title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
Ever used pairwise() ?? | find-the-original-array-of-prefix-xor | 0 | 1 | # Intuition\nI have knowledge of builtin modules.\n\n# Code\n```\nclass Solution:\n def findArray(self, pref: List[int]) -> List[int]:\n return [pref[0]] + [ a ^ b for a, b in pairwise(pref)]\n```\n\n> Comment a better solution than this. | 1 | You are given an **integer** array `pref` of size `n`. Find and return _the array_ `arr` _of size_ `n` _that satisfies_:
* `pref[i] = arr[0] ^ arr[1] ^ ... ^ arr[i]`.
Note that `^` denotes the **bitwise-xor** operation.
It can be proven that the answer is **unique**.
**Example 1:**
**Input:** pref = \[5,2,0,3,1\]
**Output:** \[5,7,2,3,2\]
**Explanation:** From the array \[5,7,2,3,2\] we have the following:
- pref\[0\] = 5.
- pref\[1\] = 5 ^ 7 = 2.
- pref\[2\] = 5 ^ 7 ^ 2 = 0.
- pref\[3\] = 5 ^ 7 ^ 2 ^ 3 = 3.
- pref\[4\] = 5 ^ 7 ^ 2 ^ 3 ^ 2 = 1.
**Example 2:**
**Input:** pref = \[13\]
**Output:** \[13\]
**Explanation:** We have pref\[0\] = arr\[0\] = 13.
**Constraints:**
* `1 <= pref.length <= 105`
* `0 <= pref[i] <= 106` | null |
Single list-comprehension (without concat or ifs) using walrus operator | find-the-original-array-of-prefix-xor | 0 | 1 | Additional solution to the list, this time using python\'s `walrus operator` which allows us to build the array result in a single list comprehension expression.\nWith the idea of `i++` in other languages, we use the value of `x` and assign it to `last` for the next iteration.\n\nExample:\n```\nlast = 3\nx = 7\nresult = last^(last:=x)\n\n# After evaluating `result`, each variable equals to:\n# result = 3^7 = 4\n# last = 7\n# x = 7\n```\n\nAdd this to a list comprehension and you get a less-readable but faster array construction. It turns out to also be shorter than other one-liner solutions.\n\n\n# Code\n```\nclass Solution:\n def findArray(self, pref: List[int]) -> List[int]:\n last = 0\n return [last^(last:=x) for x in pref]\n``` | 1 | You are given an **integer** array `pref` of size `n`. Find and return _the array_ `arr` _of size_ `n` _that satisfies_:
* `pref[i] = arr[0] ^ arr[1] ^ ... ^ arr[i]`.
Note that `^` denotes the **bitwise-xor** operation.
It can be proven that the answer is **unique**.
**Example 1:**
**Input:** pref = \[5,2,0,3,1\]
**Output:** \[5,7,2,3,2\]
**Explanation:** From the array \[5,7,2,3,2\] we have the following:
- pref\[0\] = 5.
- pref\[1\] = 5 ^ 7 = 2.
- pref\[2\] = 5 ^ 7 ^ 2 = 0.
- pref\[3\] = 5 ^ 7 ^ 2 ^ 3 = 3.
- pref\[4\] = 5 ^ 7 ^ 2 ^ 3 ^ 2 = 1.
**Example 2:**
**Input:** pref = \[13\]
**Output:** \[13\]
**Explanation:** We have pref\[0\] = arr\[0\] = 13.
**Constraints:**
* `1 <= pref.length <= 105`
* `0 <= pref[i] <= 106` | null |
✅✅OPTIMIZED PYTHON | C++ SOLUTION ✅✅ | find-the-original-array-of-prefix-xor | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Python3 solution \n```\nclass Solution:\n def findArray(self, pref: List[int]) -> List[int]:\n ans=[pref[0]]\n ans.extend(pref[i]^pref[i-1] for i in range(1,len(pref)))\n return ans \n\n \n \n```\n# C++ SOLUTION \n```\nclass Solution {\npublic:\n vector<int> findArray(vector<int>& pref) {\n std::vector<int> ans;\n ans.push_back(pref[0]);\n\n for (int i = 1; i < pref.size(); i++) {\n ans.push_back(pref[i] ^ pref[i - 1]);\n }\n\n return ans;\n }\n};\n``` | 1 | You are given an **integer** array `pref` of size `n`. Find and return _the array_ `arr` _of size_ `n` _that satisfies_:
* `pref[i] = arr[0] ^ arr[1] ^ ... ^ arr[i]`.
Note that `^` denotes the **bitwise-xor** operation.
It can be proven that the answer is **unique**.
**Example 1:**
**Input:** pref = \[5,2,0,3,1\]
**Output:** \[5,7,2,3,2\]
**Explanation:** From the array \[5,7,2,3,2\] we have the following:
- pref\[0\] = 5.
- pref\[1\] = 5 ^ 7 = 2.
- pref\[2\] = 5 ^ 7 ^ 2 = 0.
- pref\[3\] = 5 ^ 7 ^ 2 ^ 3 = 3.
- pref\[4\] = 5 ^ 7 ^ 2 ^ 3 ^ 2 = 1.
**Example 2:**
**Input:** pref = \[13\]
**Output:** \[13\]
**Explanation:** We have pref\[0\] = arr\[0\] = 13.
**Constraints:**
* `1 <= pref.length <= 105`
* `0 <= pref[i] <= 106` | null |
2433. Find The Original Array of Prefix Xor | find-the-original-array-of-prefix-xor | 0 | 1 | \n# Code\n```\nclass Solution:\n def findArray(self, pref: List[int]) -> List[int]:\n x = []\n res = 0\n for i in range(0,len(pref)):\n if(i==0):\n x.append(pref[i])\n else:\n x.append(pref[i]^pref[i-1])\n return x\n\n \n``` | 1 | You are given an **integer** array `pref` of size `n`. Find and return _the array_ `arr` _of size_ `n` _that satisfies_:
* `pref[i] = arr[0] ^ arr[1] ^ ... ^ arr[i]`.
Note that `^` denotes the **bitwise-xor** operation.
It can be proven that the answer is **unique**.
**Example 1:**
**Input:** pref = \[5,2,0,3,1\]
**Output:** \[5,7,2,3,2\]
**Explanation:** From the array \[5,7,2,3,2\] we have the following:
- pref\[0\] = 5.
- pref\[1\] = 5 ^ 7 = 2.
- pref\[2\] = 5 ^ 7 ^ 2 = 0.
- pref\[3\] = 5 ^ 7 ^ 2 ^ 3 = 3.
- pref\[4\] = 5 ^ 7 ^ 2 ^ 3 ^ 2 = 1.
**Example 2:**
**Input:** pref = \[13\]
**Output:** \[13\]
**Explanation:** We have pref\[0\] = arr\[0\] = 13.
**Constraints:**
* `1 <= pref.length <= 105`
* `0 <= pref[i] <= 106` | null |
Backward loop, Python - Easy 3 line solution. | find-the-original-array-of-prefix-xor | 0 | 1 | # Intuition\n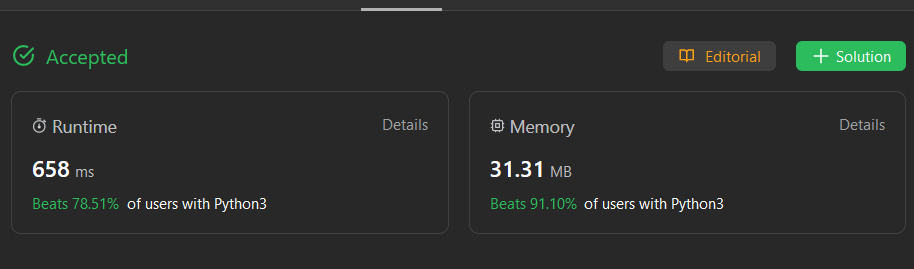\nModifying the input array to hold the result.\n\nFor the result, the result array should hold the values when xor-ed upto that index will give the value in the input array.\n\nFor this we can just use the property of XOR in which XOR when a number XORed with the same value twice returns the starting number.\n\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Looping forward(Not used): It will be easy to comprehend how the result builds up. XOR is commutative and Associative [i.e, (a^b)^c = a^(b^c)]. The value in index n in result array will be XOR of n and n-1 elements of input array.\n2. Looping backward: Looping forward will overwrite the values at each index, messing up the result, so to implement the answer without space lose we iterate through input array from backward and update pref[i] ^= pref[i-1].\n\n# Complexity\n- Time complexity:\n- O(n) time complexity due to 1 for loop.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n- O(1) space complexity due to no extra list or such datatypes made based on input values.\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findArray(self, pref: List[int]) -> List[int]:\n \n //#return [pref[0]] + [pref[i] ^ pref[i-1] for i in range(1,len(pref))]\n \n #arr = [pref[0]]\n #for i in range(1,len(pref)):\n # arr.append(pref[i] ^ pref[i-1])\n #return arr\n\n for i in range(len(pref)-1,0,-1):\n pref[i] = pref[i] ^ pref[i-1]\n return pref\n\n\n``` | 1 | You are given an **integer** array `pref` of size `n`. Find and return _the array_ `arr` _of size_ `n` _that satisfies_:
* `pref[i] = arr[0] ^ arr[1] ^ ... ^ arr[i]`.
Note that `^` denotes the **bitwise-xor** operation.
It can be proven that the answer is **unique**.
**Example 1:**
**Input:** pref = \[5,2,0,3,1\]
**Output:** \[5,7,2,3,2\]
**Explanation:** From the array \[5,7,2,3,2\] we have the following:
- pref\[0\] = 5.
- pref\[1\] = 5 ^ 7 = 2.
- pref\[2\] = 5 ^ 7 ^ 2 = 0.
- pref\[3\] = 5 ^ 7 ^ 2 ^ 3 = 3.
- pref\[4\] = 5 ^ 7 ^ 2 ^ 3 ^ 2 = 1.
**Example 2:**
**Input:** pref = \[13\]
**Output:** \[13\]
**Explanation:** We have pref\[0\] = arr\[0\] = 13.
**Constraints:**
* `1 <= pref.length <= 105`
* `0 <= pref[i] <= 106` | null |
Python3 Readable Short solution | find-the-original-array-of-prefix-xor | 0 | 1 | # Intuition\nSame intuition as given in the Hints section\n\n# Approach\nMake an extra array `arr` containing the first element of `prev`. Then append `prev[i] ^ prev[i-1]` to the array in a loop from `1` to `N-1`. \n\n# Complexity\n- Time complexity:\nO(N) as it implements a single for loop from 1 to N-1\n\n- Space complexity:\nO(N) as it takes an extra array of length N\n\n# Code\n```\nclass Solution:\n def findArray(self, pref: List[int]) -> List[int]:\n arr = [pref[0]]\n for i in range(1, len(pref)):\n arr.append(pref[i] ^ pref[i - 1])\n return arr\n``` | 1 | You are given an **integer** array `pref` of size `n`. Find and return _the array_ `arr` _of size_ `n` _that satisfies_:
* `pref[i] = arr[0] ^ arr[1] ^ ... ^ arr[i]`.
Note that `^` denotes the **bitwise-xor** operation.
It can be proven that the answer is **unique**.
**Example 1:**
**Input:** pref = \[5,2,0,3,1\]
**Output:** \[5,7,2,3,2\]
**Explanation:** From the array \[5,7,2,3,2\] we have the following:
- pref\[0\] = 5.
- pref\[1\] = 5 ^ 7 = 2.
- pref\[2\] = 5 ^ 7 ^ 2 = 0.
- pref\[3\] = 5 ^ 7 ^ 2 ^ 3 = 3.
- pref\[4\] = 5 ^ 7 ^ 2 ^ 3 ^ 2 = 1.
**Example 2:**
**Input:** pref = \[13\]
**Output:** \[13\]
**Explanation:** We have pref\[0\] = arr\[0\] = 13.
**Constraints:**
* `1 <= pref.length <= 105`
* `0 <= pref[i] <= 106` | null |
Python All Out Army Attack | maximum-enemy-forts-that-can-be-captured | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nCheck 2 possible army movements - Forward and Backward\n# Approach\n<!-- Describe your approach to solving the problem. -->\nRaise flag True when encounter 1 and set count to zero\nWhen flag True and station is 0 then increment count by 1\nIf flag False append the count to out list and set count to zero\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->O(n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->O(n)\n\n# Code\n```\nclass Solution:\n def captureForts(self, forts: List[int]) -> int:\n if 1 not in forts or -1 not in forts: return 0\n out1 = [] # movement forward\n flag = False\n count1 = 0\n for i in range(len(forts)):\n if forts[i] == 1:\n flag = True\n count1 = 0\n elif forts[i] == -1:\n flag = False\n out1.append(count1)\n count1 = 0\n elif flag and forts[i] == 0:\n count1+=1\n \n fla = False\n out2=[]\n count2=0\n for i in range(len(forts)-1, -1, -1): # movement backward\n if forts[i] == 1:\n fla = True\n count2 = 0\n elif forts[i] == -1:\n fla = False\n out2.append(count2)\n count2 = 0\n elif fla and forts[i] == 0:\n \n count2+=1\n \n out1+=out2\n return max(out1)\n\n``` | 1 | You are given a **0-indexed** integer array `forts` of length `n` representing the positions of several forts. `forts[i]` can be `-1`, `0`, or `1` where:
* `-1` represents there is **no fort** at the `ith` position.
* `0` indicates there is an **enemy** fort at the `ith` position.
* `1` indicates the fort at the `ith` the position is under your command.
Now you have decided to move your army from one of your forts at position `i` to an empty position `j` such that:
* `0 <= i, j <= n - 1`
* The army travels over enemy forts **only**. Formally, for all `k` where `min(i,j) < k < max(i,j)`, `forts[k] == 0.`
While moving the army, all the enemy forts that come in the way are **captured**.
Return _the **maximum** number of enemy forts that can be captured_. In case it is **impossible** to move your army, or you do not have any fort under your command, return `0`_._
**Example 1:**
**Input:** forts = \[1,0,0,-1,0,0,0,0,1\]
**Output:** 4
**Explanation:**
- Moving the army from position 0 to position 3 captures 2 enemy forts, at 1 and 2.
- Moving the army from position 8 to position 3 captures 4 enemy forts.
Since 4 is the maximum number of enemy forts that can be captured, we return 4.
**Example 2:**
**Input:** forts = \[0,0,1,-1\]
**Output:** 0
**Explanation:** Since no enemy fort can be captured, 0 is returned.
**Constraints:**
* `1 <= forts.length <= 1000`
* `-1 <= forts[i] <= 1` | null |
[C++|Java|Python3] scan - one pass | maximum-enemy-forts-that-can-be-captured | 1 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/3ffc910c12ff8c84890fb15351216a0fa85dc3ac) for solutions of biweekly 94. \n\n**Intuition**\nEffectively, this problem can be translated into "finding the number of 0\'s between a 1 and a -1". \n**Implementation**\n**C++**\n```\nclass Solution {\npublic: \n int captureForts(vector<int>& forts) {\n int ans = 0; \n for (int i = 0, ii = 0; i < forts.size(); ++i) \n if (forts[i]) {\n if (forts[ii] == -forts[i]) ans = max(ans, i-ii-1); \n ii = i; \n }\n return ans; \n }\n};\n```\n**Java**\n```\nclass Solution {\n\tpublic int captureForts(int[] forts) {\n\t\tint ans = 0; \n\t\tfor (int i = 0, ii = 0; i < forts.length; ++i) \n\t\t\tif (forts[i] != 0) {\n\t\t\t\tif (forts[ii] == -forts[i]) ans = Math.max(ans, i-ii-1); \n\t\t\t\tii = i; \n\t\t\t}\n\t\treturn ans; \n\t}\n}\n```\n**Python3**\n```\nclass Solution: \n def captureForts(self, forts: List[int]) -> int:\n ans = ii = 0 \n for i, x in enumerate(forts): \n if x: \n if forts[ii] == -x: ans = max(ans, i-ii-1)\n ii = i \n return ans \n```\n**Complexity**\nTime `O(N)`\nSpace `O(1)` | 31 | You are given a **0-indexed** integer array `forts` of length `n` representing the positions of several forts. `forts[i]` can be `-1`, `0`, or `1` where:
* `-1` represents there is **no fort** at the `ith` position.
* `0` indicates there is an **enemy** fort at the `ith` position.
* `1` indicates the fort at the `ith` the position is under your command.
Now you have decided to move your army from one of your forts at position `i` to an empty position `j` such that:
* `0 <= i, j <= n - 1`
* The army travels over enemy forts **only**. Formally, for all `k` where `min(i,j) < k < max(i,j)`, `forts[k] == 0.`
While moving the army, all the enemy forts that come in the way are **captured**.
Return _the **maximum** number of enemy forts that can be captured_. In case it is **impossible** to move your army, or you do not have any fort under your command, return `0`_._
**Example 1:**
**Input:** forts = \[1,0,0,-1,0,0,0,0,1\]
**Output:** 4
**Explanation:**
- Moving the army from position 0 to position 3 captures 2 enemy forts, at 1 and 2.
- Moving the army from position 8 to position 3 captures 4 enemy forts.
Since 4 is the maximum number of enemy forts that can be captured, we return 4.
**Example 2:**
**Input:** forts = \[0,0,1,-1\]
**Output:** 0
**Explanation:** Since no enemy fort can be captured, 0 is returned.
**Constraints:**
* `1 <= forts.length <= 1000`
* `-1 <= forts[i] <= 1` | null |
Simple Python Solution | maximum-enemy-forts-that-can-be-captured | 0 | 1 | ```\nclass Solution(object):\n def captureForts(self, forts):\n def solve(arr):\n max_ = 0\n count, flag = 0, False\n for num in arr:\n if num == 1: \n count, flag = 0, True\n elif num == -1: \n max_, count, flag = max(max_, count), 0, False\n else: \n if flag: count += 1\n return max_\n return max(solve(forts), solve(forts[::-1]))\n```\n**UpVote**, if you like it **:)** | 6 | You are given a **0-indexed** integer array `forts` of length `n` representing the positions of several forts. `forts[i]` can be `-1`, `0`, or `1` where:
* `-1` represents there is **no fort** at the `ith` position.
* `0` indicates there is an **enemy** fort at the `ith` position.
* `1` indicates the fort at the `ith` the position is under your command.
Now you have decided to move your army from one of your forts at position `i` to an empty position `j` such that:
* `0 <= i, j <= n - 1`
* The army travels over enemy forts **only**. Formally, for all `k` where `min(i,j) < k < max(i,j)`, `forts[k] == 0.`
While moving the army, all the enemy forts that come in the way are **captured**.
Return _the **maximum** number of enemy forts that can be captured_. In case it is **impossible** to move your army, or you do not have any fort under your command, return `0`_._
**Example 1:**
**Input:** forts = \[1,0,0,-1,0,0,0,0,1\]
**Output:** 4
**Explanation:**
- Moving the army from position 0 to position 3 captures 2 enemy forts, at 1 and 2.
- Moving the army from position 8 to position 3 captures 4 enemy forts.
Since 4 is the maximum number of enemy forts that can be captured, we return 4.
**Example 2:**
**Input:** forts = \[0,0,1,-1\]
**Output:** 0
**Explanation:** Since no enemy fort can be captured, 0 is returned.
**Constraints:**
* `1 <= forts.length <= 1000`
* `-1 <= forts[i] <= 1` | null |
[Python 3] Check left and right | maximum-enemy-forts-that-can-be-captured | 0 | 1 | ```\nclass Solution:\n def captureForts(self, forts: List[int]) -> int:\n res = 0\n \n for i in range(len(forts)):\n if forts[i] == 1:\n curr = i\n \n for l in range(i - 1, -1, -1):\n if forts[l] == 1:\n break\n if forts[l] == -1:\n res = max(res, curr - l)\n break\n \n for r in range(i + 1, len(forts)):\n if forts[r] == 1:\n break\n if forts[r] == -1:\n res = max(res, r - curr)\n break\n \n return res - 1 if res else 0\n``` | 1 | You are given a **0-indexed** integer array `forts` of length `n` representing the positions of several forts. `forts[i]` can be `-1`, `0`, or `1` where:
* `-1` represents there is **no fort** at the `ith` position.
* `0` indicates there is an **enemy** fort at the `ith` position.
* `1` indicates the fort at the `ith` the position is under your command.
Now you have decided to move your army from one of your forts at position `i` to an empty position `j` such that:
* `0 <= i, j <= n - 1`
* The army travels over enemy forts **only**. Formally, for all `k` where `min(i,j) < k < max(i,j)`, `forts[k] == 0.`
While moving the army, all the enemy forts that come in the way are **captured**.
Return _the **maximum** number of enemy forts that can be captured_. In case it is **impossible** to move your army, or you do not have any fort under your command, return `0`_._
**Example 1:**
**Input:** forts = \[1,0,0,-1,0,0,0,0,1\]
**Output:** 4
**Explanation:**
- Moving the army from position 0 to position 3 captures 2 enemy forts, at 1 and 2.
- Moving the army from position 8 to position 3 captures 4 enemy forts.
Since 4 is the maximum number of enemy forts that can be captured, we return 4.
**Example 2:**
**Input:** forts = \[0,0,1,-1\]
**Output:** 0
**Explanation:** Since no enemy fort can be captured, 0 is returned.
**Constraints:**
* `1 <= forts.length <= 1000`
* `-1 <= forts[i] <= 1` | null |
python easy solution beats 91% | maximum-enemy-forts-that-can-be-captured | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def captureForts(self, forts: List[int]) -> int:\n i = 0 \n j = i +1\n c = 0\n l = [0]\n while i < len(forts) and j < len(forts):\n if forts[i] == 1:\n while forts[j] == 0 and j < len(forts)-1:\n c += 1\n j += 1\n if forts[j] == 1:\n c = 0\n if forts[j] != -1 :\n c = 0\n l.append(c)\n c = 0\n i = j \n j = i + 1\n elif forts[i] == -1: \n while forts[j] == 0 and j < len(forts)-1:\n c += 1\n j += 1\n if forts[j] == -1:\n c = 0\n if forts[j] != 1:\n c = 0\n l.append(c)\n c = 0\n i = j\n j = i + 1\n else:\n i += 1\n j = i+1\n print(l)\n return max(l)\n\n \n``` | 2 | You are given a **0-indexed** integer array `forts` of length `n` representing the positions of several forts. `forts[i]` can be `-1`, `0`, or `1` where:
* `-1` represents there is **no fort** at the `ith` position.
* `0` indicates there is an **enemy** fort at the `ith` position.
* `1` indicates the fort at the `ith` the position is under your command.
Now you have decided to move your army from one of your forts at position `i` to an empty position `j` such that:
* `0 <= i, j <= n - 1`
* The army travels over enemy forts **only**. Formally, for all `k` where `min(i,j) < k < max(i,j)`, `forts[k] == 0.`
While moving the army, all the enemy forts that come in the way are **captured**.
Return _the **maximum** number of enemy forts that can be captured_. In case it is **impossible** to move your army, or you do not have any fort under your command, return `0`_._
**Example 1:**
**Input:** forts = \[1,0,0,-1,0,0,0,0,1\]
**Output:** 4
**Explanation:**
- Moving the army from position 0 to position 3 captures 2 enemy forts, at 1 and 2.
- Moving the army from position 8 to position 3 captures 4 enemy forts.
Since 4 is the maximum number of enemy forts that can be captured, we return 4.
**Example 2:**
**Input:** forts = \[0,0,1,-1\]
**Output:** 0
**Explanation:** Since no enemy fort can be captured, 0 is returned.
**Constraints:**
* `1 <= forts.length <= 1000`
* `-1 <= forts[i] <= 1` | null |
Python 2 pointer | maximum-enemy-forts-that-can-be-captured | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n 2 pointer\n move left pointer to right when right is not zero\n update max result when length of left to right window size > result\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n n\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def captureForts(self, forts: List[int]) -> int:\n res, i = 0, 0\n for j in range(len(forts)):\n if forts[j]:\n if forts[j] == - forts[i]:\n res = max(res,j-i-1)\n i = j\n return res\n``` | 1 | You are given a **0-indexed** integer array `forts` of length `n` representing the positions of several forts. `forts[i]` can be `-1`, `0`, or `1` where:
* `-1` represents there is **no fort** at the `ith` position.
* `0` indicates there is an **enemy** fort at the `ith` position.
* `1` indicates the fort at the `ith` the position is under your command.
Now you have decided to move your army from one of your forts at position `i` to an empty position `j` such that:
* `0 <= i, j <= n - 1`
* The army travels over enemy forts **only**. Formally, for all `k` where `min(i,j) < k < max(i,j)`, `forts[k] == 0.`
While moving the army, all the enemy forts that come in the way are **captured**.
Return _the **maximum** number of enemy forts that can be captured_. In case it is **impossible** to move your army, or you do not have any fort under your command, return `0`_._
**Example 1:**
**Input:** forts = \[1,0,0,-1,0,0,0,0,1\]
**Output:** 4
**Explanation:**
- Moving the army from position 0 to position 3 captures 2 enemy forts, at 1 and 2.
- Moving the army from position 8 to position 3 captures 4 enemy forts.
Since 4 is the maximum number of enemy forts that can be captured, we return 4.
**Example 2:**
**Input:** forts = \[0,0,1,-1\]
**Output:** 0
**Explanation:** Since no enemy fort can be captured, 0 is returned.
**Constraints:**
* `1 <= forts.length <= 1000`
* `-1 <= forts[i] <= 1` | null |
Python | O(n) | maximum-enemy-forts-that-can-be-captured | 0 | 1 | # Approach\n- So basically we just have to calculate the number of maximum continuous zerows between -1 and 1.\n- first we will find the first non zero element.\n- now we will start iteration through the array while calculating max zeros between 1 and -1.\n- every time we will find a zero we will count it and if we find 1/-1 then our previous element should be different from current element.\n\n# Complexity\n- Time complexity: O(n)\n- Space complexity: O(n)\n\n# Code\n```\nclass Solution:\n def captureForts(self, forts: List[int]) -> int:\n indx = 0\n count = 0\n prev = 0\n ans = 0\n for i in range(len(forts)):\n if forts[i]!=0:\n prev = forts[i]\n indx = i\n break\n \n for i in range(indx+1, len(forts)):\n if forts[i] == 0:\n count += 1\n else:\n if (forts[i] == 1 and prev == -1) or (forts[i] == -1 and prev == 1):\n ans = max(ans, count)\n count = 0\n prev = forts[i]\n else:\n count = 0\n \n return ans\n \n```\n---------------------\n**Upvote the post if you find it helpful.\nHappy coding.** | 1 | You are given a **0-indexed** integer array `forts` of length `n` representing the positions of several forts. `forts[i]` can be `-1`, `0`, or `1` where:
* `-1` represents there is **no fort** at the `ith` position.
* `0` indicates there is an **enemy** fort at the `ith` position.
* `1` indicates the fort at the `ith` the position is under your command.
Now you have decided to move your army from one of your forts at position `i` to an empty position `j` such that:
* `0 <= i, j <= n - 1`
* The army travels over enemy forts **only**. Formally, for all `k` where `min(i,j) < k < max(i,j)`, `forts[k] == 0.`
While moving the army, all the enemy forts that come in the way are **captured**.
Return _the **maximum** number of enemy forts that can be captured_. In case it is **impossible** to move your army, or you do not have any fort under your command, return `0`_._
**Example 1:**
**Input:** forts = \[1,0,0,-1,0,0,0,0,1\]
**Output:** 4
**Explanation:**
- Moving the army from position 0 to position 3 captures 2 enemy forts, at 1 and 2.
- Moving the army from position 8 to position 3 captures 4 enemy forts.
Since 4 is the maximum number of enemy forts that can be captured, we return 4.
**Example 2:**
**Input:** forts = \[0,0,1,-1\]
**Output:** 0
**Explanation:** Since no enemy fort can be captured, 0 is returned.
**Constraints:**
* `1 <= forts.length <= 1000`
* `-1 <= forts[i] <= 1` | null |
⬆️🔥✅ 100% | 0MS | 3 LINES | EASY | EXPLAINED | PROOF 🔥⬆️✅ | maximum-enemy-forts-that-can-be-captured | 1 | 1 | # upvote pls\n\n# calculate max distance btw 1 to -1 & viceversa \n<!-- Describe your approach to solving the problem. -->\n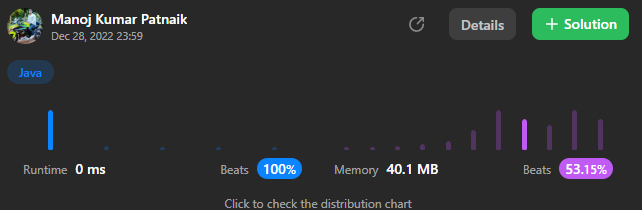\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n public int captureForts(int[] f) {\n int r=0,j=0;\n for(int i=0;i<f.length;i++){\n if(f[i]!=0){\n if(f[j]==-f[i]) r=Math.max(r,i-j-1);\n j=i;\n }\n }return r;\n }\n``` | 2 | You are given a **0-indexed** integer array `forts` of length `n` representing the positions of several forts. `forts[i]` can be `-1`, `0`, or `1` where:
* `-1` represents there is **no fort** at the `ith` position.
* `0` indicates there is an **enemy** fort at the `ith` position.
* `1` indicates the fort at the `ith` the position is under your command.
Now you have decided to move your army from one of your forts at position `i` to an empty position `j` such that:
* `0 <= i, j <= n - 1`
* The army travels over enemy forts **only**. Formally, for all `k` where `min(i,j) < k < max(i,j)`, `forts[k] == 0.`
While moving the army, all the enemy forts that come in the way are **captured**.
Return _the **maximum** number of enemy forts that can be captured_. In case it is **impossible** to move your army, or you do not have any fort under your command, return `0`_._
**Example 1:**
**Input:** forts = \[1,0,0,-1,0,0,0,0,1\]
**Output:** 4
**Explanation:**
- Moving the army from position 0 to position 3 captures 2 enemy forts, at 1 and 2.
- Moving the army from position 8 to position 3 captures 4 enemy forts.
Since 4 is the maximum number of enemy forts that can be captured, we return 4.
**Example 2:**
**Input:** forts = \[0,0,1,-1\]
**Output:** 0
**Explanation:** Since no enemy fort can be captured, 0 is returned.
**Constraints:**
* `1 <= forts.length <= 1000`
* `-1 <= forts[i] <= 1` | null |
Python3 solution | maximum-enemy-forts-that-can-be-captured | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def captureForts(self, forts: List[int]) -> int:\n lol=0\n for i in range(len(forts)):\n for j in range(len(forts)):\n \n if not i==j and (forts[j]==1 or forts[i]==1) and (forts[j]==-1 or forts[i]==-1):\n if i>j and len(set(forts[j+1:i]))==1 and forts[j+1:i][0]==0:\n lol=max(lol,len(forts[j+1:i]))\n \n if i<j and len(set(forts[j+1:i]))==1 and forts[j+1:i][0]==0:\n lol=max(lol,len(forts[j+1:i]))\n \n return lol\n``` | 1 | You are given a **0-indexed** integer array `forts` of length `n` representing the positions of several forts. `forts[i]` can be `-1`, `0`, or `1` where:
* `-1` represents there is **no fort** at the `ith` position.
* `0` indicates there is an **enemy** fort at the `ith` position.
* `1` indicates the fort at the `ith` the position is under your command.
Now you have decided to move your army from one of your forts at position `i` to an empty position `j` such that:
* `0 <= i, j <= n - 1`
* The army travels over enemy forts **only**. Formally, for all `k` where `min(i,j) < k < max(i,j)`, `forts[k] == 0.`
While moving the army, all the enemy forts that come in the way are **captured**.
Return _the **maximum** number of enemy forts that can be captured_. In case it is **impossible** to move your army, or you do not have any fort under your command, return `0`_._
**Example 1:**
**Input:** forts = \[1,0,0,-1,0,0,0,0,1\]
**Output:** 4
**Explanation:**
- Moving the army from position 0 to position 3 captures 2 enemy forts, at 1 and 2.
- Moving the army from position 8 to position 3 captures 4 enemy forts.
Since 4 is the maximum number of enemy forts that can be captured, we return 4.
**Example 2:**
**Input:** forts = \[0,0,1,-1\]
**Output:** 0
**Explanation:** Since no enemy fort can be captured, 0 is returned.
**Constraints:**
* `1 <= forts.length <= 1000`
* `-1 <= forts[i] <= 1` | null |
Python 3 || 2-6 lines, w/ explanation and example || T/M: 29 ms / 13.8 MB | maximum-enemy-forts-that-can-be-captured | 0 | 1 | Here\'s the plan:\n- First, we build a list of tuples with `itertools.groupby`. First element of each pair is the integer of the group(-1,0,or 1), and the second element is the length of the string.\n1. Second, we iterate through this list checking for whether `{grp[i-1][0], grp[i+1][0]} == {-1,1}`. If so, then `grp[i][0]==0`, and `grp[i][1]` must be a potential answer.\n- Third we check whether the `grp[i-1][0]` and `grp[i+1][0]` are 1 and -1 by whether their product is -1.\n- Fourth, we return the maximum.\n```\nclass Solution:\n def captureForts(self, forts: List[int]) -> int:\n\n grp = [(k,len(list(g))) for k,g in groupby(forts)]\n\n ans = 0 # Example: forts = [ 1, 0,0, -1, 0,0,0,0, 1]\n # | | | | |\n for i in range(1,len(grp)-1): # grp = [(1,1), (0,*2*),(-1,1),(0,*4*), (1,1)] \n if grp[i-1][0]*grp[i+1][0]==-1: # |______________||_____________|| \n ans = max(ans,grp[i][1]) # ans = max(2, 4)\n\n return ans # return 4\n```\n[https://leetcode.com/problems/maximum-enemy-forts-that-can-be-captured/submissions/864957033/](http://)\n\nI could be wrong, but I think that time is *O*(*N*) and space is *O*(*N*).\n\nBelow is the two-liner. I couldn\'t quite pull of a one-liner, maybe someone else? I promise an upvote :).\n\n```\nclass Solution:\n def captureForts(self, forts: List[int]) -> int:\n\n grp = [(k,len(list(g))) for k,g in groupby(forts)]\n return max([grp[i][1] for i in range(1,len(grp)-1) \n if grp[i-1][0]*grp[i+1][0]==-1], default = 0)\n```\n | 4 | You are given a **0-indexed** integer array `forts` of length `n` representing the positions of several forts. `forts[i]` can be `-1`, `0`, or `1` where:
* `-1` represents there is **no fort** at the `ith` position.
* `0` indicates there is an **enemy** fort at the `ith` position.
* `1` indicates the fort at the `ith` the position is under your command.
Now you have decided to move your army from one of your forts at position `i` to an empty position `j` such that:
* `0 <= i, j <= n - 1`
* The army travels over enemy forts **only**. Formally, for all `k` where `min(i,j) < k < max(i,j)`, `forts[k] == 0.`
While moving the army, all the enemy forts that come in the way are **captured**.
Return _the **maximum** number of enemy forts that can be captured_. In case it is **impossible** to move your army, or you do not have any fort under your command, return `0`_._
**Example 1:**
**Input:** forts = \[1,0,0,-1,0,0,0,0,1\]
**Output:** 4
**Explanation:**
- Moving the army from position 0 to position 3 captures 2 enemy forts, at 1 and 2.
- Moving the army from position 8 to position 3 captures 4 enemy forts.
Since 4 is the maximum number of enemy forts that can be captured, we return 4.
**Example 2:**
**Input:** forts = \[0,0,1,-1\]
**Output:** 0
**Explanation:** Since no enemy fort can be captured, 0 is returned.
**Constraints:**
* `1 <= forts.length <= 1000`
* `-1 <= forts[i] <= 1` | null |
Easy Python Solution | reward-top-k-students | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nCreate an hashmap to store the point of each student.\n\n# Code\n```\nclass Solution:\n def topStudents(self, positive_feedback: List[str], negative_feedback: List[str], report: List[str], student_id: List[int], k: int) -> List[int]:\n ans=collections.defaultdict(int)\n positive_feedback_d=defaultdict(int)\n negative_feedback_d=defaultdict(int)\n for i in positive_feedback:\n positive_feedback_d[i]+=1\n for i in negative_feedback:\n negative_feedback_d[i]+=1\n for i in range(len(report)):\n for j in report[i].split(" "):\n if positive_feedback_d[j]>=1:\n ans[student_id[i]]+=3\n elif negative_feedback_d[j]>=1:\n ans[student_id[i]]-=1\n else:\n continue\n temp=[]\n sorted_d = (sorted(ans, key=lambda x: (-ans[x], x)))\n i=0\n for j in sorted_d:\n temp.append(j)\n i+=1\n if i>=k:\n break\n return(temp)\n \n \n \n``` | 3 | You are given two string arrays `positive_feedback` and `negative_feedback`, containing the words denoting positive and negative feedback, respectively. Note that **no** word is both positive and negative.
Initially every student has `0` points. Each positive word in a feedback report **increases** the points of a student by `3`, whereas each negative word **decreases** the points by `1`.
You are given `n` feedback reports, represented by a **0-indexed** string array `report` and a **0-indexed** integer array `student_id`, where `student_id[i]` represents the ID of the student who has received the feedback report `report[i]`. The ID of each student is **unique**.
Given an integer `k`, return _the top_ `k` _students after ranking them in **non-increasing** order by their points_. In case more than one student has the same points, the one with the lower ID ranks higher.
**Example 1:**
**Input:** positive\_feedback = \[ "smart ", "brilliant ", "studious "\], negative\_feedback = \[ "not "\], report = \[ "this student is studious ", "the student is smart "\], student\_id = \[1,2\], k = 2
**Output:** \[1,2\]
**Explanation:**
Both the students have 1 positive feedback and 3 points but since student 1 has a lower ID he ranks higher.
**Example 2:**
**Input:** positive\_feedback = \[ "smart ", "brilliant ", "studious "\], negative\_feedback = \[ "not "\], report = \[ "this student is not studious ", "the student is smart "\], student\_id = \[1,2\], k = 2
**Output:** \[2,1\]
**Explanation:**
- The student with ID 1 has 1 positive feedback and 1 negative feedback, so he has 3-1=2 points.
- The student with ID 2 has 1 positive feedback, so he has 3 points.
Since student 2 has more points, \[2,1\] is returned.
**Constraints:**
* `1 <= positive_feedback.length, negative_feedback.length <= 104`
* `1 <= positive_feedback[i].length, negative_feedback[j].length <= 100`
* Both `positive_feedback[i]` and `negative_feedback[j]` consists of lowercase English letters.
* No word is present in both `positive_feedback` and `negative_feedback`.
* `n == report.length == student_id.length`
* `1 <= n <= 104`
* `report[i]` consists of lowercase English letters and spaces `' '`.
* There is a single space between consecutive words of `report[i]`.
* `1 <= report[i].length <= 100`
* `1 <= student_id[i] <= 109`
* All the values of `student_id[i]` are **unique**.
* `1 <= k <= n` | null |
Python 3 || 4 lines, nsmallest || T/M: 82% / 70% | reward-top-k-students | 0 | 1 | ```\nclass Solution:\n def topStudents(self, positive_feedback: List[str], negative_feedback: List[str], report: List[str], student_id: List[int], k: int) -> List[int]:\n\n d = [0]*len(student_id)\n\n pSet,nSet = set(positive_feedback), set(negative_feedback)\n\n for i,s in enumerate(report):\n for w in s.split(): d[i]+= 3*(w in pSet)-(w in nSet)\n\n return [id for _i, id in \n nsmallest(k, enumerate(student_id), \n key = lambda x: (-d[x[0]],x[1]))]\n```\n[https://leetcode.com/problems/reward-top-k-students/submissions/868361920/](http://)\n\n\n\nI could be wrong, but I think that time is *O*(*N*) and space is *O*(*N*). | 4 | You are given two string arrays `positive_feedback` and `negative_feedback`, containing the words denoting positive and negative feedback, respectively. Note that **no** word is both positive and negative.
Initially every student has `0` points. Each positive word in a feedback report **increases** the points of a student by `3`, whereas each negative word **decreases** the points by `1`.
You are given `n` feedback reports, represented by a **0-indexed** string array `report` and a **0-indexed** integer array `student_id`, where `student_id[i]` represents the ID of the student who has received the feedback report `report[i]`. The ID of each student is **unique**.
Given an integer `k`, return _the top_ `k` _students after ranking them in **non-increasing** order by their points_. In case more than one student has the same points, the one with the lower ID ranks higher.
**Example 1:**
**Input:** positive\_feedback = \[ "smart ", "brilliant ", "studious "\], negative\_feedback = \[ "not "\], report = \[ "this student is studious ", "the student is smart "\], student\_id = \[1,2\], k = 2
**Output:** \[1,2\]
**Explanation:**
Both the students have 1 positive feedback and 3 points but since student 1 has a lower ID he ranks higher.
**Example 2:**
**Input:** positive\_feedback = \[ "smart ", "brilliant ", "studious "\], negative\_feedback = \[ "not "\], report = \[ "this student is not studious ", "the student is smart "\], student\_id = \[1,2\], k = 2
**Output:** \[2,1\]
**Explanation:**
- The student with ID 1 has 1 positive feedback and 1 negative feedback, so he has 3-1=2 points.
- The student with ID 2 has 1 positive feedback, so he has 3 points.
Since student 2 has more points, \[2,1\] is returned.
**Constraints:**
* `1 <= positive_feedback.length, negative_feedback.length <= 104`
* `1 <= positive_feedback[i].length, negative_feedback[j].length <= 100`
* Both `positive_feedback[i]` and `negative_feedback[j]` consists of lowercase English letters.
* No word is present in both `positive_feedback` and `negative_feedback`.
* `n == report.length == student_id.length`
* `1 <= n <= 104`
* `report[i]` consists of lowercase English letters and spaces `' '`.
* There is a single space between consecutive words of `report[i]`.
* `1 <= report[i].length <= 100`
* `1 <= student_id[i] <= 109`
* All the values of `student_id[i]` are **unique**.
* `1 <= k <= n` | null |
✅ [Python] code with proper comments and approach explained. | reward-top-k-students | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\n1. Convert the both positive and negative feedback to a set for faster accessing i.e. in O(1) time.\n2. Then iterating in every string of the report list and calculating the points and store the points in the dictionary.\n3. Sort the dictionary in decreasing order of points but also in the increasing order of student_id\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(nlogn)$$\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$ for using dictionary\n\n# Code\n```\nclass Solution:\n def topStudents(self, positive_feedback: List[str], negative_feedback: List[str], report: List[str], student_id: List[int], k: int) -> List[int]:\n # converting the both positive_feedback and negative_feedback for fast accessing.\n pos= set(positive_feedback)\n neg= set(negative_feedback)\n d={} #dictionary to store every student points \n\n\n for i in range(len(report)):\n pts=0\n lst=report[i].split()\n for j in lst:\n if j in pos: # if positive feedback\n pts+=3\n if j in neg: # if negative feedback\n pts-=1\n d[student_id[i]]=pts #storing the final points\n \n #sorting the dictionary accoring to max points\n final_lst=list(sorted(d.items(),key=lambda x : (x[-1],-x[0]),reverse=True)) \n ans=[] #to store final answer\n for i in range(k):\n ans.append(final_lst[i][0])\n return ans\n \n``` | 4 | You are given two string arrays `positive_feedback` and `negative_feedback`, containing the words denoting positive and negative feedback, respectively. Note that **no** word is both positive and negative.
Initially every student has `0` points. Each positive word in a feedback report **increases** the points of a student by `3`, whereas each negative word **decreases** the points by `1`.
You are given `n` feedback reports, represented by a **0-indexed** string array `report` and a **0-indexed** integer array `student_id`, where `student_id[i]` represents the ID of the student who has received the feedback report `report[i]`. The ID of each student is **unique**.
Given an integer `k`, return _the top_ `k` _students after ranking them in **non-increasing** order by their points_. In case more than one student has the same points, the one with the lower ID ranks higher.
**Example 1:**
**Input:** positive\_feedback = \[ "smart ", "brilliant ", "studious "\], negative\_feedback = \[ "not "\], report = \[ "this student is studious ", "the student is smart "\], student\_id = \[1,2\], k = 2
**Output:** \[1,2\]
**Explanation:**
Both the students have 1 positive feedback and 3 points but since student 1 has a lower ID he ranks higher.
**Example 2:**
**Input:** positive\_feedback = \[ "smart ", "brilliant ", "studious "\], negative\_feedback = \[ "not "\], report = \[ "this student is not studious ", "the student is smart "\], student\_id = \[1,2\], k = 2
**Output:** \[2,1\]
**Explanation:**
- The student with ID 1 has 1 positive feedback and 1 negative feedback, so he has 3-1=2 points.
- The student with ID 2 has 1 positive feedback, so he has 3 points.
Since student 2 has more points, \[2,1\] is returned.
**Constraints:**
* `1 <= positive_feedback.length, negative_feedback.length <= 104`
* `1 <= positive_feedback[i].length, negative_feedback[j].length <= 100`
* Both `positive_feedback[i]` and `negative_feedback[j]` consists of lowercase English letters.
* No word is present in both `positive_feedback` and `negative_feedback`.
* `n == report.length == student_id.length`
* `1 <= n <= 104`
* `report[i]` consists of lowercase English letters and spaces `' '`.
* There is a single space between consecutive words of `report[i]`.
* `1 <= report[i].length <= 100`
* `1 <= student_id[i] <= 109`
* All the values of `student_id[i]` are **unique**.
* `1 <= k <= n` | null |
[Python 3] (Updated for new test cases) HashMap | Sorting | reward-top-k-students | 0 | 1 | ```\nclass Solution:\n def topStudents(self, positive_feedback: List[str], negative_feedback: List[str], report: List[str], stu_id: List[int], k: int) -> List[int]:\n hm = Counter()\n \n pos = set(positive_feedback)\n neg = set(negative_feedback)\n \n for rep in range(len(report)):\n temp = report[rep].split()\n for i in temp:\n if i in pos:\n hm[stu_id[rep]] += 3\n if i in neg:\n hm[stu_id[rep]] -= 1\n \n return sorted([i for i in stu_id], key = lambda x: (hm[x], -x), reverse = 1)[: k]\n``` | 1 | You are given two string arrays `positive_feedback` and `negative_feedback`, containing the words denoting positive and negative feedback, respectively. Note that **no** word is both positive and negative.
Initially every student has `0` points. Each positive word in a feedback report **increases** the points of a student by `3`, whereas each negative word **decreases** the points by `1`.
You are given `n` feedback reports, represented by a **0-indexed** string array `report` and a **0-indexed** integer array `student_id`, where `student_id[i]` represents the ID of the student who has received the feedback report `report[i]`. The ID of each student is **unique**.
Given an integer `k`, return _the top_ `k` _students after ranking them in **non-increasing** order by their points_. In case more than one student has the same points, the one with the lower ID ranks higher.
**Example 1:**
**Input:** positive\_feedback = \[ "smart ", "brilliant ", "studious "\], negative\_feedback = \[ "not "\], report = \[ "this student is studious ", "the student is smart "\], student\_id = \[1,2\], k = 2
**Output:** \[1,2\]
**Explanation:**
Both the students have 1 positive feedback and 3 points but since student 1 has a lower ID he ranks higher.
**Example 2:**
**Input:** positive\_feedback = \[ "smart ", "brilliant ", "studious "\], negative\_feedback = \[ "not "\], report = \[ "this student is not studious ", "the student is smart "\], student\_id = \[1,2\], k = 2
**Output:** \[2,1\]
**Explanation:**
- The student with ID 1 has 1 positive feedback and 1 negative feedback, so he has 3-1=2 points.
- The student with ID 2 has 1 positive feedback, so he has 3 points.
Since student 2 has more points, \[2,1\] is returned.
**Constraints:**
* `1 <= positive_feedback.length, negative_feedback.length <= 104`
* `1 <= positive_feedback[i].length, negative_feedback[j].length <= 100`
* Both `positive_feedback[i]` and `negative_feedback[j]` consists of lowercase English letters.
* No word is present in both `positive_feedback` and `negative_feedback`.
* `n == report.length == student_id.length`
* `1 <= n <= 104`
* `report[i]` consists of lowercase English letters and spaces `' '`.
* There is a single space between consecutive words of `report[i]`.
* `1 <= report[i].length <= 100`
* `1 <= student_id[i] <= 109`
* All the values of `student_id[i]` are **unique**.
* `1 <= k <= n` | null |
EASY SOLUTION USING MAX HEAP AND HASH SET!! | reward-top-k-students | 0 | 1 | # Intuition\nTo make the max heap according to the score of each student!!\n\n# Approach\n-> Fistly we will convert the both positive and negative array into hash set, so that we can find the result in O(1) time.\n\n-> Then we will start traversing the student_id array and for each student[i] we will go for report[i] which shows the report of i student.\n\n-> Then we will check for each string in report[i] whether the string is peresent in which set . If the string is present in postive set the its score will be increased by 3 and if that string is present in negative set then its score will bre decreased by 1.\n\n-> After traversing the whole report[i] then whatever the score is generated we will put this score into a max heap.\n\n-> After calculating the score for each student_id then we will want to find the top k score. For that we will remove k elements from heap and store it in result array and return the result array.\n\n\n# Complexity\n- Time complexity:\nO(K*B)\nk=lenght of student_id array.\nB=length of the report string of each student.\n\n- Space complexity:\nO(N)\n# If this explanations helps you , then don\'t forget to upvote !! Thanks ;-)\n# Code\n```\nfrom heapq import *\nclass Solution:\n def topStudents(self, positive: List[str], negative: List[str], report: List[str], student_id: List[int], k: int) -> List[int]:\n positive=set(positive)\n negative=set(negative)\n heap=[]\n for i in range(len(student_id)):\n c=0\n b="".join(report[i]).split(" ")\n for j in b:\n if j in positive:\n c+=3\n elif j in negative:\n c-=1\n heappush(heap,[-c,student_id[i]])\n res=[]\n while k:\n l=heappop(heap)\n res.append(l[1])\n k-=1\n return res\n\n``` | 1 | You are given two string arrays `positive_feedback` and `negative_feedback`, containing the words denoting positive and negative feedback, respectively. Note that **no** word is both positive and negative.
Initially every student has `0` points. Each positive word in a feedback report **increases** the points of a student by `3`, whereas each negative word **decreases** the points by `1`.
You are given `n` feedback reports, represented by a **0-indexed** string array `report` and a **0-indexed** integer array `student_id`, where `student_id[i]` represents the ID of the student who has received the feedback report `report[i]`. The ID of each student is **unique**.
Given an integer `k`, return _the top_ `k` _students after ranking them in **non-increasing** order by their points_. In case more than one student has the same points, the one with the lower ID ranks higher.
**Example 1:**
**Input:** positive\_feedback = \[ "smart ", "brilliant ", "studious "\], negative\_feedback = \[ "not "\], report = \[ "this student is studious ", "the student is smart "\], student\_id = \[1,2\], k = 2
**Output:** \[1,2\]
**Explanation:**
Both the students have 1 positive feedback and 3 points but since student 1 has a lower ID he ranks higher.
**Example 2:**
**Input:** positive\_feedback = \[ "smart ", "brilliant ", "studious "\], negative\_feedback = \[ "not "\], report = \[ "this student is not studious ", "the student is smart "\], student\_id = \[1,2\], k = 2
**Output:** \[2,1\]
**Explanation:**
- The student with ID 1 has 1 positive feedback and 1 negative feedback, so he has 3-1=2 points.
- The student with ID 2 has 1 positive feedback, so he has 3 points.
Since student 2 has more points, \[2,1\] is returned.
**Constraints:**
* `1 <= positive_feedback.length, negative_feedback.length <= 104`
* `1 <= positive_feedback[i].length, negative_feedback[j].length <= 100`
* Both `positive_feedback[i]` and `negative_feedback[j]` consists of lowercase English letters.
* No word is present in both `positive_feedback` and `negative_feedback`.
* `n == report.length == student_id.length`
* `1 <= n <= 104`
* `report[i]` consists of lowercase English letters and spaces `' '`.
* There is a single space between consecutive words of `report[i]`.
* `1 <= report[i].length <= 100`
* `1 <= student_id[i] <= 109`
* All the values of `student_id[i]` are **unique**.
* `1 <= k <= n` | null |
Tedious | reward-top-k-students | 0 | 1 | Just bunch of data manipulation. Looks a bit better in Python.\n\n**Python 3**\n```python\nclass Solution:\n def topStudents(self, pos_feed: List[str], neg_feed: List[str], report: List[str], student_id: List[int], k: int) -> List[int]:\n pos, neg, score_id = set(pos_feed), set(neg_feed), []\n for r, id in zip(report, student_id):\n score = sum(3 if w in pos else -1 if w in neg else 0 for w in r.split(" "))\n score_id.append((-score, id))\n return [id for _, id in sorted(score_id)[0 : k]]\n```\n**C++**\n```cpp\nvector<int> topStudents(vector<string>& pos_feed, vector<string>& neg_feed, vector<string>& report, vector<int>& student_id, int k) {\n unordered_set<string> pos(begin(pos_feed), end(pos_feed)), neg(begin(neg_feed), end(neg_feed));\n vector<pair<int, int>> sid;\n vector<int> res;\n for (int i = 0; i < report.size(); ++i) {\n int score = 0;\n for (int j = 0, k = 0; j <= report[i].size(); ++j)\n if (j == report[i].size() || report[i][j] == \' \') {\n score += pos.count(report[i].substr(k, j - k)) ? 3 : \n neg.count(report[i].substr(k, j - k)) ? -1 : 0;\n k = j + 1;\n }\n sid.push_back({-score, student_id[i]});\n }\n partial_sort(begin(sid), begin(sid) + k, end(sid));\n transform(begin(sid), begin(sid) + k, back_inserter(res), [](const auto &p){ return p.second; });\n return res;\n}\n``` | 16 | You are given two string arrays `positive_feedback` and `negative_feedback`, containing the words denoting positive and negative feedback, respectively. Note that **no** word is both positive and negative.
Initially every student has `0` points. Each positive word in a feedback report **increases** the points of a student by `3`, whereas each negative word **decreases** the points by `1`.
You are given `n` feedback reports, represented by a **0-indexed** string array `report` and a **0-indexed** integer array `student_id`, where `student_id[i]` represents the ID of the student who has received the feedback report `report[i]`. The ID of each student is **unique**.
Given an integer `k`, return _the top_ `k` _students after ranking them in **non-increasing** order by their points_. In case more than one student has the same points, the one with the lower ID ranks higher.
**Example 1:**
**Input:** positive\_feedback = \[ "smart ", "brilliant ", "studious "\], negative\_feedback = \[ "not "\], report = \[ "this student is studious ", "the student is smart "\], student\_id = \[1,2\], k = 2
**Output:** \[1,2\]
**Explanation:**
Both the students have 1 positive feedback and 3 points but since student 1 has a lower ID he ranks higher.
**Example 2:**
**Input:** positive\_feedback = \[ "smart ", "brilliant ", "studious "\], negative\_feedback = \[ "not "\], report = \[ "this student is not studious ", "the student is smart "\], student\_id = \[1,2\], k = 2
**Output:** \[2,1\]
**Explanation:**
- The student with ID 1 has 1 positive feedback and 1 negative feedback, so he has 3-1=2 points.
- The student with ID 2 has 1 positive feedback, so he has 3 points.
Since student 2 has more points, \[2,1\] is returned.
**Constraints:**
* `1 <= positive_feedback.length, negative_feedback.length <= 104`
* `1 <= positive_feedback[i].length, negative_feedback[j].length <= 100`
* Both `positive_feedback[i]` and `negative_feedback[j]` consists of lowercase English letters.
* No word is present in both `positive_feedback` and `negative_feedback`.
* `n == report.length == student_id.length`
* `1 <= n <= 104`
* `report[i]` consists of lowercase English letters and spaces `' '`.
* There is a single space between consecutive words of `report[i]`.
* `1 <= report[i].length <= 100`
* `1 <= student_id[i] <= 109`
* All the values of `student_id[i]` are **unique**.
* `1 <= k <= n` | null |
Python | O(logn) Runtime | With explanation | minimize-the-maximum-of-two-arrays | 0 | 1 | # Intuition\n1st Insight: Seeing that uniqueCnt1 and uniqueCnt2 are order of $$10^9$$ and our answer is also potentially of that magnitude, we might think to consider a logarithmic solution such as binary search.\n\n2nd Insight: It isn\'t obvious how to find the minimum maximum value across all arrays satisfying the condition or even construct the arrays. One question we might ask is can we determine if a maximum value $$V$$ is possible? Yes we can! \n\n*Also another reason to consider this approach is if some value $$V$$ is possible, then it is possible to construct such arrays for any value greater than $$V$$ e.g. using the same array constructed for $$V$$.\n\nLet $$k$$ be the number of elements that don\'t divide $$divisor1$$. If $$k < uniqueCnt1$$ then $$V$$ isn\'t possible as there aren\'t enough numbers from $$1$$ to $$V$$ that don\'t divide $$divisor1$$. We can make the same argument for $$divisor2$$ and $$uniqueCnt2$$.\n\nNow there is one more condition that is neccessary for $$V$$ to possible. Let $$k$$ be the number of elements that are not divisible by at least 1 of $$divisor1$$ and $$divisor2$$. We must have that $$k >= uniqueCnt1 + uniqueCnt2$$.\n\n$$V$$ is valid if and only if these three properties are satisfied.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThus we solve this problem using binary search. We can let our right pointer be $$10^{10}$$ which is sufficiently high and our left be 1. Inside the while loop we perform the checks as described above. To do the third check notice that the number of elements from $$1$$ to $$V$$ that dont divide at least one of $$divisor1$$ and $$divisor2$$ is equal to $$V$$ minus the number of elements that divide both $$divisor1$$ and $$divisor2$$. This is just \n$$V - V/lcm(divisor1,divisor2)$$. We use this binary search to find the lowest possible value of V.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(log(uniqueCnt1+uniqueCnt2))$$\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n\n```\nimport math\nclass Solution:\n def minimizeSet(self, divisor1: int, divisor2: int, uniqueCnt1: int, uniqueCnt2: int) -> int:\n \n def gcd(a, b):\n if (a == 0):\n return b\n\n if (b == 0):\n return a\n\n if (a == b):\n return a\n\n if (a > b):\n return gcd(a-b, b)\n return gcd(a, b-a)\n \n def lcm(a,b):\n return a*b//gcd(a,b)\n \n d1=divisor1\n d2=divisor2\n u1=uniqueCnt1\n u2=uniqueCnt2\n \n l=1\n r=10**(10)\n #r=7\n res=float(\'inf\')\n while l<=r:\n m=(l+r)//2\n x=m-m//d1\n y=m-m//d2\n z=m-m//(lcm(d1,d2))\n \n if x<u1 or y<u2 or z<u1+u2:\n l=m+1\n continue\n else:\n res=min(res,m)\n r=m-1\n \n \n \n \n return res\n``` | 1 | We have two arrays `arr1` and `arr2` which are initially empty. You need to add positive integers to them such that they satisfy all the following conditions:
* `arr1` contains `uniqueCnt1` **distinct** positive integers, each of which is **not divisible** by `divisor1`.
* `arr2` contains `uniqueCnt2` **distinct** positive integers, each of which is **not divisible** by `divisor2`.
* **No** integer is present in both `arr1` and `arr2`.
Given `divisor1`, `divisor2`, `uniqueCnt1`, and `uniqueCnt2`, return _the **minimum possible maximum** integer that can be present in either array_.
**Example 1:**
**Input:** divisor1 = 2, divisor2 = 7, uniqueCnt1 = 1, uniqueCnt2 = 3
**Output:** 4
**Explanation:**
We can distribute the first 4 natural numbers into arr1 and arr2.
arr1 = \[1\] and arr2 = \[2,3,4\].
We can see that both arrays satisfy all the conditions.
Since the maximum value is 4, we return it.
**Example 2:**
**Input:** divisor1 = 3, divisor2 = 5, uniqueCnt1 = 2, uniqueCnt2 = 1
**Output:** 3
**Explanation:**
Here arr1 = \[1,2\], and arr2 = \[3\] satisfy all conditions.
Since the maximum value is 3, we return it.
**Example 3:**
**Input:** divisor1 = 2, divisor2 = 4, uniqueCnt1 = 8, uniqueCnt2 = 2
**Output:** 15
**Explanation:**
Here, the final possible arrays can be arr1 = \[1,3,5,7,9,11,13,15\], and arr2 = \[2,6\].
It can be shown that it is not possible to obtain a lower maximum satisfying all conditions.
**Constraints:**
* `2 <= divisor1, divisor2 <= 105`
* `1 <= uniqueCnt1, uniqueCnt2 < 109`
* `2 <= uniqueCnt1 + uniqueCnt2 <= 109` | null |
Python 3 || 7 lines, iteration, w/ explanation & example || T/M: 99.3% / 100% | minimize-the-maximum-of-two-arrays | 0 | 1 | Here\'s the plan:\nSuppose, for example: \n`divisor1 = 2, uniqueCnt1 = 11`, and `divisor2 = 3, uniqueCnt2 = 2`\n\nWe need at least 13 elements in total in the two groups. Four elements would be eligible for either\ngroup (`[1,5,7,11]`), two for Group1 only (`[3,9]`), four for Group2 only (`[2,4,8,10]`), and two for neither group [6,12].\nThe diagram below summarizes:\n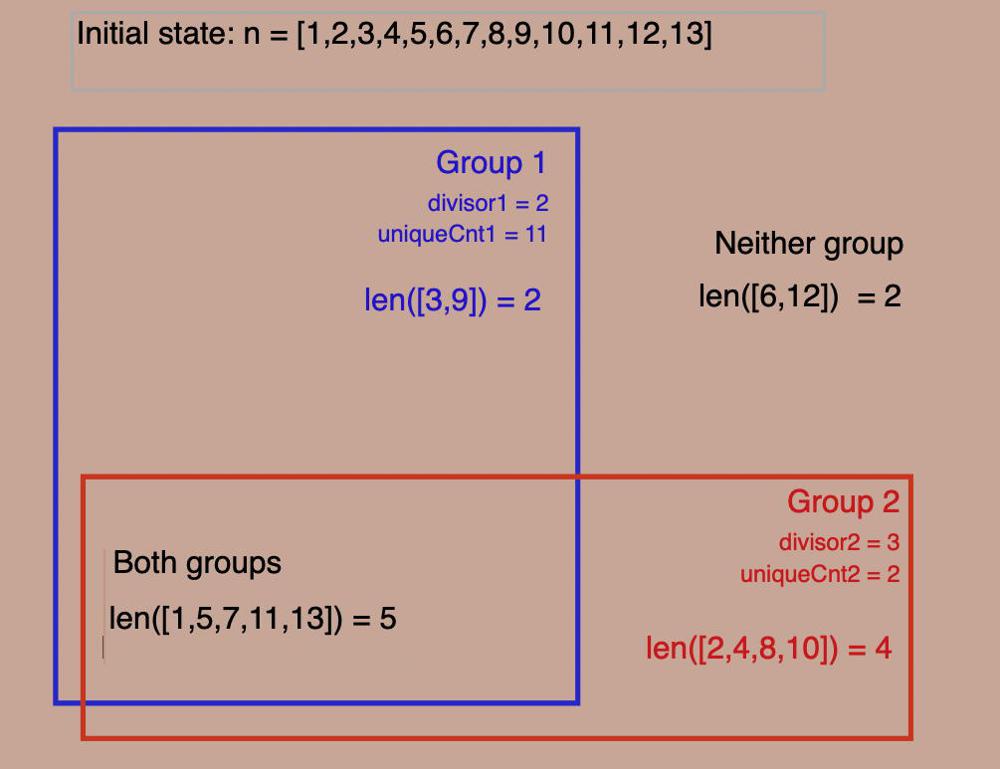\n\n\n\nClearly n needs to be increased by at least 4 (`n = 13+4 = 17`) in order for Group 1 to have 11 elements. We add 4 to n (so now n = 17), and determine the groups again:\n```\nGroup1 : `len([3,9, 15]) = 3`\nGroup2 : `len([2,4,8,10, 14,16]) = 6`\nBoth : `len([1,5,7,11,13, 17]) = 6`\nNeither: `len([6,12]) = 2\n```\nWe continue to iterate in this fashion, ending up with `n = 21`.\n```\nGroup1 : `len([3,9, 15, 21]) = 4` \u2013\nGroup2 : `len([2,4,8,10, 14,16 20 ]) = 7` 4 + 7 = 11\nBoth : `len([1,5,7,11,13, 17, 19 ]) = 7` \u2013\nNeither: `len([6,12, 18 ]) = 3```\n\n```\n```\nclass Solution:\n def minimizeSet(self, d1: int, d2: int, ct1: int, ct2: int) -> int:\n \n f = lambda x: (x+abs(x))//2 \n\n n, prev, d = ct1 + ct2, 0, lcm(d1,d2)\n\n while n > prev: # keep going until we do not \n # have to add more\n prev = n \n\n l1, l2 = n//d2 - n//d, n//d1 - n//d # l1, l2 : length of group1, group2\n\n n+= f(f(ct1 - l1) + f(ct2 - l2)- n + n//d + l2 + l1) # determine the incease in n\n\n return n\n```\n[https://leetcode.com/problems/minimize-the-maximum-of-two-arrays/submissions/866527037/](http://)\n\nI could be wrong, but I think that time is *O*(*N*) and space is *O*(*N*). | 3 | We have two arrays `arr1` and `arr2` which are initially empty. You need to add positive integers to them such that they satisfy all the following conditions:
* `arr1` contains `uniqueCnt1` **distinct** positive integers, each of which is **not divisible** by `divisor1`.
* `arr2` contains `uniqueCnt2` **distinct** positive integers, each of which is **not divisible** by `divisor2`.
* **No** integer is present in both `arr1` and `arr2`.
Given `divisor1`, `divisor2`, `uniqueCnt1`, and `uniqueCnt2`, return _the **minimum possible maximum** integer that can be present in either array_.
**Example 1:**
**Input:** divisor1 = 2, divisor2 = 7, uniqueCnt1 = 1, uniqueCnt2 = 3
**Output:** 4
**Explanation:**
We can distribute the first 4 natural numbers into arr1 and arr2.
arr1 = \[1\] and arr2 = \[2,3,4\].
We can see that both arrays satisfy all the conditions.
Since the maximum value is 4, we return it.
**Example 2:**
**Input:** divisor1 = 3, divisor2 = 5, uniqueCnt1 = 2, uniqueCnt2 = 1
**Output:** 3
**Explanation:**
Here arr1 = \[1,2\], and arr2 = \[3\] satisfy all conditions.
Since the maximum value is 3, we return it.
**Example 3:**
**Input:** divisor1 = 2, divisor2 = 4, uniqueCnt1 = 8, uniqueCnt2 = 2
**Output:** 15
**Explanation:**
Here, the final possible arrays can be arr1 = \[1,3,5,7,9,11,13,15\], and arr2 = \[2,6\].
It can be shown that it is not possible to obtain a lower maximum satisfying all conditions.
**Constraints:**
* `2 <= divisor1, divisor2 <= 105`
* `1 <= uniqueCnt1, uniqueCnt2 < 109`
* `2 <= uniqueCnt1 + uniqueCnt2 <= 109` | null |
python 3 Solution 7-lines,O(N),O(N) | minimize-the-maximum-of-two-arrays | 0 | 1 | \n```\nclass Solution:\n def minimizeSet(self, divisor1: int, divisor2: int, uniqueCnt1: int, uniqueCnt2: int) -> int:\n f=lambda x:(x+abs(x))//2\n n,prev,d=uniqueCnt1+uniqueCnt2,0,lcm(divisor1,divisor2)\n while n>prev:\n prev=n\n l1,l2=n//divisor2-n//d,n//divisor1-n//d\n n+=f(f(uniqueCnt1-l1)+f(uniqueCnt2-l2)-n+n//d+l2+l1)\n\n return n \n``` | 1 | We have two arrays `arr1` and `arr2` which are initially empty. You need to add positive integers to them such that they satisfy all the following conditions:
* `arr1` contains `uniqueCnt1` **distinct** positive integers, each of which is **not divisible** by `divisor1`.
* `arr2` contains `uniqueCnt2` **distinct** positive integers, each of which is **not divisible** by `divisor2`.
* **No** integer is present in both `arr1` and `arr2`.
Given `divisor1`, `divisor2`, `uniqueCnt1`, and `uniqueCnt2`, return _the **minimum possible maximum** integer that can be present in either array_.
**Example 1:**
**Input:** divisor1 = 2, divisor2 = 7, uniqueCnt1 = 1, uniqueCnt2 = 3
**Output:** 4
**Explanation:**
We can distribute the first 4 natural numbers into arr1 and arr2.
arr1 = \[1\] and arr2 = \[2,3,4\].
We can see that both arrays satisfy all the conditions.
Since the maximum value is 4, we return it.
**Example 2:**
**Input:** divisor1 = 3, divisor2 = 5, uniqueCnt1 = 2, uniqueCnt2 = 1
**Output:** 3
**Explanation:**
Here arr1 = \[1,2\], and arr2 = \[3\] satisfy all conditions.
Since the maximum value is 3, we return it.
**Example 3:**
**Input:** divisor1 = 2, divisor2 = 4, uniqueCnt1 = 8, uniqueCnt2 = 2
**Output:** 15
**Explanation:**
Here, the final possible arrays can be arr1 = \[1,3,5,7,9,11,13,15\], and arr2 = \[2,6\].
It can be shown that it is not possible to obtain a lower maximum satisfying all conditions.
**Constraints:**
* `2 <= divisor1, divisor2 <= 105`
* `1 <= uniqueCnt1, uniqueCnt2 < 109`
* `2 <= uniqueCnt1 + uniqueCnt2 <= 109` | null |
[C++|Java|Python3] binary search | minimize-the-maximum-of-two-arrays | 1 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/3ffc910c12ff8c84890fb15351216a0fa85dc3ac) for solutions of biweekly 94. \n\n**Intuition**\nHere, we can use binary search to look for the tightest upper bound. Given a value `x`, the condition for `x` to be a valid upper bound is that \n1) there is enough number between 1 to `x` that are not divisible by `divisor1`; \n2) there is enough number between 1 to `x` that are not divisible by `divisor2`; \n3) in the overlapping area, if some numbers are allocated to cover `uniqueCnt1` they cannot be used to cover `uniqueCnt2`. \n\n\n**C++**\n```\nclass Solution {\npublic: \n\tint minimizeSet(int divisor1, int divisor2, int uniqueCnt1, int uniqueCnt2) {\n\t\tlong lo = 0, hi = INT_MAX, mult = lcm((long) divisor1, divisor2); \n\t\twhile (lo < hi) {\n\t\t\tint mid = lo + (hi-lo)/2; \n\t\t\tif (uniqueCnt1 <= mid-mid/divisor1 && uniqueCnt2 <= mid-mid/divisor2 && uniqueCnt1+uniqueCnt2 <= mid-mid/mult) hi = mid; \n\t\t\telse lo = mid+1; \n\t\t}\n\t\treturn lo; \n\t}\n};\n```\n**Java**\n```\nclass Solution {\n\tpublic int minimizeSet(int divisor1, int divisor2, int uniqueCnt1, int uniqueCnt2) {\n\t\tint g = divisor1; \n\t\tfor (int x = divisor2; x > 0; ) {int tmp = g; g = x; x = tmp % x; }\n\t\tlong lo = 0, hi = Integer.MAX_VALUE, mult = ((long) divisor1*divisor2/g); \n\t\twhile (lo < hi) {\n\t\t\tlong mid = lo + (hi-lo)/2;\n\t\t\tif (uniqueCnt1 <= mid-mid/divisor1 && uniqueCnt2 <= mid-mid/divisor2 && uniqueCnt1+uniqueCnt2 <= mid-mid/mult) hi = mid; \n\t\t\telse lo = mid+1; \n\t\t}\n\t\treturn (int) lo; \n\t}\n}\n```\n**Python3**\n```\nclass Solution: \n\tdef minimizeSet(self, divisor1: int, divisor2: int, uniqueCnt1: int, uniqueCnt2: int) -> int: \n\t\tlo, hi = 0, 1<<32-1\n\t\tmult = lcm(divisor1, divisor2)\n\t\twhile lo < hi: \n\t\t\tmid = lo + hi >> 1\n\t\t\tif uniqueCnt1 <= mid - mid//divisor1 and uniqueCnt2 <= mid - mid//divisor2 and uniqueCnt1+uniqueCnt2 <= mid - mid//mult: hi = mid\n\t\t\telse: lo = mid+1\n\t\treturn lo \n``` | 19 | We have two arrays `arr1` and `arr2` which are initially empty. You need to add positive integers to them such that they satisfy all the following conditions:
* `arr1` contains `uniqueCnt1` **distinct** positive integers, each of which is **not divisible** by `divisor1`.
* `arr2` contains `uniqueCnt2` **distinct** positive integers, each of which is **not divisible** by `divisor2`.
* **No** integer is present in both `arr1` and `arr2`.
Given `divisor1`, `divisor2`, `uniqueCnt1`, and `uniqueCnt2`, return _the **minimum possible maximum** integer that can be present in either array_.
**Example 1:**
**Input:** divisor1 = 2, divisor2 = 7, uniqueCnt1 = 1, uniqueCnt2 = 3
**Output:** 4
**Explanation:**
We can distribute the first 4 natural numbers into arr1 and arr2.
arr1 = \[1\] and arr2 = \[2,3,4\].
We can see that both arrays satisfy all the conditions.
Since the maximum value is 4, we return it.
**Example 2:**
**Input:** divisor1 = 3, divisor2 = 5, uniqueCnt1 = 2, uniqueCnt2 = 1
**Output:** 3
**Explanation:**
Here arr1 = \[1,2\], and arr2 = \[3\] satisfy all conditions.
Since the maximum value is 3, we return it.
**Example 3:**
**Input:** divisor1 = 2, divisor2 = 4, uniqueCnt1 = 8, uniqueCnt2 = 2
**Output:** 15
**Explanation:**
Here, the final possible arrays can be arr1 = \[1,3,5,7,9,11,13,15\], and arr2 = \[2,6\].
It can be shown that it is not possible to obtain a lower maximum satisfying all conditions.
**Constraints:**
* `2 <= divisor1, divisor2 <= 105`
* `1 <= uniqueCnt1, uniqueCnt2 < 109`
* `2 <= uniqueCnt1 + uniqueCnt2 <= 109` | null |
NO Binary Search Solution [TOP 100% runtime&memory] | minimize-the-maximum-of-two-arrays | 0 | 1 | # Apporach\n<!-- Describe your approach to solving the problem. -->\nLet\'s $$f(u, d)$$ is the minimum possible maximum integer in an array of size $$u$$ that are not divisible by divisor $$d$$. There will be numbers from 1 to u, except that are divisible by $$d$$, and some numbers that are bigger than $$u$$.\n\nSo, we are missing all $$k \\cdot d $$ numbers from 1 to $$f(u, d)$$. How can we count the number of them? For each $$d - 1$$ numbers that exist in the array, we miss one $$k \\cdot d$$ number, where $$k \\in \\N$$. So the number of them equals $$floor(u / (d - 1))$$.\n\nBut we should not count the last $$k \\cdot d $$ number if $$u$$ is divisible by $$d - 1$$.\n\nSo, the final formula for $$f(u, d)$$:\n$$f(u, d) = u + floor((u - 1) / (d - 1))$$\n\nWe should calculate $$f(u, d)$$ for the first array, then for the second one, and if there are a lot of numbers from both arrays, we should calculate for both arrays: $$f(u1 + u2, LCM(d1, d2))$$.\n\nThe resulting answer will be the maximum of the three functions:\n$$max(f(u1, d1), f(u2, d2), f(u1 + u2, LCM(d1, d2)))$$\n\n\n# Complexity\n- Time complexity: $$O(log(divisor1+divisor2))$$, because it is the time complexity of LCM.\n\n- Space complexity: $$O(1)$$\n\n# Code\n```\ndef f(u, d):\n return u + (u - 1) // (d - 1)\n\n\nclass Solution:\n def minimizeSet(self, d1: int, d2: int, u1: int, u2: int) -> int:\n return max(f(u1, d1), f(u2, d2), f(u1 + u2, math.lcm(d1, d2)))\n\n``` | 14 | We have two arrays `arr1` and `arr2` which are initially empty. You need to add positive integers to them such that they satisfy all the following conditions:
* `arr1` contains `uniqueCnt1` **distinct** positive integers, each of which is **not divisible** by `divisor1`.
* `arr2` contains `uniqueCnt2` **distinct** positive integers, each of which is **not divisible** by `divisor2`.
* **No** integer is present in both `arr1` and `arr2`.
Given `divisor1`, `divisor2`, `uniqueCnt1`, and `uniqueCnt2`, return _the **minimum possible maximum** integer that can be present in either array_.
**Example 1:**
**Input:** divisor1 = 2, divisor2 = 7, uniqueCnt1 = 1, uniqueCnt2 = 3
**Output:** 4
**Explanation:**
We can distribute the first 4 natural numbers into arr1 and arr2.
arr1 = \[1\] and arr2 = \[2,3,4\].
We can see that both arrays satisfy all the conditions.
Since the maximum value is 4, we return it.
**Example 2:**
**Input:** divisor1 = 3, divisor2 = 5, uniqueCnt1 = 2, uniqueCnt2 = 1
**Output:** 3
**Explanation:**
Here arr1 = \[1,2\], and arr2 = \[3\] satisfy all conditions.
Since the maximum value is 3, we return it.
**Example 3:**
**Input:** divisor1 = 2, divisor2 = 4, uniqueCnt1 = 8, uniqueCnt2 = 2
**Output:** 15
**Explanation:**
Here, the final possible arrays can be arr1 = \[1,3,5,7,9,11,13,15\], and arr2 = \[2,6\].
It can be shown that it is not possible to obtain a lower maximum satisfying all conditions.
**Constraints:**
* `2 <= divisor1, divisor2 <= 105`
* `1 <= uniqueCnt1, uniqueCnt2 < 109`
* `2 <= uniqueCnt1 + uniqueCnt2 <= 109` | null |
Beginer Friendly | minimize-the-maximum-of-two-arrays | 0 | 1 | # Code\n```python []\nclass Solution:\n def minimizeSet(self, divisor1: int, divisor2: int, uniqueCnt1: int, uniqueCnt2: int) -> int:\n l, r = 0, 1 << 32 - 1\n\n lcm = math.lcm(divisor1, divisor2)\n def valid(num):\n div1 = num - num // divisor1\n if div1 < uniqueCnt1: \n # there isn\'t enough number between 1 to num that are not divisible by divisor1\n return False\n div2 = num - num // divisor2 \n if div2 < uniqueCnt2: \n # there isn\'t enough number between 1 to num that are not divisible by divisor2\n return False\n union = num - num // lcm\n # num // lcm: none wowrks, num: total, union: total - none works\n if union < (uniqueCnt1 + uniqueCnt2):\n # Numbers from the range [1,L] that are multiples of both C1 and C2 (thus, multiples of lcm(D1,D2)) should be skipped, thus, increasing the candidate value by 1.\n return False\n return True\n\n while l + 1 != r:\n m = (l + r) // 2\n if (valid(m)):\n r = m \n else:\n l = m\n return r\n \n```\n# Reference:\nhttps://leetcode.com/problems/minimize-the-maximum-of-two-arrays/solutions/2946508/python-lcm-and-binary-search-explained-bonus-one-liner/?orderBy=most_votes\nBisect Template:\nhttps://www.youtube.com/watch?v=JuDAqNyTG4g&ab_channel=%E4%BA%94%E7%82%B9%E4%B8%83%E8%BE%B9 | 6 | We have two arrays `arr1` and `arr2` which are initially empty. You need to add positive integers to them such that they satisfy all the following conditions:
* `arr1` contains `uniqueCnt1` **distinct** positive integers, each of which is **not divisible** by `divisor1`.
* `arr2` contains `uniqueCnt2` **distinct** positive integers, each of which is **not divisible** by `divisor2`.
* **No** integer is present in both `arr1` and `arr2`.
Given `divisor1`, `divisor2`, `uniqueCnt1`, and `uniqueCnt2`, return _the **minimum possible maximum** integer that can be present in either array_.
**Example 1:**
**Input:** divisor1 = 2, divisor2 = 7, uniqueCnt1 = 1, uniqueCnt2 = 3
**Output:** 4
**Explanation:**
We can distribute the first 4 natural numbers into arr1 and arr2.
arr1 = \[1\] and arr2 = \[2,3,4\].
We can see that both arrays satisfy all the conditions.
Since the maximum value is 4, we return it.
**Example 2:**
**Input:** divisor1 = 3, divisor2 = 5, uniqueCnt1 = 2, uniqueCnt2 = 1
**Output:** 3
**Explanation:**
Here arr1 = \[1,2\], and arr2 = \[3\] satisfy all conditions.
Since the maximum value is 3, we return it.
**Example 3:**
**Input:** divisor1 = 2, divisor2 = 4, uniqueCnt1 = 8, uniqueCnt2 = 2
**Output:** 15
**Explanation:**
Here, the final possible arrays can be arr1 = \[1,3,5,7,9,11,13,15\], and arr2 = \[2,6\].
It can be shown that it is not possible to obtain a lower maximum satisfying all conditions.
**Constraints:**
* `2 <= divisor1, divisor2 <= 105`
* `1 <= uniqueCnt1, uniqueCnt2 < 109`
* `2 <= uniqueCnt1 + uniqueCnt2 <= 109` | null |
Python3 | Binary search | minimize-the-maximum-of-two-arrays | 0 | 1 | # Python | Binary Search\nWe can think about `Given n, can we select some numbers from 1~n to have uniqueCnt1 numbers in arr1 and uniqueCnt2 in arr2?`\nWe can check by this way:\nFirst, we put in `arr1` the numbers that can fit in `arr1` but cannot fit in `arr2`, and put in `arr2` the numbers that can fit in `arr2` but cannot fit in `arr1`. \nWe define the numbers as `only1` and `only2` in the `valid` function. \nThen, if `uniqueCnt1` > `only1`, we should use the numbers that can both fit in `arr1` and `arr2`. So, we count the numbers that can both fit in `arr1` and `arr2` as the variable `both` in `valid` function.\nIf `max(uniqueCnt1-only1, 0) + max(uniqueCnt2-only2, 0) > both`, it means that we cannot fill `arr1` and `arr2` with the `1~n` limit.\nSo, we are start looking for the smallest `n` that can fulfill the case by binary search with `O(nlogn)` time complexity.\n# Code\n```python []\n\nclass Solution:\n def minimizeSet(self, divisor1: int, divisor2: int, uniqueCnt1: int, uniqueCnt2: int) -> int:\n uc1 = uniqueCnt1\n uc2 = uniqueCnt2\n d1 = divisor1\n d2 = divisor2\n def gcd(a, b):\n if a == 0:\n return b\n return gcd(b%a, a)\n def valid(n):\n only1 = n // d2 - n // lcm\n only2 = n // d1 - n // lcm\n both = n - n // d1 - n // d2 + n // lcm\n return max(0, uc1 - only1) + max(0, uc2 - only2) <= both\n \n gcd1 = gcd(d1, d2)\n lcm = d1*d2/gcd1\n return bisect_left(range(uniqueCnt1*d1+d2*uniqueCnt2+1), True, lo=1, key=valid)\n \n```\n | 3 | We have two arrays `arr1` and `arr2` which are initially empty. You need to add positive integers to them such that they satisfy all the following conditions:
* `arr1` contains `uniqueCnt1` **distinct** positive integers, each of which is **not divisible** by `divisor1`.
* `arr2` contains `uniqueCnt2` **distinct** positive integers, each of which is **not divisible** by `divisor2`.
* **No** integer is present in both `arr1` and `arr2`.
Given `divisor1`, `divisor2`, `uniqueCnt1`, and `uniqueCnt2`, return _the **minimum possible maximum** integer that can be present in either array_.
**Example 1:**
**Input:** divisor1 = 2, divisor2 = 7, uniqueCnt1 = 1, uniqueCnt2 = 3
**Output:** 4
**Explanation:**
We can distribute the first 4 natural numbers into arr1 and arr2.
arr1 = \[1\] and arr2 = \[2,3,4\].
We can see that both arrays satisfy all the conditions.
Since the maximum value is 4, we return it.
**Example 2:**
**Input:** divisor1 = 3, divisor2 = 5, uniqueCnt1 = 2, uniqueCnt2 = 1
**Output:** 3
**Explanation:**
Here arr1 = \[1,2\], and arr2 = \[3\] satisfy all conditions.
Since the maximum value is 3, we return it.
**Example 3:**
**Input:** divisor1 = 2, divisor2 = 4, uniqueCnt1 = 8, uniqueCnt2 = 2
**Output:** 15
**Explanation:**
Here, the final possible arrays can be arr1 = \[1,3,5,7,9,11,13,15\], and arr2 = \[2,6\].
It can be shown that it is not possible to obtain a lower maximum satisfying all conditions.
**Constraints:**
* `2 <= divisor1, divisor2 <= 105`
* `1 <= uniqueCnt1, uniqueCnt2 < 109`
* `2 <= uniqueCnt1 + uniqueCnt2 <= 109` | null |
A simple solution that is readable and has comments. | minimize-the-maximum-of-two-arrays | 0 | 1 | # Approach\nStep 1: Identify logic to determine if a solution is possible for some number "n".\nStep 2: Find "n". \n\n# Complexity\n- Time complexity: O(logN)\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimizeSet(self, d1: int, d2: int, c1: int, c2: int) -> int:\n LCM = lcm(d1, d2)\n def canFit(n, l):\n v1 = n//d1 #numbers divisible by d1 \n v2 = n//d2 #numbers divisible by d2\n v3 = n//l #numbers divisible by both d1 and d2\n \n guaranteedFor1 = v2-v3 #guaranteed allotment for d1\n guaranteedFor2 = v1-v3 #guaranteed allotment for d2\n\n x = max(c1-guaranteedFor1, 0) #number of elements required to complete c1\n y = max(c2-guaranteedFor2, 0) #number of elements required to complete c2\n\n # if required number of elements is less than or equal to available unalloted numbers, then its a possible solution\n if (x+y)<=(n-v1-v2+v3): return True\n return False\n\n l = 1\n r = 10**10\n\n while l<r:\n if l==r-1:\n if canFit(l, LCM): return l\n else: return r\n m = (l+r)//2\n if canFit(m, LCM): r = m\n else: l = m\n``` | 0 | We have two arrays `arr1` and `arr2` which are initially empty. You need to add positive integers to them such that they satisfy all the following conditions:
* `arr1` contains `uniqueCnt1` **distinct** positive integers, each of which is **not divisible** by `divisor1`.
* `arr2` contains `uniqueCnt2` **distinct** positive integers, each of which is **not divisible** by `divisor2`.
* **No** integer is present in both `arr1` and `arr2`.
Given `divisor1`, `divisor2`, `uniqueCnt1`, and `uniqueCnt2`, return _the **minimum possible maximum** integer that can be present in either array_.
**Example 1:**
**Input:** divisor1 = 2, divisor2 = 7, uniqueCnt1 = 1, uniqueCnt2 = 3
**Output:** 4
**Explanation:**
We can distribute the first 4 natural numbers into arr1 and arr2.
arr1 = \[1\] and arr2 = \[2,3,4\].
We can see that both arrays satisfy all the conditions.
Since the maximum value is 4, we return it.
**Example 2:**
**Input:** divisor1 = 3, divisor2 = 5, uniqueCnt1 = 2, uniqueCnt2 = 1
**Output:** 3
**Explanation:**
Here arr1 = \[1,2\], and arr2 = \[3\] satisfy all conditions.
Since the maximum value is 3, we return it.
**Example 3:**
**Input:** divisor1 = 2, divisor2 = 4, uniqueCnt1 = 8, uniqueCnt2 = 2
**Output:** 15
**Explanation:**
Here, the final possible arrays can be arr1 = \[1,3,5,7,9,11,13,15\], and arr2 = \[2,6\].
It can be shown that it is not possible to obtain a lower maximum satisfying all conditions.
**Constraints:**
* `2 <= divisor1, divisor2 <= 105`
* `1 <= uniqueCnt1, uniqueCnt2 < 109`
* `2 <= uniqueCnt1 + uniqueCnt2 <= 109` | null |
Binary search, counting with the inclusion/exclusion principle | minimize-the-maximum-of-two-arrays | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThere are 3 counts of interest that can be used to check if a given bound num provides enough space to cover the range while within the constraints. \n1. c1: how many are divisible by divisor1\n2. c2: how many are divisible by divisor2\n3. c3: how many are divisible by both\n\nMultiples of divisor2 can be used for cnt1 if not multiples of divisor1 and vice versa. This leads to reduced counts uc1 and uc2 that together have to be covered by numbers not multiples of either divisor1 or divisor2. The calculations use the inclusion/exclusion principle from set theory.\n\n\n# Approach\nBinary search for the smallest valid upper bound\n\n# Complexity\n- Time/space complexity logarithmic with respect to the search range\n\n\n# Code\n```\nclass Solution:\n def minimizeSet(self, divisor1: int, divisor2: int, uniqueCnt1: int, uniqueCnt2: int) -> int:\n # binary search for solution\n def enough(num):\n c1, c2 = num//divisor1, num//divisor2\n c3 = num//lcm(divisor1,divisor2)\n # div2 can be used for cnt1 if not multiple of div1\n uc1 = max(0,uniqueCnt1 - c2 + c3) # reduced uniqueCnt1\n # div1 can be used for cnt2 if not multiple of div2\n uc2 = max(0,uniqueCnt2 - c1 + c3) # reduced uniqueCnt1\n # numbers that are not multiples of div1, div2\n # can be used for both. \n return uc1 + uc2 <= num - c1 - c2 + c3\n\n left, right = uniqueCnt1+uniqueCnt2, pow(10,10)\n if enough(left): return left\n while left < right-1: # loop invariant ]left,right]\n mid = (left+right)//2\n if enough(mid): right = mid\n else: left = mid\n return right\n``` | 0 | We have two arrays `arr1` and `arr2` which are initially empty. You need to add positive integers to them such that they satisfy all the following conditions:
* `arr1` contains `uniqueCnt1` **distinct** positive integers, each of which is **not divisible** by `divisor1`.
* `arr2` contains `uniqueCnt2` **distinct** positive integers, each of which is **not divisible** by `divisor2`.
* **No** integer is present in both `arr1` and `arr2`.
Given `divisor1`, `divisor2`, `uniqueCnt1`, and `uniqueCnt2`, return _the **minimum possible maximum** integer that can be present in either array_.
**Example 1:**
**Input:** divisor1 = 2, divisor2 = 7, uniqueCnt1 = 1, uniqueCnt2 = 3
**Output:** 4
**Explanation:**
We can distribute the first 4 natural numbers into arr1 and arr2.
arr1 = \[1\] and arr2 = \[2,3,4\].
We can see that both arrays satisfy all the conditions.
Since the maximum value is 4, we return it.
**Example 2:**
**Input:** divisor1 = 3, divisor2 = 5, uniqueCnt1 = 2, uniqueCnt2 = 1
**Output:** 3
**Explanation:**
Here arr1 = \[1,2\], and arr2 = \[3\] satisfy all conditions.
Since the maximum value is 3, we return it.
**Example 3:**
**Input:** divisor1 = 2, divisor2 = 4, uniqueCnt1 = 8, uniqueCnt2 = 2
**Output:** 15
**Explanation:**
Here, the final possible arrays can be arr1 = \[1,3,5,7,9,11,13,15\], and arr2 = \[2,6\].
It can be shown that it is not possible to obtain a lower maximum satisfying all conditions.
**Constraints:**
* `2 <= divisor1, divisor2 <= 105`
* `1 <= uniqueCnt1, uniqueCnt2 < 109`
* `2 <= uniqueCnt1 + uniqueCnt2 <= 109` | null |
EZ understand, two lines of codes with full explanation | minimize-the-maximum-of-two-arrays | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nNote that for each bunch of divisor size integers, there are divisor size - 1 integers do not divide the divisor, \nIf no integers will be candidate for both array, the Maximum for each array can be computed with following equation:\n`uniqueCnt / (divisor - 1) * divisor`\nIf some inetgers can be considered for both array, we can treat it as a case when we have only one divisor which is `lcm(divisor1, divisor2)`\nso the Maximum for both array can be compueted with the following equation:\n`(uniqueCnt1 + uniqueCnt2) * lcm / (lcm - 1)`\nTogether, we pick the max value among the max for each single array and the max for both given the divisor as lcm.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Compute the lcm of divisor1, divisor2.\n2. Find the max value among the three possible Maximums.\n# Complexity\n- Time complexity: O(log(divisor1.divisor2)) since lcm can be computed as abs(a,b) // gcd(a, b) and gcd(a,b) is computed by binary Euclidean algorithm.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimizeSet(self, divisor1: int, divisor2: int, uniqueCnt1: int, uniqueCnt2: int) -> int:\n lcm = math.lcm(divisor1, divisor2)\n return math.ceil(max(\n uniqueCnt1 / (divisor1 - 1) * divisor1,\n uniqueCnt2 / (divisor2 - 1) * divisor2,\n (uniqueCnt1 + uniqueCnt2) * lcm / (lcm - 1)\n ))-1\n``` | 0 | We have two arrays `arr1` and `arr2` which are initially empty. You need to add positive integers to them such that they satisfy all the following conditions:
* `arr1` contains `uniqueCnt1` **distinct** positive integers, each of which is **not divisible** by `divisor1`.
* `arr2` contains `uniqueCnt2` **distinct** positive integers, each of which is **not divisible** by `divisor2`.
* **No** integer is present in both `arr1` and `arr2`.
Given `divisor1`, `divisor2`, `uniqueCnt1`, and `uniqueCnt2`, return _the **minimum possible maximum** integer that can be present in either array_.
**Example 1:**
**Input:** divisor1 = 2, divisor2 = 7, uniqueCnt1 = 1, uniqueCnt2 = 3
**Output:** 4
**Explanation:**
We can distribute the first 4 natural numbers into arr1 and arr2.
arr1 = \[1\] and arr2 = \[2,3,4\].
We can see that both arrays satisfy all the conditions.
Since the maximum value is 4, we return it.
**Example 2:**
**Input:** divisor1 = 3, divisor2 = 5, uniqueCnt1 = 2, uniqueCnt2 = 1
**Output:** 3
**Explanation:**
Here arr1 = \[1,2\], and arr2 = \[3\] satisfy all conditions.
Since the maximum value is 3, we return it.
**Example 3:**
**Input:** divisor1 = 2, divisor2 = 4, uniqueCnt1 = 8, uniqueCnt2 = 2
**Output:** 15
**Explanation:**
Here, the final possible arrays can be arr1 = \[1,3,5,7,9,11,13,15\], and arr2 = \[2,6\].
It can be shown that it is not possible to obtain a lower maximum satisfying all conditions.
**Constraints:**
* `2 <= divisor1, divisor2 <= 105`
* `1 <= uniqueCnt1, uniqueCnt2 < 109`
* `2 <= uniqueCnt1 + uniqueCnt2 <= 109` | null |
python super easy lower bound (binary search) | minimize-the-maximum-of-two-arrays | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimizeSet(self, divisor1: int, divisor2: int, uniqueCnt1: int, uniqueCnt2: int) -> int:\n \n\n l = 1\n h = 10 ** 10\n lcm = math.lcm(divisor1, divisor2)\n while l <= h:\n mid = l + (h-l) //2 \n\n if mid - mid // divisor1 < uniqueCnt1 or mid - mid // divisor2 < uniqueCnt2 or mid - mid//lcm < uniqueCnt1 + uniqueCnt2:\n l = mid +1\n else:\n h = mid -1\n return l\n``` | 0 | We have two arrays `arr1` and `arr2` which are initially empty. You need to add positive integers to them such that they satisfy all the following conditions:
* `arr1` contains `uniqueCnt1` **distinct** positive integers, each of which is **not divisible** by `divisor1`.
* `arr2` contains `uniqueCnt2` **distinct** positive integers, each of which is **not divisible** by `divisor2`.
* **No** integer is present in both `arr1` and `arr2`.
Given `divisor1`, `divisor2`, `uniqueCnt1`, and `uniqueCnt2`, return _the **minimum possible maximum** integer that can be present in either array_.
**Example 1:**
**Input:** divisor1 = 2, divisor2 = 7, uniqueCnt1 = 1, uniqueCnt2 = 3
**Output:** 4
**Explanation:**
We can distribute the first 4 natural numbers into arr1 and arr2.
arr1 = \[1\] and arr2 = \[2,3,4\].
We can see that both arrays satisfy all the conditions.
Since the maximum value is 4, we return it.
**Example 2:**
**Input:** divisor1 = 3, divisor2 = 5, uniqueCnt1 = 2, uniqueCnt2 = 1
**Output:** 3
**Explanation:**
Here arr1 = \[1,2\], and arr2 = \[3\] satisfy all conditions.
Since the maximum value is 3, we return it.
**Example 3:**
**Input:** divisor1 = 2, divisor2 = 4, uniqueCnt1 = 8, uniqueCnt2 = 2
**Output:** 15
**Explanation:**
Here, the final possible arrays can be arr1 = \[1,3,5,7,9,11,13,15\], and arr2 = \[2,6\].
It can be shown that it is not possible to obtain a lower maximum satisfying all conditions.
**Constraints:**
* `2 <= divisor1, divisor2 <= 105`
* `1 <= uniqueCnt1, uniqueCnt2 < 109`
* `2 <= uniqueCnt1 + uniqueCnt2 <= 109` | null |
Python | Easy Solution | shortest-distance-to-target-string-in-a-circular-array | 0 | 1 | # Code\n```\nclass Solution:\n def closetTarget(self, words: List[str], target: str, startIndex: int) -> int:\n n = len(words)\n # moving right\n moveright = 0\n flag = False\n for i in range(startIndex,len(words)+startIndex):\n if words[(i+1)%n] == target:\n flag = True\n moveright += 1\n break\n else:\n moveright += 1\n # If target is not present so its no use searching to the left so we return -1\n if flag == False:\n return -1\n \n # moving left\n moveleft = 0\n pointer = startIndex\n while True:\n if words[pointer] == target:\n break\n else:\n pointer = (pointer - 1 + n) % n\n moveleft += 1\n return min(moveleft,moveright)\n \n``` | 2 | You are given a **0-indexed** **circular** string array `words` and a string `target`. A **circular array** means that the array's end connects to the array's beginning.
* Formally, the next element of `words[i]` is `words[(i + 1) % n]` and the previous element of `words[i]` is `words[(i - 1 + n) % n]`, where `n` is the length of `words`.
Starting from `startIndex`, you can move to either the next word or the previous word with `1` step at a time.
Return _the **shortest** distance needed to reach the string_ `target`. If the string `target` does not exist in `words`, return `-1`.
**Example 1:**
**Input:** words = \[ "hello ", "i ", "am ", "leetcode ", "hello "\], target = "hello ", startIndex = 1
**Output:** 1
**Explanation:** We start from index 1 and can reach "hello " by
- moving 3 units to the right to reach index 4.
- moving 2 units to the left to reach index 4.
- moving 4 units to the right to reach index 0.
- moving 1 unit to the left to reach index 0.
The shortest distance to reach "hello " is 1.
**Example 2:**
**Input:** words = \[ "a ", "b ", "leetcode "\], target = "leetcode ", startIndex = 0
**Output:** 1
**Explanation:** We start from index 0 and can reach "leetcode " by
- moving 2 units to the right to reach index 3.
- moving 1 unit to the left to reach index 3.
The shortest distance to reach "leetcode " is 1.
**Example 3:**
**Input:** words = \[ "i ", "eat ", "leetcode "\], target = "ate ", startIndex = 0
**Output:** -1
**Explanation:** Since "ate " does not exist in `words`, we return -1.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` and `target` consist of only lowercase English letters.
* `0 <= startIndex < words.length` | null |
[Python3] Two simultaneous pointers to different sides | shortest-distance-to-target-string-in-a-circular-array | 0 | 1 | Modulo operation for negative numbers in python works like: `(i + l) % l if i < 0`\n```python3 []\nclass Solution:\n def closetTarget(self, words: List[str], target: str, start: int) -> int:\n ln = len(words)\n for k in range(ln//2 + 1):\n l, r = start - k, start + k\n if words[l % ln] == target: return abs(start - l)\n if words[r % ln] == target: return abs(r - start)\n return -1\n``` | 1 | You are given a **0-indexed** **circular** string array `words` and a string `target`. A **circular array** means that the array's end connects to the array's beginning.
* Formally, the next element of `words[i]` is `words[(i + 1) % n]` and the previous element of `words[i]` is `words[(i - 1 + n) % n]`, where `n` is the length of `words`.
Starting from `startIndex`, you can move to either the next word or the previous word with `1` step at a time.
Return _the **shortest** distance needed to reach the string_ `target`. If the string `target` does not exist in `words`, return `-1`.
**Example 1:**
**Input:** words = \[ "hello ", "i ", "am ", "leetcode ", "hello "\], target = "hello ", startIndex = 1
**Output:** 1
**Explanation:** We start from index 1 and can reach "hello " by
- moving 3 units to the right to reach index 4.
- moving 2 units to the left to reach index 4.
- moving 4 units to the right to reach index 0.
- moving 1 unit to the left to reach index 0.
The shortest distance to reach "hello " is 1.
**Example 2:**
**Input:** words = \[ "a ", "b ", "leetcode "\], target = "leetcode ", startIndex = 0
**Output:** 1
**Explanation:** We start from index 0 and can reach "leetcode " by
- moving 2 units to the right to reach index 3.
- moving 1 unit to the left to reach index 3.
The shortest distance to reach "leetcode " is 1.
**Example 3:**
**Input:** words = \[ "i ", "eat ", "leetcode "\], target = "ate ", startIndex = 0
**Output:** -1
**Explanation:** Since "ate " does not exist in `words`, we return -1.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` and `target` consist of only lowercase English letters.
* `0 <= startIndex < words.length` | null |
python - easy understanding | shortest-distance-to-target-string-in-a-circular-array | 0 | 1 | # Intuition\nMy first instinct was that It is circular, so in terms of calculation, I can\'t just count positive numbers, I have to count negative numbers too.\nIf I want to follow the intuitive way of writing in Python, I would just cut it into two parts, the front and the back, and then reverse them to form a new variable.\nFinally, I would find the positive and negative indices of the target, compare their sizes, and that would be the final answer.\n\nI also explained my thoughts on YouTube.\n[Link](https://youtu.be/quOs3SYgL-o)\n\n# Code\n```\nclass Solution:\n #1. circular\n #2. \u627E\u51FAstarIndex\u8207target\u4E4B\u9593\u7684\u6700\u77ED\u8DDD\u96E2\n def closetTarget(self, words: List[str], target: str, start: int) -> int:\n #\u7B2C\u4E00\u6B65\u9A5F\uFF1A\u5148\u6392\u9664\u4E0D\u53EF\u80FD\u7684\u6771\u897F\n if target not in words:\n return -1\n #\u7B2C\u4E8C\u6B65\u9A5F\uFF1Awords\u5207\u5272\u6210newWords\uFF0C\u628Astart\u653E\u5728\u6700\u524D\u9762\u7684\u4F4D\u7F6E\u4E0A\n newWords = words[start:] + words[:start] #\u65B0\u7684words\n index1 = newWords.index(target)\n for i in range(-1,-len(words)-1,-1):\n if target == newWords[i]:\n index2 = -i\n break\n\n return min(index1,index2)\n\n\n \n \n \n``` | 1 | You are given a **0-indexed** **circular** string array `words` and a string `target`. A **circular array** means that the array's end connects to the array's beginning.
* Formally, the next element of `words[i]` is `words[(i + 1) % n]` and the previous element of `words[i]` is `words[(i - 1 + n) % n]`, where `n` is the length of `words`.
Starting from `startIndex`, you can move to either the next word or the previous word with `1` step at a time.
Return _the **shortest** distance needed to reach the string_ `target`. If the string `target` does not exist in `words`, return `-1`.
**Example 1:**
**Input:** words = \[ "hello ", "i ", "am ", "leetcode ", "hello "\], target = "hello ", startIndex = 1
**Output:** 1
**Explanation:** We start from index 1 and can reach "hello " by
- moving 3 units to the right to reach index 4.
- moving 2 units to the left to reach index 4.
- moving 4 units to the right to reach index 0.
- moving 1 unit to the left to reach index 0.
The shortest distance to reach "hello " is 1.
**Example 2:**
**Input:** words = \[ "a ", "b ", "leetcode "\], target = "leetcode ", startIndex = 0
**Output:** 1
**Explanation:** We start from index 0 and can reach "leetcode " by
- moving 2 units to the right to reach index 3.
- moving 1 unit to the left to reach index 3.
The shortest distance to reach "leetcode " is 1.
**Example 3:**
**Input:** words = \[ "i ", "eat ", "leetcode "\], target = "ate ", startIndex = 0
**Output:** -1
**Explanation:** Since "ate " does not exist in `words`, we return -1.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` and `target` consist of only lowercase English letters.
* `0 <= startIndex < words.length` | null |
[Python] Easy Fast Solution With Explanation | shortest-distance-to-target-string-in-a-circular-array | 0 | 1 | # Intuition\n\n$\\cdot$ Target can appear more than once\n$\\cdot$ Each target has two possible shortest distance i.e. left or right\n$\\cdot$ Instead of infinite circle loop think of finite segment of concatenated arrays\n\n# Approach\nLoop through array to find each target. Then determine if target index is above or below starting index. This will determine where are the two shortest distances and which segment to consider.\n\nIf target below start:\nwords $words+1$\n[target, start, start+1] $[target, start, start+1]$\n\nIf target above start:\n$words-1$ words\n$[start, start+1, target]$ [start, start+1, target] \n\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def closetTarget(self, words: List[str], target: str, startIndex: int) -> int:\n if target not in words:\n return -1\n min_distance = len(words) - 1\n for index, word in enumerate(words):\n if word == target:\n if index < startIndex:\n distance = min(startIndex - index, index + len(words) - startIndex)\n else:\n distance = min(index - startIndex, startIndex + len(words) - index)\n min_distance = min(distance, min_distance)\n return min_distance\n\n\n\n\n``` | 2 | You are given a **0-indexed** **circular** string array `words` and a string `target`. A **circular array** means that the array's end connects to the array's beginning.
* Formally, the next element of `words[i]` is `words[(i + 1) % n]` and the previous element of `words[i]` is `words[(i - 1 + n) % n]`, where `n` is the length of `words`.
Starting from `startIndex`, you can move to either the next word or the previous word with `1` step at a time.
Return _the **shortest** distance needed to reach the string_ `target`. If the string `target` does not exist in `words`, return `-1`.
**Example 1:**
**Input:** words = \[ "hello ", "i ", "am ", "leetcode ", "hello "\], target = "hello ", startIndex = 1
**Output:** 1
**Explanation:** We start from index 1 and can reach "hello " by
- moving 3 units to the right to reach index 4.
- moving 2 units to the left to reach index 4.
- moving 4 units to the right to reach index 0.
- moving 1 unit to the left to reach index 0.
The shortest distance to reach "hello " is 1.
**Example 2:**
**Input:** words = \[ "a ", "b ", "leetcode "\], target = "leetcode ", startIndex = 0
**Output:** 1
**Explanation:** We start from index 0 and can reach "leetcode " by
- moving 2 units to the right to reach index 3.
- moving 1 unit to the left to reach index 3.
The shortest distance to reach "leetcode " is 1.
**Example 3:**
**Input:** words = \[ "i ", "eat ", "leetcode "\], target = "ate ", startIndex = 0
**Output:** -1
**Explanation:** Since "ate " does not exist in `words`, we return -1.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` and `target` consist of only lowercase English letters.
* `0 <= startIndex < words.length` | null |
shortest-distance-to-target-string-in-a-circular-array | shortest-distance-to-target-string-in-a-circular-array | 0 | 1 | # Code\n```javascript []\n/**\n * @param {string[]} words\n * @param {string} target\n * @param {number} startIndex\n * @return {number}\n */\nvar closetTarget = function(words, t, s) {\n if(!words.includes(t)){\n return -1\n }\n let a = words.concat(words.slice(0,s+1));\n a = a.reverse();\n let l = a.indexOf(t);\n let b = words.slice(s).concat(words)\n let m = b.indexOf(t)\n return Math.min(l,m)\n \n \n};\n```\n```python []\nclass Solution:\n def closetTarget(self, words: List[str], t: str, s: int) -> int:\n if t not in words:\n return -1\n a=words + words[:s+1]\n a = a[::-1]\n l = a.index(t)\n b = words[s:] + words\n m = b.index(t)\n return min(l,m)\n```\n\n```\n\n | 1 | You are given a **0-indexed** **circular** string array `words` and a string `target`. A **circular array** means that the array's end connects to the array's beginning.
* Formally, the next element of `words[i]` is `words[(i + 1) % n]` and the previous element of `words[i]` is `words[(i - 1 + n) % n]`, where `n` is the length of `words`.
Starting from `startIndex`, you can move to either the next word or the previous word with `1` step at a time.
Return _the **shortest** distance needed to reach the string_ `target`. If the string `target` does not exist in `words`, return `-1`.
**Example 1:**
**Input:** words = \[ "hello ", "i ", "am ", "leetcode ", "hello "\], target = "hello ", startIndex = 1
**Output:** 1
**Explanation:** We start from index 1 and can reach "hello " by
- moving 3 units to the right to reach index 4.
- moving 2 units to the left to reach index 4.
- moving 4 units to the right to reach index 0.
- moving 1 unit to the left to reach index 0.
The shortest distance to reach "hello " is 1.
**Example 2:**
**Input:** words = \[ "a ", "b ", "leetcode "\], target = "leetcode ", startIndex = 0
**Output:** 1
**Explanation:** We start from index 0 and can reach "leetcode " by
- moving 2 units to the right to reach index 3.
- moving 1 unit to the left to reach index 3.
The shortest distance to reach "leetcode " is 1.
**Example 3:**
**Input:** words = \[ "i ", "eat ", "leetcode "\], target = "ate ", startIndex = 0
**Output:** -1
**Explanation:** Since "ate " does not exist in `words`, we return -1.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` and `target` consist of only lowercase English letters.
* `0 <= startIndex < words.length` | null |
[Python 3] Check left and right | Brute Force | shortest-distance-to-target-string-in-a-circular-array | 0 | 1 | ```\nclass Solution:\n def closetTarget(self, words: List[str], target: str, startIndex: int) -> int:\n if target not in words:\n return -1\n \n words = words + words\n \n n = len(words)\n r = l = startIndex\n res = inf\n \n while words[l] != target:\n l = l - 1\n res = min(res, abs(startIndex - l))\n \n while words[r] != target:\n r = r + 1\n res = min(res, abs(startIndex - r))\n \n return res\n``` | 2 | You are given a **0-indexed** **circular** string array `words` and a string `target`. A **circular array** means that the array's end connects to the array's beginning.
* Formally, the next element of `words[i]` is `words[(i + 1) % n]` and the previous element of `words[i]` is `words[(i - 1 + n) % n]`, where `n` is the length of `words`.
Starting from `startIndex`, you can move to either the next word or the previous word with `1` step at a time.
Return _the **shortest** distance needed to reach the string_ `target`. If the string `target` does not exist in `words`, return `-1`.
**Example 1:**
**Input:** words = \[ "hello ", "i ", "am ", "leetcode ", "hello "\], target = "hello ", startIndex = 1
**Output:** 1
**Explanation:** We start from index 1 and can reach "hello " by
- moving 3 units to the right to reach index 4.
- moving 2 units to the left to reach index 4.
- moving 4 units to the right to reach index 0.
- moving 1 unit to the left to reach index 0.
The shortest distance to reach "hello " is 1.
**Example 2:**
**Input:** words = \[ "a ", "b ", "leetcode "\], target = "leetcode ", startIndex = 0
**Output:** 1
**Explanation:** We start from index 0 and can reach "leetcode " by
- moving 2 units to the right to reach index 3.
- moving 1 unit to the left to reach index 3.
The shortest distance to reach "leetcode " is 1.
**Example 3:**
**Input:** words = \[ "i ", "eat ", "leetcode "\], target = "ate ", startIndex = 0
**Output:** -1
**Explanation:** Since "ate " does not exist in `words`, we return -1.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` and `target` consist of only lowercase English letters.
* `0 <= startIndex < words.length` | null |
Python 3 || 7 lines, two loops, one pass || T/M: 42 ms / 13.9 MB | shortest-distance-to-target-string-in-a-circular-array | 0 | 1 | ```class Solution:\n def closetTarget(self, words: List[str], target: str, startIndex: int) -> int:\n\n n = len(words)\n\n for left in range(n):\n if words[(startIndex+left)%n ] == target: break\n else: return -1 \n\n for right in range(n):\n if words[(startIndex-right)%n] == target: break\n \n return min(left, right)\n```\n[https://leetcode.com/problems/shortest-distance-to-target-string-in-a-circular-array/submissions/865367549/](http://)\n\n\nI could be wrong, but I think that time is *O*(*N*) and space is *O*(1). | 10 | You are given a **0-indexed** **circular** string array `words` and a string `target`. A **circular array** means that the array's end connects to the array's beginning.
* Formally, the next element of `words[i]` is `words[(i + 1) % n]` and the previous element of `words[i]` is `words[(i - 1 + n) % n]`, where `n` is the length of `words`.
Starting from `startIndex`, you can move to either the next word or the previous word with `1` step at a time.
Return _the **shortest** distance needed to reach the string_ `target`. If the string `target` does not exist in `words`, return `-1`.
**Example 1:**
**Input:** words = \[ "hello ", "i ", "am ", "leetcode ", "hello "\], target = "hello ", startIndex = 1
**Output:** 1
**Explanation:** We start from index 1 and can reach "hello " by
- moving 3 units to the right to reach index 4.
- moving 2 units to the left to reach index 4.
- moving 4 units to the right to reach index 0.
- moving 1 unit to the left to reach index 0.
The shortest distance to reach "hello " is 1.
**Example 2:**
**Input:** words = \[ "a ", "b ", "leetcode "\], target = "leetcode ", startIndex = 0
**Output:** 1
**Explanation:** We start from index 0 and can reach "leetcode " by
- moving 2 units to the right to reach index 3.
- moving 1 unit to the left to reach index 3.
The shortest distance to reach "leetcode " is 1.
**Example 3:**
**Input:** words = \[ "i ", "eat ", "leetcode "\], target = "ate ", startIndex = 0
**Output:** -1
**Explanation:** Since "ate " does not exist in `words`, we return -1.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` and `target` consist of only lowercase English letters.
* `0 <= startIndex < words.length` | null |
Simple short Python solution | shortest-distance-to-target-string-in-a-circular-array | 0 | 1 | # Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def closetTarget(self, words: List[str], target: str, startIndex: int) -> int:\n for i in range(0, len(words)):\n if target in [words[(startIndex - i) % len(words)], words[(startIndex + i) % len(words)]]:\n return i\n return -1\n``` | 1 | You are given a **0-indexed** **circular** string array `words` and a string `target`. A **circular array** means that the array's end connects to the array's beginning.
* Formally, the next element of `words[i]` is `words[(i + 1) % n]` and the previous element of `words[i]` is `words[(i - 1 + n) % n]`, where `n` is the length of `words`.
Starting from `startIndex`, you can move to either the next word or the previous word with `1` step at a time.
Return _the **shortest** distance needed to reach the string_ `target`. If the string `target` does not exist in `words`, return `-1`.
**Example 1:**
**Input:** words = \[ "hello ", "i ", "am ", "leetcode ", "hello "\], target = "hello ", startIndex = 1
**Output:** 1
**Explanation:** We start from index 1 and can reach "hello " by
- moving 3 units to the right to reach index 4.
- moving 2 units to the left to reach index 4.
- moving 4 units to the right to reach index 0.
- moving 1 unit to the left to reach index 0.
The shortest distance to reach "hello " is 1.
**Example 2:**
**Input:** words = \[ "a ", "b ", "leetcode "\], target = "leetcode ", startIndex = 0
**Output:** 1
**Explanation:** We start from index 0 and can reach "leetcode " by
- moving 2 units to the right to reach index 3.
- moving 1 unit to the left to reach index 3.
The shortest distance to reach "leetcode " is 1.
**Example 3:**
**Input:** words = \[ "i ", "eat ", "leetcode "\], target = "ate ", startIndex = 0
**Output:** -1
**Explanation:** Since "ate " does not exist in `words`, we return -1.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` and `target` consist of only lowercase English letters.
* `0 <= startIndex < words.length` | null |
Python | Easy Solution✅ | shortest-distance-to-target-string-in-a-circular-array | 0 | 1 | # Code\u2705\n```\nclass Solution:\n def closetTarget(self, words: List[str], target: str, startIndex: int) -> int:\n if words[startIndex] == target:\n return 0\n\n for index in range(len(words)):\n if words[(startIndex+index) % len(words)] == target or words[(startIndex-index) % len(words)] == target:\n return index\n return -1\n``` | 6 | You are given a **0-indexed** **circular** string array `words` and a string `target`. A **circular array** means that the array's end connects to the array's beginning.
* Formally, the next element of `words[i]` is `words[(i + 1) % n]` and the previous element of `words[i]` is `words[(i - 1 + n) % n]`, where `n` is the length of `words`.
Starting from `startIndex`, you can move to either the next word or the previous word with `1` step at a time.
Return _the **shortest** distance needed to reach the string_ `target`. If the string `target` does not exist in `words`, return `-1`.
**Example 1:**
**Input:** words = \[ "hello ", "i ", "am ", "leetcode ", "hello "\], target = "hello ", startIndex = 1
**Output:** 1
**Explanation:** We start from index 1 and can reach "hello " by
- moving 3 units to the right to reach index 4.
- moving 2 units to the left to reach index 4.
- moving 4 units to the right to reach index 0.
- moving 1 unit to the left to reach index 0.
The shortest distance to reach "hello " is 1.
**Example 2:**
**Input:** words = \[ "a ", "b ", "leetcode "\], target = "leetcode ", startIndex = 0
**Output:** 1
**Explanation:** We start from index 0 and can reach "leetcode " by
- moving 2 units to the right to reach index 3.
- moving 1 unit to the left to reach index 3.
The shortest distance to reach "leetcode " is 1.
**Example 3:**
**Input:** words = \[ "i ", "eat ", "leetcode "\], target = "ate ", startIndex = 0
**Output:** -1
**Explanation:** Since "ate " does not exist in `words`, we return -1.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` and `target` consist of only lowercase English letters.
* `0 <= startIndex < words.length` | null |
8-Line Python Code | shortest-distance-to-target-string-in-a-circular-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def closetTarget(self, words: List[str], target: str, startIndex: int) -> int:\n if target not in words:\n return -1\n n = len(words)\n final_posn = float("inf")\n for i in range(n):\n if words[i] == target:\n final_posn = min(final_posn, abs(i-startIndex), n-abs(i-startIndex))\n return final_posn\n\n``` | 4 | You are given a **0-indexed** **circular** string array `words` and a string `target`. A **circular array** means that the array's end connects to the array's beginning.
* Formally, the next element of `words[i]` is `words[(i + 1) % n]` and the previous element of `words[i]` is `words[(i - 1 + n) % n]`, where `n` is the length of `words`.
Starting from `startIndex`, you can move to either the next word or the previous word with `1` step at a time.
Return _the **shortest** distance needed to reach the string_ `target`. If the string `target` does not exist in `words`, return `-1`.
**Example 1:**
**Input:** words = \[ "hello ", "i ", "am ", "leetcode ", "hello "\], target = "hello ", startIndex = 1
**Output:** 1
**Explanation:** We start from index 1 and can reach "hello " by
- moving 3 units to the right to reach index 4.
- moving 2 units to the left to reach index 4.
- moving 4 units to the right to reach index 0.
- moving 1 unit to the left to reach index 0.
The shortest distance to reach "hello " is 1.
**Example 2:**
**Input:** words = \[ "a ", "b ", "leetcode "\], target = "leetcode ", startIndex = 0
**Output:** 1
**Explanation:** We start from index 0 and can reach "leetcode " by
- moving 2 units to the right to reach index 3.
- moving 1 unit to the left to reach index 3.
The shortest distance to reach "leetcode " is 1.
**Example 3:**
**Input:** words = \[ "i ", "eat ", "leetcode "\], target = "ate ", startIndex = 0
**Output:** -1
**Explanation:** Since "ate " does not exist in `words`, we return -1.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` and `target` consist of only lowercase English letters.
* `0 <= startIndex < words.length` | null |
Simple Python Solution - O(N) - 3 lines of code | shortest-distance-to-target-string-in-a-circular-array | 0 | 1 | \n# Code\n```\nclass Solution:\n def closetTarget(self, words: List[str], target: str, startIndex: int) -> int:\n if words[startIndex] == target:\n return 0\n \n for i in range(1, len(words)):\n if words[(startIndex-i) % len(words)] == target or words[(startIndex+i) % len(words)] == target:\n return i\n\n return -1\n``` | 2 | You are given a **0-indexed** **circular** string array `words` and a string `target`. A **circular array** means that the array's end connects to the array's beginning.
* Formally, the next element of `words[i]` is `words[(i + 1) % n]` and the previous element of `words[i]` is `words[(i - 1 + n) % n]`, where `n` is the length of `words`.
Starting from `startIndex`, you can move to either the next word or the previous word with `1` step at a time.
Return _the **shortest** distance needed to reach the string_ `target`. If the string `target` does not exist in `words`, return `-1`.
**Example 1:**
**Input:** words = \[ "hello ", "i ", "am ", "leetcode ", "hello "\], target = "hello ", startIndex = 1
**Output:** 1
**Explanation:** We start from index 1 and can reach "hello " by
- moving 3 units to the right to reach index 4.
- moving 2 units to the left to reach index 4.
- moving 4 units to the right to reach index 0.
- moving 1 unit to the left to reach index 0.
The shortest distance to reach "hello " is 1.
**Example 2:**
**Input:** words = \[ "a ", "b ", "leetcode "\], target = "leetcode ", startIndex = 0
**Output:** 1
**Explanation:** We start from index 0 and can reach "leetcode " by
- moving 2 units to the right to reach index 3.
- moving 1 unit to the left to reach index 3.
The shortest distance to reach "leetcode " is 1.
**Example 3:**
**Input:** words = \[ "i ", "eat ", "leetcode "\], target = "ate ", startIndex = 0
**Output:** -1
**Explanation:** Since "ate " does not exist in `words`, we return -1.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` and `target` consist of only lowercase English letters.
* `0 <= startIndex < words.length` | null |
[python3] sliding window sol for reference | take-k-of-each-character-from-left-and-right | 0 | 1 | The intuition for the solution is below. \n\nlets assume that there are ta,tb and tc chars of a,b and c in the string, then we are looking for the longest window where number of a\'s is <= ta-a, b\'s is <= tb - b and c\'s is <= tc - c. \n\n```\nclass Solution:\n def takeCharacters(self, s: str, k: int) -> int:\n if k == 0: return 0\n\n ans = len(s)\n start = 0 \n ctr = Counter(s)\n h = defaultdict(int)\n \n if ctr[\'a\'] < k or ctr[\'b\'] < k or ctr[\'c\'] < k:\n return -1\n \n ctr[\'a\'] -= k\n ctr[\'b\'] -= k\n ctr[\'c\'] -= k\n \n if not ctr[\'a\'] and not ctr[\'b\'] and not ctr[\'c\']:\n return len(s)\n \n for i,c in enumerate(s):\n h[c] += 1\n \n while (h[\'a\'] > ctr[\'a\'] or h[\'b\'] > ctr[\'b\'] or h[\'c\'] > ctr[\'c\']) and start <= i: \n h[s[start]] -= 1\n start += 1\n \n if h[\'a\'] <= ctr[\'a\'] and h[\'b\'] <= ctr[\'b\'] and h[\'c\'] <= ctr[\'c\']:\n ans = min(start+len(s)-i-1, ans)\n \n return ans | 1 | You are given a string `s` consisting of the characters `'a'`, `'b'`, and `'c'` and a non-negative integer `k`. Each minute, you may take either the **leftmost** character of `s`, or the **rightmost** character of `s`.
Return _the **minimum** number of minutes needed for you to take **at least**_ `k` _of each character, or return_ `-1` _if it is not possible to take_ `k` _of each character._
**Example 1:**
**Input:** s = "aabaaaacaabc ", k = 2
**Output:** 8
**Explanation:**
Take three characters from the left of s. You now have two 'a' characters, and one 'b' character.
Take five characters from the right of s. You now have four 'a' characters, two 'b' characters, and two 'c' characters.
A total of 3 + 5 = 8 minutes is needed.
It can be proven that 8 is the minimum number of minutes needed.
**Example 2:**
**Input:** s = "a ", k = 1
**Output:** -1
**Explanation:** It is not possible to take one 'b' or 'c' so return -1.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only the letters `'a'`, `'b'`, and `'c'`.
* `0 <= k <= s.length` | null |
[Python 3] Sliding Window | take-k-of-each-character-from-left-and-right | 0 | 1 | **INTUITION** :\n\nwe have to find length of **largest window/substring** where we have\n* occurences of \'a\' in window/substring **<=** (total occurences of \'a\' in s) - k\n* occurences of \'b\' in window/substring **<=** (total occurences of \'b\' in s) - k\n* occurences of \'c\' in window/substring **<=** (total occurences of \'c\' in s) - k\n\n\n```\nclass Solution:\n def takeCharacters(self, s: str, k: int) -> int:\n ra = s.count(\'a\') - k\n rb = s.count(\'b\') - k\n rc = s.count(\'c\') - k\n \n\t\t# if any of them is less than 0, it means there are less than k occurences of a character.\n if any(i < 0 for i in [ra, rb, rc]):\n return -1\n \n hm = defaultdict(int)\n length = left = res = 0\n \n for right in s:\n hm[right] += 1\n length += 1\n \n while hm[\'a\'] > ra or hm[\'b\'] > rb or hm[\'c\'] > rc:\n hm[s[left]] -= 1\n length -= 1\n left += 1\n \n res = max(res, length)\n \n return len(s) - res\n``` | 9 | You are given a string `s` consisting of the characters `'a'`, `'b'`, and `'c'` and a non-negative integer `k`. Each minute, you may take either the **leftmost** character of `s`, or the **rightmost** character of `s`.
Return _the **minimum** number of minutes needed for you to take **at least**_ `k` _of each character, or return_ `-1` _if it is not possible to take_ `k` _of each character._
**Example 1:**
**Input:** s = "aabaaaacaabc ", k = 2
**Output:** 8
**Explanation:**
Take three characters from the left of s. You now have two 'a' characters, and one 'b' character.
Take five characters from the right of s. You now have four 'a' characters, two 'b' characters, and two 'c' characters.
A total of 3 + 5 = 8 minutes is needed.
It can be proven that 8 is the minimum number of minutes needed.
**Example 2:**
**Input:** s = "a ", k = 1
**Output:** -1
**Explanation:** It is not possible to take one 'b' or 'c' so return -1.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only the letters `'a'`, `'b'`, and `'c'`.
* `0 <= k <= s.length` | null |
Sliding Window by adding `S` to itself | take-k-of-each-character-from-left-and-right | 0 | 1 | # Intuition\nSliding Window by adding `s` to itself. After appending `s` to itself there will be 3 exit points.\n\n1. at the beginning of the string\n2. at the end of the string\n3. at the middle of the string ( since we added `s` to itself )\n\nthese are represented by `exits` variable in code below\n\n\n\n# Approach\nAdd string `s` to itself and use regular sliding window to find minimum window which contains `k` abc\'s.\n\nWhen calculating minimum window add the nearest exit point distance.\n\nExample : \n\n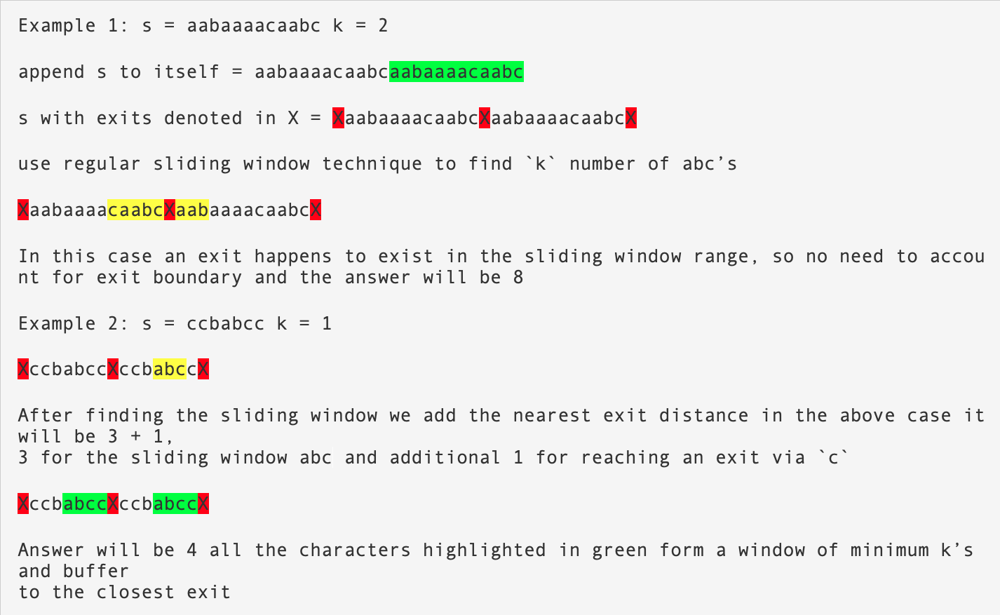\n\n\n# Complexity\n- Time complexity:\n- O(N)\n\n- Space complexity:\n- O(N)\n\n# Code\n```\nclass Solution:\n def takeCharacters(self, s: str, k: int) -> int:\n\n # early terminations\n if k == 0:\n return 0\n c = Counter(s)\n if c[\'a\'] < k or c[\'b\'] < k or c[\'c\'] < k:\n return -1\n\n # keep track of original `s` length\n slen = len(s)\n\n # append `s` to itself\n s += s\n ans = float(\'inf\')\n limit = len(s)\n\n # exits we can use after finding the sliding window which satisfies `k` abc\'s\n exits = [0, slen - 1, limit - 1]\n seen = {\'a\': 0, \'b\': 0, \'c\': 0}\n l = 0\n\n # regular sliding window approach - find k number of `abc`s\n for r in range(limit):\n seen[s[r]] += 1\n\n while l < r and seen[\'a\'] >= k and seen[\'b\'] >= k and seen[\'c\'] >= k:\n # after finding the sliding window\n # add nearest exit boundary either to left or right\n # if exit is present in the sliding window we just count the window boundary as the current best\n for e in exits:\n ans = min(ans, max(r, e) - min(e, l) + 1)\n seen[s[l]] -= 1\n l += 1\n\n return ans\n\n\n``` | 1 | You are given a string `s` consisting of the characters `'a'`, `'b'`, and `'c'` and a non-negative integer `k`. Each minute, you may take either the **leftmost** character of `s`, or the **rightmost** character of `s`.
Return _the **minimum** number of minutes needed for you to take **at least**_ `k` _of each character, or return_ `-1` _if it is not possible to take_ `k` _of each character._
**Example 1:**
**Input:** s = "aabaaaacaabc ", k = 2
**Output:** 8
**Explanation:**
Take three characters from the left of s. You now have two 'a' characters, and one 'b' character.
Take five characters from the right of s. You now have four 'a' characters, two 'b' characters, and two 'c' characters.
A total of 3 + 5 = 8 minutes is needed.
It can be proven that 8 is the minimum number of minutes needed.
**Example 2:**
**Input:** s = "a ", k = 1
**Output:** -1
**Explanation:** It is not possible to take one 'b' or 'c' so return -1.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only the letters `'a'`, `'b'`, and `'c'`.
* `0 <= k <= s.length` | null |
[Python] clean 12 line sliding window solution with explanation | take-k-of-each-character-from-left-and-right | 0 | 1 | **Explanation**\n\nInstead of taking `k` of each character from left and right, we take at most `count(c) - k` of each character from middle.\n\nFor Example 1, `s = "aabaaaacaabc"` with `k = 2`:\n- we have `freq = {\'a\': 8, \'b\': 2, \'c\': 2}`\n- this converts to `limits = freq - k = {\'a\': 6, \'b\': 0, \'c\': 0}`\n- now the problem becomes "finding the longest substring where the occurrence of each character is within `limits`"\n- we can easily solve this new problem using sliding window\n\n</br>\n\n**Complexity**\n\n- Time complexity: `O(N)`\n- Space complexity: `O(1)`\n\n</br>\n\n**Python**\n```\nclass Solution:\n def takeCharacters(self, s: str, k: int) -> int:\n limits = {c: s.count(c) - k for c in \'abc\'}\n if any(x < 0 for x in limits.values()):\n return -1\n\n cnts = {c: 0 for c in \'abc\'}\n ans = l = 0\n for r, c in enumerate(s):\n cnts[c] += 1\n while cnts[c] > limits[c]:\n cnts[s[l]] -= 1\n l += 1\n ans = max(ans, r - l + 1)\n\n return len(s) - ans\n``` | 113 | You are given a string `s` consisting of the characters `'a'`, `'b'`, and `'c'` and a non-negative integer `k`. Each minute, you may take either the **leftmost** character of `s`, or the **rightmost** character of `s`.
Return _the **minimum** number of minutes needed for you to take **at least**_ `k` _of each character, or return_ `-1` _if it is not possible to take_ `k` _of each character._
**Example 1:**
**Input:** s = "aabaaaacaabc ", k = 2
**Output:** 8
**Explanation:**
Take three characters from the left of s. You now have two 'a' characters, and one 'b' character.
Take five characters from the right of s. You now have four 'a' characters, two 'b' characters, and two 'c' characters.
A total of 3 + 5 = 8 minutes is needed.
It can be proven that 8 is the minimum number of minutes needed.
**Example 2:**
**Input:** s = "a ", k = 1
**Output:** -1
**Explanation:** It is not possible to take one 'b' or 'c' so return -1.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only the letters `'a'`, `'b'`, and `'c'`.
* `0 <= k <= s.length` | null |
Python 😎😎😎 || Explained with comments || clever? | take-k-of-each-character-from-left-and-right | 0 | 1 | # Code\n```\nclass Solution:\n def takeCharacters(self, s: str, k: int) -> int:\n # make a duplicate and count\n ss = s*2\n freq = {\'a\': s.count(\'a\'), \'b\': s.count(\'b\'), \'c\': s.count(\'c\')}\n\n if any([v < k for v in freq.values()]): # using any() to check if impossible\n return -1\n\n # just some variables\n windows = []\n start = 0\n end = len(s)-1\n\n # make sure start never crosses the boundary\n while start < len(s):\n\n # as long as the window is still valid and start doesn\'t cross the boundary\n while start < len(s) and all([v >= k for v in freq.values()]):\n # get rid of previous letter\n freq[s[start]] -= 1\n # next!\n start += 1\n\n windows.append(end-start+2)\n\n # as long as the window isn\'t valid\n while end < len(ss)-1 and any([v < k for v in freq.values()]):\n end += 1\n freq[ss[end]] += 1\n windows.append(end-start+1)\n\n # just return the smallest window size\n return min(windows)\n```\n\n | 1 | You are given a string `s` consisting of the characters `'a'`, `'b'`, and `'c'` and a non-negative integer `k`. Each minute, you may take either the **leftmost** character of `s`, or the **rightmost** character of `s`.
Return _the **minimum** number of minutes needed for you to take **at least**_ `k` _of each character, or return_ `-1` _if it is not possible to take_ `k` _of each character._
**Example 1:**
**Input:** s = "aabaaaacaabc ", k = 2
**Output:** 8
**Explanation:**
Take three characters from the left of s. You now have two 'a' characters, and one 'b' character.
Take five characters from the right of s. You now have four 'a' characters, two 'b' characters, and two 'c' characters.
A total of 3 + 5 = 8 minutes is needed.
It can be proven that 8 is the minimum number of minutes needed.
**Example 2:**
**Input:** s = "a ", k = 1
**Output:** -1
**Explanation:** It is not possible to take one 'b' or 'c' so return -1.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only the letters `'a'`, `'b'`, and `'c'`.
* `0 <= k <= s.length` | null |
[python3] time: O(n) & space: O(1) | take-k-of-each-character-from-left-and-right | 0 | 1 | # Code\n```\nclass Solution:\n def takeCharacters(self, s: str, k: int) -> int:\n a = s.count(\'a\') - k\n b = s.count(\'b\') - k\n c = s.count(\'c\') - k\n if any(i<0 for i in [a, b, c]):\n return -1\n dict = defaultdict(int)\n left = length = res = 0\n for right in s:\n dict[right] += 1\n length += 1\n while dict[\'a\'] > a or dict[\'b\'] > b or dict[\'c\'] > c:\n dict[s[left]] -= 1\n length -= 1\n left += 1\n res = max(res, length)\n return len(s) - res\n \n``` | 1 | You are given a string `s` consisting of the characters `'a'`, `'b'`, and `'c'` and a non-negative integer `k`. Each minute, you may take either the **leftmost** character of `s`, or the **rightmost** character of `s`.
Return _the **minimum** number of minutes needed for you to take **at least**_ `k` _of each character, or return_ `-1` _if it is not possible to take_ `k` _of each character._
**Example 1:**
**Input:** s = "aabaaaacaabc ", k = 2
**Output:** 8
**Explanation:**
Take three characters from the left of s. You now have two 'a' characters, and one 'b' character.
Take five characters from the right of s. You now have four 'a' characters, two 'b' characters, and two 'c' characters.
A total of 3 + 5 = 8 minutes is needed.
It can be proven that 8 is the minimum number of minutes needed.
**Example 2:**
**Input:** s = "a ", k = 1
**Output:** -1
**Explanation:** It is not possible to take one 'b' or 'c' so return -1.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only the letters `'a'`, `'b'`, and `'c'`.
* `0 <= k <= s.length` | null |
Python 3 || 12 lines, sliding window || T/M: 291 ms / 14.8 MB | take-k-of-each-character-from-left-and-right | 0 | 1 | ```\nclass Solution:\n def takeCharacters(self, s: str, k: int) -> int:\n\n d = Counter(s)\n if min(d.values()) < k: return -1\n\n d[\'a\']-= k ; d[\'b\']-= k ; d[\'c\']-= k \n\n ct = {\'a\':0, \'b\':0, \'c\':0}\n\n n, left, ans = len(s)-1, 0, inf\n\n for right, ch in enumerate(s):\n ct[ch]+= 1\n\n while ct[ch] > d[ch]:\n ct[s[left]]-= 1\n left+= 1\n\n ans = min(ans, n-right+left)\n\n return ans\n```\n[https://leetcode.com/problems/take-k-of-each-character-from-left-and-right/submissions/865427114/](http://)\n\nI could be wrong, but I think that time complexity is *O*(*N*) and space complexity is *O*(1).\n | 5 | You are given a string `s` consisting of the characters `'a'`, `'b'`, and `'c'` and a non-negative integer `k`. Each minute, you may take either the **leftmost** character of `s`, or the **rightmost** character of `s`.
Return _the **minimum** number of minutes needed for you to take **at least**_ `k` _of each character, or return_ `-1` _if it is not possible to take_ `k` _of each character._
**Example 1:**
**Input:** s = "aabaaaacaabc ", k = 2
**Output:** 8
**Explanation:**
Take three characters from the left of s. You now have two 'a' characters, and one 'b' character.
Take five characters from the right of s. You now have four 'a' characters, two 'b' characters, and two 'c' characters.
A total of 3 + 5 = 8 minutes is needed.
It can be proven that 8 is the minimum number of minutes needed.
**Example 2:**
**Input:** s = "a ", k = 1
**Output:** -1
**Explanation:** It is not possible to take one 'b' or 'c' so return -1.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only the letters `'a'`, `'b'`, and `'c'`.
* `0 <= k <= s.length` | null |
[C++, Java, Python] Binary Search and sorting | maximum-tastiness-of-candy-basket | 1 | 1 | \n\n# Intuition\nWe can use binary search to search the minimum difference. Since range is `0 - 10^9`, Time complexity will be `n * log(10 ^ 9) = 10 ^ 5 * 30`. This should be within constraints.\n\n# Approach\n* Sort `prices`\n* Run a binary search in the range `0 to 10 ^ 9`\n* The check function iterates over the array `prices` and checks if the given value `x` can be the minimum difference for any subsequence of the array prices\n\n# Complexity\n- Time complexity: O(nlog(10^9))\n\n- Space complexity: O(sort)\n\n# Code\n**Python3**:\n```\ndef maximumTastiness(self, price: List[int], k: int) -> int:\n price.sort()\n def check(x):\n last, count, i = price[0], 1, 1\n while count < k and i < len(price):\n if price[i] - last >= x:\n last, count = price[i], count + 1\n i += 1\n return count == k\n lo, hi = 0, 10 ** 9\n while lo < hi:\n mid = (lo + hi) // 2\n if check(mid): lo = mid + 1\n else: hi = mid\n return lo - 1\n```\n\n**C++**:\n```\nint maximumTastiness(vector<int>& price, int k) {\n sort(price.begin(), price.end());\n int lo = 0, hi = 1e9;\n while (lo < hi) {\n int mid = lo + (hi - lo) / 2;\n if (check(mid, price, k)) lo = mid + 1;\n else hi = mid;\n }\n return lo - 1;\n}\nbool check(int x, vector<int>& price, int k) {\n int last = price[0], count = 1, i = 1;\n while (count < k && i < price.size()) {\n if (price[i] - last >= x)\n last = price[i], count++;\n i++;\n }\n return count == k;\n}\n```\n\n**Java**:\n```\npublic int maximumTastiness(int[] price, int k) {\n Arrays.sort(price);\n int lo = 0, hi = 1000_000_000;\n while (lo < hi) {\n int mid = lo + (hi - lo) / 2;\n if (check(mid, price, k)) lo = mid + 1;\n else hi = mid;\n }\n return lo - 1;\n}\nboolean check(int x, int[] price, int k) {\n int last = price[0], count = 1, i = 1;\n while (count < k && i < price.length) {\n if (price[i] - last >= x) {\n last = price[i]; count++;\n }\n i++;\n }\n return count == k;\n}\n``` | 65 | You are given an array of positive integers `price` where `price[i]` denotes the price of the `ith` candy and a positive integer `k`.
The store sells baskets of `k` **distinct** candies. The **tastiness** of a candy basket is the smallest absolute difference of the **prices** of any two candies in the basket.
Return _the **maximum** tastiness of a candy basket._
**Example 1:**
**Input:** price = \[13,5,1,8,21,2\], k = 3
**Output:** 8
**Explanation:** Choose the candies with the prices \[13,5,21\].
The tastiness of the candy basket is: min(|13 - 5|, |13 - 21|, |5 - 21|) = min(8, 8, 16) = 8.
It can be proven that 8 is the maximum tastiness that can be achieved.
**Example 2:**
**Input:** price = \[1,3,1\], k = 2
**Output:** 2
**Explanation:** Choose the candies with the prices \[1,3\].
The tastiness of the candy basket is: min(|1 - 3|) = min(2) = 2.
It can be proven that 2 is the maximum tastiness that can be achieved.
**Example 3:**
**Input:** price = \[7,7,7,7\], k = 2
**Output:** 0
**Explanation:** Choosing any two distinct candies from the candies we have will result in a tastiness of 0.
**Constraints:**
* `2 <= k <= price.length <= 105`
* `1 <= price[i] <= 109` | null |
[Python3] | Sorting + Binary Search | maximum-tastiness-of-candy-basket | 0 | 1 | ```\nclass Solution:\n def maximumTastiness(self, price: List[int], k: int) -> int:\n if k == 0:\n return 0\n price.sort()\n def isValid(num):\n n = len(price)\n cnt = 1\n diff = price[0] + num\n for i in range(1,n):\n if price[i] >= diff:\n diff = price[i] + num\n cnt += 1\n else:\n continue\n return cnt\n low,high = 0,max(price) - min(price)\n ans = -1\n while low <= high:\n mid = (low + high) >> 1\n if isValid(mid) >= k:\n ans = mid\n low = mid + 1\n else:\n high = mid - 1\n return ans\n``` | 8 | You are given an array of positive integers `price` where `price[i]` denotes the price of the `ith` candy and a positive integer `k`.
The store sells baskets of `k` **distinct** candies. The **tastiness** of a candy basket is the smallest absolute difference of the **prices** of any two candies in the basket.
Return _the **maximum** tastiness of a candy basket._
**Example 1:**
**Input:** price = \[13,5,1,8,21,2\], k = 3
**Output:** 8
**Explanation:** Choose the candies with the prices \[13,5,21\].
The tastiness of the candy basket is: min(|13 - 5|, |13 - 21|, |5 - 21|) = min(8, 8, 16) = 8.
It can be proven that 8 is the maximum tastiness that can be achieved.
**Example 2:**
**Input:** price = \[1,3,1\], k = 2
**Output:** 2
**Explanation:** Choose the candies with the prices \[1,3\].
The tastiness of the candy basket is: min(|1 - 3|) = min(2) = 2.
It can be proven that 2 is the maximum tastiness that can be achieved.
**Example 3:**
**Input:** price = \[7,7,7,7\], k = 2
**Output:** 0
**Explanation:** Choosing any two distinct candies from the candies we have will result in a tastiness of 0.
**Constraints:**
* `2 <= k <= price.length <= 105`
* `1 <= price[i] <= 109` | null |
Python | Binary Search on answers | O(NlogN + NlogM) | maximum-tastiness-of-candy-basket | 0 | 1 | # Intuition\nIf we sort an array it becomes clear that the first $$n-1$$ minimal differences lie between neighbors (there is a less difference with neighbor than with an element next to neighbor). We can use that observation to built some backet of candies. How to decide which candies we need to put in backet? What if we somehow get the target tastiness and then we can make a backet with candies that have tastiness not less than target.\n\nGiven an array `price = [1,3,1]`. Consider a few edge cases:\n- We want to find backet with maximum possible tastiness, **which is 2 because max difference is always between min and max price**. We take 2 candies with prices 1 and 3. The difference between their prices is 2.\n- We want to find basket with minimum possible tastiness, **which is 0 because candies have same price**. We take 2 candies with price 1, difference - 0.\n\nIt looks like we have some boundaries and now we can apply binary search on answers here to check different found backets with specified tastiness. If we do not find enough candies in our backet we need to loosen the condition and try to choose candies with a smaller difference. Otherwise, we need to make the condition stricter and try to choose candies with a bigger difference.\n\n# Approach\n1. Sort array of prices\n2. Run binary search on possible answers, in the range from `0` to `max possible difference`\n - `0`, bacause it is possible to have 2 equal price in array (diff = 0)\n - `max possible difference`, which is `max_price - min_price`\n3. On each iteration: \n 1. check with how many candies can we make a backet so that the tastiness is at least the specified (our mid calculated for binary search)\n 1. compare size of built backet with `k`. If we can reach required number of candies then move left boundary to the right (make the condition more strict). Otherwise, move right boundary to the left (make the condition more weak).\n\n# Complexity\n- Time complexity:\n$$O(NlogN + NlogM)$$, where $$N$$ - number of candies, $$M$$ - maximum possible difference between any 2 candies.\n\n- Space complexity:\n$$O(logN)$$, where $$N$$ - number of candies. Because of sorting, if we assume that sort is quicksort algorithm.\n\n# Code\n```\nclass Solution:\n # Binary search by range of tastiness (range of possible differences)\n # Time: O(NlogN + NlogM), where N - number of candies, M - maximum possible difference between any 2 candies\n # Space: O(logn), for sorting if we assume sort is quicksort algorithm\n def maximumTastiness(self, price: List[int], k: int) -> int:\n price.sort()\n \n def find_at_least_tastiness_backet(min_tastiness):\n # goal: find backet with tastiness >= min_tastiness\n\n # place 1st candy to basket\n # we always place candy w/ min price coz it give us bigger differences\n last = price[0]\n cnt = 1\n\n # try to find next candy w/ difference between pair >= min_tastiness\n for i in range(1, len(price)):\n if price[i] - last >= min_tastiness: # found next candy for backet\n cnt += 1 # place candy to backet\n # doesn\'t make any sense to check [:i] candies\n # coz it will give us smaller diff between \n # current and max found price (=last)\n # so we continue to search diff starting from last found candy\n last = price[i]\n return cnt\n\n left = 0 # min possible difference (when 2 nums are equal)\n right = price[-1] - price[0] # max possble difference\n ans = 0\n while left <= right:\n mid = left + (right - left) // 2 # potential target tastiness\n if find_at_least_tastiness_backet(mid) >= k:\n left = mid + 1 # we found more candies than needed, let\'s try increase our found result, increase target tastiness of backet (assuming to find reach with less candies)\n ans = mid\n else:\n right = mid - 1\n\n return ans\n``` | 4 | You are given an array of positive integers `price` where `price[i]` denotes the price of the `ith` candy and a positive integer `k`.
The store sells baskets of `k` **distinct** candies. The **tastiness** of a candy basket is the smallest absolute difference of the **prices** of any two candies in the basket.
Return _the **maximum** tastiness of a candy basket._
**Example 1:**
**Input:** price = \[13,5,1,8,21,2\], k = 3
**Output:** 8
**Explanation:** Choose the candies with the prices \[13,5,21\].
The tastiness of the candy basket is: min(|13 - 5|, |13 - 21|, |5 - 21|) = min(8, 8, 16) = 8.
It can be proven that 8 is the maximum tastiness that can be achieved.
**Example 2:**
**Input:** price = \[1,3,1\], k = 2
**Output:** 2
**Explanation:** Choose the candies with the prices \[1,3\].
The tastiness of the candy basket is: min(|1 - 3|) = min(2) = 2.
It can be proven that 2 is the maximum tastiness that can be achieved.
**Example 3:**
**Input:** price = \[7,7,7,7\], k = 2
**Output:** 0
**Explanation:** Choosing any two distinct candies from the candies we have will result in a tastiness of 0.
**Constraints:**
* `2 <= k <= price.length <= 105`
* `1 <= price[i] <= 109` | null |
Easy to understand Python with full explanation, clear variable naming, and inline comments | maximum-tastiness-of-candy-basket | 0 | 1 | # Intuition\nThis problem presents itself as a max-min problem. In order to find the answer, we need to find the minimum absolute difference of candy prices in any k sized basket, and then find the maximum amongst those minimum values calculated.\n\n# Approach\nFirstly, we can sort the input array. This provides a useful property/assumption of the prices array: $$price_{i} <= price_{i+1}$$ which implies that the difference between $$price_{i+1} - price_{i} <= price_{i+2} - price_{i}$$ and so on. This is an important assumption of the function `checkPriceConstraint()` below because we greedily add candies to our basket.\n\nSecond, a function that checks if `k` candies can be selected that each have _at least_ a minimum absolute difference of `minPriceDiff` between them from our sorted prices input array. This is `checkPriceConstraint()` below; Greedily taking the first candy for our basket, then taking each next candy that satisfies our minimum difference constraint with the last candy\'s price we added to our basket, until `k` candies are selected.\n\nThird, where to start. An important value we can find is the global absolute maximum between any two candies in the input. This is equal to the $$max(price) - min(price)$$. And the absolute minimum differences we can have is $$0$$. Given this, we want to find the maximum value within this range of $$[0, maxDiff]$$ that has a valid minimum absolute difference between `k` selected candies. One strategy, not accepted, is to try each value from 0 to maxDiff, and stop on the first value that fails. This works, but will fail our time complexity. A binary search can optimize this linear scan of values because we know that if a value works, say $$value_{i}$$ then everything before $$i$$ must also be valid. Likewise if $$value_{i}$$ fails, then everything after $$i$$ is also invalid. So, ultimately, our binary search will resolve a maximum satisfactory minimum price difference.\n\n# Complexity\n- Time complexity:\n$$O(nlogn)$$ for the initial sort\n$$O(logn)$$ for the binary search to find the maximum valid price difference ... times the algorithm to check if the price is a valid achievable difference for k candies ... `checkPriceConstraint()` is $$O(n)$$.\nTotal we have, $$(nlog(n) + log(n)*n)$$ which is equivalent to $$nlog(n)$$\n\n\n- Space complexity:\nUnless we can sort in place, the input prices will be allocated for $$O(n)$$ space.\n\n# Code\n```\nclass Solution:\n def maximumTastiness(self, price: List[int], k: int) -> int:\n # We want a maximum of the minimums\n\n # Sorting the input array will give\n # [1, 2, 5, 8, 12, 21]\n\n # Now sorted, we can be certain the absolute difference between\n # any two candies is increasing or 0 from left to right\n\n price = sorted(price)\n\n # This function checks if we can place k candies\n # with a minimum of `minPriceDiff` between each candy\n def checkPriceConstraint(minPriceDiff):\n candiesInBasket = 1 # Always use the first one because\n lastCandyAddedIdx = 0 # there is no constraint on it\n\n for idx in range(1, len(price)):\n if price[idx] - price[lastCandyAddedIdx] >= minPriceDiff:\n lastCandyAddedIdx = idx\n candiesInBasket += 1\n \n if candiesInBasket >= k:\n return True\n return False\n\n # This value is the global max difference between any two candies\n maxDiff = abs(price[-1] - price[0])\n\n # Binary Search on the different price constraints between any 2 candies in the basket\n # While doing it, track the max of the price constraints that are valid\n # This works because for any given price constraint, if it works\n # then everything less than it will work, if it doesn\'t then anything right\n # of it won\'t\n leftPrice = 0\n rightPrice = maxDiff\n maxOfMins = 0\n while leftPrice <= rightPrice:\n midPrice = (leftPrice + rightPrice) // 2\n if checkPriceConstraint(midPrice):\n maxOfMins = midPrice\n leftPrice = midPrice + 1\n else:\n rightPrice = midPrice - 1\n \n return maxOfMins\n``` | 1 | You are given an array of positive integers `price` where `price[i]` denotes the price of the `ith` candy and a positive integer `k`.
The store sells baskets of `k` **distinct** candies. The **tastiness** of a candy basket is the smallest absolute difference of the **prices** of any two candies in the basket.
Return _the **maximum** tastiness of a candy basket._
**Example 1:**
**Input:** price = \[13,5,1,8,21,2\], k = 3
**Output:** 8
**Explanation:** Choose the candies with the prices \[13,5,21\].
The tastiness of the candy basket is: min(|13 - 5|, |13 - 21|, |5 - 21|) = min(8, 8, 16) = 8.
It can be proven that 8 is the maximum tastiness that can be achieved.
**Example 2:**
**Input:** price = \[1,3,1\], k = 2
**Output:** 2
**Explanation:** Choose the candies with the prices \[1,3\].
The tastiness of the candy basket is: min(|1 - 3|) = min(2) = 2.
It can be proven that 2 is the maximum tastiness that can be achieved.
**Example 3:**
**Input:** price = \[7,7,7,7\], k = 2
**Output:** 0
**Explanation:** Choosing any two distinct candies from the candies we have will result in a tastiness of 0.
**Constraints:**
* `2 <= k <= price.length <= 105`
* `1 <= price[i] <= 109` | null |
[Python3] [DP] Simple solution with steps and intuition | number-of-great-partitions | 0 | 1 | #### Intuition\nNotice in the problem description that k < 1000 while number of subsets of the array can go as high as 2^1000, instead of thinking from the perspextive of iterating through each subset, we should think about how to use this "k" to resolve the problem.\n#### Steps\n\n- Define a sub-problem: \n`Find the total number of subsets in the array with their sums smaller than k.`. \nThis is a classic DP problem [SubsetSum](https://leetcode.com/discuss/interview-question/1279773/google-interview-question-array-subset-sum-equals-k) with time complexity `O(N * K)`.\n- For each of the invalid subset, it can be either the first or second of an invalid tuple.\n- So, let the number of invalid subsets be X, total number of subsets pairs is 2^n, total valid subsets should be `2^n - 2 * X`.\n\n *Needs to think about edges cases where all pairs of subsets are invalid (`2 * X > 2^n`).*\n\n#### Complexity\n`O(N * K)`\n\n#### Solution\n```\nclass Solution:\n def countPartitions(self, nums: List[int], k: int) -> int:\n n = len(nums)\n dp = [[0] * (k + 1)] + [[-1] * (k + 1) for _ in range(n)]\n dp[0][0] = 1\n def subsetSumCounts(s, idx):\n if s < 0:\n \treturn 0\n if dp[idx][s] < 0:\n dp[idx][s] = subsetSumCounts(s, idx - 1) + subsetSumCounts(s - nums[idx - 1], idx - 1)\n return dp[idx][s]\n \n invalid_pairs = sum([subsetSumCounts(i, n) for i in range(k)]) * 2\n return max(2**n - invalid_pairs, 0) % (10**9 + 7)\n``` | 35 | You are given an array `nums` consisting of **positive** integers and an integer `k`.
**Partition** the array into two ordered **groups** such that each element is in exactly **one** group. A partition is called great if the **sum** of elements of each group is greater than or equal to `k`.
Return _the number of **distinct** great partitions_. Since the answer may be too large, return it **modulo** `109 + 7`.
Two partitions are considered distinct if some element `nums[i]` is in different groups in the two partitions.
**Example 1:**
**Input:** nums = \[1,2,3,4\], k = 4
**Output:** 6
**Explanation:** The great partitions are: (\[1,2,3\], \[4\]), (\[1,3\], \[2,4\]), (\[1,4\], \[2,3\]), (\[2,3\], \[1,4\]), (\[2,4\], \[1,3\]) and (\[4\], \[1,2,3\]).
**Example 2:**
**Input:** nums = \[3,3,3\], k = 4
**Output:** 0
**Explanation:** There are no great partitions for this array.
**Example 3:**
**Input:** nums = \[6,6\], k = 2
**Output:** 2
**Explanation:** We can either put nums\[0\] in the first partition or in the second partition.
The great partitions will be (\[6\], \[6\]) and (\[6\], \[6\]).
**Constraints:**
* `1 <= nums.length, k <= 1000`
* `1 <= nums[i] <= 109` | null |
[Python] DP O(n*k) - Video Solution | number-of-great-partitions | 0 | 1 | I have explained this [here](https://youtu.be/CQtLbU8x_vI).\n\n**Time:** `O(N.K)`\n\n**Space:** `O(N.K)`\n\n```\nclass Solution:\n def countPartitions(self, nums: List[int], k: int) -> int:\n n = len(nums)\n \n @cache\n def dfs(i, cur_sum):\n if i==n:\n return 1\n \n skip = dfs(i+1, cur_sum)\n include = 0\n if nums[i] + cur_sum < k:\n include = dfs(i+1, cur_sum + nums[i])\n \n return skip + include\n \n less_than_k = 2 * dfs(0,0)\n return max(2**n - less_than_k, 0) % (10**9 + 7)\n\n | 5 | You are given an array `nums` consisting of **positive** integers and an integer `k`.
**Partition** the array into two ordered **groups** such that each element is in exactly **one** group. A partition is called great if the **sum** of elements of each group is greater than or equal to `k`.
Return _the number of **distinct** great partitions_. Since the answer may be too large, return it **modulo** `109 + 7`.
Two partitions are considered distinct if some element `nums[i]` is in different groups in the two partitions.
**Example 1:**
**Input:** nums = \[1,2,3,4\], k = 4
**Output:** 6
**Explanation:** The great partitions are: (\[1,2,3\], \[4\]), (\[1,3\], \[2,4\]), (\[1,4\], \[2,3\]), (\[2,3\], \[1,4\]), (\[2,4\], \[1,3\]) and (\[4\], \[1,2,3\]).
**Example 2:**
**Input:** nums = \[3,3,3\], k = 4
**Output:** 0
**Explanation:** There are no great partitions for this array.
**Example 3:**
**Input:** nums = \[6,6\], k = 2
**Output:** 2
**Explanation:** We can either put nums\[0\] in the first partition or in the second partition.
The great partitions will be (\[6\], \[6\]) and (\[6\], \[6\]).
**Constraints:**
* `1 <= nums.length, k <= 1000`
* `1 <= nums[i] <= 109` | null |
[C++ || Java || Python] Direct approach to solve a deformed 01 knapsack problem | number-of-great-partitions | 0 | 1 | > **I know almost nothing about English, pointing out the mistakes in my article would be much appreciated.**\n\n> **In addition, I\'m too weak, please be critical of my ideas.**\n\n> **Vote welcome if this solution helped.**\n---\n\n# Intuition\n1. Step by step, each number must belong to a group. The sums of two groups will be changed with the change of belonging situation. A natural thought is to record the count of "until $i$, two groups\' sum are $x$ and $y$".\n2. Notice that $x+y=s$, here $s$ means the prefix sum until $i$. So remove one group is more clear. Define {until $i$, one of group\'s sum $j$} as the state, it convert to a classical [0/1 knapsack problem](https://en.wikipedia.org/wiki/Knapsack_problem). The problem seems to have been resolved.\n3. But there is a serious problem: the value range is too large to record.\n4. Notice $k$ is small, so we can immediately think about recording the states which not greater than $k$, and treat the sum greater than $k$ as $k$. \n5. But that will cause new question: the record of $k$ contains some illegal state (the sum of another group is less than k). It\'s hard to eliminate.\n6. The reason is we selected a group as state randomly, but the symmetry of information is destroyed during the compression process.\n7. Since we need both two groups are not less than $k$, better modify the state to {until $i$, the smaller group\'s sum $j$}. The final state will no longer contains illegal states.\n\n# Approach\n\n1. Define $dp[i][j]$ as the count of **"until $i$, the smaller group equals to $j$"**. In particular, $dp[i][k]$ saves the count of $\\geq k$. \n As a result, in each step we have two choice:\n - Add $nums[i]$ into larger group, the smaller group remains unchanged. So directly add $dp[i][j]$ into $dp[i+1][j]$.\n - Add $nums[i]$ into smaller group, this operation may lead to two different results:\n - Let the smaller group surpass the larger one. In this case we need to add $dp[i][j]$ into $dp[i+1][s-i]$, here $s$ means the prefix sum until $i$.\n - The smaller group keep smaller than the lagrer one. In this case we need to add $dp[i][j]$ into $dp[i+1][j+nums[i]]$.\n\n Finally, don\'t forget all the $j$, $s-j$, $j+nums[i]$ should keep less than $k$, the excess will be treated as $k$.\n2. **So the transfer equation seems as:**\n$$dp[i+1][t] = dp[i][j] + dp[i][t], \\space where \\space t = \\{k, s-j, j+nums[i]\\}_{min}$$\n3. Try to optimize the naive implementation:\n Easy to find the transfer always occurs between $dp[i][*]$ and $dp[i+1][*]$, so consider to compress two-dimensional array into one-dimensional.\n It\'s a common optimization, we must care about the refresh order to ensure that values will not be updated until used. In this problem, $nums[i]>0$ is always established, so $t>=j$ is always established as well. As a result, refreshing $j$ in descending order works fine.\n\n# Complexity\n- Time complexity: $$O(nk)$$\n- Space complexity: $$O(k)$$\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int countPartitions(vector<int>& nums, int k) {\n vector<int> dp(k + 1, 0);\n dp[0] = 1;\n long s = 0;\n for (auto x: nums) {\n for (int j = min<long>(k, s); j >= 0; --j) {\n long t = min<long>(min<long>(k, s - j), j + x);\n dp[t] = (dp[t] + dp[j]) % 1000000007;\n }\n s += x;\n }\n return dp[k];\n }\n};\n```\n```Java []\nclass Solution {\n public int countPartitions(int[] nums, int k) {\n int []dp = new int[k+1];\n dp[0] = 1;\n int s = 0;\n for (int x: nums) {\n for (int j = Math.min(k, s); j >= 0; --j) {\n int t = Math.min(k, Math.min(s - j, j + x));\n dp[t] = (dp[t] + dp[j]) % 1000000007;\n }\n s = Math.min(s + x, 2 * k);\n }\n return dp[k];\n }\n}\n```\n```Python3 []\nclass Solution:\n def countPartitions(self, nums: List[int], k: int) -> int:\n dp = [1] + [0] * k\n s = 0\n for x in nums:\n for j in range(min(k, s), -1, -1):\n t = min(k, s - j, j + x)\n dp[t] = (dp[t] + dp[j]) % 1000000007\n s += x\n return dp[k]\n``` | 3 | You are given an array `nums` consisting of **positive** integers and an integer `k`.
**Partition** the array into two ordered **groups** such that each element is in exactly **one** group. A partition is called great if the **sum** of elements of each group is greater than or equal to `k`.
Return _the number of **distinct** great partitions_. Since the answer may be too large, return it **modulo** `109 + 7`.
Two partitions are considered distinct if some element `nums[i]` is in different groups in the two partitions.
**Example 1:**
**Input:** nums = \[1,2,3,4\], k = 4
**Output:** 6
**Explanation:** The great partitions are: (\[1,2,3\], \[4\]), (\[1,3\], \[2,4\]), (\[1,4\], \[2,3\]), (\[2,3\], \[1,4\]), (\[2,4\], \[1,3\]) and (\[4\], \[1,2,3\]).
**Example 2:**
**Input:** nums = \[3,3,3\], k = 4
**Output:** 0
**Explanation:** There are no great partitions for this array.
**Example 3:**
**Input:** nums = \[6,6\], k = 2
**Output:** 2
**Explanation:** We can either put nums\[0\] in the first partition or in the second partition.
The great partitions will be (\[6\], \[6\]) and (\[6\], \[6\]).
**Constraints:**
* `1 <= nums.length, k <= 1000`
* `1 <= nums[i] <= 109` | null |
Python Knapsack beats 100% | number-of-great-partitions | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIt says the number can grow really big, which leads me thinking about DP, and from there goes to Knapsack problem.\n\nAnd given the constraint `1 <= nums.length, k <= 1000`, I guess this is a 2 dimensional dp (with 2 loops).\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThere are in total `2^n` combinations, if we can find the ones that do not suffice the condition, the combinations of `total sum < k`, then we can get the result by substraction. (I originally tried to get the `#combinations` that are greater than k, the number would grow too big and TLE).\n\nIn this case, the Knapsack\'s capacity is `k-1` (less than `k`), and we have `n` items. `dp[i][j]` means the total number of combinations at `ith` item given capacity `j`.\n\n# Complexity\n- Time complexity: `O(nk)`\n\n- Space complexity: `O(nk)`\n\n# Code\n```\nclass Solution:\n def countPartitions(self, nums: List[int], k: int) -> int:\n M = 10**9+7\n s, n = sum(nums), len(nums)\n if s < 2*k:\n return 0\n\n dp = [[0 for _ in range(k)] for _ in range(n)]\n for i, v in enumerate(nums):\n for j in range(k):\n if i == 0:\n if j - v >= 0:\n dp[i][j] = 2 # one can either put or not put the item\n else:\n dp[i][j] = 1 # can only not put the item\n continue\n dp[i][j] = dp[i-1][j]\n if j - v >= 0:\n dp[i][j] += dp[i-1][j-v]\n return (2**n - 2*dp[n-1][k-1]) % M\n \n``` | 2 | You are given an array `nums` consisting of **positive** integers and an integer `k`.
**Partition** the array into two ordered **groups** such that each element is in exactly **one** group. A partition is called great if the **sum** of elements of each group is greater than or equal to `k`.
Return _the number of **distinct** great partitions_. Since the answer may be too large, return it **modulo** `109 + 7`.
Two partitions are considered distinct if some element `nums[i]` is in different groups in the two partitions.
**Example 1:**
**Input:** nums = \[1,2,3,4\], k = 4
**Output:** 6
**Explanation:** The great partitions are: (\[1,2,3\], \[4\]), (\[1,3\], \[2,4\]), (\[1,4\], \[2,3\]), (\[2,3\], \[1,4\]), (\[2,4\], \[1,3\]) and (\[4\], \[1,2,3\]).
**Example 2:**
**Input:** nums = \[3,3,3\], k = 4
**Output:** 0
**Explanation:** There are no great partitions for this array.
**Example 3:**
**Input:** nums = \[6,6\], k = 2
**Output:** 2
**Explanation:** We can either put nums\[0\] in the first partition or in the second partition.
The great partitions will be (\[6\], \[6\]) and (\[6\], \[6\]).
**Constraints:**
* `1 <= nums.length, k <= 1000`
* `1 <= nums[i] <= 109` | null |
Memoization in Python, fewer than 10 lines | number-of-great-partitions | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nI am lazy so I implement the solution in memoization instead of bottom-up dynamic programming. In this simple 3-D dynamic programming, every state is defined by `(i, s1, s2)`, where i is the current number `nums[i]` to consider, `s1` and `s2` are the current sums of the first and the second arrays. The keys to getting accepted: \n\n* `s1` and `s2` are bounded by `k` as `k` can be much smaller than the sums can be made. \n* Traversing the numbers in the descending order. This reduces the search space greatly. \n\n# Code\n```\nclass Solution:\n def countPartitions(self, nums: List[int], k: int) -> int:\n n = len(nums)\n nums.sort(reverse=True)\n \n @cache\n def dp(i, s1, s2):\n if i >= n:\n return 1 if s1 >= k and s2 >= k else 0\n return (dp(i+1, min(k, s1+nums[i]), s2) + dp(i+1, s1, min(k, s2+nums[i]))) % 1000000007\n \n return dp(0, 0, 0) \n``` | 0 | You are given an array `nums` consisting of **positive** integers and an integer `k`.
**Partition** the array into two ordered **groups** such that each element is in exactly **one** group. A partition is called great if the **sum** of elements of each group is greater than or equal to `k`.
Return _the number of **distinct** great partitions_. Since the answer may be too large, return it **modulo** `109 + 7`.
Two partitions are considered distinct if some element `nums[i]` is in different groups in the two partitions.
**Example 1:**
**Input:** nums = \[1,2,3,4\], k = 4
**Output:** 6
**Explanation:** The great partitions are: (\[1,2,3\], \[4\]), (\[1,3\], \[2,4\]), (\[1,4\], \[2,3\]), (\[2,3\], \[1,4\]), (\[2,4\], \[1,3\]) and (\[4\], \[1,2,3\]).
**Example 2:**
**Input:** nums = \[3,3,3\], k = 4
**Output:** 0
**Explanation:** There are no great partitions for this array.
**Example 3:**
**Input:** nums = \[6,6\], k = 2
**Output:** 2
**Explanation:** We can either put nums\[0\] in the first partition or in the second partition.
The great partitions will be (\[6\], \[6\]) and (\[6\], \[6\]).
**Constraints:**
* `1 <= nums.length, k <= 1000`
* `1 <= nums[i] <= 109` | null |
Python (Simple DP) | number-of-great-partitions | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countPartitions(self, nums, k):\n n, mod = len(nums), 10**9+7\n\n @lru_cache(None)\n def dfs(i,total):\n if i == n:\n if total < k:\n return 1\n else:\n return 0\n\n count = 0\n\n if total + nums[i] < k:\n count += dfs(i+1,total+nums[i])\n \n count += dfs(i+1,total)\n\n return count%mod\n\n return max(0,(pow(2,n) - 2*dfs(0,0)))%mod\n\n\n\n\n``` | 0 | You are given an array `nums` consisting of **positive** integers and an integer `k`.
**Partition** the array into two ordered **groups** such that each element is in exactly **one** group. A partition is called great if the **sum** of elements of each group is greater than or equal to `k`.
Return _the number of **distinct** great partitions_. Since the answer may be too large, return it **modulo** `109 + 7`.
Two partitions are considered distinct if some element `nums[i]` is in different groups in the two partitions.
**Example 1:**
**Input:** nums = \[1,2,3,4\], k = 4
**Output:** 6
**Explanation:** The great partitions are: (\[1,2,3\], \[4\]), (\[1,3\], \[2,4\]), (\[1,4\], \[2,3\]), (\[2,3\], \[1,4\]), (\[2,4\], \[1,3\]) and (\[4\], \[1,2,3\]).
**Example 2:**
**Input:** nums = \[3,3,3\], k = 4
**Output:** 0
**Explanation:** There are no great partitions for this array.
**Example 3:**
**Input:** nums = \[6,6\], k = 2
**Output:** 2
**Explanation:** We can either put nums\[0\] in the first partition or in the second partition.
The great partitions will be (\[6\], \[6\]) and (\[6\], \[6\]).
**Constraints:**
* `1 <= nums.length, k <= 1000`
* `1 <= nums[i] <= 109` | null |
[Python3] Count the non-great partitions | number-of-great-partitions | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAs k is small(<=1000), we count the non-great partitions, which means to store all subsequence count no more than target. Counting the great partitions will get TLE. All partitions is *2 * (2 * (n - 1) - 1)*.\n\n# Code\n```\nfrom math import factorial\n\nclass Solution:\n def countPartitions(self, nums: List[int], target: int) -> int:\n s = sum(nums)\n if s < 2 * target: return 0\n cur = dict()\n for v in sorted(nums):\n if v >= target: break\n nxt = {v : 1}\n for k, t in cur.items():\n nxt[k] = nxt.get(k, 0) + t\n if k + v < target: nxt[k + v] = nxt.get(k + v, 0) + t\n cur = nxt\n return 2 * (2 ** (len(nums) - 1) - 1 - sum(v for v in cur.values())) % (10 ** 9 + 7)\n``` | 0 | You are given an array `nums` consisting of **positive** integers and an integer `k`.
**Partition** the array into two ordered **groups** such that each element is in exactly **one** group. A partition is called great if the **sum** of elements of each group is greater than or equal to `k`.
Return _the number of **distinct** great partitions_. Since the answer may be too large, return it **modulo** `109 + 7`.
Two partitions are considered distinct if some element `nums[i]` is in different groups in the two partitions.
**Example 1:**
**Input:** nums = \[1,2,3,4\], k = 4
**Output:** 6
**Explanation:** The great partitions are: (\[1,2,3\], \[4\]), (\[1,3\], \[2,4\]), (\[1,4\], \[2,3\]), (\[2,3\], \[1,4\]), (\[2,4\], \[1,3\]) and (\[4\], \[1,2,3\]).
**Example 2:**
**Input:** nums = \[3,3,3\], k = 4
**Output:** 0
**Explanation:** There are no great partitions for this array.
**Example 3:**
**Input:** nums = \[6,6\], k = 2
**Output:** 2
**Explanation:** We can either put nums\[0\] in the first partition or in the second partition.
The great partitions will be (\[6\], \[6\]) and (\[6\], \[6\]).
**Constraints:**
* `1 <= nums.length, k <= 1000`
* `1 <= nums[i] <= 109` | null |
Direct Counting DP O(NK) | number-of-great-partitions | 0 | 1 | # Approach\nThis solution does not count complement subsets like other solutions, and instead count the desired subsets directly with a DP recursive function and memoization.\n\n```dp(index, curr_sum)``` represents state of deciding for whether or not to add ```index```th number to the first group knowing that the first\'s group sum is already ```curr_sum```.\n\nThe key point here is, we don\'t need to proceed anymore if we get a sum bigger or equal to ```k```.\n\n# Complexity\n```O(NK)``` Time complexity\n\n# Code\n```\nfrom itertools import accumulate\nfrom typing import List\n\n\nclass Solution:\n def countPartitions(self, nums: List[int], k: int) -> int:\n n = len(nums)\n s = sum(nums)\n mod = int(1e9 + 7)\n mem = [[-1 for _ in range(k)] for _ in range(n + 1)]\n prefix_sum = [0] + list(accumulate(nums))\n\n def dp(index, curr_sum):\n if index == n + 1:\n return int(curr_sum >= k and (s - curr_sum) >= k)\n\n if mem[index][curr_sum] != -1:\n return mem[index][curr_sum]\n\n mem[index][curr_sum] = dp(index + 1, curr_sum)\n if curr_sum + nums[index - 1] >= k:\n if prefix_sum[index] - (curr_sum + nums[index - 1]) >= k:\n mem[index][curr_sum] += pow(2, (n - index), mod)\n else:\n mem[index][curr_sum] += dp(index + 1, curr_sum + nums[index - 1])\n return mem[index][curr_sum]\n return (2 * dp(1, 0)) % mod\n``` | 0 | You are given an array `nums` consisting of **positive** integers and an integer `k`.
**Partition** the array into two ordered **groups** such that each element is in exactly **one** group. A partition is called great if the **sum** of elements of each group is greater than or equal to `k`.
Return _the number of **distinct** great partitions_. Since the answer may be too large, return it **modulo** `109 + 7`.
Two partitions are considered distinct if some element `nums[i]` is in different groups in the two partitions.
**Example 1:**
**Input:** nums = \[1,2,3,4\], k = 4
**Output:** 6
**Explanation:** The great partitions are: (\[1,2,3\], \[4\]), (\[1,3\], \[2,4\]), (\[1,4\], \[2,3\]), (\[2,3\], \[1,4\]), (\[2,4\], \[1,3\]) and (\[4\], \[1,2,3\]).
**Example 2:**
**Input:** nums = \[3,3,3\], k = 4
**Output:** 0
**Explanation:** There are no great partitions for this array.
**Example 3:**
**Input:** nums = \[6,6\], k = 2
**Output:** 2
**Explanation:** We can either put nums\[0\] in the first partition or in the second partition.
The great partitions will be (\[6\], \[6\]) and (\[6\], \[6\]).
**Constraints:**
* `1 <= nums.length, k <= 1000`
* `1 <= nums[i] <= 109` | null |
simplest way in python!! | count-the-digits-that-divide-a-number | 0 | 1 | \n# Code\n```\nclass Solution:\n def countDigits(self, num: int) -> int:\n if len(str(num)) == 1:\n return 1\n else:\n ans = 0\n for i in str(num):\n if num % int(i) == 0:\n ans += 1\n return ans\n``` | 1 | Given an integer `num`, return _the number of digits in `num` that divide_ `num`.
An integer `val` divides `nums` if `nums % val == 0`.
**Example 1:**
**Input:** num = 7
**Output:** 1
**Explanation:** 7 divides itself, hence the answer is 1.
**Example 2:**
**Input:** num = 121
**Output:** 2
**Explanation:** 121 is divisible by 1, but not 2. Since 1 occurs twice as a digit, we return 2.
**Example 3:**
**Input:** num = 1248
**Output:** 4
**Explanation:** 1248 is divisible by all of its digits, hence the answer is 4.
**Constraints:**
* `1 <= num <= 109`
* `num` does not contain `0` as one of its digits. | null |
python3 code | count-the-digits-that-divide-a-number | 0 | 1 | # Intuition\nThe method first creates a deep copy of the input integer using the deepcopy function from the copy module. It then initializes an empty list l, a variable count1 to zero.\n\nThe method then enters a while loop that continues until num is greater than zero. In each iteration, it computes the remainder of num divided by 10 and appends it to the list l. It also updates num to be the integer division of itself by 10.\n\nFinally, the method enters a for loop that iterates over each element in l. For each element, it checks if the remainder of w divided by the element is zero. If so, it increments count1.\n\nThe method then returns count1.\n# Approach\nThe code takes an integer num as input and counts the number of digits in num that are factors of num.\n\nTo do this, the code first creates a deep copy of the input integer using the deepcopy function from the copy module. It then initializes an empty list l, and a variable count1 to zero.\n\nThe code then enters a while loop that continues until num is greater than zero. In each iteration, it computes the remainder of num divided by 10 and appends it to the list l. It also updates num to be the integer division of itself by 10.\n\nThis effectively splits num into its individual digits and stores them in the list l.\n\nThe code then enters a for loop that iterates over each element in l. For each element, it checks if the remainder of w divided by the element is zero. If so, it increments count1. This effectively counts the number of digits in num that are factors of num.\n\nFinally, the code returns count1.\n\n# Complexity\n- Time complexity:\nO(logn)\n\n- Space complexity:\nO(logn)\n\n# Code\n```\nclass Solution:\n def countDigits(self, num: int) -> int:\n import copy\n w = copy.deepcopy(num)\n l=[]\n count1=0\n while(num>0):\n n=num%10\n l.append(n)\n num = num//10 \n for i in l:\n if(w%i==0):\n count1 = count1+1\n return count1\n \n``` | 1 | Given an integer `num`, return _the number of digits in `num` that divide_ `num`.
An integer `val` divides `nums` if `nums % val == 0`.
**Example 1:**
**Input:** num = 7
**Output:** 1
**Explanation:** 7 divides itself, hence the answer is 1.
**Example 2:**
**Input:** num = 121
**Output:** 2
**Explanation:** 121 is divisible by 1, but not 2. Since 1 occurs twice as a digit, we return 2.
**Example 3:**
**Input:** num = 1248
**Output:** 4
**Explanation:** 1248 is divisible by all of its digits, hence the answer is 4.
**Constraints:**
* `1 <= num <= 109`
* `num` does not contain `0` as one of its digits. | null |
Python code for counting the digits that divide a number (TC: O(n) & SC: O(1)) | count-the-digits-that-divide-a-number | 0 | 1 | \n\n# Complexity\n- Time complexity:\nO(d), d is the number of digits.\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def countDigits(self, num: int) -> int:\n count = 0\n for i in str(num):\n if int(num)%int(i) == 0:\n count = count+1\n return count\n``` | 1 | Given an integer `num`, return _the number of digits in `num` that divide_ `num`.
An integer `val` divides `nums` if `nums % val == 0`.
**Example 1:**
**Input:** num = 7
**Output:** 1
**Explanation:** 7 divides itself, hence the answer is 1.
**Example 2:**
**Input:** num = 121
**Output:** 2
**Explanation:** 121 is divisible by 1, but not 2. Since 1 occurs twice as a digit, we return 2.
**Example 3:**
**Input:** num = 1248
**Output:** 4
**Explanation:** 1248 is divisible by all of its digits, hence the answer is 4.
**Constraints:**
* `1 <= num <= 109`
* `num` does not contain `0` as one of its digits. | null |
Python | Easy Solution✅ | count-the-digits-that-divide-a-number | 0 | 1 | # Code\u2705\nSolution 1:\n```\nclass Solution:\n def countDigits(self, num: int) -> int:\n count = [digit for digit in str(num) if num % int(digit) == 0]\n return len(count)\n```\nSolution 2:\n```\nclass Solution:\n def countDigits(self, num: int) -> int:\n count = 0\n for digit in str(num):\n if num % int(digit) == 0:\n count +=1\n return count\n``` | 4 | Given an integer `num`, return _the number of digits in `num` that divide_ `num`.
An integer `val` divides `nums` if `nums % val == 0`.
**Example 1:**
**Input:** num = 7
**Output:** 1
**Explanation:** 7 divides itself, hence the answer is 1.
**Example 2:**
**Input:** num = 121
**Output:** 2
**Explanation:** 121 is divisible by 1, but not 2. Since 1 occurs twice as a digit, we return 2.
**Example 3:**
**Input:** num = 1248
**Output:** 4
**Explanation:** 1248 is divisible by all of its digits, hence the answer is 4.
**Constraints:**
* `1 <= num <= 109`
* `num` does not contain `0` as one of its digits. | null |
simplest solution in python | count-the-digits-that-divide-a-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countDigits(self, num: int) -> int:\n temp=0\n st=str(num)\n for i in st:\n if num%int(i)==0:\n temp+=1\n return temp\n``` | 1 | Given an integer `num`, return _the number of digits in `num` that divide_ `num`.
An integer `val` divides `nums` if `nums % val == 0`.
**Example 1:**
**Input:** num = 7
**Output:** 1
**Explanation:** 7 divides itself, hence the answer is 1.
**Example 2:**
**Input:** num = 121
**Output:** 2
**Explanation:** 121 is divisible by 1, but not 2. Since 1 occurs twice as a digit, we return 2.
**Example 3:**
**Input:** num = 1248
**Output:** 4
**Explanation:** 1248 is divisible by all of its digits, hence the answer is 4.
**Constraints:**
* `1 <= num <= 109`
* `num` does not contain `0` as one of its digits. | null |
[Easy And Concise] | | Beats 93.43% ✅ | distinct-prime-factors-of-product-of-array | 0 | 1 | \n# Complexity\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n- Time complexity: **O(n * \u221An)**\n\n- Space complexity: **O(k)** *{k is distinct primes}*\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n\n``` C++ []\nclass Solution {\nprivate:\n void findprimefactors(int n){\n for(int i = 2;i * i <= n;i++){\n while(n % i == 0){\n mp[i]++; // first factor of a number is always prime \n n /= i;\n }\n // mp[n / i]++; --> this one is not goin to be prime\n }\n if(n > 1) mp[n]++; // e.g if n = 24 \n }\npublic:\n unordered_map<int,int> mp;\n int distinctPrimeFactors(vector<int>& v) {\n for(auto x: v){\n findprimefactors(x);\n }\n return mp.size();\n }\n};\n```\n``` Python []\nfrom collections import defaultdict\n\nclass Solution:\n def __init__(self):\n self.mp = defaultdict(int)\n\n def find_prime_factors(self, n):\n i = 2\n while i * i <= n:\n while n % i == 0:\n self.mp[i] += 1\n n //= i\n i += 1\n if n > 1:\n self.mp[n] += 1\n\n def distinct_prime_factors(self, v):\n for x in v:\n self.find_prime_factors(x)\n return len(self.mp)\n```\n\n\n# Plz Upvote Guys!! | 2 | Given an array of positive integers `nums`, return _the number of **distinct prime factors** in the product of the elements of_ `nums`.
**Note** that:
* A number greater than `1` is called **prime** if it is divisible by only `1` and itself.
* An integer `val1` is a factor of another integer `val2` if `val2 / val1` is an integer.
**Example 1:**
**Input:** nums = \[2,4,3,7,10,6\]
**Output:** 4
**Explanation:**
The product of all the elements in nums is: 2 \* 4 \* 3 \* 7 \* 10 \* 6 = 10080 = 25 \* 32 \* 5 \* 7.
There are 4 distinct prime factors so we return 4.
**Example 2:**
**Input:** nums = \[2,4,8,16\]
**Output:** 1
**Explanation:**
The product of all the elements in nums is: 2 \* 4 \* 8 \* 16 = 1024 = 210.
There is 1 distinct prime factor so we return 1.
**Constraints:**
* `1 <= nums.length <= 104`
* `2 <= nums[i] <= 1000` | null |
sieve || python | distinct-prime-factors-of-product-of-array | 0 | 1 | # Intuition and Approach\n* use sieve to find the prime numbers between 1 to 1000.\n* then return the no. of prime no. among them which can perfeclty divide any no. of the given list\n\n# Complexity\n- Time complexity:O(n)\n\n- Space complexity:o(1)\n\n# Code\n```\nclass Solution:\n p=[]\n def __init__(self):\n if(len(self.p)!=0):return\n self.s=[1 for i in range(1001)]\n for i in range(2,1001):\n if(not self.s[i]):continue\n self.p.append(i)\n for j in range(i*i,1001,i):\n self.s[j]=0\n \n def distinctPrimeFactors(self, nums: List[int]) -> int:\n # print(self.p)\n ans=0\n for i in range(len(self.p)):\n for j in range(len(nums)):\n if(nums[j]%self.p[i]==0):\n # print(i,j,nums[j],self.p[i])\n ans+=1\n break\n return ans\n``` | 1 | Given an array of positive integers `nums`, return _the number of **distinct prime factors** in the product of the elements of_ `nums`.
**Note** that:
* A number greater than `1` is called **prime** if it is divisible by only `1` and itself.
* An integer `val1` is a factor of another integer `val2` if `val2 / val1` is an integer.
**Example 1:**
**Input:** nums = \[2,4,3,7,10,6\]
**Output:** 4
**Explanation:**
The product of all the elements in nums is: 2 \* 4 \* 3 \* 7 \* 10 \* 6 = 10080 = 25 \* 32 \* 5 \* 7.
There are 4 distinct prime factors so we return 4.
**Example 2:**
**Input:** nums = \[2,4,8,16\]
**Output:** 1
**Explanation:**
The product of all the elements in nums is: 2 \* 4 \* 8 \* 16 = 1024 = 210.
There is 1 distinct prime factor so we return 1.
**Constraints:**
* `1 <= nums.length <= 104`
* `2 <= nums[i] <= 1000` | null |
[ Python ] ✅✅ Simple Python Solution Using Set | 100 % faster🥳✌👍 | distinct-prime-factors-of-product-of-array | 0 | 1 | # If You like the Solution, Don\'t Forget To UpVote Me, Please UpVote! \uD83D\uDD3C\uD83D\uDE4F\n# Runtime: 351 ms, faster than 100.00% of Python3 online submissions for Distinct Prime Factors of Product of Array.\n# Memory Usage: 15.2 MB, less than 100.00% of Python3 online submissions for Distinct Prime Factors of Product of Array.\n\n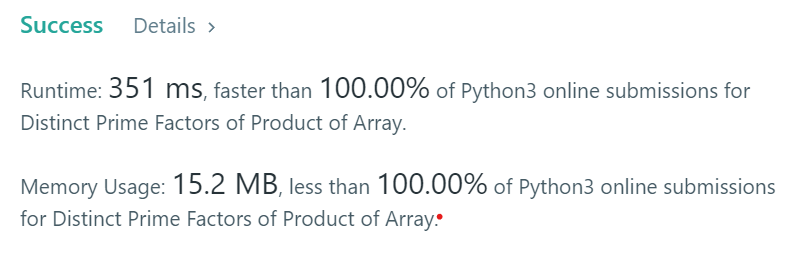\n\n\n\tclass Solution:\n\t\tdef distinctPrimeFactors(self, nums: List[int]) -> int:\n\n\t\t\tresult = []\n\n\t\t\tfor i in range (len(nums)) :\n\n\t\t\t\tsquare_root = int(math.sqrt(nums[i]))\n\n\t\t\t\tfor prime_num in range(2, square_root + 1) :\n\n\t\t\t\t\tif (nums[i] % prime_num == 0) :\n\n\t\t\t\t\t\tresult.append(prime_num)\n\n\t\t\t\t\t\twhile (nums[i] % prime_num == 0) :\n\t\t\t\t\t\t\tnums[i] = nums[i] // prime_num\n\n\t\t\t\tif (nums[i] >= 2) :\n\t\t\t\t\tresult.append(nums[i])\n\n\t\t\tresult = set(result)\n\t\t\treturn len(result)\n\t\t\t\n# Thank You \uD83E\uDD73\u270C\uD83D\uDC4D\n | 12 | Given an array of positive integers `nums`, return _the number of **distinct prime factors** in the product of the elements of_ `nums`.
**Note** that:
* A number greater than `1` is called **prime** if it is divisible by only `1` and itself.
* An integer `val1` is a factor of another integer `val2` if `val2 / val1` is an integer.
**Example 1:**
**Input:** nums = \[2,4,3,7,10,6\]
**Output:** 4
**Explanation:**
The product of all the elements in nums is: 2 \* 4 \* 3 \* 7 \* 10 \* 6 = 10080 = 25 \* 32 \* 5 \* 7.
There are 4 distinct prime factors so we return 4.
**Example 2:**
**Input:** nums = \[2,4,8,16\]
**Output:** 1
**Explanation:**
The product of all the elements in nums is: 2 \* 4 \* 8 \* 16 = 1024 = 210.
There is 1 distinct prime factor so we return 1.
**Constraints:**
* `1 <= nums.length <= 104`
* `2 <= nums[i] <= 1000` | null |
Python | O(nsqrt(n)) | distinct-prime-factors-of-product-of-array | 0 | 1 | # Complexity\n- Time complexity: O(n*sqrt(n))\n- Space complexity: O(n)\n\n# Code\n```\nclass Solution:\n def distinctPrimeFactors(self, nums: List[int]) -> int: \n def prime(a):\n ans = set() \n for i in range (len(a)) :\n if a[i] == 2:\n ans.add(2)\n continue\n sqr = int(math.sqrt(a[i]))\n for j in range(2, sqr+1) :\n if (a[i] % j == 0) :\n ans.add(j)\n while (a[i] % j == 0) :\n a[i] //= j\n if (a[i] > 2) :\n ans.add(a[i])\n return len(ans)\n\n return prime(nums)\n```\n---------------\n**Upvote the post if you find it helpful.\nHappy coding.** | 1 | Given an array of positive integers `nums`, return _the number of **distinct prime factors** in the product of the elements of_ `nums`.
**Note** that:
* A number greater than `1` is called **prime** if it is divisible by only `1` and itself.
* An integer `val1` is a factor of another integer `val2` if `val2 / val1` is an integer.
**Example 1:**
**Input:** nums = \[2,4,3,7,10,6\]
**Output:** 4
**Explanation:**
The product of all the elements in nums is: 2 \* 4 \* 3 \* 7 \* 10 \* 6 = 10080 = 25 \* 32 \* 5 \* 7.
There are 4 distinct prime factors so we return 4.
**Example 2:**
**Input:** nums = \[2,4,8,16\]
**Output:** 1
**Explanation:**
The product of all the elements in nums is: 2 \* 4 \* 8 \* 16 = 1024 = 210.
There is 1 distinct prime factor so we return 1.
**Constraints:**
* `1 <= nums.length <= 104`
* `2 <= nums[i] <= 1000` | null |
python3 solution ||100% Faster Solution | distinct-prime-factors-of-product-of-array | 0 | 1 | \n```\nclass Solution:\n def distinctPrimeFactors(self, nums: List[int]) -> int:\n s=set()\n for x in nums:\n t=2\n while t*t<=x:\n while x%t==0:\n x//=t\n s.add(t)\n\n t+=1\n\n if x>1:\n s.add(x)\n\n return len(s) \n``` | 2 | Given an array of positive integers `nums`, return _the number of **distinct prime factors** in the product of the elements of_ `nums`.
**Note** that:
* A number greater than `1` is called **prime** if it is divisible by only `1` and itself.
* An integer `val1` is a factor of another integer `val2` if `val2 / val1` is an integer.
**Example 1:**
**Input:** nums = \[2,4,3,7,10,6\]
**Output:** 4
**Explanation:**
The product of all the elements in nums is: 2 \* 4 \* 3 \* 7 \* 10 \* 6 = 10080 = 25 \* 32 \* 5 \* 7.
There are 4 distinct prime factors so we return 4.
**Example 2:**
**Input:** nums = \[2,4,8,16\]
**Output:** 1
**Explanation:**
The product of all the elements in nums is: 2 \* 4 \* 8 \* 16 = 1024 = 210.
There is 1 distinct prime factor so we return 1.
**Constraints:**
* `1 <= nums.length <= 104`
* `2 <= nums[i] <= 1000` | null |
Easy Python solution with proper comments. | distinct-prime-factors-of-product-of-array | 0 | 1 | # Intuition\nThe approch is to calculate all prime factors of every number of nums and the adding the factors to a set for getting unique prime factors.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n O(n*k)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n O(n)\n\n# Code\n```\nclass Solution:\n def distinctPrimeFactors(self, nums: List[int]) -> int:\n def factors(nums): #function to calculate prime factors\n z = 2\n s=[] # list to store prime factors\n while(nums>1) :\n if(nums%z==0):\n nums//=z\n s.append(z)\n else:\n z+=1\n return s\n\n s=set() #creating set to store the unique factors\n for k in nums:\n z = factors(k) # calculating current kth numbers factors \n for j in z:\n s.add(j) #adding factors to the set\n \n\n return len(s) #returning the length of set as ans.\n``` | 2 | Given an array of positive integers `nums`, return _the number of **distinct prime factors** in the product of the elements of_ `nums`.
**Note** that:
* A number greater than `1` is called **prime** if it is divisible by only `1` and itself.
* An integer `val1` is a factor of another integer `val2` if `val2 / val1` is an integer.
**Example 1:**
**Input:** nums = \[2,4,3,7,10,6\]
**Output:** 4
**Explanation:**
The product of all the elements in nums is: 2 \* 4 \* 3 \* 7 \* 10 \* 6 = 10080 = 25 \* 32 \* 5 \* 7.
There are 4 distinct prime factors so we return 4.
**Example 2:**
**Input:** nums = \[2,4,8,16\]
**Output:** 1
**Explanation:**
The product of all the elements in nums is: 2 \* 4 \* 8 \* 16 = 1024 = 210.
There is 1 distinct prime factor so we return 1.
**Constraints:**
* `1 <= nums.length <= 104`
* `2 <= nums[i] <= 1000` | null |
[Python3] Greedy O(N) Solution, Clean & Concise | partition-string-into-substrings-with-values-at-most-k | 0 | 1 | # Approach\nWe scan all digits in `s` from left to right, and add 1 to `ans` whenever the current value of the segment exceeds `k`.\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimumPartition(self, s: str, k: int) -> int:\n curr, ans = 0, 1\n for d in s:\n if int(d) > k:\n return -1\n curr = 10 * curr + int(d)\n if curr > k:\n ans += 1\n curr = int(d)\n return ans\n``` | 4 | You are given a string `s` consisting of digits from `1` to `9` and an integer `k`.
A partition of a string `s` is called **good** if:
* Each digit of `s` is part of **exactly** one substring.
* The value of each substring is less than or equal to `k`.
Return _the **minimum** number of substrings in a **good** partition of_ `s`. If no **good** partition of `s` exists, return `-1`.
**Note** that:
* The **value** of a string is its result when interpreted as an integer. For example, the value of `"123 "` is `123` and the value of `"1 "` is `1`.
* A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "165462 ", k = 60
**Output:** 4
**Explanation:** We can partition the string into substrings "16 ", "54 ", "6 ", and "2 ". Each substring has a value less than or equal to k = 60.
It can be shown that we cannot partition the string into less than 4 substrings.
**Example 2:**
**Input:** s = "238182 ", k = 5
**Output:** -1
**Explanation:** There is no good partition for this string.
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is a digit from `'1'` to `'9'`.
* `1 <= k <= 109`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
Beats 100% | Explanation | O(n) | CodeDominar Solution | partition-string-into-substrings-with-values-at-most-k | 0 | 1 | # Code\n```\nclass Solution:\n def minimumPartition(self, s: str, k: int) -> int:\n # Initialize a temporary string to store the current substring\n tmp = \'\'\n \n # Initialize a counter for the number of substrings\n cnt = 0\n \n # Iterate through the characters in the string s\n for c in s:\n # Add the current character to the temporary string\n tmp += c\n \n # If the value of the temporary string is greater than k, reset the temporary string to the current character\n # and increment the counter for the number of substrings\n if int(tmp) > k:\n tmp = c\n \n # If the value of the temporary string is still greater than k, return -1\n if int(tmp) > k:\n return -1\n \n cnt += 1\n \n # Return the count of substrings plus one for the final substring\n return cnt + 1\n\n \n``` | 2 | You are given a string `s` consisting of digits from `1` to `9` and an integer `k`.
A partition of a string `s` is called **good** if:
* Each digit of `s` is part of **exactly** one substring.
* The value of each substring is less than or equal to `k`.
Return _the **minimum** number of substrings in a **good** partition of_ `s`. If no **good** partition of `s` exists, return `-1`.
**Note** that:
* The **value** of a string is its result when interpreted as an integer. For example, the value of `"123 "` is `123` and the value of `"1 "` is `1`.
* A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "165462 ", k = 60
**Output:** 4
**Explanation:** We can partition the string into substrings "16 ", "54 ", "6 ", and "2 ". Each substring has a value less than or equal to k = 60.
It can be shown that we cannot partition the string into less than 4 substrings.
**Example 2:**
**Input:** s = "238182 ", k = 5
**Output:** -1
**Explanation:** There is no good partition for this string.
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is a digit from `'1'` to `'9'`.
* `1 <= k <= 109`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
Two Pointers | Explanation | partition-string-into-substrings-with-values-at-most-k | 0 | 1 | # Intuition\n\n1. Calculate the length of number `k` - > `l`\n2. Go though `s` from end to start\n a. `k` can be bigger then a number with the same length and 100% bigger then a number with `l-1` length\n b. Convert last `l` symbols to number and compare to `k`\n c. `l-1` can be equal to `0` which means no good partition\n d. Select the next last `l` symbols\n\n# Code\n```\nclass Solution:\n def minimumPartition(self, s: str, k: int) -> int:\n n = len(s)\n l = self.getIntLen(k)\n if l > n:\n return -1\n result = 0\n j = n\n i = n - l\n while i >= 0 and j > 0:\n if int(s[i:j]) <= k:\n result += 1\n i -= l\n j -= l\n elif j - i - 1 > 0:\n result += 1\n i -= l - 1\n j -= l - 1\n else:\n return -1\n i = max(i, 0)\n return result\n\n def getIntLen(self, n: int):\n result = 0\n while n > 0:\n n //= 10\n result += 1\n return result\n \n``` | 1 | You are given a string `s` consisting of digits from `1` to `9` and an integer `k`.
A partition of a string `s` is called **good** if:
* Each digit of `s` is part of **exactly** one substring.
* The value of each substring is less than or equal to `k`.
Return _the **minimum** number of substrings in a **good** partition of_ `s`. If no **good** partition of `s` exists, return `-1`.
**Note** that:
* The **value** of a string is its result when interpreted as an integer. For example, the value of `"123 "` is `123` and the value of `"1 "` is `1`.
* A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "165462 ", k = 60
**Output:** 4
**Explanation:** We can partition the string into substrings "16 ", "54 ", "6 ", and "2 ". Each substring has a value less than or equal to k = 60.
It can be shown that we cannot partition the string into less than 4 substrings.
**Example 2:**
**Input:** s = "238182 ", k = 5
**Output:** -1
**Explanation:** There is no good partition for this string.
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is a digit from `'1'` to `'9'`.
* `1 <= k <= 109`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
[python3] Greedy solution for ref | partition-string-into-substrings-with-values-at-most-k | 0 | 1 | ```\nclass Solution:\n def minimumPartition(self, s: str, k: int) -> int:\n rolling = ""\n ans = 0\n \n for i in s: \n if int(rolling + i) > k and (rolling and int(rolling) <= k):\n rolling = i\n ans += 1\n else: \n rolling += i\n \n return (ans+1) if int(rolling) <= k else -1 | 1 | You are given a string `s` consisting of digits from `1` to `9` and an integer `k`.
A partition of a string `s` is called **good** if:
* Each digit of `s` is part of **exactly** one substring.
* The value of each substring is less than or equal to `k`.
Return _the **minimum** number of substrings in a **good** partition of_ `s`. If no **good** partition of `s` exists, return `-1`.
**Note** that:
* The **value** of a string is its result when interpreted as an integer. For example, the value of `"123 "` is `123` and the value of `"1 "` is `1`.
* A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "165462 ", k = 60
**Output:** 4
**Explanation:** We can partition the string into substrings "16 ", "54 ", "6 ", and "2 ". Each substring has a value less than or equal to k = 60.
It can be shown that we cannot partition the string into less than 4 substrings.
**Example 2:**
**Input:** s = "238182 ", k = 5
**Output:** -1
**Explanation:** There is no good partition for this string.
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is a digit from `'1'` to `'9'`.
* `1 <= k <= 109`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
Python | Greedy | 100% | partition-string-into-substrings-with-values-at-most-k | 0 | 1 | # Code\n```\nclass Solution:\n def minimumPartition(self, s: str, k: int) -> int:\n if k < 10 and int(max(s)) > k: return -1\n j = 0\n n = len(s)\n cur = 0\n res = 1\n while j < n:\n cur = 10*cur + int(s[j])\n if cur > k:\n cur = int(s[j])\n res += 1\n j += 1\n return res\n \n \n \n \n``` | 1 | You are given a string `s` consisting of digits from `1` to `9` and an integer `k`.
A partition of a string `s` is called **good** if:
* Each digit of `s` is part of **exactly** one substring.
* The value of each substring is less than or equal to `k`.
Return _the **minimum** number of substrings in a **good** partition of_ `s`. If no **good** partition of `s` exists, return `-1`.
**Note** that:
* The **value** of a string is its result when interpreted as an integer. For example, the value of `"123 "` is `123` and the value of `"1 "` is `1`.
* A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "165462 ", k = 60
**Output:** 4
**Explanation:** We can partition the string into substrings "16 ", "54 ", "6 ", and "2 ". Each substring has a value less than or equal to k = 60.
It can be shown that we cannot partition the string into less than 4 substrings.
**Example 2:**
**Input:** s = "238182 ", k = 5
**Output:** -1
**Explanation:** There is no good partition for this string.
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is a digit from `'1'` to `'9'`.
* `1 <= k <= 109`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
Python Greedy and DP approach | partition-string-into-substrings-with-values-at-most-k | 0 | 1 | # DP approach\n\n# Code\n```\ndef minimumPartition(self, s: str, k: int) -> int:\n n = len(s)\n dp = [-1] * n\n\n def dfs(ind):\n if ind >=n :\n return 0\n if dp[ind]!=-1:\n return dp[ind]\n\n partitions = math.inf\n curr = 0\n for i in range(ind, n):\n curr = int(s[ind:i+1])\n if curr <= k:\n partitions = min(1 + dfs(i+1), partitions)\n\n dp[ind] = partitions\n return partitions\n\n \n ans = dfs(0)\n\n return ans if ans != math.inf else -1\n```\nThe DP solution gets timed oput\n\n# Better Approach\nGreedy\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def minimumPartition(self, s: str, k: int) -> int:\n n = len(s)\n partitions = 0\n i = 0\n\n while i<n:\n j = i\n while j<n and int(s[i:j+1])<=k:\n j+=1\n \n if i == j and int(s[i]) > k:\n return -1\n i = j\n partitions+=1\n \n return partitions\n``` | 1 | You are given a string `s` consisting of digits from `1` to `9` and an integer `k`.
A partition of a string `s` is called **good** if:
* Each digit of `s` is part of **exactly** one substring.
* The value of each substring is less than or equal to `k`.
Return _the **minimum** number of substrings in a **good** partition of_ `s`. If no **good** partition of `s` exists, return `-1`.
**Note** that:
* The **value** of a string is its result when interpreted as an integer. For example, the value of `"123 "` is `123` and the value of `"1 "` is `1`.
* A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "165462 ", k = 60
**Output:** 4
**Explanation:** We can partition the string into substrings "16 ", "54 ", "6 ", and "2 ". Each substring has a value less than or equal to k = 60.
It can be shown that we cannot partition the string into less than 4 substrings.
**Example 2:**
**Input:** s = "238182 ", k = 5
**Output:** -1
**Explanation:** There is no good partition for this string.
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is a digit from `'1'` to `'9'`.
* `1 <= k <= 109`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
Easy Solution in O(n) time with proper Comments. | partition-string-into-substrings-with-values-at-most-k | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe Approch is to calculate the sum of digits continuously and if sum exceeds the value of k then me make a partition and again start calculating sum and make partitions.\n\n\n\n# Complexity\n- Time complexity: $$O(n)$$ where n is the lenght of the string.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimumPartition(self, s: str, k: int) -> int:\n chk=0 \n partition=1\n for i in range(len(s)):\n chk = chk * 10 + ord(s[i])-ord(\'0\') #adding the new digit to the chk\n if chk > k: # if chk is greater than k than we have to make a partition \n chk = ord(s[i])-ord(\'0\') # making new chk for next iteration \n if chk>k: \n return -1\n partition+=1 # counting the partition\n \n return partition \n \n\n``` | 2 | You are given a string `s` consisting of digits from `1` to `9` and an integer `k`.
A partition of a string `s` is called **good** if:
* Each digit of `s` is part of **exactly** one substring.
* The value of each substring is less than or equal to `k`.
Return _the **minimum** number of substrings in a **good** partition of_ `s`. If no **good** partition of `s` exists, return `-1`.
**Note** that:
* The **value** of a string is its result when interpreted as an integer. For example, the value of `"123 "` is `123` and the value of `"1 "` is `1`.
* A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "165462 ", k = 60
**Output:** 4
**Explanation:** We can partition the string into substrings "16 ", "54 ", "6 ", and "2 ". Each substring has a value less than or equal to k = 60.
It can be shown that we cannot partition the string into less than 4 substrings.
**Example 2:**
**Input:** s = "238182 ", k = 5
**Output:** -1
**Explanation:** There is no good partition for this string.
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is a digit from `'1'` to `'9'`.
* `1 <= k <= 109`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
Python | 39ms, Beat 100% | Queue | closest-prime-numbers-in-range | 0 | 1 | ```\nclass Solution:\n def closestPrimes(self, left: int, right: int) -> List[int]:\n def isPrime(n):\n if n < 2: return False\n for x in range(2, int(n**0.5) + 1):\n if n % x == 0:\n return False\n return True\n q = []\n diff = float(\'inf\')\n pair = [-1,-1]\n for i in range(left,right+1):\n if isPrime(i): \n q.append(i)\n while len(q)>=2:\n if abs(q[0]-q[1])<diff:\n pair=[q[0],q[1]]\n diff=abs(q[0]-q[1]) \n if diff<=2: return pair\n q.pop(0)\n return pair\n``` | 4 | Given two positive integers `left` and `right`, find the two integers `num1` and `num2` such that:
* `left <= nums1 < nums2 <= right` .
* `nums1` and `nums2` are both **prime** numbers.
* `nums2 - nums1` is the **minimum** amongst all other pairs satisfying the above conditions.
Return _the positive integer array_ `ans = [nums1, nums2]`. _If there are multiple pairs satisfying these conditions, return the one with the minimum_ `nums1` _value or_ `[-1, -1]` _if such numbers do not exist._
A number greater than `1` is called **prime** if it is only divisible by `1` and itself.
**Example 1:**
**Input:** left = 10, right = 19
**Output:** \[11,13\]
**Explanation:** The prime numbers between 10 and 19 are 11, 13, 17, and 19.
The closest gap between any pair is 2, which can be achieved by \[11,13\] or \[17,19\].
Since 11 is smaller than 17, we return the first pair.
**Example 2:**
**Input:** left = 4, right = 6
**Output:** \[-1,-1\]
**Explanation:** There exists only one prime number in the given range, so the conditions cannot be satisfied.
**Constraints:**
* `1 <= left <= right <= 106`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
Python 3 || 8 lines, w/ example || T/M: 7937 ms /35.8 MB | closest-prime-numbers-in-range | 0 | 1 | ```\nclass Solution:\n def closestPrimes(self, left: int, right: int) -> List[int]:\n \n right+= 1 # Example: right = 6 left = 15\n\n # Before sieve: \n prime = [False,False]+[True]*(right-2) # prime = [F,F,T,T,T,T,T,T,T,T,T,T,T,T,T,T]\n # 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5\n for i in range(2,isqrt(right)+1): # After sieve: \n for j in range(i * i, right, i): # prime = [F,F,T,T,F,T,F,T,F,F,F,T,F,T,F,F]\n prime[j] = False # 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5\n print(prime) # \n prime = [i for i,p in enumerate(prime) # prime = [ 7, 11, 13, ]\n if p and left<=i<=right] \n\n if len(prime) < 2: return [-1,-1] # <-- if not two primes, no solution\n\n return list(min(list(zip(prime,prime[1:])), # min(11-7, 13-11) = 13-11 --> [11, 13]\n key = lambda x: x[1]-x[0]))\n```\n[https://leetcode.com/problems/closest-prime-numbers-in-range/submissions/869311374/](http://)\n\nNot sure about time complexity. I think space complexity is *O*(*N*). | 4 | Given two positive integers `left` and `right`, find the two integers `num1` and `num2` such that:
* `left <= nums1 < nums2 <= right` .
* `nums1` and `nums2` are both **prime** numbers.
* `nums2 - nums1` is the **minimum** amongst all other pairs satisfying the above conditions.
Return _the positive integer array_ `ans = [nums1, nums2]`. _If there are multiple pairs satisfying these conditions, return the one with the minimum_ `nums1` _value or_ `[-1, -1]` _if such numbers do not exist._
A number greater than `1` is called **prime** if it is only divisible by `1` and itself.
**Example 1:**
**Input:** left = 10, right = 19
**Output:** \[11,13\]
**Explanation:** The prime numbers between 10 and 19 are 11, 13, 17, and 19.
The closest gap between any pair is 2, which can be achieved by \[11,13\] or \[17,19\].
Since 11 is smaller than 17, we return the first pair.
**Example 2:**
**Input:** left = 4, right = 6
**Output:** \[-1,-1\]
**Explanation:** There exists only one prime number in the given range, so the conditions cannot be satisfied.
**Constraints:**
* `1 <= left <= right <= 106`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
Python Sieve of Eratosthenes | closest-prime-numbers-in-range | 0 | 1 | This solution uses the Sieve of Eratosthenes to iterate through all of the prime numbers up to `right`.\n\nOnce you can do that, simply find the pair with the smallest range.\n\nThis could be made faster by stopping as soon as $$right - left == 2$$, but this is so fast it isn\'t really necessary.\n\n# Code\n```\nclass Solution:\n def closestPrimes(self, left: int, right: int) -> list[int]:\n sieve = [False] * (right+1)\n \n last_prime = -1\n result = [-1, -1]\n for p in range(2, right+1):\n if not sieve[p]:\n for p2 in range(p*2, right+1, p):\n sieve[p2] = True\n if left <= p <= right:\n if last_prime == -1:\n last_prime = p\n elif result == [-1, -1]:\n result = [last_prime, p]\n elif p - last_prime < result[1] - result[0]:\n result = [last_prime, p]\n last_prime = p\n return result\n\n``` | 3 | Given two positive integers `left` and `right`, find the two integers `num1` and `num2` such that:
* `left <= nums1 < nums2 <= right` .
* `nums1` and `nums2` are both **prime** numbers.
* `nums2 - nums1` is the **minimum** amongst all other pairs satisfying the above conditions.
Return _the positive integer array_ `ans = [nums1, nums2]`. _If there are multiple pairs satisfying these conditions, return the one with the minimum_ `nums1` _value or_ `[-1, -1]` _if such numbers do not exist._
A number greater than `1` is called **prime** if it is only divisible by `1` and itself.
**Example 1:**
**Input:** left = 10, right = 19
**Output:** \[11,13\]
**Explanation:** The prime numbers between 10 and 19 are 11, 13, 17, and 19.
The closest gap between any pair is 2, which can be achieved by \[11,13\] or \[17,19\].
Since 11 is smaller than 17, we return the first pair.
**Example 2:**
**Input:** left = 4, right = 6
**Output:** \[-1,-1\]
**Explanation:** There exists only one prime number in the given range, so the conditions cannot be satisfied.
**Constraints:**
* `1 <= left <= right <= 106`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
SieveOfEratosthenes + Sliding Window Python Easy Solution | closest-prime-numbers-in-range | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def closestPrimes(self, left: int, right: int) -> List[int]:\n \n def sieve(n):\n prime = [True] * (n+1)\n for i in range(2, n//2):\n if prime[i]:\n for jj in range(2*i, n+1, i):\n prime[jj] = False\n return [i for i in range(2, n+1) if prime[i]]\n \n primes = sieve(right)\n inRange = False\n minDiff = float("inf")\n ans = [-1, -1]\n \n for ind in range(0, len(primes)-1):\n if not inRange:\n if primes[ind] >= left:\n inRange = True\n \n if inRange:\n l = primes[ind]\n r = primes[ind+1]\n if (r-l) < minDiff:\n minDiff = r-l\n ans = [l, r]\n return ans\n \n \n \n \n``` | 1 | Given two positive integers `left` and `right`, find the two integers `num1` and `num2` such that:
* `left <= nums1 < nums2 <= right` .
* `nums1` and `nums2` are both **prime** numbers.
* `nums2 - nums1` is the **minimum** amongst all other pairs satisfying the above conditions.
Return _the positive integer array_ `ans = [nums1, nums2]`. _If there are multiple pairs satisfying these conditions, return the one with the minimum_ `nums1` _value or_ `[-1, -1]` _if such numbers do not exist._
A number greater than `1` is called **prime** if it is only divisible by `1` and itself.
**Example 1:**
**Input:** left = 10, right = 19
**Output:** \[11,13\]
**Explanation:** The prime numbers between 10 and 19 are 11, 13, 17, and 19.
The closest gap between any pair is 2, which can be achieved by \[11,13\] or \[17,19\].
Since 11 is smaller than 17, we return the first pair.
**Example 2:**
**Input:** left = 4, right = 6
**Output:** \[-1,-1\]
**Explanation:** There exists only one prime number in the given range, so the conditions cannot be satisfied.
**Constraints:**
* `1 <= left <= right <= 106`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
[Python 3] Sieve of Eratosthenes | closest-prime-numbers-in-range | 0 | 1 | ```\nclass Solution:\n def closestPrimes(self, left: int, right: int) -> List[int]:\n prime = [True for i in range(right + 1)]\n \n p = 2\n while p ** 2 <= right:\n if prime[p]:\n for i in range(p ** 2, right + 1, p):\n prime[i] = False\n p += 1\n \n prime = [i for i in range(len(prime)) if prime[i] and max(2, left) <= i <= right]\n \n res = [-1, -1]\n diff = inf\n \n for i in range(len(prime) - 1):\n if prime[i + 1] - prime[i] < diff:\n diff = prime[i + 1] - prime[i]\n res = [prime[i], prime[i + 1]]\n \n if diff <= 2:\n return res\n \n return res\n``` | 2 | Given two positive integers `left` and `right`, find the two integers `num1` and `num2` such that:
* `left <= nums1 < nums2 <= right` .
* `nums1` and `nums2` are both **prime** numbers.
* `nums2 - nums1` is the **minimum** amongst all other pairs satisfying the above conditions.
Return _the positive integer array_ `ans = [nums1, nums2]`. _If there are multiple pairs satisfying these conditions, return the one with the minimum_ `nums1` _value or_ `[-1, -1]` _if such numbers do not exist._
A number greater than `1` is called **prime** if it is only divisible by `1` and itself.
**Example 1:**
**Input:** left = 10, right = 19
**Output:** \[11,13\]
**Explanation:** The prime numbers between 10 and 19 are 11, 13, 17, and 19.
The closest gap between any pair is 2, which can be achieved by \[11,13\] or \[17,19\].
Since 11 is smaller than 17, we return the first pair.
**Example 2:**
**Input:** left = 4, right = 6
**Output:** \[-1,-1\]
**Explanation:** There exists only one prime number in the given range, so the conditions cannot be satisfied.
**Constraints:**
* `1 <= left <= right <= 106`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
Python | Twin Primes, No Sieve | closest-prime-numbers-in-range | 0 | 1 | # Intuition\n\n[Twin primes](https://en.wikipedia.org/wiki/Twin_prime) are quite common, so it won\'t take long to find some.\n\n# Approach\n\n**Lemma**: every range $$1 \\le L \\le R \\le 10^6$$ of length at least 1\'452 contains a pair of twin primes. _Proof_: run a sieve of Eratosthenes and see for yourself.\n\nIf there are fewer than 1\'452 numbers in $$[L, R]$$ then finding all primes in $$O((R - L) \\sqrt{R})$$ time is fast enough (takes roughly $$10^6$$ operations). Otherwise, you can stop once you encounter a twin pair, since no further gap will be smaller.\n\n_Note_: In practice, finding primes in a range $$[L, R]$$ is faster than $$O((R - L) \\sqrt{R})$$. For example, all even numbers require only one step to determine that they are composite. All numbers of the form $$6k+3$$ require only two steps, etc. Amortized analysis applies here.\n\n**Conjecture**: naively finding primes in a range $$[L, R]$$ takes $$O((R - L) \\sqrt R / \\log R)$$ time. Experimentally, for $$R \\le 10^6$$ the constant factor of this estimate is roughly 2. In other words, checking if a random number close to a million is prime takes on average $$2 \\sqrt{10^6} / \\log_2(10^6) \\approx 100$$ operations, which is much better than $$\\sqrt{10^6} = 1000$$.\n\n# Complexity\n\n- Time complexity: $$O(\\min(1\'452, R - L) \\sqrt R / \\log R)$$\n\n- Space complexity: $$O(\\min(1\'452, R - L) / \\log R)$$ due to [prime number theorem](https://en.wikipedia.org/wiki/Prime_number_theorem).\n\n# Code\n\n[submission link](https://leetcode.com/problems/closest-prime-numbers-in-range/submissions/868988755/).\n\n```\nimport math\n\n\ndef is_prime(num: int) -> bool:\n if num == 1:\n return False\n for divisor in range(2, math.floor(math.sqrt(num)) + 1):\n if num % divisor == 0:\n return False\n return True\n\n\nclass Solution:\n def closestPrimes(self, left: int, right: int) -> list[int]:\n primes = []\n for candidate in range(left, right + 1):\n if is_prime(candidate):\n if primes and candidate <= primes[-1] + 2:\n return [primes[-1], candidate] # twin or [2, 3]\n primes.append(candidate)\n \n gaps = ([primes[i - 1], primes[i]]\n for i in range(1, len(primes)))\n\n return min(gaps,\n key=lambda gap: (gap[1] - gap[0], gap[0]),\n default=[-1, -1])\n\n``` | 22 | Given two positive integers `left` and `right`, find the two integers `num1` and `num2` such that:
* `left <= nums1 < nums2 <= right` .
* `nums1` and `nums2` are both **prime** numbers.
* `nums2 - nums1` is the **minimum** amongst all other pairs satisfying the above conditions.
Return _the positive integer array_ `ans = [nums1, nums2]`. _If there are multiple pairs satisfying these conditions, return the one with the minimum_ `nums1` _value or_ `[-1, -1]` _if such numbers do not exist._
A number greater than `1` is called **prime** if it is only divisible by `1` and itself.
**Example 1:**
**Input:** left = 10, right = 19
**Output:** \[11,13\]
**Explanation:** The prime numbers between 10 and 19 are 11, 13, 17, and 19.
The closest gap between any pair is 2, which can be achieved by \[11,13\] or \[17,19\].
Since 11 is smaller than 17, we return the first pair.
**Example 2:**
**Input:** left = 4, right = 6
**Output:** \[-1,-1\]
**Explanation:** There exists only one prime number in the given range, so the conditions cannot be satisfied.
**Constraints:**
* `1 <= left <= right <= 106`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
Brute force approach but terminate if you find a pair of primes whose difference is two | closest-prime-numbers-in-range | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIf you look at the list of primes, you will notice that there\nare many pairs of primes whose difference is two. \n\nFor example (3,5),(11,13),(101,103),....\n\nA pair of primes whose difference is two is called a twin prime.\nIf you are given a table of primes, you\'ll probably find a twin prime, even if the primes get big.\n\nI also knew that whether there are infinitely many twin primes is still unsolved. Thus, I thought there may be a lot of twin primes. The larger the difference between right and left, the more likely we will find a twin pair.\n\nThus, I simply searched all primes from left to right, keep track of the diff, and check if it is minimal; a brute force approach. However, if I find a twin prime, terminate. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe only time the difference between two primes equals one is (2,3). Handle this edge case first. Then brute force as written above.\n\n# Notes\n\nI realize this approach just worked luckily; If n=right-left, the time complexity is O(n sqrt(n)) which generally causes TLE. Moreover, I don\'t know if there is a mathematical theorem that gives info about how frequently twin primes appear. It just so worked.\n\nHowever, I wanted to share it because I haven\'t used complex algorithms.\n\nHave a nice day!\n\n# Complexity\nIf n = right - left,\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nWorst case: O(n * sqrt(n))\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(n)\n# Code\n```\nclass Solution:\n def closestPrimes(self, left: int, right: int) -> List[int]:\n if (left == 1 and right >=3): return [2,3]\n if (left ==2 and right >=3): return[2,3]\n if (left == 1 and right <=2):return [-1,-1]\n if (left == right): return [-1,-1]\n def isPrime(n):\n if (n==2): return True\n sq = int(math.sqrt(n))+1\n for i in range(2,sq):\n if (n %i == 0): return False\n return True\n\n primes = []\n minDiff = 99999999999999999999\n output = [-1,-1]\n \n for i in range(left,right+1):\n if (isPrime(i) == True):\n primes.append(i)\n if (len(primes)>1):\n d = i - primes[-2]\n if (d==2):\n return [primes[-2],i]\n else:\n if (d < minDiff):\n minDiff = d\n output[0] = primes[-2]\n output[1] = primes[-1]\n return output\n``` | 1 | Given two positive integers `left` and `right`, find the two integers `num1` and `num2` such that:
* `left <= nums1 < nums2 <= right` .
* `nums1` and `nums2` are both **prime** numbers.
* `nums2 - nums1` is the **minimum** amongst all other pairs satisfying the above conditions.
Return _the positive integer array_ `ans = [nums1, nums2]`. _If there are multiple pairs satisfying these conditions, return the one with the minimum_ `nums1` _value or_ `[-1, -1]` _if such numbers do not exist._
A number greater than `1` is called **prime** if it is only divisible by `1` and itself.
**Example 1:**
**Input:** left = 10, right = 19
**Output:** \[11,13\]
**Explanation:** The prime numbers between 10 and 19 are 11, 13, 17, and 19.
The closest gap between any pair is 2, which can be achieved by \[11,13\] or \[17,19\].
Since 11 is smaller than 17, we return the first pair.
**Example 2:**
**Input:** left = 4, right = 6
**Output:** \[-1,-1\]
**Explanation:** There exists only one prime number in the given range, so the conditions cannot be satisfied.
**Constraints:**
* `1 <= left <= right <= 106`
.spoilerbutton {display:block; border:dashed; padding: 0px 0px; margin:10px 0px; font-size:150%; font-weight: bold; color:#000000; background-color:cyan; outline:0; } .spoiler {overflow:hidden;} .spoiler > div {-webkit-transition: all 0s ease;-moz-transition: margin 0s ease;-o-transition: all 0s ease;transition: margin 0s ease;} .spoilerbutton\[value="Show Message"\] + .spoiler > div {margin-top:-500%;} .spoilerbutton\[value="Hide Message"\] + .spoiler {padding:5px;} | null |
EASY to UNDERSTAND || Conditional Logic | categorize-box-according-to-criteria | 0 | 1 | # Complexity\n- Time complexity: O(1)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n//Specifies a fixed set of possible values.\nfrom typing import Literal\n\nclass Solution:\n def categorizeBox(self, length: int, width: int, height: int, mass: int) -> Literal["Both", "Neither", "Bulky", "Heavy"]:\n //Preset thresholds for categorization\n bulkyLen = 10 ** 4\n bulkyVol = 10 ** 9\n heavyMass = 100\n\n //Calculates volume of box\n volume = length * width * height\n //Calculates if bulky\n bulky = max(length, width, height) >= bulkyLen or volume >= bulkyVol\n //Calculates if heavy\n heavy = mass >= heavyMass\n\n //Seperates results into categories\n if bulky and heavy:\n return "Both"\n elif not bulky and not heavy:\n return "Neither"\n elif bulky and not heavy:\n return "Bulky"\n elif not bulky and heavy:\n return "Heavy"\n``` | 1 | Given four integers `length`, `width`, `height`, and `mass`, representing the dimensions and mass of a box, respectively, return _a string representing the **category** of the box_.
* The box is `"Bulky "` if:
* **Any** of the dimensions of the box is greater or equal to `104`.
* Or, the **volume** of the box is greater or equal to `109`.
* If the mass of the box is greater or equal to `100`, it is `"Heavy ".`
* If the box is both `"Bulky "` and `"Heavy "`, then its category is `"Both "`.
* If the box is neither `"Bulky "` nor `"Heavy "`, then its category is `"Neither "`.
* If the box is `"Bulky "` but not `"Heavy "`, then its category is `"Bulky "`.
* If the box is `"Heavy "` but not `"Bulky "`, then its category is `"Heavy "`.
**Note** that the volume of the box is the product of its length, width and height.
**Example 1:**
**Input:** length = 1000, width = 35, height = 700, mass = 300
**Output:** "Heavy "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 24500000 <= 109. So it cannot be categorized as "Bulky ".
However mass >= 100, so the box is "Heavy ".
Since the box is not "Bulky " but "Heavy ", we return "Heavy ".
**Example 2:**
**Input:** length = 200, width = 50, height = 800, mass = 50
**Output:** "Neither "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 8 \* 106 <= 109. So it cannot be categorized as "Bulky ".
Its mass is also less than 100, so it cannot be categorized as "Heavy " either.
Since its neither of the two above categories, we return "Neither ".
**Constraints:**
* `1 <= length, width, height <= 105`
* `1 <= mass <= 103` | null |
Easy Solution | Java | Python | categorize-box-according-to-criteria | 1 | 1 | # Java\n```\nclass Solution {\n public boolean isBulky(int a, int b, int c, int d) {\n double val = Math.pow(10, 4);\n if(a >= val || b >= val || c >= val || d >= val) {\n return true;\n }\n else if((long) a * b * c >= Math.pow(10, 9)) {\n return true;\n }\n return false;\n }\n\n public boolean isHeavy(int mass) {\n if(mass >= 100) {\n return true;\n }\n return false;\n }\n\n public String categorizeBox(int length, int width, int height, int mass) {\n boolean x = isBulky(length, width, height, mass);\n boolean y = isHeavy(mass);\n System.out.println(x + " " + y);\n if(x && y) {\n return "Both";\n }\n else if(x && !(y)) {\n return "Bulky";\n }\n else if(y && !(x)) {\n return "Heavy";\n }\n else {\n return "Neither";\n }\n }\n}\n```\n# Python\n```\nclass Solution:\n def categorizeBox(self, length: int, width: int, height: int, mass: int) -> str:\n\n def isBulky(a, b, c, d):\n val = 10 ** 4\n\n if a >= val or b >= val or c >= val or d >= val:\n return True\n\n elif a * b * c >= (10 ** 9):\n return True\n\n return False\n\n def isHeavy(mass):\n if mass >= 100:\n return True\n\n return False\n \n x = isBulky(length, width, height, mass)\n y = isHeavy(mass)\n\n if x and y:\n return "Both"\n elif y and not x:\n return "Heavy"\n elif x and not y:\n return "Bulky"\n else:\n return "Neither"\n\n\n```\nDo upvote if you like the Solution :) | 1 | Given four integers `length`, `width`, `height`, and `mass`, representing the dimensions and mass of a box, respectively, return _a string representing the **category** of the box_.
* The box is `"Bulky "` if:
* **Any** of the dimensions of the box is greater or equal to `104`.
* Or, the **volume** of the box is greater or equal to `109`.
* If the mass of the box is greater or equal to `100`, it is `"Heavy ".`
* If the box is both `"Bulky "` and `"Heavy "`, then its category is `"Both "`.
* If the box is neither `"Bulky "` nor `"Heavy "`, then its category is `"Neither "`.
* If the box is `"Bulky "` but not `"Heavy "`, then its category is `"Bulky "`.
* If the box is `"Heavy "` but not `"Bulky "`, then its category is `"Heavy "`.
**Note** that the volume of the box is the product of its length, width and height.
**Example 1:**
**Input:** length = 1000, width = 35, height = 700, mass = 300
**Output:** "Heavy "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 24500000 <= 109. So it cannot be categorized as "Bulky ".
However mass >= 100, so the box is "Heavy ".
Since the box is not "Bulky " but "Heavy ", we return "Heavy ".
**Example 2:**
**Input:** length = 200, width = 50, height = 800, mass = 50
**Output:** "Neither "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 8 \* 106 <= 109. So it cannot be categorized as "Bulky ".
Its mass is also less than 100, so it cannot be categorized as "Heavy " either.
Since its neither of the two above categories, we return "Neither ".
**Constraints:**
* `1 <= length, width, height <= 105`
* `1 <= mass <= 103` | null |
categorize-box-according-to-criteria | categorize-box-according-to-criteria | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def categorizeBox(self, length: int, width: int, height: int, mass: int) -> str:\n volume = length*width*height\n if (volume >= 10**9 or height >= 10**4 or width >= 10**4 or length >= 10**4) and mass >=100:\n return ("Both")\n elif (volume < 10**9 or height < 10**4 or width < 10**4 or length < 10**4) and mass >=100:\n return ("Heavy")\n elif (volume >= 10**9 or height >= 10**4 or width >= 10**4 or length >= 10**4) and mass <100:\n return ("Bulky")\n else:\n return ("Neither")\n\n``` | 1 | Given four integers `length`, `width`, `height`, and `mass`, representing the dimensions and mass of a box, respectively, return _a string representing the **category** of the box_.
* The box is `"Bulky "` if:
* **Any** of the dimensions of the box is greater or equal to `104`.
* Or, the **volume** of the box is greater or equal to `109`.
* If the mass of the box is greater or equal to `100`, it is `"Heavy ".`
* If the box is both `"Bulky "` and `"Heavy "`, then its category is `"Both "`.
* If the box is neither `"Bulky "` nor `"Heavy "`, then its category is `"Neither "`.
* If the box is `"Bulky "` but not `"Heavy "`, then its category is `"Bulky "`.
* If the box is `"Heavy "` but not `"Bulky "`, then its category is `"Heavy "`.
**Note** that the volume of the box is the product of its length, width and height.
**Example 1:**
**Input:** length = 1000, width = 35, height = 700, mass = 300
**Output:** "Heavy "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 24500000 <= 109. So it cannot be categorized as "Bulky ".
However mass >= 100, so the box is "Heavy ".
Since the box is not "Bulky " but "Heavy ", we return "Heavy ".
**Example 2:**
**Input:** length = 200, width = 50, height = 800, mass = 50
**Output:** "Neither "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 8 \* 106 <= 109. So it cannot be categorized as "Bulky ".
Its mass is also less than 100, so it cannot be categorized as "Heavy " either.
Since its neither of the two above categories, we return "Neither ".
**Constraints:**
* `1 <= length, width, height <= 105`
* `1 <= mass <= 103` | null |
Easy solution. Beats 99 %. Match/Switch Case. Python. | categorize-box-according-to-criteria | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nUse match cases.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Create boolean variabls bulky and heavy.\n2. Set valus acording the task description.\n3. Make cases.\n4. Return a required value dependent on a particular case.\n# Complexity\n- Time complexity:$$O(1)$$ \n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(1)$$ \n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python []\nclass Solution:\n def categorizeBox(self, length: int, width: int, height: int, mass: int) -> str:\n bulky = False\n heavy = False\n\n if length >= 10**4 or width >= 10**4 or height >= 10**4 \\\n or (length * height * width) >= 10**9:\n bulky = True\n\n if mass >= 100:\n heavy = True\n\n match bulky, heavy:\n case bulky, heavy if bulky and heavy:\n return "Both"\n case bulky, heavy if not bulky and not heavy:\n return "Neither"\n case bulky, heavy if bulky and not heavy:\n return "Bulky"\n case bulky, heavy if not bulky and heavy:\n return "Heavy"\n \n return ""\n```\n | 0 | Given four integers `length`, `width`, `height`, and `mass`, representing the dimensions and mass of a box, respectively, return _a string representing the **category** of the box_.
* The box is `"Bulky "` if:
* **Any** of the dimensions of the box is greater or equal to `104`.
* Or, the **volume** of the box is greater or equal to `109`.
* If the mass of the box is greater or equal to `100`, it is `"Heavy ".`
* If the box is both `"Bulky "` and `"Heavy "`, then its category is `"Both "`.
* If the box is neither `"Bulky "` nor `"Heavy "`, then its category is `"Neither "`.
* If the box is `"Bulky "` but not `"Heavy "`, then its category is `"Bulky "`.
* If the box is `"Heavy "` but not `"Bulky "`, then its category is `"Heavy "`.
**Note** that the volume of the box is the product of its length, width and height.
**Example 1:**
**Input:** length = 1000, width = 35, height = 700, mass = 300
**Output:** "Heavy "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 24500000 <= 109. So it cannot be categorized as "Bulky ".
However mass >= 100, so the box is "Heavy ".
Since the box is not "Bulky " but "Heavy ", we return "Heavy ".
**Example 2:**
**Input:** length = 200, width = 50, height = 800, mass = 50
**Output:** "Neither "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 8 \* 106 <= 109. So it cannot be categorized as "Bulky ".
Its mass is also less than 100, so it cannot be categorized as "Heavy " either.
Since its neither of the two above categories, we return "Neither ".
**Constraints:**
* `1 <= length, width, height <= 105`
* `1 <= mass <= 103` | null |
Python3 | categorize-box-according-to-criteria | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n```\nCode block\n```\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def categorizeBox(self, length: int, width: int, height: int, mass: int) -> str:\n volume=length*width*height\n a=10**4\n b=10**9\n c=0\n d=0\n if (length>=a)or(width>=a)or(height>=a) or (volume>=b):\n c=1\n if mass>=100:\n d=1\n if c==1 and d==0:\n return \'Bulky\'\n elif c==0 and d==0:\n return \'Neither\'\n elif c==0 and d==1:\n return \'Heavy\'\n elif c==1 and d==1:\n return \'Both\'\n``` | 1 | Given four integers `length`, `width`, `height`, and `mass`, representing the dimensions and mass of a box, respectively, return _a string representing the **category** of the box_.
* The box is `"Bulky "` if:
* **Any** of the dimensions of the box is greater or equal to `104`.
* Or, the **volume** of the box is greater or equal to `109`.
* If the mass of the box is greater or equal to `100`, it is `"Heavy ".`
* If the box is both `"Bulky "` and `"Heavy "`, then its category is `"Both "`.
* If the box is neither `"Bulky "` nor `"Heavy "`, then its category is `"Neither "`.
* If the box is `"Bulky "` but not `"Heavy "`, then its category is `"Bulky "`.
* If the box is `"Heavy "` but not `"Bulky "`, then its category is `"Heavy "`.
**Note** that the volume of the box is the product of its length, width and height.
**Example 1:**
**Input:** length = 1000, width = 35, height = 700, mass = 300
**Output:** "Heavy "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 24500000 <= 109. So it cannot be categorized as "Bulky ".
However mass >= 100, so the box is "Heavy ".
Since the box is not "Bulky " but "Heavy ", we return "Heavy ".
**Example 2:**
**Input:** length = 200, width = 50, height = 800, mass = 50
**Output:** "Neither "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 8 \* 106 <= 109. So it cannot be categorized as "Bulky ".
Its mass is also less than 100, so it cannot be categorized as "Heavy " either.
Since its neither of the two above categories, we return "Neither ".
**Constraints:**
* `1 <= length, width, height <= 105`
* `1 <= mass <= 103` | null |
Python 3 || 2 lines, w/ explanation || T/M: 100% / 100% | categorize-box-according-to-criteria | 0 | 1 | Here\'s the plan: \n- We use this bit map for assigning a string:\n```\nBulky Heavy idx bits string\n\u2013\u2013\u2013\u2013\u2013 \u2013\u2013\u2013\u2013\u2013\u2013 \u2013\u2013\u2013\u2013\u2013\u2013\u2013 \u2013\u2013\u2013\u2013 \u2013\u2013\u2013\u2013\u2013\u2013\u2013\nFalse False 0+0 = 0 00 Neither\nTrue False 1+0 = 1 01 Bulky\nFalse True 0+2 = 2 10 Heavy\nTrue True 1+2 = 3 11 Both\n```\n- We use`idx` to address the correct string in a tuple of the possible strings `("Neither", "Bulky", "Heavy", "Both")`.\n```\nclass Solution:\n def categorizeBox(self, length: int, width: int, height: int, mass: int) -> str:\n\n idx = int(length >= 10000 or width >= 10000 or height >= 10000 or \n length * width * height >= 10**9) + 2*(mass >= 100)\n \n return ("Neither", "Bulky", "Heavy", "Both")[idx]\n```\n[https://leetcode.com/problems/categorize-box-according-to-criteria/submissions/874510700/]()\n\nI could be wrong, but I think that time complexity is O(1) and space complexity is O(1). | 33 | Given four integers `length`, `width`, `height`, and `mass`, representing the dimensions and mass of a box, respectively, return _a string representing the **category** of the box_.
* The box is `"Bulky "` if:
* **Any** of the dimensions of the box is greater or equal to `104`.
* Or, the **volume** of the box is greater or equal to `109`.
* If the mass of the box is greater or equal to `100`, it is `"Heavy ".`
* If the box is both `"Bulky "` and `"Heavy "`, then its category is `"Both "`.
* If the box is neither `"Bulky "` nor `"Heavy "`, then its category is `"Neither "`.
* If the box is `"Bulky "` but not `"Heavy "`, then its category is `"Bulky "`.
* If the box is `"Heavy "` but not `"Bulky "`, then its category is `"Heavy "`.
**Note** that the volume of the box is the product of its length, width and height.
**Example 1:**
**Input:** length = 1000, width = 35, height = 700, mass = 300
**Output:** "Heavy "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 24500000 <= 109. So it cannot be categorized as "Bulky ".
However mass >= 100, so the box is "Heavy ".
Since the box is not "Bulky " but "Heavy ", we return "Heavy ".
**Example 2:**
**Input:** length = 200, width = 50, height = 800, mass = 50
**Output:** "Neither "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 8 \* 106 <= 109. So it cannot be categorized as "Bulky ".
Its mass is also less than 100, so it cannot be categorized as "Heavy " either.
Since its neither of the two above categories, we return "Neither ".
**Constraints:**
* `1 <= length, width, height <= 105`
* `1 <= mass <= 103` | null |
Python || Simple If...Else | categorize-box-according-to-criteria | 0 | 1 | # Code\n```\nclass Solution:\n def categorizeBox(self, length: int, width: int, height: int, mass: int) -> str:\n if length>=(10**4) or width>=(10**4) or height>=(10**4) or length*width*height>=(10**9):\n if mass>=100:\n return "Both"\n else:\n return "Bulky"\n if mass>=100:\n return "Heavy"\n return "Neither" \n``` | 1 | Given four integers `length`, `width`, `height`, and `mass`, representing the dimensions and mass of a box, respectively, return _a string representing the **category** of the box_.
* The box is `"Bulky "` if:
* **Any** of the dimensions of the box is greater or equal to `104`.
* Or, the **volume** of the box is greater or equal to `109`.
* If the mass of the box is greater or equal to `100`, it is `"Heavy ".`
* If the box is both `"Bulky "` and `"Heavy "`, then its category is `"Both "`.
* If the box is neither `"Bulky "` nor `"Heavy "`, then its category is `"Neither "`.
* If the box is `"Bulky "` but not `"Heavy "`, then its category is `"Bulky "`.
* If the box is `"Heavy "` but not `"Bulky "`, then its category is `"Heavy "`.
**Note** that the volume of the box is the product of its length, width and height.
**Example 1:**
**Input:** length = 1000, width = 35, height = 700, mass = 300
**Output:** "Heavy "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 24500000 <= 109. So it cannot be categorized as "Bulky ".
However mass >= 100, so the box is "Heavy ".
Since the box is not "Bulky " but "Heavy ", we return "Heavy ".
**Example 2:**
**Input:** length = 200, width = 50, height = 800, mass = 50
**Output:** "Neither "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 8 \* 106 <= 109. So it cannot be categorized as "Bulky ".
Its mass is also less than 100, so it cannot be categorized as "Heavy " either.
Since its neither of the two above categories, we return "Neither ".
**Constraints:**
* `1 <= length, width, height <= 105`
* `1 <= mass <= 103` | null |
[C++|Java|Python3] if-else | categorize-box-according-to-criteria | 1 | 1 | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/7360be4d63ffa8b13518401baa628a6f6800d326) for solutions of weekly 95. \n\n**Intuition**\nThis is a `if-else` problem. \n**Implementation**\n**C++**\n```\nclass Solution {\npublic:\n string categorizeBox(int length, int width, int height, int mass) {\n bool bulky = max({length, width, height}) >= 1e4 || (long long) length*width*height >= 1e9, heavy = mass >= 100; \n if (bulky && heavy) return "Both"; \n if (bulky) return "Bulky"; \n if (heavy) return "Heavy"; \n return "Neither"; \n }\n};\n```\n**Java**\n```\nclass Solution {\n public String categorizeBox(int length, int width, int height, int mass) {\n boolean bulky = Math.max(length, Math.max(width, height)) >= 1e4 || (long) length*width*height >= 1e9, heavy = mass >= 100; \n if (bulky && heavy) return "Both"; \n if (bulky) return "Bulky"; \n if (heavy) return "Heavy"; \n return "Neither"; \n }\n}\n```\n**Python3**\n```\nclass Solution:\n def categorizeBox(self, length: int, width: int, height: int, mass: int) -> str:\n bulky = max(length, width, height) >= 1e4 or length*width*height >= 1e9\n heavy = mass >= 100 \n if bulky and heavy: return "Both"\n if bulky: return "Bulky"\n if heavy: return "Heavy"\n return "Neither"\n```\n**Complexity**\nTime `O(1)`\nSpace `O(1)` | 6 | Given four integers `length`, `width`, `height`, and `mass`, representing the dimensions and mass of a box, respectively, return _a string representing the **category** of the box_.
* The box is `"Bulky "` if:
* **Any** of the dimensions of the box is greater or equal to `104`.
* Or, the **volume** of the box is greater or equal to `109`.
* If the mass of the box is greater or equal to `100`, it is `"Heavy ".`
* If the box is both `"Bulky "` and `"Heavy "`, then its category is `"Both "`.
* If the box is neither `"Bulky "` nor `"Heavy "`, then its category is `"Neither "`.
* If the box is `"Bulky "` but not `"Heavy "`, then its category is `"Bulky "`.
* If the box is `"Heavy "` but not `"Bulky "`, then its category is `"Heavy "`.
**Note** that the volume of the box is the product of its length, width and height.
**Example 1:**
**Input:** length = 1000, width = 35, height = 700, mass = 300
**Output:** "Heavy "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 24500000 <= 109. So it cannot be categorized as "Bulky ".
However mass >= 100, so the box is "Heavy ".
Since the box is not "Bulky " but "Heavy ", we return "Heavy ".
**Example 2:**
**Input:** length = 200, width = 50, height = 800, mass = 50
**Output:** "Neither "
**Explanation:**
None of the dimensions of the box is greater or equal to 104.
Its volume = 8 \* 106 <= 109. So it cannot be categorized as "Bulky ".
Its mass is also less than 100, so it cannot be categorized as "Heavy " either.
Since its neither of the two above categories, we return "Neither ".
**Constraints:**
* `1 <= length, width, height <= 105`
* `1 <= mass <= 103` | null |
[python3] Sol for reference | find-consecutive-integers-from-a-data-stream | 0 | 1 | ```\nclass DataStream:\n\n def __init__(self, value: int, k: int):\n self.count = 0 \n self.value = value\n self.k = k\n\n def consec(self, num: int) -> bool:\n if num == self.value:\n self.count += 1\n else: \n self.count = 0 \n \n return self.count >= self.k | 2 | For a stream of integers, implement a data structure that checks if the last `k` integers parsed in the stream are **equal** to `value`.
Implement the **DataStream** class:
* `DataStream(int value, int k)` Initializes the object with an empty integer stream and the two integers `value` and `k`.
* `boolean consec(int num)` Adds `num` to the stream of integers. Returns `true` if the last `k` integers are equal to `value`, and `false` otherwise. If there are less than `k` integers, the condition does not hold true, so returns `false`.
**Example 1:**
**Input**
\[ "DataStream ", "consec ", "consec ", "consec ", "consec "\]
\[\[4, 3\], \[4\], \[4\], \[4\], \[3\]\]
**Output**
\[null, false, false, true, false\]
**Explanation**
DataStream dataStream = new DataStream(4, 3); //value = 4, k = 3
dataStream.consec(4); // Only 1 integer is parsed, so returns False.
dataStream.consec(4); // Only 2 integers are parsed.
// Since 2 is less than k, returns False.
dataStream.consec(4); // The 3 integers parsed are all equal to value, so returns True.
dataStream.consec(3); // The last k integers parsed in the stream are \[4,4,3\].
// Since 3 is not equal to value, it returns False.
**Constraints:**
* `1 <= value, num <= 109`
* `1 <= k <= 105`
* At most `105` calls will be made to `consec`. | null |
Python3 counting Solution without creating queue/array | find-consecutive-integers-from-a-data-stream | 0 | 1 | \n# Code\n```\nclass DataStream:\n\n def __init__(self, value: int, k: int):\n self.key_ = value\n self.target_counter_ = k\n self.counter_ = 0\n \n\n def consec(self, num: int) -> bool:\n if num == self.key_:\n self.counter_ += 1\n if self.counter_ == self.target_counter_:\n self.counter_ -= 1\n return True\n else:\n self.counter_ = 0\n return False\n\n\n# Your DataStream object will be instantiated and called as such:\n# obj = DataStream(value, k)\n# param_1 = obj.consec(num)\n``` | 3 | For a stream of integers, implement a data structure that checks if the last `k` integers parsed in the stream are **equal** to `value`.
Implement the **DataStream** class:
* `DataStream(int value, int k)` Initializes the object with an empty integer stream and the two integers `value` and `k`.
* `boolean consec(int num)` Adds `num` to the stream of integers. Returns `true` if the last `k` integers are equal to `value`, and `false` otherwise. If there are less than `k` integers, the condition does not hold true, so returns `false`.
**Example 1:**
**Input**
\[ "DataStream ", "consec ", "consec ", "consec ", "consec "\]
\[\[4, 3\], \[4\], \[4\], \[4\], \[3\]\]
**Output**
\[null, false, false, true, false\]
**Explanation**
DataStream dataStream = new DataStream(4, 3); //value = 4, k = 3
dataStream.consec(4); // Only 1 integer is parsed, so returns False.
dataStream.consec(4); // Only 2 integers are parsed.
// Since 2 is less than k, returns False.
dataStream.consec(4); // The 3 integers parsed are all equal to value, so returns True.
dataStream.consec(3); // The last k integers parsed in the stream are \[4,4,3\].
// Since 3 is not equal to value, it returns False.
**Constraints:**
* `1 <= value, num <= 109`
* `1 <= k <= 105`
* At most `105` calls will be made to `consec`. | null |
[Python3] Record the Last Index of non-Value Element in the Data Stream | find-consecutive-integers-from-a-data-stream | 0 | 1 | # Intuition\nChecking the last `k` element in the Data Stream each time is computationally expensive and will get TLE. We need to come up with better algorithms.\n\n# Approach\nGiven the objective of this problem, we don\'t need to (physically) maintain the full Data Stream, but just need to record the last index of the element not equal to the given `value` in the Data Stream.\n\n# Complexity\n- Time complexity of each operation: `O(1)`\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity of each operation: `O(1)`\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass DataStream:\n\n def __init__(self, value: int, k: int):\n self.value = value\n self.k = k\n self.currIdx = -1\n self.lastIdx = -1\n\n def consec(self, num: int) -> bool:\n self.currIdx += 1\n if num != self.value:\n self.lastIdx = self.currIdx\n return self.currIdx - self.lastIdx >= self.k\n\n\n# Your DataStream object will be instantiated and called as such:\n# obj = DataStream(value, k)\n# param_1 = obj.consec(num)\n``` | 6 | For a stream of integers, implement a data structure that checks if the last `k` integers parsed in the stream are **equal** to `value`.
Implement the **DataStream** class:
* `DataStream(int value, int k)` Initializes the object with an empty integer stream and the two integers `value` and `k`.
* `boolean consec(int num)` Adds `num` to the stream of integers. Returns `true` if the last `k` integers are equal to `value`, and `false` otherwise. If there are less than `k` integers, the condition does not hold true, so returns `false`.
**Example 1:**
**Input**
\[ "DataStream ", "consec ", "consec ", "consec ", "consec "\]
\[\[4, 3\], \[4\], \[4\], \[4\], \[3\]\]
**Output**
\[null, false, false, true, false\]
**Explanation**
DataStream dataStream = new DataStream(4, 3); //value = 4, k = 3
dataStream.consec(4); // Only 1 integer is parsed, so returns False.
dataStream.consec(4); // Only 2 integers are parsed.
// Since 2 is less than k, returns False.
dataStream.consec(4); // The 3 integers parsed are all equal to value, so returns True.
dataStream.consec(3); // The last k integers parsed in the stream are \[4,4,3\].
// Since 3 is not equal to value, it returns False.
**Constraints:**
* `1 <= value, num <= 109`
* `1 <= k <= 105`
* At most `105` calls will be made to `consec`. | null |
[Python] O(1) Time and Space | find-consecutive-integers-from-a-data-stream | 0 | 1 | ```\nclass DataStream:\n\n def __init__(self, value: int, k: int):\n self.k = k\n self.val = value\n self.t = k\n \n\n def consec(self, num: int) -> bool:\n if self.t > 0:\n self.t -= 1\n \n if num != self.val:\n self.t = self.k\n \n if self.t:\n return False\n return True\n``` | 1 | For a stream of integers, implement a data structure that checks if the last `k` integers parsed in the stream are **equal** to `value`.
Implement the **DataStream** class:
* `DataStream(int value, int k)` Initializes the object with an empty integer stream and the two integers `value` and `k`.
* `boolean consec(int num)` Adds `num` to the stream of integers. Returns `true` if the last `k` integers are equal to `value`, and `false` otherwise. If there are less than `k` integers, the condition does not hold true, so returns `false`.
**Example 1:**
**Input**
\[ "DataStream ", "consec ", "consec ", "consec ", "consec "\]
\[\[4, 3\], \[4\], \[4\], \[4\], \[3\]\]
**Output**
\[null, false, false, true, false\]
**Explanation**
DataStream dataStream = new DataStream(4, 3); //value = 4, k = 3
dataStream.consec(4); // Only 1 integer is parsed, so returns False.
dataStream.consec(4); // Only 2 integers are parsed.
// Since 2 is less than k, returns False.
dataStream.consec(4); // The 3 integers parsed are all equal to value, so returns True.
dataStream.consec(3); // The last k integers parsed in the stream are \[4,4,3\].
// Since 3 is not equal to value, it returns False.
**Constraints:**
* `1 <= value, num <= 109`
* `1 <= k <= 105`
* At most `105` calls will be made to `consec`. | null |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.