question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
russian-doll-envelopes | Simple Dp LIS Box Stacking | simple-dp-lis-box-stacking-by-chaharnish-24rf | It is a simple application of Longest Increasing subsequence. \nYou Just have to increase the sequence with a smaller element. \nThere is also a NlogN solution | chaharnishant | NORMAL | 2021-03-30T18:38:52.175839+00:00 | 2021-03-30T18:39:42.661102+00:00 | 654 | false | It is a simple application of Longest Increasing subsequence. \nYou Just have to increase the sequence with a smaller element. \nThere is also a NlogN solution do check it out!\n\n```\n\tstatic bool compare(pair<int,pair<int,int>>& a,pair<int,pair<int,int>>& b){\n return a.first>b.first;\n }\n \n int maxEnvelopes(vector<vector<int>>& env) {\n int n=env.size();\n vector<int> dp(n,1);\n vector<pair<int,pair<int,int>>> envs;\n for(int i=0;i<n;i++){\n envs.push_back({env[i][0]*env[i][1],{env[i][0],env[i][1]}});\n }\n \n sort(envs.begin(),envs.end(),compare);\n \n for(int i=1;i<n;i++){\n for(int j=i-1;j>=0;j--){\n if(envs[j].second.first>envs[i].second.first && envs[j].second.second>envs[i].second.second){\n dp[i]=max(dp[i],dp[j]+1);\n }\n }\n }\n \n return *max_element(dp.begin(),dp.end());\n }\n\t``` | 4 | 0 | ['Dynamic Programming'] | 0 |
russian-doll-envelopes | Java DP solution with comments for explanation | java-dp-solution-with-comments-for-expla-7jp0 | java\nclass Solution {\n public int maxEnvelopes(int[][] envelopes) {\n // sort the envelopes in ascending order based on width\n Arrays.sort(e | viet-quocnguyen | NORMAL | 2021-03-30T15:12:15.746741+00:00 | 2021-03-30T15:12:15.746787+00:00 | 180 | false | ```java\nclass Solution {\n public int maxEnvelopes(int[][] envelopes) {\n // sort the envelopes in ascending order based on width\n Arrays.sort(envelopes, (a, b) -> a[0] - b[0]); \n \n // keep track of max envelopes could be Russian doll for each envelop.\n int[] dp = new int[envelopes.length];\n Arrays.fill(dp, 1);\n \n int max = 1;\n \n // i is the current envelop index\n // j is the previous envelop index before i\n for(int i = 0; i < envelopes.length; i++){\n for(int j = 0; j < i; j++){\n // compare the width and height of the current envelop with all the previous ones.\n if(envelopes[j][0] < envelopes[i][0] &&\n envelopes[j][1] < envelopes[i][1])\n {\n // update the max number of envelopes the current envelop could be Russian doll\n dp[i] = Math.max(dp[i], dp[j] + 1);\n }\n }\n \n max = Math.max(dp[i], max);\n }\n \n return max; \n }\n}\n``` | 4 | 1 | [] | 1 |
russian-doll-envelopes | My Java Solution using Sort and Longest Increasing Subsequence concept | my-java-solution-using-sort-and-longest-3xibh | \nclass Solution {\n public int maxEnvelopes(int[][] envelopes) {\n // sort as \n // if widths are equal, sort based on the decreeasing height\ | vrohith | NORMAL | 2021-03-30T09:10:50.396348+00:00 | 2021-03-30T09:15:19.983172+00:00 | 315 | false | ```\nclass Solution {\n public int maxEnvelopes(int[][] envelopes) {\n // sort as \n // if widths are equal, sort based on the decreeasing height\n // else carry on with width on increasing\n Arrays.sort(envelopes, (a, b) -> a[0] == b[0] ? b[1] - a[1] : a[0] - b[0]);\n // now just find the longest increasing subsequence of height and thats the answer\n // it is a general dp question and that concept can be applied here\n int length = envelopes.length;\n int [] dp = new int [length];\n Arrays.fill(dp, 1); // this is the minimum possible value\n for (int i=0; i<length; i++) {\n for (int j=0; j<i; j++) {\n if (envelopes[i][1] > envelopes[j][1] && dp[i] < dp[j] + 1)\n dp[i] = dp[j] + 1;\n }\n }\n int max = Integer.MIN_VALUE;\n for (int number : dp) {\n max = Math.max(number, max);\n }\n return max;\n }\n}\n```\n\n```\n// since we sorted we can also use binary search concept for LIS\n int result = 0;\n for (int [] e : envelopes) {\n int index = Arrays.binarySearch(dp, 0, result, e[1]);\n if (index < 0) {\n index = -(index + 1);\n }\n dp[index] = e[1];\n if (index == result)\n result += 1;\n }\n return result;\n``` | 4 | 1 | ['Sorting', 'Binary Tree', 'Java'] | 0 |
russian-doll-envelopes | C++ | Variation of LIS | DP | c-variation-of-lis-dp-by-wh0ami-akzw | \nclass Solution {\n static bool compare(vector<int>v1, vector<int>v2) {\n return v1[0] < v2[0] || (v1[0] == v2[0] && v1[1] < v2[1]);\n }\npublic:\ | wh0ami | NORMAL | 2020-05-09T15:21:31.186380+00:00 | 2020-05-09T15:21:31.186411+00:00 | 249 | false | ```\nclass Solution {\n static bool compare(vector<int>v1, vector<int>v2) {\n return v1[0] < v2[0] || (v1[0] == v2[0] && v1[1] < v2[1]);\n }\npublic:\n int maxEnvelopes(vector<vector<int>>& envelopes) {\n \n int n = envelopes.size();\n if (n == 0) return 0;\n \n sort(envelopes.begin(), envelopes.end(), compare);\n \n int LIS[n];\n for (int i = 0; i < n; i++)\n LIS[i] = 1;\n \n int ans = 1;\n for (int i = 0; i < n; i++) {\n for (int j = 0; j < i; j++) {\n if (envelopes[i][0] > envelopes[j][0] && envelopes[i][1] > envelopes[j][1])\n LIS[i] = max(LIS[i], 1+LIS[j]);\n }\n ans = max(ans, LIS[i]);\n }\n \n return ans;\n }\n};\n``` | 4 | 1 | [] | 0 |
russian-doll-envelopes | Accepted C# (Sort + LIS) solution: Easy to understand | accepted-c-sort-lis-solution-easy-to-und-yso6 | \npublic class Solution {\n public int MaxEnvelopes(int[][] envelopes)\n {\n if (envelopes == null || envelopes.Length == 0)\n return 0; | pantigalt | NORMAL | 2020-03-06T09:20:38.917909+00:00 | 2020-03-06T09:20:38.917943+00:00 | 128 | false | ```\npublic class Solution {\n public int MaxEnvelopes(int[][] envelopes)\n {\n if (envelopes == null || envelopes.Length == 0)\n return 0;\n \n Array.Sort(envelopes, (a, b) => a[0] == b[0] ? b[1].CompareTo(a[1]) : a[0].CompareTo(b[0]));\n return LengthOfLIS(envelopes.Select(x => x[1]).ToArray());\n }\n \n public int LengthOfLIS(int[] nums)\n {\n int[] dp = new int[nums.Length];\n int len = 0;\n foreach (int n in nums)\n {\n int i = Array.BinarySearch(dp, 0, len, n);\n if (i < 0)\n i = -(i + 1);\n \n dp[i] = n;\n if (i == len)\n len++;\n }\n \n return len;\n } \n}\n``` | 4 | 0 | [] | 0 |
russian-doll-envelopes | Simple C# Solution | simple-c-solution-by-maxpushkarev-j87v | \n public class Solution\n {\n public int MaxEnvelopes(int[][] envelopes)\n {\n if (envelopes.Length == 0)\n {\n | maxpushkarev | NORMAL | 2020-03-05T03:07:00.101704+00:00 | 2020-03-05T03:07:00.101750+00:00 | 392 | false | ```\n public class Solution\n {\n public int MaxEnvelopes(int[][] envelopes)\n {\n if (envelopes.Length == 0)\n {\n return 0;\n }\n\n if (envelopes.Length == 1)\n {\n return 1;\n }\n\n Array.Sort(envelopes, (e1, e2) =>\n {\n var wCmp = e1[0].CompareTo(e2[0]);\n if (wCmp != 0)\n {\n return wCmp;\n }\n\n return e1[1].CompareTo(e2[1]);\n });\n\n int[] dp = new int[envelopes.Length];\n\n dp[0] = 1;\n\n for (int i = 1; i < envelopes.Length; i++)\n {\n dp[i] = 1;\n var curr = envelopes[i];\n\n for (int j = 0; j < i; j++)\n {\n var prevDp = dp[j];\n var prev = envelopes[j];\n\n if (prev[0] < curr[0] && prev[1] < curr[1])\n {\n if (prevDp + 1 > dp[i])\n {\n dp[i] = prevDp + 1;\n }\n }\n }\n }\n\n return dp.Max();\n }\n }\n``` | 4 | 2 | ['Dynamic Programming'] | 2 |
russian-doll-envelopes | DFS or topological sorting | dfs-or-topological-sorting-by-guagua_2-sp8d | The solution I propose may not be as efficient as many other solutions posted. But I find topological sorting is very intuitive here and is quite interesting. C | guagua_2 | NORMAL | 2019-12-30T06:30:52.439296+00:00 | 2019-12-30T06:30:52.439485+00:00 | 433 | false | The solution I propose may not be as efficient as many other solutions posted. But I find topological sorting is very intuitive here and is quite interesting. Consider each doll is a node in a graph. Two nodes are connected if one can contain another. It is easy to come up with a O(n^2) solution with DFS:\n\n```\nclass Solution {\n // Topological sorting\n public int maxEnvelopes(int[][] envelopes) {\n int[] visited = new int[envelopes.length]; // we need to memorize the longest path of each node.\n int max = 0;\n for (int i=0; i<envelopes.length; i++) {\n max = Math.max(max, dfs(i, envelopes, visited));\n }\n return max;\n }\n \n private int dfs(int cur, int[][] envelopes, int[] visited) {\n if (visited[cur] > 0) {\n return visited[cur];\n }\n \n int max = 1;\n for (int i=0; i<envelopes.length; i++) {\n if (envelopes[i][0] < envelopes[cur][0] && envelopes[i][1] < envelopes[cur][1]) {\n max = Math.max(max, dfs(i, envelopes, visited) + 1);\n }\n }\n visited[cur] = max;\n \n return max;\n }\n}\n``` | 4 | 1 | [] | 0 |
russian-doll-envelopes | Simple Java Graph based solution (bad performance) | simple-java-graph-based-solution-bad-per-thyp | Came up with this different approach to the problem. I figured we could model these envelopes as a graph where an edge from n1 -> n2 would mean that n1 can enve | dominoanty | NORMAL | 2019-09-03T06:44:38.929009+00:00 | 2019-09-03T06:44:38.929047+00:00 | 241 | false | Came up with this different approach to the problem. I figured we could model these envelopes as a graph where an edge from n1 -> n2 would mean that n1 can envelope n2 [n1.width > n2.width && n1.height > n2.height]. Now that we have the graph, we can find the longest path and the length of that would give us the answer. I cached the longest path from nodes to avoid recurring subproblems, to avoid TLE. \n\nRuntime : 1131ms. faster than 5% of the submissions\nMemory: 111.4mb, less than 7.69% of the submissions\n\nDefinitely not the best approach but a possible one. \n\n```\nclass Node {\n int width;\n int height;\n public List<Node> canHold = new ArrayList<>();\n \n @Override\n public String toString() {\n return "Node[" + this.width + "][" + this.height + "]";\n }\n Node(int width, int height) {\n this.width = width;\n this.height = height;\n }\n \n public void checkHold(Node n) {\n if(this.width > n.width && this.height > n.height) {\n canHold.add(n);\n }\n }\n}\nclass Solution {\n public List<Node> path;\n public Map<Node, Integer> cache = new HashMap<>();\n public int getMaxDistanceHelper(Node n, int distance) {\n \n if(cache.get(n) == null) {\n int result;\n if(n.canHold.size() == 0) \n result = distance;\n else {\n int max = 0;\n for(Node n2 : n.canHold) {\n int temp = 1 + getMaxDistanceHelper(n2, distance);\n if(temp > max)\n max = temp;\n }\n result = max; \n } \n cache.put(n, result);\n }\n return cache.get(n);\n }\n public int maxEnvelopes(int[][] envelopes) {\n List<Node> nodes = new ArrayList<>();\n \n for(int i = 0; i < envelopes.length; i++) {\n nodes.add(new Node(envelopes[i][0], envelopes[i][1]));\n }\n for(int i = 0; i < nodes.size(); i++) {\n for(int j = 0; j < nodes.size(); j++) {\n if(i != j) \n nodes.get(i).checkHold(nodes.get(j));\n }\n }\n\n int max = 0;\n for(Node n: nodes) {\n \n int dist = getMaxDistanceHelper(n, 1);\n if(dist > max)\n max = dist;\n }\n return max;\n }\n}\n``` | 4 | 0 | ['Graph'] | 0 |
russian-doll-envelopes | JAVA dp solution with comments | java-dp-solution-with-comments-by-siddha-6m3a | \nclass Solution {\n \n int[] dp;\n \n public int lengthOfRussianDoll(int index, int[][] envelopes){\n \n\t\t// terminating condition aka bas | siddhantiitbmittal3 | NORMAL | 2019-05-17T12:55:51.591421+00:00 | 2019-05-17T12:55:51.591486+00:00 | 661 | false | ```\nclass Solution {\n \n int[] dp;\n \n public int lengthOfRussianDoll(int index, int[][] envelopes){\n \n\t\t// terminating condition aka base case\n if(index > envelopes.length-1)\n return 0;\n \n if(dp[index] > 0)\n return dp[index];\n\n int maxCount = 0;\n \n\t\t// main recursive module\n for(int i=index+1;i<envelopes.length;i++){\n\t\t\t// check if i envelope can be put inside index envelope\n if(envelopes[index][1] > envelopes[i][1] && envelopes[index][0] > envelopes[i][0])\n maxCount = Math.max(maxCount, lengthOfRussianDoll(i,envelopes)+1);\n }\n \n dp[index] = maxCount;\n \n return dp[index];\n }\n \n public int maxEnvelopes(int[][] envelopes) {\n // edge cases\n if(envelopes == null || envelopes.length == 0)\n return 0;\n \n\t\t// sort the array on envelopes with width descending order.\n Arrays.sort(envelopes, new Comparator<int[]>(){\n @Override\n public int compare(int[] a1, int[] a2){\n return a2[0] - a1[0];\n }\n });\n \n\t\t// dp[index] gives the maxium length of russian doll taking index as the outer most envelope.\n dp = new int[envelopes.length];\n \n\t\t// Now we consider creating the russian doll sequence with considering each envelope as the\n\t\t// outermost envelope and then moving forward. The important thing about sorting is than for any\n\t\t// index i ....only possible indexes will be greater than i. \n int maxCount = 0;\n for(int i=0;i<envelopes.length;i++){\n maxCount = Math.max(maxCount, lengthOfRussianDoll(i,envelopes)+1); \n }\n \n return maxCount;\n \n }\n}\n``` | 4 | 0 | [] | 2 |
russian-doll-envelopes | Longest path in DAG. DP solution. With follow up solution. | longest-path-in-dag-dp-solution-with-fol-xpla | dp[i] = max(0, dp[j] | if rectangle j can be embedded to rectangle i) + 1\n\n public class Solution {\n \n boolean canFit(int[] a, int[] b) { | zzz1322 | NORMAL | 2016-06-07T02:09:14+00:00 | 2016-06-07T02:09:14+00:00 | 2,246 | false | **dp[i] = max(0, dp[j]** | if rectangle j can be embedded to rectangle i) **+ 1**\n\n public class Solution {\n \n boolean canFit(int[] a, int[] b) { // Rectangle a can fit into rectangle b. \n return (a[0] < b[0] && a[1] < b[1]);\n }\n \n public int maxEnvelopes(int[][] envelopes) {\n if(envelopes == null || envelopes.length == 0) return 0;\n // In the follow up question, rectangle can be rotated to fit into another. In this case, we need preprocess envelopes array to let smaller value be envelopes[0], bigger value be envelopes[1]\n Arrays.sort(envelopes, (int[] x, int[] y) -> x[0] - y[0]);\n int n = envelopes.length;\n int dp[] = new int[n];\n int rst = 0;\n for(int i = 0; i < n; ++i) {\n int max = 0;\n for(int j = 0; j < i; ++j) {\n if(canFit(envelopes[j], envelopes[i])) max = Math.max(max, dp[j]);\n }\n dp[i] = max + 1;\n rst = Math.max(dp[i], rst);\n }\n return rst;\n }\n } | 4 | 1 | [] | 1 |
russian-doll-envelopes | My Three C++ Solutions: DP, Binary Search and Lower_bound | my-three-c-solutions-dp-binary-search-an-akei | Solution One:\n\n class Solution {\n public:\n int maxEnvelopes(vector>& envelopes) {\n int res = 0, n = envelopes.size();\n \t\tvect | grandyang | NORMAL | 2016-06-07T16:36:16+00:00 | 2016-06-07T16:36:16+00:00 | 869 | false | Solution One:\n\n class Solution {\n public:\n int maxEnvelopes(vector<pair<int, int>>& envelopes) {\n int res = 0, n = envelopes.size();\n \t\tvector<int> dp(n, 1);\n \t\tsort(envelopes.begin(), envelopes.end());\n \t\tfor (int i = 0; i < n; ++i) {\n \t\t\tfor (int j = 0; j < i; ++j) {\n \t\t\t\tif (envelopes[i].first > envelopes[j].first && envelopes[i].second > envelopes[j].second) {\n \t\t\t\t\tdp[i] = max(dp[i], dp[j] + 1);\n \t\t\t\t}\n \t\t\t}\n \t\t\tres = max(res, dp[i]);\n \t\t}\n \t\treturn res;\n }\n };\n\nSolution Two:\n\n class Solution {\n public:\n int maxEnvelopes(vector<pair<int, int>>& envelopes) {\n vector<int> dp;\n \t\tsort(envelopes.begin(), envelopes.end(), [](const pair<int, int> &a, const pair<int, int> &b){\n \t\t\tif (a.first == b.first) return a.second > b.second;\n \t\t\treturn a.first < b.first;\n \t\t});\n \t\tfor (int i = 0; i < envelopes.size(); ++i) {\n \t\t\tint left = 0, right = dp.size(), t= envelopes[i].second;\n \t\t\twhile (left < right) {\n \t\t\t\tint mid = left + (right - left) / 2;\n \t\t\t\tif (dp[mid] < t) left = mid + 1;\n \t\t\t\telse right = mid;\n \t\t\t}\n \t\t\tif (right >= dp.size()) dp.push_back(t);\n \t\t\telse dp[right] = t;\n \t\t}\n \t\treturn dp.size();\n }\n };\n\nSolution Three:\n\n class Solution {\n public:\n int maxEnvelopes(vector<pair<int, int>>& envelopes) {\n vector<int> dp;\n \t\tsort(envelopes.begin(), envelopes.end(), [](const pair<int, int> &a, const pair<int, int> &b){\n \t\t\tif (a.first == b.first) return a.second > b.second;\n \t\t\treturn a.first < b.first;\n \t\t});\n \t\tfor (int i = 0; i < envelopes.size(); ++i) {\n \t\t\tauto it = lower_bound(dp.begin(), dp.end(), envelopes[i].second);\n \t\t\tif (it == dp.end()) dp.push_back(envelopes[i].second);\n \t\t\telse *it = envelopes[i].second;\n \t\t}\n \t\treturn dp.size();\n }\n }; | 4 | 1 | [] | 0 |
russian-doll-envelopes | C++ DP Solution with O(n^2) Time complexity O(n) Space complexity | c-dp-solution-with-on2-time-complexity-o-91zo | class Solution {\n public:\n int maxEnvelopes(vector<pair<int, int>>& envelopes) {\n int ans = 0;\n vector<int> dp;\n | yular | NORMAL | 2016-06-09T07:48:57+00:00 | 2016-06-09T07:48:57+00:00 | 906 | false | class Solution {\n public:\n int maxEnvelopes(vector<pair<int, int>>& envelopes) {\n int ans = 0;\n vector<int> dp;\n if(!envelopes.size())\n return ans;\n sort(envelopes.begin(), envelopes.end(), cmpfunc);\n dp.resize(envelopes.size());\n dp[0] = 1;\n ans = 1;\n for(int i = 1; i < envelopes.size(); ++ i){\n dp[i] = 0;\n for(int j = 0; j < i; ++ j){\n if(envelopes[j].first < envelopes[i].first && envelopes[j].second < envelopes[i].second)\n dp[i] = max(dp[i], dp[j]);\n }\n ++ dp[i];\n ans = max(ans, dp[i]);\n }\n return ans;\n }\n private:\n struct cmp{\n \t bool operator() (const pair<int, int> &a, const pair<int, int> &b){\n \t return a.first*a.second < b.first*b.second; \n \t }\n \t}cmpfunc;\n }; | 4 | 1 | ['Dynamic Programming', 'C++'] | 2 |
russian-doll-envelopes | Clean C++ 11 Implementation with Explaination refered from @kamyu104 | clean-c-11-implementation-with-explainat-nqmq | It is easy to relate this problem with the previous LIS problem. But how to solve it under the 2 dimensional cases. The key ideas lay at that how we deal with t | rainbowsecret | NORMAL | 2016-06-12T02:38:58+00:00 | 2018-10-20T11:37:12.659773+00:00 | 984 | false | It is easy to relate this problem with the previous LIS problem. But how to solve it under the 2 dimensional cases. The key ideas lay at that how we deal with the equal width but different hight cases. \n\nA clever solution is to sort the pair<int,int> array according the width, if the width is equal, just sort by the height reversely. \n\nThen we can get the following solutions : \n\n class Solution {\n public:\n int maxEnvelopes(vector<pair<int, int>>& envelopes) {\n int size_ = envelopes.size();\n if(size_ < 2) return size_; \n sort(envelopes.begin(), envelopes.end(), \n [](const pair<int, int>& a, const pair<int, int>& b) {\n if(a.first == b.first) {\n return a.second > b.second;\n }\n else {\n return a.first < b.first;\n }\n });\n /** find the LIS of the height, as we have filtered the width equal cases **/\n vector<int> result;\n for (const auto& iter : envelopes) {\n const auto target = iter.second;\n auto cur = lower_bound(result.begin(), result.end(), target);\n if (cur == result.end()) {\n result.emplace_back(target);\n } else {\n *cur = target;\n }\n }\n return result.size();\n }\n }; | 4 | 1 | [] | 0 |
russian-doll-envelopes | Longest increasing Subsequence || O(Nlogn) || Easy CPP Code | longest-increasing-subsequence-onlogn-ea-vuow | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | ayushrakwal94 | NORMAL | 2024-03-07T08:14:11.934568+00:00 | 2024-03-07T08:14:11.934591+00:00 | 756 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n static bool comp(pair<int,int> &a,pair<int,int>&b){\n if(a.first==b.first){\n return a.second>b.second;\n }\n else{\n return a.first<b.first;\n }\n }\n\n int maxEnvelopes(vector<vector<int>>& env) {\n vector<pair<int,int>>arr(env.size());\n for(int i = 0 ; i < env.size() ; i++){\n arr[i] = {env[i][0],env[i][1]};\n }\n\n sort(arr.begin(),arr.end(),comp);\n \n vector<int>dp(env.size());\n int ans = 0;\n\n for(int i = 0 ; i < env.size() ; i++)\n {\n int l = 0;\n int h = ans;\n while(l<h){\n int m = l +(h-l)/2;\n\n if(dp[m] < arr[i].second) l = m + 1;\n else h = m;\n }\n\n dp[l] = arr[i].second;\n if(l == ans) ans++;\n }\n return ans;\n }\n};\n``` | 3 | 0 | ['Binary Search', 'Dynamic Programming', 'Sorting', 'C++'] | 0 |
russian-doll-envelopes | C++ Solution | Stepwise Explained | Hard problem made easy | Binary Search | Sorting | DP | c-solution-stepwise-explained-hard-probl-c4jr | Intuition and Approach\n\nThe goal is to find the maximum number of envelopes that can be nested inside each other. The sorting is done based on the width, and | darkaadityaa | NORMAL | 2024-02-06T19:04:33.063616+00:00 | 2024-02-06T19:16:47.416988+00:00 | 277 | false | # Intuition and Approach\n\nThe goal is to find the maximum number of envelopes that can be nested inside each other. The sorting is done based on the width, and then the problem is reduced to finding the **Longest Increasing Subsequence (LIS)** based on the height.\n\n1) **Sort Based on Width:**\n-- Sort the envelopes based on the width in ascending order.\n-- If two envelopes have the same width, sort them in descending order of height.\n\n2) **Dynamic Programming (LIS):**\n-- Initialize a vector $$temp$$ to keep track of the heights forming the LIS. \nIterate through the sorted envelopes:\n-- For each envelope, get its height.\n-- If the height is greater than the last element in $$temp$$, add it to the end.\n-- Otherwise, find the first element in $$temp$$ that is greater than or equal to the height, and update it.\n-- The size of the final $$temp$$ vector represents the length of the LIS.\n\n**Key Idea:**\n-- The sorting step helps in simplifying the problem by reducing it to finding the LIS.\n-- The LIS calculation is often done using dynamic programming or binary search.\nThis approach is commonly employed in problems where one needs to find the \n\n\n\n--------------------------------------------------------------------\n\n# Complexity\n- Time complexity: O(N log N + N log K)\n-- where N is the number of envelopes and K is the length of the LIS.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(N)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n-------------------------------------------------------------------\n\n#### If you find this solution helpful, consider giving it an upvote!\n\n\n#### Do check out all other solutions posted by me here: https://github.com/adityajamwal02/Leetcode-Community-Solutions\n\n-------------------------------------------------------------------\n\n# Code\n```\nclass Solution {\npublic:\n static bool compare(vector<int> &a, vector<int> &b){\n if(a[0]==b[0]){\n return a[1]>b[1];\n }\n return a[0]<b[0];\n }\n int maxEnvelopes(vector<vector<int>>& envelopes) {\n int n=envelopes.size();\n sort(envelopes.begin(), envelopes.end(), compare);\n vector<int> temp;\n temp.push_back(envelopes[0][1]);\n for(int i=1;i<n;i++){\n int height=envelopes[i][1];\n if(height>temp.back()){\n temp.push_back(height);\n }else{\n auto it = lower_bound(temp.begin(),temp.end(),height);\n *it=height;\n }\n }\n return temp.size(); \n }\n};\n``` | 3 | 0 | ['Binary Search', 'Dynamic Programming', 'Sorting', 'C++'] | 0 |
russian-doll-envelopes | Easy || Relatable approach|| learn from 300. | easy-relatable-approach-learn-from-300-b-2ogf | Intuition\n Describe your first thoughts on how to solve this problem. \nSee this problem is similar to 300. Longest Increasing Subsequence , I have explained i | Abhishekkant135 | NORMAL | 2024-01-06T07:50:51.854454+00:00 | 2024-01-06T07:50:51.854489+00:00 | 1,030 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nSee this problem is similar to 300. Longest Increasing Subsequence , I have explained in detailed how patience sort works read it here https://leetcode.com/problems/longest-increasing-subsequence/solutions/4514634/easy-patience-sorting-o-nlogn/\n# Approach\n<!-- Describe your approach to solving the problem. -->\nSort the array. Ascend on width and descend on height if width are same.\nFind the longest increasing subsequence based on height of envelopea.\nAs you have arranges the height in decreasing sense for same width the code will automatically choose the least height for consideration ( read the link above).\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(nlogN)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int maxEnvelopes(int[][] envelopes) {\n Arrays.sort(envelopes, (a, b) -> {\n if (a[0] == b[0]) {\n return b[1] - a[1];\n }\n else {\n return a[0] - b[0];\n }\n });\n int nums[]=new int [envelopes.length];\n int j=0;\n for(int i=0;i<envelopes.length;i++){\n nums[j]=envelopes[i][1];\n j++;\n }\n return lengthOfLIS(nums);\n }\n// the below part is taken exact from problem 300 , read the link above.\n\n public int lengthOfLIS(int[] nums) {\n ArrayList<Integer> al=new ArrayList<>();\n for(int i=0;i<nums.length;i++){\n int index=binary(al,nums[i]);\n if(index==al.size()){\n al.add(nums[i]);\n }\n else{\n al.set(index,nums[i]);\n }\n \n }\n return al.size();\n }\n\n\n public int binary(ArrayList<Integer> al,int target){\n int s=0;\n int e=al.size();\n int result=al.size();\n while(s<e){\n int mid=(s+e)/2;\n if(al.get(mid)<target){\n s=mid+1;\n }\n else{\n e=mid;\n result=mid;\n }\n }\n return result;\n }\n} \n\n``` | 3 | 0 | ['Java'] | 1 |
russian-doll-envelopes | Most Detailed and BEGINNER FRIENDLY Explanation || Binary Search and DP (Memoization) | most-detailed-and-beginner-friendly-expl-kqpo | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \nv = [[5,4],[6,4],[6,7], | BihaniManan | NORMAL | 2023-07-19T16:11:15.224564+00:00 | 2024-09-21T10:39:47.232370+00:00 | 375 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nv = [[5,4],[6,4],[6,7],[2,3]]\n\nSort this vector in increasing order wrt to the first element.\nIf v[i].first == v[i + 1].first, in this case sort in decreasing order wrt to the second element.\n\nv = [[2,3],[5,4],[6,7],[6,4]]\n\nWhy we are sorting the vector pair like this?\n\nNotice it carefully, after sorting it like this, it **reduces to a very famous problem called Longest Increasing Subsequence**, wrt to second part of the vector array.\n\nNow you have to just ignore the first element i.e. v[i].first and solve the problem wrt to v[i].second and it is same as **Longest Increasing Subsequence**.\n\nI am attaching the problem here, for your reference.\n\n[Longest Increasing Subsequence](https://leetcode.com/submissions/detail/997895245/)\n\n\n# **Note**: \n**Recursion with Memoization won\'t work here due to constraints of the problem.**\n\nRecursion with Memoization will give **TLE**.\n\n1 <= envelopes.length <= 10^5\n\nBut still **here is the code of RECURSION with MEMOIZATION** for your better understanding.\n\n```\nclass Solution {\npublic:\n static bool sortbyCond(const pair<int, int>& a, const pair<int, int> &b)\n {\n if(a.first != b.first) return (a.first < b.first);\n else return (a.second > b.second);\n }\n\n int solveMem(vector<pair<int, int>> &nums, int curr, int prev, vector<vector<int>> &dp)\n {\n if(curr == nums.size()) return 0;\n if(dp[curr][prev + 1] != -1) return dp[curr][prev + 1];\n\n int inc = 0, exc = 0;\n\n if(prev == -1 || nums[prev].second < nums[curr].second)\n {\n inc = 1 + solveMem(nums, curr + 1, curr, dp);\n }\n exc = 0 + solveMem(nums, curr + 1, prev, dp);\n\n dp[curr][prev + 1] = max(inc, exc);\n\n return dp[curr][prev + 1];\n }\n \n int maxEnvelopes(vector<vector<int>>& envelopes) {\n vector<pair<int,int>> v;\n for(int i = 0; i < envelopes.size(); i++)\n {\n v.push_back({envelopes[i][0], envelopes[i][1]});\n }\n\n sort(v.begin(), v.end(), sortbyCond);\n\n vector<vector<int>> dp(v.size(), vector<int> (v.size(), -1));\n\n return solveMem(v, 0, -1, dp);\n }\n};\n```\n\n---\n\n# Now coming to the main solution of this problem:\n\nSo the idea here is to apply the **binary search** on the sorted array.\n\n**v = [[2,3],[5,4],[6,7],[6,4]]**\n\nFor simplicity treat the problem as Longest Increasing Subsequence on second part of the vector v, as our problem as reduced to this only.\n\nNow I\'ll take a better example to explain the binary search solution:\n**nums = [5,8,3,7,9,1]**\n\n1st iteration: LIS = [5]\n2nd iteration: LIS = [5,8]\n3rd iteration: LIS = [5,8] , [3]\n4th iteration: LIS = [5,8] , [3,7]\n5th iteration: LIS = [5,8,9] , [3,7,9]\n6th iteration: LIS = [5,8,9], [3,7,9]\n\nBut after reading the problem carefully we come to know that we just want the length of the Longest Increasing Subsequence and the order just doesn\'t matter.\n\nSo, now push the first element to the ans vector and after each iteration compare the last element of the answer vector to the next element of the vector nums and\n\nif(nums[i].second > ans.back()){\nans.push_back(nums[i].second);\n}\nelse{\nint index = lower_bound(ans.begin(), ans.end(), nums[i].second) - ans.begin();\nans[index] = nums[i].second;\n}\n\n**Dry Run:**\n**nums = [5,8,3,7,9,1]**\n\n1st iteration: ans = [5]\n2nd iteration: ans = [5,8]\n3rd iteration: ans = [3,8]\n4th iteration: ans = [3,7]\n5th iteration: ans = [3,7,9]\n6th iteration: ans = [1,7,9]\n\n**ans.size() = 3** \nwhich is our required ans.\n\n\n### Feel free to post your doubts in the comment section.\n\n# Complexity\n- Time complexity: **O(n logn)**\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: **O(n)**\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n static bool sortbyCond(const pair<int, int>& a, const pair<int, int> &b)\n {\n if(a.first != b.first) return (a.first < b.first);\n else return (a.second > b.second);\n }\n\n int solveOptimal(vector<pair<int, int>> &nums)\n {\n if(nums.size() == 0) return 0;\n vector<int> ans;\n ans.push_back(nums[0].second);\n\n for(int i = 1; i < nums.size(); i++)\n {\n if(nums[i].second > ans.back())\n {\n ans.push_back(nums[i].second);\n }\n else\n {\n int index = lower_bound(ans.begin(), ans.end(), nums[i].second) - ans.begin();\n ans[index] = nums[i].second;\n }\n }\n return ans.size();\n }\n\n \n int maxEnvelopes(vector<vector<int>>& envelopes) {\n vector<pair<int,int>> v;\n for(int i = 0; i < envelopes.size(); i++)\n {\n v.push_back({envelopes[i][0], envelopes[i][1]});\n }\n\n sort(v.begin(), v.end(), sortbyCond);\n\n return solveOptimal(v);\n }\n};\n``` | 3 | 1 | ['Binary Search', 'Dynamic Programming', 'Recursion', 'Memoization', 'C++'] | 0 |
russian-doll-envelopes | 4 APPROCH WITH EXPLAIN->SAME AS LIS | 4-approch-with-explain-same-as-lis-by-pa-1qfk | Intuition\n Describe your first thoughts on how to solve this problem. \nLONGEST INCREASING SUBSEQUENCE SAME\n# Approach\n Describe your approach to solving the | parth_gujral_ | NORMAL | 2023-03-23T13:22:13.169139+00:00 | 2023-03-23T13:22:13.169178+00:00 | 1,528 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nLONGEST INCREASING SUBSEQUENCE SAME\n# Approach\n<!-- Describe your approach to solving the problem. -->\nRussian dolls hai unko envelops me dalna hai to sort kardia width wise but agar width same hui kisi ki toh kya kre?\n\nto hmne HIEGHT ko sort krdia but descending order me an descending order me kyu kara ?\nbeacuse hum LIS lga sake uspr agar hum normal karenge to mja n aaega..\n(Complex hoega)\n\n#love babar bhaiya\n\n# Complexity\n- Time complexity:RECURSION->exponential (TLE)\n- MEMOIZATION-> O(N*N) (TLE)\n- TOP-DOWN-> O(N*N) (TLE)\n- Binary search ->O(N*LOG(N)) (optimised)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n- MEMOIZATION-> O(N*N)\n- TOP-DOWN-> O(N*N)\n- Binary search ->O(N)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n \n // int solve(int n, vector<vector<int>>& envelopes,int curr,int prev)\n // {\n // if(curr == n)\n // {\n // return 0;\n // }\n\n // int include=0;\n // if(prev == -1 or (envelopes[prev][0] < envelopes[curr][0] and \n // envelopes[prev][1] < envelopes[curr][1]))\n // {\n //check with and heigth both\n // include = 1 + solve(n,envelopes,curr+1,curr);\n // }\n\n // int exclude = 0 + solve(n,envelopes, curr+1, prev);\n\n // return max(include,exclude);\n // }\n\n // int solve_mem(int n, vector<vector<int>>& envelopes,int curr,int prev,vector<vector<int>> &dp)\n // {\n // if(curr == n)\n // {\n // return 0;\n // }\n\n // if(dp[curr][prev+1] != -1)\n // return dp[curr][prev+1];\n\n\n // int include=0;\n // if(prev == -1 or (envelopes[prev][0] < envelopes[curr][0] and \n // envelopes[prev][1] < envelopes[curr][1]))\n // {\n // include = 1 + solve_mem(n,envelopes,curr+1,curr,dp);\n // }\n\n // int exclude = 0 + solve_mem(n,envelopes, curr+1, prev,dp);\n\n // dp[curr][prev+1] = max(include,exclude);\n\n // return dp[curr][prev+1];\n // }\n\n static bool comp(const vector<int> &v1,const vector<int> &v2)\n {\n if(v1[0] == v2[0])\n {\n return v1[1] > v2[1];\n }\n else\n return v2[0] > v1[0];\n }\n\n int maxEnvelopes(vector<vector<int>>& envelopes) {\n\n\n // sort(envelopes.begin(),envelopes.end());\n\n // for(int i=0;i<envelopes.size();i++)\n // {\n // for(int j=0;j<envelopes[0].size();j++)\n // {\n // cout<<"envelopes[i][j]"<<envelopes[i][j]<<" ";\n // }\n // cout<<endl;\n // }\n\n //RECURSION\n // return solve(envelopes.size(), envelopes, 0, -1);\n \n //MEMOIZATION\n // vector<vector<int>> dp(envelopes.size()+1,vector<int>(envelopes.size()+1,-1));\n // return solve_mem(envelopes.size(), envelopes, 0, -1,dp);\n\n //TABULATION\n\n // vector<vector<int>> dp(envelopes.size()+1,vector<int>(envelopes.size()+1,0));\n // int n=envelopes.size();\n // for(int curr = n-1; curr>=0; curr--)\n // {\n // for(int prev= curr-1; prev>=-1; prev--)\n // {\n // int include=0;\n // if(prev == -1 or (envelopes[prev][0] < envelopes[curr][0] and \n // envelopes[prev][1] < envelopes[curr][1]))\n // {\n // include = 1 + dp[curr+1][curr+1];\n // }\n\n // int exclude = 0 + dp[curr+1][prev+1];\n\n // dp[curr][prev+1] = max(include,exclude);\n // }\n // }\n\n // return dp[0][0];\n\n //BINARY SEARCH\n\n // \n int n = envelopes.size();\n sort(envelopes.begin(),envelopes.end(),comp);\n vector<int>arr;\n for(int i =0;i<n;i++)\n {\n if(arr.empty() || arr.back()<envelopes[i][1])\n arr.push_back(envelopes[i][1]);\n else\n {\n int index = lower_bound(arr.begin(),arr.end(),envelopes[i][1])\n - arr.begin();\n arr[index] = envelopes[i][1];\n }\n }\n \n return arr.size();\n }\n};\n\n\n// class Solution {\n// public:\n// static bool cmp(const vector<int>& v1, const vector<int>& v2)\n// {\n// if(v1[0] == v2[0]) return v1[1] > v2[1];\n// return v1[0]<v2[0];\n// }\n// int maxEnvelopes(vector<vector<int>>& envelopes) {\n// int n = envelopes.size();\n// sort(envelopes.begin(),envelopes.end(),cmp);\n// vector<int>arr;\n// for(int i =0;i<n;i++)\n// {\n// if(arr.empty() || arr.back()<envelopes[i][1])\n// arr.push_back(envelopes[i][1]);\n// else\n// {\n// int index = lower_bound(arr.begin(),arr.end(),envelopes[i][1])\n// - arr.begin();\n// arr[index] = envelopes[i][1];\n// }\n// }\n \n// return arr.size();\n// }\n// };\n\n``` | 3 | 0 | ['C++'] | 1 |
russian-doll-envelopes | What i understood from all lis solution | what-i-understood-from-all-lis-solution-rmnom | \n// this is nlogn lis variant\nwe need to sort the weights in increasing order but if they are equal then\n heights should be in decreasing order , this is d | pranavrocksharma | NORMAL | 2022-06-20T16:03:10.944465+00:00 | 2022-06-20T16:04:52.583596+00:00 | 160 | false | \n// this is nlogn lis variant\nwe need to sort the weights in increasing order but if they are equal then\n heights should be in decreasing order , this is done to ensure that when we\n do lis on the height the lower one do not gets picked up. eg\n \n [[5,4],[6,5],[6,7],[2,3]]\n \n after sorting in inc width and height for same width\n \n [[2,3] [5,4] [6,5] [6,7]]\n \n { 3, 4, 5, 7} this is telling we can put 3rd envolope in 4th but its false\n so thats why we do reverse sort for same width\n \n [[2,3] [5,4] [6,7] [6,5]]\n {3,4,7} {3,4,5} this wont allow lower value to be part of lis as big is ahead\n \n\n // time = nlogn\n\n```\n bool static comp(vector<int> &a, vector<int> &b){\n if(a[0] == b[0]){\n return a[1] > b[1];\n }\n return a[0] < b[0];\n }\n int maxEnvelopes(vector<vector<int>>& envelopes) {\n sort(envelopes.begin(),envelopes.end(),comp);\n vector<int> temp;\n temp.push_back(envelopes[0][1]);\n for(int i=1; i<envelopes.size(); ++i){\n if(temp.back() < envelopes[i][1]){\n temp.push_back(envelopes[i][1]);\n }else{\n int idx = lower_bound(temp.begin(),temp.end(),envelopes[i][1])\n - temp.begin();\n temp[idx] = envelopes[i][1];\n }\n }\n return temp.size();\n }\n``` | 3 | 0 | ['Dynamic Programming'] | 0 |
russian-doll-envelopes | Russian Doll Envelopes || O(NlogN) || LIS || Binary Search | russian-doll-envelopes-onlogn-lis-binary-a3b2 | First, we sort the Envelopes. width in ascending order and height in decending order. Then apply LIS (Longest Increasing Subsequence) on height to find the requ | tanwar02 | NORMAL | 2022-05-25T21:19:19.779468+00:00 | 2022-05-27T12:08:59.863381+00:00 | 450 | false | First, we sort the Envelopes. width in ascending order and height in decending order. Then apply LIS (Longest Increasing Subsequence) on height to find the required length.\n\nWhy height in decending order?\nIf we sort the height in ascending order and If the width of two envelopes are same and the height of first envelope is less than the next envelope, there will be LIS of 2 length but first envelope can not fit in next envelope because the width of both envelopes are same.\nExample: If sort both width and height in ascending order : [6,4],[6,7]. Here LIS will be [4,7], lenght will be 2 but they can not fit.\nIf sort width in ascending order and height in decending order : [6,7],[6,4]. Here LIS will be either [7] or [4], length will be 1 because they can not fit.\n\nIf width of some envelopes are same, they can not fit. Here We apply the LIS with respect to height, we sort the height in decending order so that the envelopes with same width can not be in one LIS.\n\n\nHere we will use binary search to find the length of LIS. Binary Search approach can only find the length of LIS but not the elements of LIS.\nLet\'s create a list lis to store the LIS. It may not be valid LIS but length will be correct.\n\nSuppose a new element come, find the index where it can fit in lis so that lis will be in increasing subsequence. If it can not fit in lis, append in the lis.\n\nExample: [1,7,8,4,5,6,-1,9]\nlis : []\n(i) element 1 : lis -> [1]\n(ii) element 7 : lis -> [1,7]\n(iii) element 8 : lis -> [1,7,8]\n(iv) element 4 : lis -> [1,4,8] 4 fit at place of 7. Now suppose two or more elements new element come greater than 4 but less tha equal to 7 in increasing sequence (e.g. [5,6,7]), we can find a larger subsequence([1,4,5,6,7]) than the previous subsequence ([1,7,8])\n(v) element 5 : lis -> [1,4,5] 5 can fit at place of 8. You can think that a new element come that can be in increasing sequence with 8 element (e.g. 10) but That element also will be in increasing subsequence with lesser element than 8 elemnt (e.g. 5).\n(vi) element 6 : lis -> [1,4,5,6]\n(vii) element -1: lis -> [-1,4,5,6]\n(viii)element 9 : lis -> [-1,4,5,6,9]\n\nThis is not the valid LIS ([-1,4,5,6,9]) but length of the longest valid subsequence will be equal to this LIS.\nIn a valid subsequence element at a index will be greater than or equal to the element at that index in this LIS.\n\nHere we will use binary search to find the position of new element where it can fit in the lis.\n\nCollections.binarySearch(List<T>, T) return the index of the element in the list if it is present in the list.\nIf it is not present in the list, it will return -(insertion index)-1 or -(insertion index+1).\nIf it is negative, multiply by -1 and then subtract 1 from it to find the correct position in lis.\n\nclass Solution {\n \n List<Integer> lis;\n public int maxEnvelopes(int[][] envelopes) {\n \n Arrays.sort(envelopes, (a, b)-> a[0]==b[0] ? b[1]-a[1] : a[0]-b[0]); //sort width in asending order and height in desending order.\n \n lis = new ArrayList<>();\n \n lis.add(envelopes[0][1]);\n \n for(int k=1; k<envelopes.length; k++){\n \n if(lis.get(lis.size()-1) < envelopes[k][1]) lis.add(envelopes[k][1]);\n else lis.set(binarySearch(envelopes[k][1]), envelopes[k][1]);\n }\n \n return lis.size();\n }\n \n int binarySearch(int height){\n \n int i = Collections.binarySearch(lis, height);\n if(i < 0){\n \n i *= -1;\n i -= 1;\n }\n return i;\n \n /*int low = 0, high = lis.size()-1, mid, pos = 0;\n \n while(low <= high){\n \n mid = (low + high)/2;\n if(lis.get(mid) == height) return mid;\n if(lis.get(mid) < height){\n \n pos = mid+1;\n low = mid+1;\n }\n else high = mid-1;\n }\n return pos;*/\n }\n} | 3 | 0 | ['Binary Tree', 'Java'] | 0 |
russian-doll-envelopes | ✅C++ | ✅2 Approaches well Explained O(N^2) & O(NlogN) | ✅Easy & clean Code | c-2-approaches-well-explained-on2-onlogn-c74a | Approach-1: O(N^2) Approach | passed 85/87 testcases\nstep-1 sort the ds\n step-2: Solve it like LIS problem (https://leetcode.com/problems/longest-increasing-s | shm_47 | NORMAL | 2022-05-25T11:20:48.763845+00:00 | 2022-05-25T11:21:49.953061+00:00 | 335 | false | **Approach-1:** O(N^2) Approach | passed 85/87 testcases\nstep-1 sort the ds\n step-2: Solve it like LIS problem (https://leetcode.com/problems/longest-increasing-subsequence/discuss/2072338/c-on2-solution-explained-through-comments) \n\n```\nclass Solution {\npublic:\n int maxEnvelopes(vector<vector<int>>& envelopes) {\n //step-1: sort the envelopes in increasing order\n sort(envelopes.begin(), envelopes.end());\n \n int n = envelopes.size(), mx = 1; //1 envelope will be there always\n \n vector<int> LIS(n, 1); //1 for a single envelop\n \n //step-2: find LIS(Longest Increasing Sequence)\n for(int i=1;i<n; i++){\n for(int j=0; j<i; j++){\n if(envelopes[i][0]> envelopes[j][0] && envelopes[i][1]> envelopes[j][1] && LIS[i]<=LIS[j])\n LIS[i] = 1+ LIS[j];\n }\n mx = max(mx, LIS[i]);\n }\n \n return mx;\n }\n};\n```\n\n**Approach-2:** O(NlogN) Approach\n```\nclass Solution {\n static bool cmp(vector<int>& a, vector<int>& b){\n if(a[0]==b[0]) return a[1] > b[1];\n return a[0] < b[0];\n }\npublic:\n int maxEnvelopes(vector<vector<int>>& env) {\n int n = env.size();\n \n // sorting by height & if we encounter same height\n // sort by descending order of width\n sort(env.begin(), env.end(), cmp);\n \n \n vector<int> lis;\n \n for(int i = 0;i<env.size();i++){\n int ele = env[i][1];\n \n int idx = lower_bound(lis.begin(), lis.end(), ele) - lis.begin();\n \n if(idx >= lis.size()) lis.push_back(ele);\n else lis[idx] = ele;\n }\n \n return lis.size();\n }\n};\n``` | 3 | 0 | ['C'] | 1 |
russian-doll-envelopes | C++ | 4 Approaches | Recursion -> Memoization -> Tabulation -> Binary Search | c-4-approaches-recursion-memoization-tab-it88 | 1. Recursion\nCODE:\n\n // Recursion *** Will Give TLE \n int solve(int idx, int prev, vector> &arr, int n) {\n if(idx == n)\n return 0 | Kajal_39 | NORMAL | 2022-05-25T07:25:29.183951+00:00 | 2022-05-25T07:51:41.724872+00:00 | 264 | false | **1. Recursion\nCODE:**\n\n // Recursion *** Will Give TLE ***\n int solve(int idx, int prev, vector<vector<int>> &arr, int n) {\n if(idx == n)\n return 0;\n int take = 0, untake = 0;\n untake = solve(idx+1, prev, arr, n);\n if((prev == -1) || (arr[idx][0] > arr[prev][0] && arr[idx][1] > arr[prev][1])) {\n take = 1 + solve(idx+1, idx, arr, n);\n }\n return max(take, untake);\n }\n int maxEnvelopes(vector<vector<int>>& envelopes) {\n int n = envelopes.size();\n sort(envelopes.begin(), envelopes.end());\n return solve(0, -1, envelopes, n);\n }\n\t\t\n**2. Recursion + Memoization\nCODE:**\n\n\t// Memoization *** Will Give TLE ***\n int solve(int idx, int prev, vector<vector<int>> &arr, int n, vector<vector<int>>&dp) {\n if(idx == n)\n return 0;\n int take = 0, untake = 0;\n if(dp[idx][prev+1] != -1)\n return dp[idx][prev+1];\n untake = solve(idx+1, prev, arr, n, dp);\n if((prev == -1) || (arr[idx][0] > arr[prev][0] && arr[idx][1] > arr[prev][1])) {\n take = 1 + solve(idx+1, idx, arr, n, dp);\n }\n return dp[idx][prev+1] = max(take, untake);\n }\n \n int maxEnvelopes(vector<vector<int>>& envelopes) {\n int n = envelopes.size();\n sort(envelopes.begin(), envelopes.end());\n vector<vector<int>>dp(n, vector<int>(n+1, -1));\n return solve(0, -1, envelopes, n, dp);\n }\n\t\t\n\t\t\n**3. Tabulation\nCODE:**\n \n\t // Tabulation *** Will Give TLE ***\n\t\tint maxEnvelopes(vector<vector<int>>& envelopes) {\n\t\t\tint n = envelopes.size();\n\t\t\tif(n == 0)\n\t\t\t\treturn 0;\n\t\t\tsort(envelopes.begin(), envelopes.end());\n\t\t\tvector<int>dp(n, 1);\n\t\t\tint take = 0, untake = 0;\n\t\t\tfor(int idx = 0; idx < n; idx++) {\n\t\t\t\tfor(int prev = 0; prev < idx; prev++) {\n\t\t\t\t\tif(envelopes[idx][0] > envelopes[prev][0] && envelopes[idx][1] > envelopes[prev][1]) {\n\t\t\t\t\t\tdp[idx] = max(dp[idx], 1 + dp[prev]);\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t\t\treturn *max_element(dp.begin(),dp.end());\n\t\t}\n\t\t\n\t\t\n**4. Binary Search Approach\nCODE:**\n\n\t // Binary Search\n\t\t static bool comp(const vector<int> &a, const vector<int> &b) {\n\t\t\tif(a[0] < b[0])\n\t\t\t\treturn true;\n\t\t\telse if(a[0] > b[0])\n\t\t\t\treturn false;\n\t\t\telse if(a[1] > b[1])\n\t\t\t\treturn true;\n\t\t\telse\n\t\t\t\treturn false;\n\t\t } \n\t\t int maxEnvelopes(vector<vector<int>>& envelopes) {\n\t\t\tsort(envelopes.begin(), envelopes.end(), comp);\n\t\t\tvector<int> dp;\n\t\t\tfor (auto it : envelopes)\n\t\t\t{\n\t\t\t\tauto iter = lower_bound(dp.begin(), dp.end(), it[1]);\n\t\t\t\tif (iter == dp.end())\n\t\t\t\t\tdp.push_back(it[1]);\n\t\t\t\telse if (it[1] < *iter)\n\t\t\t\t\t*iter = it[1];\n\t\t\t}\n\t\t\treturn dp.size();\n\t\t}\n | 3 | 0 | ['Binary Search', 'Dynamic Programming'] | 0 |
russian-doll-envelopes | ✅ JAVA | SORTING + LIS | TLE | MLE | O(N^2) | O(N LOGN) | 👍🏼 | | java-sorting-lis-tle-mle-on2-on-logn-by-xcd9f | COUPLE OF SOLUTIONS IN JAVA\n Below is Naive approach which throws TLE.\n \nclass Solution {\n \n private int MAX_VALUE = 100002;\n \n public in | bharathkalyans | NORMAL | 2022-05-25T07:20:20.398042+00:00 | 2022-05-25T07:22:32.491276+00:00 | 531 | false | __COUPLE OF SOLUTIONS IN JAVA__\n* Below is Naive approach which throws TLE.\n ```\nclass Solution {\n \n private int MAX_VALUE = 100002;\n \n public int maxEnvelopes(int[][] envelopes) {\n Arrays.sort(envelopes, (a, b) -> a[0] - b[0]);\n int[] prev = new int[]{MAX_VALUE, MAX_VALUE};\n return LIS(envelopes, envelopes.length - 1, prev);\n }\n \n private int LIS(int[][] envelopes, int index, int[] prev){\n if(index == 0){\n return (envelopes[0][0] < prev[0] && envelopes[0][1] < prev[1]) ? 1 : 0;\n }\n int exclude = LIS(envelopes, index - 1, prev);\n int include = 0;\n if(envelopes[index][0] < prev[0] && envelopes[index][1] < prev[1]){\n include = 1 + LIS(envelopes, index - 1, envelopes[index]);\n }\n\n return Math.max(exclude, include);\n }\n}\n ```\n * Below is Memoized approach which throws MLE.\n ```\n class Solution {\n \n private int MAX_VALUE = 100002;\n \n public int maxEnvelopes(int[][] envelopes) {\n Arrays.sort(envelopes, (a, b) -> a[0] - b[0]);\n int[] prev = new int[]{MAX_VALUE, MAX_VALUE};\n int[][][] dp = new int[envelopes.length][MAX_VALUE + 1][MAX_VALUE + 1];\n \n for(int[][] grid : dp)\n for(int[] row : grid)\n Arrays.fill(row, -1);\n \n return LIS(envelopes, envelopes.length - 1, prev, dp);\n }\n \n private int LIS(int[][] envelopes, int index, int[] prev, int[][][] dp){\n if(index == 0){\n return (envelopes[0][0] < prev[0] && envelopes[0][1] < prev[1]) ? 1 : 0;\n }\n \n if(dp[index][prev[0]][prev[1]] != -1) return dp[index][prev[0]][prev[1]];\n int exclude = LIS(envelopes, index - 1, prev, dp);\n int include = 0;\n if(envelopes[index][0] < prev[0] && envelopes[index][1] < prev[1]){\n include = 1 + LIS(envelopes, index - 1, envelopes[index], dp);\n }\n\n return dp[index][prev[0]][prev[1]] = Math.max(exclude, include);\n }\n \n}\n ```\n * Below is Optmized approach which must pass all cases but sadly passes oly 85/87 TC and also throws TLE\uD83E\uDD72.\n ```\n class Solution {\n \n private int MAX_VALUE = 100002;\n \n public int maxEnvelopes(int[][] envelopes) {\n int n = envelopes.length, maxNumberOfEnvelopes = 1;\n \n // We sort According to Width!!\n Arrays.sort(envelopes, (a, b) -> a[0] - b[0]);\n \n for(int[] envelope : envelopes)\n System.out.println(envelope[0] + " " + envelope[1]);\n \n // We find LIS according to Height!!\n int prev = MAX_VALUE;\n int[] dp = new int[n + 1];\n dp[0] = 1;\n \n for(int i = 1;i < n; i++){\n dp[i] = 1;\n for(int j = 0; j < i; j++)\n if(envelopes[i][0] != envelopes[j][0] && envelopes[i][1] > envelopes[j][1])\n dp[i] = Math.max(dp[i], dp[j] + 1);\n \n maxNumberOfEnvelopes = Math.max(maxNumberOfEnvelopes, dp[i]);\n }\n \n return maxNumberOfEnvelopes;\n }\n \n}\n```\n\n* Below is the Optmized Solution which passes all test cases with TC O(N logN).\uD83E\uDEE1\n```\nclass Solution {\n \n public int maxEnvelopes(int[][] envelopes) {\n int n = envelopes.length, len = 0;\n \n // We sort According to Width!!\n Arrays.sort(envelopes, (a, b) -> a[0] == b[0] ? b[1] - a[1] : a[0] - b[0]);\n \n int dp[] = new int[n];\n for(int[] envelope : envelopes){\n int index = Arrays.binarySearch(dp, 0, len, envelope[1]);\n if(index < 0)\n index = -(index + 1);\n dp[index] = envelope[1];\n if(index == len)\n len++;\n }\n\t\t\n return len;\n } \n}\n```\n \n * Bonus Tip :: Always make helper functions as private[ABSTRACTION].\n * Happy Coding and do Upvote if Helpful!\n \n author : [@bharathkalyans](https://leetcode.com/bharathkalyans/)\nlinkedin : [@bharathkalyans](https://www.linkedin.com/in/bharathkalyans/)\ntwitter : [@bharathkalyans](https://twitter.com/bharathkalyans)\ngithub : [@bharathkalyans](https://github.com/bharathkalyans/)\n \n | 3 | 0 | ['Dynamic Programming', 'Binary Tree', 'Java'] | 3 |
russian-doll-envelopes | c++ | Russian Doll Envelopes || Simple Solution | c-russian-doll-envelopes-simple-solution-vgrp | Why we need to sort?\n\nIn these types of problem when we are dealing with two dimensions, we need to reduce the problem from two-dimensional array into a one-d | babasaheb256 | NORMAL | 2022-05-25T06:04:05.513829+00:00 | 2022-05-25T06:14:23.307342+00:00 | 357 | false | Why we need to sort?\n\nIn these types of problem when we are dealing with two dimensions, we need to reduce the problem from two-dimensional array into a one-dimensional array in order to improve time complexity.\n\t\n"**Sort first when things are undecided**", sorting can make the data orderly, reduce the degree of confusion, and often help us to sort out our thinking. the same is true with this question. Now, after doing the correct sorting, we just need to find Longest Increasing Subsequence of that one dimensional array.\n\nNow, you may be wondered what correct sorting actually is?\n \nIt is the sorting which we do to order to achieve the answer. Like, increasing, non-increasing sorting. Without any further discussion, let\'s dig into Intuition followed by algorithm.\n\nAlgorithm\n\n* We sort the array in increasing order of width. And if two widths are same, we need to sort height in decreasing order.\n* Now why we need to sort in decreasing order if two widths are same. By this practice, we\'re assuring that no width will get counted more than one time. Let\'s take an example\n* envelopes=[[3, 5], [6, 7], [7, 13], [6, 10], [8, 4], [7, 11]]\n\n* Now, if you see for a while, 6 and 7 is counted twice while we\'re calculating the length of LIS, which will give the wrong ans. As question is asking, if any width/height are less than or equal, then, it is not possible to russian doll these envelopes.\n\n\n* Now, we know the problem. So, how can we tackle these conditions when two width are same, so that it won\'t affect our answer. We can simple reverse sort the height if two width are equal, to remove duplicacy.\n\n* Now, you may question, how reverse sorting the height would remove duplicacy? As the name itself says, Longest Increasing Subsequnce, the next coming height would be less than the previous one. Hence, forbidding it to increase length count.\n\n\t* Breify speaking we sort the envelopes based on width in ascending\n\t\t* After this Envelopes look like [[3, 5], [6, 7],[6, 10], [7, 11] ,[7, 13] , [8, 4]]\n\t* Then we sort those element in decreasing order fro which width is same\n\t\t* After this Envelopes look like [[3, 5], [6, 10],[6, 7], [7, 13] ,[7, 11] , [8, 4]]\n\n\t* **Then we Count length of Longest increasing subsequence based on Height only** i.e {5,10,7,13,11,4}\n\t\t* So it comes out to be 3\n \n \n** If you don\'t understand how LIS is calculated here, I strongly refer you to follow the prerequisite.**\n \nNow, we have sucessfully reduced the problem to LIS! All you need to apply classical LIS on heights, to calculate the ans. This would be the maximum number of envelopes can be russian doll.\n\n\n```\nclass Solution {\npublic:\n \n //This compare function going to pass to sort function it should be static function\n\t\n\t// To understand this think that we take two element adjacent to each other a,b both here are vector \n\t\n\t// Based on what you want right return condition \n/* \n\t\tlike \n\t\t\t1. a<b for ascending ( first element should be less than second ) \n\t\t\t2. a>b for descending ( first element should be greater than second ) \n\n*/\n static bool cmp(vector<int>& a,vector<int>& b)\n {\n if(a[0]==b[0]) //if first val of pair are equal than for a,b than check for second val of pair\n {\n return a[1]>b[1]; // comparing second val of pair in a,b\n }\n \n return a[0]<b[0];\n }\n \n int maxEnvelopes(vector<vector<int>>& envelopes) {\n \n \n \n sort(envelopes.begin(),envelopes.end(),cmp);\n \n vector<int> v;\n \n for(auto i: envelopes)\n {\n auto it= lower_bound(v.begin(),v.end(),i[1]);\n \n if(it== v.end()) // if element greater than i[1] not present than push i[1] here i[1] represent height of envelope\n {\n v.push_back(i[1]);\n }\n else\n {\n *it= i[1]; // if element greater than i[1] not present than replace previous element as it will not impact or LIS \n }\n }\n \n return v.size();\n \n }\n};\n\n\n``` | 3 | 0 | ['C', 'Sorting', 'C++'] | 0 |
russian-doll-envelopes | [C++] Explaination why sort like that works? || LIS + Binary search | c-explaination-why-sort-like-that-works-ts8vj | The question can be confusing even though you know the solution of classic lis using binary search.I recommend learning first the Binary search solution of clas | iShubhamRana | NORMAL | 2022-05-25T03:23:44.363235+00:00 | 2022-05-25T03:41:03.852356+00:00 | 491 | false | The question can be confusing even though you know the solution of classic lis using binary search.I recommend learning first the Binary search solution of classic LIS. \nThe sorting part caused a lot of confusion to me also ;)\n\n**This post focuses on why sorting a particular way yields the solution**\n\nFor a envelope A to go inside B, lengthA < lengthB and widthA < widthB\n1. We cannot take care of both parameters simontaneously, so lets sort according to one paremeter lets say height , and take care of other parameter while forming the solution.\n2. Lets say we have dolls \n\t[2,6] , [2,3], [4,5] , [5,7] ,[8,9], [4,7], [6,9]\napply sort acc to 0\'th index , (we will see how later we need to modify the sort a little bit)\n\t[2,6], [2,3], [4,5], [4,7] , [5,7], [6,9], [8,9]\n\n**general idea behind binary search solution is that if we cant add the current candidate to the end , we should start forming a new solution simultaneously . So lets try this approach on the example and see how it fails**\n[2,6] \n\n[2,6] since [2,3] cant be fitted because of short 1\'st index(call height)\n[2,3]\n\n[2,6] height [4,5] is shorter\n[2,3], [4,5]\n\n[2,6],[4,7]\n[2,3],[4,5], [4,7] height[4,7] big enough to fit in both\n\nWait but we just hit an invalid sequence [2,3],[4,5],[4,7]. This happened because of equal widths of [4,5],[4,7] but why it didnt happen when we considered the dolls [2,6],[2,3]?\n\n**Reason**: If among envelopes having same width , if we consider any envelope having height smaller first then the higher height envelopes will start contributing the solution of smaller ones which is actually invalid.\nBut as we saw in case of [2,3] and [2,6] , [2,3] instead of contributing, started its own new solution.\n**The new new sorting we have now is**\n**sort in increasing according to width and incase of equal widths sort according to the lengths.**\n\nThe rest solution is same as the classic LIS.\n**Hope it helps:) Do upvote**\n```\nclass Solution {\npublic:\n static bool compare(vector<int> &a , vector<int> &b){\n if(a[0]==b[0]) return a[1]>b[1];\n return a[0]<b[0];\n }\n int maxEnvelopes(vector<vector<int>>& envelopes) {\n int n=envelopes.size();\n sort(envelopes.begin(),envelopes.end(),compare);\n \n vector<int> lis;\n for(int i=0;i<n;i++){\n int ht = envelopes[i][1];\n auto idx = lower_bound(lis.begin(),lis.end(),ht)-lis.begin();\n if(idx==lis.size())\n lis.push_back(ht);\n else lis[idx]=ht;\n }\n \n return lis.size();\n }\n};\n``` | 3 | 0 | [] | 0 |
minimum-operations-to-make-the-array-increasing | [Java/Python 3] Straight Forward codes. | javapython-3-straight-forward-codes-by-r-xurf | Use a dummy value of 0 to initialize the prev; \n2. Traverse the input array, compare each element cur with the modified previous element prev; If cur > prev, u | rock | NORMAL | 2021-04-17T16:04:48.515866+00:00 | 2021-04-17T17:59:36.118667+00:00 | 7,935 | false | 1. Use a dummy value of `0` to initialize the `prev`; \n2. Traverse the input array, compare each element `cur` with the **modified** previous element `prev`; If `cur > prev`, update `prev` to the value of `cur` for the prospective comparison in the next iteration; otherwise, we need to increase `cur` to at least `prev + 1` to make the array strictly increasing; in this case, update `prev` by increment of `1`.\n```java\n public int minOperations(int[] nums) {\n int cnt = 0, prev = 0;\n for (int cur : nums) {\n if (cur <= prev) {\n cnt += ++prev - cur;\n }else {\n prev = cur;\n }\n }\n return cnt;\n }\n```\n```python\n def minOperations(self, nums: List[int]) -> int:\n cnt = prev = 0\n for cur in nums:\n if cur <= prev:\n prev += 1\n cnt += prev - cur\n else:\n prev = cur\n return cnt\n```\n**Analysis:**\n\nTime: `O(n)`, space: `O(1)`, where `n = nums.length`. | 67 | 3 | [] | 10 |
minimum-operations-to-make-the-array-increasing | C++ Track Last | c-track-last-by-votrubac-q11e | cpp\nint minOperations(vector<int>& nums) {\n int res = 0, last = 0;\n for (auto n : nums) {\n res += max(0, last - n + 1);\n last = max(n, | votrubac | NORMAL | 2021-04-17T16:48:46.466909+00:00 | 2021-04-17T16:48:46.467025+00:00 | 5,681 | false | ```cpp\nint minOperations(vector<int>& nums) {\n int res = 0, last = 0;\n for (auto n : nums) {\n res += max(0, last - n + 1);\n last = max(n, last + 1);\n }\n return res;\n}\n``` | 62 | 2 | [] | 11 |
minimum-operations-to-make-the-array-increasing | Easy C++ Solution | easy-c-solution-by-anshika_28-201t | Do upvote if useful!\n\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int output=0;\n for(int i=0;i<nums.size()-1;i++){\ | anshika_28 | NORMAL | 2021-05-02T08:19:27.465721+00:00 | 2021-05-02T08:19:27.465752+00:00 | 3,895 | false | **Do upvote if useful!**\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int output=0;\n for(int i=0;i<nums.size()-1;i++){\n if(nums[i]<nums[i+1])\n continue;\n else{\n output=output+(nums[i]+1-nums[i+1]);\n nums[i+1]=nums[i]+1;\n }\n }\n return output;\n }\n};\n``` | 47 | 3 | ['C', 'C++'] | 5 |
minimum-operations-to-make-the-array-increasing | 100% 1ms Java Solution | 100-1ms-java-solution-by-rentx-v5i4 | Using num as the latest value to compare with next nums[i], instead of increasing nums[i] and using it to compare with nums[i+1]. Changing the value of nums[i] | rentx | NORMAL | 2021-05-26T23:02:02.469589+00:00 | 2021-05-26T23:02:02.469622+00:00 | 2,839 | false | Using num as the latest value to compare with next nums[i], instead of increasing nums[i] and using it to compare with nums[i+1]. Changing the value of nums[i] costs more time than changing the value of the variable num. \n\n```\nclass Solution {\n public int minOperations(int[] nums) {\n if (nums.length <= 1) {\n return 0;\n }\n\n int count = 0;\n int num = nums[0];\n for (int i = 1; i < nums.length; i++) {\n if (num == nums[i]) {\n count++;\n num++;\n } else if (num > nums[i]) {\n num++;\n count += num - nums[i];\n } else {\n num = nums[i];\n }\n }\n \n return count;\n }\n}\n``` | 21 | 0 | ['Java'] | 6 |
minimum-operations-to-make-the-array-increasing | Python3 simple solution beats 90% users | python3-simple-solution-beats-90-users-b-ai7s | \nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n count = 0\n for i in range(1,len(nums)):\n if nums[i] <= nums | EklavyaJoshi | NORMAL | 2021-04-27T03:09:05.660999+00:00 | 2021-04-27T03:09:05.661030+00:00 | 2,065 | false | ```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n count = 0\n for i in range(1,len(nums)):\n if nums[i] <= nums[i-1]:\n x = nums[i]\n nums[i] += (nums[i-1] - nums[i]) + 1\n count += nums[i] - x\n return count\n```\n**If you like this solution, please upvote for this** | 18 | 2 | ['Python3'] | 1 |
minimum-operations-to-make-the-array-increasing | ✅ Short & Easy Solution w/ Explanation | 4 Lines of Code! | short-easy-solution-w-explanation-4-line-wqhf | \u2714\uFE0F Solution - I\n\nThe problem can be solved as -\n\n1. Just iterate over the array.\n2. If at any point, nums[i] <= nums[i - 1], then we need to incr | archit91 | NORMAL | 2021-04-17T16:03:56.108367+00:00 | 2021-04-17T18:39:27.315639+00:00 | 1,997 | false | \u2714\uFE0F ***Solution - I***\n\nThe problem can be solved as -\n\n1. Just iterate over the array.\n2. If at any point, `nums[i] <= nums[i - 1]`, then we need to increment `nums[i]` to make the array strictly increasing. The number of increments needed is given by - `nums[i - 1] + nums[i] + 1`. Basically, it is the number of increments needed to take `nums[i]` to atleast `nums[i - 1] + 1`.\n3. Return the number of increments.\n\n```\nint minOperations(vector<int>& nums) {\n\tint cnt = 0;\n\tfor(int i = 1; i < size(nums); i++)\n\t\tif(nums[i] <= nums[i - 1]) { \n\t\t\tcnt += (nums[i - 1] - nums[i] + 1), // nums[i] must be made atleast equal to nums[i-1]+1\n\t\t\tnums[i] = nums[i - 1] + 1;\n\t\t}\n\treturn cnt;\n}\n```\n\n***Time Complexity :*** **`O(N)`**\n***Space Complexity :*** **`O(1)`**\n\n---\n\n\u2714\uFE0F ***Solution - II (Shorter Version of Solution - I)***\n\nThe below solution is same as solution - I, just a shorter, more concise version.\n\n```\nint minOperations(vector<int>& nums) {\n\tint cnt = 0;\n\tfor(int i = 1; i < size(nums); i++)\n\t\tcnt += max(0, nums[i - 1] + 1 - nums[i]), nums[i] = max(nums[i], nums[i - 1] + 1);\n\treturn cnt;\n}\n```\n\n---\n--- | 17 | 1 | ['C'] | 5 |
minimum-operations-to-make-the-array-increasing | Python O(n) simple and short solution | python-on-simple-and-short-solution-by-t-o13f | Python :\n\n\ndef minOperations(self, nums: List[int]) -> int:\n\tans = 0\n\n\tfor i in range(1, len(nums)):\n\t\tif nums[i] <= nums[i - 1]:\n\t\t\tans += (nums | TovAm | NORMAL | 2021-11-07T15:42:03.331855+00:00 | 2021-11-07T15:42:03.331896+00:00 | 1,672 | false | **Python :**\n\n```\ndef minOperations(self, nums: List[int]) -> int:\n\tans = 0\n\n\tfor i in range(1, len(nums)):\n\t\tif nums[i] <= nums[i - 1]:\n\t\t\tans += (nums[i - 1] - nums[i] + 1)\n\t\t\tnums[i] = (nums[i - 1] + 1)\n\n\treturn ans\n```\n\n**Like it ? please upvote !** | 13 | 1 | ['Python', 'Python3'] | 1 |
minimum-operations-to-make-the-array-increasing | Easiest Java Solution [ 100% ] [ 2ms ] | easiest-java-solution-100-2ms-by-rajarsh-t260 | Approach\n1. Initialization:\n - Get the length of the array nums and store it in len.\n - Create a new array arr of the same length as nums.\n - Initiali | RajarshiMitra | NORMAL | 2024-06-18T07:01:57.922048+00:00 | 2024-06-18T07:08:03.456238+00:00 | 506 | false | # Approach\n1. **Initialization**:\n - Get the length of the array `nums` and store it in `len`.\n - Create a new array `arr` of the same length as `nums`.\n - Initialize a variable `count` to keep track of the total number of operations.\n\n2. **Copying the Original Array**:\n - Copy each element of `nums` into `arr` to work with a separate array that will be modified.\n\n3. **Ensure Strictly Increasing Order**:\n - Iterate through the `arr` from the first element to the second-to-last element.\n - For each element, check if the next element (`arr[i+1]`) is less than or equal to the current element (`arr[i]`).\n - If this condition is true, update the next element (`arr[i+1]`) to be one more than the current element (`arr[i] + 1`).\n\n4. **Calculate the Total Number of Operations**:\n - Iterate through the `arr` and calculate the difference between each element of `arr` and the corresponding element in `nums`.\n - Add this difference to `count`.\n\n5. **Return the Result**:\n - The `count` now contains the total number of operations needed to make `nums` strictly increasing.\n - Return `count`.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int len=nums.length;\n int arr[]=new int[len];\n int count=0;\n for(int i=0; i<len; i++){\n arr[i] = nums[i];\n }\n for(int i=0; i<len-1; i++){\n if(arr[i+1] <= arr[i]){\n arr[i+1] = arr[i]+1;\n }\n }\n for(int i=0; i<len; i++){\n count+=arr[i]-nums[i];\n }\n return count;\n }\n}\n```\n\n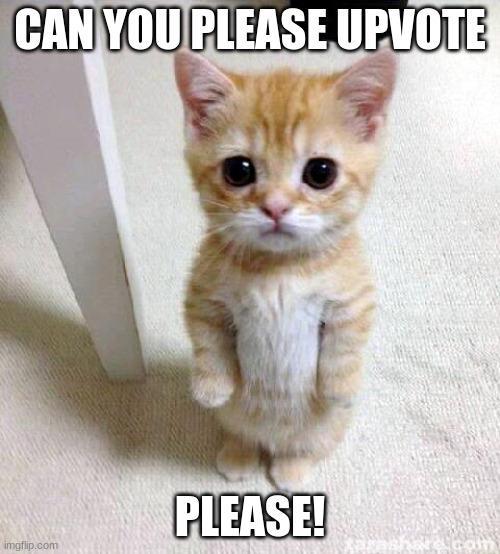\n | 12 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | [Java] - Simple One Pass Solution | java-simple-one-pass-solution-by-makubex-s5ye | Check if the next number (nums[i]), is smaller than current number (nums[i - 1]). If it is smaller, get the difference between current number nums[i - 1] and ne | makubex74 | NORMAL | 2021-04-19T05:53:07.871391+00:00 | 2021-04-20T05:26:43.788207+00:00 | 765 | false | * Check if the next number (```nums[i]```), is smaller than current number (```nums[i - 1]```). If it is smaller, get the difference between current number ```nums[i - 1]``` and next number ```nums[i]``` and add a 1 to it to make the next number strictly higher than current number.\n* Add the computed difference value to ```minOperations```.\n* Modify the next number (```nums[i]```) by adding the ```difference``` found.\n```\npublic int minOperations(int[] nums) {\n\tint minOperations = 0;\n for(int i = 1; i < nums.length; i++) {\n\t\tif(nums[i] > nums[i - 1])\n\t\t\tcontinue;\n\t\tint difference = nums[i - 1] - nums[i] + 1; \n minOperations += difference;\n nums[i] += difference;\n }\n return minOperations;\n}\n```\n**Analysis:**\nTime Complexity: O(n) where n = nums.length.\nSpace Complexity: O(1)\n\t | 9 | 2 | [] | 0 |
minimum-operations-to-make-the-array-increasing | Python [one pass solution] | python-one-pass-solution-by-it_bilim-j8t5 | \nclass Solution(object):\n def minOperations(self, nums):\n res = 0\n for i in range(1,len(nums)):\n if nums[i]<=nums[i-1]:\n | it_bilim | NORMAL | 2021-04-17T17:08:52.059753+00:00 | 2021-04-17T17:08:52.059785+00:00 | 657 | false | ```\nclass Solution(object):\n def minOperations(self, nums):\n res = 0\n for i in range(1,len(nums)):\n if nums[i]<=nums[i-1]:\n diff = nums[i-1]-nums[i]+1\n res += diff\n nums[i] = nums[i-1]+1\n return res\n``` | 9 | 3 | [] | 0 |
minimum-operations-to-make-the-array-increasing | Simple Javascript Solution | simple-javascript-solution-by-nileshsain-v53j | \nvar minOperations = function(nums) {\n if(nums.length < 2) return 0;\n let count = 0;\n for(let i = 1; i<nums.length; i++) {\n if(nums[i] <= nu | nileshsaini_99 | NORMAL | 2021-09-23T12:47:55.930629+00:00 | 2021-09-23T12:47:55.930660+00:00 | 931 | false | ```\nvar minOperations = function(nums) {\n if(nums.length < 2) return 0;\n let count = 0;\n for(let i = 1; i<nums.length; i++) {\n if(nums[i] <= nums[i-1]) {\n let change = nums[i-1] - nums[i] + 1;\n count += change;\n nums[i] += change;\n }\n }\n \n return count;\n};\n``` | 8 | 0 | ['JavaScript'] | 0 |
minimum-operations-to-make-the-array-increasing | [Java] Easy Solution with explanation beats 100% Time: O(n), Space O(1) | java-easy-solution-with-explanation-beat-krno | Approach :\n Start from index 1\n if the current element (nums[i]) is less than the previous element(nums[i-1]) then have the difference count to store the di | vinsinin | NORMAL | 2021-04-17T16:14:43.005909+00:00 | 2021-04-17T16:22:26.801419+00:00 | 1,137 | false | **Approach :**\n* Start from `index 1`\n* if the current element (`nums[i]`) is less than the previous element(`nums[i-1]`) then have the difference `count` to store the difference, also `+1` to make it increasing\n* Add the difference to the result `count += diff`\n* Add the difference to the current element `nums[i] += diff`\n* proceed till the end (`nums.length`)\n* return `count`\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int count = 0;\n for (int i = 1;i<nums.length;i++){\n if (nums[i] <= nums[i-1]) {\n int diff = nums[i-1] - nums[i] + 1;\n count += diff;\n nums[i] += diff;\n }\n }\n return count;\n }\n}\n``` | 7 | 0 | ['Java'] | 2 |
minimum-operations-to-make-the-array-increasing | ✅Easiest Basic C++ solution | Full Explanations with Dry Run | No advance Logiv✅ | easiest-basic-c-solution-full-explanatio-12yz | Very Easy solution..just imagine elements of array as I proceed\n\nSolution:\n\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n i | dev_yash_ | NORMAL | 2024-02-18T08:09:29.189034+00:00 | 2024-02-18T08:09:29.189058+00:00 | 388 | false | Very Easy solution..just imagine elements of array as I proceed\n\n**Solution:**\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int operations=0; //cant keep 1 and do operations++ as for single ele array its inv\n for(size_t i {1};i<nums.size();i++) //keep i=1 not 0 as if condition uses prev ele\n {\n if(nums[i-1]<nums[i])\n continue;\n else\n {\n //first calculate difference between previous and current term\n int diff=nums[i-1]-nums[i];\n diff++;\n \n //now add difference to the current element that is smaller than prev ele\n nums[i]=nums[i]+diff;\n \n //increment count of operations by adding difference\n operations+=diff;\n }\n }\n return operations;\n \n }\n};\n```\n***Test:***\n\nLet\'s go through a dry run of the code using an example. Consider the input array nums = [1, 5, 2, 4, 1]\n\nIteration 1:\n- i = 1\n- nums[1 - 1] = nums[0] = 1\n- nums[1] = 5\n- Since 1 < 5, we continue.\n\nIteration 2:\n- i = 2\n- nums[2 - 1] = nums[1] = 5\n- nums[2] = 2\n- Since 5 >= 2, we proceed with the operation.\n - Calculate the difference: diff = nums[1] - nums[2] + 1 = 5 - 2 + 1 = 4\n - Increment nums[2] by diff: nums[2] += 4 => nums[2] = 6\n - Increment operations by diff: operations += 4 => operations = 4\n\nIteration 3:\n- i = 3\n- nums[3 - 1] = nums[2] = 6\n- nums[3] = 4\n- Since 6 >= 4, we proceed with the operation.\n - Calculate the difference: diff = nums[2] - nums[3] + 1 = 6 - 4 + 1 = 3\n - Increment nums[3] by diff: nums[3] += 3 => nums[3] = 7\n - Increment operations by diff: operations += 3 => operations = 7\n\nIteration 4:\n- i = 4\n- nums[4 - 1] = nums[3] = 7\n- nums[4] = 1\n- Since 7 >= 1, we proceed with the operation.\n - Calculate the difference: diff = nums[3] - nums[4] + 1 = 7 - 1 + 1 = 7\n - Increment nums[4] by diff: nums[4] += 7 => nums[4] = 8\n - Increment operations by diff: operations += 7 => operations = 14\n\nEnd of iterations. Return operations = 14.\n | 6 | 0 | ['Array', 'Greedy', 'C', 'C++'] | 0 |
minimum-operations-to-make-the-array-increasing | [Python3] Easy Solution without Modifying Array | python3-easy-solution-without-modifying-3ssj5 | Modifying mutable input data is bad practice in Python for functions like this, because when using it, the user may not be aware of the changes, and this will l | rgalyeon | NORMAL | 2021-04-17T22:51:59.657645+00:00 | 2021-04-17T22:51:59.657676+00:00 | 725 | false | Modifying mutable input data is bad practice in Python for functions like this, because when using it, the user may not be aware of the changes, and this will lead to subsequent errors.\nWe can solve this problem without modifying a list by using an additional variable (`max_elem`).\n\nAlgo:\n* Initialize variables: `n_operations` with `0` and `max_elem` with `0`.\n* \tIterate over the input array\n\t* \tCompare `cur_elem` with `max_elem`\n\t* \tIf `cur_elem` > `max_elem`:\n\t\t* \tThen we update `max_elem` to the value of `cur_elem`\n\t* If `cur_elem` <= `max_elem`:\n\t\t* Then we **calculate current minimum number of operations** needed for cur_elem to be the maximum of all previous elements (`max_elem - cur_num + 1`)\n\t\t* Add the **current minimum number of operations** to the total (`n_operations`)\n\t\t* And update our current `max_elem` with `max_elem += 1`, because our new maximum is 1 more than the previous one.\n* Return result (`n_operations`) \n\n```Python\ndef minOperations(self, nums: List[int]) -> int:\n n_operations = 0\n max_elem = 0\n \n for cur_num in nums:\n if cur_num <= max_elem:\n n_operations += max_elem - cur_num + 1\n max_elem += 1\n else:\n max_elem = cur_num\n return n_operations\n```\n\n**Complexity:**\n**Time:** Linear `O(n)`\n**Memory:** `O(1)`, we use only constant memory | 6 | 0 | ['Python'] | 1 |
minimum-operations-to-make-the-array-increasing | Python Easy and Efficient Solution . | python-easy-and-efficient-solution-by-as-613p | Intuition\n Describe your first thoughts on how to solve this problem. \nWe have to Return the minimum number of operations needed to make nums strictly increas | Aswin_Bharath | NORMAL | 2023-11-01T05:05:10.815480+00:00 | 2023-11-01T05:05:10.815508+00:00 | 898 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe have to Return the minimum number of operations needed to make nums strictly increasing.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nTaking the previous value and comparing with the current value and increasing the count with the previous value to meet the rule of \n* nums[i] < nums[i+1] for all 0 <= i < nums.length - 1\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe code uses a single loop that iterates through the nums list, and for each element, it performs some constant time operations. Within the loop, there are a few simple conditional checks and arithmetic operations. The time complexity of these operations is linear in the length of the nums list, so the overall time complexity of this code is O(n), where \'n\' is the length of the nums list.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity of this code is primarily determined by the variables used. There are a few integer variables (count and prev) that are used to store intermediate results, and their memory usage does not depend on the size of the nums list. Therefore, the space complexity is O(1), which means it is constant and does not depend on the input size.\n\n\n# Code\n```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n if len(nums)==1:\n return 0\n count = prev= 0\n for i in nums:\n if i <= prev :\n prev += 1\n count += prev-i\n else:\n prev = i\n return count\n``` | 5 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-the-array-increasing | 5 LINE JAVA SOLUTION || Easy peasy lemon squeezy😊 || SIMPLE | 5-line-java-solution-easy-peasy-lemon-sq-hqjv | \n\n# Code\n\nclass Solution {\n public int minOperations(int[] nums) {\n int minimum_operations = 0;\n for(int i=1;i<nums.length;i++){\n | Sauravmehta | NORMAL | 2023-02-13T20:56:58.978116+00:00 | 2023-02-13T20:56:58.978161+00:00 | 841 | false | \n\n# Code\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int minimum_operations = 0;\n for(int i=1;i<nums.length;i++){\n minimum_operations += Math.max(nums[i-1]+1,nums[i]) - nums[i];\n nums[i] = Math.max(nums[i-1]+1,nums[i]);\n }\n return minimum_operations;\n }\n}\n``` | 5 | 0 | ['Java'] | 1 |
minimum-operations-to-make-the-array-increasing | Java Clean Solution & explanation | java-clean-solution-explanation-by-aswin-fh7m | Please \uD83D\uDD3C upvote this post if you find the answer useful & do comment about your thoughts \uD83D\uDCAC\n\n## Explanation\n\nThis is the most clean sol | aswinb | NORMAL | 2021-04-17T16:19:42.097912+00:00 | 2022-05-19T07:08:50.572107+00:00 | 264 | false | **Please** \uD83D\uDD3C **upvote this post if you find the answer useful & do comment about your thoughts** \uD83D\uDCAC\n\n## Explanation\n\nThis is the most clean solution in Java which calculates number of operations along with array updation of the operations to be made.\n\n- At first we check whether the given array contains only one element and return 0\n- Next, we loop for N times to find minOperations\n- We must compare current element and next element, hence we need to make sure that the next element exists\n- Now we check if current element is greater than next element and only then we need to perform operations.\n- We find the number of operations by taking the difference of current and next element and add 1 to make sure that we maintain increasing order in array\n- We also update the array itself, to make sure that we check the next elements properly\n- Finally we return the answer\n- Time complexity - O(N)\n- Space complexity - O(1)\n\n## Java Code\n\n```\nclass Solution {\n public int minOperations(int[] nums) {\n if(nums.length<=1) {\n return 0;\n }\n int ans = 0;\n for(int i=0; i<nums.length; i++) {\n if(i+1>=nums.length) {\n return ans;\n }\n if(nums[i+1] <= nums[i]) {\n ans += nums[i] - nums[i+1] + 1;\n nums[i+1] += nums[i] - nums[i+1] + 1;\n }\n }\n return ans;\n }\n}\n``` | 5 | 1 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | Python Simple Solution | python-simple-solution-by-lokeshsk1-fxvr | \nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n c=0\n for i in range(len(nums)-1):\n if nums[i+1] <= nums[i]: | lokeshsk1 | NORMAL | 2021-04-17T16:09:12.549316+00:00 | 2021-04-18T03:45:34.735632+00:00 | 396 | false | ```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n c=0\n for i in range(len(nums)-1):\n if nums[i+1] <= nums[i]:\n d = (nums[i] + 1) - nums[i+1]\n nums[i+1] += d\n c += d\n return c\n``` | 5 | 1 | [] | 1 |
minimum-operations-to-make-the-array-increasing | Two Solutions ✅|| Equal Complexity || Varied Runtimes ⏱️🚀 || JAVA ☕ | two-solutions-equal-complexity-varied-ru-agma | Intuition\nThe goal is to ensure each element in the array is strictly greater than the previous element. If an element is not greater than the previous one, we | Megha_Mathur18 | NORMAL | 2024-06-14T05:05:32.245752+00:00 | 2024-06-14T05:05:32.245785+00:00 | 345 | false | # Intuition\nThe goal is to ensure each element in the array is strictly greater than the previous element. If an element is not greater than the previous one, we need to increase it to be at least one more than the previous element.\n\n# Approach\n1. Initialize a counter count to keep track of the total number of operations needed.\n1. Iterate through the array starting from the second element.\n1. For each element, check if it is less than or equal to the previous element.\n1. If it is, calculate the difference needed to make it one more than the previous element.\n1. Add this difference to count and update the current element to the new value.\n1. Return count as the result.\n\n# Complexity\n**- Time complexity:** **O(n)**, where n is the length of the array. This is because we only iterate through the array once.\n\n**- Space complexity:** ****O(1)****, as we are using a constant amount of extra space.\n\n# RUNTIME : \nHere we are UPDATING an ARRAY. **Updating** **nums[i] costs more time**.\n\n \n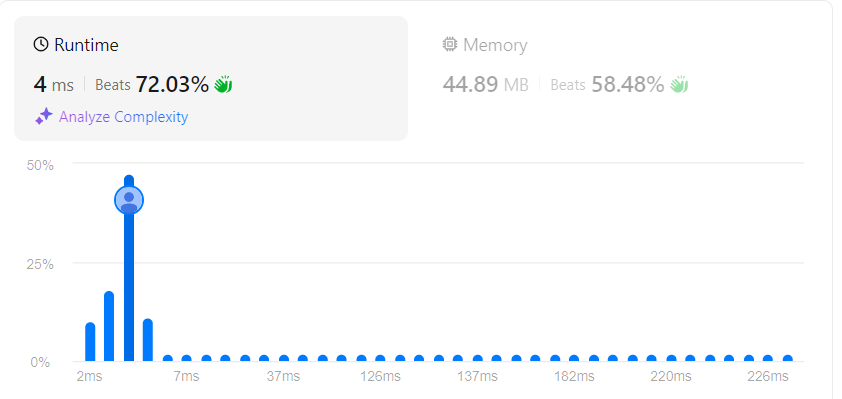\n\n\n\n\n# Code-1 : Updating nums[] -\n```\n class Solution {\n public int minOperations(int[] nums) {\n\n // UPDATING ARRAY -> cost more time\n if(nums.length <= 1) {\n return 0;\n }\n\n int count = 0;\n for(int i=1 ; i<nums.length ; i++) {\n if(nums[i] <= nums[i-1]) {\n count += nums[i-1] + 1 - (nums[i]);\n nums[i] = nums[i-1] + 1;\n }\n }\n\n return count;\n }\n }\n\n```\n\n# Code-2 : Updating variable - \n**Updating** the **value of variable** prev **cost less time** than updating the nums[i].\n\n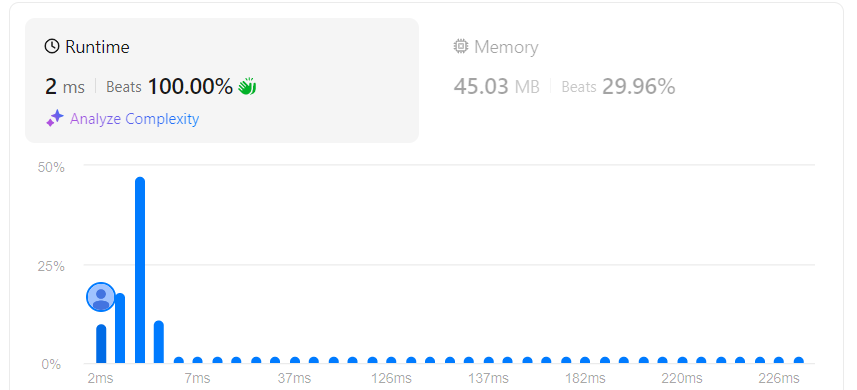\n\n\n```\n\nclass Solution {\n public int minOperations(int[] nums) {\n// UPDATING VARIABLE -> cost less time\n\n if(nums.length == 1) {\n return 0;\n }\n\n int count = 0;\n int prev = nums[0];\n for(int i=1 ; i<nums.length ; i++) {\n if(prev == nums[i]) {\n prev++;\n count++;\n }\n else if(prev > nums[i]) {\n prev++;\n count += prev - (nums[i]);\n }\n else {\n prev = nums[i];\n }\n }\n\n return count;\n }\n}\n```\n\n | 4 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | simple and easy C++ solution 😍❤️🔥 | simple-and-easy-c-solution-by-shishirrsi-3nvv | if it\'s help, please up \u2B06 vote! \u2764\uFE0F\n\n\n# Code\n\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) \n {\n int ans = | shishirRsiam | NORMAL | 2024-06-05T07:17:47.612288+00:00 | 2024-06-05T07:17:47.612307+00:00 | 408 | false | # if it\'s help, please up \u2B06 vote! \u2764\uFE0F\n\n\n# Code\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) \n {\n int ans = 0, n = nums.size();\n vector<int>temp = nums;\n for(int i=0;i<n-1;i++)\n {\n if(temp[i] >= temp[i+1])\n temp[i+1] = temp[i]+1;\n }\n for(int i=0;i<n;i++)\n ans += temp[i] - nums[i];\n return ans;\n }\n};\n``` | 4 | 0 | ['Array', 'Greedy', 'C++'] | 3 |
minimum-operations-to-make-the-array-increasing | Beginner-friendly || Simple solution in Python3/TypeScript | beginner-friendly-simple-solution-in-pyt-iukh | Intuition\nThe problem description is the following:\n- there\'s a list of nums\n- our goal is to make nums strongly increasing\n\nThere\'s no need in sorting. | subscriber6436 | NORMAL | 2023-09-26T22:37:43.547317+00:00 | 2023-09-26T22:37:43.547342+00:00 | 292 | false | # Intuition\nThe problem description is the following:\n- there\'s a list of `nums`\n- our goal is to make `nums` **strongly increasing**\n\nThere\'s no need in sorting. The approach is straightforward and requires to calculate difference between adjacent integers.\n\n# Approach\n1. initialize `ans` to store the answer\n2. iterate over `nums`, check if `nums[i] <= nums[i - 1]`, then calculate `diff` and increment `ans`\n3. return `ans`\n\n# Complexity\n- Time complexity: **O(n)**, to iterate over `nums`\n\n- Space complexity: **O(1)**, we don\'t allocate extra space.\n\n# Code in TypeScript\n```\nfunction minOperations(nums: number[]): number {\n let ans = 0\n\n for (let i = 1; i < nums.length; i++) {\n if (nums[i] <= nums[i - 1]) {\n const diff = Math.abs(nums[i] - nums[i - 1]) + 1\n nums[i] += diff \n ans += diff\n }\n }\n\n return ans\n};\n```\n\n# Code in Python3\n```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n ans = 0\n\n for i in range(1, len(nums)):\n if nums[i] <= nums[i - 1]:\n diff = abs(nums[i] - nums[i - 1]) + 1\n nums[i] += diff\n ans += diff\n\n return ans\n``` | 4 | 0 | ['Array', 'Greedy', 'Python3'] | 1 |
minimum-operations-to-make-the-array-increasing | Full Accuracy python3 | full-accuracy-python3-by-ganjinaveen-spzs | \n\n# Find difference between two elements and add 1\n\nclass Solution(object):\n def minOperations(self, nums):\n count = current= 0\n for n i | GANJINAVEEN | NORMAL | 2023-02-08T18:23:48.687612+00:00 | 2023-03-20T17:48:26.895032+00:00 | 260 | false | \n\n# Find difference between two elements and add 1\n```\nclass Solution(object):\n def minOperations(self, nums):\n count = current= 0\n for n in nums:\n if n <= current:\n current += 1\n count += current - n\n else:\n current = n\n return count\n #please upvote me it would encourage me alot\n\n \n```\n# please upvote me it would encourage me alot\n | 4 | 0 | ['Python'] | 0 |
minimum-operations-to-make-the-array-increasing | Java solution 2ms | java-solution-2ms-by-saha_souvik-jg5q | Intuition\nFor every element, which is less than or equal to its predecessor, perform the required no. of make it strictly increasing\n\n# Approach\nTraverse th | Saha_Souvik | NORMAL | 2023-01-25T13:44:48.780944+00:00 | 2023-01-25T13:44:48.780997+00:00 | 761 | false | # Intuition\nFor every element, which is less than or equal to its predecessor, perform the required no. of make it strictly increasing\n\n# Approach\nTraverse the array from left to right, for every element, ```if nums[i] <= nums[i-1] ```, then we increment the i-th element and add the required no.of steps to the result.\n\n# Complexity\n- Time complexity:\nO(N)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int ans=0;\n for(int i=1; i<nums.length; i++){\n if(nums[i] > nums[i-1]) continue;\n ans += (nums[i-1]-nums[i]+1);\n nums[i] = nums[i-1]+1;\n }\n return ans;\n }\n}\n``` | 4 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | JavaScript faster than 85% | javascript-faster-than-85-by-seredulichk-e35p | \nconst minOperations = nums => {\n let prevEl = nums[0];\n let counter = 0;\n \n for (let i = 0; i < nums.length - 1; i += 1) {\n if (nums[i | seredulichka | NORMAL | 2022-08-25T12:04:02.956745+00:00 | 2022-08-25T12:04:02.956778+00:00 | 562 | false | ```\nconst minOperations = nums => {\n let prevEl = nums[0];\n let counter = 0;\n \n for (let i = 0; i < nums.length - 1; i += 1) {\n if (nums[i + 1] <= prevEl) {\n const diff = prevEl + 1 - nums[i+1] \n counter += diff\n prevEl += 1\n } else {\n prevEl = nums[i+1]\n }\n }\n\n return counter;\n};\n``` | 4 | 0 | ['JavaScript'] | 0 |
minimum-operations-to-make-the-array-increasing | 100% Faster and 100% Less Space. Easy Solution in Python | 100-faster-and-100-less-space-easy-solut-6sjl | ```class Solution:\n def minOperations(self, nums: List[int]) -> int:\n base = nums[0]\n count = 0\n for i in range(1,len(nums)):\n | shivamsingh99 | NORMAL | 2021-04-18T14:08:52.754126+00:00 | 2021-04-18T19:56:07.217995+00:00 | 598 | false | ```class Solution:\n def minOperations(self, nums: List[int]) -> int:\n base = nums[0]\n count = 0\n for i in range(1,len(nums)):\n if nums[i] < base:\n x = base - nums[i] + 1\n count += x\n nums[i] += x\n base = nums[i]\n elif nums[i] > base:\n base = nums[i]\n else:\n nums[i] += 1\n base = nums[i]\n count += 1\n return count | 4 | 1 | ['Python'] | 3 |
minimum-operations-to-make-the-array-increasing | python 3 solution beats 99.8% TIME , O(1) SPACE used | python-3-solution-beats-998-time-o1-spac-b6a8 | stats\n Describe your first thoughts on how to solve this problem. \n\n\n\n# Approach\n Describe your approach to solving the problem. \nwe can have a temporary | Sumukha_g | NORMAL | 2023-08-10T12:59:19.808820+00:00 | 2023-08-10T12:59:19.808844+00:00 | 471 | false | # stats\n<!-- Describe your first thoughts on how to solve this problem. -->\n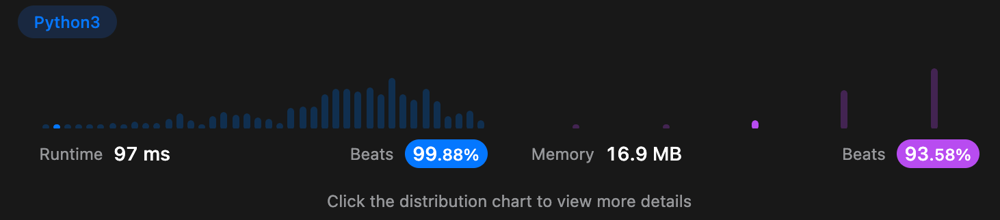\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nwe can have a temporary variable s and a counting variable c\ns initialized with first element of array\nif s is greater than or equal to current elemnet then we take difference between s and current lement and add 1 to make it number 1 greater than s\n` 5 2` , `s=5`,`c added to 5-2+1`,`s=6` the next element\nelse s is updated to new element \nreturning cdoes the job\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n**________O(N)**\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n**________O(1)**\n# Code\n```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n c=0\n s=nums[0]\n for i in range(1,len(nums)):\n if s>=nums[i]:\n c+=s-nums[i]+1\n s+=1\n else:\n s=nums[i]\n return c\n``` | 3 | 0 | ['Array', 'Python3'] | 2 |
minimum-operations-to-make-the-array-increasing | Best approach in JAVA,C++,PYTHON,GO with 0ms runtime!! | best-approach-in-javacpythongo-with-0ms-urhkn | \n\n# Approach\nThe given code defines a class named "Solution" with a member function named "minOperations". This function takes a vector of integers "nums" as | madhavbsnl013 | NORMAL | 2023-07-02T09:13:27.691516+00:00 | 2023-07-02T09:14:15.364711+00:00 | 596 | false | \n\n# Approach\nThe given code defines a class named "Solution" with a member function named "minOperations". This function takes a vector of integers "nums" as input and returns an integer.\n\nThe purpose of the function is to calculate the minimum number of operations required to make the elements in the input vector strictly increasing. An operation involves incrementing an element by a certain amount to make it greater than the next element.\n\nHere\'s how the function works:\n\n1. It declares an integer variable named "count" and initializes it to 0. This variable keeps track of the total number of operations performed.\n\n2. The function enters a for loop that iterates over each element in the input vector "nums", except for the last element.\n\n3. Inside the loop, it checks if the current element "nums[i]" is greater than or equal to the next element "nums[i+1]". If it is, it means that an operation is required to make the elements strictly increasing.\n\n4. If an operation is needed, the function calculates the difference between the current element and the next element plus 1, and adds it to the "count" variable. This accounts for the number of increments required to make the current element greater than the next element.\n\n5. Additionally, it updates the next element "nums[i+1]" to be equal to the current element plus 1. This ensures that the next element is greater than the current element after the operation is performed.\n\n6. If the current element is already less than the next element, the code continues to the next iteration of the loop.\n\n7. After the loop completes, the function returns the value of the "count" variable, which represents the minimum number of operations required to make the elements in the vector strictly increasing.\n\nIn summary, the "minOperations" function calculates the minimum number of operations needed to transform the elements in the input vector into a strictly increasing sequence. It iterates through the vector, performs operations when necessary, and keeps track of the total number of operations performed.\n\n\n# Code\n\n```java []\nimport java.util.List;\n\nclass Solution {\n public int minOperations(List<Integer> nums) {\n int count = 0;\n for (int i = 0; i < nums.size() - 1; i++) {\n if (nums.get(i) >= nums.get(i + 1)) {\n count += nums.get(i) - nums.get(i + 1) + 1;\n nums.set(i + 1, nums.get(i) + 1);\n }\n }\n return count;\n }\n}\n\n```\n```C++ []\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int count=0;\n for(int i=0;i<nums.size()-1;i++){\n if(nums[i]>=nums[i+1]){\n count=count+nums[i]-nums[i+1]+1;\n nums[i+1]=nums[i]+1;\n }\n else\n continue;\n }\n return count;\n \n \n }\n};\n```\n```Python []\nclass Solution:\n def minOperations(self, nums):\n count = 0\n for i in range(len(nums) - 1):\n if nums[i] >= nums[i + 1]:\n count += nums[i] - nums[i + 1] + 1\n nums[i + 1] = nums[i] + 1\n return count\n\n```\n```Go []\npackage main\n\nimport (\n\t"fmt"\n)\n\ntype Solution struct{}\n\nfunc (s Solution) minOperations(nums []int) int {\n\tcount := 0\n\tfor i := 0; i < len(nums)-1; i++ {\n\t\tif nums[i] >= nums[i+1] {\n\t\t\tcount += nums[i] - nums[i+1] + 1\n\t\t\tnums[i+1] = nums[i] + 1\n\t\t}\n\t}\n\treturn count\n}\n\nfunc main() {\n\ts := Solution{}\n\tnums := []int{1, 3, 1, 1, 2, 5, 2, 2}\n\tresult := s.minOperations(nums)\n\tfmt.Println("Minimum Operations:", result)\n}\n\n```\n | 3 | 0 | ['Greedy', 'Python', 'C++', 'Java', 'Go'] | 0 |
minimum-operations-to-make-the-array-increasing | Easy solution | easy-solution-by-wtfcoder-41i8 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | wtfcoder | NORMAL | 2023-06-23T14:24:28.103258+00:00 | 2023-06-23T14:24:28.103289+00:00 | 343 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\nPlease upvote if you find it helpful \n\n# Code\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int count = 0; \n for(int i=1; i<nums.size(); i++) if(nums[i] <= nums[i-1]) { count += nums[i-1]+1-nums[i]; nums[i] = nums[i-1]+1; }\n\n return count; \n }\n};\n``` | 3 | 0 | ['C++'] | 0 |
minimum-operations-to-make-the-array-increasing | FASTEST way with JAVA(2ms) | fastest-way-with-java2ms-by-amin_aziz-h20u | Code\n\nclass Solution {\n public int minOperations(int[] nums) {\n int count = 0;\n int dep = nums[0];\n for(int a = 0; a < nums.leng | amin_aziz | NORMAL | 2023-04-13T06:56:56.681525+00:00 | 2023-04-13T06:56:56.681563+00:00 | 621 | false | # Code\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int count = 0;\n int dep = nums[0];\n for(int a = 0; a < nums.length-1; a++){\n if(dep>=nums[a+1]){\n dep++;\n count += dep-nums[a+1];\n }else{\n dep = nums[a+1];\n }\n }\n return count;\n }\n}\n``` | 3 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | 8 lines solution using python3 simplest of all | 8-lines-solution-using-python3-simplest-khs9x | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Mohammad_tanveer | NORMAL | 2023-03-20T17:13:55.132520+00:00 | 2023-03-20T17:13:55.132562+00:00 | 668 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n ans=0\n for i in range(len(nums)-1):\n if nums[i]>=nums[i+1]:\n ans=ans+nums[i]-nums[i+1]+1\n nums[i+1]=nums[i]+1\n else:\n ans+=0\n return ans\n\n\n``` | 3 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-the-array-increasing | Java | Array | Easy Approach | java-array-easy-approach-by-divyansh__26-nz6i | \nclass Solution {\n public int minOperations(int[] nums) {\n int count=0;\n for(int i=1;i<nums.length;i++){\n if(nums[i]<=nums[i-1] | Divyansh__26 | NORMAL | 2022-09-14T15:45:48.037494+00:00 | 2022-09-14T15:45:48.037528+00:00 | 645 | false | ```\nclass Solution {\n public int minOperations(int[] nums) {\n int count=0;\n for(int i=1;i<nums.length;i++){\n if(nums[i]<=nums[i-1]){\n count=count+nums[i-1]-nums[i]+1;\n nums[i]=nums[i-1]+1;\n }\n }\n return count;\n }\n}\n```\nKindly upvote if you like the code. | 3 | 0 | ['Array', 'Java'] | 0 |
minimum-operations-to-make-the-array-increasing | easy python code | easy-python-code-by-dakash682-wj5h | \nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n count = 0\n for i in range(len(nums)-1):\n if nums[i] >= nums | dakash682 | NORMAL | 2022-04-09T03:26:57.696625+00:00 | 2022-04-09T03:26:57.696671+00:00 | 409 | false | ```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n count = 0\n for i in range(len(nums)-1):\n if nums[i] >= nums[i+1]:\n count += (nums[i]+1)-nums[i+1]\n nums[i+1] = nums[i]+1\n return count\n```\nhope it helped you,\nif it did, plz consider **upvote** | 3 | 0 | ['Python', 'Python3'] | 0 |
minimum-operations-to-make-the-array-increasing | C++ Easy to understand 4 Lines only | c-easy-to-understand-4-lines-only-by-nex-a5ak | \nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int res = 0, last = 0;\n for (auto n : nums) {\n res += max(0, last - | NextThread | NORMAL | 2022-01-04T15:14:46.273550+00:00 | 2022-01-04T15:14:46.273585+00:00 | 226 | false | ```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int res = 0, last = 0;\n for (auto n : nums) {\n res += max(0, last - n + 1);\n last = max(n, last + 1);\n }\n return res;\n } \n};\n``` | 3 | 0 | ['C'] | 1 |
minimum-operations-to-make-the-array-increasing | Easy Readable Solution | easy-readable-solution-by-himanshuchhika-c1gk | Explanation:\ngoal is to find the minimum number of operation so if we are going to perform operation i.e if case is nums[i-1]>=nums[i] then make make nums[i]=n | himanshuchhikara | NORMAL | 2021-04-17T16:13:40.724320+00:00 | 2021-04-17T16:41:01.820694+00:00 | 181 | false | **Explanation:**\ngoal is to find the minimum number of operation so if we are going to perform operation i.e if case is nums[i-1]>=nums[i] then make make nums[i]=nums[i-1]+1. So cost to make nums[i] equal to (nums[i-1]+1) is nums[i-1] - nums[i] + 1.\n\n**CODE:**\n```\n public int minOperations(int[] nums) {\n int operations=0;\n int prev=nums[0];\n \n for(int i=1;i<nums.length;i++){\n int curr=nums[i];\n if(curr>prev){\n prev=curr;\n continue; // nothing to do\n }\n operations+=prev-curr+1;\n prev=prev+1;\n }\n return operations;\n }\n```\n\n**Complexity:**\n`Time:O(n) and Space:O(1)`\n\nPlease **UPVOTE** if found it helpful and feel free to reach out to me or comment down if you have any doubt. | 3 | 1 | ['Java'] | 1 |
minimum-operations-to-make-the-array-increasing | Solution | solution-by-ftm_v20-ncnm | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | ftm_v20 | NORMAL | 2024-07-02T14:51:07.001079+00:00 | 2024-07-02T14:51:07.001108+00:00 | 250 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n c=0\n for i in range(1,len(nums)):\n if nums[i]<=nums[i-1]:\n diff=nums[i-1]-nums[i]+1\n nums[i]=nums[i-1]+1\n c+=diff\n return c\n``` | 2 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-the-array-increasing | Rust || O(n) || 0ms | rust-on-0ms-by-user7454af-4iwg | Complexity\n- Time complexity: O(n)\n\n- Space complexity: O(1)\n\n# Code\n\nimpl Solution {\n pub fn min_operations(mut nums: Vec<i32>) -> i32 {\n le | user7454af | NORMAL | 2024-06-06T02:37:37.205904+00:00 | 2024-06-06T02:37:37.205937+00:00 | 206 | false | # Complexity\n- Time complexity: $$O(n)$$\n\n- Space complexity: $$O(1)$$\n\n# Code\n```\nimpl Solution {\n pub fn min_operations(mut nums: Vec<i32>) -> i32 {\n let mut ans = 0;\n for i in 1..nums.len() {\n if nums[i] <= nums[i-1] {\n let change = nums[i-1] - nums[i] + 1;\n ans += change;\n nums[i] += change;\n }\n }\n ans\n }\n}\n``` | 2 | 0 | ['Rust'] | 0 |
minimum-operations-to-make-the-array-increasing | Easy C++ Solution | With Explaination | easy-c-solution-with-explaination-by-vai-fjnv | \nclass Solution {\npublic:\nint minOperations(vector<int>& nums) {\n int count=0;\n\t\t//Loop through the vector starting from the second element\n | vaibhavS_07 | NORMAL | 2024-03-06T22:26:49.259036+00:00 | 2024-03-06T22:28:20.017163+00:00 | 595 | false | ```\nclass Solution {\npublic:\nint minOperations(vector<int>& nums) {\n int count=0;\n\t\t//Loop through the vector starting from the second element\n for(int i=1;i<nums.size();i++){\n\t\t //Check if the current element is less than or equal to the previous one\n if(nums[i]<=nums[i-1]){\n\t\t\t //Increment count by the difference between the previous and current elements plus 1\n count+=nums[i-1]-nums[i]+1;\n\t\t\t\t//Increase the current element by the calculated difference\n nums[i]+=nums[i-1]-nums[i]+1;\n }\n }\n return count;\n }\n};\n``` | 2 | 0 | ['C'] | 0 |
minimum-operations-to-make-the-array-increasing | Super Easy || upvote if u like :) | super-easy-upvote-if-u-like-by-hrugved00-mzwk | \n\n# Code\n\nclass Solution {\n public int minOperations(int[] nums) {\n int step=0;\n for(int i=1;i<nums.length;i++){\n if(nums[i] | hrugved001 | NORMAL | 2023-06-16T06:42:21.871038+00:00 | 2023-06-16T06:42:21.871066+00:00 | 260 | false | \n\n# Code\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int step=0;\n for(int i=1;i<nums.length;i++){\n if(nums[i]<=nums[i-1]){\n step+=Math.abs(nums[i]-nums[i-1])+1;\n nums[i]=nums[i-1]+1;\n }\n }\n return step;\n }\n}\n``` | 2 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | Simple JAVA Solution for beginners. 2ms. Beats 95.3%. | simple-java-solution-for-beginners-2ms-b-4m98 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | sohaebAhmed | NORMAL | 2023-05-09T03:29:14.941866+00:00 | 2023-05-09T03:29:14.941903+00:00 | 307 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int count = 0;\n for(int i = 1; i < nums.length; i++) {\n if(nums[i] > nums[i - 1]) {\n continue;\n }\n count += nums[i - 1] - nums[i] + 1;\n nums[i] = nums[i - 1] + 1;\n }\n return count;\n }\n}\n``` | 2 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | C++ Solution || Simple Approach | c-solution-simple-approach-by-asad_sarwa-3k10 | Complexity\n- Time complexity: O(n)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity: O(1)\n Add your space complexity here, e.g. O(n) \n\n# Co | Asad_Sarwar | NORMAL | 2023-03-24T11:52:10.114206+00:00 | 2023-03-24T11:52:10.114238+00:00 | 1,283 | false | # Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n\n if(nums.size()==1)\n return 0;\n int n=nums.size();\n int count = 0;\n for (int i = 1; i < n; i++)\n {\n if (nums[i] <= nums[i - 1])\n {\n count += abs(nums[i - 1] + 1 - nums[i]);\n nums[i] = nums[i - 1] + 1;\n }\n }\n return count;\n\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
minimum-operations-to-make-the-array-increasing | Simple C++ code || beats 94.88% and 84.15% ✌ | simple-c-code-beats-9488-and-8415-by-kra-ozol | \n# Approach\n Describe your approach to solving the problem. \niterates through index =1, checks if the element in the current index is smaller than the elemen | Kraken_02 | NORMAL | 2023-02-07T04:48:07.598352+00:00 | 2023-02-07T04:48:07.598399+00:00 | 715 | false | \n# Approach\n<!-- Describe your approach to solving the problem. -->\niterates through index =1, checks if the element in the current index is smaller than the element in the previous one, if it is then adds value equal to previousElement - currentElement + 1 , also stores the increased number to a counter to return later, \n\nthat\'s all , \u270C\n\n- Time Complexity: O(n)\n\n- Runtime: Beats 94.88%\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n\n- Memory: Beats 84.15%\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int c=0;\n int n=nums.size();\n\n for(int i=1;i<n;i++){\n if(nums[i]<=nums[i-1]){\n c=c+(nums[i-1]-nums[i])+1;\n nums[i]=nums[i]+(nums[i-1]-nums[i])+1;\n }\n }\n return c;\n }\n};\n```\n\nHope you liked the implementation of the code, if you like it feel free to upvote \uD83D\uDC4D\nKeep Coding , Keep Chilling | 2 | 0 | ['C++'] | 1 |
minimum-operations-to-make-the-array-increasing | C++ Solution | c-solution-by-mayuri_goswami-3e39 | Intuition\nFor every element, which is less than or equal to its predecessor, we will perform the required number of operations to make it strictly increasing.\ | Mayuri_Goswami | NORMAL | 2023-01-28T03:07:45.922674+00:00 | 2023-01-28T03:07:45.922717+00:00 | 1,271 | false | # Intuition\nFor every element, which is less than or equal to its predecessor, we will perform the required number of operations to make it strictly increasing.\n\n# Approach\nFirst we will declare a variable m, and will assign the nums[0] value to it. Now, traverse the array from left to right, for every element, if nums[i] <= m , then we increment the i-th element and add the required no.of steps to the result.\n\n# Complexity\n- Time complexity:\n O(N)\n\n- Space complexity:\n O(1)\n\n# Code\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n if(nums.size()==1)\n return 0;\n int ans=0, m=nums[0];\n for(int i=1; i<nums.size(); i++){\n if(nums[i]<=m){\n ans+=(m+1-nums[i]);\n m+=1;\n }\n else{\n m=nums[i];\n }\n }\n return ans;\n }\n};\n``` | 2 | 0 | ['C++'] | 1 |
minimum-operations-to-make-the-array-increasing | Java&Javascript Solution (JW) | javajavascript-solution-jw-by-specter01w-7d07 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | specter01wj | NORMAL | 2023-01-26T15:49:27.023462+00:00 | 2023-01-26T15:49:27.023510+00:00 | 193 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\nJava:\n```\npublic int minOperations(int[] nums) {\n int cnt = 0, prev = 0;\n for (int cur : nums) {\n if (cur <= prev) {\n cnt += ++prev - cur;\n } else {\n prev = cur;\n }\n }\n return cnt;\n}\n```\nJavascript:\n```\n/**\n * @param {number[]} nums\n * @return {number}\n */\nvar minOperations = function(nums) {\n let cnt = 0, prev = 0;\n for (let cur of nums) {\n if (cur <= prev) {\n cnt += ++prev - cur;\n } else {\n prev = cur;\n }\n }\n return cnt; \n};\n``` | 2 | 0 | ['Java', 'JavaScript'] | 0 |
minimum-operations-to-make-the-array-increasing | C++ easy solution TC:O(n) SC:O(n) | c-easy-solution-tcon-scon-by-om_limbhare-ttpz | \nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n if(nums.size()==1) return 0;\n int count=0,sum=0;\n for(int i=1;i | om_limbhare | NORMAL | 2022-11-24T22:25:48.756143+00:00 | 2022-11-24T22:25:48.756190+00:00 | 353 | false | ```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n if(nums.size()==1) return 0;\n int count=0,sum=0;\n for(int i=1;i<nums.size();i++){\n if(nums[i]<=nums[i-1]){\n count+=nums[i-1]+1;\n sum+=count-nums[i];\n nums[i]=count;\n count=0;\n }\n }\n return sum;\n }\n};\n``` | 2 | 0 | ['C', 'C++'] | 0 |
minimum-operations-to-make-the-array-increasing | [ C++ ] - Runtime : 8 ms - 99.66 % faster || Easy Solution | c-runtime-8-ms-9966-faster-easy-solution-ahnw | Code\n\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int sum=0;\n for(int i=0;i<nums.size()-1;i++){\n if(num | ideepakchauhan7 | NORMAL | 2022-11-13T14:30:24.482245+00:00 | 2022-11-13T17:21:03.608449+00:00 | 575 | false | # Code\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int sum=0;\n for(int i=0;i<nums.size()-1;i++){\n if(nums[i]>nums[i+1]||nums[i]==nums[i+1]){\n sum+=nums[i]-nums[i+1]+1;\n nums[i+1]+=nums[i]-nums[i+1]+1;\n }\n }\n return sum;\n }\n};\n``` | 2 | 0 | ['Array', 'Greedy', 'C++'] | 0 |
minimum-operations-to-make-the-array-increasing | BEGINNER FRIENDLY || EASY TO UNDERSTAND [JAVA] | beginner-friendly-easy-to-understand-jav-a7ko | \nclass Solution {\n public int minOperations(int[] nums) {\n int count=0;\n \n for(int i=1;i<nums.length;i++) {\n if(nums[i]<= | priyankan_23 | NORMAL | 2022-08-31T16:48:19.218986+00:00 | 2022-08-31T16:48:19.219025+00:00 | 271 | false | ```\nclass Solution {\n public int minOperations(int[] nums) {\n int count=0;\n \n for(int i=1;i<nums.length;i++) {\n if(nums[i]<=nums[i-1]){\n count+=nums[i-1]-nums[i]+1;\n nums[i]+=nums[i-1]-nums[i]+1;\n }\n \n }\n return count;\n }\n}\n``` | 2 | 0 | [] | 0 |
minimum-operations-to-make-the-array-increasing | Java || Easy Fastest Solution || 0ms | java-easy-fastest-solution-0ms-by-ranjit-ehlw | \n int res=0;\n if(nums.length==1){\n return res;\n }\n int val=0;\n for(int i=1;i<nums.length;i++){\n \n if | Ranjit-Kumar-Nayak | NORMAL | 2022-06-16T02:30:57.778511+00:00 | 2022-06-16T02:30:57.778554+00:00 | 216 | false | ```\n int res=0;\n if(nums.length==1){\n return res;\n }\n int val=0;\n for(int i=1;i<nums.length;i++){\n \n if(nums[i-1]>=nums[i]){\n val+=(nums[i-1]- nums[i])+1;\n nums[i]=1+nums[i-1];\n }\n }\n return val;\n``` | 2 | 0 | ['Greedy', 'Java'] | 1 |
minimum-operations-to-make-the-array-increasing | C++ Simple Greedy Solution | O(n) | One-Pass | c-simple-greedy-solution-on-one-pass-by-a1igm | Every element should be atmost 1 greater than the previous element.\nSo, iterate the array & make every element nums[i] = max(nums[i], nums[i-1] + 1) and count | Mythri_Kaulwar | NORMAL | 2022-02-13T15:51:59.122661+00:00 | 2022-02-13T15:51:59.122689+00:00 | 299 | false | Every element should be atmost 1 greater than the previous element.\nSo, iterate the array & make every element ```nums[i] = max(nums[i], nums[i-1] + 1)``` and count the no. of ```1```s to be added to make this change.\n**Code :**\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int count = 0, n = nums.size();\n for(int i=1; i<n; i++){\n int temp = nums[i];\n nums[i] = max(nums[i], nums[i-1]+1);\n count += nums[i]-temp;\n }\n return count;\n }\n};\n``` | 2 | 0 | ['Greedy', 'C', 'C++'] | 0 |
minimum-operations-to-make-the-array-increasing | [ Java ] Beats 100% Very simple to understand solution with explanation | java-beats-100-very-simple-to-understand-g02f | Concept : Since we want a purely increasing array, an element at a[i] if smaller or equal to a[i-1] has to be made at least a[i-1]+1 in order to satisfy our req | KapProDes | NORMAL | 2021-12-09T02:25:29.256184+00:00 | 2021-12-09T02:25:29.256230+00:00 | 205 | false | __Concept__ : Since we want a purely increasing array, an element at __a[i]__ if smaller or equal to __a[i-1]__ has to be made at least __a[i-1]+1__ in order to satisfy our requirements.\n\n__Steps__ : \n1. check __if a[i] <= a[i-1]__. This is the only case where we need to make an operations.\n2. If we find a[i] <= a[i-1] we will increment a[i] precisely by __(a[i-1] - a[i] + 1)__ in order to make strictly increasing.\n3. Continue doing so till the end of the array keep track of the __count__\n4. Note : All previous __increments__ will automatically propagate throughout the array as we proceed.\n\n__Code__ : \n```\nclass Solution {\n public int minOperations(int[] nums) {\n int count = 0;\n int n = nums.length;\n for(int i=1;i<n;i++){\n if(nums[i]<=nums[i-1]){\n count += (nums[i-1]-nums[i]+1);\n nums[i] += (nums[i-1]-nums[i]+1);\n }\n }\n return count;\n }\n}\n```\n__If you like this solution, please upvote.\nPlease comment for clarifications/doubts.__ | 2 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | WEEB DOES PYTHON | weeb-does-python-by-skywalker5423-12e9 | \n\tclass Solution:\n\t\tdef minOperations(self, nums: List[int]) -> int:\n\t\t\tcount = 0\n\n\t\t\tfor i in range(1,len(nums)):\n\t\t\t\tif nums[i] <= nums[i-1 | Skywalker5423 | NORMAL | 2021-12-03T07:24:57.471241+00:00 | 2021-12-03T07:24:57.471285+00:00 | 164 | false | \n\tclass Solution:\n\t\tdef minOperations(self, nums: List[int]) -> int:\n\t\t\tcount = 0\n\n\t\t\tfor i in range(1,len(nums)):\n\t\t\t\tif nums[i] <= nums[i-1]:\n\t\t\t\t\tinitial = nums[i] \n\t\t\t\t\tnums[i] = nums[i-1] + 1\n\t\t\t\t\tcount += nums[i] - initial\n\n\t\t\treturn count\n\nAlright leetcoders, take a break and watch some anime\nCheck out **\u308C\u3067\u3043\xD7\u3070\u3068! (Ladies Versus Butlers)**\n\n# Episodes: 12 (1 Season) + 6 Specials\n# Genre: Comedy, Ecchi, Harem, Romance, School, Seinen\n\nGood anime, enjoy it\n | 2 | 0 | ['Python', 'Python3'] | 1 |
minimum-operations-to-make-the-array-increasing | C++ solution without updating the vector nums | c-solution-without-updating-the-vector-n-6ac7 | \nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n if(nums.size()==0)return 0;\n int op=0,count=0;\n for(int | codedguy | NORMAL | 2021-09-26T10:43:25.177949+00:00 | 2021-09-26T10:43:25.177980+00:00 | 37 | false | ```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n if(nums.size()==0)return 0;\n int op=0,count=0;\n for(int i=1;i<nums.size();i++){\n if(nums[i]<=nums[i-1]+count){\n count+=nums[i-1]-nums[i]+1; \n op+=count;\n }\n else count =0;\n } \n return op;\n \n }\n};\n``` | 2 | 0 | [] | 0 |
minimum-operations-to-make-the-array-increasing | easy python solution | easy-python-solution-by-bhupatjangid-vrae | \nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n ans=0\n for i in range(1,len(nums)):\n ans+=(max(0,nums[i-1]+ | bhupatjangid | NORMAL | 2021-08-16T15:16:31.222038+00:00 | 2021-08-16T15:16:31.222086+00:00 | 81 | false | ```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n ans=0\n for i in range(1,len(nums)):\n ans+=(max(0,nums[i-1]+1-nums[i]))\n nums[i]=max(nums[i],nums[i-1]+1)\n return ans\n``` | 2 | 0 | [] | 0 |
minimum-operations-to-make-the-array-increasing | [JAVA] s'mple short O(n) solution | java-smple-short-on-solution-by-zeldox-r1mh | if you like it pls upvote\n\nJAVA\n\nclass Solution {\n public int minOperations(int[] nums) {\n int res = 0;\n for(int i = 1;i<nums.length;i++ | zeldox | NORMAL | 2021-08-14T21:48:17.594328+00:00 | 2021-08-14T21:48:17.594356+00:00 | 110 | false | if you like it pls upvote\n\nJAVA\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int res = 0;\n for(int i = 1;i<nums.length;i++){\n if(nums[i]<=nums[i-1]){\n res+= nums[i-1]-nums[i]+1;\n nums[i] = nums[i-1]+1;\n }\n }\n return res;\n }\n}\n``` | 2 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | JavaScript reduce solution | javascript-reduce-solution-by-kkchengaf-bq56 | every time increase by (max - cur)\n\nvar minOperations = function(nums) {\n var max = 0;\n return nums.reduce((acc, cur) => { \n max = Math.max(cu | kkchengaf | NORMAL | 2021-06-09T03:52:51.979139+00:00 | 2021-06-09T03:52:51.979179+00:00 | 180 | false | every time increase by (max - cur)\n```\nvar minOperations = function(nums) {\n var max = 0;\n return nums.reduce((acc, cur) => { \n max = Math.max(cur, ++max);\n return acc + max - cur;\n }, 0)\n};\n``` | 2 | 0 | ['JavaScript'] | 2 |
minimum-operations-to-make-the-array-increasing | python | beats 99.8% | easy | python-beats-998-easy-by-abhishen99-c44v | \nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n \n \n pre,ans=0,0\n \n for i in nums:\n | Abhishen99 | NORMAL | 2021-05-21T18:12:15.271143+00:00 | 2021-05-21T18:12:46.564590+00:00 | 252 | false | ```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n \n \n pre,ans=0,0\n \n for i in nums:\n if pre < i:\n pre=i\n else:\n pre+=1\n ans+=pre-i\n return ans\n``` | 2 | 0 | ['Python', 'Python3'] | 2 |
minimum-operations-to-make-the-array-increasing | Python easy solution | python-easy-solution-by-iamkshitij77-u7dh | ```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n ans = 0\n for i in range(1,len(nums)):\n if nums[i-1]>=num | iamkshitij77 | NORMAL | 2021-05-06T20:57:10.484988+00:00 | 2021-05-06T20:57:10.485017+00:00 | 71 | false | ```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n ans = 0\n for i in range(1,len(nums)):\n if nums[i-1]>=nums[i]:\n ans+=nums[i-1]-nums[i]+1\n nums[i] = nums[i-1] + 1\n return ans\n | 2 | 0 | [] | 0 |
minimum-operations-to-make-the-array-increasing | Simple Javascript solution beats 95% | simple-javascript-solution-beats-95-by-s-c1it | \n/**\n * @param {number[]} nums\n * @return {number}\n */\nvar minOperations = function(nums) {\n let ops = 0, prevNum = nums[0]\n for(let i = 1; i < num | saynn | NORMAL | 2021-04-27T07:17:33.799652+00:00 | 2021-04-27T07:17:33.799702+00:00 | 318 | false | ```\n/**\n * @param {number[]} nums\n * @return {number}\n */\nvar minOperations = function(nums) {\n let ops = 0, prevNum = nums[0]\n for(let i = 1; i < nums.length; i++){\n if(nums[i] <= prevNum){\n ops += prevNum + 1 - nums[i]\n nums[i] = prevNum + 1\n }\n prevNum = nums[i]\n }\n return ops\n};\n``` | 2 | 0 | ['JavaScript'] | 0 |
minimum-operations-to-make-the-array-increasing | Go golang solution | go-golang-solution-by-leaf_peng-kpl6 | Runtime: 16 ms, faster than 47.56% of Go online submissions for Minimum Operations to Make the Array Increasing.\nMemory Usage: 6.2 MB, less than 6.10% of Go on | leaf_peng | NORMAL | 2021-04-25T03:19:47.199021+00:00 | 2021-04-25T03:19:47.199057+00:00 | 56 | false | >Runtime: 16 ms, faster than 47.56% of Go online submissions for Minimum Operations to Make the Array Increasing.\nMemory Usage: 6.2 MB, less than 6.10% of Go online submissions for Minimum Operations to Make the Array Increasing.\n\n```go\nfunc minOperations(nums []int) int {\n ans := 0\n for i := 1; i < len(nums); i++ {\n if nums[i - 1] >= nums[i] {\n ans += nums[i - 1] - nums[i] + 1\n nums[i] += nums[i - 1] - nums[i] + 1\n }\n }\n return ans\n}\n``` | 2 | 0 | [] | 0 |
minimum-operations-to-make-the-array-increasing | A greedy solution for a greedy problem (C++) | a-greedy-solution-for-a-greedy-problem-c-l2lz | The resultant array after performing all of the increment operations has to be strictly increasing (e.g., [1,2,3,4,5]).For a strictly increasing array each elem | vatsss | NORMAL | 2021-04-20T17:38:35.869198+00:00 | 2021-04-20T17:38:35.869246+00:00 | 277 | false | The resultant array after performing all of the increment operations has to be strictly increasing (e.g., [1,2,3,4,5]).For a strictly increasing array each element has to be greater than its previous element by atleast 1.So, we can take a greedy approach such that whenever we find an element that is not greater than the previous ones we increment it by the dfference+1 and move on with our approach.(In short,the current element has to be the greatest one **yet**)\n```\nint cnt=0;\n if(arr.size()==1)\n return cnt;\n int mx=-1;\n for(int i=0;i<arr.size();i++){\n\t\t//if the present element is already greater than the maximum element then we don\'t have to increment anything. \n if(arr[i]>mx){\n mx=arr[i]; //updating the maximum element yet.\n continue;\n }\n int temp=mx-arr[i]+1; //minimum increment that we can do will be equal to : (maximum element - present element+1)\n cnt+=mx-arr[i]+1; //updating the count factor.\n mx=temp+arr[i]; //Since we updated the present element we have to update the max. element as well (strictly increasing.)\n }\n return cnt;\n``` | 2 | 0 | ['Greedy', 'C'] | 0 |
minimum-operations-to-make-the-array-increasing | Simple C++ and Java Solution Time O(N) and Space O(1) | simple-c-and-java-solution-time-on-and-s-otuk | C++ solution: \n\n\n\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n if(nums.size()<1)return 0;\n int cost=0;\n fo | millenniumdart09 | NORMAL | 2021-04-18T10:39:22.676687+00:00 | 2021-05-25T19:13:30.571739+00:00 | 186 | false | **C++ solution:** \n```\n\n\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n if(nums.size()<1)return 0;\n int cost=0;\n for(int i=1;i<nums.size();i++)\n {\n if(nums[i]<=nums[i-1])\n {\n cost+=nums[i-1]-nums[i]+1;\n nums[i]+=nums[i-1]-nums[i]+1;\n }\n \n }\n return cost;\n }\n};\n```\n\n**Java Solution:**\n\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int cost=0;\n for(int i=1;i<nums.length;i++)\n {\n if(nums[i-1]>=nums[i])\n {\n int diff=nums[i-1]-nums[i];\n cost=cost+diff+1;\n nums[i]=nums[i]+diff+1;\n }\n }\n return cost;\n \n }\n};\n\n```\n\n\n | 2 | 0 | ['C', 'Java'] | 0 |
minimum-operations-to-make-the-array-increasing | Rust Simple Solution | rust-simple-solution-by-kohbis-fm5x | rust\nimpl Solution {\n pub fn min_operations(nums: Vec<i32>) -> i32 {\n let mut count: i32 = 0;\n let mut prev: i32 = 0;\n for num in n | kohbis | NORMAL | 2021-04-18T01:47:40.611447+00:00 | 2021-04-18T01:47:40.611478+00:00 | 77 | false | ```rust\nimpl Solution {\n pub fn min_operations(nums: Vec<i32>) -> i32 {\n let mut count: i32 = 0;\n let mut prev: i32 = 0;\n for num in nums {\n if prev >= num {\n count += (prev + 1) - num;\n prev += 1\n } else {\n prev = num\n }\n }\n count\n }\n}\n``` | 2 | 0 | ['Rust'] | 0 |
minimum-operations-to-make-the-array-increasing | python easy solution | python-easy-solution-by-akaghosting-ckdz | \tclass Solution:\n\t\tdef minOperations(self, nums: List[int]) -> int:\n\t\t\tres = 0\n\t\t\tfor i in range(1, len(nums)):\n\t\t\t\tif nums[i] <= nums[i - 1]:\ | akaghosting | NORMAL | 2021-04-17T16:16:29.266753+00:00 | 2021-04-17T16:16:29.266789+00:00 | 128 | false | \tclass Solution:\n\t\tdef minOperations(self, nums: List[int]) -> int:\n\t\t\tres = 0\n\t\t\tfor i in range(1, len(nums)):\n\t\t\t\tif nums[i] <= nums[i - 1]:\n\t\t\t\t\tres += nums[i - 1] - nums[i] + 1\n\t\t\t\t\tnums[i] = nums[i - 1] + 1\n\t\t\treturn res | 2 | 0 | [] | 0 |
minimum-operations-to-make-the-array-increasing | [Python3] sweep | python3-sweep-by-ye15-jtyk | \nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n ans = 0\n for i in range(1, len(nums)):\n if nums[i-1] >= num | ye15 | NORMAL | 2021-04-17T16:09:35.818346+00:00 | 2021-04-17T16:09:35.818385+00:00 | 136 | false | ```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n ans = 0\n for i in range(1, len(nums)):\n if nums[i-1] >= nums[i]: \n ans += 1 + nums[i-1] - nums[i]\n nums[i] = 1 + nums[i-1]\n return ans \n``` | 2 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-the-array-increasing | [C++] One pass solution (100% time & 100% space) | c-one-pass-solution-100-time-100-space-b-vxfl | \nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int result = 0;\n \n for (int i = 1; i < nums.size(); ++i) {\n | bhaviksheth | NORMAL | 2021-04-17T16:03:49.791097+00:00 | 2021-04-17T16:03:49.791130+00:00 | 235 | false | ```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int result = 0;\n \n for (int i = 1; i < nums.size(); ++i) {\n if (nums[i] <= nums[i - 1]) {\n result += nums[i - 1] + 1 - nums[i];\n nums[i] = nums[i - 1] + 1;\n }\n }\n \n return result;\n }\n``` | 2 | 0 | [] | 0 |
minimum-operations-to-make-the-array-increasing | Easy and just count it | easy-and-just-count-it-by-sairangineeni-a1ye | ApproachCreate a count variable to track the number of operations.Loop through the array from index 0 to n - 2 (we compare each element with the next).For each | Sairangineeni | NORMAL | 2025-03-31T06:05:56.507022+00:00 | 2025-03-31T06:05:56.507022+00:00 | 127 | false | # Approach
Create a count variable to track the number of operations.
Loop through the array from index 0 to n - 2 (we compare each element with the next).
For each pair nums[i] and nums[i+1]:
If nums[i] >= nums[i+1], it means we need to increase nums[i+1].
Calculate how much to increase it by: diff = nums[i] - nums[i+1] + 1.
We add +1 to make it strictly greater.
Add diff to count.
Update nums[i+1] by adding diff to it.
After the loop ends, return count.
# Code
```java []
class Solution {
public static int minOperations(int[] nums) {
int count = 0;
for (int i = 0; i < nums.length-1; i++) {
if(nums[i] >= nums[i+1]) {
int diff = nums[i] - nums[i + 1] + 1;
count += diff;
nums[i+1] += diff;
}
}
return count;
}
}
``` | 1 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | Greedy Incremental Adjustment to Enforce Strict Monotonicity | greedy-incremental-adjustment-to-enforce-bezz | IntuitionThe goal is to transform the input array into a strictly increasing sequence using the minimum number of increment operations. To achieve this, we scan | expert07 | NORMAL | 2025-03-28T12:29:50.002714+00:00 | 2025-03-28T12:29:50.002714+00:00 | 127 | false | # Intuition
The goal is to transform the input array into a strictly increasing sequence using the minimum number of increment operations. To achieve this, we scan the array from left to right. If the current element is not strictly less than the next, we increment the next element until it becomes greater. This greedy approach ensures we only perform the necessary minimum number of operations at each step.
# Approach
- Initialize a counter to keep track of operations.
- Iterate through the array from the first to the second-to-last element.
- If the next element is less than or equal to the current, increment it until it becomes strictly greater.
- Add the number of increments performed to the counter.
- Return the final count.
# Complexity
- Time complexity:
O(n) – Each element is visited once, and though inner increments happen, each increment operation corresponds to an increase in array value, bounded by the overall value range.
- Space complexity:
O(1) – No extra space is used besides a few variables; the input vector is modified in place (though Leetcode typically doesn't penalize for this).
# Code
```cpp []
class Solution {
public:
int minOperations(vector<int>& nums) {
int counter = 0;
for(int i = 0; i < nums.size() -1; i++)
{
while(nums[i] >= nums[i+1])
{
nums[i+1]++;
counter++;
}
}
return counter;
}
};
``` | 1 | 0 | ['Array', 'Greedy', 'Simulation', 'C++'] | 0 |
minimum-operations-to-make-the-array-increasing | T.C-> O(n) and S.C-> O(1) | cpp 🤩 | tc-on-and-sc-o1-cpp-by-varuntyagig-gjz1 | Complexity
Time complexity:
O(n)
Space complexity:
O(1)
Code | varuntyagig | NORMAL | 2025-03-27T21:33:40.093547+00:00 | 2025-03-27T21:33:40.093547+00:00 | 61 | false | # Complexity
- Time complexity:
$$O(n)$$
- Space complexity:
$$O(1)$$
# Code
```cpp []
class Solution {
public:
int minOperations(vector<int>& nums) {
int steps = 0;
for (int i = 0; i < nums.size() - 1; ++i) {
if (nums[i] > nums[i + 1]) {
steps += ((nums[i] - nums[i + 1]) + 1);
nums[i + 1] += ((nums[i] - nums[i + 1]) + 1);
} else if (nums[i] == nums[i + 1]) {
steps += 1;
nums[i + 1] += 1;
}
}
return steps;
}
};
``` | 1 | 0 | ['Array', 'C++'] | 0 |
minimum-operations-to-make-the-array-increasing | <<EASY THAN YOUR EXPECTATIONS>> | easy-than-your-expectations-by-dakshesh_-wob3 | PLEASE UPVOTE MECode | Dakshesh_vyas123 | NORMAL | 2025-03-20T10:41:09.631710+00:00 | 2025-03-20T10:41:09.631710+00:00 | 98 | false | # PLEASE UPVOTE ME
# Code
```cpp []
class Solution {
public:
int minOperations(vector<int>& nums) {
int a=0;
for(int i=0;i<nums.size()-1;i++){
if(nums[i+1]<=nums[i]) {
a+=(nums[i]-nums[i+1])+1;
nums[i+1]=nums[i]+1;}
}
return a;
}
};
``` | 1 | 0 | ['C++'] | 0 |
minimum-operations-to-make-the-array-increasing | C++ Solution ||n100% Beats | c-solution-n100-beats-by-jeetgajera-upuq | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Jeetgajera | NORMAL | 2024-09-12T03:44:20.384949+00:00 | 2024-09-12T03:44:20.384981+00:00 | 18 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity : O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity : O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int ans=0;\n \n for(int i=1;i<nums.size();i++){\n if(nums[i]<=nums[i-1]){\n ans+=nums[i-1]+1-nums[i];\n nums[i]=nums[i-1]+1;\n } \n }\n return ans;\n }\n};\n``` | 1 | 0 | ['Greedy', 'C++'] | 0 |
minimum-operations-to-make-the-array-increasing | Easy 100% | easy-100-by-oybek_0005-cvtg | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | oybek_0005 | NORMAL | 2024-08-12T14:43:20.171526+00:00 | 2024-08-12T14:43:20.171571+00:00 | 255 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int count =0;\n for(int i=1; i<nums.length; i++){\n if(nums[i] <= nums[i-1]){\n int dif = nums[i-1] - nums[i]+1;\n nums[i]+=dif;\n count+=dif;\n }\n \n }\n return count;\n }\n}\n``` | 1 | 0 | ['Java'] | 1 |
minimum-operations-to-make-the-array-increasing | simple C++ sol | simple-c-sol-by-vivek_0104-4hf2 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Vivek_0104 | NORMAL | 2024-03-02T07:28:27.325401+00:00 | 2024-03-02T07:28:27.325423+00:00 | 6 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(N)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n\n# Code\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int ans=0,need=1;\n for(auto x:nums){\n ans+=max(need-x,0);\n need=max(need,x)+1;\n }\n return ans;\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
minimum-operations-to-make-the-array-increasing | Simple java code 2 ms beats 99 % | simple-java-code-2-ms-beats-99-by-arobh-zxya | \n# Complexity\n- \n\n# Code\n\nclass Solution {\n public int minOperations(int[] nums) {\n int max = nums[0];\n int sum = 0;\n for(int | Arobh | NORMAL | 2024-01-14T03:41:49.603307+00:00 | 2024-01-14T03:41:49.603331+00:00 | 18 | false | \n# Complexity\n- \n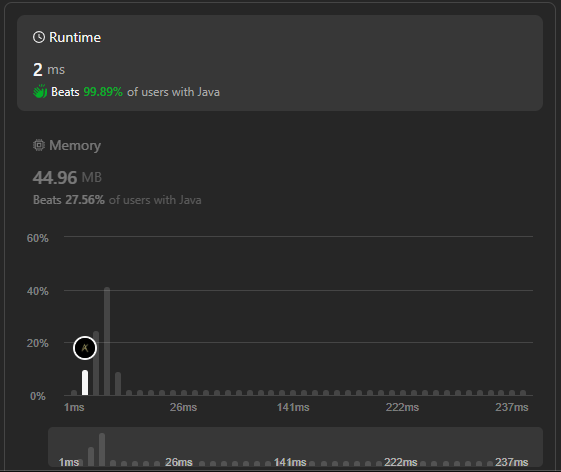\n# Code\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int max = nums[0];\n int sum = 0;\n for(int i=1; i<nums.length; i++) {\n if(nums[i]>max) {\n max = nums[i];\n } else {\n max++;\n sum += max - nums[i];\n }\n }\n return sum;\n }\n}\n``` | 1 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | Easy C++ solution || Greedy approach | easy-c-solution-greedy-approach-by-bhara-h30f | \n\n# Code\n\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int n = nums.size(), count = 0;\n if(n == 1){\n r | bharathgowda29 | NORMAL | 2024-01-07T14:08:51.579170+00:00 | 2024-01-07T14:08:51.579195+00:00 | 450 | false | \n\n# Code\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int n = nums.size(), count = 0;\n if(n == 1){\n return 0;\n }\n for(int i=1; i<n; i++){\n if(nums[i] > nums[i-1]){\n continue;\n }\n else{\n int temp = nums[i-1] - nums[i] + 1;\n nums[i] += temp;\n count += temp;\n }\n }\n\n return count;\n }\n};\n``` | 1 | 0 | ['Array', 'Greedy', 'C++'] | 0 |
minimum-operations-to-make-the-array-increasing | simplest java sol | simplest-java-sol-by-anoopchaudhary1-ncpl | \n\n# Code\n\nclass Solution {\n public int minOperations(int[] nums) {\n int[] arr = new int[nums.length];\n int count =0;\n for(int i | Anoopchaudhary1 | NORMAL | 2023-12-14T08:31:52.227610+00:00 | 2023-12-14T08:31:52.227644+00:00 | 140 | false | \n\n# Code\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int[] arr = new int[nums.length];\n int count =0;\n for(int i = 0 ; i<nums.length ; i++){\n arr[i] = nums[i];\n }\n for(int i =0 ; i<arr.length-1 ; i++){\n if(arr[i+1] <=arr[i]){\n arr[i+1] = arr[i]+1;\n }\n }\n for(int i =0 ; i< arr.length ; i++){\n count = count + arr[i]-nums[i];\n }\n return count;\n }\n}\n``` | 1 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | using adjacent difference. | using-adjacent-difference-by-akhilaaa-mimj | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Akhilaaa | NORMAL | 2023-11-17T11:44:18.420638+00:00 | 2023-11-17T11:44:18.420667+00:00 | 86 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& v) \n {\n int n=v.size(); \n int number=0;\n for(int i=1;i<n;i++)\n {\n if(v[i] <= v[i-1]){\n int p=v[i-1]-v[i];\n number=number+p+1;\n v[i]=v[i]+p+1;\n }\n\n }\n return number;\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
Subsets and Splits