question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
โ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
minimum-operations-to-make-the-array-increasing | Type Script Solution | type-script-solution-by-diyoufkv7-e11x | Intuition\nMathematic Expression Approch\n\n# Approach\n- Finding the Difference between the Elements that is smaller than the\nnext element\n\n# Complexity\n- | diyoufkv7 | NORMAL | 2023-11-03T04:14:52.942209+00:00 | 2023-11-03T04:14:52.942240+00:00 | 26 | false | # Intuition\nMathematic Expression Approch\n\n# Approach\n- Finding the Difference between the Elements that is smaller than the\nnext element\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfunction minOperations(nums: number[]): number {\n let sum = 0;\n let result = 0;\n for (let i = 1; i < nums.length; i++) {\n if (nums[i] <= nums[i - 1]) {\n sum = (nums[i - 1] + 1) - nums[i]\n nums[i] += sum\n result += sum\n }\n }\n\n return result\n};\n``` | 1 | 0 | ['TypeScript'] | 0 |
minimum-operations-to-make-the-array-increasing | Do this sample code. | do-this-sample-code-by-0varun-5xx4 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | 0varun | NORMAL | 2023-11-01T04:42:35.795915+00:00 | 2023-11-01T04:42:35.795945+00:00 | 152 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n/**\n * @param {number[]} nums\n * @return {number}\n */\nvar minOperations = function (nums) {\n var count = 0;\n for (var i = 1; i < nums.length; i++) {\n if (nums[i] <= nums[i - 1]) {\n count += nums[i - 1] - nums[i] + 1;\n nums[i] = nums[i - 1] + 1;\n }\n }\n return count;\n}\n\n\n``` | 1 | 0 | ['JavaScript'] | 2 |
minimum-operations-to-make-the-array-increasing | Simple Solution | simple-solution-by-adwxith-9yfl | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | adwxith | NORMAL | 2023-11-01T04:40:55.098722+00:00 | 2023-11-01T04:40:55.098761+00:00 | 257 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n/**\n * @param {number[]} nums\n * @return {number}\n */\nvar minOperations = function(nums) {\n let ops=0\n for(let i=1;i<nums.length;i++){\n if(nums[i]<=nums[i-1]){\n ops+=(nums[i-1]-nums[i])+1\n nums[i]=nums[i-1]+1\n }\n }\n return ops\n};\n``` | 1 | 0 | ['Array', 'JavaScript'] | 0 |
minimum-operations-to-make-the-array-increasing | Best Java Solution || Beats 100% | best-java-solution-beats-100-by-ravikuma-z18i | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | ravikumar50 | NORMAL | 2023-10-09T17:09:06.853331+00:00 | 2023-10-09T17:09:06.853349+00:00 | 987 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int minOperations(int[] arr) {\n int n = arr.length;\n \n int ans = 0;\n\n for(int i=1; i<n; i++){\n if(arr[i]<=arr[i-1]){\n ans = ans + arr[i-1]-arr[i]+1;\n arr[i] = arr[i-1]+1;\n }\n }\n return ans;\n }\n}\n``` | 1 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | Solution which beats 96% in C# | solution-which-beats-96-in-c-by-akhundov-l683 | \npublic class Solution {\n public int MinOperations(int[] nums) {\n int currElement = nums[0], count = 0;\n for (int i = 1; i < nums.Len | akhundovaykhan | NORMAL | 2023-09-08T13:24:47.441777+00:00 | 2023-09-08T13:24:47.441842+00:00 | 164 | false | ```\npublic class Solution {\n public int MinOperations(int[] nums) {\n int currElement = nums[0], count = 0;\n for (int i = 1; i < nums.Length; i++)\n {\n if (currElement < nums[i])\n {\n currElement = nums[i];\n }\n else\n {\n count += currElement - nums[i] + 1;\n currElement++;\n }\n }\n return count;\n }\n}\n``` | 1 | 0 | ['C#'] | 0 |
minimum-operations-to-make-the-array-increasing | Minimum Operations to Make the Array Increasing ๐งโ๐ป๐งโ๐ป || JAVA solution code ๐๐... | minimum-operations-to-make-the-array-inc-kzfq | Code\n\nclass Solution {\n public int minOperations(int[] nums) {\n int count = 0;\n\n for(int i=1;i<nums.length;i++){\n\n if(nums[i | Jayakumar__S | NORMAL | 2023-08-04T16:45:22.346913+00:00 | 2023-08-04T16:45:22.346942+00:00 | 526 | false | # Code\n```\nclass Solution {\n public int minOperations(int[] nums) {\n int count = 0;\n\n for(int i=1;i<nums.length;i++){\n\n if(nums[i]<=nums[i-1]){\n count += Math.abs(nums[i]-nums[i-1])+1;\n nums[i] = nums[i-1]+1;\n\n }\n }\n return count;\n }\n}\n``` | 1 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | Minimum Operations to Make the Array Increasing Solution in C++ | minimum-operations-to-make-the-array-inc-49sa | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | The_Kunal_Singh | NORMAL | 2023-03-04T05:55:20.014738+00:00 | 2023-03-04T05:55:20.014777+00:00 | 35 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n# Code\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int i, count=0;\n if(nums.size()==1)\n {\n return count;\n }\n for(i=1 ; i<nums.size() ; i++)\n {\n if(nums[i]<=nums[i-1])\n {\n count += nums[i-1] - nums[i] + 1;\n nums[i] += nums[i-1] - nums[i] + 1;\n }\n }\n return count;\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
minimum-operations-to-make-the-array-increasing | Easy c++ Solution for Beginners | easy-c-solution-for-beginners-by-sunnyya-mzi0 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | sunnyyad2002 | NORMAL | 2023-02-20T06:05:52.074071+00:00 | 2023-02-20T06:05:52.074105+00:00 | 106 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int count=0;\n for(int i=0;i<nums.size()-1;i++){\n if(nums[i] < nums[i+1])\n continue;\n else{\n count=count+(nums[i]+1-nums[i+1]);\n nums[i+1]=nums[i]+1;\n }\n\n }\n return count;\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
minimum-operations-to-make-the-array-increasing | c++ || using loop || easy approach | c-using-loop-easy-approach-by-jauliadhik-j6yf | \n# Complexity\n- Time complexity:\nO(N)\n\n- Space complexity:\nO(1)\n\n# Code\n\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) \n {\n | jauliadhikari2012 | NORMAL | 2023-01-17T03:42:46.491597+00:00 | 2023-01-17T03:42:46.491644+00:00 | 796 | false | \n# Complexity\n- Time complexity:\nO(N)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) \n {\n int count =0;\n for(int i=0;i<nums.size()-1;i++)\n {\n if(nums[i] < nums[i+1])\n {\n continue;\n }\n else\n {\n \n count = count +(nums[i] + 1-nums[i+1]);\n nums[i+1]=nums[i]+1;\n\n }\n }return count;\n }\n};\n``` | 1 | 0 | ['C++'] | 1 |
minimum-operations-to-make-the-array-increasing | C++ brute force | c-brute-force-by-mishalalshahari-dybu | \nint minOperations(vector<int>& nums) {\n int count=0;\n for(int i=0;i<nums.size()-1;i++){\n if(nums[i]<nums[i+1]){\n c | mishalalshahari | NORMAL | 2022-10-31T17:45:54.813800+00:00 | 2022-10-31T17:45:54.813858+00:00 | 16 | false | ```\nint minOperations(vector<int>& nums) {\n int count=0;\n for(int i=0;i<nums.size()-1;i++){\n if(nums[i]<nums[i+1]){\n continue;\n }\n else{\n count+=nums[i]+1-nums[i+1];\n nums[i+1]=nums[i]+1;\n }\n }\n return count;\n }\n``` | 1 | 0 | ['C++'] | 0 |
minimum-operations-to-make-the-array-increasing | 80% SPACE AND TIME BEATS || C++ || EASY || SIMPLE | 80-space-and-time-beats-c-easy-simple-by-b74t | \nclass Solution {\npublic:\n int minOperations(vector<int>& nums) \n {\n int moves=0;\n //first we will sort the array \n //reazon-->we | akshat0610 | NORMAL | 2022-09-26T14:02:25.087213+00:00 | 2022-09-26T14:02:25.087253+00:00 | 535 | false | ```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) \n {\n int moves=0;\n //first we will sort the array \n //reazon-->we will convert the array into increasign array\n //how->we will increment the curr element and make it bigger than its previous\n\n //fist 0th index element we will not consider --> nums[i-1] and nums[i] relation \n\n //iterate to all the elements from the index1\n for(int i=1;i<nums.size();i++)\n {\n if(nums[i-1]==nums[i]) //duplicate need to increment the nums[i]\n {\n moves++;\n nums[i]=nums[i-1]+1;\n }\n else if(nums[i-1]>nums[i]) //nums[i-1] is greater even after sorting beacause we incremented nums[i-1] to make it uniuq from nums[i-2]\n {\n moves=moves+abs(nums[i]-nums[i-1])+1;\n nums[i]=nums[i-1]+1;\n }\n }\n return moves; \n }\n};\n``` | 1 | 0 | ['C', 'Sorting', 'C++'] | 0 |
minimum-operations-to-make-the-array-increasing | 80% SPACE AND TIME BEATS || C++ || EASY || SIMPLE | 80-space-and-time-beats-c-easy-simple-by-3yiv | \nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int ans = 0;\n for(int i = 1; i < nums.size(); i++){\n if(num | abhay_12345 | NORMAL | 2022-09-23T19:29:30.099954+00:00 | 2022-09-23T19:29:30.099994+00:00 | 329 | false | ```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int ans = 0;\n for(int i = 1; i < nums.size(); i++){\n if(nums[i]==nums[i-1]){\n ans++;\n nums[i]++;\n }\n else if(nums[i]-nums[i-1]<0){\n ans += -nums[i]+nums[i-1]+1;\n nums[i] = nums[i-1]+1;\n }\n }\n // for(auto &i: nums)cout<<i<<" ";\n return ans;\n }\n};\n``` | 1 | 0 | ['Greedy', 'C', 'C++'] | 0 |
minimum-operations-to-make-the-array-increasing | Java Solution || 100% Optimized || 100% faster || O(1) Space || O(N) time | java-solution-100-optimized-100-faster-o-dh1t | class Solution {\n public int minOperations(int[] nums) {\n \n int count =0; // Operations Count\n int nextVal = nums[0]+1; // We ch | avadarshverma737 | NORMAL | 2022-09-13T14:01:39.722026+00:00 | 2022-09-13T14:02:14.804148+00:00 | 289 | false | class Solution {\n public int minOperations(int[] nums) {\n \n int count =0; // Operations Count\n int nextVal = nums[0]+1; // We choose the next value to be our successive element\n for(int i=1;i<nums.length;i++){\n \n if(nums[i]<=nums[i-1]){\n count += nextVal-nums[i]; // No. of operations to change the present value to the Next Succesor\n nums[i] = nextVal; // change array value\n }\n else{\n nextVal = Math.max(nextVal,nums[i]); // Change the successor to the max value that can be put\n }\n nextVal++; // for the array to be strictly increasing, always inc the nextVal ;\n }\n return count;\n }\n} | 1 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | C++ EASY SOLUTION | c-easy-solution-by-abhay18e-ffc2 | Would love your feedback\n\n int minOperations(vector<int>& nums) {\n int operationCount = 0;\n \n for(int i=1; i<nums.size(); i++){\n | abhay18e | NORMAL | 2022-08-14T12:01:23.125001+00:00 | 2022-08-14T12:01:23.125045+00:00 | 169 | false | *Would love your feedback*\n```\n int minOperations(vector<int>& nums) {\n int operationCount = 0;\n \n for(int i=1; i<nums.size(); i++){\n int diff = nums[i]-nums[i-1];\n \n if(diff<=0){\n nums[i] += abs(diff) +1;\n operationCount += abs(diff)+1;\n }\n }\n return operationCount;\n }``` | 1 | 0 | [] | 1 |
minimum-operations-to-make-the-array-increasing | Java Easiest Solution | java-easiest-solution-by-aditya001-l8y4 | ```\nclass Solution {\n public int minOperations(int[] nums) {\n int ans = 0;\n for(int i=0;i<nums.length-1;i++){\n if(nums[i+1]<=nu | Aditya001 | NORMAL | 2022-08-05T04:52:33.212971+00:00 | 2022-08-05T04:52:33.213020+00:00 | 306 | false | ```\nclass Solution {\n public int minOperations(int[] nums) {\n int ans = 0;\n for(int i=0;i<nums.length-1;i++){\n if(nums[i+1]<=nums[i]){\n ans += nums[i]-nums[i+1]+1;\n nums[i+1] = nums[i]+1;\n }\n }\n return ans;\n }\n} | 1 | 0 | ['Java'] | 0 |
minimum-operations-to-make-the-array-increasing | C++ | brute force solution | c-brute-force-solution-by-shobhit2205-dfg8 | \nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int count = 0;\n for(int i = 1; i < nums.size(); i++){\n if(n | Shobhit2205 | NORMAL | 2022-08-03T15:15:10.878738+00:00 | 2022-08-03T15:15:10.878806+00:00 | 186 | false | ```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int count = 0;\n for(int i = 1; i < nums.size(); i++){\n if(nums[i] <= nums[i - 1]) {\n count += (nums[i-1] - nums[i] + 1);\n nums[i] = nums[i-1] + 1;\n }\n }\n return count;\n }\n};\n``` | 1 | 0 | ['C'] | 0 |
minimum-operations-to-make-the-array-increasing | Java Easy Solution 2ms | java-easy-solution-2ms-by-ramsingh27-waia | class Solution {\n public int minOperations(int[] nums) {\n int n=nums.length;\n int count=0;\n for(int i=0;i<n-1;i++)\n {\n | Ramsingh27 | NORMAL | 2022-07-30T17:13:08.449013+00:00 | 2022-07-30T17:13:08.449051+00:00 | 54 | false | class Solution {\n public int minOperations(int[] nums) {\n int n=nums.length;\n int count=0;\n for(int i=0;i<n-1;i++)\n {\n if(nums[i+1]<=nums[i])\n {\n int r=nums[i]-nums[i+1]+1;\n count+=r;\n nums[i+1]+=r;\n }\n }\n return count;\n }\n} | 1 | 0 | [] | 0 |
minimum-operations-to-make-the-array-increasing | Vasu vro | vasu-vro-by-revanth2311-7gq0 | \'\'\' \nclass Solution {\npublic:\n int minOperations(vector& nums) {\n int k=0,op=0;\n for(int i=1;i=nums[i]){\n k=nums[i-1]-n | revanth2311 | NORMAL | 2022-07-28T08:21:00.727200+00:00 | 2022-07-28T08:21:00.727242+00:00 | 29 | false | \'\'\' \nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int k=0,op=0;\n for(int i=1;i<nums.size();i++){\n k=0;\n if(nums[i-1]>=nums[i]){\n k=nums[i-1]-nums[i]+1;\n nums[i]=nums[i]+k;\n }\n op=op+k;\n }\n return op;\n }\n};\n\'\'\' | 1 | 0 | [] | 0 |
minimum-operations-to-make-the-array-increasing | Easy C++ solution | easy-c-solution-by-mubin86-fikc | \nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int result = 0;\n for(int i = 1; i<nums.size(); i++){\n if(nu | mubin86 | NORMAL | 2022-07-24T17:37:25.522201+00:00 | 2022-07-24T17:37:25.522253+00:00 | 51 | false | ```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int result = 0;\n for(int i = 1; i<nums.size(); i++){\n if(nums[i] <= nums[i-1]){ \n int newNum = nums[i-1] + 1;\n result += newNum - nums[i];\n nums[i] = newNum; \n }\n }\n return result;\n }\n};\n``` | 1 | 0 | [] | 0 |
minimum-operations-to-make-the-array-increasing | Easiest Approach C++ | easiest-approach-c-by-sagarkesharwnnni-21hd | \nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int count=0;\n int temp=0;\n \n for(int i=0;i<nums.size()- | sagarkesharwnnni | NORMAL | 2022-07-20T10:41:55.133058+00:00 | 2022-07-20T10:41:55.133099+00:00 | 65 | false | ```\nclass Solution {\npublic:\n int minOperations(vector<int>& nums) {\n int count=0;\n int temp=0;\n \n for(int i=0;i<nums.size()-1;i++){\n if(nums[i]>=nums[i+1]){\n temp=nums[i]-nums[i+1]+1;\n nums[i+1]+=temp;\n count+=temp; \n \n }\n \n }\n return count;\n }\n};\n``` | 1 | 0 | ['C'] | 0 |
minimum-operations-to-make-the-array-increasing | c++|O(n)|Simple Logic | consimple-logic-by-deepakhacker21-grza | class Solution {\npublic:\n\n int minOperations(vector& nums) {\n int count = 0;\n for(int i=1;i= nums[i]) {\n count = count + ( | deepakHacker21 | NORMAL | 2022-07-14T18:45:56.130502+00:00 | 2022-07-14T18:45:56.130543+00:00 | 39 | false | class Solution {\npublic:\n\n int minOperations(vector<int>& nums) {\n int count = 0;\n for(int i=1;i<nums.size();i++) {\n if(nums[i-1] >= nums[i]) {\n count = count + ((nums[i-1]+1)-nums[i]);\n nums[i] = nums[i-1] +1;\n }\n }\n return count;\n }\n}; | 1 | 0 | ['C'] | 0 |
minimum-operations-to-make-the-array-increasing | 90% fast || c++ || O(N) | 90-fast-c-on-by-sumit_singh0044-9l26 | \n int minOperations(vector<int>& nums) {\n if(nums.size()==1)\n return 0;\n int count=0;\n for(int i=1;i<nums.size();i++)\n | sumit_singh0044 | NORMAL | 2022-07-08T11:15:22.700652+00:00 | 2022-07-08T11:15:22.700675+00:00 | 57 | false | ```\n int minOperations(vector<int>& nums) {\n if(nums.size()==1)\n return 0;\n int count=0;\n for(int i=1;i<nums.size();i++)\n {\n if(nums[i]==nums[i-1])\n {\n nums[i]++;\n count++;\n }\n else if(nums[i]<nums[i-1])\n {\n int t=nums[i-1]-nums[i];\n t++;\n nums[i]=nums[i]+t;\n count+=t;\n } \n }\n return count;\n }\n``` | 1 | 0 | ['C', 'C++'] | 0 |
minimum-operations-to-make-the-array-increasing | โ
C++ || Short && clean || O(N) && O(1) | c-short-clean-on-o1-by-abhinav_0107-gdq7 | \n\n\n\n\tclass Solution {\n\t\tpublic:\n\t\t\tint minOperations(vector& nums) {\n\t\t\t\tint k=0;\n\t\t\t\tfor(int i=0;i<nums.size()-1;i++){\n\t\t\t\t\tif(nums | abhinav_0107 | NORMAL | 2022-06-28T08:43:24.402103+00:00 | 2022-06-28T08:43:24.402140+00:00 | 149 | false | 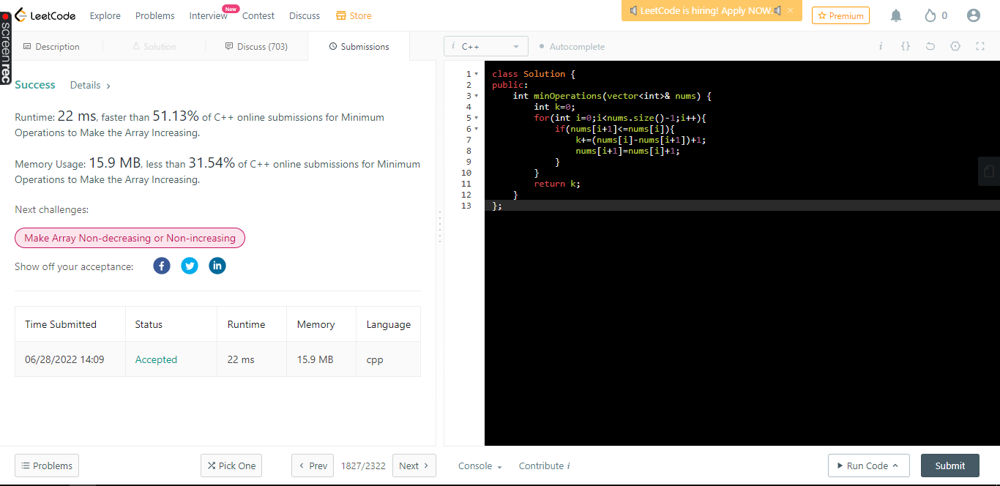\n\n\n\n\tclass Solution {\n\t\tpublic:\n\t\t\tint minOperations(vector<int>& nums) {\n\t\t\t\tint k=0;\n\t\t\t\tfor(int i=0;i<nums.size()-1;i++){\n\t\t\t\t\tif(nums[i+1]<=nums[i]){\n\t\t\t\t\t\tk+=(nums[i]-nums[i+1])+1;\n\t\t\t\t\t\tnums[i+1]=nums[i]+1;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t\treturn k;\n\t\t\t}\n\t\t}; | 1 | 0 | ['C', 'C++'] | 0 |
minimum-operations-to-make-the-array-increasing | Java solution. O(n) | java-solution-on-by-pvp-ltcb | \nclass Solution {\n public int minOperations(int[] nums) {\n int ans = 0;\n for (int i = 1; i < nums.length; i++) {\n if (nums[i] < | pvp | NORMAL | 2022-06-27T06:17:07.982618+00:00 | 2022-06-27T06:17:07.982657+00:00 | 40 | false | ```\nclass Solution {\n public int minOperations(int[] nums) {\n int ans = 0;\n for (int i = 1; i < nums.length; i++) {\n if (nums[i] <= nums[i - 1]) {\n ans += nums[i - 1] + 1 - nums[i];\n nums[i] = nums[i - 1] + 1;\n }\n }\n return ans;\n }\n}\n``` | 1 | 0 | [] | 0 |
minimum-operations-to-make-the-array-increasing | Python | Easy & Understanding Solution | python-easy-understanding-solution-by-ba-v6km | ```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n n=len(nums)\n if(n==1):\n return 0\n \n ans= | backpropagator | NORMAL | 2022-06-14T18:09:32.572031+00:00 | 2022-06-14T18:09:32.572077+00:00 | 371 | false | ```\nclass Solution:\n def minOperations(self, nums: List[int]) -> int:\n n=len(nums)\n if(n==1):\n return 0\n \n ans=0\n \n for i in range(1,n):\n if(nums[i]<=nums[i-1]):\n ans+=nums[i-1]-nums[i]+1\n nums[i]=nums[i-1]+1\n \n return ans | 1 | 0 | ['Python', 'Python3'] | 0 |
construct-string-with-repeat-limit | Easy understanding C++ code with comments | easy-understanding-c-code-with-comments-v9h2s | We want to form a lexicographically largest string using the characters in a given string.\nBut we have to make sure that a letter is not repeated more than the | venkatasaitanish | NORMAL | 2022-02-20T04:02:43.434365+00:00 | 2022-05-23T06:26:12.675157+00:00 | 7,523 | false | We want to form a lexicographically largest string using the characters in a given string.\nBut we have to make sure that a letter is not repeated more than the given limit in a row (i.e consecutively).\n\nSo we use a ***priority_queue*** to pop the element which has the highest priority. *If it\'s count is less than the repeatLimit,* we directly add to the ans.\n\n*But if it\'s count is greater than the repeatLimit*, we just add the repeatLimit amount of the present character and we pop out the next priority character and add a single letter to the ans.\nThis makes that a letter is not repeated more than limit in a row and lexicographically largest is maintained. After adding to the ans, if we have extra characters left out, we just push back into priority_queue for the next use.\n\nHere *if we are not able to pop*, the next priority character, we just return the ans so as we cannot add more than repeatedLimit charecters in a row.\n```\nclass Solution {\npublic:\n string repeatLimitedString(string s, int k) { // k is the repeatLimit\n int n = s.length();\n unordered_map<char,int> m;\n for(int i=0;i<n;i++) m[s[i]]++;\n priority_queue<pair<char,int>> pq;\n for(auto i: m){\n pq.push({i.first,i.second}); // pushing the characters with their frequencies.\n }\n \n string ans = "";\n while(!pq.empty()){\n char c1 = pq.top().first;\n int n1 = pq.top().second;\n pq.pop();\n \n int len = min(k,n1); // Adding characters upto minimum of repeatLimit and present character count.\n for(int i=0;i<len;i++){ // adding the highest priority element to the ans.\n ans += c1;\n }\n \n char c2;\n int n2=0;\n if(n1-len>0){ // If the cnt of present character is more than the limit.\n if(!pq.empty()){ //Getting the next priority character.\n c2 = pq.top().first;\n n2 = pq.top().second;\n pq.pop();\n }\n else{\n return ans; // if there is no another letter to add, we just return ans.\n }\n ans += c2; // Adding next priority character to ans.\n \n // If the elements are left out, pushing them back into priority queue for next use.\n pq.push({c1,n1-len});\n if(n2-1>0) pq.push({c2,n2-1}); \n }\n }\n return ans;\n }\n};\n```\n**Upvote if it helps!** | 115 | 8 | ['Greedy', 'C', 'Heap (Priority Queue)'] | 19 |
construct-string-with-repeat-limit | Java, Priority Queue and stack - Larger String with repeat limit | java-priority-queue-and-stack-larger-str-vh3v | At first on looking into this problem it looked little complex,\n\nBut after thinking for some time, I am able to unwire it little easily..\n\nHere is the appro | Surendaar | NORMAL | 2022-02-20T04:03:39.932617+00:00 | 2022-02-20T04:03:39.932659+00:00 | 5,579 | false | At first on looking into this problem it looked little complex,\n\nBut after thinking for some time, I am able to unwire it little easily..\n\nHere is the approach I took.\n\nIn-order to get a lexicographically larger number we know that it should have all larger alphabets in the starting of string like zzzzzzyyyyyyxxxxx.\n\nBut here they have given repeat count limit, so first things first we can sort the given string of characters using PriorityQueue.\n\n`1. Sort the given string in lexicographically large order using Max heap\n\nThen to maintain the repeat count and the lesser lexicographically small character once and then come back to larger charecter, I took the help of stack.\n\n 2. If the count of repeated character is more than the given repeat limit then add to stack\n 3. Then add the small character once and check whether the stack is empty\n 4. If its empty then you can continue in the same pattern\n 5. If stack is not empty then add the characters to its repeat count and again go for lesser character and repeat this process till the Priority Queue is empty.\n\nNow its time to look into the code :)\n\n\n```\n public String repeatLimitedString(String s, int repeatLimit) {\n PriorityQueue<Character> pq = new PriorityQueue<Character>((a,b)->b-a);\n for(char ch:s.toCharArray()){\n \tpq.add(ch);\n }\n StringBuffer res = new StringBuffer();\n ArrayList<Character> list = new ArrayList<Character>();\n Stack<Character> stk = new Stack<Character>();\n int count = 0;\n char previouschar = pq.peek();\n while(!pq.isEmpty()){\n \tchar curr = pq.poll();\n \tif(curr==previouschar){\n \t\tif(count<repeatLimit){\n \t\t\tres.append(curr);\n \t\t}\n \t\telse{\n \t\t\tstk.add(curr);\n \t\t}\n \t\tcount++;\n \t}\n \telse{\n \t\tif(stk.isEmpty()){\n \t\t\tcount=0;\n \t\t\tres.append(curr);\n \t\t\tpreviouschar = curr;\n \t\t\tcount++;\n \t\t}\n \t\telse{\n \t\t\tres.append(curr);\n \t\t\tcount=0;\n \t\t\twhile(!stk.isEmpty() && count<repeatLimit){\n \t\t\t\tres.append(stk.pop());\n \t\t\t\tcount++;\n \t\t\t}\n \t\t}\n \t}\n }\n return res.toString();\n }\n\n\nHappy learning.. upvote if its helpful\n\n | 60 | 6 | ['Stack', 'Heap (Priority Queue)', 'Java'] | 18 |
construct-string-with-repeat-limit | C++ Greedy + Counting O(N) Time O(1) space | c-greedy-counting-on-time-o1-space-by-lz-dzpf | See my latest update in repo LeetCode\n\n\n## Solution 1. Greedy + Counting\n\nStore frequency of characters in int cnt[26].\n\nWe pick characters in batches. I | lzl124631x | NORMAL | 2022-02-20T04:00:54.263930+00:00 | 2022-02-20T04:00:54.263968+00:00 | 5,276 | false | See my latest update in repo [LeetCode](https://github.com/lzl124631x/LeetCode)\n\n\n## Solution 1. Greedy + Counting\n\nStore frequency of characters in `int cnt[26]`.\n\nWe pick characters in batches. In each batch, we pick the first character from `z` to `a` whose `cnt` is positive with the following caveats:\n1. If the current character is the same as the one used in the previous batch, we need to skip it.\n2. On top of case 1, if the `cnt` of the character used in the previous batch is positive, then we can only fill a single character in this batch.\n\n```cpp\n// OJ: https://leetcode.com/contest/weekly-contest-281/problems/construct-string-with-repeat-limit/\n// Author: github.com/lzl124631x\n// Time: O(N)\n// Space: O(1)\nclass Solution {\npublic:\n string repeatLimitedString(string s, int limit) {\n int cnt[26] = {};\n string ans;\n for (char c : s) cnt[c - \'a\']++;\n while (true) {\n int i = 25;\n bool onlyOne = false;\n for (; i >= 0; --i) {\n if (ans.size() && i == ans.back() - \'a\' && cnt[i]) { // the character of our last batch still has some count left, so we only insert a single character in this batch\n onlyOne = true;\n continue;\n }\n if (cnt[i]) break; // found a character with positive count, fill with this character\n }\n if (i == -1) break; // no more characters to fill, break;\n int fill = onlyOne ? 1 : min(cnt[i], limit);\n cnt[i] -= fill;\n while (fill--) ans += \'a\' + i;\n }\n return ans;\n }\n};\n``` | 57 | 3 | [] | 13 |
construct-string-with-repeat-limit | ๐Two Pointer Simple Solution ๐ง | two-pointer-simple-solution-by-sumeet_sh-8ac1 | ๐ง IntuitionWe are tasked with constructing the lexicographically largest string possible from the string s, while ensuring that no character appears more than r | Sumeet_Sharma-1 | NORMAL | 2024-12-17T01:25:33.461938+00:00 | 2024-12-17T05:34:35.395965+00:00 | 20,125 | false | # \uD83E\uDDE0 Intuition\n\nWe are tasked with constructing the lexicographically largest string possible from the string `s`, while ensuring that no character appears more than `repeatLimit` times consecutively.\n\nTo achieve this, we need to:\n- **Maximize lexicographical order**: We prioritize placing the lexicographically largest characters in the result first.\n- **Avoid exceeding the repeat limit**: We need to carefully manage the addition of characters to avoid placing any character more than `repeatLimit` times consecutively.\n\n### Key Insights:\n1. **Sorting in Descending Order**: Sorting the characters of the string in descending order allows us to first try to place the largest characters. This helps in creating the lexicographically largest string.\n2. **Consecutive Occurrences**: We need to ensure that we don\'t violate the constraint that no character appears more than `repeatLimit` times consecutively. If we try to add a character that would exceed this limit, we must skip it and use another character.\n\n# \uD83D\uDE80 Approach\n\n1. **Sort the String**:\n - We first sort the string `s` in descending order using `sort(s.rbegin(), s.rend())`. This ensures that the largest characters appear first, giving us the lexicographically largest possible result when constructing the string.\n \n2. **Iterate Over the Sorted String**:\n - We need to iterate through the sorted string and build the result string, while managing the consecutive occurrences of characters.\n - For each character in the string:\n - If it\'s the same as the last character added to the result, we check if it has already appeared `repeatLimit` times consecutively. If it has, we skip it and attempt to add a different character.\n - If it\'s a different character, we add it directly to the result string.\n\n3. **Handling the Repeat Limit**:\n - For every character added to the result, we track how many times that character has appeared using a variable `freq`.\n - If a character appears `repeatLimit` times consecutively, we move on to the next available character, which may involve skipping over repeated characters using a `pointer`.\n\n4. **Pointer Management**:\n - If we encounter a character that has already appeared `repeatLimit` times consecutively, we use a pointer to find the next distinct character in the sorted string and add that to the result.\n - The pointer ensures that we don\'t violate the repeat limit and helps us find the next valid character to place.\n\n5. **Final Output**:\n - After processing all the characters and carefully managing the repeat limit, we return the constructed string as the result.\n\n# \u23F3 Complexity\n\n- **Time complexity**:\n - Sorting the string takes **O(n log n)**, where `n` is the length of the string `s`.\n - The iteration through the string takes **O(n)**. Hence, the overall time complexity is dominated by the sorting step, giving a total of **O(n log n)**.\n\n- **Space complexity**:\n - We use a few variables (e.g., `freq`, `pointer`) and store the result string, which gives a space complexity of **O(n)**, where `n` is the length of the string.\n\n# \uD83D\uDCBB Code\n\n```cpp []\n#include <iostream>\n#include <vector>\n#include <algorithm>\n\nusing namespace std;\n\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n sort(s.rbegin(), s.rend());\n\n string result;\n int freq = 1;\n int pointer = 0;\n\n for (int i = 0; i < s.size(); ++i) {\n if (!result.empty() && result.back() == s[i]) {\n if (freq < repeatLimit) {\n result += s[i];\n freq++;\n } else {\n pointer = max(pointer, i + 1);\n while (pointer < s.size() && s[pointer] == s[i]) {\n pointer++;\n }\n\n if (pointer < s.size()) {\n result += s[pointer];\n swap(s[i], s[pointer]);\n freq = 1;\n } else {\n break;\n }\n }\n } else {\n result += s[i];\n freq = 1;\n }\n }\n\n return result;\n }\n};\n```\n```JavaScript []\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n s = sorted(s, reverse=True)\n result = []\n freq = 1\n pointer = 0\n\n i = 0\n while i < len(s):\n if result and result[-1] == s[i]:\n if freq < repeatLimit:\n result.append(s[i])\n freq += 1\n else:\n pointer = max(pointer, i + 1)\n while pointer < len(s) and s[pointer] == s[i]:\n pointer += 1\n\n if pointer < len(s):\n result.append(s[pointer])\n s[i], s[pointer] = s[pointer], s[i]\n freq = 1\n else:\n break\n else:\n result.append(s[i])\n freq = 1\n\n i += 1\n\n return \'\'.join(result)\n\n```\n```Java []\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n char[] chars = s.toCharArray();\n Arrays.sort(chars);\n StringBuilder result = new StringBuilder();\n \n int freq = 1;\n int pointer = chars.length - 1;\n\n for (int i = chars.length - 1; i >= 0; --i) {\n if (result.length() > 0 && result.charAt(result.length() - 1) == chars[i]) {\n if (freq < repeatLimit) {\n result.append(chars[i]);\n freq++;\n } else {\n while (pointer >= 0 && (chars[pointer] == chars[i] || pointer > i)) {\n pointer--;\n }\n\n if (pointer >= 0) {\n result.append(chars[pointer]);\n char temp = chars[i];\n chars[i] = chars[pointer];\n chars[pointer] = temp;\n freq = 1;\n } else {\n break;\n }\n }\n } else {\n result.append(chars[i]);\n freq = 1;\n }\n }\n return result.toString();\n }\n}\n\n```\n```Python3 []\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n chars = sorted(s, reverse=True)\n \n result = []\n freq = 1\n pointer = 0\n \n for i in range(len(chars)):\n if result and result[-1] == chars[i]:\n if freq < repeatLimit:\n result.append(chars[i])\n freq += 1\n else:\n pointer = max(pointer, i + 1)\n while pointer < len(chars) and chars[pointer] == chars[i]:\n pointer += 1\n \n if pointer < len(chars):\n result.append(chars[pointer])\n chars[i], chars[pointer] = chars[pointer], chars[i]\n freq = 1\n else:\n break\n else:\n result.append(chars[i])\n freq = 1\n \n return \'\'.join(result)\n\n```\n```C# []\nusing System;\nusing System.Linq;\nusing System.Text;\n\npublic class Solution {\n public string RepeatLimitedString(string s, int repeatLimit) {\n char[] chars = s.ToCharArray();\n Array.Sort(chars, (a, b) => b.CompareTo(a));\n\n StringBuilder result = new StringBuilder();\n int freq = 1;\n int pointer = 0;\n\n for (int i = 0; i < chars.Length; ++i) {\n if (result.Length > 0 && result[result.Length - 1] == chars[i]) {\n if (freq < repeatLimit) {\n result.Append(chars[i]);\n freq++;\n } else {\n pointer = Math.Max(pointer, i + 1);\n while (pointer < chars.Length && chars[pointer] == chars[i]) {\n pointer++;\n }\n\n if (pointer < chars.Length) {\n result.Append(chars[pointer]);\n char temp = chars[i];\n chars[i] = chars[pointer];\n chars[pointer] = temp;\n freq = 1;\n } else {\n break;\n }\n }\n } else {\n result.Append(chars[i]);\n freq = 1;\n }\n }\n\n return result.ToString();\n }\n}\n``` | 50 | 4 | ['Two Pointers', 'C++', 'Java', 'Python3', 'Ruby', 'JavaScript', 'C#'] | 18 |
construct-string-with-repeat-limit | [Python3] priority queue | python3-priority-queue-by-ye15-pyvn | Please pull this commit for solutions of weekly 281. \n\n\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n pq = [( | ye15 | NORMAL | 2022-02-20T04:03:24.517116+00:00 | 2022-02-21T22:06:51.329967+00:00 | 3,451 | false | Please pull this [commit](https://github.com/gaosanyong/leetcode/commit/793daa0aab0733bfadd4041fdaa6f8bdd38fe229) for solutions of weekly 281. \n\n```\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n pq = [(-ord(k), v) for k, v in Counter(s).items()] \n heapify(pq)\n ans = []\n while pq: \n k, v = heappop(pq)\n if ans and ans[-1] == k: \n if not pq: break \n kk, vv = heappop(pq)\n ans.append(kk)\n if vv-1: heappush(pq, (kk, vv-1))\n heappush(pq, (k, v))\n else: \n m = min(v, repeatLimit)\n ans.extend([k]*m)\n if v-m: heappush(pq, (k, v-m))\n return "".join(chr(-x) for x in ans)\n``` | 44 | 7 | ['Python3'] | 9 |
construct-string-with-repeat-limit | Priority Queue Solution โ
โ
| priority-queue-solution-by-arunk_leetcod-99ot | IntuitionThe problem requires constructing a string where no character appears more than k consecutive times while maximizing the lexicographical order. To achi | arunk_leetcode | NORMAL | 2024-12-17T05:33:24.621350+00:00 | 2024-12-17T05:33:24.621350+00:00 | 4,711 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem requires constructing a string where no character appears more than k consecutive times while maximizing the lexicographical order. To achieve this, the largest characters should be used as much as possible, but when a character reaches its limit of k consecutive occurrences, we temporarily "pause" its usage and switch to the next largest character.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Frequency Counting: Count the frequency of each character using a frequency array.\n2. Max-Heap: Use a priority queue (max-heap) to always process the largest available character.\n3. Building the Result:\n - Append the current largest character up to k times or until it runs out.\n - If the current character has more occurrences left, we "pause" it by temporarily skipping to the next largest character.\n - Insert the skipped character back into the heap to process it later.\n4. Edge Case: If there is no second largest character to "break" the sequence, the construction ends.\n\n# Complexity\n- Time complexity: $$O(Nlog26)$$ \n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(26)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string s, int k) {\n vector<int> freq(26, 0);\n for (char c : s) freq[c - \'a\']++;\n \n priority_queue<pair<char, int>> pq;\n for (int i = 0; i < 26; i++) {\n if (freq[i] > 0) pq.push({\'a\' + i, freq[i]});\n }\n \n string result;\n while (!pq.empty()) {\n auto [ch, count] = pq.top(); pq.pop();\n int used = min(k, count);\n result.append(used, ch);\n count -= used;\n\n if (count > 0) {\n if (pq.empty()) break;\n auto [nextCh, nextCount] = pq.top(); pq.pop();\n result += nextCh;\n nextCount--;\n\n if (nextCount > 0) pq.push({nextCh, nextCount});\n pq.push({ch, count});\n }\n }\n return result;\n }\n};\n\n```\n``` Java []\nimport java.util.PriorityQueue;\n\nclass Solution {\n public String repeatLimitedString(String s, int k) {\n int[] freq = new int[26];\n for (char c : s.toCharArray()) {\n freq[c - \'a\']++;\n }\n \n PriorityQueue<int[]> pq = new PriorityQueue<>((a, b) -> b[0] - a[0]);\n for (int i = 0; i < 26; i++) {\n if (freq[i] > 0) pq.offer(new int[] {i + \'a\', freq[i]});\n }\n \n StringBuilder result = new StringBuilder();\n while (!pq.isEmpty()) {\n int[] current = pq.poll();\n int ch = current[0], count = current[1];\n int used = Math.min(k, count);\n \n for (int i = 0; i < used; i++) {\n result.append((char) ch);\n }\n count -= used;\n \n if (count > 0) {\n if (pq.isEmpty()) break;\n int[] next = pq.poll();\n result.append((char) next[0]);\n next[1]--;\n \n if (next[1] > 0) pq.offer(next);\n pq.offer(new int[] {ch, count});\n }\n }\n \n return result.toString();\n }\n}\n```\n``` Python []\nimport heapq\nfrom collections import Counter\n\nclass Solution:\n def repeatLimitedString(self, s: str, k: int) -> str:\n freq = Counter(s)\n pq = [(-ord(char), count) for char, count in freq.items()]\n heapq.heapify(pq)\n \n result = []\n while pq:\n ch, count = heapq.heappop(pq)\n ch = chr(-ch)\n used = min(k, count)\n result.append(ch * used)\n count -= used\n \n if count > 0:\n if not pq: break\n next_ch, next_count = heapq.heappop(pq)\n result.append(chr(-next_ch))\n next_count -= 1\n \n if next_count > 0:\n heapq.heappush(pq, (next_ch, next_count))\n heapq.heappush(pq, (-ord(ch), count))\n \n return "".join(result)\n``` | 25 | 0 | ['Heap (Priority Queue)', 'Python', 'C++', 'Java', 'Python3'] | 3 |
construct-string-with-repeat-limit | Greedy | greedy-by-votrubac-ayeg | Count all characters in 26 buckets (\'a\' to \'z\'). \n2. While we have characters left:\n\t- Find the largest bucket i that still has characters.\n\t- Take up | votrubac | NORMAL | 2022-02-20T04:01:57.409501+00:00 | 2022-02-21T06:20:17.477404+00:00 | 2,580 | false | 1. Count all characters in 26 buckets (\'a\' to \'z\'). \n2. While we have characters left:\n\t- Find the largest bucket `i` that still has characters.\n\t- Take up to limit characters from bucket `i`.\n\t- Find the second largest bucket `j` that has characters.\n\t- Pick 1 character from bucket `j`.\n\nCaveats:\n- The largest bucket `i` could be the same as the second largest one `j` from the previous iteration.\n\t- So we should pick one less character from there.\n- Make sure `i` and `j` only go one direction (down) for the performance.\n\n**C++**\n```cpp\nstring repeatLimitedString(string s, int lim) {\n string res;\n int cnt[26] = {};\n for (auto ch : s)\n ++cnt[ch - \'a\'];\n for (int i = 25, j = 26; j >= 0; ) {\n while (i >= 0 && cnt[i] == 0)\n --i;\n if (i >= 0) {\n res += string(min(lim - (i == j), cnt[i]), \'a\' + i);\n cnt[i] -= min(lim - (i == j), cnt[i]);\n }\n j = min(i - 1, j);\n while (j >= 0 && cnt[j] == 0)\n --j; \n if (j >=0) {\n res += string(1, \'a\' + j);\n --cnt[j];\n }\n }\n return res;\n}\n``` | 25 | 1 | [] | 6 |
construct-string-with-repeat-limit | Greedy make_heap+ C-array vs priority_queue | greedy-mak_heap-c-array-by-anwendeng-de3i | IntuitionAgain Greedy heap with k operations where k= # of of diffrent chars.
Similar process is done by used priority_queue. 2 approaches have slight different | anwendeng | NORMAL | 2024-12-17T02:02:31.593668+00:00 | 2024-12-17T06:33:46.871328+00:00 | 3,448 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAgain Greedy heap with k operations where k= # of of diffrent chars.\nSimilar process is done by used priority_queue. 2 approaches have slight different processes for the 2nd max character.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. count `freq`\n2. build `heap` with char with freq>0\n3. Use `make_heap`\n4. While the `heap` is not empty, i.e. hz>0 do the following:\n```\npop_heap(heap, heap + hz);\nchar c= heap[hz-1];\nhz--;// pop\n\nint& f=freq[c-\'a\']; \nint use=min(f, k); // Add as many characters as possible (up to k)\nans.append(use, c);\nf-= use;\n\nif (f > 0 && hz > 0) {\n // Use the next character to interleave\n pop_heap(heap, heap+hz);\n char c2=heap[hz-1];\n hz--;// pop\n\n ans.push_back(c2);\n int& f2 = freq[c2-\'a\'];\n f2--;\n\n // Reinsert both characters back \n if (f2 > 0) {\n heap[hz++]=c2;// push c2\n push_heap(heap, heap + hz);\n }\n heap[hz++] = c; // push c\n push_heap(heap, heap + hz);\n} \n```\n5. return `ans`\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nmake_heap: $$O(n\\log k)$$\npriority_queue: $O((n+k)\\log n)$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n# Code Greedy heap\n```cpp [C++ heap]\nclass Solution {\npublic:\n static string repeatLimitedString(string& s, int k) {\n int freq[26]={0}, hz=0;\n\n // Count frequency\n for (char c : s) \n freq[c-\'a\']++;\n \n // Use C-array to act as max heap\n char heap[26];\n for (int i = 0; i < 26; i++) {\n if (freq[i]> 0) \n heap[hz++]=\'a\'+ i;\n }\n make_heap(heap, heap+hz);\n\n string ans;\n ans.reserve(s.size());\n\n while (hz > 0) {\n pop_heap(heap, heap + hz);\n char c= heap[--hz];\n\n int& f=freq[c-\'a\'];\n int use=min(f, k); // Add as many characters as possible (up to k)\n ans.append(use, c);\n f-= use;\n\n if (f > 0 && hz > 0) {\n // Use the next character to interleave\n pop_heap(heap, heap+hz);\n char c2=heap[--hz];\n\n ans.push_back(c2);\n int f2 =--freq[c2 - \'a\'];\n\n // Reinsert both characters back \n if (f2 > 0) {\n heap[hz++]=c2;\n push_heap(heap, heap + hz);\n }\n heap[hz++] = c;\n push_heap(heap, heap + hz);\n } \n }\n return ans;\n }\n};\n\nauto init = []() {\n ios::sync_with_stdio(0);\n cin.tie(0);\n cout.tie(0);\n return \'c\';\n}();\n\n```\n# C++ using priority_queue\n```cpp [C++ priority queue]\nclass Solution {\npublic:\n static string repeatLimitedString(string& s, int k) {\n int freq[26] = {0};\n // Count frequency\n for (char c : s) \n freq[c-\'a\']++;\n \n // Use a max-heap to hold char\n priority_queue<char> pq;\n for (int i=0; i<26; i++) {\n if (freq[i] > 0) \n pq.emplace(\'a\'+i);\n }\n \n string ans;\n ans.reserve(s.size());\n \n while (!pq.empty()) {\n char c=pq.top();\n pq.pop();\n \n // Add as many characters as possible (up to k)\n int& f=freq[c-\'a\'];\n int use = min(f, k);\n ans.append(use, c);\n f-= use;\n\n if (f > 0) {\n if (pq.empty()) \n break; // No other character to interleave\n \n // Use the next character in priority queue to interleave\n char c2= pq.top();\n ans.push_back(c2);\n int f2=--freq[c2-\'a\'];\n\n if (f2==0) pq.pop();\n\n // Re-add the original character \n pq.emplace(c);\n }\n }\n \n return ans;\n }\n};\n\nauto init = []() {\n ios::sync_with_stdio(0);\n cin.tie(0);\n cout.tie(0);\n return \'c\';\n}();\n``` | 21 | 0 | ['String', 'Greedy', 'Heap (Priority Queue)', 'C++'] | 8 |
construct-string-with-repeat-limit | Python | Straightforward Solution | Counting | python-straightforward-solution-counting-ms2v | We store the frequency of each character of the string in an array(A[26]). Now we start from the last index of the array which will store z as we need to genera | mohd_mustufa | NORMAL | 2022-02-20T04:16:54.244534+00:00 | 2022-02-20T04:16:54.244561+00:00 | 1,221 | false | We store the frequency of each character of the string in an array(A[26]). Now we start from the last index of the array which will store z as we need to generate output in lexiographically increasing order.\n\n```\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n arr = [0] * 26\n a = ord(\'a\')\n \n for char in s:\n arr[ord(char)-a] += 1\n\t\t\t\n # Using an array to store the answer as appending to a string is a costly operation\n ans = []\n curr = 25\n prev = 24 \n\t\t\n # We are starting with curr = 25 as we need to add the largest character first\n while curr >= 0:\n if arr[curr] == 0:\n curr -= 1\n continue\n \n\t\t\t# If the character is present in the string then we add it to our answer \n for i in range(min(repeatLimit, arr[curr])):\n ans.append(chr(curr + a))\n arr[curr] -= 1\n \n\t\t\t# If the above for loop was able to add all the characters then we can move on to the next one\n if arr[curr] == 0:\n curr -= 1\n continue\n \n\t\t\t# If the above for loop was not able to add all the characters then we need to add a second largest character before we can continue adding the largest character to our answer again\n g = False\n for j in range(min(prev, curr-1), -1, -1):\n if arr[j]:\n g = True\n arr[j] -= 1\n prev = j\n ans.append(chr(j+a))\n break\n\t\t\t# If g is false then we know that there were no other characters present other than the largest character\n if not g: break\n \n return \'\'.join(ans)\n``` | 15 | 4 | [] | 2 |
construct-string-with-repeat-limit | Python | Greedy Algorithm & Priority Queue | python-greedy-algorithm-priority-queue-b-tddx | see the Successfully Accepted SubmissionCodeSteps:
Frequency Count:
Use a Counter to count the frequency of each character in the string.
Max Heap:
Use a max | Khosiyat | NORMAL | 2024-12-17T01:08:00.090981+00:00 | 2024-12-17T01:08:00.090981+00:00 | 1,233 | false | [see the Successfully Accepted Submission](https://leetcode.com/problems/construct-string-with-repeat-limit/submissions/1480683191/?envType=daily-question&envId=2024-12-17)\n\n# Code\n```python3 []\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n # Count frequencies of each character\n freq = Counter(s)\n \n # Use a max heap (negative frequencies for max heap simulation)\n max_heap = []\n for char, count in freq.items():\n heapq.heappush(max_heap, (-ord(char), count)) # Store (negative ASCII value, count)\n \n result = [] # Final result list\n while max_heap:\n # Extract the largest character\n neg_char, count = heapq.heappop(max_heap)\n char = chr(-neg_char)\n \n # Determine how many times we can use this character (up to repeatLimit)\n use_count = min(count, repeatLimit)\n result.extend([char] * use_count)\n \n count -= use_count # Remaining count after usage\n \n if count > 0: # If there are more of this character left\n if not max_heap:\n break # No other characters to use, we are done\n \n # Extract the second largest character to "break" the sequence\n neg_next_char, next_count = heapq.heappop(max_heap)\n next_char = chr(-neg_next_char)\n \n # Use the second largest character once to break the sequence\n result.append(next_char)\n next_count -= 1 # Update the count\n \n # Push both characters back into the heap if they still have remaining counts\n heapq.heappush(max_heap, (neg_char, count))\n if next_count > 0:\n heapq.heappush(max_heap, (neg_next_char, next_count))\n \n # Join the list into the final string\n return "".join(result)\n\n```\n\n## Steps:\n1. **Frequency Count**: \n Use a `Counter` to count the frequency of each character in the string.\n\n2. **Max Heap**: \n Use a max heap (priority queue) to always extract the lexicographically largest character. \n - In Python, we simulate a max heap using **negative frequencies**.\n\n3. **Construct the String**: \n - Repeatedly pick the largest character while respecting the `repeatLimit`. \n - If the `repeatLimit` for the current character is reached, temporarily use the next largest character to *break* the sequence and then return to the largest character.\n\n4. **Edge Cases**: \n If it is impossible to continue constructing the string (e.g., no more characters are available to "break" a sequence), stop.\n\n---\n\n## Explanation of Key Steps:\n\n### 1. **Heap Initialization** \n- Each character\'s negative ASCII value and count are pushed into the heap. \n- This ensures the lexicographically largest characters are prioritized.\n\n### 2. **Constructing the String** \n- While the heap is not empty: \n - Extract the largest character and use it up to `repeatLimit` times. \n - If there are still more occurrences of this character left, use the **second largest character** to *break* the consecutive sequence.\n\n### 3. **Reinserting Characters** \n- Both the largest and second largest characters are reinserted back into the heap if they have remaining occurrences.\n\n### 4. **Stopping Condition** \n- If there are no other characters to "break" the sequence, the process ends.\n\n---\n\n## Complexity Analysis:\n\n### Time Complexity:\n- Pushing and popping from the heap takes \\( O(\\log k) \\), where \\( k \\) is the number of distinct characters. \n- Each character in the string is processed once, so the overall time complexity is \\( O(n \\log k) \\), where \\( n \\) is the length of the string.\n\n### Space Complexity:\n- The heap stores at most \\( O(k) \\) characters, and the result list takes \\( O(n) \\) space. \n- Therefore, the overall space complexity is \\( O(n + k) \\).\n\n\n\n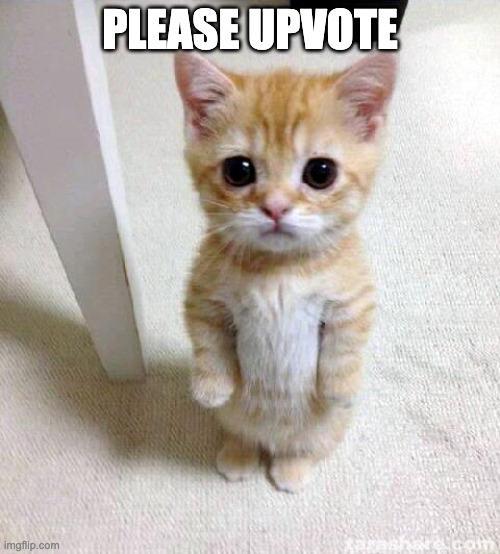 | 11 | 0 | ['Python3'] | 0 |
construct-string-with-repeat-limit | Logic explained With Comments || Priority Queue | logic-explained-with-comments-priority-q-m8x1 | As we need lexicographically largest string so start adding largest character available.\nOne thing we need to take care of is we cannot repeat a character more | kamisamaaaa | NORMAL | 2022-02-20T04:22:00.167222+00:00 | 2022-02-21T04:28:43.709618+00:00 | 879 | false | As we **need lexicographically largest string** so start **adding largest character available**.\nOne thing we need to **take care of** is we **cannot repeat a character more than repeatLimit times** so **when this happens** **add 1 next greatest charcter.**\n```\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n \n int n(size(s));\n vector <int> m(26, 0);\n for (auto ch : s) m[ch-\'a\']++;\n \n priority_queue <pair<char, int>> pq;\n for (int i=0; i<26; i++)\n if (m[i]) \n pq.push({i+\'a\', m[i]});\n \n string res;\n \n while (!pq.empty()) {\n\t\t\n auto top = pq.top(); pq.pop(); // top contains lexicographically greatest character\n here :\n int lim = min(top.second, repeatLimit); // lim is number of times top can be added\n top.second -= lim;\n\t\t\t\n while (lim--) res.push_back(top.first);\n if (top.second > 0) {\n if (pq.empty()) return res; // if we dont get next greatest character then we cannot use remaining characters so return res\n auto next = pq.top(); pq.pop(); // next contains lexicographically greatest character smaller than top\n res.push_back(next.first);\n next.second -= 1;\n if (next.second) pq.push(next);\n goto here;\n }\n }\n \n return res;\n }\n};\n```\n\n**Upvote if it helps :)** | 9 | 0 | ['C', 'Heap (Priority Queue)'] | 2 |
construct-string-with-repeat-limit | Python short solution using heap | python-short-solution-using-heap-by-cubi-n101 | \n \n \n The idea is simple, keep a max heap of all the characters and their available counts.\n If the max character element at the t | Cubicon | NORMAL | 2022-02-20T04:01:49.333320+00:00 | 2022-02-20T04:01:49.333355+00:00 | 505 | false | \n \n \n The idea is simple, keep a max heap of all the characters and their available counts.\n If the max character element at the top of the heap is already exceeding the limit interms of count,\n then pop the next one and add to heap. Also add back the unused max character element to the heap.\n \n \n```\ndef repeatLimitedString(self, s: str, limit: int) -> str:\n\theap = [ (-ord(ch), ch, count) for ch, count in Counter(s).items() ]\n\theapify(heap)\n\tres, count = [], 0\n\n\twhile heap:\n\t\tmax_heap_key, ch, rem = heappop(heap)\n\t\tif res and res[-1] == ch and (count+1) > limit:\n\t\t\tif not heap: break\n\t\t\tmax_heap_key2, ch2, rem2 = heappop(heap)\n\t\t\theappush(heap, (max_heap_key, ch, rem))\n\t\t\tmax_heap_key, ch, rem = max_heap_key2, ch2, rem2\n\n\t\tif res and res[-1] != ch:\n\t\t\tcount = 0\n\n\t\tres.append(ch)\n\t\trem -= 1\n\t\tif rem:\n\t\t\theappush(heap, (max_heap_key, ch, rem))\n\t\tcount += 1\n\treturn \'\'.join(res) \n```\n```\n\t | 9 | 0 | [] | 0 |
construct-string-with-repeat-limit | Solution for LeetCode#2182 | solution-for-leetcode2182-by-samir023041-us3r | IntuitionThe problem requires creating a lexicographically largest string with a limit on consecutive repeating characters. The intuition is to use a greedy app | samir023041 | NORMAL | 2024-12-17T05:18:14.199718+00:00 | 2024-12-17T05:21:22.713648+00:00 | 558 | false | 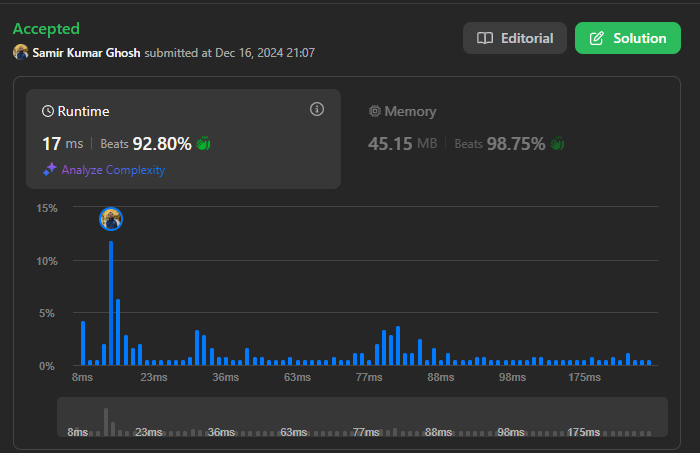\n\n# Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem requires creating a lexicographically largest string with a limit on consecutive repeating characters. The intuition is to use a greedy approach, starting with the largest character and working our way down.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Count the frequency of each character in the input string.\n2. Start from the largest character (z) and work downwards.\n3. For each character, add it to the result string up to the repeat limit.\n4. If there are more occurrences of a character than the repeat limit, find the next available smaller character to insert as a separator.\n5. Repeat this process until all characters are used.\n\n# Complexity\n- Time complexity: O(n+k)\n - n: Total number of characters in the input string.\n - k: Number of unique characters in the input string (at most 26 for lowercase letters).\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(26)\u2192O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```java []\nclass Solution { \n\n public String repeatLimitedString(String s, int repeatLimit) {\n int n=s.length();\n StringBuilder sb=new StringBuilder();\n\n // Step 1: Frequency count for each character\n int[] arr=new int[26];\n for(int i=0; i<n; i++){\n arr[s.charAt(i)-\'a\']++;\n }\n\n // Step 2: Iterate from larger characters to smaller characters\n for(int i=25; i>=0; i--){ // i: One pointer\n if(arr[i]==0) continue;\n \n int curr=Math.min(arr[i], repeatLimit);\n arr[i]-=curr;\n while(curr>0){ \n sb.append( (char)(\'a\'+i) );\n curr--;\n } \n\n // Step 3: Handle remaining occurrences by inserting smaller character\n if(arr[i]!=0){\n for(int j=i-1; j>=0; j--){ // j: Another pointer\n if(arr[j]==0) continue;\n sb.append( (char)(\'a\'+j) ); // Adding only one character \n arr[j]--;\n i++;\n break;\n } \n } \n }\n \n return sb.toString(); \n }\n\n\n}\n``` | 8 | 0 | ['Counting', 'Java'] | 1 |
construct-string-with-repeat-limit | Simple solution C++| Java| Python | Javascript | simple-solution-c-java-python-javascript-5sth | Solution in C++, Python, Java, and JavaScriptIntuitionWe need to construct a string that ensures no character appears more than repeatLimit times consecutively. | BijoySingh7 | NORMAL | 2024-12-17T04:50:51.159486+00:00 | 2024-12-17T04:50:51.159486+00:00 | 2,205 | false | \n\n###### *Solution in C++, Python, Java, and JavaScript*\n\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n vector<int> freq(26, 0);\n for (char c : s) freq[c - \'a\']++;\n priority_queue<pair<char, int>> pq;\n for (int i = 0; i < 26; i++) if (freq[i] > 0) pq.push({i + \'a\', freq[i]});\n string result;\n while (!pq.empty()) {\n auto [ch, count] = pq.top(); pq.pop();\n int use = min(repeatLimit, count);\n result.append(use, ch);\n count -= use;\n if (count > 0) {\n if (!pq.empty()) {\n auto [nextCh, nextCount] = pq.top(); pq.pop();\n result.push_back(nextCh);\n if (--nextCount > 0) pq.push({nextCh, nextCount});\n pq.push({ch, count});\n }\n }\n }\n return result;\n }\n};\n```\n\n```python []\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n from heapq import heapify, heappop, heappush\n freq = [0] * 26\n for c in s:\n freq[ord(c) - ord(\'a\')] += 1\n pq = [(-i, -count) for i, count in enumerate(freq) if count > 0]\n heapify(pq)\n result = []\n while pq:\n ch, count = heappop(pq)\n count = -count\n use = min(repeatLimit, count)\n result.append(chr(-ch + ord(\'a\')) * use)\n count -= use\n if count > 0:\n if pq:\n nextCh, nextCount = heappop(pq)\n result.append(chr(-nextCh + ord(\'a\')))\n if nextCount + 1 < 0:\n heappush(pq, (nextCh, nextCount + 1))\n heappush(pq, (ch, -count))\n return \'\'.join(result)\n```\n\n```java []\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int[] freq = new int[26];\n for (char c : s.toCharArray()) freq[c - \'a\']++;\n PriorityQueue<int[]> pq = new PriorityQueue<>((a, b) -> b[0] - a[0]);\n for (int i = 0; i < 26; i++) if (freq[i] > 0) pq.add(new int[]{i, freq[i]});\n StringBuilder result = new StringBuilder();\n while (!pq.isEmpty()) {\n int[] curr = pq.poll();\n int use = Math.min(repeatLimit, curr[1]);\n result.append(String.valueOf((char) (\'a\' + curr[0])).repeat(use));\n curr[1] -= use;\n if (curr[1] > 0 && !pq.isEmpty()) {\n int[] next = pq.poll();\n result.append((char) (\'a\' + next[0]));\n next[1]--;\n if (next[1] > 0) pq.add(next);\n pq.add(curr);\n }\n }\n return result.toString();\n }\n}\n```\n\n```javascript []\nclass Solution {\n repeatLimitedString(s, repeatLimit) {\n const freq = Array(26).fill(0);\n for (const c of s) freq[c.charCodeAt() - 97]++;\n const pq = [];\n for (let i = 25; i >= 0; i--) {\n if (freq[i] > 0) pq.push([String.fromCharCode(97 + i), freq[i]]);\n }\n const result = [];\n while (pq.length) {\n let [ch, count] = pq.shift();\n const use = Math.min(repeatLimit, count);\n result.push(ch.repeat(use));\n count -= use;\n if (count > 0 && pq.length) {\n let [nextCh, nextCount] = pq.shift();\n result.push(nextCh);\n if (--nextCount > 0) pq.unshift([nextCh, nextCount]);\n pq.push([ch, count]);\n }\n }\n return result.join(\'\');\n }\n}\n```\n\n# Intuition\nWe need to construct a string that ensures no character appears more than `repeatLimit` times consecutively. To make it lexicographically largest, we use characters in descending order.\n\n# Approach\n1. Use a frequency map to count the occurrences of each character.\n2. Use a priority queue (max-heap) to ensure we always pick the lexicographically largest available character.\n3. Append the character up to `repeatLimit` times, and if there are still remaining characters, we insert the next largest available character.\n4. Continue until all valid characters are used.\n\n# Complexity\n- Time complexity: $$O(n \\log k)$$, where `n` is the size of the string and `k` is the distinct character count.\n- Space complexity: $$O(k)$$, where `k` is the number of unique characters.\n\n### *If you have any questions or need further clarification, feel free to drop a comment! \uD83D\uDE0A* | 8 | 0 | ['String', 'Python', 'C++', 'Java', 'JavaScript'] | 7 |
construct-string-with-repeat-limit | [Java] Using char counting array with O(1) space | java-using-char-counting-array-with-o1-s-ffcx | My easy-understanding solution, O(n) time complexity and O(26) -> O(1) space.\n\nclass Solution {\n public String repeatLimitedString(String s, int repeatLim | SiyuanXing | NORMAL | 2022-02-20T04:11:44.607792+00:00 | 2022-02-20T04:34:28.607677+00:00 | 893 | false | My easy-understanding solution, O(n) time complexity and O(26) -> O(1) space.\n```\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int[] counter = new int[26];\n int max = 0;\n for (char ch : s.toCharArray()) {\n int curr = ch - \'a\';\n max = Math.max(max, curr);\n counter[curr]++;\n }\n int repeated = 0;\n StringBuilder builder = new StringBuilder();\n while (max >= 0) {\n builder.append((char)(\'a\' + max));\n counter[max]--;\n repeated++;\n if (counter[max] == 0) {\n max = findNextMax(counter, max - 1);\n repeated = 0;\n\t\t\t\tcontinue;\n } \n if (repeated == repeatLimit) {\n\t\t\t\t// Greedy, use the next possible char once and get back to curr.\n\t\t\t\t// if no other char available, the curr word is the largest subsequence. \n int lower = findNextMax(counter, max - 1);\n if (lower < 0) {\n return builder.toString();\n }\n builder.append((char)(\'a\' + lower));\n counter[lower]--;\n repeated = 0;\n }\n }\n return builder.toString();\n }\n \n private int findNextMax(int[] counter, int from) {\n int curr = from;\n while (curr >= 0) {\n if (counter[curr] > 0) {\n return curr;\n }\n curr--;\n }\n return curr;\n }\n}\n``` | 8 | 1 | ['Counting', 'Java'] | 4 |
construct-string-with-repeat-limit | Greedy Python Solution | 100% Runtime | greedy-python-solution-100-runtime-by-an-qenq | Upvote if you find this article helpful\u2B06\uFE0F.\n### STEPS:\n1. Basically we make a sorted table with freq of each character.\n2. Then we take the most lex | ancoderr | NORMAL | 2022-02-20T04:03:31.221909+00:00 | 2022-02-20T04:42:09.566575+00:00 | 1,104 | false | Upvote if you find this article helpful\u2B06\uFE0F.\n### STEPS:\n1. Basically we make a sorted table with freq of each character.\n2. Then we take the most lexicographically superior character (Call it A).\n3. If its freq is in limits, directly add it.\n4. If its freq is more than the limit. Add repeatLimit number of A\'s and then search for the next lexicographically superior character(Call it TEMP) and add it just once, as we will again add the remaining A\'s.\n5. Repeat this process until either all A\'s are consumed or we run out of TEMP characters.\n6. Repeat all these steps for all other remaining characters in lexicographic order.\n\n**NOTE: Here we make the Greedy choice of choosing a lexicographically superior character at each step, while selecting A and also while selecting TEMP.**\n**NOTE: I have not removed characters if a characters freq becomes 0. This is because random pop() operations in the middle of a list takes O(n) time. For pop() operations in default index(End of list) is O(1). Refer to this article \u27A1\uFE0F [Time Complexity of Python Library Functinons](https://wiki.python.org/moin/TimeComplexity)** \n\n### STATUS:\n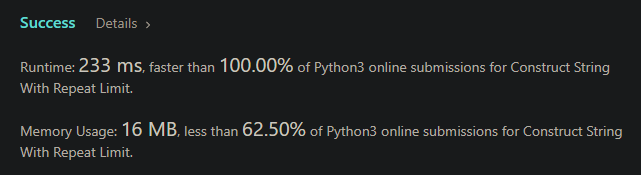\n\n### CODE:\n```\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n table = Counter(s)\n char_set = [\'0\', \'a\', \'b\', \'c\', \'d\', \'e\', \'f\', \'g\', \'h\', \'i\', \'j\', \'k\', \'l\', \'m\', \'n\', \'o\', \'p\', \'q\', \'r\', \'s\',\n \'t\', \'u\', \'v\', \'w\', \'x\', \'y\', \'z\']\n sorted_table = []\n for i in range(26,-1,-1):\n if char_set[i] in table:\n sorted_table.append((char_set[i],table[char_set[i]]))\n\n result = ""\n n = len(sorted_table)\n for i in range(n):\n char, curr_freq = sorted_table[i] # The lexicographically larger character and its frequency\n index_to_take_from = i + 1 # We take from this index the next lexicographically larger character(TEMP) if the repeatLimit for A is exceeded\n while curr_freq > repeatLimit: # Limit exceeded\n result += char*repeatLimit # Add repeatLimit number of character A\'s\n curr_freq -= repeatLimit # Decrease frequency of character A\n # Now we search for the next lexicographically superior character that can be used once\n while index_to_take_from < n: # Till we run out of characters\n ch_avail, freq_avail = sorted_table[index_to_take_from]\n if freq_avail == 0: # If its freq is 0 that means that it was previously used. This is done as we are not removing the character from table if its freq becomes 0. \n index_to_take_from += 1 # Check for next lexicographically superior character\n else:\n result += ch_avail # If found then add that character \n sorted_table[index_to_take_from] = (ch_avail,freq_avail-1) # Update the new characters frequency\n break\n else:\n break # We cannot find any lexicographically superior character\n else:\n result += char*curr_freq # If the freq is in limits then just add them\n return result\n```\n | 8 | 4 | ['Python', 'Python3'] | 2 |
construct-string-with-repeat-limit | EASY SOLUTION USING HEAP FOR BEGINNER'S!!! | easy-solution-using-heap-for-beginners-b-y5xg | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | aero_coder | NORMAL | 2024-12-17T01:08:05.768990+00:00 | 2024-12-17T21:39:02.321313+00:00 | 1,194 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution\n{\npublic:\n string repeatLimitedString(string s, int repeatLimit)\n {\n priority_queue<pair<char, int>> pq;\n map<char, int> mp;\n for (auto &it : s)\n {\n mp[it]++;\n }\n for (auto it : mp)\n {\n pq.emplace(it.first, it.second);\n }\n string ans;\n while (!pq.empty())\n {\n auto it = pq.top();\n pq.pop();\n char ch = it.first;\n int cnt = it.second;\n int use = min(cnt, repeatLimit);\n ans.append(use, ch);\n cnt -= use;\n if (cnt > 0)\n {\n if (!pq.empty())\n {\n auto sc = pq.top();\n pq.pop();\n char nextChar = sc.first;\n int nextCnt = sc.second;\n ans.push_back(nextChar);\n nextCnt--;\n if (nextCnt > 0)\n {\n pq.emplace(nextChar, nextCnt);\n }\n }\n else\n {\n break;\n }\n pq.emplace(ch, cnt);\n }\n }\n return ans;\n }\n};\n\n```\n\n | 7 | 0 | ['Heap (Priority Queue)', 'C++'] | 1 |
construct-string-with-repeat-limit | โ
Efficient๐ฅBeats 98,39%๐กBeginnerโ
C++ | Java | C# | Python๐ฅO(n) - O(1) | with-explanation-c-beats-944-on-o1-by-kh-h10u | Algorithm consists of 2 steps:Step 1) - Setup
Create 2 int[] arrays, symbolCount and indices with size of 26 (number of letters in the alphabet).
And fill them | khavv | NORMAL | 2024-12-17T22:36:48.285500+00:00 | 2024-12-19T10:02:20.970878+00:00 | 91 | false | 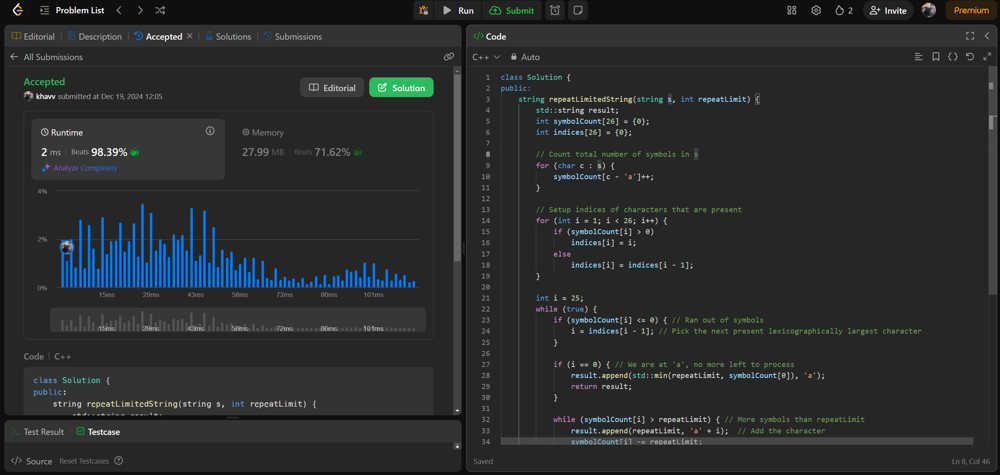
### Algorithm consists of 2 steps:
***Step 1)*** - Setup
Create 2 `int[]` arrays, `symbolCount` and `indices` with size of 26 (number of letters in the alphabet).
And fill them in the following manner:
`symbolCount` stores count of each letter in `s`
`indices` is set up in such a way, so that every `[i-1]` element would refer to the closest nonempty element of `symbolCount`
**Example** - limit the number of letters to `6` for simplicity
If `s` is `aaaceccf`, than `symbolCount`would look like this: `[3,0,3,0,1,1]` and `indices` would look like this: `[0,0,2,2,4,5]`.
Again, every element of `indices` stores the index of closest nonempty element of `symbolCount`
***Step 2)*** - Iterate
After setup what is left is the main while loop. It has pretty descriptive comments in the code section, but I'll try to summarize the algorithm in simple language. Basically, it iterates over the `symbolCount` array, from right to left. Whenever `symbolCount[i] == 0` it changes `i` to the next nonempty element of `symbolCount` which is in fact `indices[i-1]`
Time complexity: $$O(n)$$
Space complexity: $$O(1)$$
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```csharp []
public string RepeatLimitedString(string s, int repeatLimit){
StringBuilder sb = new StringBuilder();
int[] symbolCount = new int[26];
int[] indices = new int[26];
int i = 0;
for (; i < s.Length; i++) // Count total number of symbols in s
symbolCount[(int)s[i] - 97]++;
for (i = 1; i < 26; i++){ // Setup indices
if (symbolCount[i] > 0)
indices[i] = i;
else
indices[i] = indices[i-1];
}
i = 25;
while (true){ // Loop for every letter 'z'->'a'
if (symbolCount[i] <= 0) // Ran out of symbols
i = indices[i - 1]; // Pick the next present llc
if (i == 0){ // i points to 'a', so we can no longer take llc from the left
sb.Append((char)97, Math.Min(repeatLimit, symbolCount[0])); // Add whatever is left
return sb.ToString();
}
while (symbolCount[i] > repeatLimit){ // Handle the case when we have more symbols than repeatLimit
sb.Append((char)(i + 97), repeatLimit);
symbolCount[i] -= repeatLimit;
if (symbolCount[indices[i-1]] <= 0){ // If next llc is empty
if (indices[i-1] <= 0) // If indices[i-1] points to 'a' => can't take the next llc
return sb.ToString();
indices[i-1] = indices[indices[i-1]-1]; // Move to the next lexicographically largest
}
sb.Append((char)(indices[i-1] + 97)); // Add "filler" character
symbolCount[indices[i-1]]--;
}
sb.Append((char)(i + 97), symbolCount[i]); // Add remaining characters
symbolCount[i] = 0;
}
}
```
```C++ []
string repeatLimitedString(string s, int repeatLimit) {
std::string result;
int symbolCount[26] = {0};
int indices[26] = {0};
for (char c : s) { // Count total number of symbols in s
symbolCount[c - 'a']++;
}
for (int i = 1; i < 26; i++) { // Setup indices
if (symbolCount[i] > 0)
indices[i] = i;
else
indices[i] = indices[i - 1];
}
int i = 25;
while (true) { // Loop for every letter 'z'->'a'
if (symbolCount[i] <= 0) { // Ran out of symbols
i = indices[i - 1]; // Pick the next present llc
}
if (i == 0) { // i points to 'a', so we can no longer take llc from the left
result.append(std::min(repeatLimit, symbolCount[0]), 'a'); // Add whatever is left
return result;
}
while (symbolCount[i] > repeatLimit) { // Handle the case when we have more symbols than repeatLimit
result.append(repeatLimit, 'a' + i); // Add the character
symbolCount[i] -= repeatLimit;
if (symbolCount[indices[i - 1]] <= 0) { // If next llc is empty
if (indices[i - 1] <= 0) // If indices[i-1] points to 'a' => can't take the next llc
return result;
indices[i - 1] = indices[indices[i - 1] - 1]; // Move to the next lexicographically largest
}
result.push_back('a' + indices[i - 1]); // Add "filler" character
symbolCount[indices[i - 1]]--;
}
result.append(symbolCount[i], 'a' + i); // Add remaining characters
symbolCount[i] = 0;
}
}
```
```Java []
public String repeatLimitedString(String s, int repeatLimit) {
StringBuilder sb = new StringBuilder();
int[] symbolCount = new int[26];
int[] indices = new int[26];
int i = 0;
for (; i < s.length(); i++) // Count total number of symbols in s
symbolCount[s.charAt(i) - 'a']++;
for (i = 1; i < 26; i++) { // Setup indices
if (symbolCount[i] > 0)
indices[i] = i;
else
indices[i] = indices[i - 1];
}
i = 25;
while (true) { // Loop for every letter 'z'->'a'
if (symbolCount[i] <= 0) // Ran out of symbols
i = indices[i - 1]; // Pick the next present llc
if (i == 0) { // i points to 'a', so we can no longer take llc from the left
sb.append(String.valueOf((char) ('a' + 0)).repeat(Math.min(repeatLimit, symbolCount[0]))); // Add whatever is left
return sb.toString();
}
while (symbolCount[i] > repeatLimit) { // Handle the case when we have more symbols than repeatLimit
sb.append(String.valueOf((char) ('a' + i)).repeat(repeatLimit));
symbolCount[i] -= repeatLimit;
if (symbolCount[indices[i - 1]] <= 0) { // If next llc is empty
if (indices[i - 1] <= 0) // If indices[i-1] points to 'a' => can't take the next llc
return sb.toString();
indices[i - 1] = indices[indices[i - 1] - 1]; // Move to the next lexicographically largest
}
sb.append((char) ('a' + indices[i - 1])); // Add "filler" character
symbolCount[indices[i - 1]]--;
}
sb.append(String.valueOf((char) ('a' + i)).repeat(symbolCount[i])); // Add remaining characters
symbolCount[i] = 0;
}
}
```
```Python []
def repeatLimitedString(self, s: str, repeatLimit: int) -> str:
sb = []
symbol_count = [0] * 26
indices = [0] * 26
i = 0
# Count total number of symbols in s
for char in s:
symbol_count[ord(char) - 97] += 1
# Setup indices
for i in range(1, 26):
if symbol_count[i] > 0:
indices[i] = i
else:
indices[i] = indices[i - 1]
i = 25
while True: # Loop for every letter 'z' -> 'a'
if symbol_count[i] <= 0: # Ran out of symbols
i = indices[i - 1] # Pick the next present lexicographically largest character
if i == 0: # i points to 'a', so we can no longer take lexicographically largest from the left
sb.append(chr(97) * min(repeatLimit, symbol_count[0])) # Add whatever is left
return ''.join(sb)
while symbol_count[i] > repeatLimit: # Handle the case when we have more symbols than repeatLimit
sb.append(chr(i + 97) * repeatLimit)
symbol_count[i] -= repeatLimit
if symbol_count[indices[i - 1]] <= 0: # If next lexicographically largest character is empty
if indices[i - 1] <= 0: # If indices[i-1] points to 'a' => can't take the next lexicographically largest
return ''.join(sb)
indices[i - 1] = indices[indices[i - 1] - 1] # Move to the next lexicographically largest
sb.append(chr(indices[i - 1] + 97)) # Add "filler" character
symbol_count[indices[i - 1]] -= 1
sb.append(chr(i + 97) * symbol_count[i]) # Add remaining characters
symbol_count[i] = 0
``` | 5 | 0 | ['Python', 'C++', 'Java', 'C#'] | 0 |
construct-string-with-repeat-limit | Priority Queue (Max Heap) solution in easy way. (java, c++, python) | priority-queue-max-heap-solution-in-easy-lhqj | ApproachSteps to Solve the ProblemUnderstand the Problem:You are given a string s with lowercase letters.
You need to construct a new string where:
Any characte | Tanmay_bhure | NORMAL | 2024-12-17T08:48:48.005181+00:00 | 2024-12-17T08:48:48.005181+00:00 | 533 | false | # Approach\n# Steps to Solve the Problem\n\n**Understand the Problem:**\n\nYou are given a string s with lowercase letters.\nYou need to construct a new string where:\nAny character can appear consecutively at most limit times.\nThe resulting string should be lexicographically the largest possible.\nUse a greedy approach with a priority queue (max-heap) to ensure you always select the largest character available.\n\n# Steps to Solve the Problem:\n\n**Step 1: Count Frequencies**\nUse a HashMap (or similar data structure) to count the occurrences of each character in the string.\n\n**Step 2: Add Characters to a Max-Heap**\nAdd all characters to a priority queue (max-heap) so you can always get the largest available character.\n\n**Step 3: Build the Resulting String**\nUse a StringBuilder (or string in Python/C++) to append characters while respecting the limit constraint.\n\n1. If the count of the current character is less than or equal to limit, append all occurrences.\n2. If the count exceeds limit, append limit occurrences, then:\nTemporarily select the next largest character to break the repetition.\n3. Return the original character back to the heap if it still has remaining occurrences.\n\n**Step 4: Edge Cases**\nEnsure proper handling when the priority queue becomes empty or when characters are exhausted.\n\n# Complexity\n- Time complexity:\n**O( n log k )** where n= length of string and k= no of unique char in string\n\n- Space complexity:\n**O(n)**\n\n# Code\n```java []\nclass Solution {\n public String repeatLimitedString(String s, int limit) {\n HashMap<Character,Integer> map= new HashMap<>();\n for(char c: s.toCharArray()) map.put(c,map.getOrDefault(c,0)+1);\n PriorityQueue<Character> pq= new PriorityQueue<>((a,b)->b-a);\n for(char k: map.keySet()) pq.offer(k);\n StringBuilder sb= new StringBuilder();\n while(!pq.isEmpty()){\n char c=pq.poll();\n int count=map.get(c);\n if(count<=limit){\n for(int i=0;i<count;++i) sb.append(c);\n map.remove(c);\n }\n else{\n for(int i=0;i<limit;++i) sb.append(c);\n map.put(c,map.get(c)-limit);\n if(pq.isEmpty()) return sb.toString();\n char next=pq.poll();\n sb.append(next);\n map.put(next,map.get(next)-1);\n if(map.get(next)==0) map.remove(next);\n if(map.containsKey(next)) pq.offer(next);\n pq.offer(c);\n }\n }\n return sb.toString();\n }\n}\n```\n``` C++ []\n#include <queue>\n#include <unordered_map>\n#include <string>\n\nclass Solution {\npublic:\n std::string repeatLimitedString(std::string s, int limit) {\n std::unordered_map<char, int> freq;\n for (char c : s) freq[c]++;\n \n // Max-heap to store characters in decreasing order\n std::priority_queue<char> pq;\n for (auto& it : freq) pq.push(it.first);\n \n std::string result;\n \n while (!pq.empty()) {\n char curr = pq.top();\n pq.pop();\n int count = freq[curr];\n \n // If count <= limit, append all occurrences\n if (count <= limit) {\n result.append(count, curr);\n freq.erase(curr);\n }\n // If count > limit\n else {\n result.append(limit, curr);\n freq[curr] -= limit;\n\n if (pq.empty()) break; // Can\'t break repetition if no other character exists\n \n // Append the next largest character to break repetition\n char next = pq.top();\n pq.pop();\n result.push_back(next);\n freq[next]--;\n \n // Return characters with remaining occurrences back to the heap\n if (freq[next] > 0) pq.push(next);\n pq.push(curr);\n }\n }\n \n return result;\n }\n};\n```\n``` Python3 []\nimport heapq\nfrom collections import Counter\n\nclass Solution:\n def repeatLimitedString(self, s: str, limit: int) -> str:\n freq = Counter(s)\n # Max-heap (use negative values to simulate a max-heap)\n pq = [-ord(char) for char in freq]\n heapq.heapify(pq)\n \n result = []\n \n while pq:\n curr = -heapq.heappop(pq)\n char = chr(curr)\n count = freq[char]\n \n if count <= limit:\n result.append(char * count)\n del freq[char]\n else:\n result.append(char * limit)\n freq[char] -= limit\n \n if not pq:\n break # Can\'t break repetition if no other character exists\n \n # Append the next largest character to break repetition\n next_char = chr(-heapq.heappop(pq))\n result.append(next_char)\n freq[next_char] -= 1\n \n # Return characters with remaining occurrences back to the heap\n if freq[next_char] > 0:\n heapq.heappush(pq, -ord(next_char))\n heapq.heappush(pq, -ord(char))\n \n return "".join(result)\n``` | 5 | 0 | ['String', 'Heap (Priority Queue)', 'C++', 'Java', 'Python3'] | 0 |
construct-string-with-repeat-limit | โ
Simple Solution | Beginner Friendly | Easy Approach with Explanationโ
| simple-solution-beginner-friendly-easy-a-1o4f | IntuitionThe goal is to construct the largest lexicographical string such that no character appears more than repeatLimit times consecutively.To achieve this, w | Jithinp96 | NORMAL | 2024-12-17T08:01:12.720079+00:00 | 2024-12-17T08:01:12.720079+00:00 | 488 | false | # Intuition\nThe goal is to construct the largest lexicographical string such that no character appears more than repeatLimit times consecutively. \n\nTo achieve this, we can:\n1. Use a frequency count of each character in the string.\n2. Start from the largest character (lexicographically) and add it to the result as many times as allowed.\n3. If a character exceeds the limit, we add the next largest character to "break the streak."\n\n# Approach\n1. *Count frequencies* of all characters using a frequency array (size 26 for lowercase letters).\n2. Start iterating from the largest character (z -> a) using two pointers: \n - i for the current largest character.\n - j for the next largest character (to use when the limit is exceeded).\n3. For each character:\n - Add it to the result min(repeatLimit, frequency) times.\n - If there are still characters left, find the next available character (j) and add it once.\n4. Repeat until we process all characters or cannot add any more.\n\n\n# Code\n```javascript []\n/**\n * @param {string} s\n * @param {number} repeatLimit\n * @return {string}\n */\nvar repeatLimitedString = function(s, repeatLimit) {\n const freq = Array(26).fill(0);\n for (const char of s) {\n freq[char.charCodeAt(0) - \'a\'.charCodeAt(0)]++;\n }\n\n let result = [];\n let i = 25;\n let j = 24;\n\n while (i >= 0) {\n if (freq[i] === 0) {\n i--;\n continue;\n }\n\n let count = Math.min(repeatLimit, freq[i]);\n result.push(String.fromCharCode(97 + i).repeat(count));\n freq[i] -= count;\n\n if (freq[i] > 0) {\n while (j >= 0 && (freq[j] === 0 || j === i)) {\n j--;\n }\n\n if (j < 0) break;\n\n result.push(String.fromCharCode(97 + j));\n freq[j]--;\n } else {\n i--;\n }\n }\n\n return result.join(\'\');\n};\n```\n\n``` typescript []\nfunction repeatLimitedString(s: string, repeatLimit: number): string {\n const freq: number[] = new Array(26).fill(0);\n for (const char of s) {\n freq[char.charCodeAt(0) - \'a\'.charCodeAt(0)]++;\n }\n\n let result: string[] = [];\n let i: number = 25;\n let j: number = 24;\n\n while (i >= 0) {\n if (freq[i] === 0) {\n i--;\n continue;\n }\n\n let count: number = Math.min(repeatLimit, freq[i]);\n result.push(String.fromCharCode(97 + i).repeat(count));\n freq[i] -= count;\n\n if (freq[i] > 0) {\n while (j >= 0 && (freq[j] === 0 || j === i)) {\n j--;\n }\n\n if (j < 0) break;\n\n result.push(String.fromCharCode(97 + j));\n freq[j]--;\n } else {\n i--;\n }\n }\n\n return result.join(\'\');\n} | 5 | 0 | ['TypeScript', 'JavaScript'] | 0 |
construct-string-with-repeat-limit | Easy Solutionโ
| Tree Mapโ
| O(N*K)โ
| Java Solutionโ
| | easy-solution-tree-map-onk-java-solution-2xv3 | Intuition
The goal is to construct the lexicographically largest string under the condition that no character can repeat more than repeatLimit times consecutive | PRASHANT_KUMAR03 | NORMAL | 2024-12-17T05:19:19.249524+00:00 | 2024-12-17T05:19:19.249524+00:00 | 171 | false | # Intuition\n- The goal is to construct the lexicographically largest string under the condition that no character can repeat more than repeatLimit times consecutively. To achieve this:\n\n1. **Prioritize the largest characters first to make the string lexicographically larger.**\n1. **Ensure that a character is appended at most repeatLimit times consecutively. If the character appears more frequently, we must "interrupt" it with the next largest character.**\n1. **Repeat this process until all characters are used or no valid interruption is possible.**\n\n# Approach\n1. **Count Frequencies in Reverse Lexicographic Order:**\n\n- Use a TreeMap with Collections.reverseOrder() to store characters in descending order. This ensures that the largest characters are processed first.\nPopulate the TreeMap with the frequency of each character.\n2. **Prepare a List of Characters:**\n\n- Extract the keys (characters) of the TreeMap into a List. This allows us to iterate through the largest characters easily.\n3. **Construct the Result String:**\n\n- Use a StringBuilder to construct the result string efficiently.\nProcess the largest character (ch) \n- - first:\nIf its frequency (val) is greater than or equal to repeatLimit, append it repeatLimit times.\nReduce its count by repeatLimit. If the count becomes zero, remove it from the map and list.\n- - - If the count is not zero, "interrupt" the sequence by appending the next largest character (ch2) from the list.\nDecrement the frequency of ch2. If its count becomes zero, remove it from the map and list.\n- - - If no second character (ch2) is available, break the loop as no valid interruption can occur.\n4. **Edge Case for Frequency < RepeatLimit:**\n\n- If the frequency of a character is less than repeatLimit, append the character as many times as its frequency and remove it from the map and list.\n5. **Return Result:**\n\n- Finally, return the string constructed using the StringBuilder.\n\n# Complexity\n- Time complexity: **O(N*K)**\n\n- Space complexity: **O(N+K)**\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```java []\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n Map<Character,Integer> map=new TreeMap<>(Collections.reverseOrder());\n for(int i=0;i<s.length();i++){\n map.put(s.charAt(i),map.getOrDefault(s.charAt(i),0)+1);\n }\n List<Character> list=new ArrayList<>();\n for(Character i : map.keySet()){\n list.add(i);\n }\n StringBuilder stb=new StringBuilder();\n while(!list.isEmpty()){\n char ch = list.get(0);\n int val=map.get(ch);\n if(val>=repeatLimit){\n for(int i=0;i<repeatLimit;i++){\n stb.append(ch);\n }\n map.put(ch,val-repeatLimit);\n if(map.get(ch)==0){\n map.remove(ch);\n list.remove(0);\n }\n else{\n if(list.size()>1){\n char ch2=list.get(1);\n stb.append(ch2);\n map.put(ch2,map.get(ch2)-1);\n if(map.get(ch2)==0){\n map.remove(ch2);\n list.remove(1);\n }\n }\n else{\n break;\n }\n }\n }\n else{\n for(int i=0;i<val;i++){\n stb.append(ch);\n }\n map.remove(ch);\n list.remove(0);\n }\n }\n return stb.toString();\n }\n}\n``` | 5 | 0 | ['Java'] | 0 |
construct-string-with-repeat-limit | Python 3 | Priority queue | Easy understanding with comment | python-3-priority-queue-easy-understandi-46h9 | Approach\n Describe your approach to solving the problem. \nFirst we store all the characters in a heap.\nThen we pop them one by one to add them into the answe | PinkGlove | NORMAL | 2024-01-08T21:39:39.339790+00:00 | 2024-01-08T21:39:39.339824+00:00 | 276 | false | # Approach\n<!-- Describe your approach to solving the problem. -->\nFirst we store all the characters in a heap.\nThen we pop them one by one to add them into the answer.\nIf the count of the current character is smaller than limit, add it to the answer directly.\nIf the count of the current character is larger than limit, we add it to the answer with count limit. Then we can add one single next character to maximize the string. So:\n-- if there\'s nothing in the heap anymore, then we have the answer since we can\'t add anymore characters to the answer.\n-- if there\'s still characters in the heap, then we can use one of the maximum character to seperate current character.\n\n# Code\n```\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str: \n # Python only have min heap\n heap = [(-ord(key), value) for key, value in Counter(s).items()]\n heapq.heapify(heap)\n ans = ""\n\n while heap:\n # The current character\n k, v = heapq.heappop(heap)\n if v <= repeatLimit:\n # Add all this character\n ans += chr(-k) * v\n else:\n # Only add limit numbers of character\n ans += chr(-k) * repeatLimit\n # If nothing in the heap (no next character), then we have the answer\n if not heap:\n break\n # The next character\n nk, nv = heapq.heappop(heap)\n # Add one to the answer\n ans += chr(-nk)\n # If there\'s still next character remaining, add it back to the heap\n if nv > 1:\n heapq.heappush(heap, (nk, nv - 1))\n # Add the remaining current character back to the heap\n heapq.heappush(heap, (k, v - repeatLimit))\n return ans\n``` | 5 | 0 | ['String', 'Heap (Priority Queue)', 'Counting', 'Python3'] | 2 |
construct-string-with-repeat-limit | Java solution-->(Classic implementation of Map+PriorityQueue) | java-solution-classic-implementation-of-uav91 | In the priority queue sorting part, Character.compare(b,a) can be replaced by return b-a; which reduces the runtime \n\n\nclass pair{\n Character ele;\n i | Ritabrata_1080 | NORMAL | 2022-09-20T09:22:38.662990+00:00 | 2022-09-20T09:36:08.647120+00:00 | 494 | false | # In the priority queue sorting part, Character.compare(b,a) can be replaced by return b-a; which reduces the runtime \n\n```\nclass pair{\n Character ele;\n int freq;\n pair(Character ele, int freq){\n this.ele = ele;\n this.freq = freq;\n }\n}\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n PriorityQueue<pair> pq = new PriorityQueue<>((a,b) -> {\n return Character.compare(b.ele, a.ele);\n });\n Map<Character, Integer> map = new HashMap<>();\n for(char c : s.toCharArray()){\n map.put(c, map.getOrDefault(c,0)+1);\n }\n StringBuilder sb = new StringBuilder();\n for(Map.Entry<Character, Integer> entry : map.entrySet()){\n pair p = new pair(entry.getKey(), entry.getValue());\n pq.offer(p);\n }\n \n while(!pq.isEmpty()){\n pair LexicographicallyLargest = pq.poll();\n int frequency = LexicographicallyLargest.freq;\n for(int i = 0;i<repeatLimit;i++){\n sb.append(LexicographicallyLargest.ele);\n LexicographicallyLargest.freq--;\n if(LexicographicallyLargest.freq == 0){\n break;\n }\n }\n if(LexicographicallyLargest.freq > 0){\n if(!pq.isEmpty()){\n pair LexicographicallySecondLargest = pq.poll();\n sb.append(LexicographicallySecondLargest.ele);\n LexicographicallySecondLargest.freq--;\n if(LexicographicallySecondLargest.freq != 0){\n pq.offer(LexicographicallySecondLargest);\n }\n pq.offer(LexicographicallyLargest);\n }\n }\n }\n return sb.toString();\n }\n}\n``` | 5 | 0 | ['Heap (Priority Queue)', 'Java'] | 0 |
construct-string-with-repeat-limit | 3 Liner Python Solution | 3-liner-python-solution-by-subin_nair-kpxe | \n def repeatLimitedString(self, s: str, k: int) -> str:\n leters , sol, stack, rep, prev , ll = (x for x in sorted(s, reverse=True)) , [], [], 0, Non | subin_nair | NORMAL | 2022-02-20T04:24:20.080858+00:00 | 2022-02-20T16:59:48.131520+00:00 | 190 | false | ```\n def repeatLimitedString(self, s: str, k: int) -> str:\n leters , sol, stack, rep, prev , ll = (x for x in sorted(s, reverse=True)) , [], [], 0, None ,len(s)\n while ele:=stack.pop() if rep <= k and stack else next(leters, 0):_,prev = sol.append(ele) if (rep:=1 if ele != prev else rep+1) <= k else stack.append(ele) , ele\n return "".join(sol)\n```\n\t\n If you have any questions, feel free to ask.\n \n Happy Coding !! | 5 | 4 | ['Stack', 'Python'] | 1 |
construct-string-with-repeat-limit | Fastest Python3 Solution! ๐ฏ | fastest-python3-solution-by-rinsane-3j5u | Complexity
Time complexity: O(nโlog(n))
Space complexity: O(1) (only need to store the characters that are atmost 26)
Code | rinsane | NORMAL | 2024-12-18T05:30:11.159976+00:00 | 2024-12-18T05:30:11.159976+00:00 | 69 | false | # Complexity\n- Time complexity: $$O(n*log(n))$$\n- Space complexity: $$O(1)$$ (only need to store the characters that are atmost 26)\n\n# Code\n```python3 []\nclass Solution:\n def repeatLimitedString(self, s: str, rl: int) -> str:\n heapq.heapify(chars:= list(map(lambda x: [-ord(x[0]), x[1]], Counter(s).items())))\n ans = ""\n while chars:\n c, f = heapq.heappop(chars)\n c = chr(abs(c))\n cf, ff = None, 0\n ans += c * min(rl, f)\n f -= rl\n while f > 0:\n if ff == 0:\n if chars:\n cf, ff = heapq.heappop(chars)\n cf = chr(abs(cf))\n else: break\n ans += cf + c*min(rl, f)\n f -= rl\n ff -= 1\n if ff:\n heapq.heappush(chars, [-ord(cf), ff])\n\n return ans\n\n``` | 4 | 0 | ['Python3'] | 2 |
construct-string-with-repeat-limit | C++ Solution || 2 Approaches || Detailed Explanation | c-solution-2-approaches-detailed-explana-lsom | IntuitionThe key observation is that to make the string lexicographically largest, we need to use the highest possible characters first, as long as we respect t | Rohit_Raj01 | NORMAL | 2024-12-17T12:07:23.031732+00:00 | 2024-12-17T12:07:23.031732+00:00 | 159 | false | # Intuition\nThe key observation is that to make the string lexicographically largest, we need to use the highest possible characters first, as long as we respect the `repeatLimit` constraint. Once we hit the `repeatLimit`, we must insert the next lexicographically smaller character before continuing.\n\n# Approach 1: Using Priority Queue\n## Approach\n1. **Count Character Frequencies**: Use a frequency array to count occurrences of each character in the string.\n2. **Priority Queue**: Insert characters into a max priority queue so that the lexicographically largest character is processed first.\n3. **Build the Result**:\n - Append the current largest character up to `repeatLimit` times.\n - If further occurrences of the current character remain, temporarily insert the next lexicographically smaller character to "break" the limit.\n - Push back the remaining characters into the priority queue for future processing.\n4. Repeat until all characters are processed.\n\n# Complexity\n- Time complexity: $$O(n + 26log(26))$$\n - Counting frequencies takes $$\uD835\uDC42(\uD835\uDC5B)$$ time.\n - Each push and pop operation in the priority queue costs $$O(log26)$$, and we process at most 26 distinct characters.\n\n- Space complexity: $$O(26)$$\n\n# Code\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n vector<int> count(26, 0);\n for (char& ch : s) {\n count[ch - \'a\']++;\n }\n\n priority_queue<char> pq;\n for (int i = 0; i < 26; i++) {\n if (count[i] > 0) {\n pq.push(\'a\' + i);\n }\n }\n\n string res;\n while (!pq.empty()) {\n char ch = pq.top();\n pq.pop();\n int freq = min(count[ch - \'a\'], repeatLimit);\n res.append(freq, ch);\n count[ch - \'a\'] -= freq;\n\n if (count[ch - \'a\'] > 0 && !pq.empty()) {\n int nextchar = pq.top();\n pq.pop();\n res.push_back(nextchar);\n count[nextchar - \'a\']--;\n if (count[nextchar - \'a\'] > 0) {\n pq.push(nextchar);\n }\n\n if (count[ch - \'a\'] > 0) {\n pq.push(ch);\n }\n }\n }\n\n return res;\n }\n};\n```\n\n\n# Approach 2: Using Frequency Array and Greedy\n## Approach\n1. **Count Character Frequencies**: Store the frequency of each character using a frequency array.\n2. **Iterate in Reverse**: Traverse from the largest character (\'z\') to the smallest (\'a\').\n3. **Build the Result**:\n - Add the current character up to `repeatLimit` times.\n - If further occurrences remain, find the next lexicographically smaller character and append it to "break" the repetition limit.\n4. Repeat the process until all characters are exhausted.\n\n# Complexity\n- Time complexity: $$O(n + 26)$$\n - Counting frequencies takes $$\uD835\uDC42(\uD835\uDC5B)$$ time.\n - Processing all 26 characters in reverse order takes $$O(26)$$ time.\n\n- Space complexity: $$O(26)$$\n\n# Code\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n vector<int> count(26, 0);\n for(char &ch : s) {\n count[ch-\'a\']++;\n }\n\n string result;\n\n int i = 25; \n while(i >= 0) { \n if(count[i] == 0) {\n i--;\n continue;\n }\n\n char ch = \'a\' + i; \n int freq = min(count[i], repeatLimit);\n\n result.append(freq, ch);\n count[i] -= freq;\n\n if(count[i] > 0) {\n int j = i-1;\n while(j >= 0 && count[j] == 0) {\n j--;\n }\n\n if(j < 0) {\n break;\n }\n\n result.push_back(\'a\' + j);\n count[j]--;\n }\n }\n\n return result;\n }\n};\n\n```\n\n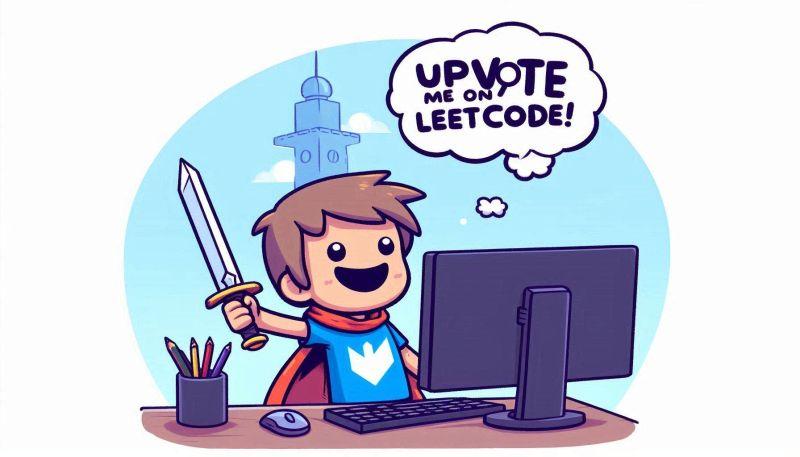\n | 4 | 0 | ['Hash Table', 'String', 'Heap (Priority Queue)', 'Counting', 'C++'] | 0 |
construct-string-with-repeat-limit | Easyyy Pessyyy Solution โ
| Beats 80% Runtime ๐ฅ | 100% Memory ๐ฅ | easyyy-pessyyy-solution-beats-80-runtime-x1ip | Intuition
The goal is to build the lexicographically largest string while ensuring no character appears more than repeatLimit times consecutively.
Count Charact | jaitaneja333 | NORMAL | 2024-12-17T05:34:31.115380+00:00 | 2024-12-17T05:34:31.115380+00:00 | 397 | false | \n# Intuition\n* The goal is to build the lexicographically largest string while ensuring no character appears more than repeatLimit times consecutively.\n\n### Count Character Frequency:\nUse a frequency array to count occurrences of each character.\n\n### Max-Heap for Character Priority:\nStore characters in a max-heap (priority queue) based on their lexicographical order and frequency. The heap ensures the largest character is processed first.\n\n### Construct the String:\n\n* Append the largest character up to repeatLimit times.\n* If it still has remaining occurrences, temporarily switch to the next largest character to break the consecutive limit.\n* Push both characters back into the heap if they have remaining occurrences.\n### Repeat until no valid characters remain.\n\n# Approach\n1. Count Frequency:\nUse an array of size 26 to count the frequency of each character.\n\n2. Max-Heap Initialization:\nInsert all characters (with positive frequency) into a max-heap. The heap will sort characters in descending lexicographical order based on their index.\n\n3. String Construction:\n* Use the largest character as much as allowed (repeatLimit) before switching to the next largest character.\n* Reinsert characters into the heap if they still have a remaining count after appending.\n\n# Complexity\n### Time complexity:\n##### Frequency Count:\nCounting the frequency of characters in the string takes O(n), where n is the size of the input string s.\n\n##### Heap Operations:\n\n* Insertion into the heap: There are at most 26 characters (O(26) operations), so this is constant in practice.\n* Processing characters: \n * Each character is pushed into and popped from the heap at most once per frequency.\n * The cost of push and pop operations in a priority queue is O(log k), where k is the number of distinct characters (at most 26).\n * In the worst case, the heap operations take O(n log k), where n is the total number of characters and k = 26.\n##### String Appending:\nAppending to the result string takes O(n) in total because the resulting string will have n characters.\n### Overall Time Complexity: `O(n+nlogk)=O(nlogk)`\n* Since k (number of distinct characters) is a small constant (26 for lowercase English letters), the complexity simplifies to O(n).\n\n### Space complexity:\n##### Frequency Array:\nThe vector<int> freq_vector uses O(26) = O(1) space.\n\n##### Priority Queue:\nThe priority queue can store up to k = 26 elements, so it uses O(k) = O(1) space.\n\n##### Result String:\nThe result string has a length of at most n, so it takes O(n) space.\n\nOverall Space Complexity: `O(n)`\n\n\n\n# Code\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n vector<int> freq_vector(26, 0);\n for (int i = 0; i < s.size(); i++) {\n freq_vector[s[i] - \'a\']++;\n }\n priority_queue<pair<int,int>> pq;\n for(int i = 0; i < 26; i++){\n if(freq_vector[i] > 0){\n pq.push(make_pair(i,freq_vector[i]));\n }\n }\n string result;\n while(!pq.empty()){\n pair<int,int> curr = pq.top();\n pq.pop();\n\n char curr_char = curr.first + \'a\' ;\n int count = min(curr.second,repeatLimit);\n curr.second -= count;\n result.append(count,curr_char);\n\n if(curr.second > 0){\n if(pq.empty()) break;\n\n pair<int,int> next = pq.top();\n pq.pop();\n\n char next_char = next.first + \'a\';\n result.push_back(next_char);\n next.second--;\n\n if(next.second > 0){\n pq.push(next);\n }\n pq.push(curr);\n }\n }\n return result;\n }\n};\n```\n\n``` Java [Java]\nimport java.util.PriorityQueue;\n\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int[] freq = new int[26];\n for (char c : s.toCharArray()) {\n freq[c - \'a\']++;\n }\n\n PriorityQueue<int[]> pq = new PriorityQueue<>((a, b) -> b[0] - a[0]); // Max-heap\n for (int i = 0; i < 26; i++) {\n if (freq[i] > 0) {\n pq.offer(new int[]{i, freq[i]}); \n }\n }\n\n StringBuilder result = new StringBuilder();\n\n while (!pq.isEmpty()) {\n int[] curr = pq.poll();\n char currChar = (char) (\'a\' + curr[0]);\n int count = Math.min(curr[1], repeatLimit);\n curr[1] -= count;\n\n for (int i = 0; i < count; i++) {\n result.append(currChar);\n }\n\n if (curr[1] > 0) {\n if (pq.isEmpty()) break;\n\n int[] next = pq.poll();\n char nextChar = (char) (\'a\' + next[0]);\n result.append(nextChar); \n next[1]--;\n\n if (next[1] > 0) {\n pq.offer(next);\n }\n pq.offer(curr);\n }\n }\n\n return result.toString();\n }\n}\n```\n\n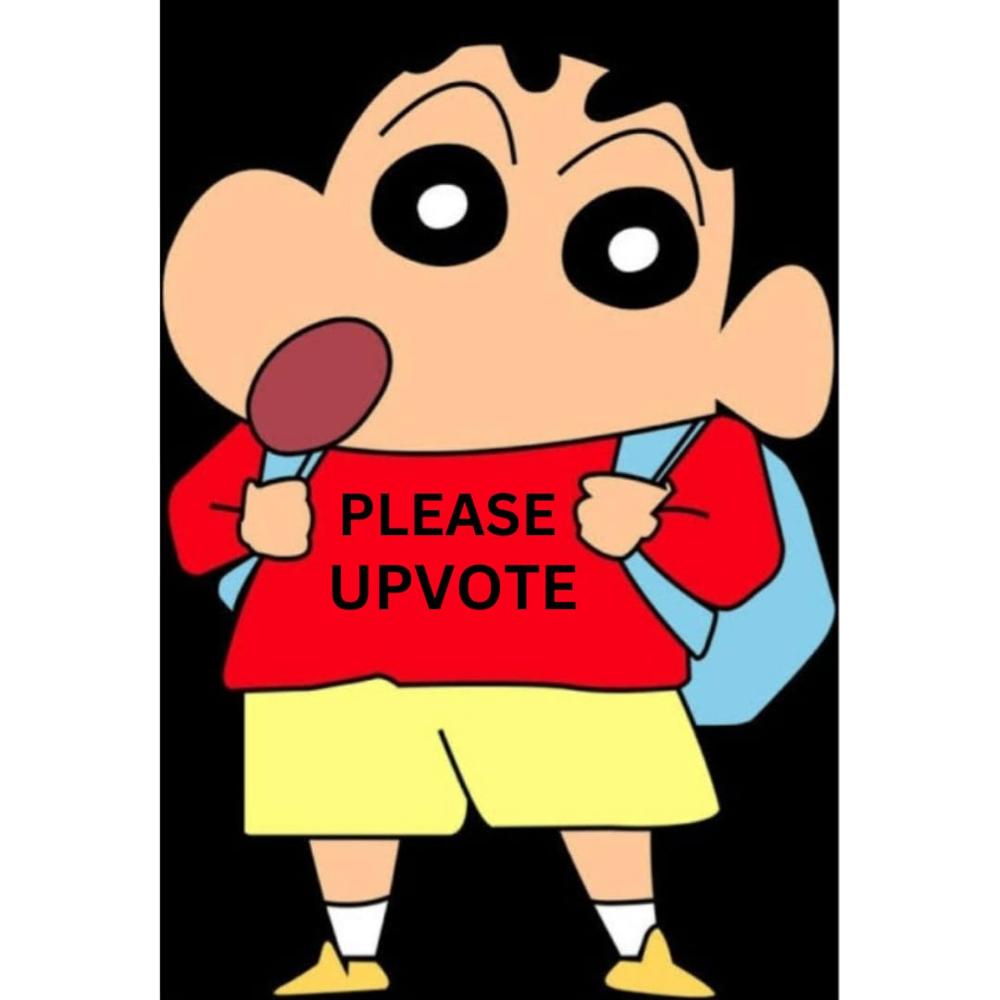\n | 4 | 0 | ['Array', 'String', 'Greedy', 'Heap (Priority Queue)', 'Counting', 'C++', 'Java'] | 1 |
construct-string-with-repeat-limit | Easy Solution in C++. | easy-solution-in-c-by-dhanu07-lu9a | Intuitionthe problem of constructing the lexicographically largest string from the characters of s while adhering to the repeatLimit, we can use a greedy approa | Dhanu07 | NORMAL | 2024-12-17T05:05:05.086672+00:00 | 2024-12-17T05:12:32.261095+00:00 | 468 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nthe problem of constructing the lexicographically largest string from the characters of s while adhering to the repeatLimit, we can use a greedy approach combined with a max-heap (priority queue). The idea is to always try to add the largest available character to the result string, while ensuring that we do not exceed the repeatLimit for consecutive characters.\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Count Character Frequencies: First, we need to count the frequency of each character in the string s. This can be done using an array of size 26 (for each letter of the alphabet).\n\n2. Use a Max-Heap: We will use a max-heap to store the characters based on their lexicographical order. The heap will allow us to efficiently retrieve the largest character available.\n\n3. Construct the Result String:\n\nWhile there are characters left in the heap, we will try to add the largest character to the result string.\nIf the largest character can be added without exceeding the repeatLimit, we add it directly.\nIf adding it would exceed the repeatLimit, we add it as many times as allowed (up to repeatLimit), and then we need to add the next largest character (if available) to break the sequence.\nAfter adding the next character, we can then add the remaining instances of the original character back to the heap if any are left.\n4. Return the Result: After constructing the string, we return it.\n\n## Explanation of the Code:\n1. Frequency Count: We count the occurrences of each character in the input string s.\n\n2. Max-Heap Initialization: We populate a max-heap with characters and their frequencies, ensuring that the largest characters are prioritized.\n\n3. Building the Result: We construct the result string by repeatedly adding the largest character while respecting the repeatLimit. If we need to break a sequence of the same character, we temporarily use the next largest character.\n\n4. Final Output: The constructed string is returned as the result.\n\n# Complexity\n- Time Complexity: The time complexity is (O(n + k \\log k)), where (n) is the length of the string and (k) is the number of unique characters (at most 26). The (O(n)) is for counting frequencies, and (O(k \\log k)) is for managing the heap operations.\n\n- Space Complexity: The space complexity is (O(1\n\n\n\n\n\n\n# Code\n```cpp []\n#include <iostream>\n#include <string>\n#include <vector>\n#include <queue>\n\nusing namespace std;\n\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n // Step 1: Count frequencies of each character\n vector<int> freq(26, 0);\n for (char c : s) {\n freq[c - \'a\']++;\n }\n\n // Step 2: Create a max-heap based on character\n priority_queue<pair<char, int>> maxHeap;\n for (char c = \'z\'; c >= \'a\'; c--) {\n if (freq[c - \'a\'] > 0) {\n maxHeap.push({c, freq[c - \'a\']});\n }\n }\n\n string result;\n \n // Step 3: Construct the result string\n while (!maxHeap.empty()) {\n auto [char1, count1] = maxHeap.top();\n maxHeap.pop();\n\n // Determine how many times we can add char1\n int timesToAdd = min(count1, repeatLimit);\n result.append(timesToAdd, char1);\n count1 -= timesToAdd;\n\n // If we still have more of char1 left, we need to add a different character\n if (count1 > 0) {\n if (maxHeap.empty()) {\n break; // No more characters to use\n }\n\n // Get the next largest character\n auto [char2, count2] = maxHeap.top();\n maxHeap.pop();\n\n // Add one instance of char2\n result += char2;\n count2--;\n\n // If char2 still has remaining instances, push it back to the heap\n if (count2 > 0) {\n maxHeap.push({char2, count2});\n }\n\n // Push char1 back to the heap with the remaining count\n maxHeap.push({char1, count1});\n }\n }\n\n return result;\n }\n};\n\n``` | 4 | 0 | ['Hash Table', 'String', 'Heap (Priority Queue)', 'Counting', 'C++'] | 4 |
construct-string-with-repeat-limit | faster than 80% of user's runtime | faster-than-80-of-users-runtime-by-dev_b-7cgw | Code | dev_bhatt202 | NORMAL | 2024-12-17T04:18:04.162255+00:00 | 2024-12-17T04:18:04.162255+00:00 | 117 | false | \n\n# Code\n```java []\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int[] freq = new int[26];\n\n for (char c : s.toCharArray())\n freq[c - \'a\']++;\n\n int pendingLetterIndex = -1;\n StringBuilder sb = new StringBuilder();\n\n for (int i = 25; i >= 0; i--) {\n if (freq[i] == 0)\n continue;\n\n if (pendingLetterIndex > 0) {\n sb.append((char) (\'a\' + i));\n freq[i]--;\n i = pendingLetterIndex;\n pendingLetterIndex = -1;\n\n } else {\n for (int j = 0; j < repeatLimit && freq[i] > 0; j++, freq[i]--)\n sb.append((char) (\'a\' + i));\n\n if (freq[i] > 0)\n pendingLetterIndex = i + 1;\n }\n }\n \n return sb.toString();\n }\n}\n``` | 4 | 0 | ['Hash Table', 'String', 'Greedy', 'Heap (Priority Queue)', 'Counting', 'Java'] | 1 |
construct-string-with-repeat-limit | max heap || easy to understand || must see | max-heap-easy-to-understand-must-see-by-fcc02 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | akshat0610 | NORMAL | 2023-03-10T10:03:43.276628+00:00 | 2023-03-10T10:03:43.276668+00:00 | 368 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n//for fast io\nstatic int fast_io = []() \n{ \n std::ios::sync_with_stdio(false); \n cin.tie(nullptr); \n cout.tie(nullptr); \n return 0; \n}();\n\n#ifdef LOCAL\n freopen("input.txt", "r" , stdin);\n freopen("output.txt", "w", stdout);\n#endif\n\n//actual code begins here\nclass Solution {\npublic:\n string repeatLimitedString(string s, int k) \n {\n //final constructed string to be returned from this function\n string ans = "";\n\n //first of all we will take the mapping of the char from string\n unordered_map<char,int>mp;\n\n //taking the mapping of the every char to keep that in heap\n for(int i=0;i<s.length();i++)\n {\n char ch = s[i];\n mp[ch]++;\n }\n\n //priority queue for keeping the large char on the top\n priority_queue<pair<char,int>>pq;\n\n for(auto it = mp.begin() ; it!=mp.end() ; it++)\n {\n char ch = it->first;\n int freq = it->second; \n pq.push(make_pair(ch,freq));\n }\n //building the string according to the given constraint\n while(!pq.empty())\n {\n pair<char,int>p1 = pq.top();\n pq.pop();\n char ele1 = p1.first;\n int freq1 = p1.second;\n\n if(freq1 < k)\n {\n //appending ch1 freq1 times to the current answer\n while(freq1 > 0)\n {\n ans.push_back(ele1);\n freq1--;\n }\n }\n else if(freq1 == k)\n {\n //appending ch1 freq1 times to the current answer\n while(freq1 > 0)\n {\n ans.push_back(ele1);\n freq1--;\n }\n }\n else if(freq1 > k)\n {\n //appending ch1 freq1 times to the current answer\n int temp = k;\n while(temp > 0)\n {\n ans.push_back(ele1);\n temp--;\n }\n freq1 = freq1 - k;\n \n //breaking the streak of the k characters\n \n //if we do not have any one to break the freq of the curr char then break\n if(pq.size() == 0)\n break;\n\n //else we have someone that can break the freq of the curr char\n pair<char,int>p2 = pq.top();\n pq.pop();\n char ele2 = p2.first;\n int freq2 = p2.second;\n \n if(freq2 > 0)\n {\n ans.push_back(ele2);\n freq2--;\n \n if(freq2 > 0)\n pq.push(make_pair(ele2,freq2));\n }\n if(freq1 > 0)\n pq.push(make_pair(ele1,freq1));\n }\n }\n return ans;\n }\n};\n``` | 4 | 0 | ['String', 'Greedy', 'Heap (Priority Queue)', 'Counting', 'C++'] | 0 |
construct-string-with-repeat-limit | [C++] Simple C++ Code || No Priority Queue || 87% time || 85% space | c-simple-c-code-no-priority-queue-87-tim-vggb | If you like the implementation then Please help me by increasing my reputation. By clicking the up arrow on the left of my image.\n\nclass Solution {\npublic:\n | _pros_ | NORMAL | 2022-07-31T09:10:13.684238+00:00 | 2022-07-31T09:10:13.684277+00:00 | 580 | false | # **If you like the implementation then Please help me by increasing my reputation. By clicking the up arrow on the left of my image.**\n```\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n vector<int> alphabet(26,0);\n int f = 0, n = s.size();\n string ans = "";\n for(char &ch : s){\n alphabet[ch-\'a\']++;\n //sum++;\n }\n while(f < n)\n {\n int x;\n for(int i = 25; i >= 0; i--)\n {\n if(!alphabet[i]) continue;\n x = i;\n break;\n }\n int mx = min(repeatLimit, alphabet[x]);\n for(int j = 0; j < mx; j++){\n ans += (\'a\'+ x);\n alphabet[x]--;\n f++;\n }\n if(alphabet[x] == 0 || f == n) continue;\n int y = x;\n for(int i = x-1; i >= 0; i--)\n {\n if(!alphabet[i]) continue;\n x = i;\n break;\n }\n if(y == x) return ans;\n ans += (\'a\' + x);\n alphabet[x]--;\n f++;\n }\n return ans;\n }\n};\n``` | 4 | 0 | ['C', 'C++'] | 1 |
construct-string-with-repeat-limit | ๐ฅ๐ฅโ
Simple Implementation using Priority Queue || T.C = O(N) || Clean Code | simple-implementation-using-priority-que-2n33 | \nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n int freq[26] = {0};\n string ans = "";\n\t\t\n f | kirtanprajapati | NORMAL | 2022-02-23T05:29:28.333103+00:00 | 2022-02-23T05:29:28.333142+00:00 | 472 | false | ```\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n int freq[26] = {0};\n string ans = "";\n\t\t\n for(auto c : s){\n freq[c-\'a\']++;\n }\n \n priority_queue<pair<char,int>>pq;\n \n for(int i=0;i<26;i++){\n if(freq[i] > 0){\n pq.emplace((i+\'a\'),freq[i]);\n }\n }\n \n while(!pq.empty()){\n \n auto [key,val] = pq.top();\n pq.pop();\n int count = val;\n \n for(int i=0;i<repeatLimit && count > 0 ; i++){\n ans += key;\n count--;\n }\n \n if(count > 0 && pq.size() > 0){\n auto [secondKey , secondVal] = pq.top();\n pq.pop();\n \n ans += secondKey;\n if(secondVal > 1){\n pq.emplace(secondKey,secondVal-1);\n }\n pq.emplace(key,count);\n } \n } \n return ans;\n }\n};\n\n``` | 4 | 0 | ['C', 'Heap (Priority Queue)'] | 0 |
construct-string-with-repeat-limit | priorityqueue + Longest Happy String | priorityqueue-longest-happy-string-by-al-kako | Found it quite close to Longest Happy String: https://leetcode.com/problems/longest-happy-string/\n\nFor solution and explanation for it, please see this (Thank | alpha_gigachad | NORMAL | 2022-02-20T17:14:15.385183+00:00 | 2022-02-20T17:14:46.219378+00:00 | 395 | false | Found it quite close to `Longest Happy String`: https://leetcode.com/problems/longest-happy-string/\n\nFor solution and explanation for it, please see this (Thanks to @lechen999): https://leetcode.com/problems/longest-happy-string/discuss/564248/Python-HEAP-solution-with-explanation\n\nWe first count each char\'s appearing times\nThen use max heap to implement `Longest Happy String`.\nHowever, please pay attention to "the actual appearing time in stack", which we\'ll also need a map to record. Whenever found the prior char `x` exceeds the `repeatLimit` (I simplified it as `k`), we heappop a less appearing char `y` and append it to stack, and `times[y]` should be 1, and clear the `times[x]` because we have a new "stack top". The same for `x` not appearing exceeds `k`. \n\nHope it helps:\n```\nfrom heapq import heapify, heappush, heappop\nfrom collections import defaultdict\n\nclass Solution:\n def repeatLimitedString(self, string: str, k: int) -> str:\n """\n look close to the problem:\n it\'s lexicographically largest \n not "longest"\n """\n appear, times = defaultdict(), defaultdict()\n pq, stack = [], []\n for s in string:\n appear[s] = appear.get(s, 0) + 1\n \n for s in appear:\n pq.append((-ord(s), appear[s]))\n \n heapify(pq)\n appear.clear()\n \n while pq:\n char, num = heappop(pq)\n s = chr(-char)\n if s in times and times[s] == k: # if reach the repeatedLimit\n if not pq:\n return \'\'.join(stack)\n char2, num2 = heappop(pq)\n token = chr(-char2)\n stack.append(token)\n if num2 - 1 > 0:\n heappush(pq, (char2, num2 - 1))\n heappush(pq, (char, num))\n del times[s]\n times[token] = 1\n continue\n if stack and stack[-1] != s:\n # reset times\n del times[stack[-1]]\n stack.append(s)\n times[s] = times.get(s, 0) + 1\n \n if num - 1 > 0:\n heappush(pq, (char, num - 1))\n return \'\'.join(stack) \n``` | 4 | 0 | ['Heap (Priority Queue)', 'Python'] | 0 |
construct-string-with-repeat-limit | Greedy + Priority Queue || Easy solution C++ | greedy-priority-queue-easy-solution-c-by-fzze | \nclass Solution {\npublic:\n string repeatLimitedString(string s, int k) \n {\n int n=s.size(),i;\n priority_queue <pair<char,int>> q;\n | Roar47 | NORMAL | 2022-02-20T04:03:30.415348+00:00 | 2022-02-20T04:03:30.415376+00:00 | 314 | false | ```\nclass Solution {\npublic:\n string repeatLimitedString(string s, int k) \n {\n int n=s.size(),i;\n priority_queue <pair<char,int>> q;\n vector <int> a(26);\n for(auto x : s)\n {\n a[x-\'a\']++;\n }\n for(i=0;i<26;i++)\n {\n if(a[i]>0)\n {\n q.push({i+\'a\',a[i]});\n }\n }\n string ans="";\n while(!q.empty())\n {\n auto f=q.top();\n q.pop();\n if(f.second>k)\n {\n for(i=0;i<k;i++)\n {\n ans.push_back(f.first);\n }\n f.second-=k;\n if(!q.empty())\n {\n auto sec=q.top();\n q.pop();\n sec.second--;\n ans.push_back(sec.first);\n if(sec.second>0)\n {\n q.push({sec.first,sec.second});\n }\n }\n else\n {\n break;\n }\n q.push({f.first,f.second});\n }\n else\n {\n for(i=0;i<f.second;i++)\n {\n ans.push_back(f.first);\n }\n }\n }\n return ans;\n }\n};\n``` | 4 | 0 | ['Greedy', 'C', 'Heap (Priority Queue)'] | 0 |
construct-string-with-repeat-limit | ๐ฅBEATS ๐ฏ % ๐ฏ |โจSUPER EASY BEGINNERS ๐ | beats-super-easy-beginners-by-codewithsp-ujsx | IntuitionThe goal is to construct a string using the characters of s such that:
No character appears consecutively more than repeatLimit times.
The resulting st | CodeWithSparsh | NORMAL | 2024-12-17T16:40:31.672200+00:00 | 2024-12-17T16:40:31.672200+00:00 | 214 | false | 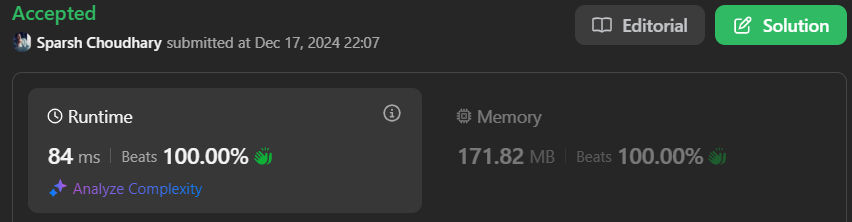\n\n\n---\n\n\n\n\n# Intuition\nThe goal is to construct a string using the characters of `s` such that:\n1. No character appears consecutively more than `repeatLimit` times.\n2. The resulting string is lexicographically largest.\n\nTo achieve this, we can:\n- Count the frequency of each character.\n- Start from the largest character (\'z\') and add it to the result as many times as allowed (up to `repeatLimit`).\n- If we hit the repeat limit but still have more of the current character left, we temporarily insert the next smaller character to "break" the repetition.\n\n---\n\n# Approach\n1. Use a frequency array to count occurrences of each character.\n2. Start from the largest character (\'z\') and try adding it up to `repeatLimit` times.\n3. If the repeat limit for the current character is reached:\n - Find the next largest character with a positive frequency.\n - Add it to the result to "break" the streak of repetitions.\n4. Continue this process until no characters remain.\n\n---\n\n# Complexity\n- **Time Complexity**: \n The time complexity is \\(O(n)\\), where \\(n\\) is the length of the string `s`. \n We process the string once to calculate frequencies and repeatedly check/insert characters based on their frequencies.\n\n- **Space Complexity**: \n \\(O(1)\\) for the frequency array since it always holds 26 entries (constant for lowercase letters).\n\n---\n\n\n```dart []\nimport \'dart:math\';\n\nclass Solution {\n String repeatLimitedString(String s, int repeatLimit) {\n List<int> freq = List.filled(26, 0);\n int a = \'a\'.codeUnitAt(0);\n\n // Step 1: Frequency of each character\n for (var char in s.split(\'\')) {\n freq[char.codeUnitAt(0) - a]++;\n }\n\n StringBuffer result = StringBuffer();\n int index = 25;\n\n // Step 2: Construct result string\n while (index >= 0) {\n if (freq[index] == 0) {\n index--;\n continue;\n }\n\n int repeatCount = min(freq[index], repeatLimit);\n for (int i = 0; i < repeatCount; i++) {\n result.write(String.fromCharCode(index + a));\n }\n\n freq[index] -= repeatCount;\n\n if (freq[index] > 0) {\n int nextIndex = index - 1;\n while (nextIndex >= 0 && freq[nextIndex] == 0) {\n nextIndex--;\n }\n\n if (nextIndex < 0) break;\n\n result.write(String.fromCharCode(nextIndex + a));\n freq[nextIndex]--;\n } else {\n index--;\n }\n }\n\n return result.toString();\n }\n}\n```\n\n\n```python []\nfrom collections import Counter\n\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n freq = [0] * 26\n a_ord = ord(\'a\')\n\n # Step 1: Frequency array\n for char in s:\n freq[ord(char) - a_ord] += 1\n\n res = []\n index = 25\n\n # Step 2: Build result\n while index >= 0:\n if freq[index] == 0:\n index -= 1\n continue\n\n repeat_count = min(freq[index], repeatLimit)\n res.extend(chr(index + a_ord) * repeat_count)\n freq[index] -= repeat_count\n\n if freq[index] > 0:\n next_index = index - 1\n while next_index >= 0 and freq[next_index] == 0:\n next_index -= 1\n\n if next_index < 0:\n break\n\n res.append(chr(next_index + a_ord))\n freq[next_index] -= 1\n else:\n index -= 1\n\n return \'\'.join(res)\n```\n\n\n```cpp []\n#include <vector>\n#include <string>\n#include <algorithm>\nusing namespace std;\n\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n vector<int> freq(26, 0);\n\n // Step 1: Frequency array\n for (char c : s) {\n freq[c - \'a\']++;\n }\n\n string result;\n int index = 25;\n\n // Step 2: Build the result string\n while (index >= 0) {\n if (freq[index] == 0) {\n index--;\n continue;\n }\n\n int repeatCount = min(freq[index], repeatLimit);\n result.append(repeatCount, \'a\' + index);\n freq[index] -= repeatCount;\n\n if (freq[index] > 0) {\n int nextIndex = index - 1;\n while (nextIndex >= 0 && freq[nextIndex] == 0) {\n nextIndex--;\n }\n\n if (nextIndex < 0) break;\n\n result += \'a\' + nextIndex;\n freq[nextIndex]--;\n } else {\n index--;\n }\n }\n\n return result;\n }\n};\n```\n\n\n```java []\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int[] freq = new int[26];\n for (char c : s.toCharArray()) {\n freq[c - \'a\']++;\n }\n\n StringBuilder result = new StringBuilder();\n int index = 25;\n\n while (index >= 0) {\n if (freq[index] == 0) {\n index--;\n continue;\n }\n\n int repeatCount = Math.min(freq[index], repeatLimit);\n for (int i = 0; i < repeatCount; i++) {\n result.append((char) (index + \'a\'));\n }\n freq[index] -= repeatCount;\n\n if (freq[index] > 0) {\n int nextIndex = index - 1;\n while (nextIndex >= 0 && freq[nextIndex] == 0) {\n nextIndex--;\n }\n\n if (nextIndex < 0) break;\n\n result.append((char) (nextIndex + \'a\'));\n freq[nextIndex]--;\n } else {\n index--;\n }\n }\n\n return result.toString();\n }\n}\n```\n\n\n```javascript []\nclass Solution {\n repeatLimitedString(s, repeatLimit) {\n const freq = Array(26).fill(0);\n const aCode = \'a\'.charCodeAt(0);\n\n for (const char of s) {\n freq[char.charCodeAt(0) - aCode]++;\n }\n\n let result = \'\';\n let index = 25;\n\n while (index >= 0) {\n if (freq[index] === 0) {\n index--;\n continue;\n }\n\n const repeatCount = Math.min(freq[index], repeatLimit);\n result += String.fromCharCode(index + aCode).repeat(repeatCount);\n freq[index] -= repeatCount;\n\n if (freq[index] > 0) {\n let nextIndex = index - 1;\n while (nextIndex >= 0 && freq[nextIndex] === 0) {\n nextIndex--;\n }\n\n if (nextIndex < 0) break;\n\n result += String.fromCharCode(nextIndex + aCode);\n freq[nextIndex]--;\n } else {\n index--;\n }\n }\n\n return result;\n }\n}\n```\n\n---\n\n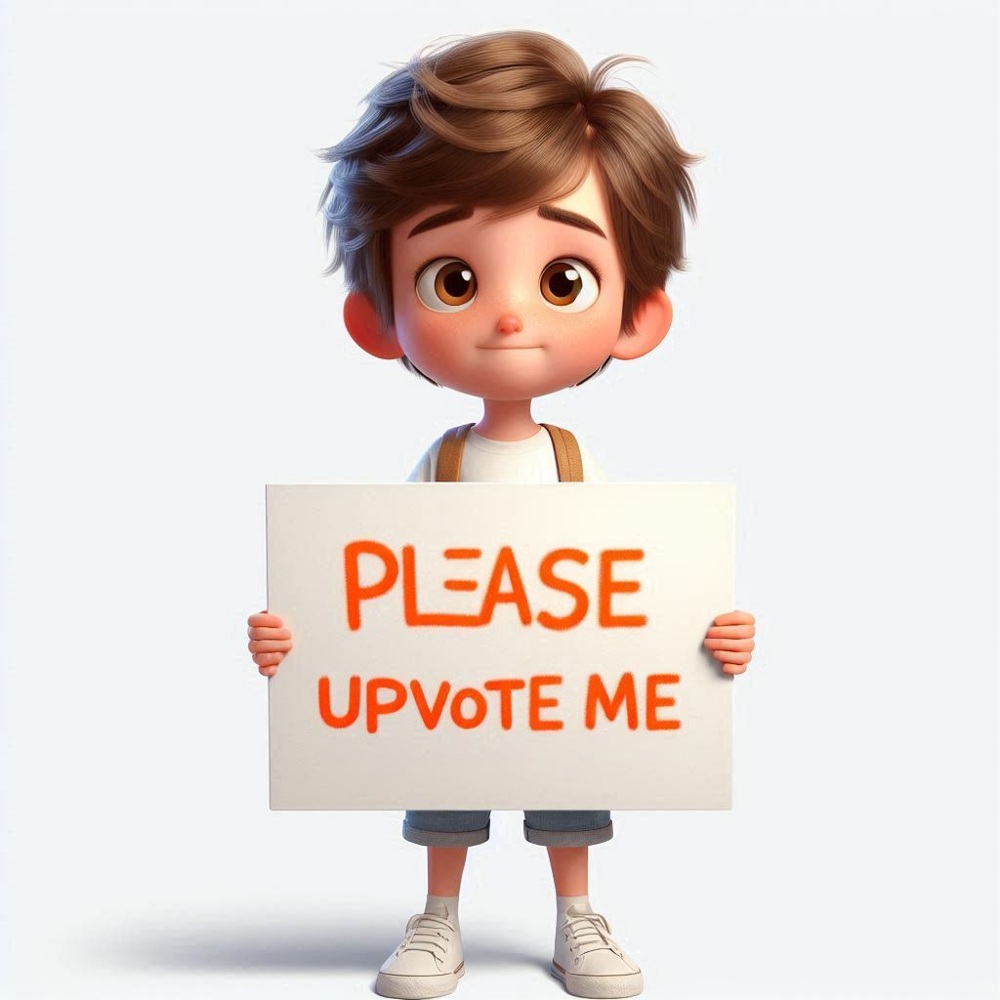 {:style=\'width:250px\'} | 3 | 0 | ['String', 'Greedy', 'C', 'Counting', 'C++', 'Java', 'Go', 'Python3', 'JavaScript', 'Dart'] | 0 |
construct-string-with-repeat-limit | โ
โ
easy and beginner friendly solution ๐ฅ๐ฅ | easy-and-beginner-friendly-solution-by-i-eyuh | Intuition
The goal is to construct the lexicographically largest string such that no character appears more than repeatLimit times consecutively. To achieve thi | ishanbagra | NORMAL | 2024-12-17T10:10:39.110313+00:00 | 2024-12-17T10:10:39.110313+00:00 | 150 | false | Intuition\nThe goal is to construct the lexicographically largest string such that no character appears more than repeatLimit times consecutively. To achieve this, we prioritize the largest characters (lexicographically) and use a greedy approach to add them to the result. If a character reaches the repeatLimit, we temporarily switch to the next largest character to break the consecutive streak.\n\nApproach\nCount Frequencies: Use a frequency array v of size 26 to count occurrences of each character in the input string s.\n\nMax-Heap: Create a max-heap (priority queue) where each element is a pair {char_index, frequency}. Characters with higher lexicographical value will have higher priority because the heap sorts in descending order.\n\nGreedy Character Insertion:\n\nExtract the character with the largest lexicographical value (curr) from the heap.\nAppend it to the result up to repeatLimit times or its remaining frequency, whichever is smaller.\nIf the character still has leftover occurrences, temporarily switch to the next largest character (next) to avoid violating the repeatLimit condition.\nAppend one occurrence of next to the result and push the updated curr and next back into the heap.\nRepeat Until Heap is Empty: Continue this process until the heap is empty or no valid characters can be inserted.\n\nComplexity\nTime Complexity:\n- The time complexity is \nO(nlog26), where \n\uD835\uDC5B\nn is the length of the string s.\n\nConstructing the frequency array takes \nO(n).\nPushing and popping from the heap takes \nO(log26), and since there are only 26 letters, the logarithm becomes constant.\n- Space Complexity:\nO(26)=O(1) because we use a fixed-size frequency array and heap.\n\n\n\n# Code\n```cpp []\n#include <string>\n#include <vector>\n#include <queue>\nusing namespace std;\n\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n vector<int> v(26, 0);\n for (int i = 0; i < s.size(); i++) v[s[i] - \'a\']++;\n\n priority_queue<pair<int, int>> maxheap;\n for (int i = 0; i < 26; i++) \n if (v[i] > 0) maxheap.push({i, v[i]});\n\n string result = "";\n\n while (!maxheap.empty()) {\n auto curr = maxheap.top();\n maxheap.pop();\n\n char curr_char = \'a\' + curr.first;\n int count = min(curr.second, repeatLimit);\n result.append(count, curr_char);\n curr.second -= count;\n\n if (curr.second > 0) {\n if (maxheap.empty()) break;\n\n auto next = maxheap.top();\n maxheap.pop();\n\n char next_char = \'a\' + next.first;\n result.push_back(next_char);\n next.second--;\n\n if (next.second > 0) maxheap.push(next);\n maxheap.push(curr);\n }\n }\n return result;\n }\n};\n\n\n\n\n\n | 3 | 0 | ['Hash Table', 'String', 'Heap (Priority Queue)', 'C++'] | 2 |
construct-string-with-repeat-limit | Two pointer approach (barely passed TCs and surpassed no one :) *fire emojis* | two-pointer-approach-barely-passed-tcs-a-512r | Intuition and ApproachSorted string in lexicographically largest way to bring bigger chars ahead and together,
Two pointers i and j used, i is current index whi | om_kumar_saini | NORMAL | 2024-12-17T09:03:14.846904+00:00 | 2024-12-18T18:29:44.113711+00:00 | 82 | false | # Intuition and Approach\nSorted string in lexicographically largest way to bring bigger chars ahead and together,\nTwo pointers `i` and `j` used, `i` is current index while `j` is index of next different character that is ready to get swapped.\nIdea is to iterate till we cross `repeatLimit` then swap `s[i]` and `s[j]` if there are more characters equal to initial `s[i]`.\n\nAfter doing this we check and append only those characters that do not cross `repeatLimit` as in question says there is no need to include every character from string `s`.\n\n# Complexity\n- Time complexity:\n$$O(n logn)$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n sort(s.rbegin(), s.rend());\n int i=0,j=0, n=s.size();\n\n while(j<n){\n while(j<n && s[i]==s[j]) j++;\n \n while(i<n && j<n && s[i]!=s[j])\n {\n char c = s[i];\n int len=1;\n while(i<n && len <= repeatLimit && s[i]==c){\n len++;\n i++;\n }\n if(s[i]==c) {\n swap(s[i],s[j]);\n i++;\n j++;\n }\n }\n }\n\n // checking and appending only valid chars\n\n string out = "";\n out += s[0];\n int len=1;\n for(int i=1;i<n;i++){\n if(s[i]==s[i-1]) len++;\n else len=1;\n if(len<=repeatLimit) out += s[i];\n }\n \n return out;\n }\n};\n```\n\n`EDIT- corrected time complexity` | 3 | 0 | ['Two Pointers', 'Sorting', 'C++'] | 1 |
construct-string-with-repeat-limit | โ
๐ฅ 95% Faster with 90% less memory | Array | No inbuilt algorithm used โ
๐ฅ | 100-faster-with-less-memory-array-no-inb-pto5 | IntuitionWe can use a basic array to find the frequency of each character from 'a' to 'z'. Then, sort the result in descending order from 'z' to 'a' based on th | Surendaar | NORMAL | 2024-12-17T04:34:11.282728+00:00 | 2024-12-17T04:36:54.781044+00:00 | 294 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe can use a basic array to find the frequency of each character from \'a\' to \'z\'. Then, sort the result in descending order from \'z\' to \'a\' based on the given conditions of maximum repetition and lexicographical order.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Find the frequency of each character.\n2. Add the values to the result string builder in descending order.\n3. If the frequency exceeds the given repeat limit, store the excess in a cache variable, insert the next smallest character once, and repeat the process.\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\nKindly upvote if you reached till here.. :)\n\n# Code\n```java []\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n StringBuilder res = new StringBuilder();\n int[] alpha = new int[26];\n for(char ch: s.toCharArray()){\n alpha[ch-\'a\']++;\n }\n char t = \'z\';\n int val = 0;\n for(int i=25; i>=0; i--){\n if(alpha[i]>0){\n while(val>0 && alpha[i]>0){\n res.append((char)(\'a\'+i));\n alpha[i]--;\n for(int j=0; j<Math.min(repeatLimit, val); j++){\n res.append(t);\n }\n val = val - Math.min(repeatLimit, val);\n }\n if(alpha[i]>0){\n for(int j=0; j<Math.min(repeatLimit, alpha[i]); j++){\n res.append((char)(\'a\'+i));\n }\n alpha[i] = alpha[i] - Math.min(repeatLimit, alpha[i]);\n }\n if(alpha[i]>0){\n t = (char)(\'a\'+i);\n val = alpha[i];\n }\n }\n }\n return res.toString();\n }\n}\n``` | 3 | 0 | ['Array', 'Greedy', 'Java'] | 0 |
construct-string-with-repeat-limit | C++ Solution || without Heap, using Map | c-solution-without-heap-using-map-by-shi-mnrn | if it's help, please up โฌ vote! โค๏ธComplexity
Overall Time Complexity: O(nlogk+26n) = O(nlogk)
Space complexity: O(k) (max 26 char)
Code | shishirRsiam | NORMAL | 2024-12-17T02:42:20.461481+00:00 | 2024-12-17T02:42:20.461481+00:00 | 328 | false | # if it\'s help, please up \u2B06 vote! \u2764\uFE0F\n\n\n\n\n## Complexity\n- Overall Time Complexity: **O(nlogk+26n) = O(nlogk)**\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(k) (max 26 char)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n void eraseData(map<char, int> &store, char ch)\n {\n if(not store[ch])\n store.erase(ch);\n }\n string repeatLimitedString(string s, int repeatLimit) \n {\n map<char, int> store;\n for(auto ch : s) store[ch] += 1;\n \n string ans;\n int count = 0;\n\n char prev = \'-\';\n while(!store.empty())\n {\n char ch = store.rbegin()->first;\n\n if(prev != ch or count < repeatLimit)\n {\n ans += ch;\n store[ch] -= 1;\n eraseData(store, ch);\n\n if(prev == ch) count += 1;\n else count = 1, prev = ch;\n }\n else \n {\n bool flag = true;\n for(char nextChar = ch - 1; nextChar >= \'a\'; nextChar--)\n {\n if(store.count(nextChar))\n {\n ans += nextChar;\n store[nextChar] -= 1;\n eraseData(store, nextChar);\n count = 1, prev = nextChar, flag = false;\n break;\n }\n }\n if(flag) break;\n }\n }\n\n return ans; \n }\n};\n``` | 3 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'C++'] | 3 |
construct-string-with-repeat-limit | For beginners - Heap Basics/Python [15 lines code] | for-beginners-heappython-by-jayanth_y-ig6k | Code | jayanth_y | NORMAL | 2024-12-17T01:46:29.252718+00:00 | 2024-12-17T22:26:42.685415+00:00 | 330 | false | # Code\n```python3 []\nclass Solution:\n def repeatLimitedString(self, s: str, rl: int) -> str:\n h = [ (-ord(x), y) for x, y in Counter(s).items() ]\n heapq.heapify(h)\n \n res = ""\n while h:\n x, y = heapq.heappop(h)\n while y > rl and h: \n res += chr(-x) * rl\n y -= rl\n a, b = heapq.heappop(h)\n res += chr(-a)\n if (b-1) > 0: heapq.heappush(h, (a, b-1))\n res += chr(-x) * min(y, rl)\n return res\n``` | 3 | 0 | ['Heap (Priority Queue)', 'Python3'] | 0 |
construct-string-with-repeat-limit | [Python3] Priority Queue + Greedy + Couting - Simple Solution | python3-priority-queue-greedy-couting-si-shnz | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | dolong2110 | NORMAL | 2023-07-11T18:42:43.698401+00:00 | 2023-07-11T18:42:43.698434+00:00 | 161 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(NlogN)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(N)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n cnt = collections.Counter(s)\n chars = [(-ord(k), k, v) for k, v in cnt.items()]\n\n heapq.heapify(chars)\n res = []\n while chars:\n o, ch, ct = heapq.heappop(chars)\n add = 0\n if len(res) >= repeatLimit and res[-1] == ch:\n if not chars: break\n no, nch, nct = heapq.heappop(chars)\n res.append(nch)\n if nct - 1 != 0: heapq.heappush(chars, (no, nch, nct - 1))\n else:\n add = min(repeatLimit, ct)\n res.extend([ch for _ in range(add)])\n if ct - add != 0: heapq.heappush(chars, (o, ch, ct - add))\n return "".join(res)\n``` | 3 | 1 | ['String', 'Greedy', 'Heap (Priority Queue)', 'Counting', 'Python3'] | 1 |
construct-string-with-repeat-limit | Java || Priority Queue || Hash Map Implementation | java-priority-queue-hash-map-implementat-cqif | \nclass Element {\n char letter;\n int frequency;\n Element(char letter, int frequency) {\n this.letter = letter;\n this.frequency = freq | devansh2805 | NORMAL | 2022-04-13T07:37:36.431672+00:00 | 2022-04-13T07:37:36.431711+00:00 | 292 | false | ```\nclass Element {\n char letter;\n int frequency;\n Element(char letter, int frequency) {\n this.letter = letter;\n this.frequency = frequency;\n }\n}\n\nclass ElementComparator implements Comparator<Element> {\n @Override\n public int compare(Element e1, Element e2) {\n return Character.compare(e2.letter, e1.letter);\n }\n}\n\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n PriorityQueue<Element> maxHeap = new PriorityQueue<>(new ElementComparator());\n Map<Character, Integer> freqMap = new HashMap<>();\n for(char letter: s.toCharArray()) {\n freqMap.put(letter, freqMap.getOrDefault(letter, 0) + 1); \n }\n for(Map.Entry<Character, Integer> entry: freqMap.entrySet()) {\n maxHeap.offer(new Element(entry.getKey(), entry.getValue()));\n }\n StringBuilder answer = new StringBuilder();\n while(!maxHeap.isEmpty()) {\n Element element = maxHeap.poll();\n for(int i=0;i<repeatLimit;i++) {\n answer.append(element.letter);\n element.frequency--;\n if(element.frequency == 0) {\n break;\n }\n }\n if(element.frequency != 0) {\n if(!maxHeap.isEmpty()) {\n Element next = maxHeap.poll();\n answer.append(next.letter);\n next.frequency--;\n if(next.frequency != 0) {\n maxHeap.offer(next);\n }\n maxHeap.offer(element);\n }\n }\n }\n return answer.toString();\n }\n}\n``` | 3 | 0 | ['Heap (Priority Queue)', 'Java'] | 1 |
construct-string-with-repeat-limit | Simple Java Solution with O(n) time complexity. | simple-java-solution-with-on-time-comple-p8en | Bullets boints to make this question super easily\n\n Construct a string which contains all the alphabet from a to z and also a integer array of length 26.\n co | htksaurav | NORMAL | 2022-03-27T16:55:26.458055+00:00 | 2022-03-27T16:55:26.458098+00:00 | 378 | false | Bullets boints to make this question super easily\n\n* Construct a string which contains all the alphabet from a to z and also a integer array of length 26.\n* count repeatation of each character and stored in integer arrat.\n* Now loop over integer array in reverse order and apply following codition.\n* if repeated char is less then equal to repeatLimit then directly add it in to answer string,\n* else first add character to answer at most repeatLimit and then loop over them till that character value becomes zero.\n```class Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n String alphabet = "abcdefghijklmnopqrstuvwxyz";\n int []arr = new int[26];\n StringBuilder str = new StringBuilder();\n for(int i=0;i<s.length();i++){\n arr[s.charAt(i)-\'a\']++;\n }\n for(int i=arr.length-1;i>=0;i--){\n if(arr[i]<=repeatLimit){\n String a = Character.toString(alphabet.charAt(i));\n str.append(a.repeat(arr[i]));\n }else{\n String a = Character.toString(alphabet.charAt(i));\n str.append(a.repeat(repeatLimit));\n arr[i]-=repeatLimit;\n int j = i-1;\n while(arr[i]>0&&j>=0){\n if(arr[j]>0){\n str.append(alphabet.charAt(j));\n arr[j]--;\n str.append(a.repeat(Math.min(repeatLimit, arr[i])));\n arr[i]-=Math.min(repeatLimit, arr[i]);\n }\n if(arr[j]==0)\n j--;\n }\n i=j+1;\n }\n }\n return str.toString();\n }\n}\n``` | 3 | 0 | ['Java'] | 0 |
construct-string-with-repeat-limit | [C++] O(n) easy to understand. | c-on-easy-to-understand-by-lovebaonvwu-8gpu | \nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n int cnt[26] = {0};\n \n for (auto c : s) {\n | lovebaonvwu | NORMAL | 2022-02-25T02:04:43.614328+00:00 | 2022-02-25T02:06:23.667267+00:00 | 259 | false | ```\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n int cnt[26] = {0};\n \n for (auto c : s) {\n ++cnt[c - \'a\'];\n }\n \n string ans;\n int prevsize = -1;\n \n int k = 0;\n while (ans.size() < s.size()) { \n for (int i = 25; i >= 0; --i) {\n \n if (cnt[i] == 0) {\n continue;\n }\n \n if ((i + \'a\') == ans.back() && k == repeatLimit) {\n continue;\n }\n \n k = (i + \'a\') == ans.back() ? k + 1 : 1;\n \n ans.push_back((i + \'a\'));\n --cnt[i];\n break;\n }\n \n if (prevsize == ans.size()) {\n break;\n }\n \n prevsize = ans.size();\n }\n \n return ans;\n }\n};\n``` | 3 | 0 | [] | 0 |
construct-string-with-repeat-limit | Java Easy to Understand | PriorityQueue Solution | java-easy-to-understand-priorityqueue-so-bvew | Add all the characters to a PriorityQueue which sorts in reverse order.\n2. Remove all the elements in the queue and add them to a String if they are occuring l | sridhar2007 | NORMAL | 2022-02-20T11:52:29.944186+00:00 | 2022-02-20T18:05:30.387180+00:00 | 435 | false | 1. Add all the characters to a PriorityQueue which sorts in reverse order.\n2. Remove all the elements in the queue and add them to a String if they are occuring less then the limit\n3. Else push them to another PriorityQueue and add them if there is a new character to go before this.\n\nUpvote if you like the solution.\n\n```\n public String repeatLimitedString(String s, int repeatLimit) {\n\n PriorityQueue<Character> q = new PriorityQueue<>(Comparator.reverseOrder());\n PriorityQueue<Character> extra = new PriorityQueue<>(Comparator.reverseOrder());\n for(char c : s.toCharArray()){\n q.add(c);\n }\n StringBuilder sb = new StringBuilder();\n\n char prev = q.poll();\n sb.append(prev);\n int count = 1;\n\n while(!q.isEmpty()){\n char c = q.poll();\n if(prev == c && count < repeatLimit) {\n sb.append(c);\n count++;\n } else if (prev == c ) {\n extra.add(c);\n } else {\n if (!extra.isEmpty() && extra.peek() > c){\n sb.append(c);\n int popCount =0;\n while (!extra.isEmpty() && extra.peek() > c && popCount < repeatLimit) {\n q.add(extra.poll());\n popCount++;\n }\n } else {\n sb.append(c);\n }\n prev = c;\n count=1;\n }\n }\n return sb.toString();\n }\n``` | 3 | 0 | ['Heap (Priority Queue)', 'Java'] | 1 |
construct-string-with-repeat-limit | [c++] Frequency Vector + Priority Queue | c-frequency-vector-priority-queue-by-gar-vgjq | \nclass Solution {\npublic:\n string repeatLimitedString(string s, int limit){\n vector<int> hash(26);\n for(int i=0;i<s.length();i++) hash[s[i | garvbareja | NORMAL | 2022-02-20T09:16:37.592248+00:00 | 2022-02-20T09:16:37.592273+00:00 | 210 | false | ```\nclass Solution {\npublic:\n string repeatLimitedString(string s, int limit){\n vector<int> hash(26);\n for(int i=0;i<s.length();i++) hash[s[i]-\'a\']++;\n priority_queue<pair<char,int>> pq; \n for(int i=0;i<26;i++) if(hash[i]) pq.push({\'a\'+i,hash[i]});\n string res="";\n while(!pq.empty()){\n char c=pq.top().first; int freq=pq.top().second; pq.pop();\n int temp=min(limit,freq);\n while(temp--) res.push_back(c);\n if(pq.empty()) return res;\n freq=freq-min(limit,freq);\n if(freq){\n char smol=pq.top().first; int smolfreq=pq.top().second; pq.pop();\n res.push_back(smol); smolfreq--;\n if(smolfreq) pq.push({smol,smolfreq});\n pq.push({c,freq});\n }\n \n }\n return res;\n }\n};\n``` | 3 | 1 | ['Greedy', 'C', 'Heap (Priority Queue)', 'C++'] | 1 |
construct-string-with-repeat-limit | C++ Easy Solution | c-easy-solution-by-simplekind-o1ev | \n#define deb(x) cout << #x << " = " << x << endl\nclass Solution {\npublic:\n string repeatLimitedString(string s, int rL) {\n map<char,int> m ;\n | simplekind | NORMAL | 2022-02-20T05:02:26.320151+00:00 | 2022-02-20T05:02:26.320178+00:00 | 440 | false | ```\n#define deb(x) cout << #x << " = " << x << endl\nclass Solution {\npublic:\n string repeatLimitedString(string s, int rL) {\n map<char,int> m ;\n for (int i =0;i<26;i++)m[i+\'a\']=0;\n for (int i = 0 ;i <s.length();i++)\n m[s[i]]++;\n string res = "";\n priority_queue <pair<int,int>>pq ;\n for(int i =0 ;i<26;i++) if(m[i+\'a\'])pq.push({i+\'a\',m[i+\'a\']});\n while(!pq.empty()){\n char c = pq.top().first;\n int x = pq.top().second;\n pq.pop();\n int leftOvers = x-rL;\n // deb(c);\n // deb(x);\n if(leftOvers<=0){\n if(res.length()){\n if(res[res.length()-1]==c) continue;\n }\n while(x){\n res+=c;\n x--;\n }\n }else{\n while(x!=leftOvers){\n res+=c;\n x--;\n }\n if(pq.empty()) return res;\n char ctemp = pq.top().first;\n int xtemp = pq.top().second;\n pq.pop();\n res+=ctemp;\n xtemp--;\n pq.push({c,leftOvers});\n if(xtemp) pq.push({ctemp,xtemp});\n }\n }\n return res;\n }\n};\n``` | 3 | 0 | ['Greedy', 'C', 'Heap (Priority Queue)', 'C++'] | 0 |
construct-string-with-repeat-limit | C++ Easy Thinking and Approach | c-easy-thinking-and-approach-by-sanheen-ree9i | \n Count all characters in 26 buckets (\'a\' to \'z\').\n While we have characters left:\n\t \tFind the largest bucket i with characters.\n\t \tPick up to limit | sanheen-sethi | NORMAL | 2022-02-20T04:43:22.026528+00:00 | 2022-02-20T04:43:22.026566+00:00 | 473 | false | \n* Count all characters in 26 buckets (\'a\' to \'z\').\n* While we have characters left:\n\t* \tFind the largest bucket i with characters.\n\t* \tPick up to limit characters from that bucket.\n\t* \tFind the second largest bucket j with characters.\n\t* \tPick 1 character from the next largest bucket.\n\n\n```\n#define debug(x) cout<<#x<<":"<<x<<endl;\n #define debug2(x,y) cout<<#x<<":"<<x<<"|"<<#y<<":"<<y<<endl;\n string repeatLimitedString(string s, int repeatLimit) {\n int arr[26] = {0};\n for(auto& val:s){\n arr[val-\'a\']++;\n }\n \n // cout<<"["<<" ";\n // for(int i = 0;i<=25;i++){\n // debug((char)(i+\'a\'),arr[i]);\n // }\n // cout<<"]"<<endl;\n \n string str = "";\n int idx = 25;\n \n while(idx >= 0){\n //debug(idx);\n int rp = repeatLimit;\n while(arr[idx] != 0 && rp){\n str += (idx + \'a\');\n //debug2(idx,str);\n arr[idx]--;\n //debug2(idx,arr[idx]);\n rp--;\n }\n if(arr[idx] == 0){\n idx--;\n continue;\n }\n if(arr[idx] != 0){\n if(idx != 0){\n int j = idx-1;\n //debug2(j,arr[j]);\n while(j >= 0 && arr[j] == 0) j--;\n //debug2(j,arr[j]);\n if(j < 0) break;\n str += (j+\'a\');\n arr[j]--;\n }else{\n break;\n }\n }\n }\n return str;\n }\n``` | 3 | 0 | ['Greedy', 'C', 'C++'] | 0 |
construct-string-with-repeat-limit | Simple JAVA Solution | simple-java-solution-by-mohit038-7lbr | \nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int i = 25, dp[] = new int[26];\n for (char c: s.toCharAr | mohit038 | NORMAL | 2022-02-20T04:02:19.366069+00:00 | 2022-02-20T04:03:42.341600+00:00 | 191 | false | ```\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int i = 25, dp[] = new int[26];\n for (char c: s.toCharArray()) dp[c - \'a\']++;\n StringBuilder sb = new StringBuilder();\n while(i > -1) {\n while (dp[i] > 0) {\n int number = Math.min(dp[i], repeatLimit);\n for (int j = 0; j < number; j++) sb.append((char)(i + \'a\'));\n dp[i] -= number;\n if (dp[i] > 0) {\n char c = getPrevChar(dp , i-1);\n if (c == \'$\') break;\n else if (c != \'$\') sb.append(c);\n }\n }\n i--;\n }\n return sb.toString();\n }\n\n private char getPrevChar(int[] dp,int i) {\n if (i < 0) return \'$\';\n if (dp[i] == 0) return getPrevChar(dp, i - 1);\n else {\n dp[i]--;\n return (char)(\'a\' + i);\n }\n }\n}\n``` | 3 | 1 | [] | 1 |
construct-string-with-repeat-limit | C++ solution O(nlogn) | c-solution-onlogn-by-adarshxd-8y9p | \nclass Solution {\npublic:\n string repeatLimitedString(string s, int rl) {\n int limit = rl;\n int n= s.size();\n sort(s.begin(), s.en | AdarshxD | NORMAL | 2022-02-20T04:02:00.481397+00:00 | 2022-02-20T04:03:20.256551+00:00 | 365 | false | ```\nclass Solution {\npublic:\n string repeatLimitedString(string s, int rl) {\n int limit = rl;\n int n= s.size();\n sort(s.begin(), s.end());\n reverse(s.begin(), s.end());\n //construction of p\n string p; \n p.push_back(s[0]);\n int size = 1;\n unordered_map<char, int> map;\n for(int i = 0; i<n; i++){\n map[s[i]]++;\n if(s[i]!= p[size-1]){\n size++;\n p.push_back(s[i]);\n }\n }\n //for(int i = 0; i<size; i++) cout<<p[i]<<" - "<<map[p[i]]<<endl;\n \n string res;\n if(size == 1){\n for(int i = 0; i< min(limit, map[p[0]]); i++) res.push_back(p[0]);\n return res;\n }\n int curr = 0;\n int next = 1;\n while(next < size){\n if(!map[p[curr]]) {\n curr = next;\n next++;\n continue;\n }\n //limit >= fre\n if(limit >= map[p[curr]] and map[p[curr]]){\n //push those\n for(int i=0; i<map[p[curr]]; i++) res.push_back(p[curr]);\n map[p[curr]] = 0;\n curr = next;\n next++;\n //limit = rl; //reseting it to rl\n continue;\n }\n //limit < fre\n for(int i = 0; i<limit; i++){\n //push limit times\n res.push_back(p[curr]);\n }\n map[p[curr]]-= limit;\n if(map[p[next]]){\n res.push_back(p[next]);\n map[p[next]]--;\n }\n else {\n next++;\n if(next == size) break;\n res.push_back(p[next]);\n map[p[next]]--;\n }\n }\n if(curr<size and map[p[curr]] and p[curr] != res[res.size()-1]){\n for(int i = 0; i< min(limit, map[p[curr]]); i++) res.push_back(p[curr]);\n }\n return res;\n }\n};\n``` | 3 | 0 | ['C'] | 1 |
construct-string-with-repeat-limit | Simple Java Solution with Comments | simple-java-solution-with-comments-by-pa-7c4s | ```\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n TreeMap tm = new TreeMap<>(Collections.reverseOrder());\n | pavankumarchaitanya | NORMAL | 2022-02-20T04:00:48.617919+00:00 | 2022-02-20T04:00:48.617953+00:00 | 406 | false | ```\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n TreeMap<Character, Integer> tm = new TreeMap<>(Collections.reverseOrder());\n StringBuffer sb = new StringBuffer();\n boolean shouldGetLargest = true;\n for(char c: s.toCharArray())\n {\n tm.put(c, tm.getOrDefault(c,0)+1); \n }\n while(tm.size()>0){\n char c = tm.firstKey();// get the largest character\n if(sb.length()!=0 && sb.charAt(sb.length()-1) == c){ // if last char of sb is same as largest character\n shouldGetLargest = false; \n }\n int charCount =0, count = 0;\n\n if(shouldGetLargest){\n charCount = tm.get(c);\n count = Math.min(charCount,repeatLimit);\n }else{\n //get second largest\n if(tm.size()>1){\n char largest = c;\n c = tm.subMap(largest, false, \'a\', true).firstKey(); // exclude largest\n charCount = tm.get(c);\n count = 1;// use second largest char only once to build lexicographically largest\n }\n // if should get next largest and only one key exists we should drop them - happens automatically because count == charCount\n }\n if(count==charCount)\n tm.remove(c);\n else\n tm.put(c, charCount-count);\n\n while(count>0){ //insert till repeat limit or available character count\n sb.append(c);\n count--;\n }\n \n if(!shouldGetLargest)// if we used second largest this time, use largest next time\n shouldGetLargest = !shouldGetLargest;\n }\n return sb.toString();\n }\n\n} | 3 | 1 | [] | 1 |
construct-string-with-repeat-limit | java easy solution || 8ms || construct string | java-easy-solution-8ms-construct-string-u0hkl | class Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int[] freq = new int[26];\n for (char ch : s.toCharArray()) | Seema_Kumari1 | NORMAL | 2024-12-18T05:17:37.521901+00:00 | 2024-12-18T05:17:37.521945+00:00 | 4 | false | class Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int[] freq = new int[26];\n for (char ch : s.toCharArray()) {\n freq[ch - \'a\']++;\n }\n\n StringBuilder result = new StringBuilder();\n int currentCharIndex = 25;\n\n while (currentCharIndex >= 0) {\n if (freq[currentCharIndex] == 0) {\n currentCharIndex--;\n continue;\n }\n\n int use = Math.min(freq[currentCharIndex], repeatLimit);\n for (int k = 0; k < use; k++) {\n result.append((char) (\'a\' + currentCharIndex));\n }\n freq[currentCharIndex] -= use;\n\n if (freq[currentCharIndex] > 0) {\n int smallerCharIndex = currentCharIndex - 1;\n while (smallerCharIndex >= 0 && freq[smallerCharIndex] == 0) {\n smallerCharIndex--;\n }\n if (smallerCharIndex < 0) {\n break; \n }\n result.append((char) (\'a\' + smallerCharIndex));\n freq[smallerCharIndex]--;\n }\n }\n\n return result.toString();\n }\n}\n | 2 | 0 | ['Java'] | 0 |
construct-string-with-repeat-limit | โ
Simple Java Solution | Map + PQ | simple-java-solution-map-pq-by-harsh__00-uiyo | CODE\nJava []\npublic String repeatLimitedString(String s, int repeatLimit) {\n\tMap<Character, Integer> map = new HashMap<>();\n\tfor(char ch : s.toCharArray() | Harsh__005 | NORMAL | 2024-12-17T18:00:39.387547+00:00 | 2024-12-17T18:00:39.387573+00:00 | 27 | false | ## **CODE**\n```Java []\npublic String repeatLimitedString(String s, int repeatLimit) {\n\tMap<Character, Integer> map = new HashMap<>();\n\tfor(char ch : s.toCharArray()){\n\t\tmap.put(ch, map.getOrDefault(ch, 0) + 1);\n\t}\n\n\tPriorityQueue<Character> pq = new PriorityQueue<>(Collections.reverseOrder());\n\tfor(char ch : map.keySet()) pq.add(ch);\n\n\tStringBuffer res = new StringBuffer("");\n\twhile(!pq.isEmpty()) {\n\t\tchar curr = pq.remove();\n\t\tint min = Math.min(map.get(curr), repeatLimit);\n\t\tres.append((""+curr).repeat(min));\n\n\t\tif(map.get(curr) == min) map.remove(curr);\n\t\telse if(!pq.isEmpty()) {\n\t\t\tchar next = pq.remove();\n\t\t\tres.append(next);\n\t\t\tif(map.get(next) == 1) map.remove(next);\n\t\t\telse {\n\t\t\t\tmap.put(next, map.get(next)-1);\n\t\t\t\tpq.add(next);\n\t\t\t}\n\n\t\t\tmap.put(curr, map.get(curr)-min);\n\t\t\tpq.add(curr);\n\t\t}\n\t}\n\treturn res.toString();\n}\n``` | 2 | 0 | ['Java'] | 1 |
construct-string-with-repeat-limit | Easy Solution using map for beginners !!! | easy-solution-using-map-for-beginners-by-fuba | IntuitionWe want to build a string by repeating characters from the input string s, but no character should appear more than k times consecutively. The idea is | sachanharshit | NORMAL | 2024-12-17T22:53:58.957393+00:00 | 2024-12-17T22:53:58.957393+00:00 | 14 | false | # Intuition\nWe want to build a string by repeating characters from the input string s, but no character should appear more than k times consecutively. The idea is to always pick the largest available character and add it to the result, respecting the k limit. If a character appears more than k times, we handle it by inserting the next largest character.\n\n# Approach\n1) Count character frequencies using a map to store how many times each character appears in the string.\n2) **Greedy selection**: Always pick the largest character available, adding it to the result up to k times.\n3) **Handle remaining characters**: If a character appears more than k times, we add the next largest character once and then put that character in map and reduce the count by 1 then put the original character back with its reduced count.\n4) Repeat this process until all characters are added to the result.\n\n# Complexity\n- Time complexity:\nO(nlogm), where n is the length of the string, and m is the number of unique characters.\n\n- Space complexity:\nO(m), where m is the number of unique characters in the string.\n\n# Code\n```cpp []\nclass Solution {\npublic:\n \n string repeatLimitedString(string s, int k) {\n map<char , int > mp;\n string ans = "";\n for(auto it : s){\n mp[it]++;\n }\n while(mp.size()>0){\n auto it = mp.rbegin();\n char hj = it->first;\n int co = it->second;\n int num = min(co , k);\n while(num--){\n ans += hj;\n }\n mp.erase(hj);\n bool flag = true;\n if(co>k && mp.size()>0){\n flag = false;\n it = mp.rbegin();\n char ch = it->first;\n int nu = it->second;\n mp.erase(ch);\n ans += ch;\n nu--;\n if(nu>0){\n mp[ch] = nu;\n }\n }\n if(co>k && !flag){\n mp[hj] = co - k;\n }\n }\n \n return ans;\n }\n};\n``` | 2 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting'] | 1 |
construct-string-with-repeat-limit | Swift | Greedy | swift-great-solution-if-i-may-say-so-by-9wenq | Code | pagafan7as | NORMAL | 2024-12-17T17:46:41.701427+00:00 | 2024-12-17T23:48:08.470877+00:00 | 22 | false | # Code\n```swift []\nclass Solution {\n func repeatLimitedString(_ s: String, _ repeatLimit: Int) -> String {\n var repeatCount = 0\n var overflow = [Character]()\n var res = ""\n\n // Greedily, go through each character by descending order.\n for c in s.sorted(by: >) {\n if repeatCount == repeatLimit && res.last == c {\n // Unable to use "c" for now. Save it for later.\n overflow.append(c)\n continue\n }\n\n append(c)\n for _ in 0..<min(repeatLimit, overflow.count) {\n append(overflow.removeLast())\n }\n }\n\n return res\n\n func append(_ c: Character) {\n if res.last != c { repeatCount = 0 }\n res.append(c)\n repeatCount += 1\n }\n }\n}\n``` | 2 | 0 | ['Swift'] | 0 |
construct-string-with-repeat-limit | ๐ Greedy Approach: Simple & Beginner-Friendly๐ | ๐ฅ Beats 95% ๐ช | greedy-approach-simple-beginner-friendly-q6uv | Intuition ๐ค:We aim to create the lexicographically largest string. Using a greedy approach:
Pick the largest character ๐ฏ (e.g., 'z') as much as allowed by repea | mansimittal935 | NORMAL | 2024-12-17T16:07:46.131722+00:00 | 2024-12-17T16:07:46.131722+00:00 | 87 | false | # Intuition \uD83E\uDD14:\nWe aim to create the lexicographically largest string. Using a greedy approach:\n\n1. Pick the largest character \uD83C\uDFAF (e.g., \'z\') as much as allowed by repeatLimit \u23F3.\n2. Once we hit the limit, move to the next largest character \uD83D\uDD3D.\n3. Repeat this until we\u2019ve used all characters from the string \uD83D\uDCDD.\n\n---\n\n# Approach \uD83D\uDEE0\uFE0F:\n1. Count frequencies of each character \uD83D\uDCCA.\n2. Greedily add characters from largest to smallest \uD83C\uDF1F.\n- Add up to repeatLimit times from the largest available letter \uD83D\uDD25.\n3. Stop when all letters are used \u23F9\uFE0F.\n\n---\n\n# Complexity\n- Time Complexity \u23F1\uFE0F:\n*$$O(n)$$*, where n is the length of the string \u2728.\n\n\n- Space Complexity \uD83D\uDCBE:\n*$$O(1)$$*, constant space used for counting 26 letters \uD83C\uDFAF.\n\n---\n\n## Why This Works \uD83D\uDCAA:\n- Greedy = Best \uD83D\uDCA1! Always choose the largest possible letter at each step for the largest lexicographical result \uD83C\uDF89.\n- The algorithm is efficient and fast\u2014Beats 95%! \uD83C\uDFC5\n\n\n# Code\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n vector<int> letterCount (26, 0);\n for(int i = 0; i < s.size(); i++) {\n letterCount[s[i]-\'a\']++;\n }\n\n string ans = "";\n int index = 25;\n while(true) {\n int countToAdd = min(repeatLimit, letterCount[index]);\n if(countToAdd > 0) {\n ans.append(countToAdd, \'a\' + index);\n letterCount[index] -= countToAdd;\n }\n\n int nextIndex = index-1;\n if(letterCount[index] > 0) {\n while(nextIndex >= 0 && letterCount[nextIndex] == 0) {\n nextIndex--;\n }\n if(nextIndex < 0) break;\n\n ans += (\'a\' + nextIndex);\n letterCount[nextIndex]--;\n } else {\n while(index >= 0 && letterCount[index] == 0) {\n index--;\n }\n if(index < 0) break;\n }\n }\n return ans;\n }\n};\n```\n\n```java []\nimport java.util.*;\n\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int[] letterCount = new int[26];\n for (char c : s.toCharArray()) {\n letterCount[c - \'a\']++;\n }\n\n StringBuilder ans = new StringBuilder();\n int index = 25;\n\n while (true) {\n int countToAdd = Math.min(repeatLimit, letterCount[index]);\n if (countToAdd > 0) {\n for (int i = 0; i < countToAdd; i++) {\n ans.append((char) (\'a\' + index));\n }\n letterCount[index] -= countToAdd;\n }\n\n int nextIndex = index - 1;\n if (letterCount[index] > 0) {\n while (nextIndex >= 0 && letterCount[nextIndex] == 0) {\n nextIndex--;\n }\n if (nextIndex < 0) break;\n\n ans.append((char) (\'a\' + nextIndex));\n letterCount[nextIndex]--;\n } else {\n while (index >= 0 && letterCount[index] == 0) {\n index--;\n }\n if (index < 0) break;\n }\n }\n\n return ans.toString();\n }\n}\n\n```\n```javascript []\nvar repeatLimitedString = function(s, repeatLimit) {\n let letterCount = new Array(26).fill(0);\n for (let char of s) {\n letterCount[char.charCodeAt(0) - \'a\'.charCodeAt(0)]++;\n }\n\n let ans = "";\n let index = 25;\n\n while (true) {\n let countToAdd = Math.min(repeatLimit, letterCount[index]);\n if (countToAdd > 0) {\n ans += String.fromCharCode(\'a\'.charCodeAt(0) + index).repeat(countToAdd);\n letterCount[index] -= countToAdd;\n }\n\n let nextIndex = index - 1;\n if (letterCount[index] > 0) {\n while (nextIndex >= 0 && letterCount[nextIndex] === 0) {\n nextIndex--;\n }\n if (nextIndex < 0) break;\n\n ans += String.fromCharCode(\'a\'.charCodeAt(0) + nextIndex);\n letterCount[nextIndex]--;\n } else {\n while (index >= 0 && letterCount[index] === 0) {\n index--;\n }\n if (index < 0) break;\n }\n }\n\n return ans;\n};\n\n```\n```python []\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n letterCount = [0] * 26\n for c in s:\n letterCount[ord(c) - ord(\'a\')] += 1\n\n ans = []\n index = 25\n\n while True:\n countToAdd = min(repeatLimit, letterCount[index])\n if countToAdd > 0:\n ans.extend(chr(ord(\'a\') + index) * countToAdd)\n letterCount[index] -= countToAdd\n\n nextIndex = index - 1\n if letterCount[index] > 0:\n while nextIndex >= 0 and letterCount[nextIndex] == 0:\n nextIndex -= 1\n if nextIndex < 0: break\n\n ans.append(chr(ord(\'a\') + nextIndex))\n letterCount[nextIndex] -= 1\n else:\n while index >= 0 and letterCount[index] == 0:\n index -= 1\n if index < 0: break\n\n return "".join(ans)\n\n```\n```ruby []\ndef repeat_limited_string(s, repeat_limit)\n letter_count = Array.new(26, 0)\n s.each_char { |c| letter_count[c.ord - \'a\'.ord] += 1 }\n\n ans = ""\n index = 25\n\n while true\n count_to_add = [repeat_limit, letter_count[index]].min\n if count_to_add > 0\n ans << ((\'a\'.ord + index).chr * count_to_add)\n letter_count[index] -= count_to_add\n end\n\n next_index = index - 1\n if letter_count[index] > 0\n next_index -= 1 while next_index >= 0 && letter_count[next_index] == 0\n break if next_index < 0\n\n ans << ((\'a\'.ord + next_index).chr)\n letter_count[next_index] -= 1\n else\n index -= 1 while index >= 0 && letter_count[index] == 0\n break if index < 0\n end\n end\n\n ans\nend\n\n```\n```rust []\nimpl Solution {\n pub fn repeat_limited_string(s: String, repeat_limit: i32) -> String {\n let mut letter_count = vec![0; 26];\n for c in s.chars() {\n letter_count[(c as usize) - (\'a\' as usize)] += 1;\n }\n\n let mut ans = String::new();\n let mut index = 25;\n\n loop {\n let count_to_add = repeat_limit.min(letter_count[index]);\n if count_to_add > 0 {\n ans.push_str(&std::iter::repeat((b\'a\' + index as u8) as char)\n .take(count_to_add as usize)\n .collect::<String>());\n letter_count[index] -= count_to_add;\n }\n\n let mut next_index = index - 1;\n if letter_count[index] > 0 {\n while next_index > 0 && letter_count[next_index] == 0 {\n next_index -= 1;\n }\n if next_index == 0 && letter_count[next_index] == 0 {\n break;\n }\n\n ans.push((b\'a\' + next_index as u8) as char);\n letter_count[next_index] -= 1;\n } else {\n while index > 0 && letter_count[index] == 0 {\n index -= 1;\n }\n if index == 0 && letter_count[index] == 0 {\n break;\n }\n }\n }\n\n ans\n }\n}\n\n```\n```c# []\nusing System;\nusing System.Text;\n\npublic class Solution {\n public string RepeatLimitedString(string s, int repeatLimit) {\n int[] letterCount = new int[26];\n foreach (char c in s) {\n letterCount[c - \'a\']++;\n }\n\n StringBuilder ans = new StringBuilder();\n int index = 25;\n\n while (true) {\n int countToAdd = Math.Min(repeatLimit, letterCount[index]);\n if (countToAdd > 0) {\n ans.Append(new string((char)(\'a\' + index), countToAdd));\n letterCount[index] -= countToAdd;\n }\n\n int nextIndex = index - 1;\n if (letterCount[index] > 0) {\n while (nextIndex >= 0 && letterCount[nextIndex] == 0) {\n nextIndex--;\n }\n if (nextIndex < 0) break;\n\n ans.Append((char)(\'a\' + nextIndex));\n letterCount[nextIndex]--;\n } else {\n while (index >= 0 && letterCount[index] == 0) {\n index--;\n }\n if (index < 0) break;\n }\n }\n\n return ans.ToString();\n }\n}\n\n```\n```go []\nfunc repeatLimitedString(s string, repeatLimit int) string {\n letterCount := make([]int, 26)\n for _, c := range s {\n letterCount[c-\'a\']++\n }\n\n ans := ""\n index := 25\n\n for {\n countToAdd := min(repeatLimit, letterCount[index])\n if countToAdd > 0 {\n ans += strings.Repeat(string(\'a\'+index), countToAdd)\n letterCount[index] -= countToAdd\n }\n\n nextIndex := index - 1\n if letterCount[index] > 0 {\n for nextIndex >= 0 && letterCount[nextIndex] == 0 {\n nextIndex--\n }\n if nextIndex < 0 {\n break\n }\n\n ans += string(\'a\' + nextIndex)\n letterCount[nextIndex]--\n } else {\n for index >= 0 && letterCount[index] == 0 {\n index--\n }\n if index < 0 {\n break\n }\n }\n }\n return ans\n}\n\nfunc min(a, b int) int {\n if a < b {\n return a\n }\n return b\n}\n\n```\n | 2 | 0 | ['Hash Table', 'Greedy', 'Python', 'C++', 'Java', 'Go', 'Rust', 'Ruby', 'JavaScript', 'C#'] | 0 |
construct-string-with-repeat-limit | โ
Easy to Understand | HashTable | Step by Step | Detailed Video Explanation๐ฅ | easy-to-understand-hashtable-step-by-ste-z2ka | IntuitionThe problem requires constructing a string such that no character repeats more than the given limit consecutively. My initial thought was to use a gree | sahilpcs | NORMAL | 2024-12-17T14:54:50.268865+00:00 | 2024-12-17T14:54:50.268865+00:00 | 60 | false | # Intuition\nThe problem requires constructing a string such that no character repeats more than the given limit consecutively. My initial thought was to use a greedy approach, always selecting the lexicographically largest available character that respects the constraints, and falling back to smaller characters when necessary to break the repetition.\n\n# Approach\n1. Count the frequency of each character using an array of size 26 (one for each letter of the alphabet).\n2. Use a greedy strategy:\n - Start with the largest available character.\n - Append it to the result up to the repeat limit.\n - If the character\'s frequency is not exhausted, find the next smaller character that can be appended to break the repetition.\n3. Continue until no more characters can be added while respecting the constraints.\n4. Return the constructed string.\n\n# Complexity\n- Time complexity: \n $$O(n + k)$$ \n - $$O(n)$$ to count the frequency of characters, where $$n$$ is the length of the string.\n - $$O(k)$$ for iterating through the characters in the array (at most 26 steps for the alphabet).\n\n- Space complexity: \n $$O(1)$$ \n - Only a fixed-size frequency array of size 26 is used, regardless of the input size.\n\n\n# Code\n```java []\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n // Create a frequency array to count occurrences of each character in the string\n int[] freq = new int[26];\n for (char ch : s.toCharArray()) {\n freq[ch - \'a\']++;\n }\n\n // StringBuilder to construct the resulting string\n StringBuilder sb = new StringBuilder();\n // Start from the largest character (\'z\') and move towards the smallest (\'a\')\n int curr = 25;\n while (curr >= 0) {\n // Skip characters that are not present in the string\n if (freq[curr] == 0) {\n curr--;\n continue;\n }\n\n // Append the current character up to the repeat limit\n int limit = repeatLimit;\n while (freq[curr] > 0 && limit > 0) {\n sb.append((char) (\'a\' + curr));\n freq[curr]--;\n limit--;\n }\n\n // If the current character still has remaining frequency\n if (freq[curr] > 0) {\n // Find the next smaller character with a non-zero frequency\n int smaller = curr - 1;\n while (smaller >= 0 && freq[smaller] == 0) {\n smaller--;\n }\n\n // If no smaller character is found, break the loop\n if (smaller < 0) {\n break;\n }\n\n // Append the smaller character to avoid exceeding the repeat limit\n sb.append((char) (\'a\' + smaller));\n freq[smaller]--;\n }\n }\n\n // Return the constructed string\n return sb.toString();\n }\n}\n\n```\n```c++ []\n#include <string>\n#include <vector>\nusing namespace std;\n\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n vector<int> freq(26, 0);\n for (char ch : s) {\n freq[ch - \'a\']++;\n }\n\n string result;\n int curr = 25;\n while (curr >= 0) {\n if (freq[curr] == 0) {\n curr--;\n continue;\n }\n\n int limit = repeatLimit;\n while (freq[curr] > 0 && limit > 0) {\n result += (char)(\'a\' + curr);\n freq[curr]--;\n limit--;\n }\n\n if (freq[curr] > 0) {\n int smaller = curr - 1;\n while (smaller >= 0 && freq[smaller] == 0) {\n smaller--;\n }\n if (smaller < 0) {\n break;\n }\n result += (char)(\'a\' + smaller);\n freq[smaller]--;\n }\n }\n\n return result;\n }\n};\n```\n```python []\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n # Frequency array for counting occurrences of each character\n freq = [0] * 26\n for ch in s:\n freq[ord(ch) - ord(\'a\')] += 1\n\n result = []\n curr = 25\n while curr >= 0:\n if freq[curr] == 0:\n curr -= 1\n continue\n\n # Append the current character up to the repeat limit\n limit = repeatLimit\n while freq[curr] > 0 and limit > 0:\n result.append(chr(curr + ord(\'a\')))\n freq[curr] -= 1\n limit -= 1\n\n # If the current character still has remaining frequency\n if freq[curr] > 0:\n # Find the next smaller character with a non-zero frequency\n smaller = curr - 1\n while smaller >= 0 and freq[smaller] == 0:\n smaller -= 1\n\n # If no smaller character is found, break\n if smaller < 0:\n break\n\n # Append the smaller character to avoid exceeding the repeat limit\n result.append(chr(smaller + ord(\'a\')))\n freq[smaller] -= 1\n\n return \'\'.join(result)\n\n```\n\n\nLeetCode 2182 Construct String With Repeat Limit | HashTable | Greedy | Asked in Microsoft\nhttps://youtu.be/19hUSyLOZag | 2 | 0 | ['Hash Table', 'String', 'Greedy', 'Java'] | 0 |
construct-string-with-repeat-limit | Simple Solution using PriorityQueue. | simple-solution-using-priorityqueue-by-s-6tfb | IntuitionThe problem involves creating a lexicographically largest string with a given constraint: no character should repeat more than repeatLimit times consec | surya04082004 | NORMAL | 2024-12-17T10:56:05.719785+00:00 | 2024-12-17T10:56:05.719785+00:00 | 8 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem involves creating a lexicographically largest string with a given constraint: no character should repeat more than `repeatLimit` times consecutively. This requires:\n\n- Tracking the frequency of each character.\n- Using a priority mechanism to always pick the largest valid character available while adhering to the repeat limit.\n\nThe approach leverages a **max-heap (PriorityQueue)** to maintain the order of characters by their frequency and lexicographical value. By repeatedly processing the most frequent characters and switching to the next available character when the limit is reached, the constraints are satisfied efficiently.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. **Frequency Count:**\nCreate a frequency array for all characters in the input string s.\n\n2. **Max-Heap Initialization:**\nPopulate a max-heap (priority queue) where each element is a Pair containing the character and its frequency. The heap orders characters by their lexicographical order and frequency (highest first).\n\n3. **String Construction:**\n- Extract the most frequent character from the heap and append it to the result string up to repeatLimit times.\n- If the character\'s frequency exceeds repeatLimit, place it back in the heap after reducing its frequency.\n- If the limit is reached, append the next largest character available in the heap to avoid consecutive repetition.\n- Repeat until the heap is empty.\n\n4. **Handle Remaining Characters:**\nAny character with leftover frequency is re-added to the heap to be processed in subsequent iterations.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n- - Constructing the frequency array: \uD835\uDC42(\uD835\uDC5B), where \uD835\uDC5B is the length of the input string `s`.\n- - Initializing the max-heap: \uD835\uDC42(\uD835\uDC58log\uD835\uDC58), where \uD835\uDC58 is the number of unique characters (at most 26 for lowercase English letters).\n- - Processing the heap: \uD835\uDC42(\uD835\uDC5Blog\uD835\uDC58), since each character insertion/removal from the heap is \uD835\uDC42(log\uD835\uDC58), and we process each character in the string.\n- - Overall: \uD835\uDC42(\uD835\uDC5Blog\uD835\uDC58), which simplifies to \uD835\uDC42(\uD835\uDC5B) for this problem as \uD835\uDC58 is a small constant (26).\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n- - Frequency array: \uD835\uDC42(26)=\uD835\uDC42(1).\n- - Max-heap storage: \uD835\uDC42(\uD835\uDC58), where \uD835\uDC58\u226426.\n- - Result string: \uD835\uDC42(\uD835\uDC5B).\n- - Overall: \uD835\uDC42(\uD835\uDC5B).\n\n# Code\n```java []\nclass Solution {\n static class Pair implements Comparable<Pair>{\n char ch;\n int freq;\n\n public Pair(char c, int f){\n ch = c;\n freq = f;\n }\n\n @Override\n public int compareTo(Pair b){\n return (int)this.ch - b.ch;\n }\n }\n public String repeatLimitedString(String s, int repeatLimit) {\n int[] freq = new int[26];\n for(char c : s.toCharArray()){\n freq[c - \'a\']++;\n }\n StringBuilder sb = new StringBuilder();\n PriorityQueue<Pair> pq = new PriorityQueue<>(Collections.reverseOrder());\n for(int i=0; i<26; i++){\n if(freq[i] != 0)\n pq.add(new Pair((char)(i+\'a\'), freq[i]));\n }\n while(!pq.isEmpty()){\n Pair p = pq.poll();\n int count = Math.min(p.freq, repeatLimit);\n sb.append(String.valueOf(p.ch).repeat(count));\n p.freq -= count;\n if(p.freq > 0){\n if(!pq.isEmpty()){\n Pair p2 = pq.poll();\n sb.append(p2.ch);\n p2.freq--;\n if(p2.freq > 0)\n pq.add(p2);\n pq.add(p);\n }\n }\n }\n return sb.toString();\n\n }\n}\n``` | 2 | 0 | ['Java'] | 0 |
construct-string-with-repeat-limit | Kotlin | Rust | kotlin-rust-by-samoylenkodmitry-8frw | Join me on Telegramhttps://t.me/leetcode_daily_unstoppable/835Problem TLDRMax lexical ordered with repeat_limit string #medium #bucket_sortIntuitionAlways peek | SamoylenkoDmitry | NORMAL | 2024-12-17T08:58:23.791982+00:00 | 2024-12-17T08:58:34.082537+00:00 | 94 | false | 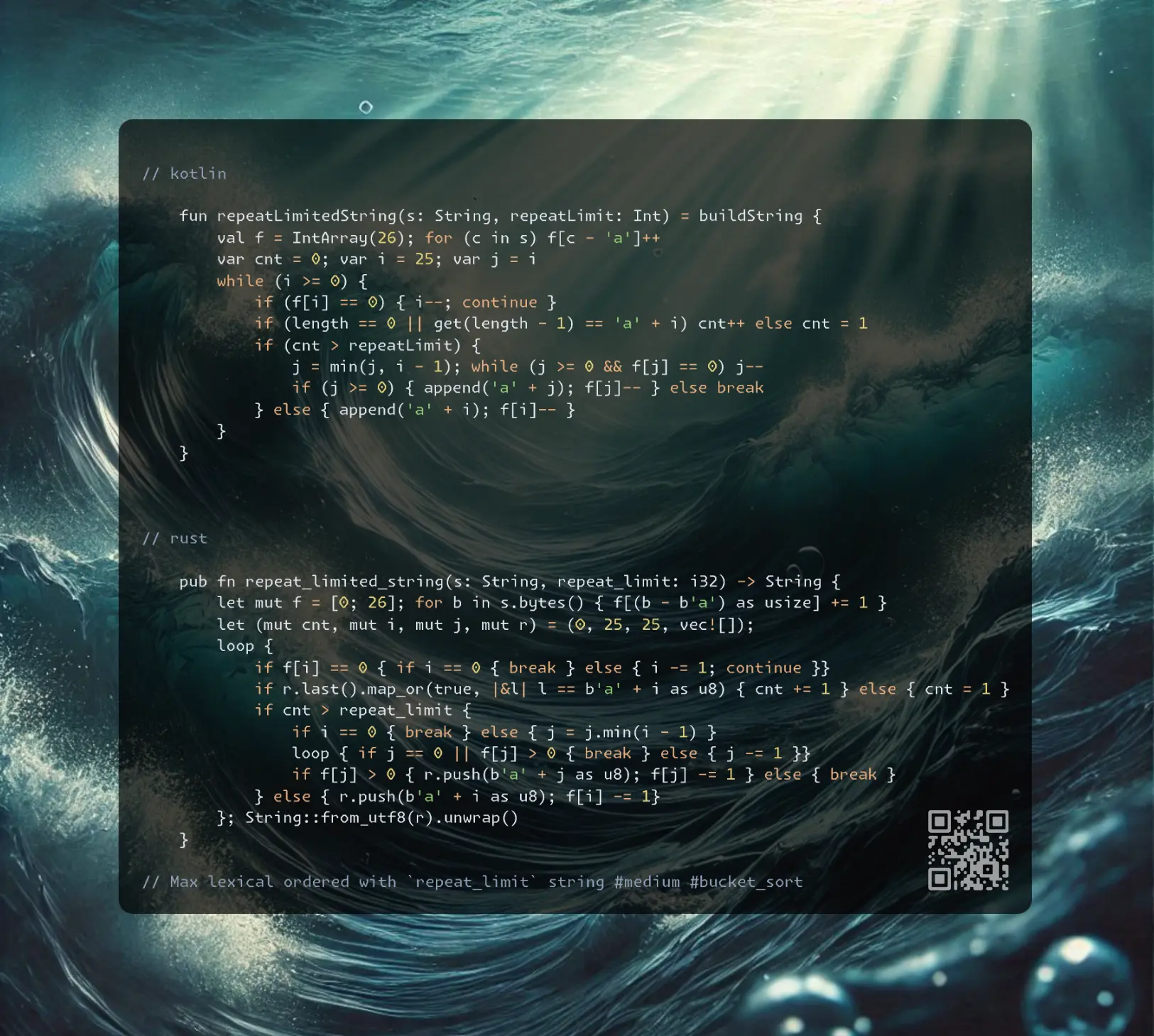\n\nhttps://youtu.be/tGaNBtqpNec\n\n#### Join me on Telegram\n\nhttps://t.me/leetcode_daily_unstoppable/835\n\n#### Problem TLDR\n\nMax lexical ordered with `repeat_limit` string #medium #bucket_sort\n\n#### Intuition\n\nAlways peek the largest value. If limit is reached peek one of the next.\n\n#### Approach\n\n* we can use a heap, but have to manage all the next chars at once\n* we can use a frequency counter and two pointers: current and next \n\n#### Complexity\n\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(n)$$\n\n#### Code\n\n```kotlin []\n\n fun repeatLimitedString(s: String, repeatLimit: Int) = buildString {\n val f = IntArray(26); for (c in s) f[c - \'a\']++\n var cnt = 0; var i = 25; var j = i\n while (i >= 0) {\n if (f[i] == 0) { i--; continue }\n if (length == 0 || get(length - 1) == \'a\' + i) cnt++ else cnt = 1\n if (cnt > repeatLimit) {\n j = min(j, i - 1); while (j >= 0 && f[j] == 0) j--\n if (j >= 0) { append(\'a\' + j); f[j]-- } else break\n } else { append(\'a\' + i); f[i]-- }\n }\n }\n\n```\n```rust []\n\n pub fn repeat_limited_string(s: String, repeat_limit: i32) -> String {\n let mut f = [0; 26]; for b in s.bytes() { f[(b - b\'a\') as usize] += 1 }\n let (mut cnt, mut i, mut j, mut r) = (0, 25, 25, vec![]);\n loop {\n if f[i] == 0 { if i == 0 { break } else { i -= 1; continue }}\n if r.last().map_or(true, |&l| l == b\'a\' + i as u8) { cnt += 1 } else { cnt = 1 }\n if cnt > repeat_limit {\n if i == 0 { break } else { j = j.min(i - 1) }\n loop { if j == 0 || f[j] > 0 { break } else { j -= 1 }}\n if f[j] > 0 { r.push(b\'a\' + j as u8); f[j] -= 1 } else { break }\n } else { r.push(b\'a\' + i as u8); f[i] -= 1}\n }; String::from_utf8(r).unwrap()\n }\n\n```\n```c++ []\n\n string repeatLimitedString(string s, int repeatLimit) {\n int f[26]; for (auto c: s) ++f[c - \'a\'];\n int cnt = 0, i = 25, j = 25, l = 26; string r;\n while (i >= 0) {\n if (!f[i]) { --i; continue; }\n l == i ? ++cnt : cnt = 1;\n if (cnt > repeatLimit) {\n j = min(j, i - 1);\n while (j > 0 && !f[j]) --j;\n if (j >= 0 && f[j]--) { r += \'a\' + j; l = j; } else break;\n } else { r += \'a\' + i; --f[i]; l = i; }\n } return r;\n }\n\n``` | 2 | 0 | ['Bucket Sort', 'C++', 'Rust', 'Kotlin'] | 1 |
construct-string-with-repeat-limit | Very Easy Detailed Solution ๐ฅ || ๐ฏ Greedy Algorithm | very-easy-detailed-solution-greedy-algor-q1rc | IntuitionWe will make a frequency array to track the frequency of each character in the string, and will iterate from last in order the maintain the lexicograph | chaturvedialok44 | NORMAL | 2024-12-17T08:14:34.054936+00:00 | 2024-12-17T08:14:34.054936+00:00 | 81 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe will make a frequency array to track the frequency of each character in the string, and will iterate from last in order the maintain the lexicographical order. We will append the char untill reached the limit of its frequency gets 0. If we sort by limit we will check for closest smaller char and will add one of that, acting as a separator.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. **Frequency Calculation:**\n - The frequency of each character in the string s is calculated and stored in an array freq[] of size 26.\n - freq[i] represents the count of character (char)(\'a\' + i).\n\n2. **Greedy Approach with Lexicographical Order:**\n - The algorithm processes characters in descending lexicographical order (from \'z\' to \'a\').\n - For each character:\n - Append the character to the result string at most repeatLimit times (using min(freq[i], repeatLimit)).\n - Decrement its frequency after appending.\n - If a character still has remaining frequency but cannot be added due to the repeatLimit, insert a smaller lexicographical character that still has frequency left:\n - Find the next valid character (j < i) with a non-zero frequency.\n - Append this smaller character to the result.\n - Decrement its frequency.\n - To ensure the larger character (i) can resume appending later, increment i (effectively reprocessing it).\n\n3. **Building the Result:**\n - Use a StringBuilder to efficiently append characters and build the result string.\n\n4. **Termination:**\n - The loop continues until all characters have been processed and their frequencies reach zero.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$O(n)$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$O(n)$\n# Code\n```java []\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int n = s.length();\n StringBuilder sb = new StringBuilder();\n\n int freq[] = new int[26];\n for(char ch : s.toCharArray()){\n freq[ch - \'a\']++;\n }\n\n for(int i=25; i>=0; i--){\n if(freq[i] == 0) continue;\n\n int minAppend = Math.min(freq[i], repeatLimit);\n freq[i] -= minAppend;\n while(minAppend > 0){\n sb.append((char)(\'a\' + i));\n minAppend--;\n }\n\n if(freq[i] != 0){\n for(int j=i-1; j>=0; j--){\n if(freq[j] == 0) continue;\n sb.append((char)(\'a\' + j));\n freq[j]--;\n i++;\n break;\n }\n }\n }\n return sb.toString();\n }\n}\n``` | 2 | 0 | ['Array', 'String', 'Greedy', 'Counting', 'Java'] | 2 |
construct-string-with-repeat-limit | Beginner Friendly || C++ || Priority Queue :) | beginner-friendly-c-priority-queue-by-ma-dy8r | Count Character Frequencies:
Use an unordered map mp to store the frequency of each character in the string s.
Max Heap (Priority Queue):
Push all characters an | mayankn31 | NORMAL | 2024-12-17T07:52:44.814648+00:00 | 2024-12-17T07:52:44.814648+00:00 | 95 | false | **Count Character Frequencies:**\n- Use an unordered map mp to store the frequency of each character in the string s.\n\n---\n\n\n**Max Heap (Priority Queue):**\n- Push all characters and their frequencies into a max heap (priority queue), sorting by character value. (lexographical)\n\n---\n\n\n**Construct Result:**\n\n- While the heap is not empty, pick the top character with the highest value.\n- Append it to the result string repeatLimit times (or until its frequency runs out).\n- If the frequency remains and another character exists in the heap, pick the next highest character, append it once, and push back both updated counts into the heap.\n\n---\n\n\n**Handle Repeat Limit:**\n- The logic ensures no character repeats more than repeatLimit times consecutively by switching to the second character when needed.\n\n---\n\n\n**Return Result:**\n- The final string is returned once all characters are processed.\n\n# Code\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n\n unordered_map<char,int>mp;\n for(char x: s) mp[x]++;\n\n priority_queue<pair<char,int>>q;\n for(auto x:mp) q.push({x.first,x.second});\n \n string result="";\n\n while(!q.empty()){\n auto x =q.top();\n q.pop();\n char c=x.first;\n int cnt=x.second;\n int limit=repeatLimit;\n while(limit--){\n result+=c;\n cnt--;\n if(cnt==0) break;\n }\n if(q.empty()) return result;\n if(cnt>0){\n auto y =q.top();\n q.pop();\n char d=y.first;\n int cn=y.second;\n result+=d;\n if(cn-1>0)q.push({d,cn-1});\n q.push({c,cnt});\n }\n }\n return result;\n }\n};\n``` | 2 | 0 | ['Hash Table', 'String', 'Heap (Priority Queue)', 'Counting', 'C++'] | 0 |
construct-string-with-repeat-limit | O(n) two pointer approach in Go | on-two-pointer-approach-in-go-by-zhengha-795v | IntuitionUse two pointers to track the current lex max rune and the next one, if the current one reaches repeatLimit, append the next one, in a loopApproachUse | zhenghaozhao | NORMAL | 2024-12-17T07:35:37.113026+00:00 | 2024-12-17T07:35:37.113026+00:00 | 140 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nUse two pointers to track the current lex max rune and the next one, if the current one reaches repeatLimit, append the next one, in a loop\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nUse strings.Repeat for constructing strings consist of the same character in constant time.\nUse strings.Builder for constant time string construction.\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```golang []\nfunc repeatLimitedString(s string, repeatLimit int) string {\n counts := make([]int, 26)\n\n for _, c := range s {\n counts[int(c-\'a\')]++\n }\n\n i, j := 25, 25\n var sb strings.Builder\n\n for j >= 0 {\n if i == j || (i >= 0 && counts[i] == 0) {\n i--\n continue\n }\n\n if counts[j] == 0 {\n j--\n continue\n }\n\n count := min(repeatLimit, counts[j])\n counts[j] -= count\n sb.WriteString(strings.Repeat(string(\'a\' + j), count))\n\n if i < 0 {\n break\n }\n\n if counts[j] > 0 {\n sb.WriteRune(rune(\'a\' + i))\n counts[i]--\n } else {\n j = i\n }\n }\n\n return sb.String()\n}\n``` | 2 | 0 | ['Go'] | 0 |
construct-string-with-repeat-limit | Swift๐ฏ | swift-by-upvotethispls-f4fs | Heap of Character Frequencies (accepted answer) | UpvoteThisPls | NORMAL | 2024-12-17T07:24:08.721196+00:00 | 2024-12-17T07:24:08.721196+00:00 | 52 | false | **Heap of Character Frequencies (accepted answer)**\n```\nimport Collections\nstruct HeapItem: Comparable {\n var ch: Character, count:Int\n init(_ a:Character, _ b:Int) {ch=a;count=b}\n static func <(l:Self, r:Self) -> Bool { l.ch < r.ch }\n}\nclass Solution {\n func repeatLimitedString(_ s: String, _ repeatLimit: Int) -> String {\n let freq = Dictionary(zip(s,repeatElement(1,count:s.count)), uniquingKeysWith: +)\n var heap = Heap(freq.map(HeapItem.init))\n var result = ""\n \n while var item = heap.popMax() {\n while true {\n result += String(repeating: item.ch, count: min(item.count, repeatLimit))\n item.count -= repeatLimit\n guard item.count > 0, let next = heap.popMax() else { break }\n result.append(next.ch) // poke one char from next largest character\n if next.count > 1 {\n heap.insert(HeapItem(next.ch, next.count-1))\n }\n }\n }\n return result\n }\n}\n``` | 2 | 0 | ['Swift'] | 0 |
construct-string-with-repeat-limit | C++ easy to understand solution | c-easy-to-understand-solution-by-dg15060-x2px | IntuitionWe need to build the lexicographically largest string with the repeatLimit number of occurences of a character at any time. The largest character shoul | dg150602 | NORMAL | 2024-12-17T07:19:51.753927+00:00 | 2024-12-17T07:19:51.753927+00:00 | 28 | false | # Intuition\nWe need to build the `lexicographically largest` string with the `repeatLimit` number of occurences of a character at any time. The largest character should come first. The trick is to keep the repetition of a particular character `rep` in `s` as `rep <= repeatLimit`\n\n# Approach\nWe store the occurences of all characters in the string in a hashmap. The character repetitions can either be smaller, equal or greater than the `repeatLimit`, so we need to check that first. If the frequency `rep` is smaller or equal, we can easily construct our string. Otherwise we search an immediate smaller character `temp`, add it in the middle and continue. As the `answer` doesn\'t need to contain all the characters of the original string `s`, we can continue with the next character if we don\'t find an immediate smaller character.\n\n# Complexity\n- Time complexity:\nO(N)\n\n- Space complexity:\nO(N)\n\n# Code\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n string ans = "";\n map<char,int> mp;\n for(char ch : s){\n mp[ch]++;\n }\n for(char ch = \'z\'; ch >= \'a\'; ch--){\n if(mp.find(ch) == mp.end()) continue;\n int rep = mp[ch];\n if(rep <= repeatLimit){\n for(int i = 0; i < rep; i++){\n ans += ch;\n }\n mp.erase(ch);\n }\n else{\n start:\n for(int i = 0; i < min(rep,repeatLimit); i++){\n ans += ch;\n }\n rep -= repeatLimit;\n if(rep > 0){\n char temp = ch;\n temp--;\n while(temp >= \'a\'){\n if(mp.find(temp) != mp.end()){\n ans += temp;\n mp[temp]--;\n if(mp[temp] == 0)\n mp.erase(temp);\n goto start;\n }\n temp--;\n }\n }\n }\n }\n return ans;\n }\n};\n``` | 2 | 0 | ['Hash Table', 'String', 'Counting', 'Iterator', 'C++'] | 0 |
construct-string-with-repeat-limit | JAVA | Simple Solution | Frequency Table | java-simple-solution-frequency-table-by-lex3l | Code | SudhikshaGhanathe | NORMAL | 2024-12-17T07:12:17.183854+00:00 | 2024-12-17T07:12:17.183854+00:00 | 131 | false | \n# Code\n```java []\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int [] vocab = new int[26]; //frequency table\n for (char ch : s.toCharArray()) vocab[ch - \'a\']++;\n StringBuilder sb = new StringBuilder();\n int idx = 25;\n while (idx >= 0) {\n if (vocab[idx] == 0) {\n idx--;\n continue;\n }\n int count = Math.min(vocab[idx], repeatLimit);\n for (int i = 0 ; i < count ; i++) sb.append((char)(idx + \'a\'));\n vocab[idx] -= count;\n if (vocab[idx] > 0) { //if there exists more characters than \'repeatLimit\'\n int next = idx - 1;\n while (next >= 0 && vocab[next] == 0) next--; //retriving the next largest idx \n if (next < 0) break;\n sb.append((char) (next + \'a\')); //adding 1 character from the next largest idx\n vocab[next]--;\n }\n }\n return sb.toString();\n }\n}\n``` | 2 | 0 | ['Hash Table', 'String', 'Counting', 'Java'] | 0 |
construct-string-with-repeat-limit | 2 solutions [array] + [maxHeap] | 2-solutions-array-maxheap-by-ajay_74-4ohc | Code | Ajay_74 | NORMAL | 2024-12-17T07:07:59.535810+00:00 | 2024-12-17T07:07:59.535810+00:00 | 109 | false | \n# Code\n```golang [Using Array]\nfunc repeatLimitedString(s string, repeatLimit int) string {\n\n\tfreq := make([]int, 26)\n\n\tfor i := 0; i < len(s); i++ {\n\t\tfreq[s[i]-\'a\']++\n\t}\n\n\tindex := 25\n\tresult := []byte{}\n\n\tfor index >= 0 {\n\t\tif freq[index] == 0 {\n\t\t\tindex--\n\t\t\tcontinue\n\t\t}\n\n\t\tk := min(repeatLimit, freq[index])\n\n\t\tfor i := 0; i < k; i++ {\n\t\t\tresult = append(result, byte(index+\'a\'))\n\t\t}\n\n\t\tfreq[index] -= k\n\t\tlettersLeft := freq[index]\n\t\tif lettersLeft > 0 {\n\t\t\tprevIndex := index - 1\n\n\t\t\tfor prevIndex >= 0 && freq[prevIndex] == 0 {\n\t\t\t\tprevIndex--\n\t\t\t}\n\n\t\t\tif prevIndex == -1 {\n\t\t\t\tbreak\n\t\t\t}\n\t\t\tresult = append(result, byte(prevIndex+\'a\'))\n\t\t\tfreq[prevIndex]--\n\t\t}\n\n\t}\n\n\treturn string(result)\n}\n\n```\n\n```golang [Using MaxHeap]\ntype pair struct {\n\tchar byte\n\tcount int\n}\n\ntype maxHeap []pair\n\nfunc (h maxHeap) Len() int { return len(h) }\nfunc (h maxHeap) Less(i, j int) bool { return h[i].char > h[j].char }\nfunc (h maxHeap) Swap(i, j int) { h[i], h[j] = h[j], h[i] }\n\nfunc (h *maxHeap) Pop() any {\n\told := *h\n\tn := len(old)\n\titem := old[n-1]\n\t*h = old[:n-1]\n\treturn item\n}\n\nfunc (h *maxHeap) Push(x any) {\n\t*h = append(*h, x.(pair))\n}\n\nfunc repeatLimitedString(s string, repeatLimit int) string {\n\tresult := []byte{}\n\tfreq := map[byte]int{}\n\n\tfor i := 0; i < len(s); i++ {\n\t\tfreq[s[i]]++\n\t}\n\n\th := maxHeap{}\n\n\tfor char, count := range freq {\n\t\th = append(h, pair{char: char, count: count})\n\t}\n\n\theap.Init(&h)\n\n\tfor len(h) > 0 {\n\t\tcurrentPair := heap.Pop(&h).(pair)\n\n\t\tk := min(currentPair.count, repeatLimit)\n\n\t\tfor i := 0; i < k; i++ {\n\t\t\tresult = append(result, currentPair.char)\n\t\t}\n\t\tcurrentPair.count -= k\n\n\t\tif currentPair.count > 0 {\n if len(h)==0{\n break\n }\n\t\t\t//add a separator\n\t\t\tnextPair := heap.Pop(&h).(pair)\n\t\t\tresult = append(result, nextPair.char)\n\t\t\tnextPair.count--\n if nextPair.count>0{\n heap.Push(&h, nextPair)\n }\n\t\t\theap.Push(&h, currentPair)\n\t\t}\n\t}\n\n\treturn string(result)\n}\n\n``` | 2 | 0 | ['Go'] | 1 |
construct-string-with-repeat-limit | Intuition, Approach and Solution using a Priority Queue in C++(Easy to Understand,Straightforward A) | intuition-approach-and-solution-using-a-cr9p8 | IntuitionAs it said lexicographically largest my main idea was to solve it using a max heap i.e. a priority queue.(As it will order the characters lexicographic | japitSaikap302 | NORMAL | 2024-12-17T06:16:59.045806+00:00 | 2024-12-17T06:16:59.045806+00:00 | 45 | false | # Intuition\nAs it said **lexicographically** largest my main idea was to solve it using a max heap i.e. a priority queue.(As it will order the characters lexicographical descending order and make it easy for me to get the correct order for the largest character)\n\n---\n\n\n# Approach\n1. Character Frequency Count\nUse a unordered map to count the frequency of each character in the string.\n2. **Use a Max-Heap (Priority Queue)**\nThe priority queue is used to process characters in lexicographically descending order.\n- Example if a test string is cczazcc\n- Then in the max-heap it would be zzccccaa (descending order)\nPush each character and its frequency into the priority queue.\n3. Build the Result String (Final Ans)\nRepeatedly:\nTake the largest character (top of the heap) and append it to the result up to repeatLimit times.\nIf the character still has remaining frequency(count):\nUse the next largest character (next top of the heap) as a **"Divider/Border"** to satisfy the repeat limit constraint(i.e. the repeatLimit).\nPush both characters (if they have remaining frequency) back into the queue(For updating the queue).\n4. Stop When No Characters Are Left\nIf the queue becomes empty and there\u2019s no character to act as a breaker, stop the process\n\n---\n\n\n# Complexity\n- Time complexity:\nTime Complexity:\nPriority Queue Operations: Each insertion and removal from the priority queue takes **\uD835\uDC42(logn)**, where n is the number of unique characters.\nString Construction: In the worst case, each character is processed multiple times (inserted back into the queue), leading to a total time complexity of **O(klogn)** where k is the length of the string.\n\n- Space complexity:\nThe space complexity is **O(n)** where n is the no of distinct characters in the string its same for both priority queue and the unordered map as both at most store the **n unique no. of characters**\n\n# Code\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n int len = s.length();\n string result="";\n\n //a map is used to count the frequency of each character\n unordered_map<char, int> mpp;\n for (char c : s) {\n mpp[c]++;\n }\n \n // a max-heap so that the largest character is processed first\n priority_queue<pair<char, int>> pq;\n for (auto it : mpp) {\n pq.push({it.first, it.second});\n }\n \n while (!pq.empty()) {\n auto [ch, count] = pq.top();\n pq.pop();\n \n int k = min(repeatLimit, count);\n result.append(k, ch);\n count -= k;\n\n if (count > 0) {\n \n if (pq.empty()) break;\n\n auto [nextCh, nextCount] = pq.top();\n pq.pop();\n\n result.push_back(nextCh);\n nextCount--;\n\n pq.push({ch, count});\n if (nextCount > 0) pq.push({nextCh, nextCount});\n }\n }\n\n return result;\n }\n};\n``` | 2 | 0 | ['Hash Table', 'String', 'Heap (Priority Queue)', 'C++'] | 0 |
construct-string-with-repeat-limit | Best solution so far without heap | best-solution-so-far-without-heap-by-rik-vn7m | Intuition: Use Freq array from 26 - 1, if freq > limit then simply grab one next char and come back to prev charComplexity
Time complexity: O(n * Min(freq, limi | rikam | NORMAL | 2024-12-17T05:32:11.676676+00:00 | 2024-12-17T05:32:11.676676+00:00 | 46 | false | # Intuition: Use Freq array from 26 - 1, if freq > limit then simply grab one next char and come back to prev char\n\n\n# Complexity\n- Time complexity: O(n * Min(freq, limit))\n- Space complexity: O(n)\n\n# Code\n```csharp []\npublic class Solution {\n public string RepeatLimitedString(string s, int repeatLimit) {\n int[] freq = new int[26];\n for(int i=0; i<s.Length; i++)\n {\n freq[s[i] - \'a\']++;\n }\n StringBuilder sb = new();\n for(int i=25; i>=0; i--)\n {\n int pos = i;\n int limit = Math.Min(freq[pos], repeatLimit);\n for(int j=0; j<limit; j++)\n {\n sb.Append((char)((pos) + \'a\'));\n freq[pos]--;\n }\n if(freq[pos] >= 1)\n { \n pos--;\n while (pos >= 0 && freq[pos] == 0) \n {\n pos--;\n }\n if (pos < 0) break; \n sb.Append((char)((pos) + \'a\'));\n freq[pos]--;\n i++;\n }\n }\n return sb.ToString();\n }\n}\n``` | 2 | 0 | ['Iterator', 'C#'] | 0 |
construct-string-with-repeat-limit | Easy and Simple Greedy Solution with Linear Time | easy-and-simple-greedy-solution-with-lin-61c7 | SolutionApproach:
Frequency Count:
Use an array hash of size 26 to store the frequency of each letter ('a' to 'z').
Greedy Selection:
Select the largest a | pavansai11 | NORMAL | 2024-12-17T04:55:13.412015+00:00 | 2024-12-17T04:55:13.412015+00:00 | 104 | false | # Solution\n\n## Approach:\n1. **Frequency Count**:\n - Use an array `hash` of size 26 to store the frequency of each letter (\'a\' to \'z\').\n\n2. **Greedy Selection**:\n - Select the largest available character (from \'z\' to \'a\') that satisfies the repeat constraint.\n\n3. **Helper Function**:\n - `getLastChar`: Finds the largest character index that is available in the `hash` array, skipping the last appended character if specified.\n\n4. **Significance of `end_char`**:\n - `end_char` is used to identify the largest available character, ignoring any restrictions on the `last_vis` (last appended character).\n - If the `last_vis` character still has occurrences left but is not the current `end_char`, it helps alternate between characters, ensuring that the result string satisfies the lexicographical order while adhering to the repeat constraints.\n\n5. **Iterate Until All Characters Are Used**:\n - Repeatedly append characters to the result string until no valid characters remain.\n - Append up to `repeatLimit` instances of the largest character. If constraints prevent appending further, switch to the next largest valid character.\n\n---\n\n## Code\n\n```cpp\nclass Solution {\n int getLastChar(const vector<int>& hash, int last_vis) {\n for (int i = 25; i >= 0; i--) \n if (i != last_vis && hash[i] > 0)\n return i;\n return -1;\n }\n\npublic:\n string repeatLimitedString(string s, int lim) {\n vector<int> hash(26, 0); // Frequency array for \'a\' to \'z\'\n string res;\n\n for (char ch : s) \n hash[ch - \'a\']++;\n\n int last_vis = -1; // Last appended character index\n\n while (true) {\n int last_char = getLastChar(hash, last_vis);\n if (last_char == -1) break; // No valid character left\n\n\n int end_char = getLastChar(hash, -1);\n\n // If we can alternate with another character\n if (last_vis != -1 && hash[last_vis] > 0 && last_char != end_char) {\n res.push_back(\'a\' + last_char);\n hash[last_char]--;\n last_vis = last_char;\n continue;\n }\n\n // Append up to `lim` instances of the largest character\n int freq = min(hash[last_char], lim);\n res.append(freq, \'a\' + last_char);\n hash[last_char] -= freq;\n last_vis = last_char;\n }\n\n return res;\n }\n};\n | 2 | 0 | ['String', 'Greedy', 'Counting', 'C++'] | 0 |
construct-string-with-repeat-limit | Efficient Max-heap solution || Easy to understand | efficient-max-heap-solution-easy-to-unde-kzhx | IntuitionThe problem asks us to construct the lexicographically largest string from the characters in s such that no character repeats more than repeatLimit tim | _adarsh_01 | NORMAL | 2024-12-17T04:31:25.811576+00:00 | 2024-12-17T04:31:25.811576+00:00 | 17 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\nThe problem asks us to construct the lexicographically largest string from the characters in s such that no character repeats more than `repeatLimit` times consecutively. This involves:\n\n 1. Using a greedy approach to select the lexicographically largest characters.\n 2. Carefully handling the repeat limit to avoid violating the constraints while maximizing the lexicographical order.\n\n\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n**1. Frequency Map and Priority Queue:**\n- First, we create a frequency map (`mp`) to count the occurrences of each character in `s`.\n- Use a max-heap (priority queue) to keep track of characters by their lexicographical order (largest characters first).\n\n**2. Building the Result:**\n- Start with an empty result string `res`.\n- While the priority queue is not empty, extract the character with the highest lexicographical order (`it`).\n- If the last character in the result string is not the same as the current character:\n - Append as many of the current character as possible, up to repeatLimit. If there are remaining occurrences, push the character back into the heap with its updated count.\n- If the last character in the result string is the same as the current character:\n - Check if the second-largest character is available in the priority queue.\n - Use that character once to break the sequence of repeated characters.\n - If no other character is available, stop (as no valid sequence can be constructed).\n\n**3. Priority Queue Updates:**\n- Whenever a character\'s count is reduced and remains non-zero, push it back into the heap with its updated count.\n\n\n\n\n\n\n# Complexity\n- **Time complexity:**\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n1. **Building the Frequency Map:** $$O(n)$$ , where `n` is the length of the string `s`.\n2. **Heap Operations:** Each character can be inserted or extracted from the heap at most once, and heap operations take $$O(log k)$$, where *k* is the number of unique characters in `s`. This results in \n$$O(klogk)$$.\n3. **Appending Characters:** Constructing the result string involves iterating through all characters of `s`, taking $$O(n)$$.\n\nOverall, the time complexity is $$O(n+klogk)$$, where k<=26 .\n\n\n\n\n\n- **Space complexity:**\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n1. **Frequency Map and Priority Queue:** Both require $$O(k)$$ space, where k<=26.\n2. **Result String:** Requires $$O(n)$$ space to store the final result.\n\n\n# Code\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n map<char,int>mp;\n priority_queue<pair<char,int>>pq;\n string res="";\n for(int i=0;i<s.size();i++){\n mp[s[i]]++;\n }\n for(auto it:mp){\n pq.push({it.first,it.second});\n }\n while(!pq.empty()){\n auto it=pq.top();\n pq.pop();\n char c=it.first;\n int cnt=it.second;\n\n if(res.empty() or res.back()!=c){\n if(cnt<=repeatLimit){\n while(cnt--){\n res+=c;\n }\n }else{\n int temp=repeatLimit;\n cnt-=repeatLimit;\n while(temp--){\n res+=c;\n }\n pq.push({c,cnt});\n }\n }else{\n if(pq.empty())break;\n char c2=pq.top().first;\n int cnt2=pq.top().second;\n pq.pop();\n res+=c2;\n cnt2--;\n if(cnt2>0)pq.push({c2,cnt2});\n pq.push({c,cnt});\n }\n }\n return res;\n }\n};\n``` | 2 | 0 | ['Hash Table', 'String', 'Greedy', 'Heap (Priority Queue)', 'C++'] | 0 |
construct-string-with-repeat-limit | โ
| Beats 95% | O(N * 26) time | O(26) Space | Greedy Strategy | beats-95-on-26-time-o26-space-greedy-str-imo4 | IntuitionFor Making a string Lexicographically largest, we will follow a Greedy Strategy
For making string lexicographically largest, always put largest alphabe | fake_name | NORMAL | 2024-12-17T04:12:50.894557+00:00 | 2024-12-17T05:42:33.733065+00:00 | 97 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFor Making a string Lexicographically largest, we will follow a **Greedy Strategy**\n> For making string lexicographically largest, always put largest alphabet in the begining (if possible), otherwise, find the next largest alphabet. \n\n---\n\n\nNow the case will come when number alphabets will exceed the repeatLimit. In that case, we will look for **next largest element** available, **take its only one occurance**, and then again continue with the largest element. \n> Ex.\n*s = "aaabbbccc*\n*repeatLimit = 2*\n*Here, first select 2 **c** --> cc*\n*Then look for next largest element, **b**. Since all **c** are not used yet, take only one **b** -> ccb*\n*Now, again take **c** --> ccbc*\n*Since **c** is exhausted, largest alphabet now is **b** --> ccbcbb*\n*Now **b** is also exhasted, take **a** --> ccbcbbaa*\n*We can only take 2 **a** otherwise it will exceed repeatLimit*\n\nWe are also making sure, if some alphabets are wasted, (in above example **a**), it should be the smallest alphabet. \n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n- Store frequency all the alphabets. \n- Greedily pick the largest alphabet, and take **atmost** **repeatLimit** occrances of it. Append it to answer string. \n- Now if all occurances are exhausted, simply move to next largest alphabet and repeat the process. Otherwise, take only one occurance of next largest alphabet and append it into the answer, and repeat step 2. \n- If next largest element does not exist, end the process as no more alphabets can be appended. \n\n# Complexity\n- Time complexity: O(N * 26)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(26)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string &s, int &repeatLimit) {\n int h[26] = {}; \n for (auto &ch: s) {\n h[ch - \'a\']++; \n }\n string ans; \n for (int i = 25; i >= 0; i--) {\n while (h[i] > 0) {\n int x = min(repeatLimit, h[i]); \n h[i] -= x; \n while (x--) {\n ans += (i + \'a\'); \n }\n if (h[i] == 0) {\n break; \n }\n bool flag = false; \n for (int j = i - 1; j >= 0; j--) {\n if (h[j]) {\n ans += (j + \'a\'); \n h[j]--; \n flag = true; \n break; \n }\n }\n if (!flag) {\n break; \n }\n }\n }\n return ans; \n }\n};\n``` | 2 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'C++'] | 0 |
construct-string-with-repeat-limit | Video | O(n) solution keep count char frequency array, with constant space | keep-count-char-frequency-array-on-solut-qdjj | YouTube solution Intuitionkeep it simple like below steps:-
create an array of 26 size and count the frequency of all characters.
int count[] = new int[26]
w | vinod_aka_veenu | NORMAL | 2024-12-17T02:09:31.448196+00:00 | 2024-12-17T03:34:19.794674+00:00 | 252 | false | # YouTube solution https://youtu.be/LGUrExMrW-c\n# Intuition\nkeep it simple like below steps:- \n\n1) create an array of 26 size and count the frequency of all characters.\nint count[] = new int[26]\n\n2) write a method that will give us next smaller char frequency in count array (remeber we are traversing from right to left **why?** we need lexicographical larger chars) \n\n**s = zzzzaaayy ===> frequency of \'z\' is at index = 25** if i call getNext(count, 25) ===> it will return next smaller index that have non zero frequency ==> **24**for \'y\' frequency.\n\n3) now we will start from **rightmost frequency** and then pick **min(repeatLimit, frequency)** times because we can append repeatLimit if frequency is greater than or equal else we need to print whatever the frequency of char.\n\nupdate the remaing frequency count[curr] -= min(repeatLimit, frequency)\n\nnow we have to two scenerios \n**a) if count[curr]>0 ===>** means we can append only one char from next smaller and get back to print again next set from same frequency.\nb) if count[curr]<=0 ==> means we have to make our curr = index of next smaller char frequency.\n now at any time if we found the there is no more next smaller available we have to break the loop and return the resultant string.\n\n\n# Code\n```java []\nclass Solution {\n public int getNext(int count[], int curr)\n {\n if(curr==0)\n return -1; \n while(curr>0)\n {\n if(count[curr-1]>0)\n return curr-1;\n curr--;\n } \n return -1; \n }\n\n public String repeatLimitedString(String s, int repeatLimit) {\n int count[] = new int[26];\n StringBuilder sb = new StringBuilder();\n for(char ch : s.toCharArray())\n count[ch -\'a\']++;\n\n int curr = getNext(count, 26); \n \n while(curr>=0)\n {\n int freq = Math.min(repeatLimit , count[curr]);\n for (int i = 0; i < freq; i++)\n sb.append((char) (curr + \'a\'));\n count[curr] -= freq;\n if(count[curr]>0)\n { \n int next = getNext(count, curr);\n if(next<0)\n break;\n sb.append((char) (next + \'a\'));\n count[next]--;\n } \n else \n curr = getNext(count, curr); \n }\n\n return sb.toString();\n\n \n }\n}\n``` | 2 | 0 | ['Java'] | 4 |
construct-string-with-repeat-limit | Construct String with Repeat Limit - Easy Explanation - Unique Solution๐๐ | construct-string-with-repeat-limit-easy-ce3xl | IntuitionWe have to reorder the given string into lexicographically longer string so that the number of repeated element in a row should be atmost repeatLimit.H | RAJESWARI_P | NORMAL | 2024-12-17T01:18:01.282792+00:00 | 2024-12-17T01:18:01.282792+00:00 | 67 | false | # Intuition\nWe have to reorder the given string into lexicographically longer string so that the number of repeated element in a row should be atmost repeatLimit.\n\nHence this can be computed by noting frequency of all the characters in the string.\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n\n**Pre-requirements**:\n\nInitialize freq array of size 26 and the datatype int to have the count of each character in the String s.\n\n int[] freq=new int[26];\n\nIterate through all the characters in a String s and increment the freq count by freq[ch-\'a\'].Since each character is at index ch-\'a\'.\n\n for(char ch:s.toCharArray())\n {\n freq[ch-\'a\']++;\n }\n\n**Construct the character array**:\n\nInitialize the character array str to be the length of the string s.\n\n char[] str=new char[s.length()];\n\nInitialize the wriIndex to be 0.Then iterate over all the elements of a frequency array.\n\n int wriIndex=0;\n for(int i=25;i>=0;i--){\n\nIterate through the value of the freq array ,freq[i] times.Construct a string by adding character as (char)(i+\'a\').\n\n for(int j=0;j<freq[i];j++)\n {\n str[wriIndex++]=(char)(i+\'a\'); \n }\n\n**Important Intialization for checking condition**:\n\nInitialize rep to be 0 to keep track of number of repeated characters in a row, cur to hold the character at the 0-th index,and next to be 1.\n\n int rep=0;\n char cur=str[0];\n int next=1;\n\nIterate through all the characters of the character array str.\n\n for (int i = 0; i < str.length; i++) {\n\nIf str[i]==cur, then increment the rep variable and if rep==repeatLimit+1, then also check if the next<i , if so increment next by i+1.\n\n if (str[i] == cur) {\n rep++;\n if (rep == repeatLimit + 1) {\n if (next < i) next = i + 1;\n\nContinuously iterate through till next is less than the str length and str[next]=cur , then increment the next.\n\n while (next < str.length && str[next] >= cur) next++;\n\nIf next equals the length of the character array str, then return the value of string as str from index 0 to i.\n\n if (next == str.length) return String.valueOf(str, 0, i);\n\nSwap str[i] and the character str[next] with the use of tmp character, then in the new iteration-> rep is again set to 0.\n\n char tmp = str[i];\n str[i] = str[next];\n str[next] = tmp;\n rep = 0;\n\nIf the rep is not equal to repeatLimit+1 , then set the cur as str[i] and rep is set as 1.\n\n else {\n cur = str[i];\n rep = 1;\n }\n\n**Returning the value**:\n\nReturn the string by converting the given character array into string by the String.valueOf(str).\n\n return String.valueOf(str);\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- ***Time complexity***: **O(n)**.\n n -> number of characters in the string s.\n\n**Frequency array population**: **O(n)**.\nThe for loop iterates over all characters in the string s.Hence it takes O(n) time.\n\n**Building the str character array**: **O(n)**.\nThe nested loops iterate over the freq array (of size 26) and populate the str array.\n\nIn the worst case, all characters in s are used, so it takes \n**O(n) time**.\n\n**Rearranging character(for loop)**: **O(n)**.\nThe loop processes the str array, which has **O(n) characters**.\n \nThe inner while loop that adjusts next depends on how many times we **skip characters**.\n \nIn the worst case, next traverses the rest of the string, but since characters are only skipped once, the total work is **O(n) time**.\n\n**Total Time Complexity**: **O(n)**.\n\nOverall Time Complexity is the time complexity due to all **3 loops**,hence it\'s time complexity is **O(n)+O(n)+O(n) = O(n) time**.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- ***Space complexity***: **O(n)**.\n n -> number of characters in the string s.\n\n**Frequency array (freq)**: **O(1)**.\n It stores fixed size of **26 characters** and hence it occupies only **constant space, O(1)**.\n\n**Character Array(str)**: **O(n)**.\nThe space occupied by the character array is proportional to the number of characters in the string s.Hence it takes **O(n) space**.\n\n**Total Space Complexity**: **O(1)**.\nHence the overall space complexity is dominated by the character array, **str**. Hence it takes **O(n) space**.\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```java []\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n //repeatLimitedString using chars of s \n // no letter appears more than repeatLimit times in a row\n //do not have to use all the characters from s\n int[] freq=new int[26];\n for(char ch:s.toCharArray())\n {\n freq[ch-\'a\']++;\n }\n char[] str=new char[s.length()];\n\n int wriIndex=0;\n for(int i=25;i>=0;i--)\n {\n for(int j=0;j<freq[i];j++)\n {\n str[wriIndex++]=(char)(i+\'a\'); \n }\n }\n int rep=0;\n char cur=str[0];\n int next=1;\n for (int i = 0; i < str.length; i++) {\n if (str[i] == cur) {\n rep++;\n if (rep == repeatLimit + 1) {\n if (next < i) next = i + 1;\n while (next < str.length && str[next] >= cur) next++;\n if (next == str.length) return String.valueOf(str, 0, i);\n char tmp = str[i];\n str[i] = str[next];\n str[next] = tmp;\n rep = 0;\n }\n } else {\n cur = str[i];\n rep = 1;\n }\n }\n return String.valueOf(str);\n }\n}\n``` | 2 | 0 | ['String', 'Counting', 'Java'] | 0 |
construct-string-with-repeat-limit | C++ O(1) space, O(N) time | explanation included | c-o1-space-on-time-explanation-included-zxxz0 | IntuitionWe need to construct a string that is lexicographically the largest and not have characters repeat more then a limit times in a row.ApproachWe will sta | LATUANKHANG | NORMAL | 2024-12-17T01:17:12.815692+00:00 | 2024-12-17T01:17:12.815692+00:00 | 397 | false | # Intuition\nWe need to construct a string that is lexicographically the largest and not have characters repeat more then a limit times in a row.\n\n# Approach\nWe will start with chracter \'z\' and make our way down the alphabet to ensure we arrive at the lexicographically largest string. \nWe first map out how many characters we have to use by iterating over th original string and keeping count of the appearences of the characters.\nWe now have a varible i and tmp for the current largest character we will be using and a backup character for when we reach the repeating limit.\nWe also create a bool \'nomore\' to check if besides our current character (i) is ther another one. Or another way to view it is if there is another characters smaller then i. This is to check if we can still continue after repeating limit amoutn of times.\nSet tmp by going down from 24 (skip 25 for i) to find the first place where cha[tmp] != 0 (means we have a letter we can use)\nWe then while loop i >= 0 then we will try to repeat limit amount of times on our current letter.\n- If we reach the limit then if there is \'nomore\' letters smaller we break (as there is no way to continue and ensure the problems conditions)\n- Else if we ran out of letters at our current position we then set i to tmp and find a new tmp\n- Else if we ran into the limit of repeating letters we then add tmp\'s charater and continue.\n**Make sure to check if tmp\'s character runs out after we add it into our ans string to look for a new one\n\n# Complexity\n- Time complexity:O(N)\n\n- Space complexity:O(1)\n\n# Code\n```cpp []\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n vector<int> cha(26, 0);\n for(int i = 0; i < s.length(); i++){\n cha[s[i]-\'a\']++;\n }\n int i = 25, tmp = i-1;\n bool nomore = false;\n while(cha[tmp] == 0){\n tmp--;\n if(tmp < 0){\n nomore = true;\n break;\n }\n }\n string ans;\n while(i >= 0){\n int k = 0;\n for(; k < repeatLimit; k++){\n if(cha[i] <= 0) break;\n ans += (\'a\'+i);\n cha[i]--;\n }\n if(nomore) break;\n if(cha[i] <= 0){\n i = tmp; tmp--;\n if(tmp < 0){\n nomore = true;\n continue;\n }\n while(cha[tmp] == 0 && !nomore){\n tmp--;\n if(tmp < 0){\n nomore = true;\n break;\n }\n }\n }else if(k == repeatLimit){\n ans += (\'a\' + tmp);\n cha[tmp]--;\n while(cha[tmp] == 0 && !nomore){\n tmp--;\n if(tmp < 0){\n nomore = true;\n break;\n }\n }\n }\n }\n return ans;\n }\n};\n``` | 2 | 0 | ['C++'] | 2 |
construct-string-with-repeat-limit | Sorted Hash Map Python | Beats 99% ๐๐ | sorted-hash-map-python-beats-99-by-rahil-u5gl | IntuitionThe problem requires constructing a string using characters from s such that:
No letter appears more than repeatLimit times consecutively.
The resultin | rahilshah01 | NORMAL | 2024-12-17T01:13:40.352576+00:00 | 2024-12-17T01:13:40.352576+00:00 | 174 | false | ## **Intuition**\nThe problem requires constructing a string using characters from `s` such that:\n1. No letter appears more than `repeatLimit` times consecutively.\n2. The resulting string is lexicographically the largest possible.\n\nThe lexicographical order constraint suggests that we should prioritize using larger characters first (characters later in the alphabet) while ensuring the `repeatLimit` condition is not violated.\n\n---\n\n## **Approach**\n1. **Count Character Frequencies**: \n Use `Counter` to get the frequency of each character in `s`. \n \n2. **Sort Characters**: \n Sort the character-frequency pairs in reverse lexicographical order so that larger characters come first.\n\n3. **Build the Result String**: \n - Start constructing the string by greedily taking as many instances of the largest available character as allowed (up to `repeatLimit`).\n - If the current largest character exceeds `repeatLimit`, insert a single instance of the next largest character to "break" the repetition, and then continue adding the first character.\n - If no second-largest character is available to break the sequence, terminate the process.\n\n4. **Edge Cases**: \n Handle the scenario where a character\'s frequency is exhausted (`pop` it from the list).\n\n---\n\n## **Complexity**\n\n### Time Complexity:\n- **Counting Frequencies**: \\(O(n)\\), where \\(n\\) is the length of `s`.\n- **Sorting Characters**: \\(O(26 \\log 26)\\) \u2192 effectively \\(O(1)\\) since there are at most 26 unique lowercase letters.\n- **Building the Result String**: \\(O(n)\\), as we iterate through all characters to construct the output.\n\nOverall time complexity: **$$ O(n) $$**\n\n\n### Space Complexity:\n- \\(O(26) = O(1)\\) for the `Counter` and sorted list of characters.\n- \\(O(n)\\) for the resulting string `repeatLimitedString`.\n\nTotal space complexity: **$$ O(n) $$**\n\n---\n\n## **Code**\n```python\nfrom collections import Counter\n\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n # Step 1: Count character frequencies and sort by lexicographical order (descending)\n char_freq = [list(i) for i in Counter(s).items()]\n char_freq.sort(reverse=True) # Sort by character (key) in descending order\n \n result = "" # Resultant string\n\n while char_freq:\n if char_freq[0][1] > repeatLimit:\n # Append repeatLimit of the largest available character\n result += char_freq[0][0] * repeatLimit\n char_freq[0][1] -= repeatLimit\n\n # If possible, append the next largest character to "break" the limit\n if len(char_freq) > 1:\n result += char_freq[1][0]\n char_freq[1][1] -= 1\n if char_freq[1][1] == 0:\n char_freq.pop(1) # Remove if exhausted\n else:\n break # No other character to use, terminate\n else:\n # Append all remaining occurrences of the largest available character\n result += char_freq[0][0] * char_freq[0][1]\n char_freq.pop(0) # Remove as frequency is exhausted\n \n return result\n | 2 | 0 | ['Hash Table', 'Greedy', 'Counting', 'Counting Sort', 'Python3'] | 0 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.