question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
construct-string-with-repeat-limit | Pretty Straightforward || Using max heap | pretty-straightforward-using-max-heap-by-1zzp | Intuition\n Describe your first thoughts on how to solve this problem. \nStore the frequency of each character in the map. Push them into the heap pair wise {c | jeet_sankala | NORMAL | 2024-01-08T00:12:08.601197+00:00 | 2024-01-08T00:12:08.601245+00:00 | 236 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nStore the frequency of each character in the map. Push them into the heap pair wise {char,freq}.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nPop the elements and take care of their freq . if(freq>limit) then you have to check the next element also else just keep poping the elements.As we want the lexicographically largest string so we\'ll use second max character only one time if(freq>limit).\nHope that helps !!\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(nlogn)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(n)\n# Code\n```\n\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n string ans="";\n unordered_map<char,int>mp;\n for(auto it:s) mp[it]++;\n priority_queue<pair<char,int>>q;\n for(auto it:mp){\n q.push({it.first,it.second});\n }\n\n while(!q.empty()){\n auto it=q.top();\n char ch=it.first;\n int freq=it.second;\n q.pop();\n \n if(freq>repeatLimit){\n int cnt=repeatLimit;\n while(cnt--){\n ans+=ch;\n }\n freq=freq-repeatLimit;\n\n if(q.size()<=0) break;\n auto itr=q.top();\n char ch1=itr.first;\n int freq1=itr.second;\n q.pop();\n\n if(freq1>=1){\n ans+=ch1;\n freq1--;\n if(freq1>0) q.push({ch1,freq1});\n }\n \n if(freq>0){\n q.push({ch,freq}); \n }\n }\n\n else{\n while(freq--){\n ans+=ch;\n }\n }\n \n }\n return ans;\n }\n};\n``` | 2 | 0 | ['Greedy', 'Heap (Priority Queue)', 'C++'] | 1 |
construct-string-with-repeat-limit | [Python3] Heap approach, beats 100% in time | python3-heap-approach-beats-100-in-time-puk1u | \n# Code\n\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n # Create max heap based on the lexigraphic order and n | pandede | NORMAL | 2024-01-05T16:53:56.487773+00:00 | 2024-01-05T16:53:56.487802+00:00 | 109 | false | 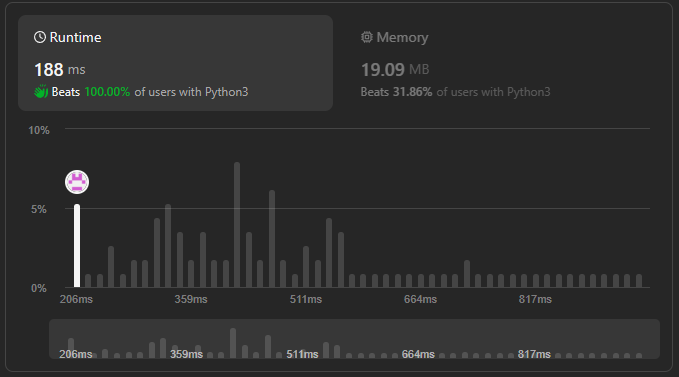\n# Code\n```\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n # Create max heap based on the lexigraphic order and number of occurrences \n heap = [\n (-ord(char), -count) \n for char, count in Counter(s).items()\n ]\n heapq.heapify(heap)\n\n res = \'\'\n while heap:\n # Take out the largest letter\n max_char, max_count = heapq.heappop(heap)\n while heap and -max_count > repeatLimit:\n # When the largest letter is appended *repeatLimit* times\n # insert ONE second largest letter\n top_char, top_count = heapq.heappop(heap)\n res += chr(-max_char) * repeatLimit + chr(-top_char)\n max_count += repeatLimit\n\n # If the second largest letter is all used up\n # don\'t push it back to the heap\n if top_count < -1:\n heapq.heappush(heap, (top_char, top_count+1))\n # Appends the remaining counting to the result\n res += chr(-max_char) * min(-max_count, repeatLimit)\n return res\n``` | 2 | 0 | ['Heap (Priority Queue)', 'Python3'] | 1 |
construct-string-with-repeat-limit | Python 3 Linked list - O(n) time and space - if you want to avoid the O(logN) heap push/pop | python-3-linked-list-on-time-and-space-i-ckv9 | Approach\nThis approach is similar to that of a priority queue / heap.\n\nHowever, using a linked list avoid the O(logN) time operations of heappush() and heapp | leokln | NORMAL | 2023-10-25T15:08:49.505412+00:00 | 2023-10-25T15:08:49.505432+00:00 | 120 | false | # Approach\nThis approach is similar to that of a priority queue / heap.\n\nHowever, using a linked list avoid the O(logN) time operations of heappush() and heappop().\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n```\n"""\nImplementation of a Node in a linked list.\n"""\nclass Node:\n def __init__(self, chr, val):\n self.chr = chr # A letter\n self.val = val # Store count of a letter \n self.next = None\n\n"""\nSolution with a linked list\n"""\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n # Count the letters and store them in an array of 26 \n counts = [0] * 26\n for i in s:\n counts[ord(i)-97] += 1\n\n # Loop through the counts and construct a linked list that link letters\n # and their counts in descending order, ignoring letters that have 0 count.\n # We have as a result:\n # root = Node(dummy, -1) -> Node(z, 2) -> Node(c, 4) -> Node(a, 1)\n root = last = Node("dummy", -1)\n for i in range(len(counts)-1, -1, -1):\n if counts[i] != 0:\n last.next = Node(chr(i+97), counts[i])\n last = last.next\n root = root.next\n \n # We go through the linked list like we do with the priority queue and\n # add letters into the answer array.\n k = repeatLimit\n ans = []\n # As long as the list is not empty, we keep going.\n while root: \n # The current node has count > repeatLimit\n if root.val > k:\n # We add the letter into ans (repeatLimit) times and update\n # the remaining count in the Node.\n ans.extend([root.chr] * k)\n root.val -= k\n \n # We need to take away one from the next Node (the next largest\n # letter) and add to the ans as separator.\n if root.next is None:\n # There are no more smaller letters we can use and cannot\n # continue adding the letter from the current Node since\n # limit is reached.\n break\n else:\n # We add the next largest letter from the next Node and\n # update remaining count. If remaining count == 0, we remove \n # that Node and link Node.next to Node.next.next.\n ans.append(root.next.chr)\n root.next.val -= 1\n if root.next.val == 0:\n root.next = root.next.next\n \n # The current node has count <= repeatLimit\n else:\n # We simply add the letters (count) times into ans and move\n # on to the next Node. \n ans.extend([root.chr] * root.val)\n root = root.next\n\n return "".join(ans)\n \n \n``` | 2 | 0 | ['Python3'] | 2 |
construct-string-with-repeat-limit | Using priority queue (faster 100%) | using-priority-queue-faster-100-by-remed-13sb | Approach\nWe count every character and use\npriority queue to achieve lexicographical order\n\nE.g zzzzccca, repeatLimit=3\n0) we build a queue: [z:4, c:3, a:1] | remedydev | NORMAL | 2023-02-24T00:16:34.535535+00:00 | 2023-02-24T00:27:14.815199+00:00 | 193 | false | # Approach\nWe count every character and use\npriority queue to achieve lexicographical order\n```\nE.g zzzzccca, repeatLimit=3\n0) we build a queue: [z:4, c:3, a:1]\n1) Start iterating a queue, on every iteration we\n 2) dequeue {z:4} \n 3) generate "zzz" (now we left with {z:1})\n 4) pick next element in a queue {c:3}, generate ONE "c", now we left with {c:2}\n 5) push {z:1} back to the queue, repeat from 2\n```\n\n\n# Complexity\n- Time complexity:\n$$O(nlogn)$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nvar repeatLimitedString = function (s, repeatLimit) {\n const freq = {}, \n //https://github.com/datastructures-js/priority-queue\n queue = new MaxPriorityQueue(), result=[];\n for(let c of s) freq[c]=freq[c]?freq[c]+1:1;\n for(let [k,v] of Object.entries(freq))\n queue.enqueue({l:k, c:v}, k.charCodeAt(0)-97);\n \n while(!queue.isEmpty()){\n const {element:top, priority:prior} = queue.dequeue();\n for(let i=0; i<repeatLimit && top.c>0;i++, top.c--)\n result.push(top.l);\n\n if(top.c>0){\n if(!queue.isEmpty()){\n const f = queue.front().element;\n result.push(f.l);\n f.c--;\n if(f.c===0) queue.dequeue();\n queue.enqueue(top, prior);\n }\n }\n }\n return result.join("");\n}\n``` | 2 | 0 | ['JavaScript'] | 0 |
construct-string-with-repeat-limit | c++ faster = and less memory than 90% with explanation | c-faster-and-less-memory-than-90-with-ex-6ib1 | Intuition\n Describe your first thoughts on how to solve this problem. \n 1. construct a map\n 2. then build a string using largest char first, wi | o2thief | NORMAL | 2023-02-19T19:32:31.634086+00:00 | 2023-02-19T19:32:31.634111+00:00 | 210 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n 1. construct a map\n 2. then build a string using largest char first, with in repeatlimit.\n 3. if reach limit, insert a second largest char and continue try using largest char\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n // construct a map\n vector<int> map(26,0);\n for (char c : s)\n map[c-\'a\']++;\n\n // then build a string using largest char first, with in repeatlimit.\n // if reach limit, insert a second largest char and continue try using largest char\n string res; \n for(int i = 25; i>=0; i--)\n {\n while (map[i] > 0)\n {\n for (int k = 0; k < min(map[i], repeatLimit); k++)\n res+= \'a\'+i; \n \n bool con = false;\n map[i]-=min(map[i], repeatLimit);\n if (map[i] > 0)\n {\n for (int j = i-1; j>=0; j--)\n {\n if(map[j] > 0)\n {\n res += \'a\'+j;\n map[j] --;\n con = true;\n break;\n }\n }\n }\n if (!con)\n break;\n }\n }\n return res; \n }\n};\n``` | 2 | 0 | ['C++'] | 1 |
construct-string-with-repeat-limit | Java | O(n) | No map or queue | Beats > 95% | java-on-no-map-or-queue-beats-95-by-judg-cyl5 | Intuition\n Describe your first thoughts on how to solve this problem. \nCreate a frequency count array for all letters in the input string. Iterate over the fr | judgementdey | NORMAL | 2023-01-12T22:22:00.847640+00:00 | 2023-01-12T22:22:45.570073+00:00 | 126 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nCreate a frequency count array for all letters in the input string. Iterate over the frequency count array from the count of `z` to `a`. Skip the letters for which the count is `0`. Append one letter at a time to the output string till the frequency count of the letter becomes `0` or the repeat limit is reached. If the repeat limit is reached, remember the letter\'s index, move on to the next lexicographically largest letter and append a single instance of that, then move back to the remembered letter and continue the procees as normal.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Build a frequency count array for all letters in the input string `s`.\n2. Iterate through the frequency count array from the index of `z` to `a`.\n3. Skip any letter for which the frequency is `0`.\n4. Append one instance of the letter at a time to the output string till the frequency of the letter becomes `0` or the repeat limit is reached.\n5. If the repeat limit is reached and the frequency of the letter is > 0, remember the letter\'s index and move on to the next iteration.\n6. In each iteration, check if there\'s a remembered letter. If yes, append a single instance of the current letter to the output string and skip back to the remembered letter\'s index. Then continue the process as normal.\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n int[] freq = new int[26];\n\n for (char c : s.toCharArray())\n freq[c - \'a\']++;\n\n int pendingLetterIndex = -1;\n StringBuilder sb = new StringBuilder();\n\n for (int i=25; i >= 0; i--) {\n if (freq[i] == 0)\n continue;\n\n if (pendingLetterIndex > 0) {\n // A lexicographically larger letter is still avaialble.\n // Append a single instance of the current letter and move\n // back to the remembered letter.\n\n sb.append((char)(\'a\' + i));\n freq[i]--;\n i = pendingLetterIndex;\n pendingLetterIndex = -1;\n } else {\n for (int j=0; j < repeatLimit && freq[i] > 0; j++, freq[i]--)\n sb.append((char)(\'a\' + i));\n\n // Repeat limit reached, remember to get back to this letter.\n if (freq[i] > 0)\n pendingLetterIndex = i+1;\n }\n }\n return sb.toString();\n }\n}\n``` | 2 | 0 | ['String', 'Greedy', 'Counting', 'Java'] | 0 |
construct-string-with-repeat-limit | python 3 || O(n)/O(1) || without priority queue | python-3-ono1-without-priority-queue-by-awudi | ```\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n count = collections.Counter(s)\n chrs = list(map(list, | derek-y | NORMAL | 2022-04-23T02:06:30.820359+00:00 | 2022-04-23T02:06:30.820406+00:00 | 228 | false | ```\nclass Solution:\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n count = collections.Counter(s)\n chrs = list(map(list, sorted(count.items(), reverse=True)))\n res = []\n first, second = 0, 1\n n = len(chrs)\n \n while second < n:\n if chrs[first][1] <= repeatLimit:\n res.append(chrs[first][0] * chrs[first][1])\n first += 1\n while chrs[first][1] == 0:\n first += 1\n if first >= second:\n second = first + 1\n else:\n res.append(chrs[first][0] * repeatLimit + chrs[second][0])\n chrs[first][1] -= repeatLimit\n chrs[second][1] -= 1\n if chrs[second][1] == 0:\n second += 1\n \n res.append(chrs[first][0] * min(repeatLimit, chrs[first][1]))\n return \'\'.join(res) | 2 | 0 | ['Greedy', 'Python', 'Python3'] | 1 |
construct-string-with-repeat-limit | C++ | Construct String with Repeat Limit. | O(n) approach using priority queue | c-construct-string-with-repeat-limit-on-nu6km | class Solution {\npublic:\n \n \n string repeatString(char ch,int num) {\n string res="";\n while(num--)\n res+=ch;\n r | vamsimudaliar | NORMAL | 2022-02-27T04:50:23.548755+00:00 | 2022-02-27T04:50:23.548785+00:00 | 161 | false | class Solution {\npublic:\n \n \n string repeatString(char ch,int num) {\n string res="";\n while(num--)\n res+=ch;\n return res;\n }\n \n string repeatLimitedString(string s, int repeatLimit) {\n \n priority_queue<pair<char,int>> pq;\n vector<int> freq(26);\n \n for(int i=0;i<s.length();i++)\n freq[s[i]-\'a\']++;\n \n for(int i=0;i<26;i++) {\n if(freq[i]) {\n char keyElement = (\'a\'+i);\n pq.push({keyElement,freq[i]});\n }\n }\n \n string resultString = "";\n \n while(pq.size()>1) {\n \n char firstChar = pq.top().first;\n int firstCharCount = pq.top().second;\n pq.pop();\n \n if(firstCharCount<=repeatLimit) {\n \n resultString+=repeatString(firstChar,firstCharCount);\n }\n else {\n firstCharCount-=repeatLimit; // 1\n \n // pulling out second char. \n char secondChar = pq.top().first; // a \n int secondCharCount = pq.top().second; // 4\n pq.pop(); \n \n resultString+= (repeatString(firstChar,repeatLimit)+secondChar); //bba\n \n if(--secondCharCount)\n pq.push({secondChar,secondCharCount}); //{a:3}\n if(firstCharCount)\n pq.push({firstChar,firstCharCount}); //{b:1}\n \n }\n }\n \n if(pq.empty()==false) // contains one element \n resultString+=repeatString(pq.top().first,min(repeatLimit,pq.top().second));\n \n \n return resultString;\n \n }\n}; | 2 | 0 | ['C', 'Heap (Priority Queue)'] | 0 |
construct-string-with-repeat-limit | Java | Time O(n) | Space O(1) | java-time-on-space-o1-by-williamhsucs-azbt | \nclass Solution {\n /**\n * Time O(n)\n * Space O(1)\n */\n public String repeatLimitedString(String s, int repeatLimit) {\n String dic = "abcdefghi | williamhsucs | NORMAL | 2022-02-24T16:13:29.946103+00:00 | 2022-02-24T16:13:29.946158+00:00 | 177 | false | ```\nclass Solution {\n /**\n * Time O(n)\n * Space O(1)\n */\n public String repeatLimitedString(String s, int repeatLimit) {\n String dic = "abcdefghijklmnopqrstuvwxyz";\n // Space O(26)\n int[] bucket = new int[26];\n // Time O(n)\n for (int i = 0; i < s.length(); i++) {\n bucket[s.charAt(i) - \'a\']++;\n }\n \n // Space O(26)\n List<Integer> trace = new ArrayList<>();\n for (int i = 25; i >= 0; i--) {\n if (bucket[i] > 0) {\n trace.add(i);\n }\n }\n\n StringBuilder str = new StringBuilder();\n int cur = 0;\n int next = 1;\n int limit = repeatLimit;\n // Time O(n)\n while (cur < trace.size() && limit > 0) {\n str.append(dic.charAt(trace.get(cur)));\n bucket[trace.get(cur)]--;\n limit--;\n if (bucket[trace.get(cur)] == 0) {\n if (next > trace.size() - 1) {\n break;\n }\n cur = next;\n next++;\n limit = repeatLimit;\n }\n if (limit == 0) {\n if (next > trace.size() - 1) {\n break;\n }\n str.append(dic.charAt(trace.get(next)));\n bucket[trace.get(next)]--;\n limit = repeatLimit;\n }\n if (next < trace.size() && bucket[trace.get(next)] == 0) {\n next++;\n }\n }\n // Time O(n)\n return str.toString();\n }\n}\n``` | 2 | 0 | ['Java'] | 0 |
construct-string-with-repeat-limit | [Python] Simple solution faster than 100%- Using 2 pointers + sorting | python-simple-solution-faster-than-100-u-233l | Hi ,\n\nHere is a simple solution. Please Upvote if you like!\n\nNote: \n\t\tletters = sorted(list(c.keys())+[\'\']) is to take care of the edgecase when seco | pal1545 | NORMAL | 2022-02-21T10:11:49.462259+00:00 | 2022-02-21T10:11:49.462289+00:00 | 222 | false | Hi ,\n\nHere is a simple solution. Please **Upvote** if you like!\n\nNote: \n\t\tletters = sorted(list(c.keys())+[\'\']) is to take care of the edgecase when `second` is None\n\n```\n\n def repeatLimitedString(self, s: str, repeatLimit: int) -> str:\n\t\n c = collections.Counter(s)\n res = []\n letters = sorted(list(c.keys())+[\'\'])\n\n first,second = letters.pop(),letters.pop()\n\n while first and second:\n if c[first] > repeatLimit:\n c[first] -= repeatLimit\n res += first * repeatLimit\n res += second\n c[second] -= 1\n if c[second]==0:\n second = letters.pop()\n\n else:\n res += first * c[first]\n c[first] = 0\n first = second\n second = letters.pop()\n if first: \n res += first * min(c[first], repeatLimit)\n return \'\'.join(res)\n \n | 2 | 0 | ['Python'] | 0 |
construct-string-with-repeat-limit | CONFUSION | HELP NEEDED | confusion-help-needed-by-aayushisingh170-y7an | Can someone explain why the answer returned for testcase #2 aababab is bbabaa and not bbaabaa even when the latter is lexicographically larger? | aayushisingh1703 | NORMAL | 2022-02-20T16:29:33.269234+00:00 | 2022-02-20T16:32:21.103160+00:00 | 57 | false | Can someone explain why the answer returned for testcase #2 ```aababab``` is ```bbabaa``` and not ```bbaabaa``` even when the latter is lexicographically larger? | 2 | 0 | [] | 1 |
construct-string-with-repeat-limit | [c++] fully commented with O(N) time complexity and O(1) space | c-fully-commented-with-on-time-complexit-zwj8 | class Solution {\npublic:\n \n string repeatLimitedString(string s, int l) {\n\t\tint m[26]; // variable for storing 26 characters\n\t\n for(char c | abhishekjha9909 | NORMAL | 2022-02-20T16:12:44.944169+00:00 | 2022-02-20T16:12:44.944205+00:00 | 81 | false | class Solution {\npublic:\n \n string repeatLimitedString(string s, int l) {\n\t\tint m[26]; // variable for storing 26 characters\n\t\n for(char c:s)m[c-\'a\']++; // frequncy count;\n \n int i=25,j; //i-> last character ie., \'z\' \n \n string res=""; //for storing ans \n \n while(i>=0){ // iterating to z-a characters \n int x=l; // temparary variable for storing limit;\n \n while(m[i] && x){ //if character remain in our map && x has value more than 0\n res+=(\'a\'+i); //appending the current character\n m[i]--; //reducing the frequency count\n x--; //reducing the limit as well\n }\n \n if(m[i]){ // current character still has more frequency to come again\n int j=i-1; //hence pointing the next character which is less than the current character;\n \n while(j>=0 && m[j]==0) // after this loop j will be a valid chacter which has frequency;\n j--;\n \n if(j>=0){ //if valid character exist then \n res+=(\'a\'+j); // add that valid character\n m[j]--; //and reduce its frequency\n }\n else //if j has value less than 0 implies no valid character exist as character can be in range [0, 25]\n break;\n }\n if(!m[i]) //if that current character has no value then decrement it\n i--;\n }\n return res;\n \n // time complexity -> O( 26*N ) == O(N) , where N is the size of string;\n // space complexity -> O(1) \n }\n};\n\n**if you find it helpful please upvote**\n**Time complexity -> O( 26*N ) == O(N) , where N is the size of string;\n space complexity -> O(1)** | 2 | 1 | ['C', 'C++'] | 1 |
construct-string-with-repeat-limit | C++, Worst to best, Bruteforce (N^2), priorityQueue(O(NlogN)), Counting + greedy(O(N)) | c-worst-to-best-bruteforce-n2-priorityqu-kmfx | Bruteforce Solution\n#1 Bruteforce Approach O(N^2) not accepted (failed at 144th testcase were the limit is \'1\')\n1. first sort the string.\n2. now traverse t | Albatross912 | NORMAL | 2022-02-20T11:26:04.116278+00:00 | 2022-02-20T11:26:04.116312+00:00 | 209 | false | # Bruteforce Solution\n**#1 Bruteforce Approach O(N^2) not accepted (failed at 144th testcase were the limit is \'1\')**\n1. first sort the string.\n2. now traverse through the string and find the index were the *limit* is reached as soon as the limit reached find the next element which is not equal to the current element \n3. then swap the both element \n4. after doing that it can be observe that the resultant string is left with the extra character at the end of the string \n\tEg:\n\tstring s = **"aaaaaaabbbccccd"**\n\tlimit = **3**\n\t* resultant string looks like \n\t\t\t **"dcccbcbbaaaaaaa"**\n\t* now we need to pop some of the last element from the string and we have done\n\t* to do so, we need to count the occurence of the last character (***in example it is \'a\' = 7*** )\n\t* Number of character to be deleted will be = **count - limit** *i.e* **7-3 = 4**\n 5. now just delete the required character from the string and done!.\n\t \n\t resultant string = **"dcccbcbbaaaaaaa"**\n\t count = 7, limit = 3;\n\t noOfdelete = 7 - 3 = 4\n\t After deletion string = **""dcccbcbbaaa | aaaa""**\n\t \n\t C++ Code : \n```\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n \n sort(s.rbegin(), s.rend());\n int count = 0;int j = 0;\n for(int i=0; i<s.size()-1; i++)\n {\n j = i;\n if(count >= repeatLimit)\n { \n for( ; j<s.size(); j++)\n {\n if(s[i] != s[j])\n {\n swap(s[i], s[j]); \n break;\n }\n }\n count = 0;\n }\n if(s[i] != s[i+1])count=0;\n else\n {\n count++;\n }\n }\n int c = 1;\n for(int i=s.size()-2; i>=0; i--)\n {\n if(s[i] != s[i+1] && i-1 >= 0)\n {\n break;\n }\n c++;\n }\n if(c <= repeatLimit)return s;\n int t = c - repeatLimit;\n while(t-- && !s.empty())s.pop_back();\n \n return s;\n }\n};\n\t \n```\n# Priority Queue Solution (Better)\n**priority queue O(NLogN), accepted**\n\n1. count all occurence of each and every character (*using map or a vector*)\n2. make a priority queue of pair and push all the element and frequency in it.\n3. Push the very first character in the answer minimum of limit and the frequency of the first character in the priority queue *i.e.* **push character X min(limit, characterFreq)**\n4. reduce the characterFreq as **characterFreq -= limit**\n5. if characterFreq is -ve we will continue\n6. else if the priority queue is empty then simply return the anwer \n7. else push one occurence of the top most character in priority queue \n8. also reduce the newFrew by 1 *i.e.* newFreq -= 1\n\nNOTE : You will understood the approach more clear by dry runnig the code \n\nCODE C++:\n```\n\nclass Solution{\n public:\n string repeatLimitedString(string s, int limit)\n {\n map<char,int> mpp;\n for(auto i:s)\n {\n mpp[i]++;\n }\n \n priority_queue<pair<char,int>> pq;\n for(auto i:mpp)\n {\n pq.push({i.first, i.second});\n }\n \n string ans;\n while(!pq.empty())\n {\n pair<char,int> temp = pq.top(); pq.pop();\n \n point : // ( * )\n \n int fill = min(limit, temp.second);\n temp.second -= fill;\n \n while(fill--)ans.push_back(temp.first);\n if(temp.second > 0)\n {\n if(pq.empty())return ans;\n \n pair<char, int> now = pq.top(); pq.pop();\n ans.push_back(now.first);\n now.second -= 1;\n if(now.second) pq.push(now);\n goto point; ( * )\n }\n }\n return ans;\n }\n};\n```\n\n\n**I personally came up with only these two approach, the best Approach actually idea of @lzl124631x Thanks @lzl124631x for giving such optimised solution**\n\nlink to his blog : https://leetcode.com/problems/construct-string-with-repeat-limit/discuss/1784718/C%2B%2B-Greedy-%2B-Counting-O(N)-Time-O(1)-space\n\n\nNOTE : This is my very first blog please forgive any mistake done in the blog.\n | 2 | 0 | ['Greedy', 'C', 'Heap (Priority Queue)', 'Counting Sort', 'C++'] | 0 |
construct-string-with-repeat-limit | priority queue C++ solution #ACCEPTED😍 | priority-queue-c-solution-accepted-by-ki-xra8 | class Solution {\npublic:\n\n string repeatLimitedString(string s, int k) {\n string ans = "";\n \n unordered_mapmp;\n \n | kinggaurav | NORMAL | 2022-02-20T11:04:02.197992+00:00 | 2022-02-20T11:04:02.198024+00:00 | 207 | false | class Solution {\npublic:\n\n string repeatLimitedString(string s, int k) {\n string ans = "";\n \n unordered_map<char , int>mp;\n \n for(int i = 0 ; i < s.size() ; i++){\n mp[s[i]]++;\n }\n \n priority_queue<pair<char , int>>pq;\n \n for(auto value : mp){\n pq.push({value.first , value.second});\n }\n \n while(!pq.empty()){\n char first = pq.top().first;\n int second = pq.top().second;\n pq.pop();\n \n int size = min(second , k);\n \n for(int i = 0 ; i < size ; i++){\n ans += first;\n }\n \n if(second - k > 0){\n if(!pq.empty()){\n char first2 = pq.top().first;\n int second2 = pq.top().second;\n pq.pop();\n ans += first2;\n if(second2 - 1 > 0){\n pq.push({first2 , second2 - 1});\n }\n }else{\n return ans;\n }\n pq.push({first , second - k});\n }\n }\n \n return ans;\n }\n}; | 2 | 0 | ['C', 'Heap (Priority Queue)'] | 0 |
construct-string-with-repeat-limit | Simple javascript solution - 159 ms | simple-javascript-solution-159-ms-by-efo-znbq | \nvar repeatLimitedString = function(s, repeatLimit) {\n const aCode = "a".charCodeAt();\n const counts = new Array(26).fill(0);\n let result = "";\n | eforce | NORMAL | 2022-02-20T08:30:16.475626+00:00 | 2022-02-20T08:30:16.475664+00:00 | 63 | false | ```\nvar repeatLimitedString = function(s, repeatLimit) {\n const aCode = "a".charCodeAt();\n const counts = new Array(26).fill(0);\n let result = "";\n let cnt, takeOne, len, lastLetter;\n \n for (let i = 0; i < s.length; ++i) {\n ++counts[s.charCodeAt(i) - aCode];\n }\n \n while (result.length < s.length) {\n takeOne = false;\n len = result.length;\n\n for (let i = 26; i >= 0; --i) {\n if (counts[i] > 0) {\n \n if (lastLetter === i) {\n takeOne = true;\n continue;\n }\n \n lastLetter = i;\n cnt = takeOne ? 1 : Math.min(counts[i], repeatLimit);\n result += String.fromCharCode(i + aCode).repeat(cnt);\n counts[i] -= cnt;\n break;\n }\n }\n \n if (result.length === len) break;\n }\n \n return result;\n};\n``` | 2 | 0 | [] | 0 |
construct-string-with-repeat-limit | Easy to understand | c++ | easy-to-understand-c-by-smit3901-mmt2 | \n// Using priority queue\n string repeatLimitedString(string s, int k) {\n string res = "";\n \n map<char,int> mp;\n \n f | smit3901 | NORMAL | 2022-02-20T08:04:45.548390+00:00 | 2022-02-20T08:04:45.548417+00:00 | 148 | false | ```\n// Using priority queue\n string repeatLimitedString(string s, int k) {\n string res = "";\n \n map<char,int> mp;\n \n for(auto x:s)\n {\n mp[x]++;\n }\n \n priority_queue<pair<char,int>> pq;\n \n for(auto x:mp)\n {\n pq.push({x.first,x.second});\n }\n \n while(!pq.empty())\n {\n char c1 = pq.top().first;\n int f1 = pq.top().second;\n pq.pop();\n \n int len = min(k,f1);\n \n for(int i=0;i<len;i++)\n {\n res += c1;\n }\n \n if(!pq.empty() and f1 - len > 0)\n {\n char c2 = pq.top().first;\n int f2 = pq.top().second;\n \n pq.pop();\n \n res += c2;\n if(f1 - len > 0)\n {\n pq.push({c1,f1-len});\n }\n if(f2 - 1 > 0)\n {\n pq.push({c2,f2-1});\n } \n } \n \n }\n return res;\n \n }\n``` | 2 | 0 | ['C', 'Heap (Priority Queue)'] | 0 |
construct-string-with-repeat-limit | Priority Queue || Max Heap || Map || C++ | priority-queue-max-heap-map-c-by-krishna-y84c | \nclass Solution {\npublic:\n string repeatLimitedString(string s, int r) {\n unordered_map<char,int>mp;\n for(auto c:s)\n {\n | krishnakant01 | NORMAL | 2022-02-20T06:12:35.434358+00:00 | 2022-02-20T06:14:02.611216+00:00 | 40 | false | ```\nclass Solution {\npublic:\n string repeatLimitedString(string s, int r) {\n unordered_map<char,int>mp;\n for(auto c:s)\n {\n mp[c]++;\n }\n // Keep the characters and it\'s count in max heap, Charater as first element\n priority_queue<pair<char,int>>pq;\n for(auto p:mp)\n {\n pq.push(p);\n }\n string ans;\n // For each top element, if it has occurance greater or equal to repeatLimit than take this character repeatLimit times \n while(!pq.empty())\n {\n auto [c,f]=pq.top();\n pq.pop();\n for(int i=0;i<r and f>0;i++)\n {\n ans+=c;\n f--;\n }\n // if frecuency of current charater>repeatLimit\n if(f)\n {\n // than check if there is any element in heap so that we can take that \n if(!pq.empty())\n {\n auto p=pq.top();\n pq.pop();\n ans+=p.first;\n if(p.second-- >1)\n {\n pq.push(p);\n }\n pq.push({c,f}); \n }\n\t\t\t\t// otherwise if this was the last element that return from here\n else\n {\n return ans;\n }\n }\n \n }\n return ans;\n }\n};\n``` | 2 | 0 | [] | 0 |
construct-string-with-repeat-limit | My Java solution with comments | my-java-solution-with-comments-by-zadelu-ozsf | The approach is to add as many copies of the "higher" characters as possible, up to repeatLimit. Then, add only 1 copy of the next highest character as a separa | zadeluca | NORMAL | 2022-02-20T04:36:10.372053+00:00 | 2022-02-20T05:32:45.979725+00:00 | 152 | false | The approach is to add as many copies of the "higher" characters as possible, up to repeatLimit. Then, add only 1 copy of the next highest character as a separator, and "go back" to the previous character.\n\nFor example, with ```s = "zzzzyy"``` and ```repeatLimit = 2```, we:\n1) Add 2 copies of \'z\'. Since we still have 2 copies remaining that we want to use later, we set ```goBack = 25```.\n2) Since ```goBack != 0```, add only 1 copy of \'y\'. Set ```i = 26``` so that on next loop iteration ```i = 25``` again, and we can add more \'z\'.\n3) Add 2 more copies of \'z\'.\n4) Add final \'y\', which produces \'zzyzzy\'.\n\n```\nclass Solution {\n public String repeatLimitedString(String s, int repeatLimit) {\n\t // count number of each character\n int[] counts = new int[26];\n for (int i = 0; i < s.length(); i++) {\n counts[s.charAt(i) - \'a\']++;\n }\n \n StringBuilder sb = new StringBuilder();\n \n\t\t// if we hit repeatLimit for a character, we can "go back"\n\t\t// to it later after adding some lower character\n int goBack = 0;\n\t\t\n for (int i = 25; i >= 0; i--) { // z -> a\n if (counts[i] > 0) {\n char c = (char)(\'a\' + i);\n\t\t\t\t\n if (goBack == 0) {\n\t\t\t\t\t// append as many copies as we have, up to repeatLimit\n for (int j = 0; j < repeatLimit && counts[i] > 0; j++) {\n sb.append(c);\n counts[i]--;\n }\n\t\t\t\t\t// if we still have more available then we hit repeatLimit, and we\'ll need\n\t\t\t\t\t// to add some lower character before we can add more of this one\n if (counts[i] > 0) {\n goBack = i;\n }\n } else {\n\t\t\t\t\t// when goBack is not 0, we want to append only 1 copy of the next lower\n\t\t\t\t\t// character, then go back to the higher character by re-setting i to goBack+1\n sb.append(c);\n counts[i]--;\n i = goBack + 1;\n goBack = 0;\n }\n }\n }\n \n return sb.toString();\n }\n}\n``` | 2 | 0 | ['Java'] | 2 |
construct-string-with-repeat-limit | Java || Simple || Map || Greedy | java-simple-map-greedy-by-lagahuahubro-silm | ```\nclass Solution {\n public String repeatLimitedString(String s, int limit) {\n int[] map = new int[26];\n int n = s.length();\n for | lagaHuaHuBro | NORMAL | 2022-02-20T04:20:09.574602+00:00 | 2022-02-20T04:24:31.629157+00:00 | 72 | false | ```\nclass Solution {\n public String repeatLimitedString(String s, int limit) {\n int[] map = new int[26];\n int n = s.length();\n for (int i = 0; i < n; i++) {\n map[s.charAt(i) - \'a\']++;\n }\n return construct(map, limit);\n }\n \n public String construct(int[] map, int k) {\n StringBuilder ans = new StringBuilder();\n for (int i = 25; i >= 0; i--) {\n while (map[i] != 0) {\n int same = 0;\n while (same < k && map[i] > 0) {\n ans.append((char)(i + \'a\'));\n same++;\n map[i]--;\n }\n if (map[i] > 0) {\n // brings the next smaller character available\n int nse = next(map, i);\n if (nse == -1) {\n break;\n } else {\n ans.append((char)(nse + \'a\'));\n map[nse]--;\n }\n }\n }\n }\n return ans.toString();\n }\n \n \n public int next(int[] map, int p) {\n for (int i = p - 1; i >= 0; i--) {\n if (map[i] != 0) {\n return i;\n }\n }\n return -1;\n }\n} | 2 | 0 | ['Greedy'] | 0 |
construct-string-with-repeat-limit | javascript greedy 192ms | javascript-greedy-192ms-by-henrychen222-wcpq | Main idea: greedy.\nto make lexical greatest, so each time use out current largest char (search from end 25 -> 0)\n (1) freq <= limit, append (freq char) direc | henrychen222 | NORMAL | 2022-02-20T04:14:09.627336+00:00 | 2022-02-20T04:28:05.317182+00:00 | 248 | false | Main idea: greedy.\nto make lexical greatest, so each time use out current largest char (search from end 25 -> 0)\n (1) freq <= limit, append (freq char) directly, f[i] = 0\n (2) freq > limit, append (limit char), find a bridge (second largest char, to make lexical greatest), append it, f[i] -= limit,\n until freq <= limit, redo (1)\n\t if cannot find a bridge char, stop now is the answer\n```\nconst ord = (c) => c.charCodeAt();\n\nconst repeatLimitedString = (s, limit) => {\n let f = Array(26).fill(0), res = \'\';\n for (const c of s) f[ord(c) - 97]++;\n for (let i = 25; ~i; i--) { // each time use out current largest char\n if (f[i] == 0) continue;\n let c = String.fromCharCode(97 + i);\n while (f[i] > limit) { // condition 2\n res += c.repeat(limit);\n f[i] -= limit;\n let findBridge = false, bridge, j;\n for (j = 25; ~j; j--) { // find second larger char\n if (j == i) continue; // should be different from current char\n if (f[j] > 0) {\n findBridge = true;\n bridge = String.fromCharCode(97 + j);\n break;\n }\n }\n if (!findBridge) return res;\n res += bridge;\n f[j]--;\n }\n res += c.repeat(f[i]); // condition 1\n f[i] = 0;\n }\n return res;\n};\n``` | 2 | 0 | ['Greedy', 'JavaScript'] | 0 |
construct-string-with-repeat-limit | C++ | Easy to understand | Hash Map | c-easy-to-understand-hash-map-by-parth_c-mvhy | \nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n vector<int> cnt(26, 0);\n for(auto &x : s){\n | parth_chovatiya | NORMAL | 2022-02-20T04:13:10.356204+00:00 | 2022-02-20T04:13:10.356242+00:00 | 74 | false | ```\nclass Solution {\npublic:\n string repeatLimitedString(string s, int repeatLimit) {\n vector<int> cnt(26, 0);\n for(auto &x : s){\n cnt[x-97]++;\n }\n \n string ans = "";\n int j = 25;\n while(j>=0 and cnt[j]==0){\n j--;\n }\n j--;\n \n for(int i=25; i>=0; i--){\n int tmp = repeatLimit;\n while(cnt[i]>0 and tmp>0){\n ans.push_back(i+97);\n cnt[i]--;\n tmp--;\n }\n if(cnt[i] != 0){\n while(j>=0 and (cnt[j]==0 or j==i)){\n j--;\n }\n if(j>=0){\n ans.push_back(j+97);\n cnt[j]--;\n i++;\n }\n }\n }\n \n return ans;\n }\n};\n``` | 2 | 2 | [] | 0 |
construct-string-with-repeat-limit | Java heap | simple | java-heap-simple-by-xavi_an-8g6x | ```\nclass Solution {\n public String repeatLimitedString(String s, int r) {\n int [] f = new int[26];\n for(int i=0;i<s.length();i++){\n | xavi_an | NORMAL | 2022-02-20T04:04:13.618306+00:00 | 2022-02-20T04:51:24.230624+00:00 | 110 | false | ```\nclass Solution {\n public String repeatLimitedString(String s, int r) {\n int [] f = new int[26];\n for(int i=0;i<s.length();i++){\n f[s.charAt(i)-\'a\']++; // frequency map with char count\n }\n PriorityQueue<int []> queue = new PriorityQueue<>((a, b) -> b[0] - a[0]); // queue ordered decending by char\n for(int i=0;i<26;i++){\n if(f[i] > 0){\n queue.add(new int[]{i, f[i]});\n }\n }\n StringBuilder sb = new StringBuilder(); \n while(!queue.isEmpty()){\n int [] c = queue.poll();\n int x = 0;\n char ch = (char) (c[0]+\'a\');\n while(x++ < r && c[1]-- > 0){\n sb.append(ch);\n }\n if(queue.isEmpty()){\n break;\n }else if(c[1] > 0){\n int [] cn = queue.poll();\n queue.add(c);\n sb.append((char)(cn[0] +\'a\'));\n if(--cn[1] > 0){\n queue.add(cn);\n }\n }\n }\n return sb.toString();\n }\n} | 2 | 1 | [] | 0 |
construct-string-with-repeat-limit | Easy Java Solution || Priority Queue | easy-java-solution-priority-queue-by-ver-usu8 | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | vermaanshul975 | NORMAL | 2025-03-28T09:46:33.462330+00:00 | 2025-03-28T09:46:33.462330+00:00 | 9 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public String repeatLimitedString(String s, int repeatLimit) {
int[] freq = new int[26];
for (char ch : s.toCharArray()) {
freq[ch - 'a']++;
}
StringBuilder sb = new StringBuilder();
PriorityQueue<Character> pq = new PriorityQueue<>(Collections.reverseOrder());
for (int i = 25; i >= 0; i--) {
if (freq[i] > 0) {
pq.add((char) (i + 'a'));
}
}
while (!pq.isEmpty()) {
char top = pq.poll();
int count = Math.min(freq[top - 'a'], repeatLimit);
for (int i = 0; i < count; i++) {
sb.append(top);
}
freq[top - 'a'] -= count;
if (freq[top - 'a'] > 0) {
if (pq.isEmpty()) break;
char next = pq.poll();
sb.append(next);
freq[next - 'a']--;
if (freq[next - 'a'] > 0) {
pq.add(next);
}
pq.add(top);
}
}
return sb.toString();
}
}
``` | 1 | 0 | ['Java'] | 0 |
construct-string-with-repeat-limit | 🔥🔥🔥🔥O(N) solution 🔥🔥🔥🔥 | on-solution-by-vadapally_varshitha-ktea | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | vadapally_varshitha | NORMAL | 2025-01-12T17:52:00.838040+00:00 | 2025-01-12T17:52:00.838040+00:00 | 12 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
string repeatLimitedString(string s, int repeatLimit) {
if (s.length() == 1)
return s;
sort(s.begin(), s.end(), greater<char>());//use count sort for O(N)
vector<int> endPos(26, 0);
int sum = -1;
for (auto ch : s) {
sum++;
endPos[ch - 'a'] = sum;
}
int i = 0, j = 1;
while (j < s.length()) {
if (s[i] == s[j]) {
if ((j - i) >= repeatLimit) {
if ((endPos[s[j] - 'a'] + 1) < s.length()) {
i = j;
endPos[s[j] - 'a']++;
swap(s[j], s[endPos[s[j] - 'a']]);
} else {
return s.substr(0, j);
}
}
j++;
} else {
i = j;
j = i + 1;
}
}
return s;
}
};
``` | 1 | 0 | ['Counting', 'C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Dynamic programming and space optimized. Beats 100%, easy to understand. | dynamic-programming-and-space-optimized-3by5a | Intuition\n Describe your first thoughts on how to solve this problem. \nKind of dynamic programming\n\n# Approach\n\nFor each number we have 2 choices, add or | null_pointer_exception | NORMAL | 2024-06-23T04:02:27.369280+00:00 | 2024-06-23T04:51:04.422506+00:00 | 4,600 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nKind of dynamic programming\n\n# Approach\n\nFor each number we have 2 choices, add or subtract to previous result.\nWe will maintain 2 result states, **addResult** and **subResult**.\n\nNow coming to current element, if we want to\n- **Add**: We can add this to maximum of addResult and subResult, because previous result doesn\'t matter.\n- **Subtract**: We need to add this to addResult, because adding it to subResult will make it more negative as well as it violates problem statement;\n\nOnce we are done with all element, return maximum of addResult and subResult\n \n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Java\n```\nclass Solution {\n public long maximumTotalCost(int[] nums) {\n int n = nums.length;\n long addResult = nums[0];\n long subResult = nums[0];\n for (int i = 1; i < n; i++) {\n long tempAdd = Math.max(addResult, subResult) + nums[i];\n long tempSub = addResult - nums[i];\n\n addResult = tempAdd;\n subResult = tempSub;\n }\n return Math.max(addResult, subResult);\n }\n}\n```\n\n#Python\n```\ndef maximumTotalCost(self, nums):\n add_result = nums[0]\n sub_result = nums[0]\n\n for i in range(1, len(nums)):\n temp_add = max(add_result, sub_result) + nums[i]\n temp_sub = add_result - nums[i]\n\n add_result = temp_add\n sub_result = temp_sub\n\n return max(add_result, sub_result)\n```\n\n\n | 53 | 5 | ['Dynamic Programming', 'Java', 'Python3'] | 7 |
maximize-total-cost-of-alternating-subarrays | Recursion + Memo | recursion-memo-by-donpaul271-fc4i | Function used in the recursion will have the following parameters.\n\npos - postion in the array\nstat - whether a subarray is already under construction or no | donpaul271 | NORMAL | 2024-06-23T04:03:48.946430+00:00 | 2024-06-26T04:19:09.576400+00:00 | 3,285 | false | Function used in the recursion will have the following parameters.\n\npos - postion in the array\nstat - whether a subarray is already under construction or not.\nsign - The sign of the current element if its added to the subarray \n\n-----\n\nWe can use a memo of the form dp[100001][2][2]\n(make sure the dp is initialized to -1e16 and not -1, as -1 is a possible answer)\n\npos<=100000\nstat can have two values 0 and 1(0 - not started, 1 - started)\nsign can have two values 0 and 1(0 implies +ve sign and 1 implies -ve sign)\n\n-----\n\nWe get a unique state using these parameters. \nRecursion calls are explained in the code. \n\n(please upvote if it helped)\n\n# Code\n```\n#define ll long long\n\nll find(int pos, int stat, int sign, vector<ll> &nums, vector<vector<vector<ll>>> &dp)\n{\n if(pos == nums.size())\n return 0;\n \n ll ans = -1e15;\n \n if(dp[pos][stat][sign]!=-1e16)\n return dp[pos][stat][sign];\n \n if(stat == 0) // we have to start the construction of a new subarray\n ans = max(ans, nums[pos] + find(pos+1, 1, 1, nums, dp)); // start the construction with sign = 1 as the next element will have negetive sign\n \n else if(stat == 1) // A subarray is already under construction\n {\n if(sign == 1) // negetive sign\n {\n ans = max(ans, -nums[pos] + find(pos+1, 1, 0, nums, dp)); // continue construction with flipped sign\n ans = max(ans, find(pos, 0, 0, nums, dp)); // end the construction and begin a new one at this index. Dont increase pos, just change stat to 0.\n }\n \n else if(sign == 0) // positive sign\n {\n ans = max(ans, nums[pos] + find(pos+1, 1, 1, nums, dp)); // continue construction with flipped sign\n ans = max(ans, find(pos, 0, 0, nums, dp)); // end the construction and begin a new one at this index. Dont increase pos, just change stat to 0.\n }\n }\n\n return dp[pos][stat][sign] = ans;\n}\n\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n\n vector<ll> t1(2, -1e16);\n vector<vector<ll>> t2(2, t1);\n vector<vector<vector<ll>>> dp(nums.size()+1, t2); //initializing to -1e16\n \n vector<ll> a(nums.begin(), nums.end()); // converted to long long just in case, to avoid overflow any kind of overflow.\n\n ll ans = find(0, 0, 0, a, dp);\n return ans;\n }\n};\n \n\n \n``` | 27 | 5 | ['C++'] | 6 |
maximize-total-cost-of-alternating-subarrays | Take not take dp! | take-not-take-dp-by-minato_10-ghe8 | Intuition\n Describe your first thoughts on how to solve this problem. \n -> This problem is similar to take not take dp the only twist\nit that we have to take | Minato_10 | NORMAL | 2024-06-23T04:02:50.242722+00:00 | 2024-06-23T04:11:16.156866+00:00 | 2,468 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n -> This problem is similar to take not take dp the only twist\nit that we have to take all the elements but we can change their sign based on the previous element.\n -> Here we are using f variable to check if we can change the sign of the current element or not (i.e if the previous element was changed than the current element cannot be changed).\n -> We cannot change the first element therefore we have just added it to the answer.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n private:\n typedef long long ll;\n vector<vector<ll>> dp;\n ll n;\n ll func(vector<int>&nums,int id,int f){\n if(id>=n) return 0;\n if(dp[id][f]!=-1) return dp[id][f];\n ll take = -1e15,ntake = -1e15;\n if(f==1){\n ntake = (-nums[id])+func(nums,id+1,0);\n }\n take = nums[id]+func(nums,id+1,1);\n return dp[id][f] = max(take,ntake);\n }\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n n = nums.size();\n dp.resize(n+1,vector<ll>(3,-1));\n return nums[0]+func(nums,1,1);\n \n }\n};\n``` | 23 | 8 | ['Dynamic Programming', 'C++'] | 3 |
maximize-total-cost-of-alternating-subarrays | Striver's appraoch take or nottake for beginners!!!! | strivers-appraoch-take-or-nottake-for-be-ioaa | Intuition\nThe intution is simple just here I have just taken the index which represents the maximum value of cost and boolean flag represents the state wether | aero_coder | NORMAL | 2024-06-23T04:10:56.535098+00:00 | 2024-07-04T20:31:07.912353+00:00 | 2,076 | false | # Intuition\nThe intution is simple just here I have just taken the index which represents the maximum value of cost and boolean flag represents the state wether we have to multipy the nums[ind] by 1 or -1.\n\n# Approach\nHere as you can see that I have taken a 2d dp array.\nIn the find function, if we reach the base case then we will return 0.\nNow there I have taken two cases, old and new_val, ie, we can either continue with the old subarray or start a new one.\nNow if we decide to continue with old subrray then we have two cases, \nwether to multiply nums[ind] by 1 or -1 depending on the value of flag.\nIf the flag is true it means multiply nums[ind] by 1 otherwise -1.\nOr we can decide to discard the current subarray and then then continue with a new subarray.\n\n# Complexity\n- Time complexity:\nO(N)\n\n- Space complexity:\nO(N)\n\n# Code\nMemoisation code\n```\nclass Solution {\n using ll = long long;\n ll find(int ind, const vector<int>& nums, bool flag, vector<vector<ll>>& dp) {\n if (ind == (int)nums.size()) return 0;\n if (dp[ind][flag] != LLONG_MIN) return dp[ind][flag];\n ll old = 0;\n if (flag) {\n old = nums[ind] + find(ind + 1, nums, false, dp);\n } else {\n old = -(nums[ind]) + find(ind + 1, nums, true, dp);\n }\n ll new_val = nums[ind] + find(ind + 1, nums, false, dp);\n return dp[ind][flag] = max(old, new_val);\n }\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n vector<vector<ll>> dp(n + 1, vector<ll>(2, LLONG_MIN)); \n return find(0, nums, true, dp);\n }\n};\n\n```\nTabulation Code \n```\nclass Solution {\n using ll = long long;\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n vector<vector<ll>> dp(n + 1, vector<ll>(2, 0));\n for (ll ind = n - 1; ind >= 0; ind--) {\n for (ll flag = 1; flag >= 0; flag--) {\n ll old = 0;\n if (flag) {\n old = nums[ind] + dp[ind + 1][0];\n } else {\n old = -(nums[ind]) + dp[ind + 1][1];\n }\n ll new_val = nums[ind] + dp[ind + 1][0];\n dp[ind][flag] = max(old, new_val);\n }\n }\n return dp[0][1];\n }\n};\n\n``` | 21 | 3 | ['Dynamic Programming', 'Memoization', 'C++'] | 5 |
maximize-total-cost-of-alternating-subarrays | [Java/C++/Python] Easy and Concise, O(1) Space | javacpython-easy-and-concise-o1-space-by-a12s | Explanation\npos is last element is positive sign.\nneg is last element is negative sign.\n\nAfter the neg, we need to append a positive A[i]\nAfter the pos, we | lee215 | NORMAL | 2024-06-23T04:09:29.028863+00:00 | 2024-06-23T07:44:47.596975+00:00 | 1,794 | false | # **Explanation**\n`pos` is last element is positive sign.\n`neg` is last element is negative sign.\n\nAfter the `neg`, we need to append a positive `A[i]`\nAfter the `pos`, we can append a positive/negative `A[i]`\n<br>\n\n# **Complexity**\nTime `O(n)`\nSpace `O(n)`\n<br>\n\n**Java**\n```java\n public long maximumTotalCost(int[] A) {\n long pos = (long)-1e15, neg = 0, tmp;\n for (int a : A) {\n tmp = neg;\n neg = pos - a;\n pos = Math.max(pos, tmp) + a;\n }\n return Math.max(pos, neg);\n }\n```\n\n**C++**\n```cpp\n long long maximumTotalCost(vector<int>& A) {\n long long pos = -1e15, neg = 0, tmp;\n for (int a : A) {\n tmp = neg;\n neg = pos - a;\n pos = max(pos, tmp) + a;\n }\n return max(pos, neg);\n }\n```\n\n**Python**\n```py\n def maximumTotalCost(self, A: List[int]) -> int:\n pos, neg = -inf, 0\n for a in A:\n pos, neg = max(pos, neg) + a, pos - a\n return max(pos, neg)\n```\n | 21 | 6 | ['C', 'Python', 'Java'] | 6 |
maximize-total-cost-of-alternating-subarrays | Masked House Robber | masked-house-robber-by-votrubac-cj9z | I did not recognize the hourse robber at first.\n\nWhen we see a positive number, we just take it (starting a new subarray).\n\nFor a streak of negative numbers | votrubac | NORMAL | 2024-06-23T04:08:12.046038+00:00 | 2024-06-23T04:32:32.637973+00:00 | 2,299 | false | I did not recognize the hourse robber at first.\n\nWhen we see a positive number, we just take it (starting a new subarray).\n\nFor a streak of negative numbers, we can flip some of them to positive. \n\nHowever, we cannot flip two numbers if they are next to each other.\n- The house robber algorithm can find the maximum value we can flip (steal) with this restriction.\n\n> Note that we need to add the **double** of the flipped value to the sum of all numbers.\n> Also note that we cannot flip the very first negative number.\n\n**C++**\n```cpp\nlong long maximumTotalCost(vector<int>& nums) {\n long long cur = 0, prev = 0;\n for (int i = 1; i < nums.size(); ++i)\n cur = exchange(prev, max(cur, prev)) - nums[i];\n return accumulate(begin(nums), end(nums), 0LL) + 2LL * max(cur, prev);\n}\n```\n\n#### Original Version\nThe equivalent original code, which follows the description above more closelly.\n\n**C++**\n```cpp\nlong long maximumTotalCost(vector<int>& nums) {\n long long res = nums[0], cur = 0, prev = 0;\n for (int i = 1; i < nums.size(); ++i) {\n res += nums[i];\n if (nums[i] >= 0) {\n res += 2LL * max(cur, prev);\n cur = prev = 0;\n }\n else\n cur = exchange(prev, max(cur, prev)) - nums[i];\n }\n return res + 2LL * max(cur, prev);\n}\n``` | 19 | 3 | ['C'] | 2 |
maximize-total-cost-of-alternating-subarrays | Easy Video Solution 🔥 || How to 🤔 in Interview || Dynamic programming || Memoization 🔥 | easy-video-solution-how-to-in-interview-zo50z | If you like the solution Please Upvote and subscribe to my youtube channel\n\n# Easy Video Explanation\n\nhttps://youtu.be/ZzOdbGx3KAs\n\n# Code\n\nclass Soluti | ayushnemmaniwar12 | NORMAL | 2024-06-23T05:50:18.922565+00:00 | 2024-06-23T07:08:01.932793+00:00 | 459 | false | ***If you like the solution Please Upvote and subscribe to my youtube channel***\n\n# ***Easy Video Explanation***\n\nhttps://youtu.be/ZzOdbGx3KAs\n\n# Code\n```\nclass Solution {\npublic:\n long long solve(int i,int f,int n,vector<int>&v,vector<vector<long long>>&dp) {\n if(i==n)\n return 0;\n if(dp[i][f]!=-1)\n return dp[i][f];\n long long a=0,b=0;\n if(f==0) {\n a=v[i]+solve(i+1,0,n,v,dp);\n b=-1*v[i]+solve(i+1,1,n,v,dp);\n } else {\n a=v[i]+solve(i+1,0,n,v,dp);\n return dp[i][f]=a;\n }\n return dp[i][f]=max(a,b);\n }\n long long maximumTotalCost(vector<int>& v) {\n int n = v.size();\n vector<vector<long long>>dp(n,vector<long long>(2,-1));\n long long ans = v[0]+solve(1,0,n,v,dp);\n return ans;\n }\n};\n``` | 10 | 3 | ['Dynamic Programming', 'Memoization', 'C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | [Java/Python 3] DP O(n) codes, from space O(n) to O(1). | javapython-3-dp-on-codes-from-space-on-t-puos | Except nums[0], we can always choose an item in the input either as original value, or flip its sign plus keep previous item as original value, and there is no | rock | NORMAL | 2024-06-23T04:02:17.847474+00:00 | 2024-06-28T18:09:35.484054+00:00 | 459 | false | Except `nums[0]`, we can always choose an item in the input either as original value, or flip its sign plus keep previous item as original value, and there is no other options; Therefore, starting from `i = 1, nums[i]`, our state transition function is `dp[i + 1] = max(dp[i] + nums[i], dp[i - 1] + nums[i - 1] - nums[i]`.\n\n\n```java\n public long maximumTotalCost(int[] nums) {\n int n = nums.length;\n long[] dp = new long[n + 1];\n dp[1] = nums[0];\n for (int i = 1; i < n; ++i) {\n dp[i + 1] = Math.max(dp[i] + nums[i], dp[i - 1] + nums[i - 1] - nums[i]);\n }\n return dp[n];\n }\n```\n\n```python\n def maximumTotalCost(self, nums: List[int]) -> int:\n dp = [0, nums[0]]\n for x, y in pairwise(nums):\n dp.append(max(dp[-1] + y, dp[-2] + x - y))\n return dp[-1]\n```\n\n**Analysis:**\nTime: O(n), space: O(n).\n\n----\n\nSpace Optimization:\n\nSince each value in `dp` array depends at most previous `2` items, we only need a `dp` array of size `3`.\n\n```java\n public long maximumTotalCost(int[] nums) {\n long[] dp = {0, nums[0], 0};\n for (int i = 1; i < nums.length; ++i) {\n dp[(i + 1) % 3] = Math.max(dp[i % 3] + nums[i], dp[(i - 1) % 3] + nums[i - 1] - nums[i]);\n }\n return dp[nums.length % 3];\n }\n```\n\n```python\n def maximumTotalCost(self, nums: List[int]) -> int:\n dp, n = [0, nums[0], 0], len(nums)\n for i in range(1, n):\n dp[(i + 1) % 3] = max(dp[i % 3] + nums[i], dp[(i - 1) % 3] + nums[i - 1] - nums[i])\n return dp[n % 3]\n```\n\n**@xxxxkav** provided more readable codes as follows:\n\n```java\n public long maximumTotalCost(int[] nums) {\n long[] dp = {0, nums[0]};\n for (int i = 1; i < nums.length; ++i) {\n dp = new long[]{dp[1], Math.max(dp[0] + nums[i - 1] - nums[i], dp[1] + nums[i])};\n }\n return dp[1];\n }\n```\n```python\n def maximumTotalCost(self, nums: List[int]) -> int:\n dp = (0, nums[0])\n for x, y in pairwise(nums):\n dp = (dp[1], max(dp[0] + x - y, dp[1] + y))\n return dp[1]\n```\n**Analysis:**\nTime: O(n), space: O(1).\n | 9 | 0 | ['Dynamic Programming', 'Java', 'Python3'] | 1 |
maximize-total-cost-of-alternating-subarrays | Python 3 || 4 lines, iteration with dp || T/S: 99% / 98% | python-3-4-lines-iteration-with-dp-ts-99-alae | Here\'s the plan:\n1. We initialize dp1 and dp2 with the first element of nums. These integer variables keep track of the current maximum total that can be obta | Spaulding_ | NORMAL | 2024-06-23T18:16:33.468146+00:00 | 2024-07-02T15:03:49.360495+00:00 | 49 | false | Here\'s the plan:\n1. We initialize `dp1` and `dp2` with the first element of `nums`. These integer variables keep track of the current maximum total that can be obtained by either adding(`dp1`) or subtracting(`dp2`) the current element to the previous maximum total.\n\n1. We iterate though `nums`, updating `dp1` and `dp2` as described in 1) above and selecting the greater of the two as the current maximum total.\n\n1. We return the maximum value as the solution.\n```\nclass Solution:\n def maximumTotalCost(self, nums: List[int]) -> int:\n\n dp1 = dp2 = nums.pop(0) # <-- 1)\n\n for n in nums:\n dp1, dp2 = max(dp1, dp2) + n, dp1 - n # <-- 2)\n\n return max(dp1, dp2) # <-- 3)\n```\n[https://leetcode.com/problems/maximize-total-cost-of-alternating-subarrays/submissions/1298064470/](https://leetcode.com/problems/maximize-total-cost-of-alternating-subarrays/submissions/1298064470/)\n\nI could be wrong, but I think that time complexity is *O*(*N*) and space complexity is *O*(1), in which *N* ~ `len(nums)`. | 8 | 0 | ['Python', 'Python3'] | 0 |
maximize-total-cost-of-alternating-subarrays | Solution By Dare2Solve | Detailed Explanation | 1D DP | solution-by-dare2solve-detailed-explanat-c23m | Explanation []\nauthorslog.vercel.app/blog/fwKyrRoXX5\n\n\n# Code\n\ncpp []\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n | Dare2Solve | NORMAL | 2024-06-23T04:06:01.352072+00:00 | 2024-06-23T04:07:24.518423+00:00 | 739 | false | ```Explanation []\nauthorslog.vercel.app/blog/fwKyrRoXX5\n```\n\n# Code\n\n```cpp []\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n vector<long long> dp(n + 1, -LLONG_MAX);\n dp[0] = 0;\n dp[1] = nums[0];\n for (int i = 1; i < n; ++i) {\n dp[i + 1] = max(dp[i] + nums[i], dp[i - 1] + nums[i - 1] - nums[i]);\n }\n return dp[n];\n }\n};\n```\n\n```Python []\nclass Solution:\n def maximumTotalCost(self, nums):\n n = len(nums)\n dp = [0] * (n + 1)\n dp[1] = nums[0]\n for i in range(1, n):\n dp[i + 1] = max(dp[i] + nums[i], dp[i - 1] + nums[i - 1] - nums[i])\n return dp[n]\n```\n\n```java []\nclass Solution {\n public long maximumTotalCost(int[] nums) {\n int n = nums.length;\n long[] dp = new long[n + 1];\n dp[0] = 0;\n dp[1] = nums[0];\n for (int i = 1; i < n; ++i) {\n dp[i + 1] = Math.max(dp[i] + nums[i], dp[i - 1] + nums[i - 1] - nums[i]);\n }\n return dp[n];\n }\n}\n```\n\n```javascript []\n/**\n * @param {number[]} nums\n * @return {number}\n */\nvar maximumTotalCost = function (nums) {\n var dp = new Array(nums.length + 1);\n dp[0] = 0;\n dp[1] = nums[0];\n for (var i = 1; i < nums.length; ++i) {\n dp[i + 1] = Math.max(dp[i] + nums[i], dp[i - 1] + nums[i - 1] - nums[i]);\n }\n return dp[nums.length];\n};\n```\n\n```Go []\nfunc maximumTotalCost(nums []int) int64 {\n\tn := len(nums)\n\tdp := make([]int64, n+1)\n\tdp[1] = int64(nums[0])\n\tfor i := 1; i < n; i++ {\n\t\tdp[i+1] = max(dp[i]+int64(nums[i]), dp[i-1]+int64(nums[i-1])-int64(nums[i]))\n\t}\n\treturn dp[n]\n}\n\nfunc max(a, b int64) int64 {\n\tif a > b {\n\t\treturn a\n\t}\n\treturn b\n}\n```\n\n```csharp []\npublic class Solution {\n public long MaximumTotalCost(int[] nums) {\n int n = nums.Length;\n long[] dp = new long[n + 1];\n dp[0] = 0;\n dp[1] = nums[0];\n for (int i = 1; i < n; ++i) {\n dp[i + 1] = Math.Max(dp[i] + nums[i], dp[i - 1] + nums[i - 1] - nums[i]);\n }\n return dp[n];\n }\n}\n``` | 7 | 0 | ['Dynamic Programming', 'Python', 'C++', 'Java', 'Go', 'Python3', 'JavaScript', 'C#'] | 0 |
maximize-total-cost-of-alternating-subarrays | DP: Recursion Memo-> tabular with space O(1)||46ms beats 100% | dp-recursion-memo-tabular-with-space-o14-w5mh | Intuition\n Describe your first thoughts on how to solve this problem. \nTry DP.\nUse Recursion with Memo-> Tabular with optimized space.\n# Approach\n Describe | anwendeng | NORMAL | 2024-06-23T07:50:49.951834+00:00 | 2024-06-23T07:50:49.951866+00:00 | 476 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nTry DP.\nUse Recursion with Memo-> Tabular with optimized space.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Define recursive function `long long f(int i, bool minus, vector<int>& nums)` with cache `long long dp[100000][2]`\n2. The recursive relations are as follows\n```\nsum=((!minus)? nums[i]: -1*nums[i]) + f(i+1, !minus, nums);\nsum= max(sum, nums[i] + f(i+1, 1, nums));\n```\n3. 2nd code is a conversion from recursive version into an iterative version in which the space need is reduced by `&1` trick\n```\nfor(int i=n-1; i>=0; i--){\n long long x=nums[i];\n dp[i&1][0]=x+dp[(i+1)&1][1];\n dp[i&1][1]=-x+dp[(i+1)&1][0];\n dp[i&1][0]=max(dp[i&1][0], x+dp[(i+1)&1][1]);\n dp[i&1][1]=max(dp[i&1][1], x+dp[(i+1)&1][1]);\n}\n```\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(n) \\to O(1)$$\n# Code recursive DP|| 59ms beats 100%\n```\nclass Solution {\npublic:\n int n;\n long long dp[100000][2];\n long long f(int i, bool minus, vector<int>& nums){\n if(i == n) return 0;\n if(dp[i][minus] != LONG_MIN) return dp[i][minus];\n long long sum=((!minus)?nums[i]:-1*nums[i])+f(i+1, !minus, nums);\n sum = max(sum, nums[i] + f(i+1, 1, nums));\n return dp[i][minus] = sum;\n }\n \n long long maximumTotalCost(vector<int>& nums) {\n n= nums.size();\n fill(&dp[0][0], &dp[0][0]+2*n, LONG_MIN);\n return f(0, 0, nums);\n }\n};\n\n\n\nauto init = []() {\n ios::sync_with_stdio(0);\n cin.tie(0);\n cout.tie(0);\n return \'c\';\n}();\n```\n# C++ iterative DP with O(1) space||49ms beats 100%\n```\nclass Solution {\npublic: \n long long maximumTotalCost(vector<int>& nums) {\n const int n = nums.size();\n long long dp[2][2]={{0}};\n for(int i=n-1; i>=0; i--){\n long long x=nums[i];\n dp[i&1][0]=x+dp[(i+1)&1][1];\n dp[i&1][1]=-x+dp[(i+1)&1][0];\n dp[i&1][0]=max(dp[i&1][0], x+dp[(i+1)&1][1]);\n dp[i&1][1]=max(dp[i&1][1], x+dp[(i+1)&1][1]);\n }\n return dp[0][0];\n }\n};\n\n\n\nauto init = []() {\n ios::sync_with_stdio(0);\n cin.tie(0);\n cout.tie(0);\n return \'c\';\n}();\n``` | 6 | 0 | ['Dynamic Programming', 'C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Simple DP Approach || Memorization || Java || C++ || Python | simple-dp-approach-memorization-java-c-p-pthc | Intuition\n Describe your first thoughts on how to solve this problem. \nWe need to consider only subarrays of size 1 and 2 to maximize the cost.\n\n\n\n\n\n\n\ | jeevanraj73 | NORMAL | 2024-06-23T05:55:11.410942+00:00 | 2024-06-23T05:55:11.410970+00:00 | 652 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe need to consider only subarrays of size 1 and 2 to maximize the cost.\n\n\n\n\n\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe proposed solution uses a dynamic programming approach with space optimization. Instead of maintaining an entire DP array, it uses two variables (secondPrev and firstPrev) to keep track of the maximum costs at previous states. This is more efficient in terms of space complexity. Let\'s go through the solution step-by-step:\n\nBase Case:\nIf there is only one element in nums, the maximum total cost is the element itself.\n\nInitial Values:\n\nsecondPrev holds the maximum cost for the subarray ending two indices before the current index.\nfirstPrev holds the maximum cost for the subarray ending at the previous index.\nInitially, secondPrev is set to the first element (nums[0]), as the subarray consisting of the first element only has that cost.\nfirstPrev is set to the maximum of two possible subarrays: [nums[0], nums[1]] or [nums[0], -nums[1]], which corresponds to nums[0] + nums[1] or nums[0] - nums[1].\nIterate Through the Array:\n\nFor each element starting from the third one (index 2), calculate the maximum cost considering extending the subarray from two indices back or extending the subarray from one index back.\nUpdate secondPrev and firstPrev for the next iteration.\nReturn Result:\n\nAfter processing all elements, firstPrev will contain the maximum total cost.\n\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n\n\n# Java Code\n```\nclass Solution {\n public long maximumTotalCost(int[] nums) {\n if (nums.length == 1) {\n return nums[0];\n }\n \n // Initial values for the dynamic programming approach\n long secondPrev = nums[0];\n long firstPrev = Math.max((long) nums[0] + nums[1], (long) nums[0] - nums[1]);\n \n for (int i = 2; i < nums.length; i++) {\n // Calculate the maximum cost for the subarray ending at the current index\n long current = Math.max(secondPrev + nums[i - 1] - nums[i], firstPrev + nums[i]);\n // Update the previous values for the next iteration\n secondPrev = firstPrev;\n firstPrev = current;\n }\n \n return firstPrev;\n }\n}\n```\n# Python Code\n```\nclass Solution:\n def maximumTotalCost(self, nums: List[int]) -> int:\n # If there is only one element in the list then the maximum total cost will be the element itself \n if len(nums) == 1:\n return nums[0]\n \n # Initial values for the dynamic programming approach\n secondPrev = nums[0]\n firstPrev = max(nums[0] + nums[1], nums[0] - nums[1])\n \n for i in range(2, len(nums)):\n # Calculate the maximum cost for the subarray ending at the current index\n current = max(secondPrev + nums[i-1] - nums[i], firstPrev + nums[i])\n # Update the previous values for the next iteration\n secondPrev = firstPrev\n firstPrev = current\n \n return firstPrev\n```\n# C++ Code\n```\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n \n if (nums.size() == 1) {\n return nums[0];\n }\n \n // Initial values for the dynamic programming approach\n long long secondPrev = nums[0];\n long long firstPrev = std::max((long long)nums[0] + nums[1], (long long)nums[0] - nums[1]);\n \n for (size_t i = 2; i < nums.size(); i++) {\n // Calculate the maximum cost for the subarray ending at the current index\n long long current = std::max(secondPrev + nums[i - 1] - nums[i], firstPrev + nums[i]);\n // Update the previous values for the next iteration\n secondPrev = firstPrev;\n firstPrev = current;\n }\n \n return firstPrev;\n }\n};\n```\n\n\n | 6 | 0 | ['Dynamic Programming', 'Memoization', 'C++', 'Java', 'Python3'] | 1 |
maximize-total-cost-of-alternating-subarrays | DP || Pick/ Not Pick || O(n) | dp-pick-not-pick-on-by-thiennk-5ohm | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Thiennk | NORMAL | 2024-06-23T04:02:11.494428+00:00 | 2024-06-23T07:09:13.469545+00:00 | 336 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(2*n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n fun maximumTotalCost(nums: IntArray): Long {\n if (nums.size == 1) return nums[0].toLong();\n if (nums.size == 2) {\n return maxOf(nums[0] + nums[1], nums[0] - nums[1]).toLong();\n }\n\n var a: Array<LongArray> = Array(nums.size, {LongArray(2) {0}})\n a[0][0] = nums[0].toLong();\n a[1][0] = a[0][0] + nums[1];\n a[1][1] = a[0][0] - nums[1];\n\n for (i in 2 until nums.size) {\n a[i][0] = maxOf(a[i-1][0] + nums[i], a[i-1][1] + nums[i])\n a[i][1] = a[i-1][0] - nums[i];\n }\n\n return maxOf(a[nums.size-1][0], a[nums.size-1][1])\n }\n}\n``` | 6 | 0 | ['Dynamic Programming', 'Kotlin'] | 0 |
maximize-total-cost-of-alternating-subarrays | C++ || Simple DP Solution | c-simple-dp-solution-by-_potter-t0lk | Code\n\nclass Solution {\npublic:\n // Consider index starts with 1\n long long dp[100001][2];\n long long topDown(int i, int oddIndex, vector<int> &nu | _Potter_ | NORMAL | 2024-06-23T04:31:44.459153+00:00 | 2024-06-23T04:38:17.067336+00:00 | 321 | false | # Code\n```\nclass Solution {\npublic:\n // Consider index starts with 1\n long long dp[100001][2];\n long long topDown(int i, int oddIndex, vector<int> &nums){\n if(i == nums.size()){\n return 0;\n }\n if(dp[i][oddIndex] != -1) {\n return dp[i][oddIndex];\n }\n // Continue in the old subarray\n long long dontBreak = 0;\n if(oddIndex){\n dontBreak = topDown(i+1, !oddIndex, nums) + nums[i];\n }\n else{\n dontBreak = topDown(i+1, !oddIndex, nums) - nums[i];\n }\n // Start new subarray\n long long breakIt = topDown(i+1, true, nums) + nums[i];\n return dp[i][oddIndex] = max(dontBreak, breakIt);\n }\n \n \n long long maximumTotalCost(vector<int>& nums) {\n memset(dp, -1, sizeof(dp));\n return topDown(0, true, nums);\n }\n};\n```\n```\ncout << "Upvote the solution if you like it!!" <<endl;\n``` | 5 | 0 | ['Dynamic Programming', 'C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | [Beats 100%] 4 approaches HOW TO BUILD UP TO OPTIMAL SOLUTION + Full thought process | beats-100-4-approaches-how-to-build-up-t-z7jv | Approach\nThe goal is to maximize the sum of alternating subarrays.\n\nAn alternating subarray starts by adding the first element, then subtracts the 2nd, adds | AlecLC | NORMAL | 2024-06-23T04:17:21.766916+00:00 | 2024-06-23T04:17:21.766938+00:00 | 205 | false | # Approach\nThe goal is to maximize the sum of alternating subarrays.\n\nAn alternating subarray starts by adding the first element, then subtracts the 2nd, adds the third, etc.\nIn other words, add up all the even indices and subtract the odd ones.\n\nThe first thing to do is look at the constraints. Solutions TLE around 10<sup>10</sup>, so with input length 10<sup>5</sup>, we need a linear or linearithmic (nlogn) solution to pass.\n\n\nYou can try to maximize elements included in even subarrays or minimize the odds, but they may disagree.\nYou can try greedy, but then a locally optimal decision may not be globally optimal.\nThis analysis is a good indicator that the best score at a position must depend on subproblems to get a globally optimal result.\n\nAt this point, dp/recursion seems like our best bet. The recurrence relation isn\'t too complicated: just take the max of extending an array or starting a new one\n\nHere\'s a basic outline of what that looks like:\n```python3\n@cache\ndef find_max(pos, cur_subarray_start):\n parity = 1 if ((pos - cur_subarray_start) % 2 == 0) else -1\n extend_subarray = nums[pos] * parity + find_max(pos + 1, cur_start)\n new_subarray = nums[pos] + find_max(pos + 1, pos + 1)\n return max(extend_subarray, new_subarray)\n```\nThis is where the problem gets tricky. The above code is $O(n^2)$ and it\'s not immediately obvious how to make it linear.\n\n**Key Insight**: We only need the position of the current subarray start to determine parity. Therefore, we can simply pass in parity as the 2nd argument.\nThen, we make at max 2 calls at each position, meaning we have linear time complexity. If this is confusing, think about the size of the memoization table. It\'s now $O(2n)$ because there could be a True and False for each item.\n\nWith this insight, we can form a working solution:\n```python3\n# TC: O(n)\n# SC: O(n)\ndef maximumTotalCost(self, nums: List[int]) -> int:\n n = len(nums)\n dp = {} # dp[i] = best score up to i\n \n def form_subarrays(pos, is_even):\n if (pos, is_even) in dp:\n return dp[(pos, is_even)]\n \n if pos == n:\n return 0\n \n extend_subarray = nums[pos] * (1 if is_even else -1) + form_subarrays(pos + 1, not is_even)\n new_subarray = nums[pos] + form_subarrays(pos+1, True)\n dp[(pos, is_even)] = max(extend_subarray, new_subarray)\n return dp[(pos, is_even)]\n \n return form_subarrays(0, True)\n```\n\nCan we do better? Yes! In order to recognize how, we first need to convert top-down to bottom up:\n```python3\n# TC: O(n)\n# SC: O(n)\ndef maximumTotalCost(nums: List[int]) -> int:\n n = len(nums)\n # dp[i][0] stores the best score up to i when the last element is at an even position\n # dp[i][1] stores the best score up to i when the last element is at an odd position\n dp = [[0] * 2 for _ in range(n + 1)]\n \n for pos in range(n - 1, -1, -1):\n dp[pos][0] = max(nums[pos] + dp[pos + 1][1], nums[pos] + dp[pos + 1][0])\n dp[pos][1] = max(-nums[pos] + dp[pos + 1][0], nums[pos] + dp[pos + 1][1])\n \n return dp[0][0]\n```\n\nThe final thing to realize is that we only use the last 2 rows of the DP table. Therefore, we can make the solution O(1) space by replacing dp[pos+1] with \n# Solution\n```python3\ndef maximumTotalCost(self, nums: List[int]) -> int:\n n = len(nums)\n \n # Initialize variables to store the current and previous states\n cur = [0, 0]\n prev = [0, 0]\n \n # Iterate from the end of the array to the beginning\n for pos in range(n - 1, -1, -1):\n # Compute the current states based on the previous states\n prev[0] = max(nums[pos] + cur[1], nums[pos] + cur[0])\n prev[1] = max(-nums[pos] + cur[0], nums[pos] + cur[1])\n \n # Update the current states to the previous states\n cur[0], cur[1] = prev[0], prev[1]\n \n # The result is the maximum cost starting from the beginning with an even position\n return cur[0]\n```\n\n# Complexity\n\n* Time Complexity: $O(n)$\n* Space Complexity: $O(1)$\n\n\n\n | 5 | 0 | ['Python3'] | 0 |
maximize-total-cost-of-alternating-subarrays | Take not take dp | take-not-take-dp-by-techtinkerer-2nk7 | \n\n# Complexity\n- Time complexity:O(n)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity:O(n)\n Add your space complexity here, e.g. O(n) \n\n | TechTinkerer | NORMAL | 2024-06-23T07:39:18.315330+00:00 | 2024-06-23T07:40:05.226438+00:00 | 256 | false | \n\n# Complexity\n- Time complexity:O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n#define ll long long\nclass Solution {\npublic:\n vector<int> nums;\n int n;\n ll dp[100001][2];\n \n ll solve(int index,bool pos){\n if(index==n)\n return 0;\n \n if(dp[index][pos]!=-1)\n return dp[index][pos];\n \n if(pos){\n return dp[index][pos]=max(1ll*nums[index]+solve(index+1,0),1ll*nums[index]+solve(index+1,1));\n }\n else{\n return dp[index][pos]=-1ll*nums[index]+solve(index+1,1);\n }\n }\n \n long long maximumTotalCost(vector<int>& nums) {\n this->nums=nums;\n this->n=nums.size();\n memset(dp,-1,sizeof(dp));\n return solve(0,1);\n }\n};\n``` | 4 | 0 | ['Dynamic Programming', 'Greedy', 'C++'] | 1 |
maximize-total-cost-of-alternating-subarrays | C++/Python3/Java Solution | Beats 100% | 4 Line ✔️ | cpython3java-solution-beats-100-4-line-b-5036 | Intuition\nUpon first glance, the problem seems to involve a pattern of alternating +ve and -ve signs. It appears as if we start with a positive sign and altern | satyam2001 | NORMAL | 2024-06-24T13:38:04.100549+00:00 | 2024-09-21T09:39:37.680342+00:00 | 174 | false | # Intuition\nUpon first glance, the problem seems to involve a pattern of alternating `+ve and -ve signs`. It appears as if we start with a positive sign and alternate signs thereafter, and we can switch all the subsequent signs if the current sign is negative. To maximize the cost, we need to determine the optimal positions where we can swap signs. Considering all possibilities, the time complexity would be $$\uD835\uDC42(2^\uD835\uDC5B)$$.\n\nHowever, a closer examination reveals that we can simplify our approach by considering two cases: treating the current element\'s sign as positive or negative and maintaining its maximum value accordingly.\n\nWe just need to store the maximum value of cost upto current element where the subarray ends with `+ve` or `-ve` sign and calculate the value of thereafter based on current `+ve` and `-ve` sign value.\n\nHere\'s how the signs of the previous element should align in these two cases:\n\n- Current element as `+ve`: The previous element can either be `+ve` (if we start a new subarray) or `-ve` (if we continue the current subarray).\n- Current element as `-ve`: The previous element must be `+ve` to maintain the alternating sign pattern.\n\n# Approach\n`a` and `b` are the value of maximum cost of nums from 0 to current element ending with `+ve` and `-ve` signs respectively.\n\n# Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(1)$$\n\n# Code\n\n```python3 []\nclass Solution:\n def maximumTotalCost(self, nums: List[int]) -> int:\n a, b = -inf, 0\n for i in nums:\n a, b = max(a, b) + i, a - i\n return max(a, b)\n```\n```C++ []\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n long long a = INT_MIN;\n long long b = 0;\n for(int& i: nums) {\n long long c = a;\n a = max(c, b) + i;\n b = c - i;\n }\n return max(a, b);\n }\n};\n```\n```java []\nclass Solution {\n public long maximumTotalCost(int[] nums) {\n long a = Integer.MIN_VALUE;\n long b = 0;\n for (int i : nums) {\n long c = a;\n a = Math.max(c, b) + i;\n b = c - i;\n }\n return Math.max(a, b);\n }\n}\n```\n\n | 3 | 0 | ['Dynamic Programming', 'C++', 'Java', 'Python3'] | 0 |
maximize-total-cost-of-alternating-subarrays | ✅✅ Detailed explanation || Recursive, Memoized Solution 😱😃 | detailed-explanation-recursive-memoized-7pzfn | Intuition\n Describe your first thoughts on how to solve this problem. \nThe problem requires splitting the array into subarrays to maximize the total cost, whe | Rishu_Raj5938 | NORMAL | 2024-06-23T13:00:16.151254+00:00 | 2024-06-23T13:02:56.564457+00:00 | 160 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem requires splitting the array into subarrays to maximize the total cost, where the cost of each subarray alternates between adding and subtracting elements. We use a recursive approach with memoization to solve this efficiently.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nRecursive Function with Memoization:\n\nThe solve function is a recursive function with memoization to avoid recalculating results for the same state multiple times.\n\nIt has two parameters: the current index idx and a boolean pos which indicates whether the current state is positive or negative.\n\nDP Table:\n\nThe DP table dp is a 2D array where dp[idx][0] and dp[idx][1] store the maximum cost starting from index idx with pos being false or true, respectively.\n\nBase Case:\n\nWhen idx is out of bounds (idx >= n), the function returns 0.\n\nMemoization Check:\n\nBefore performing any calculations, the function checks if the result is already computed and stored in dp[idx][pos].\n\nState Transition:\n\nNote -> pos indicates whether the current value should be multiplied by -1.\n\npos = true means the current value should not be multiplied by -1.\n\npos = false means the current value should be multiplied by -1.\n\nIf pos is true, it adds nums[idx] to the result and calls solve for the next index with pos being false.\n\nIf pos is false, it computes two potential results: taking the current element with a negative sign and moving to the next element with pos being true, or not taking the current element and moving to the next element with pos being false. \n\nThe maximum of these two results is stored in dp[idx][pos].\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe DP table has dimensions [n][2], where n is the size of the input array nums.\n\nThe function solve is called at most 2 * n times, each taking \nconstant time O(1) for memoization checks and state transitions.\n\nHence, the overall time complexity is O(n).\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity includes the DP table and the recursion stack.\n\nThe DP table dp requires O(2 * n) = O(n) space.\n\nThe recursion stack can go up to a maximum depth of n, requiring O(n) space.\n\nTherefore, the total space complexity is O(n).\n\n# Code\n```C++ []\n\nclass Solution {\npublic:\n \n int n;\n long long dp[100004][2];\n \n long long solve(vector<int>& nums, int idx, bool pos) {\n \n if(idx >= n) return 0;\n \n if(dp[idx][pos] != -1) return dp[idx][pos];\n \n if(pos) {\n dp[idx][pos] = nums[idx] + solve(nums, idx+1, false);\n }\n else {\n long long take = solve(nums, idx+1, true)-nums[idx];\n long long not_take = solve(nums, idx+1, false)+nums[idx];\n dp[idx][pos] = max(take, not_take);\n }\n \n return dp[idx][pos];\n }\n \n long long maximumTotalCost(vector<int>& nums) {\n \n n = nums.size();\n \n memset(dp, -1, sizeof(dp));\n \n return solve(nums, 0, true);\n }\n};\n```\n```python []\nclass Solution:\n def maximumTotalCost(self, nums: List[int]) -> int:\n n = len(nums)\n dp = [[-1, -1] for _ in range(n)]\n\n def solve(idx: int, pos: bool) -> int:\n if idx >= n:\n return 0\n\n if dp[idx][pos] != -1:\n return dp[idx][pos]\n\n if pos:\n dp[idx][pos] = nums[idx] + solve(idx + 1, False)\n else:\n take = solve(idx + 1, True) - nums[idx]\n not_take = solve(idx + 1, False) + nums[idx]\n dp[idx][pos] = max(take, not_take)\n\n return dp[idx][pos]\n\n return solve(0, True)\n\n\n```\n```java []\nimport java.util.Arrays;\n\nclass Solution {\n private int n;\n private long[][] dp;\n\n private long solve(int[] nums, int idx, boolean pos) {\n if (idx >= n) return 0;\n\n if (dp[idx][pos ? 1 : 0] != -1) return dp[idx][pos ? 1 : 0];\n\n if (pos) {\n dp[idx][1] = nums[idx] + solve(nums, idx + 1, false);\n return dp[idx][1];\n } else {\n long take = solve(nums, idx + 1, true) - nums[idx];\n long notTake = solve(nums, idx + 1, false) + nums[idx];\n dp[idx][0] = Math.max(take, notTake);\n return dp[idx][0];\n }\n }\n\n public long maximumTotalCost(int[] nums) {\n n = nums.length;\n dp = new long[n][2];\n for (int i = 0; i < n; i++) {\n Arrays.fill(dp[i], -1);\n }\n return solve(nums, 0, true);\n }\n\n public static void main(String[] args) {\n Solution sol = new Solution();\n int[] nums = {1, 2, 3, 4, 5};\n System.out.println(sol.maximumTotalCost(nums)); \n }\n}\n\n```\n | 3 | 0 | ['Memoization', 'C++', 'Java', 'Python3'] | 1 |
maximize-total-cost-of-alternating-subarrays | C++ O(N) solution . | c-on-solution-by-deleted_user-1eq7 | Intuition\n Describe your first thoughts on how to solve this problem. \nSince in a subarray, sum is el1 - el2 + el3 - el4 ....\nWe can use subarray of size ei | deleted_user | NORMAL | 2024-06-23T04:23:00.183449+00:00 | 2024-06-23T04:23:00.183467+00:00 | 43 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nSince in a subarray, sum is el1 - el2 + el3 - el4 ....\nWe can use subarray of size either 1 or 2 .\nRest is DP.\n# Complexity\n- Time complexity:$$O(N)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(N)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n#define ll long long\nclass Solution {\npublic:\n ll maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n vector<ll> dp(n, 0);\n dp[n-1] = nums[n-1];\n for (int i = n - 2; i >= 0; --i) {\n dp[i] = nums[i];\n if (i + 1 < n) {\n dp[i] = max(dp[i], nums[i] - nums[i+1] + (i + 2 < n ? dp[i+2] : 0));\n }\n dp[i] = max(dp[i], nums[i] + dp[i+1]);\n }\n return dp[0];\n }\n};\n\n``` | 3 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | 2d DP memoized solution do upvote me 😎😎 | 2d-dp-memoized-solution-do-upvote-me-by-21hns | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | rahulX64 | NORMAL | 2024-06-26T08:47:49.659066+00:00 | 2024-06-26T08:47:49.659094+00:00 | 33 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n vector<vector<long long>> dp;\n\n long long solve(int ind, vector<int>& nums, int p) {\n if (ind == nums.size())\n return 0;\n\n if (dp[ind][p] != LLONG_MIN)\n return dp[ind][p];\n\n long long cont, ncont;\n if (p == 0) {\n cont = nums[ind] + solve(ind + 1, nums, 1);\n ncont = nums[ind] + solve(ind + 1, nums, 0);\n } else {\n \n cont = (-1) * nums[ind] + solve(ind + 1, nums, 0);\n ncont = (-1) * nums[ind] + solve(ind + 1, nums, 0);\n \n }\n\n return dp[ind][p] = max(cont, ncont);\n }\n\n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n dp.resize(n, vector<long long>(2, LLONG_MIN));\n\n return solve(0, nums, 0);\n }\n};\n\n``` | 2 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Simple || DP | Easy | C++ | O(N) | Seven line code | optimal | simple-dp-easy-c-on-seven-line-code-opti-nsgh | Intuition\n Describe your first thoughts on how to solve this problem. \nTo maximize the total cost of subarrays, we need to strategically decide where to split | gavnish_kumar | NORMAL | 2024-06-24T08:46:53.279079+00:00 | 2024-06-24T08:46:53.279117+00:00 | 91 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nTo maximize the total cost of subarrays, we need to strategically decide where to split the array. The cost of a subarray nums[l..r] alternates between adding and subtracting the elements based on their positions (even-indexed elements are added, odd-indexed elements are subtracted). This gives us two main tasks:\n\nCalculate the cost of continuing the current subarray.\nCalculate the cost of starting a new subarray.\nOur goal is to use dynamic programming to determine the optimal points to split the array to achieve the maximum possible total cost.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n**Cost Function Understanding:** The cost of subarray nums[l..r] can be broken down as:\nEven indexed elements within the subarray are added.\nOdd indexed elements within the subarray are subtracted.\n\n**Dynamic Programming Setup:**\nUse a 2D DP table dp[i][check] where i represents the current index and check represents the current sign (positive if check is true, negative if check is false).\ndp[i][true] will store the maximum cost up to index i considering nums[i] as positive.\ndp[i][false] will store the maximum cost up to index i considering nums[i] as negative.\n\n**Recursive Function with Memoization:**Define a recursive function solve(nums, i, check, dp) that computes the maximum cost from index i onwards with the current sign indicated by check.\n**Base Case:** If i exceeds the array bounds, return 0.\n**Memoization: **If the result is already computed, return it.\n**Compute two possible costs:**\nContinue the current subarray.\nStart a new subarray.\nOptimal Solution:\n\nStart from the first element with a positive sign and recursively compute the maximum cost using the solve function.\nReturn the computed maximum cost.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n O(N) where N is the length of array\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n O(N) where N is the length of array\n\n# Code\n```\nclass Solution {\npublic:\n long long int solve(vector<int>&nums,int i,bool check,vector<vector<long long int>> & dp){\n if(i>=nums.size()) return 0;\n if(dp[i][check]!=-1) return dp[i][check];\n long long int part= solve(nums,i+1,true,dp)+ (check ? nums[i]: -1*nums[i]);\n long long int select= solve(nums,i+1,!check,dp)+ (check? nums[i]: -1*nums[i]);\n return dp[i][check]=max(part,select);\n }\n long long maximumTotalCost(vector<int>& nums) {\n vector<vector<long long int>> dp(nums.size(),vector<long long int >(2,-1));\n return solve(nums,0,true,dp);\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | DP. One line solution. Time O(n). Space O(1) | dp-one-line-solution-time-on-space-o1-by-jfeh | \nclass Solution:\n def maximumTotalCost(self, n: List[int]) -> int:\n return reduce(lambda d, m:(d[1], max(d[0]+m[0]-m[1], d[1]+m[1])), pairwise(n), | xxxxkav | NORMAL | 2024-06-23T22:26:37.485132+00:00 | 2024-06-23T23:35:58.247570+00:00 | 38 | false | ```\nclass Solution:\n def maximumTotalCost(self, n: List[int]) -> int:\n return reduce(lambda d, m:(d[1], max(d[0]+m[0]-m[1], d[1]+m[1])), pairwise(n), (0,n[0]))[1]\n```\n> More readable\n```\nclass Solution:\n def maximumTotalCost(self, nums: List[int]) -> int:\n dp = (0, nums[0])\n for a, b in pairwise(nums):\n dp = (dp[1], max(dp[0]+a-b, dp[1]+b))\n return dp[1]\n``` | 2 | 0 | ['Dynamic Programming', 'Python3'] | 1 |
maximize-total-cost-of-alternating-subarrays | Two Approaches - Recursive and Dynamic Programming Solutions | two-approaches-recursive-and-dynamic-pro-6q1z | Intuition\nThe problem is similar to jump by 1 step and jump by 2 step, whereas in this case, we are referring it to select the subarray of length 1 or of lengt | nanda_kumar_2005 | NORMAL | 2024-06-23T06:27:16.954123+00:00 | 2024-06-23T06:27:16.954157+00:00 | 79 | false | # Intuition\nThe problem is similar to jump by 1 step and jump by 2 step, whereas in this case, we are referring it to select the subarray of length 1 or of length 2.\n\n# Complexity\nBetter complexity when DP array is used over recursion, to pre solve the subproblems in recursion.\n\n# Tip\nBoth the solutions are given in the single code itself, if recursive approach is required, just remove dp from everywhere, else everything is for dp.\n\n# Code\n```\nclass Solution {\nprivate:\n long long func(int index, vector <int> &nums, vector <long long> &dp) {\n if (index == nums.size()) return 0;\n if (index == nums.size()-1) return nums[index] + func(index+1, nums, dp);\n\n if (dp[index] != -1) return dp[index];\n\n long long jump_1 = nums[index] + func(index+1, nums, dp);\n long long jump_2 = nums[index] - nums[index+1] + func(index+2, nums, dp);\n return dp[index] = max(jump_1, jump_2);\n }\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n long long ans = 0;\n int n = nums.size();\n if (n==1) return nums[0];\n vector <long long> dp(n+1, -1);\n return func(0, nums, dp);\n }\n};\n``` | 2 | 0 | ['Dynamic Programming', 'Recursion', 'C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | C++ Recursion, Memoization , Tabulation ✅ | c-recursion-memoization-tabulation-by-ya-r56y | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | yashwanthreddi | NORMAL | 2024-06-23T04:04:10.747164+00:00 | 2024-07-14T05:44:10.701700+00:00 | 448 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n\n**Recursion**\n```\nclass Solution {\npublic:\n \n long long fun(vector<int>& nums,int i, long long sum,int pow)\n {\n if(i>=nums.size())\n {\n return sum;\n }\n \n long long cut = fun(nums,i+1,sum+nums[i] * pow, 1);\n\n long long notcut = fun(nums,i+1,sum+nums[i] * pow,-1*pow);\n \n \n return max(cut,notcut);\n \n }\n \n long long maximumTotalCost(vector<int>& nums) {\n \n int pow=1;\n \n long long sum=0;\n \n return fun(nums,0,sum,pow);\n \n }\n};\n```\n\n**Memoization**\n```\nclass Solution {\npublic:\n \n long long fun(vector<int>& nums,int i,int pow,vector<vector<long long>>& dp)\n {\n if(i>=nums.size())\n {\n return 0;\n }\n \n if (dp[i][pow == 1 ? 0 : 1] != -1) {\n return dp[i][pow == 1 ? 0 : 1];\n }\n \n long long cut = fun(nums,i+1, 1,dp)+ nums[i] * pow;\n\n long long notcut = fun(nums,i+1,-1*pow,dp)+nums[i] * pow;\n \n \n return dp[i][pow == 1 ? 0 : 1] = max(cut, notcut);\n \n }\n \n long long maximumTotalCost(vector<int>& nums) {\n \n int pow=1;\n \n long long sum=0;\n \n vector<vector<long long>> dp(nums.size(), vector<long long>(2, -1));\n \n \n long long temp = fun(nums,0,pow,dp);\n\n \n return temp;\n }\n};\n```\n\n**Tabulation**\n```\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n vector<vector<long long>> dp(n + 1, vector<long long>(2, 0));\n\n for(int i=0;i<nums.size();i++){\n dp[i][0]=nums[i];\n if(i-1>=0){\n dp[i][0]+=max(dp[i-1][0],dp[i-1][1]);\n dp[i][1]=-nums[i];\n dp[i][1]+=dp[i-1][0];\n }else{\n dp[i][1]=nums[i];\n }\n }\n return max(dp[n-1][0], dp[n-1][1]);\n }\n};\n```\n | 2 | 0 | ['Dynamic Programming', 'Recursion', 'Memoization', 'C++'] | 1 |
maximize-total-cost-of-alternating-subarrays | C++ | | Easy Solution | | Top-Down DP | | With Explanation | c-easy-solution-top-down-dp-with-explana-et0z | Approach\nAt each i, we have 2 options:\n Just take nums[i] in the subarray.\n Take nums[i] and nums[i+1] in the subarray\n\ndp[i]: Maximum sum of subarrays fro | yash8589 | NORMAL | 2024-06-23T04:03:48.878199+00:00 | 2024-06-23T04:03:48.878228+00:00 | 76 | false | # Approach\nAt each `i`, we have 2 options:\n* Just take `nums[i]` in the subarray.\n* Take `nums[i]` and `nums[i+1]` in the subarray\n\n`dp[i]`: Maximum sum of subarrays from `i` to `n-1`.\n\nBe sure to check for bounds in case of `i+1`\n\n# Complexity\n- Time complexity: $O(n)$\n\n# Code\n```\nclass Solution {\npublic:\n vector<int> nums;\n long long helper(int i, vector<long long>& dp){\n if(i >= nums.size()) return 0;\n \n if(dp[i] != -1) return dp[i];\n \n long long a, b=0;\n a= nums[i] + helper(i+1, dp);\n if(i+1 < nums.size()){\n b = nums[i] - nums[i+1] + helper(i+2, dp);\n return dp[i] = max(a, b);\n }\n else return dp[i] = a;\n \n }\n long long maximumTotalCost(vector<int>& nums) {\n this->nums= nums;\n vector<long long> dp(nums.size(),-1);\n return helper(0,dp);\n \n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | 🔥Clean DP Code✅ || ⚡Memoization | clean-dp-code-memoization-by-adish_21-t4xo | \n\n# Complexity\n\n- Time complexity:\nO(n * 2)\n\n- Space complexity:\nO(n * 2)\n\n\n# Code\n## Please Upvote if it helps\uD83E\uDD17\n\nclass Solution {\npub | aDish_21 | NORMAL | 2024-06-23T04:02:31.441455+00:00 | 2024-06-23T08:22:09.392160+00:00 | 460 | false | \n\n# Complexity\n```\n- Time complexity:\nO(n * 2)\n\n- Space complexity:\nO(n * 2)\n```\n\n# Code\n## Please Upvote if it helps\uD83E\uDD17\n```\nclass Solution {\npublic:\n long dp[100001][2];\n long helper(int ind, int n, vector<int>& nums, bool ch){\n if(ind == n)\n return 0;\n if(dp[ind][ch] != -1)\n return dp[ind][ch];\n long maxi = LONG_MIN;\n maxi = ((!ch) ? nums[ind] : -1 * nums[ind]) + helper(ind + 1, n, nums, !ch);\n maxi = max(maxi, nums[ind] + helper(ind + 1, n, nums, true));\n return dp[ind][ch] = maxi;\n }\n \n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n memset(dp, -1, sizeof(dp));\n return helper(0, n, nums, false);\n }\n};\n``` | 2 | 0 | ['Dynamic Programming', 'Memoization', 'C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | c++ dp | c-dp-by-pb_matrix-61b9 | \nclass Solution {\npublic:\n vector<int>v;\n int n;\n long long dp[100001][2][2];\n long long fn(int ind,int st,int o){\n if(ind>=n) return | pb_matrix | NORMAL | 2024-09-29T11:18:27.198475+00:00 | 2024-09-29T11:18:27.198506+00:00 | 23 | false | ```\nclass Solution {\npublic:\n vector<int>v;\n int n;\n long long dp[100001][2][2];\n long long fn(int ind,int st,int o){\n if(ind>=n) return 0;\n if(dp[ind][st][o]!=-1) return dp[ind][st][o];\n long long x;\n if(st) x=v[ind]+max(fn(ind+1,0,1),fn(ind+1,1,0));\n else{\n if(o) x=-v[ind]+max(fn(ind+1,0,0),fn(ind+1,1,0));\n else x=v[ind]+max(fn(ind+1,0,1),fn(ind+1,1,0));\n }\n return dp[ind][st][o]=x;\n }\n long long maximumTotalCost(vector<int>&vp) {\n v=vp;\n n=v.size();\n memset(dp,-1,sizeof(dp));\n return fn(0,1,0);\n }\n};\n``` | 1 | 0 | ['Dynamic Programming', 'C'] | 1 |
maximize-total-cost-of-alternating-subarrays | beats 100% || considers all conditions seperately | beats-100-considers-all-conditions-seper-njwb | Intuition\nfirstly tried greedy,though of binary search,etc but a regular dp question\n Describe your first thoughts on how to solve this problem. \n\n\n\n# Com | mzip19 | NORMAL | 2024-06-23T20:04:14.533896+00:00 | 2024-06-23T20:04:14.533928+00:00 | 201 | false | # Intuition\nfirstly tried greedy,though of binary search,etc but a regular dp question\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n\n\n# Complexity\n- Time complexity:\n- $$O(n)$$ dp array of 2*n size\n\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n- $$O(n)$$(recursive stack and dp array)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n private long f(int[] nums,long[][] dp,int i,int flip){\n if(i==nums.length) return 0;\n if(dp[i][flip]!=-2) return dp[i][flip];\n if(nums[i]>0 || flip==0){\n long ans=f(nums,dp,i+1,1);\n \n if(nums[i]>=0) {\n dp[i][0]=ans+nums[i];\n dp[i][1]=ans+nums[i];\n }else dp[i][flip]=ans+nums[i];\n return dp[i][flip];\n \n }\n else {\n long ans1=f(nums,dp,i+1,1)+nums[i];\n long ans2=f(nums,dp,i+1,0)+nums[i]*-1;\n dp[i][0]=ans1;\n return dp[i][1]=Math.max(ans1,ans2);\n }\n \n\n } \n public long maximumTotalCost(int[] nums) {\n long[][] dp=new long[nums.length][2];\n for(int i=0;i<nums.length;i++) {\n dp[i][1]=-2;\n dp[i][0]=-2;\n }\n return f(nums,dp,0,0);\n }\n \n}\n\n//-7 -5 -2 3 -2 4\n``` | 1 | 0 | ['Dynamic Programming', 'Java'] | 2 |
maximize-total-cost-of-alternating-subarrays | Easy to Understand (intuitive) , Tabulation | easy-to-understand-intuitive-tabulation-nvkpq | Intuition\n Describe your first thoughts on how to solve this problem. \n1. There is no constraints on number of partitions.\n2. we can create as many as we wan | quack_quack | NORMAL | 2024-06-23T18:29:07.178004+00:00 | 2024-06-24T13:16:03.602509+00:00 | 244 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n1. There is no constraints on number of partitions.\n2. we can create as many as we want , think in group of 2 ,at any index i , think about i , and i - 1 only\n3. Think about these case:\n a. [-1 , -2] => 1\n b. [-1 , -2 , -5] => 2\n c. [-1 , -2 , -5 , -10] => 6\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nIf we have (pos , neg) -> we can always make 2nd element positive.\n\n\n# Complexity\n- Time complexity:\n- O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n- O(N)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n\n# Code\n```\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n \n long long ans = 0;\n vector<long long> dp(n, -1e15);\n dp[0] = nums[0];\n \n if(n == 1){\n return (long long)nums[0];\n }\n // I have created base case for first 2 element.\n if(nums[1] < 0){\n dp[1] = -1 * nums[1] + nums[0];\n }else{\n dp[1] = dp[0] + nums[1];\n }\n \n \n for(int i = 2 ; i < n ; i++){\n if(nums[i] < 0){\n dp[i] = dp[i - 2] + -1 * nums[i] + nums[i-1] ;\n }\n dp[i] = max(dp[i] , dp[i - 1] + nums[i]);\n }\n \n return dp[n - 1];\n \n }\n};\n``` | 1 | 0 | ['Dynamic Programming', 'C++'] | 2 |
maximize-total-cost-of-alternating-subarrays | Top down DP | | Space optimization(O(1)) | top-down-dp-space-optimizationo1-by-rake-mgwc | \n Describe your approach to solving the problem. \n\n# Complexity\n- Time complexity:O(N)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity:O(1 | Rakesh_kumar_reddy | NORMAL | 2024-06-23T15:49:07.585082+00:00 | 2024-06-23T15:49:07.585117+00:00 | 5 | false | \n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n long long ans=0;\n int n=nums.size();\n vector<long long> curr(3);\n vector<long long> prev(3);\n for(int ind=n-1;ind>=1;ind--){\n for(int one=1;one>=-1;one-=2){\n long long ans=0;\n if(one>0)\n ans=max(nums[ind]+prev[-one+1],nums[ind]+prev[one+1]);\n else\n ans=-nums[ind]+prev[-one+1];\n curr[one+1]=ans;\n }\n prev=curr;\n }\n return max(curr[0],max(curr[1],curr[2]))+nums[0];\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Dynamic Programming || Easy Approach with intuition || Memoization || C++ | dynamic-programming-easy-approach-with-i-ip7i | Intuition\nFor each element in the array, we have two possibilities:\n\n1. Start a new subarray from the current element.\n2. Continue the current subarray by a | arshika3086 | NORMAL | 2024-06-23T10:38:32.510008+00:00 | 2024-06-23T10:38:32.510035+00:00 | 134 | false | # Intuition\nFor each element in the array, we have two possibilities:\n\n1. Start a new subarray from the current element.\n2. Continue the current subarray by adding the current element.\n\nWe use a dynamic programming approach to keep track of these possibilities and make optimal decisions at each step.\n\n# Approach\n1. Recursive Function: We define a recursive function fun(ind, start, sign) that returns the maximum possible sum considering the following parameters:\n\n(i) ind: The current index in the nums array.\n(ii) start: A flag indicating whether we are starting fresh (0) or continuing (1).\n(iii) sign: A flag indicating the current sign of the next operation (1 for adding the element, 0 for subtracting).\n\n2. Base Case: If ind equals the size of nums, we return 0 because we\'ve processed all elements.\n\n3. Memoization: We use a 3D vector dp to store the results of subproblems to avoid redundant calculations.\n\n4. Recursive Cases:\n- If start is 0, we must start a new subarray with the current element.\n- If start is 1, we consider both adding and not adding the current element, depending on the sign.\n- If sign is 1, we subtract the current element.\n- If sign is 0, we add the current element.\n\n5. Return Value: The function maximumTotalCost initializes the dp array and starts the recursion from the first element with both start and sign set to 0.\n\n# Complexity\n- Time complexity:\nThe total number of unique states is n * 2 * 2 = 4n. Each state is computed in constant time \uD835\uDC42(1), so the overall time complexity is O(n).\n\n- Space complexity:\n The dp array requires O(n) space and the recursion stack space also uses O(n), so the overall space complexity is O(n).\n\n\n# Code\n```\nclass Solution {\npublic:\n long long fun(int ind, int start,int sign,vector<vector<vector<long long>>>&dp, vector<int>&nums)\n {\n if(ind == nums.size()) return 0;\n if(dp[ind][start][sign] != -1e13) return dp[ind][start][sign];\n long long ans = -1e13;\n if(start == 0) //means fresh start we need to take the current element\n {\n ans = max(ans,nums[ind]+fun(ind+1,1,1,dp,nums));\n }\n else //not a fresh start, we are continuing 2 cases either we continue or not means either we take or not take\n {\n if(sign == 1) //minus sign\n {\n ans = max(ans,-nums[ind]+fun(ind+1,1,0,dp,nums)); //take\n ans = max(ans,fun(ind,0,0,dp,nums)); //we are not taking that element in continuity and will start fresh from that element\n }\n else\n {\n ans = max(ans,nums[ind]+fun(ind+1,1,1,dp,nums));\n ans = max(ans,fun(ind,0,0,dp,nums));\n }\n }\n return dp[ind][start][sign] = ans;\n }\n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n vector<vector<vector<long long>>>dp(n,vector<vector<long long>>(2,vector<long long>(2,-1e13)));\n return fun(0,0,0,dp,nums);\n }\n};\n``` | 1 | 0 | ['Dynamic Programming', 'Memoization', 'C++'] | 1 |
maximize-total-cost-of-alternating-subarrays | 3Dp with state , index and minus 1 as parameters | 3dp-with-state-index-and-minus-1-as-para-4hr7 | State represents whether you are in continuation of the previous array or not (1 or 0 respectively).\n\nState is either 0 or 1 we will have 2 choices in both ca | Tilak27 | NORMAL | 2024-06-23T09:31:45.169123+00:00 | 2024-06-23T09:31:45.169147+00:00 | 21 | false | State represents whether you are in continuation of the previous array or not (1 or 0 respectively).\n\nState is either 0 or 1 we will have 2 choices in both cases i.e whether to continue the same state or not.\nend_array means we are ending the array at that index.\ncurr_array that the next call will be in continuation of the subarray.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n\n long long solve(vector<int>& nums, int i, int state, int minus, vector <vector <vector <long long>>> &dp) {\n\n if(i == nums.size()) return 0;\n\n int x = minus == -1 ? 0: 1;\n\n if(dp[i][state][x] != -1) {\n\n return dp[i][state][x];\n\n }\n\n long long curr_array = -1e9, end_array = -1e9;\n\n if(state == 1) {\n\n curr_array = nums[i]*minus + solve(nums, i+1, 1, minus*-1, dp);\n end_array = nums[i]*minus + solve(nums, i+1, 0, 1, dp);\n\n }\n\n else {\n\n curr_array = nums[i] + solve(nums, i+1, 1, -1, dp);\n end_array = nums[i] + solve(nums, i+1, 0, -1, dp);\n\n }\n\n return dp[i][state][x] = max(curr_array, end_array);\n }\n\n\n long long maximumTotalCost(vector<int>& nums) {\n\n vector <vector <vector <long long>>> dp(nums.size(), vector <vector <long long>> (2, vector <long long> (2, -1)));\n \n return solve(nums, 0, 0, 1, dp);\n }\n};\n``` | 1 | 0 | ['C++'] | 1 |
maximize-total-cost-of-alternating-subarrays | Explained tabulation dynamic programming. | explained-tabulation-dynamic-programming-a2h8 | Intuition\n Describe your first thoughts on how to solve this problem. \n\nYou are given an integer array nums with length n.\n\nThe cost of a subarray nums[l.. | ddhira123 | NORMAL | 2024-06-23T08:26:27.542484+00:00 | 2024-06-23T08:26:27.542508+00:00 | 56 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\nYou are given an integer array `nums` with length $$n$$.\n\nThe cost of a subarray `nums[l..r]`, where $$0 \\leq l \\leq r < n$$, is defined as:\n\n$$\\text{cost}(l, r) = nums[l] - nums[l + 1] + ... + nums[r] \\times (\u22121)^{r \u2212 l}$$\n\nOr in fully mathematic notation, it will be\n\n$$\\text{cost}(l, r) = \\sum_{i=l}^{r} \\text{nums}[i] \\times (-1)^{i-l} $$\n\nElements at **even** indexes (i.e. 0, 2, 4, 6, ...) in subarray would be subtracted in the cost calculation, and the ones at **odd** indexes (i.e. 1,3,5,7,...) will be added.\n\nThe total cost is sum of costs of all subarrays. In this problem, we are asked to get max possible cost by splitting the array into several subarrays.\n\nThus, we need to do this in splitting to gain maximized total cost:\n\n- If the element is **positive** or zero, split it to a subarray directly to ensure it placed at 0-th index.\n- If the element is **negative**:\n - If current element is the second element of `nums` (`nums[1]`) or _its previous element_ is positive or zero, merge it to the previously created subarray. So it would be placed at index 1.\n - Otherwise, pick the max possible cost from the possible splits.\n For example, array `[1,-3,-2]` can be splitted to :\n 1. `[1,-3]` and `[-2]`, that costs `(1 - (-3)) + (-2) = 2`, which equals to cost of `[1,-3,-2]`.\n 2. `[1]` and `[-3,-2]`, that costs `1 + (-3 - (-2)) = 0`\n\nSince we need to use previous values on the process (in consecutive negative elements case), we can use up tabulation / bottom-up dynamic programming.\n\nSimilar problem, but simpler = [House Robber](https://leetcode.com/problems/house-robber/description/)\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n1. If $$n == 1$$, return `nums[0]` directly. No need to do more calculation.\n2. Create a new array sized $$n$$ to store the total costs of subarrays from `nums[0..i]`. Because we need to revisit the previous elements `nums` to check its sign (positive / negative).\n3. Initialize the tabulation by setting:\n ```\n dp[0] = nums[0]\n ```\n4. For `i=1` to `i=nums.length-1`:\n 1. If `nums[i] >= 0`, add it immediately. Let\'s assume that we created new subarray.\n ```\n dp[i] = dp[i-1] + nums[i]\n ```\n 2. Else:\n 1. If `i==1 || nums[i-1] >= 0`, merge it directly to the last created subarray. When `i==1`, there\'s no better choice to make the cost bigger, than merging this element to be added. *Elements on even indexes will be subtracted.*\n ```\n dp[i] = dp[i-1] - nums[i]\n ```\n 2. Else, This is consecutive negative elements, so we need to recalculate for the optimal splits. In this step, we have our latest subarray: `[nums[i-2], nums[i-1]]`.\n ```\n dp[i] = max(\n // Merge directly to latest subarray\n // cost([nums[i-2], nums[i-1], nums[i]])\n dp[i-1] + nums[i], \n\n // Split the latest subarray into 2, then\n // merge this element to the one containing nums[i-1]\n // cost([nums[i-2]]) + cost([nums[i-1], nums[i]])\n dp[i-2] + nums[i-1] - nums[i]\n )\n ```\n\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public long maximumTotalCost(int[] nums) {\n if(nums.length == 1) return nums[0];\n\n long[] dp = new long[nums.length];\n dp[0] = nums[0];\n\n for(int i=1; i<nums.length; i++) {\n if(nums[i] >= 0) {\n dp[i] = dp[i-1] + nums[i];\n } else {\n if(i==1 || nums[i-1] >= 0) {\n dp[i] = dp[i-1] - nums[i];\n } else {\n dp[i] = Math.max(dp[i-1]+nums[i], dp[i-2]+nums[i-1]-nums[i]);\n }\n }\n }\n\n return dp[nums.length-1];\n }\n}\n``` | 1 | 0 | ['Dynamic Programming', 'Java'] | 1 |
maximize-total-cost-of-alternating-subarrays | Rust/Python 3 lines solution with explanation how to get there | rustpython-3-lines-solution-with-explana-ngd6 | Intuition\n\nFirst it does not name sense to have an division in which we have more than 3 elements. For example if one of the divisions is a1, a2, a3, you can | salvadordali | NORMAL | 2024-06-23T07:53:30.825045+00:00 | 2024-06-23T07:53:30.825074+00:00 | 61 | false | # Intuition\n\nFirst it does not name sense to have an division in which we have more than 3 elements. For example if one of the divisions is `a1, a2, a3`, you can do the same with divison `a1, a2` and another one `a3`.\n\nNow we can look at the problem recursively.\n\n```\nf(arr) = max(\n arr[i] + f(arr[i + 1:]),\n arr[i] - arr[i + 1] + f(arr[i + 2:]),\n)\n```\n\nJust add boundary conditions and caching to redo the same computations and you have a solution\n\n```\nclass Solution:\n\n def maximumTotalCost(self, nums: List[int]) -> int:\n @lru_cache(None)\n def dp(i):\n if i >= len(nums):\n return 0\n\n if i == len(nums) - 1:\n return nums[-1]\n\n return max(\n nums[i] + dp(i + 1),\n nums[i] - nums[i + 1] + dp(i + 2)\n )\n\n return dp(0)\n```\n\nNow you can see that you can move backwards in the array and do the same computations (you can notice that you need only last 2 results). So you can rewrite it with\n\n# Complexity\n- Time complexity: $O(n)$\n- Space complexity: $O(1)$\n\n# Code\n```Python []\nclass Solution:\n\n def maximumTotalCost(self, nums: List[int]) -> int:\n v1, v2 = nums[-1], 0\n for i in range(len(nums) - 2, -1, -1):\n v1, v2 = max(nums[i] + v1, nums[i] - nums[i + 1] + v2), v1\n return v1\n```\n```Rust []\nimpl Solution {\n pub fn maximum_total_cost(nums: Vec<i32>) -> i64 {\n let (mut v1, mut v2) = (nums[nums.len() - 1] as i64, 0);\n for i in (0 .. nums.len() - 1).rev() {\n (v1, v2) = ((nums[i] as i64 + v1).max(nums[i] as i64 - nums[i + 1] as i64 + v2), v1);\n }\n return v1;\n }\n}\n\n``` | 1 | 0 | ['Python3', 'Rust'] | 0 |
maximize-total-cost-of-alternating-subarrays | Python solution using Recursion, Dynamic Programming (DP) | python-solution-using-recursion-dynamic-9s8wx | Intuition\n Describe your first thoughts on how to solve this problem. \ngiven a nums array, calculate the maximum total of subarray, \n- when split, start the | nvhp46 | NORMAL | 2024-06-23T07:48:21.468491+00:00 | 2024-06-23T07:48:21.468522+00:00 | 82 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\ngiven a nums array, calculate the maximum total of subarray, \n- when split, start the sign as +\n- when not split, flip the sign after each element.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nUsing recursive DP: \n - stop condition, when the cursor is larger than the array length\n - 2 choices: split and no_split: split reset the sign and no_split flip the sign\n\n# Code\n```\nclass Solution:\n def maximumTotalCost(self, nums: List[int]) -> int:\n l = len(nums)\n @cache\n def dp(i, sign):\n if i >= l:\n return 0\n split = sign * nums[i] + dp(i+1, 1)\n no_split = sign*(nums[i]) + dp(i+1, -1*sign)\n return max(split,no_split)\n\n return dp(0, 1)\n``` | 1 | 0 | ['Dynamic Programming', 'Recursion', 'Python3'] | 0 |
maximize-total-cost-of-alternating-subarrays | easy dp c++ | easy-dp-c-by-shivanshu0287-x8vw | Intuition\ncase 1-when you multiply a element by -1 then you have only one option on next element to multiply it by 1(continue in same subarray or switch the su | shivanshu0287 | NORMAL | 2024-06-23T06:21:43.201023+00:00 | 2024-06-23T06:21:43.201053+00:00 | 8 | false | # Intuition\ncase 1-when you multiply a element by -1 then you have only one option on next element to multiply it by 1(continue in same subarray or switch the subarray).\n\n\ncase 2-when you multiply a element by 1 then you have 2 options:-\na-multiply by 1 (means swith the subarray).\nb-multiply by -1 (means continue in same subarray).\n\n\n\n\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(2*N);\n\n- Space complexity:\nO(2*N);\n\n# Code\n```\n#define ll long long\nclass Solution {\n ll n;\n ll dp[100001][2];\n ll solve(ll ind,vector<int>&nums,ll lst){\n if(ind==n)return 0;\n if(dp[ind][lst]!=-1)return dp[ind][lst];\n ll ans=-1e15;\n if(lst==0){\n ans=max(ans,nums[ind]+solve(ind+1,nums,1));\n }else{\n ans=max(ans,-1*nums[ind]+solve(ind+1,nums,0));\n ans=max(ans,nums[ind]+solve(ind+1,nums,1));\n }\n return dp[ind][lst]=ans;\n }\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n memset(dp,-1,sizeof(dp));\n n=nums.size();\n return solve(0,nums,0);\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Recursive + meme | TLE | recursive-meme-tle-by-vaibhav1701-2q53 | Intuition\n- At any index we have two option to be part of previous subarray or start a new subarray.\n\n# Code\n\nclass Solution {\n public static long maxi | vaibhav1701 | NORMAL | 2024-06-23T05:35:56.525985+00:00 | 2024-06-23T05:35:56.526008+00:00 | 39 | false | # Intuition\n- At any index we have two option to be part of previous subarray or start a new subarray.\n\n# Code\n```\nclass Solution {\n public static long maximumTotalCost(int[] nums) {\n HashMap<String, Long> map = new HashMap<>();\n\n return helper(0, false, nums, 0, map);\n }\n\n public static long helper(int idx, boolean isOdd, int[] nums, long sum, HashMap<String, Long> map) {\n if (idx == nums.length)\n return sum;\n\n String key = idx + " " + isOdd + " " + sum;\n if (map.containsKey(key)) {\n return map.get(key);\n }\n\n long take = helper(idx + 1, !isOdd, nums, sum + (isOdd ? -(nums[idx]) : nums[idx]), map);\n long skip = sum + helper(idx + 1, isOdd, nums, nums[idx], map);\n\n map.put(key, Math.max(take, skip));\n return Math.max(take, skip);\n }\n}\n``` | 1 | 0 | ['Hash Table', 'Recursion', 'Java'] | 0 |
maximize-total-cost-of-alternating-subarrays | Simple DP Similar to Take/Not-take✅ | simple-dp-similar-to-takenot-take-by-har-bhds | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Harshsharma08 | NORMAL | 2024-06-23T05:04:28.623230+00:00 | 2024-06-23T05:04:28.623259+00:00 | 46 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n long partition(int ar[], int i, long dp[][],int prev){\n if(i<0) return 0;\n if(i==0){\n if(prev==0) return ar[0];\n else return Integer.MIN_VALUE;\n }\n if(dp[i][prev]!=-1) return dp[i][prev];\n long ans = Integer.MIN_VALUE;\n if(prev==0){\n ans = Math.max(ans,ar[i] + partition(ar,i-1,dp,0));\n ans = Math.max(ans,ar[i] + partition(ar,i-1,dp,1));\n }\n else{\n ans = Math.max(ans, -ar[i] + partition(ar,i-1,dp,0));\n }\n return dp[i][prev] = ans;\n }\n public long maximumTotalCost(int[] nums) {\n int n = nums.length;\n long dp[][] = new long[n][2];\n for(int i=0;i<n;i++) Arrays.fill(dp[i],-1);\n long lf = partition(nums,n-1,dp,0);\n long rf = partition(nums,n-1,dp,1);\n return Math.max(lf,rf);\n }\n}\n``` | 1 | 0 | ['Array', 'Dynamic Programming', 'Memoization', 'C++', 'Java'] | 0 |
maximize-total-cost-of-alternating-subarrays | dp || c++ || 2D DP | dp-c-2d-dp-by-aditya_kunwar-x65m | Intuition\ndekhlo code accha se intutively likhe hai\n\n# Code\n\nclass Solution {\npublic:\nlong long dp[100001][2];\nlong long f(vector<int>&nums,int flag,int | aditya_kunwar | NORMAL | 2024-06-23T04:51:33.492103+00:00 | 2024-06-23T04:51:33.492136+00:00 | 10 | false | # Intuition\ndekhlo code accha se intutively likhe hai\n\n# Code\n```\nclass Solution {\npublic:\nlong long dp[100001][2];\nlong long f(vector<int>&nums,int flag,int ind){\n if(nums.size()<=ind)return 0;\n if(dp[ind][flag]!=-1)return dp[ind][flag];\n long long split=0,skip=0;\n if(flag==0)\n split=1LL*nums[ind]+f(nums,0,ind+1);\n else\n split=-nums[ind]*1LL+f(nums,0,ind+1);\n \n if(flag==1)\n skip=-nums[ind]*1LL+f(nums,0,ind+1);\n else\n skip=nums[ind]*1LL+f(nums,1,ind+1);\n return dp[ind][flag]=max(split,skip);\n}\n long long maximumTotalCost(vector<int>& nums) {\n memset(dp,-1,sizeof(dp));\n return f(nums,0,0);\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | ✔️ Beats 100% : 1D -DP : Detailed Step-by-Step Explanation - C++/Java/Python/C#/JS/Ruby/Go/Dart | beats-100-1d-dp-detailed-step-by-step-ex-njhu | Intuition\nWe need to find the maximum cost of a subarray where the cost is calculated by flipping the sign of every second element. This means alternating sign | Cregan_wolf | NORMAL | 2024-06-23T04:47:05.493829+00:00 | 2024-06-23T04:48:26.964617+00:00 | 87 | false | # Intuition\nWe need to find the maximum cost of a subarray where the cost is calculated by flipping the sign of every second element. This means alternating signs as we move through the array. Dynamic Programming (DP) is suitable to solve this problem efficiently.\n\n# Approach\n1. **Dynamic Programming Table:** Use a 2D DP table where `dp[i][j]` represents the maximum cost of the subarray starting at index `i`, with `j` indicating whether the previous number included in the cost was positive (`j=1`) or negative (`j=0`).\n\n2. **Recursive Definition:**\n - If `prevPositive` is true, we add `nums[idx]` to the cost.\n - If `prevPositive` is false, we subtract `nums[idx]` from the cost.\n\n3. **Base Case:** If we reach the end of the array, the cost is `0`.\n\n4. **Choice Diagram:**\n - Include the current element in the subarray with appropriate sign change.\n - Start a new subarray from the current element.\n\n5. **Initialization:** Initialize the DP table with `-1` to indicate uncomputed states.\n\n# Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(n)$$\n\n# Code\n```C++ []\nclass Solution {\npublic:\n // Memoization table: -1 indicates not computed yet\n long long dp[100005][2];\n\n long long maxCost(int idx, bool prevPositive, std::vector<int>& nums) {\n int n = nums.size();\n if (idx >= n) return 0;\n\n if (dp[idx][prevPositive] != -1) {\n return dp[idx][prevPositive];\n }\n\n long long includeCurrent = nums[idx];\n if (!prevPositive) {\n includeCurrent = -nums[idx];\n }\n\n long long continueSubarray = includeCurrent + maxCost(idx + 1, !prevPositive, nums);\n long long startNewSubarray = nums[idx] + maxCost(idx + 1, false, nums);\n\n if (idx == 0) {\n // If we are at the beginning, we cannot start with a negative sign\n dp[idx][prevPositive] = startNewSubarray;\n } else {\n dp[idx][prevPositive] = std::max(continueSubarray, startNewSubarray);\n }\n\n return dp[idx][prevPositive];\n }\n\n long long maximumTotalCost(std::vector<int>& nums) {\n std::memset(dp, -1, sizeof(dp));\n return maxCost(0, true, nums);\n }\n};\n```\n```python []\nclass Solution:\n def __init__(self):\n self.dp = {}\n\n def maxCost(self, idx, prevPositive, nums):\n if idx >= len(nums):\n return 0\n\n if (idx, prevPositive) in self.dp:\n return self.dp[(idx, prevPositive)]\n\n includeCurrent = nums[idx] if prevPositive else -nums[idx]\n\n continueSubarray = includeCurrent + self.maxCost(idx + 1, not prevPositive, nums)\n startNewSubarray = nums[idx] + self.maxCost(idx + 1, False, nums)\n\n if idx == 0:\n self.dp[(idx, prevPositive)] = startNewSubarray\n else:\n self.dp[(idx, prevPositive)] = max(continueSubarray, startNewSubarray)\n\n return self.dp[(idx, prevPositive)]\n\n def maximumTotalCost(self, nums):\n self.dp.clear()\n return self.maxCost(0, True, nums)\n\n```\n```C# []\npublic class Solution {\n private long[,] dp;\n\n private long MaxCost(int idx, bool prevPositive, List<int> nums) {\n int n = nums.Count;\n if (idx >= n) return 0;\n\n if (dp[idx, prevPositive ? 1 : 0] != -1) {\n return dp[idx, prevPositive ? 1 : 0];\n }\n\n long includeCurrent = nums[idx];\n if (!prevPositive) {\n includeCurrent = -nums[idx];\n }\n\n long continueSubarray = includeCurrent + MaxCost(idx + 1, !prevPositive, nums);\n long startNewSubarray = nums[idx] + MaxCost(idx + 1, false, nums);\n\n if (idx == 0) {\n dp[idx, prevPositive ? 1 : 0] = startNewSubarray;\n } else {\n dp[idx, prevPositive ? 1 : 0] = Math.Max(continueSubarray, startNewSubarray);\n }\n\n return dp[idx, prevPositive ? 1 : 0];\n }\n\n public long MaximumTotalCost(List<int> nums) {\n dp = new long[nums.Count + 1, 2];\n for (int i = 0; i <= nums.Count; i++) {\n dp[i, 0] = dp[i, 1] = -1;\n }\n return MaxCost(0, true, nums);\n }\n}\n\n```\n```Java []\nclass Solution {\n private long[][] dp;\n\n private long maxCost(int idx, boolean prevPositive, int[] nums) {\n int n = nums.length;\n if (idx >= n) return 0;\n\n if (dp[idx][prevPositive ? 1 : 0] != -1) {\n return dp[idx][prevPositive ? 1 : 0];\n }\n\n long includeCurrent = nums[idx];\n if (!prevPositive) {\n includeCurrent = -nums[idx];\n }\n\n long continueSubarray = includeCurrent + maxCost(idx + 1, !prevPositive, nums);\n long startNewSubarray = nums[idx] + maxCost(idx + 1, false, nums);\n\n if (idx == 0) {\n dp[idx][prevPositive ? 1 : 0] = startNewSubarray;\n } else {\n dp[idx][prevPositive ? 1 : 0] = Math.max(continueSubarray, startNewSubarray);\n }\n\n return dp[idx][prevPositive ? 1 : 0];\n }\n\n public long maximumTotalCost(int[] nums) {\n dp = new long[nums.length + 1][2];\n for (int i = 0; i <= nums.length; i++) {\n dp[i][0] = dp[i][1] = -1;\n }\n return maxCost(0, true, nums);\n }\n}\n\n```\n```javascript []\nclass Solution {\n constructor() {\n this.dp = {};\n }\n\n maxCost(idx, prevPositive, nums) {\n if (idx >= nums.length) {\n return 0;\n }\n\n const key = `${idx},${prevPositive}`;\n if (key in this.dp) {\n return this.dp[key];\n }\n\n let includeCurrent = nums[idx];\n if (!prevPositive) {\n includeCurrent = -nums[idx];\n }\n\n const continueSubarray = includeCurrent + this.maxCost(idx + 1, !prevPositive, nums);\n const startNewSubarray = nums[idx] + this.maxCost(idx + 1, false, nums);\n\n if (idx === 0) {\n this.dp[key] = startNewSubarray;\n } else {\n this.dp[key] = Math.max(continueSubarray, startNewSubarray);\n }\n\n return this.dp[key];\n }\n\n maximumTotalCost(nums) {\n this.dp = {};\n return this.maxCost(0, true, nums);\n }\n}\n\n```\n```Dart []\nclass Solution {\n Map<String, int> dp = {};\n\n int maxCost(int idx, bool prevPositive, List<int> nums) {\n if (idx >= nums.length) {\n return 0;\n }\n\n String key = "$idx,$prevPositive";\n if (dp.containsKey(key)) {\n return dp[key]!;\n }\n\n int includeCurrent = nums[idx];\n if (!prevPositive) {\n includeCurrent = -nums[idx];\n }\n\n int continueSubarray = includeCurrent + maxCost(idx + 1, !prevPositive, nums);\n int startNewSubarray = nums[idx] + maxCost(idx + 1, false, nums);\n\n if (idx == 0) {\n dp[key] = startNewSubarray;\n } else {\n dp[key] = continueSubarray > startNewSubarray ? continueSubarray : startNewSubarray;\n }\n\n return dp[key]!;\n }\n\n int maximumTotalCost(List<int> nums) {\n dp.clear();\n return maxCost(0, true, nums);\n }\n}\n\n```\n```ruby []\nclass Solution\n def initialize\n @dp = {}\n end\n\n def max_cost(idx, prev_positive, nums)\n return 0 if idx >= nums.length\n\n key = [idx, prev_positive]\n return @dp[key] if @dp.key?(key)\n\n include_current = prev_positive ? nums[idx] : -nums[idx]\n\n continue_subarray = include_current + max_cost(idx + 1, !prev_positive, nums)\n start_new_subarray = nums[idx] + max_cost(idx + 1, false, nums)\n\n if idx == 0\n @dp[key] = start_new_subarray\n else\n @dp[key] = [continue_subarray, start_new_subarray].max\n end\n\n @dp[key]\n end\n\n def maximum_total_cost(nums)\n @dp.clear\n max_cost(0, true, nums)\n end\nend\n\n \n\n```\n```Go []\npackage main\n\nimport (\n "fmt"\n "math"\n)\n\ntype Solution struct {\n dp map[[2]int64]int64\n}\n\nfunc NewSolution() *Solution {\n return &Solution{dp: make(map[[2]int64]int64)}\n}\n\nfunc (s *Solution) maxCost(idx int, prevPositive bool, nums []int) int64 {\n if idx >= len(nums) {\n return 0\n }\n\n key := [2]int64{int64(idx), boolToInt64(prevPositive)}\n if val, exists := s.dp[key]; exists {\n return val\n }\n\n includeCurrent := int64(nums[idx])\n if !prevPositive {\n includeCurrent = -includeCurrent\n }\n\n continueSubarray := includeCurrent + s.maxCost(idx+1, !prevPositive, nums)\n startNewSubarray := int64(nums[idx]) + s.maxCost(idx+1, false, nums)\n\n if idx == 0 {\n s.dp[key] = startNewSubarray\n } else {\n s.dp[key] = int64(math.Max(float64(continueSubarray), float64(startNewSubarray)))\n }\n\n return s.dp[key]\n}\n\nfunc (s *Solution) MaximumTotalCost(nums []int\n\n\n``` | 1 | 0 | ['Dynamic Programming', 'C++', 'Java', 'Go', 'Python3', 'JavaScript', 'C#', 'Dart'] | 1 |
maximize-total-cost-of-alternating-subarrays | Recursion+Memo-Easy Solution-O(n)-Dynamic Programming | recursionmemo-easy-solution-on-dynamic-p-cxz3 | Intuition\nSign and index represents the states of dp and if the sign remains 0 means we take the next subarray.\n\n# Approach\nTake or not take approach\n\n# C | DivyenduVimal | NORMAL | 2024-06-23T04:37:53.412344+00:00 | 2024-06-23T04:37:53.412369+00:00 | 91 | false | # Intuition\nSign and index represents the states of dp and if the sign remains 0 means we take the next subarray.\n\n# Approach\nTake or not take approach\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nusing ll=long long;\nclass Solution {\npublic:\n ll dp[100001][2];\n long long int solve(vector<int>&v,int n,int i,int sign){\n if(i>=n){\n return 0;\n }\n if(dp[i][sign]!=-1){\n return dp[i][sign];\n }\n ll ta=0;\n ll nt=0;\n if(sign==0){\n ta=v[i]+solve(v,n,i+1,1);\n nt=v[i]+solve(v,n,i+1,0);\n }\n else{\n ta=(-1*v[i])+solve(v,n,i+1,0);\n }\n return dp[i][sign]=max<ll>(ta,nt);\n }\n long long maximumTotalCost(vector<int>& nums) {\n int n=nums.size();\n memset(dp,-1,sizeof(dp));\n ll f=solve(nums,n,0,0);\n return f;\n }\n};\n``` | 1 | 0 | ['Dynamic Programming', 'Recursion', 'Memoization', 'C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Recursion -> memoization || Python code | recursion-memoization-python-code-by-rin-k4s8 | \n# Code\n\nclass Solution:\n def asd(self,i,nums,parity,dp):\n if(i>=len(nums)):\n return 0\n if(dp[i][parity]!=-1):\n r | rini03 | NORMAL | 2024-06-23T04:35:39.342892+00:00 | 2024-06-23T04:35:39.342916+00:00 | 82 | false | \n# Code\n```\nclass Solution:\n def asd(self,i,nums,parity,dp):\n if(i>=len(nums)):\n return 0\n if(dp[i][parity]!=-1):\n return dp[i][parity]\n add=nums[i]\n if(parity==1):\n dp[i][parity]=self.asd(i+1,nums,0,dp)-nums[i]\n else:\n dp[i][parity]=max(self.asd(i+1,nums,1-parity,dp)+nums[i],self.asd(i+1,nums,parity,dp)+nums[i])\n return dp[i][parity]\n \n \n \n def maximumTotalCost(self, nums: List[int]) -> int:\n # ans=0\n # start=0\n # for i in range(len(nums)):\n # if()\n dp=[]\n for i in range(len(nums)):\n a=[]\n for j in range(2):\n a.append(-1)\n dp.append(a)\n p=self.asd(0,nums,0,dp)\n # print(dp)\n return p \n``` | 1 | 0 | ['Recursion', 'Memoization', 'Python3'] | 2 |
maximize-total-cost-of-alternating-subarrays | DP Approach | Memoization | Tabulation | dp-approach-memoization-tabulation-by-ta-5wm2 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\nWe can consider this problem as a dp problem of Pattern Take/notTake, whe | tanishq0_0 | NORMAL | 2024-06-23T04:26:46.119836+00:00 | 2024-06-23T04:26:46.119859+00:00 | 89 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nWe can consider this problem as a dp problem of Pattern Take/notTake, where our Take is Positive value and not Take is our negative value. We can use a bool variable to check whether our curr idx can have negative or not.\n\n# Memoization Approach\n```\nll solve(vector<int> &nums, int i, bool neg, vector<vector<ll>> &dp){\n\n // out of bounds \n if(i >= nums.size())\n return 0;\n // if pre calculated return the val.\n if(dp[i][neg] != -1e9)\n return dp[i][neg];\n // take/ negtake cases\n ll negTake = -1e8, take = -1e8;\n\n\n // if we have previously taken a positive value\n // we can take curr as negative\n if(neg){\n negTake = (-nums[i]) + solve(nums, i + 1, false, dp);\n }\n // we take this as new subarray and make neg == true\n for the next element to be counted in the same subarray.\n\n take = (nums[i]) + solve(nums, i + 1, true, dp);\n\n // returning the ans;\n return dp[i][neg] = max(take, negTake);\n\n\n }\n\n public:\n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n\n vector<vector<ll>> dp(n, vector<ll> (2, -1e9));\n int i = 1; bool neg = true;\n return (ll) nums[0] + solve(nums, i, neg, dp);\n }\n```\n\n## Why Taking first element by default and negative as 1 in starting?\n\nWe are here taking first element as default because we have to take first element in exactly one subarray and it will start from first element only.\n\nAs for why negative == 1 since we already consider our first subarray we are checking whether the next elements will be a part of same subarray or we have to take it in another subarray\n\n# Tabulation Approach\n\n```\n#define ll long long\n\nclass Solution {\n \npublic:\n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n\n vector<vector<ll>> dp(n, vector<ll> (2, 0));\n dp[0][1] = dp[0][0] = nums[0];\n for(int i = 1; i<n; i++){\n for(int j = 0; j<2; j++){\n ll negTake = -1e5, take = -1e5;\n if(j == 1){\n negTake = (-nums[i] + dp[i-1][0]);\n }\n take = (nums[i] + dp[i-1][1]);\n dp[i][j] = max(take, negTake);\n }\n }\n\n return dp[n-1][1];\n }\n};\n```\n\n# Complexity\n- Time complexity:O(N * 2)\n\n- Space complexity: O(N) | 1 | 0 | ['Dynamic Programming', 'Memoization', 'C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Kotlin | O(n) time, O(n) space | DP | explained (655ms, 161MB) | kotlin-on-time-on-space-dp-explained-655-zlig | Intuition\n\nThe problem is equal to "you can change any two consequent items from a, b to a, -b multiple times (or zero), but each original item could be chang | tonycode88 | NORMAL | 2024-06-23T04:23:15.344064+00:00 | 2024-07-06T16:30:44.790423+00:00 | 16 | false | # Intuition\n\nThe problem is equal to "you can change any two consequent items from `a, b` to `a, -b` multiple times (or zero), but each original item could be changed only once. Maximize the sum of items."\n\n# Approach\n\nDynamic programming:\n\n- $$maxCost[0] = nums[0]$$\n- $$maxCost[i] = max\\left( maxCost[i-1] + nums[i], maxCost[i-2] + nums[i-1] - nums[i] \\right)$$\n - we either pick an item as-is or apply `a, -b` pattern\n\n# Complexity\n- Time complexity: $$O(n)$$\n\n- Space complexity: $$O(n)$$ (non-iterative impl with caching)\n\n# Code\n```\nimport kotlin.math.max\n\n\nclass Solution {\n\n private var cache: Array<Long?> = emptyArray()\n\n\n fun maximumTotalCost(nums: IntArray): Long {\n cache = arrayOfNulls(nums.size)\n return maxCostUptoIdx(nums, nums.size-1)\n }\n\n private fun maxCostUptoIdx(nums: IntArray, i: Int): Long {\n return when {\n (i < 0) -> 0L\n\n (i == 0) -> nums.first().toLong()\n\n else -> {\n if (cache[i] != null) {\n cache[i]!!\n } else {\n val result = max(\n maxCostUptoIdx(nums, i - 1) + nums[i],\n maxCostUptoIdx(nums, i - 2) + nums[i - 1] - nums[i]\n )\n cache[i] = result\n result\n }\n }\n }\n }\n\n}\n\n``` | 1 | 0 | ['Dynamic Programming', 'Kotlin'] | 0 |
maximize-total-cost-of-alternating-subarrays | Easy Python solution. O(n) time, O(1) space | easy-python-solution-on-time-o1-space-by-tj61 | Intuition\nThere is no reason to split it up into groups larger than 2 because it is alternating. We can either choose to keep the number, or flip the number (a | tyler__ | NORMAL | 2024-06-23T04:21:18.045428+00:00 | 2024-06-23T04:21:18.045491+00:00 | 10 | false | # Intuition\nThere is no reason to split it up into groups larger than 2 because it is alternating. We can either choose to keep the number, or flip the number (and not have flipped the one before it).\n\n# Approach\nIf we don\'t flip it, then we can just add it to the max of our 2 previous scenarios. If we do flip it, we have to take the last scenario where we didn\'t flip it. Also note we cannot flip the first number.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n# Code\n```\nclass Solution:\n def maximumTotalCost(self, nums: List[int]) -> int:\n n = len(nums)\n a = nums[0] #didnt flip last\n b = nums[0] #flipped last\n for i in range(1,n):\n a,b = nums[i]+max(a,b),a-nums[i]\n return max(a,b)\n``` | 1 | 0 | ['Python3'] | 1 |
maximize-total-cost-of-alternating-subarrays | Easy Iterative dynamic programming | easy-iterative-dynamic-programming-by-om-6vc3 | \n\n# Code\n\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n \n int n=nums.size();\n vector<long long>dp1(num | omrajhalwa | NORMAL | 2024-06-23T04:14:45.291131+00:00 | 2024-06-23T04:14:45.291148+00:00 | 15 | false | \n\n# Code\n```\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n \n int n=nums.size();\n vector<long long>dp1(nums.size(),0);\n vector<long long>dp2(nums.size(),0);\n dp1[0]=nums[0];dp2[0]=nums[0];\n for(int i=1;i<nums.size();i++){\n dp1[i]+=nums[i]+max(dp2[i-1],dp1[i-1]);\n dp2[i]+=(nums[i]*-1)+dp1[i-1];\n }\n \n return max(dp1[n-1],dp2[n-1]);\n }\n};\n``` | 1 | 0 | ['Dynamic Programming', 'C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Beginner friendly approach...Take Not take approach using DP | beginner-friendly-approachtake-not-take-78cd2 | Intuition\n Describe your first thoughts on how to solve this problem. \nAssume u have taken the 1st element\nnow u can include the 2nd element as part of 1st e | Tanooj13 | NORMAL | 2024-06-23T04:11:32.420065+00:00 | 2024-06-23T04:11:32.420088+00:00 | 30 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAssume u have taken the 1st element\nnow u can include the 2nd element as part of 1st element subarray if u multiply it with negative sign\nor\nu can make the 2nd element a new subarray if u dont multiply it with negative sign\n\nFor example:\n[1,-2,-7]\nhere we can take [1]\nnext [1,-(-2)] or [1][-2]\nnext A: [1,2,-7] or B: [1][-2,(-(-7))] or C: [1][-2][-7]\nchoose the maximum one\nA:-4 | B:1+(-2+7) = 6 | C:(1)+(-2)+(-7) = -8\n\nSo B is the ans which is 6.\n \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(N^2)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(2*N)\n\n# Code\n```\nclass Solution {\n long[][] dp;\n public long maximumTotalCost(int[] nums) {\n dp = new long[nums.length][2];\n for (long[] i : dp){\n Arrays.fill(i,-1);\n }\n return find(nums,0,0);\n }\n private long find(int[] nums,int ind,int sign){\n if (ind == nums.length)return 0;\n if (dp[ind][sign] != -1)return dp[ind][sign];\n long ans = Long.MIN_VALUE;\n if (sign == 0){\n long newsub = nums[ind] + find(nums,ind+1,0);\n long samesub = nums[ind] + find(nums,ind+1,1);\n ans = Math.max(ans,Math.max(newsub,samesub));\n }\n else{\n long samesub = -nums[ind] + find(nums,ind+1,0);\n ans = Math.max(ans,samesub);\n \n }\n \n return dp[ind][sign] = ans;\n }\n}\n``` | 1 | 0 | ['Dynamic Programming', 'Memoization', 'Java'] | 0 |
maximize-total-cost-of-alternating-subarrays | EZ DP 🔥🔥 | ez-dp-by-chitraksh24-ywev | Code\n\nclass Solution:\n def maximumTotalCost(self, nums: List[int]) -> int: \n @cache\n def solve(curr,sign):\n if curr | chitraksh24 | NORMAL | 2024-06-23T04:02:23.584221+00:00 | 2024-06-23T04:02:23.584258+00:00 | 161 | false | # Code\n```\nclass Solution:\n def maximumTotalCost(self, nums: List[int]) -> int: \n @cache\n def solve(curr,sign):\n if curr>=len(nums):\n return 0\n \n if sign:\n return max(-nums[curr]+solve(curr+1,not sign),nums[curr]+solve(curr+1,sign))\n else:\n return nums[curr]+solve(curr+1,True)\n return solve(0,False)\n \n``` | 1 | 0 | ['Dynamic Programming', 'Memoization', 'Python3'] | 2 |
maximize-total-cost-of-alternating-subarrays | Beats 100%, Intuitive approach with explanation, in python | beats-100-intuitive-approach-with-explan-0i73 | Intuition\n\nEach element will either have a positive multiplier (+1) or a negative multiplier(-1). We just need to consider multiplier sign of an element, with | kardeepakkumar | NORMAL | 2024-06-23T04:02:09.381450+00:00 | 2024-06-23T04:02:09.381486+00:00 | 103 | false | # Intuition\n\nEach element will either have a positive multiplier (+1) or a negative multiplier(-1). We just need to consider multiplier sign of an element, with regard to the multiplier sign of the previous element:\n- If the previous element is negative multiplied, then current has to be positive multiplied\n- If the previous element is positive multiplied, then current can be either positive or negative multiplied.\n\n# Approach\n\nRewriting the above intuition for current element:\n- For current element to be negative multiplied, we need to consider only previous element positive multiplied case\n- For current element to be positive multiplied, we can consider best of previous element negative and positive multiplied\n\nHence, we get this DP relation\n```\ndpNeg[i] = dpPos[i-1] - nums[i]\ndpPos[i] = max(dpNeg[i-1] + nums[i], dpPos[i-1] + nums[i])\n```\n\nWhere we initialise both dpNeg and dpPos with nums[0], as first element has to be positive multiplied in any case.\n\nNote: Corner case of len(nums) = 1 is automatically handled with dp initiliasation and loop range \nNote: We don\'t need to store the whole dp array, we just need the last value of the dp (saving on space complexity)\n\n# Complexity\n#### Time complexity: O(n)\nWe solve this in a single pass of the nums array\n\n#### Space complexity: O(1)\nWe use just two extra variable to store the negative and positive DP values\n\n# Code\n```python\nclass Solution:\n def maximumTotalCost(self, nums: List[int]) -> int:\n dpPos = nums[0]\n dpNeg = nums[0]\n for i in range(1, len(nums)):\n oldDpPos = dpPos\n dpPos = max(dpNeg + nums[i], dpPos + nums[i])\n dpNeg = oldDpPos - nums[i]\n return max(dpNeg, dpPos)\n``` | 1 | 0 | ['Dynamic Programming', 'Python3'] | 0 |
maximize-total-cost-of-alternating-subarrays | DP solution memoization C++ | dp-solution-memoization-c-by-yashgarala2-rdbc | \n# Code\n\nclass Solution {\npublic:\n // Function to calculate the maximum total cost using recursion and memoization\n long long calculate(vector<int> | yashgarala29 | NORMAL | 2024-06-23T04:02:00.744506+00:00 | 2024-06-23T04:02:00.744546+00:00 | 196 | false | \n# Code\n```\nclass Solution {\npublic:\n // Function to calculate the maximum total cost using recursion and memoization\n long long calculate(vector<int> &nums, int current, int sign, vector<vector<long long>> &dp) {\n int n = nums.size();\n // Base case: If we have processed all elements\n if (current >= n) {\n return 0;\n }\n // If the result is already computed, return it\n if (dp[current][sign + 1] != INT_MIN) {\n return dp[current][sign + 1];\n }\n \n // Calculate the maximum cost by either switching the sign or keeping the same sign\n long long add_sign = nums[current] * sign + calculate(nums, current + 1, -sign, dp);\n long long continue_same = nums[current] * sign + calculate(nums, current + 1, sign, dp);\n \n // Store the result in dp table\n dp[current][sign + 1] = max(add_sign, continue_same);\n \n return dp[current][sign + 1];\n }\n \n // Function to initiate the calculation\n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n // Initialize the dp table with INT_MIN to indicate uncomputed states\n vector<vector<long long>> dp(n, vector<long long>(3, INT_MIN));\n // Start the calculation from the first element with a positive sign\n return calculate(nums, 0, 1, dp);\n }\n};\n\n``` | 1 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | C++ || EASY DP | c-easy-dp-by-abhishek6487209-ii5l | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Abhishek6487209 | NORMAL | 2024-06-23T04:01:45.118590+00:00 | 2024-06-23T04:01:45.118619+00:00 | 57 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n long long dp[100000][2];\n long long vp(int j, vector<int>& nums, bool k) {\n if (j >= nums.size()) return 0;\n if (dp[j][k] != -1) {\n return dp[j][k];\n }\n \n long long ans = 0;\n if (k && nums[j] < 0) {\n ans = max(vp(j + 1, nums, true) + nums[j], vp(j + 1, nums, false) - nums[j]);\n } else {\n ans = nums[j] + vp(j + 1, nums, true);\n }\n return dp[j][k] = ans;\n }\n \n long long maximumTotalCost(vector<int>& nums) {\n memset(dp, -1, sizeof(dp));\n return nums[0] + vp(1, nums, true);\n }\n};\n\n``` | 1 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Easy to understand DP | O(n) beats 100% | easy-to-understand-dp-on-beats-100-by-pr-zfis | Intuition\nKey idea: All subarrays will either be of length $1$ or length $2$. This is because any subarray length greater than $2$ can be made with various sub | Pras28 | NORMAL | 2024-06-23T04:00:57.916373+00:00 | 2024-06-23T04:49:25.380509+00:00 | 104 | false | # Intuition\n**Key idea:** All subarrays will either be of length $1$ or length $2$. This is because any subarray length greater than $2$ can be made with various subarrays of lengths $1$ and $2$, and the sum of the scores of these smaller subarrays will be $\\geq$ the score of the larger subarray.\n\nNow, we simply have to figure out when to create a new subarray (*add* the current element to the total), and when to continue an existing subarray (*subtract* the current element from the total).\n\n# Approach\n\nWe use dynamic programming to solve this problem. The DP state is defined as:\n\n- `dp[i][0]`: Maximum total cost up to index i, ending with a subarray of length 1\n- `dp[i][1]`: Maximum total cost up to index i, ending with a subarray of length 2\n\nFor each element, we have two choices:\n1. Start a new subarray (length 1)\n2. Continue the previous subarray (length 2)\n\n### DP Transitions\n\n1. For starting a new subarray:\n `dp[i][0] = max(dp[i-1][0], dp[i-1][1]) + nums[i]`\n We take the maximum of the previous states and add the current element.\n\n2. For continuing the previous subarray:\n `dp[i][1] = dp[i-1][0] - nums[i]`\n We can only continue from a state that ended with a length 1 subarray, and we subtract the current element due to alternating signs.\n\n### Base Case\n\nWe initialize `dp[0][0] = dp[0][1] = nums[0]` as the first element can be considered both as a length 1 subarray and the start of a longer subarray.\n\n\n# Complexity\n- Time complexity:\n$O(n)$\n\n- Space complexity:\n$O(n)$\n\n# Code\n```\nclass Solution:\n def maximumTotalCost(self, nums: List[int]) -> int:\n n = len(nums)\n dp = [[0, 0] for _ in range(n)]\n \n # base case \n dp[0][0] = nums[0]\n dp[0][1] = nums[0]\n \n for i in range(1, len(nums)):\n # state 1: create a new subarray\n dp[i][0] = max(dp[i-1][0], dp[i-1][1]) + nums[i]\n # state 2: continue the previous subarray\n dp[i][1] = dp[i-1][0] - nums[i]\n \n return max(dp[n-1][0], dp[n-1][1])\n``` | 1 | 0 | ['Dynamic Programming', 'Python', 'Python3'] | 0 |
maximize-total-cost-of-alternating-subarrays | Simple recursion + memo | simple-recursion-memo-by-joe54-bjp8 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | joe54 | NORMAL | 2024-06-23T04:00:55.399096+00:00 | 2024-06-23T04:00:55.399139+00:00 | 145 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n \n long long maximumTotalCost(vector<int>& nums) {\n vector<long long> memo(nums.size() * 2 + 1, LONG_MIN);\n return dfs(nums, 0, 1, memo);\n }\n \n long long dfs(vector<int>& nums, int i, int sign, vector<long long> &memo) {\n if (i == nums.size()) return 0;\n int key = nums.size() - sign * i;\n if (memo[key] != LONG_MIN) return memo[key];\n long long res = 0;\n res += sign * nums[i];\n long long cut = dfs(nums, i + 1, 1, memo);\n long long dontCut = dfs(nums, i + 1, sign * -1, memo);\n memo[key] = res + max(cut, dontCut);\n return memo[key]; \n }\n};\n``` | 1 | 0 | ['Dynamic Programming', 'Recursion', 'Memoization', 'C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Shortest 2 line solution | shortest-2-line-solution-by-karan_udaypi-32yp | IntuitionRefer to this video in order to learn to write tabular solutions from memo solutions :- ApproachComplexity
Time complexity:
Space complexity:
Code | karan_udaypie | NORMAL | 2025-03-24T07:38:23.400744+00:00 | 2025-03-24T07:38:23.400744+00:00 | 2 | false | # Intuition
Refer to this video in order to learn to write tabular solutions from memo solutions :- https://www.youtube.com/watch?v=5p4M8RmeQCU
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
long long maximumTotalCost(vector<int>& nums) {
int n = nums.size();
vector<long long> dp(n);
dp[0] = nums[0];
for(int i = 1; i < n; i++)
dp[i] = max(nums[i] + dp[i - 1], (i >= 2 ? dp[i - 2] : 0) + (nums[i - 1] - nums[i]));
return dp[n - 1];
}
};
``` | 0 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Kadane's Algorithm (sort of...) | kadanes-algorithm-sort-of-by-vijayshanka-ksxz | Code | vijayshankarkumar | NORMAL | 2025-03-08T21:50:28.393448+00:00 | 2025-03-08T21:50:28.393448+00:00 | 1 | false | # Code
```cpp []
class Solution {
public:
long long maximumTotalCost(vector<int>& nums) {
if (nums.size() == 1) return nums.front();
vector<long long> dp(nums.size());
dp[0] = nums[0];
dp[1] = max(nums[0] + nums[1], nums[0] - nums[1]);
for (int i = 2; i < nums.size(); i++) {
dp[i] = max(dp[i - 1] + nums[i], dp[i - 2] + nums[i - 1] - nums[i]);
}
return dp.back();
}
};
``` | 0 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | scala oneliner | scala-oneliner-by-vititov-8m2g | null | vititov | NORMAL | 2025-02-16T23:45:10.551544+00:00 | 2025-02-16T23:45:10.551544+00:00 | 3 | false | ```scala []
object Solution {
def maximumTotalCost(nums: Array[Int]): Long =
nums.reverseIterator.foldLeft((0L,0L)){case ((p,n),num) =>
(num+(p max n), -num+p)
}._1
}
``` | 0 | 0 | ['Greedy', 'Scala'] | 0 |
maximize-total-cost-of-alternating-subarrays | 100% run time and 100 memory C# | 100-run-time-and-100-memory-c-by-c0ngtha-0jan | IntuitionUsing dynamic programming with pre and next for sum to i-2 number and i-1 numberApproachComplexity
Time complexity:
Space complexity:
Code | c0ngthanh | NORMAL | 2025-01-08T11:26:37.601565+00:00 | 2025-01-08T11:26:37.601565+00:00 | 3 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
Using dynamic programming with pre and next for sum to i-2 number and i-1 number
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
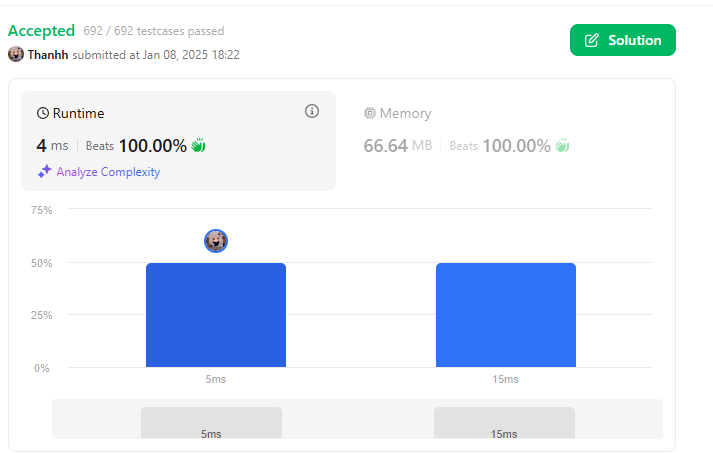
# Code
```csharp []
public class Solution {
public long MaximumTotalCost(int[] nums) {
if(nums.Length == 1){
return nums[0];
}
long pre=nums[0];
long next = nums[1] < 0 ? nums[0]-nums[1] : nums[0]+nums[1];
long temp;
for(int i=2;i<nums.Length;i++){
temp = next;
if(nums[i] <0 && nums[i-1] <0){
next = Math.Max(pre+nums[i-1]-nums[i],next+nums[i]);
}else{
next= next+(nums[i]>0?nums[i]:-nums[i]);
}
pre = temp;
}
return next;
}
}
``` | 0 | 0 | ['Dynamic Programming', 'C#'] | 0 |
maximize-total-cost-of-alternating-subarrays | C++ | c-by-tinachien-r65j | \nusing LL = long long;\nclass Solution {\n LL dp[100005][2];\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n dp[0][0] = nums[0];\n | TinaChien | NORMAL | 2024-12-07T06:04:52.630024+00:00 | 2024-12-07T06:04:52.630066+00:00 | 3 | false | ```\nusing LL = long long;\nclass Solution {\n LL dp[100005][2];\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n dp[0][0] = nums[0];\n dp[0][1] = LLONG_MIN/2;\n int n = nums.size();\n for(int i = 1; i < n; i++){\n dp[i][0] = max(dp[i-1][0], dp[i-1][1]) + nums[i];\n dp[i][1] = dp[i-1][0] - nums[i];\n }\n return max(dp[n-1][0], dp[n-1][1]);\n }\n};\n``` | 0 | 0 | [] | 0 |
maximize-total-cost-of-alternating-subarrays | DP? | dp-by-iitjsagar-u88u | Approach\n Describe your approach to solving the problem. \nEvery number can be either have a negative multiplier or not: \n1. If nums[i] has negative multiplie | iitjsagar | NORMAL | 2024-11-27T05:35:49.823097+00:00 | 2024-11-27T05:35:49.823132+00:00 | 1 | false | # Approach\n<!-- Describe your approach to solving the problem. -->\nEvery number can be either have a negative multiplier or not: \n1. If nums[i] has negative multiplier, then current cost = (max cost till nums[i-2]) + nums[i-1] - nums[i]\n2. If nums[i] has positive multiplier, then current cost = (max cost till nums[i-1]) + nums[i]\n\nMax cost till nums[i] = max of the two options\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python []\nclass Solution(object):\n def maximumTotalCost(self, nums):\n """\n :type nums: List[int]\n :rtype: int\n """\n \n L = len(nums)\n\n if L == 1:\n return nums[0]\n\n p2 = 0\n p1 = nums[0]\n\n for i in range(1,L):\n pn = max(p2 + nums[i-1] - nums[i], p1 + nums[i])\n p2 = p1\n p1 = pn\n \n return pn\n``` | 0 | 0 | ['Dynamic Programming', 'Python'] | 0 |
maximize-total-cost-of-alternating-subarrays | 10 Lines|O(1) Space|Faster than 100% | 10-lineso1-spacefaster-than-100-by-saiki-3aut | Complexity\n- Time complexity: O(n)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity: O(1)\n Add your space complexity here, e.g. O(n) \n\n# Co | saikiranpatil | NORMAL | 2024-11-07T18:36:48.340193+00:00 | 2024-11-07T18:59:04.606926+00:00 | 10 | false | # Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n long long pp, pm, cp, cm;\n cp = cm = pp = pm = nums[0];\n for(int i=1;i<nums.size();i++){\n cp=max(pp+nums[i], pm+nums[i]);\n cm=pp-nums[i];\n\n pp=cp;\n pm=cm;\n }\n return max(cp, cm);\n }\n};\n```\nSilimar to [CSES - Counting Towers](https://cses.fi/problemset/task/2413)\n\n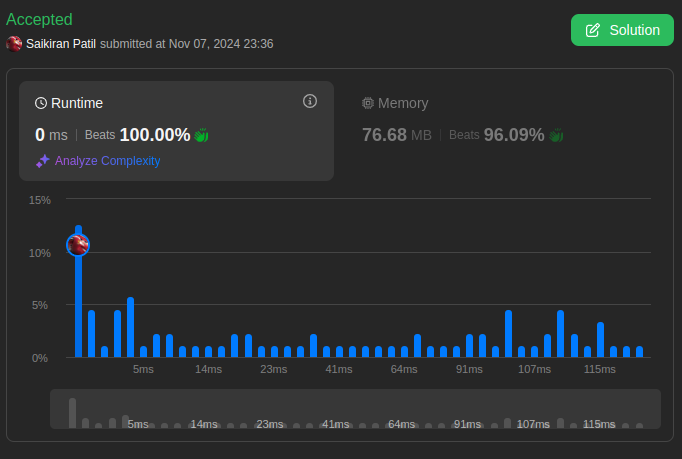 | 0 | 0 | ['Dynamic Programming', 'C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Straightforward DP solution with concise explanation. Time O(n) and Space O(1). | straightforward-dp-solution-with-concise-w0pw | Intuition\nIt is a dynamic programming problem. Each element in the input will be either taken as is or taken with a flipped sign in the final cost. \n\n# Appro | 00_11_00 | NORMAL | 2024-10-24T00:29:39.648426+00:00 | 2024-10-24T00:29:39.648468+00:00 | 2 | false | # Intuition\nIt is a dynamic programming problem. Each element in the input will be either taken as is or taken with a flipped sign in the final cost. \n\n# Approach\nWe can assume that DP_p[i] is the maximum cost of the arry [0, i] with the number at index i taken as is and DP_p[i] is the maximum cost of the arry [0, i] with the number at index i taken with a flipped sign.\n\nThen we will have the following induction rule:\n\n**Case 1**: the number at position is taken as is. It doesn\'t matter what sigan the previous number is taken.\n\nDP_p[i] = max(DP_p[i-1], DP_n[i-1]) + nums[i]\n\n**Case 1**: the number at position is taken with a flipped sign. Thi case, we can only combine this number with its previous number and its previous number is taken as is then we could have nums[i-1] - nums[i] such that the sign of nums[i] is flipped in the cost calculation.\nDP_p[i] = DP_p[i-1] - nums[i]\n\n**Base case**: We can only take the first number as is.\nDP_p[0] = nums[0]\nDP_n[0] = nums[0]\n\n**Return** max(DP_p[n - 1], DP_n[n - 1]) where n is the size of the input.\n\nPlease note that the calclation of DP_p[i] and DP_n[i] only rely on (i-1)\'s result which means we just need to record its previous result. So we can use rolling array to reduce the space complexity to constant.\n\n# Complexity\n- Time complexity: $$O(n)$$\n\n- Space complexity: $$O(1)$$\n\n# Code\n```cpp []\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n if (nums.empty()) {\n return 0;\n }\n long long kept_p = nums[0];\n long long kept_n = nums[0];\n for (int i = 1; i < nums.size(); ++i) {\n long long previois_kept_p = kept_p;\n kept_p = max(kept_p, kept_n) + nums[i];\n kept_n = previois_kept_p - nums[i];\n }\n return max(kept_p, kept_n);\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Detailed explanation using 1D dp | detailed-explanation-using-1d-dp-by-raja-ojai | Intuition\nThe idea is to use dynamic programming.\n\n# Approach\nLet dp[i] stores the answer to the array from [i...n - 1] where n is the size of the array. Ou | rajat8020 | NORMAL | 2024-10-17T20:19:41.811032+00:00 | 2024-10-17T20:20:30.304239+00:00 | 3 | false | # Intuition\nThe idea is to use dynamic programming.\n\n# Approach\nLet dp[i] stores the answer to the array from [i...n - 1] where n is the size of the array. Our answer will be $$dp[0]$$. \n\n# Observation\nIt is never necessary to partition the array into size more than 2. In other words the size of the partition will either be 1 or 2. The reason is we want to have more and more positive numbers in the array. If the number is already positive then we separate that out into a subarray of size 1. If the number is negative then to make it positive we have to start creating the subarray from one element before eat. For example if array is $$[1, -2, 3, -4]$$ then the optimmal answer will be $$[1 + -1*(-2) + 3 + -1*(-4)]$$ because it makes both $$-2$$ and $$-4$$ positive. But the same result can also be achieved by making splitting the array into $$[1, -2]$$ and $$[3, -4]$$. Hence as soon as we make a -ve number positive we need not worry about whats ahead of it. \n\n## Recurrence\nThe $$dp[i]$$ state would go like:\n$$p1 = arr[i] + dp[i + 1]$$ taking $$arr[i]$$ into a separate subarray\n$$p2 = arr[i] + (-1*arr[i + 1]) + dp[i + 2]$$ changing sign of $$arr[i + 1]$$\n\n$$dp[i] = max(p1, p2)$$\n \n\n# Complexity\n- Time complexity: $$O(n)$$ \n\n- Space complexity: $$O(n)$$\n\n# Code\n```cpp []\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size(), i;\n vector<long long> dp(n + 1);\n dp[n - 1] = nums.back();\n for(int i = n - 2; i >= 0; --i) {\n long long p1 = nums[i] + dp[i + 1];\n long long p2 = nums[i] + (-1) * nums[i + 1] + dp[i + 2];\n dp[i] = max(p1, p2);\n }\n return dp[0];\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Beat 100% in both || O(1) space solution || easy to understand | beat-100-in-both-o1-space-solution-easy-bmbfz | Intuition\n- a means flip nums[i-1]\n- b means not flip nums[i-1]\n- i can flip if only i-1 not flip\n- i can flip or not flip, dependent from the state of i-1, | dnanper | NORMAL | 2024-10-12T09:19:59.538369+00:00 | 2024-10-12T09:22:45.216156+00:00 | 2 | false | # Intuition\n- a means flip nums[i-1]\n- b means not flip nums[i-1]\n- i can flip if only i-1 not flip\n- i can flip or not flip, dependent from the state of i-1, so we need to find max value of that two states.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n```cpp []\n;class Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n ios::sync_with_stdio(false); cin.tie(0);\n long long res = 0, a = 0, b = 0;\n int n = nums.size();\n for (int i = 0; i < n; i++)\n {\n if (i == 0)\n {\n res += nums[i];\n continue;\n }\n if (nums[i] >= 0)\n {\n res += max(a, b);\n res += nums[i];\n a = 0;\n b = 0;\n }\n else\n {\n long long tmp1 = a, tmp2 = b;\n a = tmp2 + (-1)*nums[i];\n b = max(tmp1 + nums[i], tmp2 + nums[i]);\n }\n }\n return res += max(a, b);\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | TC : O(n), SC: O(1) DP | tc-on-sc-o1-dp-by-anikets2002-s18a | \n# Code\ntypescript []\nfunction maximumTotalCost(nums: number[]): number {\n // we only take positive this mean we are startign a new sub array from here\n | anikets2002 | NORMAL | 2024-10-09T07:23:55.960832+00:00 | 2024-10-09T07:23:55.960869+00:00 | 0 | false | \n# Code\n```typescript []\nfunction maximumTotalCost(nums: number[]): number {\n // we only take positive this mean we are startign a new sub array from here\n let n = nums.length;\n if(n === 1)return nums[0];\n let maxYet = nums[0];\n let posPrev = 0, posCurr = 0, negPrev = 0, negCurr = 0;\n for(let i = 0; i < n; i++)\n {\n posCurr = nums[i] + (i === 0 ? 0 : maxYet)\n if(i > 0)\n {\n negCurr = posPrev - nums[i];\n maxYet = Math.max(posCurr, negCurr)\n }\n posPrev = posCurr;\n negPrev = negCurr;\n }\n return Math.max(posCurr, negCurr)\n};\n``` | 0 | 0 | ['TypeScript'] | 0 |
maximize-total-cost-of-alternating-subarrays | Simplest C++ Solution | simplest-c-solution-by-pushpendra_singh-1ugi | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | pushpendra_singh_ | NORMAL | 2024-09-24T18:36:31.323134+00:00 | 2024-09-24T18:36:31.323158+00:00 | 1 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n vector<long long int>dp(nums.size()+1,0);\n //hrer state fxn is till size i max sum\n dp[1]=nums[0];\n for(int i=2;i<dp.size();i++){\n if(nums[i-1]>=0) dp[i]=dp[i-1]+nums[i-1];\n else{\n if(nums[i-2]>0) dp[i]=dp[i-2]+nums[i-2]+abs(nums[i-1]);\n else dp[i]=max(dp[i-2]+nums[i-2]+abs(nums[i-1]),dp[i-1]+nums[i-1]);\n }\n }\n return dp[nums.size()];\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | coded with very hit and trial process || cpp | coded-with-very-hit-and-trial-process-cp-bl8s | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | chanderveersinghchauhan08 | NORMAL | 2024-09-12T17:55:46.200602+00:00 | 2024-09-12T17:55:46.200656+00:00 | 0 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n\n long long f(int ind , bool prev , vector<int> &nums , int n , vector<vector<long long >> &dp){\n if(ind == n) return 0;\n if(dp[ind][prev] != -1) return dp[ind][prev];\n long long op1 = LLONG_MIN;\n long long op2 = LLONG_MIN;\n long long op3 = LLONG_MIN;\n long long op4 = LLONG_MIN;\n if(prev == 1 ){\n op1 = (-nums[ind] + f(ind+1 , 0 ,nums , n , dp));// adding in prev subArray\n }\n else if(prev == 0){\n op2 = (nums[ind] + f(ind+1, 1 , nums ,n , dp )); // adding in prev subArray\n }\n \n // creating new subArray from this index\n op3 = nums[ind] + f(ind+1 , 0 , nums , n , dp);\n return dp[ind][prev] = max(op1 , max(op2 , max(op3 , op4)));\n }\n\n long long maximumTotalCost(vector<int>& nums) {\n int n = nums.size();\n bool prev = 0;\n vector<vector<long long >> dp(n , vector<long long > (2,-1));\n long long ans1 = f(0 , 0 , nums , n , dp);\n return ans1;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Positive OR negative sign | positive-or-negative-sign-by-tejasuwarad-974o | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Tejasuwarade | NORMAL | 2024-09-12T16:30:03.063819+00:00 | 2024-09-12T16:30:03.063857+00:00 | 0 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nTime Complexity: O(n)\n\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nSpace Complexity: O(n)\n\n# Code\n```cpp []\nclass Solution {\npublic:\n\n long long max_cost(int i,int sign, vector<int>&nums, int n,vector<vector<long long>> &dp){\n //base\n if(i==n){\n return 0;\n }\n if(dp[i][sign]!=-1){\n return dp[i][sign];\n }\n long long pos=LLONG_MIN,neg=LLONG_MIN;\n if(sign==0){\n pos=nums[i]+max_cost(i+1,1,nums,n,dp);\n }else{\n if(sign==1){\n pos=nums[i]+max_cost(i+1,1,nums,n,dp);\n neg=nums[i]*-1+max_cost(i+1,0,nums,n,dp);\n }else{\n pos=nums[i]+max_cost(i+1,1,nums,n,dp);\n }\n }\n return dp[i][sign]=max(pos,neg);\n }\n long long maximumTotalCost(vector<int>& nums) {\n int n=nums.size();\n vector<vector<long long>>dp(n,vector<long long>(2,-1));\n long long ans=max_cost(0,0,nums,n,dp);\n return ans; \n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Most Optimized O(n) time and O(1) Space | most-optimized-on-time-and-o1-space-by-m-0wam | Intuition\nThe last subarray can end with subtraction or addition, If the last subarray was addition your option for the next element is to either continue that | mkshv | NORMAL | 2024-09-03T16:22:01.114911+00:00 | 2024-09-03T16:22:01.114946+00:00 | 2 | false | # Intuition\nThe last subarray can end with subtraction or addition, If the last subarray was addition your option for the next element is to either continue that subarray with a subtraction, OR start a new subarray with addition. \n\nIf the last subarray was a subtraction, you only have one option, that you can only add the current element, this can be considered as the start of the new subarray. \n\n\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```python3 []\nclass Solution:\n def maximumTotalCost(self,nums):\n n = len(nums)\n a,b = nums[0], -float("inf")\n for i in range(1,n):\n c = max( b + nums[i], a + nums[i])\n d = a - nums[i]\n a , b = c, d\n return max(a,b)\n\n``` | 0 | 0 | ['Python3'] | 0 |
maximize-total-cost-of-alternating-subarrays | 4 lines Java Easy | 4-lines-java-easy-by-parishithada-u62v | Complexity\n- Time complexity:\n Add your time complexity here, e.g. O(n) \nO(n2) \n\n- Space complexity:\n Add your space complexity here, e.g. O(n) \nO(n2) + | parishithada | NORMAL | 2024-08-09T17:51:39.601164+00:00 | 2024-08-09T17:51:39.601187+00:00 | 4 | false | # Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n*2)$$ \n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(n*2) + (Stack Space- O(n))$$\n\n# Code\n```\nclass Solution {\n public long maximumTotalCost(int[] nums) {\n t=new Long[nums.length][2];\n return rec(0,1,nums);\n }\n Long[][] t;\n public long rec(int i,int f,int[] arr){\n if(i>=arr.length) return 0;\n if(t[i][Math.max(f,0)]!=null) return t[i][Math.max(f,0)];\n long take=f*arr[i]+rec(i+1,f*-1,arr);\n long startnew=arr[i]+rec(i+1,-1,arr);\n return t[i][Math.max(f,0)]=Math.max(take,startnew);\n }\n}\n``` | 0 | 0 | ['Java'] | 0 |
maximize-total-cost-of-alternating-subarrays | dp ^^ | dp-by-andrii_khlevniuk-nbty | time: O(N); space: O(1)\n\n\n\n\nlong long maximumTotalCost(vector<int>& n)\n{ \n\tlong long pp{}, p{n[0]};\n\tfor(int i{1}; i<size(n); ++i)\n\t{\n\t\taut | andrii_khlevniuk | NORMAL | 2024-08-06T09:38:11.256811+00:00 | 2024-08-06T12:26:55.738130+00:00 | 3 | false | **time: `O(N)`; space: `O(1)`**\n\n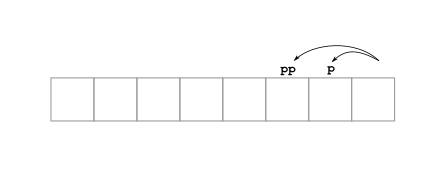\n\n```\nlong long maximumTotalCost(vector<int>& n)\n{ \n\tlong long pp{}, p{n[0]};\n\tfor(int i{1}; i<size(n); ++i)\n\t{\n\t\tauto t = max(p+n[i], pp+n[i-1]-n[i]);\n\t\tpp = p;\n\t\tp = t;\n\t}\n\treturn p;\n}\n```\n**Notation:**\n* `p` - the best previous solution;\n* `pp` - the best previous previous solution;\n\n\n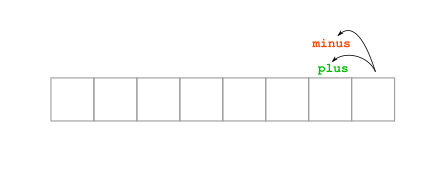\n\n```\nlong long maximumTotalCost(vector<int>& n)\n{ \n\tlong long plus{n[0]}, minus{n[0]};\n\tfor(int i{1}; i<size(n); ++i)\n\t{\n\t\tauto old_plus = plus;\n\t\tplus = max(plus, minus) + n[i];\n\t\tminus = old_plus - n[i];\n\t}\n\treturn max(plus, minus); \n}\n```\n**Notation:**\n* `plus` - the best solution ending with `+`;\n* `minus` - the best solution ending with `-`;\n\nThis two solution remind order reduction technique in differential equations:\n\n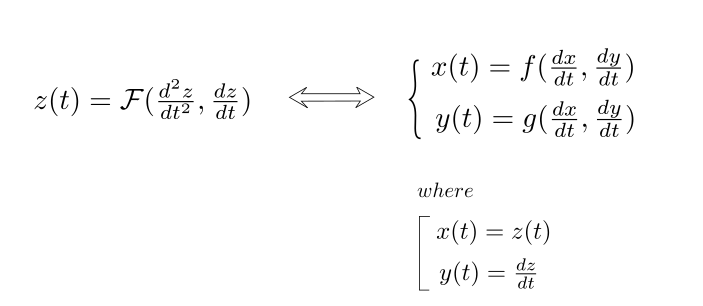\n\n We can either store 2 pieces of data for the previous moment in time `t-dt` or store 1 piece of data for 2 previous moments in time `t-dt` and `t-2*dt`. | 0 | 0 | ['C', 'C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Max Cost of Alt Subarrays | max-cost-of-alt-subarrays-by-jain47040-7mqr | \n# Code\n\nclass Solution {\npublic:\nlong long maximumTotalCost(vector<int>& nums){\n\n int n = nums.size();\n vector<vector<long long>> Dp(n,vector<lon | jain47040 | NORMAL | 2024-08-04T13:18:39.374494+00:00 | 2024-08-04T13:18:39.374528+00:00 | 0 | false | \n# Code\n```\nclass Solution {\npublic:\nlong long maximumTotalCost(vector<int>& nums){\n\n int n = nums.size();\n vector<vector<long long>> Dp(n,vector<long long>(2));\n\n Dp[0][0] = nums[0];\n Dp[0][1] = nums[0];\n\n if(n == 1){\n return Dp[0][0];\n }\n\n Dp[1][0] = nums[1] + max(Dp[0][0],Dp[0][1]);\n Dp[1][1] = -nums[1] + Dp[0][0];\n\n for(int i = 2;i < n;i++){\n Dp[i][0] = nums[i] + max(Dp[i-1][1],Dp[i-1][0]);\n Dp[i][1] = -nums[i] + Dp[i-1][0] ;\n }\n\n for(int i = 0;i < n;i++){\n cout << Dp[i][0] << " " << Dp[i][1];\n // nline\n }\n\n return max(Dp[n-1][0],Dp[n-1][1]);\n\n}\n\n\n\n\n};\n``` | 0 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Very concise and simple O(n) time/O(1) Space Solution | very-concise-and-simple-on-timeo1-space-4o55o | Intuition\nNo matter what happened before any num x, we can always add x to our running total. Conversely, we can only subtract x, if we added the previous num. | lifran | NORMAL | 2024-08-02T13:04:07.154250+00:00 | 2024-08-02T13:04:07.154288+00:00 | 2 | false | # Intuition\nNo matter what happened before any num `x`, we can always *add* `x` to our running total. Conversely, we can only *subtract* `x`, if we added the previous num.\nSo if we can keep track of the maximum possible cost for the cases when we *add* and when we *subtract* the *last* num, we can then calculate the maximum possible cost for the current num.\n\n# Approach\nWe use a size-2 array `dp` to keep track of the two possible maximum cost states as described above and iterate over all of `nums`. Our answer at the end is going to be the `max` over `dp`.\n\n# Complexity\n- Time complexity: O(`|nums.size|`)\n\n- Space complexity: O(`1`)\n\n# Kotlin\n```Kotlin\nclass Solution {\n fun maximumTotalCost(nums: IntArray): Long {\n val dp = LongArray(2) { nums[0].toLong() }\n for (x in nums.drop(1)) {\n val plus = dp.max() + x\n val minus = dp[0] - x\n dp[0] = plus\n dp[1] = minus\n }\n\n return dp.max()\n }\n}\n``` | 0 | 0 | ['Dynamic Programming', 'Kotlin'] | 0 |
maximize-total-cost-of-alternating-subarrays | DP+Space optimized .....||Beats 99.90% ✅✅ | dpspace-optimized-beats-9990-by-soy_yo_m-5brh | \n\n# Intuition\n Describe your first thoughts on how to solve this problem. \nGoal was to maximise the total cost where cost is computed using alternative sum. | Soy_yo_mani | NORMAL | 2024-07-31T18:42:19.161441+00:00 | 2024-07-31T18:42:19.161479+00:00 | 1 | false | 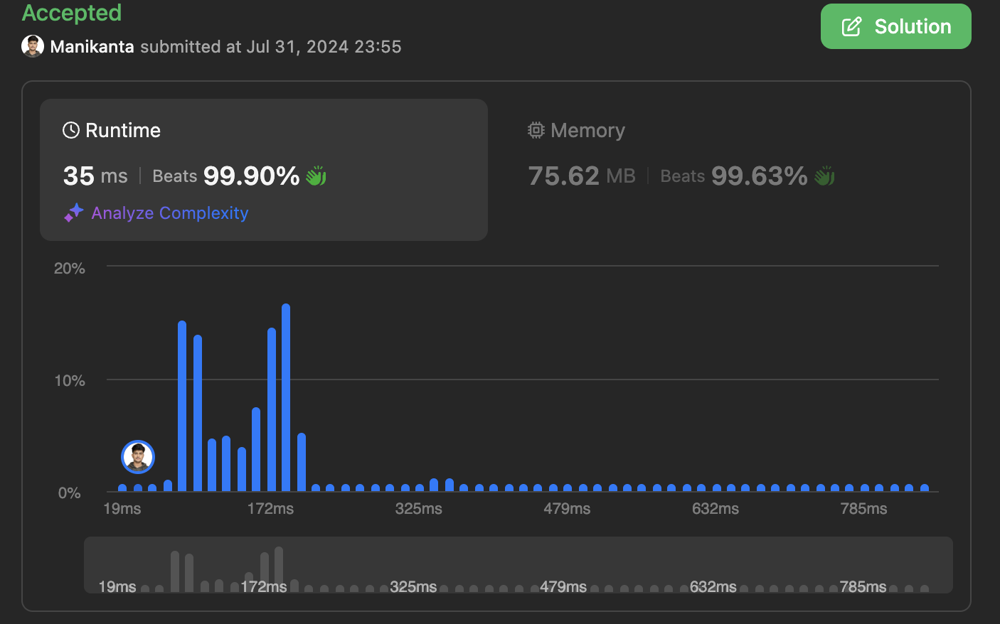\n\n# Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nGoal was to maximise the total cost where cost is computed using alternative sum...use dp to track the sum.For space optimization instead of using 2D dp use variables to store previous result/state.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n \n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n\n# Code\n```\nclass Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) \n {\n ios_base::sync_with_stdio(false); \n cin.tie(NULL);\n int n = nums.size();\n if (n == 1) \n {\n return nums[0];\n }\n // Initialize variables for dp[i-1]\n long long prev0 = nums[0], prev1 = LLONG_MIN;\n long long curr0, curr1;\n\n for (int i = 1; i < n; i++) \n {\n curr0 = max(prev0, prev1) + nums[i];\n curr1 = prev0 - nums[i];\n \n prev0 = curr0;\n prev1 = curr1;\n }\n\n return max(prev0, prev1);\n }\n};\n\n\nUpvote if you find it easy to analyse??!!\n``` | 0 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Simple Recursive DP | simple-recursive-dp-by-smartchuza-34ox | Complexity\n- Time complexity: O(2N)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity: O(2N)\n Add your space complexity here, e.g. O(n) \n\n# | smartchuza | NORMAL | 2024-07-27T17:15:35.319828+00:00 | 2024-07-27T17:15:35.319883+00:00 | 0 | false | # Complexity\n- Time complexity: O(2*N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(2*N)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n long long dp[100001][2];\n long long go(int i, int pos, vector<int>& nums)\n {\n if (i == nums.size())\n return 0;\n if (dp[i][pos]!=-1)\n return dp[i][pos];\n if (pos)\n {\n return dp[i][pos] = nums[i] + go(i+1, 1-pos, nums);\n }\n return dp[i][pos] = max(-nums[i] + go(i+1, 1-pos, nums), nums[i] + go(i+1, pos, nums));\n }\n long long maximumTotalCost(vector<int>& nums) {\n memset(dp, -1, sizeof(dp));\n return go(0,1,nums);\n }\n};\n\n\n``` | 0 | 0 | ['C++'] | 0 |
maximize-total-cost-of-alternating-subarrays | Java Dp and without Dp solution with explanation | java-dp-and-without-dp-solution-with-exp-9pj1 | Intuition\nAt each index we can \n-> start a new subarray with the current index or\n-> it would be part of the previous subarray. \n\nWe find maximum sum at ea | prathihaspodduturi | NORMAL | 2024-07-26T23:19:52.075935+00:00 | 2024-07-26T23:19:52.076000+00:00 | 3 | false | # Intuition\nAt each index we can \n-> start a new subarray with the current index or\n-> it would be part of the previous subarray. \n\nWe find maximum sum at each index using the above two points.\n\n# Approach\nlet dp[n] be the maximum sum at each index of the array.\n\nusing the two points in the intutuion :\n\nstarting the subarray with the current index: \ndp[currentIndex] = Math.max(dp[currentIndex], dp[currentIndex] + dp[currentIndex-1])\n\nit would be part of a previous subarray:\ndp[currentIndex] = Math.max(dp[currentIndex], dp[currentIndex-1] - dp[currentIndex] + dp[currentIndex-2])\n\nHow I got to this is:\n\nLets say we are at index k.\n\nif \'K\' is part of previous subarray it must either fall in a even postion or odd postion \nlets say s is the starting index of the a subarray where k is part of it \n (-1)^(k-s) : if k-s is even then its on even postion\n if k-s is odd then its on odd position\n\nlets say if (s-k) is even then (s-k) is even position, \nthen \ndp[k] = nums[s] - nums[s+1] + nums[s+2].... - nums[k-1] + nums[k]\ndp[k] = nums[k] + dp[k-1]\nIf k is on even postion, then we can start the subarray at k as it is also an even postion.\n\nif (s-k) is odd then k is on odd position,\nIf we consider k-1 as start index instead of s. then K is still on the same odd position which doesn\'t effect the k\' position.\n\ndp[k] = nums[s] - nums[s+1] + .... + nums[k-1] - nums[k]\ndp[k] = dp[k-2] + nums[k-1] - nums[k]\n\nwe cannot directly use dp[k] = dp[k-1] - nums[k] as dp[k-1] also includes the value of k-1 at odd position, as K is in odd position K-1 must be on even position.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution {\n public long maximumTotalCost(int[] nums) {\n \n int n = nums.length;\n\n long dp[] = new long[n];\n \n dp[0] = nums[0];\n for(int i=1;i<n;i++)\n {\n dp[i] = Math.max(dp[i], nums[i] + dp[i-1]);\n\n if(i-2>=0)\n dp[i] = Math.max(dp[i], nums[i-1] - nums[i] + dp[i-2]);\n }\n\n return dp[n-1];\n }\n}\n\n```\n\nSpace optimizing, just using the previous value and previous\'s previous value\n\n```\n\nclass Solution {\n public long maximumTotalCost(int[] nums) {\n \n int n = nums.length;\n\n long prev=nums[0], prevsprev=0;\n \n for(int i=1;i<n;i++)\n {\n long curSum = nums[i] + prev;\n \n curSum = Math.max(curSum, nums[i-1] - nums[i] + prevsprev);\n\n prevsprev = prev;\n prev = curSum;\n }\n\n return prev;\n }\n}\n\n```\n\nIf you like the solution, do upvote | 0 | 0 | ['Java'] | 0 |
maximize-total-cost-of-alternating-subarrays | Kotlin | Dynamic Programming | Iterative | O(n) time | O(1) space | kotlin-dynamic-programming-iterative-on-m35ss | 1. DP array\n\nfun maximumTotalCost(nums: IntArray): Long {\n val dp = LongArray(nums.size + 1)\n\n for (i in nums.lastIndex downTo 0) {\n dp[i] = | IrregularOne | NORMAL | 2024-07-22T18:52:51.874099+00:00 | 2024-07-22T19:05:05.999073+00:00 | 3 | false | # 1. DP array\n```\nfun maximumTotalCost(nums: IntArray): Long {\n val dp = LongArray(nums.size + 1)\n\n for (i in nums.lastIndex downTo 0) {\n dp[i] = nums[i].toLong() + dp[i + 1]\n\n if (i < nums.lastIndex) {\n dp[i] = maxOf(dp[i], (nums[i] - nums[i + 1]).toLong() + dp[i + 2])\n }\n }\n\n return dp[0]\n}\n\n```\n# Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(n)$$\n\n# 2. Space optimization\nWe may see that at each iteration we are only using results from 1 and 2 steps before. Thus, we can use just 2 variables for this, instead of array.\n```\nfun maximumTotalCost(nums: IntArray): Long {\n var max = Long.MIN_VALUE\n var prev = 0L\n var prevPrev = 0L\n\n for (i in nums.lastIndex downTo 0) {\n max = nums[i].toLong() + prev\n\n if (i < nums.lastIndex) {\n max = maxOf(max, (nums[i] - nums[i + 1]).toLong() + prevPrev)\n }\n\n prevPrev = prev\n prev = max\n }\n\n return max\n}\n```\n# Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(1)$$ | 0 | 0 | ['Kotlin'] | 0 |
Subsets and Splits