the string "abacad", the letter "a" appears 3 times. How many substrings begin with the first "a" and end with any "a"? There are 3 substrings ("a", "aba", and "abaca"). How many substrings begin with the second "a" and end with any "a"? How about the third? 2 substrings begin with the second "a" ("a", and "aca") and 1 substring begins with the third "a" ("a"). There is a total of 3 + 2 + 1 = 6 substrings that begin and end with "a". If a character appears i times in the string, there are i * (i + 1) / 2 substrings that begin and end with that character. |
Most space optimized python solution | find-players-with-zero-or-one-losses | 0 | 1 | ```\nfrom collections import defaultdict\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n dct=defaultdict(lambda :[0,0])\n for p1,p2 in matches:\n dct[p1][0]+=1\n dct[p1][1]+=1\n dct[p2][0]+=1\n wonAll=[]\n lossOne=[]\n for i in dct:\n if dct[i][0]==dct[i][1]:\n wonAll.append(i)\n elif dct[i][0]-dct[i][1]==1:\n lossOne.append(i)\n wonAll.sort()\n lossOne.sort()\n return [wonAll,lossOne]\n``` | 5 | You are given an array `target` of n integers. From a starting array `arr` consisting of `n` 1's, you may perform the following procedure :
* let `x` be the sum of all elements currently in your array.
* choose index `i`, such that `0 <= i < n` and set the value of `arr` at index `i` to `x`.
* You may repeat this procedure as many times as needed.
Return `true` _if it is possible to construct the_ `target` _array from_ `arr`_, otherwise, return_ `false`.
**Example 1:**
**Input:** target = \[9,3,5\]
**Output:** true
**Explanation:** Start with arr = \[1, 1, 1\]
\[1, 1, 1\], sum = 3 choose index 1
\[1, 3, 1\], sum = 5 choose index 2
\[1, 3, 5\], sum = 9 choose index 0
\[9, 3, 5\] Done
**Example 2:**
**Input:** target = \[1,1,1,2\]
**Output:** false
**Explanation:** Impossible to create target array from \[1,1,1,1\].
**Example 3:**
**Input:** target = \[8,5\]
**Output:** true
**Constraints:**
* `n == target.length`
* `1 <= n <= 5 * 104`
* `1 <= target[i] <= 109` | Count the number of times a player loses while iterating through the matches. |
Python in 2 lines | find-players-with-zero-or-one-losses | 0 | 1 | \n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n win, lose = zip(*matches)\n return [sorted(set(win) - set(lose)), sorted(k for k, v in Counter(lose).items() if v == 1)]\n``` | 4 | You are given an integer array `matches` where `matches[i] = [winneri, loseri]` indicates that the player `winneri` defeated player `loseri` in a match.
Return _a list_ `answer` _of size_ `2` _where:_
* `answer[0]` is a list of all players that have **not** lost any matches.
* `answer[1]` is a list of all players that have lost exactly **one** match.
The values in the two lists should be returned in **increasing** order.
**Note:**
* You should only consider the players that have played **at least one** match.
* The testcases will be generated such that **no** two matches will have the **same** outcome.
**Example 1:**
**Input:** matches = \[\[1,3\],\[2,3\],\[3,6\],\[5,6\],\[5,7\],\[4,5\],\[4,8\],\[4,9\],\[10,4\],\[10,9\]\]
**Output:** \[\[1,2,10\],\[4,5,7,8\]\]
**Explanation:**
Players 1, 2, and 10 have not lost any matches.
Players 4, 5, 7, and 8 each have lost one match.
Players 3, 6, and 9 each have lost two matches.
Thus, answer\[0\] = \[1,2,10\] and answer\[1\] = \[4,5,7,8\].
**Example 2:**
**Input:** matches = \[\[2,3\],\[1,3\],\[5,4\],\[6,4\]\]
**Output:** \[\[1,2,5,6\],\[\]\]
**Explanation:**
Players 1, 2, 5, and 6 have not lost any matches.
Players 3 and 4 each have lost two matches.
Thus, answer\[0\] = \[1,2,5,6\] and answer\[1\] = \[\].
**Constraints:**
* `1 <= matches.length <= 105`
* `matches[i].length == 2`
* `1 <= winneri, loseri <= 105`
* `winneri != loseri`
* All `matches[i]` are **unique**. | In the string "abacad", the letter "a" appears 3 times. How many substrings begin with the first "a" and end with any "a"? There are 3 substrings ("a", "aba", and "abaca"). How many substrings begin with the second "a" and end with any "a"? How about the third? 2 substrings begin with the second "a" ("a", and "aca") and 1 substring begins with the third "a" ("a"). There is a total of 3 + 2 + 1 = 6 substrings that begin and end with "a". If a character appears i times in the string, there are i * (i + 1) / 2 substrings that begin and end with that character. |
Python in 2 lines | find-players-with-zero-or-one-losses | 0 | 1 | \n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n win, lose = zip(*matches)\n return [sorted(set(win) - set(lose)), sorted(k for k, v in Counter(lose).items() if v == 1)]\n``` | 4 | You are given an array `target` of n integers. From a starting array `arr` consisting of `n` 1's, you may perform the following procedure :
* let `x` be the sum of all elements currently in your array.
* choose index `i`, such that `0 <= i < n` and set the value of `arr` at index `i` to `x`.
* You may repeat this procedure as many times as needed.
Return `true` _if it is possible to construct the_ `target` _array from_ `arr`_, otherwise, return_ `false`.
**Example 1:**
**Input:** target = \[9,3,5\]
**Output:** true
**Explanation:** Start with arr = \[1, 1, 1\]
\[1, 1, 1\], sum = 3 choose index 1
\[1, 3, 1\], sum = 5 choose index 2
\[1, 3, 5\], sum = 9 choose index 0
\[9, 3, 5\] Done
**Example 2:**
**Input:** target = \[1,1,1,2\]
**Output:** false
**Explanation:** Impossible to create target array from \[1,1,1,1\].
**Example 3:**
**Input:** target = \[8,5\]
**Output:** true
**Constraints:**
* `n == target.length`
* `1 <= n <= 5 * 104`
* `1 <= target[i] <= 109` | Count the number of times a player loses while iterating through the matches. |
Easy Python Solution | find-players-with-zero-or-one-losses | 0 | 1 | # Code\n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n win=[]\n lose=[]\n for i in matches:\n win.append(i[0])\n lose.append(i[1])\n l=[] #0 lose\n m=[] #1 lose\n c=Counter(lose)\n for i in range(len(win)):\n if c[win[i]]==0:\n l.append(win[i])\n if c[win[i]]==1:\n m.append(win[i])\n if c[lose[i]]==1:\n m.append(lose[i])\n \n l=list(set(l))\n m=list(set(m))\n l.sort()\n m.sort()\n\n return [l,m]\n``` | 2 | You are given an integer array `matches` where `matches[i] = [winneri, loseri]` indicates that the player `winneri` defeated player `loseri` in a match.
Return _a list_ `answer` _of size_ `2` _where:_
* `answer[0]` is a list of all players that have **not** lost any matches.
* `answer[1]` is a list of all players that have lost exactly **one** match.
The values in the two lists should be returned in **increasing** order.
**Note:**
* You should only consider the players that have played **at least one** match.
* The testcases will be generated such that **no** two matches will have the **same** outcome.
**Example 1:**
**Input:** matches = \[\[1,3\],\[2,3\],\[3,6\],\[5,6\],\[5,7\],\[4,5\],\[4,8\],\[4,9\],\[10,4\],\[10,9\]\]
**Output:** \[\[1,2,10\],\[4,5,7,8\]\]
**Explanation:**
Players 1, 2, and 10 have not lost any matches.
Players 4, 5, 7, and 8 each have lost one match.
Players 3, 6, and 9 each have lost two matches.
Thus, answer\[0\] = \[1,2,10\] and answer\[1\] = \[4,5,7,8\].
**Example 2:**
**Input:** matches = \[\[2,3\],\[1,3\],\[5,4\],\[6,4\]\]
**Output:** \[\[1,2,5,6\],\[\]\]
**Explanation:**
Players 1, 2, 5, and 6 have not lost any matches.
Players 3 and 4 each have lost two matches.
Thus, answer\[0\] = \[1,2,5,6\] and answer\[1\] = \[\].
**Constraints:**
* `1 <= matches.length <= 105`
* `matches[i].length == 2`
* `1 <= winneri, loseri <= 105`
* `winneri != loseri`
* All `matches[i]` are **unique**. | In the string "abacad", the letter "a" appears 3 times. How many substrings begin with the first "a" and end with any "a"? There are 3 substrings ("a", "aba", and "abaca"). How many substrings begin with the second "a" and end with any "a"? How about the third? 2 substrings begin with the second "a" ("a", and "aca") and 1 substring begins with the third "a" ("a"). There is a total of 3 + 2 + 1 = 6 substrings that begin and end with "a". If a character appears i times in the string, there are i * (i + 1) / 2 substrings that begin and end with that character. |
Easy Python Solution | find-players-with-zero-or-one-losses | 0 | 1 | # Code\n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n win=[]\n lose=[]\n for i in matches:\n win.append(i[0])\n lose.append(i[1])\n l=[] #0 lose\n m=[] #1 lose\n c=Counter(lose)\n for i in range(len(win)):\n if c[win[i]]==0:\n l.append(win[i])\n if c[win[i]]==1:\n m.append(win[i])\n if c[lose[i]]==1:\n m.append(lose[i])\n \n l=list(set(l))\n m=list(set(m))\n l.sort()\n m.sort()\n\n return [l,m]\n``` | 2 | You are given an array `target` of n integers. From a starting array `arr` consisting of `n` 1's, you may perform the following procedure :
* let `x` be the sum of all elements currently in your array.
* choose index `i`, such that `0 <= i < n` and set the value of `arr` at index `i` to `x`.
* You may repeat this procedure as many times as needed.
Return `true` _if it is possible to construct the_ `target` _array from_ `arr`_, otherwise, return_ `false`.
**Example 1:**
**Input:** target = \[9,3,5\]
**Output:** true
**Explanation:** Start with arr = \[1, 1, 1\]
\[1, 1, 1\], sum = 3 choose index 1
\[1, 3, 1\], sum = 5 choose index 2
\[1, 3, 5\], sum = 9 choose index 0
\[9, 3, 5\] Done
**Example 2:**
**Input:** target = \[1,1,1,2\]
**Output:** false
**Explanation:** Impossible to create target array from \[1,1,1,1\].
**Example 3:**
**Input:** target = \[8,5\]
**Output:** true
**Constraints:**
* `n == target.length`
* `1 <= n <= 5 * 104`
* `1 <= target[i] <= 109` | Count the number of times a player loses while iterating through the matches. |
Beats 99% | python | hashmap | find-players-with-zero-or-one-losses | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n d = {}\n for i in matches:\n if i[1] in d:\n d[i[1]] +=1\n else:\n d[i[1]] = 1\n a,b =set(),[]\n\n for i in matches:\n if i[0] not in d:\n a.add(i[0])\n if d[i[1]] == 1:\n b.append(i[1])\n a = sorted(list(a))\n b = sorted(b)\n return [a,b]\n``` | 1 | You are given an integer array `matches` where `matches[i] = [winneri, loseri]` indicates that the player `winneri` defeated player `loseri` in a match.
Return _a list_ `answer` _of size_ `2` _where:_
* `answer[0]` is a list of all players that have **not** lost any matches.
* `answer[1]` is a list of all players that have lost exactly **one** match.
The values in the two lists should be returned in **increasing** order.
**Note:**
* You should only consider the players that have played **at least one** match.
* The testcases will be generated such that **no** two matches will have the **same** outcome.
**Example 1:**
**Input:** matches = \[\[1,3\],\[2,3\],\[3,6\],\[5,6\],\[5,7\],\[4,5\],\[4,8\],\[4,9\],\[10,4\],\[10,9\]\]
**Output:** \[\[1,2,10\],\[4,5,7,8\]\]
**Explanation:**
Players 1, 2, and 10 have not lost any matches.
Players 4, 5, 7, and 8 each have lost one match.
Players 3, 6, and 9 each have lost two matches.
Thus, answer\[0\] = \[1,2,10\] and answer\[1\] = \[4,5,7,8\].
**Example 2:**
**Input:** matches = \[\[2,3\],\[1,3\],\[5,4\],\[6,4\]\]
**Output:** \[\[1,2,5,6\],\[\]\]
**Explanation:**
Players 1, 2, 5, and 6 have not lost any matches.
Players 3 and 4 each have lost two matches.
Thus, answer\[0\] = \[1,2,5,6\] and answer\[1\] = \[\].
**Constraints:**
* `1 <= matches.length <= 105`
* `matches[i].length == 2`
* `1 <= winneri, loseri <= 105`
* `winneri != loseri`
* All `matches[i]` are **unique**. | In the string "abacad", the letter "a" appears 3 times. How many substrings begin with the first "a" and end with any "a"? There are 3 substrings ("a", "aba", and "abaca"). How many substrings begin with the second "a" and end with any "a"? How about the third? 2 substrings begin with the second "a" ("a", and "aca") and 1 substring begins with the third "a" ("a"). There is a total of 3 + 2 + 1 = 6 substrings that begin and end with "a". If a character appears i times in the string, there are i * (i + 1) / 2 substrings that begin and end with that character. |
Beats 99% | python | hashmap | find-players-with-zero-or-one-losses | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n d = {}\n for i in matches:\n if i[1] in d:\n d[i[1]] +=1\n else:\n d[i[1]] = 1\n a,b =set(),[]\n\n for i in matches:\n if i[0] not in d:\n a.add(i[0])\n if d[i[1]] == 1:\n b.append(i[1])\n a = sorted(list(a))\n b = sorted(b)\n return [a,b]\n``` | 1 | You are given an array `target` of n integers. From a starting array `arr` consisting of `n` 1's, you may perform the following procedure :
* let `x` be the sum of all elements currently in your array.
* choose index `i`, such that `0 <= i < n` and set the value of `arr` at index `i` to `x`.
* You may repeat this procedure as many times as needed.
Return `true` _if it is possible to construct the_ `target` _array from_ `arr`_, otherwise, return_ `false`.
**Example 1:**
**Input:** target = \[9,3,5\]
**Output:** true
**Explanation:** Start with arr = \[1, 1, 1\]
\[1, 1, 1\], sum = 3 choose index 1
\[1, 3, 1\], sum = 5 choose index 2
\[1, 3, 5\], sum = 9 choose index 0
\[9, 3, 5\] Done
**Example 2:**
**Input:** target = \[1,1,1,2\]
**Output:** false
**Explanation:** Impossible to create target array from \[1,1,1,1\].
**Example 3:**
**Input:** target = \[8,5\]
**Output:** true
**Constraints:**
* `n == target.length`
* `1 <= n <= 5 * 104`
* `1 <= target[i] <= 109` | Count the number of times a player loses while iterating through the matches. |
Python one liner (maybe some fooling around involved :P) | find-players-with-zero-or-one-losses | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFirst I tried to solve it with a hashmap to count the winners and loosers. Later I tried to solve it with a one-liner, which is actually not as readable as my original solution, but I want to post it anyway. :)\n\nHere are some explanations:\n\nFirst part "Zero losses"\n```\nlist(zip(*matches))\n```\nlist(zip(*matches)) converts the list into the form [["winner", "winner", ...], ["looser", "looser", ...]]\n\n```\nset(list(zip(*matches))[0])\n```\nconverts the winners list into a set\n\n```\nset(list(zip(*matches))[1])\n```\nconverts the loosers list into a set\n\n```\nset(list(zip(*matches))[0]) - set(list(zip(*matches))[1])\n```\nRemoves the players that are also in the loosers set from the winners set.\n\n```\nsorted(set(list(zip(*matches))[0]) - set(list(zip(*matches))[1]))\n\n```\nThe sorted method also takes care of converting it into a list and sorts the values.\n\nSecond part "One loss"\n\n```\nnumpy.unique(list(zip(*matches))[1], return_counts=True)\n```\nnumpy unique converts it basically to a set, but due to the return_counts flag set to true it will also return how often it was in the original list. For the second part it should only add the players to the result where the count is 1.\n\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nI guess it is: O(n) * O(n*log(n))\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nI consume extra space, because I don\'t save some of the results in variables, due to trying to solve it in one line.\n\n# Code\n```\nimport numpy\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n return sorted(set(list(zip(*matches))[0]) - set(list(zip(*matches))[1])), sorted([l[0] for l in zip(*numpy.unique(list(zip(*matches))[1], return_counts=True)) if l[1] == 1])\n``` | 1 | You are given an integer array `matches` where `matches[i] = [winneri, loseri]` indicates that the player `winneri` defeated player `loseri` in a match.
Return _a list_ `answer` _of size_ `2` _where:_
* `answer[0]` is a list of all players that have **not** lost any matches.
* `answer[1]` is a list of all players that have lost exactly **one** match.
The values in the two lists should be returned in **increasing** order.
**Note:**
* You should only consider the players that have played **at least one** match.
* The testcases will be generated such that **no** two matches will have the **same** outcome.
**Example 1:**
**Input:** matches = \[\[1,3\],\[2,3\],\[3,6\],\[5,6\],\[5,7\],\[4,5\],\[4,8\],\[4,9\],\[10,4\],\[10,9\]\]
**Output:** \[\[1,2,10\],\[4,5,7,8\]\]
**Explanation:**
Players 1, 2, and 10 have not lost any matches.
Players 4, 5, 7, and 8 each have lost one match.
Players 3, 6, and 9 each have lost two matches.
Thus, answer\[0\] = \[1,2,10\] and answer\[1\] = \[4,5,7,8\].
**Example 2:**
**Input:** matches = \[\[2,3\],\[1,3\],\[5,4\],\[6,4\]\]
**Output:** \[\[1,2,5,6\],\[\]\]
**Explanation:**
Players 1, 2, 5, and 6 have not lost any matches.
Players 3 and 4 each have lost two matches.
Thus, answer\[0\] = \[1,2,5,6\] and answer\[1\] = \[\].
**Constraints:**
* `1 <= matches.length <= 105`
* `matches[i].length == 2`
* `1 <= winneri, loseri <= 105`
* `winneri != loseri`
* All `matches[i]` are **unique**. | In the string "abacad", the letter "a" appears 3 times. How many substrings begin with the first "a" and end with any "a"? There are 3 substrings ("a", "aba", and "abaca"). How many substrings begin with the second "a" and end with any "a"? How about the third? 2 substrings begin with the second "a" ("a", and "aca") and 1 substring begins with the third "a" ("a"). There is a total of 3 + 2 + 1 = 6 substrings that begin and end with "a". If a character appears i times in the string, there are i * (i + 1) / 2 substrings that begin and end with that character. |
Python one liner (maybe some fooling around involved :P) | find-players-with-zero-or-one-losses | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFirst I tried to solve it with a hashmap to count the winners and loosers. Later I tried to solve it with a one-liner, which is actually not as readable as my original solution, but I want to post it anyway. :)\n\nHere are some explanations:\n\nFirst part "Zero losses"\n```\nlist(zip(*matches))\n```\nlist(zip(*matches)) converts the list into the form [["winner", "winner", ...], ["looser", "looser", ...]]\n\n```\nset(list(zip(*matches))[0])\n```\nconverts the winners list into a set\n\n```\nset(list(zip(*matches))[1])\n```\nconverts the loosers list into a set\n\n```\nset(list(zip(*matches))[0]) - set(list(zip(*matches))[1])\n```\nRemoves the players that are also in the loosers set from the winners set.\n\n```\nsorted(set(list(zip(*matches))[0]) - set(list(zip(*matches))[1]))\n\n```\nThe sorted method also takes care of converting it into a list and sorts the values.\n\nSecond part "One loss"\n\n```\nnumpy.unique(list(zip(*matches))[1], return_counts=True)\n```\nnumpy unique converts it basically to a set, but due to the return_counts flag set to true it will also return how often it was in the original list. For the second part it should only add the players to the result where the count is 1.\n\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nI guess it is: O(n) * O(n*log(n))\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nI consume extra space, because I don\'t save some of the results in variables, due to trying to solve it in one line.\n\n# Code\n```\nimport numpy\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n return sorted(set(list(zip(*matches))[0]) - set(list(zip(*matches))[1])), sorted([l[0] for l in zip(*numpy.unique(list(zip(*matches))[1], return_counts=True)) if l[1] == 1])\n``` | 1 | You are given an array `target` of n integers. From a starting array `arr` consisting of `n` 1's, you may perform the following procedure :
* let `x` be the sum of all elements currently in your array.
* choose index `i`, such that `0 <= i < n` and set the value of `arr` at index `i` to `x`.
* You may repeat this procedure as many times as needed.
Return `true` _if it is possible to construct the_ `target` _array from_ `arr`_, otherwise, return_ `false`.
**Example 1:**
**Input:** target = \[9,3,5\]
**Output:** true
**Explanation:** Start with arr = \[1, 1, 1\]
\[1, 1, 1\], sum = 3 choose index 1
\[1, 3, 1\], sum = 5 choose index 2
\[1, 3, 5\], sum = 9 choose index 0
\[9, 3, 5\] Done
**Example 2:**
**Input:** target = \[1,1,1,2\]
**Output:** false
**Explanation:** Impossible to create target array from \[1,1,1,1\].
**Example 3:**
**Input:** target = \[8,5\]
**Output:** true
**Constraints:**
* `n == target.length`
* `1 <= n <= 5 * 104`
* `1 <= target[i] <= 109` | Count the number of times a player loses while iterating through the matches. |
PYTHON | Hashmap and Set | find-players-with-zero-or-one-losses | 0 | 1 | Keep the loosers loosing count in hashmap , if player belongs to looser set then player must have lost exactly one match if not remove the player from looser set ( maybe lost 2 or more matches ) , if player belongs to winner set he will mot be in hashmap so add him to winner set if adding any looser in winner set remove him fron winner \n\t\n\t\n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n \n lookup = defaultdict(int)\n winners = set()\n loosers = set()\n \n for win, loss in matches:\n lookup[loss] += 1\n if win not in lookup:\n winners.add(win)\n \n if loss in winners:\n winners.remove(loss)\n \n if lookup[loss] == 1:\n loosers.add(loss)\n else:\n if loss in loosers:\n loosers.remove(loss)\n \n w = sorted(list(winners))\n l = sorted(list(loosers))\n \n \xA0 \xA0 \xA0 \xA0return [w, l] \n``` | 1 | You are given an integer array `matches` where `matches[i] = [winneri, loseri]` indicates that the player `winneri` defeated player `loseri` in a match.
Return _a list_ `answer` _of size_ `2` _where:_
* `answer[0]` is a list of all players that have **not** lost any matches.
* `answer[1]` is a list of all players that have lost exactly **one** match.
The values in the two lists should be returned in **increasing** order.
**Note:**
* You should only consider the players that have played **at least one** match.
* The testcases will be generated such that **no** two matches will have the **same** outcome.
**Example 1:**
**Input:** matches = \[\[1,3\],\[2,3\],\[3,6\],\[5,6\],\[5,7\],\[4,5\],\[4,8\],\[4,9\],\[10,4\],\[10,9\]\]
**Output:** \[\[1,2,10\],\[4,5,7,8\]\]
**Explanation:**
Players 1, 2, and 10 have not lost any matches.
Players 4, 5, 7, and 8 each have lost one match.
Players 3, 6, and 9 each have lost two matches.
Thus, answer\[0\] = \[1,2,10\] and answer\[1\] = \[4,5,7,8\].
**Example 2:**
**Input:** matches = \[\[2,3\],\[1,3\],\[5,4\],\[6,4\]\]
**Output:** \[\[1,2,5,6\],\[\]\]
**Explanation:**
Players 1, 2, 5, and 6 have not lost any matches.
Players 3 and 4 each have lost two matches.
Thus, answer\[0\] = \[1,2,5,6\] and answer\[1\] = \[\].
**Constraints:**
* `1 <= matches.length <= 105`
* `matches[i].length == 2`
* `1 <= winneri, loseri <= 105`
* `winneri != loseri`
* All `matches[i]` are **unique**. | In the string "abacad", the letter "a" appears 3 times. How many substrings begin with the first "a" and end with any "a"? There are 3 substrings ("a", "aba", and "abaca"). How many substrings begin with the second "a" and end with any "a"? How about the third? 2 substrings begin with the second "a" ("a", and "aca") and 1 substring begins with the third "a" ("a"). There is a total of 3 + 2 + 1 = 6 substrings that begin and end with "a". If a character appears i times in the string, there are i * (i + 1) / 2 substrings that begin and end with that character. |
PYTHON | Hashmap and Set | find-players-with-zero-or-one-losses | 0 | 1 | Keep the loosers loosing count in hashmap , if player belongs to looser set then player must have lost exactly one match if not remove the player from looser set ( maybe lost 2 or more matches ) , if player belongs to winner set he will mot be in hashmap so add him to winner set if adding any looser in winner set remove him fron winner \n\t\n\t\n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n \n lookup = defaultdict(int)\n winners = set()\n loosers = set()\n \n for win, loss in matches:\n lookup[loss] += 1\n if win not in lookup:\n winners.add(win)\n \n if loss in winners:\n winners.remove(loss)\n \n if lookup[loss] == 1:\n loosers.add(loss)\n else:\n if loss in loosers:\n loosers.remove(loss)\n \n w = sorted(list(winners))\n l = sorted(list(loosers))\n \n \xA0 \xA0 \xA0 \xA0return [w, l] \n``` | 1 | You are given an array `target` of n integers. From a starting array `arr` consisting of `n` 1's, you may perform the following procedure :
* let `x` be the sum of all elements currently in your array.
* choose index `i`, such that `0 <= i < n` and set the value of `arr` at index `i` to `x`.
* You may repeat this procedure as many times as needed.
Return `true` _if it is possible to construct the_ `target` _array from_ `arr`_, otherwise, return_ `false`.
**Example 1:**
**Input:** target = \[9,3,5\]
**Output:** true
**Explanation:** Start with arr = \[1, 1, 1\]
\[1, 1, 1\], sum = 3 choose index 1
\[1, 3, 1\], sum = 5 choose index 2
\[1, 3, 5\], sum = 9 choose index 0
\[9, 3, 5\] Done
**Example 2:**
**Input:** target = \[1,1,1,2\]
**Output:** false
**Explanation:** Impossible to create target array from \[1,1,1,1\].
**Example 3:**
**Input:** target = \[8,5\]
**Output:** true
**Constraints:**
* `n == target.length`
* `1 <= n <= 5 * 104`
* `1 <= target[i] <= 109` | Count the number of times a player loses while iterating through the matches. |
Identifying Players by Match Outcomes | find-players-with-zero-or-one-losses | 0 | 1 | # Intuition\nThe goal of this code is to find the players who have lost lost once or less in a given list of matches.\n\n# Approach\nThe approach taken by the code is as follows:\n\n1. Initialize a dictionary `loss` to store the number of losses for each player, and an empty list `res` to store the players who have won or lost only once.\n2. Iterate over the matches and update the loss count for each player.\n3. Iterate over all possible players and, for each player that has a loss count in the loss dictionary and a loss count of at most 1, append the player to the appropriate sublist of `res`.\n4. Return `res`.\n\n## Time Complexity\nThe time complexity of this code is O(n), where n is the maximum player number in the given list of matches. This is because we need to iterate over all possible players and check their loss count in the loss dictionary.\n\n## Space Complexity\nThe space complexity of this code is O(n), where n is the maximum player number in the given list of matches. This is because we need to store the loss dictionary with at most n elements and the res list with at most n elements.\n\n\n\n# Code\n```python []\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n loss, res = {}, [[], []]\n for winner, loser in matches:\n loss[winner] = loss.get(winner, 0)\n loss[loser] = loss.get(loser, 0) + 1\n for player in range(max(chain(*matches)) + 1):\n if player in loss and loss[player] <= 1:\n res[loss[player]].append(player)\n return res\n``` | 1 | You are given an integer array `matches` where `matches[i] = [winneri, loseri]` indicates that the player `winneri` defeated player `loseri` in a match.
Return _a list_ `answer` _of size_ `2` _where:_
* `answer[0]` is a list of all players that have **not** lost any matches.
* `answer[1]` is a list of all players that have lost exactly **one** match.
The values in the two lists should be returned in **increasing** order.
**Note:**
* You should only consider the players that have played **at least one** match.
* The testcases will be generated such that **no** two matches will have the **same** outcome.
**Example 1:**
**Input:** matches = \[\[1,3\],\[2,3\],\[3,6\],\[5,6\],\[5,7\],\[4,5\],\[4,8\],\[4,9\],\[10,4\],\[10,9\]\]
**Output:** \[\[1,2,10\],\[4,5,7,8\]\]
**Explanation:**
Players 1, 2, and 10 have not lost any matches.
Players 4, 5, 7, and 8 each have lost one match.
Players 3, 6, and 9 each have lost two matches.
Thus, answer\[0\] = \[1,2,10\] and answer\[1\] = \[4,5,7,8\].
**Example 2:**
**Input:** matches = \[\[2,3\],\[1,3\],\[5,4\],\[6,4\]\]
**Output:** \[\[1,2,5,6\],\[\]\]
**Explanation:**
Players 1, 2, 5, and 6 have not lost any matches.
Players 3 and 4 each have lost two matches.
Thus, answer\[0\] = \[1,2,5,6\] and answer\[1\] = \[\].
**Constraints:**
* `1 <= matches.length <= 105`
* `matches[i].length == 2`
* `1 <= winneri, loseri <= 105`
* `winneri != loseri`
* All `matches[i]` are **unique**. | In the string "abacad", the letter "a" appears 3 times. How many substrings begin with the first "a" and end with any "a"? There are 3 substrings ("a", "aba", and "abaca"). How many substrings begin with the second "a" and end with any "a"? How about the third? 2 substrings begin with the second "a" ("a", and "aca") and 1 substring begins with the third "a" ("a"). There is a total of 3 + 2 + 1 = 6 substrings that begin and end with "a". If a character appears i times in the string, there are i * (i + 1) / 2 substrings that begin and end with that character. |
Identifying Players by Match Outcomes | find-players-with-zero-or-one-losses | 0 | 1 | # Intuition\nThe goal of this code is to find the players who have lost lost once or less in a given list of matches.\n\n# Approach\nThe approach taken by the code is as follows:\n\n1. Initialize a dictionary `loss` to store the number of losses for each player, and an empty list `res` to store the players who have won or lost only once.\n2. Iterate over the matches and update the loss count for each player.\n3. Iterate over all possible players and, for each player that has a loss count in the loss dictionary and a loss count of at most 1, append the player to the appropriate sublist of `res`.\n4. Return `res`.\n\n## Time Complexity\nThe time complexity of this code is O(n), where n is the maximum player number in the given list of matches. This is because we need to iterate over all possible players and check their loss count in the loss dictionary.\n\n## Space Complexity\nThe space complexity of this code is O(n), where n is the maximum player number in the given list of matches. This is because we need to store the loss dictionary with at most n elements and the res list with at most n elements.\n\n\n\n# Code\n```python []\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n loss, res = {}, [[], []]\n for winner, loser in matches:\n loss[winner] = loss.get(winner, 0)\n loss[loser] = loss.get(loser, 0) + 1\n for player in range(max(chain(*matches)) + 1):\n if player in loss and loss[player] <= 1:\n res[loss[player]].append(player)\n return res\n``` | 1 | You are given an array `target` of n integers. From a starting array `arr` consisting of `n` 1's, you may perform the following procedure :
* let `x` be the sum of all elements currently in your array.
* choose index `i`, such that `0 <= i < n` and set the value of `arr` at index `i` to `x`.
* You may repeat this procedure as many times as needed.
Return `true` _if it is possible to construct the_ `target` _array from_ `arr`_, otherwise, return_ `false`.
**Example 1:**
**Input:** target = \[9,3,5\]
**Output:** true
**Explanation:** Start with arr = \[1, 1, 1\]
\[1, 1, 1\], sum = 3 choose index 1
\[1, 3, 1\], sum = 5 choose index 2
\[1, 3, 5\], sum = 9 choose index 0
\[9, 3, 5\] Done
**Example 2:**
**Input:** target = \[1,1,1,2\]
**Output:** false
**Explanation:** Impossible to create target array from \[1,1,1,1\].
**Example 3:**
**Input:** target = \[8,5\]
**Output:** true
**Constraints:**
* `n == target.length`
* `1 <= n <= 5 * 104`
* `1 <= target[i] <= 109` | Count the number of times a player loses while iterating through the matches. |
✔Python3 | Dictionary | Hashmap | Concise | easy-understanding 🔥 | find-players-with-zero-or-one-losses | 0 | 1 | # Approach\n* Traverse the array and store the loss count in a Dictionary for each player\n* Traverse the dictionary and store the players with Zero value in one list and One value in another list\n* Sort these lists and put them in a single list to return the expected answer\n# Code\n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n lossTable = dict()\n noLoss=[]\n oneLoss=[]\n for i in matches:\n if i[0] not in lossTable:\n lossTable[i[0]]=0\n if i[1] not in lossTable:\n lossTable[i[1]]=0\n lossTable[i[1]]+=1 #increment the loss\n for i in lossTable:\n if lossTable[i]==0:\n noLoss.append(i)\n if lossTable[i]==1:\n oneLoss.append(i)\n #print(lossTable)\n #print(noLoss,oneLoss)\n res=[sorted(noLoss),sorted(oneLoss)]\n return res\n``` | 1 | You are given an integer array `matches` where `matches[i] = [winneri, loseri]` indicates that the player `winneri` defeated player `loseri` in a match.
Return _a list_ `answer` _of size_ `2` _where:_
* `answer[0]` is a list of all players that have **not** lost any matches.
* `answer[1]` is a list of all players that have lost exactly **one** match.
The values in the two lists should be returned in **increasing** order.
**Note:**
* You should only consider the players that have played **at least one** match.
* The testcases will be generated such that **no** two matches will have the **same** outcome.
**Example 1:**
**Input:** matches = \[\[1,3\],\[2,3\],\[3,6\],\[5,6\],\[5,7\],\[4,5\],\[4,8\],\[4,9\],\[10,4\],\[10,9\]\]
**Output:** \[\[1,2,10\],\[4,5,7,8\]\]
**Explanation:**
Players 1, 2, and 10 have not lost any matches.
Players 4, 5, 7, and 8 each have lost one match.
Players 3, 6, and 9 each have lost two matches.
Thus, answer\[0\] = \[1,2,10\] and answer\[1\] = \[4,5,7,8\].
**Example 2:**
**Input:** matches = \[\[2,3\],\[1,3\],\[5,4\],\[6,4\]\]
**Output:** \[\[1,2,5,6\],\[\]\]
**Explanation:**
Players 1, 2, 5, and 6 have not lost any matches.
Players 3 and 4 each have lost two matches.
Thus, answer\[0\] = \[1,2,5,6\] and answer\[1\] = \[\].
**Constraints:**
* `1 <= matches.length <= 105`
* `matches[i].length == 2`
* `1 <= winneri, loseri <= 105`
* `winneri != loseri`
* All `matches[i]` are **unique**. | In the string "abacad", the letter "a" appears 3 times. How many substrings begin with the first "a" and end with any "a"? There are 3 substrings ("a", "aba", and "abaca"). How many substrings begin with the second "a" and end with any "a"? How about the third? 2 substrings begin with the second "a" ("a", and "aca") and 1 substring begins with the third "a" ("a"). There is a total of 3 + 2 + 1 = 6 substrings that begin and end with "a". If a character appears i times in the string, there are i * (i + 1) / 2 substrings that begin and end with that character. |
✔Python3 | Dictionary | Hashmap | Concise | easy-understanding 🔥 | find-players-with-zero-or-one-losses | 0 | 1 | # Approach\n* Traverse the array and store the loss count in a Dictionary for each player\n* Traverse the dictionary and store the players with Zero value in one list and One value in another list\n* Sort these lists and put them in a single list to return the expected answer\n# Code\n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n lossTable = dict()\n noLoss=[]\n oneLoss=[]\n for i in matches:\n if i[0] not in lossTable:\n lossTable[i[0]]=0\n if i[1] not in lossTable:\n lossTable[i[1]]=0\n lossTable[i[1]]+=1 #increment the loss\n for i in lossTable:\n if lossTable[i]==0:\n noLoss.append(i)\n if lossTable[i]==1:\n oneLoss.append(i)\n #print(lossTable)\n #print(noLoss,oneLoss)\n res=[sorted(noLoss),sorted(oneLoss)]\n return res\n``` | 1 | You are given an array `target` of n integers. From a starting array `arr` consisting of `n` 1's, you may perform the following procedure :
* let `x` be the sum of all elements currently in your array.
* choose index `i`, such that `0 <= i < n` and set the value of `arr` at index `i` to `x`.
* You may repeat this procedure as many times as needed.
Return `true` _if it is possible to construct the_ `target` _array from_ `arr`_, otherwise, return_ `false`.
**Example 1:**
**Input:** target = \[9,3,5\]
**Output:** true
**Explanation:** Start with arr = \[1, 1, 1\]
\[1, 1, 1\], sum = 3 choose index 1
\[1, 3, 1\], sum = 5 choose index 2
\[1, 3, 5\], sum = 9 choose index 0
\[9, 3, 5\] Done
**Example 2:**
**Input:** target = \[1,1,1,2\]
**Output:** false
**Explanation:** Impossible to create target array from \[1,1,1,1\].
**Example 3:**
**Input:** target = \[8,5\]
**Output:** true
**Constraints:**
* `n == target.length`
* `1 <= n <= 5 * 104`
* `1 <= target[i] <= 109` | Count the number of times a player loses while iterating through the matches. |
Python using Hashmap | find-players-with-zero-or-one-losses | 0 | 1 | \n# Code\n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n count = {}\n for match in matches:\n if match[0] not in count.keys():\n count[match[0]] = [1]\n else:\n count[match[0]].append(1)\n if match[1] not in count.keys():\n count[match[1]] = [-1]\n else:\n count[match[1]].append(-1)\n not_lost = []\n single_lost = []\n for player in count.keys():\n if -1 not in count[player]:\n not_lost.append(player)\n elif count[player].count(-1)==1:\n single_lost.append(player)\n not_lost.sort()\n single_lost.sort()\n return [not_lost,single_lost]\n \n \n``` | 1 | You are given an integer array `matches` where `matches[i] = [winneri, loseri]` indicates that the player `winneri` defeated player `loseri` in a match.
Return _a list_ `answer` _of size_ `2` _where:_
* `answer[0]` is a list of all players that have **not** lost any matches.
* `answer[1]` is a list of all players that have lost exactly **one** match.
The values in the two lists should be returned in **increasing** order.
**Note:**
* You should only consider the players that have played **at least one** match.
* The testcases will be generated such that **no** two matches will have the **same** outcome.
**Example 1:**
**Input:** matches = \[\[1,3\],\[2,3\],\[3,6\],\[5,6\],\[5,7\],\[4,5\],\[4,8\],\[4,9\],\[10,4\],\[10,9\]\]
**Output:** \[\[1,2,10\],\[4,5,7,8\]\]
**Explanation:**
Players 1, 2, and 10 have not lost any matches.
Players 4, 5, 7, and 8 each have lost one match.
Players 3, 6, and 9 each have lost two matches.
Thus, answer\[0\] = \[1,2,10\] and answer\[1\] = \[4,5,7,8\].
**Example 2:**
**Input:** matches = \[\[2,3\],\[1,3\],\[5,4\],\[6,4\]\]
**Output:** \[\[1,2,5,6\],\[\]\]
**Explanation:**
Players 1, 2, 5, and 6 have not lost any matches.
Players 3 and 4 each have lost two matches.
Thus, answer\[0\] = \[1,2,5,6\] and answer\[1\] = \[\].
**Constraints:**
* `1 <= matches.length <= 105`
* `matches[i].length == 2`
* `1 <= winneri, loseri <= 105`
* `winneri != loseri`
* All `matches[i]` are **unique**. | In the string "abacad", the letter "a" appears 3 times. How many substrings begin with the first "a" and end with any "a"? There are 3 substrings ("a", "aba", and "abaca"). How many substrings begin with the second "a" and end with any "a"? How about the third? 2 substrings begin with the second "a" ("a", and "aca") and 1 substring begins with the third "a" ("a"). There is a total of 3 + 2 + 1 = 6 substrings that begin and end with "a". If a character appears i times in the string, there are i * (i + 1) / 2 substrings that begin and end with that character. |
Python using Hashmap | find-players-with-zero-or-one-losses | 0 | 1 | \n# Code\n```\nclass Solution:\n def findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n count = {}\n for match in matches:\n if match[0] not in count.keys():\n count[match[0]] = [1]\n else:\n count[match[0]].append(1)\n if match[1] not in count.keys():\n count[match[1]] = [-1]\n else:\n count[match[1]].append(-1)\n not_lost = []\n single_lost = []\n for player in count.keys():\n if -1 not in count[player]:\n not_lost.append(player)\n elif count[player].count(-1)==1:\n single_lost.append(player)\n not_lost.sort()\n single_lost.sort()\n return [not_lost,single_lost]\n \n \n``` | 1 | You are given an array `target` of n integers. From a starting array `arr` consisting of `n` 1's, you may perform the following procedure :
* let `x` be the sum of all elements currently in your array.
* choose index `i`, such that `0 <= i < n` and set the value of `arr` at index `i` to `x`.
* You may repeat this procedure as many times as needed.
Return `true` _if it is possible to construct the_ `target` _array from_ `arr`_, otherwise, return_ `false`.
**Example 1:**
**Input:** target = \[9,3,5\]
**Output:** true
**Explanation:** Start with arr = \[1, 1, 1\]
\[1, 1, 1\], sum = 3 choose index 1
\[1, 3, 1\], sum = 5 choose index 2
\[1, 3, 5\], sum = 9 choose index 0
\[9, 3, 5\] Done
**Example 2:**
**Input:** target = \[1,1,1,2\]
**Output:** false
**Explanation:** Impossible to create target array from \[1,1,1,1\].
**Example 3:**
**Input:** target = \[8,5\]
**Output:** true
**Constraints:**
* `n == target.length`
* `1 <= n <= 5 * 104`
* `1 <= target[i] <= 109` | Count the number of times a player loses while iterating through the matches. |
Only using Simple Set Operations | Easy Solution | Python | find-players-with-zero-or-one-losses | 0 | 1 | ```\n#thanks to the legend who gave me an upvote :D, cuz that\'s my first one\nclass Solution(object):\n def findWinners(self, matches):\n """\n :type matches: List[List[int]]\n :rtype: List[List[int]]\n """\n win=set()\n loss=set()\n twicelost=set()\n for i in matches:\n win.add(i[0])\n if i[1] in loss:\n twicelost.add(i[1])\n else:\n loss.add(i[1])\n return [sorted(list(win-loss)),sorted(list(loss-twicelost))]\n``` | 1 | You are given an integer array `matches` where `matches[i] = [winneri, loseri]` indicates that the player `winneri` defeated player `loseri` in a match.
Return _a list_ `answer` _of size_ `2` _where:_
* `answer[0]` is a list of all players that have **not** lost any matches.
* `answer[1]` is a list of all players that have lost exactly **one** match.
The values in the two lists should be returned in **increasing** order.
**Note:**
* You should only consider the players that have played **at least one** match.
* The testcases will be generated such that **no** two matches will have the **same** outcome.
**Example 1:**
**Input:** matches = \[\[1,3\],\[2,3\],\[3,6\],\[5,6\],\[5,7\],\[4,5\],\[4,8\],\[4,9\],\[10,4\],\[10,9\]\]
**Output:** \[\[1,2,10\],\[4,5,7,8\]\]
**Explanation:**
Players 1, 2, and 10 have not lost any matches.
Players 4, 5, 7, and 8 each have lost one match.
Players 3, 6, and 9 each have lost two matches.
Thus, answer\[0\] = \[1,2,10\] and answer\[1\] = \[4,5,7,8\].
**Example 2:**
**Input:** matches = \[\[2,3\],\[1,3\],\[5,4\],\[6,4\]\]
**Output:** \[\[1,2,5,6\],\[\]\]
**Explanation:**
Players 1, 2, 5, and 6 have not lost any matches.
Players 3 and 4 each have lost two matches.
Thus, answer\[0\] = \[1,2,5,6\] and answer\[1\] = \[\].
**Constraints:**
* `1 <= matches.length <= 105`
* `matches[i].length == 2`
* `1 <= winneri, loseri <= 105`
* `winneri != loseri`
* All `matches[i]` are **unique**. | In the string "abacad", the letter "a" appears 3 times. How many substrings begin with the first "a" and end with any "a"? There are 3 substrings ("a", "aba", and "abaca"). How many substrings begin with the second "a" and end with any "a"? How about the third? 2 substrings begin with the second "a" ("a", and "aca") and 1 substring begins with the third "a" ("a"). There is a total of 3 + 2 + 1 = 6 substrings that begin and end with "a". If a character appears i times in the string, there are i * (i + 1) / 2 substrings that begin and end with that character. |
Only using Simple Set Operations | Easy Solution | Python | find-players-with-zero-or-one-losses | 0 | 1 | ```\n#thanks to the legend who gave me an upvote :D, cuz that\'s my first one\nclass Solution(object):\n def findWinners(self, matches):\n """\n :type matches: List[List[int]]\n :rtype: List[List[int]]\n """\n win=set()\n loss=set()\n twicelost=set()\n for i in matches:\n win.add(i[0])\n if i[1] in loss:\n twicelost.add(i[1])\n else:\n loss.add(i[1])\n return [sorted(list(win-loss)),sorted(list(loss-twicelost))]\n``` | 1 | You are given an array `target` of n integers. From a starting array `arr` consisting of `n` 1's, you may perform the following procedure :
* let `x` be the sum of all elements currently in your array.
* choose index `i`, such that `0 <= i < n` and set the value of `arr` at index `i` to `x`.
* You may repeat this procedure as many times as needed.
Return `true` _if it is possible to construct the_ `target` _array from_ `arr`_, otherwise, return_ `false`.
**Example 1:**
**Input:** target = \[9,3,5\]
**Output:** true
**Explanation:** Start with arr = \[1, 1, 1\]
\[1, 1, 1\], sum = 3 choose index 1
\[1, 3, 1\], sum = 5 choose index 2
\[1, 3, 5\], sum = 9 choose index 0
\[9, 3, 5\] Done
**Example 2:**
**Input:** target = \[1,1,1,2\]
**Output:** false
**Explanation:** Impossible to create target array from \[1,1,1,1\].
**Example 3:**
**Input:** target = \[8,5\]
**Output:** true
**Constraints:**
* `n == target.length`
* `1 <= n <= 5 * 104`
* `1 <= target[i] <= 109` | Count the number of times a player loses while iterating through the matches. |
[ Python ] ✅✅ Simple Python Solution Using HashMap | Dictionary 🥳✌👍 | find-players-with-zero-or-one-losses | 0 | 1 | # If You like the Solution, Don\'t Forget To UpVote Me, Please UpVote! \uD83D\uDD3C\uD83D\uDE4F\n# Runtime: 2054 ms, faster than 84.05% of Python3 online submissions for Find Players With Zero or One Losses.\n# Memory Usage: 68.7 MB, less than 77.41% of Python3 online submissions for Find Players With Zero or One Losses.\n\n\tclass Solution:\n\t\tdef findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n\n\t\t\tchance = {}\n\n\t\t\tfor match in matches:\n\n\t\t\t\tp1, p2 = match\n\n\t\t\t\tif p1 not in chance:\n\t\t\t\t\tchance[p1] = [1, 0]\n\t\t\t\telse:\n\t\t\t\t\tchance[p1] = [chance[p1][0] + 1, chance[p1][1]]\n\n\t\t\t\tif p2 not in chance:\n\t\t\t\t\tchance[p2] = [0, 1]\n\t\t\t\telse:\n\t\t\t\t\tchance[p2] = [chance[p2][0], chance[p2][1] + 1]\n\n\t\t\tanswer_zero = []\n\t\t\tanswer_one = []\n\n\t\t\tfor key in sorted(chance):\n\n\t\t\t\tif chance[key][0] != 0 and chance[key][1] == 0:\n\t\t\t\t\tanswer_zero.append(key)\n\n\t\t\t\tif chance[key][1] == 1:\n\t\t\t\t\tanswer_one.append(key)\n\n\t\t\tresult = [answer_zero, answer_one]\n\n\t\t\treturn result\n\n# Thank You \uD83E\uDD73\u270C\uD83D\uDC4D | 1 | You are given an integer array `matches` where `matches[i] = [winneri, loseri]` indicates that the player `winneri` defeated player `loseri` in a match.
Return _a list_ `answer` _of size_ `2` _where:_
* `answer[0]` is a list of all players that have **not** lost any matches.
* `answer[1]` is a list of all players that have lost exactly **one** match.
The values in the two lists should be returned in **increasing** order.
**Note:**
* You should only consider the players that have played **at least one** match.
* The testcases will be generated such that **no** two matches will have the **same** outcome.
**Example 1:**
**Input:** matches = \[\[1,3\],\[2,3\],\[3,6\],\[5,6\],\[5,7\],\[4,5\],\[4,8\],\[4,9\],\[10,4\],\[10,9\]\]
**Output:** \[\[1,2,10\],\[4,5,7,8\]\]
**Explanation:**
Players 1, 2, and 10 have not lost any matches.
Players 4, 5, 7, and 8 each have lost one match.
Players 3, 6, and 9 each have lost two matches.
Thus, answer\[0\] = \[1,2,10\] and answer\[1\] = \[4,5,7,8\].
**Example 2:**
**Input:** matches = \[\[2,3\],\[1,3\],\[5,4\],\[6,4\]\]
**Output:** \[\[1,2,5,6\],\[\]\]
**Explanation:**
Players 1, 2, 5, and 6 have not lost any matches.
Players 3 and 4 each have lost two matches.
Thus, answer\[0\] = \[1,2,5,6\] and answer\[1\] = \[\].
**Constraints:**
* `1 <= matches.length <= 105`
* `matches[i].length == 2`
* `1 <= winneri, loseri <= 105`
* `winneri != loseri`
* All `matches[i]` are **unique**. | In the string "abacad", the letter "a" appears 3 times. How many substrings begin with the first "a" and end with any "a"? There are 3 substrings ("a", "aba", and "abaca"). How many substrings begin with the second "a" and end with any "a"? How about the third? 2 substrings begin with the second "a" ("a", and "aca") and 1 substring begins with the third "a" ("a"). There is a total of 3 + 2 + 1 = 6 substrings that begin and end with "a". If a character appears i times in the string, there are i * (i + 1) / 2 substrings that begin and end with that character. |
[ Python ] ✅✅ Simple Python Solution Using HashMap | Dictionary 🥳✌👍 | find-players-with-zero-or-one-losses | 0 | 1 | # If You like the Solution, Don\'t Forget To UpVote Me, Please UpVote! \uD83D\uDD3C\uD83D\uDE4F\n# Runtime: 2054 ms, faster than 84.05% of Python3 online submissions for Find Players With Zero or One Losses.\n# Memory Usage: 68.7 MB, less than 77.41% of Python3 online submissions for Find Players With Zero or One Losses.\n\n\tclass Solution:\n\t\tdef findWinners(self, matches: List[List[int]]) -> List[List[int]]:\n\n\t\t\tchance = {}\n\n\t\t\tfor match in matches:\n\n\t\t\t\tp1, p2 = match\n\n\t\t\t\tif p1 not in chance:\n\t\t\t\t\tchance[p1] = [1, 0]\n\t\t\t\telse:\n\t\t\t\t\tchance[p1] = [chance[p1][0] + 1, chance[p1][1]]\n\n\t\t\t\tif p2 not in chance:\n\t\t\t\t\tchance[p2] = [0, 1]\n\t\t\t\telse:\n\t\t\t\t\tchance[p2] = [chance[p2][0], chance[p2][1] + 1]\n\n\t\t\tanswer_zero = []\n\t\t\tanswer_one = []\n\n\t\t\tfor key in sorted(chance):\n\n\t\t\t\tif chance[key][0] != 0 and chance[key][1] == 0:\n\t\t\t\t\tanswer_zero.append(key)\n\n\t\t\t\tif chance[key][1] == 1:\n\t\t\t\t\tanswer_one.append(key)\n\n\t\t\tresult = [answer_zero, answer_one]\n\n\t\t\treturn result\n\n# Thank You \uD83E\uDD73\u270C\uD83D\uDC4D | 1 | You are given an array `target` of n integers. From a starting array `arr` consisting of `n` 1's, you may perform the following procedure :
* let `x` be the sum of all elements currently in your array.
* choose index `i`, such that `0 <= i < n` and set the value of `arr` at index `i` to `x`.
* You may repeat this procedure as many times as needed.
Return `true` _if it is possible to construct the_ `target` _array from_ `arr`_, otherwise, return_ `false`.
**Example 1:**
**Input:** target = \[9,3,5\]
**Output:** true
**Explanation:** Start with arr = \[1, 1, 1\]
\[1, 1, 1\], sum = 3 choose index 1
\[1, 3, 1\], sum = 5 choose index 2
\[1, 3, 5\], sum = 9 choose index 0
\[9, 3, 5\] Done
**Example 2:**
**Input:** target = \[1,1,1,2\]
**Output:** false
**Explanation:** Impossible to create target array from \[1,1,1,1\].
**Example 3:**
**Input:** target = \[8,5\]
**Output:** true
**Constraints:**
* `n == target.length`
* `1 <= n <= 5 * 104`
* `1 <= target[i] <= 109` | Count the number of times a player loses while iterating through the matches. |
[Python] Hashmap & Counter Solution with Detailed Explanations, Very Clean & Concise | encrypt-and-decrypt-strings | 0 | 1 | This is the first time I get AK over the past few months. I would like to share my solution with the LC community.\n\n**Intuition**\nThe given constraints are not large, we can pre-compute all necessary quantities only once.\n\n\n**Explanation**\nWe can pre-compute two hashmaps as follows:\n\n(1) The first hashmap `self.hashmap` is a encryption map - we map each key with their encrypted values, which is straightforward.\n(2) The second hashmap `self.dictmap` is a `Counter` - we encrypt each word in the given `dictionary` and use the encrypted string as the key and increase the counter by 1. As such, we have solved the duplication problem of the `decrypt()` method.\n\n**Complexity**\nTime in `__init__()`: `O(sum(dictionary[i].length))`, which gets amortized over later function calls\nSpace in `__init__()`: `O(dictionary.length)`\nTime in `encrypt()`: `O(word1.length)`\nSpace in `encrypt()`: `O(1)` (if not counting the `output` string)\nTime in `decrypt()`: `O(1)`\nSpace in `decrypt()`: `O(1)`\n\n\nBelow is my in-contest solution. Please upvote if you find this solution helpful. Thanks!\n```\nclass Encrypter:\n\n def __init__(self, keys: List[str], values: List[str], dictionary: List[str]):\n self.hashmap = dict()\n for i in range(len(keys)):\n self.hashmap[keys[i]] = values[i]\n self.dictmap = defaultdict(int)\n for word in dictionary:\n self.dictmap[self.encrypt(word)] += 1\n\n def encrypt(self, word1: str) -> str:\n output = \'\'\n for char in word1:\n output += self.hashmap[char]\n return output\n\n def decrypt(self, word2: str) -> int:\n return self.dictmap[word2]\n```\n\n**Follow up** (for self-learning purpose): The `encrypt()` method can be written as follows to handle more general test cases (given in the Comments section).\n```\ndef encrypt(self, word1: str) -> str:\n output = \'\'\n for char in word1:\n if char in self.hashmap:\n output += self.hashmap[char]\n else:\n return \'\'\n return output\n``` | 26 | You are given a character array `keys` containing **unique** characters and a string array `values` containing strings of length 2. You are also given another string array `dictionary` that contains all permitted original strings after decryption. You should implement a data structure that can encrypt or decrypt a **0-indexed** string.
A string is **encrypted** with the following process:
1. For each character `c` in the string, we find the index `i` satisfying `keys[i] == c` in `keys`.
2. Replace `c` with `values[i]` in the string.
Note that in case a character of the string is **not present** in `keys`, the encryption process cannot be carried out, and an empty string `" "` is returned.
A string is **decrypted** with the following process:
1. For each substring `s` of length 2 occurring at an even index in the string, we find an `i` such that `values[i] == s`. If there are multiple valid `i`, we choose **any** one of them. This means a string could have multiple possible strings it can decrypt to.
2. Replace `s` with `keys[i]` in the string.
Implement the `Encrypter` class:
* `Encrypter(char[] keys, String[] values, String[] dictionary)` Initializes the `Encrypter` class with `keys, values`, and `dictionary`.
* `String encrypt(String word1)` Encrypts `word1` with the encryption process described above and returns the encrypted string.
* `int decrypt(String word2)` Returns the number of possible strings `word2` could decrypt to that also appear in `dictionary`.
**Example 1:**
**Input**
\[ "Encrypter ", "encrypt ", "decrypt "\]
\[\[\['a', 'b', 'c', 'd'\], \[ "ei ", "zf ", "ei ", "am "\], \[ "abcd ", "acbd ", "adbc ", "badc ", "dacb ", "cadb ", "cbda ", "abad "\]\], \[ "abcd "\], \[ "eizfeiam "\]\]
**Output**
\[null, "eizfeiam ", 2\]
**Explanation**
Encrypter encrypter = new Encrypter(\[\['a', 'b', 'c', 'd'\], \[ "ei ", "zf ", "ei ", "am "\], \[ "abcd ", "acbd ", "adbc ", "badc ", "dacb ", "cadb ", "cbda ", "abad "\]);
encrypter.encrypt( "abcd "); // return "eizfeiam ".
// 'a' maps to "ei ", 'b' maps to "zf ", 'c' maps to "ei ", and 'd' maps to "am ".
encrypter.decrypt( "eizfeiam "); // return 2.
// "ei " can map to 'a' or 'c', "zf " maps to 'b', and "am " maps to 'd'.
// Thus, the possible strings after decryption are "abad ", "cbad ", "abcd ", and "cbcd ".
// 2 of those strings, "abad " and "abcd ", appear in dictionary, so the answer is 2.
**Constraints:**
* `1 <= keys.length == values.length <= 26`
* `values[i].length == 2`
* `1 <= dictionary.length <= 100`
* `1 <= dictionary[i].length <= 100`
* All `keys[i]` and `dictionary[i]` are **unique**.
* `1 <= word1.length <= 2000`
* `1 <= word2.length <= 200`
* All `word1[i]` appear in `keys`.
* `word2.length` is even.
* `keys`, `values[i]`, `dictionary[i]`, `word1`, and `word2` only contain lowercase English letters.
* At most `200` calls will be made to `encrypt` and `decrypt` **in total**. | Can you get the max/min of a certain subarray by using the max/min of a smaller subarray within it? Notice that the max of the subarray from index i to j is equal to max of (max of the subarray from index i to j-1) and nums[j]. |
[Python] Hashmap & Counter Solution with Detailed Explanations, Very Clean & Concise | encrypt-and-decrypt-strings | 0 | 1 | This is the first time I get AK over the past few months. I would like to share my solution with the LC community.\n\n**Intuition**\nThe given constraints are not large, we can pre-compute all necessary quantities only once.\n\n\n**Explanation**\nWe can pre-compute two hashmaps as follows:\n\n(1) The first hashmap `self.hashmap` is a encryption map - we map each key with their encrypted values, which is straightforward.\n(2) The second hashmap `self.dictmap` is a `Counter` - we encrypt each word in the given `dictionary` and use the encrypted string as the key and increase the counter by 1. As such, we have solved the duplication problem of the `decrypt()` method.\n\n**Complexity**\nTime in `__init__()`: `O(sum(dictionary[i].length))`, which gets amortized over later function calls\nSpace in `__init__()`: `O(dictionary.length)`\nTime in `encrypt()`: `O(word1.length)`\nSpace in `encrypt()`: `O(1)` (if not counting the `output` string)\nTime in `decrypt()`: `O(1)`\nSpace in `decrypt()`: `O(1)`\n\n\nBelow is my in-contest solution. Please upvote if you find this solution helpful. Thanks!\n```\nclass Encrypter:\n\n def __init__(self, keys: List[str], values: List[str], dictionary: List[str]):\n self.hashmap = dict()\n for i in range(len(keys)):\n self.hashmap[keys[i]] = values[i]\n self.dictmap = defaultdict(int)\n for word in dictionary:\n self.dictmap[self.encrypt(word)] += 1\n\n def encrypt(self, word1: str) -> str:\n output = \'\'\n for char in word1:\n output += self.hashmap[char]\n return output\n\n def decrypt(self, word2: str) -> int:\n return self.dictmap[word2]\n```\n\n**Follow up** (for self-learning purpose): The `encrypt()` method can be written as follows to handle more general test cases (given in the Comments section).\n```\ndef encrypt(self, word1: str) -> str:\n output = \'\'\n for char in word1:\n if char in self.hashmap:\n output += self.hashmap[char]\n else:\n return \'\'\n return output\n``` | 26 | Given two strings: `s1` and `s2` with the same size, check if some permutation of string `s1` can break some permutation of string `s2` or vice-versa. In other words `s2` can break `s1` or vice-versa.
A string `x` can break string `y` (both of size `n`) if `x[i] >= y[i]` (in alphabetical order) for all `i` between `0` and `n-1`.
**Example 1:**
**Input:** s1 = "abc ", s2 = "xya "
**Output:** true
**Explanation:** "ayx " is a permutation of s2= "xya " which can break to string "abc " which is a permutation of s1= "abc ".
**Example 2:**
**Input:** s1 = "abe ", s2 = "acd "
**Output:** false
**Explanation:** All permutations for s1= "abe " are: "abe ", "aeb ", "bae ", "bea ", "eab " and "eba " and all permutation for s2= "acd " are: "acd ", "adc ", "cad ", "cda ", "dac " and "dca ". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
**Example 3:**
**Input:** s1 = "leetcodee ", s2 = "interview "
**Output:** true
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `1 <= n <= 10^5`
* All strings consist of lowercase English letters. | For encryption, use hashmap to map each char of word1 to its value. For decryption, use trie to prune when necessary. |
Two Hashmaps, pay attention when a letter is not present in keys | encrypt-and-decrypt-strings | 0 | 1 | I gotta say this is not a very clean and hard enough hard question\n# Code\n```\nclass Encrypter:\n\n def __init__(self, keys: List[str], values: List[str], dictionary: List[str]):\n self.reflection = {k:v for k,v in zip(keys,values)}\n for a in \'abcdefghijklmnopqrstuvwxyz\':\n if a not in self.reflection:\n self.reflection[a] = \'#\'\n self.newdic = {word:\'\'.join(self.reflection[a] for a in word) for word in dictionary}\n for d in self.newdic:\n if \'#\' in self.newdic[d]: self.newdic[d] = \'\'\n self.antinewdic = {}\n for word in self.newdic:\n if self.newdic[word] not in self.antinewdic:\n self.antinewdic[self.newdic[word]] = 0\n self.antinewdic[self.newdic[word]] += 1\n \n\n def encrypt(self, word1: str) -> str:\n if word1 in self.newdic: return self.newdic[word1]\n res = \'\'.join(self.reflection[a] for a in word1)\n if \'#\' in res: return \'\'\n else: return res\n\n def decrypt(self, word2: str) -> int:\n if word2 not in self.antinewdic: return 0\n return self.antinewdic[word2]\n \n \n\n\n# Your Encrypter object will be instantiated and called as such:\n# obj = Encrypter(keys, values, dictionary)\n# param_1 = obj.encrypt(word1)\n# param_2 = obj.decrypt(word2)\n``` | 0 | You are given a character array `keys` containing **unique** characters and a string array `values` containing strings of length 2. You are also given another string array `dictionary` that contains all permitted original strings after decryption. You should implement a data structure that can encrypt or decrypt a **0-indexed** string.
A string is **encrypted** with the following process:
1. For each character `c` in the string, we find the index `i` satisfying `keys[i] == c` in `keys`.
2. Replace `c` with `values[i]` in the string.
Note that in case a character of the string is **not present** in `keys`, the encryption process cannot be carried out, and an empty string `" "` is returned.
A string is **decrypted** with the following process:
1. For each substring `s` of length 2 occurring at an even index in the string, we find an `i` such that `values[i] == s`. If there are multiple valid `i`, we choose **any** one of them. This means a string could have multiple possible strings it can decrypt to.
2. Replace `s` with `keys[i]` in the string.
Implement the `Encrypter` class:
* `Encrypter(char[] keys, String[] values, String[] dictionary)` Initializes the `Encrypter` class with `keys, values`, and `dictionary`.
* `String encrypt(String word1)` Encrypts `word1` with the encryption process described above and returns the encrypted string.
* `int decrypt(String word2)` Returns the number of possible strings `word2` could decrypt to that also appear in `dictionary`.
**Example 1:**
**Input**
\[ "Encrypter ", "encrypt ", "decrypt "\]
\[\[\['a', 'b', 'c', 'd'\], \[ "ei ", "zf ", "ei ", "am "\], \[ "abcd ", "acbd ", "adbc ", "badc ", "dacb ", "cadb ", "cbda ", "abad "\]\], \[ "abcd "\], \[ "eizfeiam "\]\]
**Output**
\[null, "eizfeiam ", 2\]
**Explanation**
Encrypter encrypter = new Encrypter(\[\['a', 'b', 'c', 'd'\], \[ "ei ", "zf ", "ei ", "am "\], \[ "abcd ", "acbd ", "adbc ", "badc ", "dacb ", "cadb ", "cbda ", "abad "\]);
encrypter.encrypt( "abcd "); // return "eizfeiam ".
// 'a' maps to "ei ", 'b' maps to "zf ", 'c' maps to "ei ", and 'd' maps to "am ".
encrypter.decrypt( "eizfeiam "); // return 2.
// "ei " can map to 'a' or 'c', "zf " maps to 'b', and "am " maps to 'd'.
// Thus, the possible strings after decryption are "abad ", "cbad ", "abcd ", and "cbcd ".
// 2 of those strings, "abad " and "abcd ", appear in dictionary, so the answer is 2.
**Constraints:**
* `1 <= keys.length == values.length <= 26`
* `values[i].length == 2`
* `1 <= dictionary.length <= 100`
* `1 <= dictionary[i].length <= 100`
* All `keys[i]` and `dictionary[i]` are **unique**.
* `1 <= word1.length <= 2000`
* `1 <= word2.length <= 200`
* All `word1[i]` appear in `keys`.
* `word2.length` is even.
* `keys`, `values[i]`, `dictionary[i]`, `word1`, and `word2` only contain lowercase English letters.
* At most `200` calls will be made to `encrypt` and `decrypt` **in total**. | Can you get the max/min of a certain subarray by using the max/min of a smaller subarray within it? Notice that the max of the subarray from index i to j is equal to max of (max of the subarray from index i to j-1) and nums[j]. |
Two Hashmaps, pay attention when a letter is not present in keys | encrypt-and-decrypt-strings | 0 | 1 | I gotta say this is not a very clean and hard enough hard question\n# Code\n```\nclass Encrypter:\n\n def __init__(self, keys: List[str], values: List[str], dictionary: List[str]):\n self.reflection = {k:v for k,v in zip(keys,values)}\n for a in \'abcdefghijklmnopqrstuvwxyz\':\n if a not in self.reflection:\n self.reflection[a] = \'#\'\n self.newdic = {word:\'\'.join(self.reflection[a] for a in word) for word in dictionary}\n for d in self.newdic:\n if \'#\' in self.newdic[d]: self.newdic[d] = \'\'\n self.antinewdic = {}\n for word in self.newdic:\n if self.newdic[word] not in self.antinewdic:\n self.antinewdic[self.newdic[word]] = 0\n self.antinewdic[self.newdic[word]] += 1\n \n\n def encrypt(self, word1: str) -> str:\n if word1 in self.newdic: return self.newdic[word1]\n res = \'\'.join(self.reflection[a] for a in word1)\n if \'#\' in res: return \'\'\n else: return res\n\n def decrypt(self, word2: str) -> int:\n if word2 not in self.antinewdic: return 0\n return self.antinewdic[word2]\n \n \n\n\n# Your Encrypter object will be instantiated and called as such:\n# obj = Encrypter(keys, values, dictionary)\n# param_1 = obj.encrypt(word1)\n# param_2 = obj.decrypt(word2)\n``` | 0 | Given two strings: `s1` and `s2` with the same size, check if some permutation of string `s1` can break some permutation of string `s2` or vice-versa. In other words `s2` can break `s1` or vice-versa.
A string `x` can break string `y` (both of size `n`) if `x[i] >= y[i]` (in alphabetical order) for all `i` between `0` and `n-1`.
**Example 1:**
**Input:** s1 = "abc ", s2 = "xya "
**Output:** true
**Explanation:** "ayx " is a permutation of s2= "xya " which can break to string "abc " which is a permutation of s1= "abc ".
**Example 2:**
**Input:** s1 = "abe ", s2 = "acd "
**Output:** false
**Explanation:** All permutations for s1= "abe " are: "abe ", "aeb ", "bae ", "bea ", "eab " and "eba " and all permutation for s2= "acd " are: "acd ", "adc ", "cad ", "cda ", "dac " and "dca ". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
**Example 3:**
**Input:** s1 = "leetcodee ", s2 = "interview "
**Output:** true
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `1 <= n <= 10^5`
* All strings consist of lowercase English letters. | For encryption, use hashmap to map each char of word1 to its value. For decryption, use trie to prune when necessary. |
Python Simple solution beats 96.92% | encrypt-and-decrypt-strings | 0 | 1 | # Code\n```\nclass Encrypter:\n def __init__(self, keys: List[str], values: List[str], dictionary: List[str]):\n self.key2value={}\n for k,v in zip(keys,values):\n self.key2value[k]=v\n self.dictionary=Counter()\n for d in dictionary:\n self.dictionary[self.encrypt(d)]+=1\n\n def encrypt(self, word1: str) -> str:\n res=[]\n for c in word1:\n if c in self.key2value:\n res.append(self.key2value[c])\n else:\n return ""\n return "".join(res)\n\n def decrypt(self, word2: str) -> int:\n return self.dictionary[word2]\n``` | 0 | You are given a character array `keys` containing **unique** characters and a string array `values` containing strings of length 2. You are also given another string array `dictionary` that contains all permitted original strings after decryption. You should implement a data structure that can encrypt or decrypt a **0-indexed** string.
A string is **encrypted** with the following process:
1. For each character `c` in the string, we find the index `i` satisfying `keys[i] == c` in `keys`.
2. Replace `c` with `values[i]` in the string.
Note that in case a character of the string is **not present** in `keys`, the encryption process cannot be carried out, and an empty string `" "` is returned.
A string is **decrypted** with the following process:
1. For each substring `s` of length 2 occurring at an even index in the string, we find an `i` such that `values[i] == s`. If there are multiple valid `i`, we choose **any** one of them. This means a string could have multiple possible strings it can decrypt to.
2. Replace `s` with `keys[i]` in the string.
Implement the `Encrypter` class:
* `Encrypter(char[] keys, String[] values, String[] dictionary)` Initializes the `Encrypter` class with `keys, values`, and `dictionary`.
* `String encrypt(String word1)` Encrypts `word1` with the encryption process described above and returns the encrypted string.
* `int decrypt(String word2)` Returns the number of possible strings `word2` could decrypt to that also appear in `dictionary`.
**Example 1:**
**Input**
\[ "Encrypter ", "encrypt ", "decrypt "\]
\[\[\['a', 'b', 'c', 'd'\], \[ "ei ", "zf ", "ei ", "am "\], \[ "abcd ", "acbd ", "adbc ", "badc ", "dacb ", "cadb ", "cbda ", "abad "\]\], \[ "abcd "\], \[ "eizfeiam "\]\]
**Output**
\[null, "eizfeiam ", 2\]
**Explanation**
Encrypter encrypter = new Encrypter(\[\['a', 'b', 'c', 'd'\], \[ "ei ", "zf ", "ei ", "am "\], \[ "abcd ", "acbd ", "adbc ", "badc ", "dacb ", "cadb ", "cbda ", "abad "\]);
encrypter.encrypt( "abcd "); // return "eizfeiam ".
// 'a' maps to "ei ", 'b' maps to "zf ", 'c' maps to "ei ", and 'd' maps to "am ".
encrypter.decrypt( "eizfeiam "); // return 2.
// "ei " can map to 'a' or 'c', "zf " maps to 'b', and "am " maps to 'd'.
// Thus, the possible strings after decryption are "abad ", "cbad ", "abcd ", and "cbcd ".
// 2 of those strings, "abad " and "abcd ", appear in dictionary, so the answer is 2.
**Constraints:**
* `1 <= keys.length == values.length <= 26`
* `values[i].length == 2`
* `1 <= dictionary.length <= 100`
* `1 <= dictionary[i].length <= 100`
* All `keys[i]` and `dictionary[i]` are **unique**.
* `1 <= word1.length <= 2000`
* `1 <= word2.length <= 200`
* All `word1[i]` appear in `keys`.
* `word2.length` is even.
* `keys`, `values[i]`, `dictionary[i]`, `word1`, and `word2` only contain lowercase English letters.
* At most `200` calls will be made to `encrypt` and `decrypt` **in total**. | Can you get the max/min of a certain subarray by using the max/min of a smaller subarray within it? Notice that the max of the subarray from index i to j is equal to max of (max of the subarray from index i to j-1) and nums[j]. |
Python Simple solution beats 96.92% | encrypt-and-decrypt-strings | 0 | 1 | # Code\n```\nclass Encrypter:\n def __init__(self, keys: List[str], values: List[str], dictionary: List[str]):\n self.key2value={}\n for k,v in zip(keys,values):\n self.key2value[k]=v\n self.dictionary=Counter()\n for d in dictionary:\n self.dictionary[self.encrypt(d)]+=1\n\n def encrypt(self, word1: str) -> str:\n res=[]\n for c in word1:\n if c in self.key2value:\n res.append(self.key2value[c])\n else:\n return ""\n return "".join(res)\n\n def decrypt(self, word2: str) -> int:\n return self.dictionary[word2]\n``` | 0 | Given two strings: `s1` and `s2` with the same size, check if some permutation of string `s1` can break some permutation of string `s2` or vice-versa. In other words `s2` can break `s1` or vice-versa.
A string `x` can break string `y` (both of size `n`) if `x[i] >= y[i]` (in alphabetical order) for all `i` between `0` and `n-1`.
**Example 1:**
**Input:** s1 = "abc ", s2 = "xya "
**Output:** true
**Explanation:** "ayx " is a permutation of s2= "xya " which can break to string "abc " which is a permutation of s1= "abc ".
**Example 2:**
**Input:** s1 = "abe ", s2 = "acd "
**Output:** false
**Explanation:** All permutations for s1= "abe " are: "abe ", "aeb ", "bae ", "bea ", "eab " and "eba " and all permutation for s2= "acd " are: "acd ", "adc ", "cad ", "cda ", "dac " and "dca ". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
**Example 3:**
**Input:** s1 = "leetcodee ", s2 = "interview "
**Output:** true
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `1 <= n <= 10^5`
* All strings consist of lowercase English letters. | For encryption, use hashmap to map each char of word1 to its value. For decryption, use trie to prune when necessary. |
python3 | HashMap solution | encrypt-and-decrypt-strings | 0 | 1 | \n# Code\n```\nclass Encrypter:\n\n def __init__(self, keys: List[str], values: List[str], dictionary: List[str]):\n self.mapping = {}\n self.countDict = defaultdict(int)\n for i in range(len(keys)):\n self.mapping[keys[i]]=values[i]\n for key in dictionary:\n self.countDict[self.encrypt(key,0)] += 1\n def encrypt(self, word1: str,init = 1) -> str:\n ans = ""\n for ch in word1:\n if ch in self.mapping:\n ans+=self.mapping[ch]\n else:\n if init==0: return ""\n ans+=ch\n return ans\n\n def decrypt(self, word2: str) -> int:\n return self.countDict[word2]\n\n\n# Your Encrypter object will be instantiated and called as such:\n# obj = Encrypter(keys, values, dictionary)\n# param_1 = obj.encrypt(word1)\n# param_2 = obj.decrypt(word2)\n``` | 0 | You are given a character array `keys` containing **unique** characters and a string array `values` containing strings of length 2. You are also given another string array `dictionary` that contains all permitted original strings after decryption. You should implement a data structure that can encrypt or decrypt a **0-indexed** string.
A string is **encrypted** with the following process:
1. For each character `c` in the string, we find the index `i` satisfying `keys[i] == c` in `keys`.
2. Replace `c` with `values[i]` in the string.
Note that in case a character of the string is **not present** in `keys`, the encryption process cannot be carried out, and an empty string `" "` is returned.
A string is **decrypted** with the following process:
1. For each substring `s` of length 2 occurring at an even index in the string, we find an `i` such that `values[i] == s`. If there are multiple valid `i`, we choose **any** one of them. This means a string could have multiple possible strings it can decrypt to.
2. Replace `s` with `keys[i]` in the string.
Implement the `Encrypter` class:
* `Encrypter(char[] keys, String[] values, String[] dictionary)` Initializes the `Encrypter` class with `keys, values`, and `dictionary`.
* `String encrypt(String word1)` Encrypts `word1` with the encryption process described above and returns the encrypted string.
* `int decrypt(String word2)` Returns the number of possible strings `word2` could decrypt to that also appear in `dictionary`.
**Example 1:**
**Input**
\[ "Encrypter ", "encrypt ", "decrypt "\]
\[\[\['a', 'b', 'c', 'd'\], \[ "ei ", "zf ", "ei ", "am "\], \[ "abcd ", "acbd ", "adbc ", "badc ", "dacb ", "cadb ", "cbda ", "abad "\]\], \[ "abcd "\], \[ "eizfeiam "\]\]
**Output**
\[null, "eizfeiam ", 2\]
**Explanation**
Encrypter encrypter = new Encrypter(\[\['a', 'b', 'c', 'd'\], \[ "ei ", "zf ", "ei ", "am "\], \[ "abcd ", "acbd ", "adbc ", "badc ", "dacb ", "cadb ", "cbda ", "abad "\]);
encrypter.encrypt( "abcd "); // return "eizfeiam ".
// 'a' maps to "ei ", 'b' maps to "zf ", 'c' maps to "ei ", and 'd' maps to "am ".
encrypter.decrypt( "eizfeiam "); // return 2.
// "ei " can map to 'a' or 'c', "zf " maps to 'b', and "am " maps to 'd'.
// Thus, the possible strings after decryption are "abad ", "cbad ", "abcd ", and "cbcd ".
// 2 of those strings, "abad " and "abcd ", appear in dictionary, so the answer is 2.
**Constraints:**
* `1 <= keys.length == values.length <= 26`
* `values[i].length == 2`
* `1 <= dictionary.length <= 100`
* `1 <= dictionary[i].length <= 100`
* All `keys[i]` and `dictionary[i]` are **unique**.
* `1 <= word1.length <= 2000`
* `1 <= word2.length <= 200`
* All `word1[i]` appear in `keys`.
* `word2.length` is even.
* `keys`, `values[i]`, `dictionary[i]`, `word1`, and `word2` only contain lowercase English letters.
* At most `200` calls will be made to `encrypt` and `decrypt` **in total**. | Can you get the max/min of a certain subarray by using the max/min of a smaller subarray within it? Notice that the max of the subarray from index i to j is equal to max of (max of the subarray from index i to j-1) and nums[j]. |
python3 | HashMap solution | encrypt-and-decrypt-strings | 0 | 1 | \n# Code\n```\nclass Encrypter:\n\n def __init__(self, keys: List[str], values: List[str], dictionary: List[str]):\n self.mapping = {}\n self.countDict = defaultdict(int)\n for i in range(len(keys)):\n self.mapping[keys[i]]=values[i]\n for key in dictionary:\n self.countDict[self.encrypt(key,0)] += 1\n def encrypt(self, word1: str,init = 1) -> str:\n ans = ""\n for ch in word1:\n if ch in self.mapping:\n ans+=self.mapping[ch]\n else:\n if init==0: return ""\n ans+=ch\n return ans\n\n def decrypt(self, word2: str) -> int:\n return self.countDict[word2]\n\n\n# Your Encrypter object will be instantiated and called as such:\n# obj = Encrypter(keys, values, dictionary)\n# param_1 = obj.encrypt(word1)\n# param_2 = obj.decrypt(word2)\n``` | 0 | Given two strings: `s1` and `s2` with the same size, check if some permutation of string `s1` can break some permutation of string `s2` or vice-versa. In other words `s2` can break `s1` or vice-versa.
A string `x` can break string `y` (both of size `n`) if `x[i] >= y[i]` (in alphabetical order) for all `i` between `0` and `n-1`.
**Example 1:**
**Input:** s1 = "abc ", s2 = "xya "
**Output:** true
**Explanation:** "ayx " is a permutation of s2= "xya " which can break to string "abc " which is a permutation of s1= "abc ".
**Example 2:**
**Input:** s1 = "abe ", s2 = "acd "
**Output:** false
**Explanation:** All permutations for s1= "abe " are: "abe ", "aeb ", "bae ", "bea ", "eab " and "eba " and all permutation for s2= "acd " are: "acd ", "adc ", "cad ", "cda ", "dac " and "dca ". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
**Example 3:**
**Input:** s1 = "leetcodee ", s2 = "interview "
**Output:** true
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `1 <= n <= 10^5`
* All strings consist of lowercase English letters. | For encryption, use hashmap to map each char of word1 to its value. For decryption, use trie to prune when necessary. |
Python (Simple Hashmap) | encrypt-and-decrypt-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Encrypter:\n def __init__(self, keys, values, dictionary):\n self.dict1 = {i:j for i,j in zip(keys,values)}\n self.dict2 = defaultdict(list)\n self.dictionary = dictionary\n\n for i,j in zip(values,keys):\n self.dict2[i].append(j)\n\n def encrypt(self, word1):\n str1 = ""\n\n for i in word1:\n if i not in self.dict1:\n return ""\n else:\n str1 += self.dict1[i]\n\n return str1\n\n def decrypt(self, word2):\n total = 0\n\n for i in self.dictionary:\n if self.encrypt(i) == word2:\n total += 1\n\n return total\n\n\n\n``` | 0 | You are given a character array `keys` containing **unique** characters and a string array `values` containing strings of length 2. You are also given another string array `dictionary` that contains all permitted original strings after decryption. You should implement a data structure that can encrypt or decrypt a **0-indexed** string.
A string is **encrypted** with the following process:
1. For each character `c` in the string, we find the index `i` satisfying `keys[i] == c` in `keys`.
2. Replace `c` with `values[i]` in the string.
Note that in case a character of the string is **not present** in `keys`, the encryption process cannot be carried out, and an empty string `" "` is returned.
A string is **decrypted** with the following process:
1. For each substring `s` of length 2 occurring at an even index in the string, we find an `i` such that `values[i] == s`. If there are multiple valid `i`, we choose **any** one of them. This means a string could have multiple possible strings it can decrypt to.
2. Replace `s` with `keys[i]` in the string.
Implement the `Encrypter` class:
* `Encrypter(char[] keys, String[] values, String[] dictionary)` Initializes the `Encrypter` class with `keys, values`, and `dictionary`.
* `String encrypt(String word1)` Encrypts `word1` with the encryption process described above and returns the encrypted string.
* `int decrypt(String word2)` Returns the number of possible strings `word2` could decrypt to that also appear in `dictionary`.
**Example 1:**
**Input**
\[ "Encrypter ", "encrypt ", "decrypt "\]
\[\[\['a', 'b', 'c', 'd'\], \[ "ei ", "zf ", "ei ", "am "\], \[ "abcd ", "acbd ", "adbc ", "badc ", "dacb ", "cadb ", "cbda ", "abad "\]\], \[ "abcd "\], \[ "eizfeiam "\]\]
**Output**
\[null, "eizfeiam ", 2\]
**Explanation**
Encrypter encrypter = new Encrypter(\[\['a', 'b', 'c', 'd'\], \[ "ei ", "zf ", "ei ", "am "\], \[ "abcd ", "acbd ", "adbc ", "badc ", "dacb ", "cadb ", "cbda ", "abad "\]);
encrypter.encrypt( "abcd "); // return "eizfeiam ".
// 'a' maps to "ei ", 'b' maps to "zf ", 'c' maps to "ei ", and 'd' maps to "am ".
encrypter.decrypt( "eizfeiam "); // return 2.
// "ei " can map to 'a' or 'c', "zf " maps to 'b', and "am " maps to 'd'.
// Thus, the possible strings after decryption are "abad ", "cbad ", "abcd ", and "cbcd ".
// 2 of those strings, "abad " and "abcd ", appear in dictionary, so the answer is 2.
**Constraints:**
* `1 <= keys.length == values.length <= 26`
* `values[i].length == 2`
* `1 <= dictionary.length <= 100`
* `1 <= dictionary[i].length <= 100`
* All `keys[i]` and `dictionary[i]` are **unique**.
* `1 <= word1.length <= 2000`
* `1 <= word2.length <= 200`
* All `word1[i]` appear in `keys`.
* `word2.length` is even.
* `keys`, `values[i]`, `dictionary[i]`, `word1`, and `word2` only contain lowercase English letters.
* At most `200` calls will be made to `encrypt` and `decrypt` **in total**. | Can you get the max/min of a certain subarray by using the max/min of a smaller subarray within it? Notice that the max of the subarray from index i to j is equal to max of (max of the subarray from index i to j-1) and nums[j]. |
Python (Simple Hashmap) | encrypt-and-decrypt-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Encrypter:\n def __init__(self, keys, values, dictionary):\n self.dict1 = {i:j for i,j in zip(keys,values)}\n self.dict2 = defaultdict(list)\n self.dictionary = dictionary\n\n for i,j in zip(values,keys):\n self.dict2[i].append(j)\n\n def encrypt(self, word1):\n str1 = ""\n\n for i in word1:\n if i not in self.dict1:\n return ""\n else:\n str1 += self.dict1[i]\n\n return str1\n\n def decrypt(self, word2):\n total = 0\n\n for i in self.dictionary:\n if self.encrypt(i) == word2:\n total += 1\n\n return total\n\n\n\n``` | 0 | Given two strings: `s1` and `s2` with the same size, check if some permutation of string `s1` can break some permutation of string `s2` or vice-versa. In other words `s2` can break `s1` or vice-versa.
A string `x` can break string `y` (both of size `n`) if `x[i] >= y[i]` (in alphabetical order) for all `i` between `0` and `n-1`.
**Example 1:**
**Input:** s1 = "abc ", s2 = "xya "
**Output:** true
**Explanation:** "ayx " is a permutation of s2= "xya " which can break to string "abc " which is a permutation of s1= "abc ".
**Example 2:**
**Input:** s1 = "abe ", s2 = "acd "
**Output:** false
**Explanation:** All permutations for s1= "abe " are: "abe ", "aeb ", "bae ", "bea ", "eab " and "eba " and all permutation for s2= "acd " are: "acd ", "adc ", "cad ", "cda ", "dac " and "dca ". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
**Example 3:**
**Input:** s1 = "leetcodee ", s2 = "interview "
**Output:** true
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `1 <= n <= 10^5`
* All strings consist of lowercase English letters. | For encryption, use hashmap to map each char of word1 to its value. For decryption, use trie to prune when necessary. |
max heap || easy | largest-number-after-digit-swaps-by-parity | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def largestInteger(self, num: int) -> int:\n evenlist=[]\n oddlist=[]\n nums= [int(x) for x in str(num)]\n for i in nums:\n if i%2==0:\n evenlist.append(i)\n else:\n oddlist.append(i)\n even= [-x for x in evenlist]\n odd = [-x for x in oddlist]\n heapq.heapify(even)\n heapq.heapify(odd)\n result=[]\n for ele in nums:\n if ele in evenlist:\n result+=[-heapq.heappop(even)]\n if ele in oddlist:\n result+=[-heapq.heappop(odd)]\n result =[str(x) for x in result] \n return int(\'\'.join(result))\n\n\n \n\n\n \n\n\n``` | 1 | You are given a positive integer `num`. You may swap any two digits of `num` that have the same **parity** (i.e. both odd digits or both even digits).
Return _the **largest** possible value of_ `num` _after **any** number of swaps._
**Example 1:**
**Input:** num = 1234
**Output:** 3412
**Explanation:** Swap the digit 3 with the digit 1, this results in the number 3214.
Swap the digit 2 with the digit 4, this results in the number 3412.
Note that there may be other sequences of swaps but it can be shown that 3412 is the largest possible number.
Also note that we may not swap the digit 4 with the digit 1 since they are of different parities.
**Example 2:**
**Input:** num = 65875
**Output:** 87655
**Explanation:** Swap the digit 8 with the digit 6, this results in the number 85675.
Swap the first digit 5 with the digit 7, this results in the number 87655.
Note that there may be other sequences of swaps but it can be shown that 87655 is the largest possible number.
**Constraints:**
* `1 <= num <= 109` | Iterate through the elements in order. As soon as the current element is a palindrome, return it. To check if an element is a palindrome, can you reverse the string? |
Python Solution using Sorting | largest-number-after-digit-swaps-by-parity | 0 | 1 | Since we need the largest number, what we want is basically to have the larger digits upfront and then the smaller ones. But we are given a parity condition that we need to comply to. We are allowed to swap odd digits with odd ones and even with even ones. So we simply maintain two arrays with odd and even digits. The we construct out answer by taking values from the odd or even arrays as per the parity of ith index. \n\n**STEPS**\n1. First make a array with the digits from nums.\n2. Then we traverse through the array and insert odd and even values into `odd` and `even` lists.\n3. We sort these `odd` and `even` lists.\n4. Then traverse from 0 to n, at each step we check for the parity at that index. For even parity we take the largest value from even array and for odd parity we take from odd array. **WE TAKE THE LARGEST VALUE AS A GREEDY CHOICE TO MAKE THE RESULTING NUMBER LARGER.**\n5. Return the result.\n\n**CODE**\n```\nclass Solution:\n def largestInteger(self, num: int):\n n = len(str(num))\n arr = [int(i) for i in str(num)]\n odd, even = [], []\n for i in arr:\n if i % 2 == 0:\n even.append(i)\n else:\n odd.append(i)\n odd.sort()\n even.sort()\n res = 0\n for i in range(n):\n if arr[i] % 2 == 0:\n res = res*10 + even.pop()\n else:\n res = res*10 + odd.pop()\n return res\n``` | 25 | You are given a positive integer `num`. You may swap any two digits of `num` that have the same **parity** (i.e. both odd digits or both even digits).
Return _the **largest** possible value of_ `num` _after **any** number of swaps._
**Example 1:**
**Input:** num = 1234
**Output:** 3412
**Explanation:** Swap the digit 3 with the digit 1, this results in the number 3214.
Swap the digit 2 with the digit 4, this results in the number 3412.
Note that there may be other sequences of swaps but it can be shown that 3412 is the largest possible number.
Also note that we may not swap the digit 4 with the digit 1 since they are of different parities.
**Example 2:**
**Input:** num = 65875
**Output:** 87655
**Explanation:** Swap the digit 8 with the digit 6, this results in the number 85675.
Swap the first digit 5 with the digit 7, this results in the number 87655.
Note that there may be other sequences of swaps but it can be shown that 87655 is the largest possible number.
**Constraints:**
* `1 <= num <= 109` | Iterate through the elements in order. As soon as the current element is a palindrome, return it. To check if an element is a palindrome, can you reverse the string? |
Python easy solution using heap | faster than 99% | largest-number-after-digit-swaps-by-parity | 0 | 1 | \nI have maintained two heaps: `odd_x` and `even_x`\nIf number is odd push into `odd_x` else push into`even_x`, also I am adding true (wherever odd number is encountered) so that its easy to track positions of odd numbers\n\nFor generating ans, I am popping out highest odd number for positions using heap where odd numbers were present (`ans[i] = -heapq.heappop(odd_x)`) \nand similarly doing for even numbers\n\n```python\n\nclass Solution:\n def largestInteger(self, num: int) -> int:\n\n odd_x = []\n even_x = []\n ans = []\n for i in str(num):\n i = int(i)\n if i % 2:\n odd_x.append(-i)\n ans.append(True)\n else:\n even_x.append(-i)\n ans.append(False)\n\n heapq.heapify(odd_x)\n heapq.heapify(even_x)\n\n for i in range(len(ans)):\n if ans[i]:\n ans[i] = -heapq.heappop(odd_x)\n else:\n ans[i] = -heapq.heappop(even_x)\n\n return int("".join(str(n) for n in ans))\n\n``` | 3 | You are given a positive integer `num`. You may swap any two digits of `num` that have the same **parity** (i.e. both odd digits or both even digits).
Return _the **largest** possible value of_ `num` _after **any** number of swaps._
**Example 1:**
**Input:** num = 1234
**Output:** 3412
**Explanation:** Swap the digit 3 with the digit 1, this results in the number 3214.
Swap the digit 2 with the digit 4, this results in the number 3412.
Note that there may be other sequences of swaps but it can be shown that 3412 is the largest possible number.
Also note that we may not swap the digit 4 with the digit 1 since they are of different parities.
**Example 2:**
**Input:** num = 65875
**Output:** 87655
**Explanation:** Swap the digit 8 with the digit 6, this results in the number 85675.
Swap the first digit 5 with the digit 7, this results in the number 87655.
Note that there may be other sequences of swaps but it can be shown that 87655 is the largest possible number.
**Constraints:**
* `1 <= num <= 109` | Iterate through the elements in order. As soon as the current element is a palindrome, return it. To check if an element is a palindrome, can you reverse the string? |
Python Solution using 2 Pointers Brute Force | minimize-result-by-adding-parentheses-to-expression | 0 | 1 | *Since the given maximum size is only 10, we can easily cover all possible options without TLE. Thus we use 2 pointers to cover all options.*\n\n**STEPS**\n1. Traverse two pointers `l` & `r`. **L** pointer goes from 0 to **plus_index** and **R** pointer goes from **plus_index+1** to **N**. **plus_index** is the index of \'+\' in the expression. The **L** pointer is the position of \'(\' and **R** is for \')\'.\n2. Use f string formatting to generate a expression. \n3. Use evaulate function to get the result of the following expression.\n4. If the new result is better than the old one, then update otherwise continue.\n \n**NOTE** \n\u27A1\uFE0F**The `evaluate` function basically converts "23(44+91)2" into "23 X (44+91) X 2" without ever having any * sign in the edges(used strip methods for that). `eval()` is a library function that evaluates a mathematical expression given in string form.**\n\u27A1\uFE0F **`ans` is a list of 2 values, first is the smalles result of any expression and second is the expression itself.**\n\n**CODE**\n```\nclass Solution:\n def minimizeResult(self, expression: str) -> str:\n plus_index, n, ans = expression.find(\'+\'), len(expression), [float(inf),expression] \n def evaluate(exps: str):\n return eval(exps.replace(\'(\',\'*(\').replace(\')\', \')*\').lstrip(\'*\').rstrip(\'*\'))\n for l in range(plus_index):\n for r in range(plus_index+1, n):\n exps = f\'{expression[:l]}({expression[l:plus_index]}+{expression[plus_index+1:r+1]}){expression[r+1:n]}\'\n res = evaluate(exps)\n if ans[0] > res:\n ans[0], ans[1] = res, exps\n return ans[1]\n``` | 16 | You are given a **0-indexed** string `expression` of the form `"+ "` where and represent positive integers.
Add a pair of parentheses to `expression` such that after the addition of parentheses, `expression` is a **valid** mathematical expression and evaluates to the **smallest** possible value. The left parenthesis **must** be added to the left of `'+'` and the right parenthesis **must** be added to the right of `'+'`.
Return `expression` _after adding a pair of parentheses such that_ `expression` _evaluates to the **smallest** possible value._ If there are multiple answers that yield the same result, return any of them.
The input has been generated such that the original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer.
**Example 1:**
**Input:** expression = "247+38 "
**Output:** "2(47+38) "
**Explanation:** The `expression` evaluates to 2 \* (47 + 38) = 2 \* 85 = 170.
Note that "2(4)7+38 " is invalid because the right parenthesis must be to the right of the `'+'`.
It can be shown that 170 is the smallest possible value.
**Example 2:**
**Input:** expression = "12+34 "
**Output:** "1(2+3)4 "
**Explanation:** The expression evaluates to 1 \* (2 + 3) \* 4 = 1 \* 5 \* 4 = 20.
**Example 3:**
**Input:** expression = "999+999 "
**Output:** "(999+999) "
**Explanation:** The `expression` evaluates to 999 + 999 = 1998.
**Constraints:**
* `3 <= expression.length <= 10`
* `expression` consists of digits from `'1'` to `'9'` and `'+'`.
* `expression` starts and ends with digits.
* `expression` contains exactly one `'+'`.
* The original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer. | Create a new string, initially empty, as the modified string. Iterate through the original string and append each character of the original string to the new string. However, each time you reach a character that requires a space before it, append a space before appending the character. Since the array of indices for the space locations is sorted, use a pointer to keep track of the next index to place a space. Only increment the pointer once a space has been appended. Ensure that your append operation can be done in O(1). |
Python 3 || 5 lines, w/ example || T/M: 82%/99% | minimize-result-by-adding-parentheses-to-expression | 0 | 1 | ```\nclass Solution:\n def minimizeResult(self, expression: str) -> str: # Example: "247+38" \n # left, right = "247","38"\n left, right = expression.split(\'+\') \n value = lambda s:eval(s.replace(\'(\',\'*(\').replace(\')\',\')*\').strip(\'*\')) \n \n lft = [ left[0:i]+\'(\'+ left[i:] for i in range( len(left ) )] # lft = [\'(247\', \'2(47\', \'24(7\']\n rgt = [right[0:i]+\')\'+right[i:] for i in range(1,len(right)+1)] # rgt = [\'3)8\', \'38)\']\n \n return min([l+\'+\'+r for l in lft for r in rgt], key = value) # = min[(247+3)8,(247+38),2(47+3)8,2(47+38),24(7+3)8,24(7+38)]\n # | | | | | | \n # 2000 285 800 170 1920 1080 \n # |\n # return 2(47+38)\n\n```\n[https://leetcode.com/submissions/detail/848284729/](http://)\n\nI could be wrong, but I think time complexity is *O*(*N*^2) and space complexity is *O*(*N*). | 5 | You are given a **0-indexed** string `expression` of the form `"+ "` where and represent positive integers.
Add a pair of parentheses to `expression` such that after the addition of parentheses, `expression` is a **valid** mathematical expression and evaluates to the **smallest** possible value. The left parenthesis **must** be added to the left of `'+'` and the right parenthesis **must** be added to the right of `'+'`.
Return `expression` _after adding a pair of parentheses such that_ `expression` _evaluates to the **smallest** possible value._ If there are multiple answers that yield the same result, return any of them.
The input has been generated such that the original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer.
**Example 1:**
**Input:** expression = "247+38 "
**Output:** "2(47+38) "
**Explanation:** The `expression` evaluates to 2 \* (47 + 38) = 2 \* 85 = 170.
Note that "2(4)7+38 " is invalid because the right parenthesis must be to the right of the `'+'`.
It can be shown that 170 is the smallest possible value.
**Example 2:**
**Input:** expression = "12+34 "
**Output:** "1(2+3)4 "
**Explanation:** The expression evaluates to 1 \* (2 + 3) \* 4 = 1 \* 5 \* 4 = 20.
**Example 3:**
**Input:** expression = "999+999 "
**Output:** "(999+999) "
**Explanation:** The `expression` evaluates to 999 + 999 = 1998.
**Constraints:**
* `3 <= expression.length <= 10`
* `expression` consists of digits from `'1'` to `'9'` and `'+'`.
* `expression` starts and ends with digits.
* `expression` contains exactly one `'+'`.
* The original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer. | Create a new string, initially empty, as the modified string. Iterate through the original string and append each character of the original string to the new string. However, each time you reach a character that requires a space before it, append a space before appending the character. Since the array of indices for the space locations is sorted, use a pointer to keep track of the next index to place a space. Only increment the pointer once a space has been appended. Ensure that your append operation can be done in O(1). |
Python | Intuitive method | minimize-result-by-adding-parentheses-to-expression | 0 | 1 | # Intuition\nJust split the string into three parts: \n- left before \'(\'\n- middle in the parenthisis\n- right after \')\'.\n\n# Complexity\n- Time complexity:\nO(N^2)\n\n- Space complexity:\nO(N^2)\n\n# Code\n```\nclass Solution:\n def minimizeResult(self, expression: str) -> str:\n n = len(expression)\n \n def dfs(l, r):\n nonlocal min_value, res\n\n # boundary check \n if l == 0 and r == n: \n return\n elif l < 0 or r > n:\n return\n\n # devide string into three parts\n # if parenthesis reach the bound, The side part becomes 1 for production\n left = eval(expression[:l]) if l != 0 else 1\n right = eval(expression[r:]) if r != n else 1\n val = left * eval(expression[l:r]) * right\n\n # check new value\n if val < min_value:\n min_value = val\n res = [l, r]\n\n dfs(l - 1, r) \n dfs(l, r + 1)\n\n \n res = [0, n]\n min_value = eval(expression)\n \n start = expression.find(\'+\')\n dfs(start-1, start+2)\n\n l, r = res\n return expression[:l] + \'(\' + expression[l:r] + ")" + expression[r:]\n \n``` | 0 | You are given a **0-indexed** string `expression` of the form `"+ "` where and represent positive integers.
Add a pair of parentheses to `expression` such that after the addition of parentheses, `expression` is a **valid** mathematical expression and evaluates to the **smallest** possible value. The left parenthesis **must** be added to the left of `'+'` and the right parenthesis **must** be added to the right of `'+'`.
Return `expression` _after adding a pair of parentheses such that_ `expression` _evaluates to the **smallest** possible value._ If there are multiple answers that yield the same result, return any of them.
The input has been generated such that the original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer.
**Example 1:**
**Input:** expression = "247+38 "
**Output:** "2(47+38) "
**Explanation:** The `expression` evaluates to 2 \* (47 + 38) = 2 \* 85 = 170.
Note that "2(4)7+38 " is invalid because the right parenthesis must be to the right of the `'+'`.
It can be shown that 170 is the smallest possible value.
**Example 2:**
**Input:** expression = "12+34 "
**Output:** "1(2+3)4 "
**Explanation:** The expression evaluates to 1 \* (2 + 3) \* 4 = 1 \* 5 \* 4 = 20.
**Example 3:**
**Input:** expression = "999+999 "
**Output:** "(999+999) "
**Explanation:** The `expression` evaluates to 999 + 999 = 1998.
**Constraints:**
* `3 <= expression.length <= 10`
* `expression` consists of digits from `'1'` to `'9'` and `'+'`.
* `expression` starts and ends with digits.
* `expression` contains exactly one `'+'`.
* The original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer. | Create a new string, initially empty, as the modified string. Iterate through the original string and append each character of the original string to the new string. However, each time you reach a character that requires a space before it, append a space before appending the character. Since the array of indices for the space locations is sorted, use a pointer to keep track of the next index to place a space. Only increment the pointer once a space has been appended. Ensure that your append operation can be done in O(1). |
Python | Brute Force | minimize-result-by-adding-parentheses-to-expression | 0 | 1 | # Code\n```\nclass Solution:\n def minimizeResult(self, e: str) -> str:\n lft, rgt = e.split("+")\n mi = inf\n for l in range(len(lft)):\n for r in range(1, len(rgt) + 1):\n cur = int(lft[:l] or 1) * (int(lft[l:]) + int(rgt[:r])) * int(rgt[r:] or 1)\n if cur < mi:\n res = f"{lft[:l]}({lft[l:]}+{rgt[:r]}){rgt[r:]}"\n mi = min(mi, cur)\n return res\n``` | 0 | You are given a **0-indexed** string `expression` of the form `"+ "` where and represent positive integers.
Add a pair of parentheses to `expression` such that after the addition of parentheses, `expression` is a **valid** mathematical expression and evaluates to the **smallest** possible value. The left parenthesis **must** be added to the left of `'+'` and the right parenthesis **must** be added to the right of `'+'`.
Return `expression` _after adding a pair of parentheses such that_ `expression` _evaluates to the **smallest** possible value._ If there are multiple answers that yield the same result, return any of them.
The input has been generated such that the original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer.
**Example 1:**
**Input:** expression = "247+38 "
**Output:** "2(47+38) "
**Explanation:** The `expression` evaluates to 2 \* (47 + 38) = 2 \* 85 = 170.
Note that "2(4)7+38 " is invalid because the right parenthesis must be to the right of the `'+'`.
It can be shown that 170 is the smallest possible value.
**Example 2:**
**Input:** expression = "12+34 "
**Output:** "1(2+3)4 "
**Explanation:** The expression evaluates to 1 \* (2 + 3) \* 4 = 1 \* 5 \* 4 = 20.
**Example 3:**
**Input:** expression = "999+999 "
**Output:** "(999+999) "
**Explanation:** The `expression` evaluates to 999 + 999 = 1998.
**Constraints:**
* `3 <= expression.length <= 10`
* `expression` consists of digits from `'1'` to `'9'` and `'+'`.
* `expression` starts and ends with digits.
* `expression` contains exactly one `'+'`.
* The original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer. | Create a new string, initially empty, as the modified string. Iterate through the original string and append each character of the original string to the new string. However, each time you reach a character that requires a space before it, append a space before appending the character. Since the array of indices for the space locations is sorted, use a pointer to keep track of the next index to place a space. Only increment the pointer once a space has been appended. Ensure that your append operation can be done in O(1). |
minimize-result-by-adding-parentheses-to-expression | minimize-result-by-adding-parentheses-to-expression | 0 | 1 | # Code\n```\nclass Solution:\n def minimizeResult(self, e: str) -> str:\n p = []\n b = e.split("+")\n p.append([int(b[0]) + int(b[1]),"(" + e + ")"]) \n for i in range(len(b[0])):\n a = b[0][:i]\n if len(a)>0:\n h = int(a)* (int(b[0][i:]) + int(b[1]))\n p.append([h,a + "(" + b[0][i:] +"+" + b[1] +")"])\n else:\n h = (int(b[0]) + int(b[1]))\n p.append([h,"(" + b[0] +"+" + b[1] +")"])\n for j in range(1,len(b[1])):\n if len(a)>0:\n h = int(a)* (int(b[0][i:]) + int(b[1][:j])) * int(b[1][j:])\n print(h)\n p.append([h,a + "(" + b[0][i:] +"+" + b[1][:j] + ")"+ b[1][j:]])\n else:\n h = (int(b[0]) + (int(b[1][:j]))) * int(b[1][j:])\n p.append([h,"(" + b[0] +"+" + b[1][:j] + ")"+ b[1][j:]])\n p.sort()\n return p[0][1]\n \n\n\n \n``` | 0 | You are given a **0-indexed** string `expression` of the form `"+ "` where and represent positive integers.
Add a pair of parentheses to `expression` such that after the addition of parentheses, `expression` is a **valid** mathematical expression and evaluates to the **smallest** possible value. The left parenthesis **must** be added to the left of `'+'` and the right parenthesis **must** be added to the right of `'+'`.
Return `expression` _after adding a pair of parentheses such that_ `expression` _evaluates to the **smallest** possible value._ If there are multiple answers that yield the same result, return any of them.
The input has been generated such that the original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer.
**Example 1:**
**Input:** expression = "247+38 "
**Output:** "2(47+38) "
**Explanation:** The `expression` evaluates to 2 \* (47 + 38) = 2 \* 85 = 170.
Note that "2(4)7+38 " is invalid because the right parenthesis must be to the right of the `'+'`.
It can be shown that 170 is the smallest possible value.
**Example 2:**
**Input:** expression = "12+34 "
**Output:** "1(2+3)4 "
**Explanation:** The expression evaluates to 1 \* (2 + 3) \* 4 = 1 \* 5 \* 4 = 20.
**Example 3:**
**Input:** expression = "999+999 "
**Output:** "(999+999) "
**Explanation:** The `expression` evaluates to 999 + 999 = 1998.
**Constraints:**
* `3 <= expression.length <= 10`
* `expression` consists of digits from `'1'` to `'9'` and `'+'`.
* `expression` starts and ends with digits.
* `expression` contains exactly one `'+'`.
* The original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer. | Create a new string, initially empty, as the modified string. Iterate through the original string and append each character of the original string to the new string. However, each time you reach a character that requires a space before it, append a space before appending the character. Since the array of indices for the space locations is sorted, use a pointer to keep track of the next index to place a space. Only increment the pointer once a space has been appended. Ensure that your append operation can be done in O(1). |
Brute force Python solution, beats 100% runtime | minimize-result-by-adding-parentheses-to-expression | 0 | 1 | # Intuition\nBrute force\n\n# Approach\nIterate through all possible left and right parenthesis positions and remember when the value is the smallest.\n\nWe could bring down the complexity to $$O(n^2)$$ by keeping track of the current values inside the parentheses and calculating the impact of moving the parenthesis left or right arithmetically. However, for small inputs like these, it wouldn\'t make a difference.\n\n# Complexity\n- Time complexity: $$O(n^3)$$\n\n- Space complexity: $$O(1)$$\n\n# Code\n```\nclass Solution:\n def minimizeResult(self, expression: str) -> str:\n plus_pos = expression.find(\'+\')\n min_val, min_lp, min_rp = None, 0, 0\n\n for lp in range(0, plus_pos):\n for rp in range(plus_pos+2, len(expression)+1):\n val = int(expression[:lp] or 1) * \\\n (int(expression[lp:plus_pos]) + int(expression[plus_pos+1:rp])) * \\\n int(expression[rp:] or 1)\n if min_val is None or val < min_val:\n min_val = val\n min_lp = lp\n min_rp = rp\n \n return expression[:min_lp] + \'(\' + expression[min_lp:min_rp] + \')\' + expression[min_rp:]\n\n``` | 0 | You are given a **0-indexed** string `expression` of the form `"+ "` where and represent positive integers.
Add a pair of parentheses to `expression` such that after the addition of parentheses, `expression` is a **valid** mathematical expression and evaluates to the **smallest** possible value. The left parenthesis **must** be added to the left of `'+'` and the right parenthesis **must** be added to the right of `'+'`.
Return `expression` _after adding a pair of parentheses such that_ `expression` _evaluates to the **smallest** possible value._ If there are multiple answers that yield the same result, return any of them.
The input has been generated such that the original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer.
**Example 1:**
**Input:** expression = "247+38 "
**Output:** "2(47+38) "
**Explanation:** The `expression` evaluates to 2 \* (47 + 38) = 2 \* 85 = 170.
Note that "2(4)7+38 " is invalid because the right parenthesis must be to the right of the `'+'`.
It can be shown that 170 is the smallest possible value.
**Example 2:**
**Input:** expression = "12+34 "
**Output:** "1(2+3)4 "
**Explanation:** The expression evaluates to 1 \* (2 + 3) \* 4 = 1 \* 5 \* 4 = 20.
**Example 3:**
**Input:** expression = "999+999 "
**Output:** "(999+999) "
**Explanation:** The `expression` evaluates to 999 + 999 = 1998.
**Constraints:**
* `3 <= expression.length <= 10`
* `expression` consists of digits from `'1'` to `'9'` and `'+'`.
* `expression` starts and ends with digits.
* `expression` contains exactly one `'+'`.
* The original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer. | Create a new string, initially empty, as the modified string. Iterate through the original string and append each character of the original string to the new string. However, each time you reach a character that requires a space before it, append a space before appending the character. Since the array of indices for the space locations is sorted, use a pointer to keep track of the next index to place a space. Only increment the pointer once a space has been appended. Ensure that your append operation can be done in O(1). |
python bruteforce | minimize-result-by-adding-parentheses-to-expression | 0 | 1 | ```\nclass Solution:\n def minimizeResult(self, expression: str) -> str:\n n,_,m = map(len, expression.partition(\'+\'))\n solved = \'\'\n res = inf\n for i in range(n):\n for j in range(1, m+1):\n if int(expression[:i] or \'1\')*eval(expression[i:n+j+1])*int(expression[n+j+1:] or \'1\') < res:\n res = int(expression[:i] or \'1\')*eval(expression[i:n+j+1])*int(expression[n+j+1:] or \'1\')\n solved = expression[:i]+\'(\'+expression[i:n+j+1]+\')\'+expression[n+j+1:]\n\n return solved if eval(expression) > res else f\'({expression})\'\n \n``` | 0 | You are given a **0-indexed** string `expression` of the form `"+ "` where and represent positive integers.
Add a pair of parentheses to `expression` such that after the addition of parentheses, `expression` is a **valid** mathematical expression and evaluates to the **smallest** possible value. The left parenthesis **must** be added to the left of `'+'` and the right parenthesis **must** be added to the right of `'+'`.
Return `expression` _after adding a pair of parentheses such that_ `expression` _evaluates to the **smallest** possible value._ If there are multiple answers that yield the same result, return any of them.
The input has been generated such that the original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer.
**Example 1:**
**Input:** expression = "247+38 "
**Output:** "2(47+38) "
**Explanation:** The `expression` evaluates to 2 \* (47 + 38) = 2 \* 85 = 170.
Note that "2(4)7+38 " is invalid because the right parenthesis must be to the right of the `'+'`.
It can be shown that 170 is the smallest possible value.
**Example 2:**
**Input:** expression = "12+34 "
**Output:** "1(2+3)4 "
**Explanation:** The expression evaluates to 1 \* (2 + 3) \* 4 = 1 \* 5 \* 4 = 20.
**Example 3:**
**Input:** expression = "999+999 "
**Output:** "(999+999) "
**Explanation:** The `expression` evaluates to 999 + 999 = 1998.
**Constraints:**
* `3 <= expression.length <= 10`
* `expression` consists of digits from `'1'` to `'9'` and `'+'`.
* `expression` starts and ends with digits.
* `expression` contains exactly one `'+'`.
* The original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer. | Create a new string, initially empty, as the modified string. Iterate through the original string and append each character of the original string to the new string. However, each time you reach a character that requires a space before it, append a space before appending the character. Since the array of indices for the space locations is sorted, use a pointer to keep track of the next index to place a space. Only increment the pointer once a space has been appended. Ensure that your append operation can be done in O(1). |
Beats 91.27% Runtime | minimize-result-by-adding-parentheses-to-expression | 0 | 1 | # Approach\nSplit the expression by `+` to simplify indexing. Left bracket can be placed at `l_idx` from `0` to `l_num - 1`. Right bracket can be placed at `r_idx` from `1` to `r_num`. Edge cases at ends can have the default value as `1` using `or`. Intuitive and straightforward solution.\n\n# Code\n```\nclass Solution:\n def minimizeResult(self, expression: str) -> str:\n min_val = float("inf")\n l_num, r_num = expression.split("+")\n l_idx, r_idx = None, None\n for i in range(len(l_num)):\n for j in range(len(r_num)):\n new_val = int(l_num[:i] or 1) * (int(l_num[i:]) + int(r_num[:j+1])) * int(r_num[j+1:] or 1)\n if new_val < min_val:\n min_val, l_idx, r_idx = new_val, i, j + 1\n l_num = l_num[:l_idx] + "(" + l_num[l_idx:]\n r_num = r_num[:r_idx] + ")" + r_num[r_idx:]\n \n return l_num + "+" + r_num\n``` | 0 | You are given a **0-indexed** string `expression` of the form `"+ "` where and represent positive integers.
Add a pair of parentheses to `expression` such that after the addition of parentheses, `expression` is a **valid** mathematical expression and evaluates to the **smallest** possible value. The left parenthesis **must** be added to the left of `'+'` and the right parenthesis **must** be added to the right of `'+'`.
Return `expression` _after adding a pair of parentheses such that_ `expression` _evaluates to the **smallest** possible value._ If there are multiple answers that yield the same result, return any of them.
The input has been generated such that the original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer.
**Example 1:**
**Input:** expression = "247+38 "
**Output:** "2(47+38) "
**Explanation:** The `expression` evaluates to 2 \* (47 + 38) = 2 \* 85 = 170.
Note that "2(4)7+38 " is invalid because the right parenthesis must be to the right of the `'+'`.
It can be shown that 170 is the smallest possible value.
**Example 2:**
**Input:** expression = "12+34 "
**Output:** "1(2+3)4 "
**Explanation:** The expression evaluates to 1 \* (2 + 3) \* 4 = 1 \* 5 \* 4 = 20.
**Example 3:**
**Input:** expression = "999+999 "
**Output:** "(999+999) "
**Explanation:** The `expression` evaluates to 999 + 999 = 1998.
**Constraints:**
* `3 <= expression.length <= 10`
* `expression` consists of digits from `'1'` to `'9'` and `'+'`.
* `expression` starts and ends with digits.
* `expression` contains exactly one `'+'`.
* The original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer. | Create a new string, initially empty, as the modified string. Iterate through the original string and append each character of the original string to the new string. However, each time you reach a character that requires a space before it, append a space before appending the character. Since the array of indices for the space locations is sorted, use a pointer to keep track of the next index to place a space. Only increment the pointer once a space has been appended. Ensure that your append operation can be done in O(1). |
Easy Python Solution | minimize-result-by-adding-parentheses-to-expression | 0 | 1 | # Code\n```\nclass Solution:\n def minimizeResult(self, expression: str) -> str:\n s=expression.split("+") #s=[\'247\',\'38\']\n left,right=list(s[0]),list(s[1])\n len_left,len_right=len(list(left)),len(list(right))\n res_left,res_right,res=[],[],float(\'inf\')\n for i in range(len_left):\n if i!=0:\n left.insert(i, \'*(\')\n else:\n left.insert(i, \'(\')\n res_left.append("".join(left))\n left.pop(i) \n for i in range(len_right):\n if i<len_right-1:\n right.insert(i+1,")*")\n else:\n right.insert(i+1,")")\n res_right.append("".join(right))\n right.pop(i+1)\n for i in range(len_left):\n for j in range(len_right):\n val=eval(res_left[i]+\'+\'+res_right[j])\n if res>val:\n res=val\n x=res_left[i]+\'+\'+res_right[j]\n return x.replace("*","")\n``` | 0 | You are given a **0-indexed** string `expression` of the form `"+ "` where and represent positive integers.
Add a pair of parentheses to `expression` such that after the addition of parentheses, `expression` is a **valid** mathematical expression and evaluates to the **smallest** possible value. The left parenthesis **must** be added to the left of `'+'` and the right parenthesis **must** be added to the right of `'+'`.
Return `expression` _after adding a pair of parentheses such that_ `expression` _evaluates to the **smallest** possible value._ If there are multiple answers that yield the same result, return any of them.
The input has been generated such that the original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer.
**Example 1:**
**Input:** expression = "247+38 "
**Output:** "2(47+38) "
**Explanation:** The `expression` evaluates to 2 \* (47 + 38) = 2 \* 85 = 170.
Note that "2(4)7+38 " is invalid because the right parenthesis must be to the right of the `'+'`.
It can be shown that 170 is the smallest possible value.
**Example 2:**
**Input:** expression = "12+34 "
**Output:** "1(2+3)4 "
**Explanation:** The expression evaluates to 1 \* (2 + 3) \* 4 = 1 \* 5 \* 4 = 20.
**Example 3:**
**Input:** expression = "999+999 "
**Output:** "(999+999) "
**Explanation:** The `expression` evaluates to 999 + 999 = 1998.
**Constraints:**
* `3 <= expression.length <= 10`
* `expression` consists of digits from `'1'` to `'9'` and `'+'`.
* `expression` starts and ends with digits.
* `expression` contains exactly one `'+'`.
* The original value of `expression`, and the value of `expression` after adding any pair of parentheses that meets the requirements fits within a signed 32-bit integer. | Create a new string, initially empty, as the modified string. Iterate through the original string and append each character of the original string to the new string. However, each time you reach a character that requires a space before it, append a space before appending the character. Since the array of indices for the space locations is sorted, use a pointer to keep track of the next index to place a space. Only increment the pointer once a space has been appended. Ensure that your append operation can be done in O(1). |
Python solution without using heaps | maximum-product-after-k-increments | 0 | 1 | # Intuition\nOnly dictionaries are used in approach to increment the smallest number and calculate the maximum product\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(max(N,K))\n- Space complexity:\nO(N) {Dictionary}\n\n# Code\n```\nclass Solution:\n def maximumProduct(self, nums: List[int], n: int) -> int:\n hash_map = {}\n min_num = sys.maxsize\n for num in nums:\n min_num = min(min_num, num)\n if num not in hash_map:\n hash_map[num] = 1\n else:\n hash_map[num] += 1\n while n>0:\n n -= 1\n hash_map[min_num] -= 1\n if hash_map[min_num] == 0:\n min_num = min_num+1\n curr_num = min_num\n else:\n curr_num = min_num+1\n if curr_num not in hash_map:\n hash_map[curr_num] = 1\n else:\n hash_map[curr_num] += 1\n prod = 1\n for key, val in hash_map.items():\n while val > 0:\n prod = (prod*key)%1000000007\n val -= 1\n return prod\n``` | 2 | You are given an array of non-negative integers `nums` and an integer `k`. In one operation, you may choose **any** element from `nums` and **increment** it by `1`.
Return _the **maximum** **product** of_ `nums` _after **at most**_ `k` _operations._ Since the answer may be very large, return it **modulo** `109 + 7`. Note that you should maximize the product before taking the modulo.
**Example 1:**
**Input:** nums = \[0,4\], k = 5
**Output:** 20
**Explanation:** Increment the first number 5 times.
Now nums = \[5, 4\], with a product of 5 \* 4 = 20.
It can be shown that 20 is maximum product possible, so we return 20.
Note that there may be other ways to increment nums to have the maximum product.
**Example 2:**
**Input:** nums = \[6,3,3,2\], k = 2
**Output:** 216
**Explanation:** Increment the second number 1 time and increment the fourth number 1 time.
Now nums = \[6, 4, 3, 3\], with a product of 6 \* 4 \* 3 \* 3 = 216.
It can be shown that 216 is maximum product possible, so we return 216.
Note that there may be other ways to increment nums to have the maximum product.
**Constraints:**
* `1 <= nums.length, k <= 105`
* `0 <= nums[i] <= 106` | Any array is a series of adjacent longest possible smooth descent periods. For example, [5,3,2,1,7,6] is [5] + [3,2,1] + [7,6]. Think of a 2-pointer approach to traverse the array and find each longest possible period. Suppose you found the longest possible period with a length of k. How many periods are within that period? How can you count them quickly? Think of the formula to calculate the sum of 1, 2, 3, ..., k. |
Python | Min heap | Priority Queue | maximum-product-after-k-increments | 0 | 1 | \n def maximumProduct(self, nums: List[int], k: int) -> int:\n heapify(nums)\n for i in range(k):\n heappush(nums, heappop(nums)+1) \n result = 1\n for i in nums:\n result *= i\n result %= 10**9 + 7\n return result | 1 | You are given an array of non-negative integers `nums` and an integer `k`. In one operation, you may choose **any** element from `nums` and **increment** it by `1`.
Return _the **maximum** **product** of_ `nums` _after **at most**_ `k` _operations._ Since the answer may be very large, return it **modulo** `109 + 7`. Note that you should maximize the product before taking the modulo.
**Example 1:**
**Input:** nums = \[0,4\], k = 5
**Output:** 20
**Explanation:** Increment the first number 5 times.
Now nums = \[5, 4\], with a product of 5 \* 4 = 20.
It can be shown that 20 is maximum product possible, so we return 20.
Note that there may be other ways to increment nums to have the maximum product.
**Example 2:**
**Input:** nums = \[6,3,3,2\], k = 2
**Output:** 216
**Explanation:** Increment the second number 1 time and increment the fourth number 1 time.
Now nums = \[6, 4, 3, 3\], with a product of 6 \* 4 \* 3 \* 3 = 216.
It can be shown that 216 is maximum product possible, so we return 216.
Note that there may be other ways to increment nums to have the maximum product.
**Constraints:**
* `1 <= nums.length, k <= 105`
* `0 <= nums[i] <= 106` | Any array is a series of adjacent longest possible smooth descent periods. For example, [5,3,2,1,7,6] is [5] + [3,2,1] + [7,6]. Think of a 2-pointer approach to traverse the array and find each longest possible period. Suppose you found the longest possible period with a length of k. How many periods are within that period? How can you count them quickly? Think of the formula to calculate the sum of 1, 2, 3, ..., k. |
[Python3] semi-math greedy solution | maximum-product-after-k-increments | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/af907302ab84ad94ecb97eeeb9b0bfa529041e30) for solutions of weekly 288. \n\n```\nclass Solution:\n def maximumProduct(self, nums: List[int], k: int) -> int:\n mod = 1_000_000_007\n nums.sort()\n for i, x in enumerate(nums): \n target = nums[i+1] if i+1 < len(nums) else inf\n diff = (target-x) * (i+1)\n if diff <= k: k -= diff \n else: break \n q, r = divmod(k, i+1)\n ans = pow(x+q+1, r, mod) * pow(x+q, i+1-r, mod) % mod\n for ii in range(i+1, len(nums)): \n ans = ans * nums[ii] % mod\n return ans\n``` | 1 | You are given an array of non-negative integers `nums` and an integer `k`. In one operation, you may choose **any** element from `nums` and **increment** it by `1`.
Return _the **maximum** **product** of_ `nums` _after **at most**_ `k` _operations._ Since the answer may be very large, return it **modulo** `109 + 7`. Note that you should maximize the product before taking the modulo.
**Example 1:**
**Input:** nums = \[0,4\], k = 5
**Output:** 20
**Explanation:** Increment the first number 5 times.
Now nums = \[5, 4\], with a product of 5 \* 4 = 20.
It can be shown that 20 is maximum product possible, so we return 20.
Note that there may be other ways to increment nums to have the maximum product.
**Example 2:**
**Input:** nums = \[6,3,3,2\], k = 2
**Output:** 216
**Explanation:** Increment the second number 1 time and increment the fourth number 1 time.
Now nums = \[6, 4, 3, 3\], with a product of 6 \* 4 \* 3 \* 3 = 216.
It can be shown that 216 is maximum product possible, so we return 216.
Note that there may be other ways to increment nums to have the maximum product.
**Constraints:**
* `1 <= nums.length, k <= 105`
* `0 <= nums[i] <= 106` | Any array is a series of adjacent longest possible smooth descent periods. For example, [5,3,2,1,7,6] is [5] + [3,2,1] + [7,6]. Think of a 2-pointer approach to traverse the array and find each longest possible period. Suppose you found the longest possible period with a length of k. How many periods are within that period? How can you count them quickly? Think of the formula to calculate the sum of 1, 2, 3, ..., k. |
[Python 3] Simple interface, Explained — O(NlogN), O(1) | maximum-total-beauty-of-the-gardens | 0 | 1 | # Intuition\nWe can forget about gardens that are originally complete, because we can\'t change their state. Let\'s assume that we don\'t have such gardens, then:\n* There are actually only $N+1$ candidates for an answer:\n\n 1 \u2014 $0$ complete gardens and $N$ incomplete gardens (with maximized minimum amount of flowers)\n 2 \u2014 $1$ complete gardens and $N-1$ incomplete gardens (with maximized minimum amount of flowers)\n ...\n N+1 \u2014 $N$ complete gardens and $0$ incomplete gardens (with maximized minimum amount of flowers)\n\nNow all we need is to find efficient approach to iterate over these candidates.\n\n* We can notice that we can do only 4 actions\n 1. Make garden complete\n 2. Maximize minimum number of flowers among incomplete gardens\n 3. Revert operation (1)\n 4. Revert operation (2)\n\nUsing this interface we can easily write an algorithm (pseudo-code):\n```\nm = 0 # number of complete gardens\nwhile can_make_more_gardens_complete:\n make_garden_complete() # Operation 1\n m += 1\nmaximize_min_number_of_flowers() # Operation 2\nans = ... # update answer\nfor _ in range(m):\n make_garden_incomplete() # Operation 3\n maximize_min_number_of_flowers() # Operation 2\n ans = max(ans, ...) # update answer\nreturn ans\n```\n\n* If we sort `flowers`: Operations (1), (3) can be implemented with $O(1)$ time, operation (2) can be implemented with $\\Omega(1)$ time and we don\'t actually need operation (4)\n\n\n# Complexity\n- Time complexity: $$O(N\\log N)$$\n\n- Space complexity: $$O(1)$$\n\n# Code\n```\nclass Solution:\n def maximumBeauty(self, flowers: List[int], newFlowers: int, target: int, full: int, partial: int) -> int:\n n = len(flowers)\n flowers.sort() \n originally_complete = sum(flowers[i] >= target for i in range(n))\n work_end = n - originally_complete\n # [0, j-1] \u2014 incomplete (with >=`level` flowers each, where `0 <= `level` <= target - 1) \n # [j, work_end-1] \u2014 complete\n # [work_end, n-1] \u2014 originally complete\n\n i = -1\n level = 0\n def maximize_min_number_of_flowers() -> None:\n nonlocal i, level, newFlowers\n if j == 0:\n return\n if i == -1:\n i = 0\n level = flowers[0]\n while i < j:\n next_level = flowers[i+1] if i + 1 < j else target - 1\n max_boost = min(next_level - level, newFlowers // (i+1))\n level += max_boost\n newFlowers -= max_boost * (i+1)\n if level != next_level:\n break\n i += 1\n \n j = work_end\n def add_complete_garden() -> bool:\n nonlocal j, newFlowers\n if j - 1 < 0 or newFlowers < target - flowers[j-1]:\n return False\n newFlowers -= target - flowers[j-1]\n j -= 1\n return True\n def remove_complete_garden() -> None:\n nonlocal j, newFlowers\n assert j < work_end\n newFlowers += target - flowers[j]\n flowers[j] = flowers[j]\n j += 1\n\n while add_complete_garden():\n pass\n maximize_min_number_of_flowers()\n ans = full * (n - j) + partial * level\n while j < work_end:\n remove_complete_garden()\n maximize_min_number_of_flowers()\n ans = max(ans, full * (n - j) + partial * level)\n return ans\n``` | 1 | Alice is a caretaker of `n` gardens and she wants to plant flowers to maximize the total beauty of all her gardens.
You are given a **0-indexed** integer array `flowers` of size `n`, where `flowers[i]` is the number of flowers already planted in the `ith` garden. Flowers that are already planted **cannot** be removed. You are then given another integer `newFlowers`, which is the **maximum** number of flowers that Alice can additionally plant. You are also given the integers `target`, `full`, and `partial`.
A garden is considered **complete** if it has **at least** `target` flowers. The **total beauty** of the gardens is then determined as the **sum** of the following:
* The number of **complete** gardens multiplied by `full`.
* The **minimum** number of flowers in any of the **incomplete** gardens multiplied by `partial`. If there are no incomplete gardens, then this value will be `0`.
Return _the **maximum** total beauty that Alice can obtain after planting at most_ `newFlowers` _flowers._
**Example 1:**
**Input:** flowers = \[1,3,1,1\], newFlowers = 7, target = 6, full = 12, partial = 1
**Output:** 14
**Explanation:** Alice can plant
- 2 flowers in the 0th garden
- 3 flowers in the 1st garden
- 1 flower in the 2nd garden
- 1 flower in the 3rd garden
The gardens will then be \[3,6,2,2\]. She planted a total of 2 + 3 + 1 + 1 = 7 flowers.
There is 1 garden that is complete.
The minimum number of flowers in the incomplete gardens is 2.
Thus, the total beauty is 1 \* 12 + 2 \* 1 = 12 + 2 = 14.
No other way of planting flowers can obtain a total beauty higher than 14.
**Example 2:**
**Input:** flowers = \[2,4,5,3\], newFlowers = 10, target = 5, full = 2, partial = 6
**Output:** 30
**Explanation:** Alice can plant
- 3 flowers in the 0th garden
- 0 flowers in the 1st garden
- 0 flowers in the 2nd garden
- 2 flowers in the 3rd garden
The gardens will then be \[5,4,5,5\]. She planted a total of 3 + 0 + 0 + 2 = 5 flowers.
There are 3 gardens that are complete.
The minimum number of flowers in the incomplete gardens is 4.
Thus, the total beauty is 3 \* 2 + 4 \* 6 = 6 + 24 = 30.
No other way of planting flowers can obtain a total beauty higher than 30.
Note that Alice could make all the gardens complete but in this case, she would obtain a lower total beauty.
**Constraints:**
* `1 <= flowers.length <= 105`
* `1 <= flowers[i], target <= 105`
* `1 <= newFlowers <= 1010`
* `1 <= full, partial <= 105` | Can we divide the array into non-overlapping subsequences and simplify the problem? In the final array, arr[i-k] ≤ arr[i] should hold. We can use this to divide the array into at most k non-overlapping sequences, where arr[i] will belong to the (i%k)th sequence. Now our problem boils down to performing the minimum operations on each sequence such that it becomes non-decreasing. Our answer will be the sum of operations on each sequence. Which indices of a sequence should we not change in order to count the minimum operations? Can finding the longest non-decreasing subsequence of the sequence help? |
[Python3] 2-pointer | maximum-total-beauty-of-the-gardens | 0 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/af907302ab84ad94ecb97eeeb9b0bfa529041e30) for solutions of weekly 288. \n\n```\nclass Solution:\n def maximumBeauty(self, flowers: List[int], newFlowers: int, target: int, full: int, partial: int) -> int:\n flowers = sorted(min(target, x) for x in flowers)\n prefix = [0]\n ii = -1 \n for i in range(len(flowers)): \n if flowers[i] < target: ii = i \n if i: prefix.append(prefix[-1] + (flowers[i]-flowers[i-1])*i)\n ans = 0 \n for k in range(len(flowers)+1): \n if k: newFlowers -= target - flowers[-k]\n if newFlowers >= 0: \n while 0 <= ii and (ii+k >= len(flowers) or prefix[ii] > newFlowers): ii -= 1\n if 0 <= ii: kk = min(target-1, flowers[ii] + (newFlowers - prefix[ii])//(ii+1))\n else: kk = 0 \n ans = max(ans, k*full + kk*partial)\n return ans \n``` | 1 | Alice is a caretaker of `n` gardens and she wants to plant flowers to maximize the total beauty of all her gardens.
You are given a **0-indexed** integer array `flowers` of size `n`, where `flowers[i]` is the number of flowers already planted in the `ith` garden. Flowers that are already planted **cannot** be removed. You are then given another integer `newFlowers`, which is the **maximum** number of flowers that Alice can additionally plant. You are also given the integers `target`, `full`, and `partial`.
A garden is considered **complete** if it has **at least** `target` flowers. The **total beauty** of the gardens is then determined as the **sum** of the following:
* The number of **complete** gardens multiplied by `full`.
* The **minimum** number of flowers in any of the **incomplete** gardens multiplied by `partial`. If there are no incomplete gardens, then this value will be `0`.
Return _the **maximum** total beauty that Alice can obtain after planting at most_ `newFlowers` _flowers._
**Example 1:**
**Input:** flowers = \[1,3,1,1\], newFlowers = 7, target = 6, full = 12, partial = 1
**Output:** 14
**Explanation:** Alice can plant
- 2 flowers in the 0th garden
- 3 flowers in the 1st garden
- 1 flower in the 2nd garden
- 1 flower in the 3rd garden
The gardens will then be \[3,6,2,2\]. She planted a total of 2 + 3 + 1 + 1 = 7 flowers.
There is 1 garden that is complete.
The minimum number of flowers in the incomplete gardens is 2.
Thus, the total beauty is 1 \* 12 + 2 \* 1 = 12 + 2 = 14.
No other way of planting flowers can obtain a total beauty higher than 14.
**Example 2:**
**Input:** flowers = \[2,4,5,3\], newFlowers = 10, target = 5, full = 2, partial = 6
**Output:** 30
**Explanation:** Alice can plant
- 3 flowers in the 0th garden
- 0 flowers in the 1st garden
- 0 flowers in the 2nd garden
- 2 flowers in the 3rd garden
The gardens will then be \[5,4,5,5\]. She planted a total of 3 + 0 + 0 + 2 = 5 flowers.
There are 3 gardens that are complete.
The minimum number of flowers in the incomplete gardens is 4.
Thus, the total beauty is 3 \* 2 + 4 \* 6 = 6 + 24 = 30.
No other way of planting flowers can obtain a total beauty higher than 30.
Note that Alice could make all the gardens complete but in this case, she would obtain a lower total beauty.
**Constraints:**
* `1 <= flowers.length <= 105`
* `1 <= flowers[i], target <= 105`
* `1 <= newFlowers <= 1010`
* `1 <= full, partial <= 105` | Can we divide the array into non-overlapping subsequences and simplify the problem? In the final array, arr[i-k] ≤ arr[i] should hold. We can use this to divide the array into at most k non-overlapping sequences, where arr[i] will belong to the (i%k)th sequence. Now our problem boils down to performing the minimum operations on each sequence such that it becomes non-decreasing. Our answer will be the sum of operations on each sequence. Which indices of a sequence should we not change in order to count the minimum operations? Can finding the longest non-decreasing subsequence of the sequence help? |
Python3 with comments | maximum-total-beauty-of-the-gardens | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n- We will sort the gardens to have the small values in front and big values in the end.\n- We will first remove as many full gardens as possible.\n- We will then spend all the remaining newFlowers to increase the minimum\n- We will then return the removed gardens one by one and repeat the previous step to find the max beauty at each step.\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: nlogn\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: n\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maximumBeauty(self, flowers: List[int], newFlowers: int, target: int, full: int, partial: int) -> int:\n\n flowers.sort()\n\n #Remove all already full gardens\n count = 0\n while flowers and flowers[-1] >= target:\n count += 1\n flowers.pop()\n\n #Complete as many gardens as possible\n stack = []\n while flowers and newFlowers >= target - flowers[-1]:\n stack.append(max(0, target - flowers.pop()))\n newFlowers -= stack[-1]\n \n mini = 0\n i = 0\n #Spend all available newFlowers \n if flowers:\n while i < len(flowers) and (flowers[i] - mini) * i <= newFlowers:\n newFlowers -= (flowers[i] - mini) * i\n mini = flowers[i]\n i += 1\n mini += newFlowers // i\n newFlowers %= i\n \n #Max beauty\n maxi = (count + len(stack)) * full + mini * partial\n\n while stack:\n\n #Unplant flowers from one of the completed gardens\n newFlowers += stack[-1]\n flowers.append(target - stack.pop())\n\n #Reduce mini to match the added garden if necessary\n if flowers[-1] < mini:\n newFlowers += i * (mini - flowers[-1])\n mini = flowers[-1]\n\n #Spend available newFlowers\n while i < len(flowers) and (flowers[i] - mini) * i <= newFlowers:\n newFlowers -= (flowers[i] - mini) * i\n mini = flowers[i]\n i += 1\n mini += newFlowers // i\n newFlowers %= i\n\n #Update max beauty\n maxi = max(maxi, (count + len(stack)) * full + min(mini, target - 1) * partial)\n \n return maxi\n``` | 0 | Alice is a caretaker of `n` gardens and she wants to plant flowers to maximize the total beauty of all her gardens.
You are given a **0-indexed** integer array `flowers` of size `n`, where `flowers[i]` is the number of flowers already planted in the `ith` garden. Flowers that are already planted **cannot** be removed. You are then given another integer `newFlowers`, which is the **maximum** number of flowers that Alice can additionally plant. You are also given the integers `target`, `full`, and `partial`.
A garden is considered **complete** if it has **at least** `target` flowers. The **total beauty** of the gardens is then determined as the **sum** of the following:
* The number of **complete** gardens multiplied by `full`.
* The **minimum** number of flowers in any of the **incomplete** gardens multiplied by `partial`. If there are no incomplete gardens, then this value will be `0`.
Return _the **maximum** total beauty that Alice can obtain after planting at most_ `newFlowers` _flowers._
**Example 1:**
**Input:** flowers = \[1,3,1,1\], newFlowers = 7, target = 6, full = 12, partial = 1
**Output:** 14
**Explanation:** Alice can plant
- 2 flowers in the 0th garden
- 3 flowers in the 1st garden
- 1 flower in the 2nd garden
- 1 flower in the 3rd garden
The gardens will then be \[3,6,2,2\]. She planted a total of 2 + 3 + 1 + 1 = 7 flowers.
There is 1 garden that is complete.
The minimum number of flowers in the incomplete gardens is 2.
Thus, the total beauty is 1 \* 12 + 2 \* 1 = 12 + 2 = 14.
No other way of planting flowers can obtain a total beauty higher than 14.
**Example 2:**
**Input:** flowers = \[2,4,5,3\], newFlowers = 10, target = 5, full = 2, partial = 6
**Output:** 30
**Explanation:** Alice can plant
- 3 flowers in the 0th garden
- 0 flowers in the 1st garden
- 0 flowers in the 2nd garden
- 2 flowers in the 3rd garden
The gardens will then be \[5,4,5,5\]. She planted a total of 3 + 0 + 0 + 2 = 5 flowers.
There are 3 gardens that are complete.
The minimum number of flowers in the incomplete gardens is 4.
Thus, the total beauty is 3 \* 2 + 4 \* 6 = 6 + 24 = 30.
No other way of planting flowers can obtain a total beauty higher than 30.
Note that Alice could make all the gardens complete but in this case, she would obtain a lower total beauty.
**Constraints:**
* `1 <= flowers.length <= 105`
* `1 <= flowers[i], target <= 105`
* `1 <= newFlowers <= 1010`
* `1 <= full, partial <= 105` | Can we divide the array into non-overlapping subsequences and simplify the problem? In the final array, arr[i-k] ≤ arr[i] should hold. We can use this to divide the array into at most k non-overlapping sequences, where arr[i] will belong to the (i%k)th sequence. Now our problem boils down to performing the minimum operations on each sequence such that it becomes non-decreasing. Our answer will be the sum of operations on each sequence. Which indices of a sequence should we not change in order to count the minimum operations? Can finding the longest non-decreasing subsequence of the sequence help? |
Python3 fast solution | maximum-total-beauty-of-the-gardens | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->O(n log n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ --> O(n)\n\n# Code\n```\nclass Solution:\n def maximumBeauty(self, flowers: List[int], newFlowers: int, target: int, full: int, partial: int) -> int:\n\n flowers.sort()\n n = len(flowers)\n\n # 1: exclude complete gardens\n m = bisect.bisect_left(flowers, target)\n init = (n - m) * full\n \n if m == 0:\n return init\n\n # 2. pre-processing\n need = [0] * m # to flood till flowers[index], how many more flowers needed\n for index in range(1, m):\n need[index] = need[index - 1] + index * (flowers[index] - flowers[index - 1])\n\n # gardens are all partials:\n ans = self.partial_garden(need, newFlowers, flowers, target, partial, m)\n \n # 3. loop all possible new complete garden index numbers\n for index in range(m - 1, -1, -1):\n if newFlowers + flowers[index] < target:\n break\n else:\n newFlowers -= (target - flowers[index]) # fill current \'index\' garden to be full\n \n cur_partial = self.partial_garden(need, newFlowers, flowers, target, partial, index)\n \n ans = max(ans, cur_partial + full * (m - index))\n \n return ans + init\n\n\n def partial_garden(self, nums, newFlowers, flowers, target, partial, ind):\n if ind == 0:\n return 0\n index = bisect.bisect_right(nums, newFlowers)\n index = min(index, ind)\n min_val = min(target - 1, flowers[index - 1] + (newFlowers - nums[index - 1]) // index)\n return min_val * partial\n \n\n\n``` | 0 | Alice is a caretaker of `n` gardens and she wants to plant flowers to maximize the total beauty of all her gardens.
You are given a **0-indexed** integer array `flowers` of size `n`, where `flowers[i]` is the number of flowers already planted in the `ith` garden. Flowers that are already planted **cannot** be removed. You are then given another integer `newFlowers`, which is the **maximum** number of flowers that Alice can additionally plant. You are also given the integers `target`, `full`, and `partial`.
A garden is considered **complete** if it has **at least** `target` flowers. The **total beauty** of the gardens is then determined as the **sum** of the following:
* The number of **complete** gardens multiplied by `full`.
* The **minimum** number of flowers in any of the **incomplete** gardens multiplied by `partial`. If there are no incomplete gardens, then this value will be `0`.
Return _the **maximum** total beauty that Alice can obtain after planting at most_ `newFlowers` _flowers._
**Example 1:**
**Input:** flowers = \[1,3,1,1\], newFlowers = 7, target = 6, full = 12, partial = 1
**Output:** 14
**Explanation:** Alice can plant
- 2 flowers in the 0th garden
- 3 flowers in the 1st garden
- 1 flower in the 2nd garden
- 1 flower in the 3rd garden
The gardens will then be \[3,6,2,2\]. She planted a total of 2 + 3 + 1 + 1 = 7 flowers.
There is 1 garden that is complete.
The minimum number of flowers in the incomplete gardens is 2.
Thus, the total beauty is 1 \* 12 + 2 \* 1 = 12 + 2 = 14.
No other way of planting flowers can obtain a total beauty higher than 14.
**Example 2:**
**Input:** flowers = \[2,4,5,3\], newFlowers = 10, target = 5, full = 2, partial = 6
**Output:** 30
**Explanation:** Alice can plant
- 3 flowers in the 0th garden
- 0 flowers in the 1st garden
- 0 flowers in the 2nd garden
- 2 flowers in the 3rd garden
The gardens will then be \[5,4,5,5\]. She planted a total of 3 + 0 + 0 + 2 = 5 flowers.
There are 3 gardens that are complete.
The minimum number of flowers in the incomplete gardens is 4.
Thus, the total beauty is 3 \* 2 + 4 \* 6 = 6 + 24 = 30.
No other way of planting flowers can obtain a total beauty higher than 30.
Note that Alice could make all the gardens complete but in this case, she would obtain a lower total beauty.
**Constraints:**
* `1 <= flowers.length <= 105`
* `1 <= flowers[i], target <= 105`
* `1 <= newFlowers <= 1010`
* `1 <= full, partial <= 105` | Can we divide the array into non-overlapping subsequences and simplify the problem? In the final array, arr[i-k] ≤ arr[i] should hold. We can use this to divide the array into at most k non-overlapping sequences, where arr[i] will belong to the (i%k)th sequence. Now our problem boils down to performing the minimum operations on each sequence such that it becomes non-decreasing. Our answer will be the sum of operations on each sequence. Which indices of a sequence should we not change in order to count the minimum operations? Can finding the longest non-decreasing subsequence of the sequence help? |
[Python] Prefix sum with binary search O(NlogN) + intuition explained | maximum-total-beauty-of-the-gardens | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIntuition would be first sort the array, since the position of the garden doesn\'t matter.\n\nThen we consider the following:\n## Do we binary search the maximum score?\n - After some thinking, determine whether a value can be achieved is hard, since there\'s no greedy approach to get an achievable solution.\n\n## Do we binary search the number of garden to fill & threshold to be partial?\n - Notice that when total full garden increase(total score +), the minimum value of partial garden decreses(total score -), which don\'t look like a monotonic problem.\n\n## Then we have an observation of the cost, apparently it consist of 3 parts, fill_cost, align_cost and spread cost. \n- Fill cost: the cost to fill $i$ largerst garden to target\n- Align cost: the cost to align previous garden to $i_{th}$ garden (which increase the minimun value).\n- Speard cost: left flowers can\'t align to $i+1_{th}$ element, but can increase the minimum value per the number of all aligned elements. \n## How to calculate the each cost?\n- using prefix sum to avoid summing up the previous k cost everytime\n- Fill cost:\n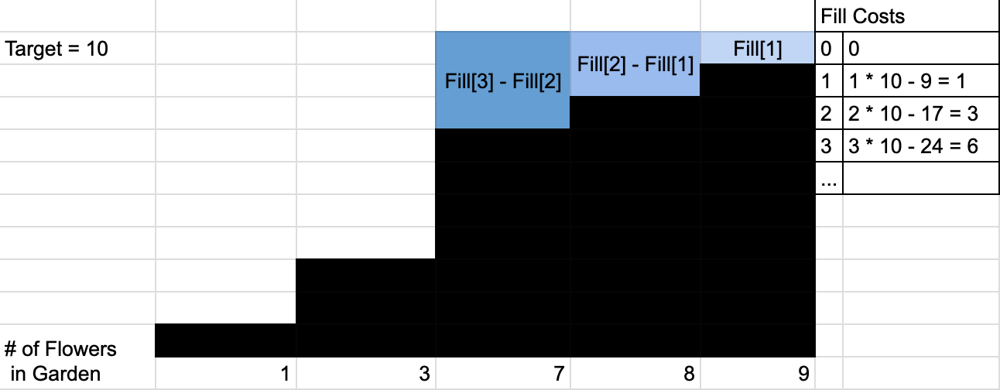\n- Align cost:\n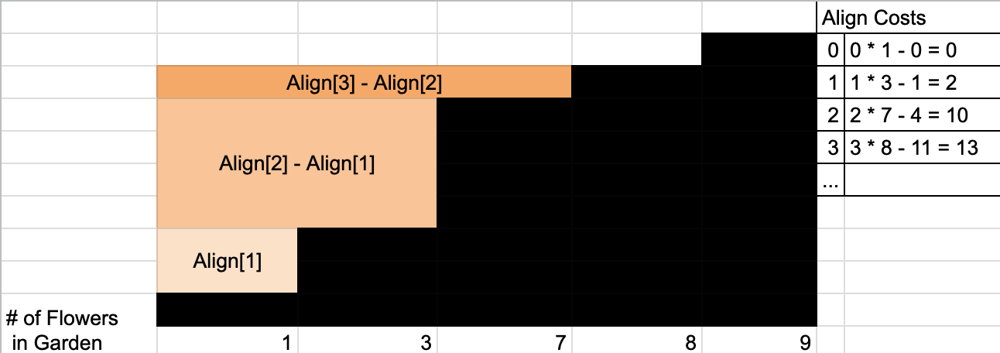\n- Spread cost:\n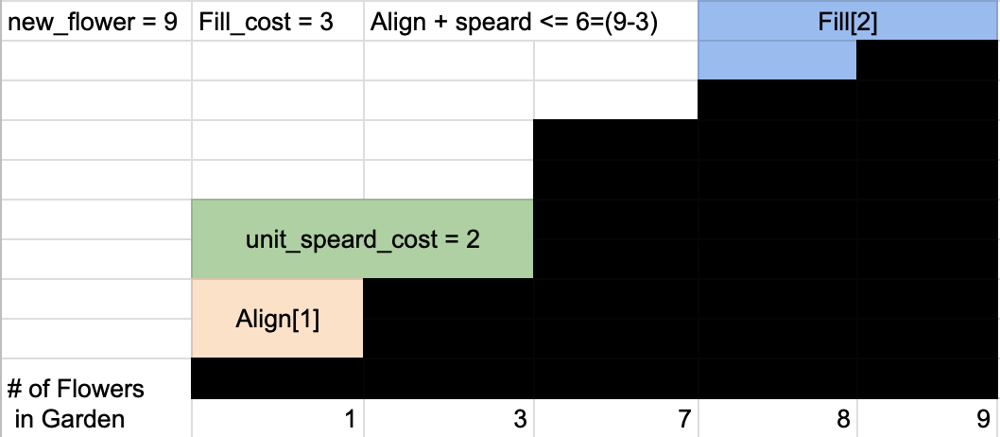\n\n## Why align cost then other?\nThe pain point is to determine what\'s min partial after the fill, by using bisect_right, we not only have the cost of align previous $i - 1$ element to $i_{th}$, but also get the unit cost to increase the minimum value by 1.\n\n# Edge cases\n- new flower too small / =0?\n- new flower too big?\n - If we have enough flowers to fill all garden to target, the optimal solution would be fill all garden or left 1 garden to be target - 1 since partial >= 0\n - We should cap the \n- All garden already reach the target?\n - Do nothing, return # of garden * full\n- \n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nsort: $O(NlogN)$\niterate thru c1: $O(N)$\nbinary search thru c2: $O(logN)$\ntotal: $O(NlogN) + O(N) * O(logN) = O(NlogN)$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$O(N)$\n# Code\n```\nclass Solution:\n def maximumBeauty(self, flowers: List[int], nf: int, target: int, full: int, partial: int) -> int:\n flowers, base = sorted([v for v in flowers if v < target], reverse=True), len([v for v in flowers if v >= target]) * full\n if flowers == []:\n return base\n n = len(flowers)\n c1, c2 = [], []\n # calculate C1, the fill cost\n ttl = 0\n for i, v in enumerate(flowers):\n c1.append(i * target - ttl)\n ttl += v\n c1.append(n * target - ttl)\n\n # calculate C2, the align cost\n flowers.reverse()\n ttl = 0\n for i, v in enumerate(flowers):\n c2.append(i * v - ttl)\n ttl += v\n c2.append(n * target - ttl)\n\n # \n if nf >= c2[-1]:\n return base + full * (n-1) + max(full, (target - 1) * partial)\n\n maxV = 0\n for k1, fc in enumerate(c1):\n # only continue if we have enough flower to fill k1 number of garden\n if fc > nf:\n break\n # set the upper bound to n - k1 to avoid overlap\n j = bisect_right(c2, nf - fc, 0, n - k1)\n\n # flowers_left = total_flower - fill_cost[k1] - align_cost[j - 1]\n npv = min(flowers[j - 1] + (nf - fc - c2[j - 1]) // j, target - 1)\n nv = full * k1 + npv * partial\n maxV = max(maxV, nv)\n return base + maxV\n\n \n``` | 0 | Alice is a caretaker of `n` gardens and she wants to plant flowers to maximize the total beauty of all her gardens.
You are given a **0-indexed** integer array `flowers` of size `n`, where `flowers[i]` is the number of flowers already planted in the `ith` garden. Flowers that are already planted **cannot** be removed. You are then given another integer `newFlowers`, which is the **maximum** number of flowers that Alice can additionally plant. You are also given the integers `target`, `full`, and `partial`.
A garden is considered **complete** if it has **at least** `target` flowers. The **total beauty** of the gardens is then determined as the **sum** of the following:
* The number of **complete** gardens multiplied by `full`.
* The **minimum** number of flowers in any of the **incomplete** gardens multiplied by `partial`. If there are no incomplete gardens, then this value will be `0`.
Return _the **maximum** total beauty that Alice can obtain after planting at most_ `newFlowers` _flowers._
**Example 1:**
**Input:** flowers = \[1,3,1,1\], newFlowers = 7, target = 6, full = 12, partial = 1
**Output:** 14
**Explanation:** Alice can plant
- 2 flowers in the 0th garden
- 3 flowers in the 1st garden
- 1 flower in the 2nd garden
- 1 flower in the 3rd garden
The gardens will then be \[3,6,2,2\]. She planted a total of 2 + 3 + 1 + 1 = 7 flowers.
There is 1 garden that is complete.
The minimum number of flowers in the incomplete gardens is 2.
Thus, the total beauty is 1 \* 12 + 2 \* 1 = 12 + 2 = 14.
No other way of planting flowers can obtain a total beauty higher than 14.
**Example 2:**
**Input:** flowers = \[2,4,5,3\], newFlowers = 10, target = 5, full = 2, partial = 6
**Output:** 30
**Explanation:** Alice can plant
- 3 flowers in the 0th garden
- 0 flowers in the 1st garden
- 0 flowers in the 2nd garden
- 2 flowers in the 3rd garden
The gardens will then be \[5,4,5,5\]. She planted a total of 3 + 0 + 0 + 2 = 5 flowers.
There are 3 gardens that are complete.
The minimum number of flowers in the incomplete gardens is 4.
Thus, the total beauty is 3 \* 2 + 4 \* 6 = 6 + 24 = 30.
No other way of planting flowers can obtain a total beauty higher than 30.
Note that Alice could make all the gardens complete but in this case, she would obtain a lower total beauty.
**Constraints:**
* `1 <= flowers.length <= 105`
* `1 <= flowers[i], target <= 105`
* `1 <= newFlowers <= 1010`
* `1 <= full, partial <= 105` | Can we divide the array into non-overlapping subsequences and simplify the problem? In the final array, arr[i-k] ≤ arr[i] should hold. We can use this to divide the array into at most k non-overlapping sequences, where arr[i] will belong to the (i%k)th sequence. Now our problem boils down to performing the minimum operations on each sequence such that it becomes non-decreasing. Our answer will be the sum of operations on each sequence. Which indices of a sequence should we not change in order to count the minimum operations? Can finding the longest non-decreasing subsequence of the sequence help? |
My most difficult problem that Leedcode has solved 😂😂 | add-two-integers | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def sum(self, num1: int, num2: int) -> int:\n return num1 + num2\n \n``` | 2 | Given two integers `num1` and `num2`, return _the **sum** of the two integers_.
**Example 1:**
**Input:** num1 = 12, num2 = 5
**Output:** 17
**Explanation:** num1 is 12, num2 is 5, and their sum is 12 + 5 = 17, so 17 is returned.
**Example 2:**
**Input:** num1 = -10, num2 = 4
**Output:** -6
**Explanation:** num1 + num2 = -6, so -6 is returned.
**Constraints:**
* `-100 <= num1, num2 <= 100` | Firstly, try to find all the words present in the string. On the basis of each word's lengths, simulate the process explained in Problem. |
Simple Integer Addition using Python | add-two-integers | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe need to calculate and return the sum of two integers, which can be done using the \'+\' operator in Python.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nOur approach is simple: we will use the \'+\' operator to add the two input integers and return the result.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity of this approach is O(1) because the operation itself is constant time and the input is fixed. Regardless of the input, the operation will always take the same time.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity is also O(1) because we are not using any additional data structures that depend on the input size.\n\n# Code\n```\nclass Solution:\n def sum(self, num1: int, num2: int) -> int:\n return num1 + num2\n``` | 4 | Given two integers `num1` and `num2`, return _the **sum** of the two integers_.
**Example 1:**
**Input:** num1 = 12, num2 = 5
**Output:** 17
**Explanation:** num1 is 12, num2 is 5, and their sum is 12 + 5 = 17, so 17 is returned.
**Example 2:**
**Input:** num1 = -10, num2 = 4
**Output:** -6
**Explanation:** num1 + num2 = -6, so -6 is returned.
**Constraints:**
* `-100 <= num1, num2 <= 100` | Firstly, try to find all the words present in the string. On the basis of each word's lengths, simulate the process explained in Problem. |
One line solution of add two Integers problem | add-two-integers | 0 | 1 | # Code\n```\nclass Solution:\n def sum(self, num1: int, num2: int) -> int:\n return num1 + num2\n``` | 1 | Given two integers `num1` and `num2`, return _the **sum** of the two integers_.
**Example 1:**
**Input:** num1 = 12, num2 = 5
**Output:** 17
**Explanation:** num1 is 12, num2 is 5, and their sum is 12 + 5 = 17, so 17 is returned.
**Example 2:**
**Input:** num1 = -10, num2 = 4
**Output:** -6
**Explanation:** num1 + num2 = -6, so -6 is returned.
**Constraints:**
* `-100 <= num1, num2 <= 100` | Firstly, try to find all the words present in the string. On the basis of each word's lengths, simulate the process explained in Problem. |
Python simple solution | add-two-integers | 0 | 1 | # Complexity\n- Time complexity:\nO(1)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def sum(self, num1: int, num2: int) -> int:\n return num1 + num2\n``` | 8 | Given two integers `num1` and `num2`, return _the **sum** of the two integers_.
**Example 1:**
**Input:** num1 = 12, num2 = 5
**Output:** 17
**Explanation:** num1 is 12, num2 is 5, and their sum is 12 + 5 = 17, so 17 is returned.
**Example 2:**
**Input:** num1 = -10, num2 = 4
**Output:** -6
**Explanation:** num1 + num2 = -6, so -6 is returned.
**Constraints:**
* `-100 <= num1, num2 <= 100` | Firstly, try to find all the words present in the string. On the basis of each word's lengths, simulate the process explained in Problem. |
Python simple solution | add-two-integers | 0 | 1 | **There\u2019s nothing to write on it write a recipe for pancakes**\n\n# Code\n```\nclass Solution:\n def sum(self, num1: int, num2: int) -> int:\n return num1 + num2\n \n```\n\n**Pancake Ingredients**\nYou likely already have everything you need to make this pancake recipe. If not, here\'s what to add to your grocery list:\n\n\xB7 Flour: This homemade pancake recipe starts with all-purpose flour.\n\xB7 Baking powder: Baking powder, a leavener, is the secret to fluffy pancakes.\n\xB7 Sugar: Just a tablespoon of white sugar is all you\'ll need for subtly sweet pancakes.\n\xB7 Salt: A pinch of salt will enhance the overall flavor without making your pancakes taste salty.\n\xB7 Milk and butter: Milk and butter add moisture and richness to the pancakes.\n\xB7 Egg: A whole egg lends even more moisture. Plus, it helps bind the pancake batter together.\n\n**How to Make Pancakes From Scratch**\nIt\'s not hard to make homemade pancakes \u2014 you just need a good recipe. That\'s where we come in! You\'ll find the step-by-step recipe below, but here\'s a brief overview of what you can expect:\n\n1. Sift the dry ingredients together.\n2. Make a well, then add the wet ingredients. Stir to combine.\n3. Scoop the batter onto a hot griddle or pan.\n4. Cook for two to three minutes, then flip.\n5. Continue cooking until brown on both sides.\n\n**When to Flip Pancakes**\nYour pancake will tell you when it\'s ready to flip. Wait until bubbles start to form on the top and the edges look dry and set. This will usually take about two to\nthree minutes on each side.\n\n# Code\n```\nclass Solution:\n def sum(self, num1: int, num2: int) -> int:\n return num1 + num2\n \n``` | 12 | Given two integers `num1` and `num2`, return _the **sum** of the two integers_.
**Example 1:**
**Input:** num1 = 12, num2 = 5
**Output:** 17
**Explanation:** num1 is 12, num2 is 5, and their sum is 12 + 5 = 17, so 17 is returned.
**Example 2:**
**Input:** num1 = -10, num2 = 4
**Output:** -6
**Explanation:** num1 + num2 = -6, so -6 is returned.
**Constraints:**
* `-100 <= num1, num2 <= 100` | Firstly, try to find all the words present in the string. On the basis of each word's lengths, simulate the process explained in Problem. |
Python using list | add-two-integers | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def sum(self, num1: int, num2: int) -> int:\n return sum([num1, num2])\n``` | 2 | Given two integers `num1` and `num2`, return _the **sum** of the two integers_.
**Example 1:**
**Input:** num1 = 12, num2 = 5
**Output:** 17
**Explanation:** num1 is 12, num2 is 5, and their sum is 12 + 5 = 17, so 17 is returned.
**Example 2:**
**Input:** num1 = -10, num2 = 4
**Output:** -6
**Explanation:** num1 + num2 = -6, so -6 is returned.
**Constraints:**
* `-100 <= num1, num2 <= 100` | Firstly, try to find all the words present in the string. On the basis of each word's lengths, simulate the process explained in Problem. |
Solutions in Every Language *on leetcode* | One-Liner ✅ | add-two-integers | 1 | 1 | **1. C++**\n```\nclass Solution {\npublic:\n int sum(int num1, int num2) {\n return num1 + num2;\n }\n};\n```\n**2. Java**\n```\nclass Solution {\n public int sum(int num1, int num2) {\n return num1 + num2;\n }\n}\n```\n**3. Python3**\n```\nclass Solution:\n def sum(self, num1: int, num2: int) -> int:\n return num1 + num2\n```\n\u261D\uFE0F which is not actually necessary\n\nYou can do this instead:\n```\nclass Solution:\n sum = add\n```\n\n**4. C**\n```\nint sum(int num1, int num2){\n return num1 + num2;\n}\n```\n**5. C#**\n```\npublic class Solution {\n public int Sum(int num1, int num2) {\n return num1 + num2;\n }\n}\n```\n**6. JavaScript**\n```\nvar sum = function(num1, num2) {\n return num1 + num2;\n};\n```\n**7. Ruby**\n```\ndef sum(num1, num2)\n num1 + num2\nend\n```\n**8. Swift**\n```\nclass Solution {\n func sum(_ num1: Int, _ num2: Int) -> Int {\n return num1 + num2\n }\n}\n```\n**9. Go**\n```\nfunc sum(num1 int, num2 int) int {\n return num1 + num2\n}\n```\n**10. Scala**\n```\nobject Solution {\n def sum(num1: Int, num2: Int): Int = {\n return num1 + num2\n }\n}\n```\n**11. Kotlin**\n```\nclass Solution {\n fun sum(num1: Int, num2: Int): Int {\n return num1 + num2\n }\n}\n```\n**12. Rust**\n```\nimpl Solution {\n pub fn sum(num1: i32, num2: i32) -> i32 {\n return num1 + num2\n }\n}\n```\n**13. PHP**\n```\nclass Solution {\n function sum($num1, $num2) {\n return $num1 + $num2;\n }\n}\n```\n**14. Typescript**\n```\nfunction sum(num1: number, num2: number): number {\n return num1 + num2\n};\n```\n**15. Racket**\n```\n(define/contract (sum num1 num2)\n (-> exact-integer? exact-integer? exact-integer?)\n (+ num1 num2)\n )\n```\n**16. Erlang**\n```\n-spec sum(Num1 :: integer(), Num2 :: integer()) -> integer().\nsum(Num1, Num2) ->\n Num1 + Num2.\n```\n**17. Elixir**\n```\ndefmodule Solution do\n @spec sum(num1 :: integer, num2 :: integer) :: integer\n def sum(num1, num2) do\n num1 + num2\n end\nend\n```\n**18. Dart**\n```\nclass Solution {\n int sum(int num1, int num2) {\n return num1 + num2;\n }\n}\n```\nThat\'s it... Upvote if you liked this solution | 23 | Given two integers `num1` and `num2`, return _the **sum** of the two integers_.
**Example 1:**
**Input:** num1 = 12, num2 = 5
**Output:** 17
**Explanation:** num1 is 12, num2 is 5, and their sum is 12 + 5 = 17, so 17 is returned.
**Example 2:**
**Input:** num1 = -10, num2 = 4
**Output:** -6
**Explanation:** num1 + num2 = -6, so -6 is returned.
**Constraints:**
* `-100 <= num1, num2 <= 100` | Firstly, try to find all the words present in the string. On the basis of each word's lengths, simulate the process explained in Problem. |
Solution | root-equals-sum-of-children | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n bool checkTree(TreeNode* root) {\n if(root==NULL)\n return false;\n if(root->left->val+root->right->val==root->val)\n return true;\n else\n return false;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def checkTree(self, root: Optional[TreeNode]) -> bool:\n return root.val == (root.left.val + root.right.val)\n```\n\n```Java []\nclass Solution {\n public boolean checkTree(TreeNode root) {\n return root.left.val + root.right.val == root.val ? true : false; \n }\n}\n```\n | 4 | You are given the `root` of a **binary tree** that consists of exactly `3` nodes: the root, its left child, and its right child.
Return `true` _if the value of the root is equal to the **sum** of the values of its two children, or_ `false` _otherwise_.
**Example 1:**
**Input:** root = \[10,4,6\]
**Output:** true
**Explanation:** The values of the root, its left child, and its right child are 10, 4, and 6, respectively.
10 is equal to 4 + 6, so we return true.
**Example 2:**
**Input:** root = \[5,3,1\]
**Output:** false
**Explanation:** The values of the root, its left child, and its right child are 5, 3, and 1, respectively.
5 is not equal to 3 + 1, so we return false.
**Constraints:**
* The tree consists only of the root, its left child, and its right child.
* `-100 <= Node.val <= 100` | How can "reversing" a part of the linked list help find the answer? We know that the nodes of the first half are twins of nodes in the second half, so try dividing the linked list in half and reverse the second half. How can two pointers be used to find every twin sum optimally? Use two different pointers pointing to the first nodes of the two halves of the linked list. The second pointer will point to the first node of the reversed half, which is the (n-1-i)th node in the original linked list. By moving both pointers forward at the same time, we find all twin sums. |
Python || One Line Solution || Easy | root-equals-sum-of-children | 0 | 1 | ```\nclass Solution:\n def checkTree(self, root: Optional[TreeNode]) -> bool:\n return root.val==root.left.val+root.right.val\n```\n**An upvote will be encouraging**\n | 11 | You are given the `root` of a **binary tree** that consists of exactly `3` nodes: the root, its left child, and its right child.
Return `true` _if the value of the root is equal to the **sum** of the values of its two children, or_ `false` _otherwise_.
**Example 1:**
**Input:** root = \[10,4,6\]
**Output:** true
**Explanation:** The values of the root, its left child, and its right child are 10, 4, and 6, respectively.
10 is equal to 4 + 6, so we return true.
**Example 2:**
**Input:** root = \[5,3,1\]
**Output:** false
**Explanation:** The values of the root, its left child, and its right child are 5, 3, and 1, respectively.
5 is not equal to 3 + 1, so we return false.
**Constraints:**
* The tree consists only of the root, its left child, and its right child.
* `-100 <= Node.val <= 100` | How can "reversing" a part of the linked list help find the answer? We know that the nodes of the first half are twins of nodes in the second half, so try dividing the linked list in half and reverse the second half. How can two pointers be used to find every twin sum optimally? Use two different pointers pointing to the first nodes of the two halves of the linked list. The second pointer will point to the first node of the reversed half, which is the (n-1-i)th node in the original linked list. By moving both pointers forward at the same time, we find all twin sums. |
Easy to understand for beginners 🍾🍾💯 Python Easy | root-equals-sum-of-children | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nDirect Tree approach ATTACKKKKKKKKK\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def checkTree(self, root: Optional[TreeNode]) -> bool:\n return root.val==root.left.val + root.right.val\n``` | 15 | You are given the `root` of a **binary tree** that consists of exactly `3` nodes: the root, its left child, and its right child.
Return `true` _if the value of the root is equal to the **sum** of the values of its two children, or_ `false` _otherwise_.
**Example 1:**
**Input:** root = \[10,4,6\]
**Output:** true
**Explanation:** The values of the root, its left child, and its right child are 10, 4, and 6, respectively.
10 is equal to 4 + 6, so we return true.
**Example 2:**
**Input:** root = \[5,3,1\]
**Output:** false
**Explanation:** The values of the root, its left child, and its right child are 5, 3, and 1, respectively.
5 is not equal to 3 + 1, so we return false.
**Constraints:**
* The tree consists only of the root, its left child, and its right child.
* `-100 <= Node.val <= 100` | How can "reversing" a part of the linked list help find the answer? We know that the nodes of the first half are twins of nodes in the second half, so try dividing the linked list in half and reverse the second half. How can two pointers be used to find every twin sum optimally? Use two different pointers pointing to the first nodes of the two halves of the linked list. The second pointer will point to the first node of the reversed half, which is the (n-1-i)th node in the original linked list. By moving both pointers forward at the same time, we find all twin sums. |
easy python solution | find-closest-number-to-zero | 0 | 1 | \nclass Solution:\n def findClosestNumber(self, nums: List[int]) -> int:\n min=1000000\n for i in nums:\n if min>abs(i):\n min=abs(i)\n if min in nums:\n return min\n else:\n return -1*min\n \n \n``` | 2 | Given an integer array `nums` of size `n`, return _the number with the value **closest** to_ `0` _in_ `nums`. If there are multiple answers, return _the number with the **largest** value_.
**Example 1:**
**Input:** nums = \[-4,-2,1,4,8\]
**Output:** 1
**Explanation:**
The distance from -4 to 0 is |-4| = 4.
The distance from -2 to 0 is |-2| = 2.
The distance from 1 to 0 is |1| = 1.
The distance from 4 to 0 is |4| = 4.
The distance from 8 to 0 is |8| = 8.
Thus, the closest number to 0 in the array is 1.
**Example 2:**
**Input:** nums = \[2,-1,1\]
**Output:** 1
**Explanation:** 1 and -1 are both the closest numbers to 0, so 1 being larger is returned.
**Constraints:**
* `1 <= n <= 1000`
* `-105 <= nums[i] <= 105` | The constraints are not very large. Can we simulate the execution by starting from each index of s? Before any of the stopping conditions is met, stop the simulation for that index and set the answer for that index. |
Self-Explanatory Code! | find-closest-number-to-zero | 0 | 1 | \n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findClosestNumber(self, nums: List[int]) -> int:\n diff = 99999999\n ans = 999999999\n for i in nums:\n if abs(0-i) < diff:\n diff = abs(0-i)\n ans = i\n if diff == abs(0-i):\n diff = abs(0-i)\n ans = max(ans, i)\n return ans\n``` | 1 | Given an integer array `nums` of size `n`, return _the number with the value **closest** to_ `0` _in_ `nums`. If there are multiple answers, return _the number with the **largest** value_.
**Example 1:**
**Input:** nums = \[-4,-2,1,4,8\]
**Output:** 1
**Explanation:**
The distance from -4 to 0 is |-4| = 4.
The distance from -2 to 0 is |-2| = 2.
The distance from 1 to 0 is |1| = 1.
The distance from 4 to 0 is |4| = 4.
The distance from 8 to 0 is |8| = 8.
Thus, the closest number to 0 in the array is 1.
**Example 2:**
**Input:** nums = \[2,-1,1\]
**Output:** 1
**Explanation:** 1 and -1 are both the closest numbers to 0, so 1 being larger is returned.
**Constraints:**
* `1 <= n <= 1000`
* `-105 <= nums[i] <= 105` | The constraints are not very large. Can we simulate the execution by starting from each index of s? Before any of the stopping conditions is met, stop the simulation for that index and set the answer for that index. |
Python | Easy Solution✅ | find-closest-number-to-zero | 0 | 1 | # Code\u2705\n```\nclass Solution:\n def findClosestNumber(self, nums: List[int]) -> int: #// nums = [-4, -2, 1, 4, 8]\n pos, neg = [], []\n for item in nums:\n if item < 0:\n neg.append(item)\n elif item > 0:\n pos.append(item)\n else:\n return 0\n #// neg = [-4, -2] pos = [1, 4, 8] \n if not neg:\n return sorted(pos)[0]\n if not pos:\n return sorted(neg)[-1]\n \n if abs(sorted(neg)[-1]) < sorted(pos)[0]:\n return sorted(neg)[-1]\n return sorted(pos)[0]\n\n\n``` | 5 | Given an integer array `nums` of size `n`, return _the number with the value **closest** to_ `0` _in_ `nums`. If there are multiple answers, return _the number with the **largest** value_.
**Example 1:**
**Input:** nums = \[-4,-2,1,4,8\]
**Output:** 1
**Explanation:**
The distance from -4 to 0 is |-4| = 4.
The distance from -2 to 0 is |-2| = 2.
The distance from 1 to 0 is |1| = 1.
The distance from 4 to 0 is |4| = 4.
The distance from 8 to 0 is |8| = 8.
Thus, the closest number to 0 in the array is 1.
**Example 2:**
**Input:** nums = \[2,-1,1\]
**Output:** 1
**Explanation:** 1 and -1 are both the closest numbers to 0, so 1 being larger is returned.
**Constraints:**
* `1 <= n <= 1000`
* `-105 <= nums[i] <= 105` | The constraints are not very large. Can we simulate the execution by starting from each index of s? Before any of the stopping conditions is met, stop the simulation for that index and set the answer for that index. |
🔥100% EASY TO UNDERSTAND/SIMPLE/CLEAN🔥 | find-closest-number-to-zero | 0 | 1 | ```\nclass Solution:\n def findClosestNumber(self, nums: List[int]) -> int:\n smallest = 0\n for i in nums:\n if abs(i) == 0:\n return 0\n elif smallest == 0 or abs(i) < abs(smallest):\n smallest = i\n elif abs(i) == abs(smallest):\n if i > smallest:\n smallest = i\n return smallest | 0 | Given an integer array `nums` of size `n`, return _the number with the value **closest** to_ `0` _in_ `nums`. If there are multiple answers, return _the number with the **largest** value_.
**Example 1:**
**Input:** nums = \[-4,-2,1,4,8\]
**Output:** 1
**Explanation:**
The distance from -4 to 0 is |-4| = 4.
The distance from -2 to 0 is |-2| = 2.
The distance from 1 to 0 is |1| = 1.
The distance from 4 to 0 is |4| = 4.
The distance from 8 to 0 is |8| = 8.
Thus, the closest number to 0 in the array is 1.
**Example 2:**
**Input:** nums = \[2,-1,1\]
**Output:** 1
**Explanation:** 1 and -1 are both the closest numbers to 0, so 1 being larger is returned.
**Constraints:**
* `1 <= n <= 1000`
* `-105 <= nums[i] <= 105` | The constraints are not very large. Can we simulate the execution by starting from each index of s? Before any of the stopping conditions is met, stop the simulation for that index and set the answer for that index. |
Python solution using lambda function || easy to understand for begineers | number-of-ways-to-buy-pens-and-pencils | 0 | 1 | ```\nfrom math import floor\nclass Solution:\n def waysToBuyPensPencils(self, total: int, cost1: int, cost2: int) -> int:\n ans = 0\n y = lambda x: (total-x*cost1)/cost2\n for i in range(total+1):\n c = floor(y(i))\n if c>=0:\n ans += c+1\n return ans\n``` | 2 | You are given an integer `total` indicating the amount of money you have. You are also given two integers `cost1` and `cost2` indicating the price of a pen and pencil respectively. You can spend **part or all** of your money to buy multiple quantities (or none) of each kind of writing utensil.
Return _the **number of distinct ways** you can buy some number of pens and pencils._
**Example 1:**
**Input:** total = 20, cost1 = 10, cost2 = 5
**Output:** 9
**Explanation:** The price of a pen is 10 and the price of a pencil is 5.
- If you buy 0 pens, you can buy 0, 1, 2, 3, or 4 pencils.
- If you buy 1 pen, you can buy 0, 1, or 2 pencils.
- If you buy 2 pens, you cannot buy any pencils.
The total number of ways to buy pens and pencils is 5 + 3 + 1 = 9.
**Example 2:**
**Input:** total = 5, cost1 = 10, cost2 = 10
**Output:** 1
**Explanation:** The price of both pens and pencils are 10, which cost more than total, so you cannot buy any writing utensils. Therefore, there is only 1 way: buy 0 pens and 0 pencils.
**Constraints:**
* `1 <= total, cost1, cost2 <= 106` | For each unique value found in the array, store a sorted list of indices of elements that have this value in the array. One way of doing this is to use a HashMap that maps the values to their list of indices. Update this mapping as you iterate through the array. Process each list of indices separately and get the sum of intervals for the elements of that value by utilizing prefix sums. For each element, keep track of the sum of indices of the identical elements that have come before and that will come after respectively. Use this to calculate the sum of intervals for that element to the rest of the elements with identical values. |
Python easy solution faster than 90% | number-of-ways-to-buy-pens-and-pencils | 0 | 1 | ```\nclass Solution:\n def waysToBuyPensPencils(self, total: int, cost1: int, cost2: int) -> int:\n if total < cost1 and total < cost2:\n return 1\n ways = 0\n if cost1 > cost2:\n for i in range(0, (total // cost1)+1):\n rem = total - (i * cost1)\n ways += (rem // cost2) + 1\n return ways\n for i in range(0, (total // cost2)+1):\n rem = total - (i * cost2)\n ways += (rem // cost1) + 1\n return ways | 2 | You are given an integer `total` indicating the amount of money you have. You are also given two integers `cost1` and `cost2` indicating the price of a pen and pencil respectively. You can spend **part or all** of your money to buy multiple quantities (or none) of each kind of writing utensil.
Return _the **number of distinct ways** you can buy some number of pens and pencils._
**Example 1:**
**Input:** total = 20, cost1 = 10, cost2 = 5
**Output:** 9
**Explanation:** The price of a pen is 10 and the price of a pencil is 5.
- If you buy 0 pens, you can buy 0, 1, 2, 3, or 4 pencils.
- If you buy 1 pen, you can buy 0, 1, or 2 pencils.
- If you buy 2 pens, you cannot buy any pencils.
The total number of ways to buy pens and pencils is 5 + 3 + 1 = 9.
**Example 2:**
**Input:** total = 5, cost1 = 10, cost2 = 10
**Output:** 1
**Explanation:** The price of both pens and pencils are 10, which cost more than total, so you cannot buy any writing utensils. Therefore, there is only 1 way: buy 0 pens and 0 pencils.
**Constraints:**
* `1 <= total, cost1, cost2 <= 106` | For each unique value found in the array, store a sorted list of indices of elements that have this value in the array. One way of doing this is to use a HashMap that maps the values to their list of indices. Update this mapping as you iterate through the array. Process each list of indices separately and get the sum of intervals for the elements of that value by utilizing prefix sums. For each element, keep track of the sum of indices of the identical elements that have come before and that will come after respectively. Use this to calculate the sum of intervals for that element to the rest of the elements with identical values. |
Check then withdraw | design-an-atm-machine | 0 | 1 | We first try to `take` the amount using available bills. If we can get the exact amount, then we complete the withdraw operation.\n\n**Python 3**\n```python\nclass ATM:\n bank, val = [0] * 5, [20, 50, 100, 200, 500]\n \n def deposit(self, banknotesCount: List[int]) -> None:\n self.bank = [a + b for a, b in zip(self.bank, banknotesCount)]\n \n def withdraw(self, amount: int) -> List[int]:\n take = [0] * 5\n for i in range(4, -1, -1):\n take[i] = min(self.bank[i], amount // self.val[i])\n amount -= take[i] * self.val[i]\n if amount == 0:\n self.bank = [a - b for a, b in zip(self.bank, take)]\n return [-1] if amount else take\n```\n**C++**\n```cpp\nclass ATM {\npublic:\n long long bank[5] = {}, val[5] = {20, 50, 100, 200, 500};\n void deposit(vector<int> banknotesCount) {\n for (int i = 0; i < 5; ++i)\n bank[i] += banknotesCount[i];\n }\n vector<int> withdraw(int amount) {\n vector<int> take(5);\n for (int i = 4; i >= 0; --i) {\n take[i] = min(bank[i], amount / val[i]);\n amount -= take[i] * val[i];\n }\n if (amount)\n return { -1 };\n for (int i = 0; i < 5; ++i)\n bank[i] -= take[i]; \n return take;\n }\n};\n``` | 72 | There is an ATM machine that stores banknotes of `5` denominations: `20`, `50`, `100`, `200`, and `500` dollars. Initially the ATM is empty. The user can use the machine to deposit or withdraw any amount of money.
When withdrawing, the machine prioritizes using banknotes of **larger** values.
* For example, if you want to withdraw `$300` and there are `2` `$50` banknotes, `1` `$100` banknote, and `1` `$200` banknote, then the machine will use the `$100` and `$200` banknotes.
* However, if you try to withdraw `$600` and there are `3` `$200` banknotes and `1` `$500` banknote, then the withdraw request will be rejected because the machine will first try to use the `$500` banknote and then be unable to use banknotes to complete the remaining `$100`. Note that the machine is **not** allowed to use the `$200` banknotes instead of the `$500` banknote.
Implement the ATM class:
* `ATM()` Initializes the ATM object.
* `void deposit(int[] banknotesCount)` Deposits new banknotes in the order `$20`, `$50`, `$100`, `$200`, and `$500`.
* `int[] withdraw(int amount)` Returns an array of length `5` of the number of banknotes that will be handed to the user in the order `$20`, `$50`, `$100`, `$200`, and `$500`, and update the number of banknotes in the ATM after withdrawing. Returns `[-1]` if it is not possible (do **not** withdraw any banknotes in this case).
**Example 1:**
**Input**
\[ "ATM ", "deposit ", "withdraw ", "deposit ", "withdraw ", "withdraw "\]
\[\[\], \[\[0,0,1,2,1\]\], \[600\], \[\[0,1,0,1,1\]\], \[600\], \[550\]\]
**Output**
\[null, null, \[0,0,1,0,1\], null, \[-1\], \[0,1,0,0,1\]\]
**Explanation**
ATM atm = new ATM();
atm.deposit(\[0,0,1,2,1\]); // Deposits 1 $100 banknote, 2 $200 banknotes,
// and 1 $500 banknote.
atm.withdraw(600); // Returns \[0,0,1,0,1\]. The machine uses 1 $100 banknote
// and 1 $500 banknote. The banknotes left over in the
// machine are \[0,0,0,2,0\].
atm.deposit(\[0,1,0,1,1\]); // Deposits 1 $50, $200, and $500 banknote.
// The banknotes in the machine are now \[0,1,0,3,1\].
atm.withdraw(600); // Returns \[-1\]. The machine will try to use a $500 banknote
// and then be unable to complete the remaining $100,
// so the withdraw request will be rejected.
// Since the request is rejected, the number of banknotes
// in the machine is not modified.
atm.withdraw(550); // Returns \[0,1,0,0,1\]. The machine uses 1 $50 banknote
// and 1 $500 banknote.
**Constraints:**
* `banknotesCount.length == 5`
* `0 <= banknotesCount[i] <= 109`
* `1 <= amount <= 109`
* At most `5000` calls **in total** will be made to `withdraw` and `deposit`.
* At least **one** call will be made to each function `withdraw` and `deposit`. | If we fix the value of k, how can we check if an original array exists for the fixed k? The smallest value of nums is obtained by subtracting k from the smallest value of the original array. How can we use this to reduce the search space for finding a valid k? You can compute every possible k by using the smallest value of nums (as lower[i]) against every other value in nums (as the corresponding higher[i]). For every computed k, greedily pair up the values in nums. This can be done sorting nums, then using a map to store previous values and searching that map for a corresponding lower[i] for the current nums[j] (as higher[i]). |
Python3 Very Pythonic and clean Logical process flow | design-an-atm-machine | 0 | 1 | It\'s pretty straightforward, once you do it. It\'s the typical sort of thing, the first time you do it, it\'s 5x-10x longer to do then it\'s obvious.\n\n```\nclass ATM:\n def __init__(self):\n self.cash = [0] * 5\n self.values = [20, 50, 100, 200, 500]\n\n def deposit(self, banknotes_count: List[int]) -> None:\n for i, n in enumerate(banknotes_count):\n self.cash[i] += n\n\n def withdraw(self, amount: int) -> List[int]:\n res = []\n for val, n in zip(self.values[::-1], self.cash[::-1]):\n need = min(n, amount // val)\n res = [need] + res\n amount -= (need * val)\n if amount == 0:\n self.deposit([-x for x in res])\n return res\n else:\n return [-1]\n\n``` | 6 | There is an ATM machine that stores banknotes of `5` denominations: `20`, `50`, `100`, `200`, and `500` dollars. Initially the ATM is empty. The user can use the machine to deposit or withdraw any amount of money.
When withdrawing, the machine prioritizes using banknotes of **larger** values.
* For example, if you want to withdraw `$300` and there are `2` `$50` banknotes, `1` `$100` banknote, and `1` `$200` banknote, then the machine will use the `$100` and `$200` banknotes.
* However, if you try to withdraw `$600` and there are `3` `$200` banknotes and `1` `$500` banknote, then the withdraw request will be rejected because the machine will first try to use the `$500` banknote and then be unable to use banknotes to complete the remaining `$100`. Note that the machine is **not** allowed to use the `$200` banknotes instead of the `$500` banknote.
Implement the ATM class:
* `ATM()` Initializes the ATM object.
* `void deposit(int[] banknotesCount)` Deposits new banknotes in the order `$20`, `$50`, `$100`, `$200`, and `$500`.
* `int[] withdraw(int amount)` Returns an array of length `5` of the number of banknotes that will be handed to the user in the order `$20`, `$50`, `$100`, `$200`, and `$500`, and update the number of banknotes in the ATM after withdrawing. Returns `[-1]` if it is not possible (do **not** withdraw any banknotes in this case).
**Example 1:**
**Input**
\[ "ATM ", "deposit ", "withdraw ", "deposit ", "withdraw ", "withdraw "\]
\[\[\], \[\[0,0,1,2,1\]\], \[600\], \[\[0,1,0,1,1\]\], \[600\], \[550\]\]
**Output**
\[null, null, \[0,0,1,0,1\], null, \[-1\], \[0,1,0,0,1\]\]
**Explanation**
ATM atm = new ATM();
atm.deposit(\[0,0,1,2,1\]); // Deposits 1 $100 banknote, 2 $200 banknotes,
// and 1 $500 banknote.
atm.withdraw(600); // Returns \[0,0,1,0,1\]. The machine uses 1 $100 banknote
// and 1 $500 banknote. The banknotes left over in the
// machine are \[0,0,0,2,0\].
atm.deposit(\[0,1,0,1,1\]); // Deposits 1 $50, $200, and $500 banknote.
// The banknotes in the machine are now \[0,1,0,3,1\].
atm.withdraw(600); // Returns \[-1\]. The machine will try to use a $500 banknote
// and then be unable to complete the remaining $100,
// so the withdraw request will be rejected.
// Since the request is rejected, the number of banknotes
// in the machine is not modified.
atm.withdraw(550); // Returns \[0,1,0,0,1\]. The machine uses 1 $50 banknote
// and 1 $500 banknote.
**Constraints:**
* `banknotesCount.length == 5`
* `0 <= banknotesCount[i] <= 109`
* `1 <= amount <= 109`
* At most `5000` calls **in total** will be made to `withdraw` and `deposit`.
* At least **one** call will be made to each function `withdraw` and `deposit`. | If we fix the value of k, how can we check if an original array exists for the fixed k? The smallest value of nums is obtained by subtracting k from the smallest value of the original array. How can we use this to reduce the search space for finding a valid k? You can compute every possible k by using the smallest value of nums (as lower[i]) against every other value in nums (as the corresponding higher[i]). For every computed k, greedily pair up the values in nums. This can be done sorting nums, then using a map to store previous values and searching that map for a corresponding lower[i] for the current nums[j] (as higher[i]). |
Python Easy O(1) | design-an-atm-machine | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nAll of the iterations are Range of 5 so, the time complexity is O(1)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nsince there is no dynamic storage alocation the space complexity is O(1)\n# Code\n```\nclass ATM:\n\n def __init__(self):\n self.banknotes=[0,0,0,0,0]\n\n def deposit(self, banknotesCount: List[int]) -> None:\n for i in range(5):\n self.banknotes[i] += banknotesCount[i]\n\n def withdraw(self, amount: int) -> List[int]:\n out_order = [500,200,100,50,20]\n with_amount = [0,0,0,0,0]\n for i in range(5):\n a = self.banknotes[abs(i-4)]\n with_amount[i] = amount//out_order[i]\n if with_amount[i] > a:\n with_amount[i] = a\n amount -= a*out_order[i]\n else :\n amount = amount % out_order[i]\n if amount == 0:\n for i in range(5):\n if self.banknotes[abs(4-i)] - with_amount[i] < 0:\n return ([-1])\n for i in range(5):\n self.banknotes[abs(4-i)] -= with_amount[i]\n return (with_amount[::-1])\n else :\n return ([-1])\n \n\n\n# Your ATM object will be instantiated and called as such:\n# obj = ATM()\n# obj.deposit(banknotesCount)\n# param_2 = obj.withdraw(amount)\n``` | 0 | There is an ATM machine that stores banknotes of `5` denominations: `20`, `50`, `100`, `200`, and `500` dollars. Initially the ATM is empty. The user can use the machine to deposit or withdraw any amount of money.
When withdrawing, the machine prioritizes using banknotes of **larger** values.
* For example, if you want to withdraw `$300` and there are `2` `$50` banknotes, `1` `$100` banknote, and `1` `$200` banknote, then the machine will use the `$100` and `$200` banknotes.
* However, if you try to withdraw `$600` and there are `3` `$200` banknotes and `1` `$500` banknote, then the withdraw request will be rejected because the machine will first try to use the `$500` banknote and then be unable to use banknotes to complete the remaining `$100`. Note that the machine is **not** allowed to use the `$200` banknotes instead of the `$500` banknote.
Implement the ATM class:
* `ATM()` Initializes the ATM object.
* `void deposit(int[] banknotesCount)` Deposits new banknotes in the order `$20`, `$50`, `$100`, `$200`, and `$500`.
* `int[] withdraw(int amount)` Returns an array of length `5` of the number of banknotes that will be handed to the user in the order `$20`, `$50`, `$100`, `$200`, and `$500`, and update the number of banknotes in the ATM after withdrawing. Returns `[-1]` if it is not possible (do **not** withdraw any banknotes in this case).
**Example 1:**
**Input**
\[ "ATM ", "deposit ", "withdraw ", "deposit ", "withdraw ", "withdraw "\]
\[\[\], \[\[0,0,1,2,1\]\], \[600\], \[\[0,1,0,1,1\]\], \[600\], \[550\]\]
**Output**
\[null, null, \[0,0,1,0,1\], null, \[-1\], \[0,1,0,0,1\]\]
**Explanation**
ATM atm = new ATM();
atm.deposit(\[0,0,1,2,1\]); // Deposits 1 $100 banknote, 2 $200 banknotes,
// and 1 $500 banknote.
atm.withdraw(600); // Returns \[0,0,1,0,1\]. The machine uses 1 $100 banknote
// and 1 $500 banknote. The banknotes left over in the
// machine are \[0,0,0,2,0\].
atm.deposit(\[0,1,0,1,1\]); // Deposits 1 $50, $200, and $500 banknote.
// The banknotes in the machine are now \[0,1,0,3,1\].
atm.withdraw(600); // Returns \[-1\]. The machine will try to use a $500 banknote
// and then be unable to complete the remaining $100,
// so the withdraw request will be rejected.
// Since the request is rejected, the number of banknotes
// in the machine is not modified.
atm.withdraw(550); // Returns \[0,1,0,0,1\]. The machine uses 1 $50 banknote
// and 1 $500 banknote.
**Constraints:**
* `banknotesCount.length == 5`
* `0 <= banknotesCount[i] <= 109`
* `1 <= amount <= 109`
* At most `5000` calls **in total** will be made to `withdraw` and `deposit`.
* At least **one** call will be made to each function `withdraw` and `deposit`. | If we fix the value of k, how can we check if an original array exists for the fixed k? The smallest value of nums is obtained by subtracting k from the smallest value of the original array. How can we use this to reduce the search space for finding a valid k? You can compute every possible k by using the smallest value of nums (as lower[i]) against every other value in nums (as the corresponding higher[i]). For every computed k, greedily pair up the values in nums. This can be done sorting nums, then using a map to store previous values and searching that map for a corresponding lower[i] for the current nums[j] (as higher[i]). |
Python list traversal | design-an-atm-machine | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nTwo lists in __init__:\n1. self.bank = [0] * 5\n2. self.notes = [20, 50, 100, 200, 500].\n\n# Code\n```\nclass ATM:\n\n def __init__(self):\n self.bank = [0, 0, 0, 0, 0]\n self.notes = [20, 50, 100, 200, 500]\n\n def deposit(self, banknotesCount: List[int]) -> None:\n self.bank = [a + b for a, b in zip(self.bank, banknotesCount)]\n\n def withdraw(self, amount: int) -> List[int]:\n out = [0] * 5\n for i in range(4, -1, -1):\n out[i] = min(self.bank[i], amount // self.notes[i])\n amount -= out[i] * self.notes[i]\n if amount == 0:\n break\n \n if amount == 0:\n self.bank = [x - y for x, y in zip(self.bank, out)]\n return out\n else:\n return [-1]\n\n\n# Your ATM object will be instantiated and called as such:\n# obj = ATM()\n# obj.deposit(banknotesCount)\n# param_2 = obj.withdraw(amount)\n``` | 0 | There is an ATM machine that stores banknotes of `5` denominations: `20`, `50`, `100`, `200`, and `500` dollars. Initially the ATM is empty. The user can use the machine to deposit or withdraw any amount of money.
When withdrawing, the machine prioritizes using banknotes of **larger** values.
* For example, if you want to withdraw `$300` and there are `2` `$50` banknotes, `1` `$100` banknote, and `1` `$200` banknote, then the machine will use the `$100` and `$200` banknotes.
* However, if you try to withdraw `$600` and there are `3` `$200` banknotes and `1` `$500` banknote, then the withdraw request will be rejected because the machine will first try to use the `$500` banknote and then be unable to use banknotes to complete the remaining `$100`. Note that the machine is **not** allowed to use the `$200` banknotes instead of the `$500` banknote.
Implement the ATM class:
* `ATM()` Initializes the ATM object.
* `void deposit(int[] banknotesCount)` Deposits new banknotes in the order `$20`, `$50`, `$100`, `$200`, and `$500`.
* `int[] withdraw(int amount)` Returns an array of length `5` of the number of banknotes that will be handed to the user in the order `$20`, `$50`, `$100`, `$200`, and `$500`, and update the number of banknotes in the ATM after withdrawing. Returns `[-1]` if it is not possible (do **not** withdraw any banknotes in this case).
**Example 1:**
**Input**
\[ "ATM ", "deposit ", "withdraw ", "deposit ", "withdraw ", "withdraw "\]
\[\[\], \[\[0,0,1,2,1\]\], \[600\], \[\[0,1,0,1,1\]\], \[600\], \[550\]\]
**Output**
\[null, null, \[0,0,1,0,1\], null, \[-1\], \[0,1,0,0,1\]\]
**Explanation**
ATM atm = new ATM();
atm.deposit(\[0,0,1,2,1\]); // Deposits 1 $100 banknote, 2 $200 banknotes,
// and 1 $500 banknote.
atm.withdraw(600); // Returns \[0,0,1,0,1\]. The machine uses 1 $100 banknote
// and 1 $500 banknote. The banknotes left over in the
// machine are \[0,0,0,2,0\].
atm.deposit(\[0,1,0,1,1\]); // Deposits 1 $50, $200, and $500 banknote.
// The banknotes in the machine are now \[0,1,0,3,1\].
atm.withdraw(600); // Returns \[-1\]. The machine will try to use a $500 banknote
// and then be unable to complete the remaining $100,
// so the withdraw request will be rejected.
// Since the request is rejected, the number of banknotes
// in the machine is not modified.
atm.withdraw(550); // Returns \[0,1,0,0,1\]. The machine uses 1 $50 banknote
// and 1 $500 banknote.
**Constraints:**
* `banknotesCount.length == 5`
* `0 <= banknotesCount[i] <= 109`
* `1 <= amount <= 109`
* At most `5000` calls **in total** will be made to `withdraw` and `deposit`.
* At least **one** call will be made to each function `withdraw` and `deposit`. | If we fix the value of k, how can we check if an original array exists for the fixed k? The smallest value of nums is obtained by subtracting k from the smallest value of the original array. How can we use this to reduce the search space for finding a valid k? You can compute every possible k by using the smallest value of nums (as lower[i]) against every other value in nums (as the corresponding higher[i]). For every computed k, greedily pair up the values in nums. This can be done sorting nums, then using a map to store previous values and searching that map for a corresponding lower[i] for the current nums[j] (as higher[i]). |
Python really easy to understand | design-an-atm-machine | 0 | 1 | # Approach\nClean solution\n\n# Code\n```\nclass ATM:\n def __init__(self):\n self.money = [0]*5\n self.order = [20, 50, 100, 200, 500]\n\n def deposit(self, banknotesCount: List[int]) -> None:\n for i, amount in enumerate(banknotesCount):\n self.money[i] += amount\n\n def withdraw(self, amount: int) -> List[int]:\n output = [0]*5\n // We can start from i = 4 as len(answer) == 5 always and we need to go from the end to the start\n i = 4\n while amount and i >= 0:\n // We check for each value whether or not we will add all banknotes with this value we have or just some of them. \n output[i] = min(self.money[i], amount//self.order[i])\n // Reduce rest amount with already used money\n amount -= output[i]*self.order[i]\n i -= 1\n \n if amount:\n // If we still have some uncovered amount after iteration, we should return [-1]\n return [-1]\n else:\n // Else we should update amount of banknotes and return banknotes we gave \n for i, mon in enumerate(self.money):\n self.money[i] = mon - output[i]\n return output\n\n\n\n# Your ATM object will be instantiated and called as such:\n# obj = ATM()\n# obj.deposit(banknotesCount)\n# param_2 = obj.withdraw(amount)\n``` | 0 | There is an ATM machine that stores banknotes of `5` denominations: `20`, `50`, `100`, `200`, and `500` dollars. Initially the ATM is empty. The user can use the machine to deposit or withdraw any amount of money.
When withdrawing, the machine prioritizes using banknotes of **larger** values.
* For example, if you want to withdraw `$300` and there are `2` `$50` banknotes, `1` `$100` banknote, and `1` `$200` banknote, then the machine will use the `$100` and `$200` banknotes.
* However, if you try to withdraw `$600` and there are `3` `$200` banknotes and `1` `$500` banknote, then the withdraw request will be rejected because the machine will first try to use the `$500` banknote and then be unable to use banknotes to complete the remaining `$100`. Note that the machine is **not** allowed to use the `$200` banknotes instead of the `$500` banknote.
Implement the ATM class:
* `ATM()` Initializes the ATM object.
* `void deposit(int[] banknotesCount)` Deposits new banknotes in the order `$20`, `$50`, `$100`, `$200`, and `$500`.
* `int[] withdraw(int amount)` Returns an array of length `5` of the number of banknotes that will be handed to the user in the order `$20`, `$50`, `$100`, `$200`, and `$500`, and update the number of banknotes in the ATM after withdrawing. Returns `[-1]` if it is not possible (do **not** withdraw any banknotes in this case).
**Example 1:**
**Input**
\[ "ATM ", "deposit ", "withdraw ", "deposit ", "withdraw ", "withdraw "\]
\[\[\], \[\[0,0,1,2,1\]\], \[600\], \[\[0,1,0,1,1\]\], \[600\], \[550\]\]
**Output**
\[null, null, \[0,0,1,0,1\], null, \[-1\], \[0,1,0,0,1\]\]
**Explanation**
ATM atm = new ATM();
atm.deposit(\[0,0,1,2,1\]); // Deposits 1 $100 banknote, 2 $200 banknotes,
// and 1 $500 banknote.
atm.withdraw(600); // Returns \[0,0,1,0,1\]. The machine uses 1 $100 banknote
// and 1 $500 banknote. The banknotes left over in the
// machine are \[0,0,0,2,0\].
atm.deposit(\[0,1,0,1,1\]); // Deposits 1 $50, $200, and $500 banknote.
// The banknotes in the machine are now \[0,1,0,3,1\].
atm.withdraw(600); // Returns \[-1\]. The machine will try to use a $500 banknote
// and then be unable to complete the remaining $100,
// so the withdraw request will be rejected.
// Since the request is rejected, the number of banknotes
// in the machine is not modified.
atm.withdraw(550); // Returns \[0,1,0,0,1\]. The machine uses 1 $50 banknote
// and 1 $500 banknote.
**Constraints:**
* `banknotesCount.length == 5`
* `0 <= banknotesCount[i] <= 109`
* `1 <= amount <= 109`
* At most `5000` calls **in total** will be made to `withdraw` and `deposit`.
* At least **one** call will be made to each function `withdraw` and `deposit`. | If we fix the value of k, how can we check if an original array exists for the fixed k? The smallest value of nums is obtained by subtracting k from the smallest value of the original array. How can we use this to reduce the search space for finding a valid k? You can compute every possible k by using the smallest value of nums (as lower[i]) against every other value in nums (as the corresponding higher[i]). For every computed k, greedily pair up the values in nums. This can be done sorting nums, then using a map to store previous values and searching that map for a corresponding lower[i] for the current nums[j] (as higher[i]). |
[Java/Python] Keep 3 Biggest Neighbours | maximum-score-of-a-node-sequence | 1 | 1 | # **Intuition**\nWe don\'t need to check all possible sequences,\nbut only some big nodes.\n<br>\n\n# **Explanation**\nFor each edge `(i, j)` in `edges`,\nwe find a neighbour `ii` of node `i`,\nwe find a neighbour `jj` of node `i`,\nIf `ii, i, j,jj` has no duplicate, then that\'s a valid sequence.\n\nAd the intuition mentioned,\nwe don\'t have to enumearte all neignbours,\nbut only some nodes with big value.\n\nBut how many is enough?\nI\'ll say 3.\nFor example, we have `ii, i, j` now,\nwe can enumerate 3 of node `j` biggest neighbour,\nthere must be at least one node different node `ii` and node `i`.\n\nSo we need to iterate all edges `(i, j)`,\nfor each node we keep at most 3 biggest neighbour, which this can be done in `O(3)` or `O(log3)`.\n<br>\n\n# **Complexity**\nTime `O(n + m)`\nSpace `O(n + m)`\n<br>\n\n**Java**\n```java\n public int maximumScore(int[] A, int[][] edges) {\n int n = A.length;\n PriorityQueue<Integer>[] q = new PriorityQueue[n];\n for (int i = 0; i < n; i++)\n q[i] = new PriorityQueue<>((a, b) -> A[a] - A[b]);\n for (int[] e : edges) {\n q[e[0]].offer(e[1]);\n q[e[1]].offer(e[0]);\n if (q[e[0]].size() > 3) q[e[0]].poll();\n if (q[e[1]].size() > 3) q[e[1]].poll();\n }\n int res = -1;\n for (int[] edge : edges)\n for (int i : q[edge[0]])\n for (int j : q[edge[1]])\n if (i != j && i != edge[1] && j != edge[0])\n res = Math.max(res, A[i] + A[j] + A[edge[0]] + A[edge[1]]); \n return res;\n }\n```\n**Python**\n```py\n def maximumScore(self, A, edges):\n n = len(A)\n G = [[] for i in range(n)]\n for i,j in edges:\n G[i].append([A[j], j])\n G[j].append([A[i], i])\n for i in range(n):\n G[i] = nlargest(3, G[i])\n \n res = -1\n for i,j in edges:\n for vii, ii in G[i]:\n for vjj, jj in G[j]:\n if ii != jj and ii != j and j != ii:\n res = max(res, vii + vjj + A[i] + A[j])\n return res\n```\n | 126 | There is an **undirected** graph with `n` nodes, numbered from `0` to `n - 1`.
You are given a **0-indexed** integer array `scores` of length `n` where `scores[i]` denotes the score of node `i`. You are also given a 2D integer array `edges` where `edges[i] = [ai, bi]` denotes that there exists an **undirected** edge connecting nodes `ai` and `bi`.
A node sequence is **valid** if it meets the following conditions:
* There is an edge connecting every pair of **adjacent** nodes in the sequence.
* No node appears more than once in the sequence.
The score of a node sequence is defined as the **sum** of the scores of the nodes in the sequence.
Return _the **maximum score** of a valid node sequence with a length of_ `4`_._ If no such sequence exists, return `-1`.
**Example 1:**
**Input:** scores = \[5,2,9,8,4\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[0,2\],\[1,3\],\[2,4\]\]
**Output:** 24
**Explanation:** The figure above shows the graph and the chosen node sequence \[0,1,2,3\].
The score of the node sequence is 5 + 2 + 9 + 8 = 24.
It can be shown that no other node sequence has a score of more than 24.
Note that the sequences \[3,1,2,0\] and \[1,0,2,3\] are also valid and have a score of 24.
The sequence \[0,3,2,4\] is not valid since no edge connects nodes 0 and 3.
**Example 2:**
**Input:** scores = \[9,20,6,4,11,12\], edges = \[\[0,3\],\[5,3\],\[2,4\],\[1,3\]\]
**Output:** -1
**Explanation:** The figure above shows the graph.
There are no valid node sequences of length 4, so we return -1.
**Constraints:**
* `n == scores.length`
* `4 <= n <= 5 * 104`
* `1 <= scores[i] <= 108`
* `0 <= edges.length <= 5 * 104`
* `edges[i].length == 2`
* `0 <= ai, bi <= n - 1`
* `ai != bi`
* There are no duplicate edges. | Is the sum of two even numbers even or odd? How about two odd numbers? One odd number and one even number? If there is an even number of odd numbers, the sum will be even and vice versa. Create an integer array to store all the even numbers in nums and another array to store all the odd numbers in nums. Sort both arrays. |
[Python3] Top 3 neighbors in adjacency list using heap | maximum-score-of-a-node-sequence | 0 | 1 | The solution here converts the adjacency list into a heap. We just need the top 3 neighbors for any node to solve this problem. Since I am using heap, I can extract the top 3 neighbors in O(3*log(n)). \n\n**Main point**: For every edge, say a---b, we can search for the best neighbor of a (that is not b) and the best neighbor of b (that is not a). We also need to make sure the neighbors are not equal (i.e. we are not forming a triangle). Then, we have a valid sequence of length 4. Here a---b is the middle of the sequence.\n\nWe can iterate over all edges and perform the checks described above and find the best valid sequence. We are destined to find the best valid sequence in this algorithm.\n\nTime Complexity: O(e*log(n)), e is no. of edges, n is no. of nodes.\n\nPS: I\'ve come realize we don\'t need to use heaps. We can just save the top 3 neighbors while creating the adjacency list. We will only need the top 3 in our algorithm, so there is no point in saving the entire adjacency list for every node and heapifying it. This makes the time complexity O(e).\n\n```\nimport heapq\n\nclass Pair:\n def __init__(self, node, score):\n self.node = node\n self.score = score\n def __lt__(self, other):\n return self.score < other.score\n\nclass Solution: \n def maximumScore(self, scores: List[int], edges: List[List[int]]) -> int:\n edgeList = [[] for i in range(len(scores))]\n for edge in edges:\n edgeList[edge[0]].append(Pair(edge[1], -1*scores[edge[1]]))\n edgeList[edge[1]].append(Pair(edge[0], -1*scores[edge[0]]))\n \n for nodeEdges in edgeList:\n heapq.heapify(nodeEdges)\n \n best = -1\n for e in edges:\n n0 = []\n n1 = []\n while(len(edgeList[e[0]])>0 and len(n0)<3):\n x = heapq.heappop(edgeList[e[0]])\n n0.append(x)\n while(len(edgeList[e[1]])>0 and len(n1)<3):\n x = heapq.heappop(edgeList[e[1]])\n n1.append(x)\n \n m0 = [x for x in n0 if x.node!=e[1]]\n m1 = [x for x in n1 if x.node!=e[0]]\n score = scores[e[0]] + scores[e[1]]\n \n if(len(m0)!=0 and len(m1)!=0):\n if(m0[0].node==m1[0].node):\n if(len(m0)>1 and len(m1)==1):\n score += scores[m0[1].node] + scores[m1[0].node]\n best = max(best, score)\n elif(len(m0)==1 and len(m1)>1):\n score += scores[m0[0].node] + scores[m1[1].node]\n best = max(best, score)\n elif(len(m0)>1 and len(m1)>1):\n score += scores[m0[0].node] + max(scores[m0[1].node], scores[m1[1].node])\n best = max(best, score)\n else:\n score += scores[m0[0].node] + scores[m1[0].node]\n best = max(best, score)\n \n for n in n0:\n heapq.heappush(edgeList[e[0]], n)\n for n in n1:\n heapq.heappush(edgeList[e[1]], n)\n\n\n return best\n``` | 4 | There is an **undirected** graph with `n` nodes, numbered from `0` to `n - 1`.
You are given a **0-indexed** integer array `scores` of length `n` where `scores[i]` denotes the score of node `i`. You are also given a 2D integer array `edges` where `edges[i] = [ai, bi]` denotes that there exists an **undirected** edge connecting nodes `ai` and `bi`.
A node sequence is **valid** if it meets the following conditions:
* There is an edge connecting every pair of **adjacent** nodes in the sequence.
* No node appears more than once in the sequence.
The score of a node sequence is defined as the **sum** of the scores of the nodes in the sequence.
Return _the **maximum score** of a valid node sequence with a length of_ `4`_._ If no such sequence exists, return `-1`.
**Example 1:**
**Input:** scores = \[5,2,9,8,4\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[0,2\],\[1,3\],\[2,4\]\]
**Output:** 24
**Explanation:** The figure above shows the graph and the chosen node sequence \[0,1,2,3\].
The score of the node sequence is 5 + 2 + 9 + 8 = 24.
It can be shown that no other node sequence has a score of more than 24.
Note that the sequences \[3,1,2,0\] and \[1,0,2,3\] are also valid and have a score of 24.
The sequence \[0,3,2,4\] is not valid since no edge connects nodes 0 and 3.
**Example 2:**
**Input:** scores = \[9,20,6,4,11,12\], edges = \[\[0,3\],\[5,3\],\[2,4\],\[1,3\]\]
**Output:** -1
**Explanation:** The figure above shows the graph.
There are no valid node sequences of length 4, so we return -1.
**Constraints:**
* `n == scores.length`
* `4 <= n <= 5 * 104`
* `1 <= scores[i] <= 108`
* `0 <= edges.length <= 5 * 104`
* `edges[i].length == 2`
* `0 <= ai, bi <= n - 1`
* `ai != bi`
* There are no duplicate edges. | Is the sum of two even numbers even or odd? How about two odd numbers? One odd number and one even number? If there is an even number of odd numbers, the sum will be even and vice versa. Create an integer array to store all the even numbers in nums and another array to store all the odd numbers in nums. Sort both arrays. |
[Python 3] Hint solution | maximum-score-of-a-node-sequence | 0 | 1 | ```\n# key is to store top 3 neighbors for each node\n# for each pair of edges (two middle nodes): find the other two nodes with highest score\n# we can\'t store top 1 node (highest node is neighbor in the edge) or 2 nodes (two middle nodes share the same highest adjacent node)\n\n\nclass Solution:\n def maximumScore(self, scores: List[int], edges: List[List[int]]) -> int:\n \n g = defaultdict(list)\n for a, b in edges:\n heappush(g[a], (scores[b], b))\n if len(g[a]) > 3:\n heappop(g[a])\n heappush(g[b], (scores[a], a))\n if len(g[b]) > 3:\n heappop(g[b])\n \n ans = -1\n for a, b in edges:\n for s1, n1 in g[a]:\n if n1 == b: continue\n for s2, n2 in g[b]:\n if n2 == n1 or n2 == a: continue\n ans = max(ans, scores[a] + scores[b] + s1 + s2)\n return ans | 3 | There is an **undirected** graph with `n` nodes, numbered from `0` to `n - 1`.
You are given a **0-indexed** integer array `scores` of length `n` where `scores[i]` denotes the score of node `i`. You are also given a 2D integer array `edges` where `edges[i] = [ai, bi]` denotes that there exists an **undirected** edge connecting nodes `ai` and `bi`.
A node sequence is **valid** if it meets the following conditions:
* There is an edge connecting every pair of **adjacent** nodes in the sequence.
* No node appears more than once in the sequence.
The score of a node sequence is defined as the **sum** of the scores of the nodes in the sequence.
Return _the **maximum score** of a valid node sequence with a length of_ `4`_._ If no such sequence exists, return `-1`.
**Example 1:**
**Input:** scores = \[5,2,9,8,4\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[0,2\],\[1,3\],\[2,4\]\]
**Output:** 24
**Explanation:** The figure above shows the graph and the chosen node sequence \[0,1,2,3\].
The score of the node sequence is 5 + 2 + 9 + 8 = 24.
It can be shown that no other node sequence has a score of more than 24.
Note that the sequences \[3,1,2,0\] and \[1,0,2,3\] are also valid and have a score of 24.
The sequence \[0,3,2,4\] is not valid since no edge connects nodes 0 and 3.
**Example 2:**
**Input:** scores = \[9,20,6,4,11,12\], edges = \[\[0,3\],\[5,3\],\[2,4\],\[1,3\]\]
**Output:** -1
**Explanation:** The figure above shows the graph.
There are no valid node sequences of length 4, so we return -1.
**Constraints:**
* `n == scores.length`
* `4 <= n <= 5 * 104`
* `1 <= scores[i] <= 108`
* `0 <= edges.length <= 5 * 104`
* `edges[i].length == 2`
* `0 <= ai, bi <= n - 1`
* `ai != bi`
* There are no duplicate edges. | Is the sum of two even numbers even or odd? How about two odd numbers? One odd number and one even number? If there is an even number of odd numbers, the sum will be even and vice versa. Create an integer array to store all the even numbers in nums and another array to store all the odd numbers in nums. Sort both arrays. |
Python3 O(|E|) solution | maximum-score-of-a-node-sequence | 0 | 1 | ```\nclass Solution:\n def maximumScore(self, scores: List[int], edges: List[List[int]]) -> int:\n \n connection = {}\n \n for source, target in edges:\n if source not in connection: connection[source] = [target]\n else: connection[source].append(target)\n \n if target not in connection: connection[target] = [source]\n else: connection[target].append(source)\n \n res = -1\n \n max_dict = {}\n for key, value in connection.items():\n max1, max2, max3 = -sys.maxsize, -sys.maxsize, -sys.maxsize\n n1, n2, n3 = None, None, None\n for element in value:\n if scores[element] > max1:\n max1, max2, max3 = scores[element], max1, max2\n n1, n2, n3 = element, n1, n2\n elif scores[element] > max2:\n max2, max3 = scores[element], max2\n n2, n3 = element, n2\n elif scores[element] > max3:\n max3 = scores[element]\n n3 = element\n max_dict[key] = []\n if n1 != None: max_dict[key].append(n1)\n if n2 != None: max_dict[key].append(n2)\n if n3 != None: max_dict[key].append(n3)\n \n for source, target in edges:\n base = scores[source] + scores[target]\n \n n_s = max_dict[source]\n n_t = max_dict[target]\n if len(n_s) == 1 or len(n_t) == 1:\n pass\n else:\n new_n_s = [x for x in n_s if x != target]\n new_n_t = [x for x in n_t if x != source]\n if new_n_s[0] != new_n_t[0]:\n res = max(res, base + scores[new_n_s[0]] + scores[new_n_t[0]])\n else:\n if len(new_n_s) > 1:\n res = max(res, base + scores[new_n_s[1]] + scores[new_n_t[0]])\n if len(new_n_t) > 1:\n res = max(res, base + scores[new_n_s[0]] + scores[new_n_t[1]]) \n \n return res\n \n``` | 2 | There is an **undirected** graph with `n` nodes, numbered from `0` to `n - 1`.
You are given a **0-indexed** integer array `scores` of length `n` where `scores[i]` denotes the score of node `i`. You are also given a 2D integer array `edges` where `edges[i] = [ai, bi]` denotes that there exists an **undirected** edge connecting nodes `ai` and `bi`.
A node sequence is **valid** if it meets the following conditions:
* There is an edge connecting every pair of **adjacent** nodes in the sequence.
* No node appears more than once in the sequence.
The score of a node sequence is defined as the **sum** of the scores of the nodes in the sequence.
Return _the **maximum score** of a valid node sequence with a length of_ `4`_._ If no such sequence exists, return `-1`.
**Example 1:**
**Input:** scores = \[5,2,9,8,4\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[0,2\],\[1,3\],\[2,4\]\]
**Output:** 24
**Explanation:** The figure above shows the graph and the chosen node sequence \[0,1,2,3\].
The score of the node sequence is 5 + 2 + 9 + 8 = 24.
It can be shown that no other node sequence has a score of more than 24.
Note that the sequences \[3,1,2,0\] and \[1,0,2,3\] are also valid and have a score of 24.
The sequence \[0,3,2,4\] is not valid since no edge connects nodes 0 and 3.
**Example 2:**
**Input:** scores = \[9,20,6,4,11,12\], edges = \[\[0,3\],\[5,3\],\[2,4\],\[1,3\]\]
**Output:** -1
**Explanation:** The figure above shows the graph.
There are no valid node sequences of length 4, so we return -1.
**Constraints:**
* `n == scores.length`
* `4 <= n <= 5 * 104`
* `1 <= scores[i] <= 108`
* `0 <= edges.length <= 5 * 104`
* `edges[i].length == 2`
* `0 <= ai, bi <= n - 1`
* `ai != bi`
* There are no duplicate edges. | Is the sum of two even numbers even or odd? How about two odd numbers? One odd number and one even number? If there is an even number of odd numbers, the sum will be even and vice versa. Create an integer array to store all the even numbers in nums and another array to store all the odd numbers in nums. Sort both arrays. |
Need Help [ Python3 ] | maximum-score-of-a-node-sequence | 0 | 1 | # Can anyone explain me, why?\n**scores = [16,21,22,2,24,21,12,17,2,24]\nedges = [[0,1],[1,2],[2,3],[3,4],[4,5],[5,6],[6,7],[7,8],[8,9],[9,0]]**\n\nwhy this is giving ***83*** , it should be ***61***\n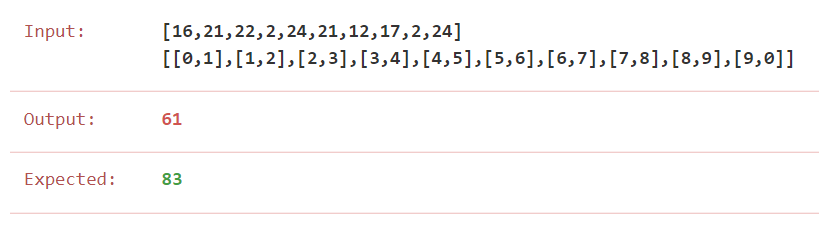\n\n\nand \n**scores = [18,6,4,9,8,2]**\n**edges = [[0,1],[0,2],[0,3],[0,4],[0,5],[1,2],[1,3],[1,4],[1,5],[2,3],[2,4],[2,5],[3,4],[3,5],[4,5]]**\n\nis giving ***41***? it should be ***47***\n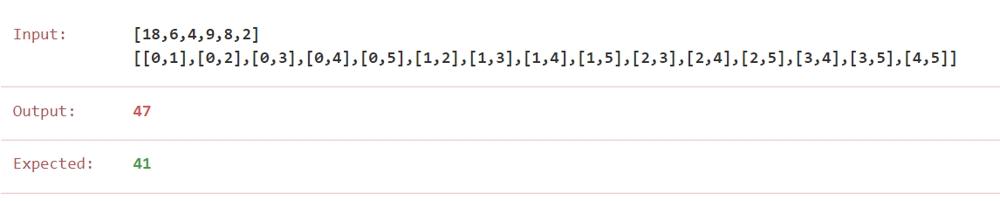\n\n | 0 | There is an **undirected** graph with `n` nodes, numbered from `0` to `n - 1`.
You are given a **0-indexed** integer array `scores` of length `n` where `scores[i]` denotes the score of node `i`. You are also given a 2D integer array `edges` where `edges[i] = [ai, bi]` denotes that there exists an **undirected** edge connecting nodes `ai` and `bi`.
A node sequence is **valid** if it meets the following conditions:
* There is an edge connecting every pair of **adjacent** nodes in the sequence.
* No node appears more than once in the sequence.
The score of a node sequence is defined as the **sum** of the scores of the nodes in the sequence.
Return _the **maximum score** of a valid node sequence with a length of_ `4`_._ If no such sequence exists, return `-1`.
**Example 1:**
**Input:** scores = \[5,2,9,8,4\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[0,2\],\[1,3\],\[2,4\]\]
**Output:** 24
**Explanation:** The figure above shows the graph and the chosen node sequence \[0,1,2,3\].
The score of the node sequence is 5 + 2 + 9 + 8 = 24.
It can be shown that no other node sequence has a score of more than 24.
Note that the sequences \[3,1,2,0\] and \[1,0,2,3\] are also valid and have a score of 24.
The sequence \[0,3,2,4\] is not valid since no edge connects nodes 0 and 3.
**Example 2:**
**Input:** scores = \[9,20,6,4,11,12\], edges = \[\[0,3\],\[5,3\],\[2,4\],\[1,3\]\]
**Output:** -1
**Explanation:** The figure above shows the graph.
There are no valid node sequences of length 4, so we return -1.
**Constraints:**
* `n == scores.length`
* `4 <= n <= 5 * 104`
* `1 <= scores[i] <= 108`
* `0 <= edges.length <= 5 * 104`
* `edges[i].length == 2`
* `0 <= ai, bi <= n - 1`
* `ai != bi`
* There are no duplicate edges. | Is the sum of two even numbers even or odd? How about two odd numbers? One odd number and one even number? If there is an even number of odd numbers, the sum will be even and vice versa. Create an integer array to store all the even numbers in nums and another array to store all the odd numbers in nums. Sort both arrays. |
List of Heaps | Commented and Explained | maximum-score-of-a-node-sequence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe want the maximum score of a node sequence \nWe know that the nodes are of length 4 \nThis means we have 3 edges \nWe also know that we need them to be such that the middle edge is fixed and other two can swing \nThis tells us we really only need the best three options any node has for neighbors since \n- One neighbor is a pair match up \n- The other two are our best options we would pick \n- If we had more than 3 options, we still would stick with our best two after an edge join \n\nFrom this we can see that each node only keeps it\'s best 3 neighbors and all others can go swing away for all we care. Based on this we can do a push pop approach with a min heap that will keep our maximals nice and cozy. \n\nAfter that it is just process of elimination to find the best combination. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nSet up your graph as a list of list for each node \nFor each edge \n- get the heap nodes as the score of an edge end and the edge end \n- if the length of the graph at an edge end is currently 3 \n - do a heap push pop of the other edge end heap node to this node in the graph \n - otherwise just do a heappush \n\nFor each edge \n- get the edge score (scores[a] + scores[b]) \n- for each neighbor of a that is not b \n - for each neighbor of b that is not the a neighbor nor a nor b \n - maximize answer versus edge score + score of neighbors \n\nreturn maximized answer if you ever did or -1 \n\n# Complexity\n- Time complexity : O(E log N) \n - O(E) to loop through edges \n - O(E) to loop through edges \n - log(N) to get the neighbors of specifications as we can simply skip over non-matches and have eliminated others \n - Total is O(E log N) \n\n- Space complexity : O(N)\n - Store 3 edges for each N nodes so O(N) \n\n# Code\n```\nclass Solution:\n def maximumScore(self, scores: List[int], edges: List[List[int]]) -> int:\n # build graph as list of heaps of score of move to neighbor and neighbor of size 3 \n graph = [[] for i in range(len(scores))]\n # for a, b in edges \n for a, b in edges : \n # make your nodes \n b_node = (scores[b], b)\n a_node = (scores[a], a)\n # if len of heap is 3 -> heapq.heappushpop is faster than a push and then a pop after a check \n # otherwise, just push it in. \n # do for both heaps with opposite nodes \n if len(graph[a]) == 3 : \n heapq.heappushpop(graph[a], b_node)\n else : \n heapq.heappush(graph[a], b_node)\n if len(graph[b]) == 3 : \n heapq.heappushpop(graph[b], a_node)\n else : \n heapq.heappush(graph[b], a_node)\n \n # start at -1 as default \n answer = -1 \n # for pair in edges \n for a, b in edges : \n # get the edge score \n edge_score = scores[a] + scores[b]\n # for score, neighbor in graph[a] where neighbor is not b and cannot be a \n for a_score, a_neighbor in graph[a] : \n if a_neighbor == b : \n continue \n else : \n # for score, neighbor in graph[b] where neighbor is not a, b, or a_neighbor \n for b_score, b_neighbor in graph[b] : \n if b_neighbor == a_neighbor or b_neighbor == a : \n continue \n else : \n # maximize answer vs edge_score with travel scores associated \n answer = max(answer, edge_score + a_score + b_score) \n # return maximal \n return answer \n``` | 0 | There is an **undirected** graph with `n` nodes, numbered from `0` to `n - 1`.
You are given a **0-indexed** integer array `scores` of length `n` where `scores[i]` denotes the score of node `i`. You are also given a 2D integer array `edges` where `edges[i] = [ai, bi]` denotes that there exists an **undirected** edge connecting nodes `ai` and `bi`.
A node sequence is **valid** if it meets the following conditions:
* There is an edge connecting every pair of **adjacent** nodes in the sequence.
* No node appears more than once in the sequence.
The score of a node sequence is defined as the **sum** of the scores of the nodes in the sequence.
Return _the **maximum score** of a valid node sequence with a length of_ `4`_._ If no such sequence exists, return `-1`.
**Example 1:**
**Input:** scores = \[5,2,9,8,4\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[0,2\],\[1,3\],\[2,4\]\]
**Output:** 24
**Explanation:** The figure above shows the graph and the chosen node sequence \[0,1,2,3\].
The score of the node sequence is 5 + 2 + 9 + 8 = 24.
It can be shown that no other node sequence has a score of more than 24.
Note that the sequences \[3,1,2,0\] and \[1,0,2,3\] are also valid and have a score of 24.
The sequence \[0,3,2,4\] is not valid since no edge connects nodes 0 and 3.
**Example 2:**
**Input:** scores = \[9,20,6,4,11,12\], edges = \[\[0,3\],\[5,3\],\[2,4\],\[1,3\]\]
**Output:** -1
**Explanation:** The figure above shows the graph.
There are no valid node sequences of length 4, so we return -1.
**Constraints:**
* `n == scores.length`
* `4 <= n <= 5 * 104`
* `1 <= scores[i] <= 108`
* `0 <= edges.length <= 5 * 104`
* `edges[i].length == 2`
* `0 <= ai, bi <= n - 1`
* `ai != bi`
* There are no duplicate edges. | Is the sum of two even numbers even or odd? How about two odd numbers? One odd number and one even number? If there is an even number of odd numbers, the sum will be even and vice versa. Create an integer array to store all the even numbers in nums and another array to store all the odd numbers in nums. Sort both arrays. |
Short Linear-Time Solution in Python, Faster than 100% | maximum-score-of-a-node-sequence | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nThe solution is three connected edges that cover four nodes where the sum of the four nodes are maximized. \nFor each edge `(u, v)` we can find two best neighboring edges `(p, u)` and `(v, q)` so that `u, v, p, q` are four distinct nodes and their sum is maximized. \nTo speed up, we precompute the best 3 edges of each node in the first scan of all edges in $$O(|E|)$$. As a result, the second scan of all edges can find the solution in $$O(|E|)$$.\n\n# Complexity\n- Time complexity: $$O(|E|)$$ where $|E|$ is the number of edges. \n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(|V|)$$ where $|V|$ is the number of nodes. \n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maximumScore(self, scores: List[int], edges: List[List[int]]) -> int:\n closest = defaultdict(set)\n for u, v in edges:\n for p, q in [(u, v), (v, u)]:\n if len(closest[p]) < 3:\n closest[p].add(q)\n else:\n r = min(closest[p], key=lambda x: scores[x])\n if scores[r] < scores[q]:\n closest[p].remove(r)\n closest[p].add(q)\n return max((scores[u] + scores[v] + scores[p] + scores[q] \n for u, v in edges for p in closest[u] if p != v for q in closest[v] if q != p and q != u),\n default=-1)\n \n``` | 0 | There is an **undirected** graph with `n` nodes, numbered from `0` to `n - 1`.
You are given a **0-indexed** integer array `scores` of length `n` where `scores[i]` denotes the score of node `i`. You are also given a 2D integer array `edges` where `edges[i] = [ai, bi]` denotes that there exists an **undirected** edge connecting nodes `ai` and `bi`.
A node sequence is **valid** if it meets the following conditions:
* There is an edge connecting every pair of **adjacent** nodes in the sequence.
* No node appears more than once in the sequence.
The score of a node sequence is defined as the **sum** of the scores of the nodes in the sequence.
Return _the **maximum score** of a valid node sequence with a length of_ `4`_._ If no such sequence exists, return `-1`.
**Example 1:**
**Input:** scores = \[5,2,9,8,4\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[0,2\],\[1,3\],\[2,4\]\]
**Output:** 24
**Explanation:** The figure above shows the graph and the chosen node sequence \[0,1,2,3\].
The score of the node sequence is 5 + 2 + 9 + 8 = 24.
It can be shown that no other node sequence has a score of more than 24.
Note that the sequences \[3,1,2,0\] and \[1,0,2,3\] are also valid and have a score of 24.
The sequence \[0,3,2,4\] is not valid since no edge connects nodes 0 and 3.
**Example 2:**
**Input:** scores = \[9,20,6,4,11,12\], edges = \[\[0,3\],\[5,3\],\[2,4\],\[1,3\]\]
**Output:** -1
**Explanation:** The figure above shows the graph.
There are no valid node sequences of length 4, so we return -1.
**Constraints:**
* `n == scores.length`
* `4 <= n <= 5 * 104`
* `1 <= scores[i] <= 108`
* `0 <= edges.length <= 5 * 104`
* `edges[i].length == 2`
* `0 <= ai, bi <= n - 1`
* `ai != bi`
* There are no duplicate edges. | Is the sum of two even numbers even or odd? How about two odd numbers? One odd number and one even number? If there is an even number of odd numbers, the sum will be even and vice versa. Create an integer array to store all the even numbers in nums and another array to store all the odd numbers in nums. Sort both arrays. |
Considering top 3 neighbours || Graphs || Python3 | maximum-score-of-a-node-sequence | 0 | 1 | # Code\n```\nfrom collections import defaultdict\n\nclass Solution:\n def maximumScore(self, scores: List[int], edges: List[List[int]]) -> int:\n\n n = len(scores)\n graphs = defaultdict(list)\n\n for a, b in edges:\n graphs[a].append([scores[b], b])\n graphs[b].append([scores[a], a])\n\n for i in range(n):\n graphs[i] = nlargest(3, graphs[i])\n\n res = -1\n\n for i, j in edges:\n for vii, ii in graphs[i]:\n for vjj, jj in graphs[j]:\n if ii != jj and ii != j and jj != i:\n res = max(res, vii + vjj + scores[i] + scores[j])\n return res\n``` | 0 | There is an **undirected** graph with `n` nodes, numbered from `0` to `n - 1`.
You are given a **0-indexed** integer array `scores` of length `n` where `scores[i]` denotes the score of node `i`. You are also given a 2D integer array `edges` where `edges[i] = [ai, bi]` denotes that there exists an **undirected** edge connecting nodes `ai` and `bi`.
A node sequence is **valid** if it meets the following conditions:
* There is an edge connecting every pair of **adjacent** nodes in the sequence.
* No node appears more than once in the sequence.
The score of a node sequence is defined as the **sum** of the scores of the nodes in the sequence.
Return _the **maximum score** of a valid node sequence with a length of_ `4`_._ If no such sequence exists, return `-1`.
**Example 1:**
**Input:** scores = \[5,2,9,8,4\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[0,2\],\[1,3\],\[2,4\]\]
**Output:** 24
**Explanation:** The figure above shows the graph and the chosen node sequence \[0,1,2,3\].
The score of the node sequence is 5 + 2 + 9 + 8 = 24.
It can be shown that no other node sequence has a score of more than 24.
Note that the sequences \[3,1,2,0\] and \[1,0,2,3\] are also valid and have a score of 24.
The sequence \[0,3,2,4\] is not valid since no edge connects nodes 0 and 3.
**Example 2:**
**Input:** scores = \[9,20,6,4,11,12\], edges = \[\[0,3\],\[5,3\],\[2,4\],\[1,3\]\]
**Output:** -1
**Explanation:** The figure above shows the graph.
There are no valid node sequences of length 4, so we return -1.
**Constraints:**
* `n == scores.length`
* `4 <= n <= 5 * 104`
* `1 <= scores[i] <= 108`
* `0 <= edges.length <= 5 * 104`
* `edges[i].length == 2`
* `0 <= ai, bi <= n - 1`
* `ai != bi`
* There are no duplicate edges. | Is the sum of two even numbers even or odd? How about two odd numbers? One odd number and one even number? If there is an even number of odd numbers, the sum will be even and vice versa. Create an integer array to store all the even numbers in nums and another array to store all the odd numbers in nums. Sort both arrays. |
Elog(something) solution for u shit | maximum-score-of-a-node-sequence | 0 | 1 | \n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom sortedcontainers import SortedList\nclass Solution:\n def maximumScore(self, scores: List[int], edges: List[List[int]]) -> int:\n ans,g=-1,defaultdict(SortedList)\n for x,y in edges:\n print(x,y)\n g[x].add((scores[y],y))\n g[y].add((scores[x],x))\n ans=-1\n for x,y in edges:\n res=scores[x]+scores[y]\n g[x].remove((scores[y],y))\n g[y].remove((scores[x],x))\n i=len(g[x])-1\n j=len(g[y])-1\n if i>=0 and j>=0 and g[x][i]==g[y][j]:\n if j-1>=0 and i-1>=0:\n if g[x][i-1][0]>=g[y][j-1][0]:\n i-=1\n else:\n j-=1\n elif j-1>=0:\n j-=1\n elif i-1>=0:\n i-=1\n else:\n j-=1\n if i>=0 and j>=0:\n ans=max(ans,res+g[x][i][0]+g[y][j][0])\n g[x].add((scores[y],y))\n g[y].add((scores[x],x))\n return ans\n``` | 0 | There is an **undirected** graph with `n` nodes, numbered from `0` to `n - 1`.
You are given a **0-indexed** integer array `scores` of length `n` where `scores[i]` denotes the score of node `i`. You are also given a 2D integer array `edges` where `edges[i] = [ai, bi]` denotes that there exists an **undirected** edge connecting nodes `ai` and `bi`.
A node sequence is **valid** if it meets the following conditions:
* There is an edge connecting every pair of **adjacent** nodes in the sequence.
* No node appears more than once in the sequence.
The score of a node sequence is defined as the **sum** of the scores of the nodes in the sequence.
Return _the **maximum score** of a valid node sequence with a length of_ `4`_._ If no such sequence exists, return `-1`.
**Example 1:**
**Input:** scores = \[5,2,9,8,4\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[0,2\],\[1,3\],\[2,4\]\]
**Output:** 24
**Explanation:** The figure above shows the graph and the chosen node sequence \[0,1,2,3\].
The score of the node sequence is 5 + 2 + 9 + 8 = 24.
It can be shown that no other node sequence has a score of more than 24.
Note that the sequences \[3,1,2,0\] and \[1,0,2,3\] are also valid and have a score of 24.
The sequence \[0,3,2,4\] is not valid since no edge connects nodes 0 and 3.
**Example 2:**
**Input:** scores = \[9,20,6,4,11,12\], edges = \[\[0,3\],\[5,3\],\[2,4\],\[1,3\]\]
**Output:** -1
**Explanation:** The figure above shows the graph.
There are no valid node sequences of length 4, so we return -1.
**Constraints:**
* `n == scores.length`
* `4 <= n <= 5 * 104`
* `1 <= scores[i] <= 108`
* `0 <= edges.length <= 5 * 104`
* `edges[i].length == 2`
* `0 <= ai, bi <= n - 1`
* `ai != bi`
* There are no duplicate edges. | Is the sum of two even numbers even or odd? How about two odd numbers? One odd number and one even number? If there is an even number of odd numbers, the sum will be even and vice versa. Create an integer array to store all the even numbers in nums and another array to store all the odd numbers in nums. Sort both arrays. |
Python Solution with comments [DFS and Top 3 neighbors with Min Heap] | maximum-score-of-a-node-sequence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFirst solution is intuitive approach using DFS+backtracking, but this approach gets TLE. \n\nSecond solution uses top 3 neighbors, I just implemented this appoach https://leetcode.com/problems/maximum-score-of-a-node-sequence/solutions/1953669/python-explanation-with-pictures-top-3-neighbors/ using heap. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\nDFS+Backtracking\nV: number of vertices\n\n- Time complexity: O(V^2)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(V)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n\n\nTop 3 neighbor\n- Time complexity: O(V) \n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(V)\n\n# Code\n```\n# DFS Approach with backtracking\n\'\'\'\nDesc:\n* undirected graph\n* n nodes [0, n-1]\n* scores: integer array, length is n, index [0, ...n-1]\n scores[i] denotes the score of the node i\n* edges: [a,b] = node a connected to node b (bi-directional connection)\n* node sequence is VALID if:\n there is an edge connecting every pair of adjacent nodes in the sequence\n no node repetition in the sequence\n\n* score of the sequence = sum of node scores inside the sequence\n* RETURN = maximum score of a VALID node sequence with a length of 4, if not return -1\n\n\nApproach 1: DFS + Backtracking\n1 - build a graph from edges array\n2 - find each unique path with length of 4 which is VALID path\n3 - get the maximum valued path\n\n\nTime Complexity: O(V^2)\nSpace Complexity: O(V)\n\nDry on for the this input: \nscores = [5,2,9,8,4], edges = [[0,1],[1,2],[2,3],[0,2],[1,3],[2,4]]\n\n getMax(0, 0, ()) 24\n / \n getMax(1, 1, (0)) 19 \n /\n getMax(2, 2, (0, 1)) 17 \n / \\\n getMax(3, 3, (0, 1,2)) get(4,3,(0,1,2))\n / \\\n 8 4\n\'\'\'\n\n\nclass Solution:\n def maximumScore(self, scores: List[int], edges: List[List[int]]) -> int:\n # 1 - build a graph\n graph = defaultdict(set)\n\n for a, b in edges:\n graph[a].add(b)\n graph[b].add(a)\n\n def getMaxPathScore(vertex, level, visited):\n if level == 3:\n return scores[vertex]\n\n visited.add(vertex)\n score = -1\n\n for neighbor in graph[vertex]:\n if neighbor in visited:\n continue\n\n visited.add(neighbor)\n score = max(score, getMaxPathScore(neighbor, level+1, visited))\n visited.remove(neighbor)\n\n return -1 if score == -1 else score + scores[vertex] \n \n # 2 - iterate each vertex for path with 4 nodes\n ans, n = -1, len(scores)\n\n for vertex in range(n):\n ans = max(ans, getMaxPathScore(vertex, 0, set()))\n\n return ans\n```\n\n\n```\nfrom collections import defaultdict\nfrom heapq import heappop, heappush\n\nclass Solution:\n def maximumScore(self, scores: List[int], edges: List[List[int]]) -> int:\n graph = defaultdict(list)\n\n # uses min heap to keep the maximum scored neighbors\n def keepTop3(a, b):\n if len(graph[a]) >= 3:\n if graph[a][0][0] < scores[b]:\n heappop(graph[a])\n heappush(graph[a], (scores[b], b))\n else:\n heappush(graph[a], (scores[b], b))\n \n # build a graph by keeping maximum scored 3 neighbors using min heap\n for a, b in edges:\n keepTop3(a, b)\n keepTop3(b, a)\n\n ans = -1\n\n # iterate each edge one by one, and find the node with 3 edges c-a-b-d\n for a, b in edges:\n # because both a and b vertices are the middle two vertices. \n # their neighbor counts has to be bigger than 1, since a is connected to c and d (need at least 2 edges), b is connected to a and d (needs at least 2 edges)\n if len(graph[a]) <= 1 or len(graph[b]) <= 1: \n continue\n\n for score_c, c in graph[a]:\n for score_d, d in graph[b]:\n if c not in [a,b] and d not in [a,b] and c != d:\n ans = max(ans, scores[a] + scores[b] + score_c + score_d)\n\n return ans\n \n``` | 0 | There is an **undirected** graph with `n` nodes, numbered from `0` to `n - 1`.
You are given a **0-indexed** integer array `scores` of length `n` where `scores[i]` denotes the score of node `i`. You are also given a 2D integer array `edges` where `edges[i] = [ai, bi]` denotes that there exists an **undirected** edge connecting nodes `ai` and `bi`.
A node sequence is **valid** if it meets the following conditions:
* There is an edge connecting every pair of **adjacent** nodes in the sequence.
* No node appears more than once in the sequence.
The score of a node sequence is defined as the **sum** of the scores of the nodes in the sequence.
Return _the **maximum score** of a valid node sequence with a length of_ `4`_._ If no such sequence exists, return `-1`.
**Example 1:**
**Input:** scores = \[5,2,9,8,4\], edges = \[\[0,1\],\[1,2\],\[2,3\],\[0,2\],\[1,3\],\[2,4\]\]
**Output:** 24
**Explanation:** The figure above shows the graph and the chosen node sequence \[0,1,2,3\].
The score of the node sequence is 5 + 2 + 9 + 8 = 24.
It can be shown that no other node sequence has a score of more than 24.
Note that the sequences \[3,1,2,0\] and \[1,0,2,3\] are also valid and have a score of 24.
The sequence \[0,3,2,4\] is not valid since no edge connects nodes 0 and 3.
**Example 2:**
**Input:** scores = \[9,20,6,4,11,12\], edges = \[\[0,3\],\[5,3\],\[2,4\],\[1,3\]\]
**Output:** -1
**Explanation:** The figure above shows the graph.
There are no valid node sequences of length 4, so we return -1.
**Constraints:**
* `n == scores.length`
* `4 <= n <= 5 * 104`
* `1 <= scores[i] <= 108`
* `0 <= edges.length <= 5 * 104`
* `edges[i].length == 2`
* `0 <= ai, bi <= n - 1`
* `ai != bi`
* There are no duplicate edges. | Is the sum of two even numbers even or odd? How about two odd numbers? One odd number and one even number? If there is an even number of odd numbers, the sum will be even and vice versa. Create an integer array to store all the even numbers in nums and another array to store all the odd numbers in nums. Sort both arrays. |
Python 6line code || 98.60 %Beats || Simple Code | calculate-digit-sum-of-a-string | 0 | 1 | **If you got help from this,... Plz Upvote .. it encourage me**\n# Code\nShort Way:\n```\nclass Solution:\n def digitSum(self, s: str, k: int) -> str:\n while len(s) > k:\n new_s = \'\'\n for i in range(0,len(s), k):\n new_s += str(sum(int(d) for d in s[i:i+k]))\n s = new_s \n return s\n\n \n```\n.\nSame Approach but long way to understand.\n```\nclass Solution:\n def digitSum(self, s: str, k: int) -> str:\n while len(s) > k:\n new_s = \'\'\n for i in range(0,len(s), k):\n temp = 0\n for d in s[i:i+k]:\n temp += int(d)\n \n new_s += str(temp)\n\n s = new_s\n \n return s\n``` | 5 | You are given a string `s` consisting of digits and an integer `k`.
A **round** can be completed if the length of `s` is greater than `k`. In one round, do the following:
1. **Divide** `s` into **consecutive groups** of size `k` such that the first `k` characters are in the first group, the next `k` characters are in the second group, and so on. **Note** that the size of the last group can be smaller than `k`.
2. **Replace** each group of `s` with a string representing the sum of all its digits. For example, `"346 "` is replaced with `"13 "` because `3 + 4 + 6 = 13`.
3. **Merge** consecutive groups together to form a new string. If the length of the string is greater than `k`, repeat from step `1`.
Return `s` _after all rounds have been completed_.
**Example 1:**
**Input:** s = "11111222223 ", k = 3
**Output:** "135 "
**Explanation:**
- For the first round, we divide s into groups of size 3: "111 ", "112 ", "222 ", and "23 ".
Then we calculate the digit sum of each group: 1 + 1 + 1 = 3, 1 + 1 + 2 = 4, 2 + 2 + 2 = 6, and 2 + 3 = 5.
So, s becomes "3 " + "4 " + "6 " + "5 " = "3465 " after the first round.
- For the second round, we divide s into "346 " and "5 ".
Then we calculate the digit sum of each group: 3 + 4 + 6 = 13, 5 = 5.
So, s becomes "13 " + "5 " = "135 " after second round.
Now, s.length <= k, so we return "135 " as the answer.
**Example 2:**
**Input:** s = "00000000 ", k = 3
**Output:** "000 "
**Explanation:**
We divide s into "000 ", "000 ", and "00 ".
Then we calculate the digit sum of each group: 0 + 0 + 0 = 0, 0 + 0 + 0 = 0, and 0 + 0 = 0.
s becomes "0 " + "0 " + "0 " = "000 ", whose length is equal to k, so we return "000 ".
**Constraints:**
* `1 <= s.length <= 100`
* `2 <= k <= 100`
* `s` consists of digits only. | You can check the opposite: check if there is a ‘b’ before an ‘a’. Then, negate and return that answer. s should not have any occurrences of “ba” as a substring. |
Python Elegant & Short | Pythonic | Iterative + Recursive | calculate-digit-sum-of-a-string | 0 | 1 | \tclass Solution:\n\t\tdef digitSum(self, s: str, k: int) -> str:\n\t\t\t"""Iterative version"""\n\t\t\twhile len(s) > k:\n\t\t\t\ts = self.round(s, k)\n\t\t\treturn s\n\t\n\t\tdef digitSum(self, s: str, k: int) -> str:\n\t\t\t"""Recursive version"""\n\t\t\tif len(s) <= k:\n\t\t\t\treturn s\n\t\t\treturn self.digitSum(self.round(s, k), k)\n\n\t\t@classmethod\n\t\tdef round(cls, s: str, k: int) -> str:\n\t\t\treturn \'\'.join(map(cls.add_digits, cls.slice(s, k)))\n\n\t\t@staticmethod\n\t\tdef add_digits(s: str) -> str:\n\t\t\treturn str(sum(int(d) for d in s))\n\n\t\t@staticmethod\n\t\tdef slice(s: str, k: int):\n\t\t\tfor i in range(0, len(s), k):\n\t\t\t\tyield s[i:i + k]\n | 2 | You are given a string `s` consisting of digits and an integer `k`.
A **round** can be completed if the length of `s` is greater than `k`. In one round, do the following:
1. **Divide** `s` into **consecutive groups** of size `k` such that the first `k` characters are in the first group, the next `k` characters are in the second group, and so on. **Note** that the size of the last group can be smaller than `k`.
2. **Replace** each group of `s` with a string representing the sum of all its digits. For example, `"346 "` is replaced with `"13 "` because `3 + 4 + 6 = 13`.
3. **Merge** consecutive groups together to form a new string. If the length of the string is greater than `k`, repeat from step `1`.
Return `s` _after all rounds have been completed_.
**Example 1:**
**Input:** s = "11111222223 ", k = 3
**Output:** "135 "
**Explanation:**
- For the first round, we divide s into groups of size 3: "111 ", "112 ", "222 ", and "23 ".
Then we calculate the digit sum of each group: 1 + 1 + 1 = 3, 1 + 1 + 2 = 4, 2 + 2 + 2 = 6, and 2 + 3 = 5.
So, s becomes "3 " + "4 " + "6 " + "5 " = "3465 " after the first round.
- For the second round, we divide s into "346 " and "5 ".
Then we calculate the digit sum of each group: 3 + 4 + 6 = 13, 5 = 5.
So, s becomes "13 " + "5 " = "135 " after second round.
Now, s.length <= k, so we return "135 " as the answer.
**Example 2:**
**Input:** s = "00000000 ", k = 3
**Output:** "000 "
**Explanation:**
We divide s into "000 ", "000 ", and "00 ".
Then we calculate the digit sum of each group: 0 + 0 + 0 = 0, 0 + 0 + 0 = 0, and 0 + 0 = 0.
s becomes "0 " + "0 " + "0 " = "000 ", whose length is equal to k, so we return "000 ".
**Constraints:**
* `1 <= s.length <= 100`
* `2 <= k <= 100`
* `s` consists of digits only. | You can check the opposite: check if there is a ‘b’ before an ‘a’. Then, negate and return that answer. s should not have any occurrences of “ba” as a substring. |
[Python 3] Convert string to list and use brute-force | calculate-digit-sum-of-a-string | 0 | 1 | ```python3 []\nclass Solution:\n def digitSum(self, s: str, k: int) -> str:\n s = list(map(int, s))\n while len(s) > k:\n tmp = []\n for i in range(0, len(s), k):\n S = sum(s[i:i+k])\n for d in (str(S)):\n tmp.append(int(d))\n s = tmp\n\n return \'\'.join(map(str, s))\n``` | 4 | You are given a string `s` consisting of digits and an integer `k`.
A **round** can be completed if the length of `s` is greater than `k`. In one round, do the following:
1. **Divide** `s` into **consecutive groups** of size `k` such that the first `k` characters are in the first group, the next `k` characters are in the second group, and so on. **Note** that the size of the last group can be smaller than `k`.
2. **Replace** each group of `s` with a string representing the sum of all its digits. For example, `"346 "` is replaced with `"13 "` because `3 + 4 + 6 = 13`.
3. **Merge** consecutive groups together to form a new string. If the length of the string is greater than `k`, repeat from step `1`.
Return `s` _after all rounds have been completed_.
**Example 1:**
**Input:** s = "11111222223 ", k = 3
**Output:** "135 "
**Explanation:**
- For the first round, we divide s into groups of size 3: "111 ", "112 ", "222 ", and "23 ".
Then we calculate the digit sum of each group: 1 + 1 + 1 = 3, 1 + 1 + 2 = 4, 2 + 2 + 2 = 6, and 2 + 3 = 5.
So, s becomes "3 " + "4 " + "6 " + "5 " = "3465 " after the first round.
- For the second round, we divide s into "346 " and "5 ".
Then we calculate the digit sum of each group: 3 + 4 + 6 = 13, 5 = 5.
So, s becomes "13 " + "5 " = "135 " after second round.
Now, s.length <= k, so we return "135 " as the answer.
**Example 2:**
**Input:** s = "00000000 ", k = 3
**Output:** "000 "
**Explanation:**
We divide s into "000 ", "000 ", and "00 ".
Then we calculate the digit sum of each group: 0 + 0 + 0 = 0, 0 + 0 + 0 = 0, and 0 + 0 = 0.
s becomes "0 " + "0 " + "0 " = "000 ", whose length is equal to k, so we return "000 ".
**Constraints:**
* `1 <= s.length <= 100`
* `2 <= k <= 100`
* `s` consists of digits only. | You can check the opposite: check if there is a ‘b’ before an ‘a’. Then, negate and return that answer. s should not have any occurrences of “ba” as a substring. |
Python3 elegant pythonic clean and easy to understand | calculate-digit-sum-of-a-string | 0 | 1 | Simple while loop and slicing to repeat 3 set process and aggregation\n\n```\nclass Solution:\n def digitSum(self, s: str, k: int) -> str:\n while len(s) > k:\n set_3 = [s[i:i+k] for i in range(0, len(s), k)]\n s = \'\'\n for e in set_3:\n val = 0\n for n in e:\n val += int(n)\n s += str(val)\n return s\n```\n\ncan be condensed and more pythonic:\n\n```\n while len(s) > k:\n set_3 = [s[i:i+k] for i in range(0, len(s), k)]\n s = \'\'\n for e in set_3:\n s += str(sum([int(n) for n in e]))\n return s\n``` | 14 | You are given a string `s` consisting of digits and an integer `k`.
A **round** can be completed if the length of `s` is greater than `k`. In one round, do the following:
1. **Divide** `s` into **consecutive groups** of size `k` such that the first `k` characters are in the first group, the next `k` characters are in the second group, and so on. **Note** that the size of the last group can be smaller than `k`.
2. **Replace** each group of `s` with a string representing the sum of all its digits. For example, `"346 "` is replaced with `"13 "` because `3 + 4 + 6 = 13`.
3. **Merge** consecutive groups together to form a new string. If the length of the string is greater than `k`, repeat from step `1`.
Return `s` _after all rounds have been completed_.
**Example 1:**
**Input:** s = "11111222223 ", k = 3
**Output:** "135 "
**Explanation:**
- For the first round, we divide s into groups of size 3: "111 ", "112 ", "222 ", and "23 ".
Then we calculate the digit sum of each group: 1 + 1 + 1 = 3, 1 + 1 + 2 = 4, 2 + 2 + 2 = 6, and 2 + 3 = 5.
So, s becomes "3 " + "4 " + "6 " + "5 " = "3465 " after the first round.
- For the second round, we divide s into "346 " and "5 ".
Then we calculate the digit sum of each group: 3 + 4 + 6 = 13, 5 = 5.
So, s becomes "13 " + "5 " = "135 " after second round.
Now, s.length <= k, so we return "135 " as the answer.
**Example 2:**
**Input:** s = "00000000 ", k = 3
**Output:** "000 "
**Explanation:**
We divide s into "000 ", "000 ", and "00 ".
Then we calculate the digit sum of each group: 0 + 0 + 0 = 0, 0 + 0 + 0 = 0, and 0 + 0 = 0.
s becomes "0 " + "0 " + "0 " = "000 ", whose length is equal to k, so we return "000 ".
**Constraints:**
* `1 <= s.length <= 100`
* `2 <= k <= 100`
* `s` consists of digits only. | You can check the opposite: check if there is a ‘b’ before an ‘a’. Then, negate and return that answer. s should not have any occurrences of “ba” as a substring. |
Simple Python Solution | calculate-digit-sum-of-a-string | 0 | 1 | ```\nclass Solution:\n def digitSum(self, s: str, k: int) -> str:\n def divideString(s: str, k: int) -> List[str]: # Utility function to return list of divided groups.\n l, n = [], len(s)\n for i in range(0, n, k):\n l.append(s[i:min(i + k, n)])\n return l\n while len(s)>k: # Till size of s is greater than k\n arr, temp = divideString(s, k), [] # arr is the list of divided groups, temp will be the list of group sums\n for group in arr: # Traverse the group and add its digits\n group_sum = 0\n for digit in group:\n group_sum += int(digit)\n temp.append(str(group_sum)) # Sum of digits of current group\n s = \'\'.join(temp) # s is replaced by the group digit sum for each group.\n return s\n``` | 6 | You are given a string `s` consisting of digits and an integer `k`.
A **round** can be completed if the length of `s` is greater than `k`. In one round, do the following:
1. **Divide** `s` into **consecutive groups** of size `k` such that the first `k` characters are in the first group, the next `k` characters are in the second group, and so on. **Note** that the size of the last group can be smaller than `k`.
2. **Replace** each group of `s` with a string representing the sum of all its digits. For example, `"346 "` is replaced with `"13 "` because `3 + 4 + 6 = 13`.
3. **Merge** consecutive groups together to form a new string. If the length of the string is greater than `k`, repeat from step `1`.
Return `s` _after all rounds have been completed_.
**Example 1:**
**Input:** s = "11111222223 ", k = 3
**Output:** "135 "
**Explanation:**
- For the first round, we divide s into groups of size 3: "111 ", "112 ", "222 ", and "23 ".
Then we calculate the digit sum of each group: 1 + 1 + 1 = 3, 1 + 1 + 2 = 4, 2 + 2 + 2 = 6, and 2 + 3 = 5.
So, s becomes "3 " + "4 " + "6 " + "5 " = "3465 " after the first round.
- For the second round, we divide s into "346 " and "5 ".
Then we calculate the digit sum of each group: 3 + 4 + 6 = 13, 5 = 5.
So, s becomes "13 " + "5 " = "135 " after second round.
Now, s.length <= k, so we return "135 " as the answer.
**Example 2:**
**Input:** s = "00000000 ", k = 3
**Output:** "000 "
**Explanation:**
We divide s into "000 ", "000 ", and "00 ".
Then we calculate the digit sum of each group: 0 + 0 + 0 = 0, 0 + 0 + 0 = 0, and 0 + 0 = 0.
s becomes "0 " + "0 " + "0 " = "000 ", whose length is equal to k, so we return "000 ".
**Constraints:**
* `1 <= s.length <= 100`
* `2 <= k <= 100`
* `s` consists of digits only. | You can check the opposite: check if there is a ‘b’ before an ‘a’. Then, negate and return that answer. s should not have any occurrences of “ba” as a substring. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.