question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Java (Using Queue | simple dfs) | java-using-queue-simple-dfs-by-dhruvpanw-ennc | First approach:(Using a queue)\n1. Make a boolean array hasReached[] where hasReached[i] means ith vertex can now reach 0.\n2. Push all edges in a queue\n3. Pop | dhruvpanwar31 | NORMAL | 2021-08-13T21:32:26.651543+00:00 | 2021-08-13T21:32:26.651585+00:00 | 458 | false | First approach:(Using a queue)\n1. Make a boolean array hasReached[] where hasReached[i] means ith vertex can now reach 0.\n2. Push all edges in a queue\n3. Pop queue, see if in the edge (u,v) we have a node that reaches 0.( hasReached[u] or hasReached[v] ) \n4. Let the node that reaches 0 be \'u\'.\n5. So now for \'v\' to reach 0 it has to go through \'u\'. So we have to change edge u->v to v->u. Hence we increment the answer as we changed orientation. Also mark hasReached[v] = true.\n6. If \'v\' was reachable i.e. hasReached[v] == true , then there is no need to change orientation as \'u\' can reach \'v\' and from there 0. Mark hasReached[u] = true\n7. If none of the vertices in the current edge were leading to 0 push them back in queue.\n8. Continue till the queue is empty. \n```\nclass Solution {\n static class Pair{\n public int first;\n public int second;\n public Pair(int first, int second){\n this.first = first;\n this.second = second;\n }\n }\n public int minReorder(int n, int[][] connections) {\n LinkedList<Pair> q = new LinkedList<>();\n for(int i=0;i<n-1;i++){\n q.add(new Pair(connections[i][0], connections[i][1]));\n }\n boolean[] hasReached = new boolean[n];\n hasReached[0] = true;\n int ans = 0;\n while(q.size()>0){\n Pair temp = q.get(0);\n q.removeFirst();\n if(hasReached[temp.first]){\n hasReached[temp.second] = true;\n ans++;\n }\n else if(hasReached[temp.second]){\n hasReached[temp.first] = true;\n }else{\n q.add(temp);\n }\n }\n return ans;\n }\n}\n```\n\nSecond approach: (Simple dfs in undirected graph)\n1. Make an adjacency list , but make the graph undirected.\n2. Make a parent array of size n, where parent[i] is parent of i , when the tree is rooted at zero.\n3. Do a dfs with 0 as root and fill the parent array\n4. Now as we know who is parent of whom traverse the given edges and see what edges are from parent to child in original directed graph. These edges have to be changed, hence increment the answer everytime you find such an edge.\n\n```\nclass Solution {\n public int minReorder(int n, int[][] connections) {\n ArrayList<ArrayList<Integer>> adj = new ArrayList<>();\n for(int i=0;i<n;i++){\n adj.add(new ArrayList<Integer>());\n } \n \n for(int i=0;i<n-1;i++){\n adj.get(connections[i][0]).add(connections[i][1]);\n adj.get(connections[i][1]).add(connections[i][0]);\n }\n \n int[] parent = new int[n];\n parent[0] = -1; \n dfs(adj,0,-1,parent);\n \n int ans = 0;\n for(int i=0;i<n-1;i++){\n int child = connections[i][0];\n int pat = connections[i][1];\n if(parent[child]!=pat){\n ans++;\n }\n }\n \n return ans;\n }\n \n private static void dfs(ArrayList<ArrayList<Integer>> adj,int source, int p, int[] parent){\n for(int s: adj.get(source)){\n if(s!=p){\n parent[s] = source;\n dfs(adj,s,source,parent);\n }\n }\n }\n}\n``` | 4 | 0 | ['Depth-First Search', 'Queue', 'Java'] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | C++ | DFS with explanation | c-dfs-with-explanation-by-xpharry-38vt | Assume each city can reach the city 0, then from the city 0, it can always reach all the cities if the connections are all reversed. Then we can traverse this r | xpharry | NORMAL | 2021-03-09T23:15:53.069256+00:00 | 2021-03-09T23:15:53.069289+00:00 | 331 | false | Assume each city can reach the city 0, then from the city 0, it can always reach all the cities if the connections are all reversed. Then we can traverse this reversed directed-graph and check each connection without repeatation, we can get the minimum number of edges to change by counting the connection that can be found in "connections".\n\nUse the example from the description as below, the desired changes of the connections are shown in red. After changing, it becomes a directed-graph connection each leave to the centroid, namely the city 0. Assume we can flip the connection here, then the graph becomes a directed graph from city 0 to each leave.\n\nBy traversing from the centroid, the city 0, it goes through each connection but with the opposite direction. Once you find any connection is along with your moving direction then it should be counted.\n\n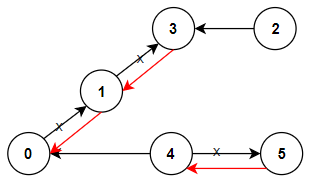\n\nIt is straight forward to use DFS to traverse the graph here.\n\nThe code is as below.\n\n```\nclass Solution {\npublic:\n int minReorder(int n, vector<vector<int>>& connections) {\n // build undirected graph\n vector<vector<int>> graph(n);\n for(auto& conn : connections) {\n graph[conn[0]].push_back(conn[1]);\n graph[conn[1]].push_back(conn[0]);\n }\n \n // set\n set<vector<int>> conn_set(connections.begin(), connections.end());\n \n // dfs\n int ans = 0;\n vector<bool> visited(n);\n dfs(graph, conn_set, visited, 0, ans);\n \n return ans;\n }\n \n void dfs(vector<vector<int>>& graph, set<vector<int>>& conn_set, vector<bool>& visited, int cur, int& ans) {\n if(visited[cur]) return;\n \n visited[cur] = true;\n\n for(int next : graph[cur]) { \n if(!visited[next]) {\n if(conn_set.count({cur, next})) {\n ans += 1;\n }\n dfs(graph, conn_set, visited, next, ans);\n }\n }\n }\n};\n``` | 4 | 1 | [] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Java || BFS || O(n) | java-bfs-on-by-legendarycoder-8hkb | \n public int minReorder(int n, int[][] connections) {\n int count = 0;\n\t\tList[] graph = new ArrayList[n];\n\t\tfor (int i = 0; i < n; i++)\n\t\t\t | LegendaryCoder | NORMAL | 2021-02-20T06:48:17.723022+00:00 | 2021-02-20T06:48:17.723051+00:00 | 285 | false | \n public int minReorder(int n, int[][] connections) {\n int count = 0;\n\t\tList<Integer>[] graph = new ArrayList[n];\n\t\tfor (int i = 0; i < n; i++)\n\t\t\tgraph[i] = new ArrayList<Integer>();\n\n\t\tfor (int[] connection : connections) {\n\t\t\tgraph[connection[0]].add(connection[1]);\n\t\t\tgraph[connection[1]].add(-connection[0]);\n\t\t}\n\n\t\tQueue<Integer> queue = new LinkedList<Integer>();\n\t\tboolean[] visited = new boolean[n];\n\t\tvisited[0] = true;\n\t\tqueue.add(0);\n\n\t\twhile (!queue.isEmpty()) {\n\t\t\tint temp = queue.poll();\n\t\t\tList<Integer> nbrs = graph[temp];\n\t\t\tfor (int nbr : nbrs) {\n\t\t\t\tif (visited[Math.abs(nbr)])\n\t\t\t\t\tcontinue;\n visited[Math.abs(nbr)] = true;\n\t\t\t\tif (nbr > 0)\n\t\t\t\t\tcount++;\n\t\t\t\tqueue.add(Math.abs(nbr));\n\t\t\t}\n\t\t}\n\n\t\treturn count;\n }\n | 4 | 0 | [] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Java 100%, simplified topological sort, no tree/graph needed | java-100-simplified-topological-sort-no-fa2wg | Simplified topological sort, keep iterate cities that can be reached by flipping direction until all cities can be reached.\n\nclass Solution {\n public int | tao68 | NORMAL | 2021-01-04T22:41:43.470108+00:00 | 2021-01-04T22:41:43.470146+00:00 | 302 | false | Simplified topological sort, keep iterate cities that can be reached by flipping direction until all cities can be reached.\n```\nclass Solution {\n public int minReorder(int n, int[][] connections) {\n boolean[] reach = new boolean[n]; // this array tracks cities reachable so far\n reach[0] = true; //mark city 0 as reachable\n int sum = 0; // result\n int count = 1; // this counter counts how many reachable cities, once it reaches n, terminate\n while (count < n){ \n for (int[] connection : connections){\n int source = connection[0], destination = connection[1];\n\t\t\t\t\n\t\t\t\t//if destination is reachable, but source is not, source can be marked as reachable\n if (reach[destination] && !reach[source]) {\n reach[source] = true;\n count++;\n continue;\n }\n\t\t\t\t\n //if source is reachable, but destination is not, we can flip the direction and mark destination reachable.\n if (reach[source] == true && !reach[destination]){\n reach[destination] = true;\n connection[0] = destination;\n connection[1] = source;\n count++;\n sum ++;\n }\n }\n }\n return sum;\n }\n}\n``` | 4 | 0 | [] | 2 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Javascript BFS, easy to understand. | javascript-bfs-easy-to-understand-by-and-4jd9 | Thinking process:\nSince the target is to minimize the number of road to reverse so that all cities can reach city 0, then for those directly connected to 0, sa | andyoung | NORMAL | 2020-10-03T22:28:30.287882+00:00 | 2020-10-03T22:58:45.890791+00:00 | 721 | false | **Thinking process:**\nSince the target is to minimize the number of road to reverse so that all cities can reach city `0`, then for those directly connected to `0`, say `nodes` , if it\'s already in the right direction, no change; if otherwise, reverse it. Same idea applies to the remaining cities that connected to `nodes`.\n\n```javascript\nvar minReorder = function(n, connections) {\n let ans = 0, nodes = [0];\n const connSet = new Set(connections);\n while (nodes.length) {\n const tmp = new Set(nodes);\n nodes = [];\n [...connSet].forEach(c => {\n if (tmp.has(c[0])) {\n ans += 1;\n nodes.push(c[1]);\n connSet.delete(c);\n } else if (tmp.has(c[1])) {\n nodes.push(c[0]);\n connSet.delete(c);\n }\n });\n }\n return ans;\n};\n```\n\n**Updated:**\nGot this code below (top 1 performance) from the Submission Detail page, very nice idea. Put it here just for references (modified a little bit to make it more readable for me). Not 100% sure about the rationale behind this code though, I\'ll try my best to interpret it.\n\nThe basic idea is to record the distances for all cities to city `0`, regardless of the direction. So imagine the goal is to reach city `0`, and for one of the roads `[src, dest]`, if the distance from `src` to `0` is greater than distance from `dest` to `0`, it means you are heading to the right direction, like getting nearer towards city `0`. Otherwise, it means you are heading farther from city `0`, then the road should be reversed.\n\n```javascript\nvar minReorder = function(n, connections) {\n const dist = Array(n).fill(-1);\n dist[0] = 0;\n let unknowns = n - 1;\n while (unknowns) {\n connections.forEach(([src, dest]) => {\n if (dist[src] != -1 && dist[dest] == -1) {\n dist[dest] = dist[src] + 1;\n --unknowns;\n } else if (dist[dest] != -1 && dist[src] == -1) {\n dist[src] = dist[dest] + 1;\n --unknowns;\n }\n });\n }\n let ans = 0;\n connections.forEach(([src, dest]) => {\n if (dist[src] < dist[dest]) ++ans;\n });\n return ans;\n};\n``` | 4 | 0 | ['Breadth-First Search', 'JavaScript'] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | [Python] 7-line DFS solution | python-7-line-dfs-solution-by-m-just-2n03 | python\ndef minReorder(self, n: int, connections: List[List[int]]) -> int:\n edges = collections.defaultdict(list)\n for a, b in connections:\n edg | m-just | NORMAL | 2020-09-21T19:51:19.182805+00:00 | 2020-09-21T19:51:19.182837+00:00 | 425 | false | ```python\ndef minReorder(self, n: int, connections: List[List[int]]) -> int:\n edges = collections.defaultdict(list)\n for a, b in connections:\n edges[a].append((b, 1))\n edges[b].append((a, 0))\n\n def dfs(i, p):\n return sum(d + dfs(j, i) for j, d in edges[i] if j != p)\n\n return dfs(0, -1)\n``` | 4 | 0 | ['Depth-First Search', 'Python'] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Python Beats 100% with Explanation (Better than Editorial) | python-beats-100-with-explanation-better-p63j | Approach: BFS/Greedy Search with a Seen SetProblem Breakdown
You are givenncities labeled from0ton-1and a list ofconnections, whereconnections[i] = [a, b]repres | jxmils | NORMAL | 2025-02-19T14:20:00.915629+00:00 | 2025-02-19T14:20:00.915629+00:00 | 744 | false | ### `Approach: BFS/Greedy Search with a Seen Set`
#### `Problem Breakdown`
- You are given `n` cities labeled from `0` to `n-1` and a list of `connections`, where `connections[i] = [a, b]` represents a directed road from city `a` to city `b`.
- You must reorder some roads so that **every city can reach city `0`**.
- Return the **minimum number of roads that need to be reversed**.
---
### `Step-by-Step Breakdown`
1. **Initialize Data Structures**
- `seen = {0}`: A set to track visited cities, starting with city `0`.
- `ans = 0`: A counter to track the number of edges that need to be reversed.
2. **Iterate Until All Cities Are Visited**
- Continue processing while `len(seen) < n` (until all cities are visited).
- Create a temporary `check = []` list to store unprocessed connections.
3. **Process Each Connection**
- If `path[1]` is already in `seen`, it means the road is **correctly directed** (`b → a`), so just mark `path[0]` as seen.
- If `path[0]` is already in `seen`, it means we must **reverse the road** (`a → b`), so:
- Mark `path[1]` as seen.
- Increase `ans` since we are reversing the edge.
- Otherwise, add the unprocessed connection to `check`.
4. **Reverse Remaining Paths in `connections`**
- At the end of each iteration, update `connections = check[::-1]` to process remaining roads in the next loop.
5. **Return the Number of Reversals (`ans`)**
- This ensures that all roads lead to city `0` using the minimum number of reversals.
---
### `Time and Space Complexity Analysis`
- **Time Complexity: `O(n²)` in the worst case**
- We may check all `n` edges multiple times (`O(n)` iterations × `O(n)` operations per iteration).
- However, in most cases, it is **closer to `O(n)`** since we gradually reduce the number of unprocessed edges.
- **Space Complexity: `O(n)`**
- The `seen` set stores up to `n` cities.
---
### `Example Walkthrough`
#### `Input`
```python
from typing import List
class Solution:
def minReorder(self, n: int, connections: List[List[int]]) -> int:
# Initialize a set to track visited cities
seen = {0}
# Counter for the number of roads that need to be reversed
ans = 0
while len(seen) < n:
check = []
for path in connections:
if path[1] in seen:
seen.add(path[0])
elif path[0] in seen:
seen.add(path[1])
ans += 1
else:
check.append(path)
# Reverse the order of unprocessed connections for the next iteration
connections = check[::-1]
return ans
```
| 3 | 0 | ['Python3'] | 3 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Python, beats 87%, clean solution with recursion | python-beats-87-clean-solution-with-recu-wsot | IntuitionDespite directioned edge, we should setup graph with biderectional edges, with marking current direction.
Setup recursion, which will consider conectio | dev_lvl_80 | NORMAL | 2025-02-03T06:43:51.289009+00:00 | 2025-02-03T06:43:51.289009+00:00 | 488 | false | # Intuition
Despite directioned edge, we should setup graph with biderectional edges, with marking current direction.
Setup recursion, which will consider conection existance and current direction
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
O(N)
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def minReorder(self, n: int, connections: List[List[int]]) -> int:
g = defaultdict(lambda : [])
for a, b in connections:
g[a].append([b, 1]) # direction out
g[b].append([a, 0])
def traverse(node, prev_node=None, cnt_changes=0) ->int:
for next_node, direction in g[node]:
if next_node == prev_node:
continue
cnt_changes += direction + traverse(next_node, node)
return cnt_changes
return traverse(0)
``` | 3 | 0 | ['Python3'] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Easy to Understand simple Bfs beats 100% O(n) | easy-to-understand-simple-bfs-beats-100-en6f0 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | hemanth2-ko | NORMAL | 2024-10-23T17:02:25.007594+00:00 | 2024-10-23T17:02:25.007631+00:00 | 240 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n int minReorder(int n, vector<vector<int>>& connections) {\n unordered_set<int> passingcities;\n unordered_map<int, vector<int>> graph;\n for(int i = 0; i < connections.size(); i++) {\n graph[connections[i][0]].push_back(connections[i][1]);\n }\n\n unordered_map<int, vector<int>> cities_passed;\n for(int i = 0; i < connections.size(); i++) {\n cities_passed[connections[i][1]].push_back(connections[i][0]);\n }\n\n queue<int> q;\n q.push(0);\n while(!q.empty()) {\n int temp = q.front();\n q.pop();\n passingcities.insert(temp);\n int i = 0;\n while(i < cities_passed[temp].size()) {\n if(passingcities.find(cities_passed[temp][i]) == passingcities.end()) {\n q.push(cities_passed[temp][i]);\n }\n i++;\n }\n }\n if(passingcities.size() == n) return 0;\n\n queue<int> map;\n for(int num : passingcities) {\n map.push(num);\n }\n int count = 0;\n while(!map.empty()) {\n int temp = map.front();\n map.pop();\n int i = 0;\n for(int num : cities_passed[temp]) {\n if(passingcities.find(num) == passingcities.end()) {\n passingcities.insert(num);\n cout << "added " << num << " due to " << temp << endl;\n map.push(num);\n }\n }\n while(i < graph[temp].size()) {\n if(passingcities.find(graph[temp][i]) == passingcities.end()) {\n passingcities.insert(graph[temp][i]);\n count++;\n cout << "edge changed for " << temp << " " << graph[temp][i] << endl;\n map.push(graph[temp][i]);\n cout << "passing cities " << graph[temp][i] << endl;\n }\n i++;\n }\n }\n return count;\n }\n};\n``` | 3 | 0 | ['C++'] | 3 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Solution By Dare2Solve | Detailed Explanation | Clean Code | solution-by-dare2solve-detailed-explanat-59kg | Explanation []\nauthorslog.com/blog/a89c2S0zHB\n\n# Code\n\ncpp []\nclass Solution {\npublic:\n int minReorder(int n, vector<vector<int>>& connections) {\n | Dare2Solve | NORMAL | 2024-08-22T08:44:24.649503+00:00 | 2024-08-22T08:44:24.649532+00:00 | 1,265 | false | ```Explanation []\nauthorslog.com/blog/a89c2S0zHB\n```\n# Code\n\n```cpp []\nclass Solution {\npublic:\n int minReorder(int n, vector<vector<int>>& connections) {\n vector<vector<int>> grid(n);\n vector<int> vis(n, 0);\n\n for (const auto& conn : connections) {\n int src = conn[0];\n int dest = conn[1];\n grid[src].push_back(-1 * dest);\n grid[dest].push_back(src);\n }\n\n return solve(grid, 0, vis);\n }\n\nprivate:\n int solve(vector<vector<int>>& grid, int val, vector<int>& vis) {\n if (vis[val] == 1) {\n return 0;\n }\n int ans = 0;\n vis[val] = 1;\n for (int ele : grid[val]) {\n if (vis[abs(ele)] == 1) {\n continue;\n }\n if (ele < 0) {\n ans += 1;\n }\n ans += solve(grid, abs(ele), vis);\n }\n return ans;\n }\n};\n```\n\n```python []\nclass Solution:\n def minReorder(self, n, connections):\n grid = [[] for _ in range(n)]\n vis = [False] * n\n\n for src, dest in connections:\n grid[src].append(-dest)\n grid[dest].append(src)\n\n return self.solve(grid, 0, vis)\n\n def solve(self, grid, val, vis):\n if vis[val]:\n return 0\n ans = 0\n vis[val] = True\n for ele in grid[val]:\n if vis[abs(ele)]:\n continue\n if ele < 0:\n ans += 1\n ans += self.solve(grid, abs(ele), vis)\n return ans\n\n# Example usage:\nsol = Solution()\nn = 6\nconnections = [[0, 1], [1, 3], [2, 3], [4, 0], [4, 5]]\nprint(sol.minReorder(n, connections))\n```\n\n```java []\npublic class Solution {\n\n private int solve(List<List<Integer>> grid, int val, boolean[] vis) {\n if (vis[val]) {\n return 0;\n }\n int ans = 0;\n vis[val] = true;\n for (int ele : grid.get(val)) {\n if (vis[Math.abs(ele)]) {\n continue;\n }\n if (ele < 0) {\n ans += 1;\n }\n ans += solve(grid, Math.abs(ele), vis);\n }\n return ans;\n }\n\n public int minReorder(int n, int[][] connections) {\n List<List<Integer>> grid = new ArrayList<>();\n boolean[] vis = new boolean[n];\n\n for (int i = 0; i < n; i++) {\n grid.add(new ArrayList<>());\n }\n\n for (int[] conn : connections) {\n int src = conn[0];\n int dest = conn[1];\n grid.get(src).add(-1 * dest);\n grid.get(dest).add(src);\n }\n\n return solve(grid, 0, vis);\n }\n\n public static void main(String[] args) {\n Solution solution = new Solution();\n int n = 6;\n int[][] connections = {{0, 1}, {1, 3}, {2, 3}, {4, 0}, {4, 5}};\n System.out.println(solution.minReorder(n, connections));\n }\n}\n```\n\n```javascript []\nvar minReorder = function (n, connections) {\n let adj = [], grid = [], vis = [], len = connections.length;\n for (let i = 0; i < n; i++) {\n grid.push([]);\n vis[i] = 0;\n }\n for (let i = 0; i < len; i++) {\n let src = connections[i][0];\n let dest = connections[i][1];\n grid[src].push(-1 * dest);\n grid[dest].push(src);\n }\n console.log(grid);\n return solve(grid, 0, vis);\n};\nfunction solve(grid, val, vis) {\n if (vis[val] === 1) {\n return 0;\n }\n let ans = 0;\n vis[val] = 1;\n for (let i = 0; i < grid[val].length; i++) {\n let ele = grid[val][i];\n if (vis[Math.abs(ele)] == 1) {\n continue;\n }\n if (ele < 0) {\n ans += 1;\n }\n ans += solve(grid, Math.abs(ele), vis);\n }\n return ans;\n}\n``` | 3 | 1 | ['Breadth-First Search', 'Graph', 'Python', 'C++', 'Java', 'Python3', 'JavaScript'] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Java Level-Order Traversal Solution Using BFS with Detailed Explanations | java-level-order-traversal-solution-usin-bxqr | Intuition\nWhen should we change the direction of a route? If the route starts from a city close to city 0 and ends at a city far from city 0, we need to change | Michael-Qu | NORMAL | 2024-06-17T22:38:51.968529+00:00 | 2024-06-17T22:38:51.968550+00:00 | 287 | false | # Intuition\nWhen should we change the direction of a route? If the route starts from a city close to city 0 and ends at a city far from city 0, we need to change its direction since it brings people "away" from city 0. Otherwise we don\'t need to change its direction since it already meets our need. In this case, once we get the distance from each city to city 0, we can run a simple iteration to count the number of routes that needs to be changed.\n\nTo get the distance from each city to city 0, we can traverse the associated undirected graph in the level order using breath-first search (BFS).\n\n# Approach\nThe algorithm is as follows.\n1. Create the adjacency list representation of the associated undirected graph.\n2. Initialize the adjacent list by iterate over all the edges `connections`.\n3. Conduct BFS on the undirected graph in the level order, starting from city 0. Update `distToZero` during the traversal.\n4. Initialize the counter `ans` for final output. Iterate over `connections` again, if `distToZero[e[0]] < distToZero[e[1]]`, this edge leads away from city 0 so we need to increment the counter `ans`.\n5. Return `ans` as the final result.\n\n# Complexity\n- Time complexity: $$O(n)$$\n\n- Space complexity: $$O(n)$$\n\n# Code\n\n```\nclass Solution {\n public int minReorder(int n, int[][] connections) {\n // BFS Approach\n // TC: O(n), SC: O(n)\n // distToZero[i] = distance from city i to city 0\n int[] distToZero = new int[n];\n List<List<Integer>> adjList = new ArrayList<>();\n for (int i = 0; i < n; i++) {\n adjList.add(new ArrayList<>());\n }\n for (int[] e : connections) {\n adjList.get(e[0]).add(e[1]);\n adjList.get(e[1]).add(e[0]);\n }\n // BFS\n Queue<Integer> queue = new ArrayDeque<>();\n queue.offer(0);\n boolean[] visited = new boolean[n];\n visited[0] = true;\n int dist = 0;\n while (!queue.isEmpty()) {\n int size = queue.size();\n for (int i = 0; i < size; i++) {\n int curr = queue.poll();\n distToZero[curr] = dist;\n for (int nei : adjList.get(curr)) {\n if (!visited[nei]) {\n queue.offer(nei);\n visited[nei] = true;\n }\n }\n }\n dist++;\n }\n int ans = 0;\n for (int[] e : connections) {\n // If the edge directs from near to far, we change the direction\n if (distToZero[e[0]] < distToZero[e[1]]) {\n ans++;\n }\n }\n return ans;\n \n }\n}\n``` | 3 | 0 | ['Tree', 'Breadth-First Search', 'Graph', 'Queue', 'Java'] | 1 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Python | BFS | DFS | Graphs | Easy to Understand | python-bfs-dfs-graphs-easy-to-understand-zlw9 | Approach: Create a graph with both original edges and their reversed counterparts (with scores 1 and 0, respectively). Traverse the graph starting from node \' | Coder1918 | NORMAL | 2024-04-01T20:52:22.027053+00:00 | 2024-04-01T21:37:17.015638+00:00 | 933 | false | **Approach:** Create a graph with both original edges and their reversed counterparts (with scores 1 and 0, respectively). Traverse the graph starting from node \'0\', incrementing a count for each original edge that points away from node \'0\'. This count represents the necessary reorientations.\nBelow code provides explanations through comments.\n\n**Please Feel free to suggest optimizations!**\n\n### **Python Solution:**\n\n**BFS -- Beats 95%**\n```\nclass Solution:\n def minReorder(self, n: int, connections: List[List[int]]) -> int:\n """ BFS """\n # Construct graph\n graph = defaultdict(dict)\n for a, b in connections:\n graph[a][b] = 1 # Original Edge with score 1\n graph[b][a] = 0 # Fake edge with score 0\n \n # Initialize a deque with start node as 0\n queue = deque([0,])\n wrong_edges = 0 # Keeps track of the edges away from \'0\'\n visited = [False] * n # Avoids cyclic traversal\n visited[0] = True # Mark 0 as visited since we start from it\n \n # BFS Traversal\n while queue:\n start = queue.popleft()\n for nei, score in graph[start].items():\n if not visited[nei]:\n # When the original edge is towards \'0\' then score is 0 otherwise 1\n wrong_edges += score\n queue.append(nei)\n visited[nei] = True\n \n return wrong_edges\n```\n**DFS**\n```\nclass Solution:\n def minReorder(self, n: int, connections: List[List[int]]) -> int:\n graph = defaultdict(set)\n for a, b in connections:\n graph[a].add((b, 1)) # Original Edge\n graph[b].add((a, 0)) # Fake Edge to enable complete tree traversal\n \n # DFS to traverse over the edges and\n # increment self.count when an edge directs away from \'0\'\n def dfs(start):\n for nei, is_real in graph[start]:\n if not visited[nei]:\n self.count += is_real\n visited[nei] = True\n dfs(nei)\n \n # To avoid visiting the same node again\n visited = [False] * n\n # Mark the first node as visited\n visited[0] = True\n # To keep track of edges that go away from \'0\'\n self.count = 0\n \n # Start traversing the graph from \'0\'\n dfs(0)\n \n return self.count\n``` | 3 | 0 | ['Depth-First Search', 'Breadth-First Search', 'Graph', 'Python3'] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Breadth-first search | breadth-first-search-by-burkh4rt-dnfi | Approach\nFirst create a lookup dictionary adj that maps vertices to adjacent/contiguous edges. Initialize 0 as the current vertex set curr and the set seen of | burkh4rt | NORMAL | 2024-01-28T15:02:28.931059+00:00 | 2024-01-28T15:02:28.931094+00:00 | 911 | false | # Approach\nFirst create a lookup dictionary `adj` that maps vertices to adjacent/contiguous edges. Initialize 0 as the current vertex set `curr` and the set `seen` of vertices that have been visited. Iteratively update `curr` by adding vertices adjacent to the current set if they have not already been seen. Edges `e` where `e[1] in curr` and `e[0] not in seen` are already pointing in the correct direction; edges `e` where `e[0] in curr` and `e[1] not in seen`must be flipped. Keep track of the flips `s` and return the total when there are no more vertices in `curr`.\n\n# Code\n```python\nclass Solution:\n def minReorder(self, n: int, connections: List[List[int]]) -> int:\n s = 0\n # create dictionary mapping vertices to their adjacent edges\n adj = defaultdict(list)\n for c in connections:\n adj[c[0]].append(c)\n adj[c[1]].append(c)\n\n # run the search\n curr = seen = {0}\n while curr:\n new = set() # will contain neighbors of `curr` not in `seen`\n for v in curr:\n for e in adj[v]:\n if e[0] not in seen and e[1] == v:\n # pointing the \'right\' direction\n new.add(e[0])\n elif e[0] == v and e[1] not in seen:\n # pointing the \'wrong\' direction\n new.add(e[1])\n s += 1\n curr = new\n seen.update(new)\n\n return s\n``` | 3 | 0 | ['Breadth-First Search', 'Python3'] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Reorder Nodes | reorder-nodes-by-noob_1012-q88m | \n/**\n * @param {number} n\n * @param {number[][]} connections\n * @return {number}\n */\nvar minReorder = function(n, connections) {\n let visitSet = new S | noob_1012 | NORMAL | 2024-01-08T14:13:58.358096+00:00 | 2024-01-08T14:14:21.137845+00:00 | 151 | false | ```\n/**\n * @param {number} n\n * @param {number[][]} connections\n * @return {number}\n */\nvar minReorder = function(n, connections) {\n let visitSet = new Set();\n let count = 0;\n let neighbours = new Map();\n let edges = new Set();\n for(let i=0;i<n;i++){\n neighbours[i] = [];\n }\n \n for(let [n1,n2] of connections){\n neighbours[n1].push(n2);\n neighbours[n2].push(n1);\n edges.add(`${n1}.${n2}`)\n }\n \n console.log(\'edges\',edges);\n \n const dfs = city => {\n for(let neighbour of neighbours[city]){\n if(visitSet.has(neighbour))\n continue;\n if(!edges.has(`${neighbour}.${city}`)){\n console.log(`${neighbour}.${city}`);\n count++;\n }\n visitSet.add(neighbour);\n dfs(neighbour);\n }\n }\n visitSet.add(0);\n dfs(0);\n return count;\n};\n``` | 3 | 0 | ['Depth-First Search', 'JavaScript'] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | [C] DFS, graph | c-dfs-graph-by-leetcodebug-im4d | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | leetcodebug | NORMAL | 2023-07-17T15:36:33.281927+00:00 | 2023-07-17T15:36:33.281977+00:00 | 24 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n/*\n * 1466. Reorder Routes to Make All Paths Lead to the City Zero\n *\n * There are n cities numbered from 0 to n - 1 and n - 1 roads \n * such that there is only one way to travel between two different \n * cities (this network form a tree). Last year, The ministry of \n * transport decided to orient the roads in one direction because \n * they are too narrow.\n * \n * Roads are represented by connections where connections[i] = [ai, bi] \n * represents a road from city ai to city bi.\n * \n * This year, there will be a big event in the capital (city 0), and many \n * people want to travel to this city.\n * \n * Your task consists of reorienting some roads such that each city can \n * visit the city 0. Return the minimum number of edges changed.\n * \n * It\'s guaranteed that each city can reach city 0 after reorder.\n *\n * 2 <= n <= 5 * 10^4\n * connections.length == n - 1\n * connections[i].length == 2\n * 0 <= ai, bi <= n - 1\n * ai != bi\n *\n *\n */\n \ntypedef struct dst {\n struct dst *next;\n int id;\n} dst_t;\n \nint dfs(dst_t **orig_adj, dst_t **bidir_adj, int n, int curr, bool *visited)\n{\n int cnt = 0;\n bool same_dir = false;\n \n visited[curr] = true;\n \n /* Check if we can move to next node (away from node 0) */\n for (dst_t *tmp1 = bidir_adj[curr]; tmp1; tmp1 = tmp1->next) {\n\n if (visited[tmp1->id] == false) {\n \n same_dir = false;\n \n /* \n * \n * Check the original neighbor list, check if it is possible to \n * move from the next city back to the current city.\n * If not, we need to reverse the original road direction\n */\n for (dst_t *tmp2 = orig_adj[tmp1->id]; tmp2; tmp2 = tmp2->next) {\n if (tmp2->id == curr) {\n same_dir = true;\n break;\n }\n }\n \n if (same_dir == false) {\n cnt++;\n }\n \n /* Move to next city, and contiune to check the roads between different cities */\n cnt += dfs(orig_adj, bidir_adj, n, tmp1->id, visited);\n }\n \n }\n\n return cnt;\n}\n\n/*\n * Algorithm:\n * We know that all the cities are connected together\n * but some of roads have incorrect direct so this \n * cause some cities can visit city 0\n *\n * E.g:\n * 0 <- 1 -> 2 <- 3 -> 4\n *\n * We can create a bidirectional map\n * 0 <- 1 -> 2 <- 3 -> 4\n * -> -> -> ->\n *\n * Start to city 0, traverse to other cities and also compare the\n * original road direction between these cities, its the direction is different\n * we need to reverse the road direction\n *\n * 0 <- 1 -> 2 <- 3 -> 4\n * -> -> -> ->\n * 1 2 => Ans = 2;\n */\n \nint minReorder(int n, int** connections, int connectionsSize, int* connectionsColSize){\n\n /*\n * Input:\n * n, n cities\n * connections, roads between cities\n * connectionsSize, number of roads\n * connectionsColSize\n */\n\n int ans = 0;\n bool *visited = (bool *)calloc(1, sizeof(bool) * n);\n dst_t **orig_adj = (dst_t **)calloc(1, sizeof(dst_t *) * n);\n dst_t **bidir_adj = (dst_t **)calloc(1, sizeof(dst_t *) * n);\n dst_t *tmp, *del;\n \n /* Build origianl adjacent list and a bidirectional adjacent list */\n for (int i = 0; i < connectionsSize; i++) {\n /* orig_adj[from][to] */\n tmp = (dst_t *)malloc(sizeof(dst_t));\n tmp->id = connections[i][1];\n tmp->next = orig_adj[connections[i][0]];\n orig_adj[connections[i][0]] = tmp;\n \n /* Bidirection */\n tmp = (dst_t *)malloc(sizeof(dst_t));\n tmp->id = connections[i][1];\n tmp->next = bidir_adj[connections[i][0]];\n bidir_adj[connections[i][0]] = tmp;\n \n tmp = (dst_t *)malloc(sizeof(dst_t));\n tmp->id = connections[i][0];\n tmp->next = bidir_adj[connections[i][1]];\n bidir_adj[connections[i][1]] = tmp;\n }\n \n ans = dfs(orig_adj, bidir_adj, n, 0, visited);\n \n for (int i = 0; i < n; i++) {\n \n for (tmp = orig_adj[i]; tmp; ) {\n del = tmp;\n tmp = tmp->next;\n free(del);\n }\n \n for (tmp = bidir_adj[i]; tmp; ) {\n del = tmp;\n tmp = tmp->next;\n free(del);\n }\n\n }\n\n free(orig_adj);\n free(bidir_adj);\n free(visited);\n \n /*\n * Output:\n * Return the minimum number of edges changed.\n */\n \n return ans;\n}\n``` | 3 | 0 | ['Depth-First Search', 'Graph', 'C'] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Java easy with bfs | java-easy-with-bfs-by-mohd_danish_khan-g019 | Approach\ncreate a map {city : neighbours} and set of all the edges\n\nnow do a bfs and check on every city if there is an edge (neigh, city) in our edge set, i | Mohd_Danish_Khan | NORMAL | 2023-07-13T21:53:39.227780+00:00 | 2023-07-13T21:53:39.227797+00:00 | 632 | false | # Approach\ncreate a map {city : neighbours} and set of all the edges\n\nnow do a bfs and check on every city if there is an edge (neigh, city) in our edge set, if its not their increase the changes count. and move on to next city. keep visited set to avoid checking the already checked node.\n\n# Code\n```\nclass Solution {\n public int minReorder(int n, int[][] connections) {\n\n HashMap<Integer,List<Integer>> adjc = new HashMap<>();\n HashSet<List<Integer>> edges = new HashSet<>();\n HashSet<Integer> visited = new HashSet();\n\n Queue<Integer> q = new LinkedList<>();\n int changes =0;\n\n\n for( int[] a : connections)\n {\n List<Integer> edge = new ArrayList();\n edge.add(a[0]);\n edge.add(a[1]);\n edges.add(edge);\n System.out.println(edge);\n\n List<Integer> l1 = adjc.getOrDefault(a[0],new ArrayList());\n List<Integer> l2 = adjc.getOrDefault(a[1],new ArrayList());\n\n l1.add(a[1]);\n l2.add(a[0]);\n adjc.put(a[0],l1);\n adjc.put(a[1],l2);\n }\n\n q.add(0);\n\n while(!q.isEmpty())\n {\n int node = q.poll();\n visited.add(node);\n\n for(Integer neigh : adjc.get(node))\n {\n if(!visited.contains(neigh))\n {\n q.add(neigh);\n List<Integer> nedge = new ArrayList();\n nedge.add(neigh);\n nedge.add(node);\n \n if(!edges.contains(nedge)) changes++;\n \n }\n }\n }\n return changes;\n }\n}\n``` | 3 | 0 | ['Java'] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Solution in C++ | solution-in-c-by-ashish_madhup-x8ch | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | ashish_madhup | NORMAL | 2023-03-24T15:16:22.745508+00:00 | 2023-03-24T15:16:22.745532+00:00 | 203 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minReorder(int n, vector<vector<int>>& con)\n {\n vector<vector<int>> adj(n),bk(n);\n vector<int> vis(n,0);\n vis[0] =1;\n for(auto a : con)\n {\n adj[a[0]].push_back(a[1]);\n bk[a[1]].push_back(a[0]);\n }\n int ans=0;\n queue<int> q;\n q.push(0);\n while(!q.empty())\n {\n int a = q.front();\n vis[a] =1;\n q.pop();\n for( int no : adj[a])\n {\n if(!vis[no]){\n ans++;\n q.push(no);\n }\n }\n for( int no : bk[a])\n {\n if(!vis[no])\n {\n q.push(no);\n }\n }\n }\n return ans;\n }\n};\n``` | 3 | 0 | ['C++'] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | reorder routes to make all paths lead to the city zero | reorder-routes-to-make-all-paths-lead-to-e7eu | Intuition\nstore all given connections in a adjaceny list smartly (in pair form) such that given connections can be checked and created connection can be checke | nobita_n0bi | NORMAL | 2023-03-24T13:56:23.061090+00:00 | 2023-03-24T13:56:23.061127+00:00 | 340 | false | # Intuition\nstore all given connections in a adjaceny list smartly (in pair form) such that given connections can be checked and created connection can be checked at the time of dfs function working\n\n# Approach\nnow let\'s talk about complete approach i stored all given connection in a adjaency list of pairs such that storing connected node contains 0 with other node and myself created node contains -1 with other node (why i do it because when i call dfs i can know when i call dfs for child then i can checked if it comes from given connection or it comes from my created connections and increase the counter if it comes from my created connections ( how i checked from where it comes > just check if its pair\'s second element if 0 or 1 (see code for clarification)))\n\n# Complexity\n- Time complexity:\nO(n+m)\n\n- Space complexity:\nO(n+m)\n\n# Code\n```\nclass Solution {\npublic:\n\n void dfs(int node,vector<pair<int,int>>adj[],int &cnt,vector<bool>&vis)\n {\n vis[node]=true;\n for(auto child:adj[node])\n {\n auto it=child.first;\n if(!vis[it])\n {\n if(child.second)\n {\n cnt++;\n }\n dfs(it,adj,cnt,vis);\n }\n }\n }\n int minReorder(int n, vector<vector<int>>& v) {\n vector<pair<int,int>>adj[n];\n for(int i=0;i<v.size();i++)\n {\n adj[v[i][0]].push_back({v[i][1],-1});\n adj[v[i][1]].push_back({v[i][0],0});\n }\n int cnt=0;\n vector<bool>vis(n,false);\n dfs(0,adj,cnt,vis);\n return cnt;\n\n }\n};\n``` | 3 | 0 | ['Greedy', 'Graph', 'C++'] | 2 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Easy understand graph problems(C++) | easy-understand-graph-problemsc-by-vikas-z7nr | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | vikash_2003 | NORMAL | 2023-03-24T12:35:21.127995+00:00 | 2023-03-24T12:35:21.128048+00:00 | 6,118 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\nint ans=0;\n void dfs(unordered_map<int,vector<int>>&mp ,unordered_map<int,bool>&vis,int m){\n vis[m]=true;\n for(auto i:mp[m]){\n if(i>=0){\n if(!vis[i]){ \n dfs(mp,vis,i);\n }\n }\n else{\n i=abs(i);\n if(!vis[i]){ \n ans++; \n dfs(mp,vis,i);\n }\n }\n }\n }\n // ans++\n // void sol(unordered_map<int,vector<int>>&mp ,unordered_map<int,bool>&vis,int m){\n // for(auto i:mp[m]) {\n // if(!vis[i]){\n // dfs(mp,vis,i);\n // ans++;\n // }\n // }\n // }\n int minReorder(int n, vector<vector<int>>& c) {\n int s=c.size();\n unordered_map<int,vector<int>>mp;\n unordered_map<int,bool>vis;\n for(int i=0;i<s;i++){\n mp[c[i][1]].push_back(c[i][0]);\n mp[c[i][0]].push_back(-c[i][1]);\n }\n dfs(mp,vis,0);\n if(ans==0)return ans;\n return ans;\n }\n};\n``` | 3 | 0 | ['C++'] | 1 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | Using BFS C++ Easy Solution | using-bfs-c-easy-solution-by-kisna-i69m | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | kisna | NORMAL | 2023-03-24T12:01:53.872379+00:00 | 2023-03-24T12:01:53.872410+00:00 | 888 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minReorder(int n, vector<vector<int>>& connections) {\n unordered_map<int,vector<pair<int,bool>>> mp;\n for(auto it:connections)\n {\n mp[it[0]].push_back({it[1],1});\n mp[it[1]].push_back({it[0],0});\n }\n vector<int> vis(n,0);\n int ans=0;\n for(int i=0;i<n;i++)\n {\n if(!vis[i])\n {\n queue<int> q;\n q.push(i);\n vis[i]=1;\n while(!q.empty())\n {\n int node=q.front();\n q.pop();\n vis[node]=1;\n for(auto it:mp[node])\n {\n if(!vis[it.first])\n {\n if(it.second==1)\n {\n ans++;\n }\n q.push(it.first);\n vis[it.first]=1;\n }\n }\n }\n\n }\n }\n return ans;\n }\n};\n``` | 3 | 0 | ['Breadth-First Search', 'C++'] | 0 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | C# DFS | c-dfs-by-dmitriy-maksimov-13sq | Approach\nTreat the graph as undirected. Start a dfs from the root, if you come across an edge in the forward direction, you need to reverse the edge.\n\n# Comp | dmitriy-maksimov | NORMAL | 2023-03-24T10:39:52.431748+00:00 | 2023-03-24T10:39:52.431779+00:00 | 667 | false | # Approach\nTreat the graph as undirected. Start a dfs from the root, if you come across an edge in the forward direction, you need to reverse the edge.\n\n# Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(n)$$\n\n# Code\n```\npublic class Solution\n{\n private readonly Dictionary<int, List<(int city, bool direction)>> _graph = new();\n private readonly HashSet<int> _visited = new();\n private int _result;\n\n public int MinReorder(int n, int[][] connections)\n {\n foreach (var connection in connections)\n {\n var cityA = connection[0];\n var cityB = connection[1];\n\n _graph[cityA] = _graph.GetValueOrDefault(cityA, new List<(int, bool)>());\n _graph[cityA].Add((cityB, true));\n\n _graph[cityB] = _graph.GetValueOrDefault(cityB, new List<(int, bool)>());\n _graph[cityB].Add((cityA, false));\n }\n \n dfs(0);\n\n return _result;\n }\n\n private void dfs(int city)\n {\n _visited.Add(city);\n\n foreach (var (node, direction) in _graph[city])\n {\n if (_visited.Contains(node)) continue;\n\n if (direction)\n {\n ++_result;\n }\n\n dfs(node);\n }\n }\n}\n``` | 3 | 0 | ['C#'] | 2 |
reorder-routes-to-make-all-paths-lead-to-the-city-zero | dfs | dfs-by-mr_stark-3nhb | \nclass Solution {\npublic:\n \n void dfs(vector<vector<pair<int,int>>> &g,int s, int &cnt,vector<bool> &v)\n {\n if(v[s]){\n return | mr_stark | NORMAL | 2023-03-24T08:46:27.338388+00:00 | 2023-03-24T08:50:22.777067+00:00 | 275 | false | ```\nclass Solution {\npublic:\n \n void dfs(vector<vector<pair<int,int>>> &g,int s, int &cnt,vector<bool> &v)\n {\n if(v[s]){\n return ;\n }\n v[s] = true;\n \n for(auto &i: g[s])\n { \n if(!v[i.first]){\n cnt+=i.second;\n dfs(g,i.first,cnt,v);\n }\n }\n }\n \n \n int minReorder(int n, vector<vector<int>>& c) {\n vector<vector<pair<int,int>>> g(n);\n vector<bool> v(n,false);\n \n for(auto &i: c)\n {\n g[i[0]].push_back({i[1],1});\n g[i[1]].push_back({i[0],0});\n }\n \n int cnt = 0;\n dfs(g,0,cnt,v);\n return cnt;\n }\n};\n``` | 3 | 0 | ['C'] | 0 |
smallest-string-with-a-given-numeric-value | [Java/C++] Easiest Possible Exaplained!! | javac-easiest-possible-exaplained-by-hi-4imxc | How\'s going Ladies - n - Gentlemen, today we are going to solve another coolest problem i.e. Smallest String With A Given Numeric Value\n\nWhat the problem is | hi-malik | NORMAL | 2022-03-22T00:54:32.589593+00:00 | 2022-03-22T01:01:25.144052+00:00 | 7,304 | false | How\'s going Ladies - n - Gentlemen, today we are going to solve another coolest problem i.e. **Smallest String With A Given Numeric Value**\n\nWhat the problem is saying, we have to generate the **string** with **numeric sum** equals to `k` & have the `n` characters\n\nLet\'s understand with an example,\n\n**Input**: n = 3, k = 27\n**Output**: "aay"\n\nAlright, so we can have upto 3 character\'s and their sum has to be equals to k.\n\nSo, what could be our `n = 3` character\'s is **`a a a`** why these because in the problem they have mentioned that they have to be in lexicographically order.\n\n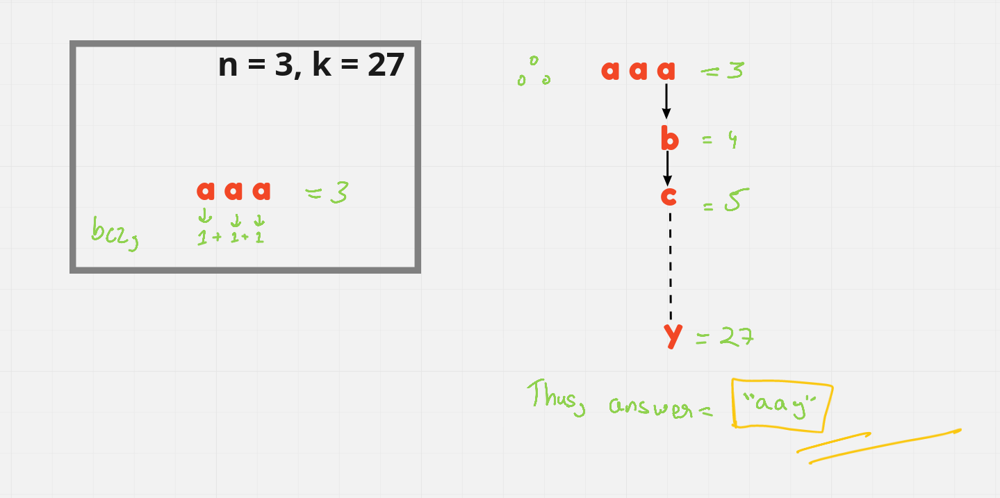\n\n```\nYou can do this same thing like in this way as well:-\na a a = 3\n|\nb = 4\n|\n|\n|\ny = 27\n\nbut this is not a lexicographicall order!!\n```\n\nSo, i hope so now you have understaood why we start from the end of the string\n\n<hr>\n\nLet\'s look at our **`Approach`** take an example:-\n\n<hr>\n\n**Input**: n = 5, k = 73\n**Output**: "aaszz"\n\nSo, we have `n = 5` & our starting character\'s could be `a a a a a`.\n* First our job is subtract **5** from **k** which will comes out `73 - 5 = 68`\n* As you can see **68 is too big**, because the maximum we can form is by adding **z into it i.e. 25**\n* So, before doing that we will check whether **k is minimum or the 25**, which ever is minimum we\'ll add into the last character.\n* And **subtract the minimum from the k**\n* Forgot to mention that, we\'ll be having one array to store the result.\n\n```\nres [] = a a a a a\n\nres[--n] += min(25, k) // get the last value min\nk -= min(25, k) // and subtarct it from k as well\n\nSo, these are the 2 thing\'s we\'ll gonna follow until k > 0\n```\n\nLet\'s implement this into our example:-\n\n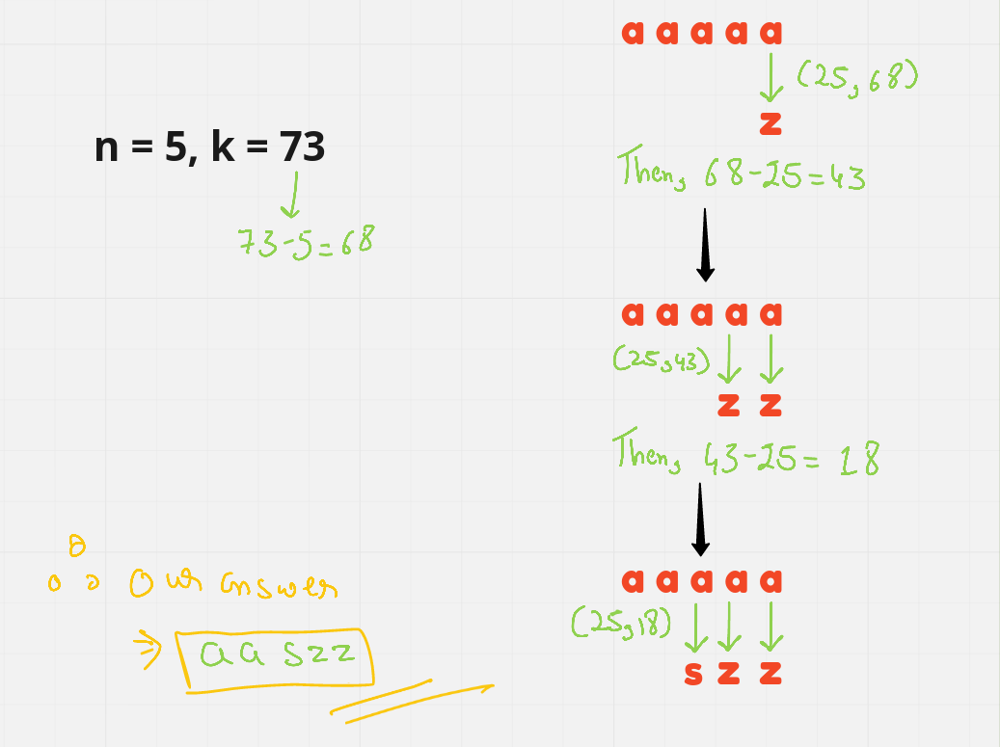\n\n*I hope so, ladies - n - gentlemen approach is absolute clear.* **Let\'s code it, up**\n\n**Java**\n```\nclass Solution {\n public String getSmallestString(int n, int k) {\n char res[] = new char[n];\n Arrays.fill(res, \'a\');\n k -= n;\n \n while(k > 0){\n res[--n] += Math.min(25, k);\n k -= Math.min(25, k);\n }\n return String.valueOf(res);\n }\n}\n```\n\n**C++**\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string res(n,\'a\');\n k -= n;\n \n while(k > 0){\n res[--n] += min(25, k);\n k -= min(25, k);\n }\n return res;\n }\n};\n```\nANALYSIS :-\n* **Time Complexity :-** BigO(N)\n\n* **Space Complexity :-** BigO(N) | 220 | 7 | [] | 30 |
smallest-string-with-a-given-numeric-value | [C++] Simple O(N) with explanation | c-simple-on-with-explanation-by-cgsgak1-rjhq | The idea is simple:\n1) Build the string of length k, which consists of letter \'a\' (lexicographically smallest string).\n2) Increment string from right to lef | cgsgak1 | NORMAL | 2020-11-22T04:01:59.788252+00:00 | 2021-03-18T20:13:00.454231+00:00 | 6,930 | false | The idea is simple:\n1) Build the string of length k, which consists of letter \'a\' (lexicographically smallest string).\n2) Increment string from right to left until it\'s value won\'t reach the target.\n\nTime complexity O(n), space complexity O(1) - no additional space used except for result string.\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string res(n, \'a\');\n k -= n;\n \n int i = res.size() - 1;\n while (k > 0) {\n int tmp = min(k, 25);\n res[i] += tmp;\n k -= tmp;\n --i;\n }\n \n return res;\n }\n}; | 131 | 10 | ['C', 'C++'] | 13 |
smallest-string-with-a-given-numeric-value | 🌻|| C++|| Easy Approach || PROPER EXPLANATION | c-easy-approach-proper-explanation-by-ab-kik0 | ALGORITHM\n\nStep - 1 Initialize a string s with n number of \'a\' to make it as a smallest possible lexiographic.\nStep - 2 Count of \'a = 1\' , so if i have | Abhay_Rautela | NORMAL | 2022-03-22T01:24:48.597520+00:00 | 2022-03-22T01:24:48.597550+00:00 | 7,437 | false | ### ALGORITHM\n```\nStep - 1 Initialize a string s with n number of \'a\' to make it as a smallest possible lexiographic.\nStep - 2 Count of \'a = 1\' , so if i have n number of \'a\' it means it sum is also \'n\'.\nStep - 3 Reduce the value of k by n (i.e k=k-n);\nStep - 4 Start traversing the string from the end because if we append any character from starting then there is chances of not getting smallest possible lexiographic order. \n\t\t Ex if we have to append \'z\' then from starting it would be like zaaa and from end it would be like aaaz which clearly shows that aaaz<zaaa.\nStep - 5 If \'k\' becomes \'0\' stop the traversal because that would be our answer.\nStep - 6 Else if k < 25, it means for getting the sum just replace ith character by \'a\'+k. And update k by k=0.\nStep - 7 Else k > 25 so iterate more and replace ith character by (\'a\'+25) and move ahead. And update k by k-25.\n\nLets take an example.\n\tI/P n=3 k=27\n\tNow follow step by step.\n\tstep-1 s=aaa (initialize a string s with n number of a)\n\tstep-2 k=k-n=27-3=24\n\tstep-3 start iterating from right most side.\n\tstep 4 check k, here k < 25, therefore update ith place of string by \'a\'+k i.e \'y\' because ASCII value of \'a\' is a 97 and 97+24 is 121 i.e \'y\'. Update k by 0. As we get our sum 27.\n\tStep 5 check k which is zero. Therefore stop iteration and return the string as it was our answer.\n```\n### CODE\n\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string s="";\n for(int i=0;i<n;i++)\n s+=\'a\';\n k=k-n;\n for(int i=n-1;i>=0;i--)\n {\n if(k==0)\n break;\n else if(k<25)\n {\n s[i] = (char)(\'a\'+k); //using (char) to convert ASCII to respective character\n k=0;\n }\n else{\n s[i] = (char)(\'a\'+25);\n k = k - 25;\n }\n }\n return s;\n }\n};\n``` | 88 | 1 | ['C'] | 15 |
smallest-string-with-a-given-numeric-value | C++ Reverse Fill | c-reverse-fill-by-votrubac-iazt | Generate the initial string with all \'a\' characters. This will reduce k by n. \n \nThen, turn rightmost \'a\' into \'z\' (\'a\' + 25, or \'a\' + k) while k | votrubac | NORMAL | 2020-11-22T04:13:25.815258+00:00 | 2020-11-22T06:21:44.391096+00:00 | 3,634 | false | Generate the initial string with all \'a\' characters. This will reduce `k` by `n`. \n \nThen, turn rightmost \'a\' into \'z\' (\'a\' + 25, or \'a\' + k) while `k` is positive.\n\n```cpp\nstring getSmallestString(int n, int k) {\n string res = string(n, \'a\');\n k -= n;\n while (k > 0) {\n res[--n] += min(25, k);\n k -= min(25, k);\n }\n return res;\n}\n``` | 85 | 7 | [] | 10 |
smallest-string-with-a-given-numeric-value | ✔️ [Python3] GREEDY FILLING (🌸¬‿¬), Explained | python3-greedy-filling-_-explained-by-ar-itri | UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.\n\nSince we are forming the lexicographically smallest string, | artod | NORMAL | 2022-03-22T02:12:40.544744+00:00 | 2022-03-22T03:22:21.950923+00:00 | 4,360 | false | **UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.**\n\nSince we are forming the lexicographically smallest string, we just simply fill our result with `a`s ( o\u02D8\u25E1\u02D8o). But hold on, that result will not necessarily have the required score c(\uFF9F.\uFF9F*) Ummm\u2026 Ok, then we can add some `z`s to the back of the result until the score reaches the required value, so be it (\uFFE2_\uFFE2;). Ofc if we are missing less than 26 to the required score, we add something that is less than `z`.\n\nTime: **O(n)** - iterations\nSpace: **O(n)** - for list of chars\n\nRuntime: 393 ms, faster than **73.27%** of Python3 online submissions for Smallest String With A Given Numeric Value.\nMemory Usage: 15.4 MB, less than **68.20%** of Python3 online submissions for Smallest String With A Given Numeric Value.\n\n```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n res, k, i = [\'a\'] * n, k - n, n - 1\n while k:\n k += 1\n if k/26 >= 1:\n res[i], k, i = \'z\', k - 26, i - 1\n else:\n res[i], k = chr(k + 96), 0\n\n return \'\'.join(res)\n```\n\n**UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.** | 66 | 2 | ['Python3'] | 7 |
smallest-string-with-a-given-numeric-value | ✅ C++ || Dry Run || Easy || Simple & Short || O(N) | c-dry-run-easy-simple-short-on-by-knockc-vmd3 | 1663. Smallest String With A Given Numeric Value\nKNOCKCAT\n\n\n1. Easy C++\n2. Line by Line Explanation with Comments.\n3. Detailed Explanation \u2705\n4. Lexi | knockcat | NORMAL | 2022-03-22T00:47:14.032205+00:00 | 2022-12-31T07:04:57.128016+00:00 | 2,027 | false | # 1663. Smallest String With A Given Numeric Value\n**KNOCKCAT**\n\n```\n1. Easy C++\n2. Line by Line Explanation with Comments.\n3. Detailed Explanation \u2705\n4. Lexicographically smallest string of length n and sum k with Dry Run.\n5. Please Upvote if it helps\u2B06\uFE0F\n```\n``` ```\n[LeetCode](http://github.com/knockcat/Leetcode) **LINK TO LEETCODE REPOSITORY**\n``` ```\n\n\nPlease upvote my comment so that i get to win the 2022 giveaway and motivate to make such discussion post.\n**Happy new Year 2023 to all of you**\n**keep solving keep improving**\nLink To comment\n[Leetcode Give away comment](https://leetcode.com/discuss/general-discussion/2946993/2022-Annual-Badge-and-the-Giveaway/1734919)\n\n**ALGORITHM**\n* **Initialise an string** of length n **with all \'a\'s**.\n* Start **traversing from the end of the string** and **add the element of the string by \'z\'** if **k >= 25. as a is already inserted with ascii 1** , adding 25 to 1 **result in 26 i.e \'z\'.**\n* At the **same time decrease the value of K** **by replaced element value i.e. for a = 1, b = 2, c = 3, \u2026, z = 26.**\n* Check for **K < 0** condition and **break the for loop.**\n* **Return the new string formed.**\n``` ```\n\n**DRY RUN**\n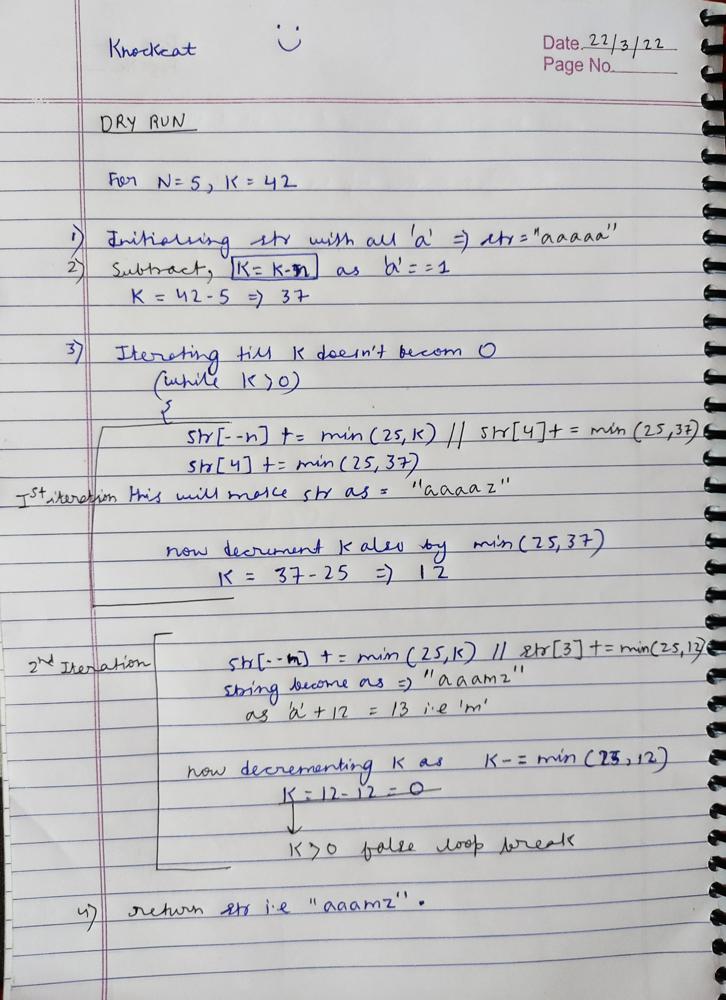\n\n``` ```\n**CODE WITH EXPLANATION**\n```\n\t\t\t\t\t// \uD83D\uDE09\uD83D\uDE09\uD83D\uDE09\uD83D\uDE09Please upvote if it helps \uD83D\uDE09\uD83D\uDE09\uD83D\uDE09\uD83D\uDE09\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n \n // initialising string of length n with all \'a\';\n string str(n,\'a\');\n \n // as all \'a\' are 1 and therefore we have subtract there sum;\n k -= n;\n \n while( k > 0)\n {\n // turning rightmost digit , \'a\' into \'z\' (\'a\' + 25, or \'a\' + k)\n // while k is positive.\n str[--n] += min(25,k);\n k -= min(25,k);\n }\n \n return str;\n }\n\t// for github repository link go to my profile.\n};\n```\n | 65 | 2 | ['String', 'C'] | 9 |
smallest-string-with-a-given-numeric-value | [Java/Python 3] Two O(n) codes w/ brief explanation and analysis. | javapython-3-two-on-codes-w-brief-explan-v67l | Mehtod 1: Greedily reversely place values\n\n1. Make sure each value of the n characters is at least 1: initialized all as \'a\';\n2. Put as more value at the | rock | NORMAL | 2020-11-22T04:25:28.789229+00:00 | 2022-03-22T12:50:20.922441+00:00 | 4,650 | false | **Mehtod 1: Greedily reversely place values**\n\n1. Make sure each value of the n characters is at least `1`: initialized all as `\'a\'`;\n2. Put as more value at the end of the String as possible.\n```java\n public String getSmallestString(int n, int k) {\n k -= n;\n char[] ans = new char[n];\n Arrays.fill(ans, \'a\');\n while (k > 0) {\n ans[--n] += Math.min(k, 25);\n k -= Math.min(k, 25);\n }\n return String.valueOf(ans);\n }\n```\n```python\n def getSmallestString(self, n: int, k: int) -> str:\n ans = [\'a\'] * n\n k -= n\n while k > 0:\n n -= 1 \n ans[n] = chr(ord(\'a\') + min(25, k))\n k -= min(25, k)\n return \'\'.join(ans)\n```\n\n**Analysis:**\nTime & space: O(n)\n\n----\n**Method 2: Math**\nCompute the total number of `z` at the end, and the number of not `a` characters right before those `z`: `r`; The remaining are `a`\'s .\n```java\n public String getSmallestString(int n, int k) {\n int z = (k - n) / 25, r = (k - n) % 25;\n return (z == n ? "" : "a".repeat(n - z - 1) + (char)(\'a\' + r)) + "z".repeat(z);\n }\n```\n```python\n def getSmallestString(self, n: int, k: int) -> str:\n z, r = divmod(k - n, 25)\n return (\'\' if z == n else \'a\' * (n - z - 1) + chr(ord(\'a\') + r)) + \'z\' * z\n```\nThe above codes can be further simplified as follows:\n\n```java\n public String getSmallestString(int n, int k) {\n int z = (k - n - 1) / 25, r = (k - n - 1) % 25;\n return "a".repeat(n - z - 1) + (char)(\'a\' + r + 1) + "z".repeat(z);\n }\n```\n```python\n def getSmallestString(self, n: int, k: int) -> str:\n z, r = divmod(k - n, 25)\n return \'a\' * (n - z - 1) + chr(ord(\'a\') + r) * (n > z) + \'z\' * z\n```\n**Analysis:**\nTime & space: O(n)\n | 65 | 1 | ['Java', 'Python3'] | 9 |
smallest-string-with-a-given-numeric-value | [Python] O(n) math solution, explained | python-on-math-solution-explained-by-dba-yiis | In this problem we need to construct lexicographically smallest string with given properties, and here we can use greedy strategy: try to take as small symbol a | dbabichev | NORMAL | 2021-01-28T08:59:35.172473+00:00 | 2021-01-28T08:59:35.172508+00:00 | 2,378 | false | In this problem we need to construct **lexicographically smallest string** with given properties, and here we can use **greedy** strategy: try to take as small symbol as possible until we can. Smallest letter means `a`, so our string in general case will look like this:\n\n`aa...aa?zz...zz`,\n\nwhere some of the groups can be empty. Now question what is the biggest numbers of `a` we can take. Denote this number by `p` and by `q` we denote value of `?` symbol. Then we have the following equation:\n`p * 1 + q + 26*(n - p - 1) = k`, which is equivalent to:\n`p = (26*n - k + q - 26)/25`.\n\nWe need to find the biggest possible value of `p`: we want as much `a` symbols at the beginning of our string as possible. Value of `q` is not equal to `26`, because then we put it to group of `z` in the end, so we can estimate `p` as:\n`p <= (26*n - k - 1)//25`. From the other side, we always can take `p = (26*n - k - 1)//25`, because we have `n - p` places left with total sum `k - p` and it is enough to show that `(n-p)*26 >= (k-p)`. One more point, given `p` can be negative, so we return `0` in this case.\n\nFinally, we can evaluate `q` as `k - 26*n + 25*p + 26` and get corresponing symbol.\n\n**Complexity**: it is still `O(n)` however, because we need to construct string in the end, what a pity. Space complexity is also `O(n)`.\n\n```\nclass Solution:\n def getSmallestString(self, n, k):\n p = max(0, (26*n - k - 1)//25)\n q = k - 26*n + 25*p + 26\n return "a"*p + chr(96 + q) + "z"*(n-p-1)\n```\n\nIf you have any questions, feel free to ask. If you like solution and explanations, please **Upvote!** | 59 | 8 | ['Greedy'] | 5 |
smallest-string-with-a-given-numeric-value | ✅ Explained with Comments | Easy | 90% | O(n) | JS | explained-with-comments-easy-90-on-js-by-uetk | \n/* \nIdea is to build the string from the end.\n1. We create an array (numericArr) to store string (1 indexed) corresponding to it\'s \nnumeric value as given | _kapi1 | NORMAL | 2022-03-22T02:09:30.664841+00:00 | 2022-03-22T02:09:30.664883+00:00 | 6,684 | false | ```\n/* \nIdea is to build the string from the end.\n1. We create an array (numericArr) to store string (1 indexed) corresponding to it\'s \nnumeric value as given i.e [undefined,a,b,c,......,z] \n\nNow we have to fill n places i.e _ _ _ _ _ _ (Think it as fill in the blanks)\n\nWe will fill this from the end. The highest value last bank can have is "z" i.e 26 numeric value\nbut we also have to fill remaining n-1 blanks and minimum value they can have is "a" i.e 1 numeric\nvalue. So it is clear the we will have to subtract these n-1 min values to fill nth blank\nso our nth value will be k-(n-1). In below code n===i\n\n2. So we calculate nth blank value i.e val=k - (n-1)\n\n3. Now if value >=26 then we fill this blank with z i.e numericArr[26] and reduce k by 26\ni.e k=k-26\n\nelse our value is <26 so we pick the string as numericArr[val] and k=k-val\n\nIn both if else case in step 3 we update our final string as str = numericArr[index] + str; (see below code)\n*/\nvar getSmallestString = function (n, k) {\n\tlet numericArr = [],\n\t\tstr = \'\';\n\t// Step 1 to build string numeric array\n\tfor (let i = 1; i <= 26; i++) {\n\t\tnumericArr[i] = String.fromCharCode(96 + i);\n\t}\n\tfor (let i = n; i > 0; i--) {\n\t\t// Step 2: Find nth blanks value\n\t\tlet val = k - (i - 1);\n\t\t// Step 3\n\t\tif (val >= 26) {\n\t\t\tstr = numericArr[26] + str;\n\t\t\tk = k - 26;\n\t\t} else {\n\t\t\tstr = numericArr[val] + str;\n\t\t\tk = k - val;\n\t\t}\n\t}\n\treturn str;\n};\ngetSmallestString(3, 27);\n``` | 26 | 1 | ['JavaScript'] | 11 |
smallest-string-with-a-given-numeric-value | C++ Simple and Short O(n) Solution, faster than 97% | c-simple-and-short-on-solution-faster-th-pu91 | At first, we will initialize the resulting string in the target size with \'a\'s.\nSo we used \'n\' out of the target \'k\' - we can reduce n from k.\nThen, we | yehudisk | NORMAL | 2021-01-28T09:05:10.113896+00:00 | 2021-01-28T15:58:12.696838+00:00 | 1,469 | false | At first, we will initialize the resulting string in the target size with \'a\'s.\nSo we used \'n\' out of the target \'k\' - we can reduce n from k.\nThen, we go from left to right and while k > 0 we turn the \'a\'s to \'z\'s, then there might be a middle character with some letter that equals to the leftover of k.\nOur string in general will look like this: a...a?z...z, where ? is any letter.\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string res(n, \'a\');\n int ptr = n-1;\n \n k -= n;\n while (k > 0) {\n res[ptr--] += min(k, 25);\n k -= min(k, 25);\n }\n \n return res;\n }\n};\n```\n**Like it? please upvote...** | 26 | 4 | ['C'] | 4 |
smallest-string-with-a-given-numeric-value | Python without any loop | python-without-any-loop-by-jaguary-wskm | \n num2 = (k-n) // 25\n if num2 == n: return \'z\' * n\n\n num1 = n - num2 - 1\n num = k - (num1 + num2 * 26)\n return \'a\' | JaguarY | NORMAL | 2020-11-22T04:11:52.431191+00:00 | 2020-11-25T08:04:15.130111+00:00 | 1,616 | false | ```\n num2 = (k-n) // 25\n if num2 == n: return \'z\' * n\n\n num1 = n - num2 - 1\n num = k - (num1 + num2 * 26)\n return \'a\' * num1 + chr(num+96) + \'z\' * num2\n```\nBasically, this result will start with a bunch of "a"(num1) and end with a bunch of "z"(num2), with at most one other letter in between(num). e.g. aaaayzzzz | 24 | 4 | [] | 7 |
smallest-string-with-a-given-numeric-value | [Python] Simple solution - Greedy - O(N) [Explanation + Code + Comment] | python-simple-solution-greedy-on-explana-qmjr | The lexicographically smallest string of length n could be \'aaa\'.. of length n\nThe constraint is that the numeric value must be k.\nTo satisfy this constrain | mihirrane | NORMAL | 2020-11-22T04:17:33.309872+00:00 | 2021-01-29T00:24:38.358491+00:00 | 1,504 | false | The lexicographically smallest string of length n could be \'aaa\'.. of length n\nThe constraint is that the numeric value must be k.\nTo satisfy this constraint, we will greedily add either \'z\' or character form of (k - value) to the string.\n\nFor eg. \nn = 3, k = 32\nInitially, ans = \'aaa\', val = 3, i =2\nSince val!=k\nans = \'aaz\', val = 28, i = 1\nSince, val!=k\nk - val = 4\nans = \'adz\', val = 0 \nSince, val == k\nbreak\n\nFinal answer = \'adz\'\n\nRunning time: O(N)\nMemory: O(1)\n\n```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n ans = [\'a\']*n # Initialize the answer to be \'aaa\'.. length n\n val = n #Value would be length as all are \'a\'\n \n for i in range(n-1, -1, -1): \n if val == k: # if value has reached k, we have created our lexicographically smallest string\n break\n val -= 1 # reduce value by one as we are removing \'a\' and replacing by a suitable character\n ans[i] = chr(96 + min(k - val, 26)) # replace with a character which is k - value or \'z\'\n val += ord(ans[i]) - 96 # add the value of newly appended character to value\n \n return \'\'.join(ans) # return the ans string in the by concatenating the list\n``` | 19 | 0 | ['Greedy', 'Python', 'Python3'] | 2 |
smallest-string-with-a-given-numeric-value | [C++, Python, Javascript] Easy Solution w/ Explanation | beats 100% / 100% | c-python-javascript-easy-solution-w-expl-74zj | (Note: This is part of a series of Leetcode solution explanations (index). If you like this solution or find it useful, please upvote this post.)\n\n---\n\nIdea | sgallivan | NORMAL | 2021-01-28T09:33:38.406672+00:00 | 2021-02-03T05:07:07.182952+00:00 | 882 | false | *(Note: This is part of a series of Leetcode solution explanations ([**index**](https://dev.to/seanpgallivan/leetcode-solutions-index-57fl)). If you like this solution or find it useful,* ***please upvote*** *this post.)*\n\n---\n\n***Idea:***\n\nThe basic idea is simple: in order for the string to be as lexigraphically "small" as it can be, you want to move as much of the "weight" towards the rear of the string as possible. \n\nThat means you want as many "**z**"s at the end of the answer and as many "**a**"s at the beginning of the answer as you can get. This also means that there will only be at most one character that is neither an "**a**" or a "**z**".\n\nFirst, remove **n** from **k** in order to leave yourself "room" to fill the remaining space with "**a**"s when you\'re done. This is as if you\'re spending **n** value of **k** to initially fill each position with an "**a**". After that, each character will be considered by how much of an increase it is over "**a**", thus a "**z**" has a value of **25**, because it\'s **25** "more" than an "**a**". That also coincidentally makes thing easier with a **0**-indexed alphabet reference string.\n\nThen start with as many "**z**"s as you can fit in **k**, and use any leftover **k** for the middle character. Then fill out the start of **ans** with "**a**"s.\n\n---\n\n***C++ Code:***\n\nThe best result for the code below is **20ms / 28.4MB** (beats 100%)\n```c++\nclass Solution {\n public:\n string getSmallestString(int n, int k) {\n k -= n;\n string alpha = "_bcdefghijklmnopqrstuvwxy_";\n string ans = std::string(~~(k / 25), \'z\');\n if (k % 25) {\n ans = alpha[k % 25] + ans;\n }\n return std::string(n - ans.size(), \'a\') + ans;\n }\n };\n```\n\n---\n\n***Python Code:***\n\nThe best result for the code below is **16ms / 13.9MB** (beats 100% / 100%)\n```python\nclass Solution(object):\n def getSmallestString(self, n, k):\n k -= n\n alpha = \'_bcdefghijklmnopqrstuvwxy_\'\n ans = \'z\' * (k // 25)\n if k % 25:\n ans = alpha[k % 25] + ans\n return \'a\' * (n - len(ans)) + ans\n```\n\n---\n\n***Javascript Code:***\n\nThe best result for the code below is **80ms / 44.1MB** (beats 100% / 100%)\n```javascript\nvar getSmallestString = function(n, k) {\n k -= n\n let alpha =\'_bcdefghijklmnopqrstuvwxy_\',\n ans = \'z\'.repeat(~~(k / 25))\n if (k % 25) ans = alpha[k % 25] + ans\n return ans.padStart(n, \'a\')\n};\n``` | 16 | 0 | ['C', 'Python', 'JavaScript'] | 3 |
smallest-string-with-a-given-numeric-value | Java Simple Solution with explanation | java-simple-solution-with-explanation-by-4eaa | Imaging an array with length n and value between 1-26 representing the character values\nFill all slots with minimumr equirement a, then we have value k-a left\ | htttth | NORMAL | 2020-11-22T04:02:26.863151+00:00 | 2020-11-22T18:26:00.795485+00:00 | 1,164 | false | Imaging an array with length n and value between 1-26 representing the character values\nFill all slots with minimumr equirement a, then we have value k-a left\nstart filling slots up to 26 (\'z\') fromt he end of the array, we can calculate with:\nnumber of \'z\' = k%25\na single character with remaining value of k%25\nnumber of \'a\' = what ever left in the front\n\nthen the final String is number of \'a\' + single character + number of \'z\'\n \n public String getSmallestString(int n, int k) {\n k = k-n;\n\n int num = k/25;\n int value = k%25;\n \n String result = "a".repeat(n-1-num);\n result += String.valueOf((char)((int)\'a\'+value));\n result += "z".repeat(num);\n\n\n return result;\n } | 14 | 5 | [] | 7 |
smallest-string-with-a-given-numeric-value | ✅[Python] Only 2 Lines Solution || O(1) || Greedy | python-only-2-lines-solution-o1-greedy-b-0rrv | \nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n z, alp = divmod(k-n-1, 25)\n return (n-z-1)*\'a\'+chr(ord(\'a\')+alp+ | subinium | NORMAL | 2022-03-22T00:47:14.219204+00:00 | 2022-03-22T00:49:50.425814+00:00 | 1,248 | false | ```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n z, alp = divmod(k-n-1, 25)\n return (n-z-1)*\'a\'+chr(ord(\'a\')+alp+1)+z*\'z\'\n``` | 12 | 0 | ['Greedy', 'Python'] | 2 |
smallest-string-with-a-given-numeric-value | [Python3] Runtime: 24 ms, faster than 99.60% | Memory: 14.8 MB, less than 95.18% | python3-runtime-24-ms-faster-than-9960-m-z0pe | \nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n diff = k - n\n q = diff // 25\n r = diff % 25\n ans = " | anubhabishere | NORMAL | 2022-03-22T05:18:17.558364+00:00 | 2022-03-22T05:18:17.558390+00:00 | 737 | false | ```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n diff = k - n\n q = diff // 25\n r = diff % 25\n ans = "a"*(n-q-1) + chr(97+r) + "z"*qt if r else "a"*(n-q)+ "z"*q\n return ans\n``` | 9 | 0 | ['Math', 'Greedy', 'Python'] | 0 |
smallest-string-with-a-given-numeric-value | Python - very easy to follow - O(N) - Char Dict | python-very-easy-to-follow-on-char-dict-6dxao | \n\ndef getSmallestString(self, n: int, k: int) -> str:\n\tc_dict = {i+1: chr(ord(\'a\') + i) for i in range(26)} # {1:\'a\', 2:\'b\' ... 26:\'z\'}\n\n\tlst = | vvvirenyu | NORMAL | 2021-01-28T09:05:03.384979+00:00 | 2021-01-28T09:12:53.323592+00:00 | 403 | false | \n```\ndef getSmallestString(self, n: int, k: int) -> str:\n\tc_dict = {i+1: chr(ord(\'a\') + i) for i in range(26)} # {1:\'a\', 2:\'b\' ... 26:\'z\'}\n\n\tlst = [\'\']*n\n \n\tfor i in range(n-1, -1, -1): # Reverse fill\n\t\tc = min(26, k-i) # if k-i > 26, character is \'z\' else c_dict[k-i]\n\t\tlst[i] = c_dict[c]\n\t\tk -= c\n\n\treturn \'\'.join(lst)\n``` | 9 | 0 | [] | 2 |
smallest-string-with-a-given-numeric-value | Easy code with comments and key idea | easy-code-with-comments-and-key-idea-by-2jxx8 | \n// Key idea is to have as many "a"s at the beginning as possible, so that the \n// string will be lexicographically smallest.\n// Similarly, maximize "z"s at | interviewrecipes | NORMAL | 2020-11-22T04:02:25.869336+00:00 | 2020-11-22T04:02:55.717266+00:00 | 712 | false | ```\n// Key idea is to have as many "a"s at the beginning as possible, so that the \n// string will be lexicographically smallest.\n// Similarly, maximize "z"s at the end so that the character will be between \n// "a"s and "z"s will be as small as possible.\n// Say, n = 6 and k is 57, then we can\'t have more than 3 "a"s because 4 x "a"s\n// would mean remaining 2 characters should add up to (57-4) which is not possible.\n// So initial 3 characters are "aaa". Now we want to make 54 from 3 characters.\n// Because we want the first character to be as small as possible, it only makes sense\n// to have as many "z"s at the end as possible.\n// So although, "aaacyz" and "aaabzz" add to 57, "aaabzz" is the answer because it is\n// smaller of the two.\n\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string answer = "";\n int a = n; // try to maximize "a"s at the beginning for \n // getting the lexicographically smallest string.\n while ((k-a) > 26*(n-a)) {\n a--;\n }\n // Now the variable `a` holds how many "a"s should be at the beginning.\n k = k - a; \n \n int z = 0; // maximize "z"s at the end.\n while (k >= (z+1)*26) {\n z++;\n }\n // Now the variable `z` holds how many "z"s should be at the end.\n \n // Construct the answer now.\n for (int i=0; i<a; i+=1) { // all "a"s at the beginning.\n answer += "a";\n }\n if (a + z < n) { // if the string can not be made of only a and z, there \n // is a need of a character in between.\n answer += (\'a\'+k-26*z-1);\n }\n for (int i=0; i<z; i+=1) { // all "z"s at the end.\n answer += "z";\n }\n return answer;\n }\n};\n``` | 9 | 2 | [] | 2 |
smallest-string-with-a-given-numeric-value | Simple java solution with explaination| o(n) time,o(n) space | simple-java-solution-with-explaination-o-mcy2 | we want String of length n and lexographic shortest string as possible.\n2. so take an character array of size n and initialize every index value with \'a\'. an | kushguptacse | NORMAL | 2021-01-29T06:33:04.067322+00:00 | 2022-03-22T06:51:55.405084+00:00 | 613 | false | 1. we want String of length n and lexographic shortest string as possible.\n2. so take an character array of size n and initialize every index value with \'a\'. and decrement k.\n3. by doing above step if k reaches 0 it means it is the desired answer. why? becuase all a together are enough to make k sum. \n4. run repeat below steps till k>0. (k>0 indicates we need to increase some characters values from \'a\' to any value till \'z\').\n5. since we want lexographic min String we will try to increase character from backward directions. and try to satisfy sum=k or in our case k=0.\n6. check Min(25,k) as it is max by which we can increase. i.e. \'a\'+25 = 26 and if k is already less then 25 it means we can just increase a by k and we will have desired sum.\n7. now decrement k with Min(25,k). and repeat the process.\n8. once k reaches 0 we have reached desired sum.\n\ntime complexity is o(n)\n```\nclass Solution {\n public String getSmallestString(int n, int k) {\n char[] ch = new char[n];\n for(int i=0;i<n;i++) {\n ch[i]=\'a\';\n k--;\n }\n int currChar=0;\n while(k>0) {\n currChar=Math.min(25,k);\n ch[--n]+=currChar;\n k-=currChar;\n }\n return String.valueOf(ch);\n }\n}\n``` | 8 | 1 | ['Greedy', 'Java'] | 1 |
smallest-string-with-a-given-numeric-value | simple java solution | simple-java-solution-by-manishkumarsah-3rq7 | \nclass Solution {\n public String getSmallestString(int n, int k) {\n char[] res = new char[n];\n Arrays.fill(res,\'a\'); // filling the whole | manishkumarsah | NORMAL | 2021-01-28T08:43:57.422720+00:00 | 2021-01-28T08:44:47.349626+00:00 | 335 | false | ```\nclass Solution {\n public String getSmallestString(int n, int k) {\n char[] res = new char[n];\n Arrays.fill(res,\'a\'); // filling the whole array with starting alphabet a\n \n // now update the k as we have fill the array with character a\n k = k-n;\n \n while(k>0)\n {\n res[n-1] += Math.min(25, k); // like this-- a+25 = z\n n--;\n k = k- Math.min(25,k);\n }\n \n return String.valueOf(res);\n }\n}\n``` | 7 | 0 | [] | 0 |
smallest-string-with-a-given-numeric-value | C++ O(N) Time Greedy | c-on-time-greedy-by-lzl124631x-0874 | See my latest update in repo LeetCode\n\n## Solution 1. Greedy\n\nIntuition: We can do it greedily:\n\n If picking a won\'t result in unsolvable problem, we pre | lzl124631x | NORMAL | 2020-11-22T04:03:04.578325+00:00 | 2020-11-22T07:00:15.137565+00:00 | 602 | false | See my latest update in repo [LeetCode](https://github.com/lzl124631x/LeetCode)\n\n## Solution 1. Greedy\n\n**Intuition**: We can do it greedily:\n\n* If picking `a` won\'t result in unsolvable problem, we prepend `a` to the start of the string.\n* Otherwise, if picking `z` won\'t result in unsolvable problem, we append `z` to the end of the string.\n* Otherwise, if there is still a non-zero `k` value left (which must be smaller than 26), we add `\'a\' + k - 1` in the middle.\n\n**Algorithm**:\n\nHow to check if it will become unsolvable problem?\n\nIf after picking `\'a\'`, the remainder numeric value `k - 1` can\'t be formed even if using all `\'z\'`s, i.e. `k - 1 >= (n - 1) * 26`, then it\'s unsolvable.\n\nSo if `n - 1 > 0 && k - 1 < (n - 1) * 26`, we should keep prepending `\'a\'`.\n\nIf after picking `\'z\'`, the remainder numeric value `k - 26` can\'t be formed even if using all `\'a\'`s, i.e. `k - 26 < n - 1`, then it\'s unsolvable.\n\nSo if `n - 1 > 0 && k - 26 >= n - 1`, we should keep appending `\'z\'`.\n\n\n```cpp\n// OJ: https://leetcode.com/contest/weekly-contest-216/problems/smallest-string-with-a-given-numeric-value/\n// Author: github.com/lzl124631x\n// Time: O(N)\n// Space: O(1)\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string s;\n int a = 0, z = 0;\n while (n - 1 > 0 && k - 1 < (n - 1) * 26) ++a, --k, --n;\n while (n - 1 > 0 && k - 26 >= n - 1) ++z, k -= 26, --n;\n while (a-- > 0) s += \'a\';\n if (k) s += \'a\' + k - 1;\n while (z-- > 0) s += \'z\';\n return s;\n }\n};\n```\n\n## Solution 2.\n\nStarts with all `\'a\'`, fill the `k` value from the end of the array backwards.\n\n```cpp\n// OJ: https://leetcode.com/problems/smallest-string-with-a-given-numeric-value/\n// Author: github.com/lzl124631x\n// Time: O(N)\n// Space: O(1)\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string s(n, \'a\');\n k -= n;\n for (int i = n - 1; k > 0; --i) {\n int d = min(k, 25);\n s[i] += d;\n k -= d;\n }\n return s;\n }\n};\n``` | 7 | 1 | [] | 0 |
smallest-string-with-a-given-numeric-value | java || Simple solution || O(n) | java-simple-solution-on-by-funingteng-cca1 | Assume we have n length of array with all \'a\', e.g. [\'a\', \'a\', \'a\'] for n = 3, k = 27. The total remaing is 27 - 3 = 24 now. Now we just need to allocat | funingteng | NORMAL | 2022-03-24T18:45:25.700945+00:00 | 2022-03-28T19:44:01.140198+00:00 | 419 | false | Assume we have n length of array with all \'a\', e.g. [\'a\', \'a\', \'a\'] for n = 3, k = 27. The total remaing is 27 - 3 = 24 now. Now we just need to allocate the remaining numbers from right to left. Then iterate the array in reverse order, if larger than 25 (26 - 1), we should allocate \'z\', otherwise we should allocate the remaining. The rest part should all be \'a\'s.\n```\nclass Solution {\n public String getSmallestString(int n, int k) {\n char[] list = new char[n];\n k -= n;\n for (int i = n - 1; i >= 0 ; i--) {\n if (k > 25) {\n k -= 25;\n list[i] = \'z\';\n } else {\n list[i] = (char)(97 + k);\n k = 0;\n }\n }\n return String.valueOf(list);\n }\n}\n``` | 6 | 0 | ['Java'] | 0 |
smallest-string-with-a-given-numeric-value | Java Code | java-code-by-rizon__kumar-iezr | \nclass Solution {\n public String getSmallestString(int n, int k) {\n char[] c = new char[n];\n \n for(int i = n-1; i>=0; i--){\n | rizon__kumar | NORMAL | 2022-03-22T02:50:56.101607+00:00 | 2022-03-22T02:50:56.101638+00:00 | 174 | false | ```\nclass Solution {\n public String getSmallestString(int n, int k) {\n char[] c = new char[n];\n \n for(int i = n-1; i>=0; i--){\n int val = Math.min(26,k - i);\n c[i] = (char)(\'a\'+val-1); //using (char) to convert ASCII to respective character\n k=k-val;\n }\n return new String(c);\n }\n}\n``` | 6 | 0 | ['Greedy'] | 1 |
smallest-string-with-a-given-numeric-value | [Java] Intuitive solution. | java-intuitive-solution-by-sadriddin17-dkjj | The idea is to initialize char array with all value with \'a\' then to reach k we\'ll increase array elements to max from the end to the beginning.\n\n\t\tchar | sadriddin17 | NORMAL | 2022-03-22T01:42:14.977519+00:00 | 2022-03-22T01:43:29.058685+00:00 | 756 | false | The idea is to initialize char array with all value with \'a\' then to reach k we\'ll increase array elements to max from the end to the beginning.\n```\n\t\tchar[] result = new char[n];\n for (int i = 0; i < n; i++) {\n result[i] = \'a\';//initialize all array element with \'a\'\n k--;\n }\n int numValue;\n while (k > 0) {\n numValue = Math.min(25, k);//building the string from the end to the beginning, it will always be optimal.\n result[--n] += numValue;\n k -= numValue;\n }\n return String.valueOf(result);\n``` | 6 | 0 | ['Java'] | 2 |
smallest-string-with-a-given-numeric-value | Correct simple Python solution without loop | correct-simple-python-solution-without-l-fjgg | \nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n q, r = divmod(k - n, 25)\n return (\'a\'*(n - q - 1) + chr(ord(\'a\') | dpustovarov | NORMAL | 2020-11-23T15:58:22.025745+00:00 | 2020-11-23T16:23:25.074807+00:00 | 441 | false | ```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n q, r = divmod(k - n, 25)\n return (\'a\'*(n - q - 1) + chr(ord(\'a\') + r) if q < n else \'\') + \'z\'*q\n```\n\n* the smallest string is a string like aaa...aszzz...z\n-- a - smallest char (0 to n length)\n-- s - char on the border (0 or 1 length)\n-- z - largest char (0 to n length)\n\n\n\nP.S. The solution correctly handles edge cases n=3, k=78. | 6 | 0 | ['Math', 'Python'] | 0 |
smallest-string-with-a-given-numeric-value | c++ || very simple solution || O(N) | c-very-simple-solution-on-by-shubhangnau-v3qy | PLEASE UPVOTE IF IT HELPS YOU\n\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n { \n string arr = ""; \n \n for(int i = | shubhangnautiyal | NORMAL | 2022-03-22T04:57:21.304686+00:00 | 2022-03-22T04:57:21.304726+00:00 | 420 | false | **PLEASE UPVOTE IF IT HELPS YOU**\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n { \n string arr = ""; \n \n for(int i = 0; i < n; i++) \n arr += \'a\'; \n for (int i = n - 1; i >= 0; i--) \n { \n k -= i; \n if (k >= 0) \n { \n if (k >= 26) \n { \n arr[i] = \'z\'; \n k -= 26; \n } \n else\n { \n char c= (char)(k + 97 - 1); \n arr[i] = c; \n k -= arr[i] - \'a\' + 1; \n } \n } \n \n else\n break; \n \n k += i; \n } \n return arr; \n }\n \n }\n};\n``` | 5 | 0 | ['String', 'C'] | 1 |
smallest-string-with-a-given-numeric-value | ✔️ C++ concise solution | Explanation | minimum time and space complexity | c-concise-solution-explanation-minimum-t-gcd5 | Intuition:\nAs we have to create a lexicographically smallest string with a length of n. We have to place as many as the largest number back of the output strin | foysol_ahmed | NORMAL | 2022-03-22T04:47:06.673943+00:00 | 2022-03-22T16:26:36.190312+00:00 | 252 | false | **Intuition:**\nAs we have to create ***a lexicographically smallest string with a length of n***. We have to place as many as the largest number back of the output string. To be more precise, for every iteration, our target is to place ***(i-1) a*** at the beginning, and for the last index, we just put the minimum number which remains from k.\n\n***Note:** During iteration, for any index, if we got the k is greater than 26, we just return 26 as we have only 26 characters possible. \n\n**Accepted Code:**\n```\nstring getSmallestString(int n, int k) {\n\n\tstring res(n, \' \');\n\n\tfor(int i=n; i>0; i--)\n\t{\n\t\tint charIdx = min(26, (k-i+1));\n\t\tres[i-1] = \'a\' + (charIdx - 1);\n\t\tk -= charIdx;\n\t}\n\n\treturn res;\n}\n```\n\n**Complexity:**\nTime Complexity: O(n)\nSpace Complexity: O(1) without considering the output string\n\n\n\n\n\n | 5 | 0 | ['Greedy'] | 1 |
smallest-string-with-a-given-numeric-value | Python. 3 lines. faster than 100.00%. cool & clear solution. | python-3-lines-faster-than-10000-cool-cl-kjzn | \tclass Solution:\n\t\tdef getSmallestString(self, n: int, k: int) -> str:\n\t\t\tz = (k - n) // 25\n\t\t\tunique = chr(k - z * 25 - n + 97) if n - z else ""\n\ | m-d-f | NORMAL | 2021-01-28T12:14:12.458078+00:00 | 2021-01-28T12:15:25.030201+00:00 | 472 | false | \tclass Solution:\n\t\tdef getSmallestString(self, n: int, k: int) -> str:\n\t\t\tz = (k - n) // 25\n\t\t\tunique = chr(k - z * 25 - n + 97) if n - z else ""\n\t\t\treturn "a"*(n-z-1) + unique + "z"*z | 5 | 1 | ['Python', 'Python3'] | 0 |
smallest-string-with-a-given-numeric-value | Python Math | python-math-by-twerpapple-0vhk | \n"""\nfind the greatest x such that \n 1*x + 26*(n-x) >= k\n 26n - 25x >= k\n 26n - k >= 25x\n (26n-k)/25 >= x\n \n x = floor((26n-k)/25)\n | twerpapple | NORMAL | 2020-11-29T17:38:53.097236+00:00 | 2020-11-29T17:39:28.743732+00:00 | 136 | false | ```\n"""\nfind the greatest x such that \n 1*x + 26*(n-x) >= k\n 26n - 25x >= k\n 26n - k >= 25x\n (26n-k)/25 >= x\n \n x = floor((26n-k)/25)\n \n numeric_val = 1*x + 26*(n-x)\n string = [1]*x + [26-(numeric_val-k)] + [26]*(n-x-1)\n"""\n\n\nclass Solution:\n def getSmallestString(self, n, k):\n """\n Args:\n n (int): length of string\n k (int): numeric value of string (sum of value of char)\n \n Returns:\n res (string): smalleest string\n """\n num_a = floor((26*n-k)/25)\n numeric_val = 1*num_a + 26*(n-num_a)\n mid = chr(ord(\'a\')+26-(numeric_val-k)-1) # careful for 1-index\n res = \'a\'*num_a + mid*1 + \'z\'*(n-num_a-1)\n return res\n``` | 5 | 0 | [] | 0 |
smallest-string-with-a-given-numeric-value | Simple Basic C++ Soln. Only Loop | simple-basic-c-soln-only-loop-by-vardaan-pd93 | Intuition\nWe need the smallest string therefore I followed these simple steps to get my ans\n1--> add \'a\' till possible \n2--> add a char \n3--> now add \'z\ | vardaanpahwa02 | NORMAL | 2023-10-27T13:00:18.711045+00:00 | 2023-10-27T13:00:18.711070+00:00 | 342 | false | # Intuition\nWe need the smallest string therefore I followed these simple steps to get my ans\n1--> add \'a\' till possible \n2--> add a char \n3--> now add \'z\'\n\n# Approach\nNow the question arrises add \'a\' but till when . \nwe will use a number for example let n=3 and k=30.\n1--> add \'a\' then n=2 and k=29\n2--> add \'a\' again then n=1 and k=28 which is not possible bcoz last char is \'z\' with number 26.\n2--> we need to add 29-26=3 this is \'c\'\n3--> now add \'z\' \n\nWhich implies you can add \'a\' till your k is less than equal to(n-1)*26 .\nadd \'a\' till \n\n k <= (n-1)*26\n k-(n-1)*26 <= 0\n\nnow if it is not possible then add char with value\n\n k-(n-2)*26 \n\nbcoz you would need to n-2 \'z\' after this \n\nn is continuosly changing is this\n\nDo a dry Run for k=30,n=3 and any k=27,n=3 to understand it better.\n# Complexity\n- Time complexity:\nO(n) : where n is charater is output string\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string ans;\n while(n!=0){\n int ch = k-(n-1)*26;\n if(ch<=0){\n ans.push_back(\'a\');\n k--;\n n--;\n }\n else{\n ans.push_back(ch+\'a\'-1);\n n--;\n k -= (ch);\n }\n }\n return ans;\n }\n};\n``` | 4 | 0 | ['C++'] | 2 |
smallest-string-with-a-given-numeric-value | Javascript | Recursive | javascript-recursive-by-vihangpatel-h9ty | Here we try to find out how many \'z\'s can be placed at the end of the desired output \n Let\'s say n = 3, k = 3; n <= k <= 78 -> All three places are required | vihangpatel | NORMAL | 2022-03-23T05:44:29.775750+00:00 | 2022-03-23T05:44:29.775778+00:00 | 178 | false | Here we try to find out how many \'z\'s can be placed at the end of the desired output \n Let\'s say n = 3, k = 3; n <= k <= 78 -> All three places are required to be filled\n\n 3 -> aaa\n 4 -> aab\n .\n .\n 27 -> aay\n 28 -> aaz\n 29 -> abz\n 30 -> acz\n \nSo, with n = 3, k = 3, lexicographically we can have aaa -> aaz strings.\nIn each iteration, we will try to find out a single character with its frequency which can be placed \nat the end of the string. \n \n\tFor e.g n = 5, k = 82, output => "aczzz"\n\tIn first iteration, we will try to find out a char starting from z, then try out => y, x, w, .... c, b, a\n\tThen subtract z * 2 => 26 * 2 => 52.\n\tnewK = k - z * n(z) = 82 - 52 => 30\n\tnewN = k - n(z) = 5 - 2 = 3\nSo, if we trace it, then calls will be like \n \n \n getSmallestString(5, 82)\n /\\\n / \\\n / \\\n / \\\n / \\\n / \\\n getSmallestString(3, 30) "zz"\n /\\\n / \\\n / \\\n / \\\n / \\\n / \\ \n getSmallestString(2, 4) "z"\n / \\\n / \\\n / \\\n / \\\n / \\ \n getSmallestString(1, 1) "c" \n / \\\n / \\\n / \\\n / \\\n getSmallestString(0, 0) "a"\n |\n |\n |\n \u25BC\n ""\n \n \n Some, other examples: \n \n\tn = 5, k = 5 => aaaaa\n n = 5, k = 29 => aaaay\n n = 5, k = 30 => aaaaz\n n = 5, k = 31 => aaabz\n n = 5, k = 55 => aaazz\n n = 5, k = 56 => aabzz\n \nLet\'s trace n = 5, k = 56:\t\n \n getSmallestString(5, 56)\n /\\\n / \\\n / \\\n / \\\n / \\\n / \\\n getSmallestString(4, 3) "zz"\n /\\\n / \\\n / \\\n / \\\n / \\\n / \\ \n getSmallestString(2, 2) "b"\n / \\\n / \\\n / \\\n / \\\n / \\ \n getSmallestString(1, 1) "a" \n / \\\n / \\\n / \\\n / \\\n getSmallestString(0, 0) "a"\n |\n |\n |\n \u25BC\n ""\n\n\n```\n/**\n * @param {number} n\n * @param {number} k\n * @return {string}\n */\n\nconst TO_ADD = 26 - 1;\n\nvar getSmallestString = function(n, k) { \n if (n <= 0 || k <= 0) {\n return \'\';\n }\n \n /* Refer comment#1 for this logic */\n let highestCharFreq = Math.floor(k / (TO_ADD + n));\n let i = 0; \n \n while(highestCharFreq === 0 && i <= TO_ADD) {\n i++;\n highestCharFreq = Math.floor(k / (TO_ADD + n - i)) \n }\n \n const highestCharCode = TO_ADD - i;\n const highestChar = String.fromCharCode(97 + highestCharCode);\n const newK = k - highestCharFreq * (highestCharCode + 1);\n const newN = n - highestCharFreq;\n \n \n \n const output = `${getSmallestString(newN, newK)}${highestChar.repeat(highestCharFreq)}`; \n return output;\n};\n```\n\n | 4 | 0 | ['JavaScript'] | 0 |
smallest-string-with-a-given-numeric-value | Easy Concise C++ O(n) Solution(Faster than 99%) | easy-concise-c-on-solutionfaster-than-99-ooht | class Solution {\npublic:\n string getSmallestString(int n, int k) {\n int curr=1;\n string ans=""; \n for(int i=1;i<=n;i++){\n if( | kartiksaxenaabcd | NORMAL | 2022-03-22T11:30:28.243614+00:00 | 2022-03-22T11:34:12.129254+00:00 | 142 | false | class Solution {\npublic:\n string getSmallestString(int n, int k) {\n int curr=1;\n string ans=""; \n for(int i=1;i<=n;i++){\n if((k-curr)< 26*(n-i)){\n ans+=char(\'a\'+curr-1);\n k-=curr;\n }\n else{\n while((k-curr)>26*(n-i))\n curr++;\n ans+=char(\'a\'+curr-1);\n k-=curr;\n }}\n return ans;\n }}; | 4 | 0 | ['Greedy', 'C'] | 0 |
smallest-string-with-a-given-numeric-value | Backward-filling with an early exit [bad code!] | backward-filling-with-an-early-exit-bad-s1iya | There is one optimization in the code below that is helpful for large n: As soon as k == n, we know that the rest of the sought string is \'a\' (s. && k > n in | anikit | NORMAL | 2022-03-22T10:24:23.935345+00:00 | 2023-01-03T23:08:09.311835+00:00 | 101 | false | There is one optimization in the code below that is helpful for large `n`: As soon as `k == n`, we know that the rest of the sought string is `\'a\'` (s. `&& k > n` in the `while` condition).\n\nWhile this code works, there are a few bad coding practices here which should be avoided:\n1. Re-use of variables: the arguments `n` and `k` are used and modified directly. It would be better to have local variables with speaking names instead.\n2. `--` is used inline twice. Yes, it makes the code more concise, but also less readable.\n3. No comments whatsoever. If you implement an algorithm, its non-trivial parts should be commented.\n\nAnd now the code:\n```csharp\npublic string GetSmallestString(int n, int k)\n{ \n var res = new char[n--];\n Array.Fill(res, \'a\');\n \n while (n >= 0 && k > n)\n {\n int c = Math.Min(26, k - n);\n k -= c;\n res[n--] = (char)(c + \'a\' - 1);\n }\n \n return new String(res);\n}\n``` | 4 | 0 | ['C#'] | 0 |
smallest-string-with-a-given-numeric-value | C++ two approaches || commented | c-two-approaches-commented-by-suryapsg-wxh4 | Approach 1:\n\nstring getSmallestString(int n, int k) {\n\tstring res;\n\tint c;\n\tfor(int i=0;i<n;i++){\n\t\t//start with \'a\'\n\t\tc=1;\n\t\t//If current in | SuryaPSG | NORMAL | 2022-03-22T03:06:46.622178+00:00 | 2022-03-22T04:16:55.727661+00:00 | 305 | false | **Approach 1:**\n```\nstring getSmallestString(int n, int k) {\n\tstring res;\n\tint c;\n\tfor(int i=0;i<n;i++){\n\t\t//start with \'a\'\n\t\tc=1;\n\t\t//If current index holds \'a\' then remaining n-i-1 length should be enough to hold atleast \'z\' in every index\n\t\t//if it is not enough we make the current char b,c,d till the above condition satisfies\n\t\twhile((n-i-1)*26<(k-c)) c++;\n\t\tres.push_back(\'a\'+c-1);\n\t\tk=k-c;\n\t}\n\treturn res;\n}\n```\n\n**Approach 2 (EFFICIENT):**\n```\nstring getSmallestString(int n, int k) {\n\t//start with all a\'s\n\tstring res(n,\'a\');\n\t//reduce k by n as we used 1(a) for every index\n\tk=k-n;\n\t//lexicographically smaller string ===> insert the highest possible char at the end \n\twhile(k>0){\n\t\t//find the min(k,25) to find the lexicographically large characters possible based on k\n\t\t//if k>25 we make the char z and reduce the k by 25\n\t\tres[--n]+=min(k,25);\n\t\tk=k-min(k,25);\n\t}\n\treturn res;\n}\n```\n\n**Please Upvote : )** | 4 | 0 | ['C'] | 1 |
smallest-string-with-a-given-numeric-value | [Simple and readable] - O(N) TypeScript/JavaScript | simple-and-readable-on-typescriptjavascr-aq9w | \nfunction getSmallestString(n: number, k: number): string {\n const alphabet = [\'\',\'a\',\'b\',\'c\',\'d\',\'e\',\'f\',\'g\',\'h\',\'i\',\'j\',\'k\',\'l\' | dustinkiselbach | NORMAL | 2021-01-28T15:33:47.613795+00:00 | 2021-01-28T15:33:47.613836+00:00 | 275 | false | ```\nfunction getSmallestString(n: number, k: number): string {\n const alphabet = [\'\',\'a\',\'b\',\'c\',\'d\',\'e\',\'f\',\'g\',\'h\',\'i\',\'j\',\'k\',\'l\',\'m\',\'n\',\'o\',\'p\',\'q\',\'r\',\'s\',\'t\',\'u\',\'v\',\'w\',\'x\',\'y\',\'z\']\n let sum = 0\n let s = \'\'\n \n for (let i = n - 1; i >= 0; i--) {\n let target = (k - i) - sum\n if (target > 26) target = 26\n s = alphabet[target] + s\n sum += target\n }\n\n return s\n};\n``` | 4 | 0 | ['TypeScript', 'JavaScript'] | 1 |
smallest-string-with-a-given-numeric-value | Smallest String With A Given Numeric Value: Python, 3 LoC | smallest-string-with-a-given-numeric-val-3qmq | python\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n if k == 26*n: return \'z\' * n\n nz, nshift = divmod(k - n, 25) | sjoin | NORMAL | 2021-01-28T15:18:45.019804+00:00 | 2021-01-28T15:18:45.019838+00:00 | 185 | false | ```python\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n if k == 26*n: return \'z\' * n\n nz, nshift = divmod(k - n, 25)\n return \'a\' * (n - nz - 1) + chr(ord(\'a\') + nshift) + \'z\' * nz\n```\n\n---\nIf you find this helpful, please **upvote**! Thank you! :-) | 4 | 0 | ['Math', 'Python'] | 0 |
smallest-string-with-a-given-numeric-value | O(n) Better Solution Greedy [Cpp] | on-better-solution-greedy-cpp-by-anil111-54jb | \nclass Solution {\npublic:\n string getSmallestString(int n, int k) \n {\n string str(n,\'a\');\n k=k-n;\n int ri=n-1;\n whil | anil111 | NORMAL | 2021-01-28T08:55:35.295039+00:00 | 2021-01-28T09:05:27.751520+00:00 | 276 | false | ```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) \n {\n string str(n,\'a\');\n k=k-n;\n int ri=n-1;\n while(k>0)\n {\n str[ri]=str[ri]+min(25,k);\n ri--;\n k=k-25;\n }\n return str;\n }\n};\n``` | 4 | 0 | ['Greedy', 'C++'] | 0 |
smallest-string-with-a-given-numeric-value | Python - !beautiful && !concise && !short | python-beautiful-concise-short-by-kafola-h41x | Start with all "a" then from the end bump it up as much as posible (25 max to turn "a" into "z")\n\n\ndef getSmallestString(self, n: int, k: int) -> str:\n\t\ta | kafola | NORMAL | 2020-11-22T04:04:18.287214+00:00 | 2020-11-22T04:04:18.287246+00:00 | 313 | false | Start with all "a" then from the end bump it up as much as posible (25 max to turn "a" into "z")\n\n```\ndef getSmallestString(self, n: int, k: int) -> str:\n\t\tarr = [97] * n\n k -= n\n \n i = n - 1\n while k > 0 and i > -1:\n arr[i] += min(k, 25)\n k -= min(k, 25)\n i -= 1\n \n return "".join([chr(i) for i in arr])\n``` | 4 | 0 | [] | 2 |
smallest-string-with-a-given-numeric-value | C++ Easy Code || Fully Explained in Simple Steps || Clean and Clear code + Explanation | c-easy-code-fully-explained-in-simple-st-ej36 | \nThe most clear explanation of the algorithm is as:\n\nStep - 1 Initialize a string s with n number of \'a\' to make it as a smallest possible lexiographic.\n | dee_stroyer | NORMAL | 2022-03-23T19:34:14.637065+00:00 | 2022-03-23T19:34:35.067496+00:00 | 140 | false | \n**The most clear explanation of the algorithm is as:**\n\n*Step - 1 Initialize a string s with n number of \'a\' to make it as a smallest possible lexiographic.\nStep - 2 Count of \'a = 1\' , so if i have n number of \'a\' it means it sum is also \'n\'.\nStep - 3 Reduce the value of k by n (i.e k=k-n);\nStep - 4 Start traversing the string from the end because if we append any character from starting then there is chances of not getting smallest possible lexiographic order. \n\t\t Ex if we have to append \'z\' then from starting it would be like zaaa and from end it would be like aaaz which clearly shows that aaaz<zaaa.\nStep - 5 If \'k\' becomes \'0\' stop the traversal because that would be our answer.\nStep - 6 Else if k < 25, it means for getting the sum just replace i th character by \'a\'+k. And update k by k=0.\nStep - 7 Else k=> 25 so iterate more and replace i th character by (\'a\'+25) and move ahead. And update k by k-25*\n\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string ans;\n ans.insert(ans.begin(),n, \'a\');\n k-=n;\n \n for(int i = n-1; i>=0; i--){\n if(k>=25){\n ans[i]+=25;\n k-=25;\n }\n \n else{\n ans[i]+=k;\n k = 0;\n }\n \n if(k == 0){\n break;\n }\n }\n \n return ans;\n }\n};\n``` | 3 | 0 | ['String', 'Greedy', 'C', 'C++'] | 0 |
smallest-string-with-a-given-numeric-value | 1663 | C++ | Easy O(N) solution with explanation | 1663-c-easy-on-solution-with-explanation-9s7f | Please upvote if you like this solution :)\n\nApproach:\n For getting smallest lexicographical order of string, Initially we define n size string and put \'a\'s | Yash2arma | NORMAL | 2022-03-22T18:33:21.921805+00:00 | 2022-03-22T18:33:21.921875+00:00 | 72 | false | **Please upvote if you like this solution :)**\n\n**Approach:**\n* For getting smallest lexicographical order of string, Initially we define n size string and put \'a\'s into it.\n* Since string only contains \'a\'s we subtract n from k i.e. k=k-n;\n* Now, we traverse from the end of the string because we want smallest lexicographical order and that is only possible when we put large value characters at the end of the string.\n* We define 3 conditions for putting character in string these are....\ni) If k==0 break iteration.\nii) if k<25 then replace s[i] with (char)(\'a\'+k) and update k by k=0.\niii) and if k>25 then replace s[i] with (char)(\'a\'+25) and update k by k-25.\n* At the end return string\n\n**Time Complexity: O(N), Space Complexity: O(1)**\n\n**Code:**\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) \n {\n string s = "";\n \n //we put \'a\' in the nth size string to get smallest lexicographical order\n for(int i=0; i<n; i++)\n s += \'a\';\n \n k = k - n; //since we put \'a\'s in the string of size n\n \n //replace \'a\' from the end\n for(int i=n-1; i>=0; i--)\n {\n if(k==0) break;\n \n else if(k<25)\n {\n s[i] = (char) (\'a\'+k); //use (char) for getting character of ASCII code\n k=0;\n }\n else\n {\n s[i] = (char) (\'a\'+25);\n k = k-25;\n }\n }\n return s;\n \n }\n};\n```\n**Please upvote if you like this solution :)** | 3 | 0 | ['Greedy', 'C'] | 0 |
smallest-string-with-a-given-numeric-value | Simple java solution | simple-java-solution-by-siddhant_1602-4rwo | class Solution {\n\n public String getSmallestString(int n, int k) {\n char c[]=new char[n];\n Arrays.fill(c,\'a\');\n int i=n-1;\n | Siddhant_1602 | NORMAL | 2022-03-22T17:49:02.895517+00:00 | 2022-03-22T17:49:02.895562+00:00 | 203 | false | class Solution {\n\n public String getSmallestString(int n, int k) {\n char c[]=new char[n];\n Arrays.fill(c,\'a\');\n int i=n-1;\n k-=n;\n while(k>0)\n {\n if(k>25)\n {\n c[i--]=\'z\';\n k-=25;\n }\n else\n {\n c[i--]=(char)(\'a\'+k);\n k=0;\n }\n }\n return String.valueOf(c);\n }\n} | 3 | 0 | ['Java'] | 0 |
smallest-string-with-a-given-numeric-value | O(n) C++ solution with explanation and dry run | on-c-solution-with-explanation-and-dry-r-g7gd | We have to find the smallest lexiographical string with size of n and numeric value of k.\n\nFor a string to be lexiographically small, it\'s beginning charact | shivansh961 | NORMAL | 2022-03-22T13:23:20.176319+00:00 | 2022-03-22T13:52:57.518385+00:00 | 135 | false | We have to find the smallest lexiographical string with size of n and numeric value of k.\n\n**For a string to be lexiographically small, it\'s beginning characters must be smallest.**\n\nWe initialise a string as **ans** with size n, having each character as **\'a\'**. \n\nLet\'s take an example for the better understanding:\n\nn=5, k(numeric value)=73\n\n**ans = "aaaaa", k=68**, this is the result after initialization. Now if the value of k would have been 0 that means we already got our answer. There are no invalid test cases(n=3, k=2) here, so we do not need to worry about the cases resulting in our code failure.\n\n**"aaaaa" is the smallest string of size 5 that exists.**\n\nWe will update the value of ans in reverse order, because the beginning elements must be smallest to get the smallest lexiographical string.\n\nIf k>25, that means we can place \'z\' here because the numeric value required is must greater, and we update the k as k-25, we keep on doing it until it reaches the condition of k<25.\nThe reason we are subtracting 25 not 26 is because we already considered the numeric value of a in one of the initial steps where we wrote n=n-k.\n\nelse(k<=25), that means this is the last update required\n\n\n\nLet me dry run it for the better understanding.\n\n\n**After Initial Steps(For n=5, k=73)**\nans = "aaaaa", k=68\n\n**Iteration of loop**\n**i=4**\nBefore Update : ans="aaaaa", k=68\nAfter Update : ans="aaaaz", k=43\n\n**i=3**\nBefore Update : ans="aaaaz", k=43\nAfter Update : ans="aaazz", k=18\n\n**i=2**\nBefore Update : ans="aaazz", k=18\nAfter Update : ans="aarzz", k=0, **break;**\n\n**Note**\n\nFor those who might have trouble understanding the meaning of **char ch = char(96+k+1)**\nAscii value of a lowercase letter starts with 97, if k=18 like in above dry run for i=2\n\n\'a\' was already the initial value, therefore we have added 96 not 97.\nk is added because it is the numeric value(meaning, we are calcualting the the no. of letters ahead of \'a\').\n1 is added because it is 1-indexed, meaning, the numeric value of a was 1 not 0.\n\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string ans = "";\n \n for(int i=0 ; i<n ; i++)\n ans += \'a\';\n \n k=k-n;\n \n if(k==0)\n return ans;\n \n for(int i=n-1 ; i>=0 ; i--){\n if(k>25){\n k=k-25;\n ans[i]=\'z\';\n }\n else{\n char ch = char(96+k+1);\n ans[i] = ch;\n break;\n }\n }\n \n return ans;\n }\n};\n``` | 3 | 0 | [] | 2 |
smallest-string-with-a-given-numeric-value | ✅ Python Solution | python-solution-by-dhananjay79-qk56 | \nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n ans = \'\'\n while (n - 1) * 26 >= k:\n ans += \'a\'\n | dhananjay79 | NORMAL | 2022-03-22T12:40:26.217732+00:00 | 2022-03-22T12:40:26.217764+00:00 | 309 | false | ```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n ans = \'\'\n while (n - 1) * 26 >= k:\n ans += \'a\'\n n -= 1; k -= 1\n ans += chr(ord(\'a\') + (k % 26 or 26) - 1)\n ans += \'z\' * (n - 1)\n return ans\n``` | 3 | 0 | ['Python', 'Python3'] | 0 |
smallest-string-with-a-given-numeric-value | Simple Java Code | simple-java-code-by-sai_prashant-z8ia | Idea is simple, we will make the string from end greedily i.e, putting the lexographically biggest possible character. Here is my code \n\n\nclass Solution {\n | sai_prashant | NORMAL | 2022-03-22T06:04:36.355636+00:00 | 2022-03-22T06:04:36.355687+00:00 | 169 | false | Idea is simple, we will make the string from end greedily i.e, putting the lexographically biggest possible character. Here is my code \n\n```\nclass Solution {\n public String getSmallestString(int n, int k) {\n StringBuilder sb=new StringBuilder("");\n for(int i=0;i<n;i++){\n int val=k-(n-i-1);\n if(val>26)val=26;\n k=k-val;\n sb.append((char)(96+val));\n }\n return sb.reverse().toString();\n }\n}\n``` | 3 | 0 | ['Greedy'] | 0 |
smallest-string-with-a-given-numeric-value | 1. Failed DP, 2. Greedy | 1-failed-dp-2-greedy-by-akshay3213-5qsb | \n\n\n# Using dynamic programming - Gave me memory limit exceeding\n# \n\n\nclass Solution {\n\t//O(n * k)\n public String getSmallestString(int n, int k) {\ | akshay3213 | NORMAL | 2022-03-22T03:53:58.020877+00:00 | 2022-03-22T03:59:52.624913+00:00 | 144 | false | 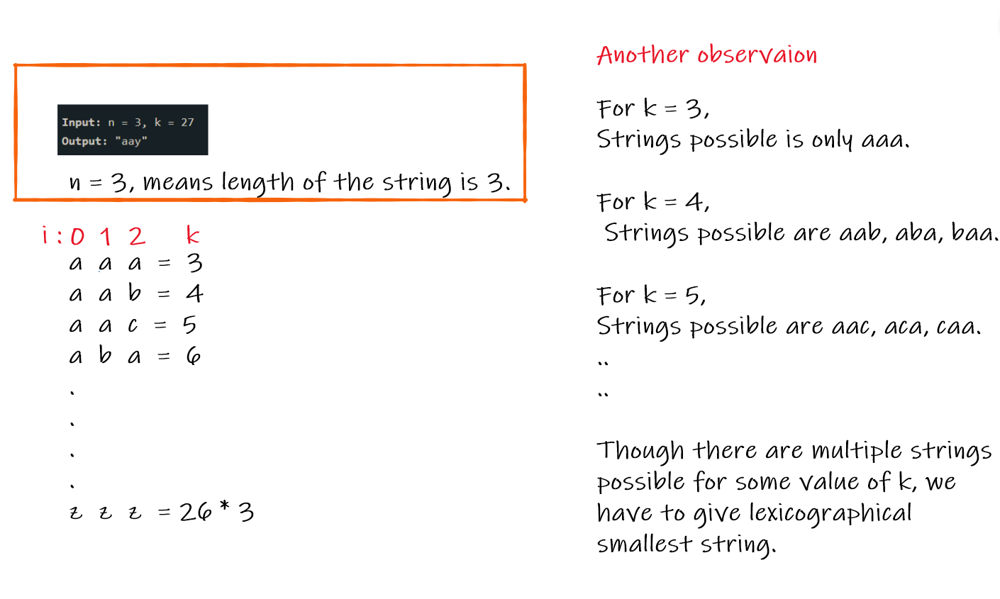\n\n\n# Using dynamic programming - Gave me memory limit exceeding\n# 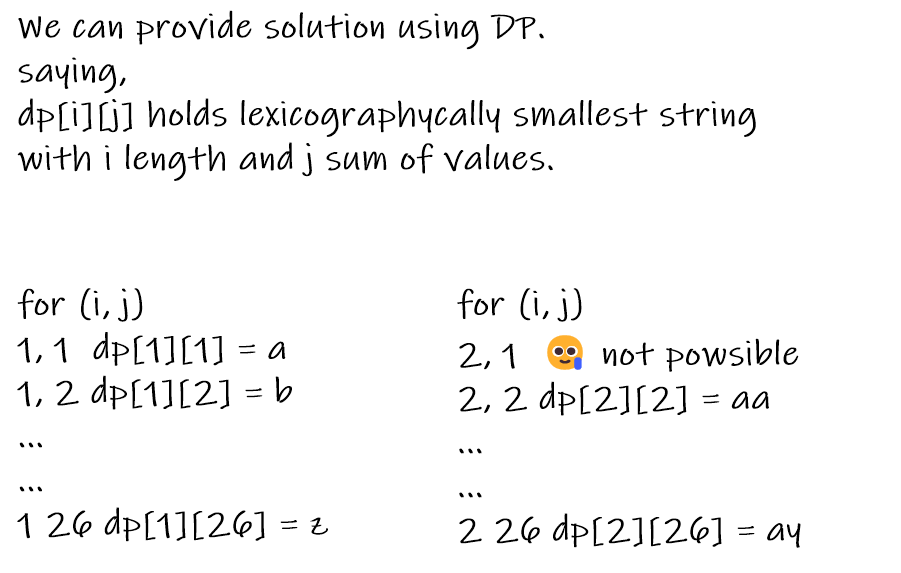\n\n```\nclass Solution {\n\t//O(n * k)\n public String getSmallestString(int n, int k) {\n String dp[][] = new String[n + 1][k + 1];\n \n return smallestString(n, k, dp);\n }\n \n\t//O(l * sum)\n String smallestString(int l, int sum, String dp[][]) {\n if (l == 0 && sum == 0) {\n return "";\n }\n \n if (l == 0 || sum < 0) {\n return "-1";\n }\n \n if (dp[l][sum] != null) {\n return dp[l][sum];\n }\n \n\t\t//O(26)\n for (int i = 0; i < 26; i++) {\n String str = smallestString(l - 1, sum - i - 1, dp);\n if (str.equals("-1")) {\n continue;\n }\n \n return dp[l][sum] = (char) (\'a\' + i) +str;\n }\n \n return dp[l][sum] = "-1";\n }\n}\n```\n\n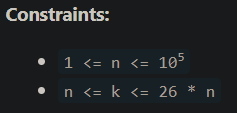\n\nConstraints are higher hence gives MLE.\n\n# Using greedy approach.\n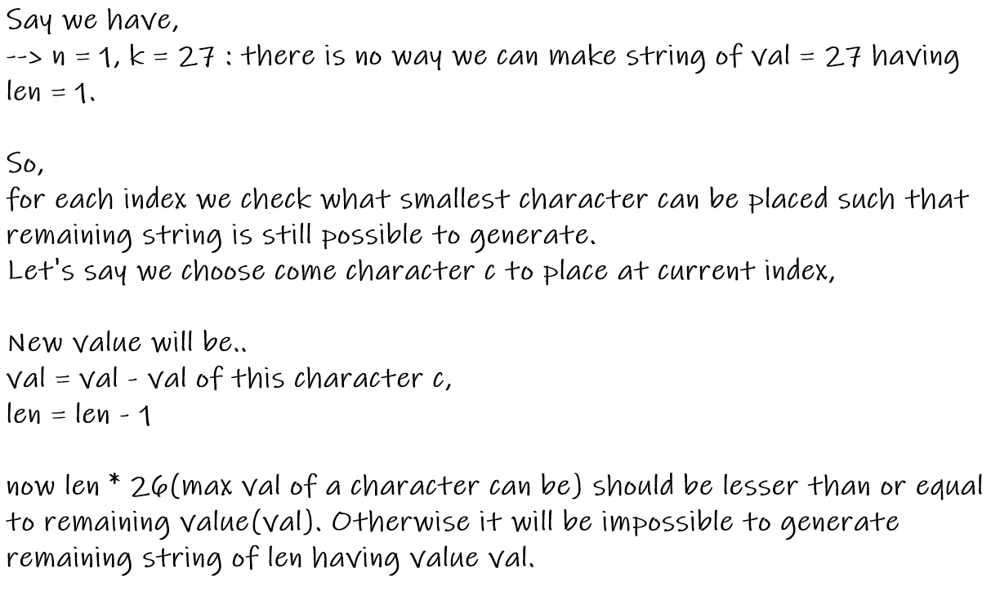\n```\nclass Solution {\n public String getSmallestString(int n, int k) {\n \n StringBuilder sb = new StringBuilder();\n \n\t\t//O(N)\n while (n > 0 && k > 0) {\n int ind = 0;\n //find appropriate character - O(26)\n while ((n - 1) * 26 < k - ind -1 && ind < 26) {\n ind++;\n }\n \n sb.append((char) (\'a\' + ind));//append it to string\n k -= (ind + 1); // update k\n n--; // update length\n }\n \n return sb.toString();\n }\n}\n```\n\nTC : O(N)\nSC : O(N)\n\n\n\n\n | 3 | 0 | ['Dynamic Programming', 'Greedy'] | 0 |
smallest-string-with-a-given-numeric-value | [Python3] O(n) time and O(1) space complexity solution | python3-on-time-and-o1-space-complexity-cuqu1 | python3\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n result =[0]*n\n for position in range(n-1,-1,-1):\n | bhushandhumal | NORMAL | 2022-03-22T03:20:49.899761+00:00 | 2022-03-22T04:12:13.413894+00:00 | 360 | false | ``` python3\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n result =[0]*n\n for position in range(n-1,-1,-1):\n add = min(k -position,26)\n result[position] = chr(ord("a")+add -1)\n k-=add\n \n return "".join(result)\n```\n\nComplexity :\n\nTime : O(n), as we iterate over n positions to build the result.\n\nSpace : O(1), as we use constant extra space to store add and position variables. | 3 | 0 | ['Python', 'Python3'] | 1 |
smallest-string-with-a-given-numeric-value | python 3 || O(n) | python-3-on-by-derek-y-3xzb | ```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n res = \'\'\n for i in range(n):\n q, r = divmod(k, 26)\ | derek-y | NORMAL | 2022-03-22T01:36:15.876719+00:00 | 2022-03-24T03:38:39.913835+00:00 | 194 | false | ```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n res = \'\'\n for i in range(n):\n q, r = divmod(k, 26)\n if r == 0:\n q -= 1\n r = 26\n\n if n - q - i >= 2:\n res += \'a\'\n k -= 1\n else:\n res += chr(r + 96) + \'z\' * (n - i - 1)\n break\n \n return res | 3 | 0 | ['Greedy', 'Python', 'Python3'] | 0 |
smallest-string-with-a-given-numeric-value | C++ || Easy Explanation || With Comments || TC - O(N) | c-easy-explanation-with-comments-tc-on-b-hml3 | \n\n1. Create a string of size n with all \'a\'s in it\n2. Start from end of the created string \n3. Create a variable \'remaining\' and put \'z\' at index i if | krishna__1902 | NORMAL | 2022-03-22T01:12:48.084374+00:00 | 2022-03-22T01:16:06.898575+00:00 | 59 | false | \n\n**1. Create a string of size n with all \'a\'s in it\n2. Start from end of the created string \n3. Create a variable \'remaining\' and put \'z\' at index i if remaining>25 (i.e, \'a\'+25 , as a is already there so \'a\'+25 = \'z\'\n4. If remaining becomes less than or equal to 25, put \'a\' + remaining value at that index and break the loop\n5. Return the modified string...**\n\n\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n \n string s = "";\n \n //Creating string of size n with only \'a\' in it\n for(int i=0;i<n;i++)\n s +=\'a\';\n \n //Putting i at end index of string "s"\n int i(n-1);\n int remaining(k-n);\n while(remaining>0)\n {\n if(remaining<=25)\n {\n s[i]=\'a\'+ remaining;\n break;\n }\n else\n {\n s[i]=\'a\'+25;\n remaining -=25;\n i--;\n }\n }\n return s;\n }\n};\n``` | 3 | 0 | ['Greedy', 'C'] | 0 |
smallest-string-with-a-given-numeric-value | [Python] O(1) with explanation | python-o1-with-explanation-by-shoomoon-dudy | Accoding to the defination, the smallest lexicographically string must have the patten as \n\n\t\ts = \'a\' * p + t * q + \'z\' * r\n\t\twhere p, r >= 0, \'a\' | shoomoon | NORMAL | 2021-02-25T19:59:43.811115+00:00 | 2021-02-25T21:45:42.951225+00:00 | 139 | false | Accoding to the defination, the smallest lexicographically string must have the patten as \n\n\t\ts = \'a\' * p + t * q + \'z\' * r\n\t\twhere p, r >= 0, \'a\' < t < \'z\', q = 0 or 1\n\nThus, we have:\n```\n1. k = 1 * p + val_t * q + 26 * r\n2. p + q + r = n\n```\n\nThen k = (n - q - r) + val_t * q + 26 * r = n + q * (val_t - 1) + r * 25.\nLet tt = val_t - 1, then 0 < tt < 25, hence:\n\n\t\tk - n = r * 25 + q * tt, where 0 <= q * tt < 25\n\t\t\t\t\nso we have:\n\n\t\tr, q * tt = divmod(k - n, 25)\n\t\tq = 1 if q * tt > 0 else 0\n\t\ttt = q * tt\n\t\tp = n - r - q\n\nThen the answer will be \n\n\t\ts = \'a\' * p + t * q + \'z\' * r\n\n```\nclass Solution(object):\n def getSmallestString(self, n, k):\n """\n :type n: int\n :type k: int\n :rtype: str\n """\n r, qt = divmod(k - n, 25)\n q = 1 if qt else 0\n p = n - q - r\n return \'a\' * p + chr(ord(\'a\') + qt) * q + \'z\' * r\n```\n\n | 3 | 0 | [] | 0 |
smallest-string-with-a-given-numeric-value | C# - O(N) - Fill array from end | c-on-fill-array-from-end-by-christris-wob7 | csharp\npublic string GetSmallestString(int n, int rem)\n{\n\t char[] result = new char[n];\n\t Array.Fill(result, \'a\');\n\n\t // We have already filled array | christris | NORMAL | 2021-01-29T02:15:54.808001+00:00 | 2021-01-29T02:17:22.806458+00:00 | 95 | false | ```csharp\npublic string GetSmallestString(int n, int rem)\n{\n\t char[] result = new char[n];\n\t Array.Fill(result, \'a\');\n\n\t // We have already filled array with \'a\' with value 1 for each char\n\t rem -= n;\n\n\t for(int i = n - 1; i >= 0; i--)\n\t {\n\t\t int diff = Math.Min(rem, 25);\n\t\t result[i] = (char)(\'a\' + diff);\n\t\t rem -= diff;\n\t }\n\n\t return new string(result);\n}\n``` | 3 | 0 | [] | 0 |
smallest-string-with-a-given-numeric-value | [C++] Greedy Approach | c-greedy-approach-by-codedayday-p178 | Approach 1: Greedy Solution\nIdea:\nGenerate the initial string with all \'a\' characters. This will reduce k by n.\nThen, turn rightmost \'a\' into \'z\' (\'a\ | codedayday | NORMAL | 2021-01-28T15:12:32.183431+00:00 | 2021-01-28T15:12:32.183471+00:00 | 141 | false | Approach 1: Greedy Solution\nIdea:\nGenerate the initial string with all \'a\' characters. This will reduce k by n.\nThen, turn rightmost \'a\' into \'z\' (\'a\' + 25, or \'a\' + k) while k is positive.\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string ans(n, \'a\');\n k -= n;\n while(k>0){\n ans[--n] += min(25, k);\n k -= min(25, k);\n }\n return ans;\n }\n};\n```\n\nReference:\n[1] https://leetcode.com/problems/smallest-string-with-a-given-numeric-value/discuss/944538/C%2B%2B-Reverse-Fill | 3 | 0 | [] | 1 |
smallest-string-with-a-given-numeric-value | [C++] Single-pass Solution Explained, ~100% Time, ~98% Space | c-single-pass-solution-explained-100-tim-iumc | Alright: while this problem might tempt you to actually compute all/some permutations, the size of the expected string is such that it should definitely make us | ajna | NORMAL | 2021-01-28T14:15:36.723067+00:00 | 2021-01-28T14:15:36.723093+00:00 | 201 | false | Alright: while this problem might tempt you to actually compute all/some permutations, the size of the expected string is such that it should definitely make us very wary about how to proceed in terms of performance.\n\nBut we can go through an actually smarter way, once we figure out that you average result will have a series of initial `\'a\'`s, a central character of some sort and the rest followed by `\'z\'`s, since that is how you get the least lexicographic order out of the given requirements.\n\nTo do so, we will start with 2 support variables:\n* `res`, already initialised to be of the right size in order to avoid painful and expensive reallocation as it would otherwise grow;\n* `i`, our pointer, initially set to address the last cell of `res`.\n\nWe will then start from the right (much more convenient to think that way, IMHO, but feel free to prove me wrong with better ideas!) and we will assign as many characters as we can to be `\'z\'`s, and at the same time reducing `k` by the same amount (`\'z\' == 26`, for this problem), stopping only when we do not have enough "score" to put any more.\n\nTime then to figure out the central character, if any: this will be possible only when `k > i` (since `i` represents how many characters we have left, with `k == i`, it would mean that we can just assign `\'a\'` to all of them) and we will proceed in a similar fashion:\n* we will reduce `k` by the appropriate value (`i + 1`);\n* assign the result of it to `res[i--]`, incremented by `\'a\'` to make it work.\n\nFinally, one last loop that we know will end up just blindly assigning `\'a\'`s to the remaining `i` characters, since we know for sure now that `k == i`.\n\nOnce done, we can return `res` :)\n\nThe code:\n\n```cpp\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n // support variables\n string res(n, \'*\');\n int i = n - 1;\n // getting rid of the largest "load"\n while (k - 26 > i) {\n k -= 26;\n res[i--] = \'z\';\n }\n // possible central character\n if (i < k) res[i--] = \'a\' + (k -= i + 1);\n // \'a\'s to the end of the world and beyond!\n while (i > -1) res[i--] = \'a\';\n return res;\n }\n};\n``` | 3 | 0 | ['C', 'Combinatorics', 'Probability and Statistics', 'C++'] | 0 |
smallest-string-with-a-given-numeric-value | Rust 4ms solution | rust-4ms-solution-by-sugyan-xdfc | \nimpl Solution {\n pub fn get_smallest_string(n: i32, k: i32) -> String {\n let mut v = vec![0; n as usize];\n let mut k = k - n;\n for | sugyan | NORMAL | 2021-01-28T08:53:01.060448+00:00 | 2021-01-28T08:53:01.060498+00:00 | 78 | false | ```\nimpl Solution {\n pub fn get_smallest_string(n: i32, k: i32) -> String {\n let mut v = vec![0; n as usize];\n let mut k = k - n;\n for e in v.iter_mut().rev() {\n let m = std::cmp::min(25, k);\n *e = m as u8 + b\'a\';\n k -= m;\n }\n String::from_utf8(v).unwrap()\n }\n}\n``` | 3 | 0 | ['Rust'] | 1 |
smallest-string-with-a-given-numeric-value | [Java] O(logN) Solution, Divide And Conquer, 3ms Faster than 100% | java-ologn-solution-divide-and-conquer-3-3f95 | Use divide and conquer when adding \'a\' and \'z\'.\n\nclass Solution {\n public String getSmallestString(int n, int k) {\n int subK = k - n; \n | applechen | NORMAL | 2020-11-22T06:44:16.119975+00:00 | 2020-11-22T06:44:16.120009+00:00 | 326 | false | Use divide and conquer when adding \'a\' and \'z\'.\n```\nclass Solution {\n public String getSmallestString(int n, int k) {\n int subK = k - n; \n int numZ = subK / 25;\n char mid = (char)(\'a\' + subK % 25);\n int numA = n - numZ - 1;\n \n StringBuilder res = new StringBuilder();\n res.append(repChar(\'a\', numA));\n res.append(mid);\n res.append(repChar(\'z\', numZ));\n return res.toString();\n }\n \n public String repChar(char c, int reps) {\n StringBuilder adder = new StringBuilder(Character.toString(c));\n StringBuilder result = new StringBuilder();\n while (reps > 0) {\n if (reps % 2 == 1) {\n result.append(adder);\n }\n adder.append(adder);\n reps /= 2;\n } \n return result.toString();\n }\n}\n```\n\nI will add more explanation later...(Maybe) | 3 | 1 | ['Divide and Conquer', 'Java'] | 4 |
smallest-string-with-a-given-numeric-value | Python 3 | 5-line Greedy O(N) | Explanation | python-3-5-line-greedy-on-explanation-by-n6we | Understand the question\n- Question is asking for smallest string, that being said, \n\t- we want to have as most a on the left side as possible\n\t- If using a | idontknoooo | NORMAL | 2020-11-22T05:29:53.794647+00:00 | 2020-11-22T07:27:44.812235+00:00 | 227 | false | ### Understand the question\n- Question is asking for *smallest string*, that being said, \n\t- we want to have as most *a* on the left side as possible\n\t- If using *a* doesn\'t match given number, we can try some letter with larger number \n\t- *z* will be the last letter we want to use, so we want to have them all the way to the right\n- The more *z* we use on the right, the less *a* we need on the left\n- For example, `n=2, k=27`\n\t- best case (smallest) `az` = 1 + 26\n\t- `za` is worst, since we put *z* on the left\n\t- `aa` is smaller than `az` but it\'s not a valid solution\n\t- `by` is a valid solution, but it\'s larger than `az` since we didn\'t start from *a*\n### Explanation\n- So the idea is\n\t- First, make sure string have enough length `n` by initializing string with all letters as *a* of length `n`\n\t- Adjust the string so that it can fully cover the given number `k`\n\t\t- We do this by using *z* from the right side\n\t- Ultimately, there **might** have some left over that using *z* will cause a overflow (`k` becomes negative)\n\t\t- then we use corresponding letter for it\n\t- for example, `n=3, k=29`\n\t\t- start with `aaa`, now `k` is 26\n\t\t- start putting `z` from the right, we can get `aaz`, now `k` is 1\n\t\t- since `z` will overflow 1, we use `b` instead, to **just** cover k\n\t\t- final result will be `abz`\n- `ans`: final answer in list format; `z` number of *z* I need, `nz` the letter **just** to cover the left over\n### Implementation\n```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n ans = [\'a\'] * n\n k, i = k-n, n-1\n z, nz = divmod(k, 25) # `z`: number of *z* I need, `nz`: ascii of the letter just to cover the leftover\n ans[n-1-z] = chr(nz + ord(\'a\')) # adjust the left over `k` using mod\n return \'\'.join(ans[:n-z]) + \'z\' * z # make final string & append `z` to the end\n``` | 3 | 0 | ['Greedy', 'Python', 'Python3'] | 2 |
smallest-string-with-a-given-numeric-value | [C++] Simple O(N) Solution | Brute Force Solution | 2 Solutions | c-simple-on-solution-brute-force-solutio-fv09 | \nclass Solution\n{\npublic:\n // Best Solution\n string getSmallestString(int n, int k)\n {\n string res(n, \'a\');\n k -= n;\n i | ravireddy07 | NORMAL | 2020-11-22T04:51:07.569238+00:00 | 2020-11-22T04:52:08.277399+00:00 | 58 | false | ```\nclass Solution\n{\npublic:\n // Best Solution\n string getSmallestString(int n, int k)\n {\n string res(n, \'a\');\n k -= n;\n int midChar = 0;\n while (k > 0)\n {\n midChar = min(k, 25);\n k -= midChar;\n res[n - 1] += midChar;\n n--;\n }\n return res;\n }\n};\n```\n\n\n```\nclass Solution\n{\npublic:\n // Brute Force\n string getSmallestString(int n, int k)\n {\n char al[] = {\'\\n\', \'a\', \'b\', \'c\', \'d\', \'e\', \'f\', \'g\', \'h\', \'i\', \'j\', \'k\', \'l\', \'m\', \'n\', \'o\', \'p\', \'q\', \'r\', \'s\', \'t\', \'u\', \'v\', \'w\', \'x\', \'y\', \'z\'};\n string res = "";\n int mid = 0;\n do\n {\n if (k > 26)\n {\n if (k - 26 >= n - 1)\n {\n res += al[26];\n k -= 26;\n }\n else\n {\n mid = k - n + 1;\n res += al[mid];\n k -= mid;\n }\n }\n else if (k != n)\n {\n mid = k - n + 1;\n res += al[mid];\n k -= mid;\n }\n else if (k == n)\n {\n res += al[1];\n k -= 1;\n }\n n--;\n } while (n > 0);\n reverse(res.begin(), res.end());\n return res;\n }\n};\n``` | 3 | 1 | [] | 0 |
smallest-string-with-a-given-numeric-value | Python simple O(N) math solution with comments | python-simple-on-math-solution-with-comm-wiwp | k = 73, n = 5\n526 -73 = 57\n\nz z z z z\n26 26 26 26 26\n| | | | |\n25 + 25 + 7 + 0 + 0 | SonicLLM | NORMAL | 2020-11-22T04:48:35.332032+00:00 | 2020-11-22T21:33:10.247673+00:00 | 286 | false | k = 73, n = 5\n5*26 -73 = 57\n```\nz z z z z\n26 26 26 26 26\n| | | | |\n25 + 25 + 7 + 0 + 0 = 57\n| | | | |\n1 1 19 0 0 \na a s z z\n```\n\n57 = 2 * 25 + 7 * 1\ndiv = 2, remain = 1\n=> "a"*div + "s" * remain = "a"*2 + "s" * 1\nsuffix = k - (div+remain) = 5 - (2+1) =2\n => "a"*div + "s" * remain + "z" *suffix = "a"*2 + "s" * 1 + "z" *2 = "aaszz"\n\n\n```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n\t\t # 97:a, 122:z\n sub = n*26 - k\n div, remain = divmod(sub, 25)\n if remain:\n suffix = n - (div+1) if n - (div+1) else 0\n else:\n suffix = n - div if n - div else 0\n return "a"*div + (chr(122-remain) if remain else "") + "z"*suffix\n``` | 3 | 0 | ['Math', 'Python'] | 3 |
smallest-string-with-a-given-numeric-value | [c++] easy with example | c-easy-with-example-by-sanjeev1709912-1f8s | My idea behind this code to take character gridily \nmeans first i try to pick 26 if possible \n\nNow the question is how to check if possible \nSo here is answ | sanjeev1709912 | NORMAL | 2020-11-22T04:32:17.625153+00:00 | 2020-11-22T05:08:24.565124+00:00 | 122 | false | My idea behind this code to take character gridily \nmeans first i try to pick 26 if possible \n\nNow the question is how to check if possible \nSo here is answer like if we pick 26 and the remaining value is geater then required number of element then it safe to pick 26 thats it\n\nok now see example \n```\nlet think n=5 and k=73 and e=0 (e repesent number of element currently picked)\npick 26 means \'z\' condition 73-26>n-e-1 // n=5 and we pick 26 out of 76 and its safe\n\n73-26=47\nnow again pick 26 condition 47-26>n-e-1 //n=5 and e=1 as we pick z priviously\n\n47-26=21 \nnow we are not able to pick 26,25,24,22,21 and same way the consicutive value \nbut we can pick 19 ans 21-19 =2 and after that we can pick two consicutive \'a\'\n\nso at last reverse your answer as we have to find minimum\n```\n\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n int a=26;\n int e=0;\n string ans="";\n for(int i=0;i<n;)\n {\n if(k-a>=n-e-1)\n {\n k-=a;\n ans+=(\'a\'+(a-1));\n i++;\n e++;\n }\n else\n {\n a-=1;\n }\n }\n reverse(ans.begin(),ans.end());\n return ans;\n }\n};\n```\n\n**please up vote if you have query comment down bellow** | 3 | 0 | [] | 0 |
smallest-string-with-a-given-numeric-value | [Ruby] 3 lines. Intuitive + math solution with system of linear equations | ruby-3-lines-intuitive-math-solution-wit-pv0t | Algorithm\n\nSearch an answer in a form aa...aa[LETTER]zz...zz\nNumeric Value of that string is a number of a chars * 1 + number of z chars * 26 + value of LETT | shhavel | NORMAL | 2020-11-22T04:17:54.246832+00:00 | 2021-01-28T08:51:33.962698+00:00 | 203 | false | # Algorithm\n\nSearch an answer in a form `aa...aa[LETTER]zz...zz`\nNumeric Value of that string is a number of `a` chars * 1 + number of `z` chars * 26 + value of `LETTER`.\n\nMin value of a string of len `n` is `n` for string `aa...aaa`.\nAfter decreasing needed sum (value of string result) by n count of `z` is `s / 25` (how many times 25 points fits in remaining value).\n\n```ruby\nCHARS = (1..26).zip(\'a\'..\'z\').to_h\ndef get_smallest_string(n, s) # k is a sum\n s -= n\n z, r = s.divmod(25) # s = 25*z + r\n return \'z\'*z if z == n\n \'a\'*(n-z-1) + CHARS[r+1] + \'z\'*z\nend\n```\n\n# Explicit math way to obtain the same solution\nSearch an answer in a form `aa...aa[LETTER]zz...zz` . `LETTER` is a character in a range `a..z`.\nNumeric Value of that string is a number of `a` chars * 1 + number of `z` chars * 26 + value of `LETTER`.\nLet `a` is a number of letters `a`, `z` is a number of letters `z`.\nCompose a system of linear equations:\n\n**\u23B0 a + z = n - 1** *(1)* , where `a >= 0`, `z >= 0`\n**\u23B1 a + l + 26z = s** *(2)*, where`1 \u2264 l \u2264 26`.\n\nfrom *(1)*: **a = n - z - 1**, where `z <= n - 1`\nthen put this into *(2)*: **n-z-1+l+26z = s** *=>* **l-1+25z = s-n** *=>* **l-1 = (s-n)%25**, **z = (s-n)/25** (unless z == n)\n\n```\ndef get_smallest_string(n, s)\n z, r = (s-n).divmod(25)\n return \'z\'*z if z == n\n \'a\'*(n-z-1) + (97+r).chr + \'z\'*z\nend\n``` | 3 | 0 | ['Math', 'Ruby'] | 0 |
smallest-string-with-a-given-numeric-value | BEATS 99% || EASY with Eg|| In Depth Commented CODE. | beats-99-easy-with-eg-in-depth-commented-5oc4 | This provided code defines a function getSmallestString(n, k) that generates a lexicographically smallest string with the following properties:\n\n- Length: The | Abhishekkant135 | NORMAL | 2024-06-26T10:22:19.698413+00:00 | 2024-06-26T10:22:19.698440+00:00 | 218 | false | This provided code defines a function `getSmallestString(n, k)` that generates a lexicographically smallest string with the following properties:\n\n- **Length:** The string has a length of `n` characters.\n- **Maximum Zs:** The string can contain at most `k` characters \'z\'.\n\n**Functionality Breakdown:**\n\n1. **Character Array:**\n - `char[] ans = new char[n];`: This line creates a character array named `ans` of size `n`. This array will hold the characters of the resulting string.\n\n2. **Distributing Zs:**\n - `k -= n;`: This line subtracts `n` from `k`. The intuition behind this is that initially, we can consider all characters in the string as \'a\'. Since each \'a\' has a value of 1 (in the lexicographic order), subtracting `n` from `k` effectively represents the remaining value (number of \'z\'s) we can distribute in the string.\n\n3. **Filling the String (Loop):**\n - `for (int i = n - 1; i >= 0; i--) { ... }`: This loop iterates from the end of the `ans` array (`n - 1`) down to the beginning (`i >= 0`). Here\'s what happens inside the loop:\n - `if (k >= 25) { ... }`: This condition checks if the remaining value `k` is greater than or equal to 25 (the value of \'z\' in the lexicographic order).\n - If true:\n - `ans[i] = \'z\';`: A \'z\' is assigned to the current character (`ans[i]`) in the array.\n - `k -= 25;`: The value `k` is decremented by 25, accounting for the added \'z\'.\n - `else { ... }`: If the condition is false (meaning `k` is less than 25):\n - `ans[i] = (char) (\'a\' + k);`: This line calculates the character to be assigned based on the remaining value `k`. It adds `k` to the ASCII value of \'a\' (97) and casts the result to a character. This ensures we assign characters from \'a\' to \'z\' (based on their order) depending on the remaining value.\n - `k = 0;`: Since all the remaining value (`k`) has been used to assign a character, we set `k` to 0.\n\n4. **Returning the Result:**\n - `return new String(ans);`: This line creates a new String object from the `ans` character array and returns it.\n\n**Output:**\n\nThis function generates the lexicographically smallest string possible considering the constraints of length `n` and maximum `k` \'z\' characters.\n\n**Example:**\n\nFor `n = 3` and `k = 2`, the code would return "aa`". Here\'s the explanation:\n\n- Initially, `k` is 2 (maximum \'z\'s).\n- Since `k` is less than 25, the loop assigns characters from \'a\' to \'z\'.\n- In the first two iterations (`i = 1` and `i = 0`), `k` is sufficient to assign \'a\' (remaining value 2 is enough for two \'a\'s).\n- In the third iteration (`i = 2`), `k` becomes 0, and the loop terminates.\n- The resulting string is "aa`".\n\n**Key Points:**\n\n- The code prioritizes using \'a\' as much as possible since it has the lowest lexicographic value.\n- It strategically distributes \'z\'s only when the remaining value (`k`) allows it.\n- This approach ensures the generated string is lexicographically the smallest possible.\n\n# Code\n```\nclass Solution {\n public String getSmallestString(int n, int k) {\n // Initialize an array to hold the characters of the resulting string\n char[] ans = new char[n];\n \n // Adjust k to account for the minimum possible sum of n \'a\'s (i.e., n)\n k -= n;\n \n // Fill the array from the end to the beginning\n for (int i = n - 1; i >= 0; i--) {\n // Determine the value to place at the current position\n if (k >= 25) {\n // Place \'z\' at the current position if k allows\n ans[i] = \'z\';\n k -= 25;\n } else {\n // Place the appropriate character to match the remaining k\n ans[i] = (char) (\'a\' + k);\n k = 0;\n }\n }\n \n // Convert the character array to a string and return it\n return new String(ans);\n }\n}\n\n``` | 2 | 0 | ['String', 'Greedy', 'Java'] | 0 |
smallest-string-with-a-given-numeric-value | c++ | easy | fast | c-easy-fast-by-venomhighs7-stso | \n\n# Code\n\n//from hi-malik\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string res(n,\'a\');\n k -= n;\n \ | venomhighs7 | NORMAL | 2022-11-22T12:17:02.074263+00:00 | 2022-11-22T12:17:02.074298+00:00 | 224 | false | \n\n# Code\n```\n//from hi-malik\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string res(n,\'a\');\n k -= n;\n \n while(k > 0){\n res[--n] += min(25, k);\n k -= min(25, k);\n }\n return res;\n }\n};\n``` | 2 | 0 | ['C++'] | 1 |
smallest-string-with-a-given-numeric-value | Easy to understand with comments | easy-to-understand-with-comments-by-shas-76en | we start from the back , keep adding the highest letter possible to the result , highest possible letter is k-(remaining spaces)(remaining as we subtract the va | shashank_c10 | NORMAL | 2022-03-23T17:47:24.950988+00:00 | 2022-03-23T17:47:24.951048+00:00 | 200 | false | we start from the back , keep adding the highest letter possible to the result , highest possible letter is k-(remaining spaces)(remaining as we subtract the value of k by the value of each letter added, the remaining spaces are n - current length of result)\n```\ndef getSmallestString(self, n: int, k: int) -> str:\n l=[]\n count=0\n for i in range(n):\n # check if we can put \'z\' \n if k-26>((n-2)-i): \n l.append(chr(26+96))\n k=k-26\n # if we cant we put highest possible letter and put a\'s after it\n else:\n l.append(chr(k-(n-1-i)+96))\n break\n while len(l)!=n:\n l.append(chr(97))\n return ("".join(l))[::-1]\n\'\'\' | 2 | 0 | ['Greedy', 'Python', 'Python3'] | 0 |
smallest-string-with-a-given-numeric-value | ✅C++ || TC - O(N), SC- O(1) || 🗓️ Daily LeetCoding Challenge || March || Day 22 | c-tc-on-sc-o1-daily-leetcoding-challenge-0sja | Approach: \n1) Since we want to make a string lexicographically smaller so we will make a string of size n with \'a\'.\n2) Start from the right to left characte | shm_47 | NORMAL | 2022-03-22T18:08:49.871209+00:00 | 2022-03-22T18:19:37.371832+00:00 | 38 | false | **Approach:** \n1) Since we want to make a string lexicographically smaller so we will make a string of size n with \'a\'.\n2) Start from the right to left character, increase the char value by min(25, k) until k will reach to 0.\n\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string res(n, \'a\'); \n k -= n; //a= 1, substract all n* a value\n \n int i = res.size()-1;\n while(k>0){\n int temp = min(25, k); //1(a)+25 = z\n res[i] += temp; //a = 1+25 = 26 = z\n k -= temp;\n i--;\n }\n \n return res;\n }\n};class Solution {\npublic:\n string getSmallestString(int n, int k) {\n string res(n, \'a\'); \n k -= n; //a= 1, substract all n* a value\n \n int i = res.size()-1;\n while(k>0){\n int temp = min(25, k); //1(a)+25 = z\n res[i] += temp; //a = 1+25 = 26 = z\n k -= temp;\n i--;\n }\n \n return res;\n }\n};\n``` | 2 | 0 | [] | 0 |
smallest-string-with-a-given-numeric-value | ✅ C++ || Dry Run || Easy || 🗓️ Daily LeetCoding Challenge || March || Day 22 | c-dry-run-easy-daily-leetcoding-challeng-scvt | Please Upvote If It Helps\n\nAlgorithm\n Initialise an string of length n with all \'a\'s\n\n Start traversing from the end of the string while k is positive\n | mayanksamadhiya12345 | NORMAL | 2022-03-22T17:12:17.052153+00:00 | 2022-03-22T17:12:17.052200+00:00 | 34 | false | **Please Upvote If It Helps**\n\n**Algorithm**\n* **Initialise** an string of length **n** with all **\'a\'s**\n\n* **Start traversing** from the **end of the string** while **k is positive**\n* add the element of the string by **\'z\' if k >= 25**\n* else add the **ascii value character** \n* At the same time decrease the **value of K** by **replaced element value**\n* Break the loop if **k==0**\n* return the final **result string** \n\n```\nclass Solution \n{\npublic:\n string getSmallestString(int n, int k) \n {\n // declaring a string with size n with all values a \n string res(n,\'a\');\n \n // substracting the n because we used the n times a in our string \n k -= n;\n \n // make a while loop \n // and iterate it till k gets 0\n while(k>0)\n {\n // start changing the characters from behind for maintaining the lexicographically smallest string \n // take the min of (k,25) if its 25 the replace with z otherwise replace with Kth character\n // and decraese that value from k\n res[--n] += min(25,k);\n k -= min(25,k);\n }\n return res;\n }\n};\n```\n\n\n**Please Upvote If It Helps**\n// Dry Run \n// n = 4 , k = 37\n \n// res = "aaaa"\n// k = (37-4) = 33\n \n// iteration 1.\n// res[4] = min(25,33) = 25 = (\'a\'+25) = \'z\'\n// k = (33-25) = 8\n// 4--\n \n// iteration 2.\n// res[3] = min(25,8) = 8 = (\'a\'+8) = \'i\'\n// k = (8-8) = 0\n// 3--\n \n// k == 0 (break the loop) | 2 | 0 | [] | 1 |
smallest-string-with-a-given-numeric-value | Python | Reverse filling | Easy and clean solution | python-reverse-filling-easy-and-clean-so-j5oz | \nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n result, k, i = [\'a\']*n, k-n, n-1\n while k:\n | revanthnamburu | NORMAL | 2022-03-22T13:46:42.035521+00:00 | 2022-03-22T13:46:42.035565+00:00 | 143 | false | ```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n result, k, i = [\'a\']*n, k-n, n-1\n while k:\n k = k + 1\n if k/26 >= 1:\n result[i], k, i = \'z\', k-26, i-1\n else:\n result[i], k = chr(k + 96), 0\n return \'\'.join(result)\n```\n**I hope that you\'ve found this useful.**\n**In that case, please upvote. It motivates me to write more such posts\uD83D\uDE03**\nComment below if you have any queries. | 2 | 0 | ['String', 'Python'] | 0 |
smallest-string-with-a-given-numeric-value | ✅ C++ Solution with explanation | O(n) | c-solution-with-explanation-on-by-karyga-45yw | Explanation\n\n1. Initialise a string ans all with \'a\'s (to ensure that the answer will be lexicographically small\n2. Start traversing the string from the en | karygauss03 | NORMAL | 2022-03-22T10:25:49.631977+00:00 | 2022-03-22T10:25:49.632001+00:00 | 32 | false | **Explanation**\n```\n1. Initialise a string ans all with \'a\'s (to ensure that the answer will be lexicographically small\n2. Start traversing the string from the end, and if k >= 25 we add \'z\' instead of \'a\' else we add \n\'b\' or \'c\' or .... \'y\' depending on k. (ans[i] += min(25, k)).\n3. We decrease the value of k by the replaced element. (k -= min(25, k)).\n4. If k < 0 we break the loop.\n5. Finally, we return the updated string ans.\n```\n**Code**\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n string ans = "";\n\t\t//Initialise string ans with \'a\'s\n for (int i = 0 ; i < n ; i++){\n ans += "a";\n }\n\t\t// The value of \'a\' is 1 so we decrease k by n\n k -= n;\n\t\t//Index j for the last position\n int j = n - 1;\n while (k > 0){\n\t\t\t//Update the rightmost caracter by min(25, k)\n\t\t\t//It means : ans[j] = min(\'a\' + 25, \'a\' + k);\n ans[j] += min(25, k);\n\t\t\t//Decrease K & j\n k -= min(25, k);\n j--;\n }\n\t\t//Return the answer\n return ans;\n }\n};\n```\n# Please Upvote if that helps you | 2 | 0 | [] | 0 |
smallest-string-with-a-given-numeric-value | C++|| easy to understand || with comments || greedy | c-easy-to-understand-with-comments-greed-kce5 | \nstring getSmallestString(int n, int k) {\n string ans="";\n for(int i=0;i<n;i++)\n {\n //for getting the lexicographically smalles | priyanshu12k5 | NORMAL | 2022-03-22T09:46:02.014049+00:00 | 2022-03-22T09:46:02.014082+00:00 | 71 | false | ```\nstring getSmallestString(int n, int k) {\n string ans="";\n for(int i=0;i<n;i++)\n {\n //for getting the lexicographically smallest string\n ans+=\'a\';\n }\n k=k-n;\n int j=n-1;\n while(k!=0 && j>=0)\n {\n// as we have added a now when ever we replace a we need to add an extra 1 as a=1\n k=k+1;\n \n int m=k%26;\n int p=k/26;\n// if element have k>26 than we can add a z in the end replacing it by a\n if(p>=1)\n {\n ans[j]=\'z\';\n k=k-26;\n \n }\n// if j<26 now we cn hust replace it by one char \n else{\n cout<<m<<" ";\n ans[j]=char(m+97-1);\n k=k-m;\n }\n j--;\n \n }\n \n return ans;\n }\n``` | 2 | 0 | ['Greedy', 'C'] | 0 |
smallest-string-with-a-given-numeric-value | ✔️[C++] || 6 line Simple Code || Easy to understand || TC: O( n ) , SC: O( 1 ) | c-6-line-simple-code-easy-to-understand-8025t | ```\nstring getSmallestString(int n, int k) {\n string res(n,\'a\');\n for(int i=0;i=26) res[n-1-i]=\'z\' , k-=26;\n else res[n-1-i]=c | anant_0059 | NORMAL | 2022-03-22T09:34:45.675105+00:00 | 2022-03-22T09:34:45.675151+00:00 | 289 | false | ```\nstring getSmallestString(int n, int k) {\n string res(n,\'a\');\n for(int i=0;i<n;++i){\n int remain=k-(n-1-i);\n if(remain>=26) res[n-1-i]=\'z\' , k-=26;\n else res[n-1-i]=char(\'a\'+remain-1) , k=n-i-1;\n }\n return res;\n } | 2 | 0 | ['Greedy', 'C'] | 0 |
smallest-string-with-a-given-numeric-value | C++ Easy Explanation Optimal Approach | c-easy-explanation-optimal-approach-by-j-w827 | Approach\nstart with a string of all a\'s of length n as it is the lexicographically smallest string possible\nupdate k as k-n\n\nnow we traverse our string fro | jay_suk23 | NORMAL | 2022-03-22T09:27:50.231788+00:00 | 2022-03-22T09:27:50.231835+00:00 | 26 | false | **Approach**\nstart with a string of all a\'s of length n as it is the lexicographically smallest string possible\nupdate k as k-n\n\nnow we traverse our string from right to left and try to fill it with the maximum character possible (this will make sure that we get the lowest characters to the left)\n\n\n**fillling indexes with characters**\n--whenever we get our k>25,\nwe fill that index with z and update k=k-25\n--whenever we get k<25\nwe fill that index with (\'a\'+k)th character and update k=0\n\n**we are using 25 instead of 26 coz our indexex are already filled with value 1 aka character \'a\'**\n\nwe will stop when we traverse all the index or **k becomes 0**\n\n**Code**\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n \n string ans(n,\'a\');\n \n k=k-n;\n \n for(int i=n-1;i>=0;i--)\n {\n if(k==0)\n break;\n if(k<25)\n {\n ans[i]=\'a\'+k;\n k=0;\n }\n else\n {\n ans[i]=\'z\';\n k=k-25;\n }\n }\n return ans;\n }\n};\n\nPLEASE UPVOTE | 2 | 0 | ['String', 'Greedy', 'C'] | 0 |
smallest-string-with-a-given-numeric-value | c++ | Easy approach | Greedy | with explanation | with example | c-easy-approach-greedy-with-explanation-qz9m3 | Input: n = 5, k = 73\nOutput: "aaszz"\n\nstep 1: make string of length n=5 and fill it with \'a\'. after step 1 our ans string will look like ans="aaaaa"\nstep | akashjoshi99 | NORMAL | 2022-03-22T09:15:48.145528+00:00 | 2022-03-22T09:15:48.145562+00:00 | 140 | false | Input: n = 5, k = 73\nOutput: "aaszz"\n\nstep 1: make string of length n=5 and fill it with \'a\'. after step 1 our **ans** string will look like **ans="aaaaa"**\nstep 2: iterate from back and keep the track of **sumOfString** after replacing ith index with \'z\'. sumOfString(aaaaz) is 1+1+1+1+26=30.\nstep 3: if sumOfString less than k then will update our \'ans\' string, repeat this untill we find sumOfString greater than k. \n\nsumOfString(aazzz) is 1+1+26+26+26=80, (80>k) so we can\'t add \'z\' at 2nd index. we need to find something smaller than \'z\'. till here \'ans\' string is aaazz and its sum is 55\n\nstep 4: replace ans[i] with char at \n(k-sumOfString+1) => ( 73-55+1)=19 (\'s\') \n**ans=aaszz**\n \n\n\n\n\n\n\n\n```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n\t\t//creating string of length n while initializing with minimum lexicographical order\n string ans(n,\'a\');\n int stringSum=n;\n \n \n for(int i=n-1;i>=0;i--){\n\t\t\t//calculating sum of string after appending \'z\' at ith index\n\t\t\t// if (k-sumOfString) >=0 then we\'ll add \'z\' at ith index\n if(k-(i+(n-i)*(\'z\'-\'a\'+1))>=0){\n ans[i]=\'z\';\n stringSum=(i+(n-i)*(\'z\'-\'a\'+1));\n }else{\n\t\t\t\t//else add char smaller than \'z\' \n ans[i]=\'a\'+(k-stringSum);\n break;\n }\n }\n return ans;\n }\n};\n``` | 2 | 0 | ['Greedy', 'C'] | 0 |
smallest-string-with-a-given-numeric-value | Math with short proof | beats 98.62% / 99.54% | math-with-short-proof-beats-9862-9954-by-efnq | This solution is based on the fact that every output will always be of the form aaa...aaa?zzz...zzz regardless of the values of k and n.\n\nThis submission ran | sr_vrd | NORMAL | 2022-03-22T08:09:59.103240+00:00 | 2022-03-22T12:57:59.394816+00:00 | 55 | false | This solution is based on the fact that every output will always be of the form `aaa...aaa?zzz...zzz` *regardless* of the values of `k` and `n`.\n\nThis submission ran at **32 ms** and used **14.8 MB** of memory. At the time of submission it was **faster than 98.62%** Python3 submissions, and used **less memory than 99.54%** Python3 submissions.\n\nWe will first prove the following assertion:\n\n**Assertion:** Since we have all possible (integer) scores from 1 to 26 available, and *n ≤ k ≤ 26 × n*, it is **always** possible to write *k* as the sum of *n* integers from *[1,26]* with **at most one** of them not *1* or *26*.\n\nWe will construct a proof of this assertion, and then we will replicate this construction in our solution.\n\n**Proof:** Since *0 ≤ k - n*, the division algorithm guarantees that there exist **unique** integers *0 ≤ n<sub>z</sub>* and *0 ≤ r < 25* such that *k - n = 25 × n<sub>z</sub> + r*. Rearranging, we can rewrite this same expression as *k = 1 × (n - n<sub>z</sub> - 1) + (r + 1) + 26 × n<sub>z</sub>*. In other words, we can write *k* as the sum of *(n - n<sub>z</sub> - 1)* *1*\'s, one integer between *1* and *25*, and *n<sub>z</sub>* *26*\'s. ∎\n\n* Note there is nothing special about 26 in this proof, so this can be generalized to:\n**Assertion:** Let *n ≥ 1*, *b ≥ 1*, and *n ≤ k ≤ b × n* be integers. Then it is always possible to write *k* as the sum of *n* integers in *[1, b]*, where at most one of them is not *1* or *b*.\n\nThis assertion tells us then that all of the lexicographically smallest strings we can obtain are of the form `aaa...aaa?zzz...zzz`, with *(n - n<sub>z</sub> - 1)* `a`\'s, one character between `a` and `y`, and *n<sub>z</sub>* `z`\'s.\n\nFrom the proof, we can see that `nz = (k - n) // 25`, `na = n - nz - 1` and the score of the character in the middle is `mid = k - na - 26 * nz`. So we can construct our solution as\n```\n return \'a\' * na + chr(96 + mid) + \'z\' * nz # char(97) = a\n```\nThere is one problen, though. When *k = 26 × n* we have that *n<sub>z</sub> = n*, so there should be *n* `z`\'s, *(n - n - 1) = -1* `a`\'s, and the score of the character in between should be *26n - (-1) - 26n = 1* (so it\'s an `a`). Theoretically the solution should work, however Python does not handle an expression as `\'a\' * (-1) + \'a\'` as one would like, and this would output `azzz...zzz` instead of the desired `zzz...zzz`.\n\nThis edge case can be fixed with\n```\n if k == 26 * n:\n return \'z\' * n\n```\n∎\n\nThe whole solution is:\n```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n\n if k == 26 * n:\n return \'z\' * n\n \n nz = (k - n) // 25\n na = n - nz - 1\n mid = k - na - 26 * nz\n \n return \'a\' * na + chr(96 + mid) + \'z\' * nz\n``` | 2 | 0 | ['Math'] | 0 |
smallest-string-with-a-given-numeric-value | ✅ JAVA || 10 LINES || ONE TRAVERSAL || EASY || SIMPLE || | java-10-lines-one-traversal-easy-simple-q4dhh | JAVAEASY CLEAN & READABLE\n\n\n\n* Our main goal is to build a string lexiographically smallest.\n* So the smallest possible string for any n would be full of \ | bharathkalyans | NORMAL | 2022-03-22T07:50:01.168608+00:00 | 2022-03-22T07:50:01.168640+00:00 | 95 | false | ``` ```**JAVA**``` ```**EASY**``` ``` ``` ```**CLEAN & READABLE**``` ```\n\n\n```\n* Our main goal is to build a string lexiographically smallest.\n* So the smallest possible string for any n would be full of \'a\'.\n* Now we have to fuflill another condition that is we want the sum of characters to be some k.\n* By maintaining the lexiograhically smallest string we have to full fill this condition.\n* So we start from rightmost part.\n* If we have k greater than 25 [\'z\'] we replace it with \'z\' and subtract it with 25 == \'z\'.\n* Else replace it with the required character with k as number.\n* Do this until k > 0.\n```\n```\nclass Solution {\n public String getSmallestString(int n, int k) {\n char[] answer = new char[n];\n Arrays.fill(answer, \'a\');\n k = k - n;\n while (n > 0 && k > 0) {\n int min = Math.min(25, k);\n answer[--n] = (char) (min + \'a\');\n k -= min;\n }\n return String.valueOf(answer);\n }\n}\n```\n**UPVOTE IF HELPFUL \uD83D\uDE4F** | 2 | 1 | ['Java'] | 1 |
smallest-string-with-a-given-numeric-value | C++ || Greedy Approch && Reverse filling || O(n) time | c-greedy-approch-reverse-filling-on-time-rtzl | \nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n \n string res = "";\n \n while(n>0){\n \n | pandeyji2023 | NORMAL | 2022-03-22T07:00:26.458645+00:00 | 2022-03-22T07:00:26.458670+00:00 | 26 | false | ```\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n \n string res = "";\n \n while(n>0){\n \n for(int i=26;i>=1;i--){\n \n int rem = k-i;\n if(rem >= n-1){\n char c = i-1+\'a\';\n res += c;\n k -= i;\n break;\n }\n }\n n--;\n }\n reverse(res.begin(),res.end());\n return res;\n }\n};\n``` | 2 | 0 | [] | 0 |
smallest-string-with-a-given-numeric-value | You will love it || Java || Simple Solution || Well Commented | you-will-love-it-java-simple-solution-we-uzt8 | Very Easy Solution. I have commented all steps.\n\nclass Solution {\n public String getSmallestString(int n, int k) {\n char[] ch = new char[n]; // In | samyakdarshan97 | NORMAL | 2022-03-22T06:21:30.311106+00:00 | 2022-03-22T06:21:30.311147+00:00 | 175 | false | Very Easy Solution. I have commented all steps.\n```\nclass Solution {\n public String getSmallestString(int n, int k) {\n char[] ch = new char[n]; // Initially taking a character array and later we will convert it to String.\n int i=0;\n for(;i<n;i++){\n ch[i] = \'a\'; // Initially keeping all characters as \'a\' bcoz we need to use all n places of the string.\n }\n i--; //for keeping track of i. Here it is n-1;\n k = k-n; // after setting initially keeping all as \'a\' so we have k-n values left.\n while(k>0){\n if(k>25){ //if leftover value of k is more than 25, we add it to the current i\'th index since we can\'t cross 26 for a particular index.\n ch[i] += 25;\n i--; //now going to the next index;\n k-=25; //and decreasing value of k as well since we used 25 for last index.\n }\n else{\n ch[i]+=k; // if k is < 25 then we simply add it to the present index and end the loop\n k=0; //by setting k value to 0.\n }\n }\n return String.copyValueOf(ch); //converting char array to String.\n }\n}\n``` | 2 | 0 | ['String', 'Greedy', 'Java'] | 0 |
smallest-string-with-a-given-numeric-value | By Math | by-math-by-haiyunjin-ktx7 | This is simply a math problem.\n\n1. Fill all with "a".\n2. Then for each extra number, add from the right. The number of "z"\'s on the right can be calculated | haiyunjin | NORMAL | 2022-03-22T06:05:28.215800+00:00 | 2022-03-22T06:05:51.132263+00:00 | 31 | false | This is simply a math problem.\n\n1. Fill all with "a".\n2. Then for each extra number, add from the right. The number of "z"\'s on the right can be calculated as `extra/25`.\n3. The letter right after "a"\'s can also be calculated by `\'a\' + extra%25`\n4. Then just add "a"\'s, first non-\'a\' and fill rest with "z".\n\nA corner case to handle is when all are "z"\'s. \n\n\n```\nclass Solution {\n public String getSmallestString(int n, int k) {\n // fill all with 1, then fill from the back\n int extra = k - n;\n int zs = extra/25;\n int lastletter = extra%25;\n \n StringBuilder sb = new StringBuilder();\n for (int i = 1 ; i < n - zs ; i++) {\n sb.append("a");\n }\n if (n > zs) {\n sb.append((char)(\'a\' + lastletter));\n }\n for (int i = 0 ; i < zs ; i++) {\n sb.append("z");\n }\n \n return sb.toString();\n }\n}\n``` | 2 | 0 | ['Math', 'Java'] | 0 |
smallest-string-with-a-given-numeric-value | ✅ Easy-Peasy Solution | Greedy | easy-peasy-solution-greedy-by-igi17-pbaq | Intuition- At each index of string take smallest character possible such that remaining character \n are possible to fill with remaining k value. | igi17 | NORMAL | 2022-03-22T05:56:39.141779+00:00 | 2022-03-22T05:59:54.515901+00:00 | 78 | false | ***Intuition***- At each index of string take smallest character possible such that remaining character \n are possible to fill with remaining k value.\n\n\tclass Solution {\n\tpublic:\n\t\tstring getSmallestString(int n, int k) {\n\t\t\tstring ans;\n\t\t\tfor(int i=0;i<n;i++){\n\t\t\t\tfor(int j=0;j<26;j++){\n\t\t\t\t\t int t=k-(j+1);\n\t\t\t\t\t if(t<=26*(n-i-1))\n\t\t\t\t\t {\n\t\t\t\t\t\t ans.push_back(\'a\'+j);\n\t\t\t\t\t\t k=t;\n\t\t\t\t\t\t break;\n\t\t\t\t\t }\n\t\t\t\t}\n\t\t\t}\n\t\t\treturn ans;\n\t\t}\n\t};\n\t\nDO \u2B06\uFE0F ***UPVOTE*** IF IT WAS HELPFUL !\t | 2 | 0 | ['Greedy', 'C'] | 0 |
smallest-string-with-a-given-numeric-value | C++ | one line solution, fast solution and explanation |O(n) or can O(1)? | c-one-line-solution-fast-solution-and-ex-tfp2 | 1. One line solution\nThe solution can be combined by three kind of string, so we have the one line solution. \nC++\nclass Solution {\npublic:\n string getSm | milochen | NORMAL | 2022-03-22T05:39:13.563841+00:00 | 2022-03-22T05:39:13.563867+00:00 | 141 | false | # 1. One line solution\nThe solution can be combined by three kind of string, so we have the one line solution. \n```C++\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n\t\treturn string(n-(k-n)/25-((k-n)%25!=0), \'a\') + string(((k-n)%25!=0),\'a\'+(k-n)%25) + string((k-n)/25,\'z\');\n\t}\n};\n```\n\nThe truth is that my one line code is made from this following code. \nBut code become slow if you use this one line solution. So I prefer\nthe following fast solution. It\'s faster to be executed and easier to be read. \n# 2. Fast solution\n```C++\nclass Solution {\npublic:\n string getSmallestString(int n, int k) {\n int total = k-n;\n int z_cnt = total / 25;\n int middle_val = total % 25;\n string ans = string(n,\'a\');\n int i = n-1;\n for(int j =0;j<z_cnt ;i--,j++)ans[i]=\'z\';\n if(i>=0) ans[i]=\'a\'+middle_val;\n return ans; \n\t}\n};\n```\n\n# 3. Explanation of the fast solution \nAt the first, we would try our best to find the lexicographically smallest string. \nSo the solution will like aaaaa?zzzzz. So we will be intresting on three thing\n(1) how many a?\n(2) what ? it is ?\n(3) how many z?\n\n\nIn the original problem, there are input n,k with this mapping table 1\n```txt\n a b c d e f g h i j k l m n o p q r s t u v w x y z \n 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26\n```\nBut we can get simple observation if we can change our view into mapping table 2\n```txt\n a b c d e f g h i j k l m n o p q r s t u v w x y z \n 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 \n```\nand focus on the value `k-n`. The observation become simple.\nLet me explain following.\n\n```txt\ncase n=3, k=27 (k-n=24)\n24 = 0+0+24 -> "aay" (refer mapping table 2)\n\ncase n=5, k=73 (k-n=68)\n68 = 0+0+18+25+25 -> "aaszz" (refer mapping table 2)\n\ncase n=4, k=54 (k-n = 50) \n50 = 0+0+25+25 -> "aazz" (refer mapping table 2)\n```\n\nThus, this observation is good for us to write a code for it.\nThat\'s why fast solution look like that.\n\n\n# 4. Complexity analysis for fast solution\n**Time complexity O(n)**\nBecause allocate n number of memory with time O(n) and use time O(n) to process rewirte z in the result.\n**Space complexity O(n)**\nBecause output string is using n number of space. \nBut if some people think output space should not be in space complexity, then they will think this as a space O(1) solution. \n\n\n\n# 5. What if time complexity of memory allocation can be faster?\nIf the time complexity of memory allocation can be O(1) and your can control where to allocated, then \nthe time complexity will be O(1) for one line solution\n\nFor example, a realtime system might use an heap allocator like TLSF (Two-Level Segregate Fit), which has a O(1) allocation cost. http://www.gii.upv.es/tlsf/ \n\nif we have the such system, then one line solution is the best choice because time complexity is O(1). \nBut for almost case, fast solution with time complexity O(n) always be the good solution. \n | 2 | 0 | ['C'] | 0 |
smallest-string-with-a-given-numeric-value | Greedy Python Solution with Explanation | greedy-python-solution-with-explanation-c78xw | Observation:\nFor any test case we will have some number of \'a\' in the start one or zero character from \'b\' to \'y\' in the middle and some number of \'z\' | ancoderr | NORMAL | 2022-03-22T04:47:28.337047+00:00 | 2022-03-22T04:52:46.093766+00:00 | 195 | false | **Observation:**\nFor any test case we will have some number of \'a\' in the start one or zero character from \'b\' to \'y\' in the middle and some number of \'z\' in the end.\n\n**Intuition:**\nWe want the lexicographically smallest string. If we have a choice to either chose b/w **`az`** and **`by`**, we will chose **`az`** as it is smaller. This suggests that at each step we can make a greedy choice of taking the smallest possible character. However this greedy choice must be made considering that at **i\'th** iteration after taking the smallest character can we still divide the remaining number of k into **n-i-1** ***(remaining number of n)*** number of groups of 26 ***(character \'z\')*** . In simpler terms we want to take the smallest possible character while considering that can the remaining numeric value k be divided into remaining number of \'z\' character. We are counting on the fact that k is divisible by n-i-1.\nk -> Reamining numeric value.\nn - i - 1 -> Remaining number of characters exclusive of the current character in iteration.\nj -> Numeric value of current character.\nSince, `{ (k - j) / (n - i - 1) } <= 26` **(if not it will go beyond character \'z\')**\n=> `26 * (n - i - 1) >= k - j` **(This condition is used in the code)**\n\n**Steps:**\n1. Iterate over range(n).\n2. For each iteration check if taking \'a\' is possible.\n3. If not then find that middle element b/w \'b\' and \'y\' by iterating over 1 to 26 till the remaining number of k can be divided into remaining number of characters(n-i-1).\n4. Till now we found the starting and middle elements. We now need to find the number of \'z\' elements.\n5. If k is still available then check if it is <26. If is is then append that character.\n6. If k is >= 26 then it can be divided into k//26 number of \'z\' characters. So append \'z\' times k//26.\n7. Join the array and return it.\n\n**Code:**\n```\nclass Solution:\n def getSmallestString(self, n: int, k: int) -> str:\n offset, arr = 96, []\n for i in range(n):\n j = 1\n # Meaning that if we consider the current char to be \'a\' then the remaining\n # number of numeric value k cannot be divided into required number of \'z\'\n if 26*(n-i-1) < k-j:\n for j in range(1, 27): # Check which middle character to take\n if j > k or 26*(n-i-1) >= k-j: # If remaining number of k cannot be divided into n-i-1 groups of \'z\'(26)\n break\n k -= j\n arr.append(chr(j+offset))\n # Since latest element is not \'a\', we most probably found the middle character(\'b\' to \'y\')\n if arr[-1] != \'a\' and k > 0:\n if k < 26: # If k < 26 then we cannot fill in \'z\'\n arr.append(chr(k+offset))\n else: # If k > 26 then we can fill k//2 number of \'z\'\n arr.append(\'z\' * (k // 26))\n break # All k have been expired by this point. Thus break and return \n return \'\'.join(arr)\n``` | 2 | 0 | ['Greedy', 'Python', 'Python3'] | 0 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.