question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
maximum-average-pass-ratio | Priority Queue | Greedy | C++ | Easy Solution | Comments | priority-queue-greedy-c-easy-solution-co-img2 | Explaination:\nOur goal is to maximize average. So, we should add student in the class which can give us highest difference of pass ratio. To pick up highest di | harsh_114 | NORMAL | 2021-03-14T04:35:37.713874+00:00 | 2021-03-14T04:35:37.713902+00:00 | 186 | false | ### Explaination:\nOur goal is to maximize average. So, we should add student in the class which can give us highest difference of pass ratio. To pick up highest difference from given difference Priority Queue(Max Heap) is useful. \n\n### Algorithm:\n1. Add one to pass and total for each class. Calculate new pass ratio and compute difference between old pass ratio and new pass ratio for each class and push difference and class index in Priority Queue. \n2. For each new student we have to determine in which class we can include it. So take top element of Priority Queue(having max diff. b/w new pass ration and old pass ratio). Increase classes[ind][0] and classes[ind][1] by one. Compute difference as mentioned in step 1 for ind and again push difference and class index in Priority Queue.\n3. After that for each class compute pass ratio and add them into one variable. Return sum of pass ratio / number of classes.\n\n### Code:\n\nclass Solution {\npublic:\n```\n double maxAverageRatio(vector<vector<int>>& classes, int extraStudents) {\n cout<<setprecision(5); //to set prcision upto 10^-5\n priority_queue<pair<double,int>> pq;\n int n=classes.size(); \n for(int i=0;i<n;i++){\n int num=classes[i][0];\n int den=classes[i][1];\n int n_num=num+1;\n int n_den=den+1;\n double dif=(double)(n_num*1.0/n_den)-(double)(num*1.0/den);\n // calculating difference between old pass ratio and after adding a student pass ratio\n pq.push(make_pair(dif,i));\n\t\t\t// pushing pass ratio and index into priority queue\n }\n while(extraStudents--){\n int tind=pq.top().second;\n int tdiff=pq.top().first;\n // tind dontes top index or index of class having highest difference after adding a student\n pq.pop();\n\t\t\t// poping out top pair\n classes[tind][0]++;\n classes[tind][1]++;\n\t\t\t// as we have decided to add a student into class with index tind we have to increase total number of students and number of students who can pass\n int num=classes[tind][0];\n int den=classes[tind][1];\n int n_num=num+1;\n int n_den=den+1;\n double dif=(double)(n_num*1.0/n_den)-(double)(num*1.0/den);\n // calculated difference after adding one student\n pq.push(make_pair(dif,tind));\n // pushing new difference to the priority queue\n }\n double sum=0;\n for(int i=0;i<n;i++){\n sum+=(double)(classes[i][0]*1.0/classes[i][1]);\n // adding pass ration to sum variable\n }\n \n return sum/n;\n }\n};\n```\n\n### Complexity Analysis:\nSuppose there are n number of classes. So complexity of step1 is O(n).\nSuppose there are m number of students. So complexity of step2 is O(mlog(n)) because getting max element is constant time operation and insertion in heap is log(n) time operation.\nComplexity of step3 is O(n).\n\nAs 1<=n,m<=10^5\n we can take m=n.\n \n So all over complexity O(n) + O(nlog(n)) + O(n) = O(nlogn).\n \n If you like this solution please upvote :) | 2 | 0 | ['Greedy', 'Heap (Priority Queue)', 'C++'] | 0 |
maximum-average-pass-ratio | Java O(mlogc) solution with clear explanation and comments | java-omlogc-solution-with-clear-explanat-day0 | The challenge that I faced was to know where to add the extra student. We cannot simply add a student to a class with lowest pass ratio.\n\nWhy? For eg: consid | karthiko | NORMAL | 2021-03-14T04:24:04.811531+00:00 | 2021-03-14T04:24:04.811557+00:00 | 70 | false | The challenge that I faced was to know where to add the extra student. We cannot simply add a student to a class with lowest pass ratio.\n\nWhy? For eg: consider the ratios 2/3 (0.6666) and 3/5 (0.6) \nif we add 1 student to 2/3 -> 3/4 (0.75)\nor\nif we add 1 student to 3/5 -> 4/6 (0.6666)\n\nFrom above we see that we cannot just simply add the student to lowest ratio (3/5).\n\nSo the idea is to compute the ratio increases (if we add one student) for each class and store in maxHeap.\nThe top of the heap will always have the class which is the best candidate to add the new student.\n\n**Code:**\n```\nclass Solution {\n\n // T = O(mlogc) S=O(c) c=no. of classes m= extra students\n public double maxAverageRatio(int[][] classes, int extraStudents) {\n \n double totalPassRatios = 0.0;\n // track the max new Pass Ratio (if a student is added to a class)\n // format: double[] {passRatio increase if a student is added, pass count, student count}\n PriorityQueue<double[]> maxHeap = new PriorityQueue<>((a,b) -> Double.compare(b[0], a[0]));\n \n for(int i=0; i< classes.length; i++) {\n // pass ratio\n double passRatio = (double) classes[i][0]/classes[i][1];\n // alternate pass ratio if a student is added\n double altPassRatio = (double) (classes[i][0]+1)/(classes[i][1]+1);\n \n maxHeap.add(new double[]{altPassRatio-passRatio, classes[i][0], classes[i][1]});\n }\n \n while(!maxHeap.isEmpty() && extraStudents > 0) {\n double[] top = maxHeap.poll();\n double passRatio = (double) (top[1]+1)/(top[2]+1);\n double altPassRatio = (double) (top[1]+2)/(top[2]+2);\n // update the maxHeap\n maxHeap.add(new double[]{altPassRatio-passRatio, top[1]+1, top[2]+1});\n \n extraStudents--; // decrement the extraStudents\n }\n \n // add all the ratios from Heap\n while(!maxHeap.isEmpty()) { \n double[] top = maxHeap.poll();\n double passRatio = (double) (top[1])/(top[2]);\n totalPassRatios += passRatio;\n }\n \n return totalPassRatios / classes.length;\n }\n}\n``` | 2 | 0 | [] | 0 |
maximum-average-pass-ratio | [JS] Greedy Heap O(M log N) O(N) | js-greedy-heap-om-log-n-on-by-stevenkino-8zof | javascript\nvar maxAverageRatio = function(classes, extraStudents) {\n // the heap will automatically find the class that will benefit\n // the most by ad | stevenkinouye | NORMAL | 2021-03-14T04:10:45.081215+00:00 | 2021-03-14T04:16:49.933252+00:00 | 277 | false | ```javascript\nvar maxAverageRatio = function(classes, extraStudents) {\n // the heap will automatically find the class that will benefit\n // the most by adding a passing student\n const heap = new MaxHeap();\n \n // push all the classes into the heap so that\n // the heap can find the class that will benefit the most\n for (const x of classes) {\n heap.push(x);\n }\n \n // while there are extra students\n while (extraStudents) {\n \n // add the extra student to the class that will benefit the most\n heap.peak()[0] += 1;\n heap.peak()[1] += 1;\n \n // heapify down so that the heap remains valid\n heap.heapifyDown(0);\n \n extraStudents--;\n }\n \n \n // calculate the new average of all the classes\n let total = 0;\n for (const [x , y] of heap.store) {\n total += (x / y);\n }\n return total / heap.store.length;\n};\n\n\nclass MaxHeap {\n constructor() {\n this.store = [];\n }\n \n peak() {\n return this.store[0];\n }\n \n size() {\n return this.store.length;\n }\n \n pop() {\n if (this.store.length < 2) {\n return this.store.pop();\n }\n const result = this.store[0];\n this.store[0] = this.store.pop();\n this.heapifyDown(0);\n return result;\n }\n \n push(val) {\n this.store.push(val);\n this.heapifyUp(this.store.length - 1);\n }\n \n heapifyUp(child) {\n while (child) {\n const parent = Math.floor((child - 1) / 2);\n if (this.shouldSwap(child, parent)) {\n [this.store[child], this.store[parent]] = [this.store[parent], this.store[child]]\n child = parent;\n } else {\n return child;\n }\n }\n }\n \n heapifyDown(parent) {\n while (true) {\n let [child, child2] = [1,2].map((x) => parent * 2 + x).filter((x) => x < this.size());\n if (this.shouldSwap(child2, child)) {\n child = child2\n }\n if (this.shouldSwap(child, parent)) {\n [this.store[child], this.store[parent]] = [this.store[parent], this.store[child]]\n parent = child;\n } else {\n return parent;\n }\n }\n }\n \n shouldSwap(child, parent) {\n if (!child) return false;\n const c = (this.store[child][0] + 1) / (this.store[child][1] + 1) - (this.store[child][0]) / (this.store[child][1]);\n const p = (this.store[parent][0] + 1) / (this.store[parent][1] + 1) - (this.store[parent][0]) / (this.store[parent][1]);\n return c > p;\n }\n}\n``` | 2 | 0 | ['Heap (Priority Queue)', 'JavaScript'] | 0 |
maximum-average-pass-ratio | Python [greedy] | python-greedy-by-gsan-jyz3 | In this question we want to modify ratios r1, r2, ..., rn such that (r1 + r2 + ... + rn) / n is maximized. As n is common, equivalently maximize (r1 + r2 + ... | gsan | NORMAL | 2021-03-14T04:02:28.504932+00:00 | 2021-03-14T04:02:28.504963+00:00 | 134 | false | In this question we want to modify ratios `r1, r2, ..., rn` such that `(r1 + r2 + ... + rn) / n` is maximized. As `n` is common, equivalently maximize `(r1 + r2 + ... + rn)`.\n\nSuppose that we only have 1 extra student. We should choose the ratio `ri = xi/yi` such that the difference `(xi+1)/(yi+1) - xi/yi` is maximum. Suppose that this is true for `k` extra students. If we have a `k+1`-st student, then we should once again find the ratio such that the above target difference is maximized. It then follows from the Principle of Induction that this stepwise approach is optimal for all values of `extraStudents`.\n\nThis is now nothing but a greedy solution. We can easily code using a max heap.\n\n```python\nclass Solution:\n def maxAverageRatio(self, classes, extraStudents):\n hp = []\n for x, y in classes:\n target_difference = (x+1)/(y+1) - x/y\n heapq.heappush(hp, (-target_difference, x, y))\n \n for _ in range(extraStudents):\n _, x, y = heapq.heappop(hp)\n target_difference = (x+2)/(y+2) - (x+1)/(y+1)\n heapq.heappush(hp, (-target_difference, x+1, y+1))\n \n ans = 0\n while hp:\n _, x, y = heapq.heappop(hp)\n ans += x / y / len(classes)\n return ans\n``` | 2 | 0 | [] | 0 |
maximum-average-pass-ratio | [Python3] Heap solution with explanation | python3-heap-solution-with-explanation-b-499f | \u2018Assign each of the extraStudents students to a class to maximize the average pass ratio across all the classes\u2019 \n-> For each extraStudents find pair | danghuybk | NORMAL | 2021-03-14T04:00:53.656716+00:00 | 2021-03-14T04:04:10.010919+00:00 | 119 | false | - \u2018Assign each of the extraStudents students to a class to maximize the average pass ratio across all the classes\u2019 \n-> For each extraStudents find pair [pass, total] where **increase = (pass + 1) / (total + 1) - pass / total** maximum.\n\n- The idea is using a max heap to maintain pair [pass, total] with max increase.\n\n```\nExample: classes = [[1,2],[3,5],[2,2]], extraStudents = 2\nWith first student:\n\t* \tincrease = [0.16666, 0.06666, 0] -> Choose class (1, 2)\n\t\tmax_heap = [(-0.16666, 1, 2), (-0.06666, 3, 5), (-0.0, 2, 2)] \n\tIf you wonder why store (-increase) instead of increase, the reason is Python only support min_heap and -min_heap is a max_heap.\n\n\t* \tAt # 1: Pop (-0.16666, 1, 2) and add increase to total_increase\n\n\t* \tAt # 2: After assign 1 extraStudents to class (1, 2), class will be (2, 3). \n\tPush it again to max_heap: (-0.08333, 2, 3) and continue with second student.\n```\t\t\n\n\n\n```\nclass Solution:\n def maxAverageRatio(self, classes: List[List[int]], extraStudents: int) -> float:\n N = len(classes)\n ini_pass = 0\n total_increase = 0\n \n max_heap = []\n for p, c in classes:\n\t\t\t# Calculate total pass ratio at first\n ini_pass += p / c\n\t\t\t\n\t\t\t# Store increase pass ratio to max_heap\n increase = (p + 1) / (c + 1) - p / c\n heappush(max_heap, (-increase, p, c))\n \n while extraStudents:\n\t\t\t# Pop the pair with max increase\n increase, p, c = heappop(max_heap)\t\t\t# 1\n total_increase -= increase\n\t\t\t\n\t\t\t# Push the pair to max_heap again\n p, c = p + 1, c + 1\n increase = (p + 1) / (c + 1) - p / c\n heappush(max_heap, (-increase, p, c))\t\t# 2\n \n extraStudents -= 1\n \n return (ini_pass + total_increase) / N\n``` | 2 | 0 | [] | 0 |
maximum-average-pass-ratio | Easy Java solution | Beats 90% | easy-java-solution-beats-90-by-hee_maan_-u6xo | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | hee_maan_shee | NORMAL | 2025-01-15T15:53:36.300045+00:00 | 2025-01-15T15:53:36.300045+00:00 | 7 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Gain{
double gain;
double ratio;
int pass;
int total;
Gain(){}
Gain(double gain, double ratio, int pass, int total){
this.gain = gain;
this.ratio = ratio;
this.pass = pass;
this.total = total;
}
}
class Solution {
public double maxAverageRatio(int[][] classes, int extraStudents) {
PriorityQueue<Gain> pq = new PriorityQueue<>((a, b) -> Double.compare(b.gain, a.gain));
double sum = 0.0;
int n = classes.length;
for(int i=0; i<classes.length; i++){
int pass = classes[i][0];
int total = classes[i][1];
double currRatio = (double) pass/total;
double incRatio = ((double) (pass+1) / (total+1));
double gain = incRatio - currRatio;
sum += currRatio;
pq.add(new Gain(gain, currRatio, pass, total));
}
for(int i=0; i<extraStudents; i++){
Gain g = pq.poll();
double gain = g.gain;
double prevRatio = g.ratio;
int pass = g.pass;
int total = g.total;
sum -= prevRatio;
pass += 1;
total += 1;
double currRatio = (double) pass/total;
sum += currRatio;
double newGain = ((double) (pass+1) / (total+1)) - currRatio;
pq.add(new Gain(newGain, currRatio, pass, total));
}
return sum/n;
}
}
``` | 1 | 0 | ['Java'] | 0 |
maximum-average-pass-ratio | **easy understandable soln** | easy-understandable-soln-by-xxzq8mtv4e-vhh0 | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | XxZq8mTv4e | NORMAL | 2025-01-01T10:41:58.091601+00:00 | 2025-01-01T10:41:58.091601+00:00 | 8 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
double maxAverageRatio(vector<vector<int>>& classes, int extraStudents) {
auto calcGain = [](int pass, int total) -> double {
return (double)(pass + 1) / (total + 1) - (double)pass / total;
};
priority_queue<pair<double, pair<int, int>>> pq;
for (const auto& cls : classes) {
int pass = cls[0], total = cls[1];
pq.push({calcGain(pass, total), {pass, total}});
}
while (extraStudents > 0) {
auto [gain, cls] = pq.top();
pq.pop();
int pass = cls.first, total = cls.second;
pass += 1;
total += 1;
pq.push({calcGain(pass, total), {pass, total}});
extraStudents--;
}
double sum = 0.0;
while (!pq.empty()) {
auto [gain, cls] = pq.top();
pq.pop();
int pass = cls.first, total = cls.second;
sum += (double)pass / total;
}
return sum / classes.size();
}
};
``` | 1 | 0 | ['Array', 'Greedy', 'Sorting', 'Heap (Priority Queue)', 'C++'] | 0 |
maximum-average-pass-ratio | 100% working Java Code , Fixed Editorial Code. Java Double Precision Problem | 100-working-java-code-fixed-editorial-co-5156 | Complexity
Time complexity:
O(nlogn)
Space complexity:
O(n)
Code | doravivek | NORMAL | 2024-12-28T19:32:59.781034+00:00 | 2024-12-28T19:32:59.781034+00:00 | 21 | false |
# Complexity
- Time complexity:
$$O(nlogn)$$
- Space complexity:
$$O(n)$$
# Code
```java []
import java.util.PriorityQueue;
class Solution {
private double f(int p, int t) {
return ((double) (p + 1) / (t + 1)) - ((double) p / t);
}
public double maxAverageRatio(int[][] classes, int extraStudents) {
// Max heap to prioritize classes with the highest gain
PriorityQueue<double[]> pq = new PriorityQueue<>(
(a, b) -> Double.compare(b[0], a[0])
);
// Initialize the priority queue with the current gain and class data
for (int[] x : classes) {
int p = x[0];
int t = x[1];
double gain = f(p, t);
pq.add(new double[]{gain, p, t});
}
// Distribute extra students
while (extraStudents-- > 0) {
double[] curr = pq.poll();
int p = (int) curr[1];
int t = (int) curr[2];
pq.add(new double[]{f(p + 1, t + 1), p + 1, t + 1});
}
// Calculate the total average ratio
double sol = 0;
while (!pq.isEmpty()) {
double[] curr = pq.poll();
int p = (int) curr[1];
int t = (int) curr[2];
sol += (double) p / t;
}
return sol / classes.length;
}
}
``` | 1 | 0 | ['Array', 'Greedy', 'Heap (Priority Queue)', 'C++', 'Java', 'MySQL'] | 1 |
maximum-average-pass-ratio | 📊 Max Average Ratio | 🚀 Greedy + Priority Queue Solution 💡 | max-average-ratio-greedy-priority-queue-nhyzx | IntuitionThe problem asks for maximizing the average passing ratio after distributing extra students to various classes. Each class has a passing-to-total ratio | Raza-Jaun | NORMAL | 2024-12-17T23:46:20.079783+00:00 | 2024-12-17T23:46:20.079783+00:00 | 13 | false | # Intuition\nThe problem asks for maximizing the average passing ratio after distributing extra students to various classes. Each class has a passing-to-total ratio, and by adding a student to the class with the most potential improvement in its ratio, we can maximize the overall average.\n\n---\n\n\n\n# Approach\nWe first calculate the initial average passing ratio for each class and store the potential improvement (calculated using the difference between the new and old ratios when adding a student). This potential improvement is stored in a priority queue (max-heap), where classes with the highest improvement come first. For each extra student, we pop the class with the highest potential improvement, increment its pass count and total, update the sum, and push the class back into the priority queue. This process continues until all extra students are assigned. Finally, the total sum is averaged by the number of classes.\n\n\n\n---\n\n\n\n# Complexity\n- Time complexity:\n$$O(n log n + m log n)$$\n\n- Space complexity:\n$$O(n)$$\n\n---\n\n\n\n# Code\n```cpp []\nclass Solution {\npublic:\n double calcAverage(int pass, int total){\n return (double)(pass+1)/(total+1) - (double)pass/total;\n }\n\n double maxAverageRatio(vector<vector<int>>& classes, int extraStudents) {\n if(!classes.size()) return 0;\n priority_queue<pair<double,pair<int,int>>> pq;\n double sum = 0.0;\n for(auto x : classes){\n int pass = x[0];\n int total = x[1];\n sum += (double)pass/total;\n pq.push({calcAverage(pass,total),{pass,total}});\n }\n\n while(extraStudents--){\n auto [average,data] = pq.top();\n pq.pop();\n int pass = data.first;\n int total = data.second;\n sum -= (double)pass/total;\n pass++;\n total++;\n sum += (double)pass/total;\n pq.push({calcAverage(pass,total),{pass,total}});\n }\n return sum / classes.size();\n }\n};\n```\n\n---\n\n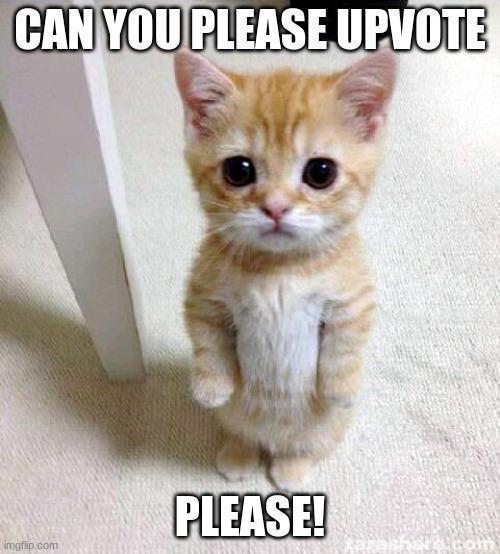\n | 1 | 0 | ['Array', 'Math', 'Binary Search', 'Greedy', 'Heap (Priority Queue)', 'C++'] | 0 |
maximum-average-pass-ratio | Very Optimized Solution (Beats 100%) With Explanation || Time Complexity = O(N logN) | very-optimized-solution-beats-100-with-e-2zm3 | Code | dhruvdangi03 | NORMAL | 2024-12-16T07:04:49.445183+00:00 | 2024-12-16T07:04:49.445183+00:00 | 9 | false | \n\n# Code\n```\nclass Solution {\n public double maxAverageRatio(int[][] classes, int extraStudents) {\n // First Store the number of classes in n.\n int n = classes.length;\n\n /* Now make a MaxHeap of Pairs.\n * A Pair will store the index and the change in the pass ratio of that class after adding a brilliant student.\n * And the Heap will be updated based on change. */\n PriorityQueue<Pair> heap = new PriorityQueue<>();\n\n // Now loop over classes and add them in heap.\n for (int i = 0; i < n; i++){\n // Calculate the amount of change\n double change = divide(classes[i][0] +1, classes[i][1] +1) \n - divide(classes[i][0], classes[i][1]);\n\n // Now make a new Pair and put change and index in it\n Pair pair = new Pair(change, i);\n \n // Now add the Pair in heap;\n heap.add(pair);\n }\n\n // Now Make a loop and Run it for the extraStudents numbers of time\n for (int i = 1; i <= extraStudents; i++) {\n Pair pair = heap.poll();\n\n // Update the Heap and also the Classes Array\n heap.add(update(classes, pair));\n }\n\n // Make a variable to store the Answer.\n double ans = 0;\n\n // Now loop over the classes array and calculate the sum of the pass ratios of the classes.\n for (int i = 0; i < n; i++)\n ans += divide(classes[i][0], classes[i][1]);\n\n // Now return the Average pass ratio.\n return ans / (double) n;\n }\n\n // This Method First casts the int into double then returns there division as double.\n double divide(int first, int sec) {\n return (double) first / (double) sec;\n }\n\n // This method Updates the Classes Array \n // And also returns the Updated Pair.\n Pair update(int[][] classes, Pair pair) {\n int idx = pair.idx;\n\n classes[idx][0]++;\n classes[idx][1]++;\n\n double change = divide(classes[idx][0] +1, classes[idx][1] +1)\n - divide(classes[idx][0], classes[idx][1]);\n\n return new Pair(change, idx);\n }\n}\n\n// This is the implementation of Pair Class\nclass Pair implements Comparable<Pair> {\n double change;\n int idx;\n\n Pair(double change, int idx){\n this.change = change;\n this.idx = idx;\n }\n\n // I have added this so the PriorityQueue will save the Pair in the order according to me.\n @Override\n public int compareTo(Pair other){\n if(this.change == other.change) return 0;\n\n return this.change > other.change ? -1 : 1;\n }\n}\n``` | 1 | 0 | ['Greedy', 'Heap (Priority Queue)', 'Java'] | 0 |
maximum-average-pass-ratio | 🚀Beats 99%, Python3, Heap, easy to understand🚀 | beats-99-python3-heap-easy-to-understand-16d9 | Complexity
Time complexity: O(nlogn)
Space complexity: O(n)
Code | mak2rae | NORMAL | 2024-12-15T22:43:32.410178+00:00 | 2024-12-15T22:43:32.410178+00:00 | 10 | false | # Complexity\n- Time complexity: O(nlogn)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python3 []\nclass Solution:\n def maxAverageRatio(self, classes: List[List[int]], extraStudents: int) -> float:\n def possible_gain(passed, total):\n return (passed + 1) / (total + 1) - passed / total\n\n max_heap = []\n pass_rate_sum = 0.0\n\n for passed, total in classes:\n pass_rate_sum += passed / total\n heapq.heappush(max_heap, (-possible_gain(passed, total), passed, total))\n\n for _ in range(extraStudents):\n current_gain, passed, total = heapq.heappop(max_heap)\n pass_rate_sum += -current_gain\n passed, total = passed + 1, total + 1\n heapq.heappush(max_heap, (-possible_gain(passed, total), passed, total))\n\n return pass_rate_sum / len(classes)\n``` | 1 | 0 | ['Heap (Priority Queue)', 'Python3'] | 0 |
maximum-average-pass-ratio | Explained briefly | explained-briefly-by-pranju-unxb | Intuition
The goal is to maximize the average pass ratio across all classes by strategically assigning the extra students. To achieve this, we should prioritize | pranju | NORMAL | 2024-12-15T18:24:14.400720+00:00 | 2024-12-15T18:24:14.400720+00:00 | 18 | false | **Intuition**\nThe goal is to maximize the average pass ratio across all classes by strategically assigning the extra students. To achieve this, we should prioritize adding students to the classes that yield the highest incremental gain in their pass ratios.\n\nThe incremental gain for a class depends on its current pass and total values. A max-heap can help us efficiently select the class with the largest gain after adding an extra student.\n\n**Approach**\nIncremental Gain Calculation:\nFor a class \u200Btotal students, adding one student updates the pass ratio. The incremental gain is:\ndouble diffinpassratio=(double)(pass+1)/(total+1)-(double)(pass)/(total);\nUsing a Max-Heap:\nExtract the class with the maximum gain, update its pass and total values after adding a student, and push the updated class back into the heap.\nAllocate Students:\nIteratively allocate all extra students to the classes that maximize the average pass ratio.\nCalculate Final Average Pass Ratio:\nAfter assigning all extra students, compute the average pass ratio by summing up the pass ratios of all classes and dividing by the number of classes.\n\n\n# Code\n```cpp []\nclass Solution {\npublic:\n double maxAverageRatio(vector<vector<int>>& classes, int extraStudents) {\n priority_queue<pair<double,pair<int,int>>>pq;//max heap of diff and class index\n for(int i=0;i<classes.size();i++)\n {\n int pass=classes[i][0];\n int total=classes[i][1];\n double diffinpassratio=(double)(pass+1)/(total+1)-(double)(pass)/(total);\n pq.push({diffinpassratio,{pass,total}});\n }\n while(extraStudents--)\n {\n int pass=pq.top().second.first;\n int total=pq.top().second.second;\n pass++;\n total++;\n pq.pop();\n double newdiff=(double)(pass+1)/(total+1)-(double)(pass)/(total);\n pq.push({newdiff,{pass,total}});\n }\n double totalaverage=0;\n while(!pq.empty())\n {\n int pass=pq.top().second.first;\n int total=pq.top().second.second;\n pq.pop();\n totalaverage+=(double)(pass)/(total);\n }\n return totalaverage/classes.size();\n }\n};\n``` | 1 | 0 | ['Heap (Priority Queue)', 'C++'] | 0 |
maximum-average-pass-ratio | C# Solution for Maximum Average Pass Ratio Problem | c-solution-for-maximum-average-pass-rati-2eoo | IntuitionThe goal is to maximize the average pass ratio across all classes after assigning a number of extra students. To achieve this:
• The pass ratio improve | Aman_Raj_Sinha | NORMAL | 2024-12-15T17:49:04.220500+00:00 | 2024-12-15T17:49:04.220500+00:00 | 20 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe goal is to maximize the average pass ratio across all classes after assigning a number of extra students. To achieve this:\n\u2022\tThe pass ratio improvement for each class is calculated when adding an extra passing student.\n\u2022\tAssign the extra students to the classes where they will have the greatest impact on improving the average.\n\u2022\tThis requires identifying the class with the maximum improvement efficiently after each assignment, which can be managed using a priority queue (or SortedSet in C#).\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1.\tPass Ratio Improvement Calculation:\n\t\u2022\tThe improvement is calculated as the difference between the current pass ratio and the new pass ratio after adding one extra passing student:\n\n{Improvement} = frac{{passi} + 1}{{totali} + 1} - frac{{passi}}{{totali}}\n\n\u2022\tThis ensures we always add an extra student to the class that yields the greatest improvement.\n2.\tData Structure:\n\t\u2022\tUse a priority queue (via SortedSet in C#) to efficiently retrieve and update the class with the maximum improvement.\n3.\tSteps:\n\t\u2022\tInitialization: Calculate the improvement for all classes and store them in the priority queue.\n\t\u2022\tExtra Student Allocation:\n\t\u2022\tExtract the class with the maximum improvement.\n\t\u2022\tAssign one extra passing student to this class and update its improvement.\n\t\u2022\tReinsert the updated class back into the priority queue.\n\t\u2022\tFinal Average Calculation:\n\t\u2022\tAfter all extra students are assigned, calculate the total average pass ratio by summing the pass ratios of all classes.\n4.\tTie-Breaking:\n\t\u2022\tIn case two classes have the same improvement, sort them by their index to ensure deterministic behavior.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n1.\tInitialization:\n\t\u2022\tCalculating the initial improvement for all classes and inserting them into the priority queue: O(n . log n)\n\n2.\tExtra Student Assignment:\n\t\u2022\tFor each of the extraStudents, extracting and reinserting the class with the maximum improvement takes: O(log n)\n\n\t\u2022\tFor k = {extraStudents}, this results in: O(k . log n)\n\n3.\tFinal Calculation:\n\t\u2022\tCalculating the total average pass ratio involves a single pass over all classes: O(n)\n\nTotal Time Complexity: O((n + k) . log n)\n\nWhere n is the number of classes and k is the number of extra students.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n1.\tPriority Queue:\n\t\u2022\tStores up to n entries, one for each class: O(n)\n\n2.\tAuxiliary Space:\n\t\u2022\tA constant amount of space is used for intermediate calculations.\n\nTotal Space Complexity: O(n)\n\n# Code\n```csharp []\npublic class Solution {\n public double MaxAverageRatio(int[][] classes, int extraStudents) {\n var pq = new SortedSet<(double improvement, int index)>(\n Comparer<(double improvement, int index)>.Create((a, b) =>\n a.improvement == b.improvement ? a.index.CompareTo(b.index) : b.improvement.CompareTo(a.improvement))\n );\n for (int i = 0; i < classes.Length; i++) {\n double improvement = GetImprovement(classes[i][0], classes[i][1]);\n pq.Add((improvement, i));\n }\n while (extraStudents > 0) {\n var (maxImprovement, index) = pq.Min;\n pq.Remove(pq.Min);\n classes[index][0]++;\n classes[index][1]++;\n double newImprovement = GetImprovement(classes[index][0], classes[index][1]);\n pq.Add((newImprovement, index));\n\n extraStudents--;\n }\n\n double totalRatio = 0;\n foreach (var c in classes) {\n totalRatio += (double)c[0] / c[1];\n }\n\n return totalRatio / classes.Length;\n }\n private double GetImprovement(int passi, int totali) {\n double currentRatio = (double)passi / totali;\n double newRatio = (double)(passi + 1) / (totali + 1);\n return newRatio - currentRatio;\n }\n}\n``` | 1 | 0 | ['C#'] | 0 |
maximum-average-pass-ratio | Java Solution for Maximum Average Pass Ratio Problem | java-solution-for-maximum-average-pass-r-onb5 | IntuitionThe problem requires maximizing the average pass ratio across multiple classes by distributing a limited number of extra students. The key observation | Aman_Raj_Sinha | NORMAL | 2024-12-15T17:40:37.704200+00:00 | 2024-12-15T17:40:37.704200+00:00 | 37 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem requires maximizing the average pass ratio across multiple classes by distributing a limited number of extra students. The key observation is that the increase in pass ratio diminishes as more students are added to a class. Therefore, we should prioritize assigning students to the class where the addition results in the largest increase in pass ratio.\n\nTo achieve this, a greedy approach is used, where a priority queue (max heap) is leveraged to repeatedly pick the class that benefits the most from an extra student.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1.\tInitial Calculation:\n\t\u2022\tFor each class, calculate the potential improvement in pass ratio by adding one student.\n\t\u2022\tStore these improvements in a max heap along with the current number of passing and total students.\n2.\tDistribute Extra Students:\n\t\u2022\tWhile there are extra students to assign:\n\t\u2022\tExtract the class with the highest improvement from the heap.\n\t\u2022\tAdd an extra student to this class (i.e., increment passi and totali).\n\t\u2022\tRecalculate the new improvement and push the updated class back into the heap.\n3.\tCompute Final Average:\n\t\u2022\tOnce all extra students are assigned, calculate the average pass ratio by summing up the pass ratios of all classes and dividing by the number of classes.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n1.\tBuilding the Heap:\n\t\u2022\tFor n classes, inserting them into the heap takes O(n . log n).\n2.\tAssigning Extra Students:\n\t\u2022\tFor each of the extraStudents, we:\n\t\u2022\tExtract the maximum improvement from the heap (O(log n)).\n\t\u2022\tRecalculate the improvement and push it back into the heap (O(log n)).\n\t\u2022\tThis step takes O(extraStudents . log n).\n3.\tFinal Computation:\n\t\u2022\tIterating over the heap to compute the final pass ratio takes O(n).\n\nTotal Time Complexity: O((n + extraStudents) . log n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n1.\tHeap Storage:\n\t\u2022\tThe heap stores up to n classes, where each entry includes the improvement, passing students, and total students.\n\t\u2022\tSpace required: O(n).\n2.\tAuxiliary Space:\n\t\u2022\tA few variables are used to store intermediate results, so additional space usage is negligible.\n\nTotal Space Complexity: O(n)\n\n# Code\n```java []\nclass Solution {\n public double maxAverageRatio(int[][] classes, int extraStudents) {\n PriorityQueue<double[]> maxHeap = new PriorityQueue<>((a, b) -> Double.compare(b[0], a[0]));\n for (int[] clazz : classes) {\n int passi = clazz[0];\n int totali = clazz[1];\n double improvement = improvement(passi, totali);\n maxHeap.offer(new double[]{improvement, passi, totali});\n }\n \n while (extraStudents > 0) {\n double[] top = maxHeap.poll();\n int passi = (int) top[1];\n int totali = (int) top[2];\n \n passi++;\n totali++;\n extraStudents--;\n \n double newImprovement = improvement(passi, totali);\n maxHeap.offer(new double[]{newImprovement, passi, totali});\n }\n double totalRatio = 0.0;\n while (!maxHeap.isEmpty()) {\n double[] clazz = maxHeap.poll();\n int passi = (int) clazz[1];\n int totali = (int) clazz[2];\n totalRatio += (double) passi / totali;\n }\n \n return totalRatio / classes.length;\n }\n private double improvement(int passi, int totali) {\n return ((double) (passi + 1) / (totali + 1)) - ((double) passi / totali);\n }\n}\n``` | 1 | 0 | ['Java'] | 0 |
maximum-average-pass-ratio | Java solution with detailed explanation | Solved using Max-Heap | Time complexity: O(nlogn) | java-solution-with-detailed-explanation-yzjbx | Problem
Each class has some students who passed the exam and some who didn't.
You want to improve the school's average pass rate:
Pass rate of a class = Studen | SaiGopalChalla | NORMAL | 2024-12-15T17:36:55.737445+00:00 | 2024-12-15T17:36:55.737445+00:00 | 21 | false | # Problem\n\n1. Each class has some students who passed the exam and some who didn\'t.\n2. You want to improve the school\'s average pass rate:\n - Pass rate of a class = Students who passed \xF7 Total students in that class.\n - Average pass rate = Sum of pass rates of all classes \xF7 Number of classes.\n3. You have some extra "super students" who will always pass the exam if they join a class.\n4. You can put these extra students into any class, one at a time.\n5. Your job is to decide which classes to put these extra students into, so the average pass rate becomes as high as possible.\n\n# Intution\n\n- Adding students to a class increases its pass rate, but:\n - The first extra student you add makes the biggest improvement in the pass rate.\n - The more students you add to the same class, the less improvement you see.\n- So, to get the best results, you should:\n - Always give the next extra student to the class where it helps the most.\n\n# Plan \n\nTo solve this step by step:\n\n1. Find out how much each class improves if you add one extra student.\n2. Always pick the class with the biggest improvement and give it one extra student.\n3. Repeat this until you\'ve assigned all the extra students.\n4. Calculate the average pass rate after all extra students are assigned.\n\n# Measuring "Improvement"?\nThe improvement in the pass rate for a class if you add one extra student is:\n\n\n\n- This tells you how much better the class\'s pass rate will be after adding a student.\n- We call this "improvement" the gain.\n# Solving with a Max-Heap\nNow, to assign the extra students smartly:\n\n1. Make a list of all classes and calculate their gain for adding one student.\n2. Put all these gains in a "max-heap." A max-heap is a structure where you can always quickly find the class with the highest gain.\n3. For each extra student:\n - Take the class with the highest gain from the heap.\n - Add the student to that class.\n - Recalculate the new gain for that class and put it back in the heap.\n4. After all extra students are assigned, calculate the final average pass rate.\n# Complexity\n- Time complexity: $$O(nlogn)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```java []\nclass Solution {\n public double maxAverageRatio(int[][] classes, int extraStudents) {\n // Max-Heap to store the classes based on the gain in pass ratio\n PriorityQueue<double[]> maxHeap = new PriorityQueue<>(\n (a, b) -> Double.compare(b[0], a[0]) \n );\n\n // fill the heap with initial values\n for (int[] c : classes) {\n int passi = c[0], totali = c[1];\n double gain = calculateGain(passi, totali);\n maxHeap.offer(new double[] {gain, passi, totali});\n }\n\n // Assign extra students\n while (extraStudents > 0) {\n // Extract the class with the maximum gain\n double[] top = maxHeap.poll();\n double gain = top[0];\n int passi = (int) top[1];\n int totali = (int) top[2];\n\n // Update the class with additional student\n passi++;\n totali++;\n\n // Recompute the gain and reinsert into the heap\n double newGain = calculateGain(passi, totali);\n maxHeap.offer(new double[] {newGain, passi, totali});\n\n // Decrement extra students\n extraStudents--;\n }\n\n // Compute the final average pass ratio\n double totalRatio = 0.0;\n for (double[] entry : maxHeap) {\n int passi = (int) entry[1];\n int totali = (int) entry[2];\n totalRatio += (double) passi / totali;\n }\n\n return totalRatio / classes.length;\n }\n\n // Helper method to compute the gain of adding one student\n private double calculateGain(int passi, int totali) {\n double currentRatio = (double) passi / totali;\n double newRatio = (double) (passi + 1) / (totali + 1);\n return newRatio - currentRatio;\n }\n}\n``` | 1 | 0 | ['Math', 'Greedy', 'Heap (Priority Queue)', 'Java'] | 1 |
maximum-average-pass-ratio | Easy Solution, Priority Queue | easy-solution-priority-queue-by-dotuangv-5qiu | IntuitionApproachComplexity
Time complexity: O(nlog(n))
Space complexity: O(n)
Code | dotuangv | NORMAL | 2024-12-15T17:31:43.926850+00:00 | 2024-12-15T17:31:43.926850+00:00 | 15 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(nlog(n))\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n double maxAverageRatio(vector<vector<int>>& classes, int extraStudents) {\n priority_queue<pair<double, pair<int, int>>> pq;\n for(int i = 0; i < classes.size(); i++){\n pq.push({(classes[i][0] + 1)*1.0 /(classes[i][1] + 1) - classes[i][0]*1.0/classes[i][1], {classes[i][0], classes[i][1]}});\n }\n while(extraStudents--){\n auto [a, b] = pq.top().second;\n pq.pop();\n pq.push({(a + 2)*1.0/(b + 2) - (a + 1)*1.0/(b + 1), {a + 1, b + 1}});\n }\n double ans = 0;\n while(!pq.empty()){\n auto [a, b] = pq.top().second;\n ans += a * 1.0 / b;\n pq.pop();\n }\n return ans/classes.size();\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
maximum-average-pass-ratio | Simple and Understand the maxAverageRatio | simple-and-understand-the-maxaveragerati-n74u | ** Proper Explanation for Each steps**Step 1: Priority Queue Initialization
A priority queue is created to store classes.
Classes are sorted based on how much t | user6465gj | NORMAL | 2024-12-15T17:24:47.012526+00:00 | 2024-12-15T17:24:47.012526+00:00 | 36 | false | ### ** Proper Explanation for Each steps**\n\n### **Step 1: Priority Queue Initialization**\n```java\nPriorityQueue<int[]> pq = new PriorityQueue<>((a, b) -> Double.compare(diff(b), diff(a)));\n```\n- A **priority queue** is created to store classes.\n- Classes are sorted based on how much their pass rate would improve if one extra student is added.\n- The class with the **highest improvement** comes first in the queue.\n\n---\n\n### **Step 2: Add All Classes to the Queue**\n```java\nfor (int[] cl : classes) pq.add(cl);\n```\n- Each class (represented as an array of `[pass, total]`) is added to the priority queue for evaluation.\n\n---\n\n### **Step 3: Distribute Extra Students**\n```java\nfor (int i = 0; i < extraStudents; i++) {\n int[] next = pq.poll();\n next[0]++;\n next[1]++;\n pq.add(next);\n}\n```\n- For each extra student:\n - **Poll** the class with the highest improvement.\n - **Update** the class by adding one more pass and one more total student.\n - **Re-add** the updated class back to the queue.\n\n---\n\n### **Step 4: Calculate the Final Average Pass Rate**\n```java\ndouble sum = 0.0;\nwhile (!pq.isEmpty()) {\n int[] next = pq.poll();\n sum += (double) next[0] / next[1];\n}\nreturn sum / classes.length;\n```\n- After distributing all students:\n - **Calculate** the pass rate for each class.\n - **Sum** up all the pass rates.\n - **Return** the average pass rate across all classes.\n\n---\n\n### **Helper Function: `diff`**\n```java\nprivate double diff(int[] a) {\n return (double)(a[0] + 1) / (a[1] + 1) - (double) a[0] / a[1];\n}\n```\n- This function calculates the **improvement in pass rate** if one extra student is added to a class. \n\n---\n\nThis entire process ensures the **extra students** are distributed in a way that maximizes the overall pass rate across all classes.\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n\n# Code\n```java []\nimport java.util.PriorityQueue;\n\nclass Solution {\n public double maxAverageRatio(int[][] classes, int extraStudents) {\n PriorityQueue<int[]> pq = new PriorityQueue<>((a, b) -> Double.compare(diff(b), diff(a)));\n for (int[] cl : classes) pq.add(cl);\n\n for (int i = 0; i < extraStudents; i++) {\n int[] next = pq.poll();\n next[0]++;\n next[1]++;\n pq.add(next);\n }\n\n double sum = 0.0;\n while (!pq.isEmpty()) {\n int[] next = pq.poll();\n sum += (double) next[0] / next[1];\n }\n return sum / classes.length;\n }\n\n private double diff(int[] a) {\n return (double)(a[0] + 1) / (a[1] + 1) - (double) a[0] / a[1];\n }\n}\n\n``` | 1 | 0 | ['Java'] | 0 |
maximum-average-pass-ratio | Intuition building from brute to optimal approach. T.C --> O(extraStudents x logn) | intuition-building-from-brute-to-optimal-xijz | ApproachInitially, tried solving this question using a greedy approach by adding 1 extra student to the class having the smallest pass-ratio, which is students/ | iamarunaksha | NORMAL | 2024-12-15T15:00:46.454429+00:00 | 2024-12-15T15:02:05.437297+00:00 | 30 | false | # Approach\n\nInitially, tried solving this question using a greedy approach by adding 1 extra student to the class having the smallest pass-ratio, which is students/totalNoOfStudents in a class, and repeating the same process till there exists extraStudents.\n\nBut, this approach fails in the very first example given here on Leetcode.\n\nTherefore, correct approach for this problem is to consider the difference between the new pass ratio by adding 1 extra student to a class & current pass ratio (existing pass-ratio).\n\nSolutions, given here is based on considering the delta/difference between the new pass ratio & existing pass ratio.\n\n# Complexity\n- Time complexity:\nFor 1st solution -> O(2n) + O(extraStudents x n) == O(n + (extraStudents x n))\n\nFor 2nd (Optimal) solution -> O(nlogn) + O(extraStudents x logn) + O(n) == O(nlogn + (extraStudents x logn) + n)\n\n- Space complexity:\nFor both solutions -> O(n)\n\n# Code\nKind of brute force based on difference of pass ratios. Gives TLE\n```cpp []\nclass Solution {\npublic:\n double maxAverageRatio(vector<vector<int>>& classes, int extraStudents) {\n \n int n = classes.size(); //T.C --> O(2n) + O(extraStudents x n) || S.C --> O(n)\n\n vector<double> pass_ratio(n);\n\n for(int i=0; i<n; i++) { //O(n)\n\n double pr = (classes[i][0] * 1.0)/classes[i][1];\n\n pass_ratio[i] = pr;\n }\n\n while(extraStudents--) { //O(extraStudents x n)\n\n double maxNewPR = INT_MIN, maxNewPRDiff = INT_MIN;\n\n int idx = 0;\n\n for(int i=0; i<n; i++) {\n\n double newPR = ((classes[i][0] + 1) * 1.0)/(classes[i][1] + 1);\n\n double diff = newPR - pass_ratio[i];\n\n if(diff > maxNewPRDiff) {\n\n maxNewPR = newPR;\n\n maxNewPRDiff = diff;\n\n idx = i;\n }\n }\n\n pass_ratio[idx] = maxNewPR;\n\n classes[idx][0]++;\n classes[idx][1]++;\n }\n\n double sum = 0;\n\n for(auto &it : pass_ratio) //O(n)\n sum += it;\n \n double avg = (sum * 1.0) / n;\n\n return avg;\n }\n};\n\n\n```\n\nOptimal Approach using max heap.\n```cpp []\n#define pdd pair<double, double>\n\nclass Solution {\npublic:\n double maxAverageRatio(vector<vector<int>>& classes, int extraStudents) {\n \n int n = classes.size(); //T.C --> O(nlogn + (extraStudents x logn) + n) || S.C --> O(n)\n\n priority_queue<pdd> maxHeap; //{delta, idx}\n\n //O(nlogn)\n for(int i=0; i<n; i++) { //Pre-processing for 1 extra student allotted to every class\n\n double pr = (classes[i][0] * 1.0)/classes[i][1];\n\n double newPR = ((classes[i][0] + 1) * 1.0)/(classes[i][1] + 1);\n\n double delta = newPR - pr;\n\n maxHeap.push({delta, i});\n }\n\n while(extraStudents--) { //O(extraStudents x log(n))\n\n auto curr = maxHeap.top();\n maxHeap.pop(); //log(n)\n\n double delta = curr.first;\n int idx = curr.second;\n\n //Updating the classes for max delta idx\n classes[idx][0]++;\n classes[idx][1]++;\n\n //Now adding 1 extra student to idx and pushing new delta to max heap\n double currPRForIdx = (classes[idx][0] * 1.0)/classes[idx][1];\n\n double newPRForIdx = ((classes[idx][0] + 1) * 1.0)/(classes[idx][1] + 1); //After adding 1 extra student\n\n double newDelta = newPRForIdx - currPRForIdx;\n\n maxHeap.push({newDelta, idx}); //log(n)\n }\n\n double sum = 0;\n\n for(int i=0; i<n; i++) { //O(n)\n\n double pr = (classes[i][0] * 1.0)/classes[i][1];\n\n sum += pr;\n }\n \n double avg = (sum * 1.0) / n;\n\n return avg;\n }\n};\n``` | 1 | 0 | ['Array', 'Greedy', 'Heap (Priority Queue)', 'C++'] | 0 |
maximum-average-pass-ratio | Using PriorityQueue || O(K logn) | using-priorityqueue-ok-logn-by-satwikpre-6l73 | IntuitionUse PriorityQueue to get next best pass, total after using each of extraStudent.ApproachPriorityQueue is used to store nextPassRatioDiff i.e. (pass + 1 | satwikprem | NORMAL | 2024-12-15T14:53:35.462438+00:00 | 2024-12-15T14:53:35.462438+00:00 | 57 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nUse PriorityQueue to get next best pass, total after using each of extraStudent.\n\n---\n\n---\n\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nPriorityQueue is used to store nextPassRatioDiff i.e. (pass + 1) / (total + 1) - (pass / total). This tells us what will be next best class to add new student.\n\n---\n\n---\n\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n O(n) + O(K logn) + O(n) = O(K logn)\n**K = 2** times the extraStudents.\nAnd, **logn** for offering or polling from the heap.\n\n---\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n O(n) \nFor PriorityQueue.\n\n---\n\n\n---\n\n\n# Code\n```java []\nclass Solution {\n public static double nextPassRatioDiff(double pass, double total){\n return (pass + 1) / (total + 1) - (pass / total);\n }\n\n\n public double maxAverageRatio(int[][] classes, int extraStudents) {\n int n = classes.length;\n PriorityQueue<double[] > maxHeap = new PriorityQueue<>((a, b) -> Double.compare(b[0], a[0]));\n //entry = {nextPassRatioDiff, pass, total}\n for(int[] arr: classes){\n int pass = arr[0];\n int total = arr[1];\n double next = nextPassRatioDiff(pass, total);\n maxHeap.offer(new double[]{next, pass, total});\n }\n while(extraStudents > 0){\n double[] top= maxHeap.poll();\n double pass = top[1] + 1;\n double total = top[2] + 1;\n double next = nextPassRatioDiff(pass, total);\n maxHeap.offer(new double[]{next, pass, total});\n extraStudents--;\n }\n double totalPassRatio = 0;\n while(!maxHeap.isEmpty()){\n double[] arr = maxHeap.poll();\n double pass = arr[1];\n double total = arr[2];\n totalPassRatio += (pass / total);\n }\n return totalPassRatio / n;\n }\n\n}\n``` | 1 | 0 | ['Array', 'Greedy', 'Heap (Priority Queue)', 'Java'] | 0 |
maximum-average-pass-ratio | Java Solution BEATS 77% | java-solution-beats-77-by-yogesh__jswl-j6ga | Code | yogesh__jswl_ | NORMAL | 2024-12-15T14:42:48.754958+00:00 | 2024-12-15T14:42:48.754958+00:00 | 29 | false | # Code\n```java []\nclass Pair{\n double ratio;\n int index;\n Pair(double ratio,int index)\n {\n this.ratio=ratio;\n this.index=index;\n }\n}\n\nclass Solution {\n public double maxAverageRatio(int[][] classes, int extraStudents) {\n PriorityQueue<Pair> pq=new PriorityQueue<>((a,b)->Double.compare(b.ratio,a.ratio));\n int n=classes.length;\n for(int i=0;i<n;i++)\n {\n double r=(classes[i][0]*1.0)/(classes[i][1]*1.0);\n double r2=((classes[i][0]+1)*1.0)/((classes[i][1]+1)*1.0);\n pq.add(new Pair(r2-r,i));\n }\n while(extraStudents>0)\n {\n int ind=pq.peek().index;\n pq.remove();\n classes[ind][0]++;\n classes[ind][1]++;\n double r=(classes[ind][0]*1.0)/(classes[ind][1]*1.0);\n double r2=((classes[ind][0]+1)*1.0)/((classes[ind][1]+1)*1.0);\n pq.add(new Pair(r2-r,ind));\n extraStudents--;\n }\n double ans=0;\n for(int i=0;i<n;i++)\n {\n ans+=(classes[i][0]*1.0)/(classes[i][1]*1.0);\n }\n return ans/n;\n }\n}\n``` | 1 | 0 | ['Array', 'Math', 'Greedy', 'Memoization', 'Queue', 'Sorting', 'Heap (Priority Queue)', 'Counting', 'Java'] | 0 |
base-7 | [Java/C++/Python] Iteration and Recursion | javacpython-iteration-and-recursion-by-l-l6ji | Iterative Solution\n\nPython\n\n def convertToBase7(self, num):\n n, res = abs(num), \'\'\n while n:\n res = str(n % 7) + res\n | lee215 | NORMAL | 2017-02-12T21:13:24.307000+00:00 | 2018-10-26T04:13:06.156261+00:00 | 18,268 | false | ## Iterative Solution\n\n**Python**\n```\n def convertToBase7(self, num):\n n, res = abs(num), \'\'\n while n:\n res = str(n % 7) + res\n n /= 7\n return \'-\' * (num < 0) + res or "0"\n```\n\n\n\n## Recursive Solution\n\n**Java:**\n```\n public String convertToBase7(int n) {\n if (n < 0) return "-" + convertToBase7(-n);\n if (n < 7) return Integer.toString(n);\n return convertToBase7(n / 7) + Integer.toString(n % 7);\n }\n```\n\n**C++:**\n```\n string convertToBase7(int n) {\n if (n < 0) return "-" + convertToBase7(-n);\n if (n < 7) return to_string(n);\n return convertToBase7(n / 7) + to_string(n % 7);\n }\n```\n\n**Python:**\n```\n def convertToBase7(self, n):\n if n < 0: return \'-\' + self.convertToBase7(-n)\n if n < 7: return str(n)\n return self.convertToBase7(n // 7) + str(n % 7)\n```\n | 133 | 4 | [] | 13 |
base-7 | Simple Java, oneliner Ruby | simple-java-oneliner-ruby-by-stefanpochm-mr0a | Java recursion:\n\n public String convertTo7(int num) {\n if (num < 0)\n return '-' + convertTo7(-num);\n if (num < 7)\n | stefanpochmann | NORMAL | 2017-02-12T06:19:14.691000+00:00 | 2018-10-11T14:40:45.049851+00:00 | 13,041 | false | **Java recursion:**\n\n public String convertTo7(int num) {\n if (num < 0)\n return '-' + convertTo7(-num);\n if (num < 7)\n return num + "";\n return convertTo7(num / 7) + num % 7;\n }\n\n**Ruby oneliner:**\n\n```\ndef convert_to7(num)\n num.to_s(7)\nend\n``` | 55 | 1 | [] | 8 |
base-7 | Verbose Java Solution | verbose-java-solution-by-shawngao-kvf9 | Just keep dividing the current number by 7...\n\n\npublic class Solution {\n public String convertTo7(int num) {\n if (num == 0) return "0";\n | shawngao | NORMAL | 2017-02-12T04:31:52.102000+00:00 | 2017-02-12T04:31:52.102000+00:00 | 8,455 | false | Just keep dividing the current number by 7...\n\n```\npublic class Solution {\n public String convertTo7(int num) {\n if (num == 0) return "0";\n \n StringBuilder sb = new StringBuilder();\n boolean negative = false;\n \n if (num < 0) {\n negative = true;\n }\n while (num != 0) {\n sb.append(Math.abs(num % 7));\n num = num / 7;\n }\n \n if (negative) {\n sb.append("-");\n }\n \n return sb.reverse().toString();\n }\n}\n``` | 32 | 0 | [] | 10 |
base-7 | 3-liner C++ to build string backward + 1-liner recursive solutions | 3-liner-c-to-build-string-backward-1-lin-eqg6 | Iterative Version: Note that the input n is guaranteed to be in range of [-1e7, 1e7] by the problem, so abs(n) won't overflow since n cannot be INT_MIN.\n\n | zzg_zzm | NORMAL | 2017-02-13T20:52:18.191000+00:00 | 2018-09-29T17:47:17.358331+00:00 | 5,651 | false | **Iterative Version:** Note that the input `n` is guaranteed to be in range of [-1e7, 1e7] by the problem, so `abs(n)` won't overflow since `n` cannot be `INT_MIN`.\n```\n string convertToBase7(int n) {\n int x = abs(n); string res;\n do res = to_string(x%7)+res; while(x/=7);\n return (n>=0? "" : "-") + res;\n }\n```\n**Recursive Version:**\n```\n string convertToBase7(int n) {\n return n>=0? n>=7? convertToBase7(n/7)+to_string(n%7) : to_string(n) : '-'+convertToBase7(-n);\n }\n``` | 22 | 4 | ['String', 'C++'] | 5 |
base-7 | my java solution w/o string helper | my-java-solution-wo-string-helper-by-hol-53ay | \npublic class Solution {\n public String convertToBase7(int num) {\n int base = 1, result = 0;\n while (num != 0) {\n result += bas | holyghost | NORMAL | 2017-02-17T15:29:34.187000+00:00 | 2018-09-30T17:05:01.265573+00:00 | 1,432 | false | ```\npublic class Solution {\n public String convertToBase7(int num) {\n int base = 1, result = 0;\n while (num != 0) {\n result += base * (num % 7);\n num /= 7;\n base *= 10;\n }\n return String.valueOf(result);\n }\n}\n``` | 22 | 1 | [] | 0 |
base-7 | Python 3 || 7 lines, modular arithmetic || T/S: 99% / 99% | python-3-7-lines-modular-arithmetic-ts-9-2q5b | \nclass Solution:\n def convertToBase7(self, num: int) -> str:\n\n if num == 0: return \'0\'\n\n ans, n = \'\', abs(num)\n\n while n:\n | Spaulding_ | NORMAL | 2023-06-16T16:29:10.380725+00:00 | 2024-06-23T00:49:20.432309+00:00 | 1,856 | false | ```\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n\n if num == 0: return \'0\'\n\n ans, n = \'\', abs(num)\n\n while n:\n n, m = divmod(n,7)\n ans+=str(m)\n\n if num < 0: ans+= \'-\'\n\n return ans[::-1]\n```\n[https://leetcode.com/problems/base-7/submissions/587231651/](https://leetcode.com/problems/base-7/submissions/587231651/)\n\nI could be wrong, but I think that time complexity is *O*(log*N*) and space complexity is *O*(log*N*), in which *N* ~`int(num)`. | 14 | 0 | ['Python3'] | 1 |
base-7 | Python - Short and Simple with Explanation and Resources | python-short-and-simple-with-explanation-crq5 | Explanation below code snippet - hope this helps!\n\nIf you are confused about the conversion process from base 10 (numbers we are used to) to base 7 read this: | rowe1227 | NORMAL | 2020-06-05T20:00:37.769068+00:00 | 2020-06-05T20:04:12.938948+00:00 | 1,932 | false | Explanation below code snippet - hope this helps!\n\nIf you are confused about the conversion process from base 10 (numbers we are used to) to base 7 read this:\n\nhttps://runestone.academy/runestone/books/published/pythonds/BasicDS/ConvertingDecimalNumberstoBinaryNumbers.html\n\n```\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n \n if not num:\n return \'0\'\n \n sign = num < 0\n num = abs(num)\n \n stack = []\n while num:\n stack.append(str(num % 7))\n num = num // 7\n \n return \'-\'*sign + \'\'.join(stack[::-1])\n```\n\nThe first two lines simply handle the edge case that num is zero. \n\nNext, we check to see if num is negative, if it is, lets make it positive using abs, but remember that it was negative with the variable sign. (We will take the original sign of the number into account in the return statement.)\n\nNext, iteratively divide num by 7 and at each iteration, keep track of the remainder in a stack. These remainders are the digits that will go into our result. However, they are in the reverse order of what we need. \n\nOnce num equals zero, the while loop will break. Join all of the elements in the stack together in reverse order, and add the sign back in. If the number was positive, then ```sign = False``` and ```"-" * False = ""``` so the negative sign will not be included in the result. \n\nSide note: The list we use to store the digits was named stack because using:\n\n```\nwhile stack:\n\tres += stack.pop()\n```\n\nwould achieve the same outcome as ```\'\'.join(stack[::-1])``` | 14 | 0 | [] | 2 |
base-7 | 1 LINNER SOLUTION (EK LINE KA JUGAD) BY TRIPPY THE CODER :) | 1-linner-solution-ek-line-ka-jugad-by-tr-9koj | Intuition\nTO MAKE SOLUTION CRISP AND TO THE POINT.\n\n# Approach\nSS APPROACH\nJUST SMILE AND SOLVE\n\n# Complexity\nNOTHING COMPLEX\n\n# HUMBLE REQUEST\nPLEAS | gurnainwadhwa | NORMAL | 2023-03-24T12:05:11.357646+00:00 | 2023-03-24T12:05:11.357678+00:00 | 1,214 | false | # Intuition\nTO MAKE SOLUTION CRISP AND TO THE POINT.\n\n# Approach\nSS APPROACH\nJUST SMILE AND SOLVE\n\n# Complexity\nNOTHING COMPLEX\n\n# HUMBLE REQUEST\nPLEASE UPVOTE IF I HELPED YOU\nTHANK YOU.\nKEEP IT UP.\nLOVE BY TRIPPY THE CODER\n\u2764\uFE0F\n\n# Code\n```\nimport java.math.*;\nclass Solution {\n public String convertToBase7(int num) {\n BigInteger m = new BigInteger(""+num);\n return m.toString(7);\n }\n}\n``` | 11 | 0 | ['String', 'Java'] | 2 |
base-7 | JS One-liner: simple is the best | js-one-liner-simple-is-the-best-by-hbjor-4qes | ```\n/*\n * @param {number} num\n * @return {string}\n /\nvar convertToBase7 = function(num) {\n return num.toString(7);\n}; | hbjorbj | NORMAL | 2020-03-24T17:57:16.263464+00:00 | 2020-03-24T17:57:16.263518+00:00 | 627 | false | ```\n/**\n * @param {number} num\n * @return {string}\n */\nvar convertToBase7 = function(num) {\n return num.toString(7);\n}; | 11 | 5 | ['JavaScript'] | 1 |
base-7 | python - easy to understand (with comments) | python-easy-to-understand-with-comments-tzbgc | \nclass Solution:\n def convertToBase7(self, num: int) -> str:\n\t\t# Store each digit in array as we iterate\n s = []\n\t\n\t\t# Base case where whil | jackkelley | NORMAL | 2019-06-24T07:53:18.940178+00:00 | 2019-06-24T07:53:18.940219+00:00 | 1,521 | false | ```\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n\t\t# Store each digit in array as we iterate\n s = []\n\t\n\t\t# Base case where while loop never runs\n if num == 0:\n return "0"\n\t\t\n\t\t# If num is negative, make it positive so the calculation is easier\n\t\t# Add a boolean flag\t\n\t\tf = False\n if num < 0:\n f = True\n num = -num\n\t\t\n\t\t# While our division (into base 7) requires more steps to finish adding digits\n while num > 0:\n\t\t\t# Append the next digit to the array\n\t\t\t# This begins from the smallest digit (rightmost)\n\t\t\t# Thus, we must reverse the string after we finish\n\t\t\t# (Last computation => Largest digit)\n\t\t\t\n s.append(str(num%7))\n\t\t\t\n\t\t\t# Perform the actual division by our base, 7, on num\n num //= 7\n\t\t\n\t\t# If it is negative add a \'-\', then join into a string and return\n\t\t# Again, s is reversed in order to place larger digits first\n return (\'-\' if f else \'\') +\'\'.join(s[::-1])\n``` | 10 | 0 | ['Python', 'Python3'] | 0 |
base-7 | 2 Solutions ( 0 ms & 1ms) || 2 Different Approaches Explained || Super Easy || Java😊 | 2-solutions-0-ms-1ms-2-different-approac-th24 | Explaination for Approach 1 :\n\nThe equation calculation formula for 100 number to 7 is like this below.\n7|100\n7|14|2\n7|2|0\n7|2|2\nAns:202(base 7)\n\n# Req | N7_BLACKHAT | NORMAL | 2023-01-02T05:53:35.077393+00:00 | 2023-01-02T05:53:35.077439+00:00 | 1,148 | false | # Explaination for Approach 1 :\n```\nThe equation calculation formula for 100 number to 7 is like this below.\n7|100\n7|14|2\n7|2|0\n7|2|2\nAns:202(base 7)\n```\n# Request \uD83D\uDE0A :\n```\nIf you find these solutions easy to understand and helpful, then\nPlease Upvote\uD83D\uDC4D\uD83D\uDC4D (Scroll Down for fastest [0 ms] approach).\n```\n# Approach 1 : 1ms Solution\n```\nclass Solution \n{\n public String convertToBase7(int num) \n {\n if(num<0)\n return "-"+convertToBase7(-num);//if negative make posiitve input in function\n else if(num < 7) \n return Integer.toString(num);//return as string the same number\n else\n return convertToBase7(num/7) + convertToBase7(num%7);//greater than 7\n }\n}\n```\n# Approach 2 : 0ms Solution [Fastest]\n```\nclass Solution \n{\n public String convertToBase7(int num) \n {\n return Integer.toString(num, 7);\n }\n}\n``` | 9 | 1 | ['Math', 'Java'] | 1 |
base-7 | Fastest |JAVA | 0ms | fastest-java-0ms-by-aliasifk-ozd7 | \nclass Solution {\n public String convertToBase7(int num) {\n if(num == 0){\n return "0";\n }\n StringBuilder sb = new Strin | aliasifk | NORMAL | 2021-03-16T07:22:04.262215+00:00 | 2021-03-16T07:22:04.262244+00:00 | 529 | false | ```\nclass Solution {\n public String convertToBase7(int num) {\n if(num == 0){\n return "0";\n }\n StringBuilder sb = new StringBuilder();\n boolean isNeg = num < 0;\n num = Math.abs(num);\n while(num != 0){\n sb.append(num%7);\n num = num/7;\n }\n if(isNeg)\n sb.append(\'-\');\n return sb.reverse().toString();\n }\n}\n``` | 9 | 0 | [] | 0 |
base-7 | 504: Solution with step by step explanation | 504-solution-with-step-by-step-explanati-2hrb | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n1. Check if the input number is 0, return "0" in this case.\n2. Initializ | Marlen09 | NORMAL | 2023-03-12T04:29:21.766128+00:00 | 2023-03-12T04:29:21.766166+00:00 | 2,095 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. Check if the input number is 0, return "0" in this case.\n2. Initialize a flag to keep track of whether the number is negative or not.\n3. Convert the input number to a positive integer if it\'s negative by taking the absolute value.\n4. Initialize an empty string variable to store the base 7 representation of the number.\n5. Loop until the input number becomes 0.\n6. At each iteration of the loop, compute the remainder when the input number is divided by 7.\n7. Add the remainder to the base 7 representation string variable.\n8. Update the input number to be the quotient when it\'s divided by 7.\n9. If the original input number was negative, add a \'-\' sign to the base 7 representation.\n10. Return the base 7 representation.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n # Handle the edge case where num is 0\n if num == 0:\n return "0"\n \n # Initialize a flag to keep track of whether num is negative or not\n is_negative = num < 0\n \n # Convert num to positive if it\'s negative\n if is_negative:\n num = -num\n \n # Initialize a variable to keep track of the base 7 representation\n base_7 = ""\n \n # Loop until num becomes 0\n while num > 0:\n # Compute the remainder when num is divided by 7\n remainder = num % 7\n \n # Add the remainder to the base 7 representation\n base_7 = str(remainder) + base_7\n \n # Update num to be the quotient when it\'s divided by 7\n num //= 7\n \n # If num was originally negative, add a \'-\' sign to the base 7 representation\n if is_negative:\n base_7 = \'-\' + base_7\n \n # Return the base 7 representation\n return base_7\n\n``` | 8 | 0 | ['Math', 'Python', 'Python3'] | 0 |
base-7 | Simple c++ solution with explanation || 0ms | simple-c-solution-with-explanation-0ms-b-58o7 | Approach:\n\t1) First we will check for number, if(num == 0), then we return "0".\n\t2) We are using flag variable for identifying the number as positive or neg | tle_exe | NORMAL | 2021-09-02T12:06:43.560992+00:00 | 2022-02-15T10:33:23.890293+00:00 | 1,339 | false | **Approach:**\n\t1) First we will check for number, if(num == 0), then we return "0".\n\t2) We are using flag variable for identifying the number as positive or negative.\n\t3) we are converting the number into positive.\n\t4) Then we are iterating and each time we push the remainder into the string and number is decremented to num/7 until the number is zero.\n\t5) After looping we are reversing the string and checking for the negative number if flag==1 then it is negative so we are adding negative sign and beginning and then returning the string.\n```\nstring convertToBase7(int num) {\n if(num==0) return "0";\n string ret;\n int flag=0;\n if(num<0)\n flag=1;\n num = abs(num);\n while(num != 0){\n ret.push_back((num%7)+\'0\');\n num/=7;\n }\n reverse(ret.begin(),ret.end());\n if(flag==1){\n ret.insert(ret.begin(),\'-\');\n }\n return ret;\n }\n```\nIf you get some value from this, then show some love by upvoting it !!\nHappy Coding \uD83D\uDE0A\uD83D\uDE0A | 8 | 0 | ['C', 'C++'] | 1 |
base-7 | Java easy solution for cheaters 100% | java-easy-solution-for-cheaters-100-by-m-2on4 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Madatbek | NORMAL | 2023-11-03T10:07:27.227974+00:00 | 2023-11-03T10:07:27.227996+00:00 | 559 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public String convertToBase7(int num) {\n return Integer.toString(num,7);\n }\n}\n``` | 7 | 0 | ['Java'] | 0 |
base-7 | 1 line | 1-line-by-lceveryday-yqs4 | \n return Integer.toString(Integer.parseInt(num+"", 10), 7); \nor just: \n\n return Integer.toString(num, 7);\n\nThe formula for convert | lceveryday | NORMAL | 2017-02-12T04:10:57.143000+00:00 | 2017-02-12T04:10:57.143000+00:00 | 3,382 | false | \n return Integer.toString(Integer.parseInt(num+"", 10), 7); \nor just: \n\n return Integer.toString(num, 7);\n\nThe formula for converting from one base to another is: \n\n return Integer.toString(Integer.parseInt(number, base1), base2); | 7 | 5 | [] | 4 |
base-7 | easy c program | easy-c-program-by-bharadwajthangella-0xpo | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | bharadwajthangella | NORMAL | 2024-07-09T08:29:30.576979+00:00 | 2024-07-09T08:29:30.577012+00:00 | 520 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nchar* convertToBase7(int num) {\n int sz = 0;\n char *v = malloc(16);\n\n int mask = num >> 31;\n num = (num ^ mask)-mask;\n\tint neg = !!mask;\n v[sz] = \'-\';\n sz |= neg; /* sz += 1 */\n \n do {\n v[sz++] = (num % 7) + \'0\'; \n num /= 7;\n } while(num);\n\n for(int i = neg, j = sz - 1; i < j; i++, j--) {\n char t = v[i];\n v[i] = v[j];\n v[j] = t;\n } \n v[sz] = \'\\0\';\n\n return v;\n}\n``` | 6 | 0 | ['C'] | 2 |
base-7 | Ugly but works | ugly-but-works-by-georgeofgeorgia-dffv | \nclass Solution:\n def convertToBase7(self, num: int) -> str:\n s=""\n x=0\n p=1\n if num==0:\n return "0"\n i | georgeofgeorgia | NORMAL | 2022-11-01T02:55:48.983487+00:00 | 2022-11-01T02:55:48.983528+00:00 | 538 | false | ```\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n s=""\n x=0\n p=1\n if num==0:\n return "0"\n if num<0:\n p=-1\n num*=-1\n while num>0:\n t=num//7\n s+=str(num%7)\n num//=7\n \n x=int(s[::-1])*p\n return str(x)\n``` | 6 | 0 | [] | 0 |
base-7 | C++ iterative and Recursive solution 3 lines code faster than 100% | c-iterative-and-recursive-solution-3-lin-lg6m | Iterative approach\n\nclass Solution {\npublic:\n string convertToBase7(int num) {\n int res = 0;\n int p = 1;\n while(num != 0)\n | Hardikjain_ | NORMAL | 2021-09-16T11:05:53.103291+00:00 | 2021-09-16T11:05:53.103335+00:00 | 233 | false | Iterative approach\n```\nclass Solution {\npublic:\n string convertToBase7(int num) {\n int res = 0;\n int p = 1;\n while(num != 0)\n {\n res += (num % 7) * p;\n num /= 7;\n p *= 10;\n }\n return to_string(res);\n }\n};\n```\nRecursive approach\n```\nclass Solution {\npublic:\n string convertToBase7(int n) {\n if(n < 0) \n {\n return "-" + convertToBase7(-n);\n }\n else if(n < 7)\n {\n return to_string(n);\n }\n return convertToBase7(n/7) + to_string(n % 7);\n }\n};\n``` | 6 | 0 | ['Recursion'] | 1 |
base-7 | 3ms C++ 3 lines Solution | 3ms-c-3-lines-solution-by-iamxiaobai-hr8h | \n string convertToBase7(int num) {\n int res = 0;\n for(int i=0; num!=0; res += pow(10,i++)*(num % 7), num /= 7) {}\n return to_string(res | IamXiaoBai | NORMAL | 2017-08-22T21:24:42.406000+00:00 | 2017-08-22T21:24:42.406000+00:00 | 1,274 | false | ```` \n string convertToBase7(int num) {\n int res = 0;\n for(int i=0; num!=0; res += pow(10,i++)*(num % 7), num /= 7) {}\n return to_string(res);\n }\n```` | 6 | 2 | [] | 1 |
base-7 | Java 98% Faster | java-98-faster-by-tbekpro-qmnp | Code\n\nclass Solution {\n public String convertToBase7(int num) {\n StringBuilder sb = new StringBuilder();\n while(Math.abs(num) > 6) {\n | tbekpro | NORMAL | 2022-12-24T06:25:42.557964+00:00 | 2022-12-24T06:25:42.557997+00:00 | 1,204 | false | # Code\n```\nclass Solution {\n public String convertToBase7(int num) {\n StringBuilder sb = new StringBuilder();\n while(Math.abs(num) > 6) {\n sb.append(num % 7);\n num = num / 7;\n }\n if (num < 0) {\n sb.append(Math.abs(num)).append("-");\n } else {\n sb.append(num);\n }\n for (int i = 0; i < sb.length() - 1; i++) {\n if (!Character.isDigit(sb.charAt(i))) {\n sb.replace(i, i + 1, "");\n }\n }\n return sb.reverse().toString();\n }\n}\n``` | 5 | 0 | ['Java'] | 0 |
base-7 | Simple and easy - faster than 99.31% | simple-and-easy-faster-than-9931-by-this-6p5c | \nclass Solution:\n def convertToBase7(self, num: int) -> str:\n if not num:\n return "0"\n l=[]\n x=num\n if num<0:\n | thisisakshat | NORMAL | 2021-01-13T14:36:49.403894+00:00 | 2021-01-13T14:36:49.403928+00:00 | 1,308 | false | ```\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n if not num:\n return "0"\n l=[]\n x=num\n if num<0:\n num=-num\n while num>0:\n r=num%7\n l.append(str(r))\n num//=7\n return "".join(l[::-1]) if x>=0 else "-"+ "".join(l[::-1]) \n```\nIf you like, please **UPVOTE**\nHappy Coding :) | 5 | 0 | ['Python', 'Python3'] | 1 |
base-7 | C++ BEST SOLUTION || EASY APPROACH || O(log base 7) | c-best-solution-easy-approach-olog-base-8a6oy | Intuition\n Describe your first thoughts on how to solve this problem. \nbasic convesion technique is used by dividing the num by 7 and storing the remainder as | Priyansh_saxena_01 | NORMAL | 2023-05-09T20:20:01.401962+00:00 | 2023-05-09T20:20:01.402002+00:00 | 678 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nbasic convesion technique is used by dividing the num by 7 and storing the remainder as a string.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nfirstly the number is converted to base 7 then it is checked if it was a negetive integer then a neetive string is added to the resultant string else the original string is returned.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(log base 7)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n\n# Code\n```\nclass Solution {\npublic:\n string convertToBase7(int n) {\n string str="";\n int rem;\n int num=abs(n);\n if(n==0) return "0";\n while(num!=0){\n rem=num%7;\n str=to_string(rem)+str;\n num/=7;\n }\n if(n<0) return "-"+str;\n return str;\n }\n};\n``` | 4 | 0 | ['Math', 'String', 'C++'] | 2 |
base-7 | ⬆️✅ One-Liner ka baap || java/python/js | 100.00% | 0 ms 🔥🔥🔥🔥🔥🔥🔥 | one-liner-ka-baap-javapythonjs-10000-0-m-ur3x | \n**upvote please**\n\njava\nreturn Integer.toString(num,7);\n\npy\nimport numpy as np\nreturn np.base_repr(num, base=7)\n\n\njs:\nconst convertToBase7 = num = | ManojKumarPatnaik | NORMAL | 2022-09-11T16:25:26.134946+00:00 | 2022-09-11T16:25:48.082553+00:00 | 339 | false | ```\n**upvote please**\n\njava\nreturn Integer.toString(num,7);\n\npy\nimport numpy as np\nreturn np.base_repr(num, base=7)\n\n\njs:\nconst convertToBase7 = num => num.toString(7);\n``` | 4 | 0 | ['C', 'Python', 'Java', 'JavaScript'] | 0 |
base-7 | Simple 0ms || (100%) c++ solution | simple-0ms-100-c-solution-by-anmol_2001-1aop | \n\t\tclass Solution {\n\t\tpublic:\n\t\tstring convertToBase7(int num) {\n if(num==0)\n return "0";\n int res,n1=num;\n string | anmol_2001 | NORMAL | 2022-02-22T05:35:07.472539+00:00 | 2023-03-03T17:21:39.974103+00:00 | 362 | false | \n\t\tclass Solution {\n\t\tpublic:\n\t\tstring convertToBase7(int num) {\n if(num==0)\n return "0";\n int res,n1=num;\n string s1="";\n\t\tvector<int> v1;\n\t\t\n if(num<0)\n {\n num*=-1;\n s1="-";\n }\n \n while(num)\n {\n res=num%7;\n v1.push_back(res);\n num/=7;\n }\n reverse(v1.begin(),v1.end());\n \n for(int i=0;i<v1.size();i++)\n s1=s1+to_string(v1[i]);\n\n return s1; \n }\n }; | 4 | 0 | ['C++'] | 0 |
base-7 | [JavaScript] Recursion | javascript-recursion-by-mulyk-jasu | \nvar convertToBase7 = function(num) {\n if(num < 0) return \'-\' + convertToBase7(num*(-1));\n if(num < 7) return num.toString();\n return convertToBa | mulyk | NORMAL | 2020-10-09T19:59:32.935530+00:00 | 2020-10-09T20:08:59.443553+00:00 | 356 | false | ```\nvar convertToBase7 = function(num) {\n if(num < 0) return \'-\' + convertToBase7(num*(-1));\n if(num < 7) return num.toString();\n return convertToBase7(Math.floor(num/7)) + (num%7).toString();\n};\n``` | 4 | 0 | ['JavaScript'] | 0 |
base-7 | Java 1 Liner + Standard Solution | java-1-liner-standard-solution-by-compto-0rqr | \npublic String convertTo7(int num) {\n return Integer.toString(num, 7);\n}\n\n\nNot using standard library:\n\n\n public String convertTo7(int num) {\n | compton_scatter | NORMAL | 2017-02-12T04:11:38.032000+00:00 | 2017-02-12T04:11:38.032000+00:00 | 1,677 | false | ```\npublic String convertTo7(int num) {\n return Integer.toString(num, 7);\n}\n```\n\nNot using standard library:\n\n```\n public String convertTo7(int num) {\n if (num == 0) return "0";\n String res = "";\n boolean isNeg = num < 0;\n while (num != 0) {\n res = Math.abs((num % 7)) + res;\n num /= 7;\n }\n return isNeg ? "-" + res : res;\n }\n``` | 4 | 0 | [] | 0 |
base-7 | 🔢 Convert to Base 7 | Beats 100% in all Languages 🚀 | convert-to-base-7-beats-100-in-all-langu-fafs | IntuitionThe simplest way to convert a number to base 7 is by leveraging built-in conversion functions. Many languages have direct methods to convert a number t | shomalikdavlatov | NORMAL | 2025-03-25T09:22:05.471358+00:00 | 2025-03-25T09:23:21.756743+00:00 | 190 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
The simplest way to convert a number to base 7 is by leveraging built-in conversion functions. Many languages have direct methods to convert a number to a different base.
# Approach
<!-- Describe your approach to solving the problem. -->
1. Use a built-in function (if available) to convert `num` to a `base 7` string.
2. If not available, manually implement base conversion by repeatedly dividing the number by `7` and collecting remainders.
# Complexity
- Time complexity: `O(log n)`
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
Since the number is divided by 7 in each step
- Space complexity: `O(1)`
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
Constant space usage
# Code
```javascript []
/**
* @param {number} num
* @return {string}
*/
var convertToBase7 = function(num) {
return num.toString(7);
};
```
``` Python3 []
import numpy as np
class Solution:
def convertToBase7(self, num: int) -> str:
return '-' + np.base_repr(-num, 7) if num < 0 else np.base_repr(num, 7)
```
``` C++ []
class Solution {
public:
string convertToBase7(int num) {
if (num == 0) return "0";
string res;
bool neg = num < 0;
num = abs(num);
while (num > 0) {
res = to_string(num % 7) + res;
num /= 7;
}
return neg ? "-" + res : res;
}
};
```
``` Java []
class Solution {
public String convertToBase7(int num) {
return Integer.toString(num, 7);
}
}
```
``` Go []
import "strconv"
func convertToBase7(num int) string {
return strconv.FormatInt(int64(num), 7)
}
```
``` Swift []
class Solution {
func convertToBase7(_ num: Int) -> String {
return String(num, radix: 7)
}
}
```
``` Dart []
class Solution {
String convertToBase7(int num) {
return num.toRadixString(7);
}
}
``` Kotlin []
class Solution {
fun convertToBase7(num: Int): String {
return num.toString(7)
}
}
``` Ruby []
# @param {Integer} num
# @return {String}
def convert_to_base7(num)
num.to_s(7)
end
```
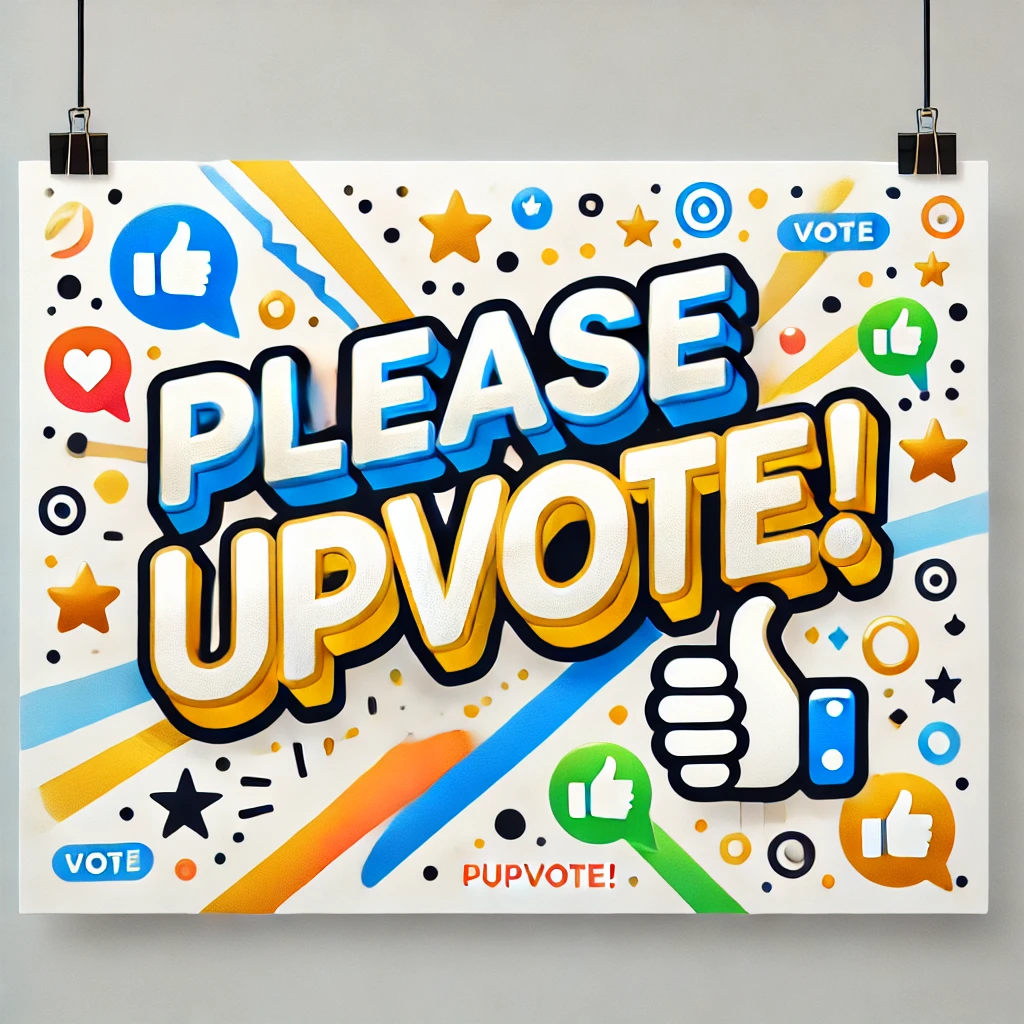
| 3 | 0 | ['Math', 'Swift', 'C++', 'Go', 'Python3', 'JavaScript', 'Dart'] | 2 |
base-7 | EFFICIENT PYTHON SOLUTION | efficient-python-solution-by-krish2213-yp3c | Given an integer num, return a string of its base 7 representation.\n\n \n# Code\n\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n \n | krish2213 | NORMAL | 2023-06-30T05:30:48.537570+00:00 | 2023-06-30T05:30:48.537606+00:00 | 291 | false | Given an integer num, return a string of its base 7 representation.\n\n \n# Code\n```\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n \n ans=""\n temp=num\n num = abs(num)\n while(num>0):\n ans+=str(num%7)\n num//=7\n neg_case="-"+ans[::-1]\n return ans[::-1] if temp>0 else "0" if temp==0 else neg_case\n\n``` | 3 | 0 | ['Python3'] | 0 |
base-7 | Solution | solution-by-deleted_user-xfyo | C++ []\nclass Solution {\n public:\n string convertToBase7(int num) {\n if (num < 0)\n return "-" + convertToBase7(-num);\n if (num < 7)\n retu | deleted_user | NORMAL | 2023-04-05T06:24:37.168693+00:00 | 2023-04-05T06:41:15.677632+00:00 | 1,480 | false | ```C++ []\nclass Solution {\n public:\n string convertToBase7(int num) {\n if (num < 0)\n return "-" + convertToBase7(-num);\n if (num < 7)\n return to_string(num);\n return convertToBase7(num / 7) + to_string(num % 7);\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def convertToBase7(self, n: int) -> str:\n num, s = abs(n), \'\'\n while num:\n num, remain = divmod(num,7)\n s = str(remain) + s\n return "-" * (n < 0) + s or "0"\n```\n\n```Java []\nclass Solution {\n public String convertToBase7(int num) {\n return Integer.toString(num, 7);\n }\n}\n```\n | 3 | 0 | ['C++', 'Java', 'Python3'] | 2 |
base-7 | JavaScript | javascript-by-1rosehip-xcsm | \nvar convertToBase7 = function(num) {\n if(num === 0) return \'0\';\n \n let temp = Math.abs(num);\n let res = \'\';\n \n while(temp !== 0){\ | 1rosehip | NORMAL | 2022-11-13T07:41:41.982087+00:00 | 2022-11-13T07:41:41.982135+00:00 | 570 | false | ```\nvar convertToBase7 = function(num) {\n if(num === 0) return \'0\';\n \n let temp = Math.abs(num);\n let res = \'\';\n \n while(temp !== 0){\n res = temp % 7 + res;\n temp = Math.floor(temp / 7);\n }\n \n return num >= 0 ? res : `-${res}`;\n};\n``` | 3 | 0 | ['JavaScript'] | 1 |
base-7 | Java Easy Solution | java-easy-solution-by-priyanshurawat-z8dq | \npublic String convertToBase7(int num) {\n int count = 0, res = 0;\n while(num != 0)\n {\n int dig = num % 7;\n res | priyanshurawat | NORMAL | 2022-09-16T15:47:51.603944+00:00 | 2022-09-16T15:47:51.603986+00:00 | 599 | false | ```\npublic String convertToBase7(int num) {\n int count = 0, res = 0;\n while(num != 0)\n {\n int dig = num % 7;\n res += dig * (int)Math.pow(10, count);\n count++;\n num /= 7;\n }\n return Integer.toString(res);\n }\n``` | 3 | 0 | ['Java'] | 0 |
base-7 | Rust solution | rust-solution-by-bigmih-tbxs | \nimpl Solution {\n pub fn convert_to_base7(mut num: i32) -> String {\n let (mut res, mut base) = (0, 1);\n while num != 0 {\n res + | BigMih | NORMAL | 2022-03-07T19:20:41.590580+00:00 | 2022-03-07T19:20:41.590629+00:00 | 131 | false | ```\nimpl Solution {\n pub fn convert_to_base7(mut num: i32) -> String {\n let (mut res, mut base) = (0, 1);\n while num != 0 {\n res += base * (num % 7);\n base *= 10;\n num /= 7;\n }\n res.to_string()\n }\n}\n``` | 3 | 0 | ['Rust'] | 0 |
base-7 | Base 7 C++|| 0ms(100%) | base-7-c-0ms100-by-whkshitij13-qfqs | \t\tclass Solution {\n\t\tpublic:\n\t\t\tstring convertToBase7(int num) {\n\t\t\tint sum=0;\n string ans;\n int temp=num;\n vectorarr;\n | whkshitij13 | NORMAL | 2022-02-22T05:33:43.274620+00:00 | 2022-02-22T16:41:37.895130+00:00 | 164 | false | \t\tclass Solution {\n\t\tpublic:\n\t\t\tstring convertToBase7(int num) {\n\t\t\tint sum=0;\n string ans;\n int temp=num;\n vector<int>arr;\n string str="";\n if(temp<0)\n {str="-";\n num=abs(num);\n }if(num==0)\n return "0";\n while(num)\n {\n arr.push_back(num%7);\n num=num/7;\n \n }\n for(int i=arr.size()-1;i>=0;i--)\n { str=str+to_string(arr[i]);\n } \n \n return str;\n\t\t\t}\n\t\t}; | 3 | 0 | [] | 0 |
base-7 | C++ || 2 approaches || std::to_chars and handrolled | c-2-approaches-stdto_chars-and-handrolle-3j0w | -\nTitle: C++ || 2 approaches || std::to_chars and handrolled\nProblem: 504\nURL: https://leetcode.com/problems/base-7/discuss/221488/c-3-liner-using-stdto_char | heder | NORMAL | 2019-01-19T07:38:07.572449+00:00 | 2022-11-04T20:27:35.582868+00:00 | 191 | false | <!---\nTitle: C++ || 2 approaches || std::to_chars and handrolled\nProblem: 504\nURL: https://leetcode.com/problems/base-7/discuss/221488/c-3-liner-using-stdto_chars\n-->\n\n# Approach 1: std::to_chars\n\n```cpp\n static std::string convertToBase7(int num) {\n std::array<char, sizeof(int) * 4> buf = {0};\n std::to_chars(buf.begin(), buf.end(), num, 7);\n return std::string(buf.data());\n }\n```\n\n# Approach 2: handrolled\n\n```cpp\n static string convertToBase7(int num) {\n if (num < 0) return "-" + convertToBase7(-num);\n \n if (num == 0) return "0";\n\n string ans;\n while (num) {\n ans.push_back(num % 7 + \'0\');\n num /= 7;\n }\n reverse(ans.begin(), ans.end());\n return ans;\n }\n```\n\n_As always: Feedback, questions, and comments are welcome. Leaving an upvote sparks joy! :)_\n\n**p.s. Join us on the [LeetCode The Hard Way Discord Server](https://discord.gg/hFUyVyWy2E)!**\n | 3 | 0 | ['C'] | 0 |
base-7 | EASY C++ 100% O(log N) | easy-c-100-olog-n-by-hnmali-4u33 | Complexity
Time complexity: O(logn)
Space complexity: O(1)
Code | hnmali | NORMAL | 2025-01-27T19:22:41.099324+00:00 | 2025-01-27T19:22:41.099324+00:00 | 503 | false |
# Complexity
- Time complexity: $$O(log n)$$
- Space complexity: $$O(1)$$
# Code
```cpp []
class Solution {
public:
string convertToBase7(int num) {
string ans;
bool flag = false;
if(num < 0) {
flag = true;
num *= -1;
}
while(num >= 7) {
char ch = (num%7)+'0';
ans = ch + ans;
num /= 7;
}
char ch = num +'0';
ans = ch + ans;
if(flag)
ans = "-" + ans;
return ans;
}
};
``` | 2 | 0 | ['Math', 'C++'] | 0 |
base-7 | Easy Solution || Python || Maths || Beats 100% | easy-solution-python-maths-beats-100-by-v6zic | My Solution Beats 100% in runtime\n# Intuition\n Describe your first thoughts on how to solve this problem. \nThe main motive of this problem is to find the bas | siri_chandana17 | NORMAL | 2024-10-09T11:58:54.118925+00:00 | 2024-10-09T11:59:35.972475+00:00 | 564 | false | # My Solution Beats 100% in runtime\n# Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe main motive of this problem is to find the base 7 value of given input integer number and return as string.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nUsing Loops and conditional statements..\nFind whether the given number or interger is 0 or positive or negative.Generate the base 7 value accordingly.\n\n# Complexity\n- Time complexity:O(log n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(log n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python3 []\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n s=""\n if num>0:\n while num!=0:\n n=num//7\n a=num%7\n s+=str(a)\n num=n\n return s[::-1]\n elif num==0:\n return \'0\'\n else:\n num=num*-1\n while num!=0:\n n=num//7\n a=num%7\n s+=str(a)\n num=n\n \n return \'-\'+s[::-1]\n\n```\n# Please UPVOTE me..! | 2 | 0 | ['Math', 'Python3'] | 0 |
base-7 | Interview Prep!! | interview-prep-by-abinayaprakash-f1fj | Intuition\n Describe your first thoughts on how to solve this problem. \nThe problem is asking to convert a given integer to its base 7 representation. My first | Abinayaprakash | NORMAL | 2024-09-09T14:24:36.881496+00:00 | 2024-09-09T14:24:36.881533+00:00 | 237 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem is asking to convert a given integer to its base 7 representation. My first thought is to repeatedly divide the number by 7 and collect the remainders, which would give the digits of the base 7 number in reverse order. If the number is negative, we handle it separately by converting the absolute value and then prepending a negative sign to the result.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. **Initial Check**: If the input number is 0, return "0" immediately as the base 7 representation of 0 is simply "0".\n2. **Negative Number Handling**: If the number is negative, convert it to its absolute value and set a flag `f` to indicate that it was negative.\n3. **Digit Collection**: Use a `while` loop to repeatedly divide the number by 7 and collect the remainders. These remainders represent the digits of the base 7 number but in reverse order.\n4. **Reverse the String**: Convert the collected digits into a string and reverse it to get the correct order.\n5. **Negative Sign Handling**: If the original number was negative, prepend a \'-\' to the result.\n6. **Return the Result**: Return the final base 7 representation.\n\n# Complexity\n- **Time complexity**: \n The time complexity is $$O(\\log_7(\\text{num}))$$ because the loop iterates for the number of digits in the base 7 representation, which is proportional to the logarithm of the number to the base 7.\n\n- **Space complexity**:\n The space complexity is also $$O(\\log_7(\\text{num}))$$ due to the space required to store the digits in the `StringBuilder`.\n\n# Code\n```java\nclass Solution {\n public String convertToBase7(int num) {\n if(num == 0){\n return "0";\n }\n \n int f = 0;\n StringBuilder sb = new StringBuilder();\n \n if(num < 0){\n f = 1;\n num = -num;\n }\n \n while(num > 0){\n sb.append(num % 7);\n num /= 7;\n }\n \n String ori = sb.reverse().toString();\n \n if(f == 1){\n return "-" + ori;\n }\n \n return ori;\n }\n}\n\n```\n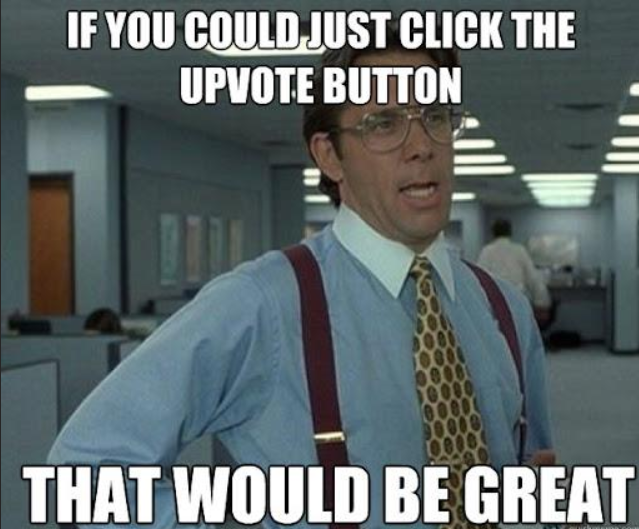\n\n | 2 | 0 | ['Math', 'Java'] | 0 |
base-7 | T.C : O(logN) || EASY C++ Solution || CPP | tc-ologn-easy-c-solution-cpp-by-ganesh_a-livq | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Ganesh_ag10 | NORMAL | 2024-06-21T16:17:06.167316+00:00 | 2024-06-21T16:17:06.167333+00:00 | 498 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n string convertToBase7(int num) {\n if(num==0) return "0";\n int n=abs(num);\n string ans="";\n while(n!=0){\n ans+=(to_string(n%7));\n n/=7;\n }\n if(num<0) ans+=\'-\';\n reverse(ans.begin(),ans.end());\n return ans;\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
base-7 | Easy and simple c++ solution beats 100% 0ms Solution | easy-and-simple-c-solution-beats-100-0ms-rzya | Code\n\nclass Solution {\npublic:\n string convertToBase7(int num) {\n bool sign=0;\n if(num<0){\n sign=1;\n }\n num=a | Kritvik07 | NORMAL | 2024-05-07T08:16:18.195665+00:00 | 2024-05-07T08:16:18.195687+00:00 | 985 | false | # Code\n```\nclass Solution {\npublic:\n string convertToBase7(int num) {\n bool sign=0;\n if(num<0){\n sign=1;\n }\n num=abs(num);\n string str="";\n while(num>=7){\n str+=num%7+48;\n num/=7;\n }\n str+=num+48;\n if(sign){\n str+=\'-\';\n }\n reverse(str.begin(),str.end());\n return str;\n }\n};\n``` | 2 | 0 | ['C++'] | 2 |
base-7 | java 100 beats | java-100-beats-by-mantosh_kumar04-xa2d | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | mantosh_kumar04 | NORMAL | 2024-04-25T17:10:22.853357+00:00 | 2024-04-25T17:10:22.853375+00:00 | 636 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public String convertToBase7(int num) {\n return Integer.toString(num, 7);\n }\n}\n``` | 2 | 0 | ['Java'] | 0 |
base-7 | java code easy to implement | java-code-easy-to-implement-by-ragulrame-xjig | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | ragulramesh1819 | NORMAL | 2024-01-06T07:22:51.615964+00:00 | 2024-01-06T07:22:51.615993+00:00 | 1,151 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public String convertToBase7(int num) {\n if(num==0)\n return "0";\n String s="";\n String o="-";\n int y=num;\n num=Math.abs(num);\n while(num>0)\n {\n s+=num%7;\n num/=7;\n }\n StringBuilder k=new StringBuilder();\n k.append(s);\n String z=String.valueOf(k.reverse());\n o+=z;\n if(y<0)\n return o;\n return z;\n \n }\n}\n``` | 2 | 0 | ['Java'] | 1 |
base-7 | 0ms Solution ~VP | 0ms-solution-vp-by-vishnuprasath011-a3sq | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | vishnuprasath011 | NORMAL | 2024-01-06T05:58:58.812511+00:00 | 2024-01-06T05:58:58.812541+00:00 | 200 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public String convertToBase7(int num) {\n return Integer.toString(num,7);\n }\n}\n``` | 2 | 0 | ['Java'] | 1 |
base-7 | Python Easy Solution | python-easy-solution-by-sumedh0706-t0g9 | Code\n\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n if num==0:\n return "0"\n res=""\n flg=0\n if n | Sumedh0706 | NORMAL | 2023-07-17T06:17:24.174844+00:00 | 2023-07-17T06:17:24.174875+00:00 | 964 | false | # Code\n```\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n if num==0:\n return "0"\n res=""\n flg=0\n if num<0:\n flg=1\n num=abs(num)\n while(num>0):\n res+=str(num%7)\n num//=7\n if flg==1:\n res+="-"\n return res[::-1]\n``` | 2 | 0 | ['Python3'] | 0 |
base-7 | ✅✅Single Line Java Solution || Beats 100% 🚀🚀 | single-line-java-solution-beats-100-by-r-sr3u | Code\n\nclass Solution {\n public String convertToBase7(int num) {\n return Integer.toString(num, 7);\n }\n}\n\nPlease upvote if you like the solut | rirefat | NORMAL | 2023-05-30T17:54:38.281690+00:00 | 2023-05-30T17:54:38.281727+00:00 | 299 | false | # Code\n```\nclass Solution {\n public String convertToBase7(int num) {\n return Integer.toString(num, 7);\n }\n}\n```\nPlease upvote if you like the solution.\nHappy Coding! \uD83D\uDE0A | 2 | 1 | ['Math', 'Java'] | 0 |
base-7 | recursion🔥 | recursion-by-tanmay_jaiswal-hde2 | \nclass Solution {\n string s = "";\npublic:\n string convertToBase7(int num, bool flag = 0) {\n if (num==0) {\n if (s.size() == 0) s = | tanmay_jaiswal_ | NORMAL | 2023-05-28T20:23:58.594275+00:00 | 2023-05-28T20:23:58.594312+00:00 | 394 | false | ```\nclass Solution {\n string s = "";\npublic:\n string convertToBase7(int num, bool flag = 0) {\n if (num==0) {\n if (s.size() == 0) s = "0";\n else reverse(begin(s), end(s)); \n return flag? "-"+s: s;\n }\n\n if (num<0) flag = 1;\n s.push_back(abs(num)%7 + \'0\');\n\n return convertToBase7(abs(num)/7, flag);\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
base-7 | c++ | | O(N) | c-on-by-_akshay_raj_singh_108-lf7f | \n<!-- Describe your first thoughts on how to solve this problem. -\n Describe your approach to solving the problem. \n\n# Complexity\n- Time complexity:O(n)\n | _Akshay_Raj_Singh_108 | NORMAL | 2023-04-30T11:04:28.548417+00:00 | 2023-04-30T11:04:28.548456+00:00 | 541 | false | \n<!-- Describe your first thoughts on how to solve this problem. -\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n string convertToBase7(int num) {\n string s="";\n int l=0;\n if(num==0){\n return "0";\n }\n if(num>0)\n { int p=num;\n while (p!=0){\n l=p%7;\n string h=to_string(l);\n s=h+s;\n p=p/7;\n }\n }else if (num<0){\n int p=-num;\n while (p!=0){\n l=p%7;\n string h=to_string(l);\n s=h+s;\n p=p/7;\n }\n s=\'-\'+s;\n }\n return s;\n\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
base-7 | Best solution ever (Just One line) | best-solution-ever-just-one-line-by-deva-disg | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | devansh_1703 | NORMAL | 2023-03-10T05:09:46.589807+00:00 | 2023-03-10T05:09:46.589847+00:00 | 1,191 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:$$ O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public String convertToBase7(int num) {\n return Integer.toString(num,7);\n }\n}\n``` | 2 | 0 | ['Java'] | 0 |
base-7 | C++ Solution || Beats 100% || Runtime 0ms || | c-solution-beats-100-runtime-0ms-by-asad-m626 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Asad_Sarwar | NORMAL | 2023-02-18T22:17:34.896431+00:00 | 2023-02-18T22:17:34.896460+00:00 | 2,515 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n string convertToBase7(int num) {\n\n int ans = 0;\n int i = 0;\n\n while (num != 0)\n {\n int rem = num % 7;\n ans = ans + rem * pow(10, i);\n i++;\n num = num / 7;\n }\n cout << ans << endl;\n string Ans;\n Ans = to_string(ans);\n return Ans;\n\n }\n};\n``` | 2 | 0 | ['C++'] | 1 |
base-7 | Python one-liner with numpy for fun :) | python-one-liner-with-numpy-for-fun-by-t-4iz0 | \n\nimport numpy as np\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n return np.base_repr(num, base=7)\n | tryingall | NORMAL | 2023-01-15T11:04:43.831311+00:00 | 2023-01-15T11:04:43.831364+00:00 | 1,330 | false | \n```\nimport numpy as np\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n return np.base_repr(num, base=7)\n``` | 2 | 0 | ['Python3'] | 0 |
base-7 | ✅✅✅ Faster than 98% | Easy to understand | faster-than-98-easy-to-understand-by-naj-ulqg | \nclass Solution:\n def convertToBase7(self, num: int) -> str:\n if num==0: return "0"\n a=\'\'\n if num<0:\n num=-num\n | Najmiddin1 | NORMAL | 2022-12-18T15:32:11.832506+00:00 | 2022-12-18T15:32:11.832565+00:00 | 1,089 | false | ```\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n if num==0: return "0"\n a=\'\'\n if num<0:\n num=-num\n a = \'-\'\n base7 = \'\'\n while num>0:\n base7+=str(num%7)\n num = num//7\n return a+base7[::-1]\n``` | 2 | 0 | ['Python3'] | 1 |
base-7 | Beats 99% - Easy Python Solution | beats-99-easy-python-solution-by-pranavb-8xpq | \nclass Solution:\n def convertToBase7(self, num: int) -> str:\n s = ""\n n = abs(num)\n while n != 0:\n s = str(n % 7) + s\n | PranavBhatt | NORMAL | 2022-12-02T15:40:31.256141+00:00 | 2022-12-02T15:40:31.256179+00:00 | 734 | false | ```\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n s = ""\n n = abs(num)\n while n != 0:\n s = str(n % 7) + s\n n = int(n // 7)\n if num > 0:\n return s\n elif num == 0:\n return "0"\n else:\n return "-" + s\n``` | 2 | 0 | ['Python'] | 0 |
base-7 | C++ | 0ms | c-0ms-by-_kitish-29uu | \nclass Solution {\nprivate:\n string ans;\npublic:\n string convertToBase7(int num) {\n if(!num) return "0";\n convertToBase7(num/7);\n | _kitish | NORMAL | 2022-11-17T05:25:36.461138+00:00 | 2022-11-17T05:25:36.461175+00:00 | 689 | false | ```\nclass Solution {\nprivate:\n string ans;\npublic:\n string convertToBase7(int num) {\n if(!num) return "0";\n convertToBase7(num/7);\n ans += (abs(num)%7) + \'0\';\n return num < 0 ? \'-\' + ans : ans;\n }\n};\n``` | 2 | 0 | ['Recursion'] | 0 |
base-7 | ✅ Runtime: 0 ms, faster than 100.00% of C++ online submissions | runtime-0-ms-faster-than-10000-of-c-onli-qzkz | \n\n/*** 504. Base 7 - CPP17 ***/\n\nclass Solution {\npublic:\n string convertToBase7(int num) {\n string x = "";\n int temp = 0;\n i | Adarsh_Shukla | NORMAL | 2022-09-16T05:00:15.401761+00:00 | 2022-09-16T05:00:15.401796+00:00 | 985 | false | ```\n\n/*** 504. Base 7 - CPP17 ***/\n\nclass Solution {\npublic:\n string convertToBase7(int num) {\n string x = "";\n int temp = 0;\n if(num<0){\n temp=-1*num;\n }\n else {\n temp=num;\n }\n vector<int> ans;\n while(temp>=7){\n int a = temp%7;\n ans.push_back(a);\n temp/=7;\n }\n ans.push_back(temp);\n for(int i=0;i<ans.size();i++){\n string m = to_string(ans[i]);\n x+=m;\n }\n reverse(x.begin(),x.end());\n if(num<0){\n x.insert(x.begin(),\'-\');\n }\n return x;\n }\n};\n\n``` | 2 | 0 | ['String', 'C'] | 0 |
base-7 | C++ Simple and Clean Code | Faster than 100% | c-simple-and-clean-code-faster-than-100-7ddh2 | Consider 0 is the special case.\n2. Store a flag indicating if the number is negative or not.\n3. Convert the absolute value of the number.\n4. Because string i | mohamedshiha | NORMAL | 2022-09-15T12:09:47.725545+00:00 | 2022-09-15T12:09:47.725595+00:00 | 408 | false | 1. Consider `0` is the special case.\n2. Store a flag indicating if the number is negative or not.\n3. Convert the absolute value of the number.\n4. Because `string` is a last-in-first-out (LIFO) data structure, it is possible to add result in the correct order by either:\n\t1. Using `append` or `push_back` and reverse the string after the conversion loop.\n\t2. Using a `queue` instead of `string` which is still a good solution considering the space comlexity is `O(logn)`\n\n```\nstring convertToBase7(int num) {\n if(num == 0)\n return "0";\n string base7Num;\n bool isNegative = num < 0;\n num = abs(num);\n while (num) {\n base7Num += to_string(num % 7);\n num /= 7;\n }\n reverse(base7Num.begin(), base7Num.end());\n return isNegative ? "-" + base7Num : base7Num;\n}\n``` | 2 | 0 | ['C'] | 0 |
base-7 | [Swift] Simplest solution | swift-simplest-solution-by-athleteg-m58n | Please, upvote if you found the solution useful.\n\nclass Solution {\n\t/// Time: O(n). Space: O(n).\n func convertToBase7(_ num: Int) -> String {\n v | Athleteg | NORMAL | 2022-09-01T08:31:59.634439+00:00 | 2022-09-01T08:33:13.278256+00:00 | 121 | false | Please, upvote if you found the solution useful.\n```\nclass Solution {\n\t/// Time: O(n). Space: O(n).\n func convertToBase7(_ num: Int) -> String {\n var n = abs(num)\n var i = 1\n var result = 0\n\n while n > 0 {\n result += (n % 7) * i\n i *= 10\n n /= 7\n }\n return num.signum() == -1 ? "-\\(result)" : "\\(result)"\n }\n}\n``` | 2 | 0 | ['Swift'] | 1 |
base-7 | Java Solution with simple explanation. | java-solution-with-simple-explanation-by-pzh5 | Code \n\n\nclass Solution {\n public String convertToBase7(int num) {\n if (num == 0) return "0";\n StringBuilder sb = new StringBuilder();\n | aayushkumar20 | NORMAL | 2022-07-27T19:58:58.378637+00:00 | 2022-07-27T19:58:58.378673+00:00 | 565 | false | ## Code \n\n```\nclass Solution {\n public String convertToBase7(int num) {\n if (num == 0) return "0";\n StringBuilder sb = new StringBuilder();\n boolean isNegative = num < 0;\n if (isNegative) num = -num;\n while (num > 0) {\n sb.append(num % 7);\n num /= 7;\n }\n if (isNegative) sb.append("-");\n return sb.reverse().toString(); \n }\n}\n```\n\n## Explanation\n\n1. If num is 0, return "0".\n2. Initialize a StringBuilder object sb.\n3. Initialize a boolean isNegative to false.\n4. If num is negative, set isNegative to true and set num to -num.\n5. While num is greater than 0, append num % 7 to sb.\n6. If isNegative is true, append "-" to sb.\n7. Reverse the string in sb and return it. | 2 | 0 | ['Java'] | 0 |
base-7 | Simple C++ || solution 100% fast || iterative | simple-c-solution-100-fast-iterative-by-cbyqf | \nclass Solution {\npublic:\n string convertToBase7(int num) {\n int ans =0;\n int j = 1;\n while(num)\n { \n ans += ( | kunalavghade | NORMAL | 2022-07-06T19:18:47.814175+00:00 | 2022-07-06T19:18:47.814211+00:00 | 374 | false | ```\nclass Solution {\npublic:\n string convertToBase7(int num) {\n int ans =0;\n int j = 1;\n while(num)\n { \n ans += (num%7)*j;\n num /=7;\n j*=10;\n }\n return to_string(ans);\n }\n};\n``` | 2 | 0 | ['C'] | 0 |
base-7 | C++ Recursive || Easy Solution | c-recursive-easy-solution-by-yashprataps-1r93 | \nclass Solution {\npublic:\n string convertToBase7(int num) {\n if(num<0) return "-" + convertToBase7(-num);\n if(num<7) return to_string(num) | yashpratapsingh11 | NORMAL | 2022-04-24T09:09:21.329885+00:00 | 2022-04-24T09:09:21.329932+00:00 | 170 | false | ```\nclass Solution {\npublic:\n string convertToBase7(int num) {\n if(num<0) return "-" + convertToBase7(-num);\n if(num<7) return to_string(num);\n return convertToBase7(num/7) + to_string(num%7);\n }\n};\n``` | 2 | 0 | ['Recursion', 'C', 'Java'] | 0 |
base-7 | Java 0ms Solution using StringBuilder | java-0ms-solution-using-stringbuilder-by-37so | It is recommended using StringBuilder instead of String concatenation since, the latter is more expensive. Additionally, StringBuilder has .insert() method whic | grustamli | NORMAL | 2022-03-19T20:22:02.368676+00:00 | 2022-03-19T20:29:14.314537+00:00 | 96 | false | It is recommended using StringBuilder instead of String concatenation since, the latter is more expensive. Additionally, StringBuilder has .insert() method which can be used to insert the character at 0th index. However, since StringBuilder uses array under the hood, it has to shift every character to the right in order to insert at the index. Thus I chose to append the characters and reverse it at once at return.\n```\nclass Solution {\n public String convertToBase7(int num) {\n StringBuilder s = new StringBuilder();\n int n = Math.abs(num);\n while(n / 7 != 0){\n s.append(n % 7);\n n /= 7;\n }\n s.append(n);\n if(num < 0) s.append(\'-\');\n return s.reverse().toString();\n }\n}\n``` | 2 | 0 | ['String', 'Java'] | 0 |
base-7 | Base 7 Solution Java | base-7-solution-java-by-bhupendra786-1uic | class Solution {\n public String convertToBase7(int num) {\n if (num < 0)\n return "-" + convertToBase7(-num);\n if (num < 7)\n return String.v | bhupendra786 | NORMAL | 2022-03-10T07:43:09.531133+00:00 | 2022-03-10T07:43:09.531160+00:00 | 109 | false | class Solution {\n public String convertToBase7(int num) {\n if (num < 0)\n return "-" + convertToBase7(-num);\n if (num < 7)\n return String.valueOf(num);\n return convertToBase7(num / 7) + String.valueOf(num % 7);\n }\n}\n | 2 | 0 | ['Math', 'String'] | 0 |
base-7 | [Java Solution] One Line - 0ms | java-solution-one-line-0ms-by-ethanrao-p8qo | java []\nclass Solution {\n public String convertToBase7(int num) {\n return Integer.toString(num, 7);\n }\n}\n | ethanrao | NORMAL | 2022-03-06T19:57:39.978778+00:00 | 2022-10-03T21:50:56.383076+00:00 | 278 | false | ```java []\nclass Solution {\n public String convertToBase7(int num) {\n return Integer.toString(num, 7);\n }\n}\n``` | 2 | 0 | ['Java'] | 0 |
base-7 | Go lang | go-lang-by-vladverpeta-hbsd | \nfunc convertToBase7(num int) string {\n if(num == 0) {\n return "0";\n }\n return fmt.Sprintf("%d", TtoS(Abs(num))*Abs(num)/num)\n}\n\nfunc Tt | vladverpeta | NORMAL | 2021-11-17T21:49:16.627549+00:00 | 2021-11-17T21:50:19.961597+00:00 | 160 | false | ```\nfunc convertToBase7(num int) string {\n if(num == 0) {\n return "0";\n }\n return fmt.Sprintf("%d", TtoS(Abs(num))*Abs(num)/num)\n}\n\nfunc TtoS(num int) int {\n\tdivided := int(math.Ceil(float64(num / 7)))\n\n\tif num < 7 {\n\t\treturn num\n\t}\n\treturn TtoS(divided)*10 + num%7\n}\n\nfunc Abs(x int) int {\n\tif x < 0 {\n\t\treturn -x\n\t}\n\treturn x\n}\n``` | 2 | 0 | ['Go'] | 0 |
base-7 | JAVA 0ms | java-0ms-by-movsar-92fx | \n\n\nclass Solution {\n public String convertToBase7(int num) {\n char sign = \'+\';\n if (num < 0) {\n sign = \'-\';\n | movsar | NORMAL | 2021-10-25T08:37:11.663067+00:00 | 2021-10-25T08:37:11.663111+00:00 | 319 | false | 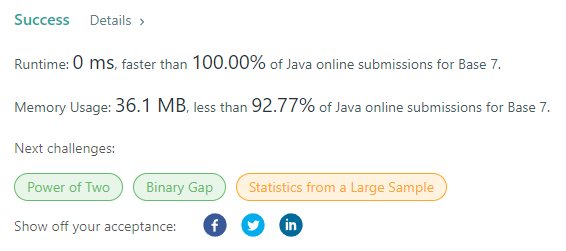\n\n```\nclass Solution {\n public String convertToBase7(int num) {\n char sign = \'+\';\n if (num < 0) {\n sign = \'-\';\n num *= -1;\n }\n \n if (num == 0) return "0";\n \n StringBuilder res = new StringBuilder();\n \n while (num != 0) {\n res.append(num % 7);\n num /= 7;\n }\n \n if (sign == \'-\') res.append(\'-\');\n \n return res.reverse().toString();\n }\n}\n``` | 2 | 0 | ['Java'] | 0 |
base-7 | [JavaScript] JS 1 liner - Easiest/Fastest | javascript-js-1-liner-easiestfastest-by-4lj9d | \nvar convertToBase7 = function(num) {\n return num.toString(7)\n};\n | gnktgc | NORMAL | 2021-09-22T15:25:08.171598+00:00 | 2021-09-22T15:25:08.171650+00:00 | 278 | false | ```\nvar convertToBase7 = function(num) {\n return num.toString(7)\n};\n``` | 2 | 0 | ['JavaScript'] | 1 |
base-7 | C solution | c-solution-by-linhbk93-94gv | \nchar * convertToBase7(int num){\n if(num == 0) return "0";\n char * result = (char *)malloc(12*sizeof(char));\n int count = 0;\n bool checkNeg = n | linhbk93 | NORMAL | 2021-09-01T04:35:58.987101+00:00 | 2021-09-01T04:35:58.987147+00:00 | 338 | false | ```\nchar * convertToBase7(int num){\n if(num == 0) return "0";\n char * result = (char *)malloc(12*sizeof(char));\n int count = 0;\n bool checkNeg = num < 0 ? true : false;\n if(num < 0) num = abs(num);\n while(num > 0)\n { \n result[count++] = num % 7 + \'0\';\n num /= 7;\n }\n if(checkNeg) result[count++] = \'-\';\n for(int i=0; i<count/2; i++)\n {\n int temp = result[i];\n result[i] = result[count-i-1];\n result[count-i-1] = temp;\n }\n result[count] = 0;\n return result;\n}\n``` | 2 | 0 | ['C'] | 1 |
base-7 | Easy, Fast Python Solutions (2 Approaches - 24ms, 32ms; Faster than 95%) | easy-fast-python-solutions-2-approaches-0vsjz | Easy, Fast Python Solutions (2 Approaches - 24ms, 32ms; Faster than 95%)\n\n## Approach 1 - Using List\nRuntime: 24 ms, faster than 95% of Python3 online submis | the_sky_high | NORMAL | 2021-07-23T08:58:28.452581+00:00 | 2021-07-23T08:58:28.452622+00:00 | 419 | false | # Easy, Fast Python Solutions (2 Approaches - 24ms, 32ms; Faster than 95%)\n\n## Approach 1 - Using List\n**Runtime: 24 ms, faster than 95% of Python3 online submissions for Base 7.**\n**Memory Usage: 14 MB, less than 90% of Python3 online submissions for Base 7.**\n```\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n answer = []\n sign = num\n\n if not num:\n return "0"\n elif num < 0:\n num *= -1\n\n while num:\n a = str(num % 7)\n answer.insert(0, a)\n num = num // 7\n\n if sign < 0:\n return "-" + "".join(answer)\n else:\n return "".join(answer)\n```\n\n\n## Aproach 2 - Using String\n**Runtime: 32 ms, faster than 62% of Python3 online submissions for Base 7.**\n**Memory Usage: 14.2 MB, less than 70% of Python3 online submissions for Base 7.**\n\n```\nclass Solution:\n def convertToBase7(self, num: int) -> str:\n answer = ""\n sign = num\n\n if not num:\n return "0"\n elif num < 0:\n num *= -1\n\n while num:\n a = str(num % 7)\n answer = a + answer\n num = num // 7\n\n if sign < 0:\n answer = "-" + answer\n\n return answer\n``` | 2 | 0 | ['String', 'Python', 'Python3'] | 0 |
base-7 | Easy Solution 0ms (100%) c++ | easy-solution-0ms-100-c-by-moazmar-h73b | \nclass Solution {\npublic:\n string convertToBase7(int num) {\n string res="";\n bool neg=false;\n if(num==0)return "0";\n if(nu | moazmar | NORMAL | 2020-12-27T16:04:53.080905+00:00 | 2020-12-27T16:04:53.080944+00:00 | 394 | false | ```\nclass Solution {\npublic:\n string convertToBase7(int num) {\n string res="";\n bool neg=false;\n if(num==0)return "0";\n if(num<0){\n neg=true;\n num=-num;\n }\n while(num>0){\n int x=num%7;\n num/=7;\n res=to_string(x)+res;\n }\n if(neg)res="-"+res;\n return res;\n }\n};\n``` | 2 | 0 | ['C', 'C++'] | 0 |
base-7 | Simple JS Solution | simple-js-solution-by-_kamal_jain-k8lb | \n/**\n * @param {number} num\n * @return {string}\n */\nvar convertToBase7 = function(num) {\n if (num === 0) return \'0\';\n const sign = num < 0 ? \'-\ | _kamal_jain | NORMAL | 2020-08-22T11:02:18.911407+00:00 | 2020-08-22T11:02:18.911499+00:00 | 273 | false | ```\n/**\n * @param {number} num\n * @return {string}\n */\nvar convertToBase7 = function(num) {\n if (num === 0) return \'0\';\n const sign = num < 0 ? \'-\' : \'+\';\n num = Math.abs(num);\n let res = \'\';\n while (num) {\n res = num % 7 + res;\n num = Math.floor(num / 7);\n }\n return sign === \'+\' ? res : \'-\' + res;\n};\n``` | 2 | 0 | ['JavaScript'] | 0 |
base-7 | 1 line Java Solution | 1-line-java-solution-by-shubhijain67-yiis | \nclass Solution {\n public String convertToBase7(int num) {\n return Integer.toString(Integer.parseInt(num+""),7);\n }\n}\n | shubhijain67 | NORMAL | 2020-05-07T18:12:09.483600+00:00 | 2020-05-07T18:12:09.483651+00:00 | 403 | false | ```\nclass Solution {\n public String convertToBase7(int num) {\n return Integer.toString(Integer.parseInt(num+""),7);\n }\n}\n``` | 2 | 0 | ['Java'] | 1 |
base-7 | Easy C# Solution | easy-c-solution-by-maxpushkarev-0wxq | \npublic class Solution\n{\n public string ConvertToBase7(int num)\n {\n if (num == 0)\n {\n return "0";\n }\n\n in | maxpushkarev | NORMAL | 2020-01-05T02:57:36.881718+00:00 | 2020-01-05T02:57:36.881754+00:00 | 172 | false | ```\npublic class Solution\n{\n public string ConvertToBase7(int num)\n {\n if (num == 0)\n {\n return "0";\n }\n\n int sign = Math.Sign(num);\n Stack<int> mods = new Stack<int>();\n\n num = Math.Abs(num);\n\n while (num != 0)\n {\n mods.Push(num % 7);\n num /= 7;\n }\n\n StringBuilder sb = new StringBuilder(mods.Count + 1);\n if (sign < 0)\n {\n sb.Append(\'-\');\n }\n while (mods.Count != 0)\n {\n sb.Append(mods.Pop());\n }\n\n return sb.ToString();\n }\n}\n``` | 2 | 0 | [] | 0 |
base-7 | python beats 100% | python-beats-100-by-doqin-rh5s | \nclass Solution(object):\n def convertToBase7(self, num):\n """\n :type num: int\n :rtype: str\n """\n isNegative = False | doqin | NORMAL | 2018-12-10T02:59:48.561297+00:00 | 2018-12-10T02:59:48.561343+00:00 | 807 | false | ```\nclass Solution(object):\n def convertToBase7(self, num):\n """\n :type num: int\n :rtype: str\n """\n isNegative = False if num > 0 else True\n lst = []\n res = 0\n num = abs(num)\n while num > 0:\n lst.append(num%7)\n num = num/7\n for i in range(len(lst)):\n res += 10**i * lst[i]\n return str(-res if isNegative == True else res)\n``` | 2 | 0 | [] | 1 |
base-7 | C# 3 line fastest! | c-3-line-fastest-by-hoangyuh-65pg | public string ConvertToBase7(int num) { if (num < 0) return "-" + ConvertToBase7(-num); if (num < 7) return num.ToString(); return Conve | hoangyuh | NORMAL | 2018-10-22T03:39:20.129844+00:00 | 2018-10-22T03:39:20.129893+00:00 | 211 | false | ```
public string ConvertToBase7(int num) {
if (num < 0) return "-" + ConvertToBase7(-num);
if (num < 7) return num.ToString();
return ConvertToBase7(num / 7) + (num % 7).ToString();
}
``` | 2 | 0 | [] | 0 |
base-7 | Simple 2 lines solution in C++ | simple-2-lines-solution-in-c-by-fockl-cyc2 | class Solution {\npublic:\n string convertToBase7(int num) {\n if(num < 0) return "-" + convertToBase7(-num);\n return (num/7>0) ? convertToBase7(n | fockl | NORMAL | 2018-06-20T11:52:49.093784+00:00 | 2018-09-05T11:30:24.620644+00:00 | 348 | false | ```class Solution {\npublic:\n string convertToBase7(int num) {\n if(num < 0) return "-" + convertToBase7(-num);\n return (num/7>0) ? convertToBase7(num/7) + to_string(num%7) : to_string(num%7);\n }\n};\n``` | 2 | 1 | [] | 0 |
base-7 | C++ solution | c-solution-by-kevin36-uu9l | ```\nclass Solution {\npublic:\n string convertTo7(int num) {\n if(num == 0) return "0";\n string str ="";\n \n if(num < 0) retur | kevin36 | NORMAL | 2017-02-12T04:13:06.589000+00:00 | 2017-02-12T04:13:06.589000+00:00 | 978 | false | ```\nclass Solution {\npublic:\n string convertTo7(int num) {\n if(num == 0) return "0";\n string str ="";\n \n if(num < 0) return "-" + convertTo7(-num);\n while( num > 0){\n int rem = num % 7;\n num = num/7;\n \n str = to_string(rem) + str;\n }\n \n return str;\n }\n\n return str; | 2 | 0 | [] | 1 |
base-7 | Intuitive Javascript Solution | intuitive-javascript-solution-by-dawchih-hoaf | \nvar convertToBase7 = function(num) {\n // use Number's native api to convert to base 7 presentation\n return Number(num).toString(7);\n};\n | dawchihliou | NORMAL | 2017-03-03T02:01:45.371000+00:00 | 2017-03-03T02:01:45.371000+00:00 | 728 | false | ```\nvar convertToBase7 = function(num) {\n // use Number's native api to convert to base 7 presentation\n return Number(num).toString(7);\n};\n``` | 2 | 1 | ['JavaScript'] | 3 |
base-7 | 100% beats in c++ solution. | 100-beats-in-c-solution-by-jahidulcse15-hyty | IntuitionApproachComplexity
Time complexity:O(log7(N))
Space complexity:O(log7(N))
Code | jahidulcse15 | NORMAL | 2025-04-12T03:47:18.407574+00:00 | 2025-04-12T03:47:18.407574+00:00 | 2 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:O(log7(N))
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:O(log7(N))
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
string convertToBase7(int num) {
if(num==0){
return "0";
}
string s;
int minus=0;
if(num<0){
minus=-1;
num*=-1;
}
while(num){
s+=(num%7)+'0';
num/=7;
}
reverse(s.begin(),s.end());
if(minus==-1){
s="-"+s;
}
return s;
}
};
``` | 1 | 0 | ['C++'] | 0 |
base-7 | 0 ms | Beats 100.00% | Easy Code | 0-ms-beats-10000-easy-code-by-r_nirmal_r-6an4 | Code | R_Nirmal_Rajan | NORMAL | 2025-03-24T18:17:55.816069+00:00 | 2025-03-24T18:17:55.816069+00:00 | 157 | false | # Code
```python3 []
class Solution:
def convertToBase7(self, num: int) -> str:
if num == 0:
return "0"
negative = num < 0
num = abs(num)
base7 = []
while num > 0:
base7.append(str(num % 7))
num //= 7
if negative:
base7.append('-')
return ''.join(base7[::-1])
``` | 1 | 0 | ['Math', 'Python3'] | 0 |
base-7 | 100 Beats Solution 👏| Math ⚡| Java 🎯 | 100-beats-solution-math-java-by-user8688-nrzl | 📌 Step-by-Step Breakdown:Handling Negative Numbers:
If num is negative, it is converted to a positive number for easier calculation, and a flag is set to track | user8688F | NORMAL | 2025-03-21T09:30:22.582693+00:00 | 2025-03-21T09:30:22.582693+00:00 | 163 | false |
# 📌 Step-by-Step Breakdown:
## **Handling Negative Numbers:**
- If num is negative, it is converted to a positive number for easier calculation, and a flag is set to track the sign.
## **Extracting Base-7 Digits:**
- The while(num > 0) loop repeatedly divides the number by 7 and collects the remainders (digits in Base-7).
Each digit is multiplied by powers of 10 to form the final number (n).
## **Restoring Sign:**
- If the flag indicates the original number was negative, the result is returned as a negative value.
## **Edge Case:**
- If the input num is 0, the function directly returns "0".
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity: O(log7(𝑛))
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(log7(𝑛))
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
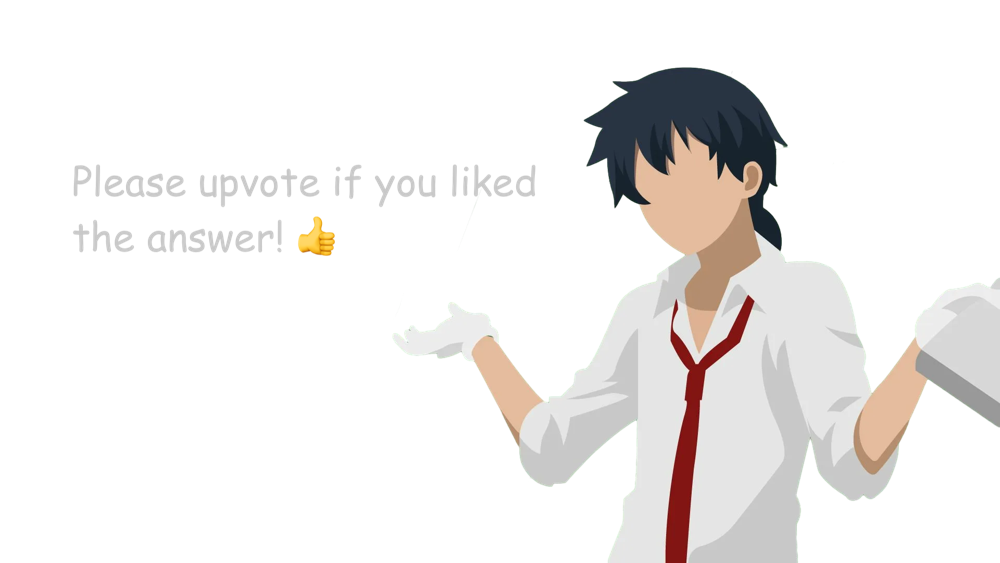
# Code
```java []
class Solution {
static int base(int num ){
int flag=0 ,n=0,pov=1;
if(num < 0){
num*=-1;
flag=1;
}
while(num > 0){
int l= num % 7;
n+=(l*(pov));
pov*=10;
num = num / 7;
}
return (flag == 1) ? -n : n;
}
public String convertToBase7(int num) {
if(num == 0 ) return "0";
return String.valueOf(base(num));
}
}
``` | 1 | 0 | ['Math', 'Java'] | 0 |
base-7 | Base 7 | base-7-by-717822s103-jiib | IntuitionThe basic idea is to repeatedly divide the number by 7 and collect the remainders. The remainders represent the digits of the number in base-7, but sin | Alexsha | NORMAL | 2025-02-24T07:35:41.100075+00:00 | 2025-02-24T07:35:41.100075+00:00 | 324 | false | # Intuition
The basic idea is to repeatedly divide the number by 7 and collect the remainders. The remainders represent the digits of the number in base-7, but since the process gives us digits in reverse order, we will need to reverse the result at the end.
# Approach
1.We handle the special case where num = 0 and return "0".
2.We convert the number to its absolute value, track the sign, and repeatedly divide by 7, collecting remainders as digits.
3.We append the digits to a StringBuilder and reverse the order.
4.If the number was negative, we add a "-" sign and return the final base-7 representation.
# Complexity
- Time complexity:
The time complexity is O(log7(N))
- Space complexity:
The space complexity is O(log7(N))
# Code
```java []
class Solution {
public String convertToBase7(int num) {
if(num==0)return "0";
StringBuilder sb=new StringBuilder();
int sign=(num>=0)?1:-1;
num=Math.abs(num);
while(num>0)
{
sb.append(num%7);
num/=7;
}
if(sign==-1)sb.append('-');
return sb.reverse().toString();
}
}
``` | 1 | 0 | ['Java'] | 0 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.